Lec 7 The dowhile Statement Syntax do statements
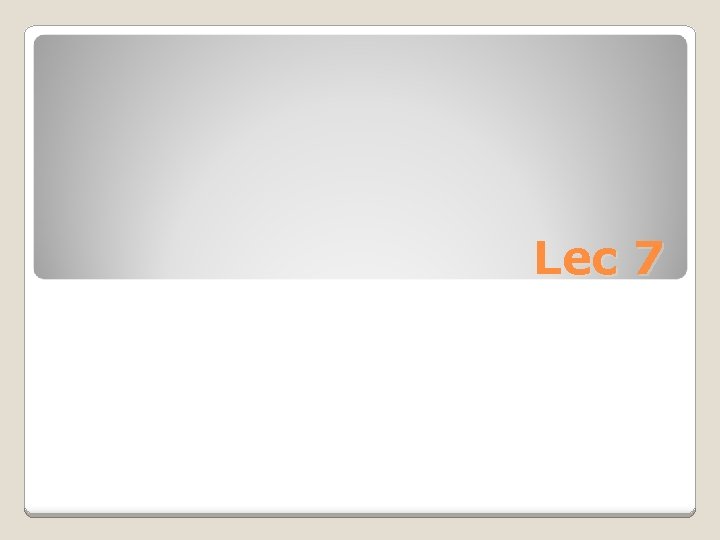
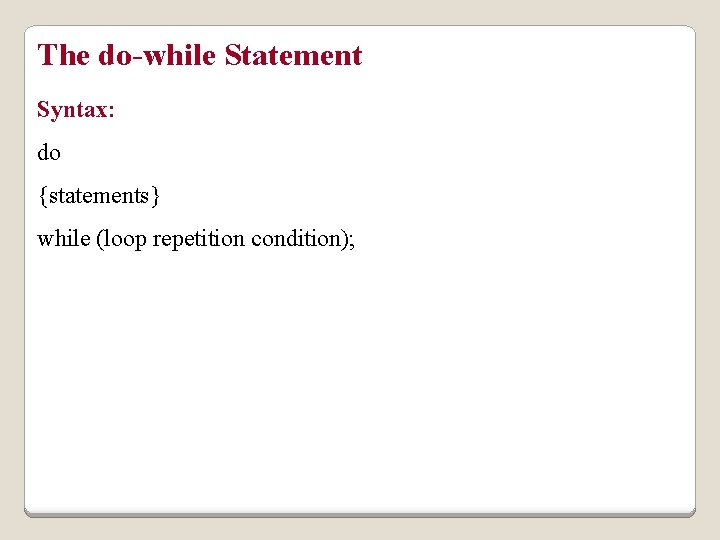
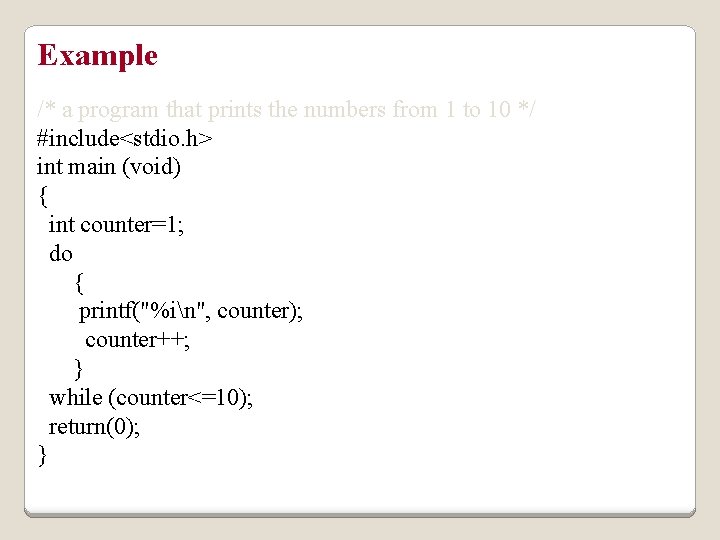
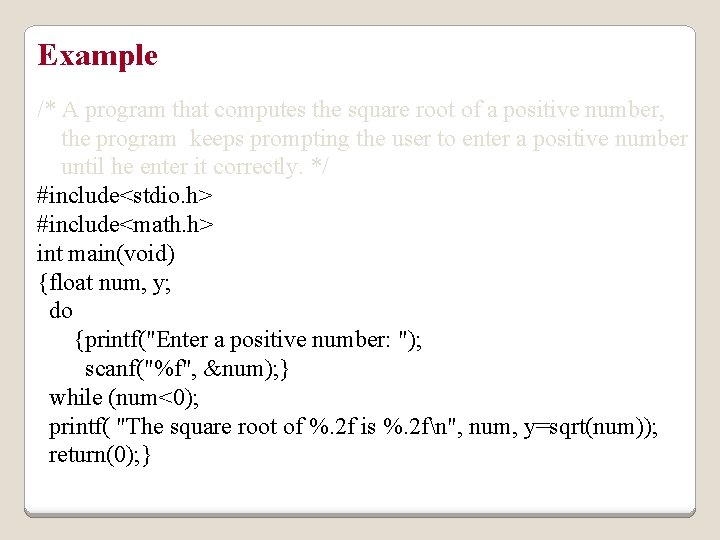
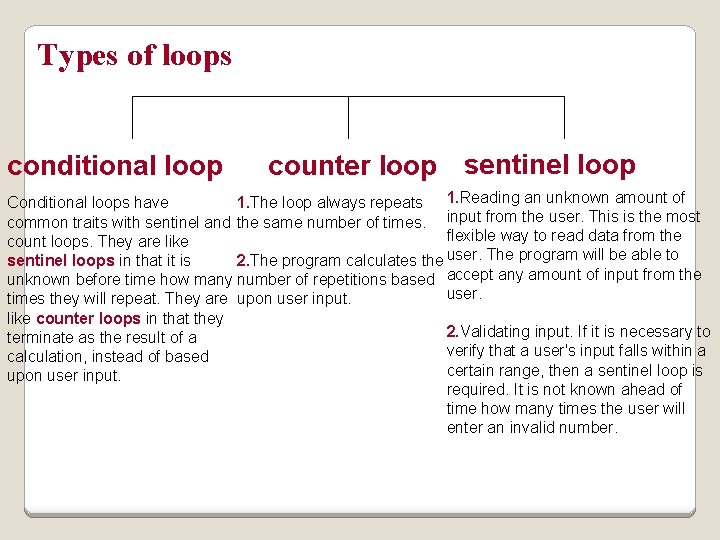
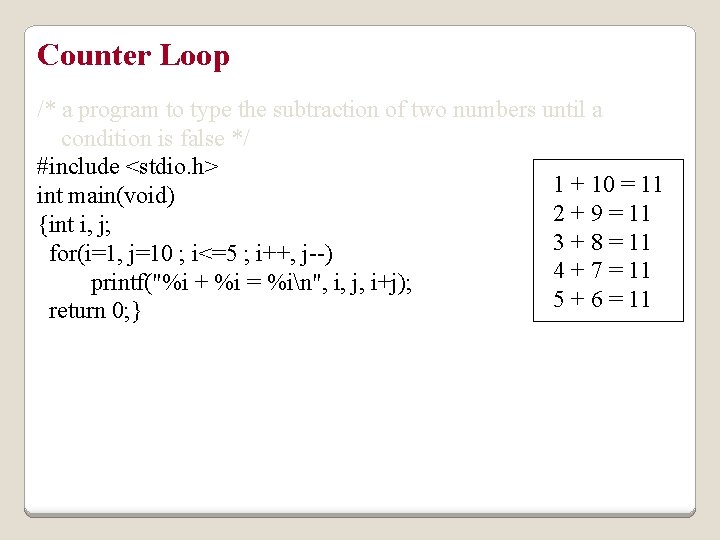
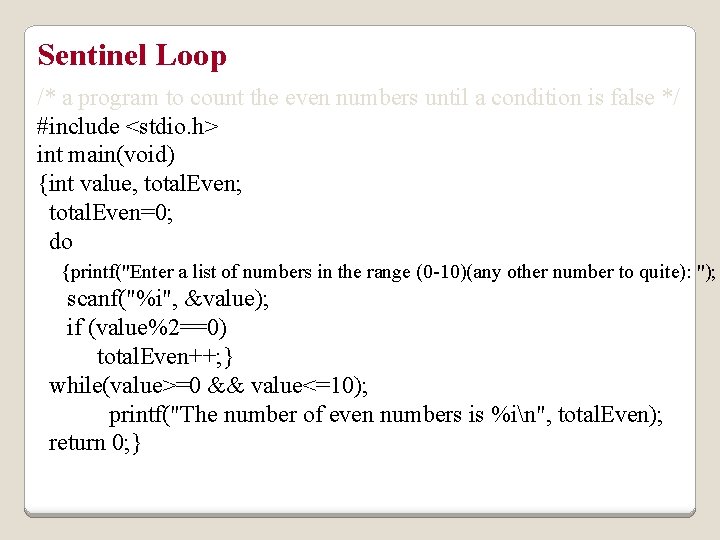
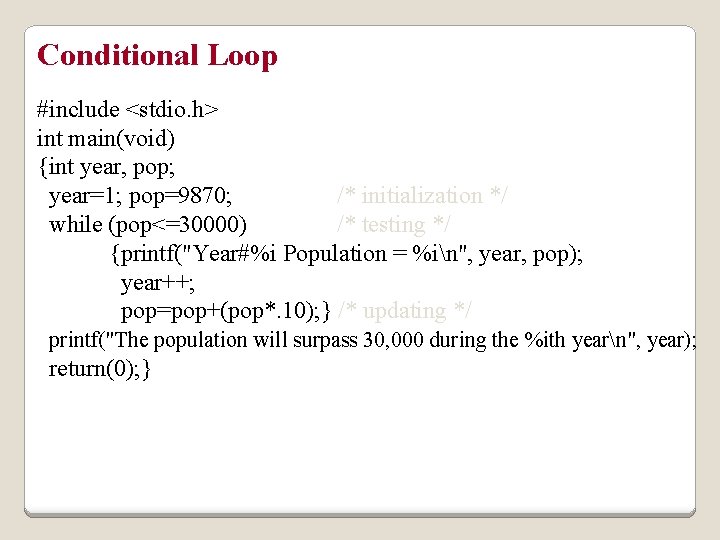
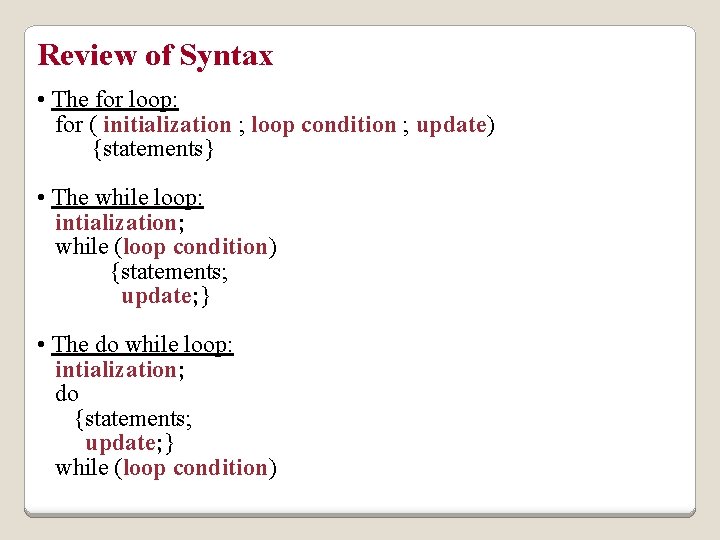
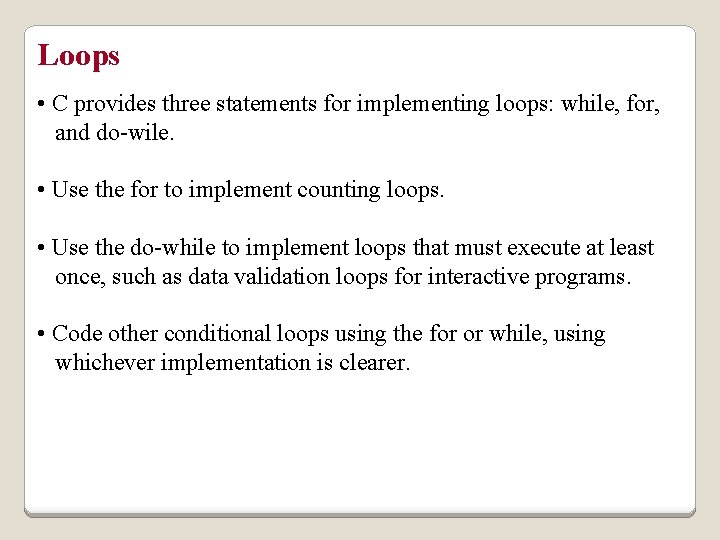
