Memory and Addresses Pointers in C 1 Memory
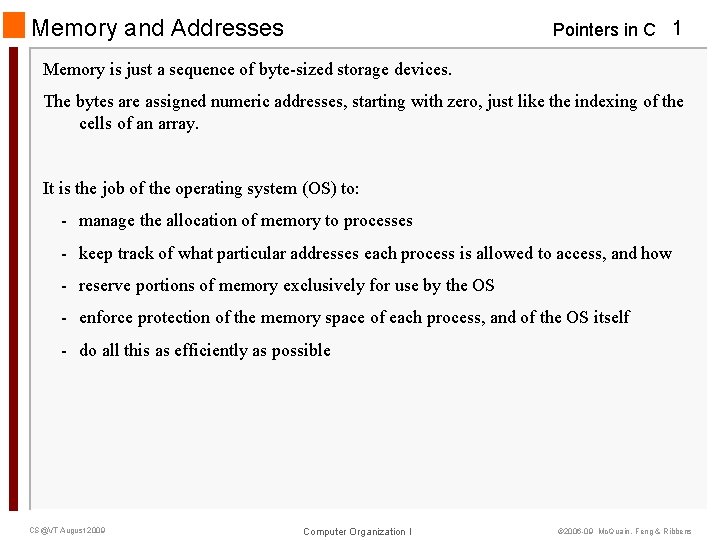
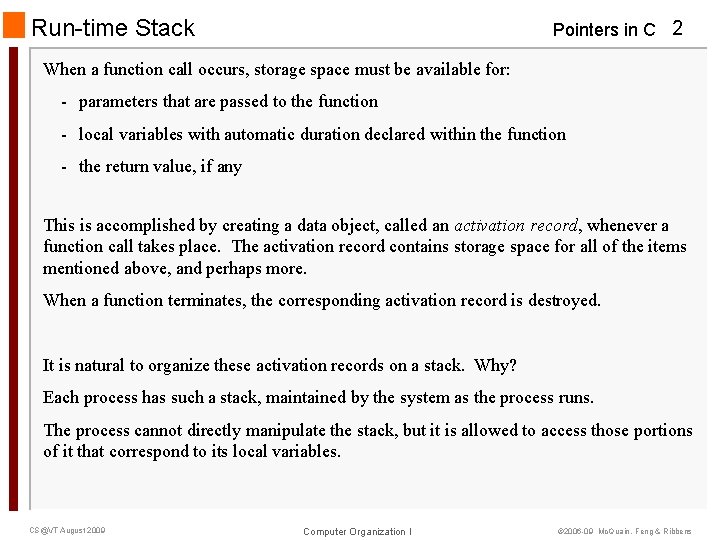
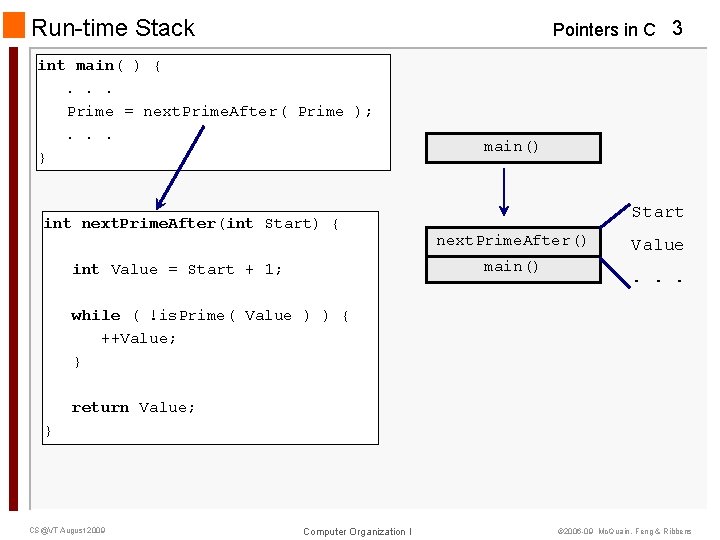
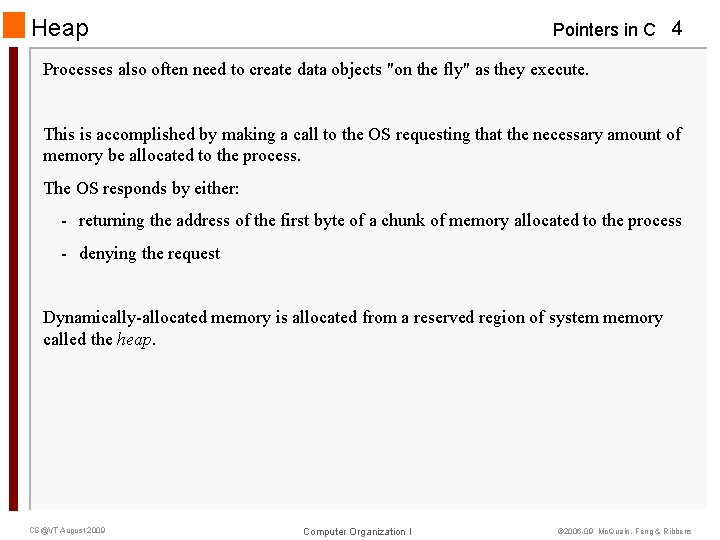
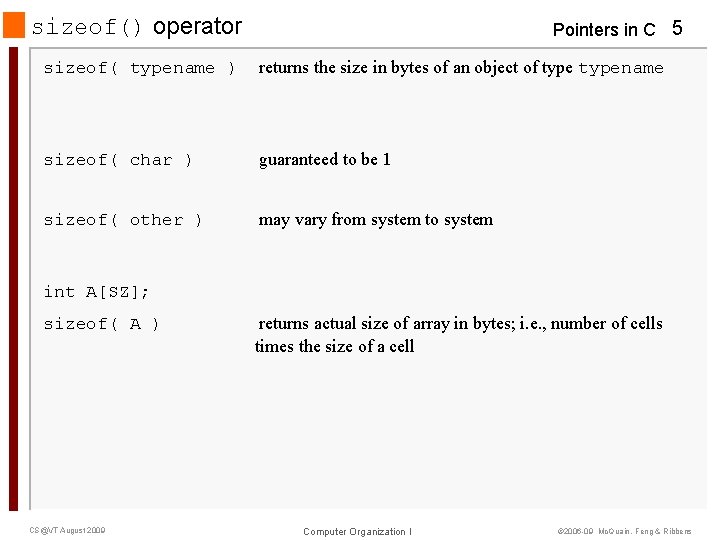
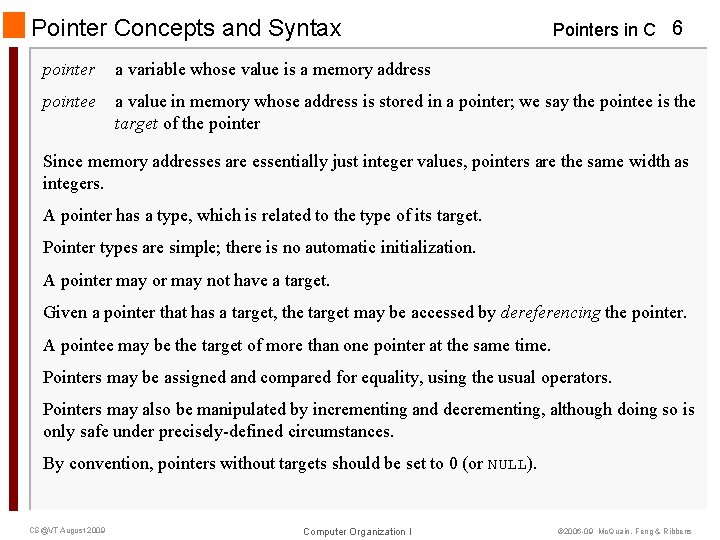
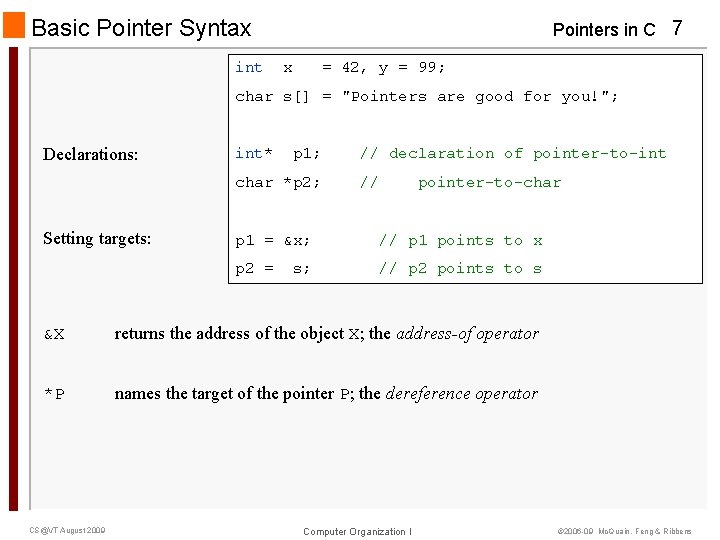
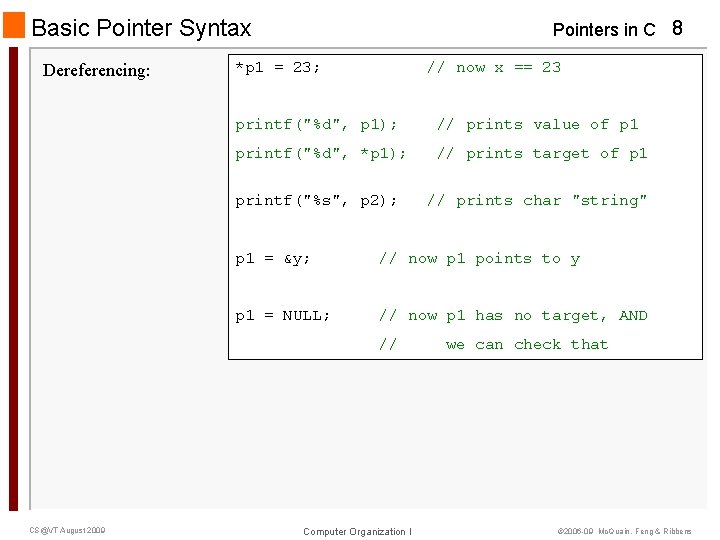
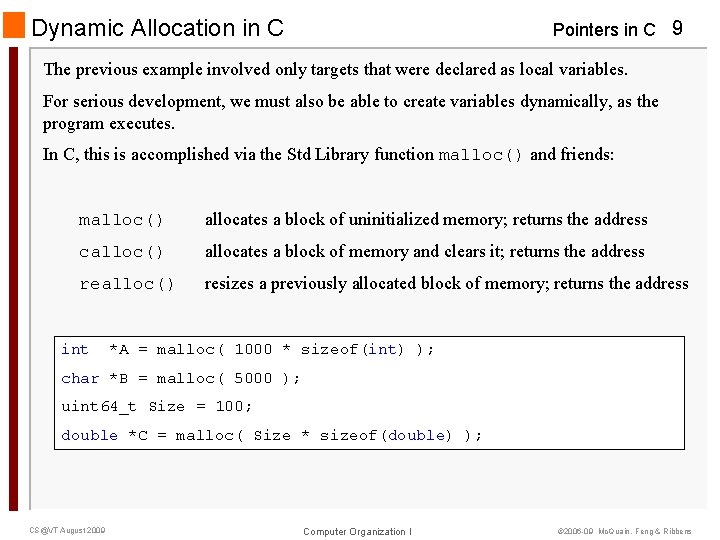
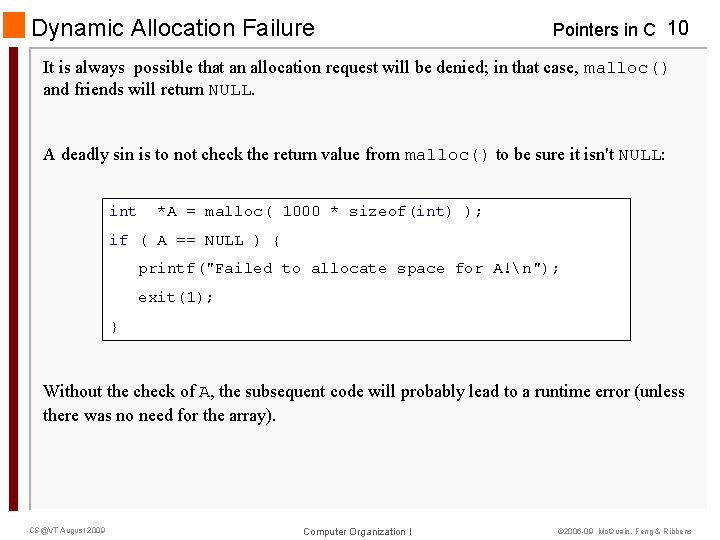
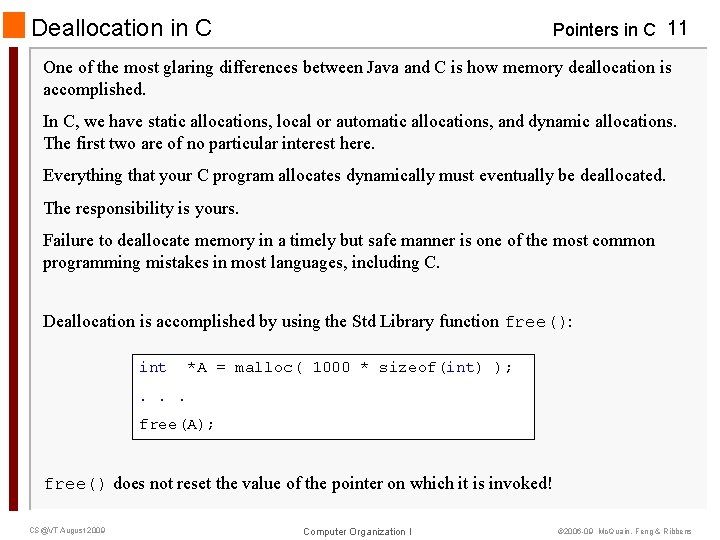
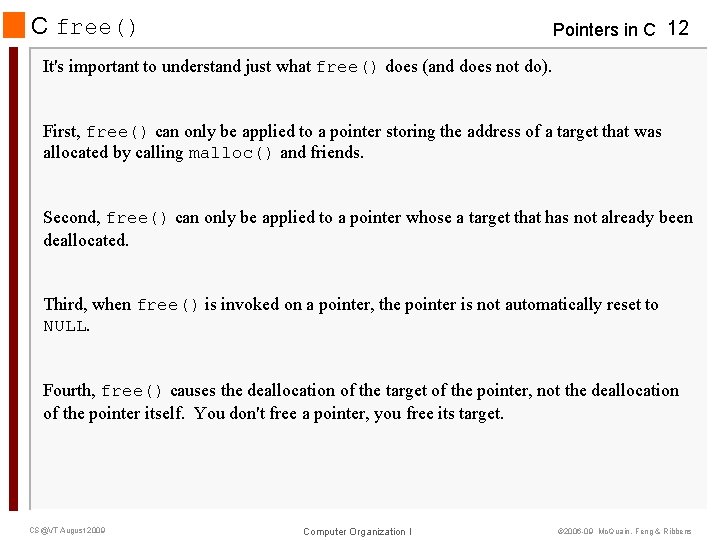
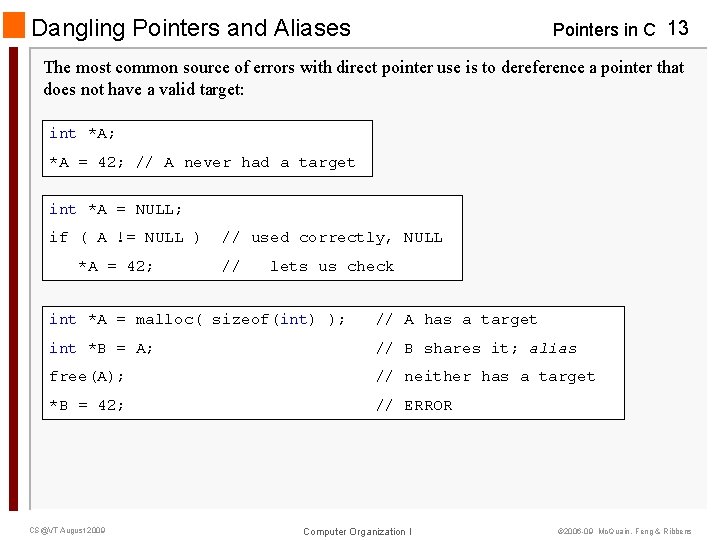
- Slides: 13
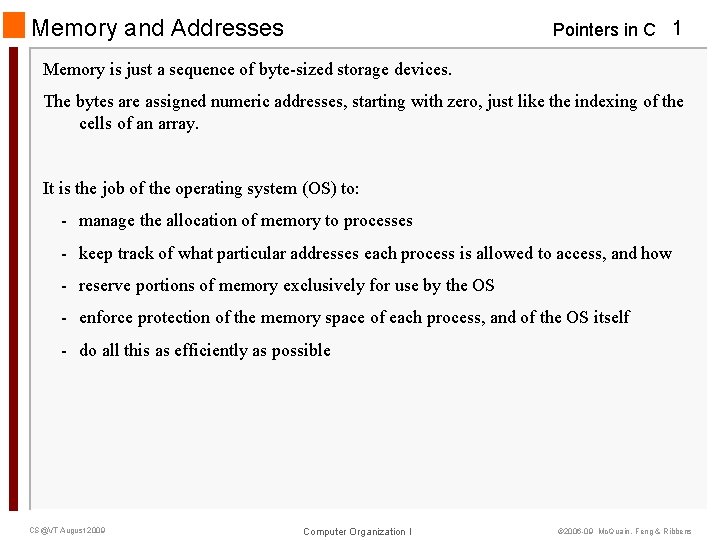
Memory and Addresses Pointers in C 1 Memory is just a sequence of byte-sized storage devices. The bytes are assigned numeric addresses, starting with zero, just like the indexing of the cells of an array. It is the job of the operating system (OS) to: - manage the allocation of memory to processes - keep track of what particular addresses each process is allowed to access, and how - reserve portions of memory exclusively for use by the OS - enforce protection of the memory space of each process, and of the OS itself - do all this as efficiently as possible CS@VT August 2009 Computer Organization I © 2006 -09 Mc. Quain, Feng & Ribbens
