Program Development Procedures 1 Program definition clearly define
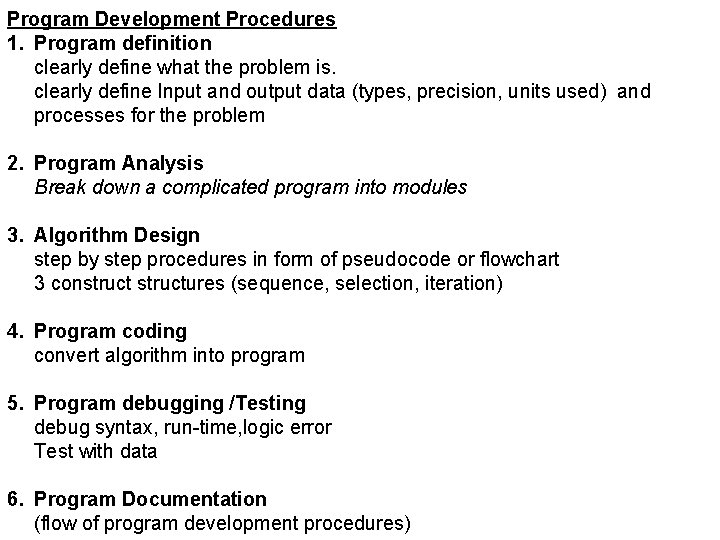
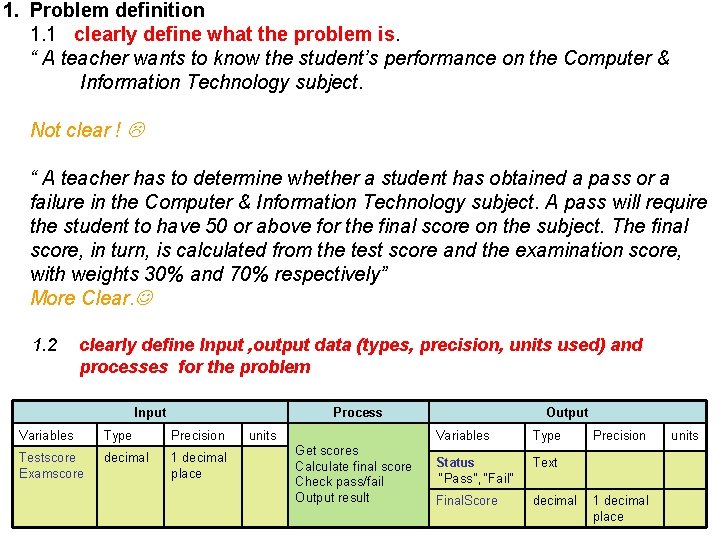
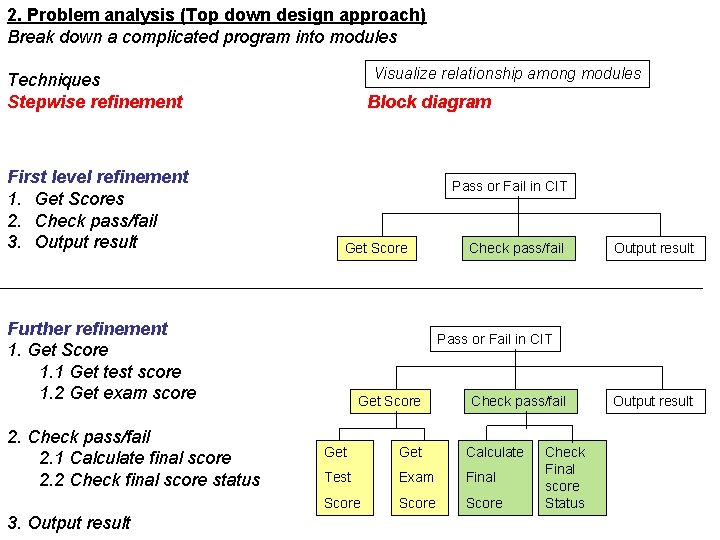
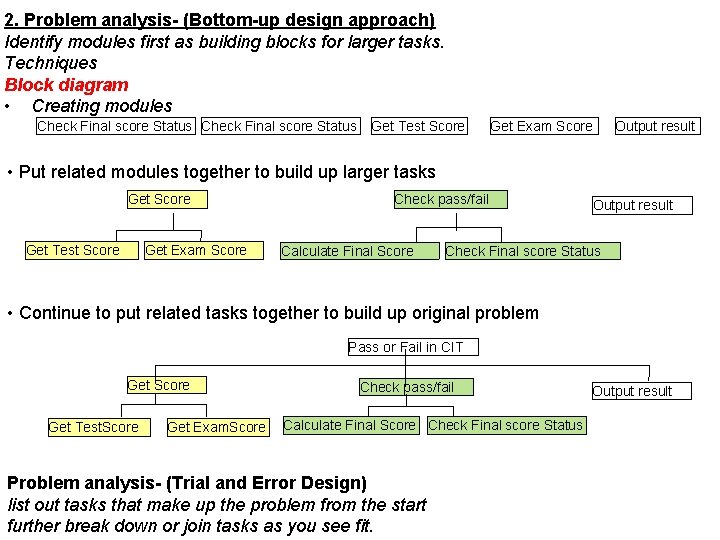
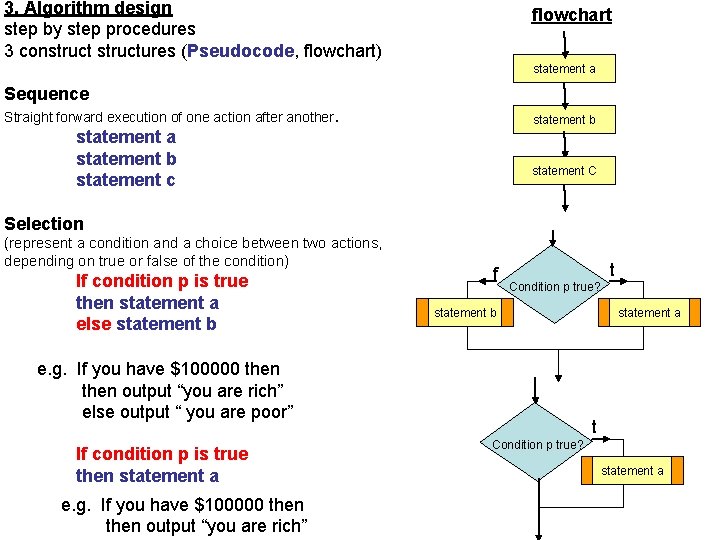
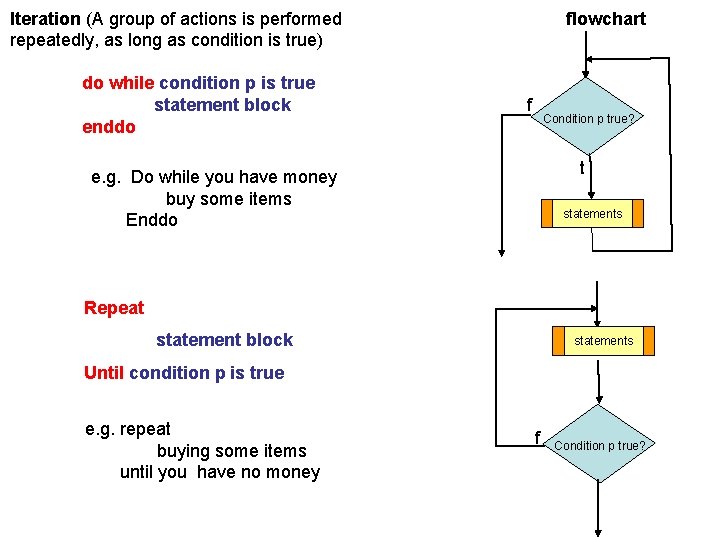
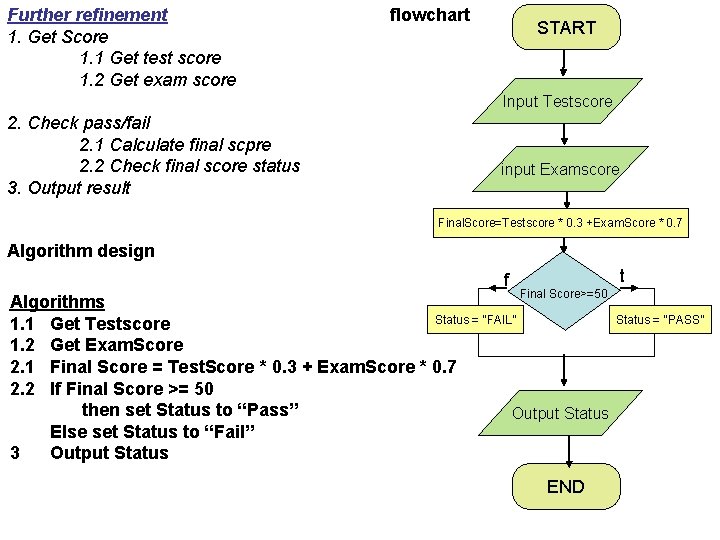
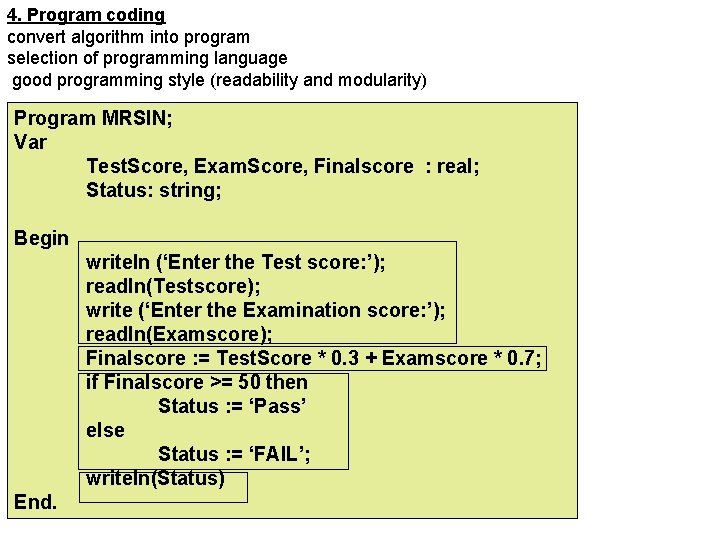
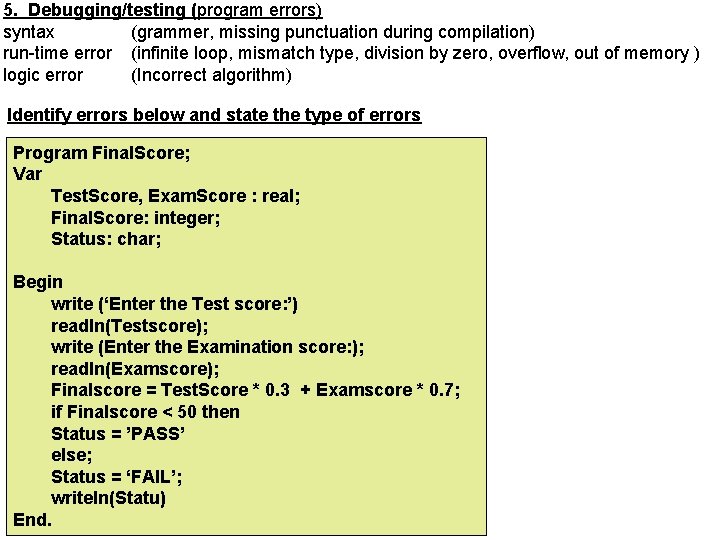
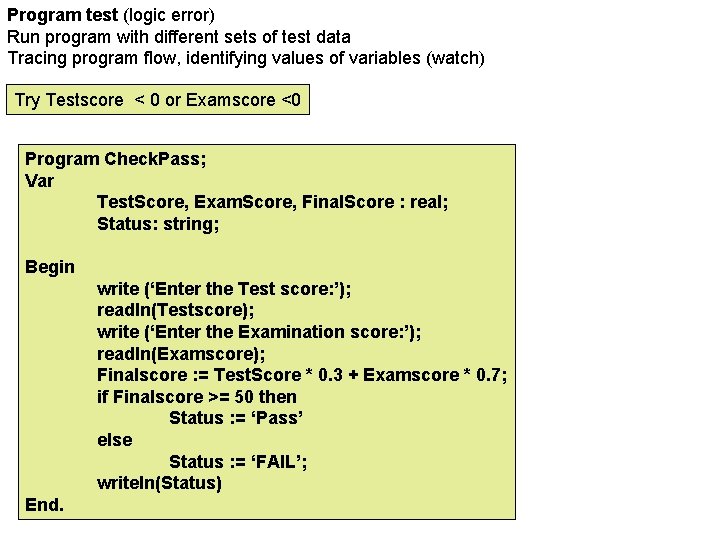
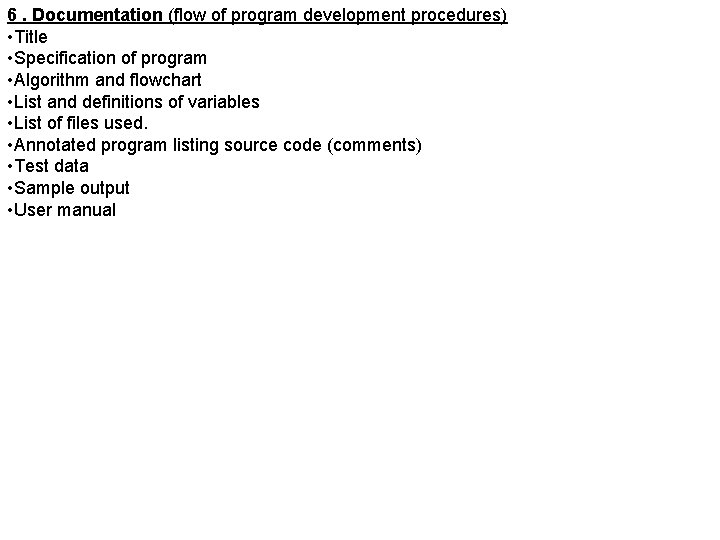
- Slides: 11
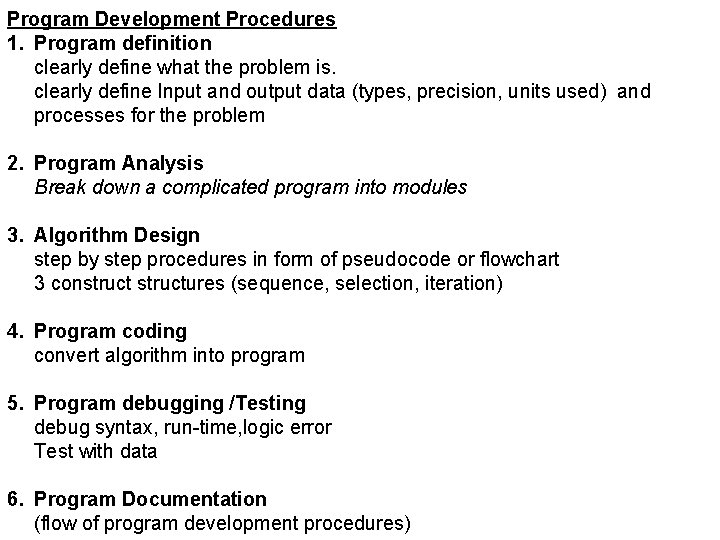
Program Development Procedures 1. Program definition clearly define what the problem is. clearly define Input and output data (types, precision, units used) and processes for the problem 2. Program