Java Unit 9 Arrays Array Lists Array List
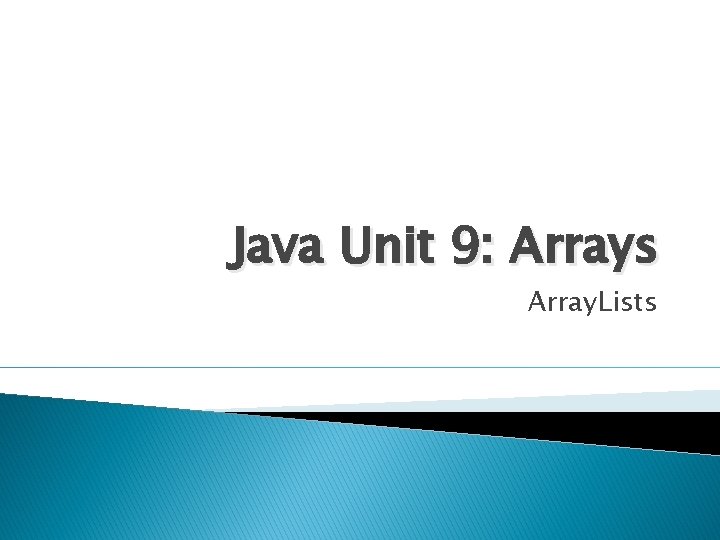
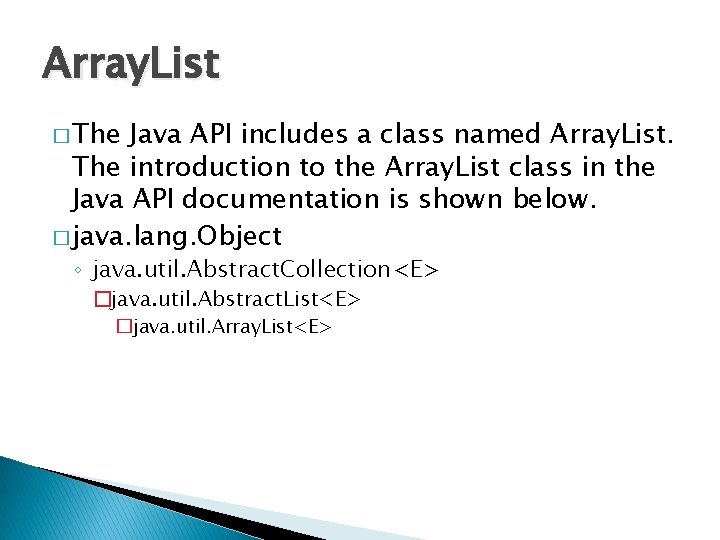
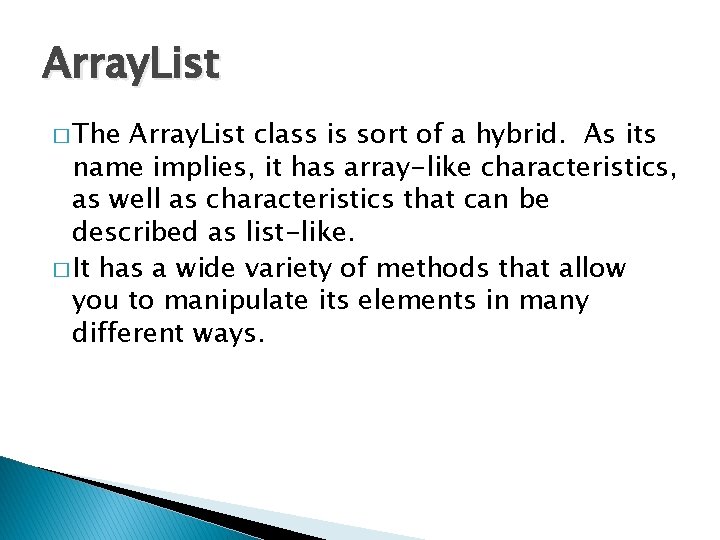
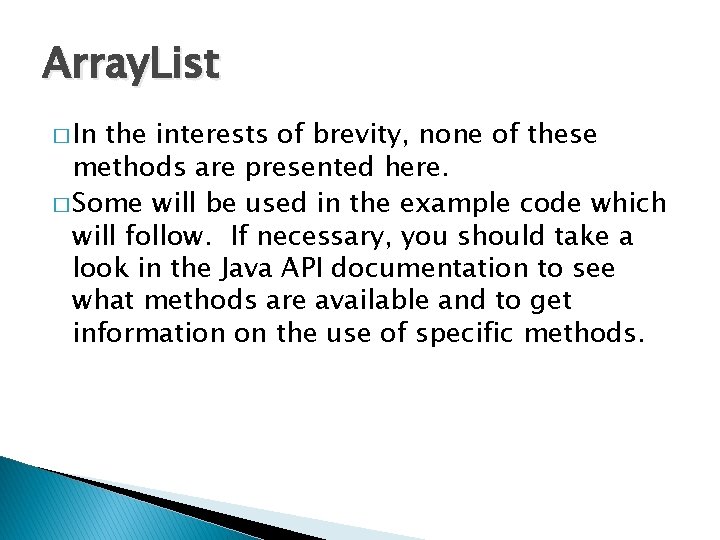
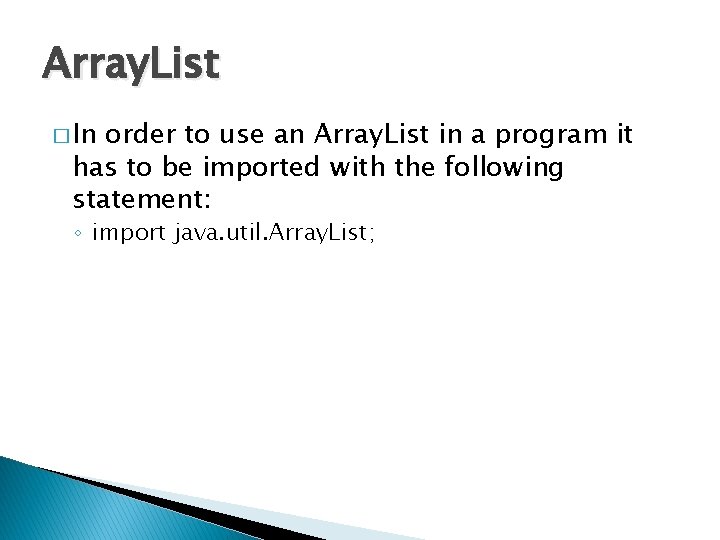
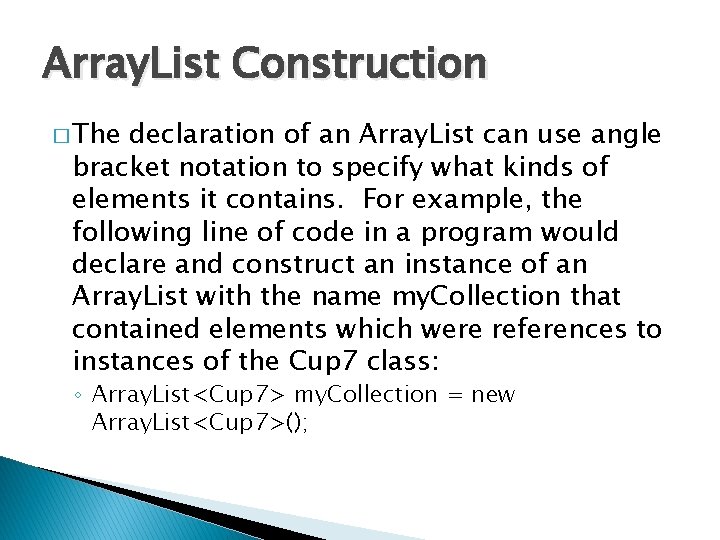
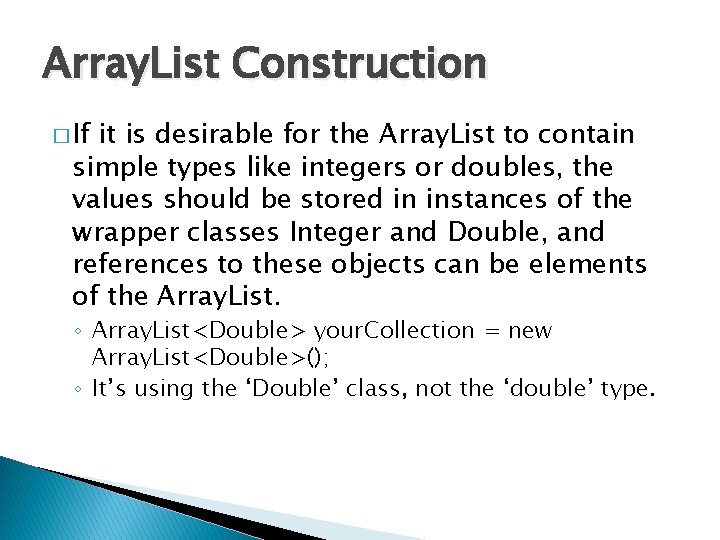
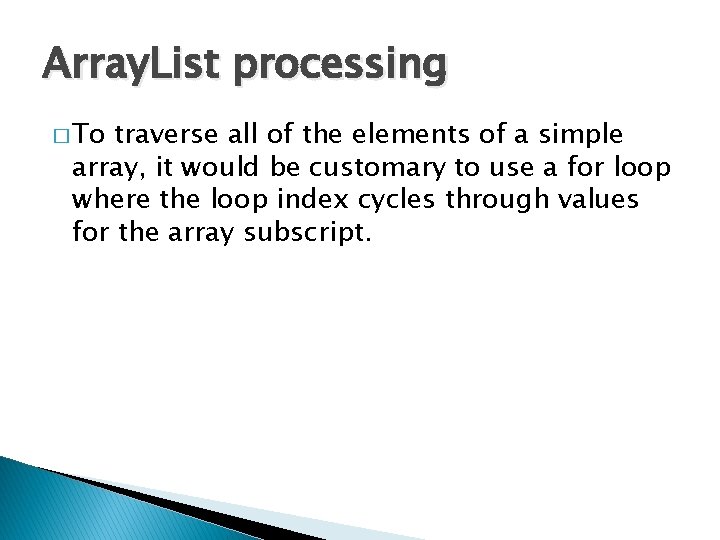
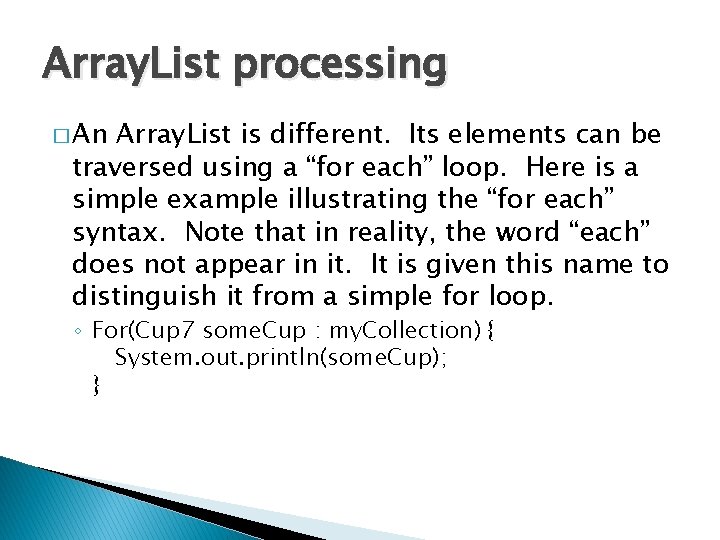
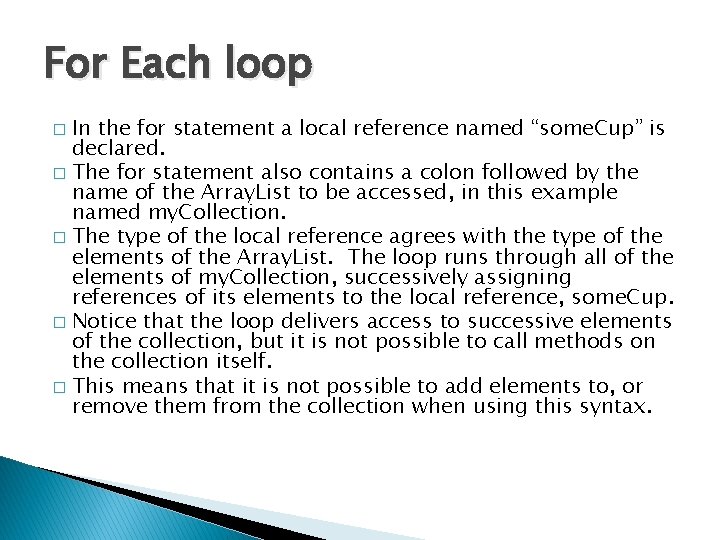
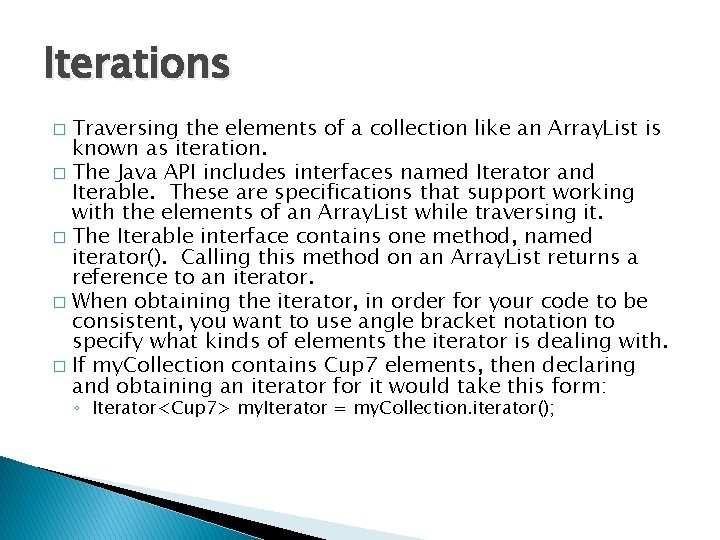
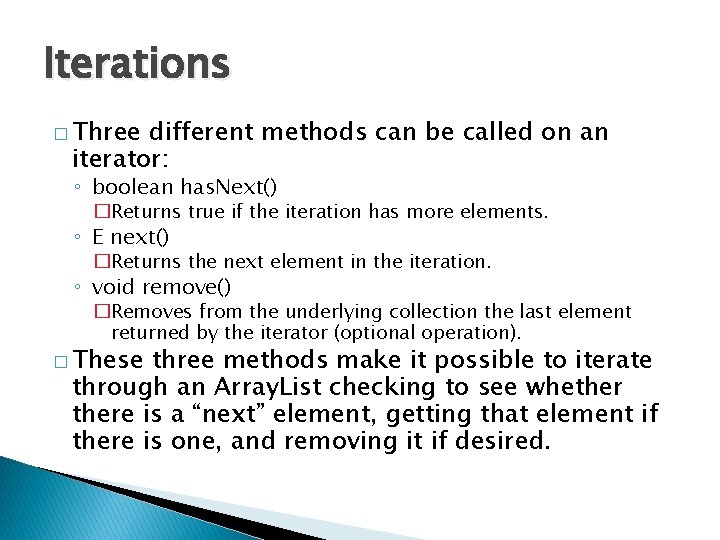
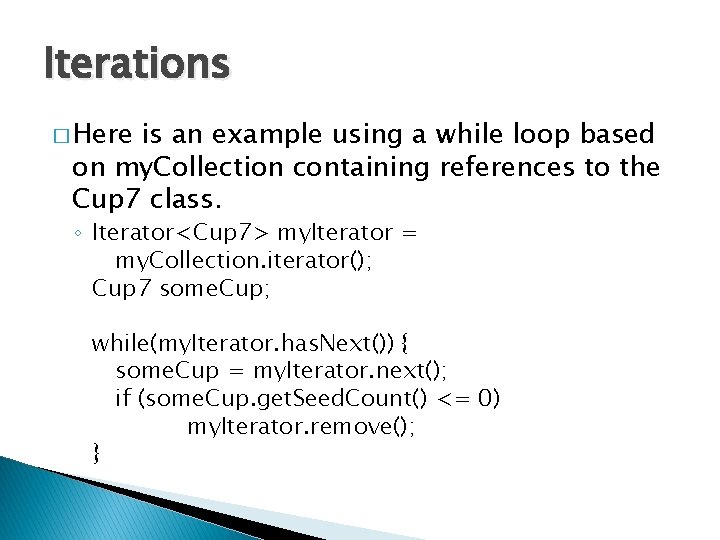
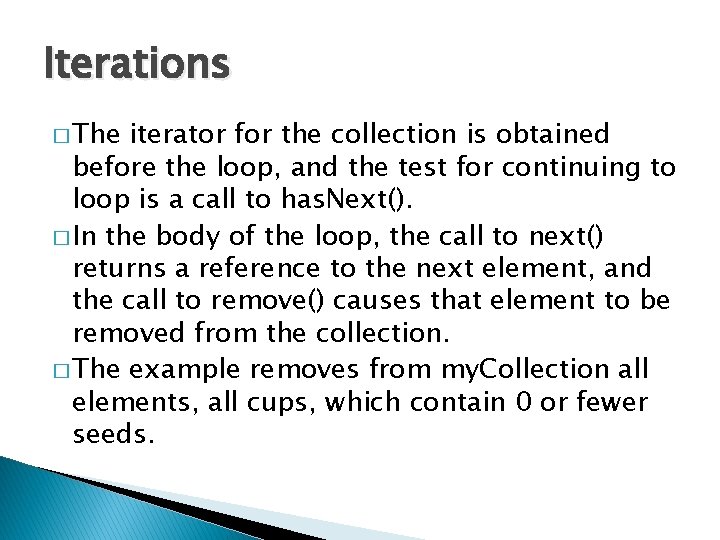
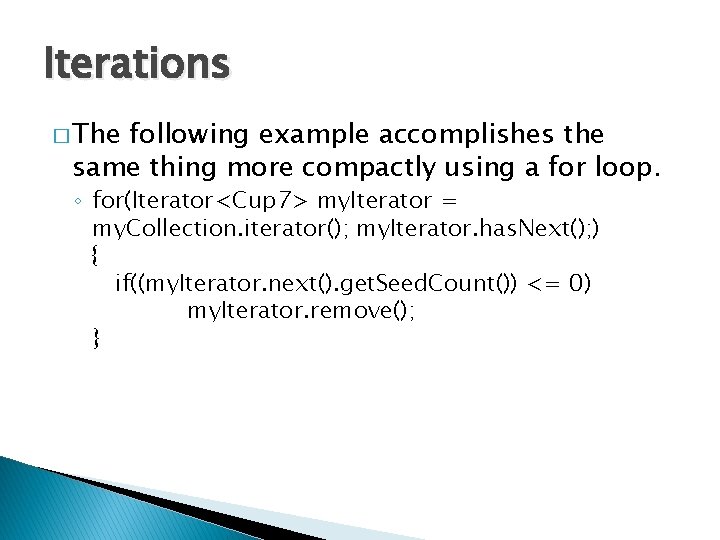
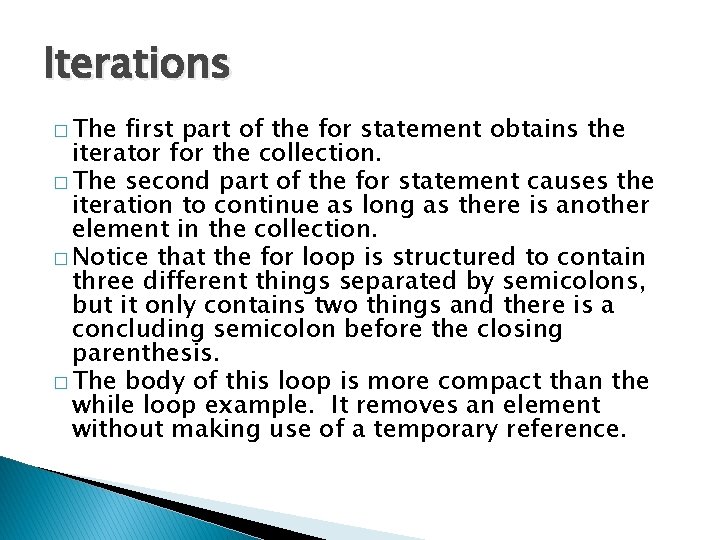
- Slides: 16
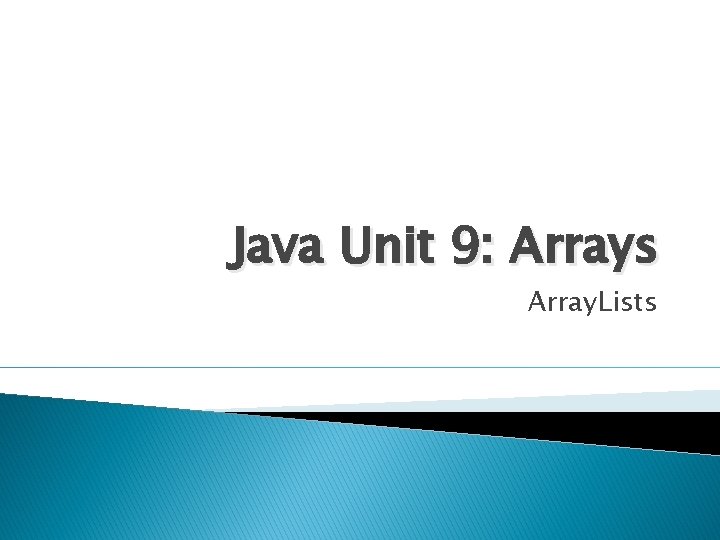
Java Unit 9: Arrays Array. Lists
