Recitation Nov 15 HW 5 Huffman EncodingDecoding Task
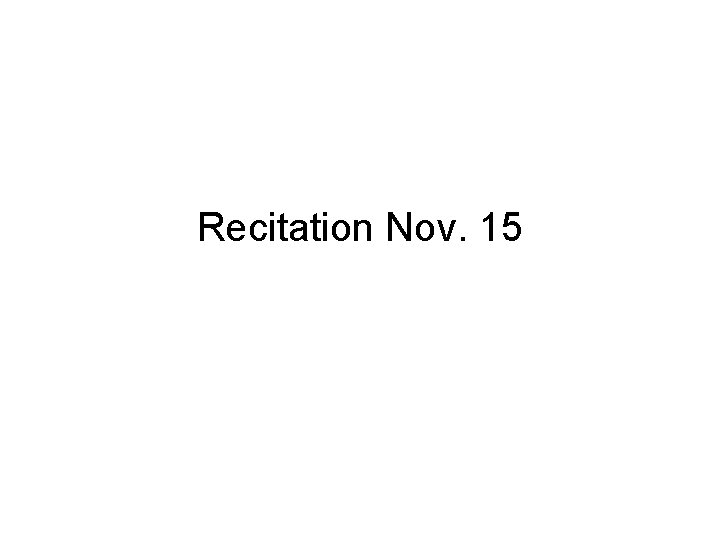
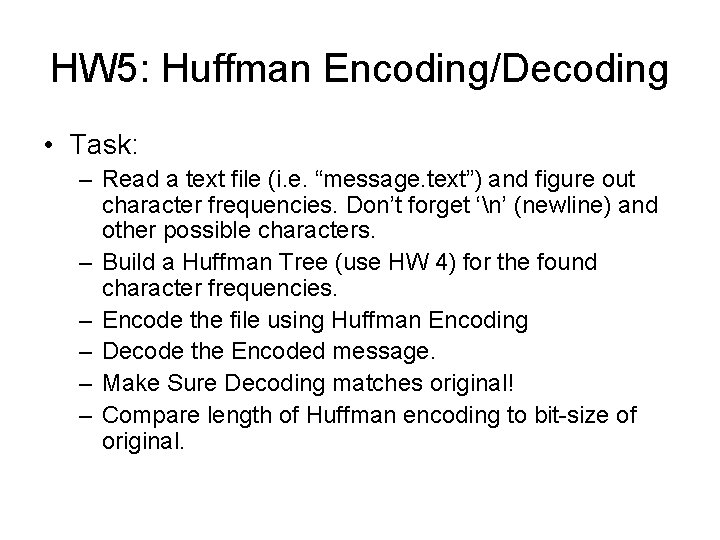
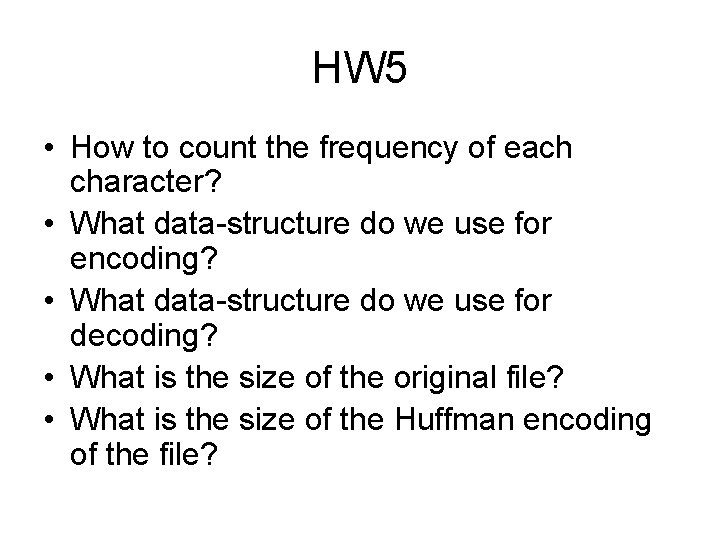
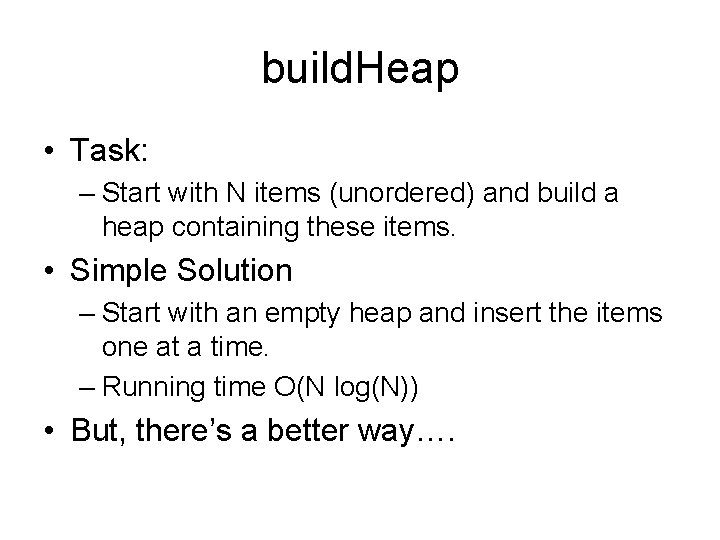
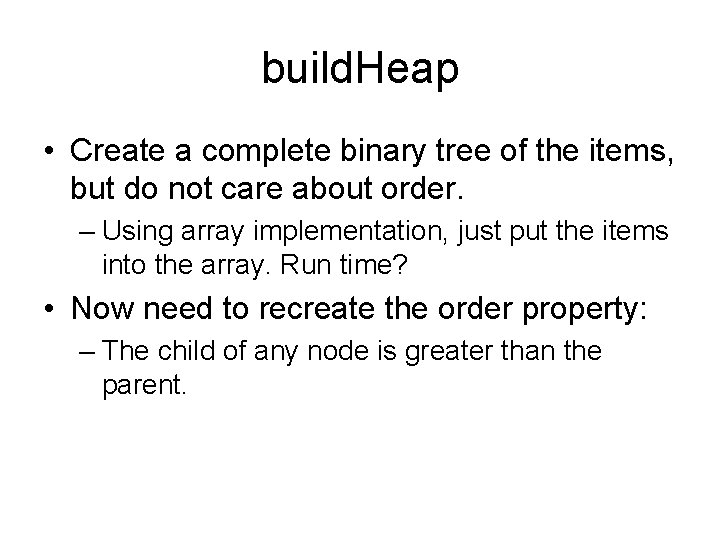
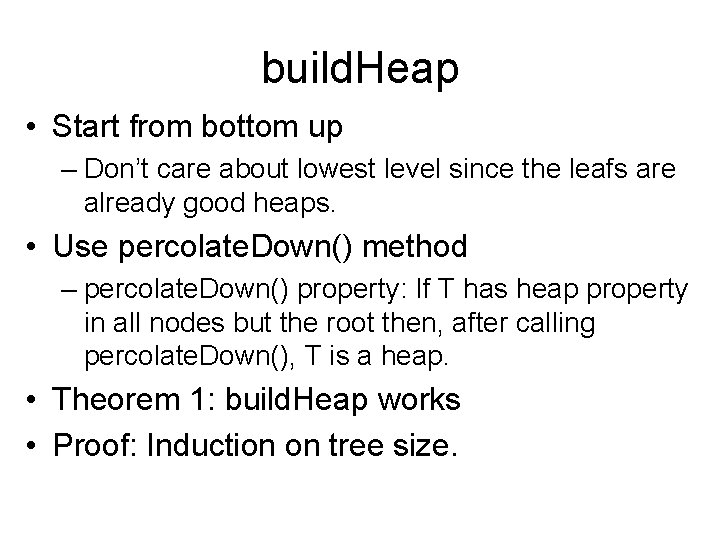
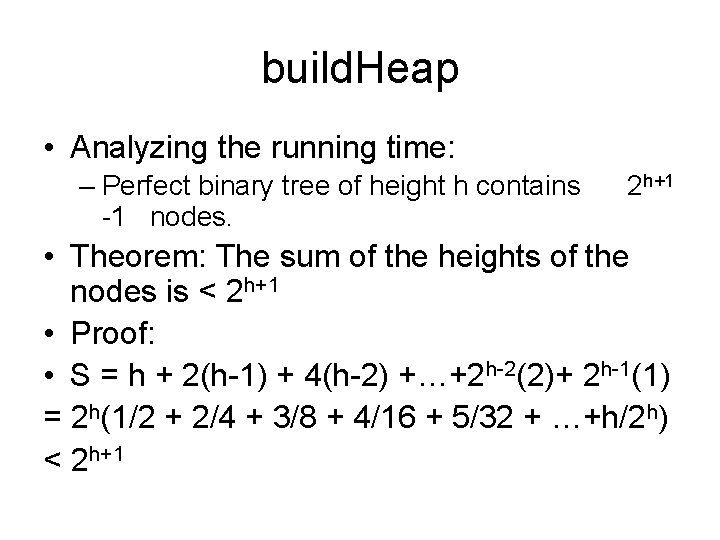
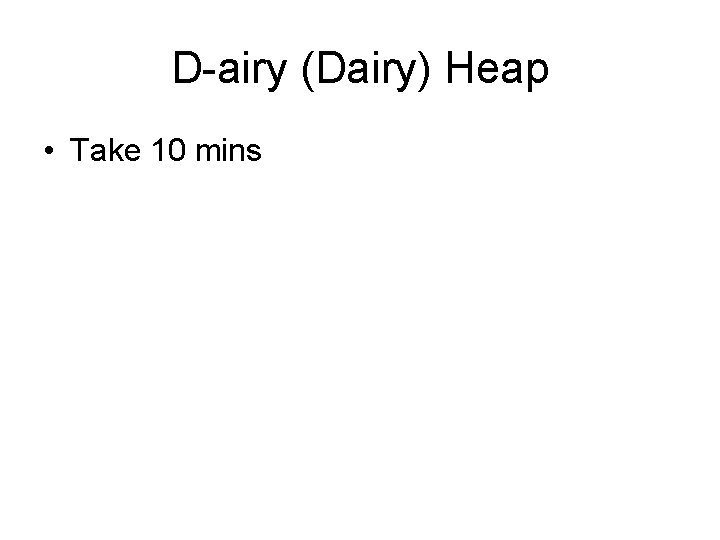
