7 2 Array Lists Array List class manages
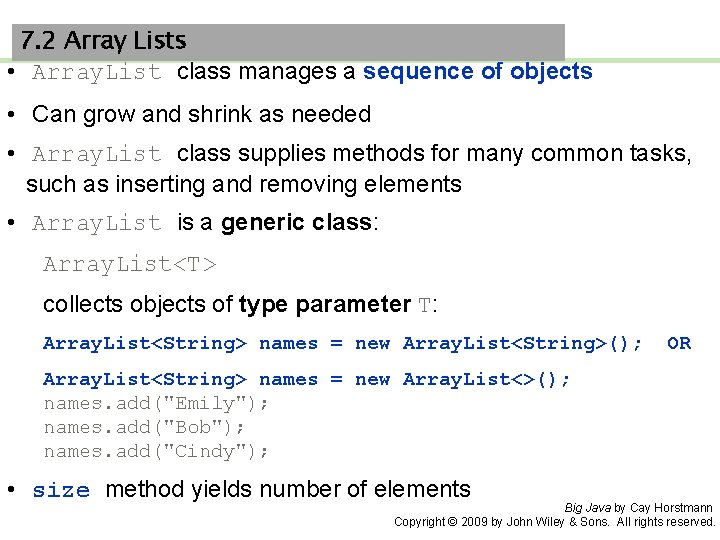
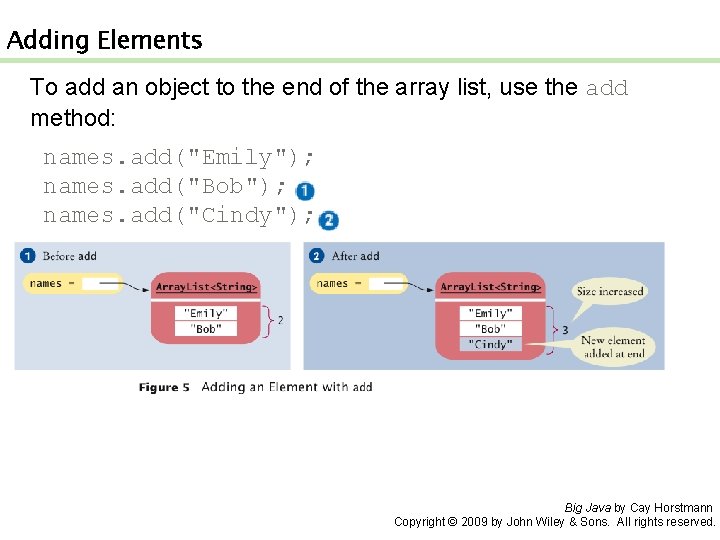
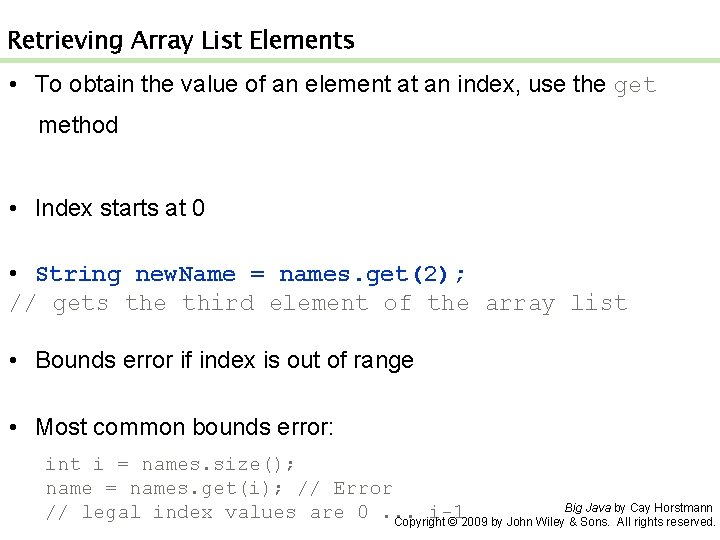
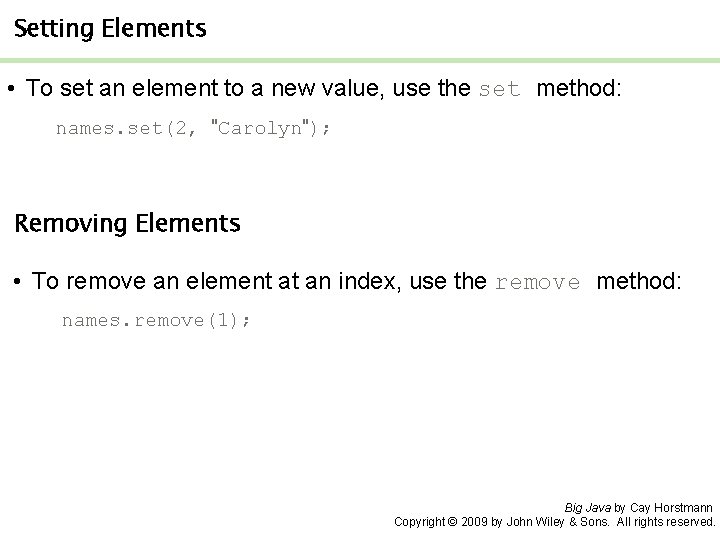
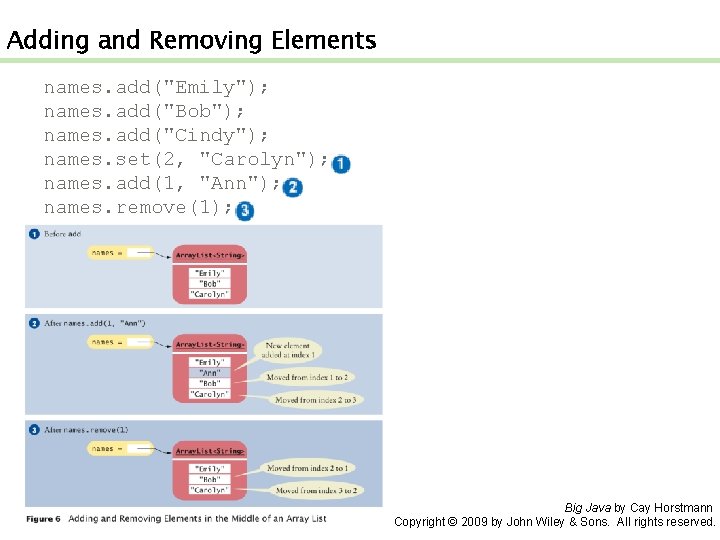
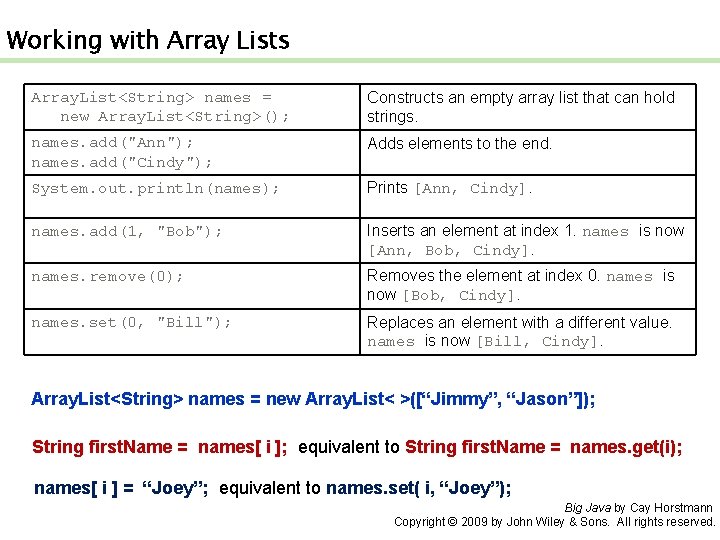
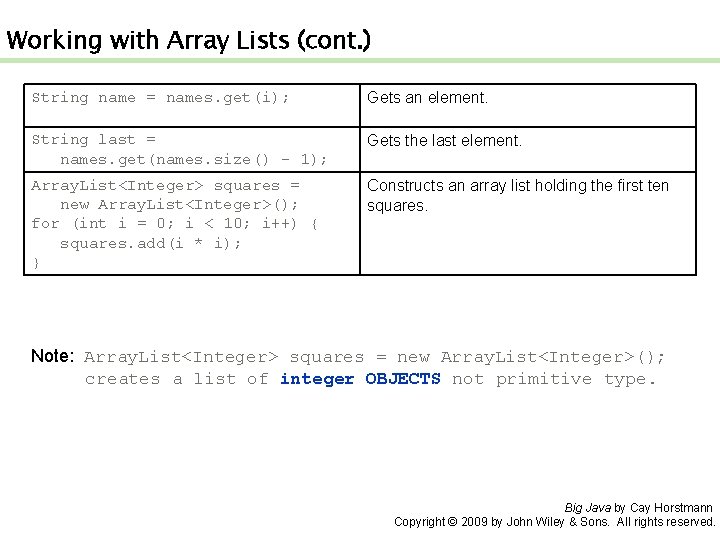
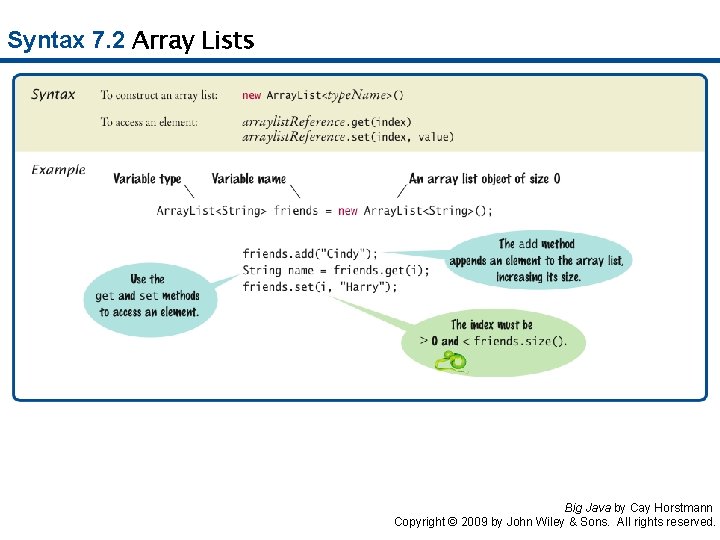
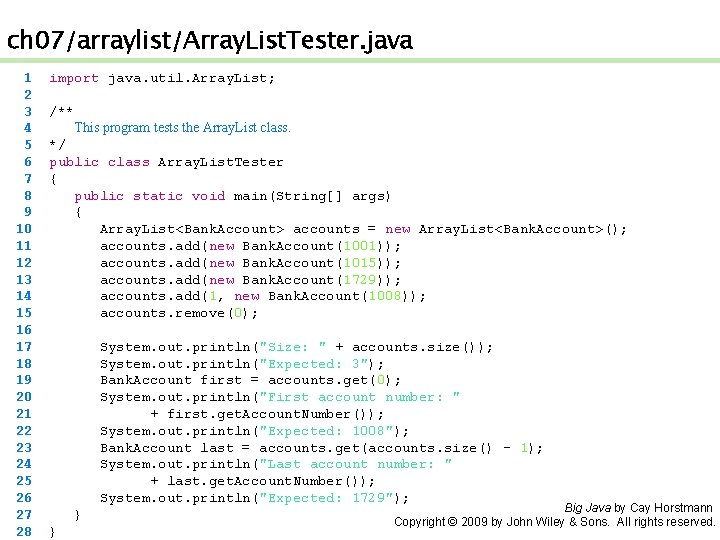
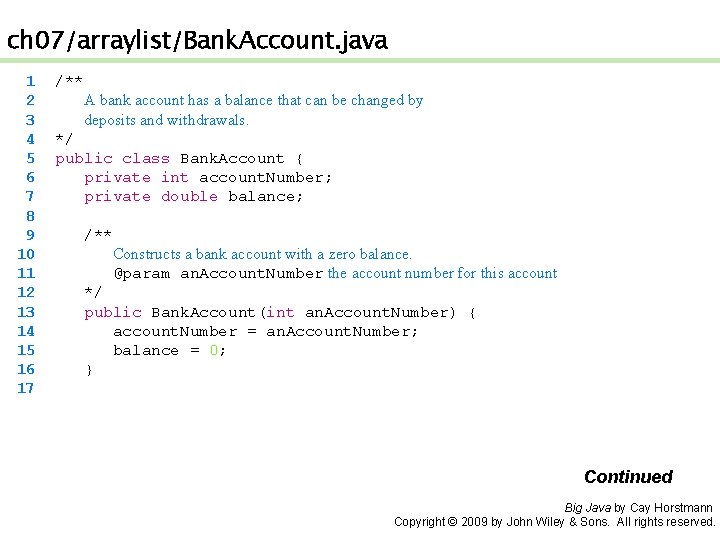
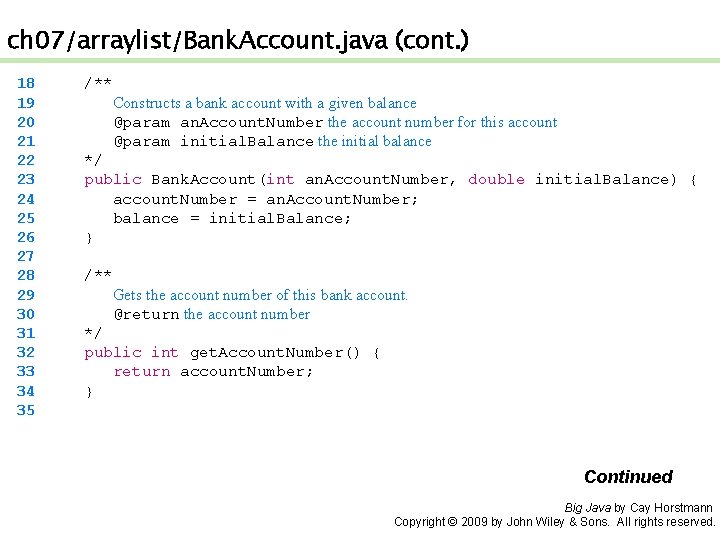
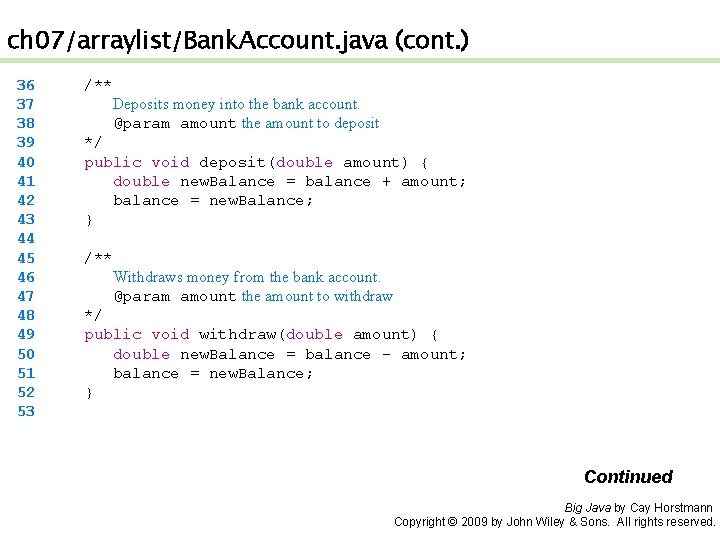
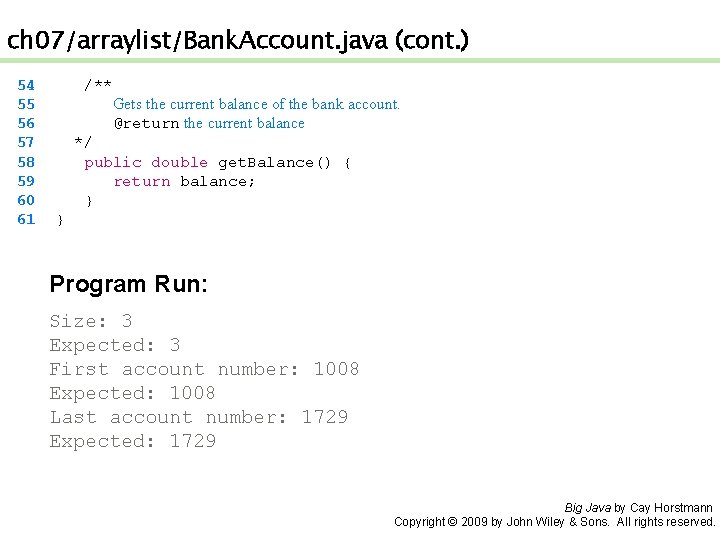
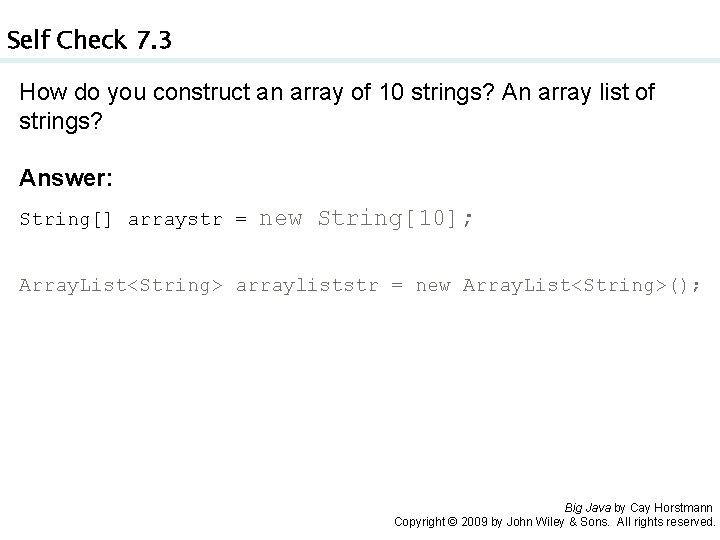
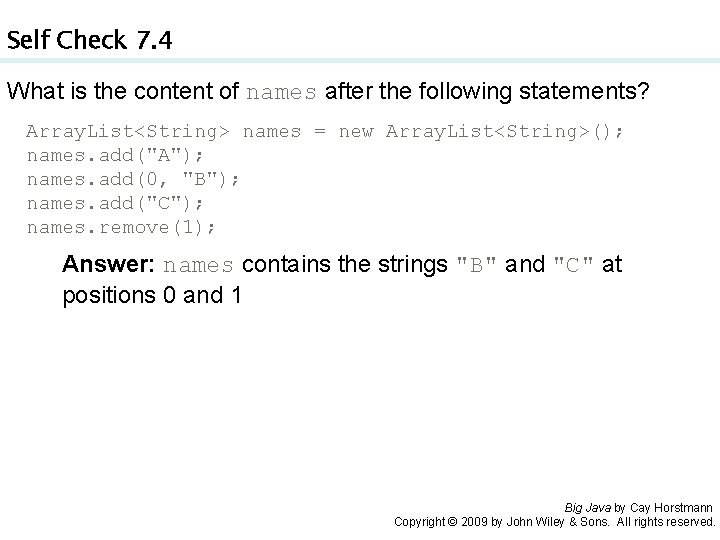
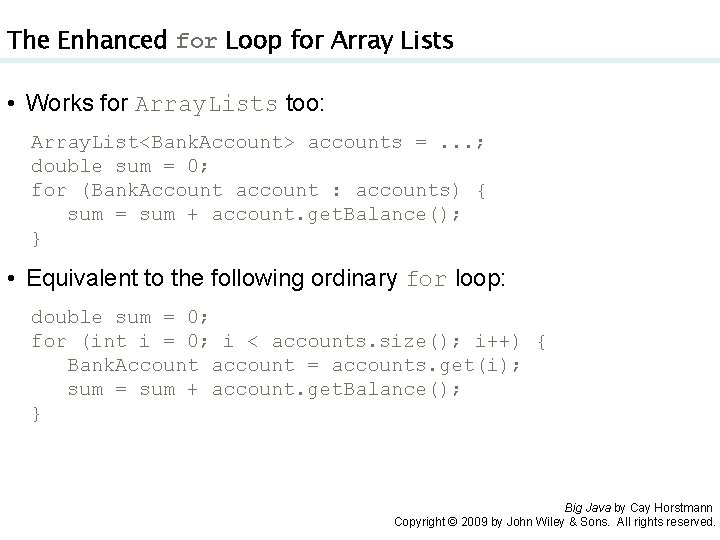
- Slides: 16
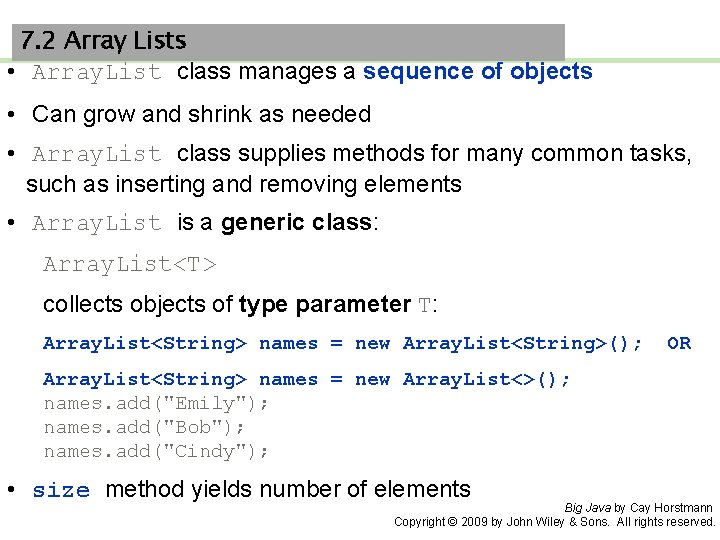
7. 2 Array Lists • Array. List class manages a sequence of objects • Can grow and shrink as needed • Array. List class supplies methods for many common tasks, such as inserting and removing elements • Array. List is a generic class: Array. List<T> collects objects of type parameter T: Array. List<String> names = new Array. List<String>(); OR Array. List<String> names = new Array. List<>(); names. add("Emily"); names. add("Bob"); names. add("Cindy"); • size method yields number of elements Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
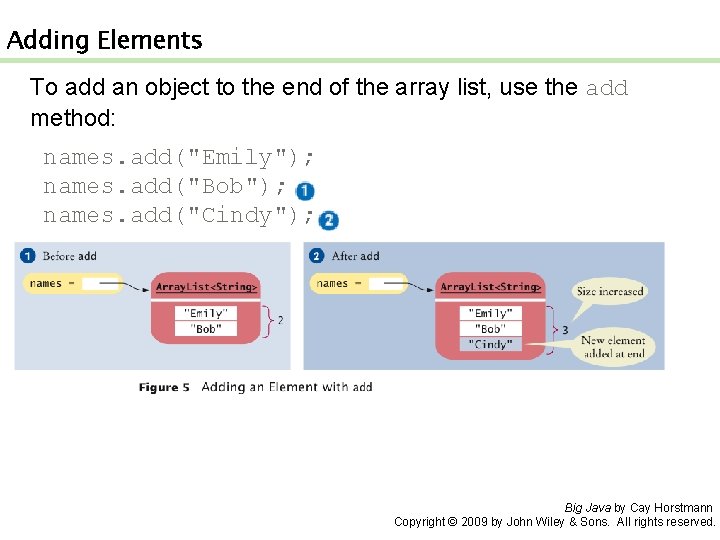
Adding Elements To add an object to the end of the array list, use the add method: names. add("Emily"); names. add("Bob"); names. add("Cindy"); Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
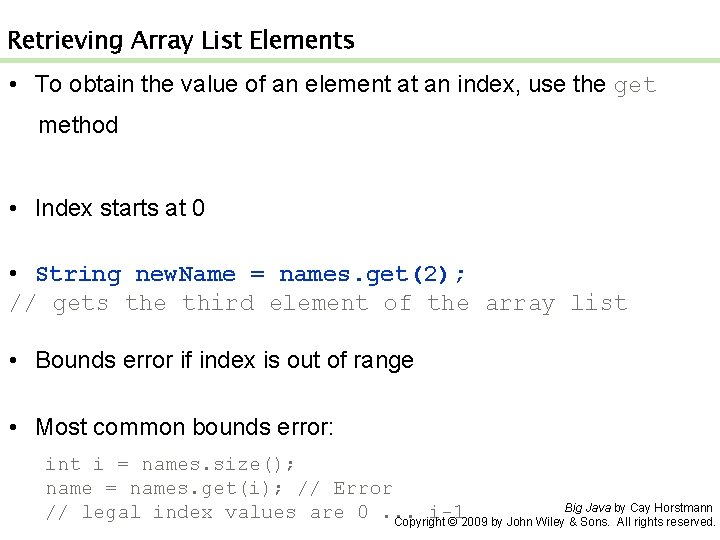
Retrieving Array List Elements • To obtain the value of an element at an index, use the get method • Index starts at 0 • String new. Name = names. get(2); // gets the third element of the array list • Bounds error if index is out of range • Most common bounds error: int i = names. size(); name = names. get(i); // Error Big Java by Cay Horstmann // legal index values are 0. . . i-1 Copyright © 2009 by John Wiley & Sons. All rights reserved.
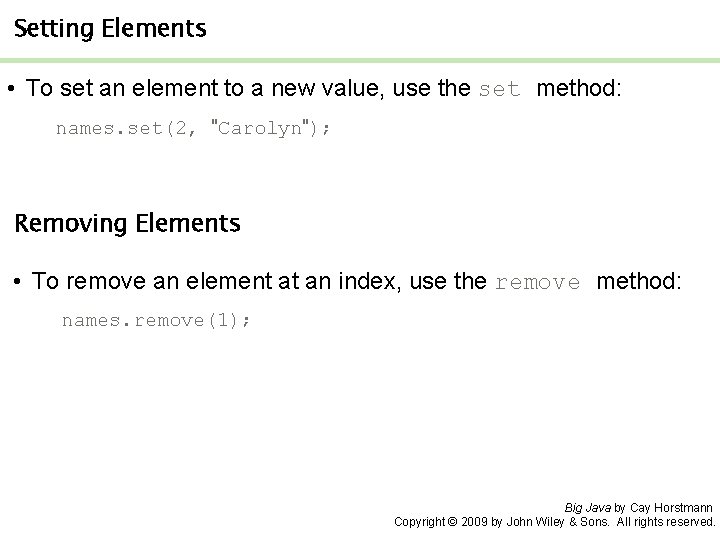
Setting Elements • To set an element to a new value, use the set method: names. set(2, "Carolyn"); Removing Elements • To remove an element at an index, use the remove method: names. remove(1); Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