Analysis Break down a complicated program into modules 3. Algorithm Design step by step procedures in form of pseudocode or flowchart 3 constructures (sequence, selection, iteration) 4. Program coding convert algorithm into program 5. Program debugging /Testing debug syntax, run-time, logic error Test with data 6. Program Documentation (flow of program development procedures)
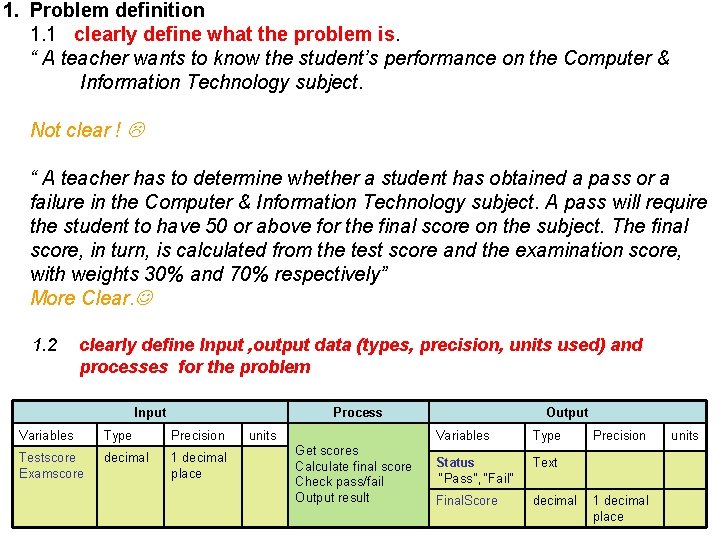
1. Problem definition 1. 1 clearly define what the problem is. “ A teacher wants to know the student’s performance on the Computer & Information Technology subject. Not clear ! “ A teacher has to determine whether a student has obtained a pass or a failure in the Computer & Information Technology subject. A pass will require the student to have 