Particle System Design The Challenge Particle systems vary
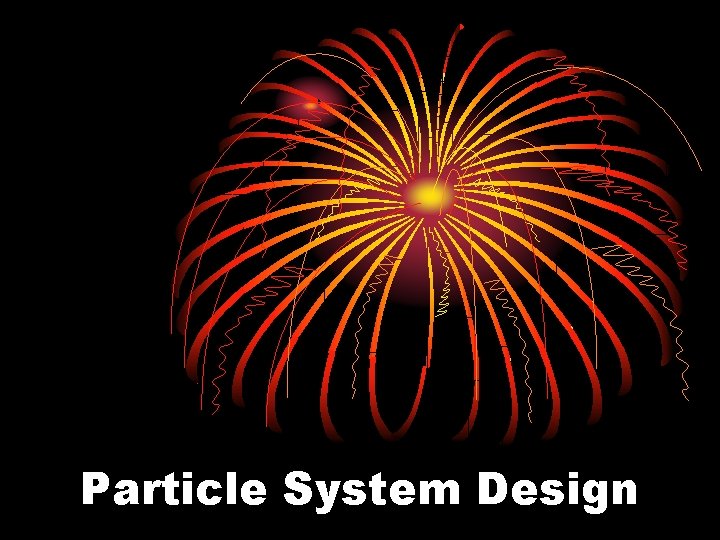
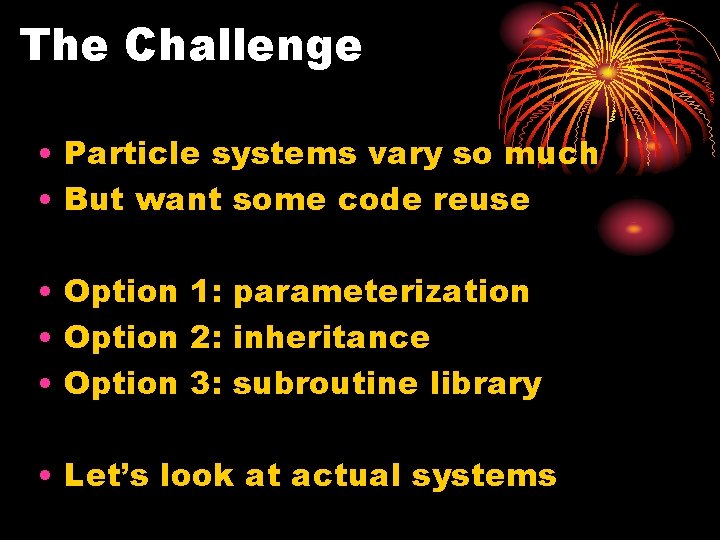
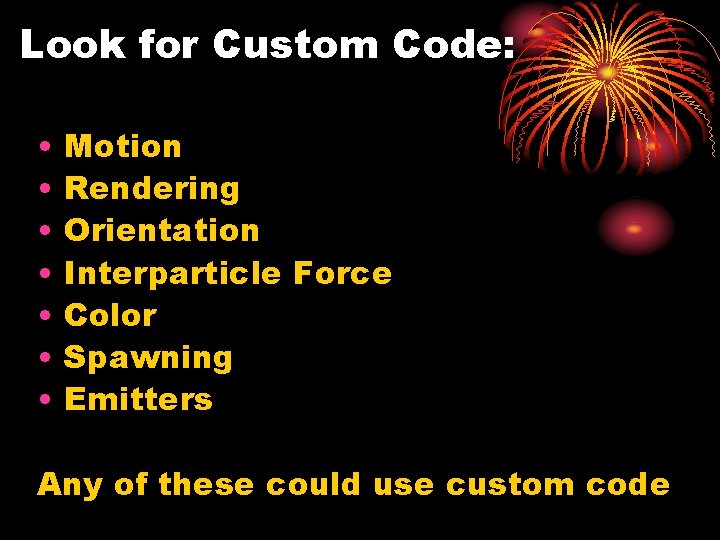
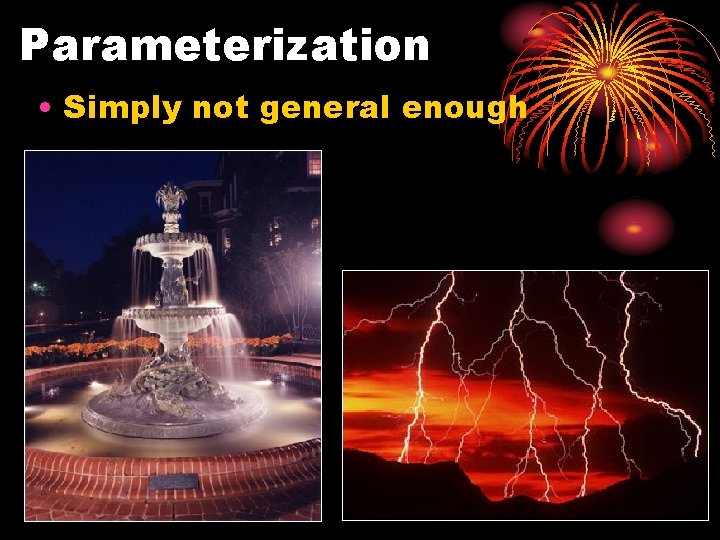
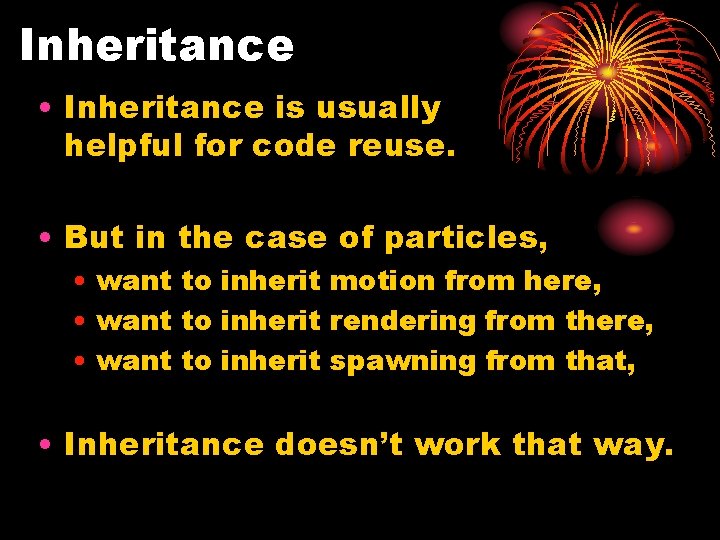
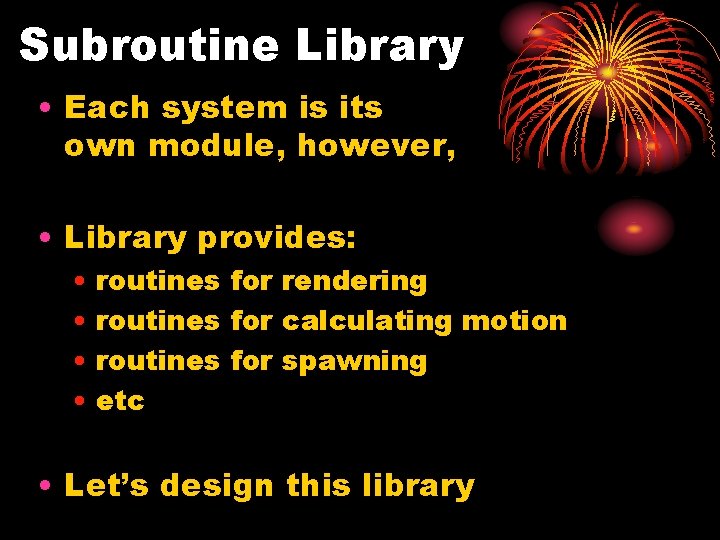
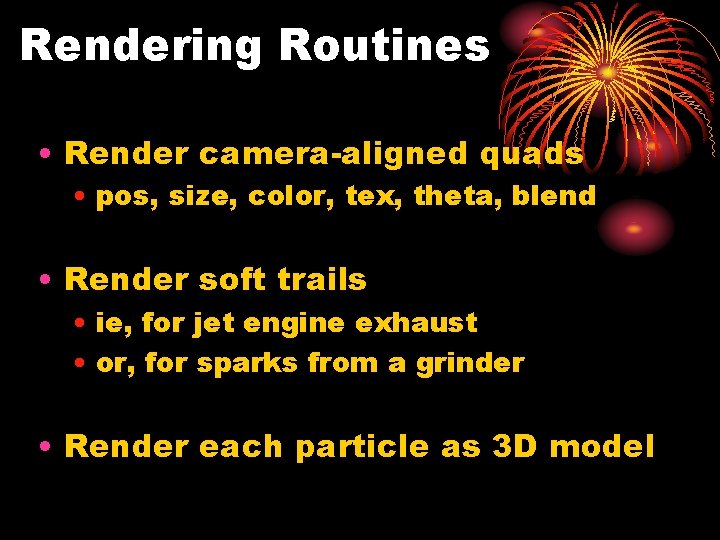
