Multidimensional Arrays and other Array Oddities Rudra Dutta
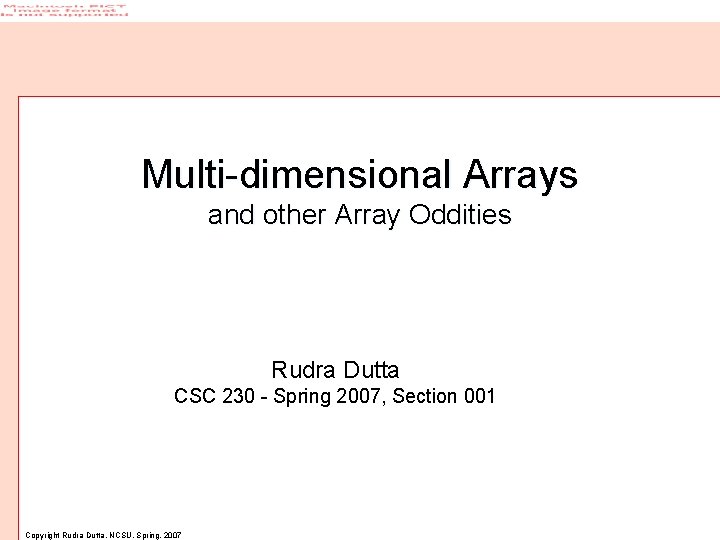
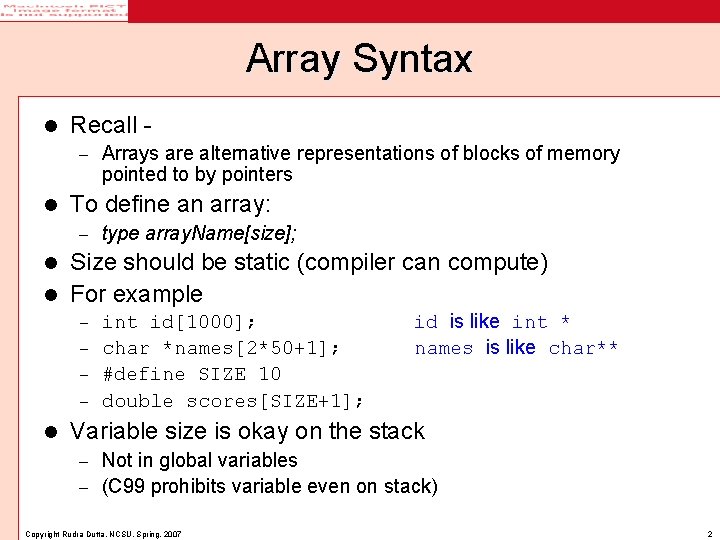
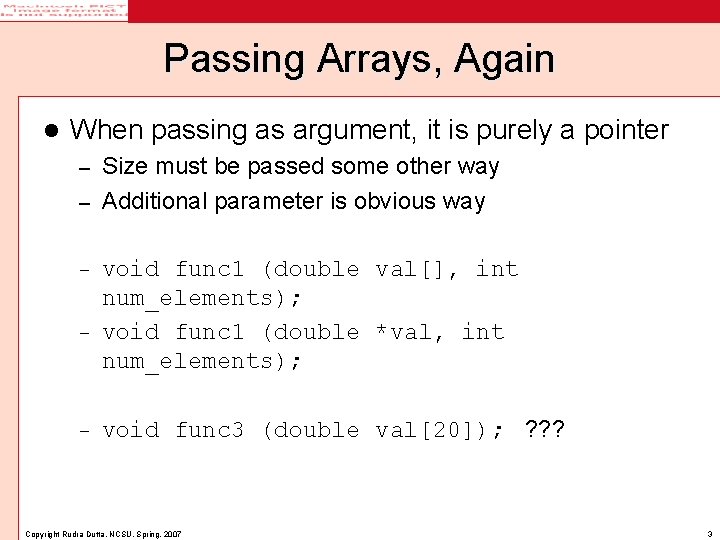
![Arrays and sizeof int id [1000]; int *pointer. Id; l l l sizeof(id) is Arrays and sizeof int id [1000]; int *pointer. Id; l l l sizeof(id) is](https://slidetodoc.com/presentation_image_h2/cc2cdc4f556b9256b0f19cd9759accc5/image-4.jpg)
![Passing Arrays id[1] = 20; pid[20] = 1; vid[0] = 6; tid[6] = 21; Passing Arrays id[1] = 20; pid[20] = 1; vid[0] = 6; tid[6] = 21;](https://slidetodoc.com/presentation_image_h2/cc2cdc4f556b9256b0f19cd9759accc5/image-5.jpg)
![2 -dimensional Arrays int values[2][3]; l Recall that values[] looks just like a pointer 2 -dimensional Arrays int values[2][3]; l Recall that values[] looks just like a pointer](https://slidetodoc.com/presentation_image_h2/cc2cdc4f556b9256b0f19cd9759accc5/image-6.jpg)
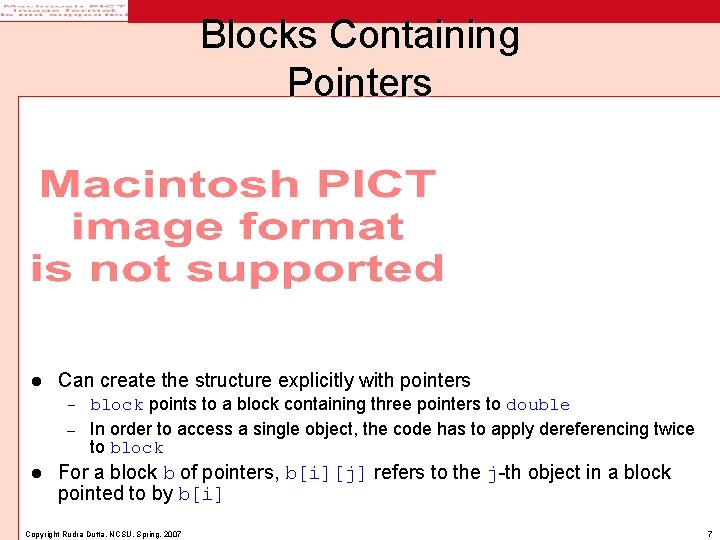