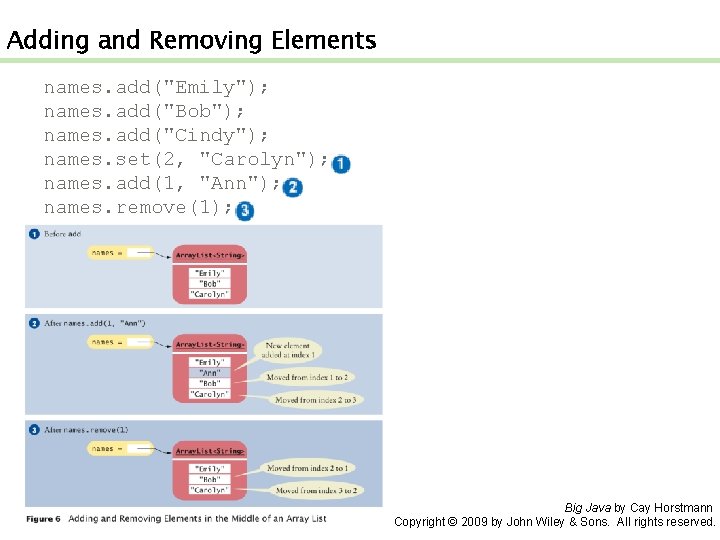
Adding and Removing Elements names. add("Emily"); names. add("Bob"); names. add("Cindy"); names. set(2, "Carolyn"); names. add(1, "Ann"); names. remove(1); Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
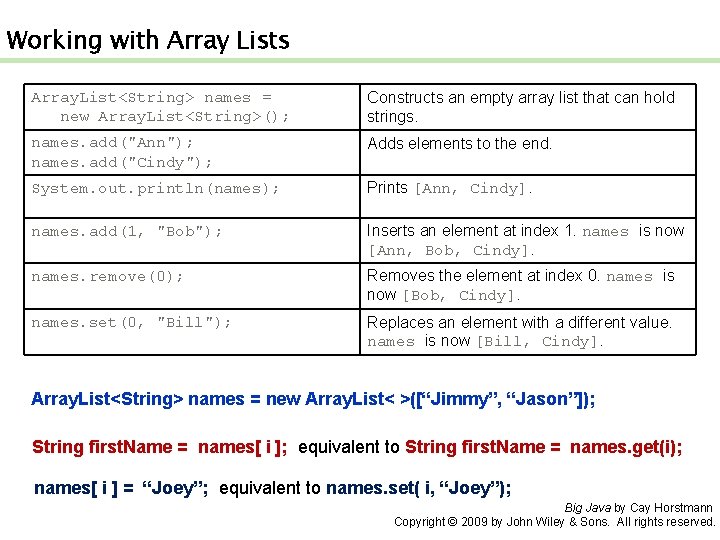
Working with Array Lists Array. List<String> names = new Array. List<String>(); Constructs an empty array list that can hold strings. names. add("Ann"); names. add("Cindy"); Adds elements to the end. System. out. println(names); Prints [Ann, Cindy]. names. add(1, "Bob"); Inserts an element at index 1. names is now [Ann, Bob, Cindy]. names. remove(0); Removes the element at index 0. names is now [Bob, Cindy]. names. set(0, "Bill"); Replaces an element with a different value. names is now [Bill, Cindy]. Array. List<String> names = new Array. List< >([“Jimmy”, “Jason”]); String first. Name = names[ i ]; equivalent to String first. Name = names. get(i); names[ i ] = “Joey”; equivalent to names. set( i, “Joey”); Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