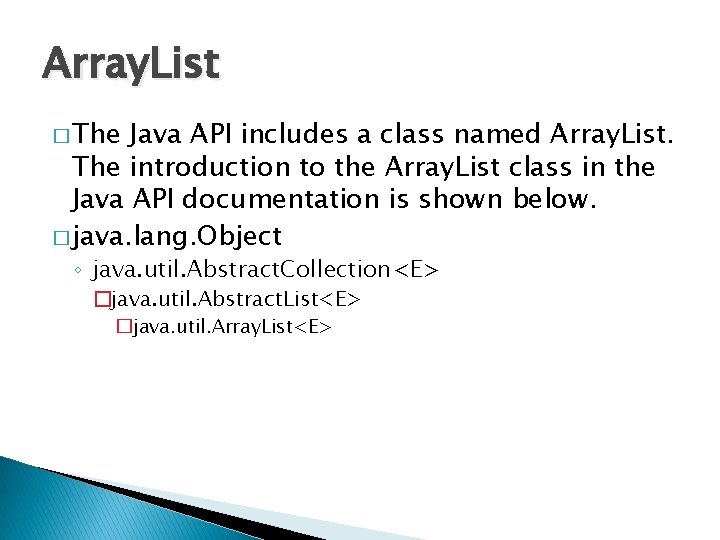
Array. List � The Java API includes a class named Array. List. The introduction to the Array. List class in the Java API documentation is shown below. � java. lang. Object ◦ java. util. Abstract. Collection<E> �java. util. Abstract. List<E> �java. util. Array. List<E>
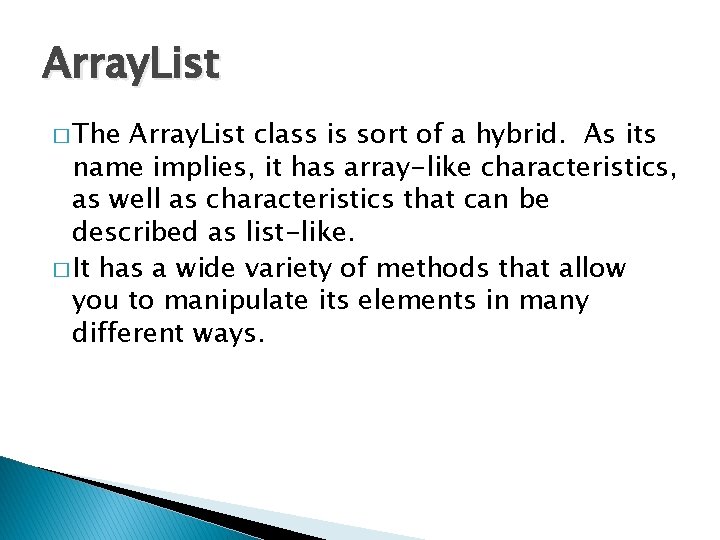
Array. List � The Array. List class is sort of a hybrid. As its name implies, it has array-like characteristics, as well as characteristics that can be described as list-like. � It has a wide variety of methods that allow you to manipulate its elements in many different ways.
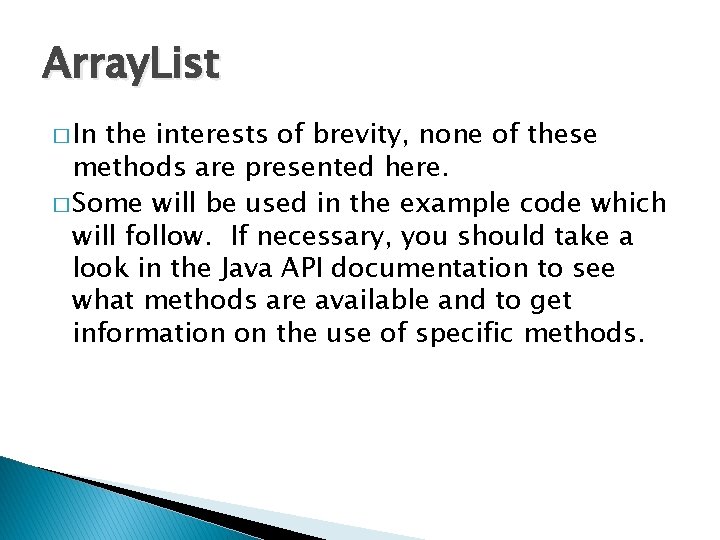
Array. List � In the interests of brevity, none of these methods are presented here. � Some will be used in the example code which will