LINUX System Process additional slides Process Control Control
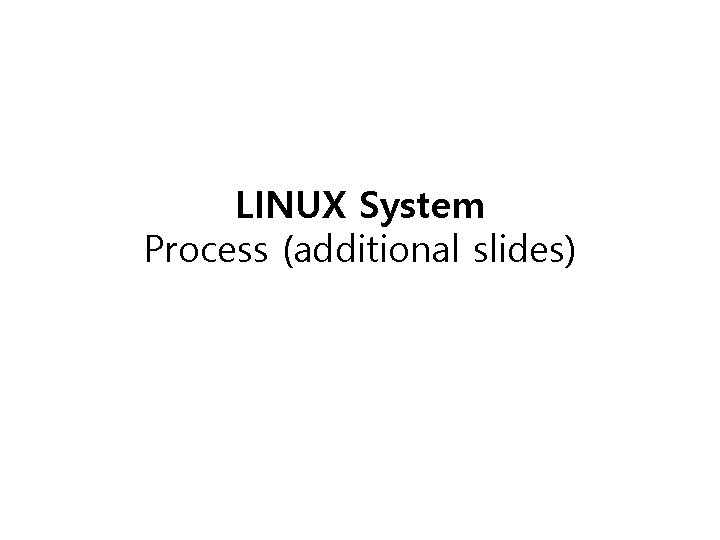
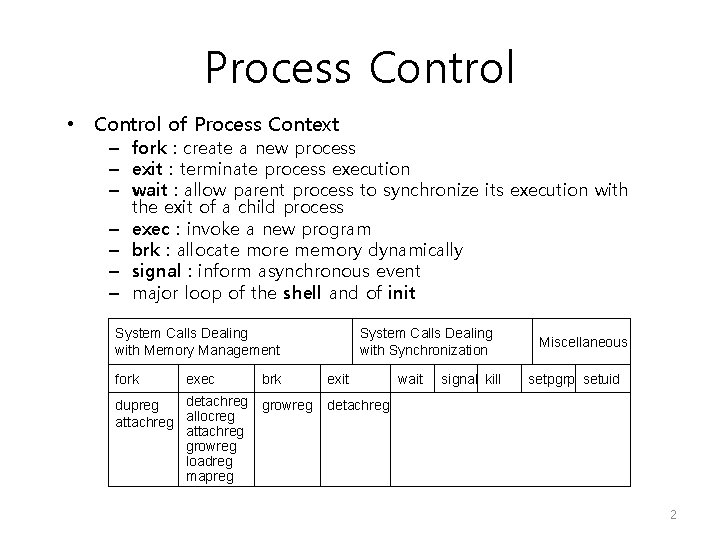
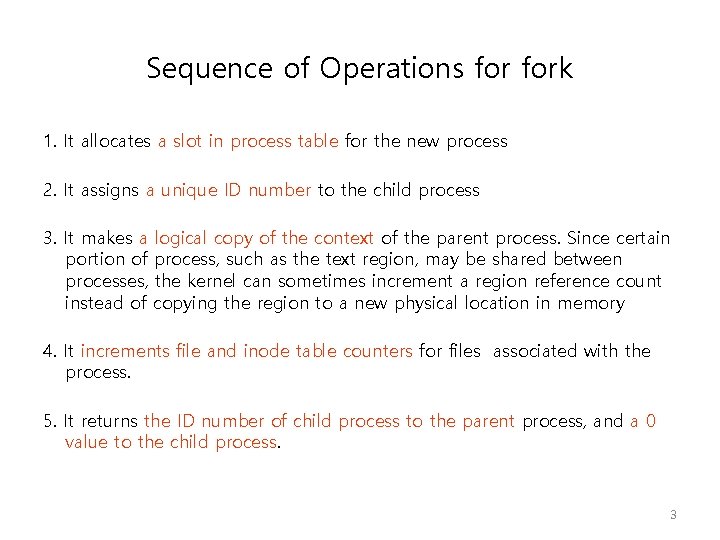
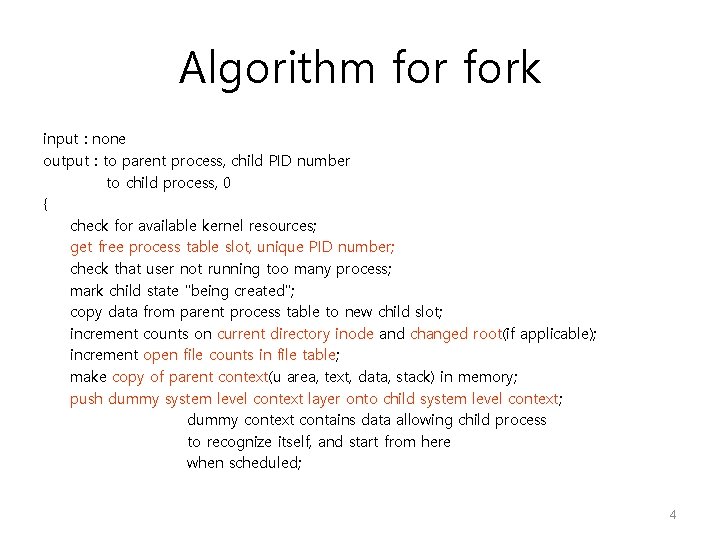
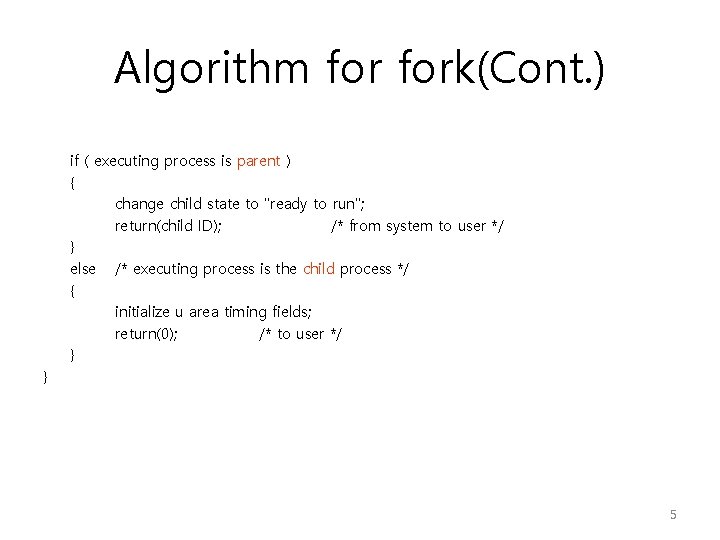
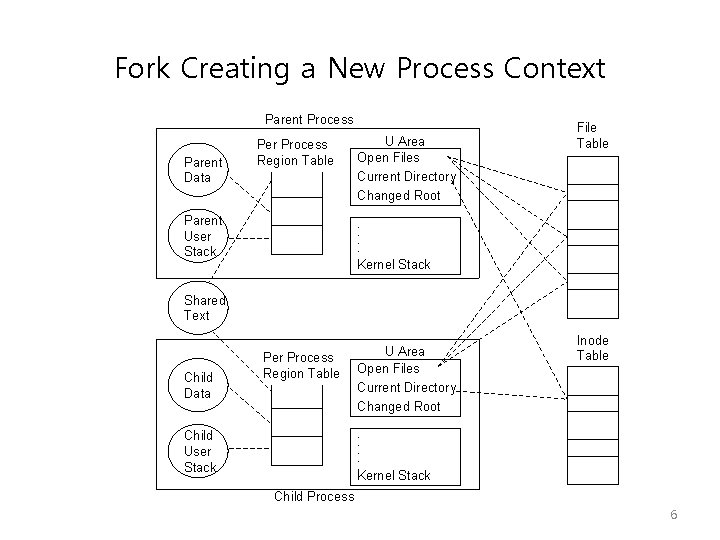
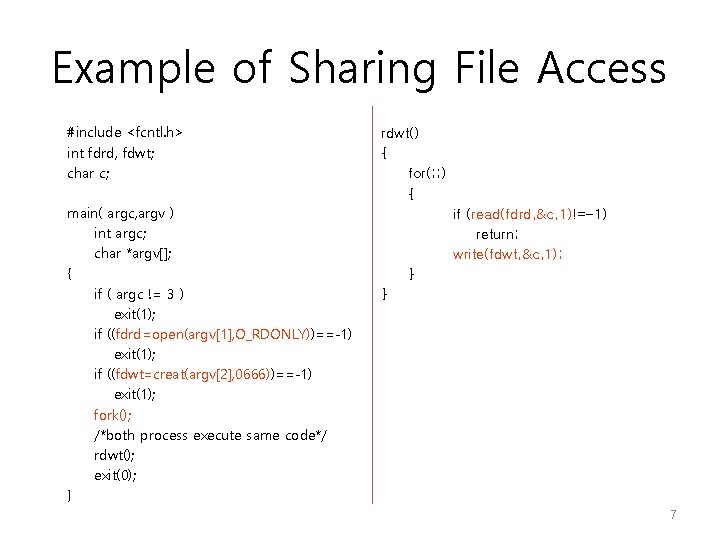
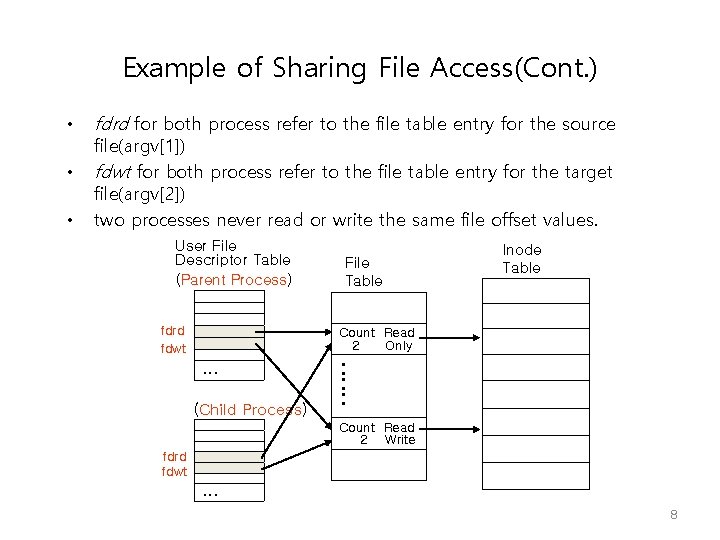
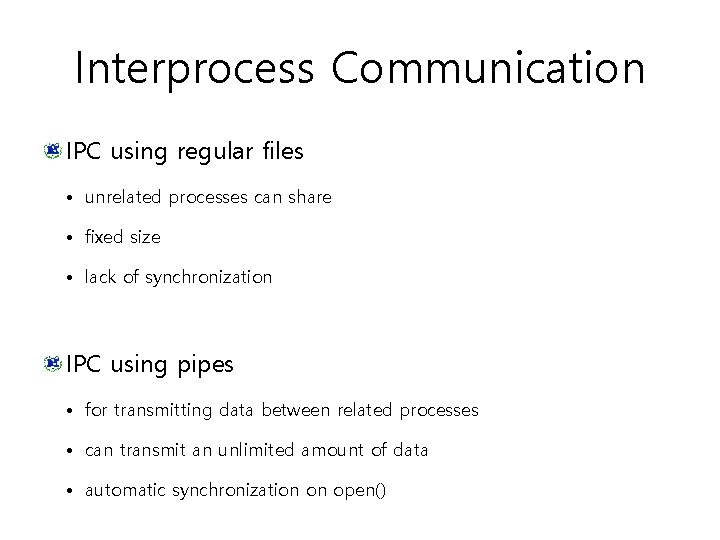
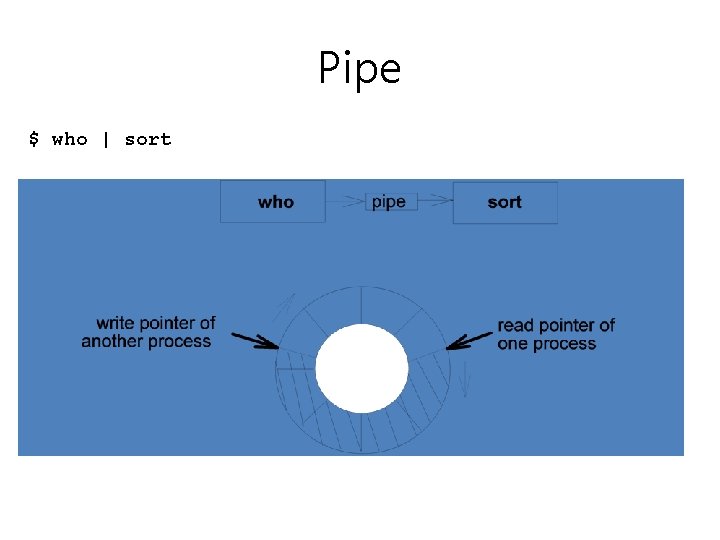
![pipes #include <unistd. h> int pipe(int fd[2]) Returns: 0 if OK, -1 on error pipes #include <unistd. h> int pipe(int fd[2]) Returns: 0 if OK, -1 on error](https://slidetodoc.com/presentation_image/3d61ca6eab26705c798aeb5ee1e2db64/image-11.jpg)
![pipe after fork user process fd[0] fd[1] parent process fd[0] child process fd[1] fd[0] pipe after fork user process fd[0] fd[1] parent process fd[0] child process fd[1] fd[0]](https://slidetodoc.com/presentation_image/3d61ca6eab26705c798aeb5ee1e2db64/image-12.jpg)
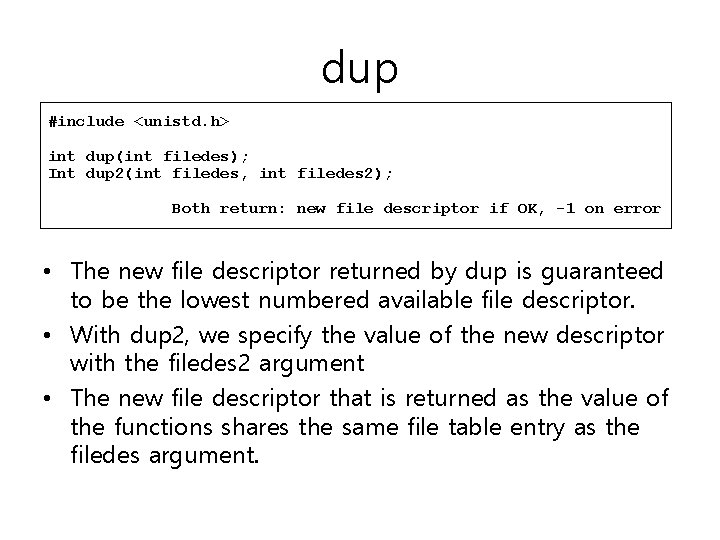
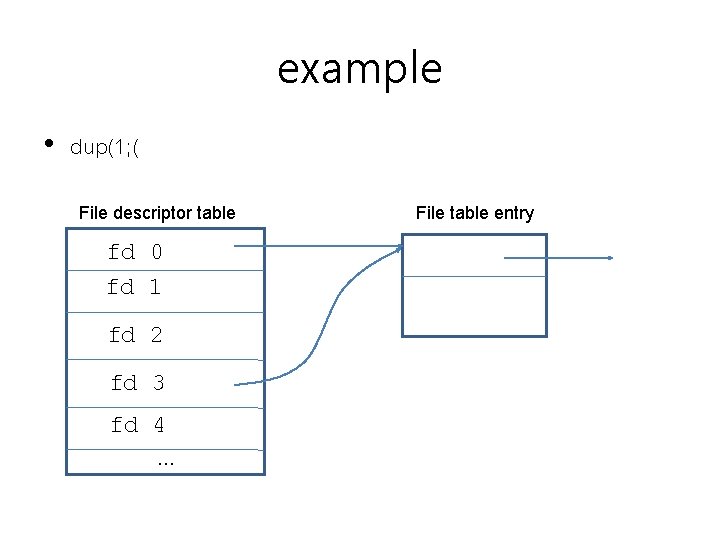
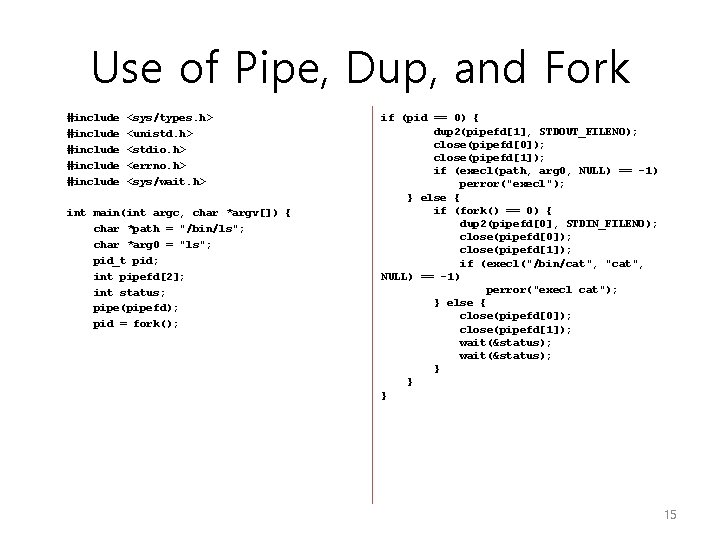
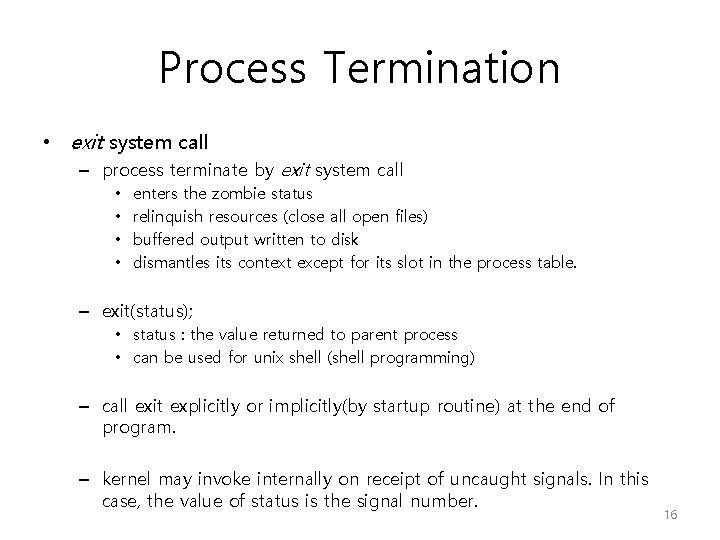
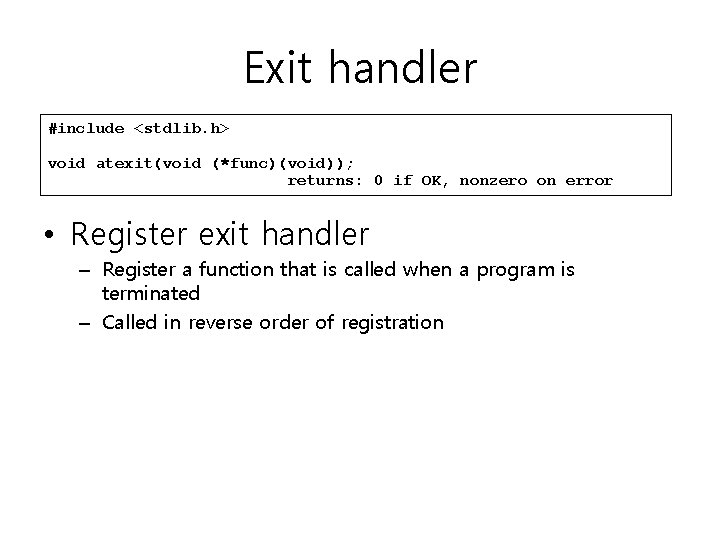
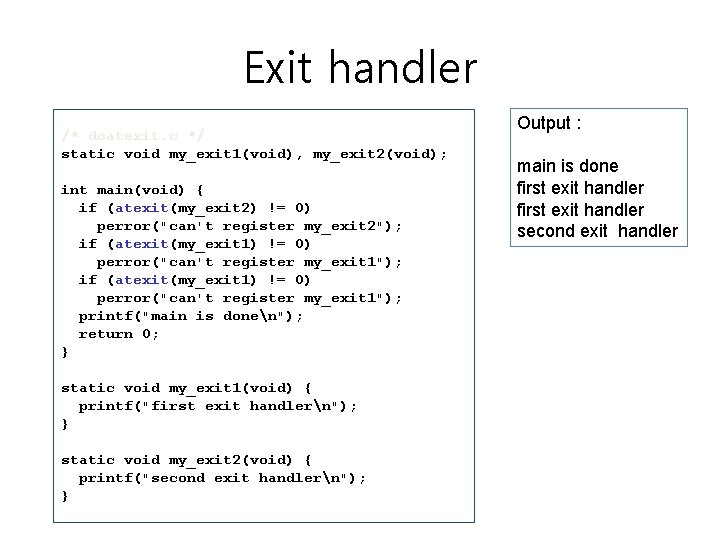
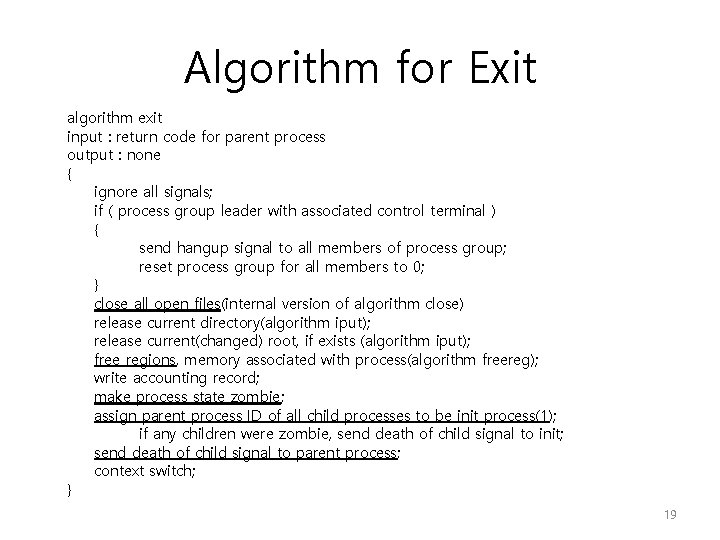