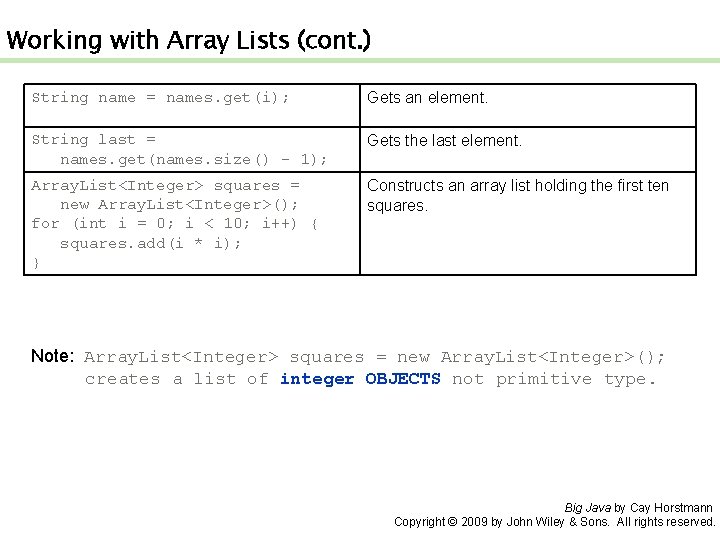
Working with Array Lists (cont. ) String name = names. get(i); Gets an element. String last = names. get(names. size() - 1); Gets the last element. Array. List<Integer> squares = new Array. List<Integer>(); for (int i = 0; i < 10; i++) { squares. add(i * i); } Constructs an array list holding the first ten squares. Note: Array. List<Integer> squares = new Array. List<Integer>(); creates a list of integer OBJECTS not primitive type. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
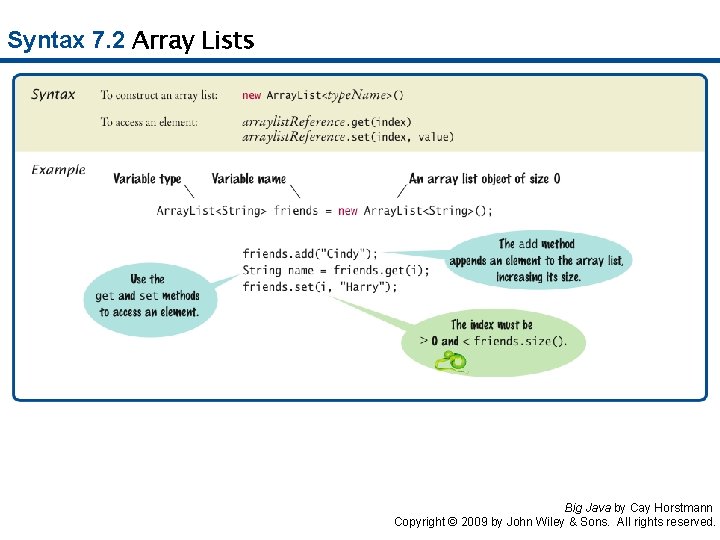
Syntax 7. 2 Array Lists Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
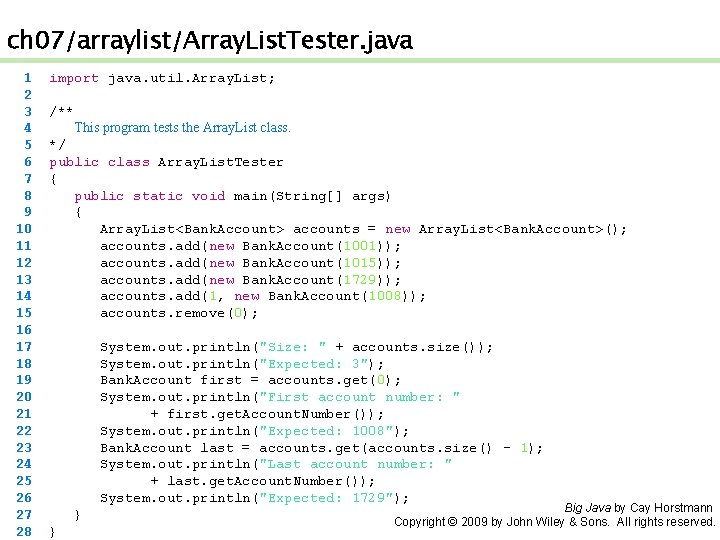
ch 07/arraylist/Array. List. Tester. java 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 import java. util. Array. List; /** This program tests the Array. List class. */ public class Array. List. Tester { public static void main(String[] args) { Array. List<Bank. Account> accounts = new Array. List<Bank. Account>(); accounts. add(new Bank. Account(1001)); accounts. add(new Bank. Account(1015)); accounts. add(new