Namespaces Calculator Casestudy Consider a simple desk calculator
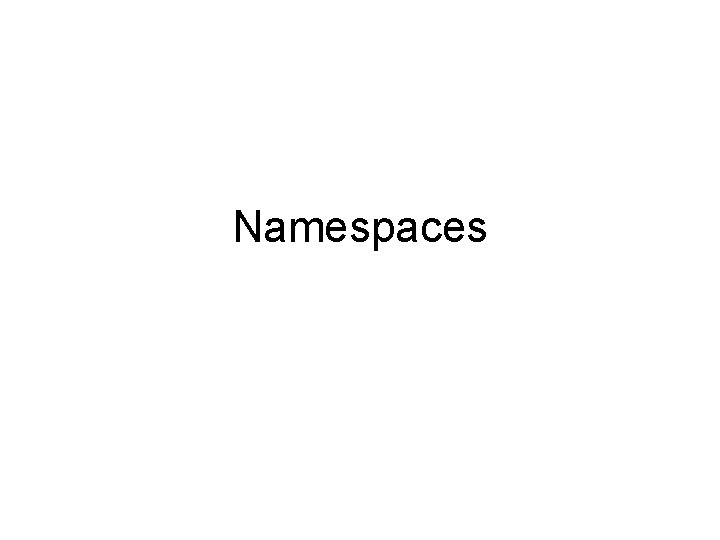
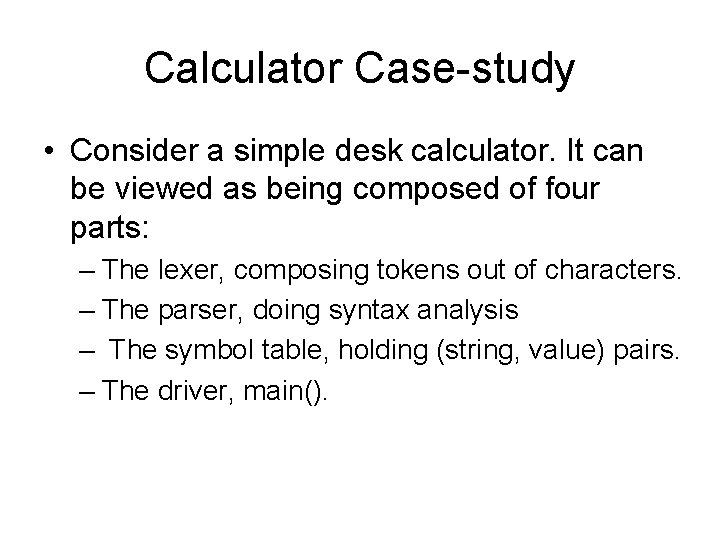
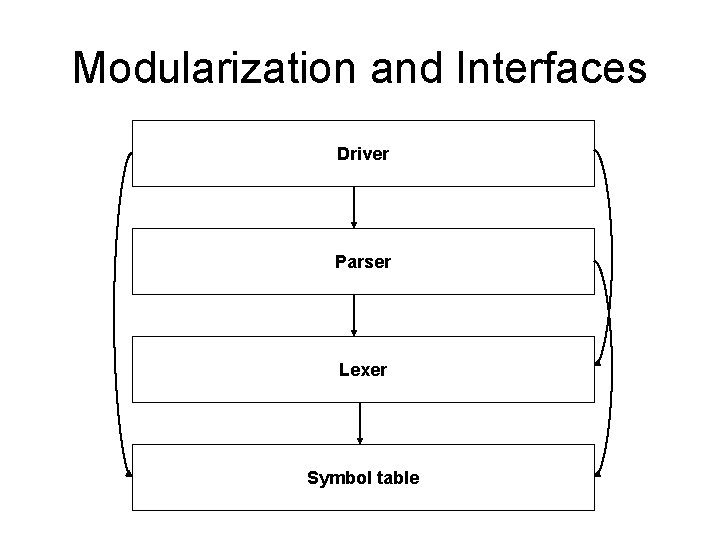
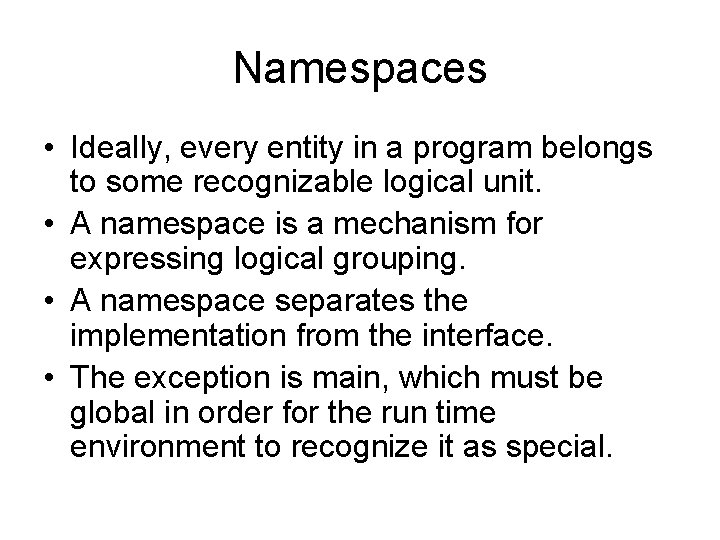
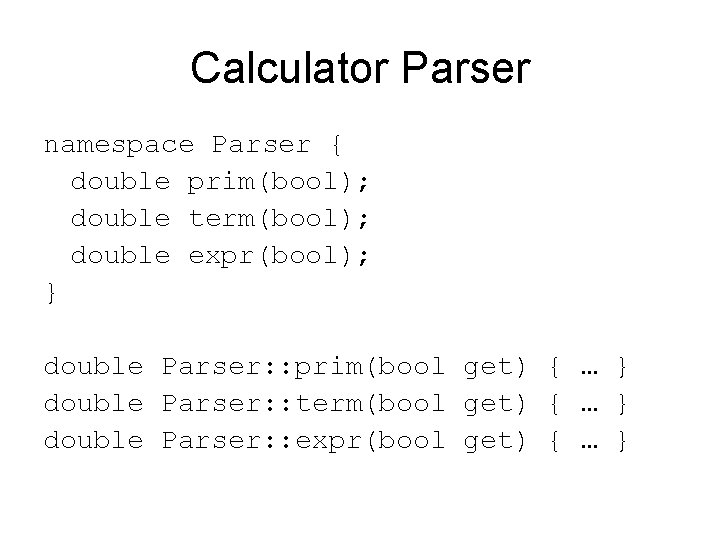
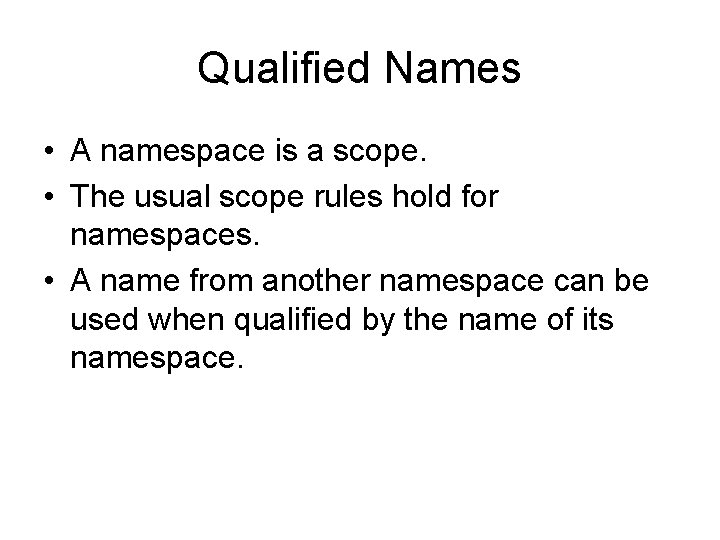
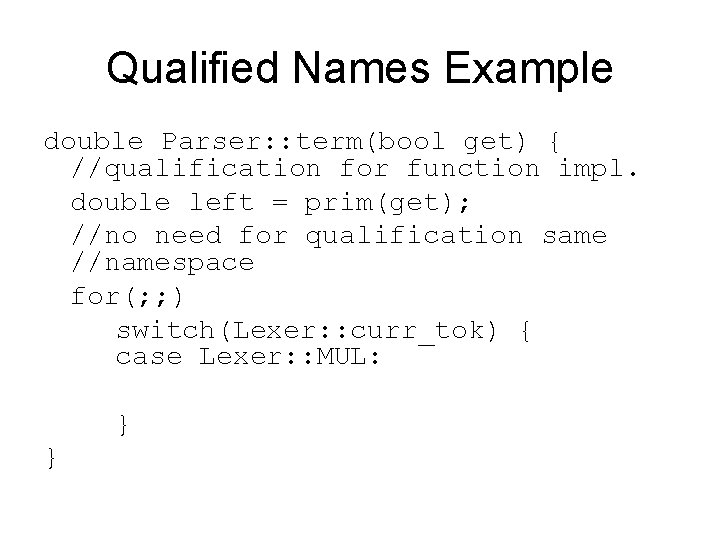
