IO Streams A stream is a sequence of
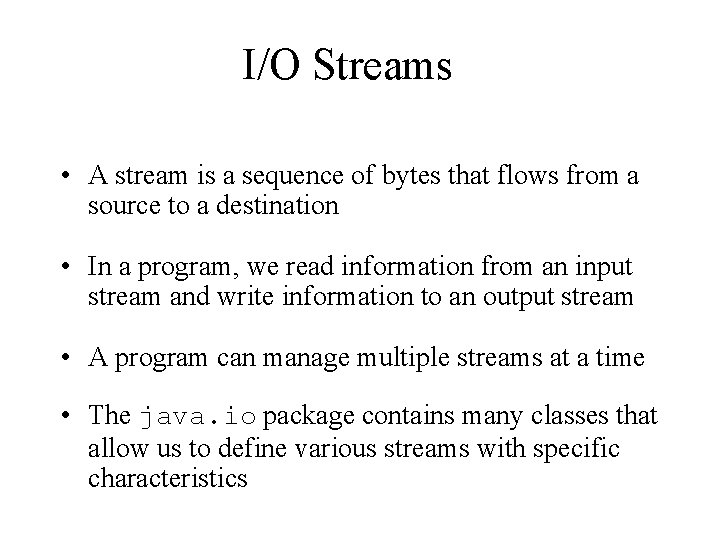
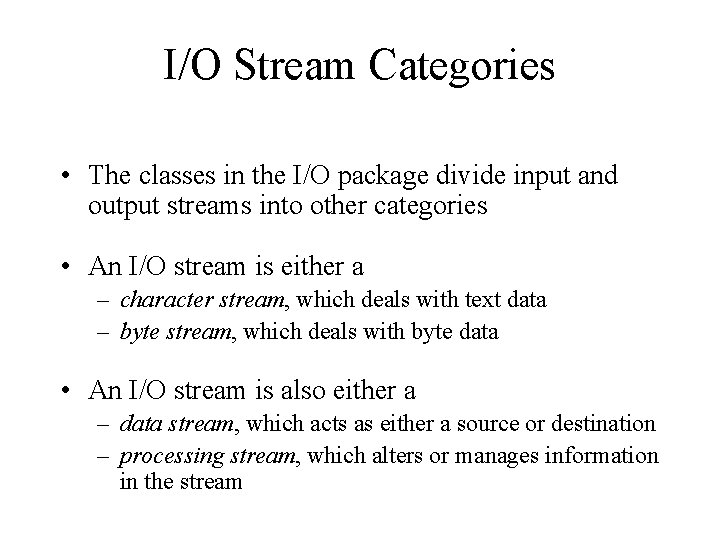
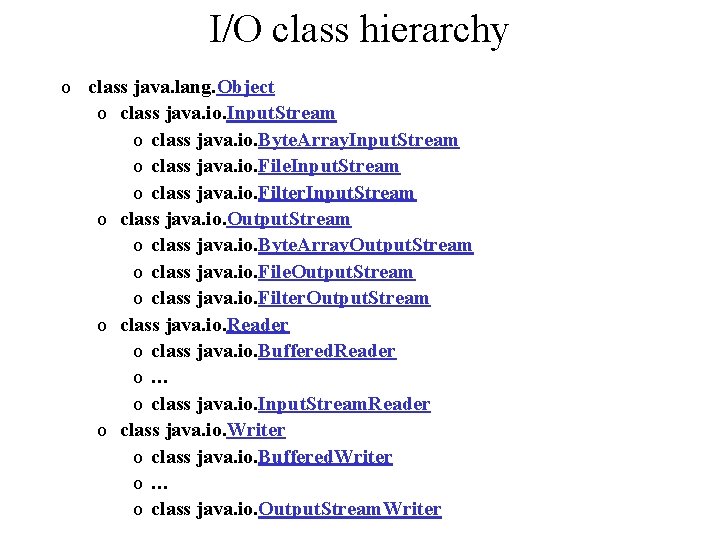
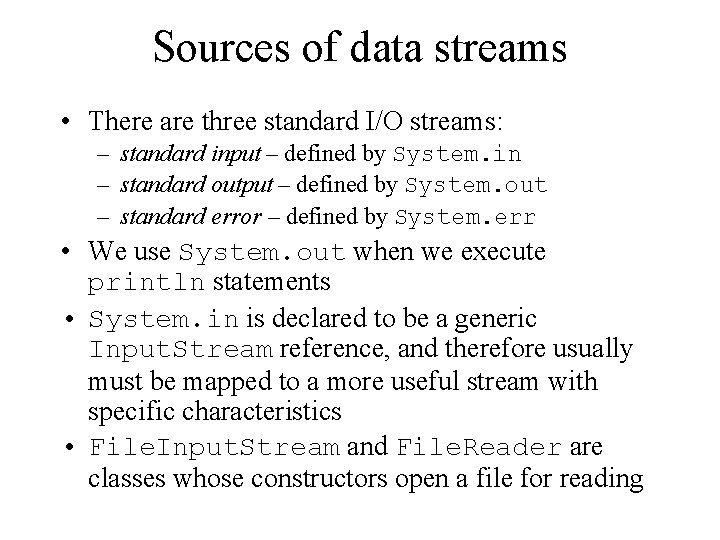
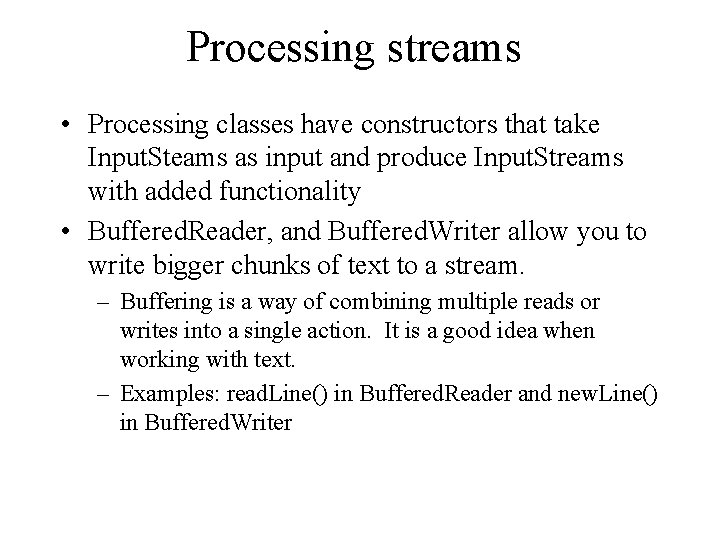
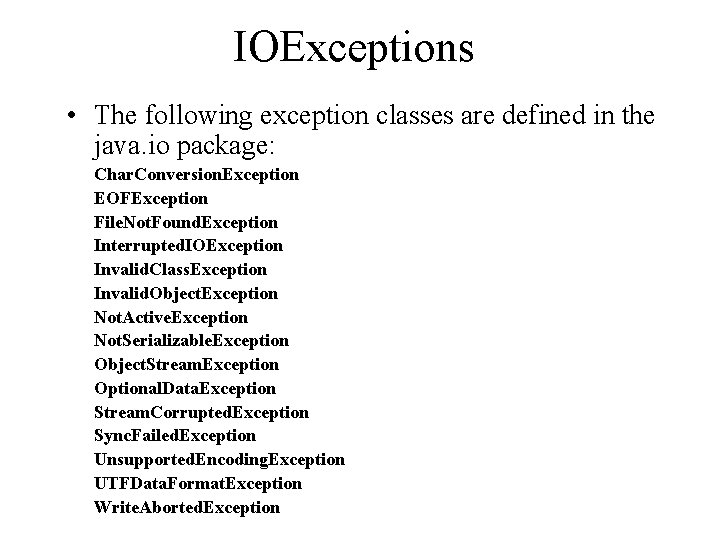
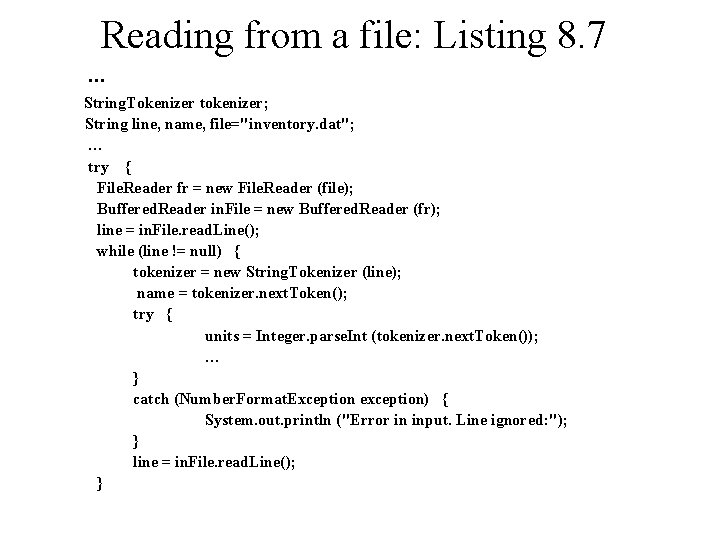
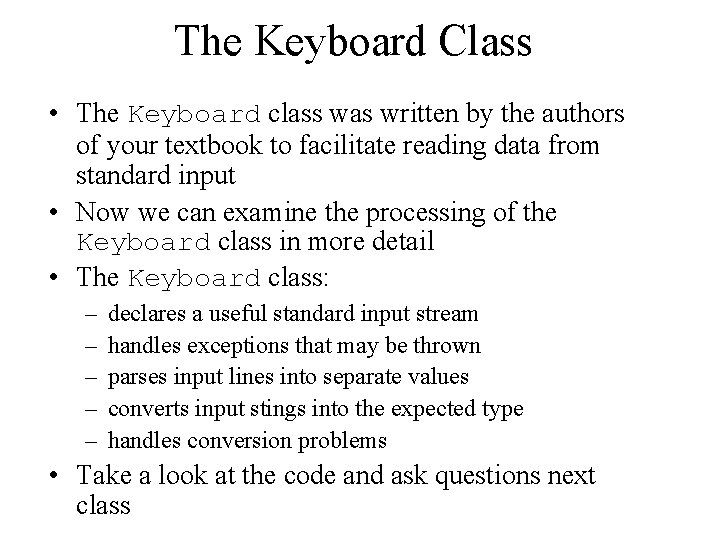
- Slides: 8
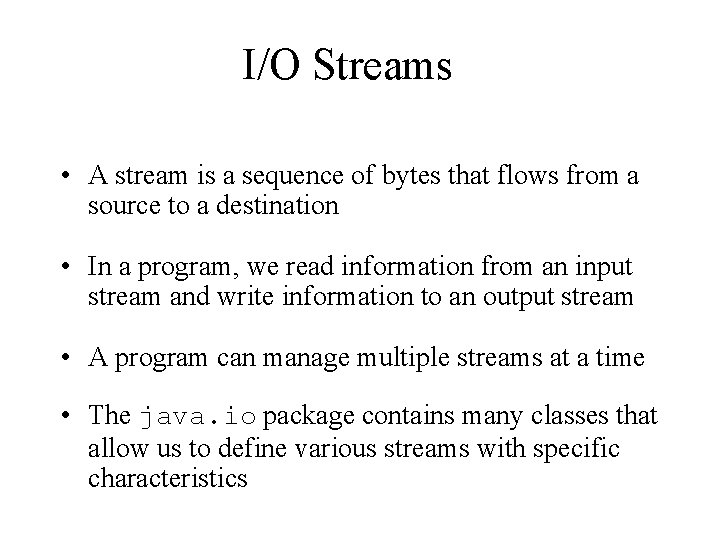
I/O Streams • A stream is a sequence of bytes that flows from a source to a destination • In a program, we read information from an input stream and write information to an output stream • A program can manage multiple streams at a time • The java. io package contains many classes that allow us to define various streams with specific characteristics