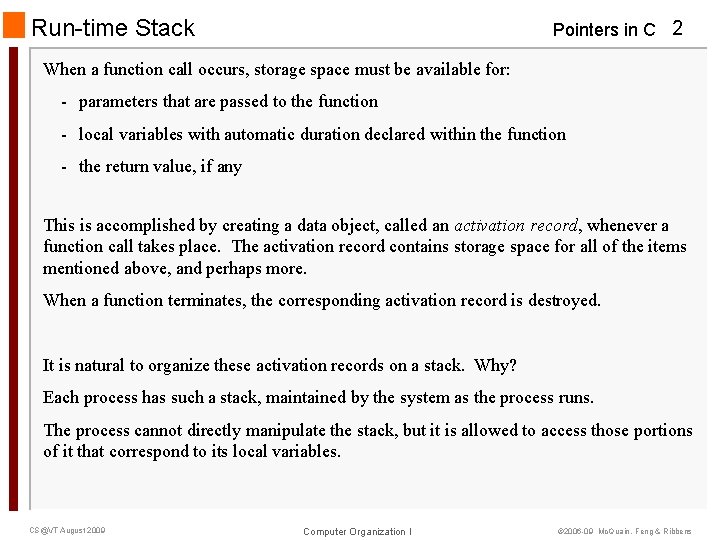
Run-time Stack Pointers in C 2 When a function call occurs, storage space must be available for: - parameters that are passed to the function - local variables with automatic duration declared within the function - the return value, if any This is accomplished by creating a data object, called an activation record, whenever a function call takes place. The activation record contains storage space for all of the items mentioned above, and perhaps more. When a function terminates, the corresponding activation record is destroyed. It is natural to organize these activation records on a stack. Why? Each process has such a stack, maintained by the system as the process runs. The process cannot directly manipulate the stack, but it is allowed to access those portions of it that correspond to its local variables. CS@VT August 2009 Computer Organization I © 2006 -09 Mc. Quain, Feng & Ribbens
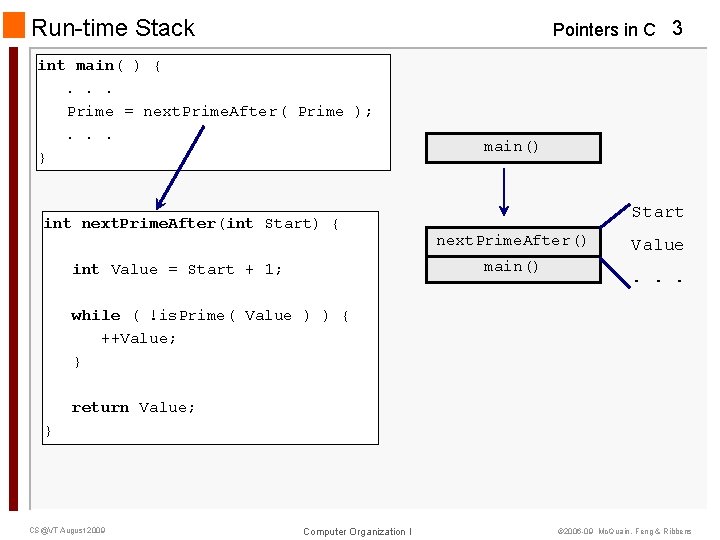