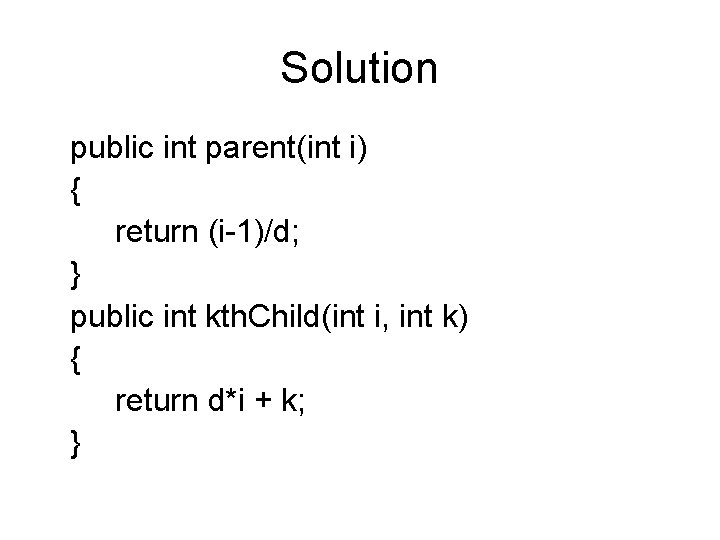
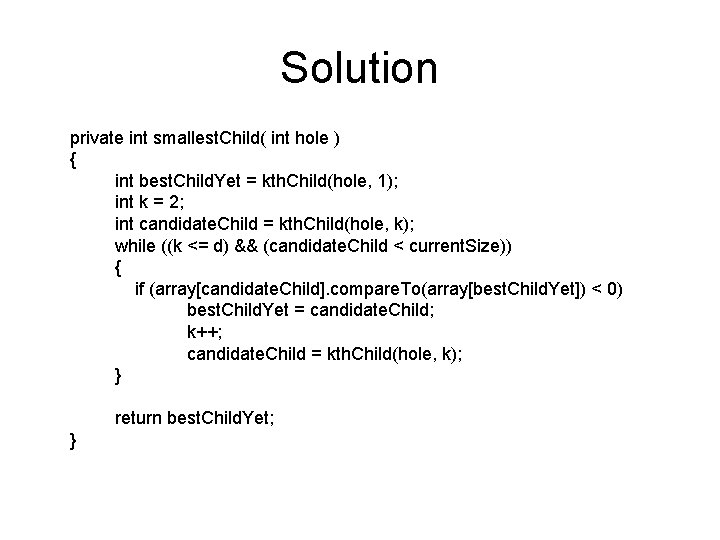
![Solution private void percolate. Up ( int hole ) { Comparable tmp = array[hole]; Solution private void percolate. Up ( int hole ) { Comparable tmp = array[hole];](https://slidetodoc.com/presentation_image/3a4ba97368b7f96702dc179f62637faa/image-11.jpg)
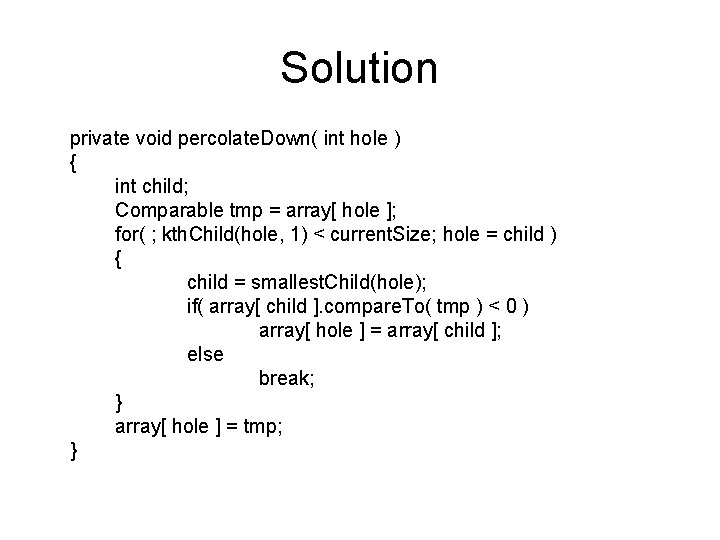