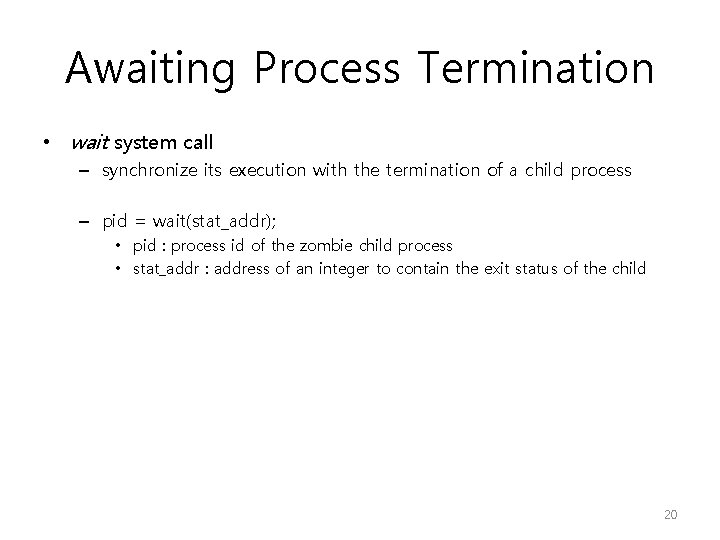
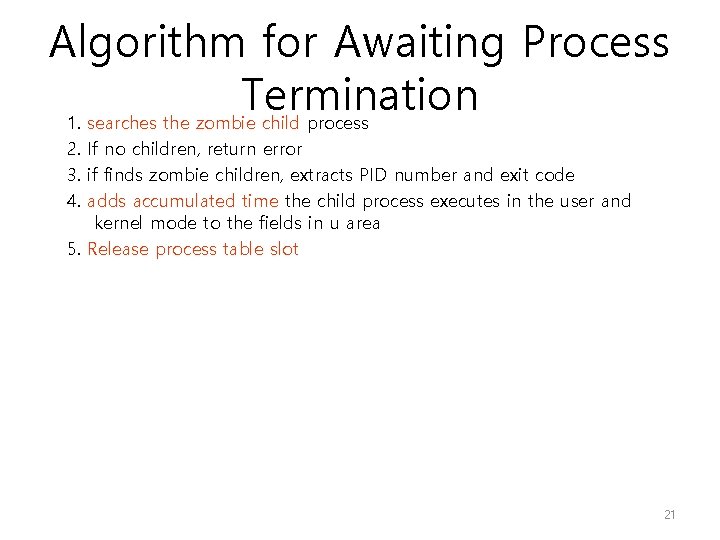
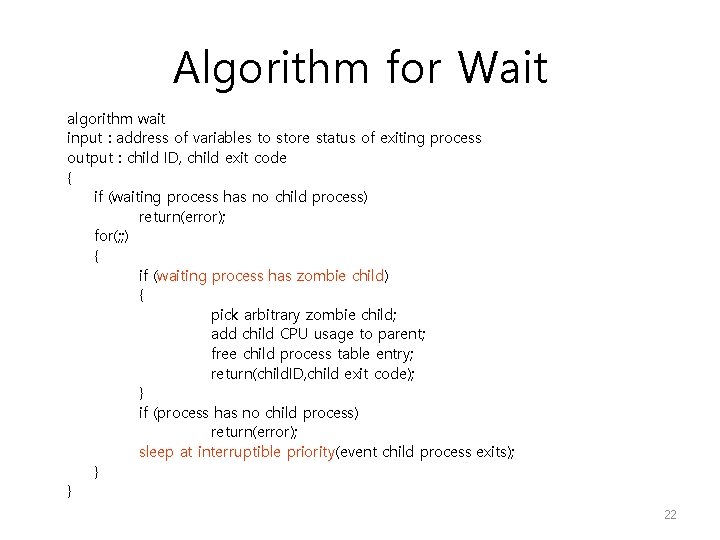
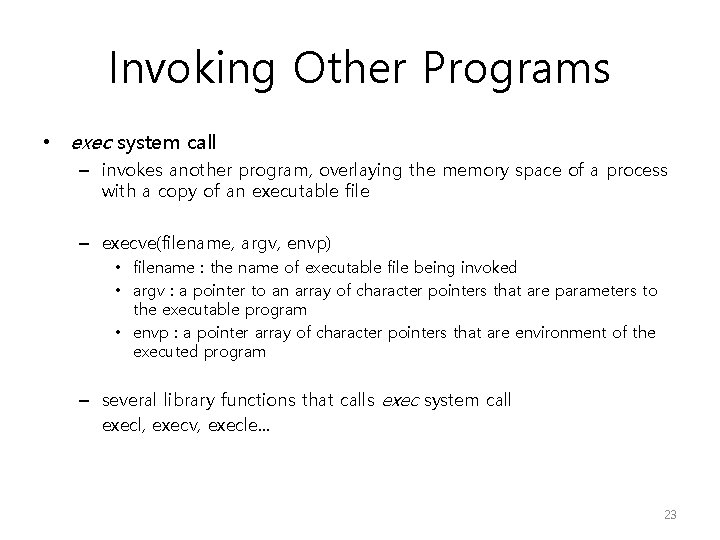
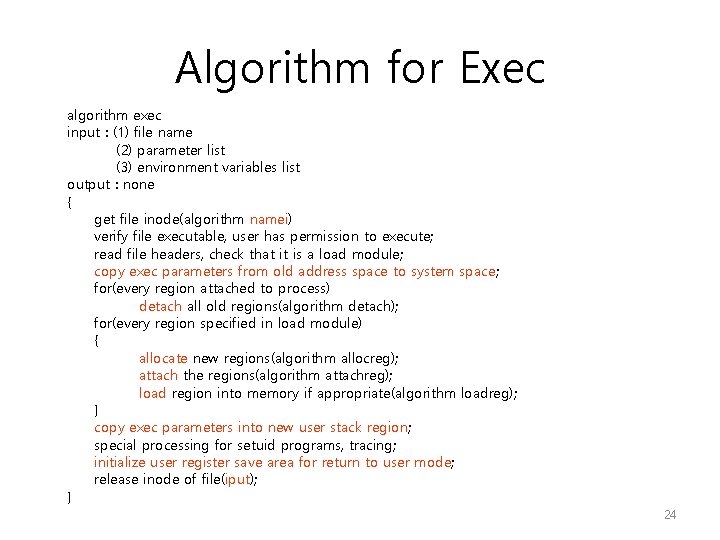
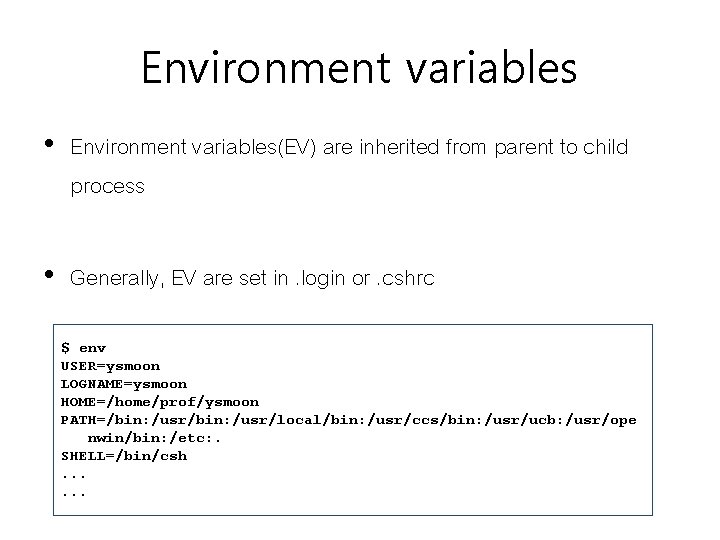
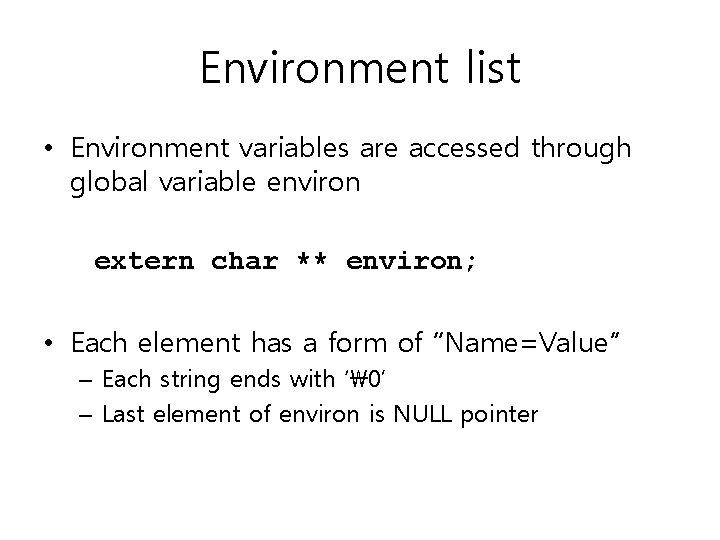
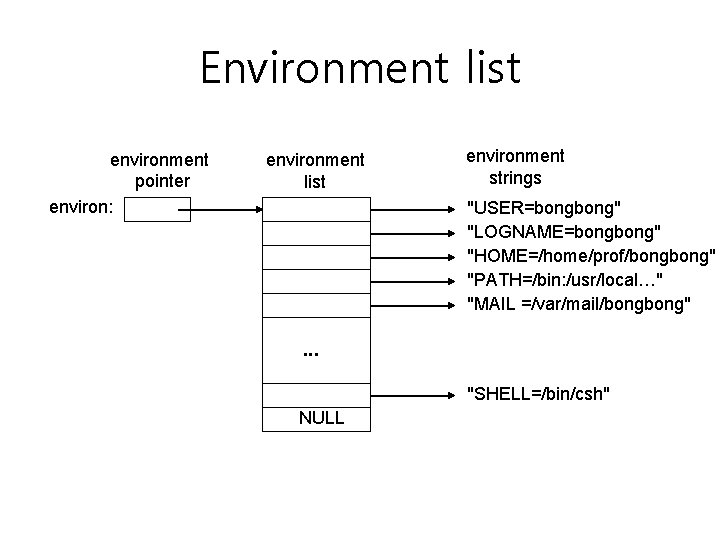
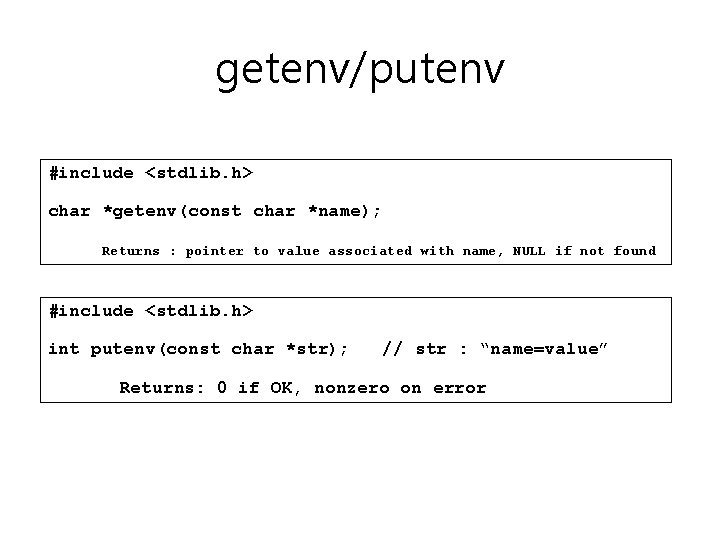
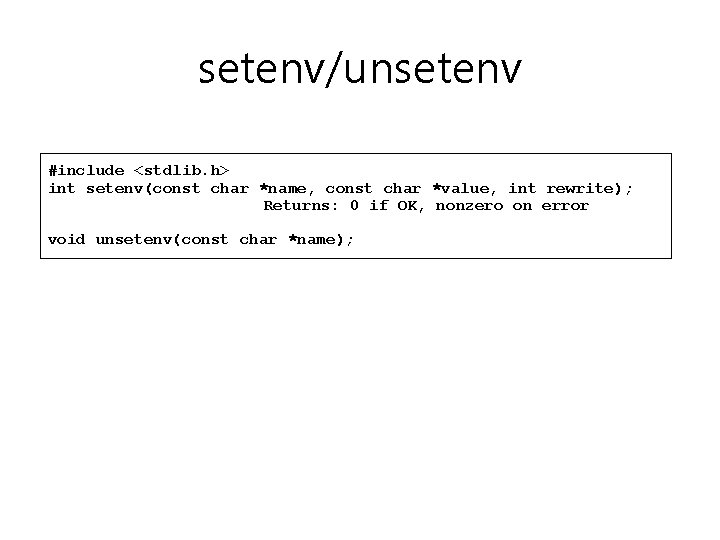
- Slides: 29
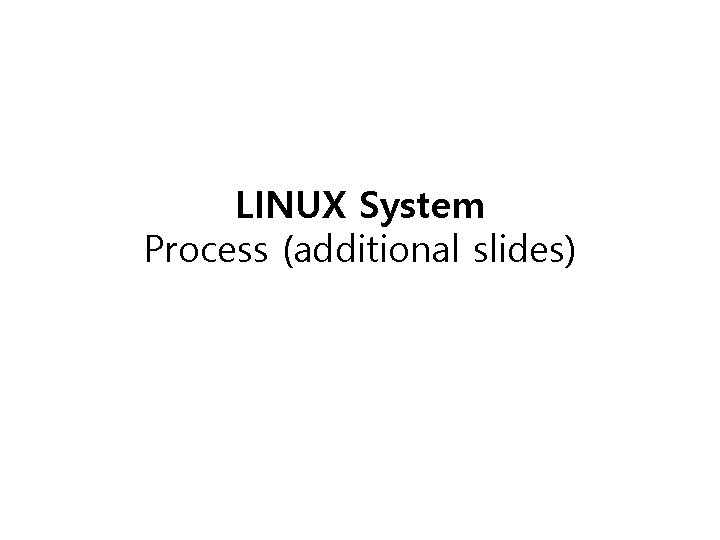
LINUX System Process (additional slides)
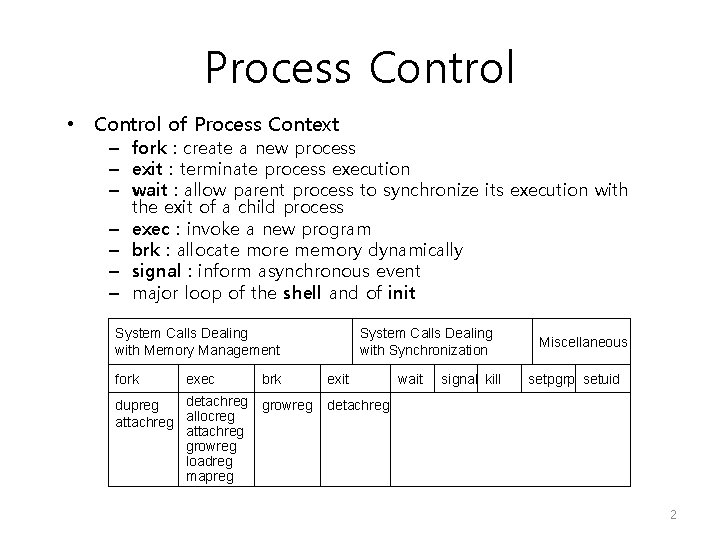
Process Control • Control of Process Context – fork : create a new process – exit : terminate process execution – wait : allow parent process to synchronize its execution with the exit of a child process – exec : invoke a new program – brk : allocate more memory dynamically – signal : inform asynchronous event – major loop of the shell and of init System Calls Dealing with Memory Management fork exec brk detachreg growreg dupreg attachreg allocreg attachreg growreg loadreg mapreg System Calls Dealing with Synchronization exit wait signal kill Miscellaneous setpgrp setuid detachreg 2
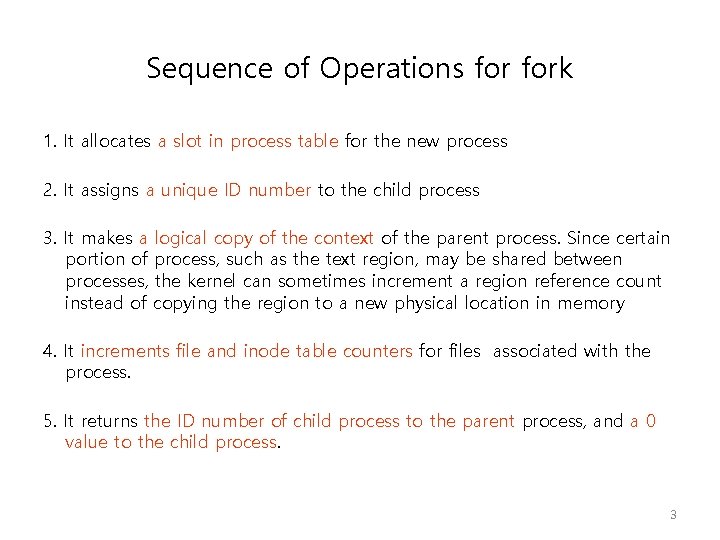
