ITM 352 Simple Arrays ITM 352 Port Kazman
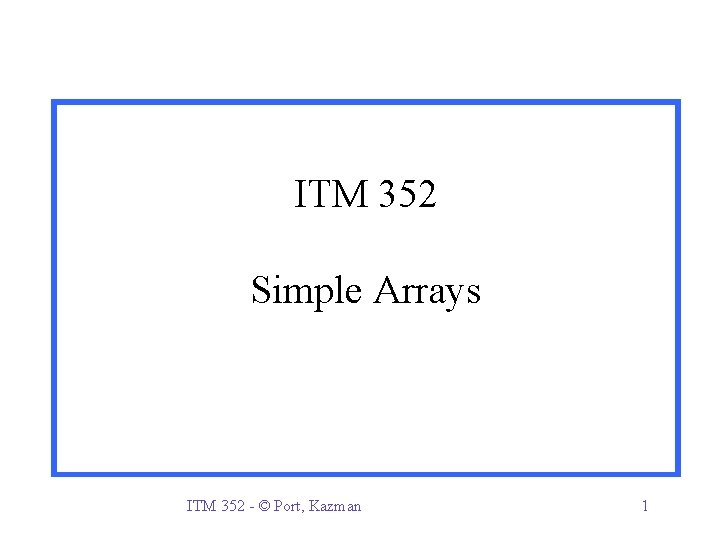
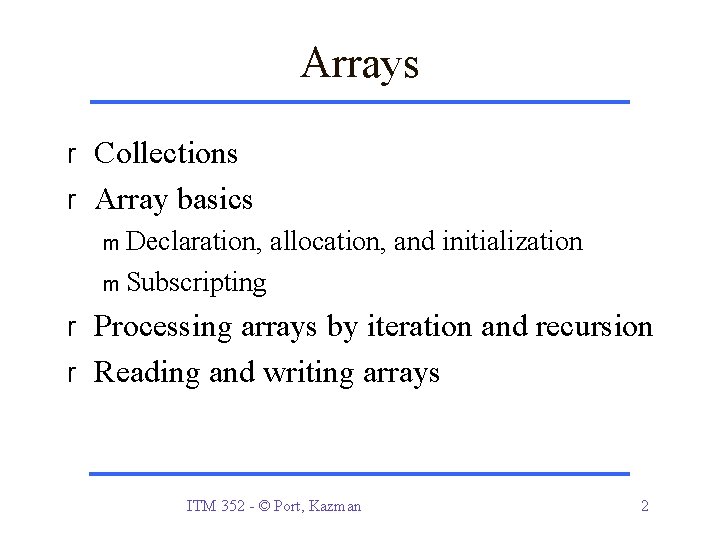
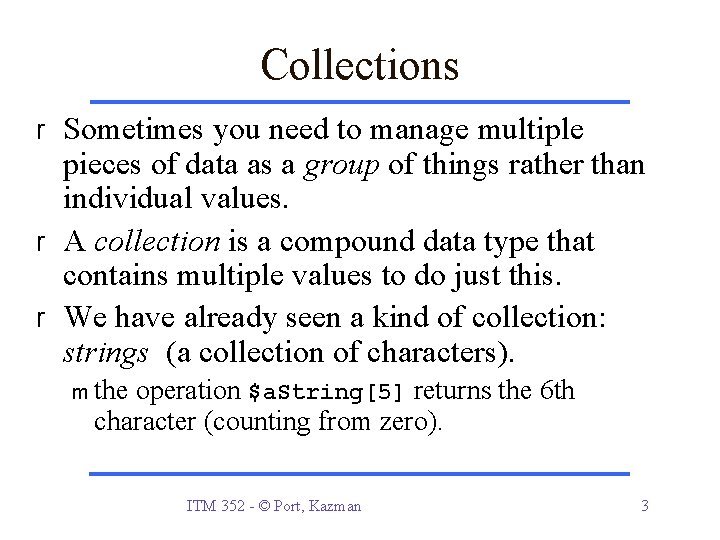
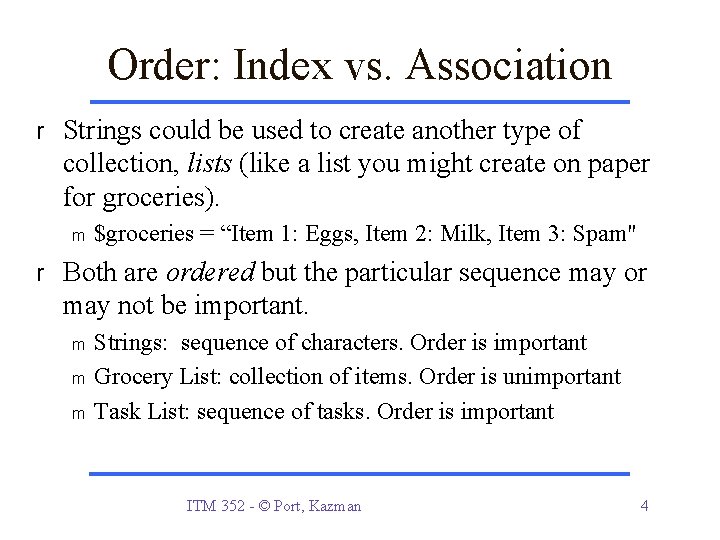
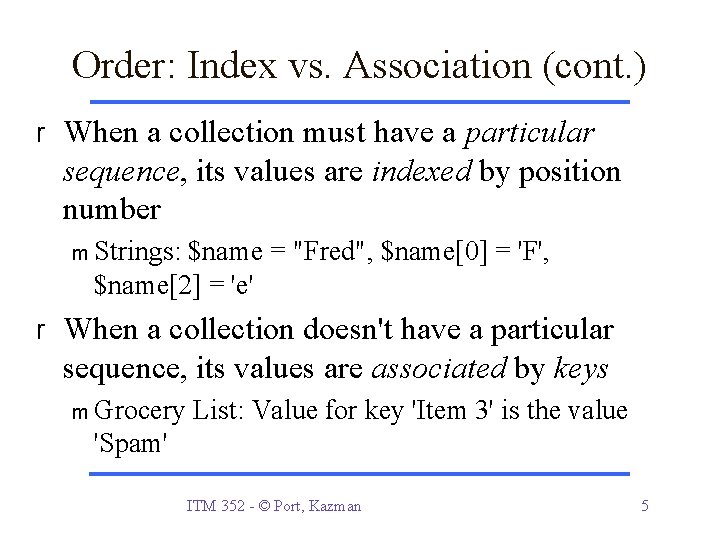
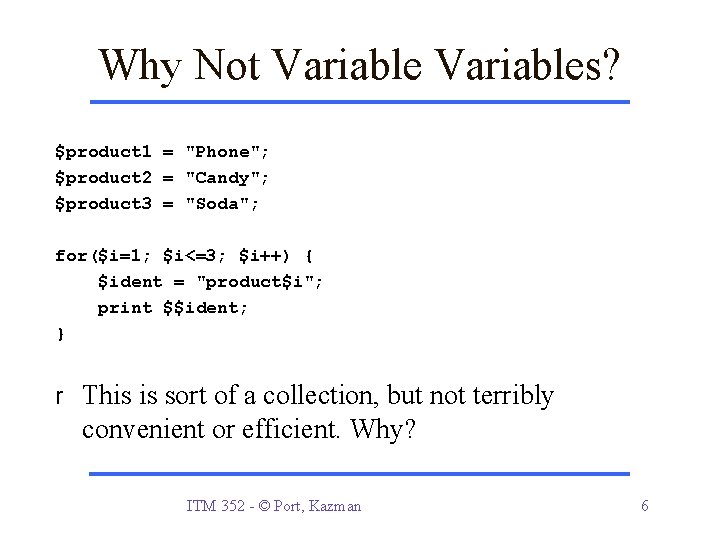
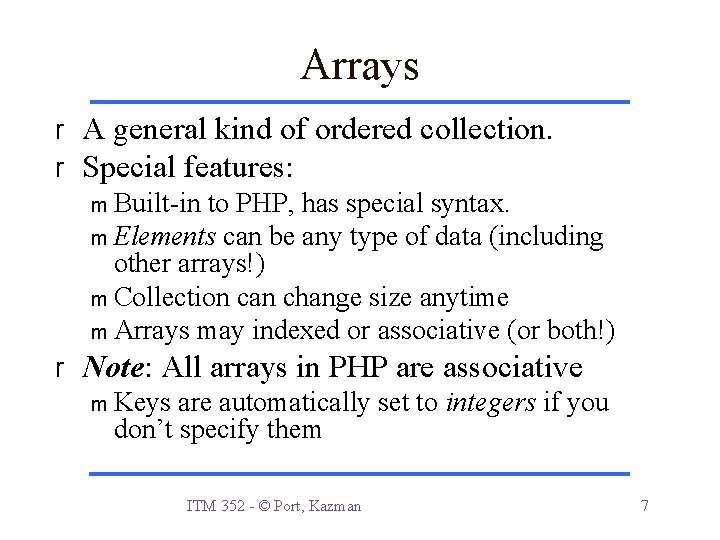
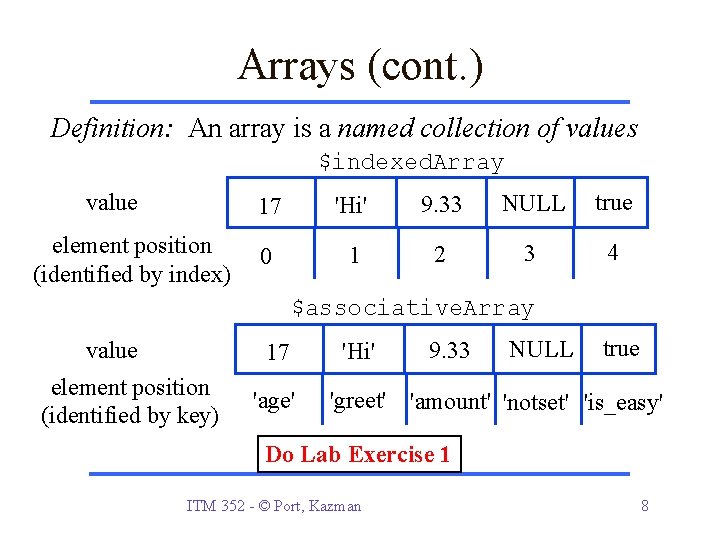
![Some Array Terminology Array name $product['price'] key - also called a subscript - must Some Array Terminology Array name $product['price'] key - also called a subscript - must](https://slidetodoc.com/presentation_image_h2/e06e2001b02e745182f0d78f63ce0ef3/image-9.jpg)
![Some Array Terminology (indexed) Array name $temperature[$n + 2] Index - also called a Some Array Terminology (indexed) Array name $temperature[$n + 2] Index - also called a](https://slidetodoc.com/presentation_image_h2/e06e2001b02e745182f0d78f63ce0ef3/image-10.jpg)
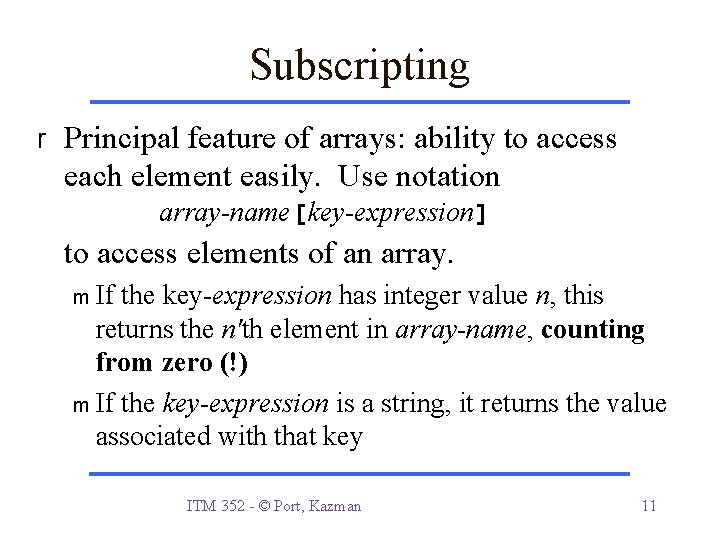
![Subscripting Examples $grades[0] = 75; echo $grades[0]; This is how you add elements to Subscripting Examples $grades[0] = 75; echo $grades[0]; This is how you add elements to](https://slidetodoc.com/presentation_image_h2/e06e2001b02e745182f0d78f63ce0ef3/image-12.jpg)