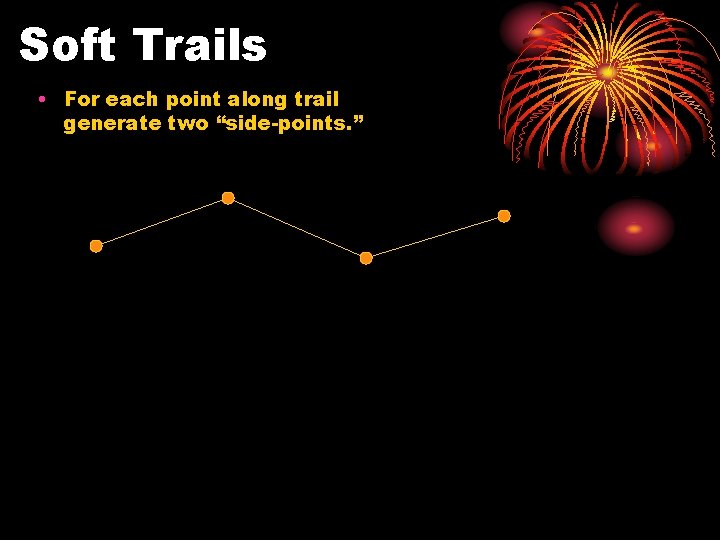
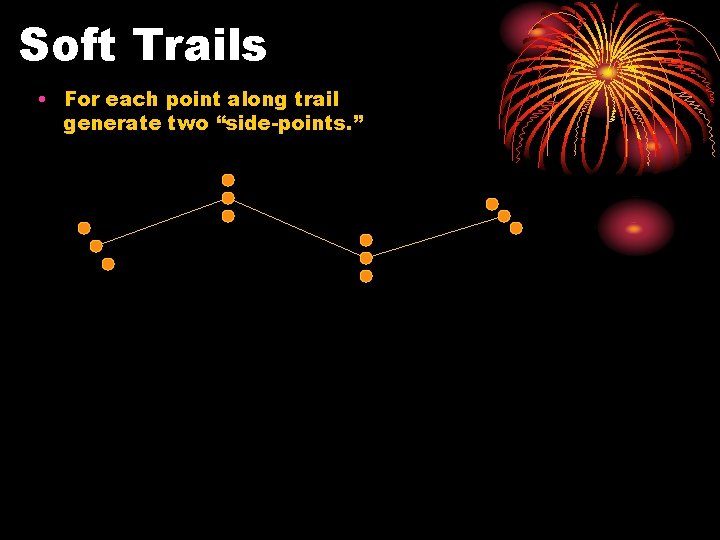
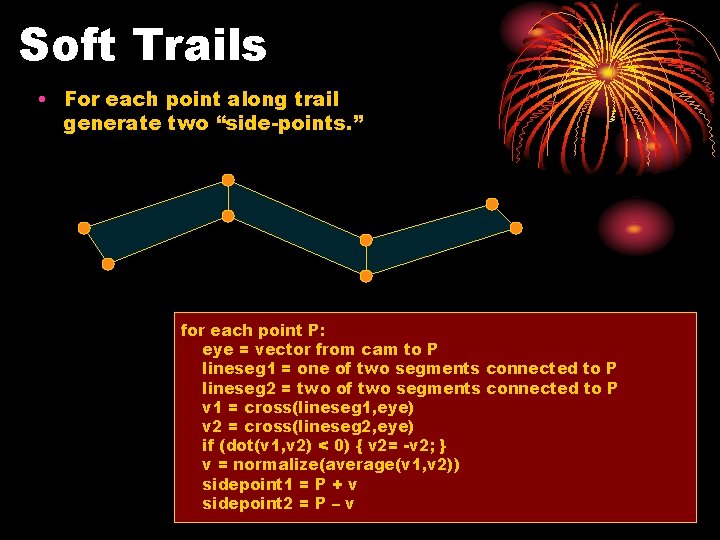
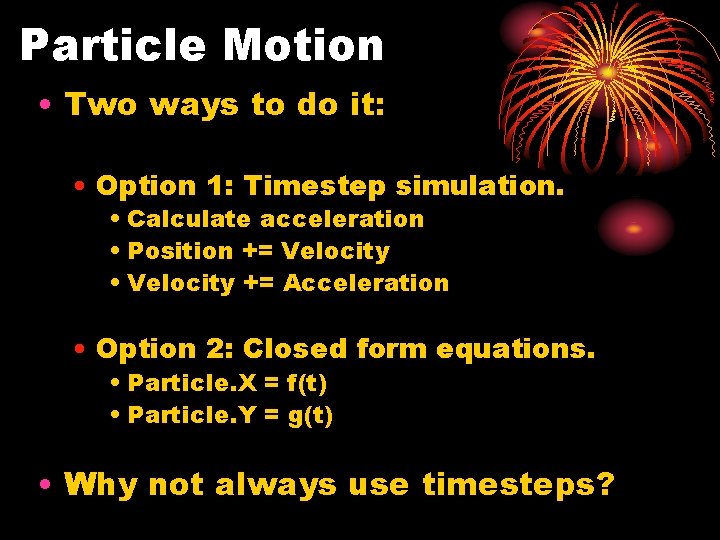
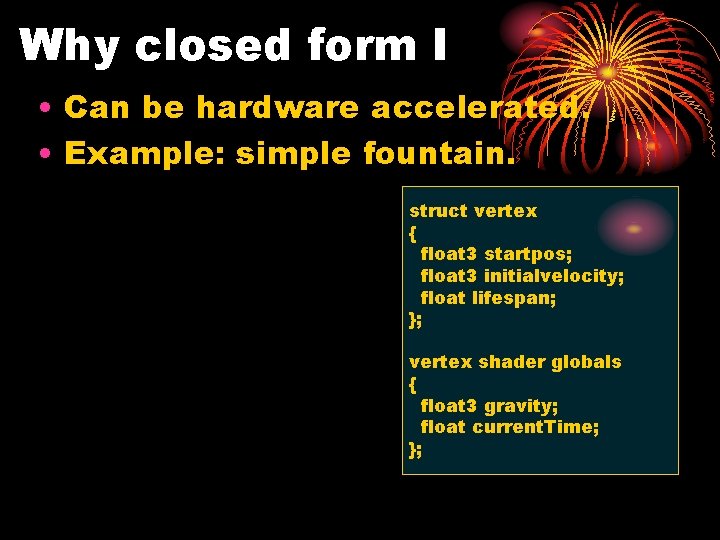
![Why closed form II • Can use prerecorded paths. • float position. X[1024]; • Why closed form II • Can use prerecorded paths. • float