Sequence of Operations fork 1. It allocates a slot in process table for the new process 2. It assigns a unique ID number to the child process 3. It makes a logical copy of the context of the parent process. Since certain portion of process, such as the text region, may be shared between processes, the kernel can sometimes increment a region reference count instead of copying the region to a new physical location in memory 4. It increments file and inode table counters for files associated with the process. 5. It returns the ID number of child process to the parent process, and a 0 value to the child process. 3
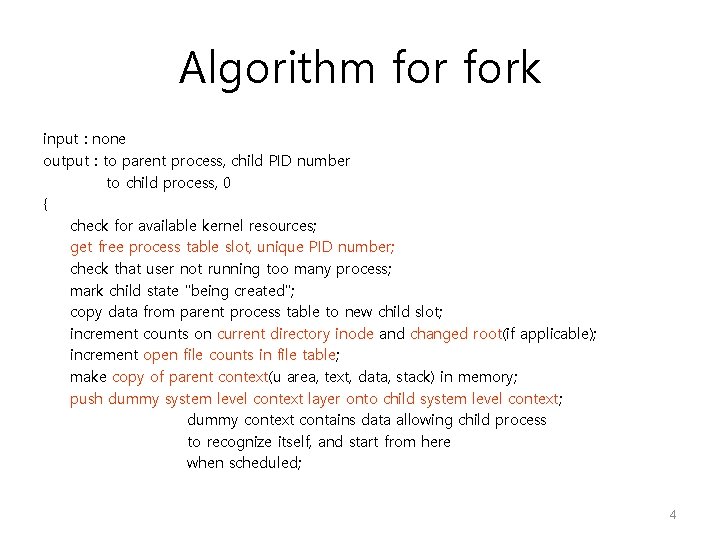
Algorithm fork input : none output : to parent process, child PID number to child process, 0 { check for available kernel resources; get free process table slot, unique PID number; check that user not running too many process; mark child state "being created"; copy data from parent process table to new child slot; increment counts on current directory inode and changed root(if applicable); increment open file counts in file table; make copy of parent context(u area, text, data, stack) in memory; push dummy system level context layer onto child system level context; dummy context contains data allowing child process to recognize itself, and start from here when scheduled; 4
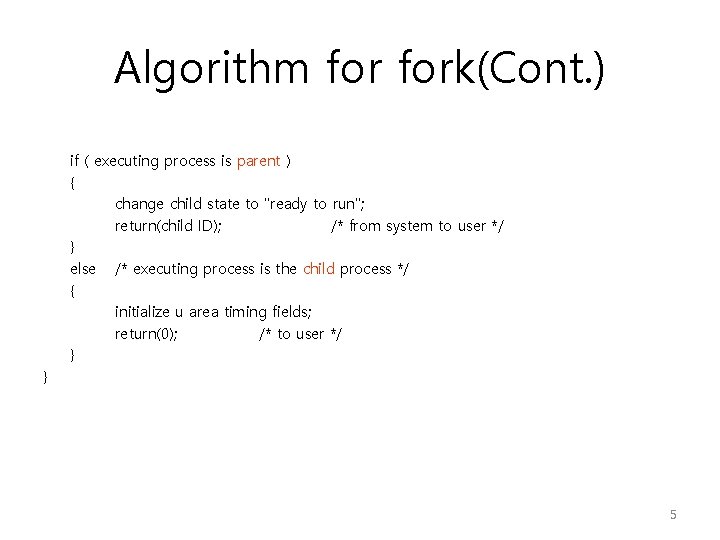