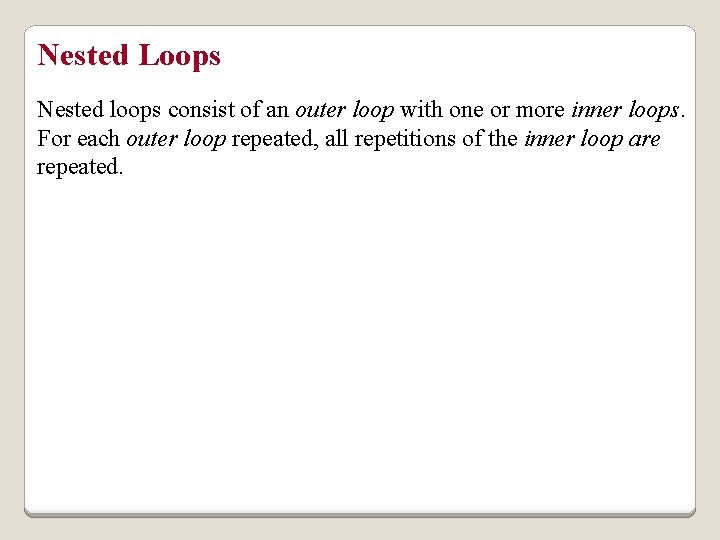
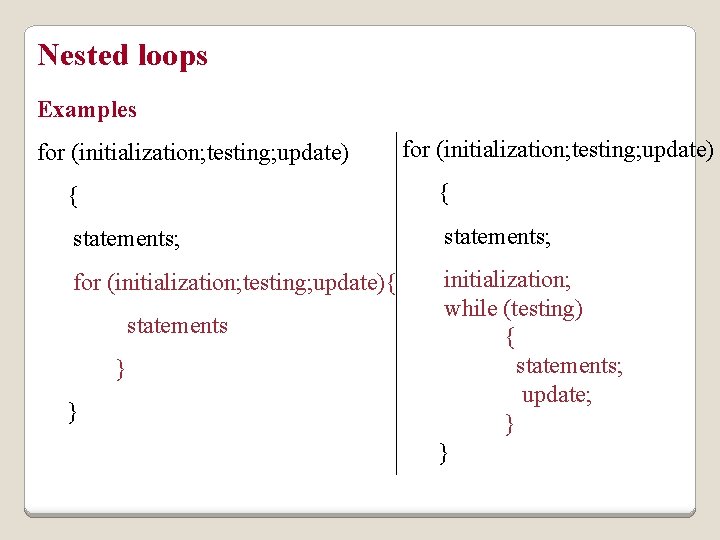
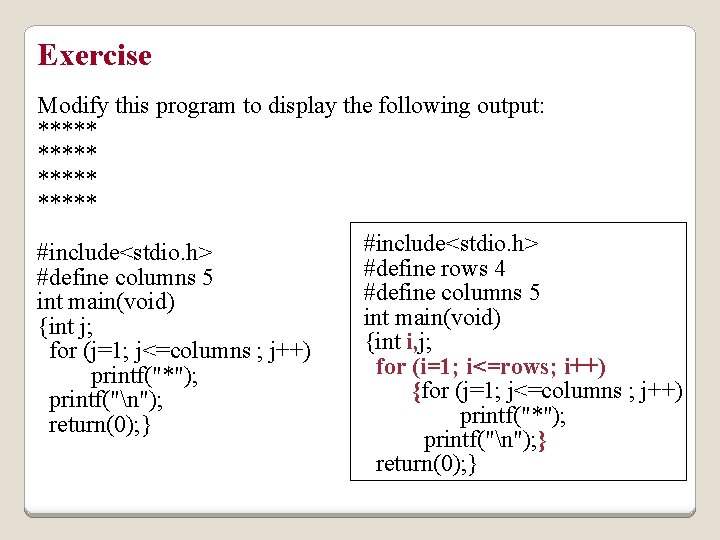
- Slides: 13
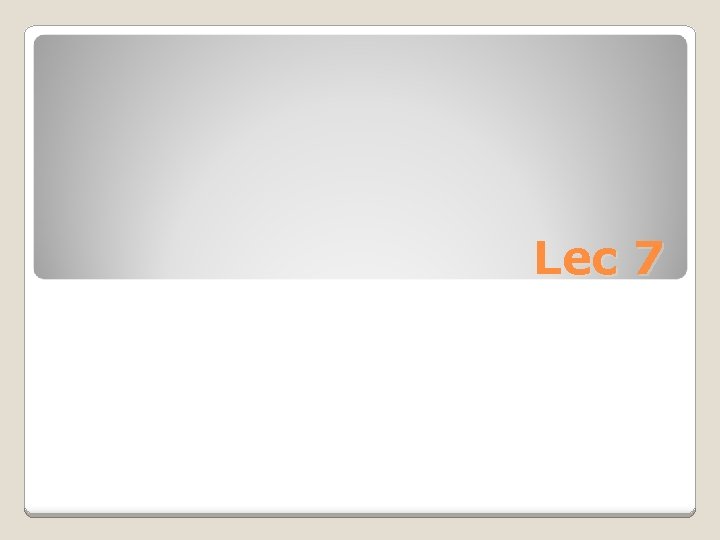
Lec 7
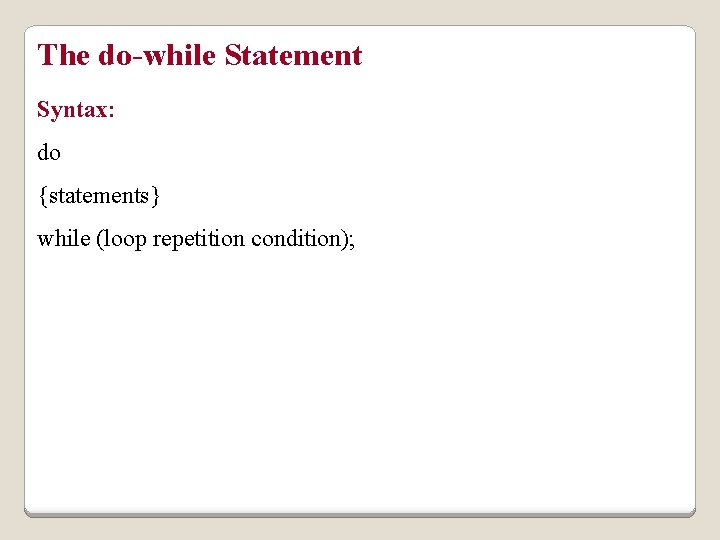
The do-while Statement Syntax: do {statements} while (loop repetition condition);
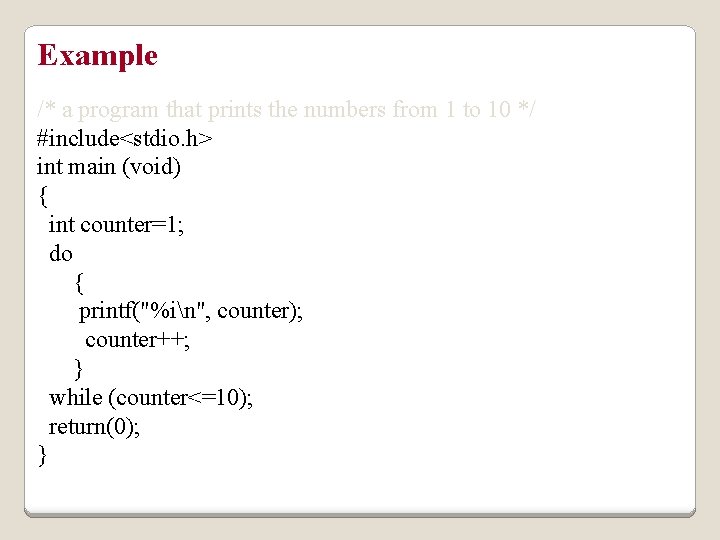
Example /* a program that prints the numbers from 1 to 10 */ #include<stdio. h> int main (void) { int counter=1; do { printf("%in", counter); counter++; } while (counter<=10); return(0); }