position. X[1024]; •](https://slidetodoc.com/presentation_image/409331f4335111945b135417356b1199/image-13.jpg)
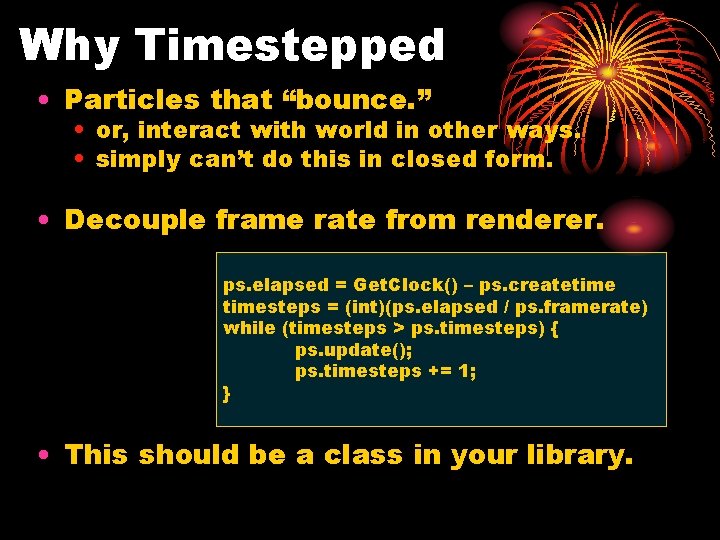
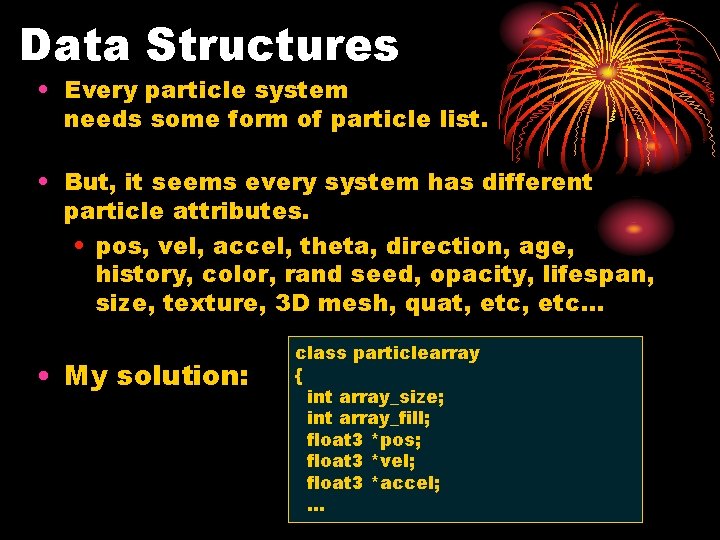
- Slides: 15
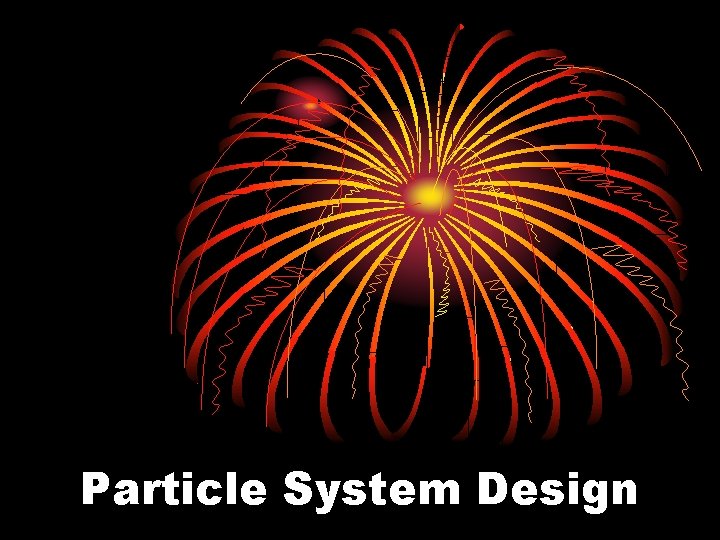
Particle System Design
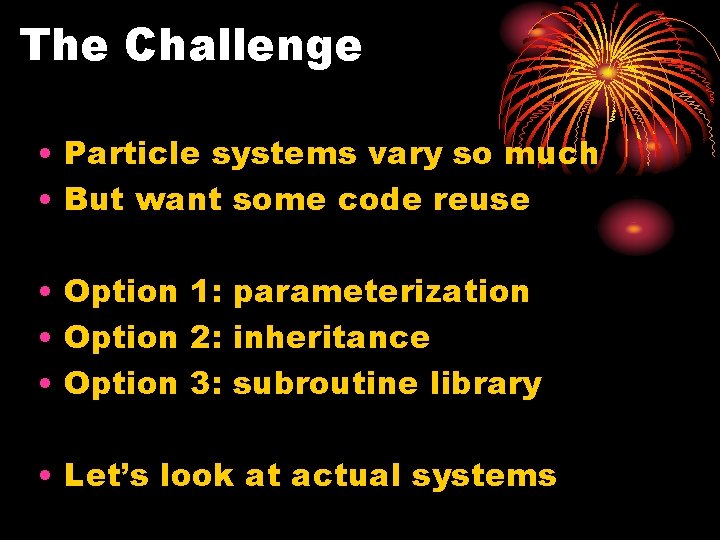