Algorithm fork(Cont. ) if ( executing process is parent ) { change child state to "ready to run"; return(child ID); /* from system to user */ } else /* executing process is the child process */ { initialize u area timing fields; return(0); /* to user */ } } 5
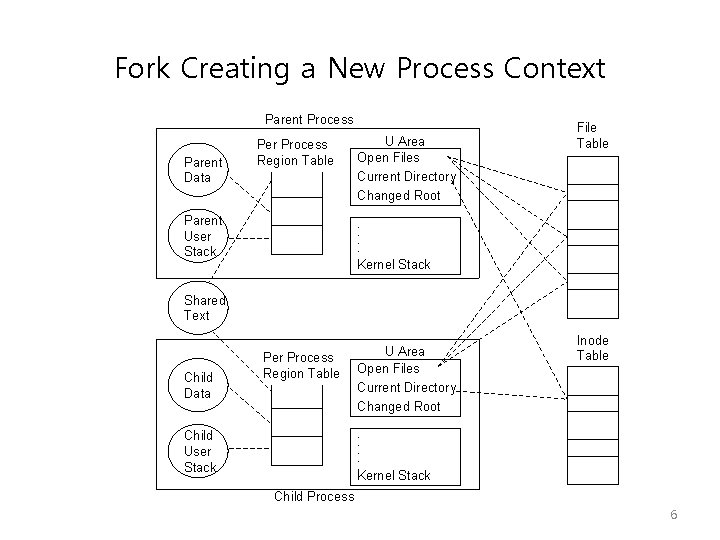
Fork Creating a New Process Context Parent Process Parent Data Per Process Region Table Parent User Stack U Area Open Files Current Directory Changed Root File Table . . . Kernel Stack Shared Text Child Data Per Process Region Table U Area Open Files Current Directory Changed Root Inode Table . . . Kernel Stack Child User Stack Child Process 6
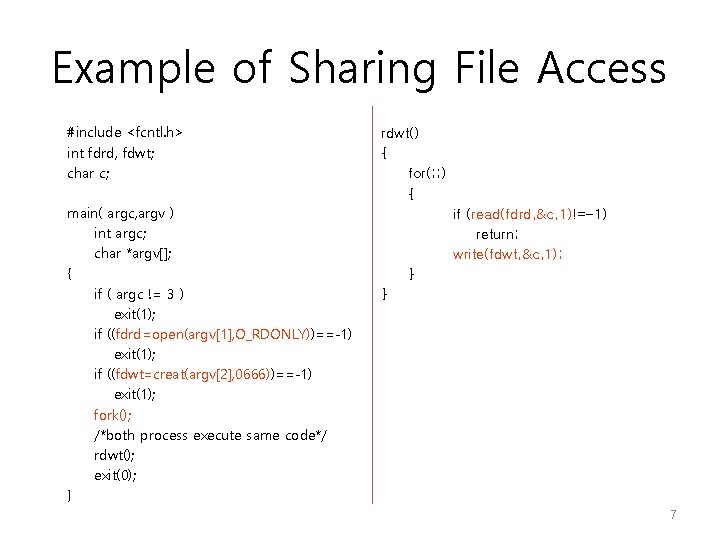
Example of Sharing File Access #include <fcntl. h> int fdrd, fdwt; char c; main( argc, argv ) int argc; char *argv[]; { if ( argc != 3 ) exit(1); if ((fdrd=open(argv[1], O_RDONLY))==-1) exit(1); if ((fdwt=creat(argv[2], 0666))==-1) exit(1); fork(); /*both process execute same code*/ rdwt(); exit(0); } rdwt() { for(; ; ) { if (read(fdrd, &c, 1)!=-1) return; write(fdwt, &c, 1); } } 7
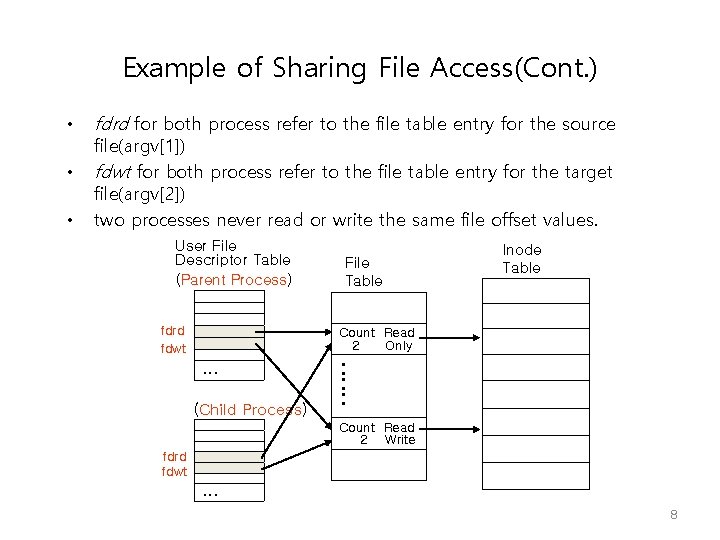
Example of Sharing File Access(Cont. ) • • • fdrd for both process refer to the file table entry for the source file(argv[1]) fdwt for both process refer to the file table entry for the target file(argv[2]) two processes