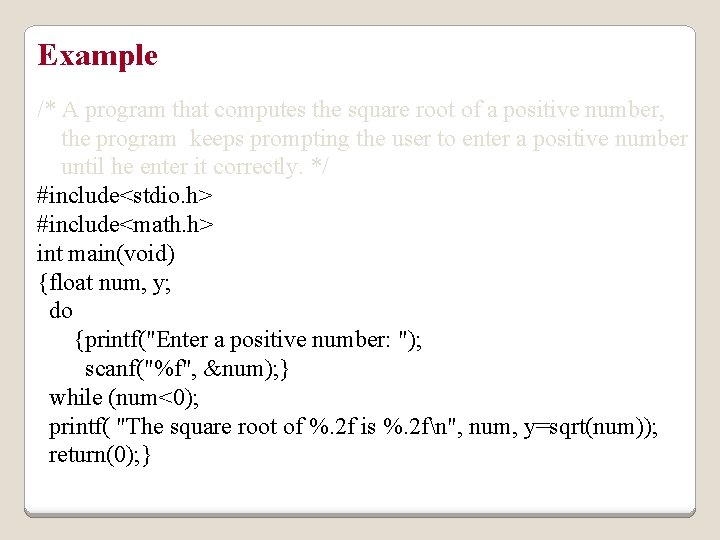
Example /* A program that computes the square root of a positive number, the program keeps prompting the user to enter a positive number until he enter it correctly. */ #include<stdio. h> #include<math. h> int main(void) {float num, y; do {printf("Enter a positive number: "); scanf("%f", &num); } while (num<0); printf( "The square root of %. 2 f is %. 2 fn", num, y=sqrt(num)); return(0); }
