Security with Angular JS Krishna Mohan Koyya Glarimy
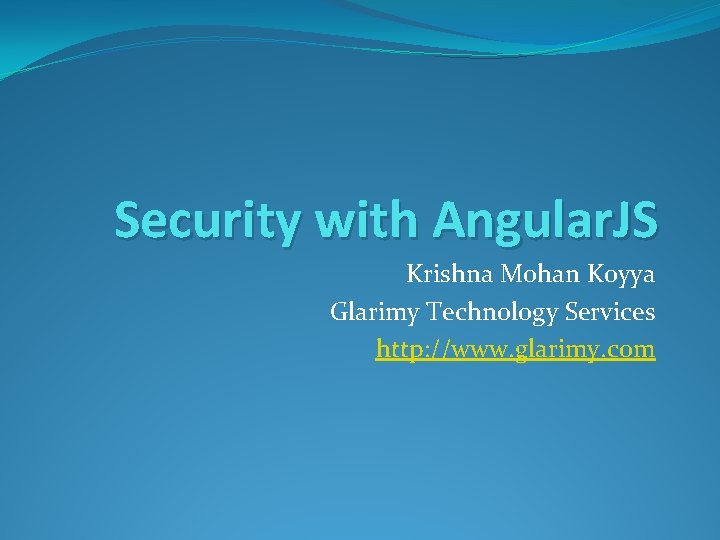
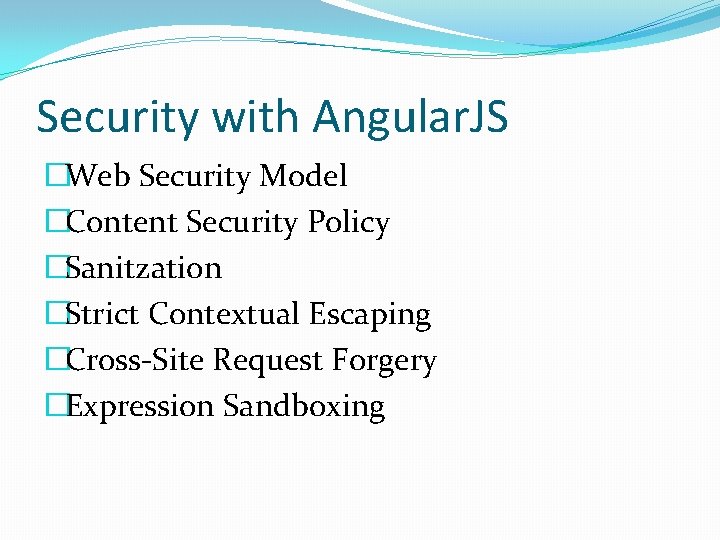
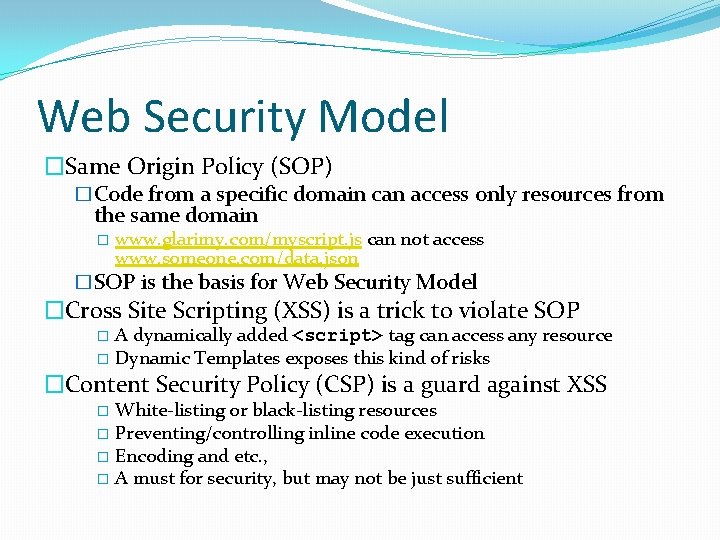
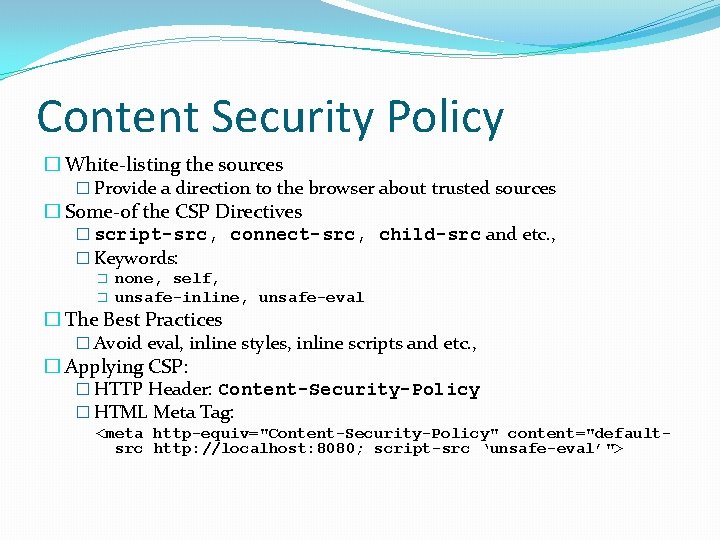
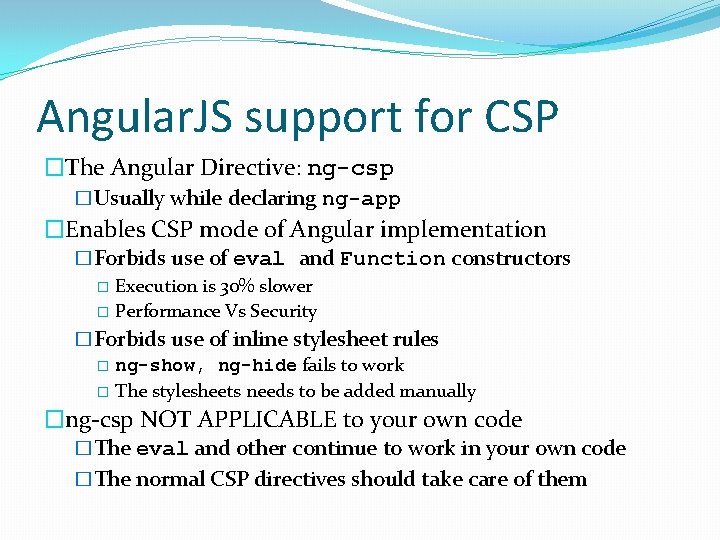
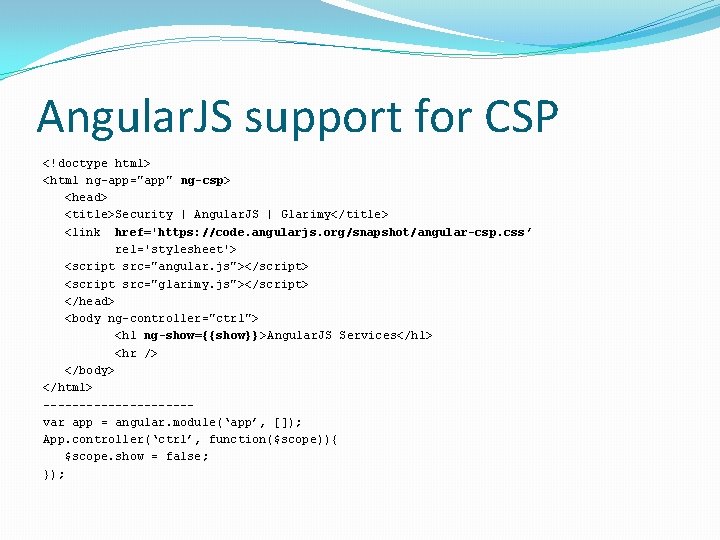
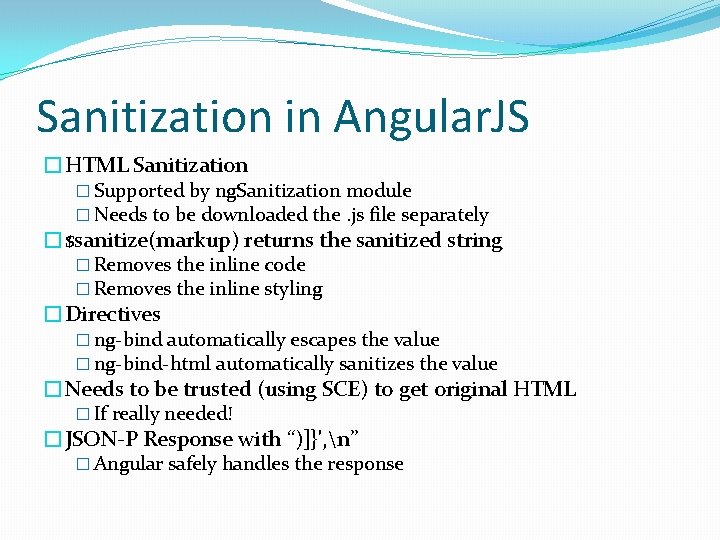
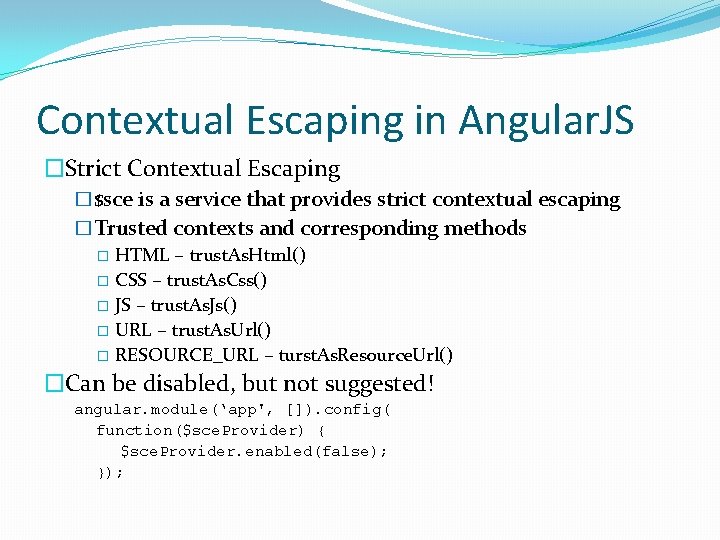
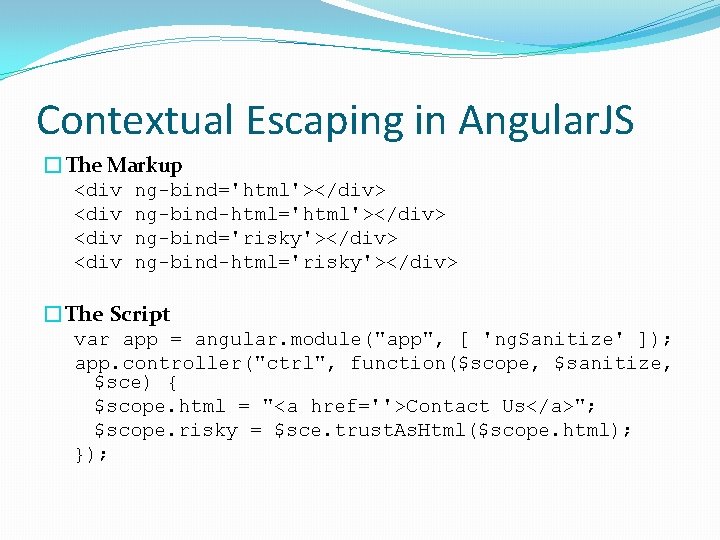
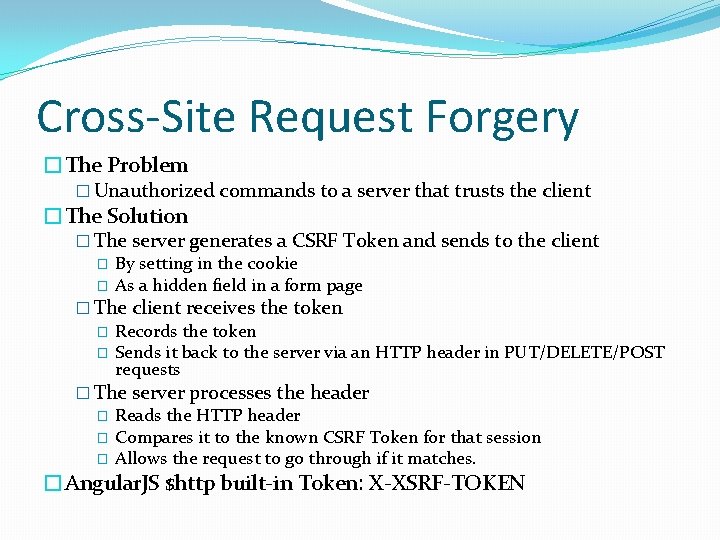
- Slides: 10
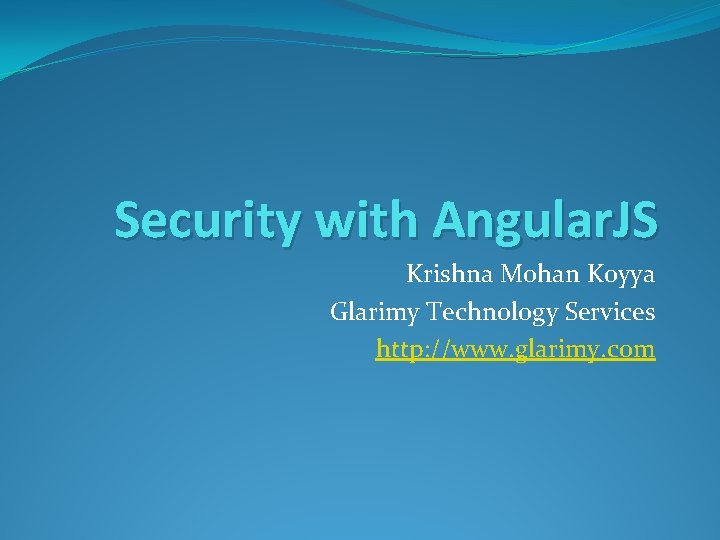
Security with Angular. JS Krishna Mohan Koyya Glarimy Technology Services http: //www. glarimy. com
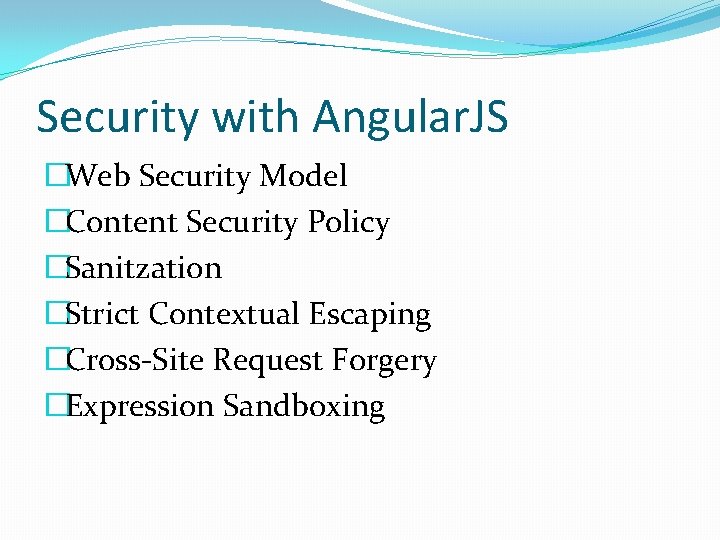