50 or above for the final score on the subject. The final score, in turn, is calculated from the test score and the examination score, with weights 30% and 70% respectively” More Clear. 1. 2 clearly define Input , output data (types, precision, units used) and processes for the problem Input Process Variables Type Precision Testscore Examscore decimal 1 decimal place units Get scores Calculate final score Check pass/fail Output result Output Variables Type Status “Pass”, “Fail” Text Final. Score decimal Precision 1 decimal place units
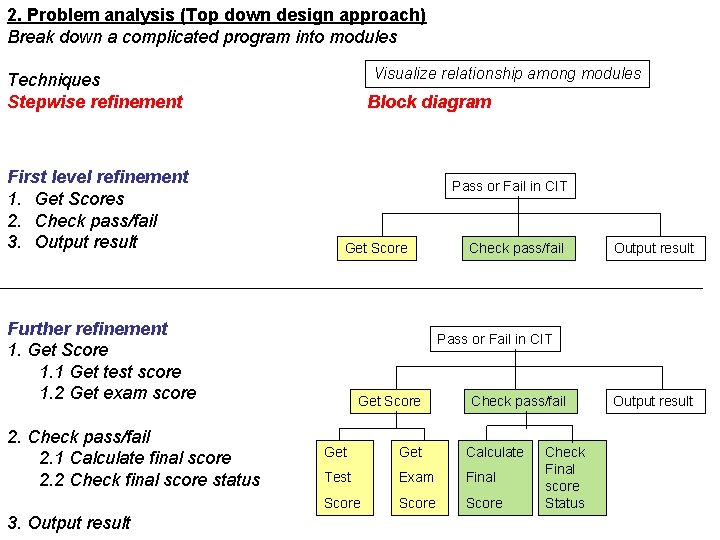
2. Problem analysis (Top down design approach) Break down a complicated program into modules Visualize relationship among modules Techniques Stepwise refinement First level refinement 