Run-time Stack Pointers in C 3 int main( ) {. . . Prime = next. Prime. After( Prime ); . . . } int next. Prime. After(int Start) { main() Start next. Prime. After() main() int Value = Start + 1; Value. . . while ( !is. Prime( Value ) ) { ++Value; } return Value; } CS@VT August 2009 Computer Organization I © 2006 -09 Mc. Quain, Feng & Ribbens
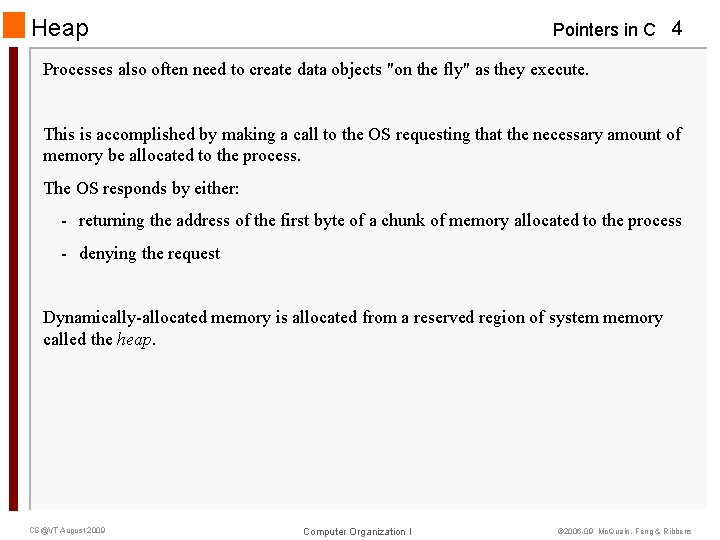
Heap Pointers in C 4 Processes also often need to create data objects "on the fly" as they execute. This is accomplished by making a call to the OS requesting that the necessary amount of memory be allocated to the process. The OS responds by either: - returning the address of the first byte of a chunk of memory allocated to the process - denying the request Dynamically-allocated memory is allocated from a reserved region of system memory called the heap. CS@VT August 2009 Computer Organization I © 2006 -09 Mc. Quain, Feng & Ribbens
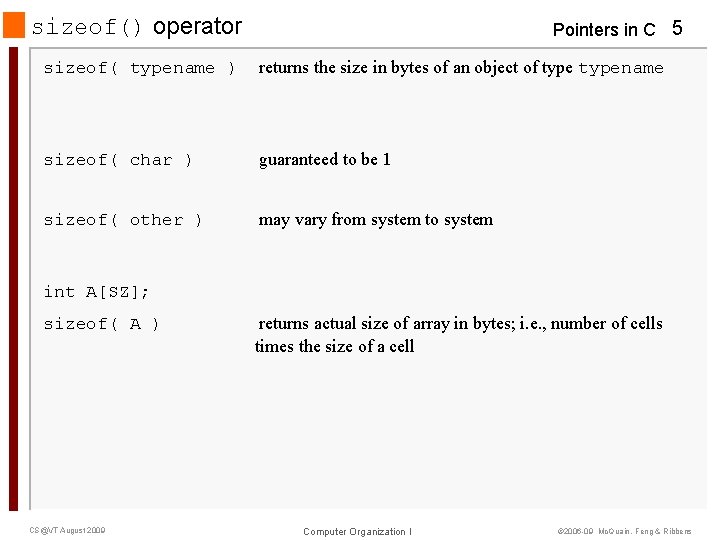
sizeof() operator Pointers in C 5 sizeof( typename ) returns the size in bytes of an object of typename sizeof( char ) guaranteed to be 1 sizeof( other ) may vary from system