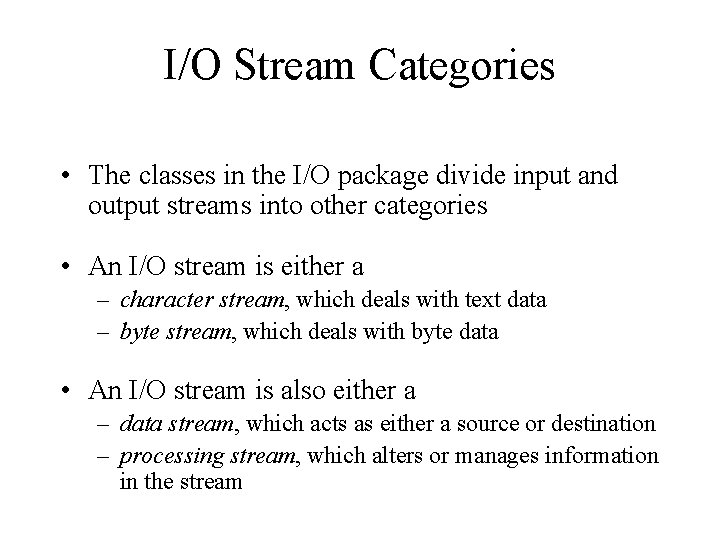
I/O Stream Categories • The classes in the I/O package divide input and output streams into other categories • An I/O stream is either a – character stream, which deals with text data – byte stream, which deals with byte data • An I/O stream is also either a – data stream, which acts as either a source or destination – processing stream, which alters or manages information in the stream
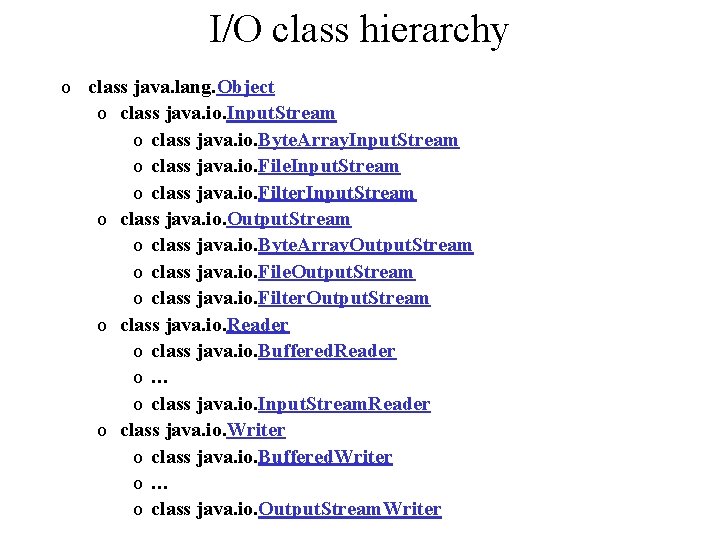
I/O class hierarchy o class java. lang. Object o class java. io. Input. Stream o class java. io. Byte. Array. Input. Stream o class java. io. File. Input. Stream o class java. io. Filter. Input. Stream o class java. io. Output. Stream o class