1. Get Scores 2. Check pass/fail 3. Output result Block diagram Pass or Fail in CIT Get Score Further refinement 1. Get Score 1. 1 Get test score 1. 2 Get exam score 2. Check pass/fail 2. 1 Calculate final score 2. 2 Check final score status 3. Output result Check pass/fail Output result Pass or Fail in CIT Get Score Check pass/fail Get Calculate Test Exam Final Score Check Final score Status Output result
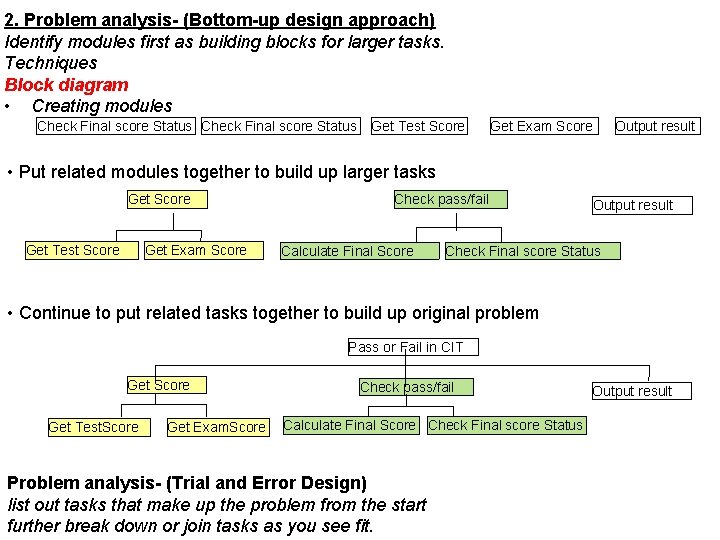
2. Problem analysis- (Bottom-up design approach) Identify modules first as building blocks for larger tasks. Techniques Block diagram • Creating modules Check Final score Status Get Test Score Get Exam Score Output result • Put related modules together to build up larger tasks Get Score Get Test Score Get Exam Score