to system int A[SZ]; sizeof( A ) CS@VT August 2009 returns actual size of array in bytes; i. e. , number of cells times the size of a cell Computer Organization I © 2006 -09 Mc. Quain, Feng & Ribbens
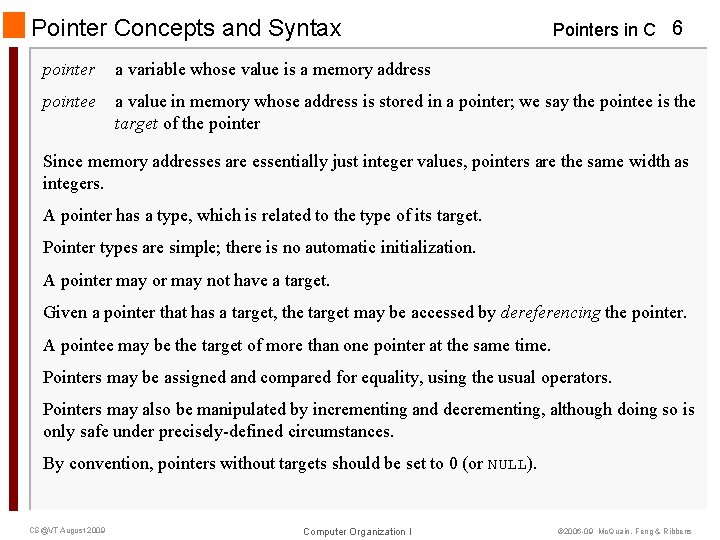
Pointer Concepts and Syntax Pointers in C 6 pointer a variable whose value is a memory address pointee a value in memory whose address is stored in a pointer; we say the pointee is the target of the pointer Since memory addresses are essentially just integer values, pointers are the same width as integers. A pointer has a type, which is related to the type of its target. Pointer types are simple; there is no automatic initialization. A pointer may or may not have a target. Given a pointer that has a target, the target may be accessed by dereferencing the pointer. A pointee may be the target of more than one pointer at the same time. Pointers may be assigned and compared for equality, using the usual operators. Pointers may also be manipulated by incrementing and decrementing, although doing so is only safe under precisely-defined circumstances. By convention, pointers without targets should be set to 0 (or NULL). CS@VT August 2009 Computer Organization I © 2006 -09 Mc. Quain, Feng & Ribbens
