Overview of Twitter API Nathan Liu Twitter API
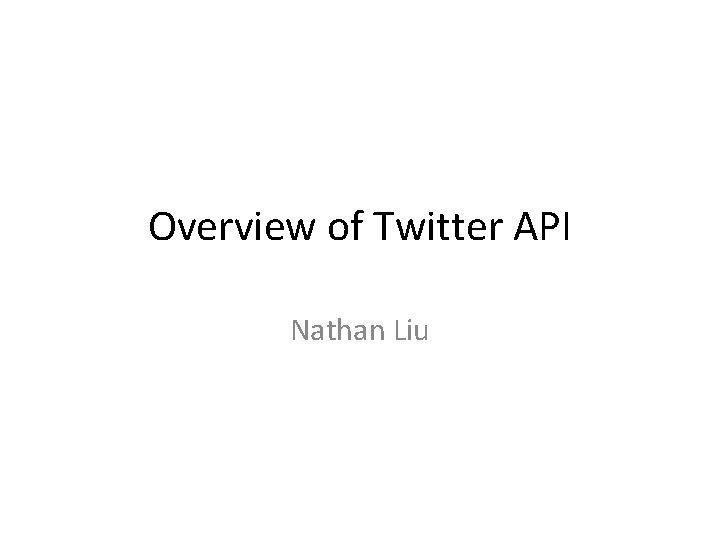
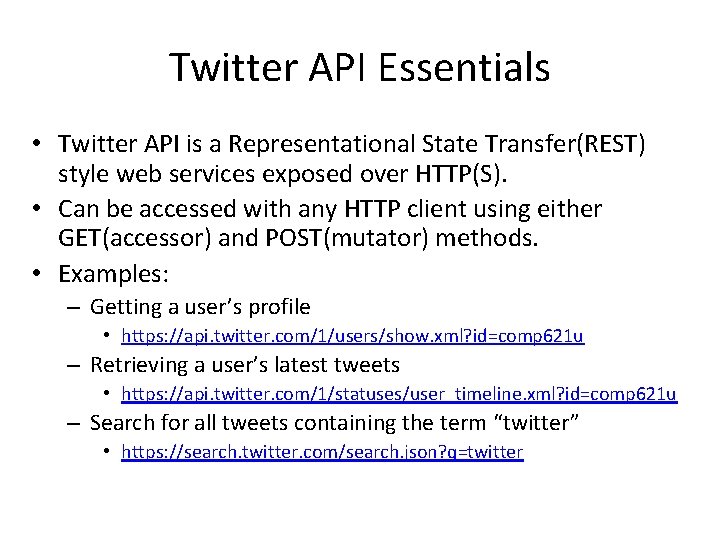
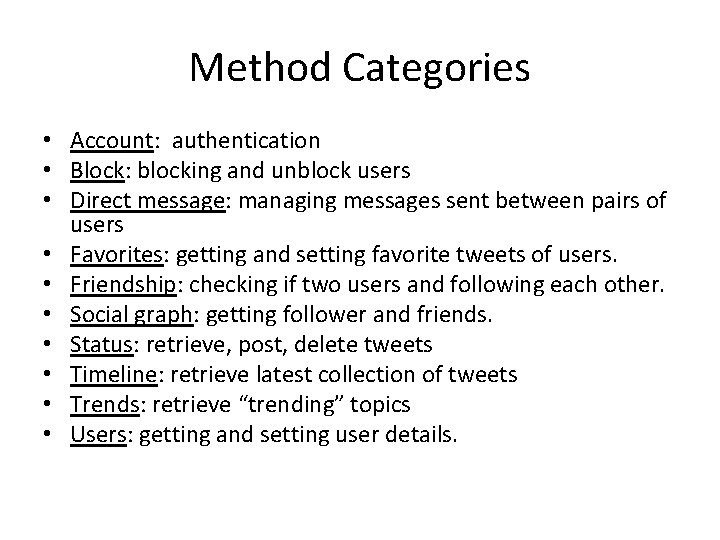
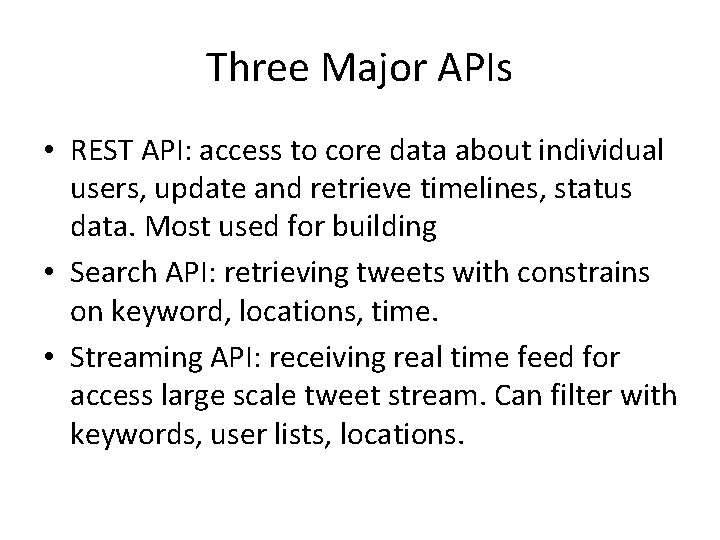
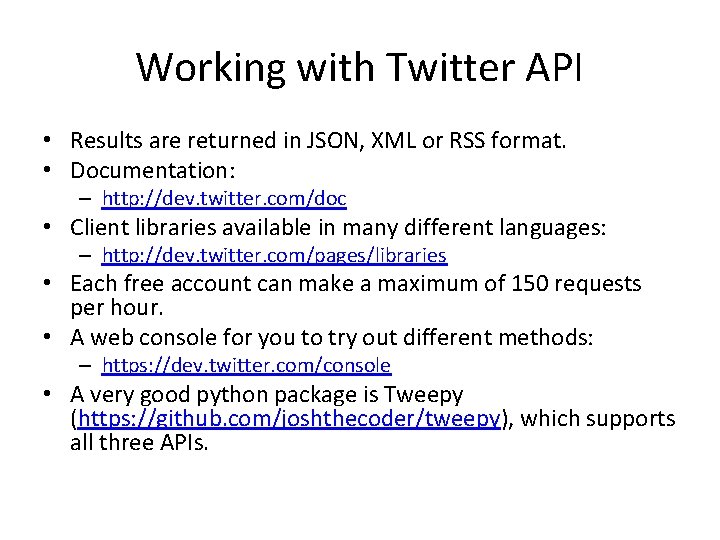
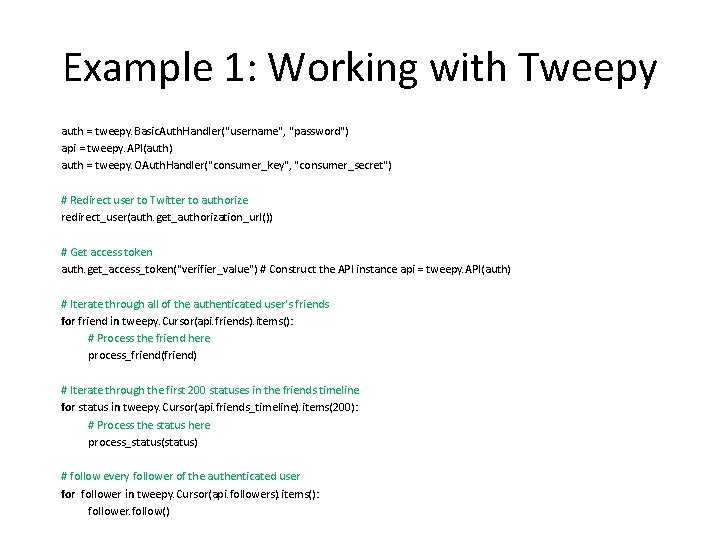
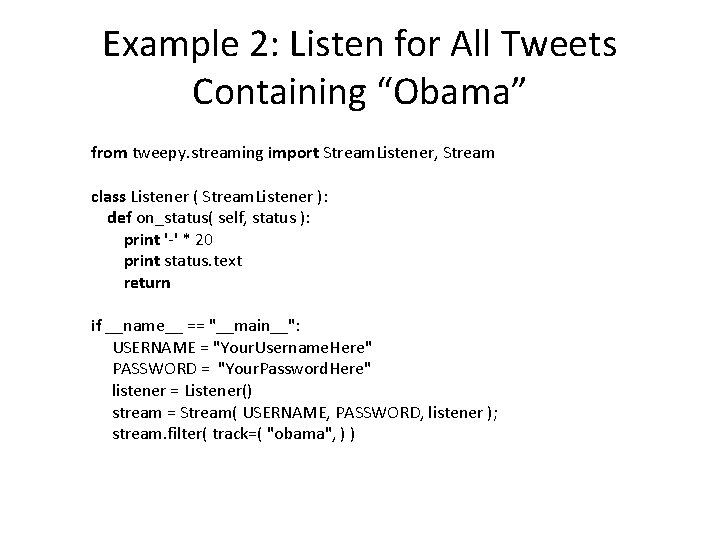
- Slides: 7
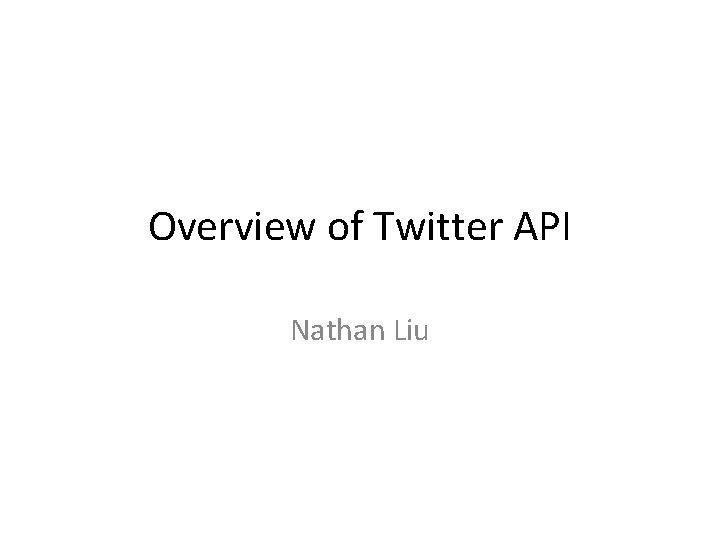
Overview of Twitter API Nathan Liu
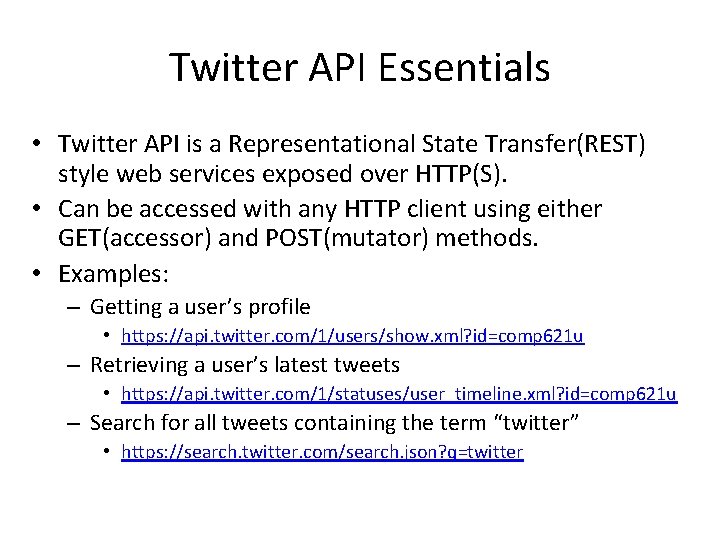
Twitter API Essentials • Twitter API is a Representational State Transfer(REST) style web services exposed over HTTP(S). • Can be accessed with any