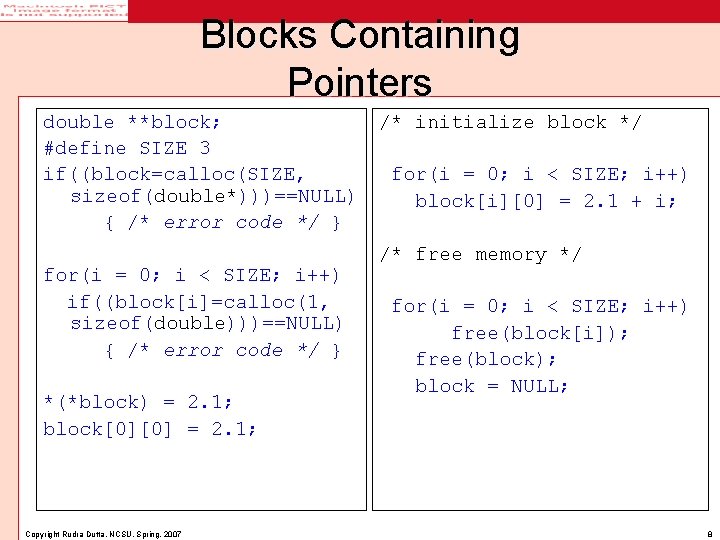
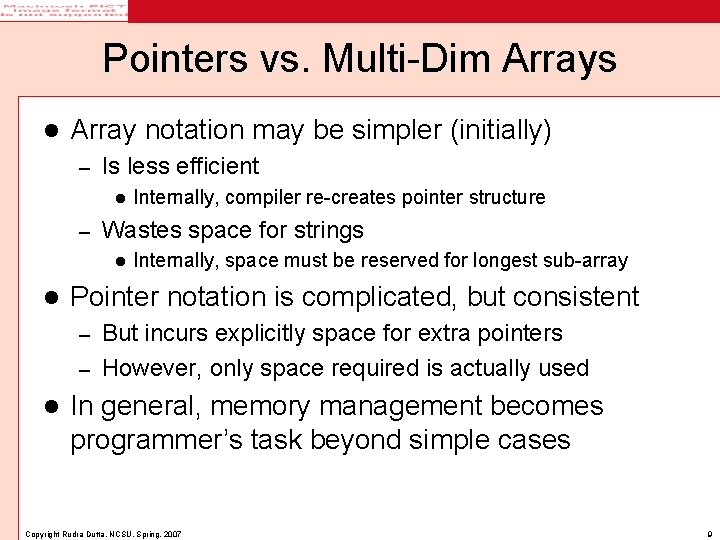
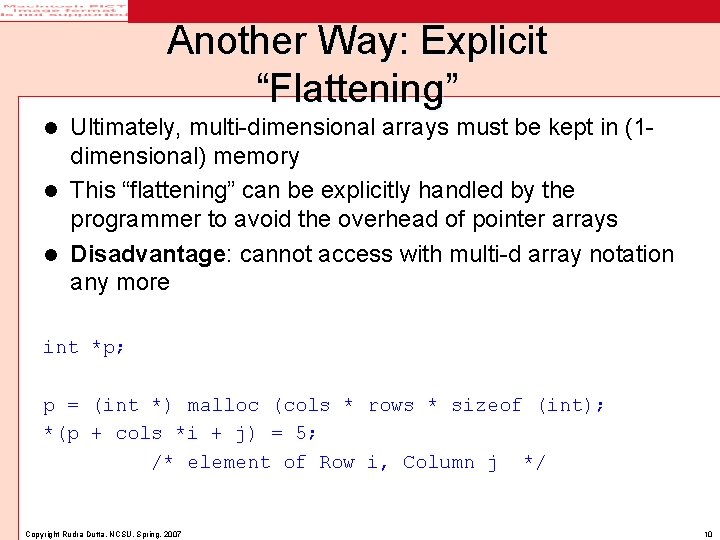
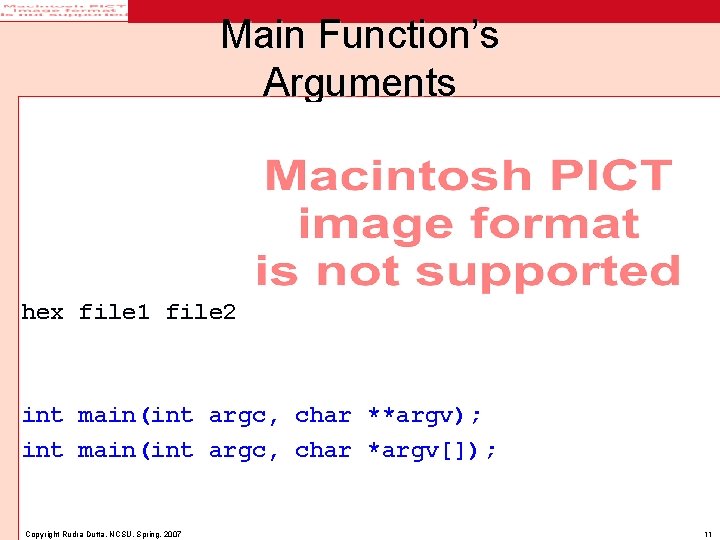
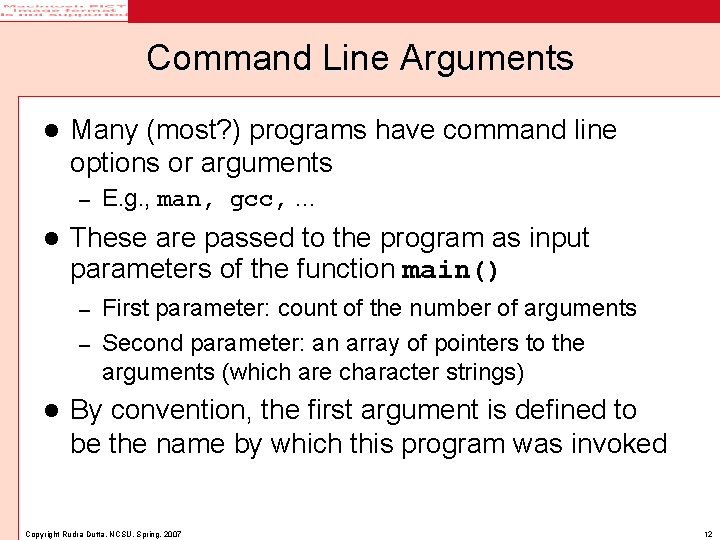
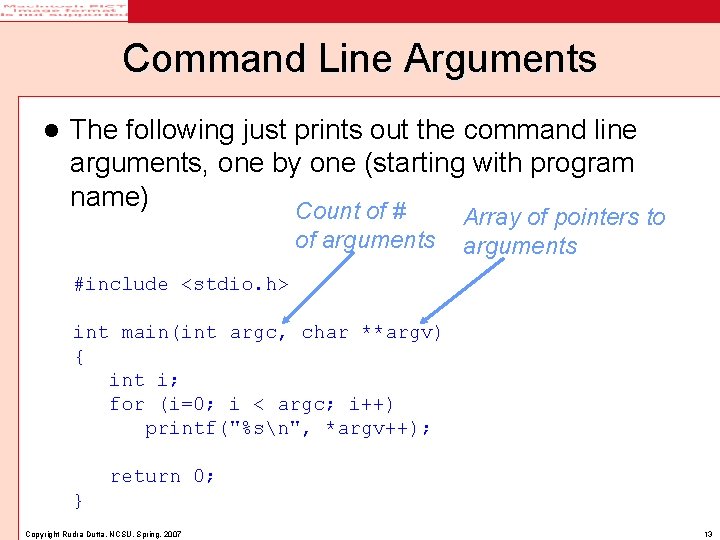
- Slides: 13
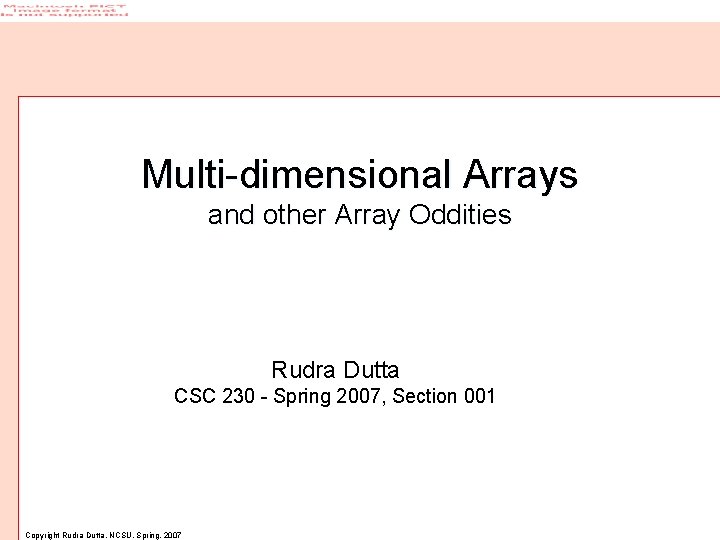
Multi-dimensional Arrays and other Array Oddities Rudra Dutta CSC 230 - Spring 2007, Section 001 Copyright Rudra Dutta, NCSU, Spring, 2007
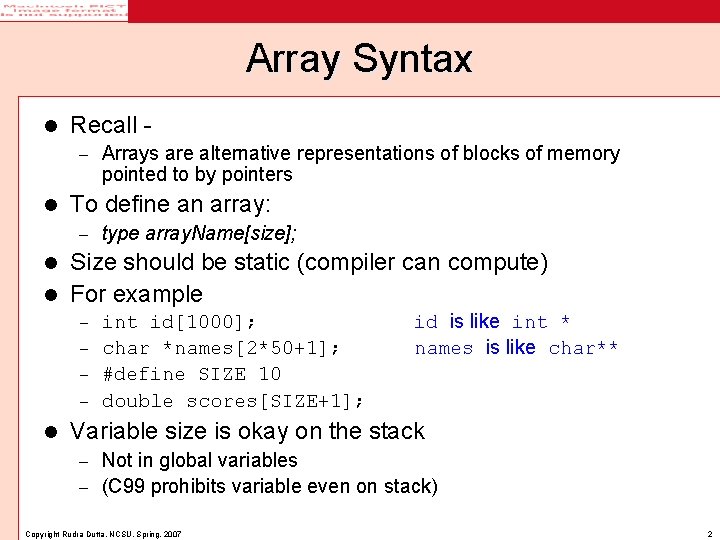
Array Syntax l Recall – l Arrays are alternative representations of blocks of memory pointed to by pointers To define an array: – type array. Name[size]; Size should be static (compiler can compute) l For example l int id[1000]; – char *names[2*50+1]; – #define SIZE 10 – double scores[SIZE+1]; – l id is like int * names is like char** Variable size is okay on the stack Not in global variables – (C 99 prohibits variable even on stack) – Copyright Rudra Dutta, NCSU, Spring, 2007 2