Check pass/fail Calculate Final Score Output result Check Final score Status • Continue to put related tasks together to build up original problem Pass or Fail in CIT Get Score Get Test. Score Get Exam. Score Check pass/fail Calculate Final Score Check Final score Status Problem analysis- (Trial and Error Design) list out tasks that make up the problem from the start further break down or join tasks as you see fit. Output result
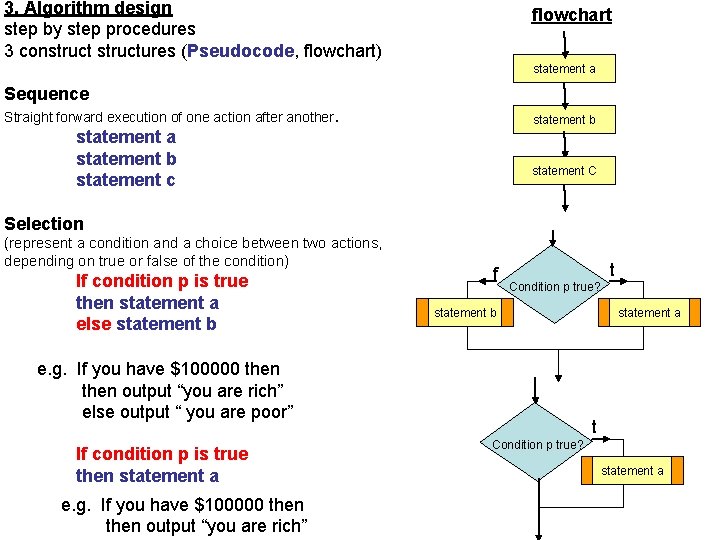
3. Algorithm design step by step procedures 3 constructures (Pseudocode, flowchart) flowchart statement a Sequence Straight forward execution of one action after another. statement b statement a statement b statement c statement C Selection (represent a condition and a choice between two