java. io. Byte. Array. Output. Stream o class java. io. File. Output. Stream o class java. io. Filter. Output. Stream o class java. io. Reader o class java. io. Buffered. Reader o… o class java. io. Input. Stream. Reader o class java. io. Writer o class java. io. Buffered. Writer o… o class java. io. Output. Stream. Writer
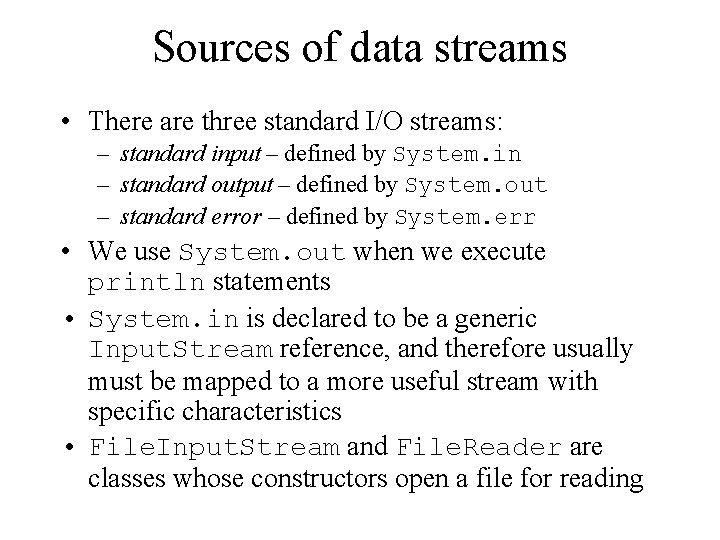
Sources of data streams • There are three standard I/O streams: – standard input – defined by System. in – standard output – defined by System. out – standard error – defined by System. err • We use System. out when we execute println statements • System. in is declared to be a generic Input. Stream reference, and therefore usually must be mapped to a more useful stream