Bank. Account(1729)); accounts. add(1, new Bank. Account(1008)); accounts. remove(0); System. out. println("Size: " + accounts. size()); System. out. println("Expected: 3"); Bank. Account first = accounts. get(0); System. out. println("First account number: " + first. get. Account. Number()); System. out. println("Expected: 1008"); Bank. Account last = accounts. get(accounts. size() - 1); System. out. println("Last account number: " + last. get. Account. Number()); System. out. println("Expected: 1729"); } } Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
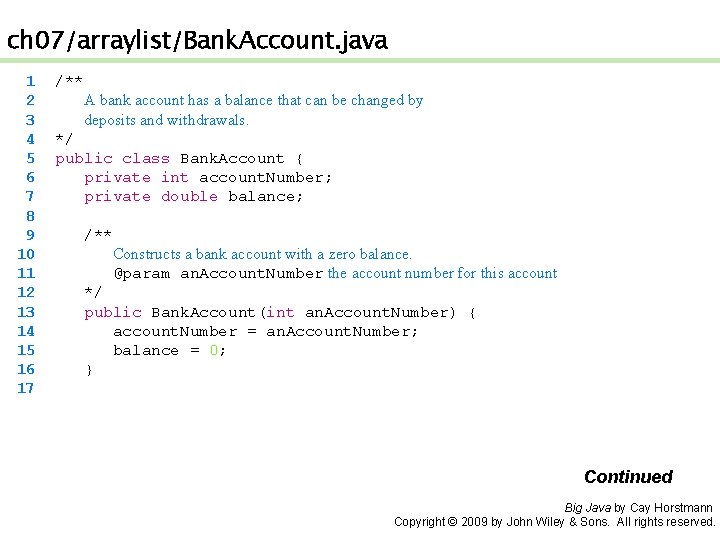
ch 07/arraylist/Bank. Account. java 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 /** A bank account has a balance that can be changed by deposits and withdrawals. */ public class Bank. Account { private int account. Number; private double balance; /** Constructs a bank account with a zero balance. @param an. Account. Number the account number for this account */ public Bank. Account(int an. Account. Number) { account. Number = an. Account. Number; balance = 0; } Continued Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