follow. If necessary, you should take a look in the Java API documentation to see what methods are available and to get information on the use of specific methods.
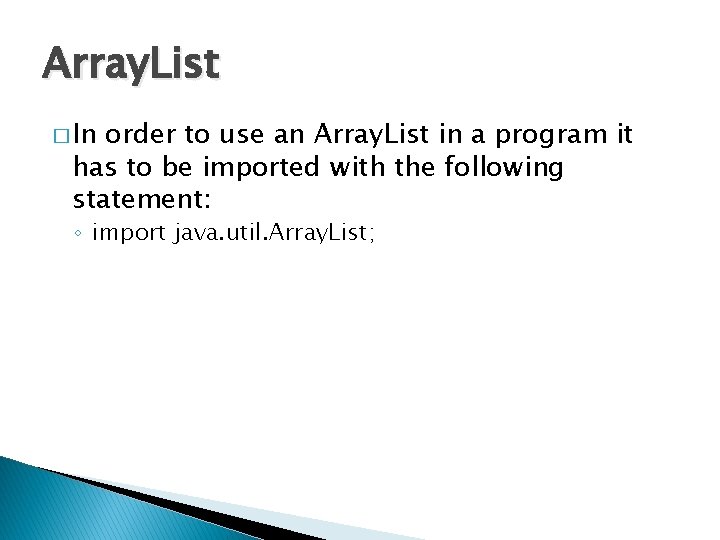
Array. List � In order to use an Array. List in a program it has to be imported with the following statement: ◦ import java. util. Array. List;
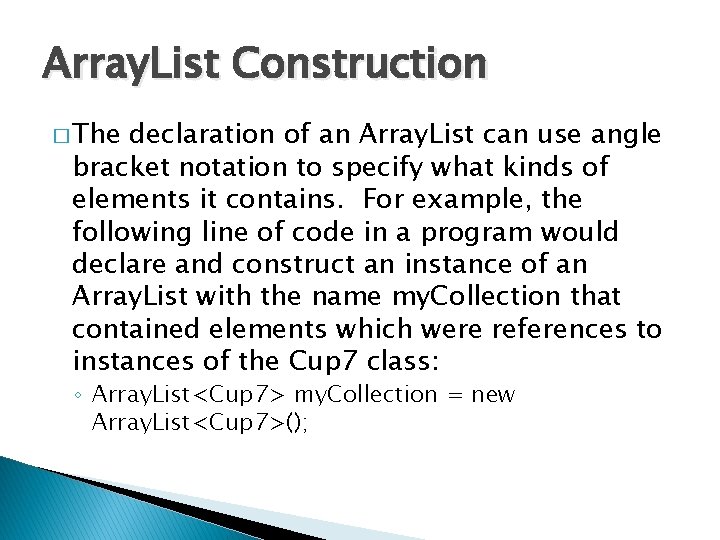
Array. List Construction � The declaration of an Array. List can use angle bracket notation to specify what kinds of elements it contains. For example, the following line of code in a program would declare and construct an instance of an Array. List with the name my. Collection that contained elements which were references to instances of the Cup 7 class: ◦ Array. List<Cup 7> my. Collection = new Array. List<Cup 7>();