with specific characteristics • File. Input. Stream and File. Reader are classes whose constructors open a file for reading
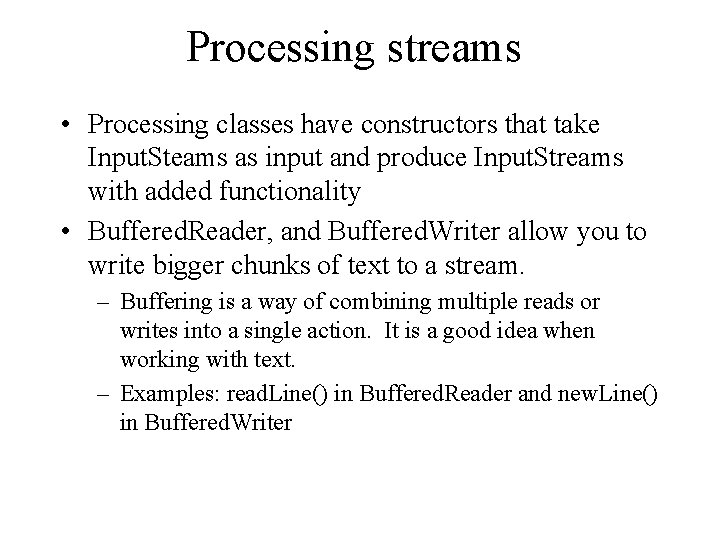
Processing streams • Processing classes have constructors that take Input. Steams as input and produce Input. Streams with added functionality • Buffered. Reader, and Buffered. Writer allow you to write bigger chunks of text to a stream. – Buffering is a way of combining multiple reads or writes into a single action. It is a good idea when working with text. – Examples: read. Line() in Buffered. Reader and new. Line() in Buffered. Writer
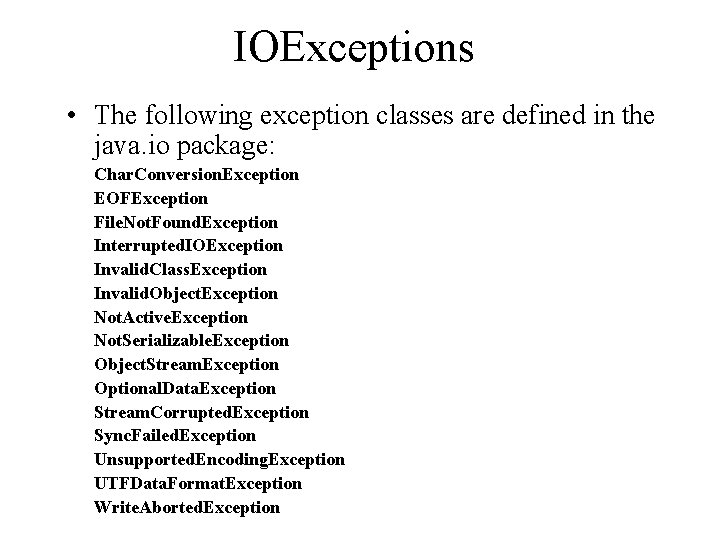