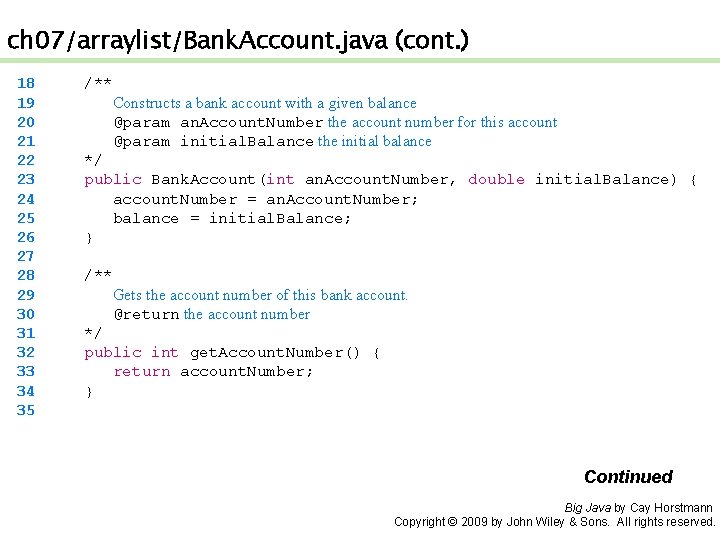
ch 07/arraylist/Bank. Account. java (cont. ) 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 /** Constructs a bank account with a given balance @param an. Account. Number the account number for this account @param initial. Balance the initial balance */ public Bank. Account(int an. Account. Number, double initial. Balance) { account. Number = an. Account. Number; balance = initial. Balance; } /** Gets the account number of this bank account. @return the account number */ public int get. Account. Number() { return account. Number; } Continued Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
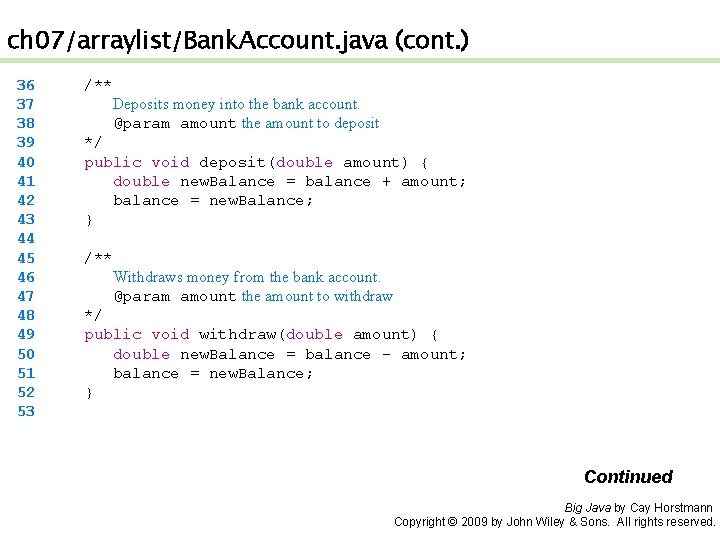
ch 07/arraylist/Bank. Account. java (cont. ) 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 /** Deposits money into the bank account. @param amount the amount to deposit */ public void deposit(double amount) { double new. Balance = balance + amount; balance = new. Balance; } /** Withdraws money from the bank account. @param amount the amount to withdraw */ public void withdraw(double amount) { double new. Balance = balance - amount; balance = new. Balance; } Continued Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