The Challenge • Particle systems vary so much • But want some code reuse • Option 1: parameterization • Option 2: inheritance • Option 3: subroutine library • Let’s look at actual systems
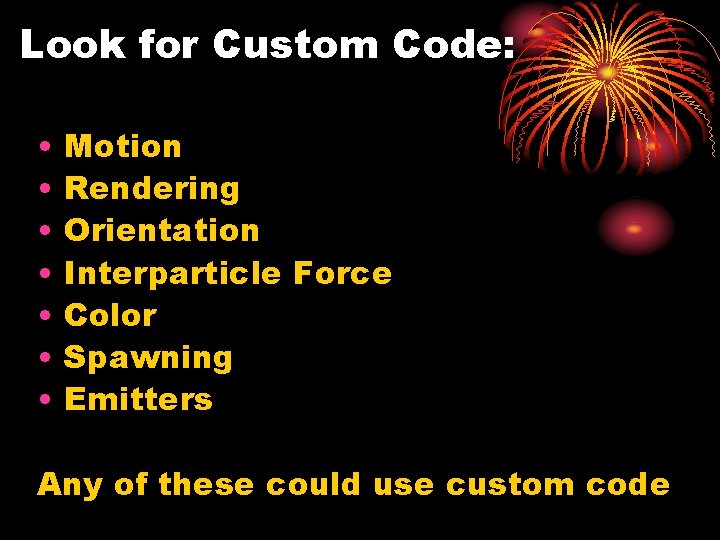
Look for Custom Code: • • Motion Rendering Orientation Interparticle Force Color Spawning Emitters Any of these could use custom code
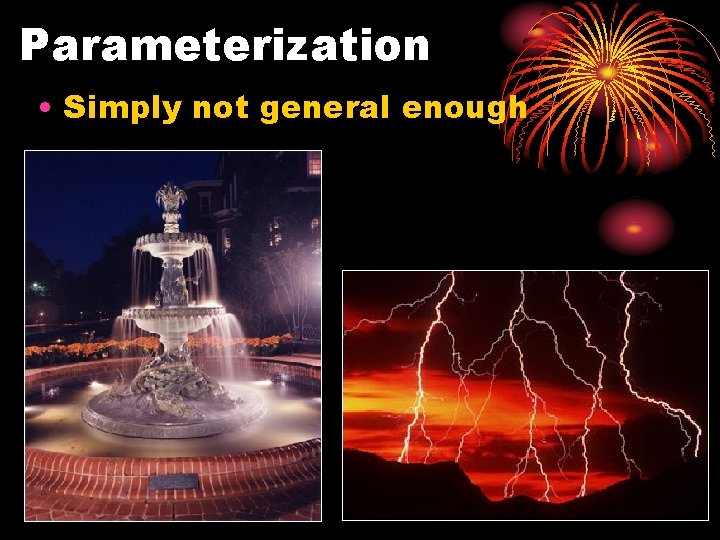