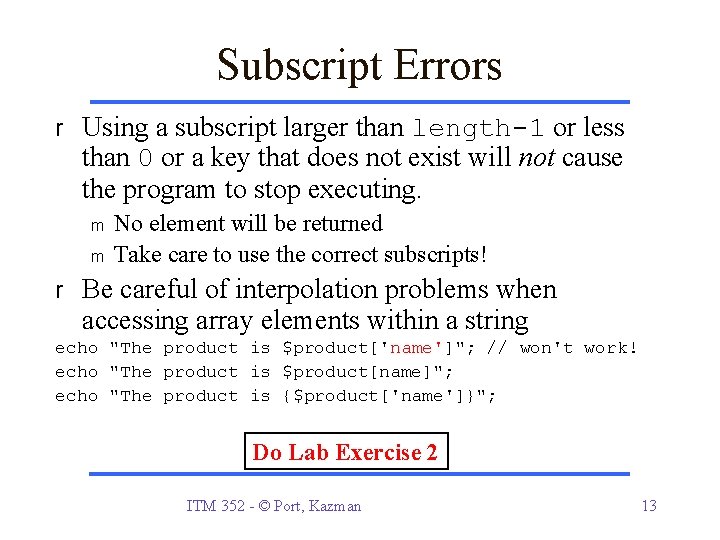
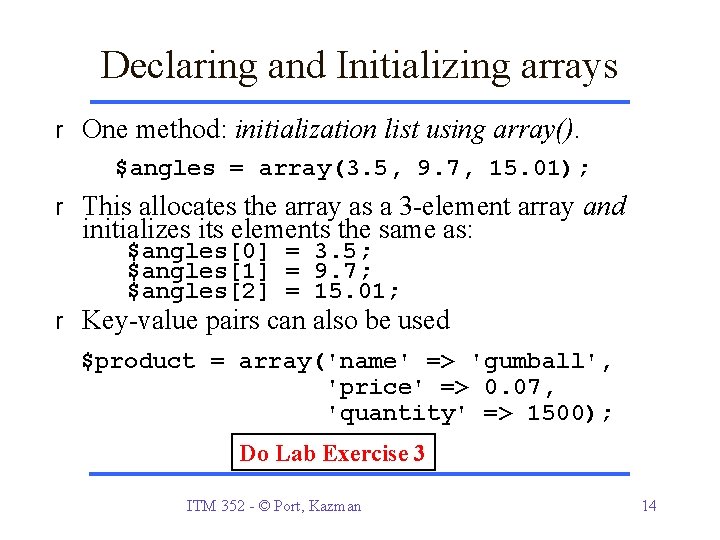
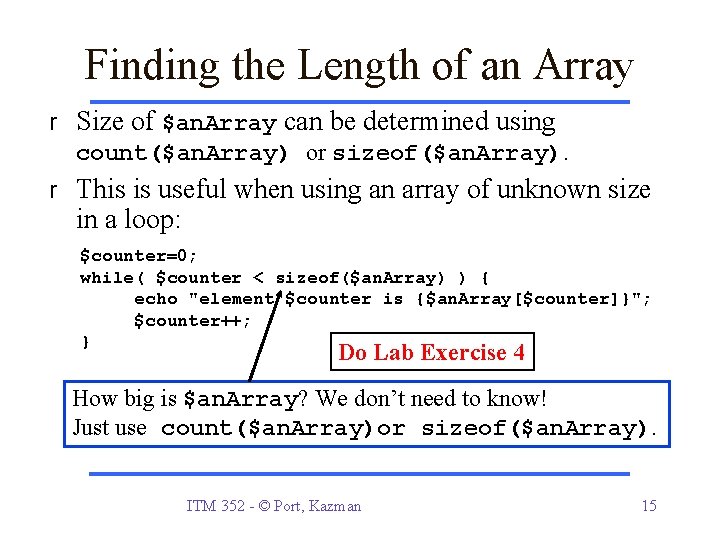
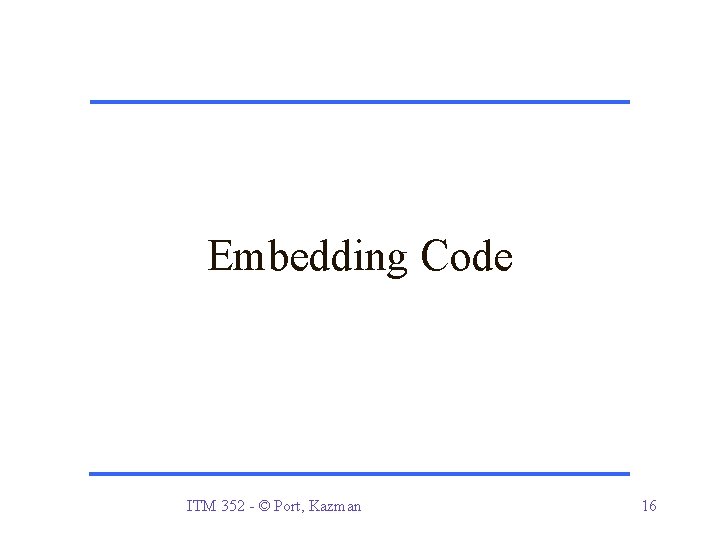
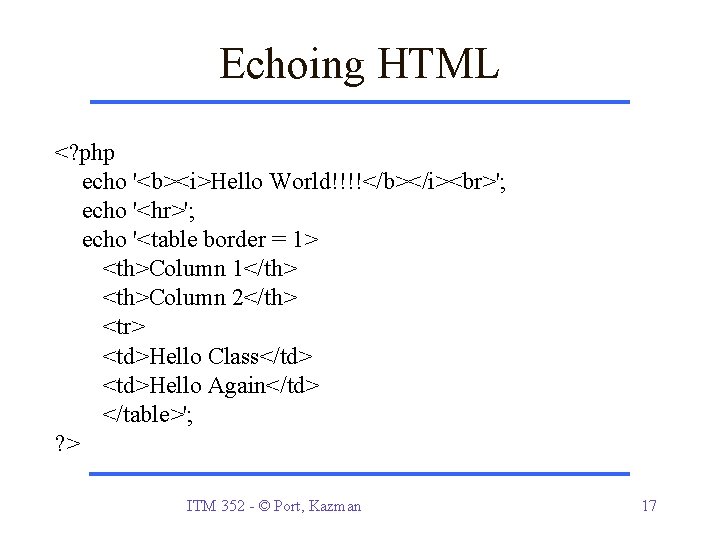
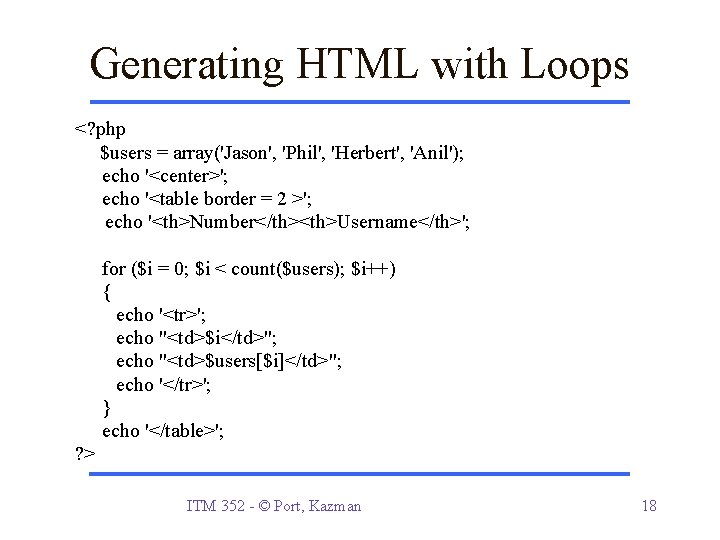
- Slides: 18
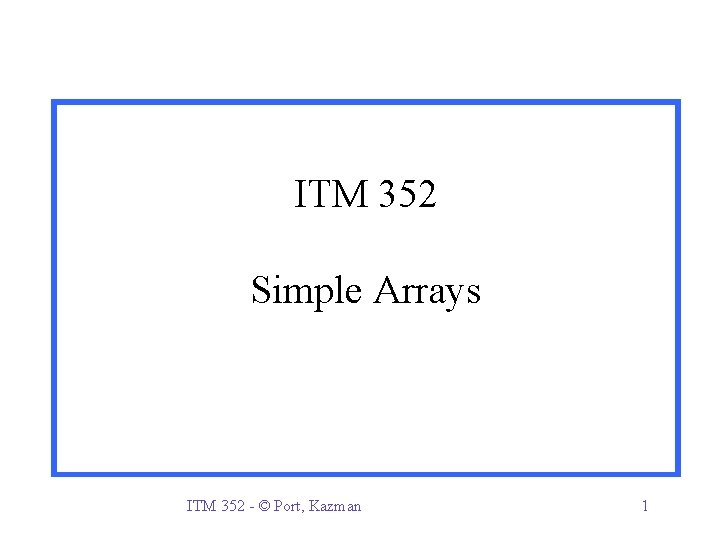
ITM 352 Simple Arrays ITM 352 - © Port, Kazman 1
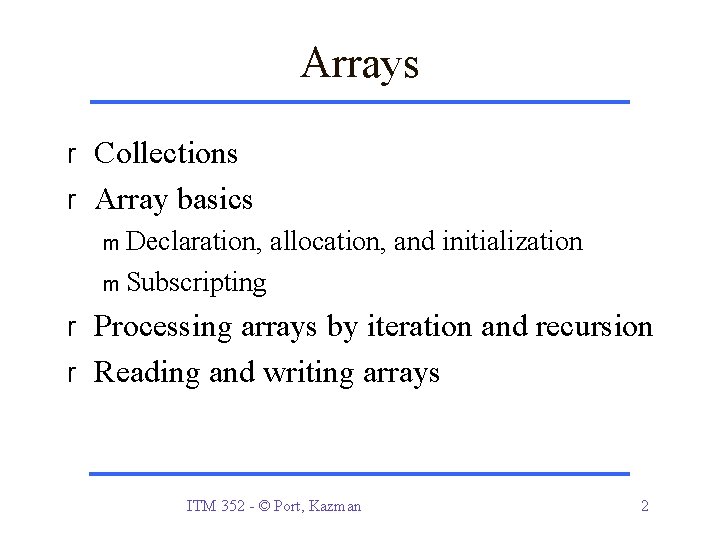
Arrays r Collections r Array basics m Declaration, allocation, and initialization m Subscripting r Processing arrays by iteration and recursion r Reading and writing arrays ITM 352 - © Port, Kazman 2
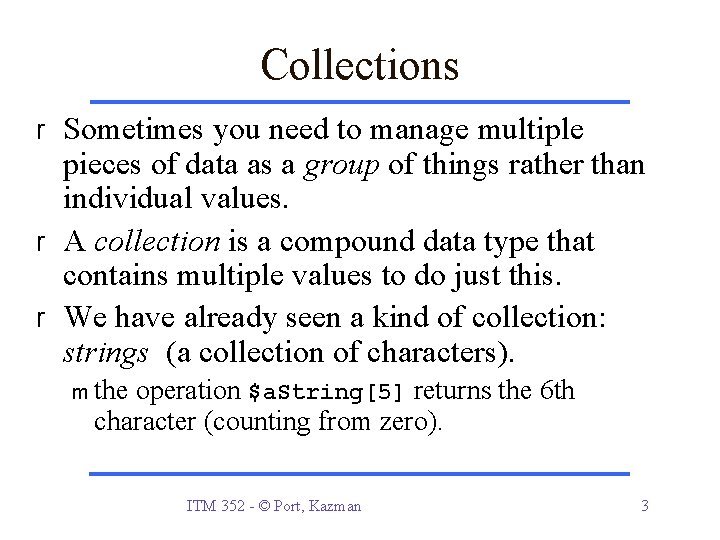
Collections r Sometimes you need to manage multiple pieces of data as a group of things rather than individual values. r A collection is a compound data type that contains multiple values to do just this. r We have already seen a kind of collection: strings (a collection of characters). m the operation $a. String[5] returns the 6 th character (counting from zero). ITM 352 - © Port, Kazman 3
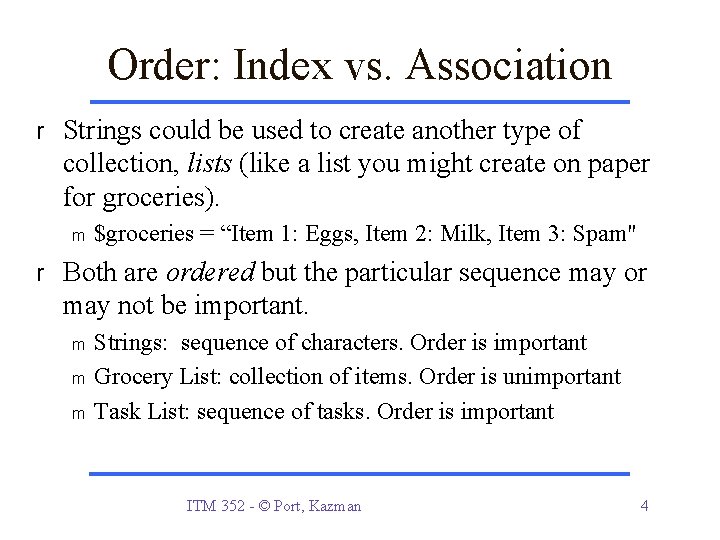
