Introduction to Software Testing Chapter 3 3 Logic
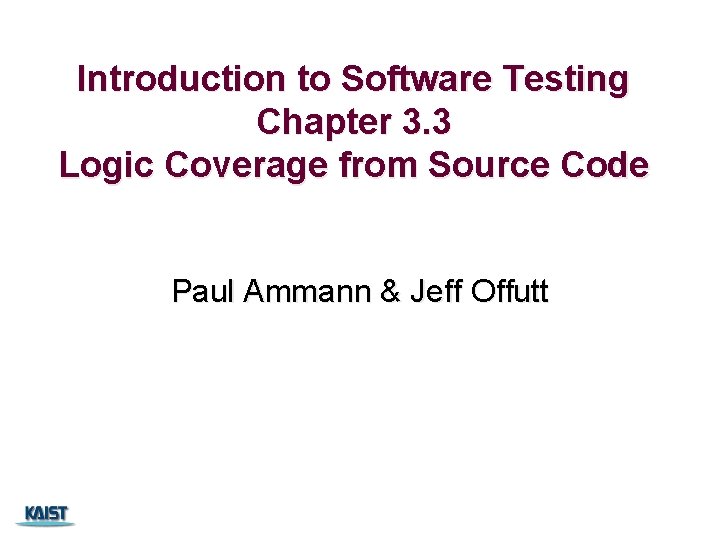
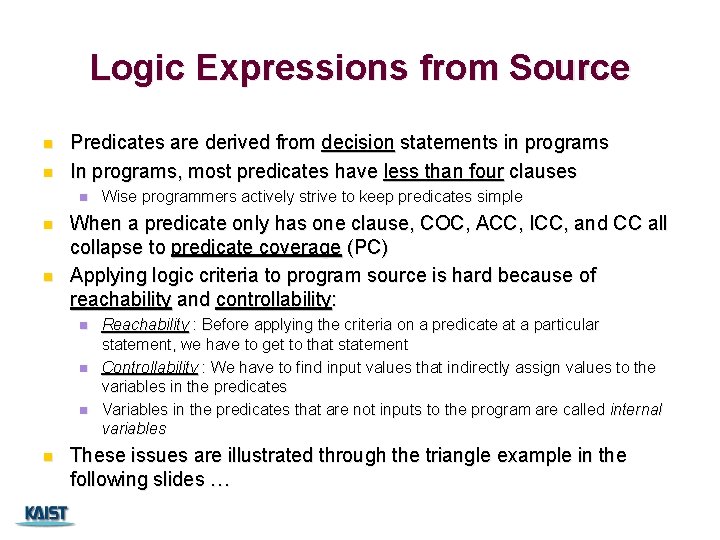
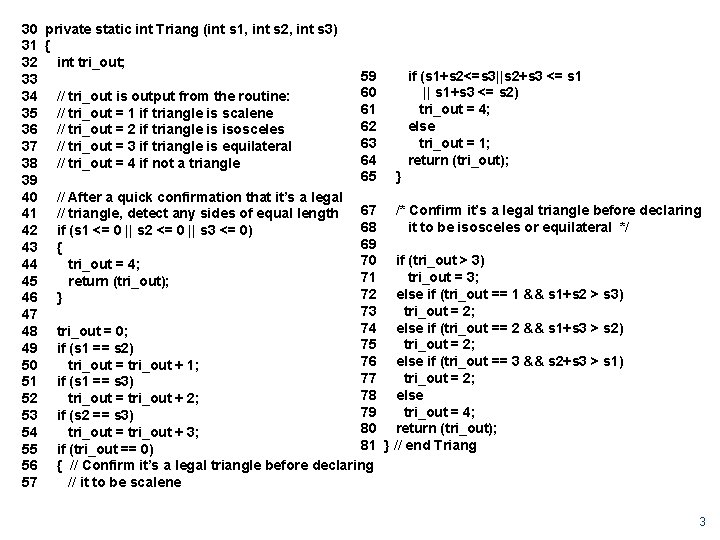
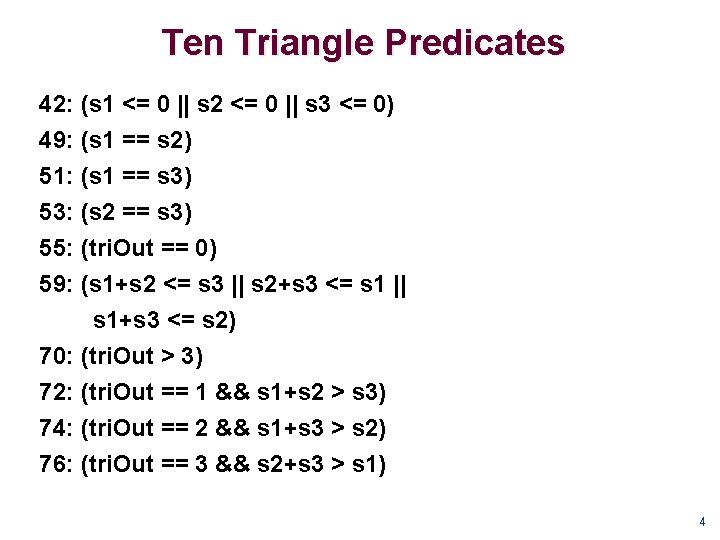
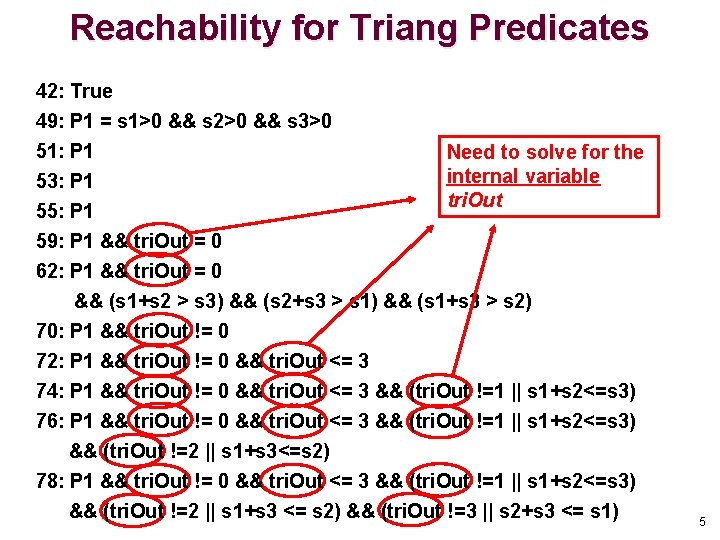
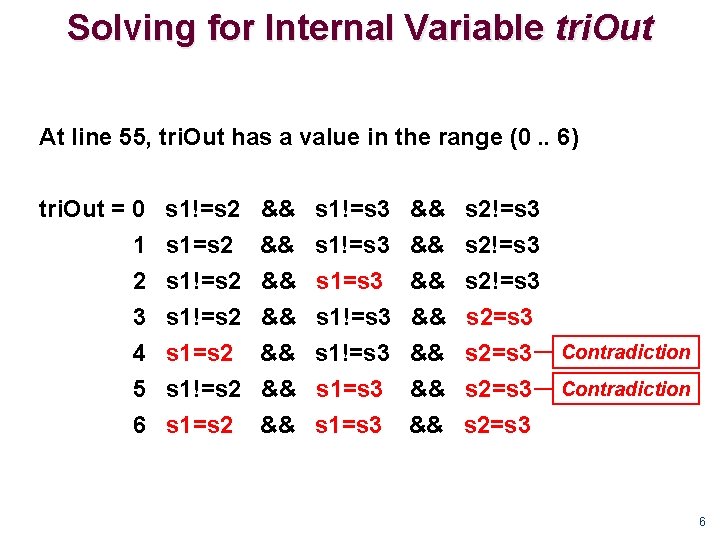
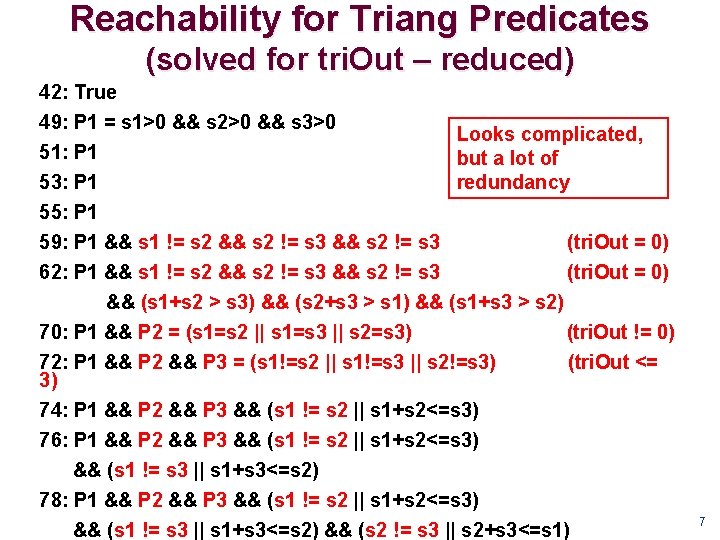
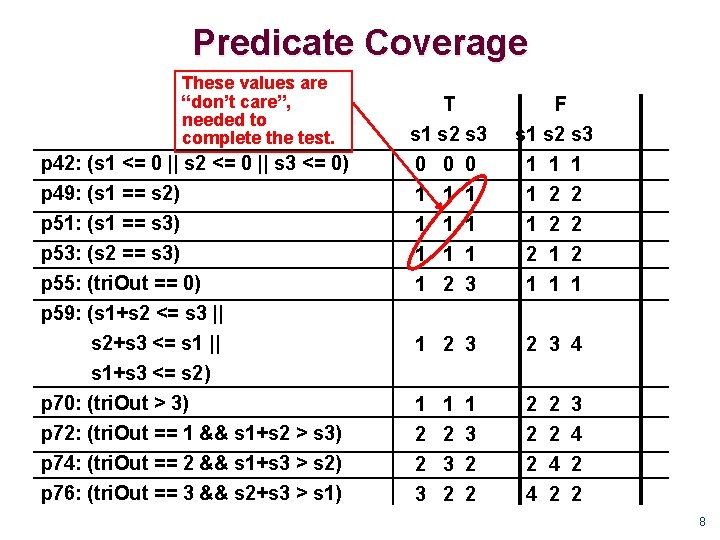
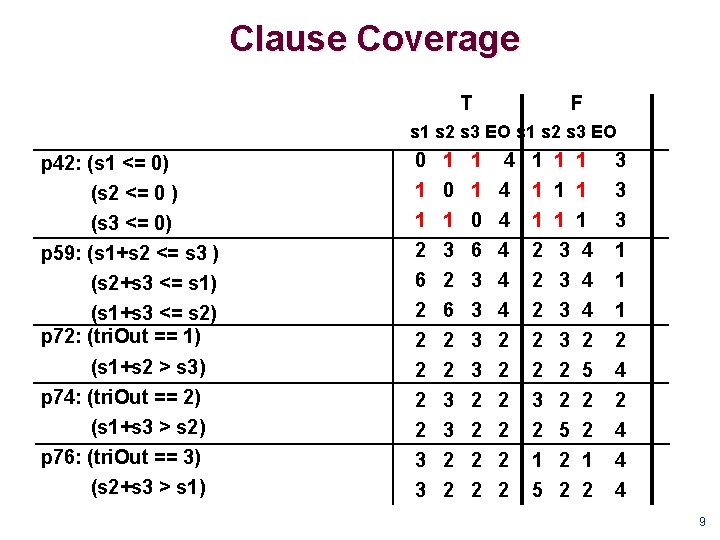
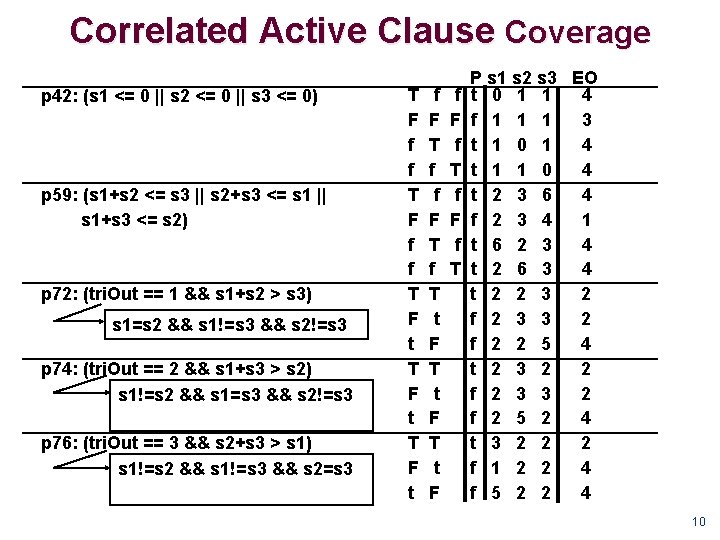
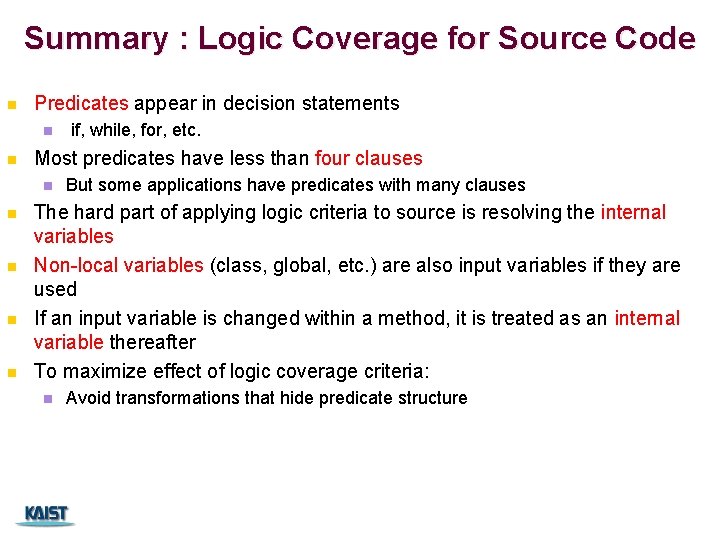
- Slides: 11
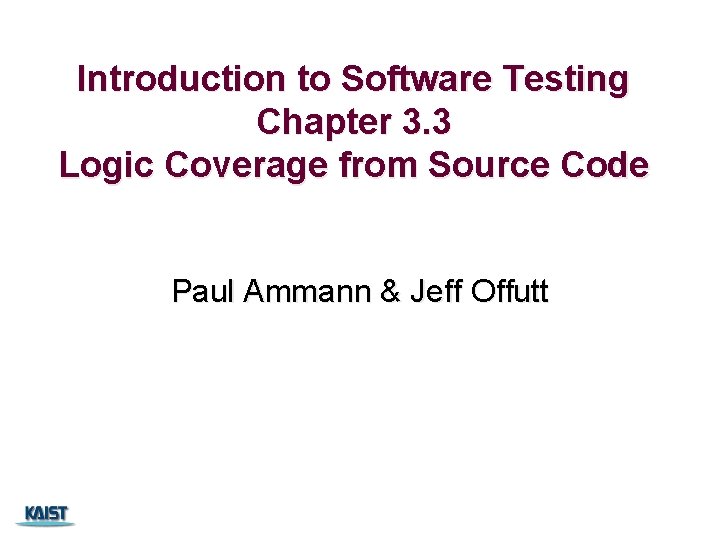
Introduction to Software Testing Chapter 3. 3 Logic Coverage from Source Code Paul Ammann & Jeff Offutt