HTTP client using either GET(accessor) and POST(mutator) methods. • Examples: – Getting a user’s profile • https: //api. twitter. com/1/users/show. xml? id=comp 621 u – Retrieving a user’s latest tweets • https: //api. twitter. com/1/statuses/user_timeline. xml? id=comp 621 u – Search for all tweets containing the term “twitter” • https: //search. twitter. com/search. json? q=twitter
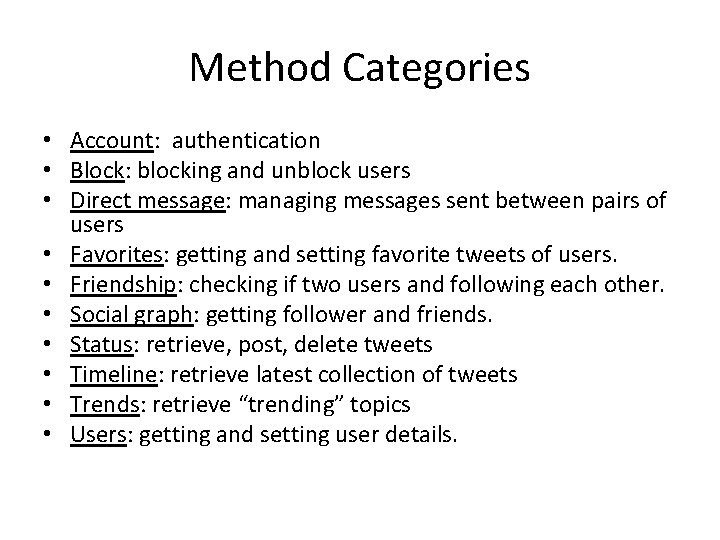
Method Categories • Account: authentication • Block: blocking and unblock users • Direct message: managing messages sent between pairs of users • Favorites: getting and setting favorite