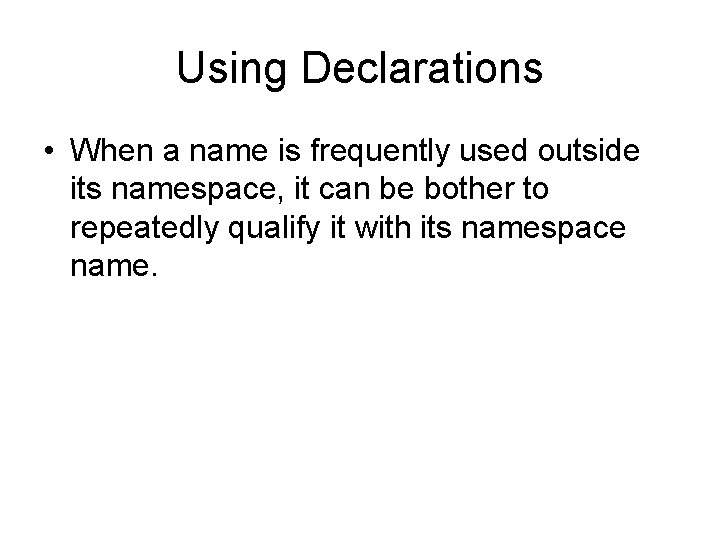
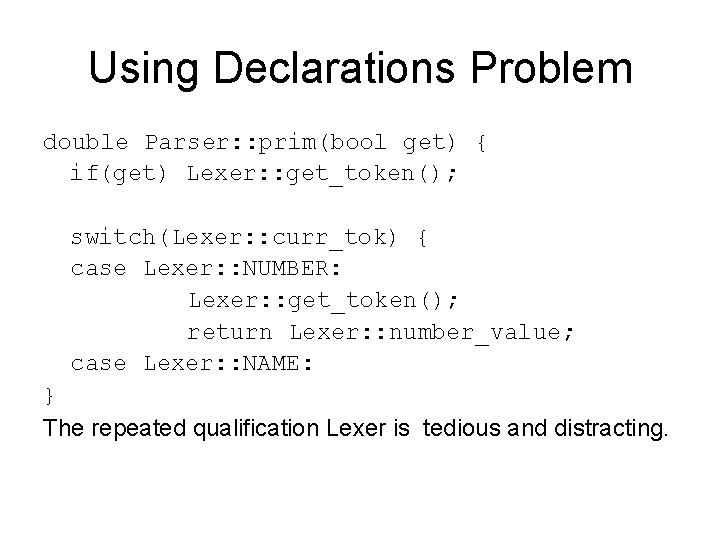
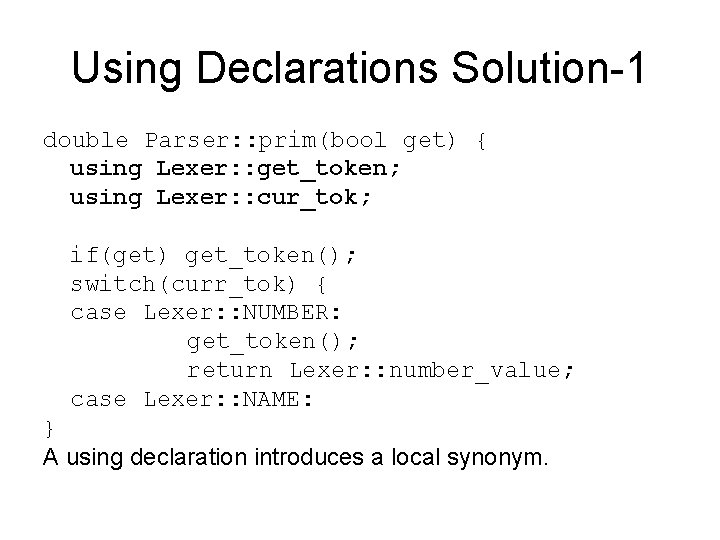
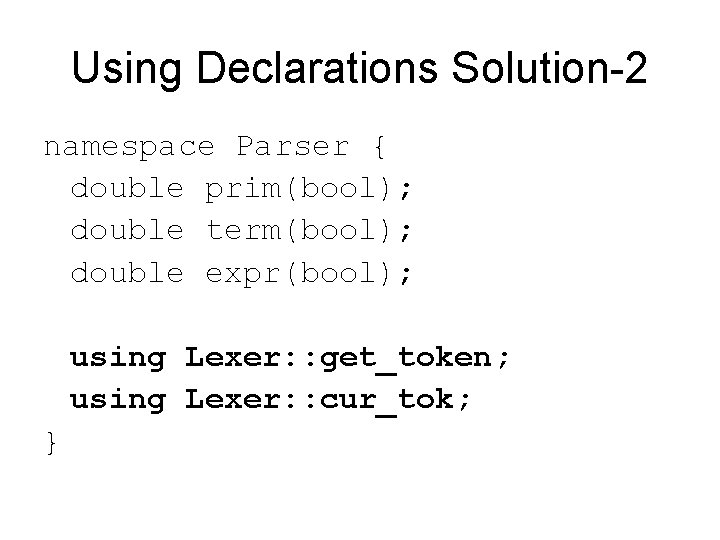
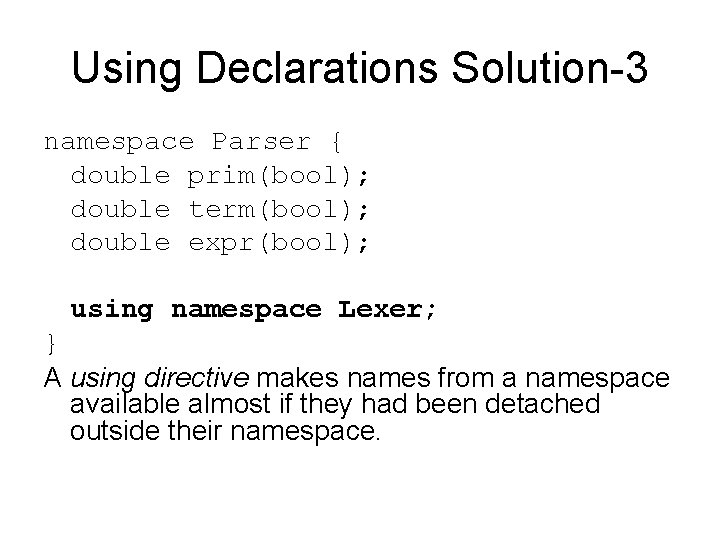
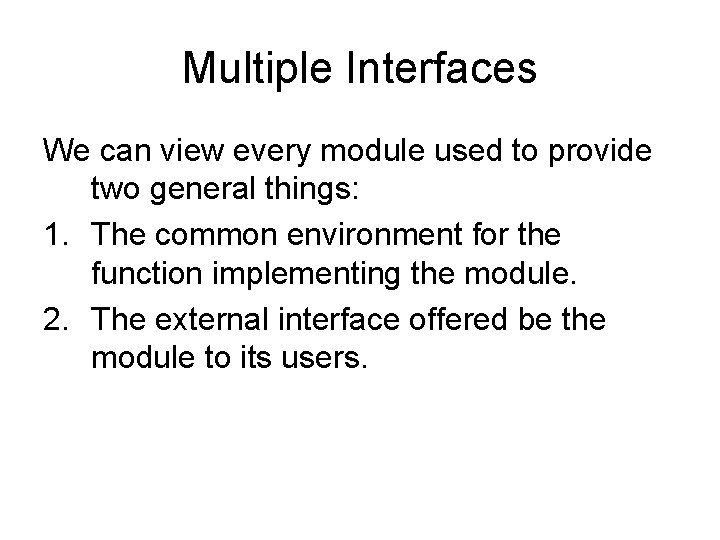
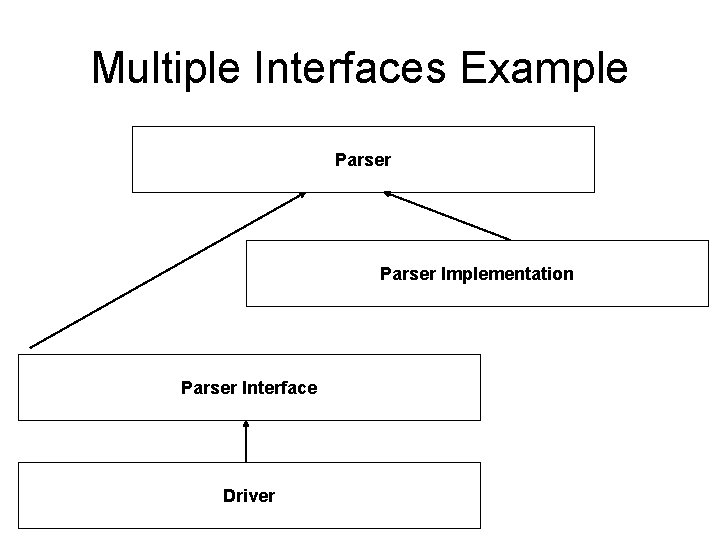
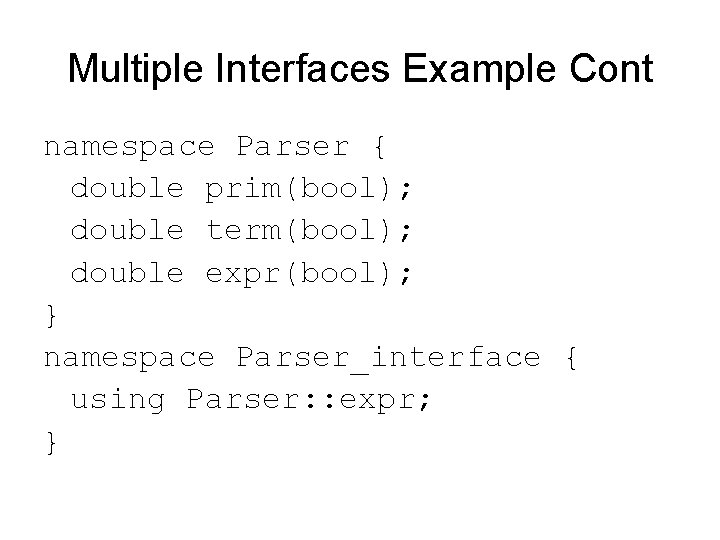
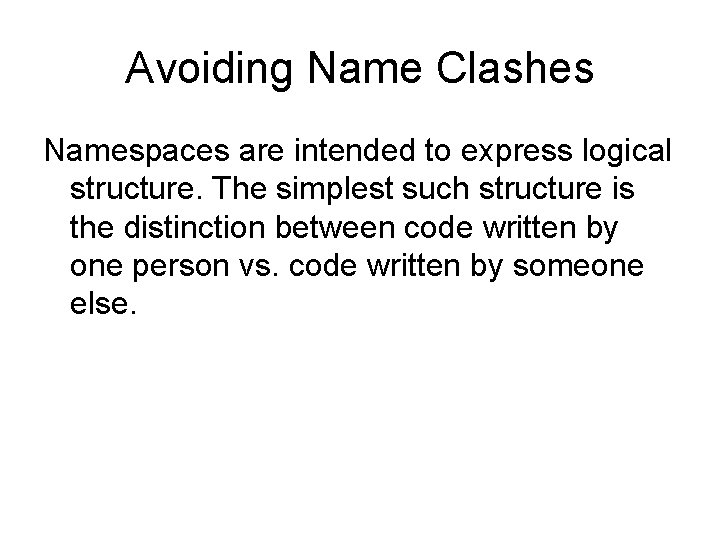