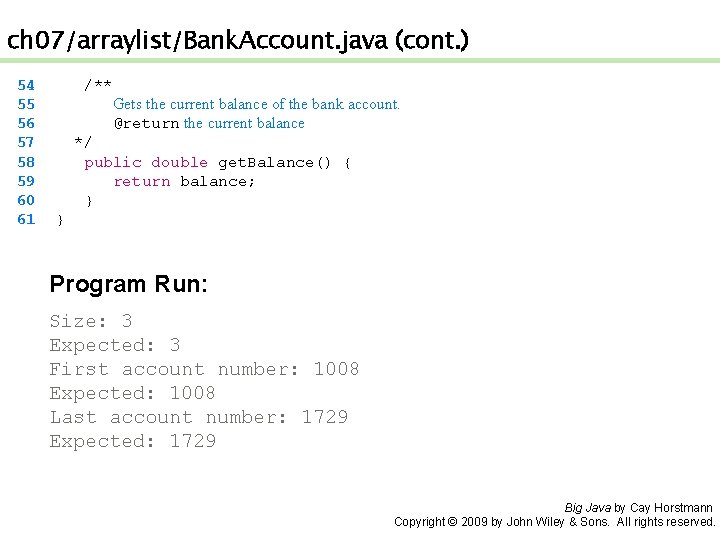
ch 07/arraylist/Bank. Account. java (cont. ) 54 55 56 57 58 59 60 61 /** Gets the current balance of the bank account. @return the current balance */ public double get. Balance() { return balance; } } Program Run: Size: 3 Expected: 3 First account number: 1008 Expected: 1008 Last account number: 1729 Expected: 1729 Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
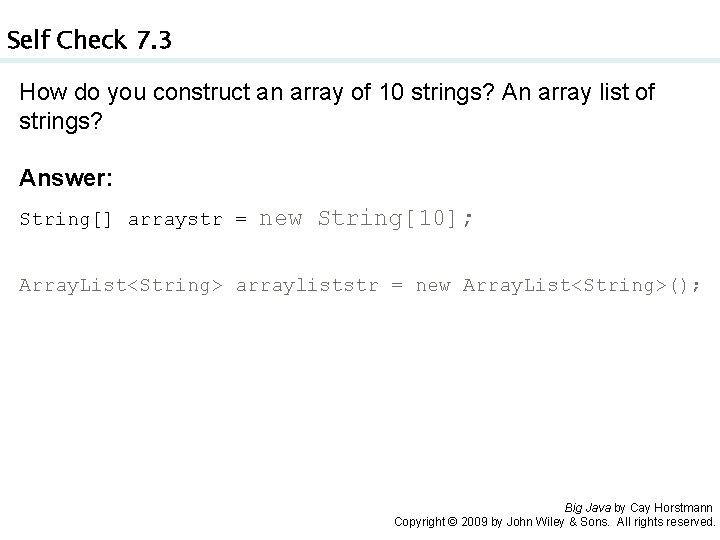
Self Check 7. 3 How do you construct an array of 10 strings? An array list of strings? Answer: String[] arraystr = new String[10]; Array. List<String> arrayliststr = new Array. List<String>(); Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
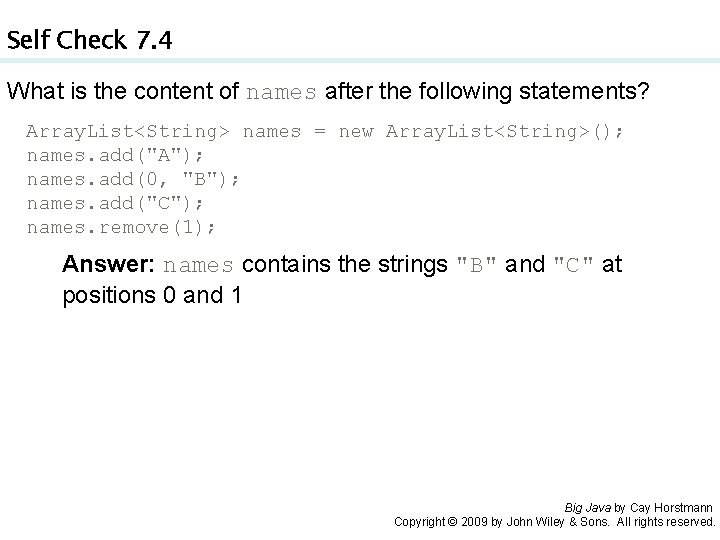
Self Check 7. 4 What is the content of names after the following statements? Array. List<String> names = new Array. List<String>(); names. add("A"); names. add(0, "B"); names. add("C"); names. remove(1); Answer: names contains the strings "B" and "C" at positions 0 and 1 Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