tweets of users. • Friendship: checking if two users and following each other. • Social graph: getting follower and friends. • Status: retrieve, post, delete tweets • Timeline: retrieve latest collection of tweets • Trends: retrieve “trending” topics • Users: getting and setting user details.
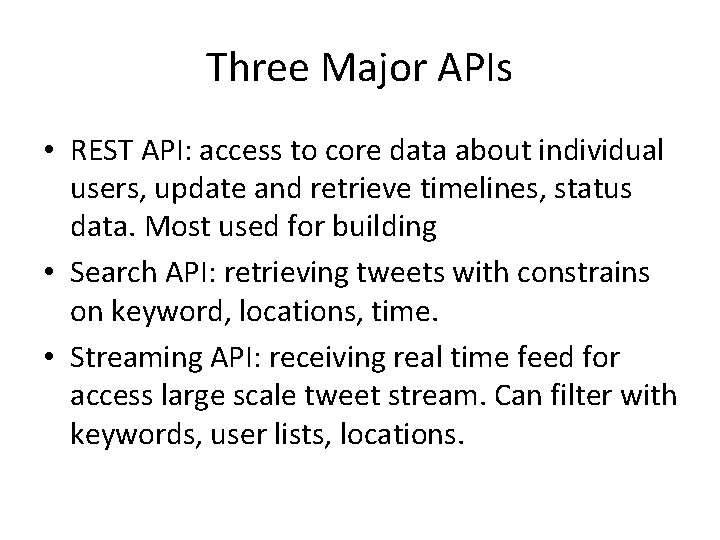
Three Major APIs • REST API: access to core data about individual users, update and retrieve timelines, status data. Most used for building • Search API: retrieving tweets with constrains on keyword, locations, time. • Streaming API: receiving real time feed for access large scale tweet stream. Can filter with keywords, user lists, locations.