actions, depending on true or false of the condition) If condition p is true then statement a else statement b f t Condition p true? statement b e. g. If you have $100000 then output “you are rich” else output “ you are poor” If condition p is true then statement a e. g. If you have $100000 then output “you are rich” statement a t Condition p true? statement a
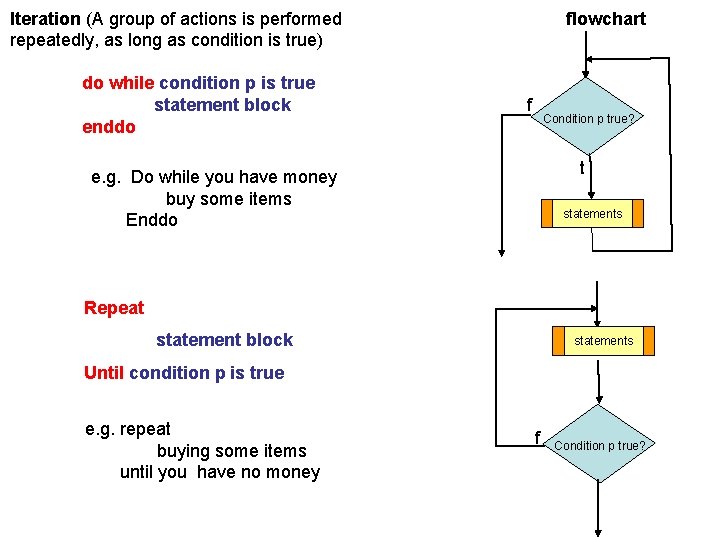
Iteration (A group of actions is performed repeatedly, as long as condition is true) do while condition p is true statement block enddo flowchart f Condition p true? t e. g. Do while you have money buy some items Enddo statements Repeat statement block statements Until condition p is true e. g. repeat buying some items until you have no money f Condition p true?