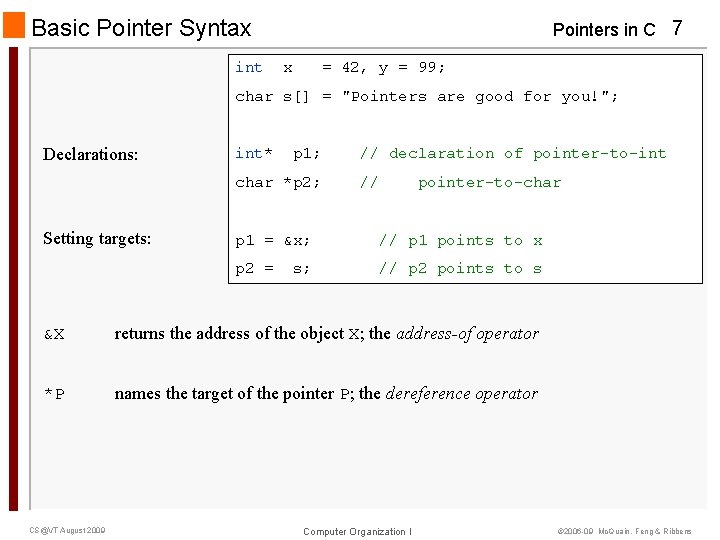
Basic Pointer Syntax int Pointers in C 7 x = 42, y = 99; char s[] = "Pointers are good for you!"; Declarations: int* p 1; char *p 2; Setting targets: // declaration of pointer-to-int // pointer-to-char p 1 = &x; // p 1 points to x p 2 = // p 2 points to s s; &X returns the address of the object X; the address-of operator *P names the target of the pointer P; the dereference operator CS@VT August 2009 Computer Organization I © 2006 -09 Mc. Quain, Feng & Ribbens
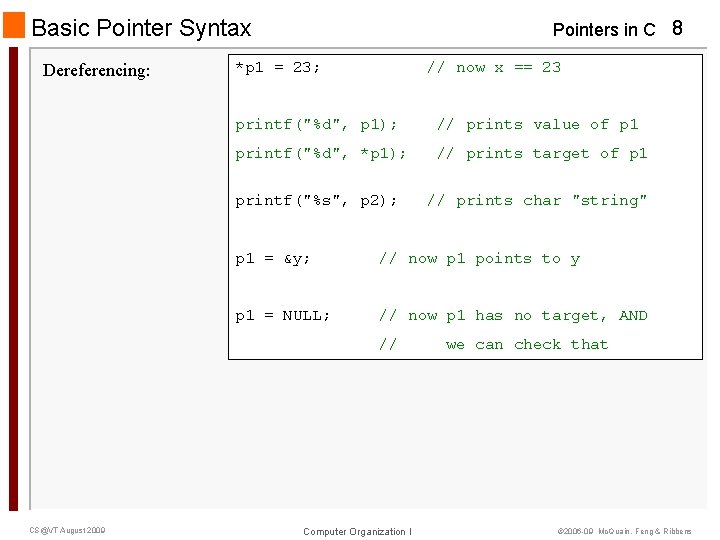
Basic Pointer Syntax Dereferencing: Pointers in C 8 *p 1 = 23; // now x == 23 printf("%d", p 1); // prints value of p 1 printf("%d", *p 1); // prints target of p 1 printf("%s", p 2); // prints char "string" p 1 = &y; // now p 1 points to y p 1 = NULL; // now p 1 has no target, AND // CS@VT August 2009 Computer Organization I we can check that © 2006 -09 Mc. Quain, Feng & Ribbens
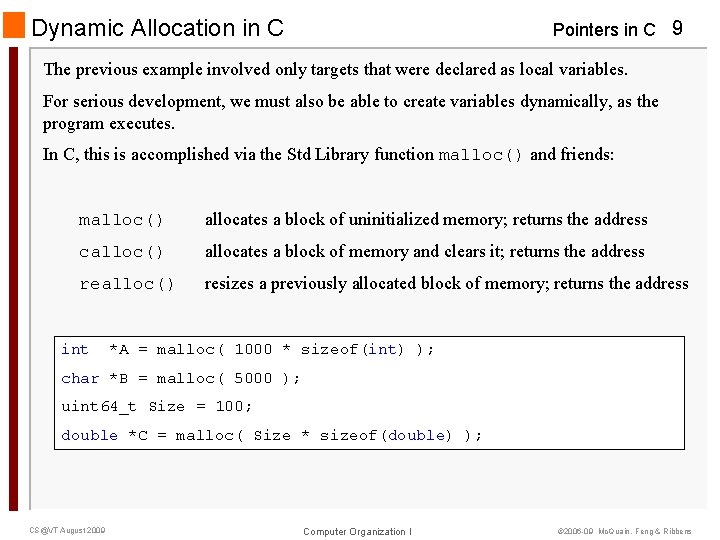