Security with Angular. JS �Web Security Model �Content Security Policy �Sanitzation �Strict Contextual Escaping �Cross-Site Request Forgery �Expression Sandboxing
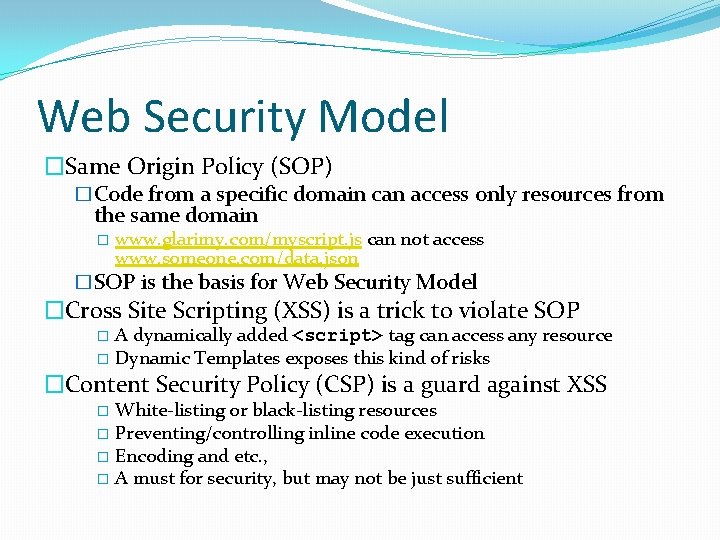
Web Security Model �Same Origin Policy (SOP) �Code from a specific domain can access only resources from the same domain � www. glarimy. com/myscript. js can not access www. someone. com/data. json �SOP is the basis for Web Security Model �Cross Site Scripting (XSS) is a trick to violate SOP A dynamically added <script> tag can access any resource � Dynamic Templates exposes this kind of risks � �Content Security Policy (CSP) is a guard against XSS White-listing or black-listing resources � Preventing/controlling inline code execution � Encoding and etc. , � A must for security, but may not be just sufficient �
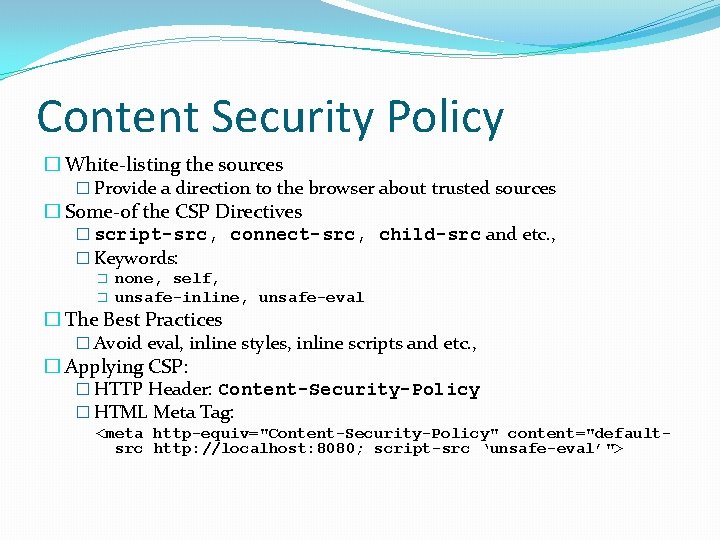
Content Security Policy � White-listing the sources � Provide a direction to the browser about trusted sources � Some-of the CSP Directives � script-src, connect-src, child-src and etc. , � Keywords: � � none, self, unsafe-inline, unsafe-eval � The Best Practices � Avoid eval, inline styles, inline scripts and etc. , � Applying CSP: � HTTP Header: Content-Security-Policy � HTML Meta Tag: <meta http-equiv="Content-Security-Policy" content="defaultsrc http: //localhost: 8080; script-src ‘unsafe-eval’">
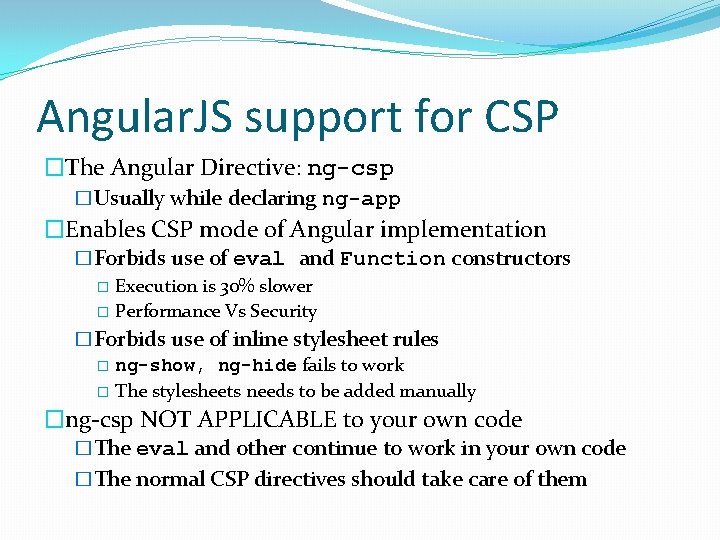
Angular. JS support for CSP �The Angular Directive: ng-csp �Usually while declaring ng-app �Enables CSP mode of Angular implementation �Forbids