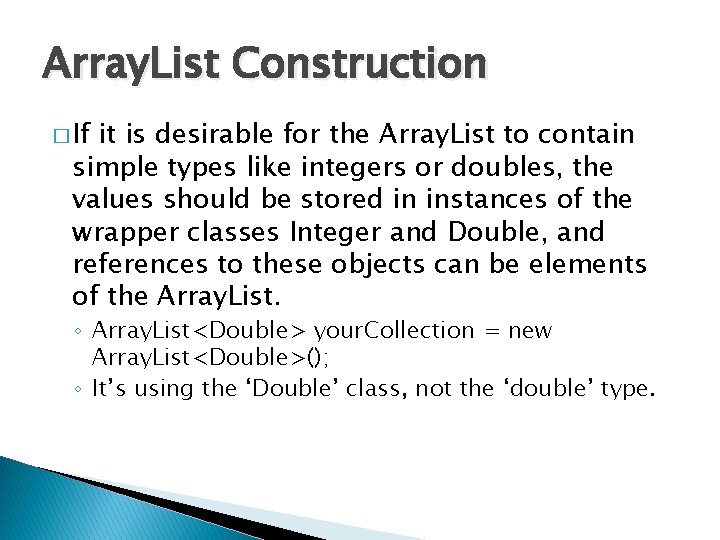
Array. List Construction � If it is desirable for the Array. List to contain simple types like integers or doubles, the values should be stored in instances of the wrapper classes Integer and Double, and references to these objects can be elements of the Array. List. ◦ Array. List<Double> your. Collection = new Array. List<Double>(); ◦ It’s using the ‘Double’ class, not the ‘double’ type.
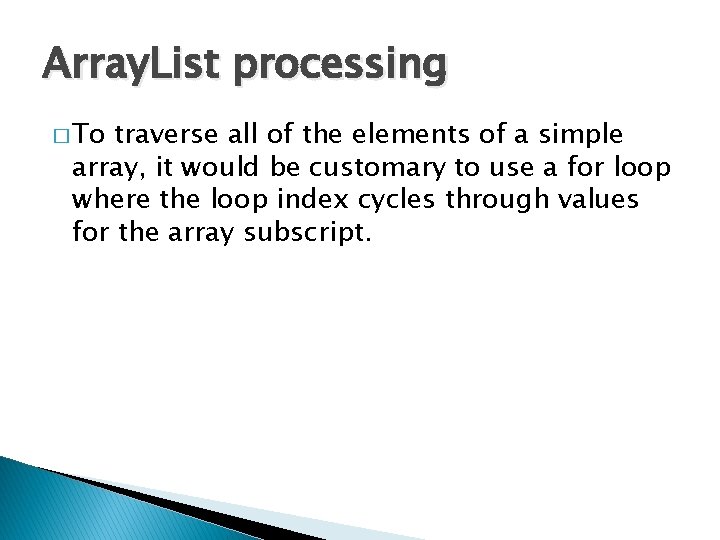
Array. List processing � To traverse all of the elements of a simple array, it would be customary to use a for loop where the loop index cycles through values for the array subscript.
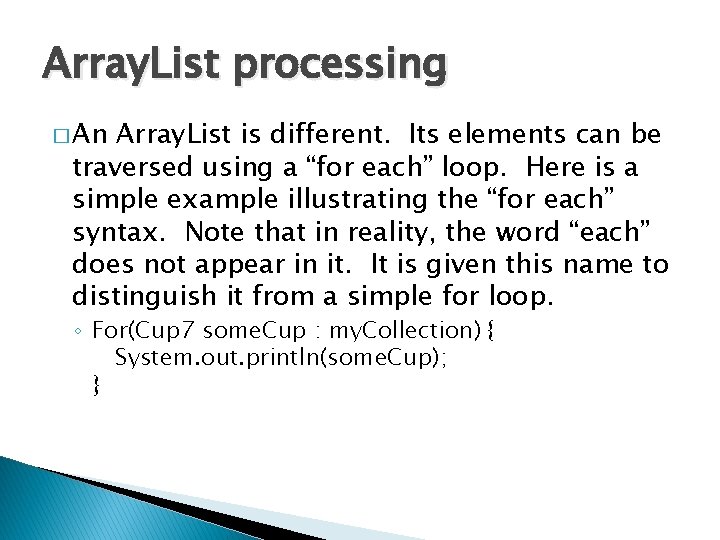