Order: Index vs. Association r Strings could be used to create another type of collection, lists (like a list you might create on paper for groceries). m $groceries = “Item 1: Eggs, Item 2: Milk, Item 3: Spam" r Both are ordered but the particular sequence may or may not be important. m m m Strings: sequence of characters. Order is important Grocery List: collection of items. Order is unimportant Task List: sequence of tasks. Order is important ITM 352 - © Port, Kazman 4
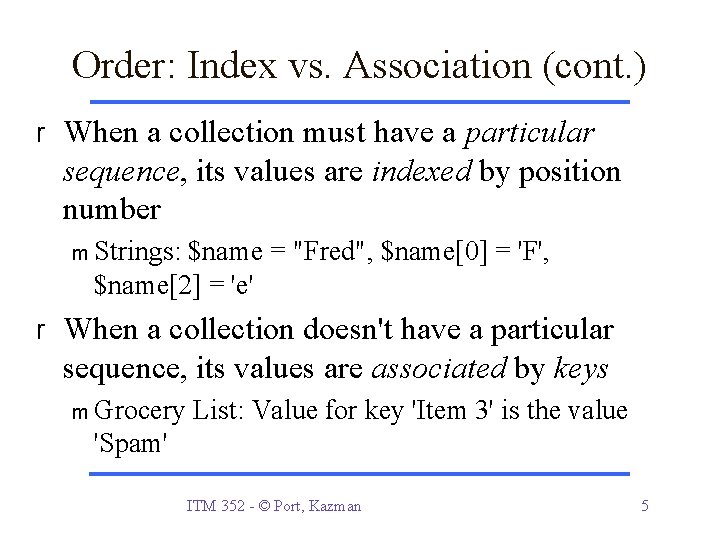
Order: Index vs. Association (cont. ) r When a collection must have a particular sequence, its values are indexed by position number m Strings: $name = "Fred", $name[0] = 'F', $name[2] = 'e' r When a collection doesn't have a particular sequence, its values are associated by keys m Grocery List: Value for key 'Item 3' is the value 'Spam' ITM 352 - © Port, Kazman 5
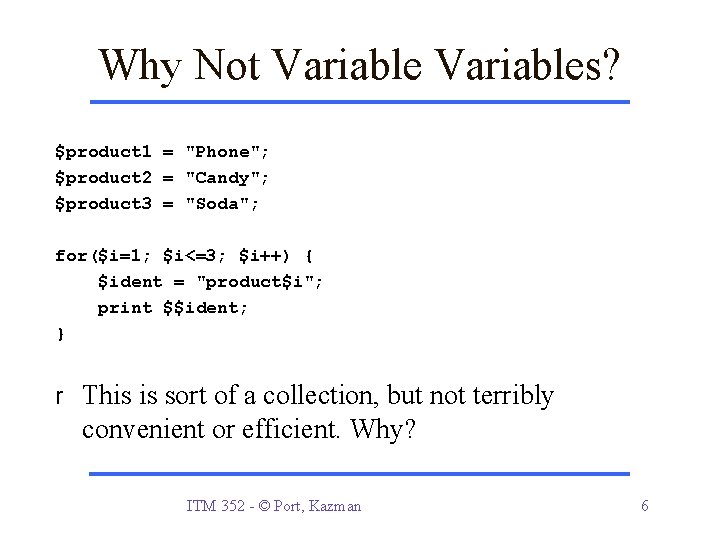
Why Not Variables? $product 1 = "Phone"; $product 2 = "Candy"; $product 3 = "Soda"; for($i=1; $i<=3; $i++) { $ident = "product$i"; print $$ident; } r This is sort of a collection, but not terribly convenient or efficient. Why? ITM 352 - © Port, Kazman 6
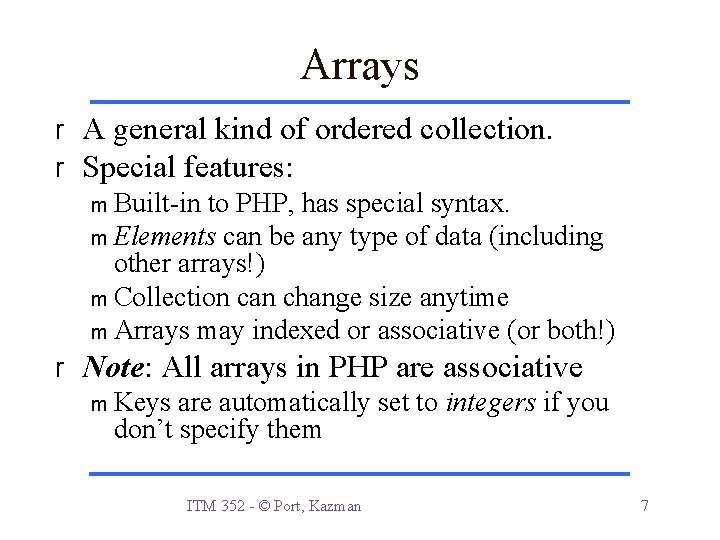