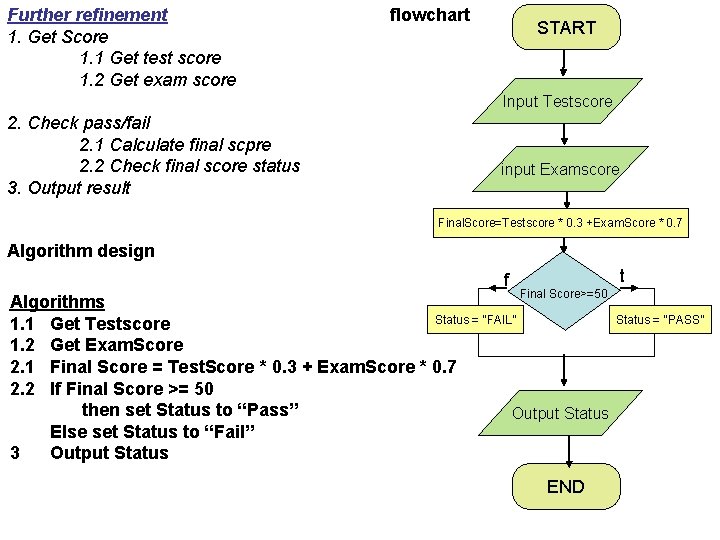
Further refinement 1. Get Score 1. 1 Get test score 1. 2 Get exam score flowchart START Input Testscore 2. Check pass/fail 2. 1 Calculate final scpre 2. 2 Check final score status 3. Output result input Examscore Final. Score=Testscore * 0. 3 +Exam. Score * 0. 7 Algorithm design f t Final Score>=50 Algorithms Status = “FAIL” 1. 1 Get Testscore 1. 2 Get Exam. Score 2. 1 Final Score = Test. Score * 0. 3 + Exam. Score * 0. 7 2. 2 If Final Score >= 50 then set Status to “Pass” Output Status Else set Status to “Fail” 3 Output Status END Status = “PASS”
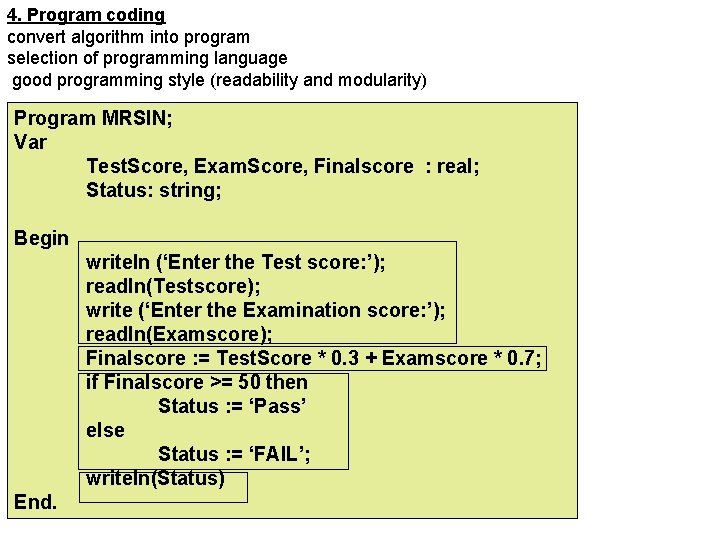
4. Program coding convert algorithm into program selection of programming language good programming style (readability and modularity) Program MRSIN; Var Test. Score, Exam. Score, Finalscore : real; Status: string; Begin writeln (‘Enter the Test score: ’); readln(Testscore); write (‘Enter the Examination score: ’); readln(Examscore); Finalscore : = Test. Score * 0. 3 + Examscore * 0. 7; if Finalscore >= 50 then Status : = ‘Pass’ else Status : = ‘FAIL’; writeln(Status) End.
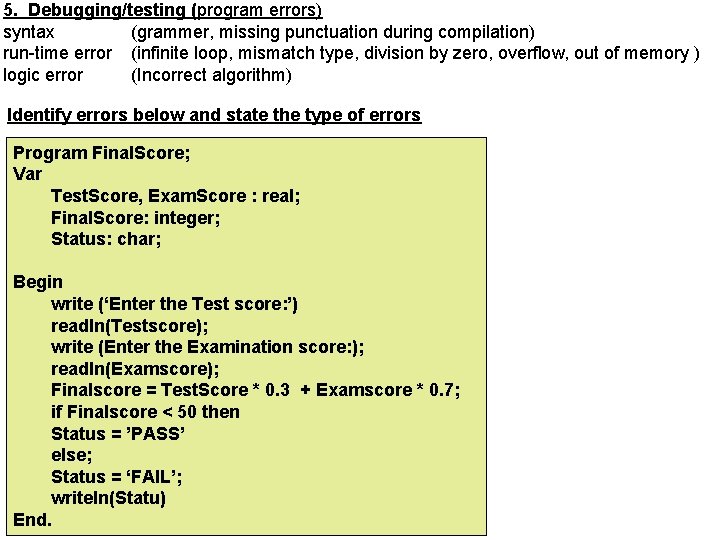
5. Debugging/testing (program errors) syntax (grammer, missing punctuation during compilation) run-time error (infinite loop, mismatch type, division by zero, overflow, out of memory ) logic error (Incorrect algorithm) Identify errors below and state the type of errors Program Final. Score; Var Test. Score, Exam. Score : real; Final. Score: integer; Status: char; Begin write (‘Enter the Test score: ’) readln(Testscore); write (Enter the Examination score: ); readln(Examscore); Finalscore = Test. Score * 0. 3 + Examscore * 0. 7; if Finalscore < 50 then Status = ’PASS’ else; Status = ‘FAIL’; writeln(Statu) End.