Parameterization • Simply not general enough
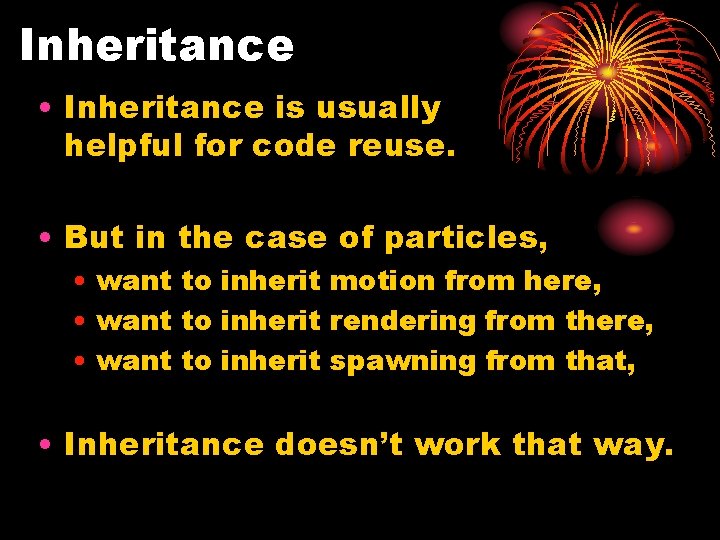
Inheritance • Inheritance is usually helpful for code reuse. • But in the case of particles, • want to inherit motion from here, • want to inherit rendering from there, • want to inherit spawning from that, • Inheritance doesn’t work that way.