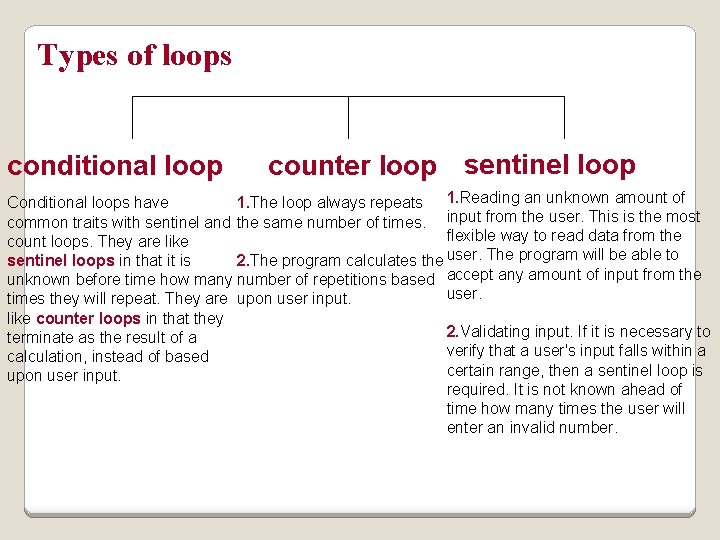
Types of loops conditional loop counter loop sentinel loop Conditional loops have 1. The loop always repeats 1. Reading an unknown amount of common traits with sentinel and the same number of times. input from the user. This is the most flexible way to read data from the count loops. They are like sentinel loops in that it is 2. The program calculates the user. The program will be able to unknown before time how many number of repetitions based accept any amount of input from the user. times they will repeat. They are upon user input. like counter loops in that they 2. Validating input. If it is necessary to terminate as the result of a verify that a user's input falls within a calculation, instead of based certain range, then a sentinel loop is upon user input. required. It is not known ahead of time how many times the user will enter an invalid number.