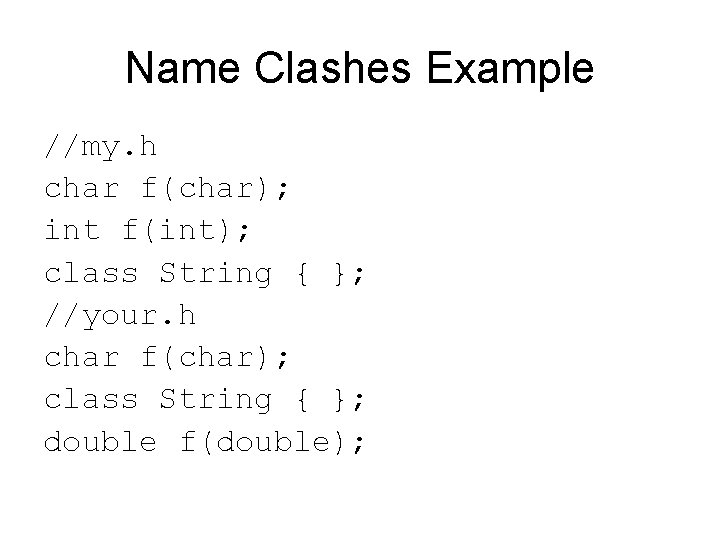
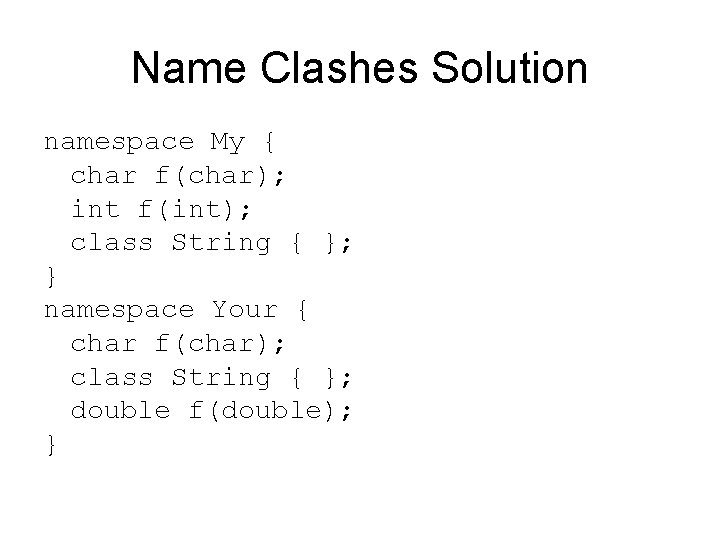
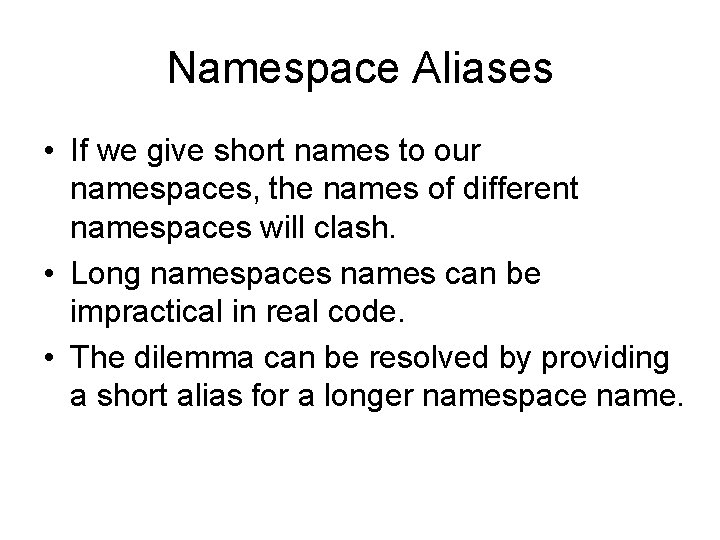
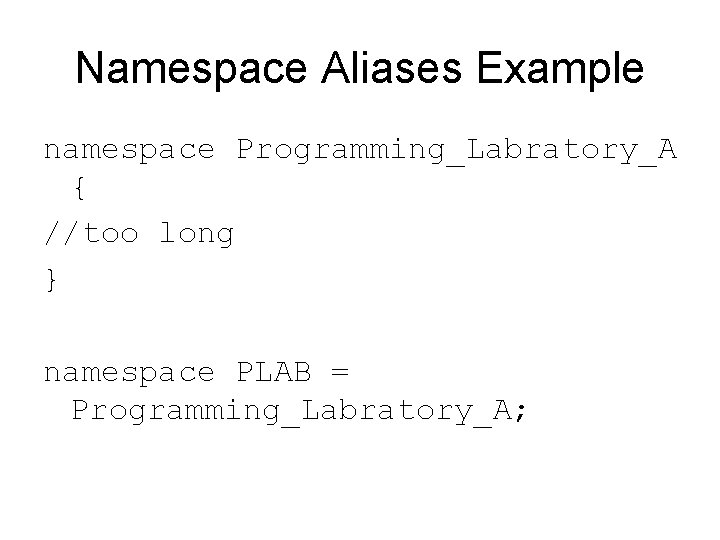
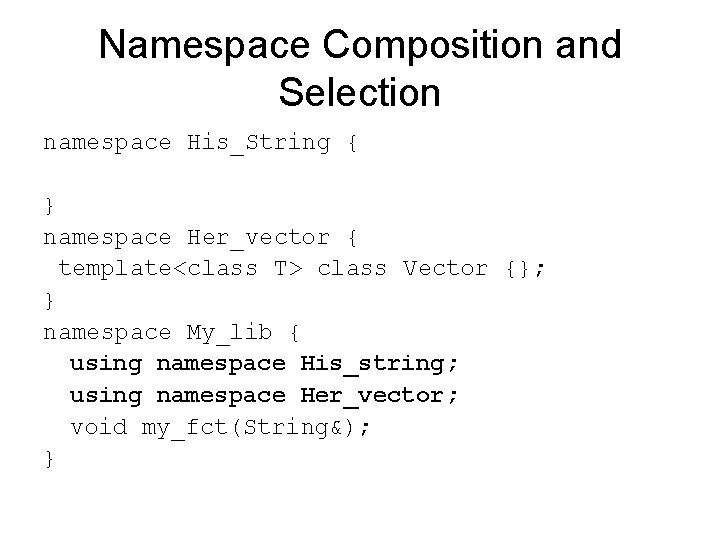
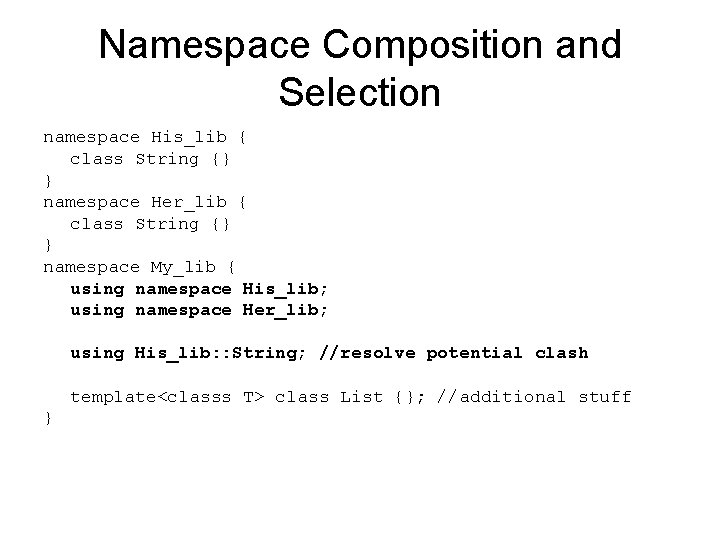
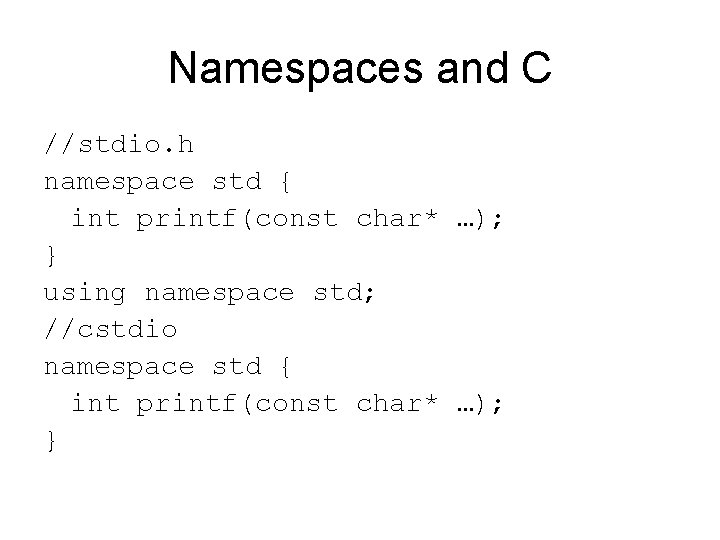
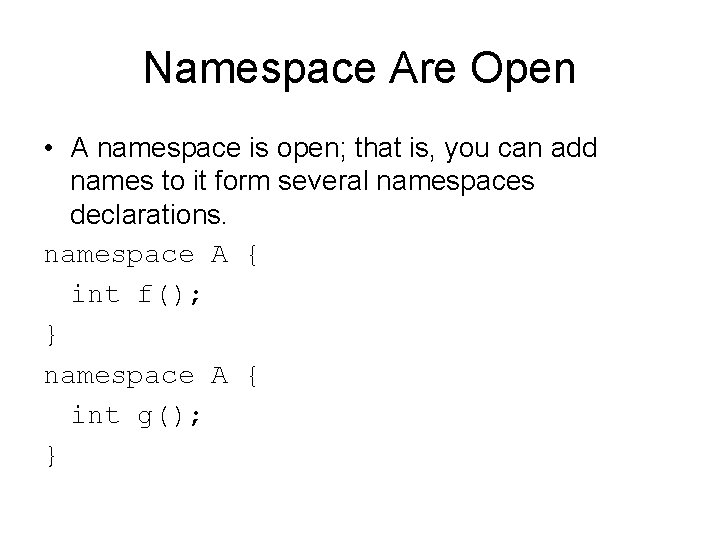
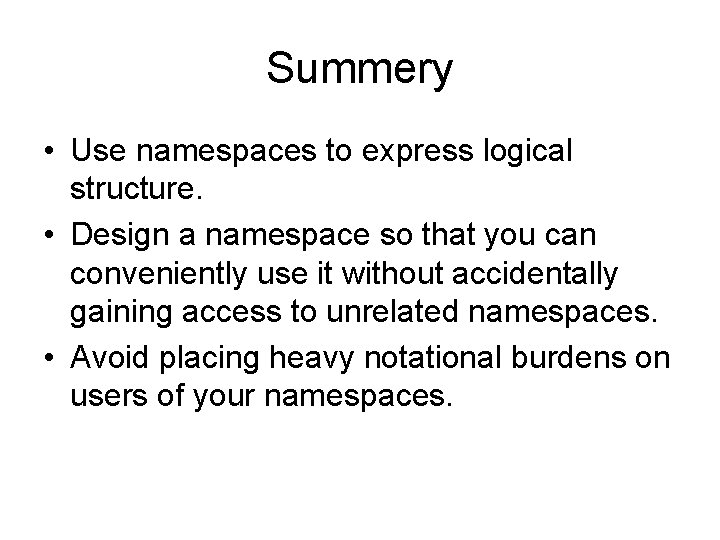
- Slides: 25