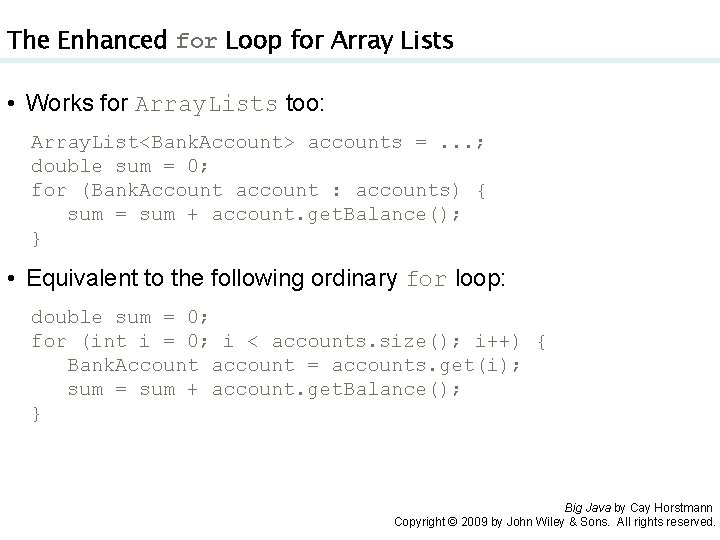
The Enhanced for Loop for Array Lists • Works for Array. Lists too: Array. List<Bank. Account> accounts =. . . ; double sum = 0; for (Bank. Account account : accounts) { sum = sum + account. get. Balance(); } • Equivalent to the following ordinary for loop: double sum = 0; for (int i = 0; i < accounts. size(); i++) { Bank. Account account = accounts. get(i); sum = sum + account. get. Balance(); } Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
Python plot list of lists
Prolog list of lists
Benefits of operating system
The operating system manages
Aloha cn
Hedging manages risk that are manageable
Dbms manages the interaction between and database.
North bridge south bridge
Diketahui suatu array segitiga memiliki 4 baris dan kolom
Jagged array vs multidimensional array
Associative array vs indexed array
Endfire array
Menginisialisasi artinya
Sparse array adalah
Multidimensional array python
Photovoltaic array maximum power point tracking array
Colon and semicolon