- Slides: 12
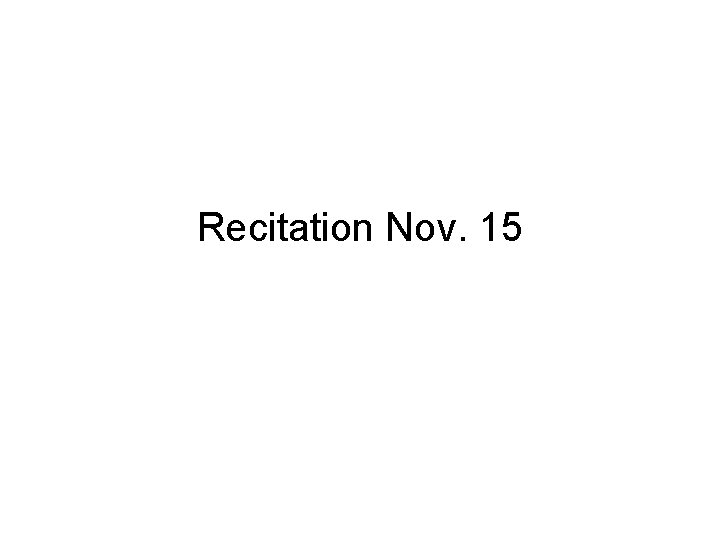
Recitation Nov. 15
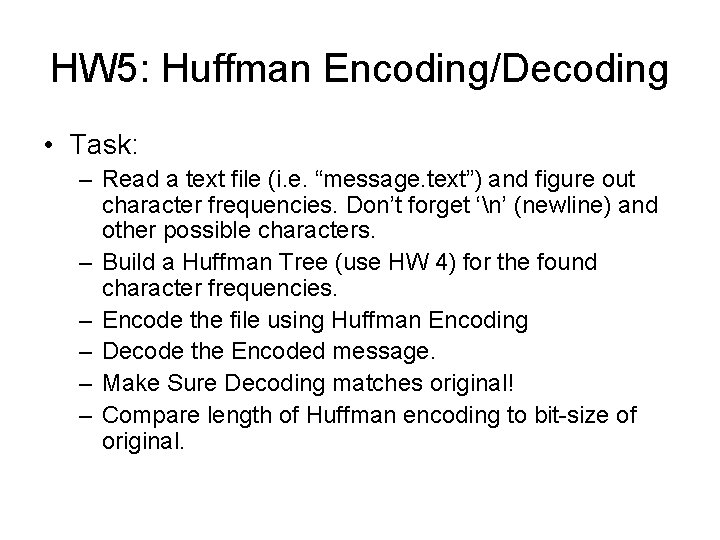
HW 5: Huffman Encoding/Decoding • Task: – Read a text file (i. e. “message. text”) and figure out character frequencies. Don’t forget ‘n’ (newline) and other possible characters. – Build a Huffman Tree (use HW 4) for the found character