Dynamic Allocation in C Pointers in C 9 The previous example involved only targets that were declared as local variables. For serious development, we must also be able to create variables dynamically, as the program executes. In C, this is accomplished via the Std Library function malloc() and friends: malloc() allocates a block of uninitialized memory; returns the address calloc() allocates a block of memory and clears it; returns the address realloc() resizes a previously allocated block of memory; returns the address int *A = malloc( 1000 * sizeof(int) ); char *B = malloc( 5000 ); uint 64_t Size = 100; double *C = malloc( Size * sizeof(double) ); CS@VT August 2009 Computer Organization I © 2006 -09 Mc. Quain, Feng & Ribbens
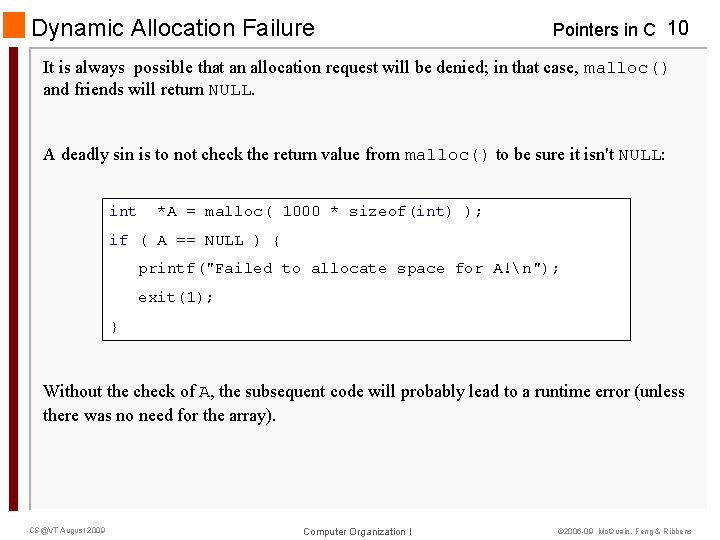
Dynamic Allocation Failure Pointers in C 10 It is always possible that an allocation request will be denied; in that case, malloc() and friends will return NULL. A deadly sin is to not check the return value from malloc() to be sure it isn't NULL: int *A = malloc( 1000 * sizeof(int) ); if ( A == NULL ) { printf("Failed to allocate space for A!n"); exit(1); } Without the check of A, the