Arrays r A general kind of ordered collection. r Special features: m Built-in to PHP, has special syntax. m Elements can be any type of data (including other arrays!) m Collection can change size anytime m Arrays may indexed or associative (or both!) r Note: All arrays in PHP are associative m Keys are automatically set to integers if you don’t specify them ITM 352 - © Port, Kazman 7
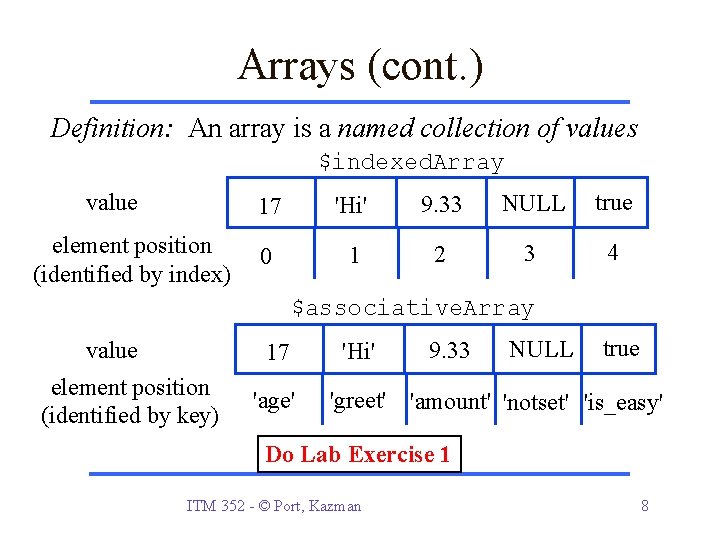
Arrays (cont. ) Definition: An array is a named collection of values $indexed. Array value element position (identified by index) 17 'Hi' 9. 33 NULL true 0 1 2 3 4 $associative. Array value 17 element position (identified by key) 'age' 'Hi' 9. 33 NULL true 'greet' 'amount' 'notset' 'is_easy' Do Lab Exercise 1 ITM 352 - © Port, Kazman 8
![Some Array Terminology Array name productprice key also called a subscript must Some Array Terminology Array name $product['price'] key - also called a subscript - must](https://slidetodoc.com/presentation_image_h2/e06e2001b02e745182f0d78f63ce0ef3/image-9.jpg)
Some Array Terminology Array name $product['price'] key - also called a subscript - must be an int, - or an expression that evaluates to an int $product['price'] keyed variable - also called an element or subscripted variable $product['price'] Value of the indexed variable; also called an element of the array $product['price'] = 32; Note that "element" may refer to either a single indexed variable in the array or the value of a single indexed variable. Kazman ITM 352 - © Port, 9
![Some Array Terminology indexed Array name temperaturen 2 Index also called a Some Array Terminology (indexed) Array name $temperature[$n + 2] Index - also called a](https://slidetodoc.com/presentation_image_h2/e06e2001b02e745182f0d78f63ce0ef3/image-10.jpg)
Some Array Terminology (indexed) Array name $temperature[$n + 2] Index - also called a subscript - must be an int, - or an expression that evaluates to an int $temperature[$n + 2] Indexed variable - also called an element or subscripted variable $temperature[$n + 2] Value of the indexed variable; also called an element of the array $temperature[$n + 2] = 32; Note that "element" may refer to either a single indexed variable in the array or the value of a single indexed variable. Kazman ITM 352 - © Port, 10
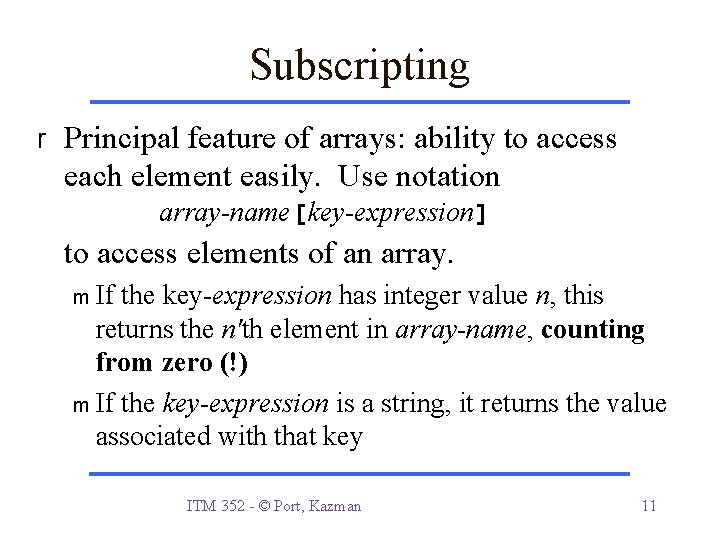