frequencies. – Encode the file using Huffman Encoding – Decode the Encoded message. – Make Sure Decoding matches original! – Compare length of Huffman encoding to bit-size of original.
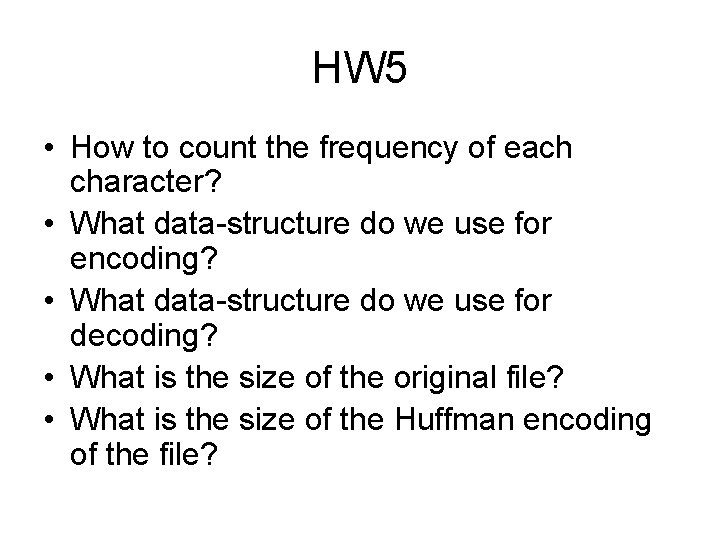
HW 5 • How to count the frequency of each character? • What data-structure do we use for encoding? • What data-structure do we use for decoding? • What