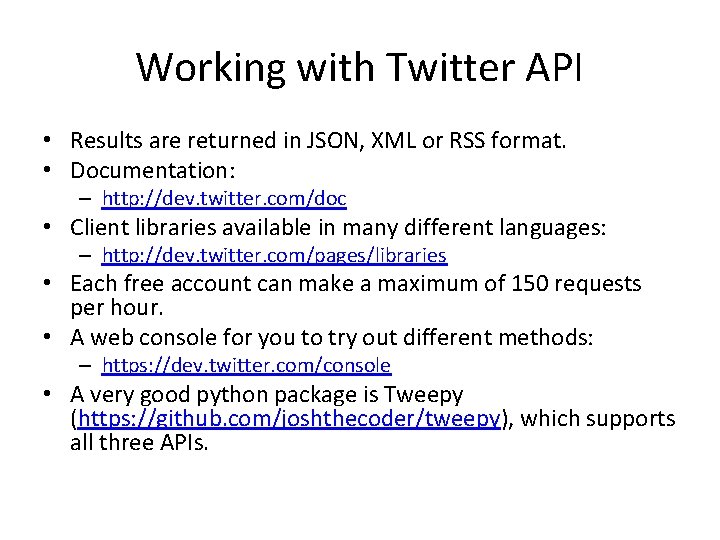
Working with Twitter API • Results are returned in JSON, XML or RSS format. • Documentation: – http: //dev. twitter. com/doc • Client libraries available in many different languages: – http: //dev. twitter. com/pages/libraries • Each free account can make a maximum of 150 requests per hour. • A web console for you to try out different methods: – https: //dev. twitter. com/console • A very good python package is Tweepy (https: //github. com/joshthecoder/tweepy), which supports all three APIs.