Subscripting r Principal feature of arrays: ability to access each element easily. Use notation array-name[key-expression] to access elements of an array. m If the key-expression has integer value n, this returns the n'th element in array-name, counting from zero (!) m If the key-expression is a string, it returns the value associated with that key ITM 352 - © Port, Kazman 11
![Subscripting Examples grades0 75 echo grades0 This is how you add elements to Subscripting Examples $grades[0] = 75; echo $grades[0]; This is how you add elements to](https://slidetodoc.com/presentation_image_h2/e06e2001b02e745182f0d78f63ce0ef3/image-12.jpg)
Subscripting Examples $grades[0] = 75; echo $grades[0]; This is how you add elements to an existing indexed array. $i = 2; $clocks[$i-1] = time(); $clocks[] = time()+1; printf("<BR>time[1] is %s and time[2] is %s", $clocks[$i-1], $clocks[2]); $name['first'] = "Rumple"; $name['last'] = "Stilskin"; echo "<BR>name is {$name['first']} {$name['last']}"; $thingy[] = 1; $thingy[] = 4; echo "<BR> $thingy[0] and $thingy[1]"; ITM 352 - © Port, Kazman 12
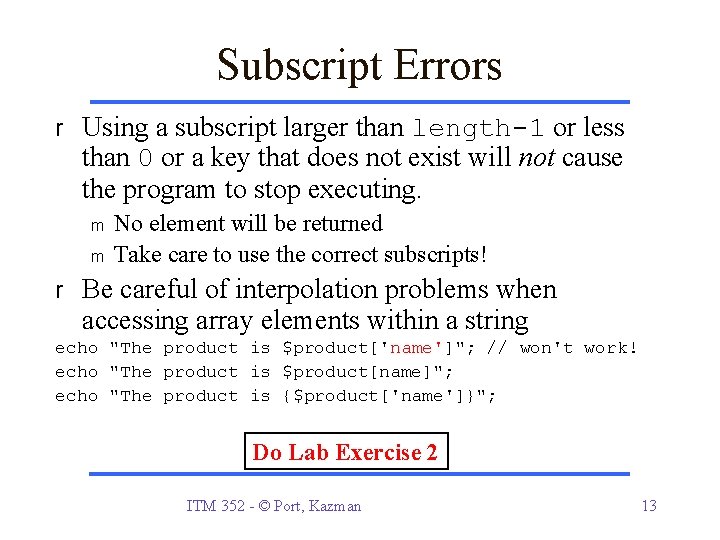
