Module 4 LOO PS AND REPET ITION Initial
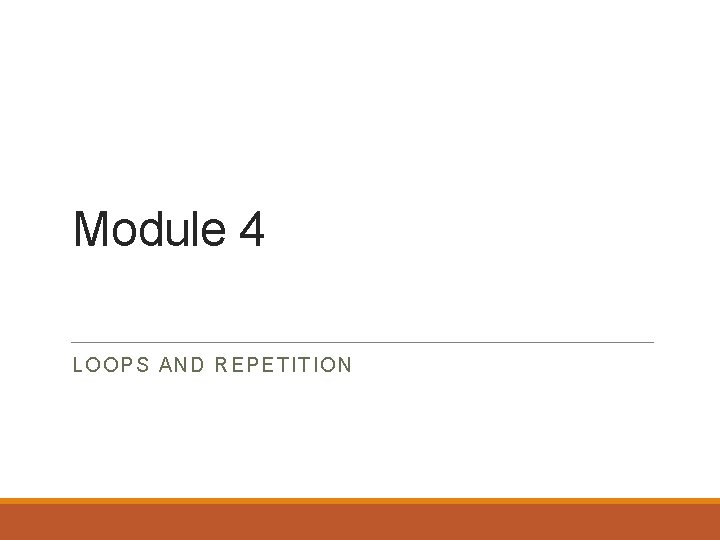
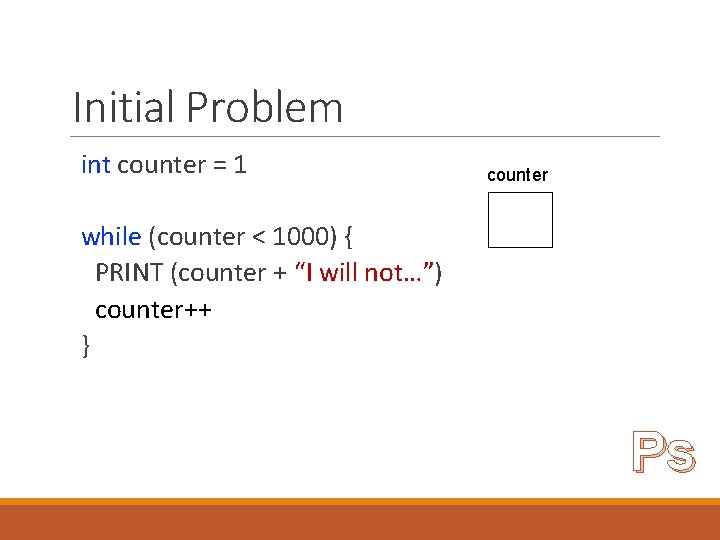
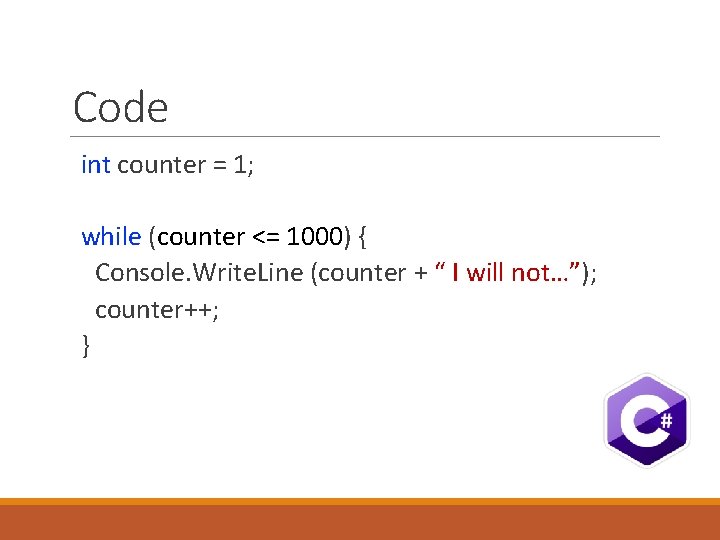
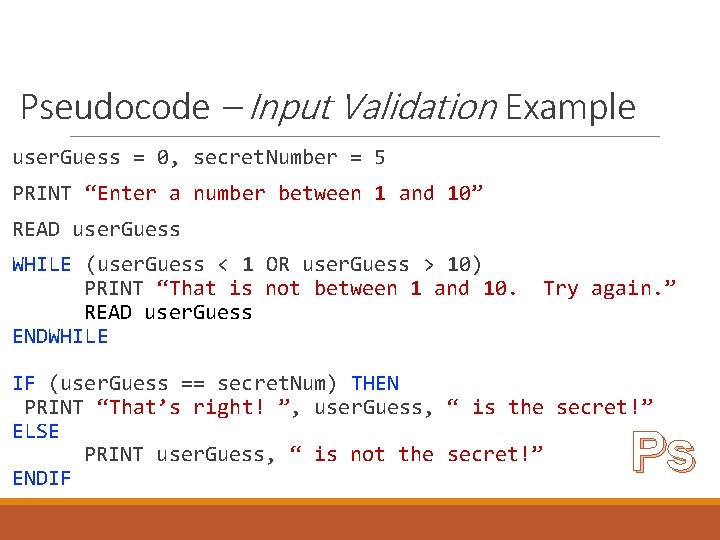
![Input Validation Example public static void Main(string[] args) { int user. Guess = 0, Input Validation Example public static void Main(string[] args) { int user. Guess = 0,](https://slidetodoc.com/presentation_image_h2/2c8e39f7a6f2c02bffebf5d7cc2bbc9a/image-5.jpg)
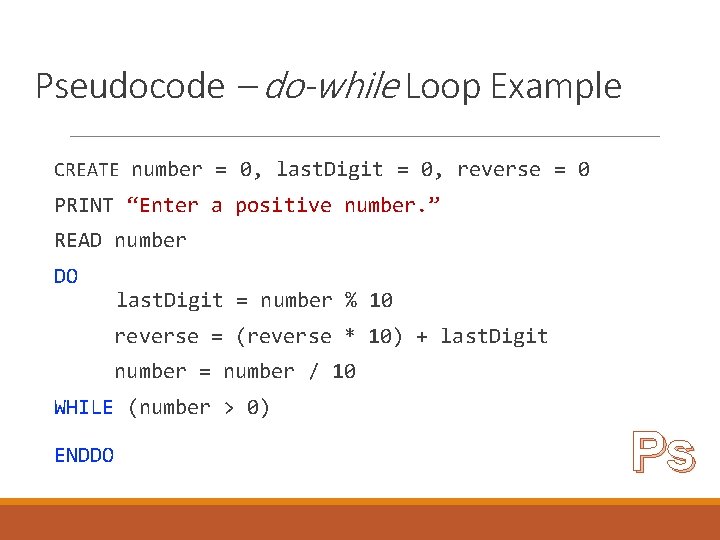
![C# - do-while Loop Example public static void Main(string[] args) { int number=0, last. C# - do-while Loop Example public static void Main(string[] args) { int number=0, last.](https://slidetodoc.com/presentation_image_h2/2c8e39f7a6f2c02bffebf5d7cc2bbc9a/image-7.jpg)
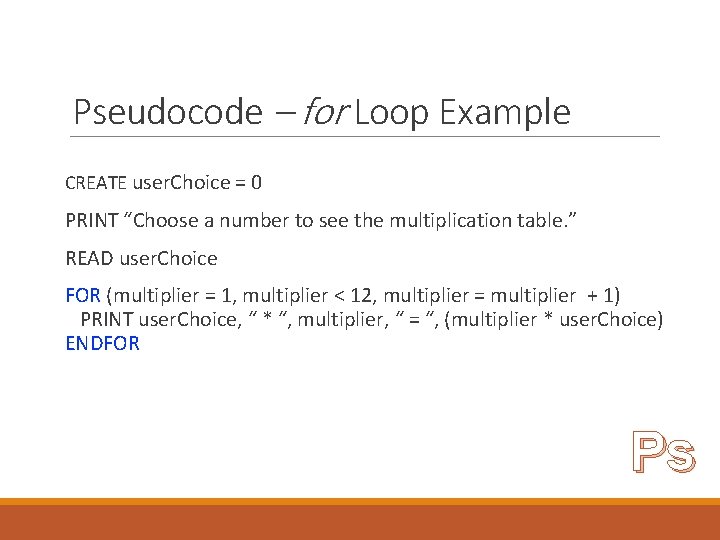
![C# - for Loop Example public static void Main(string[] args) { int choice = C# - for Loop Example public static void Main(string[] args) { int choice =](https://slidetodoc.com/presentation_image_h2/2c8e39f7a6f2c02bffebf5d7cc2bbc9a/image-9.jpg)