is the size of the original file? • What is the size of the Huffman encoding of the file?
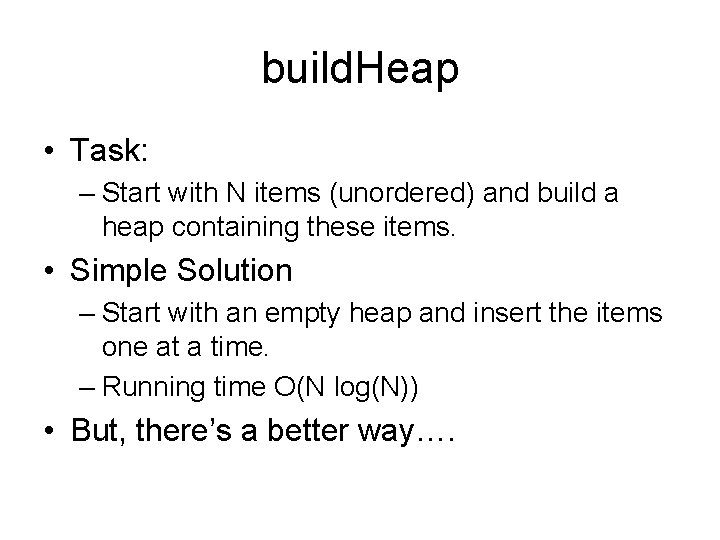
build. Heap • Task: – Start with N items (unordered) and build a heap containing these items. • Simple Solution – Start with an empty heap and insert the items one at a time. – Running time O(N log(N)) • But, there’s a better way….