use of eval and Function constructors � Execution is 30% slower � Performance Vs Security �Forbids use of inline stylesheet rules � ng-show, ng-hide fails to work � The stylesheets needs to be added manually �ng-csp NOT APPLICABLE to your own code �The eval and other continue to work in your own code �The normal CSP directives should take care of them
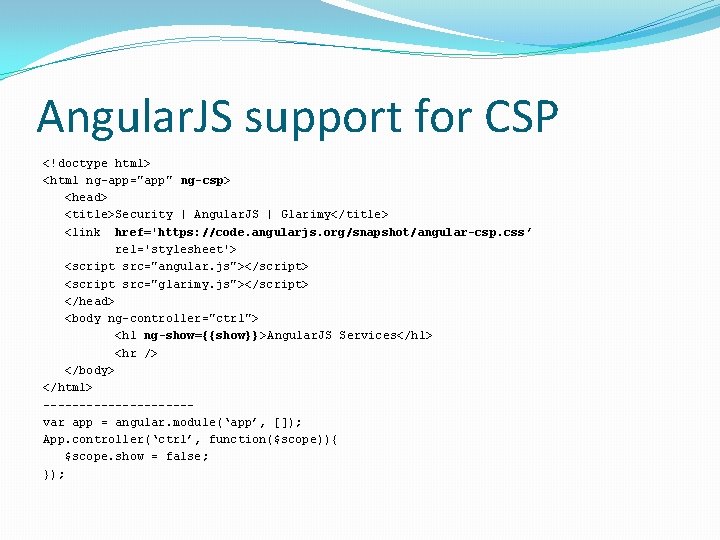
Angular. JS support for CSP <!doctype html> <html ng-app="app" ng-csp> <head> <title>Security | Angular. JS | Glarimy</title> <link href='https: //code. angularjs. org/snapshot/angular-csp. css’ rel='stylesheet'> <script src="angular. js"></script> <script src="glarimy. js"></script> </head> <body ng-controller="ctrl"> <h 1 ng-show={{show}}>Angular. JS