never read or write the same file offset values. User File Descriptor Table (Parent Process) fdrd fdwt File Table Inode Table Count Read 2 Only . . . (Child Process) : : : Count Read 2 Write fdrd fdwt . . . 8
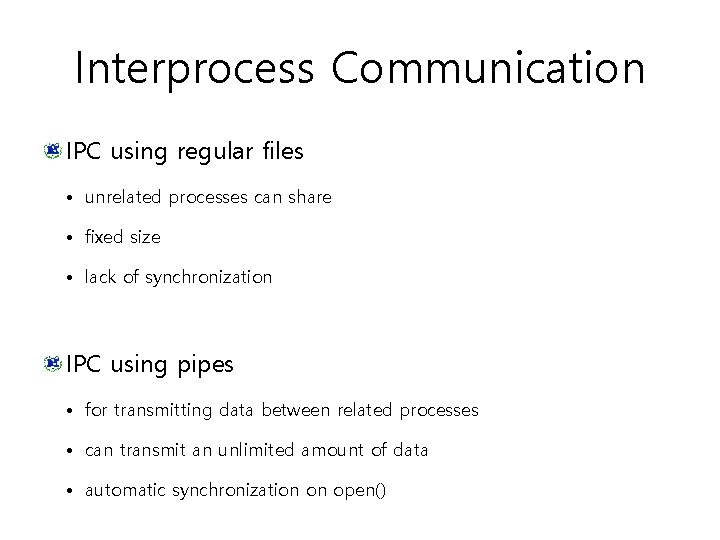
Interprocess Communication IPC using regular files • unrelated processes can share • fixed size • lack of synchronization IPC using pipes • for transmitting data between related processes • can transmit an unlimited amount of data • automatic synchronization on open()
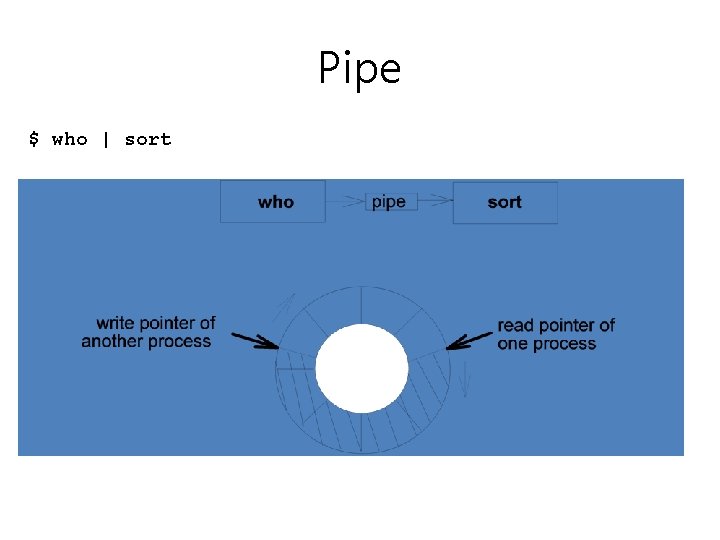
Pipe $ who | sort
![pipes include unistd h int pipeint fd2 Returns 0 if OK 1 on error pipes #include <unistd. h> int pipe(int fd[2]) Returns: 0 if OK, -1 on error](https://slidetodoc.com/presentation_image/3d61ca6eab26705c798aeb5ee1e2db64/image-11.jpg)
pipes #include <unistd. h> int pipe(int fd[2]) Returns: 0 if OK, -1 on error Two file descriptors are returned through the fd argument • fd[0]: can be used to read from the pipe, and • fd[1]: can be used to write to the pipe Anything that is written on fd[1] may be read by fd[0]. • This is of no use in a single process. • However, between processes, it gives a method of communication The pipe() system call gives parent-child processes a way to communicate with each other.