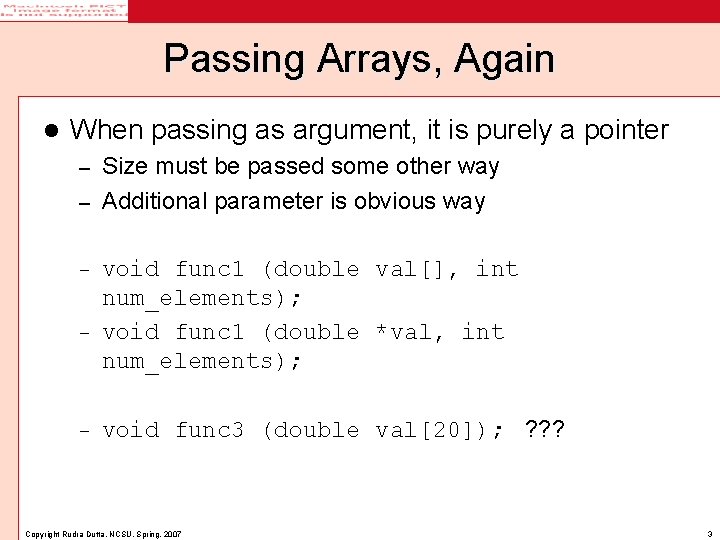
Passing Arrays, Again l When passing as argument, it is purely a pointer Size must be passed some other way – Additional parameter is obvious way – void func 1 (double val[], int num_elements); – void func 1 (double *val, int num_elements); – – void func 3 (double val[20]); ? ? ? Copyright Rudra Dutta, NCSU, Spring, 2007 3
![Arrays and sizeof int id 1000 int pointer Id l l l sizeofid is Arrays and sizeof int id [1000]; int *pointer. Id; l l l sizeof(id) is](https://slidetodoc.com/presentation_image_h2/cc2cdc4f556b9256b0f19cd9759accc5/image-4.jpg)
Arrays and sizeof int id [1000]; int *pointer. Id; l l l sizeof(id) is 1000*sizeof(int) – NOT 1000 l sizeof(pointer. Id) is the number of bytes used to store a pointer to an int. l The following sets pointer. Id to the last element of the array id: pointer. Id = id + sizeof(id)/sizeof(id[0]) - 1; Copyright Rudra Dutta, NCSU, Spring, 2007 4