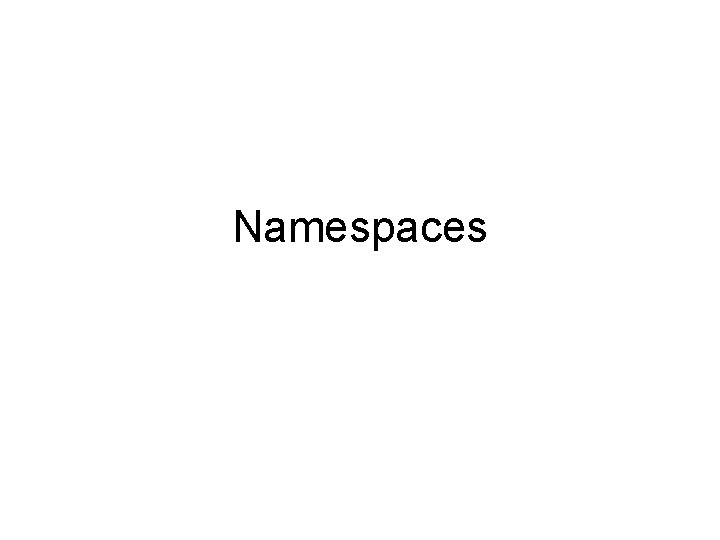
Namespaces
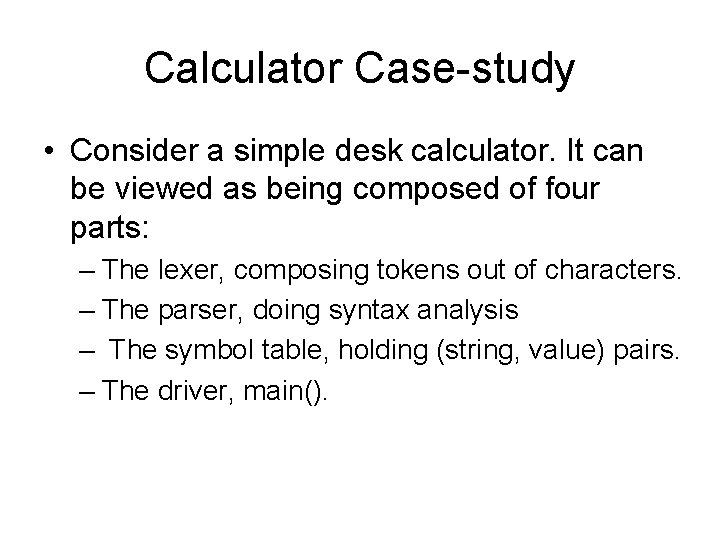
Calculator Case-study • Consider a simple desk calculator. It can be viewed as being composed of four parts: – The lexer, composing tokens out of characters. – The parser, doing syntax analysis – The symbol table, holding (string, value) pairs. – The driver, main().