IOExceptions • The following exception classes are defined in the java. io package: Char. Conversion. Exception EOFException File. Not. Found. Exception Interrupted. IOException Invalid. Class. Exception Invalid. Object. Exception Not. Active. Exception Not. Serializable. Exception Object. Stream. Exception Optional. Data. Exception Stream. Corrupted. Exception Sync. Failed. Exception Unsupported. Encoding. Exception UTFData. Format. Exception Write. Aborted. Exception
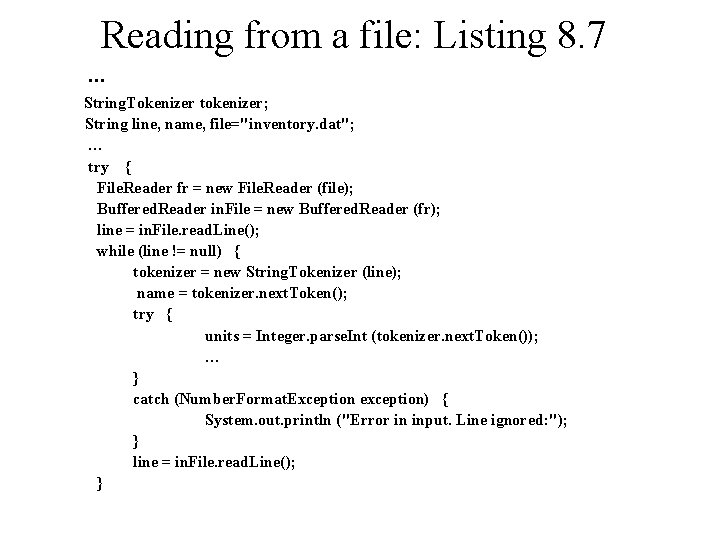
Reading from a file: Listing 8. 7 … String. Tokenizer tokenizer; String line, name, file="inventory. dat"; … try { File. Reader fr = new File. Reader (file); Buffered. Reader in. File = new Buffered. Reader (fr); line = in. File. read. Line(); while (line != null) { tokenizer = new String. Tokenizer (line); name = tokenizer. next. Token(); try { units = Integer. parse. Int (tokenizer. next. Token()); … } catch (Number. Format. Exception exception) { System. out. println ("Error in input. Line ignored: "); } line = in. File. read. Line(); }
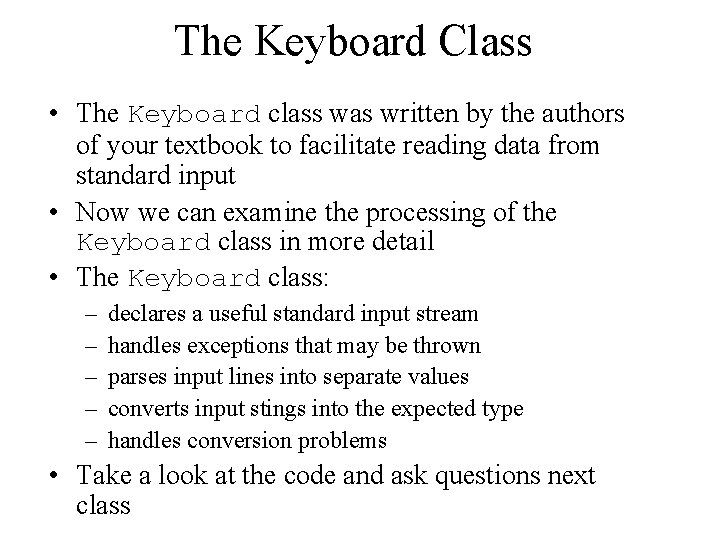
The Keyboard Class • The Keyboard class was written by the authors of your textbook to facilitate reading data from standard input • Now we can examine the processing of the Keyboard class in more detail • The Keyboard class: – – – declares a useful standard input stream handles exceptions that may be thrown parses input lines into separate values converts input stings into the expected type handles conversion problems • Take a look at the code and ask questions next class