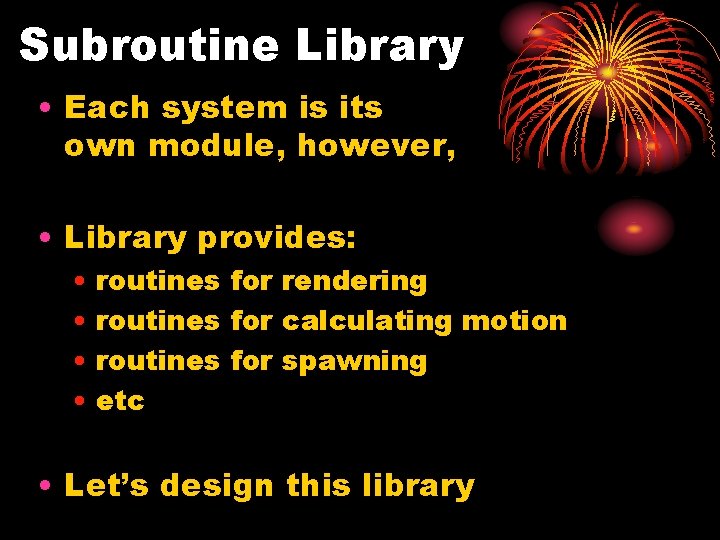
Subroutine Library • Each system is its own module, however, • Library provides: • routines for rendering • routines for calculating motion • routines for spawning • etc • Let’s design this library
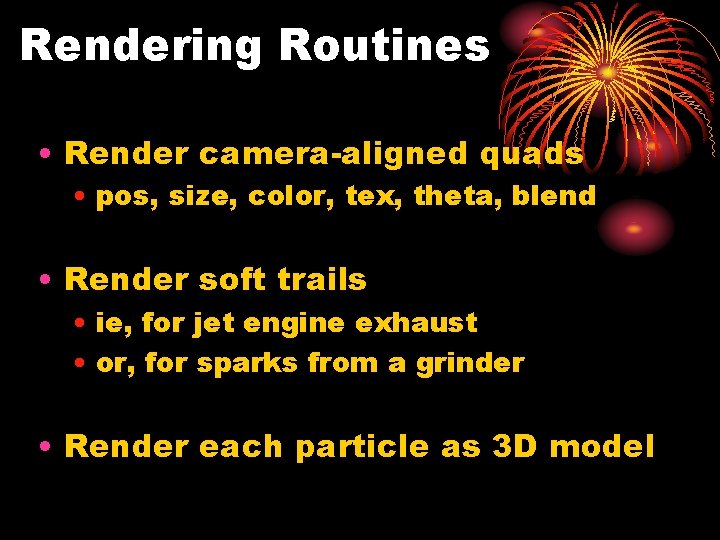
Rendering Routines • Render camera-aligned quads • pos, size, color, tex, theta, blend • Render soft trails • ie, for jet engine exhaust • or, for sparks from a grinder • Render each particle as 3 D model
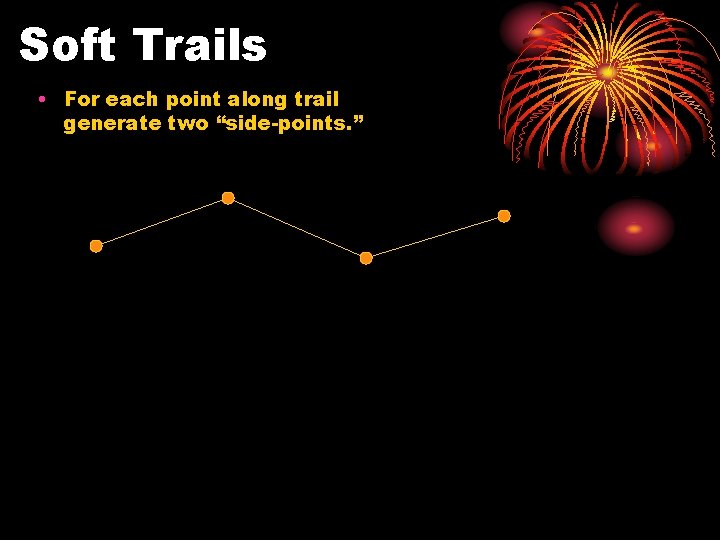
Soft Trails • For each point along trail generate two “side-points. ”
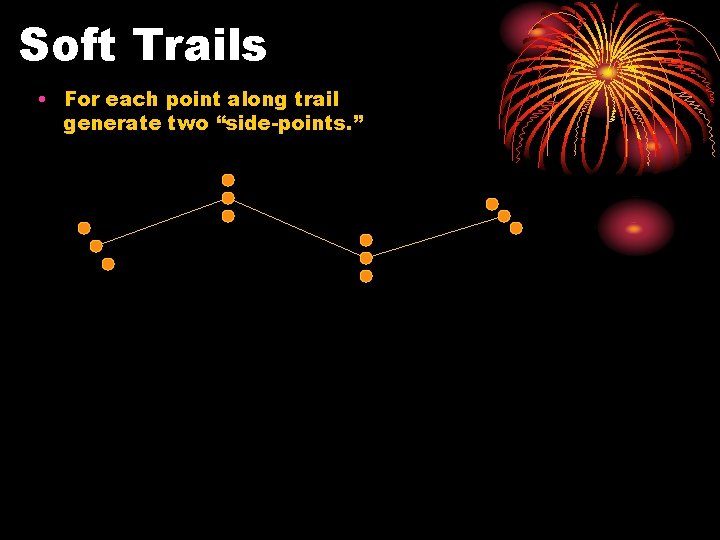
Soft Trails • For each point along trail generate two “side-points. ”