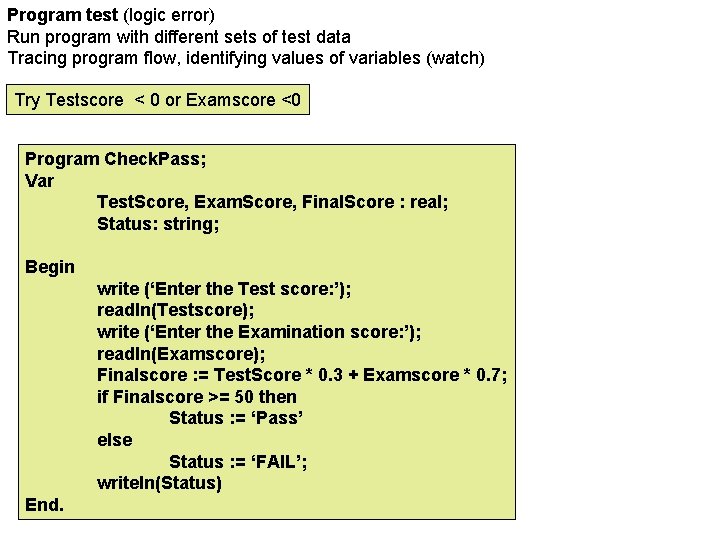
Program test (logic error) Run program with different sets of test data Tracing program flow, identifying values of variables (watch) Try Testscore < 0 or Examscore <0 Program Check. Pass; Var Test. Score, Exam. Score, Final. Score : real; Status: string; Begin write (‘Enter the Test score: ’); readln(Testscore); write (‘Enter the Examination score: ’); readln(Examscore); Finalscore : = Test. Score * 0. 3 + Examscore * 0. 7; if Finalscore >= 50 then Status : = ‘Pass’ else Status : = ‘FAIL’; writeln(Status) End.
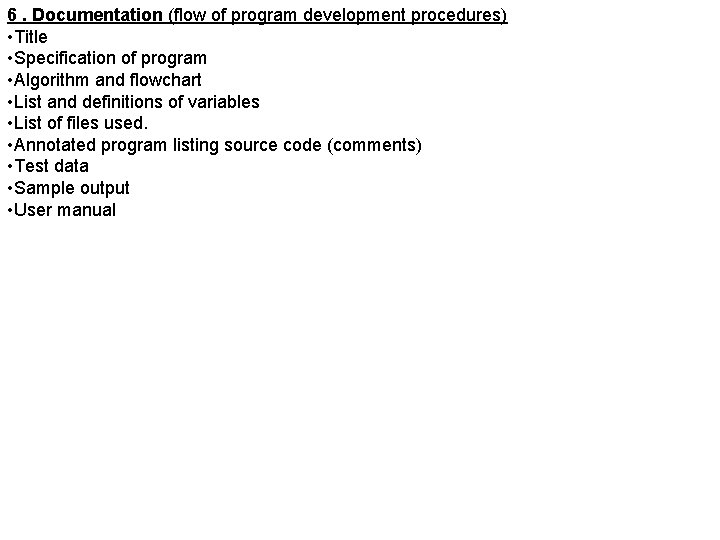
6. Documentation (flow of program development procedures) • Title • Specification of program • Algorithm and flowchart • List and definitions of variables • List of files used. • Annotated program listing source code (comments) • Test data • Sample output • User manual
Constrictures meaning
Eye can see clearly now
Response blocking vs extinction
Semantic elements
Two closed pyramid shaped beakers containing clearly
Modifiers of comparatives
Clearly trivial threshold
Site:slidetodoc.com
Modifiers of comparisons
How to write a supported opinion paragraph
Josh starmer
In the help strategy, the h stands for healthful.