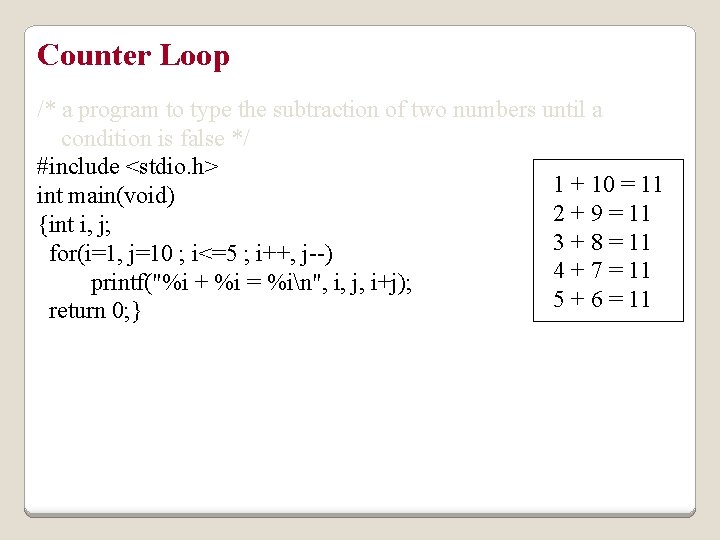
Counter Loop /* a program to type the subtraction of two numbers until a condition is false */ #include <stdio. h> 1 + 10 = 11 int main(void) 2 + 9 = 11 {int i, j; 3 + 8 = 11 for(i=1, j=10 ; i<=5 ; i++, j--) 4 + 7 = 11 printf("%i + %i = %in", i, j, i+j); 5 + 6 = 11 return 0; }
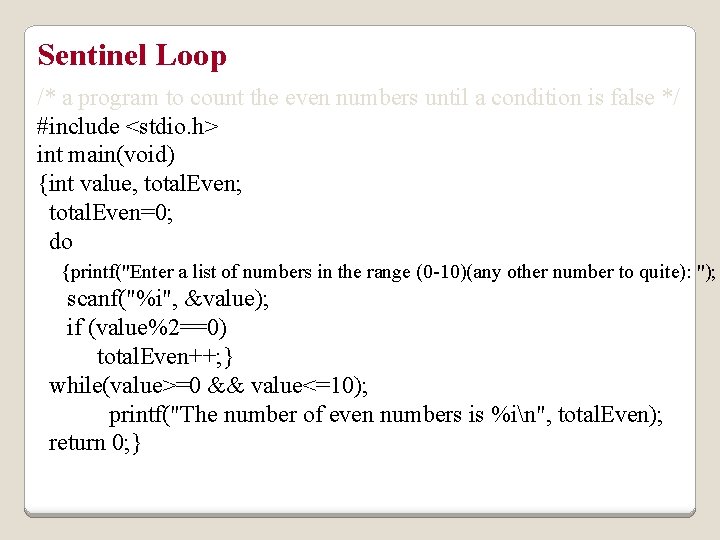
Sentinel Loop /* a program to count the even numbers until a condition is false */ #include <stdio. h> int main(void) {int value, total. Even; total. Even=0; do {printf("Enter a list of numbers in the range (0 -10)(any other number to quite): "); scanf("%i", &value); if (value%2==0) total. Even++; } while(value>=0 && value<=10); printf("The number of even numbers is %in", total. Even); return 0; }
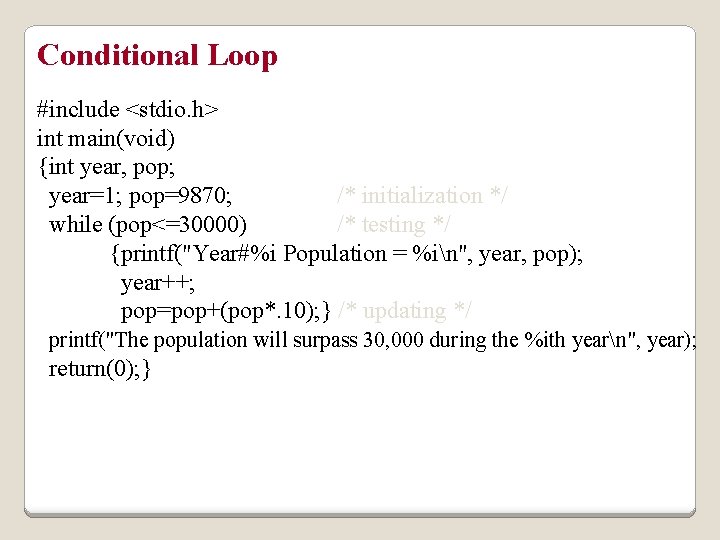