Services</h 1> <hr /> </body> </html> ----------var app = angular. module(‘app’, []); App. controller(‘ctrl’, function($scope)){ $scope. show = false; });
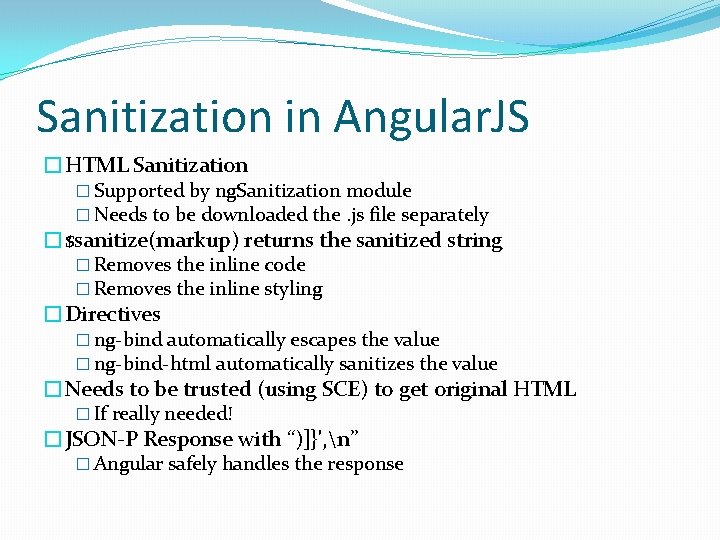
Sanitization in Angular. JS �HTML Sanitization � Supported by ng. Sanitization module � Needs to be downloaded the. js file separately �$sanitize(markup) returns the sanitized string � Removes the inline code � Removes the inline styling �Directives � ng-bind automatically escapes the value � ng-bind-html automatically sanitizes the value �Needs to be trusted (using SCE) to get original HTML � If really needed! �JSON-P Response with “)]}', n” � Angular safely handles the response
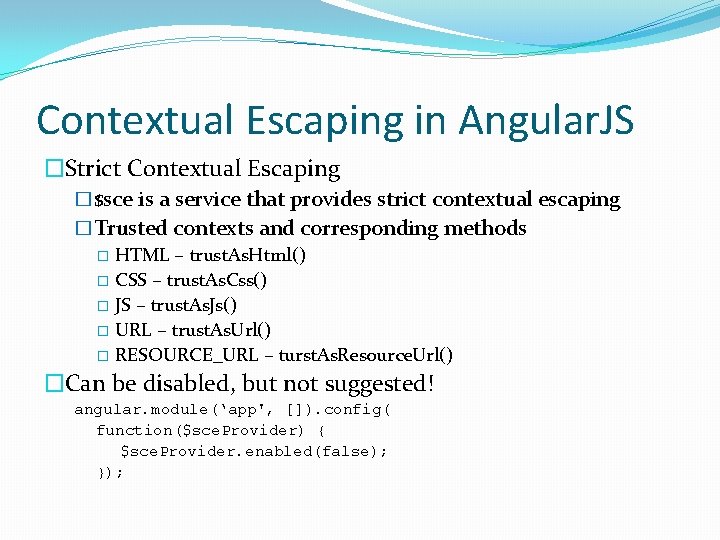