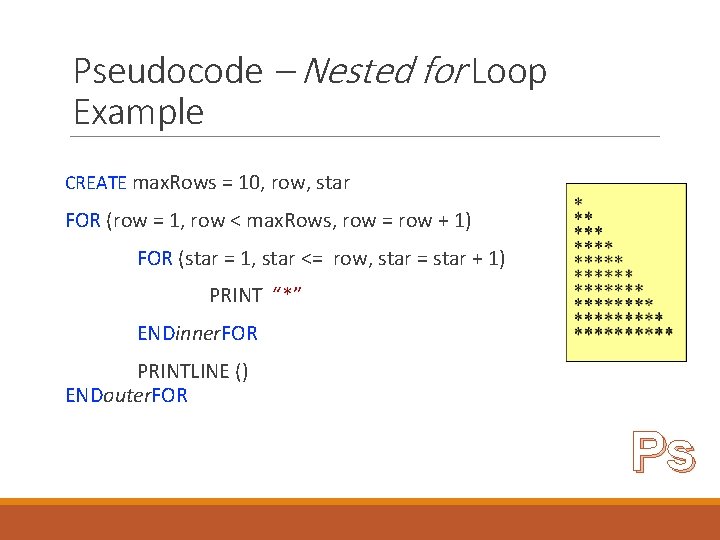
![C# - Nested for Loop Example public static void Main(string[] args) { int max. C# - Nested for Loop Example public static void Main(string[] args) { int max.](https://slidetodoc.com/presentation_image_h2/2c8e39f7a6f2c02bffebf5d7cc2bbc9a/image-11.jpg)
- Slides: 11
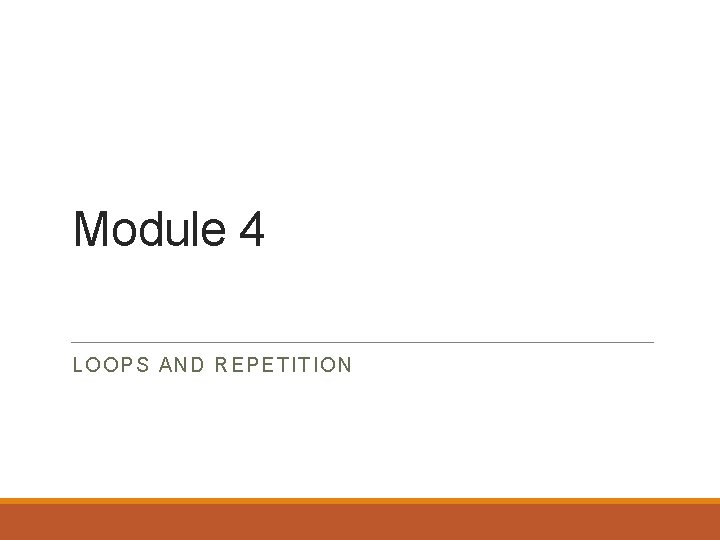
Module 4 LOO PS AND REPET ITION
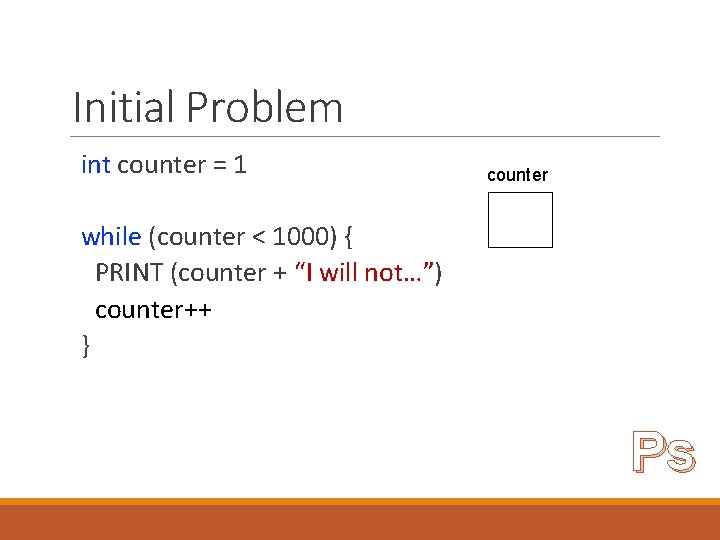
Initial Problem int counter = 1 counter while (counter < 1000) { PRINT (counter + “I will not…”) counter++ } Ps
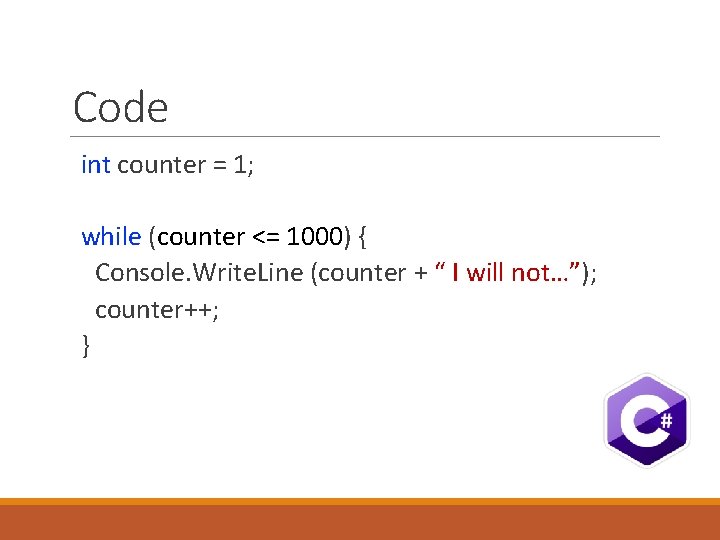
Code int counter = 1; while (counter <= 1000) { Console. Write. Line (counter + “ I will not…”); counter++; }
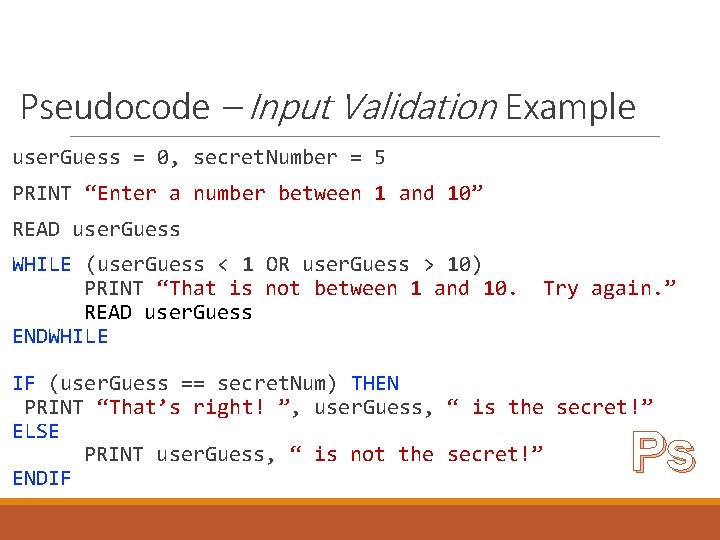
Pseudocode – Input Validation Example user. Guess = 0, secret. Number = 5 PRINT “Enter a number between 1 and 10” READ user. Guess WHILE (user. Guess < 1 OR user. Guess > 10) PRINT “That is not