subsequent code will probably lead to a runtime error (unless there was no need for the array). CS@VT August 2009 Computer Organization I © 2006 -09 Mc. Quain, Feng & Ribbens
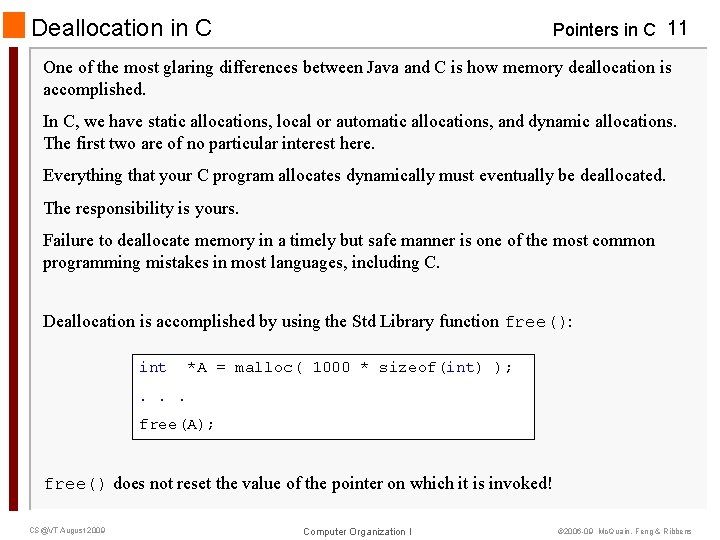
Deallocation in C Pointers in C 11 One of the most glaring differences between Java and C is how memory deallocation is accomplished. In C, we have static allocations, local or automatic allocations, and dynamic allocations. The first two are of no particular interest here. Everything that your C program allocates dynamically must eventually be deallocated. The responsibility is yours. Failure to deallocate memory in a timely but safe manner is one of the most common programming mistakes in most languages, including C. Deallocation is accomplished by using the Std Library function free(): int *A = malloc( 1000 * sizeof(int) ); . . . free(A); free() does not reset the value of the pointer on which it is invoked! CS@VT August 2009 Computer Organization I © 2006 -09 Mc. Quain, Feng & Ribbens
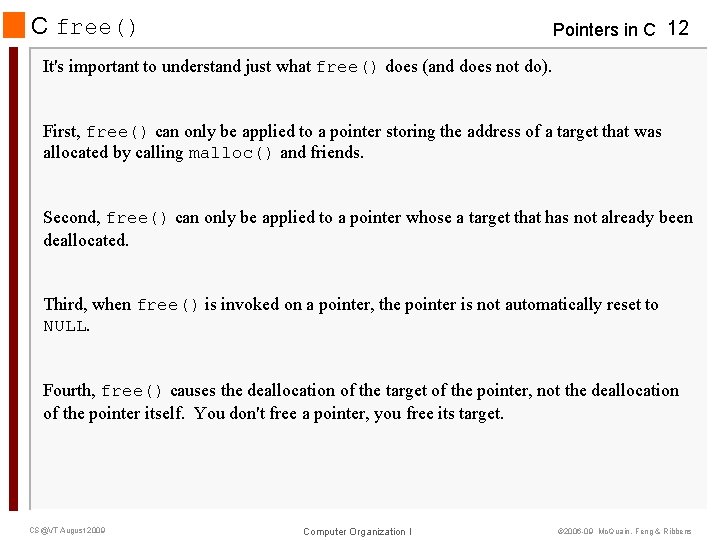