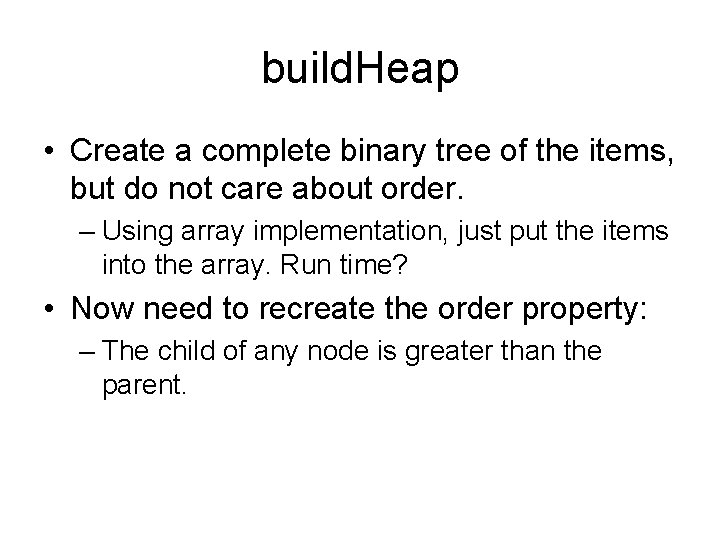
build. Heap • Create a complete binary tree of the items, but do not care about order. – Using array implementation, just put the items into the array. Run time? • Now need to recreate the order property: – The child of any node is greater than the parent.
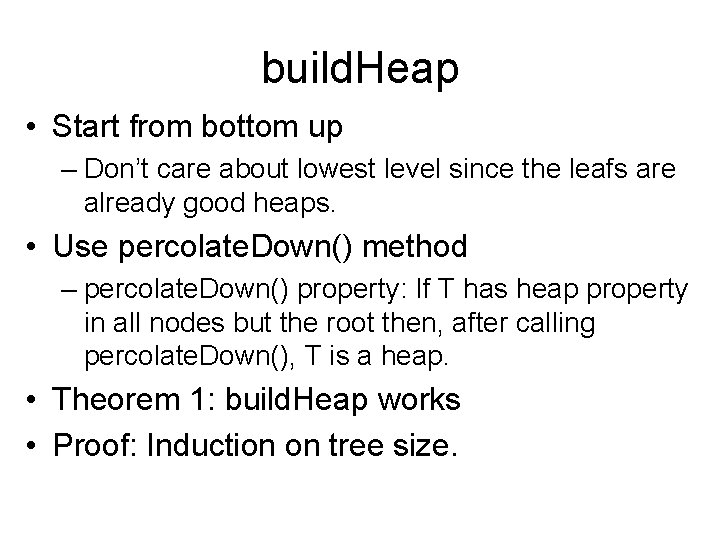
build. Heap • Start from bottom up – Don’t care about lowest level since the leafs are already good heaps. • Use percolate. Down() method – percolate. Down() property: If T has heap property in all nodes but the root then, after calling percolate. Down(), T is a heap. • Theorem 1: build. Heap works • Proof: Induction on tree size.