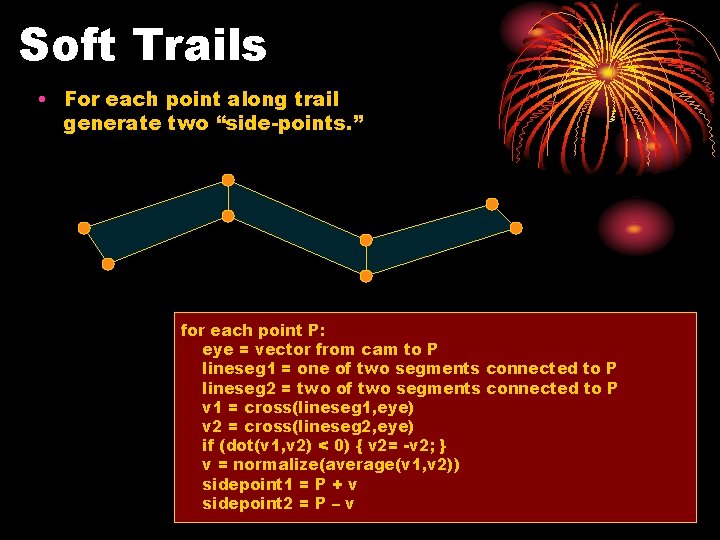
Soft Trails • For each point along trail generate two “side-points. ” for each point P: eye = vector from cam to P lineseg 1 = one of two segments connected to P lineseg 2 = two of two segments connected to P v 1 = cross(lineseg 1, eye) v 2 = cross(lineseg 2, eye) if (dot(v 1, v 2) < 0) { v 2= -v 2; } v = normalize(average(v 1, v 2)) sidepoint 1 = P + v sidepoint 2 = P – v
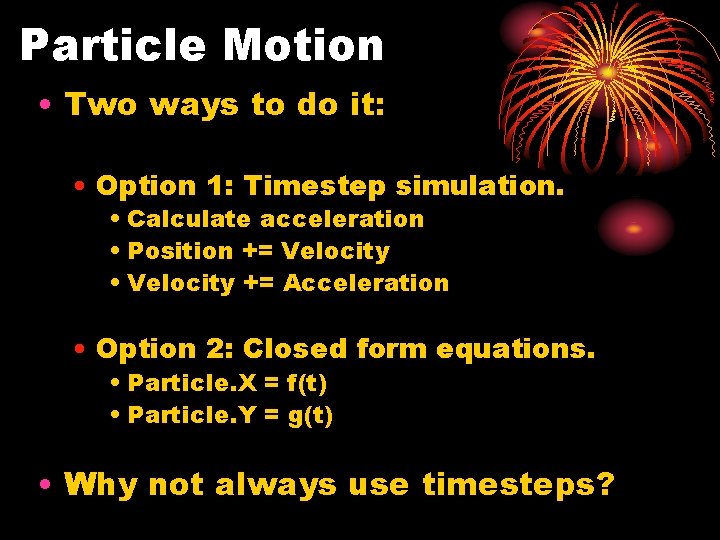
Particle Motion • Two ways to do it: • Option 1: Timestep simulation. • Calculate acceleration • Position += Velocity • Velocity += Acceleration • Option 2: Closed form equations. • Particle. X = f(t) • Particle. Y = g(t) • Why not always use timesteps?