Conditional Loop #include <stdio. h> int main(void) {int year, pop; year=1; pop=9870; /* initialization */ while (pop<=30000) /* testing */ {printf("Year#%i Population = %in", year, pop); year++; pop=pop+(pop*. 10); } /* updating */ printf("The population will surpass 30, 000 during the %ith yearn", year); return(0); }
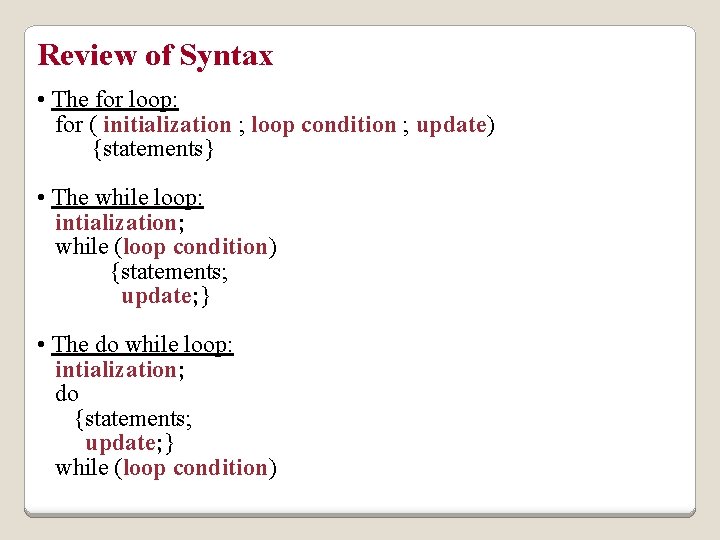
Review of Syntax • The for loop: for ( initialization ; loop condition ; update) {statements} • The while loop: intialization; while (loop condition) {statements; update; } • The do while loop: intialization; do {statements; update; } while (loop condition)
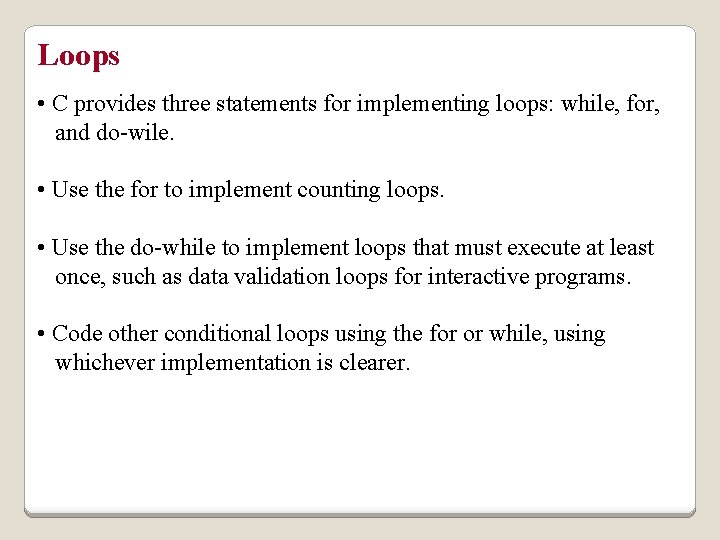
Loops • C provides three statements for implementing loops: while, for, and do-wile. • Use the for to implement counting loops. • Use the do-while to implement loops that must execute at least once, such as data validation loops for interactive programs. • Code other conditional loops using the for or while, using whichever implementation is clearer.