Array. List processing � An Array. List is different. Its elements can be traversed using a “for each” loop. Here is a simple example illustrating the “for each” syntax. Note that in reality, the word “each” does not appear in it. It is given this name to distinguish it from a simple for loop. ◦ For(Cup 7 some. Cup : my. Collection) { System. out. println(some. Cup); }
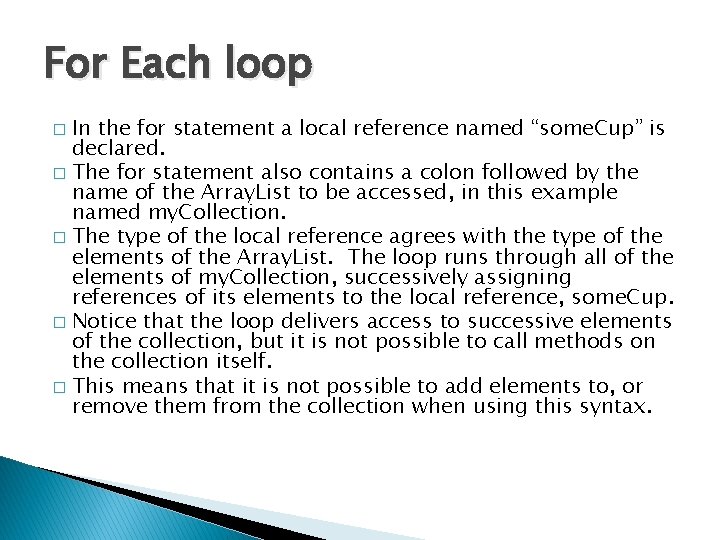
For Each loop In the for statement a local reference named “some. Cup” is declared. � The for statement also contains a colon followed by the name of the Array. List to be accessed, in this example named my. Collection. � The type of the local reference agrees with the type of the elements of the Array. List. The loop runs through all of the elements of my. Collection, successively assigning references of its elements to the local reference, some. Cup. � Notice