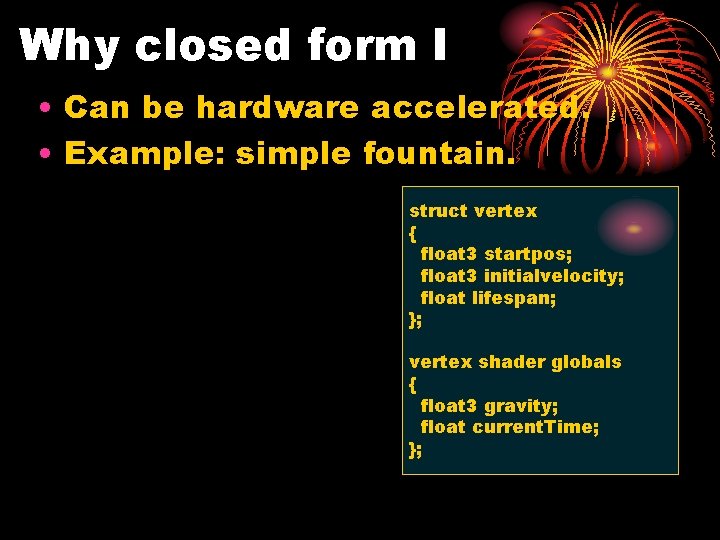
Why closed form I • Can be hardware accelerated. • Example: simple fountain. struct vertex { float 3 startpos; float 3 initialvelocity; float lifespan; }; vertex shader globals { float 3 gravity; float current. Time; };
![Why closed form II Can use prerecorded paths float position X1024 Why closed form II • Can use prerecorded paths. • float position. X[1024]; •](https://slidetodoc.com/presentation_image/409331f4335111945b135417356b1199/image-13.jpg)
Why closed form II • Can use prerecorded paths. • float position. X[1024]; • float position. Y[1024]; • Can add several prerecorded paths • multiple circles • wiggle + parabola • Can transform prerecorded path • smoke example • Library should contain many paths.
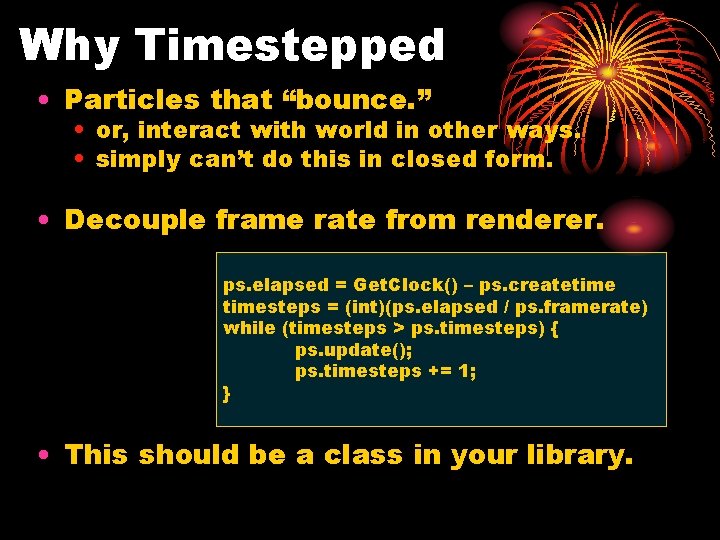
Why Timestepped • Particles that “bounce. ” • or, interact with world in other ways. • simply can’t do this in closed form. • Decouple frame rate from renderer. ps. elapsed = Get. Clock() – ps. createtimesteps = (int)(ps. elapsed / ps. framerate) while (timesteps > ps. timesteps) { ps. update(); ps. timesteps += 1; } • This should be a class in your library.
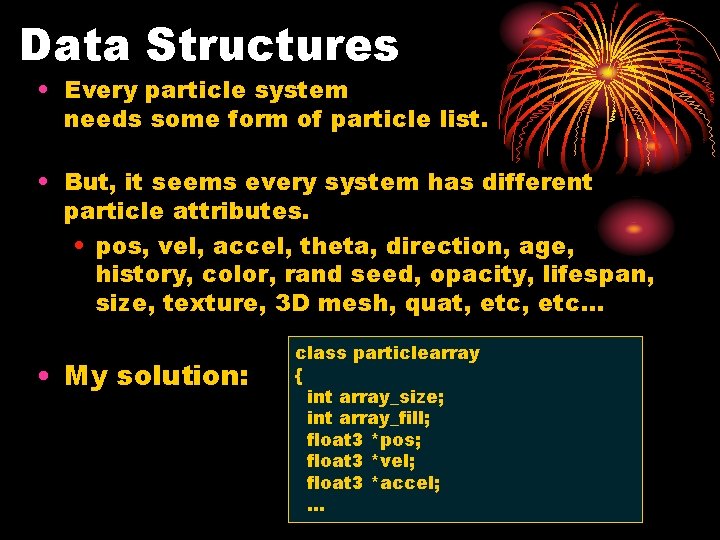
Data Structures • Every particle system needs some form of particle list. • But, it seems every system has different particle attributes. • pos, vel, accel, theta, direction, age, history, color, rand seed, opacity, lifespan, size, texture, 3 D mesh, quat, etc. . . • My solution: class particlearray { int array_size; int array_fill; float 3 *pos; float 3 *vel; float 3 *accel; . . .
Welcome to teen challenge uk - teen challenge uk
How do individual use languages vary among places
Vary your sentences
Lesson 1 darwins voyage of discovery
Species vary locally examples
Darwin's voyage of discovery
Pematex karlovy vary
Y varies inversely as x example
What should an abstract include
Why does development vary among countries?
Answers will vary
Adj of vary
What is an adverbial opener
Pr��ce karlovy vary
Key issue 3: why do individual languages vary among places?
People vary