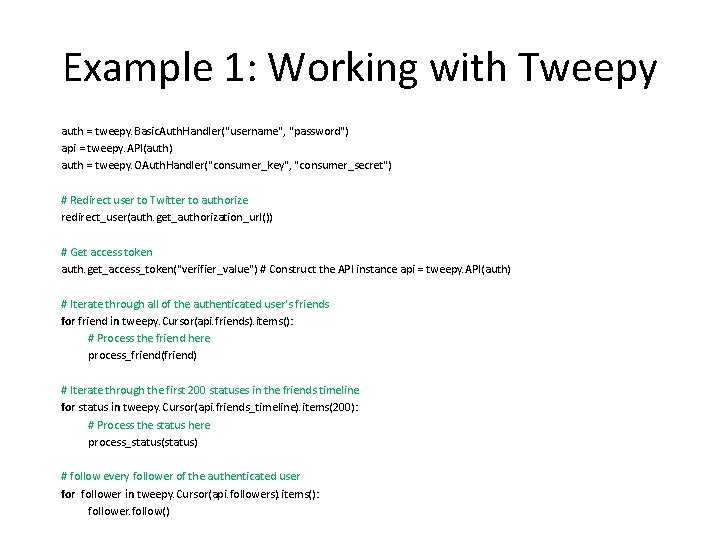
Example 1: Working with Tweepy auth = tweepy. Basic. Auth. Handler("username", "password") api = tweepy. API(auth) auth = tweepy. OAuth. Handler("consumer_key", "consumer_secret") # Redirect user to Twitter to authorize redirect_user(auth. get_authorization_url()) # Get access token auth. get_access_token("verifier_value") # Construct the API instance api = tweepy. API(auth) # Iterate through all of the authenticated user's friends for friend in tweepy. Cursor(api. friends). items(): # Process the friend here process_friend(friend) # Iterate through the first 200 statuses in the friends timeline for status in tweepy. Cursor(api. friends_timeline). items(200): # Process the status here process_status(status) # follow every follower of the authenticated user follower in tweepy. Cursor(api. followers). items(): follower. follow()
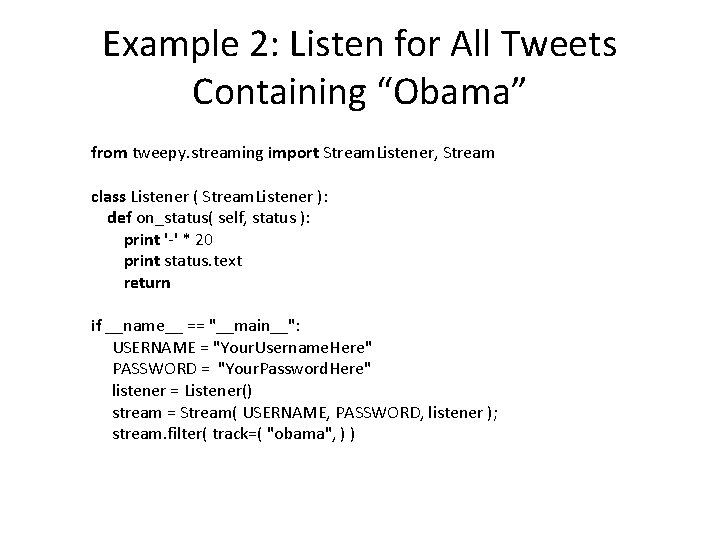
Example 2: Listen for All Tweets Containing “Obama” from tweepy. streaming import Stream. Listener, Stream class Listener ( Stream. Listener ): def on_status( self, status ): print '-' * 20 print status. text return if __name__ == "__main__": USERNAME = "Your. Username. Here" PASSWORD = "Your. Password. Here" listener = Listener() stream = Stream( USERNAME, PASSWORD, listener ); stream. filter( track=( "obama", ) )