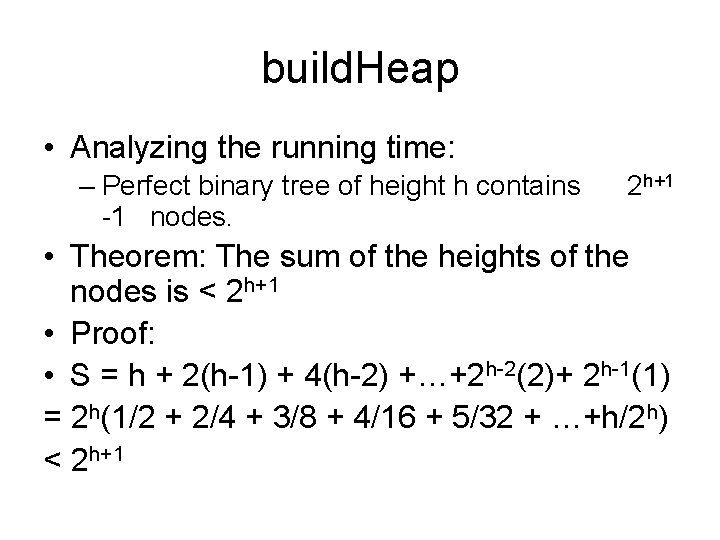
build. Heap • Analyzing the running time: – Perfect binary tree of height h contains -1 nodes. 2 h+1 • Theorem: The sum of the heights of the nodes is < 2 h+1 • Proof: • S = h + 2(h-1) + 4(h-2) +…+2 h-2(2)+ 2 h-1(1) = 2 h(1/2 + 2/4 + 3/8 + 4/16 + 5/32 + …+h/2 h) < 2 h+1
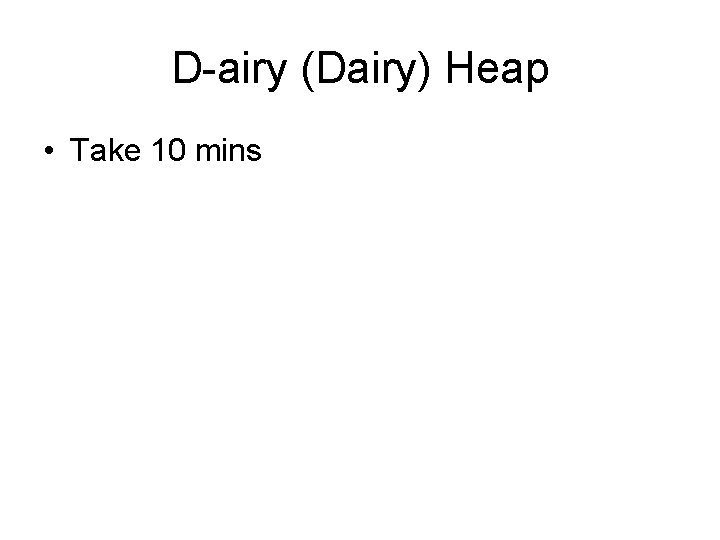
D-airy (Dairy) Heap • Take 10 mins
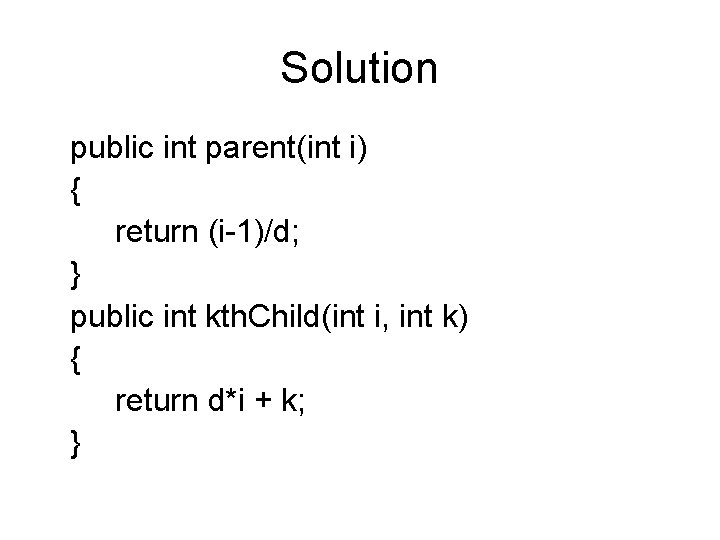
Solution public int parent(int i) { return (i-1)/d; } public int kth. Child(int i, int k) { return d*i + k; }
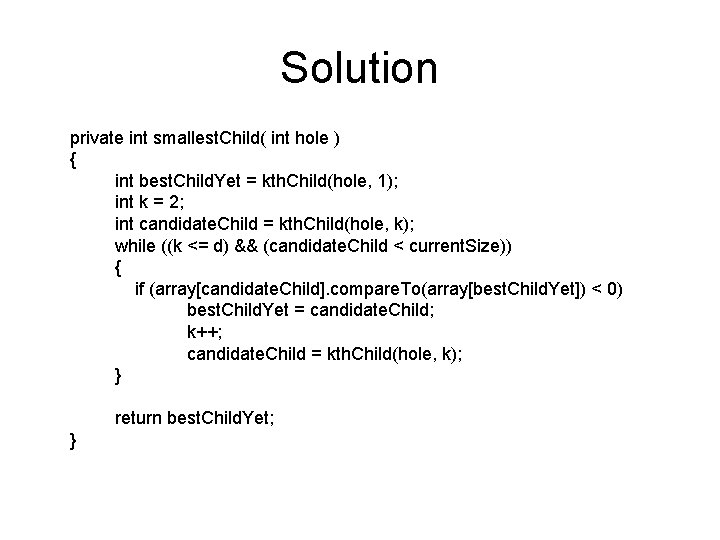
Solution private int smallest. Child( int hole ) { int best. Child. Yet = kth. Child(hole, 1); int k = 2; int candidate. Child = kth. Child(hole, k); while ((k <= d) && (candidate. Child < current. Size)) { if (array[candidate. Child]. compare. To(array[best. Child. Yet]) < 0) best. Child. Yet = candidate. Child; k++; candidate. Child = kth. Child(hole, k); } return best. Child. Yet; }