![Passing Arrays id1 20 pid20 1 vid0 6 tid6 21 Passing Arrays id[1] = 20; pid[20] = 1; vid[0] = 6; tid[6] = 21;](https://slidetodoc.com/presentation_image_h2/cc2cdc4f556b9256b0f19cd9759accc5/image-5.jpg)
Passing Arrays id[1] = 20; pid[20] = 1; vid[0] = 6; tid[6] = 21; #include <stdio. h> #include <stdlib. h> printf ("%d %dn", sizeof(id), sizeof(pid), sizeof(vid), k, sizeof(tid), sizeof(iid)); typedef int mintar[1000]; void test (int fid[20]) { printf ("%d n", sizeof(fid)); } test(id); } int main () { int id[1000]; int * const pid = malloc (1000 * sizeof(int)); int k = rand(); int vid[k]; mintar tid; int iid[] = {1, 2, 3}; Copyright Rudra Dutta, NCSU, Spring, 2007 5
![2 dimensional Arrays int values23 l Recall that values looks just like a pointer 2 -dimensional Arrays int values[2][3]; l Recall that values[] looks just like a pointer](https://slidetodoc.com/presentation_image_h2/cc2cdc4f556b9256b0f19cd9759accc5/image-6.jpg)
2 -dimensional Arrays int values[2][3]; l Recall that values[] looks just like a pointer access l int a [3] l – l “a is a pointer to a block of 3 ints” int values [2] [3]; – “values is an array of 2 pointers to block of 3 ints” – “Pointer to block of 2 pointers to block of 3 ints” But internally “flattened” l Can be accessed as pointers l Copyright Rudra Dutta, NCSU, Spring, 2007 6
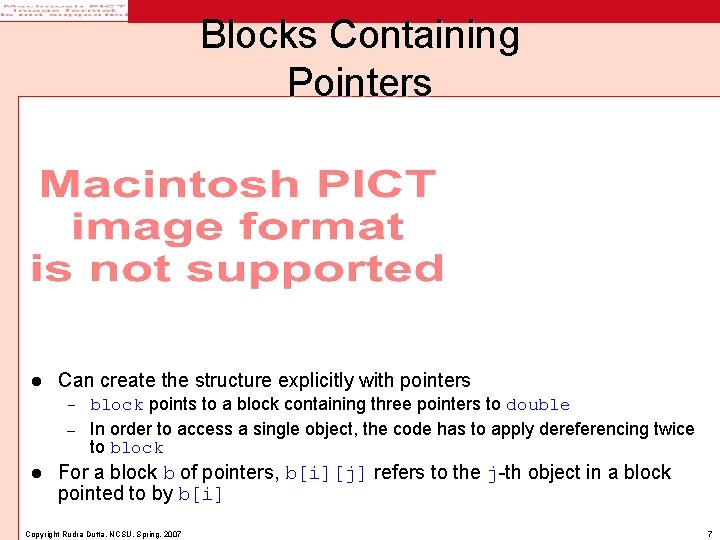
Blocks Containing Pointers l Can create the structure explicitly with pointers block points to a block containing three pointers to double – In order to access a single object, the code has to apply dereferencing twice to block – l For a block b of pointers, b[i][j] refers to the j-th object in a block pointed to by b[i] Copyright Rudra Dutta, NCSU, Spring, 2007 7
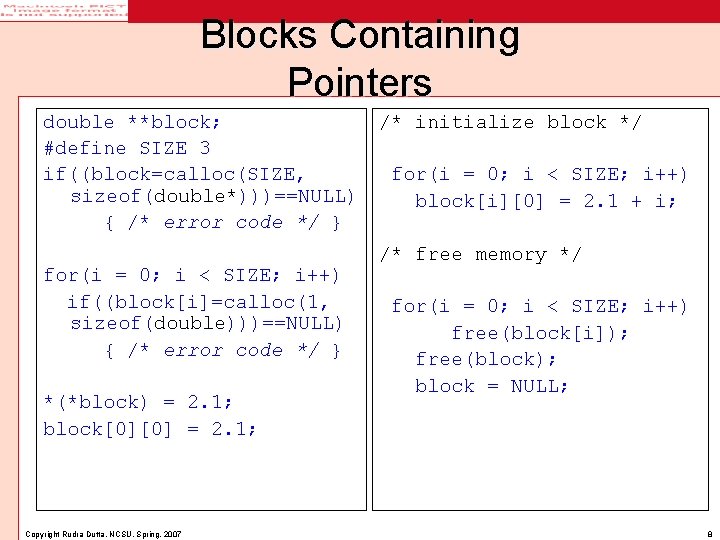