![pipe after fork user process fd0 fd1 parent process fd0 child process fd1 fd0 pipe after fork user process fd[0] fd[1] parent process fd[0] child process fd[1] fd[0]](https://slidetodoc.com/presentation_image/3d61ca6eab26705c798aeb5ee1e2db64/image-12.jpg)
pipe after fork user process fd[0] fd[1] parent process fd[0] child process fd[1] fd[0] pipe kernel fd[1]
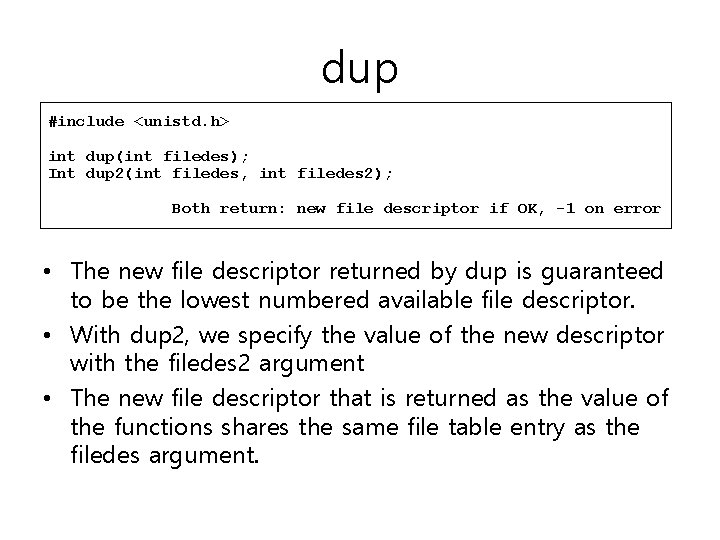
dup #include <unistd. h> int dup(int filedes); Int dup 2(int filedes, int filedes 2); Both return: new file descriptor if OK, -1 on error • The new file descriptor returned by dup is guaranteed to be the lowest numbered available file descriptor. • With dup 2, we specify the value of the new descriptor with the filedes 2 argument • The new file descriptor that is returned as the value of the functions shares the same file table entry as the filedes argument.
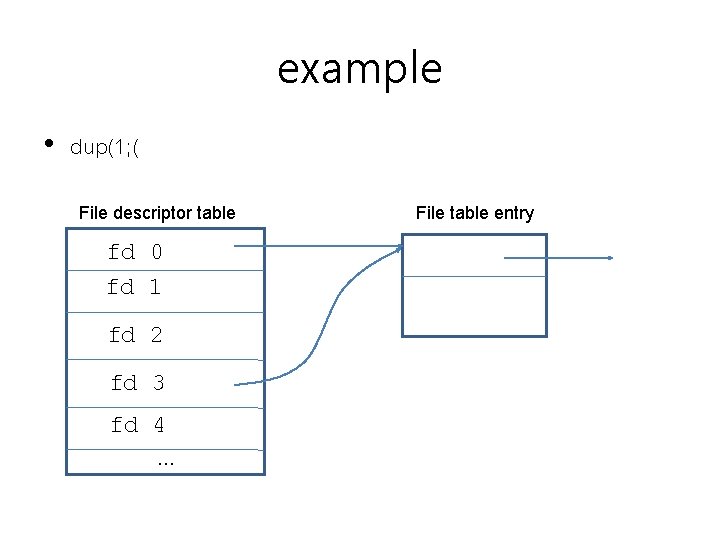
example • dup(1; ( File descriptor table fd 0 fd 1 fd 2 fd 3 fd 4 … File table entry
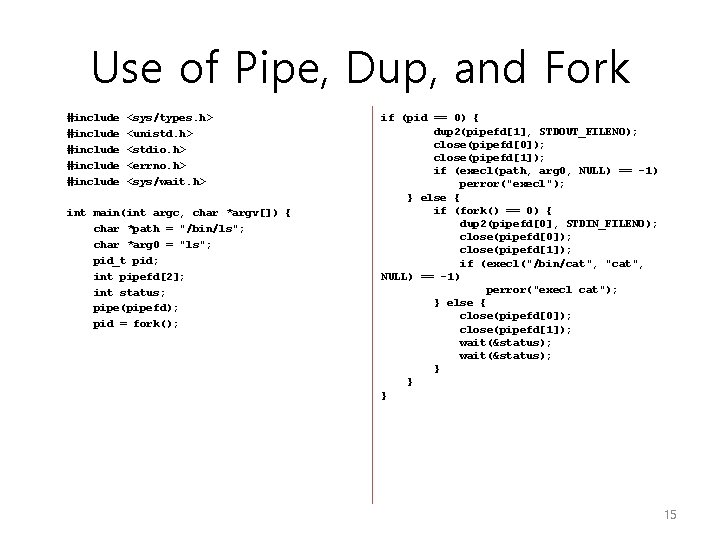
Use of Pipe, Dup, and Fork #include #include <sys/types. h> <unistd. h> <stdio. h> <errno. h> <sys/wait. h> int main(int argc, char *argv[]) { char *path = "/bin/ls"; char *arg 0 = "ls"; pid_t pid; int pipefd[2]; int status; pipe(pipefd); pid = fork(); if (pid == 0) { dup 2(pipefd[1], STDOUT_FILENO); close(pipefd[0]); close(pipefd[1]); if (execl(path, arg 0, NULL) == -1) perror("execl"); } else { if (fork() == 0) { dup 2(pipefd[0], STDIN_FILENO); close(pipefd[0]); close(pipefd[1]); if (execl("/bin/cat", "cat", NULL) == -1) perror("execl cat"); } else { close(pipefd[0]); close(pipefd[1]); wait(&status); } } } 15
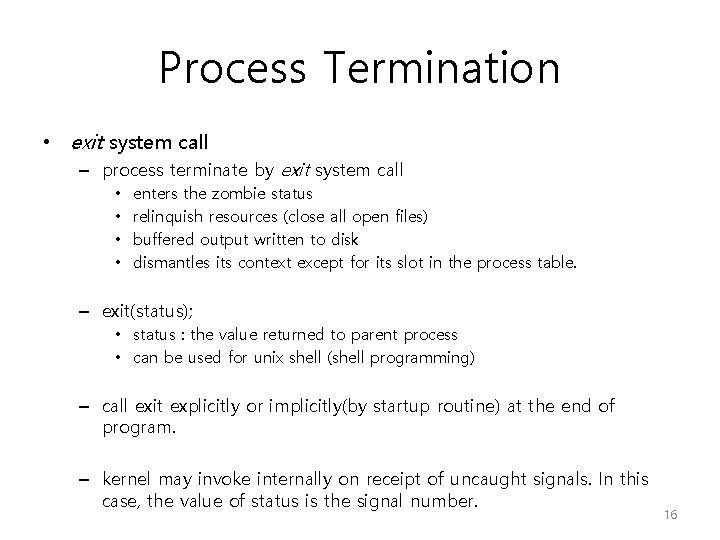
Process Termination • exit system call – process terminate by exit system call • • enters the zombie status relinquish resources (close all open files) buffered output written to disk dismantles its context except for its slot in the process table. – exit(status); • status : the value returned to parent process • can be used for unix shell (shell programming) – call exit explicitly or implicitly(by startup routine) at the end of program. – kernel may invoke internally on receipt of uncaught signals. In this case, the value of status is the signal number. 16
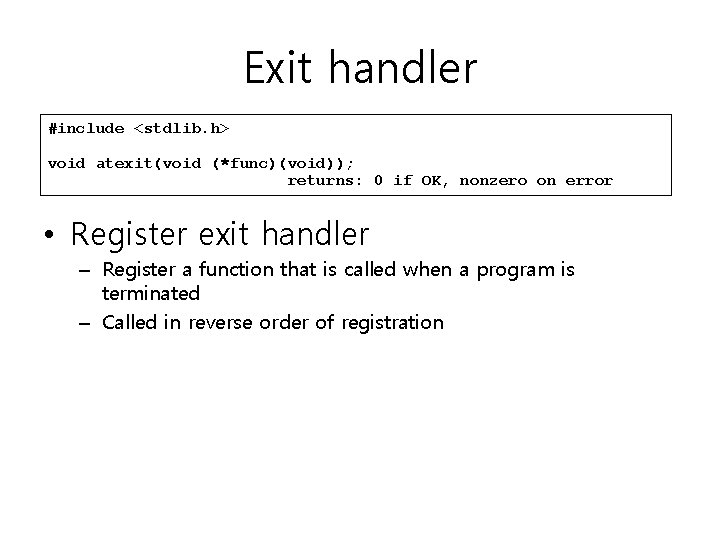
Exit handler #include <stdlib. h> void atexit(void (*func)(void)); returns: 0 if OK, nonzero on error • Register exit handler – Register a function that is called when a program is terminated – Called in reverse order of registration
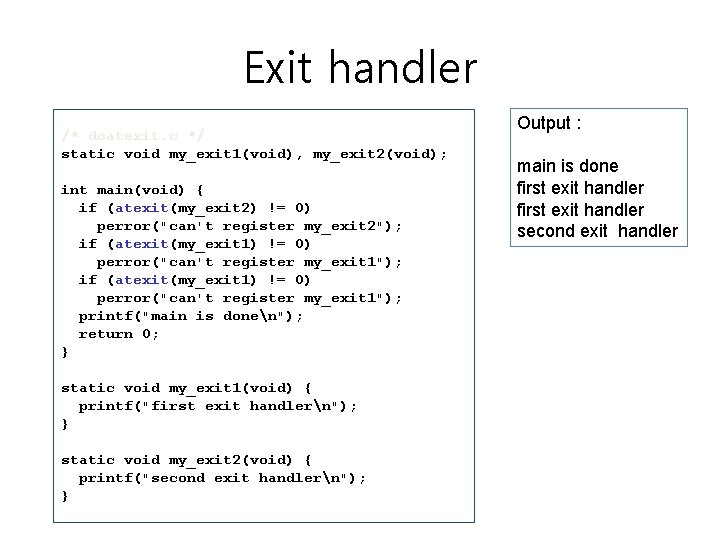
Exit handler /* doatexit. c */ static void my_exit 1(void), my_exit 2(void); int main(void) { if (atexit(my_exit 2) != 0) perror("can't register my_exit 2"); if (atexit(my_exit 1) != 0) perror("can't register my_exit 1"); printf("main is donen"); return 0; } static void my_exit 1(void) { printf("first exit handlern"); } static void my_exit 2(void) { printf("second exit handlern"); } Output : main is done first exit handler second exit handler