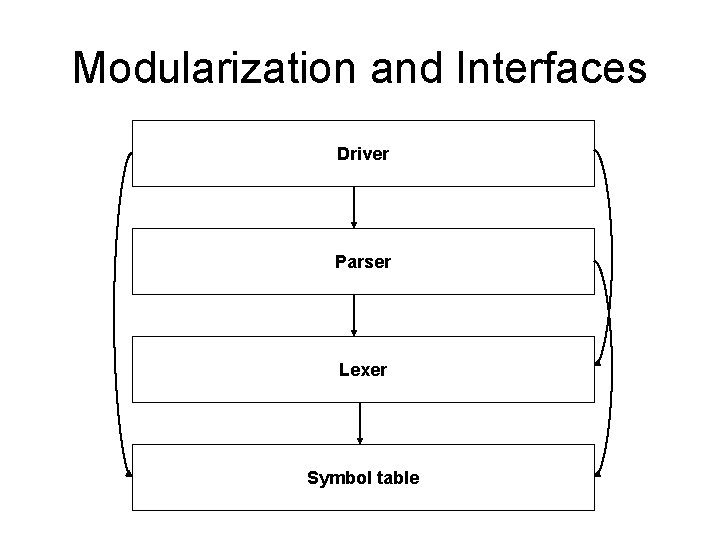
Modularization and Interfaces Driver Parser Lexer Symbol table
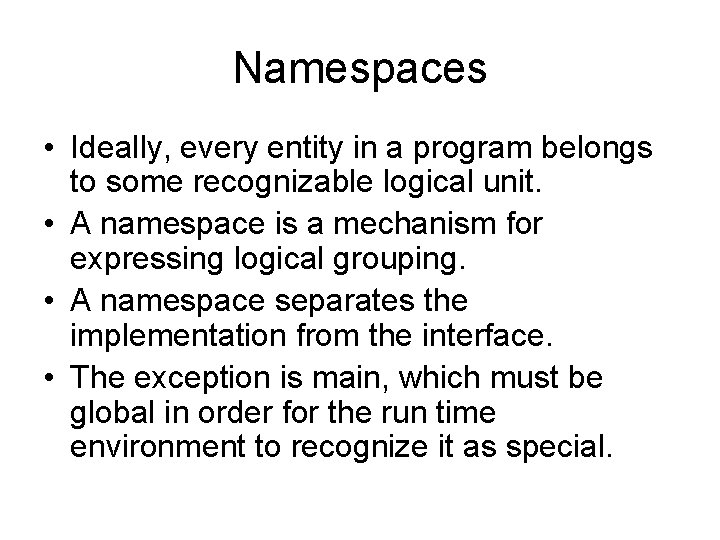
Namespaces • Ideally, every entity in a program belongs to some recognizable logical unit. • A namespace is a mechanism for expressing logical grouping. • A namespace separates the implementation from the interface. • The exception is main, which must be global in order for the run time environment to recognize it as special.
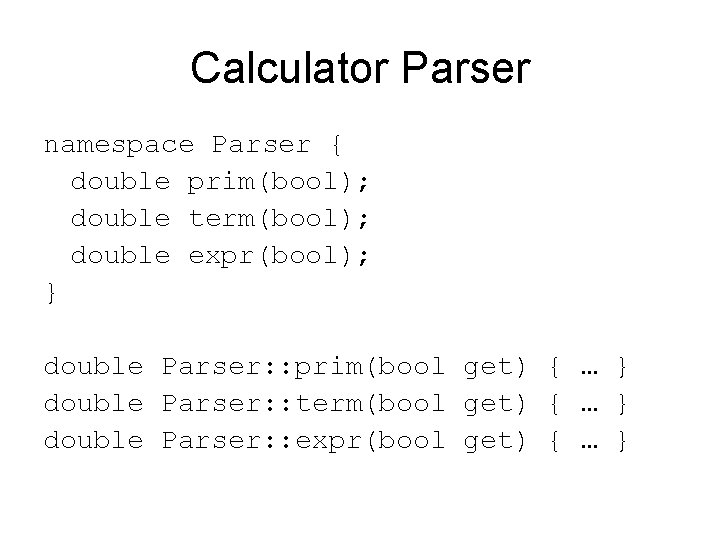
Calculator Parser namespace Parser { double prim(bool); double term(bool); double expr(bool); } double Parser: : prim(bool get) { … } double Parser: : term(bool get) { … } double Parser: : expr(bool get) { … }
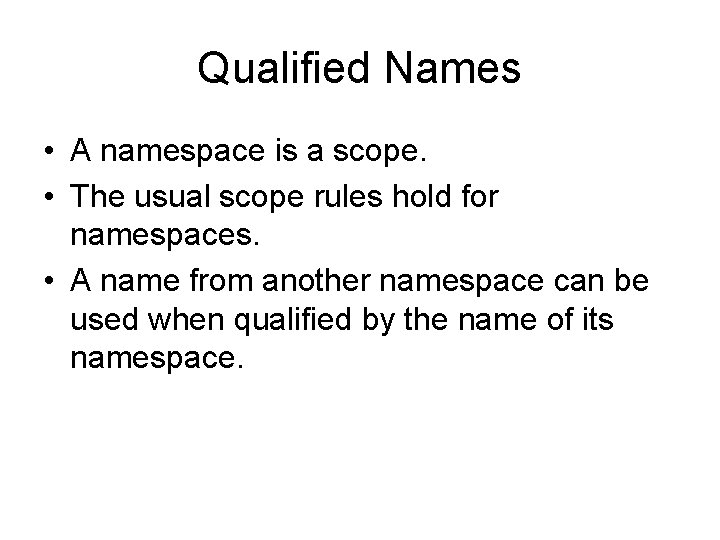
Qualified Names • A namespace is a scope. • The usual scope rules hold for namespaces. • A name from another namespace can be used when qualified by the name of its namespace.
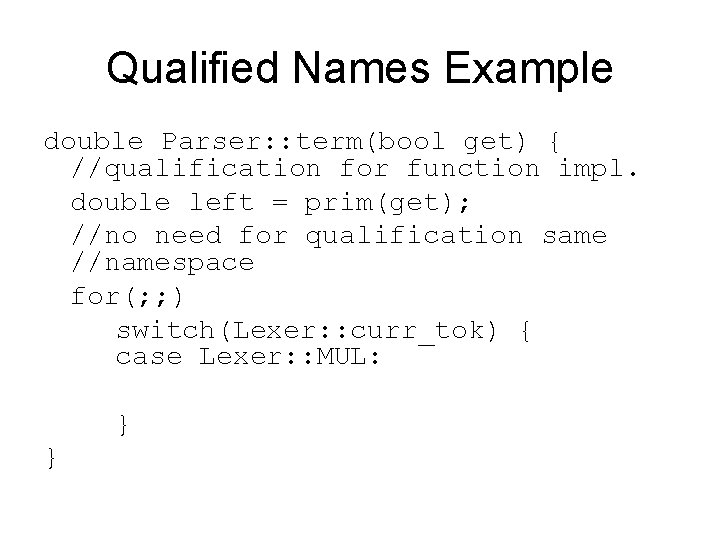
Qualified Names Example double Parser: : term(bool get) { //qualification for function impl. double left = prim(get); //no need for qualification same //namespace for(; ; ) switch(Lexer: : curr_tok) { case Lexer: : MUL: } }
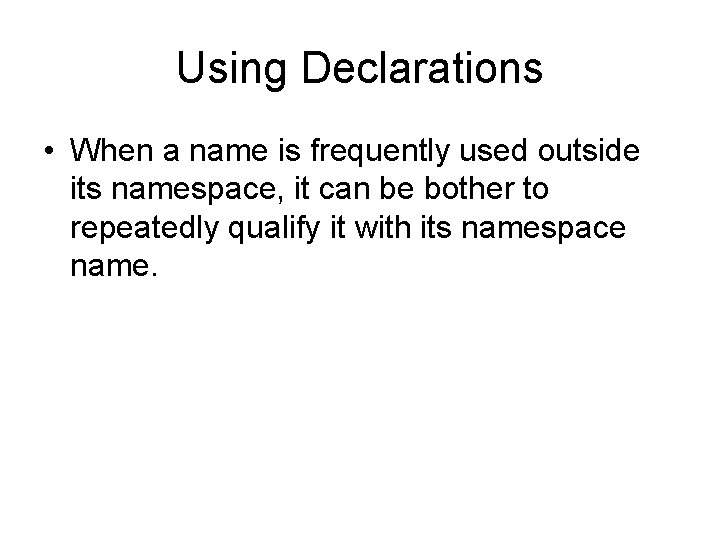
Using Declarations • When a name is frequently used outside its namespace, it can be bother to repeatedly qualify it with its namespace name.