between 1 and 10. READ user. Guess ENDWHILE Try again. ” IF (user. Guess == secret. Num) THEN PRINT “That’s right! ”, user. Guess, “ is the secret!” ELSE PRINT user. Guess, “ is not the secret!” ENDIF Ps
![Input Validation Example public static void Mainstring args int user Guess 0 Input Validation Example public static void Main(string[] args) { int user. Guess = 0,](https://slidetodoc.com/presentation_image_h2/2c8e39f7a6f2c02bffebf5d7cc2bbc9a/image-5.jpg)
Input Validation Example public static void Main(string[] args) { int user. Guess = 0, secret. Num = 5; Console. Write. Line("Enter a number between 1 and 10: "); user. Guess = Convert. To. Int 32(Console. Read. Line()); while(user. Guess < 1 || user. Guess > 10) { Console. Write. Line("That’s not between 1 and 10. Try again!"); user. Guess = Convert. To. Int 32(Console. Read. Line()); } if (user. Guess == secret. Num) { Console. Write. Line(user. Guess + " is the secret number!"); } else { Console. Write. Line(user. Guess + " is not the secret number!"); } }
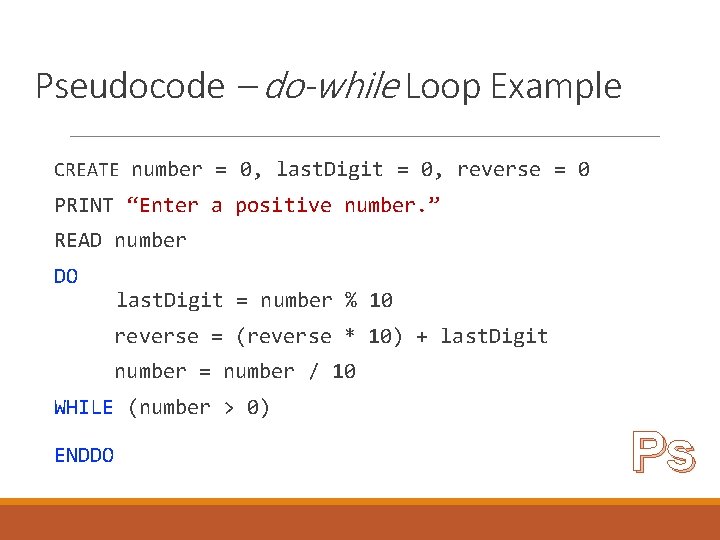
Pseudocode – do-while Loop Example CREATE number = 0, last. Digit = 0, reverse = 0 PRINT “Enter a positive number. ” READ number DO last. Digit = number % 10 reverse = (reverse * 10) + last. Digit number = number / 10 WHILE (number > 0) ENDDO Ps
![C dowhile Loop Example public static void Mainstring args int number0 last C# - do-while Loop Example public static void Main(string[] args) { int number=0, last.](https://slidetodoc.com/presentation_image_h2/2c8e39f7a6f2c02bffebf5d7cc2bbc9a/image-7.jpg)
C# - do-while Loop Example public static void Main(string[] args) { int number=0, last. Digit=0, reverse = 0; Console. Write. Line("Enter a positive integer: "); number = Convert. To. Int 32(Console. Read. Line()); do { last. Digit = number % 10; reverse = (reverse * 10) + last. Digit; number = number / 10; } while (number > 0); // NOTE THE SEMICOLON!!!! Console. Write. Line("That number reversed is " + reverse); }