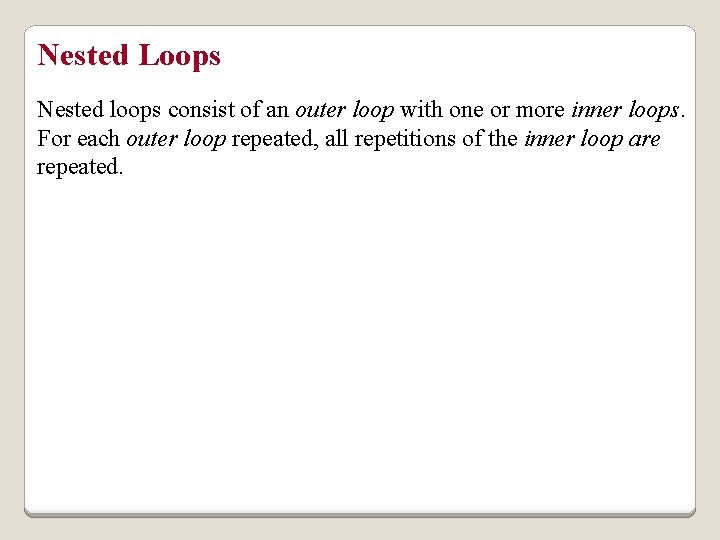
Nested Loops Nested loops consist of an outer loop with one or more inner loops. For each outer loop repeated, all repetitions of the inner loop are repeated.
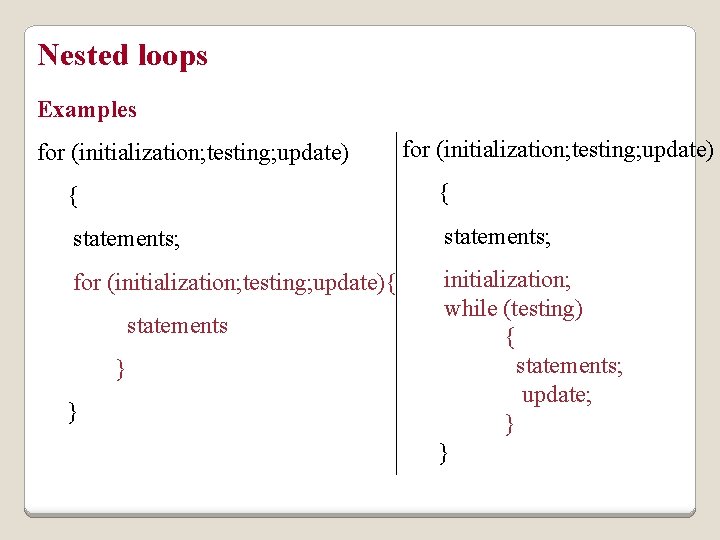
Nested loops Examples for (initialization; testing; update) { { statements; for (initialization; testing; update){ initialization; while (testing) { statements; update; } } statements } }
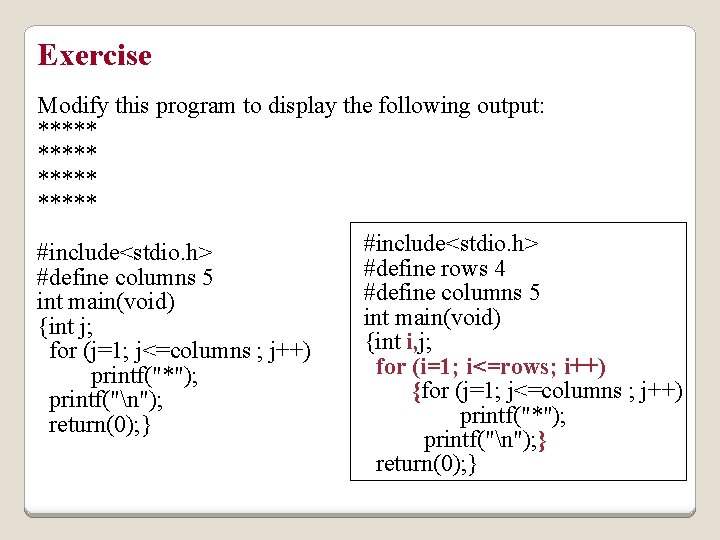
Exercise Modify this program to display the following output: ***** #include<stdio. h> #define columns 5 int main(void) {int j; for (j=1; j<=columns ; j++) printf("*"); printf("n"); return(0); } #include<stdio. h> #define rows 4 #define columns 5 int main(void) {int i, j; for (i=1; i<=rows; i++) {for (j=1; j<=columns ; j++) printf("*"); printf("n"); } return(0); }