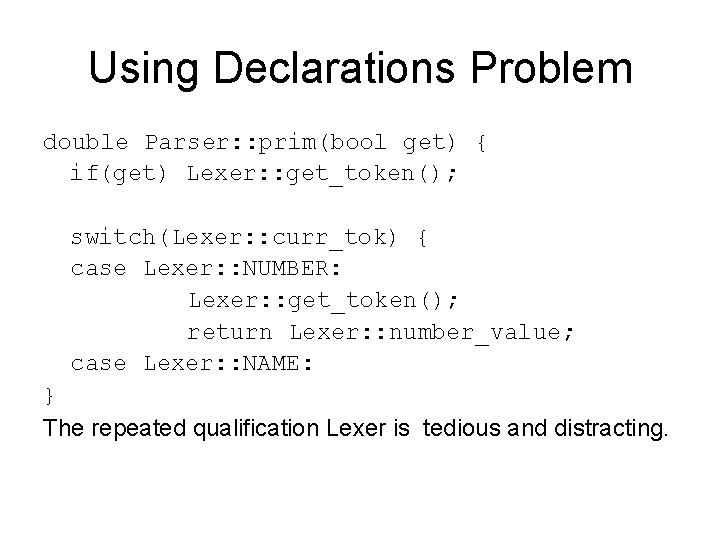
Using Declarations Problem double Parser: : prim(bool get) { if(get) Lexer: : get_token(); switch(Lexer: : curr_tok) { case Lexer: : NUMBER: Lexer: : get_token(); return Lexer: : number_value; case Lexer: : NAME: } The repeated qualification Lexer is tedious and distracting.
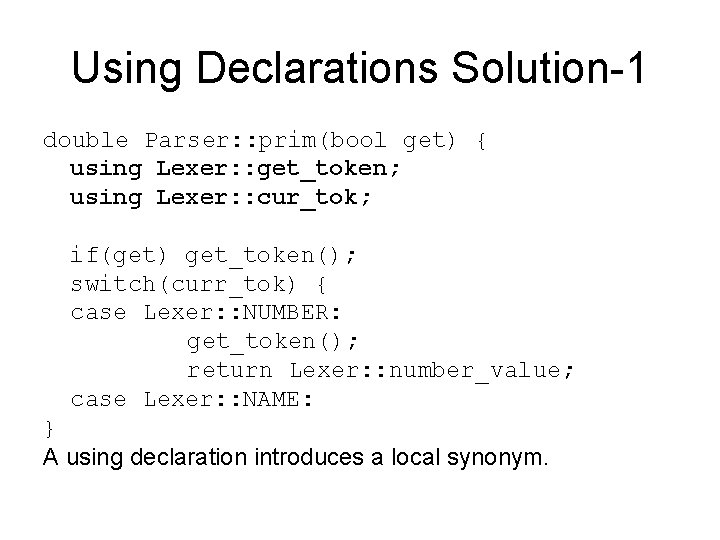
Using Declarations Solution-1 double Parser: : prim(bool get) { using Lexer: : get_token; using Lexer: : cur_tok; if(get) get_token(); switch(curr_tok) { case Lexer: : NUMBER: get_token(); return Lexer: : number_value; case Lexer: : NAME: } A using declaration introduces a local synonym.
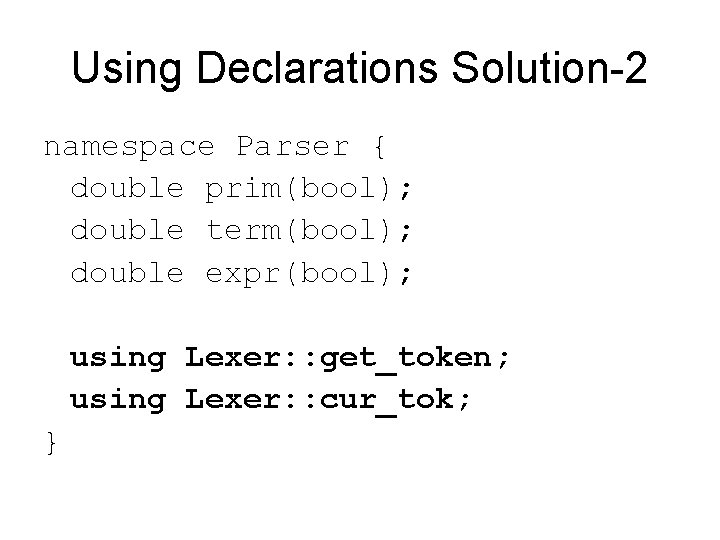
Using Declarations Solution-2 namespace Parser { double prim(bool); double term(bool); double expr(bool); using Lexer: : get_token; using Lexer: : cur_tok; }
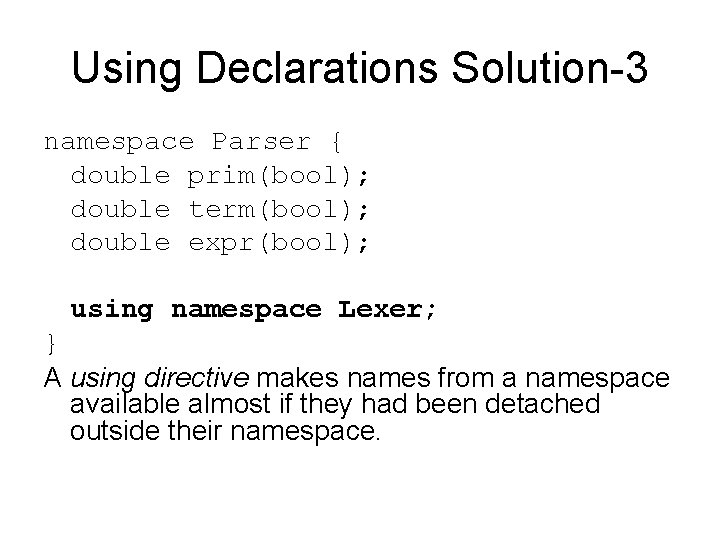
Using Declarations Solution-3 namespace Parser { double prim(bool); double term(bool); double expr(bool); using namespace Lexer; } A using directive makes names from a namespace available almost if they had been detached outside their namespace.
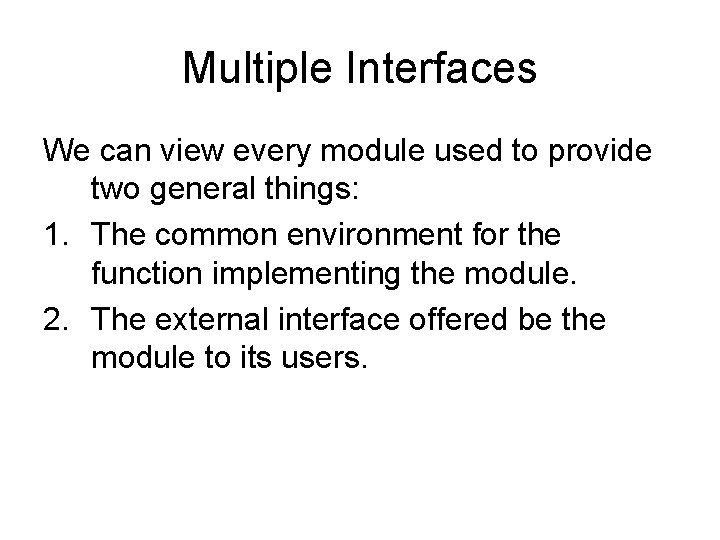
Multiple Interfaces We can view every module used to provide two general things: 1. The common environment for the function implementing the module. 2. The external interface offered be the module to its users.