Contextual Escaping in Angular. JS �Strict Contextual Escaping �$sce is a service that provides strict contextual escaping �Trusted contexts and corresponding methods � HTML – trust. As. Html() � CSS – trust. As. Css() � JS – trust. As. Js() � URL – trust. As. Url() � RESOURCE_URL – turst. As. Resource. Url() �Can be disabled, but not suggested! angular. module(‘app', []). config( function($sce. Provider) { $sce. Provider. enabled(false); });
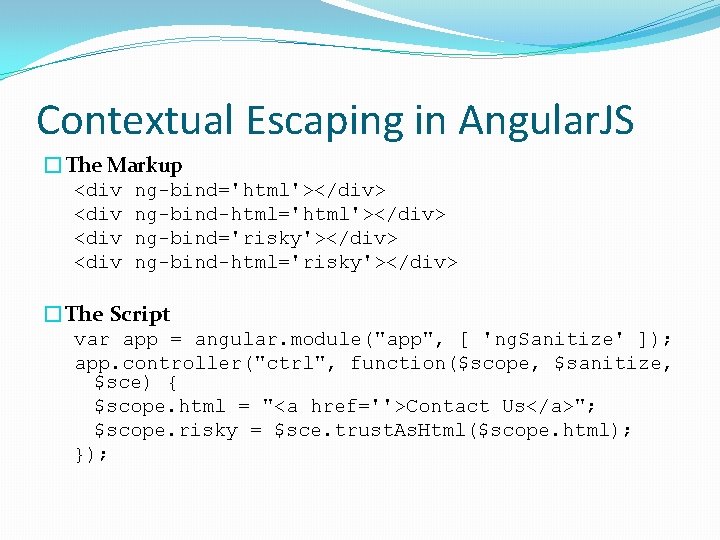
Contextual Escaping in Angular. JS �The Markup <div ng-bind='html'></div> ng-bind-html='html'></div> ng-bind='risky'></div> ng-bind-html='risky'></div> �The Script var app = angular. module("app", [ 'ng. Sanitize' ]); app. controller("ctrl", function($scope, $sanitize, $sce) { $scope. html = "<a href=''>Contact Us</a>"; $scope. risky = $sce. trust. As. Html($scope. html); });
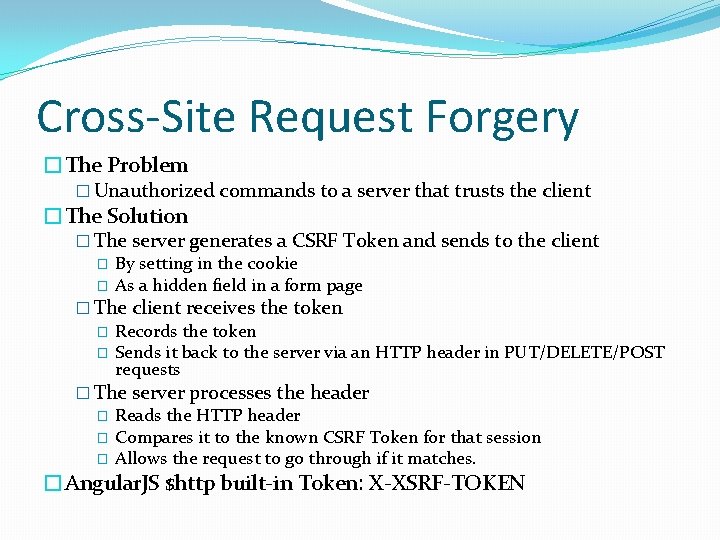
Cross-Site Request Forgery �The Problem � Unauthorized commands to a server that trusts the client �The Solution � The server generates a CSRF Token and sends to the client � By setting in the cookie � As a hidden field in a form page � The client receives the token � Records the token � Sends it back to the server via an HTTP header in PUT/DELETE/POST requests � The server processes the header � Reads the HTTP header � Compares it to the known CSRF Token for that session � Allows the request to go through if it matches. �Angular. JS $http built-in Token: X-XSRF-TOKEN