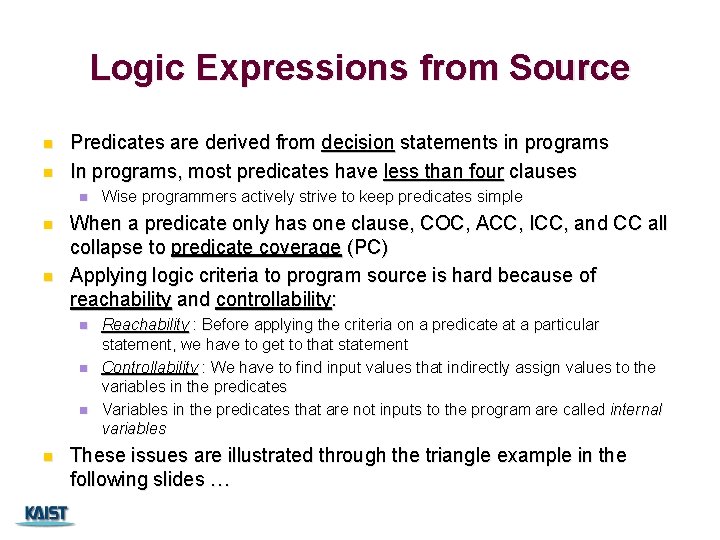
Logic Expressions from Source n n Predicates are derived from decision statements in programs In programs, most predicates have less than four clauses n n n When a predicate only has one clause, COC, ACC, ICC, and CC all collapse to predicate coverage (PC) Applying logic criteria to program source is hard because of reachability and controllability: n n Wise programmers actively strive to keep predicates simple Reachability : Before applying the criteria on a predicate at a particular statement, we have to get to that statement Controllability : We have to find input values that