Blocks Containing Pointers double **block; #define SIZE 3 if((block=calloc(SIZE, sizeof(double*)))==NULL) { /* error code */ } for(i = 0; i < SIZE; i++) if((block[i]=calloc(1, sizeof(double)))==NULL) { /* error code */ } *(*block) = 2. 1; block[0][0] = 2. 1; Copyright Rudra Dutta, NCSU, Spring, 2007 /* initialize block */ for(i = 0; i < SIZE; i++) block[i][0] = 2. 1 + i; /* free memory */ for(i = 0; i < SIZE; i++) free(block[i]); free(block); block = NULL; 8
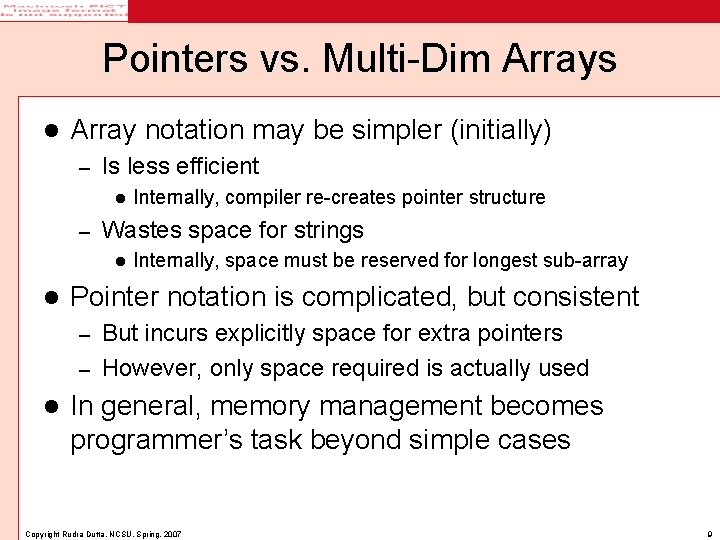
Pointers vs. Multi-Dim Arrays l Array notation may be simpler (initially) – Is less efficient l – Wastes space for strings l l Internally, compiler re-creates pointer structure Internally, space must be reserved for longest sub-array Pointer notation is complicated, but consistent But incurs explicitly space for extra pointers – However, only space required is actually used – l In general, memory management becomes programmer’s task beyond simple cases Copyright Rudra Dutta, NCSU, Spring, 2007 9