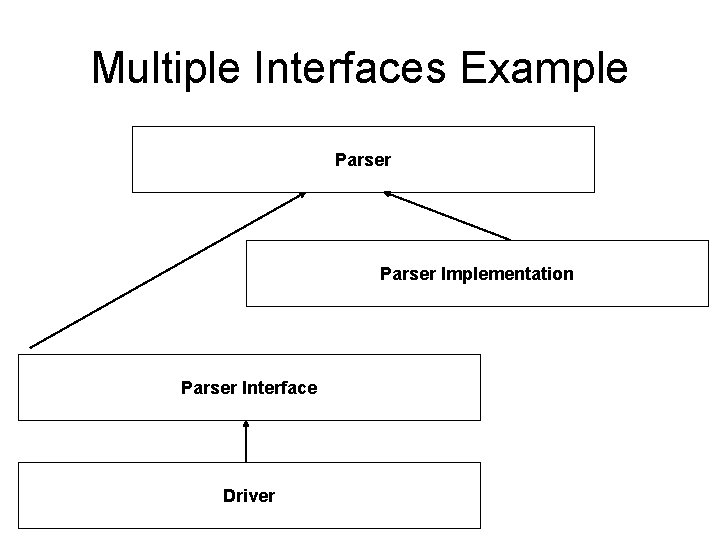
Multiple Interfaces Example Parser Implementation Parser Interface Driver
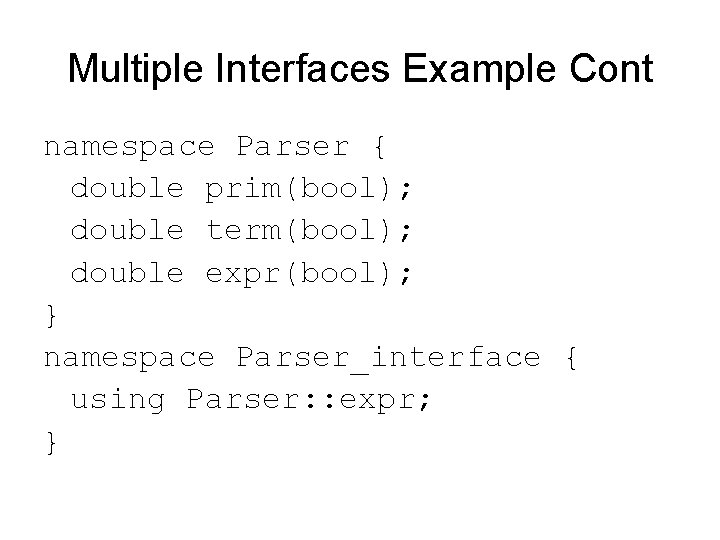
Multiple Interfaces Example Cont namespace Parser { double prim(bool); double term(bool); double expr(bool); } namespace Parser_interface { using Parser: : expr; }
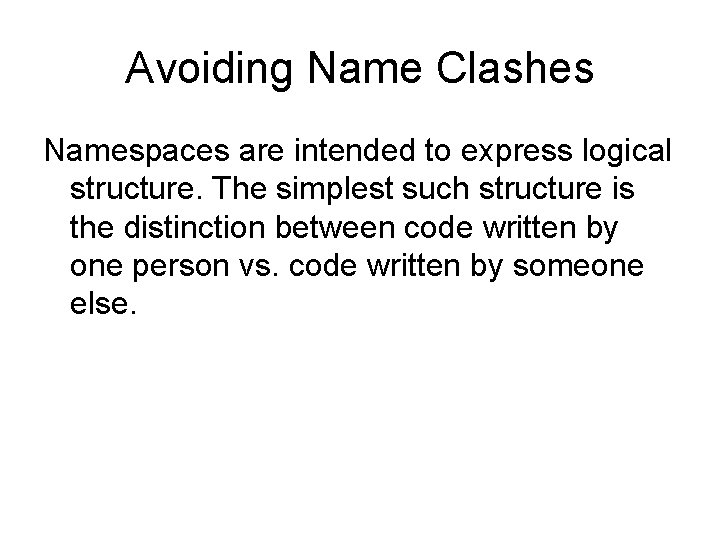
Avoiding Name Clashes Namespaces are intended to express logical structure. The simplest such structure is the distinction between code written by one person vs. code written by someone else.
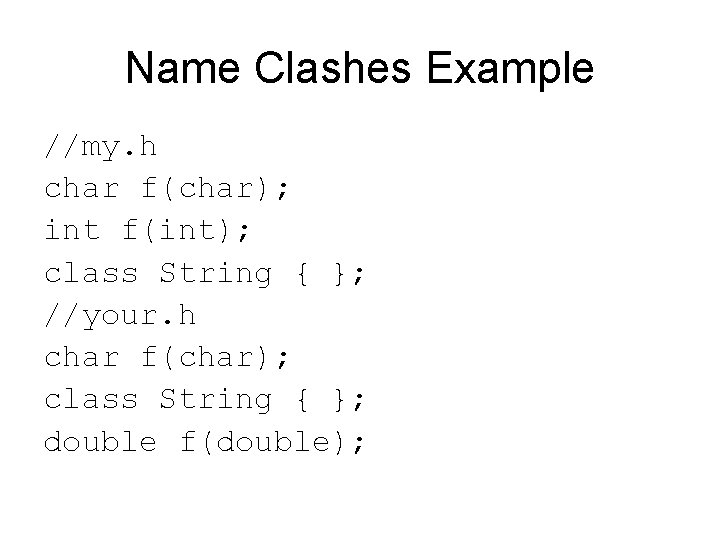
Name Clashes Example //my. h char f(char); int f(int); class String { }; //your. h char f(char); class String { }; double f(double);
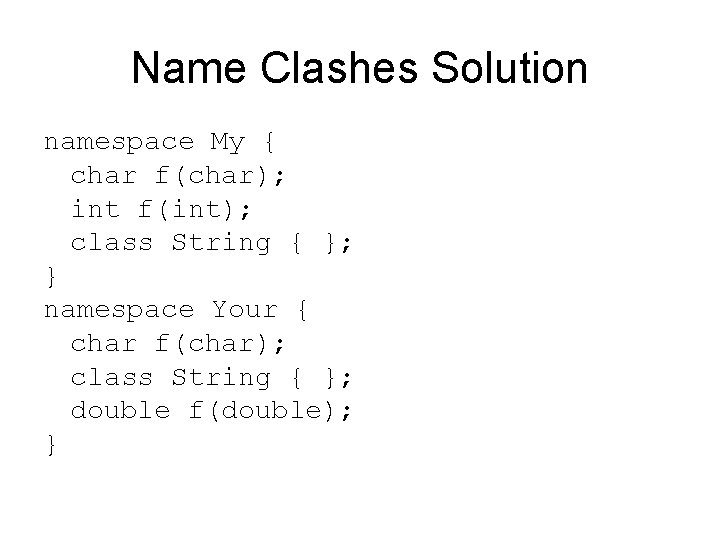
Name Clashes Solution namespace My { char f(char); int f(int); class String { }; } namespace Your { char f(char); class String { }; double f(double); }
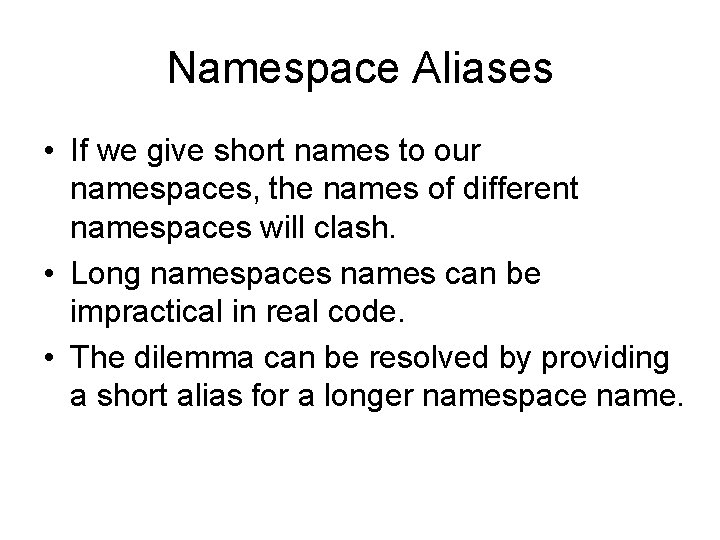
Namespace Aliases • If we give short names to our namespaces, the names of different namespaces will clash. • Long namespaces names can be impractical in real code. • The dilemma can be resolved by providing a short alias for a longer namespace name.