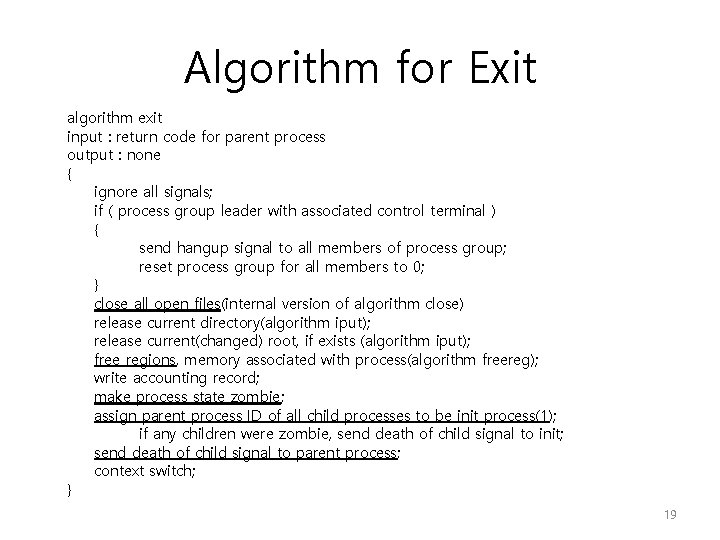
Algorithm for Exit algorithm exit input : return code for parent process output : none { ignore all signals; if ( process group leader with associated control terminal ) { send hangup signal to all members of process group; reset process group for all members to 0; } close all open files(internal version of algorithm close) release current directory(algorithm iput); release current(changed) root, if exists (algorithm iput); free regions, memory associated with process(algorithm freereg); write accounting record; make process state zombie; assign parent process ID of all child processes to be init process(1); if any children were zombie, send death of child signal to init; send death of child signal to parent process; context switch; } 19
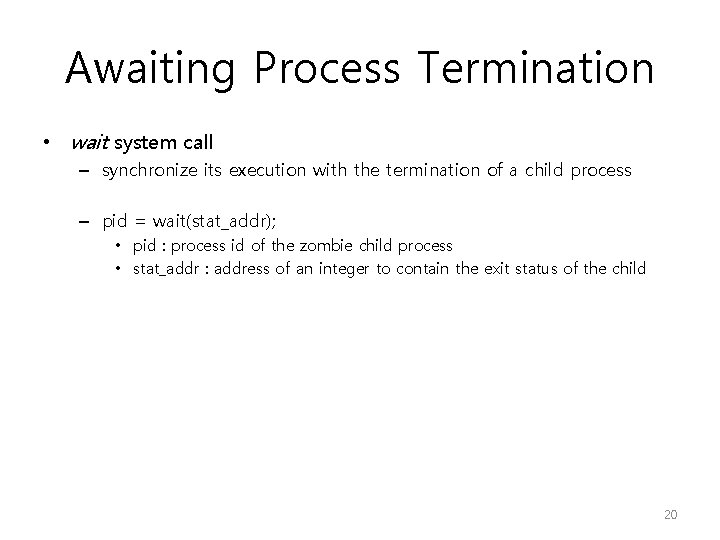
Awaiting Process Termination • wait system call – synchronize its execution with the termination of a child process – pid = wait(stat_addr); • pid : process id of the zombie child process • stat_addr : address of an integer to contain the exit status of the child 20
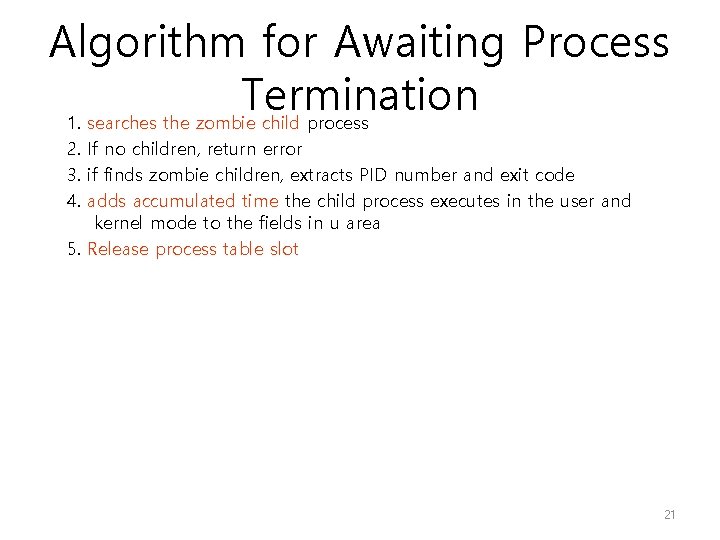
Algorithm for Awaiting Process Termination 1. searches the zombie child process 2. If no children, return error 3. if finds zombie children, extracts PID number and exit code 4. adds accumulated time the child process executes in the user and kernel mode to the fields in u area 5. Release process table slot 21
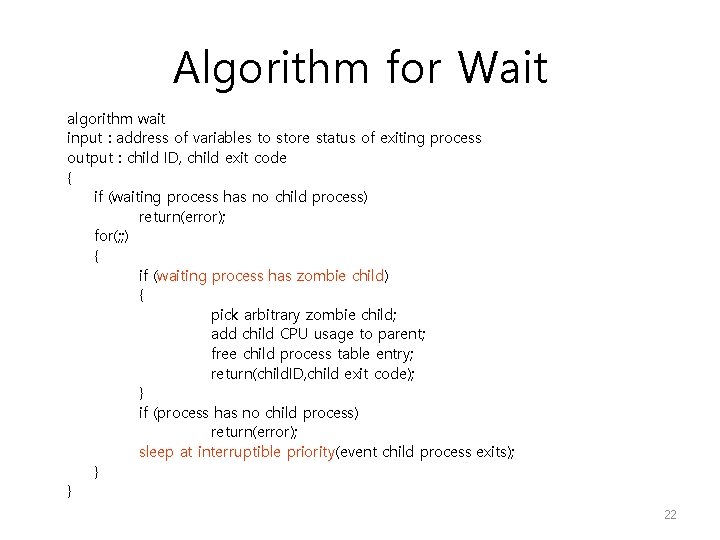
Algorithm for Wait algorithm wait input : address of variables to store status of exiting process output : child ID, child exit code { if (waiting process has no child process) return(error); for(; ; ) { if (waiting process has zombie child) { pick arbitrary zombie child; add child CPU usage to parent; free child process table entry; return(child. ID, child exit code); } if (process has no child process) return(error); sleep at interruptible priority(event child process exits); } } 22
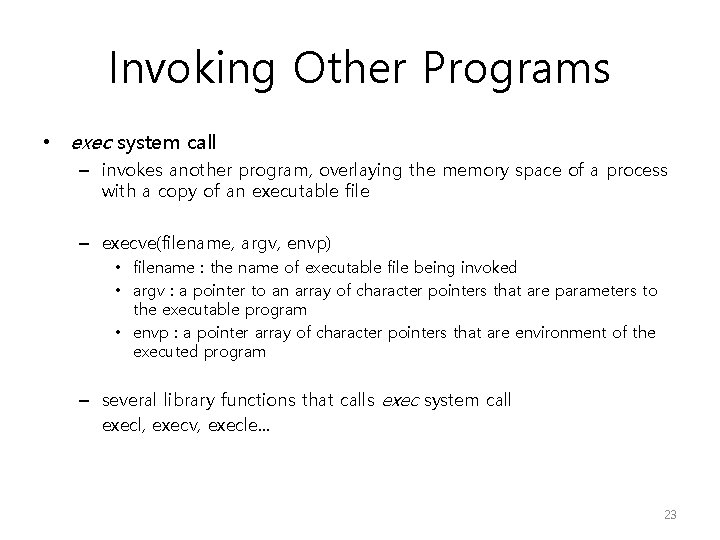
Invoking Other Programs • exec system call – invokes another program, overlaying the memory space of a process with a copy of an executable file – execve(filename, argv, envp) • filename : the name of executable file being invoked • argv : a pointer to an array of character pointers that are parameters to the executable program • envp : a pointer array of character pointers that are environment of the executed program – several library functions that calls exec system call execl, execv, execle. . . 23
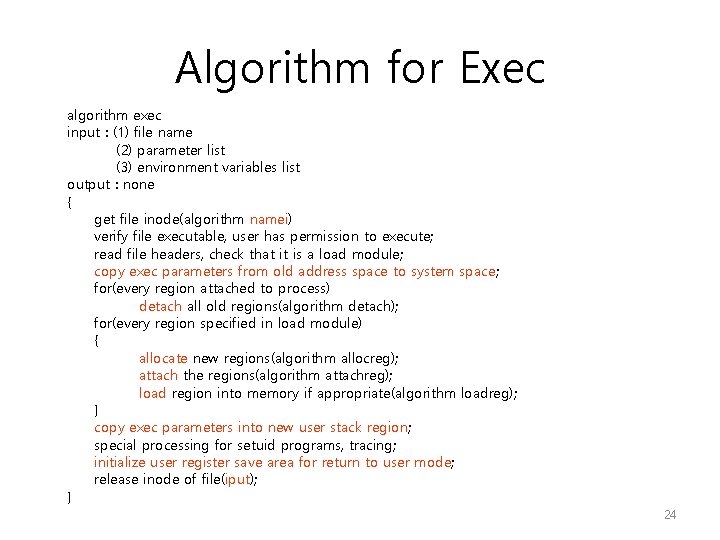