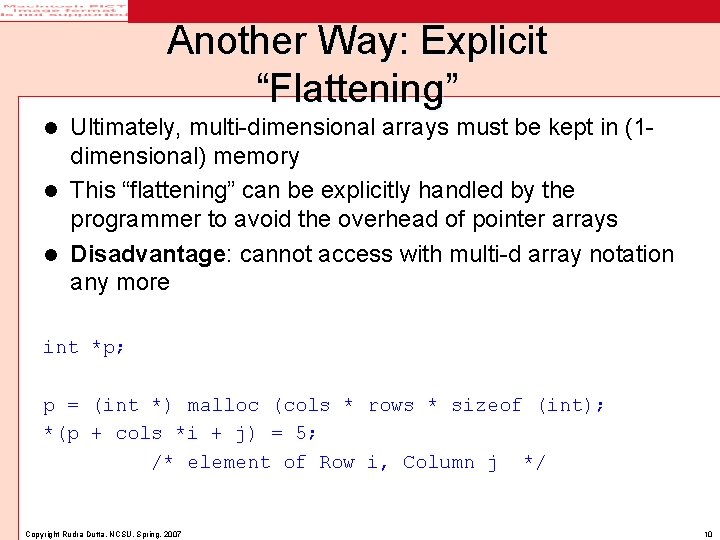
Another Way: Explicit “Flattening” Ultimately, multi-dimensional arrays must be kept in (1 dimensional) memory l This “flattening” can be explicitly handled by the programmer to avoid the overhead of pointer arrays l Disadvantage: cannot access with multi-d array notation any more l int *p; p = (int *) malloc (cols * rows * sizeof (int); *(p + cols *i + j) = 5; /* element of Row i, Column j */ Copyright Rudra Dutta, NCSU, Spring, 2007 10
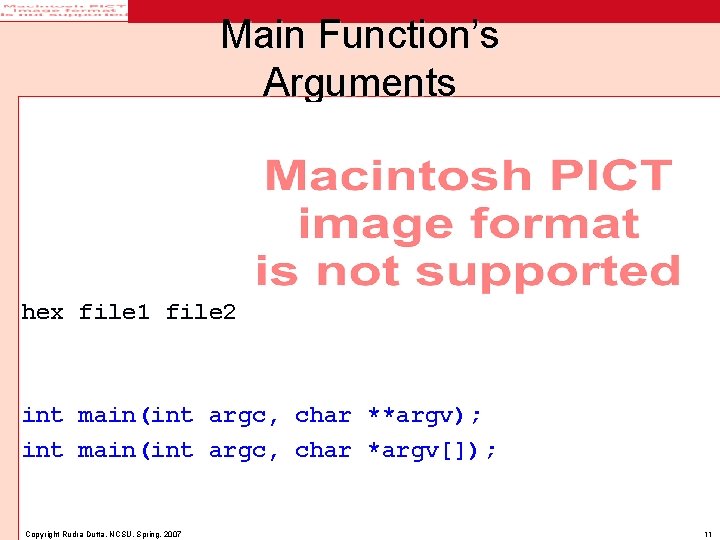
Main Function’s Arguments hex file 1 file 2 int main(int argc, char **argv); int main(int argc, char *argv[]); Copyright Rudra Dutta, NCSU, Spring, 2007 11
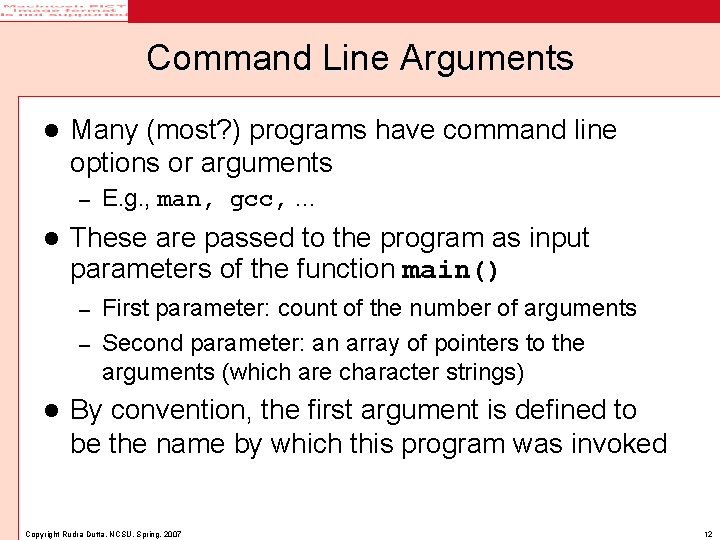
Command Line Arguments l Many (most? ) programs have command line options or arguments – l E. g. , man, gcc, … These are passed to the program as input parameters of the function main() First parameter: count of the number of arguments – Second parameter: an array of pointers to the arguments (which are character strings) – l By convention, the first argument is defined to be the name by which this program was invoked Copyright Rudra Dutta, NCSU, Spring, 2007 12
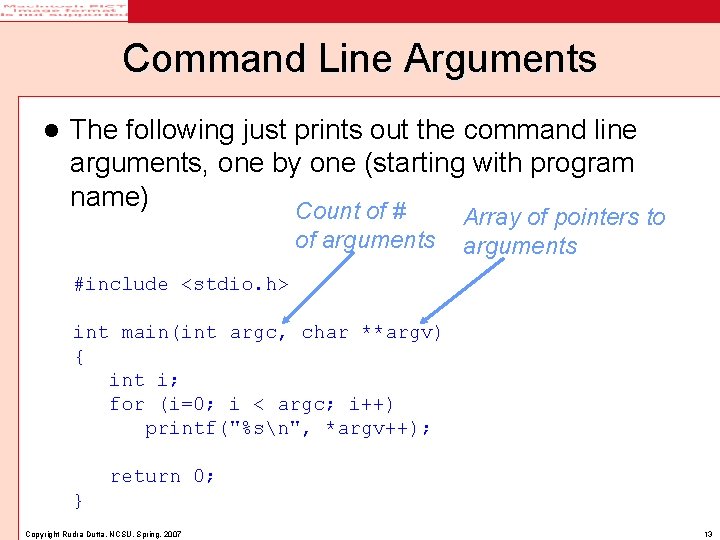
Command Line Arguments l The following just prints out the command line arguments, one by one (starting with program name) Count of # of arguments Array of pointers to arguments #include <stdio. h> int main(int argc, char **argv) { int i; for (i=0; i < argc; i++) printf("%sn", *argv++); return 0; } Copyright Rudra Dutta, NCSU, Spring, 2007 13
Jagged array
Array of arrays c++
Multidimensional array
Arrays in pascal
Systemverilog multidimensional array initialization
Processing multidimensional array
Array 1 dimensi dan 2 dimensi
C# multidimensional array initialization
Java copy array
Niloy dutta uconn
Chris keane wsu
Anup dutta hcl
Prabal dutta
Dipanwita dutta