that the loop delivers access to successive elements of the collection, but it is not possible to call methods on the collection itself. � This means that it is not possible to add elements to, or remove them from the collection when using this syntax. �
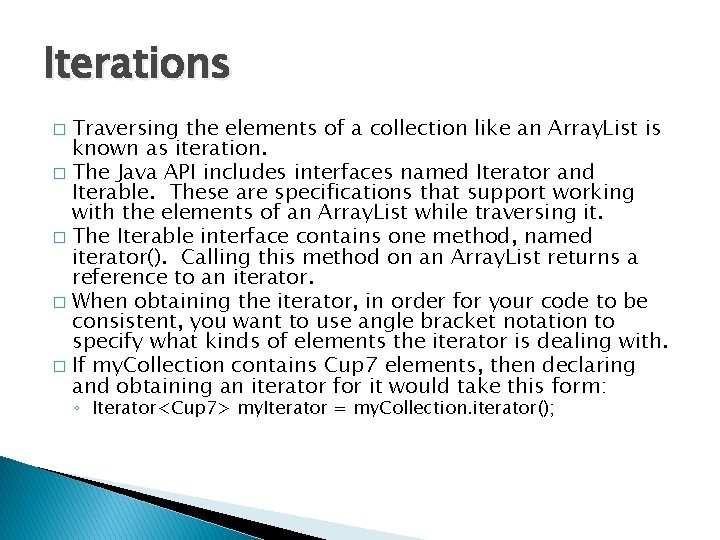
Iterations Traversing the elements of a collection like an Array. List is known as iteration. � The Java API includes interfaces named Iterator and Iterable. These are specifications that support working with the elements of an Array. List while traversing it. � The Iterable interface contains one method, named iterator(). Calling this method on an Array. List returns a reference to an iterator. � When obtaining the iterator, in order for your code to be consistent, you want to use angle