Algorithm for Exec algorithm exec input : (1) file name (2) parameter list (3) environment variables list output : none { get file inode(algorithm namei) verify file executable, user has permission to execute; read file headers, check that it is a load module; copy exec parameters from old address space to system space; for(every region attached to process) detach all old regions(algorithm detach); for(every region specified in load module) { allocate new regions(algorithm allocreg); attach the regions(algorithm attachreg); load region into memory if appropriate(algorithm loadreg); } copy exec parameters into new user stack region; special processing for setuid programs, tracing; initialize user register save area for return to user mode; release inode of file(iput); } 24
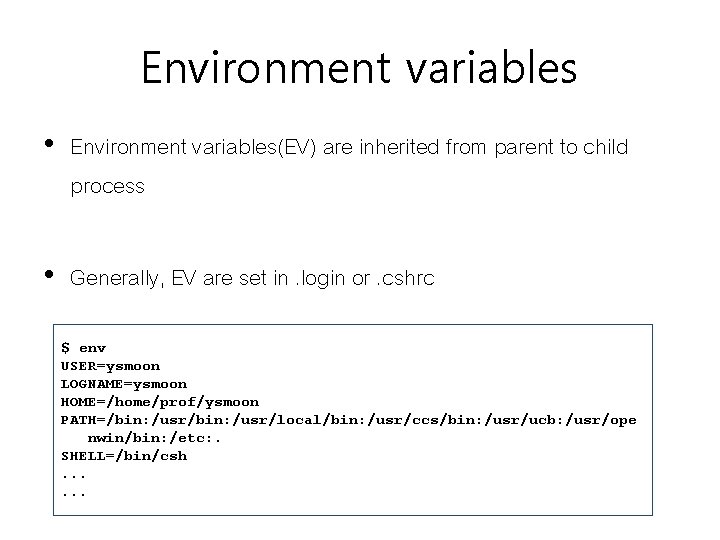
Environment variables • Environment variables(EV) are inherited from parent to child process • Generally, EV are set in. login or. cshrc $ env USER=ysmoon LOGNAME=ysmoon HOME=/home/prof/ysmoon PATH=/bin: /usr/local/bin: /usr/ccs/bin: /usr/ucb: /usr/ope nwin/bin: /etc: . SHELL=/bin/csh. . .
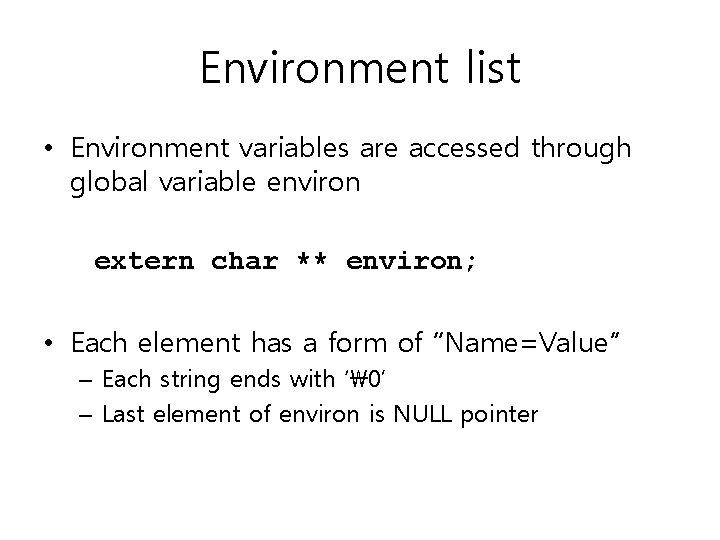
Environment list • Environment variables are accessed through global variable environ extern char ** environ; • Each element has a form of “Name=Value” – Each string ends with ‘ ’ – Last element of environ is NULL pointer
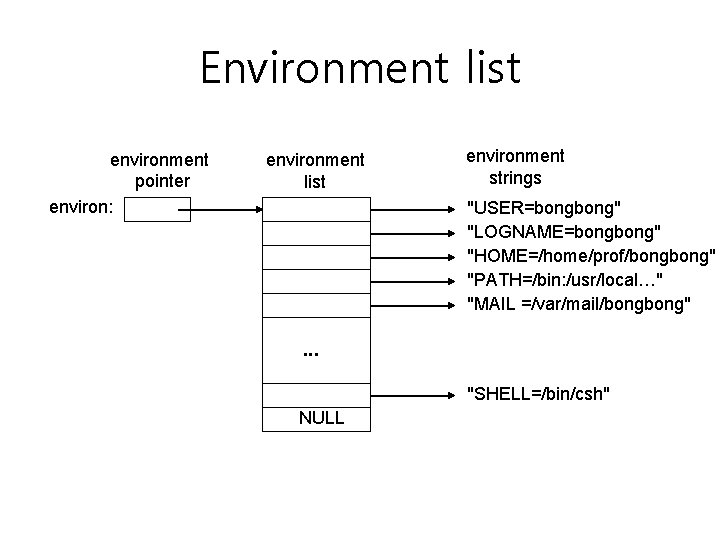
Environment list environment pointer environ: environment list environment strings "USER=bong" "LOGNAME=bong" "HOME=/home/prof/bong" "PATH=/bin: /usr/local…" "MAIL =/var/mail/bong" . . . "SHELL=/bin/csh" NULL
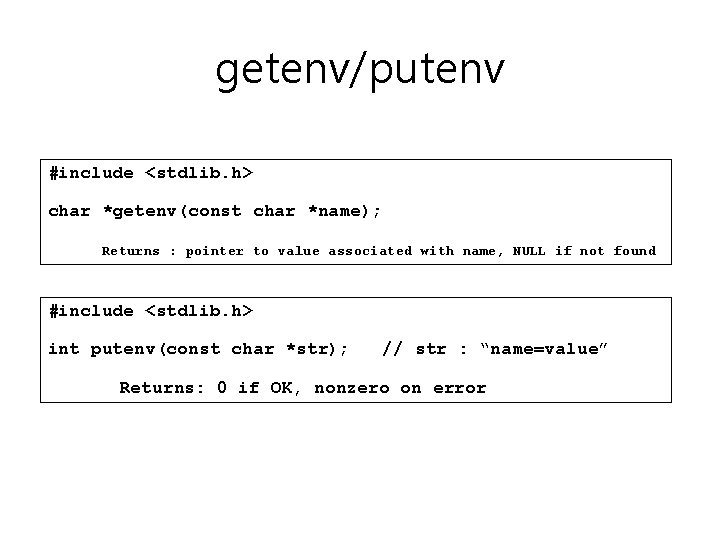
getenv/putenv #include <stdlib. h> char *getenv(const char *name); Returns : pointer to value associated with name, NULL if not found #include <stdlib. h> int putenv(const char *str); // str : “name=value” Returns: 0 if OK, nonzero on error
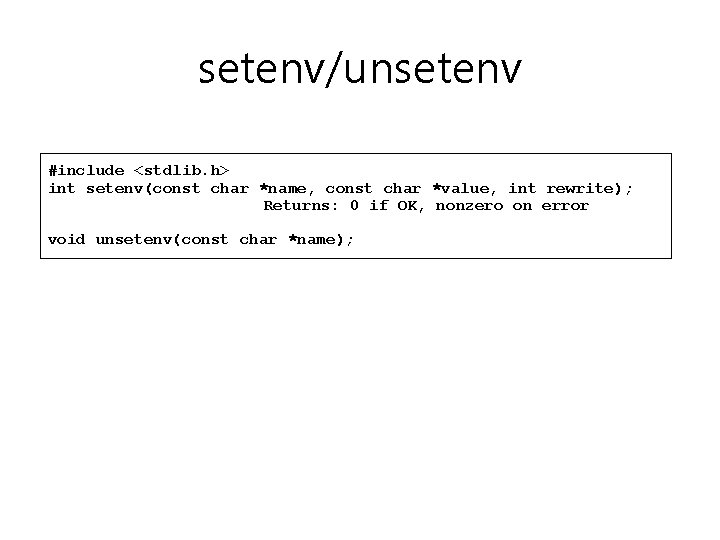
setenv/unsetenv #include <stdlib. h> int setenv(const char *name, const char *value, int rewrite); Returns: 0 if OK, nonzero on error void unsetenv(const char *name);
General security
Guilots
A small child slides down the four frictionless slides
John pushes hector on a plastic toboggan
Pcb kernel
Sculpture is created by shaping or combining the materials
Additional process
Additional member system scotland
Fptp pros and cons
Fire watchers are additional personnel
Supporting individuals with additional needs
Presenting yourself on the uc application
Objectives of school health programme
Ipo chart
Additional aspects of aqueous equilibria
Additional aspects of aqueous equilibria
10-5 additional practice secant lines and segments
Bridge of adams clasp is fabricated on what angulation
Activity 15 quadrilaterals and their properties
Surface area of pyramids and cones
Space figures and cross sections
Writing a letter asking for information
Additional roles reimbursements scheme
6-1 additional practice the polygon angle-sum theorems
School health additional referral program
Additional support for learning act 2004
Attach additional responsibilities to an object dynamically
Database system ppt
5-3 additional vocabulary support bisectors in triangles
5-1 perpendicular and angle bisectors worksheet