Subscript Errors r Using a subscript larger than length-1 or less than 0 or a key that does not exist will not cause the program to stop executing. m m No element will be returned Take care to use the correct subscripts! r Be careful of interpolation problems when accessing array elements within a string echo "The product is $product['name']"; // won't work! echo "The product is $product[name]"; echo "The product is {$product['name']}"; Do Lab Exercise 2 ITM 352 - © Port, Kazman 13
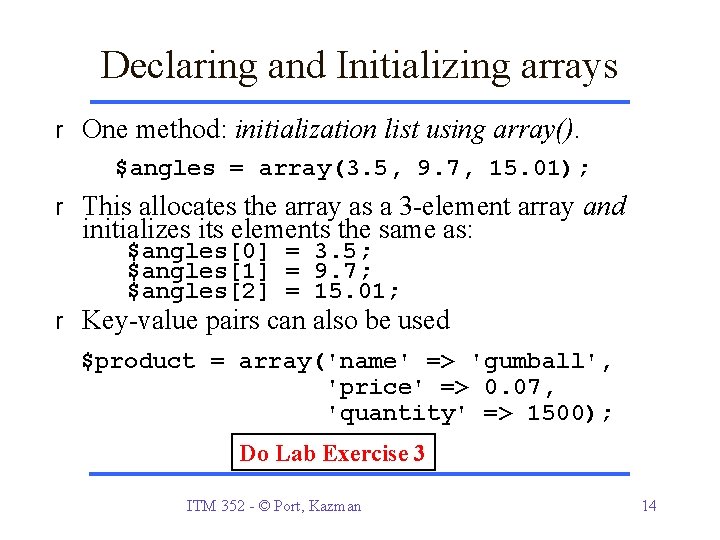
Declaring and Initializing arrays r One method: initialization list using array(). $angles = array(3. 5, 9. 7, 15. 01); r This allocates the array as a 3 -element array and initializes its elements the same as: $angles[0] = 3. 5; $angles[1] = 9. 7; $angles[2] = 15. 01; r Key-value pairs can also be used $product = array('name' => 'gumball', 'price' => 0. 07, 'quantity' => 1500); Do Lab Exercise 3 ITM 352 - © Port, Kazman 14
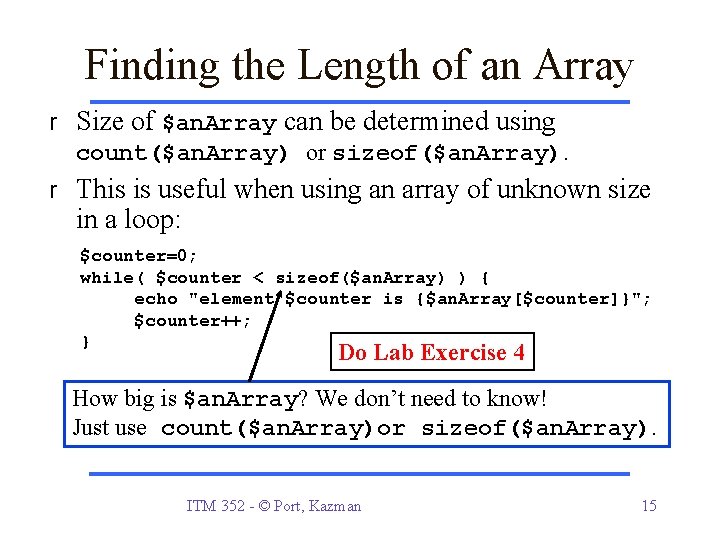
Finding the Length of an Array r Size of $an. Array can be determined using count($an. Array) or sizeof($an. Array). r This is useful when using an array of unknown size in a loop: $counter=0; while( $counter < sizeof($an. Array) ) { echo "element $counter is {$an. Array[$counter]}"; $counter++; } Do Lab Exercise 4 How big is $an. Array? We don’t need to know! Just use count($an. Array)or sizeof($an. Array). ITM 352 - © Port, Kazman 15