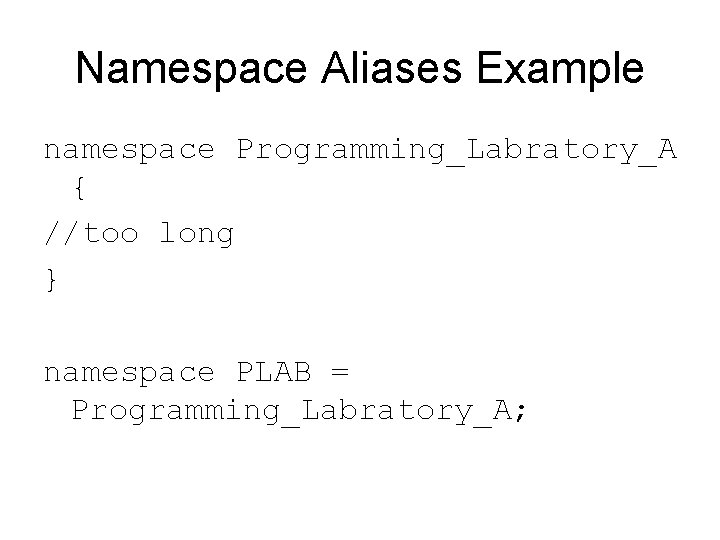
Namespace Aliases Example namespace Programming_Labratory_A { //too long } namespace PLAB = Programming_Labratory_A;
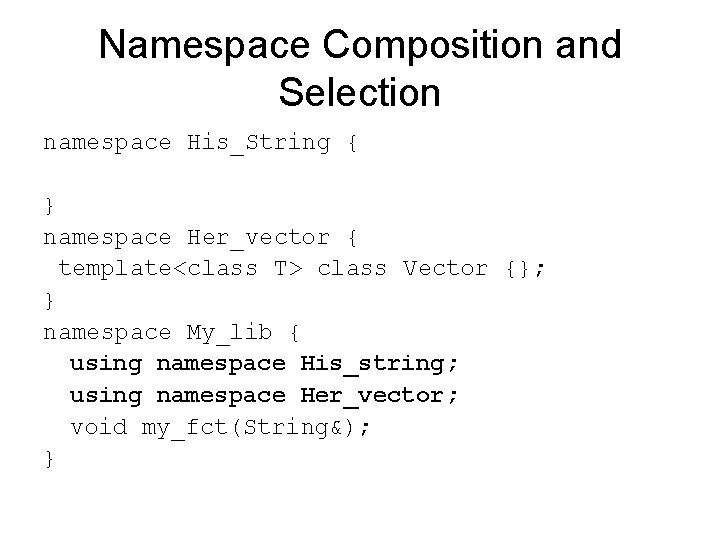
Namespace Composition and Selection namespace His_String { } namespace Her_vector { template<class T> class Vector {}; } namespace My_lib { using namespace His_string; using namespace Her_vector; void my_fct(String&); }
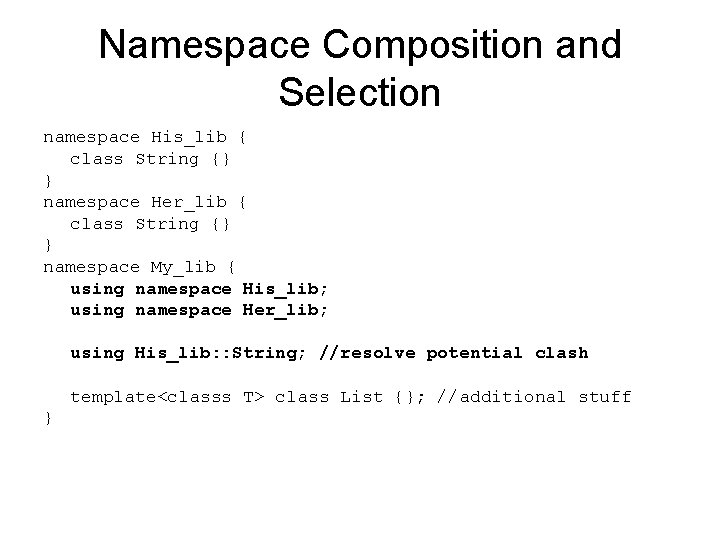
Namespace Composition and Selection namespace His_lib { class String {} } namespace Her_lib { class String {} } namespace My_lib { using namespace His_lib; using namespace Her_lib; using His_lib: : String; //resolve potential clash template<classs T> class List {}; //additional stuff }
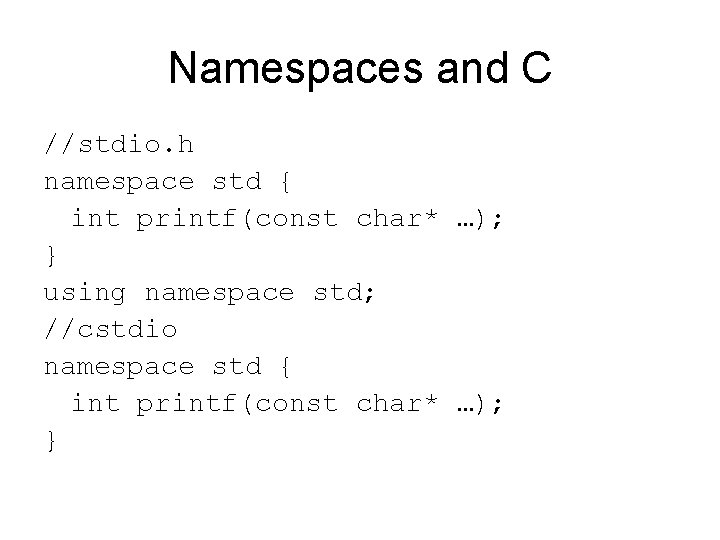
Namespaces and C //stdio. h namespace std { int printf(const char* …); } using namespace std; //cstdio namespace std { int printf(const char* …); }
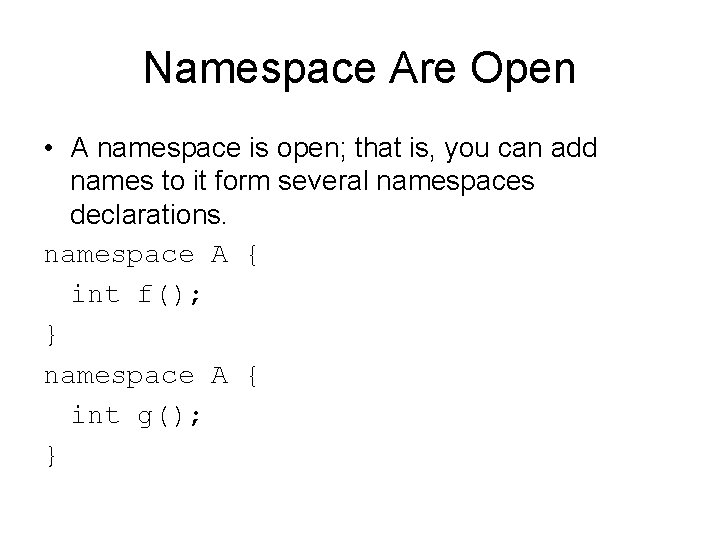
Namespace Are Open • A namespace is open; that is, you can add names to it form several namespaces declarations. namespace A { int f(); } namespace A { int g(); }
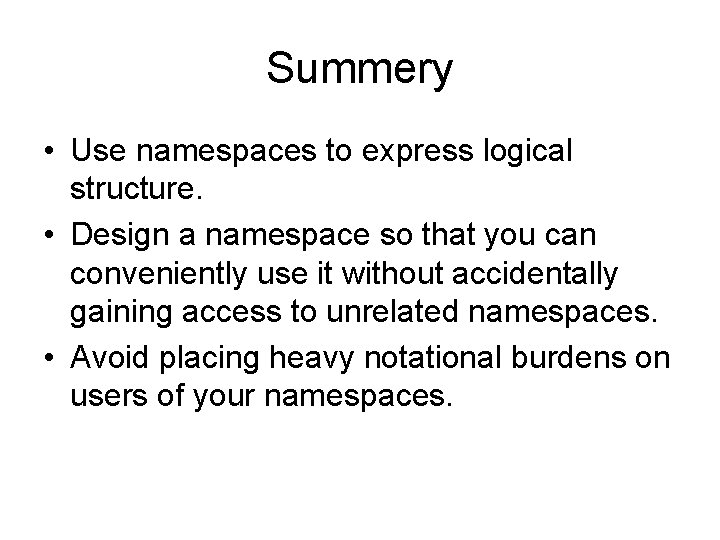
Summery • Use namespaces to express logical structure. • Design a namespace so that you can conveniently use it without accidentally gaining access to unrelated namespaces. • Avoid placing heavy notational burdens on users of your namespaces.
Consider simple past
Simple present simple past simple future exercises
Present simple past simple future simple
Present simple present continuous past simple future simple
Present simple present continuous past simple
Simple past simple present simple future
Present simple past simple future simple exercises
Past tense present tense future tense
Simple present tense review
Future simple present simple
Www.bmi-calculator.net/bmr-calculator
I consider your behavior rude irresponsible and offensive
Satan loves to take what's beautiful
Consider a person standing in a breezy room at 20
Consider all relevant criteria
Verb infinitive
Labour saving devices in food and nutrition
Consider transferring an enormous file of l
Consider christ lyrics
Caf consider all factors
Disadvantages of rsa retail savings bonds
What did shakespeare consider his main profession to be?
Prefix of consider
Wjec hospitality and catering unit 2 examples
Consider a u-tube whose arms are open
Consider the balance of a commercial bank