bracket notation to specify what kinds of elements the iterator is dealing with. � If my. Collection contains Cup 7 elements, then declaring and obtaining an iterator for it would take this form: � ◦ Iterator<Cup 7> my. Iterator = my. Collection. iterator();
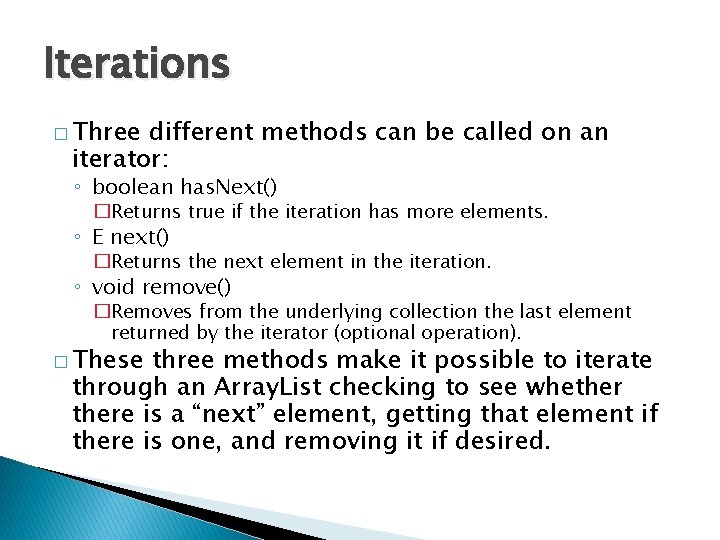
Iterations � Three different methods can be called on an iterator: ◦ boolean has. Next() �Returns true if the iteration has more elements. ◦ E next() �Returns the next element in the iteration. ◦ void remove() �Removes from the underlying collection the last element returned by the iterator (optional operation). � These three methods make it possible to iterate through an Array. List checking to see whethere is a “next” element, getting that element if there is one, and removing it if desired.