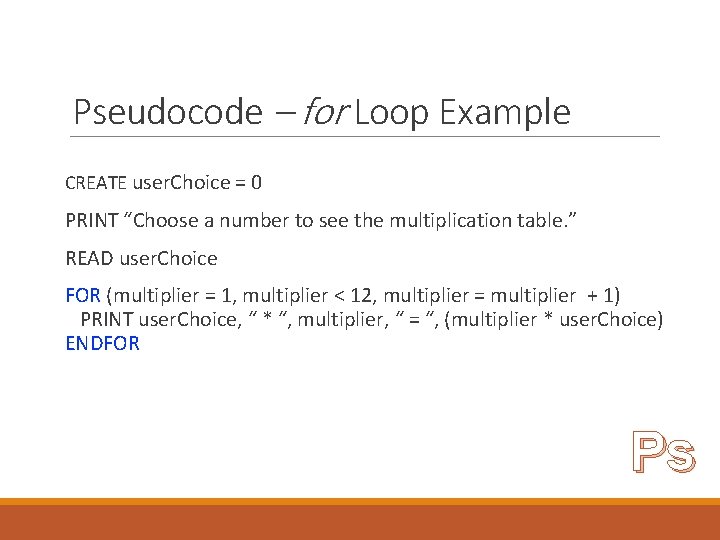
Pseudocode – for Loop Example CREATE user. Choice = 0 PRINT “Choose a number to see the multiplication table. ” READ user. Choice FOR (multiplier = 1, multiplier < 12, multiplier = multiplier + 1) PRINT user. Choice, “ * “, multiplier, “ = “, (multiplier * user. Choice) ENDFOR Ps
![C for Loop Example public static void Mainstring args int choice C# - for Loop Example public static void Main(string[] args) { int choice =](https://slidetodoc.com/presentation_image_h2/2c8e39f7a6f2c02bffebf5d7cc2bbc9a/image-9.jpg)
C# - for Loop Example public static void Main(string[] args) { int choice = 0; Console. Write. Line("Enter a number for the table. "); choice = Convert. To. Int 32(Console. Read. Line()); for(int multiplier = 1; multiplier <= 12; multiplier += 1) { Console. Write. Line(multiplier + " * " + choice + " = " + (multiplier * choice)); } }
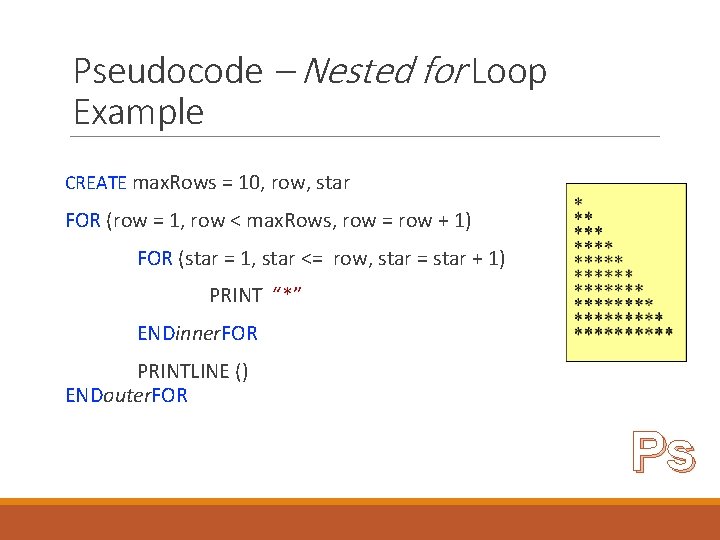
Pseudocode – Nested for Loop Example CREATE max. Rows = 10, row, star FOR (row = 1, row < max. Rows, row = row + 1) FOR (star = 1, star <= row, star = star + 1) PRINT “*” ENDinner. FOR PRINTLINE () ENDouter. FOR Ps
![C Nested for Loop Example public static void Mainstring args int max C# - Nested for Loop Example public static void Main(string[] args) { int max.](https://slidetodoc.com/presentation_image_h2/2c8e39f7a6f2c02bffebf5d7cc2bbc9a/image-11.jpg)
C# - Nested for Loop Example public static void Main(string[] args) { int max. Rows = 10; for (int row = 1; row <= max. Rows; row++) { for (int star = 1; star <= row; star ++) { Console. Write("*"); } Console. Write. Line(); } }
Suffixes ion ation ition
Lucy liu what my girl do
Loo wit the firekeeper theme
Byzantine generals problem solution
Fons van de loo
Janneke van der loo
Yong loo lin school of medicine
Kaganapan sa el filibusterismo noong 1889
C device module module 1
System planning and initial investigation
Descriptors grading scale and remarks
Initial velocity and final velocity formula