C free() Pointers in C 12 It's important to understand just what free() does (and does not do). First, free() can only be applied to a pointer storing the address of a target that was allocated by calling malloc() and friends. Second, free() can only be applied to a pointer whose a target that has not already been deallocated. Third, when free() is invoked on a pointer, the pointer is not automatically reset to NULL. Fourth, free() causes the deallocation of the target of the pointer, not the deallocation of the pointer itself. You don't free a pointer, you free its target. CS@VT August 2009 Computer Organization I © 2006 -09 Mc. Quain, Feng & Ribbens
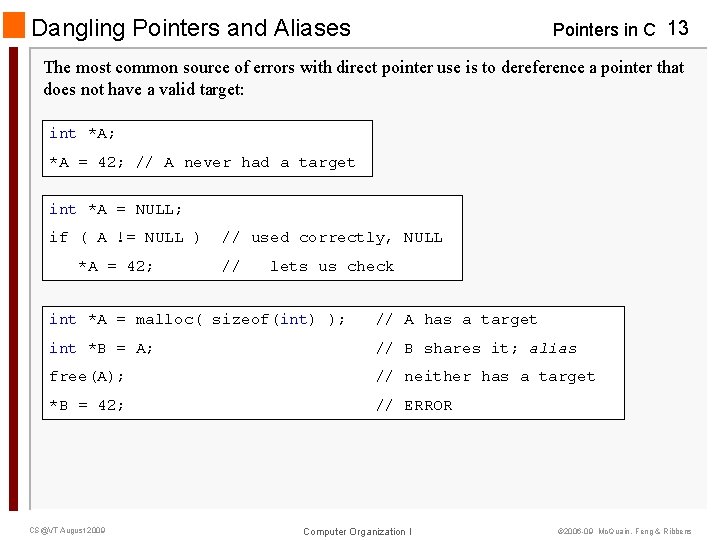
Dangling Pointers and Aliases Pointers in C 13 The most common source of errors with direct pointer use is to dereference a pointer that does not have a valid target: int *A; *A = 42; // A never had a target int *A = NULL; if ( A != NULL ) *A = 42; // used correctly, NULL // lets us check int *A = malloc( sizeof(int) ); // A has a target int *B = A; // B shares it; alias free(A); // neither has a target *B = 42; // ERROR CS@VT August 2009 Computer Organization I © 2006 -09 Mc. Quain, Feng & Ribbens