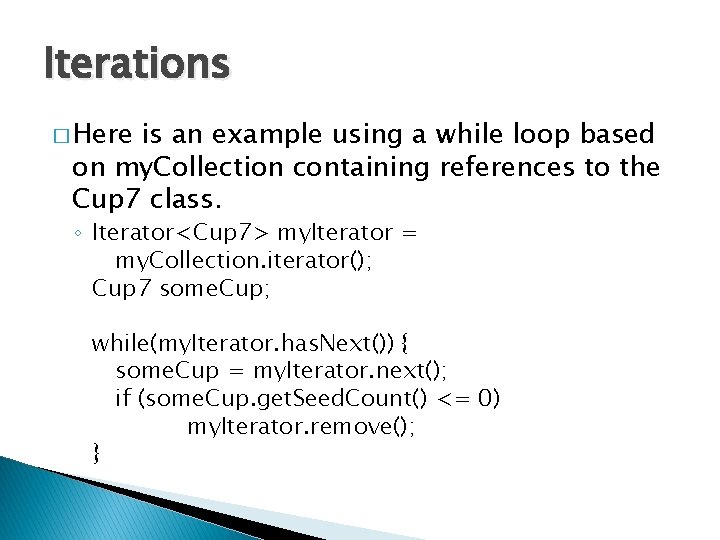
Iterations � Here is an example using a while loop based on my. Collection containing references to the Cup 7 class. ◦ Iterator<Cup 7> my. Iterator = my. Collection. iterator(); Cup 7 some. Cup; while(my. Iterator. has. Next()) { some. Cup = my. Iterator. next(); if (some. Cup. get. Seed. Count() <= 0) my. Iterator. remove(); }
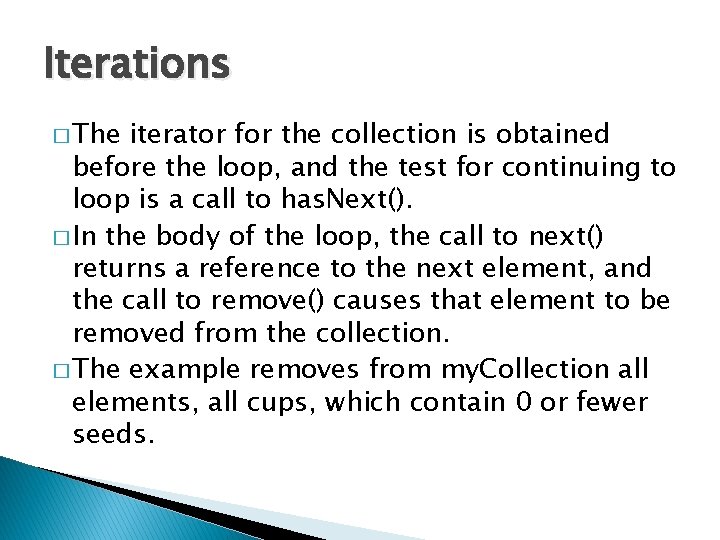
Iterations � The iterator for the collection is obtained before the loop, and the test for continuing to loop is a call to has. Next(). � In the body of the loop, the call to next() returns a reference to the next element, and the call to remove() causes that element to be removed from the collection. � The example removes from my. Collection all elements, all cups, which contain 0 or fewer seeds.
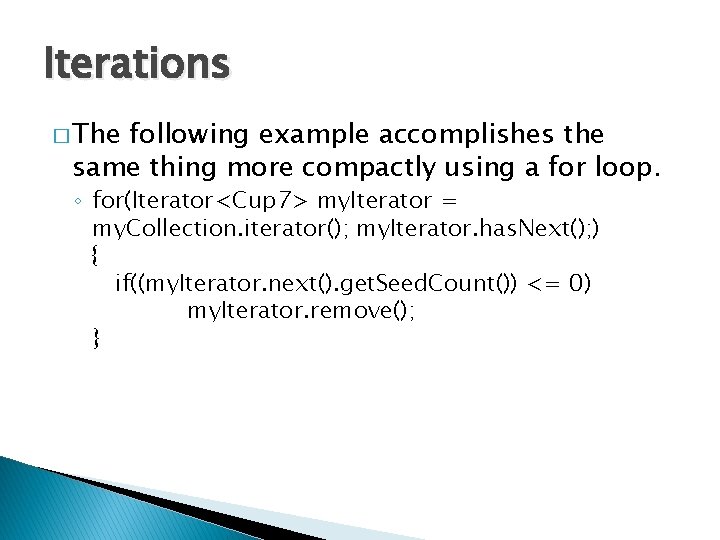
Iterations � The following example accomplishes the same thing more compactly using a for loop. ◦ for(Iterator<Cup 7> my. Iterator = my. Collection. iterator(); my. Iterator. has. Next(); ) { if((my. Iterator. next(). get. Seed. Count()) <= 0) my. Iterator. remove(); }
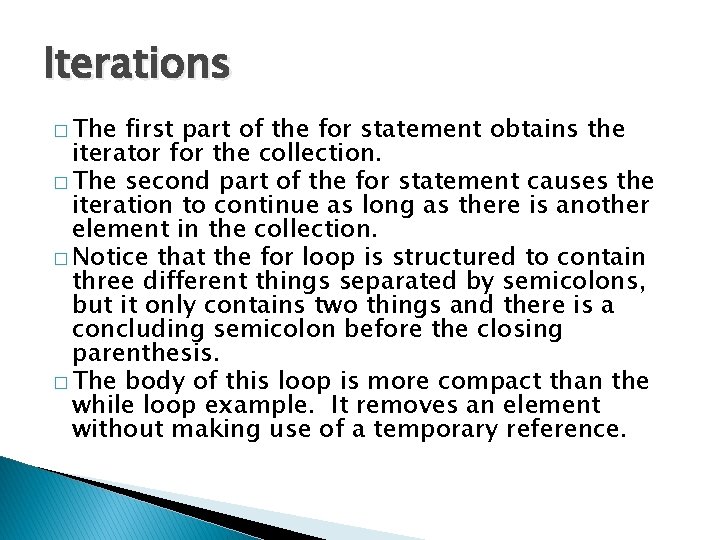
Iterations � The first part of the for statement obtains the iterator for the collection. � The second part of the for statement causes the iteration to continue as long as there is another element in the collection. � Notice that the for loop is structured to contain three different things separated by semicolons, but it only contains two things and there is a concluding semicolon before the closing parenthesis. � The body of this loop is more compact than the while loop example. It removes an element without making use of a temporary reference.
Array of arrays c++
Parallel arrays
Suma de arreglos unidimensionales en c
Arrays bidimensionales java
Python list of arrays
Java types of lists
Python plot list of lists
Prolog empty list
Java exceptions list
Difference between array and vector in java
What is collection
Ragged array
Veteork
Parallel arrays
Why do we need arrays?
Dynamic arrays and amortized analysis
Array mips