![Solution private void percolate Up int hole Comparable tmp arrayhole Solution private void percolate. Up ( int hole ) { Comparable tmp = array[hole];](https://slidetodoc.com/presentation_image/3a4ba97368b7f96702dc179f62637faa/image-11.jpg)
Solution private void percolate. Up ( int hole ) { Comparable tmp = array[hole]; for (; hole > 0 && tmp. compare. To(array[parent(hole)] ) < 0; hole = parent(hole)) array[ hole ] = array[ parent(hole) ]; array[ hole ] = tmp; }
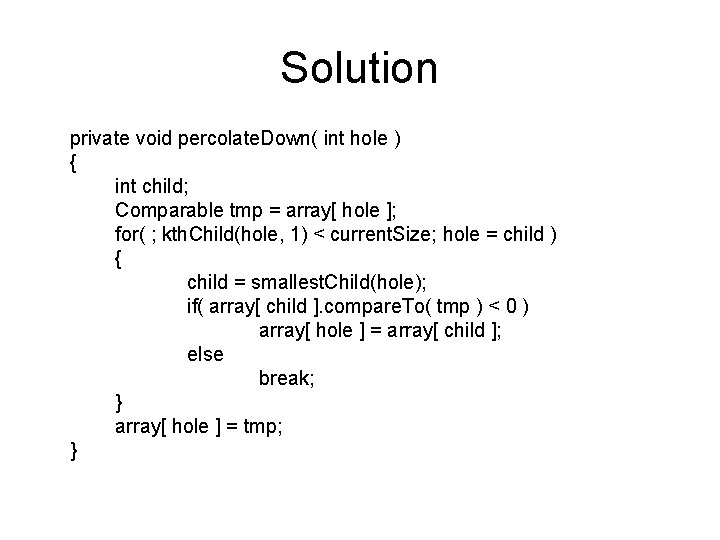
Solution private void percolate. Down( int hole ) { int child; Comparable tmp = array[ hole ]; for( ; kth. Child(hole, 1) < current. Size; hole = child ) { child = smallest. Child(hole); if( array[ child ]. compare. To( tmp ) < 0 ) array[ hole ] = array[ child ]; else break; } array[ hole ] = tmp; }
Rote recitation of a written message
Xvvvxv
Objectives of poem recitation
Criteria for performance task
Active recitation
Know your material
100belaud
Quood posture 8
What is meant by etiquette of recitation of the holy quran
Colbert, stephen. home page. 1 nov. 2006.
Nov 19 1863
"set out nov dez levantamento bibliográfico"
Söz birləşmələri sintaktik əlaqələr