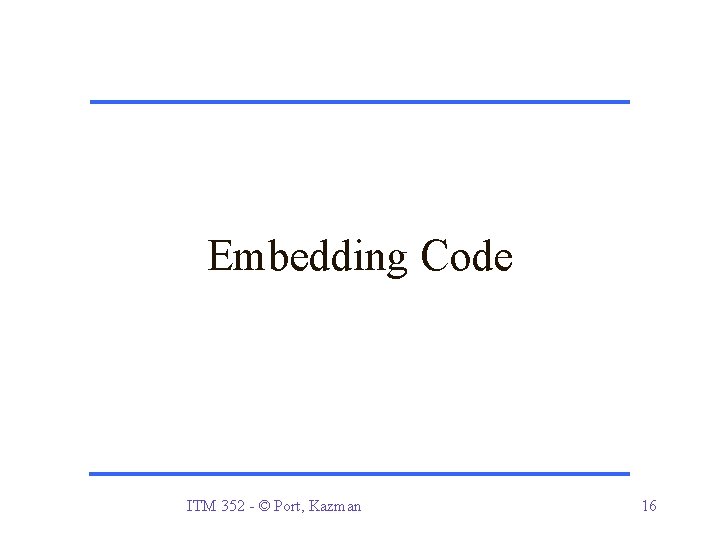
Embedding Code ITM 352 - © Port, Kazman 16
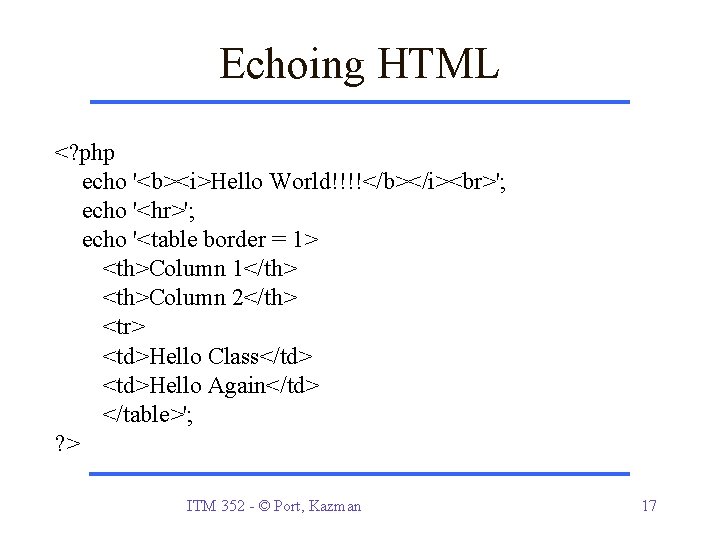
Echoing HTML <? php echo '<b><i>Hello World!!!!</b></i> '; echo '<hr>'; echo '<table border = 1> <th>Column 1</th> <th>Column 2</th> <tr> <td>Hello Class</td> <td>Hello Again</td> </table>'; ? > ITM 352 - © Port, Kazman 17
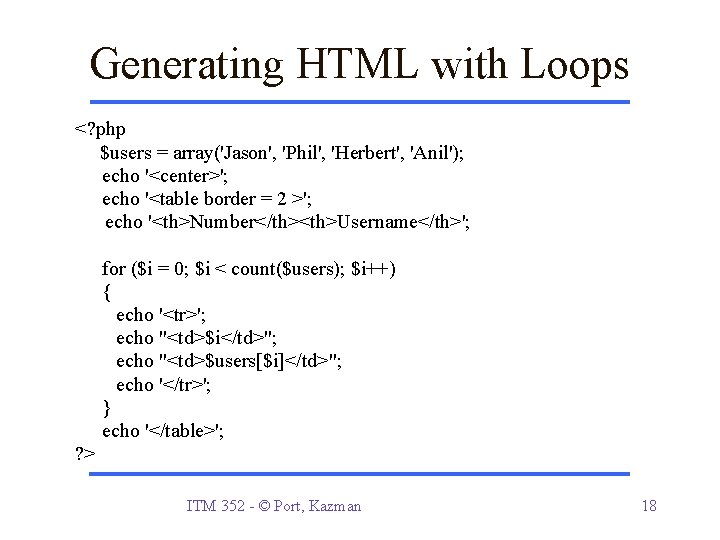
Generating HTML with Loops <? php $users = array('Jason', 'Phil', 'Herbert', 'Anil'); echo '<center>'; echo '<table border = 2 >'; echo '<th>Number</th><th>Username</th>'; for ($i = 0; $i < count($users); $i++) { echo '<tr>'; echo "<td>$i</td>"; echo "<td>$users[$i]</td>"; echo '</tr>'; } echo '</table>'; ? > ITM 352 - © Port, Kazman 18
Rick kazman
8255 a
Which one is programmable communication interface
Wave port in hfss
Ion itm
Itm monitoring tool
/index2.php?include= si|e: itm
Itm giurgiu
Akta 1976 itm
Network topology generator
Itm vrancea
Itm maquillage
Parallel arrays in c
Array of arrays c++
Java array operations
Partially filled arrays
C++ parallel arrays
Why do we need arrays?
Dynamic arrays and amortized analysis