indirectly assign values to the variables in the predicates Variables in the predicates that are not inputs to the program are called internal variables These issues are illustrated through the triangle example in the following slides …
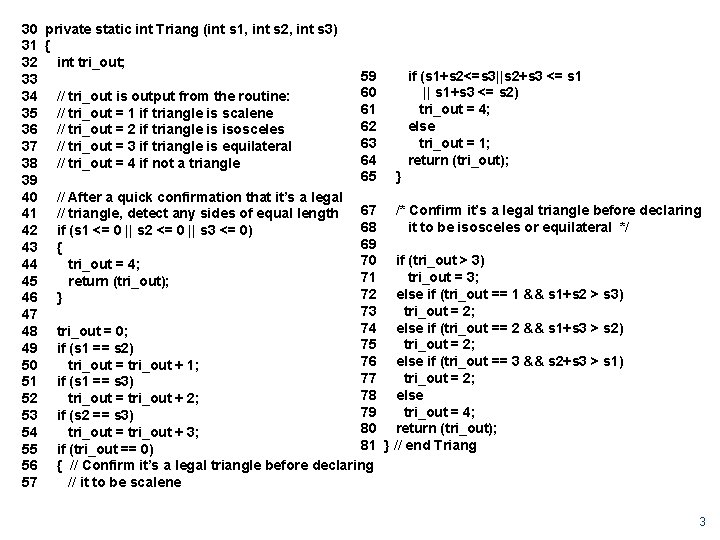
30 private static int Triang (int s 1, int s 2, int s 3) 31 { 32 int tri_out; 59 if (s 1+s 2<=s 3||s 2+s 3 <= s 1 33 60 || s 1+s 3 <= s 2) 34 // tri_out is output from the routine: 61 tri_out = 4; 35 // tri_out = 1 if triangle is scalene 62 else 36 // tri_out = 2 if triangle is isosceles 63 tri_out = 1; 37 // tri_out = 3 if triangle is equilateral 64 return (tri_out); 38 // tri_out = 4 if not a triangle 65 } 39 40 // After a quick confirmation that it’s a legal 67 /* Confirm it’s a legal triangle before declaring 41 // triangle, detect any sides of equal length 68 it to be isosceles or equilateral */ 42 if (s 1 <= 0 || s 2 <= 0 || s 3 <= 0) 69 43 { 70 if (tri_out > 3) 44 tri_out = 4; 71 tri_out = 3; 45 return (tri_out); 72 else if (tri_out == 1 && s 1+s 2 > s 3) 46 } 73 tri_out = 2; 47 74 else if (tri_out == 2 && s 1+s 3 > s 2) 48 tri_out = 0; 75 tri_out = 2; 49 if (s 1 == s 2) 76 else if (tri_out == 3 && s 2+s 3 > s 1) 50 tri_out = tri_out + 1; 77 tri_out = 2; 51 if (s 1 == s 3) 78 else 52 tri_out = tri_out + 2; 79 tri_out = 4; 53 if (s 2 == s 3) 80 return (tri_out); 54 tri_out = tri_out + 3; 81 } // end Triang 55 if (tri_out == 0) 56 { // Confirm it’s a legal triangle before declaring 57 // it to be scalene 3
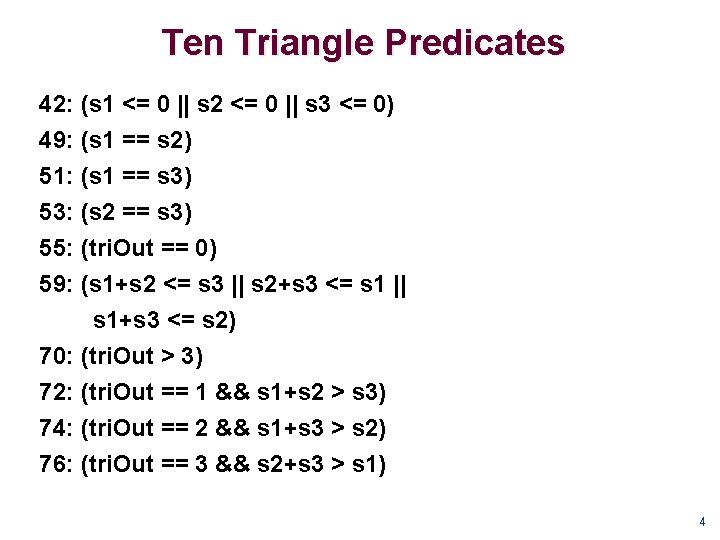
Ten Triangle Predicates 42: (s 1 <= 0 || s 2 <= 0 || s 3 <= 0) 49: (s 1 == s 2) 51: (s 1 == s 3) 53: (s 2 == s 3) 55: (tri. Out == 0) 59: (s 1+s 2 <= s 3 || s 2+s 3 <= s 1 || s 1+s 3 <= s 2) 70: (tri. Out > 3) 72: (tri. Out == 1 && s 1+s 2 > s 3) 74: (tri. Out == 2 && s 1+s 3 > s 2) 76: (tri. Out == 3 && s 2+s 3 > s 1) 4
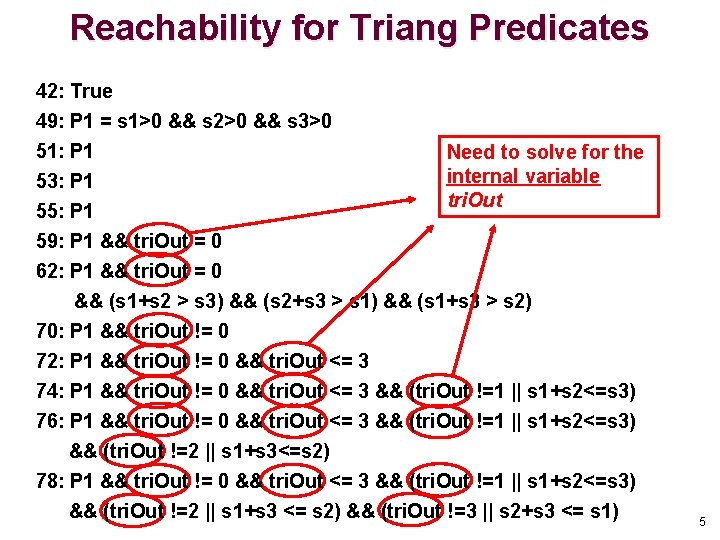
Reachability for Triang Predicates 42: True 49: P 1 = s 1>0 && s 2>0 && s 3>0 51: P 1 Need to solve for the internal variable 53: P 1 tri. Out 55: P 1 59: P 1 && tri. Out = 0 62: P 1 && tri. Out = 0 && (s 1+s 2 > s 3) && (s 2+s 3 > s 1) && (s 1+s 3 > s 2) 70: P 1 && tri. Out != 0 72: P 1 && tri. Out != 0 && tri. Out <= 3 74: P 1 && tri. Out != 0 && tri. Out <= 3 && (tri. Out !=1 || s 1+s 2<=s 3) 76: P 1 && tri. Out != 0 && tri. Out <= 3 && (tri. Out !=1 || s 1+s 2<=s 3) && (tri. Out !=2 || s 1+s 3<=s 2) 78: P 1 && tri. Out != 0 && tri. Out <= 3 && (tri. Out !=1 || s 1+s 2<=s 3) && (tri. Out !=2 || s 1+s 3 <= s 2) && (tri. Out !=3 || s 2+s 3 <= s 1) 5
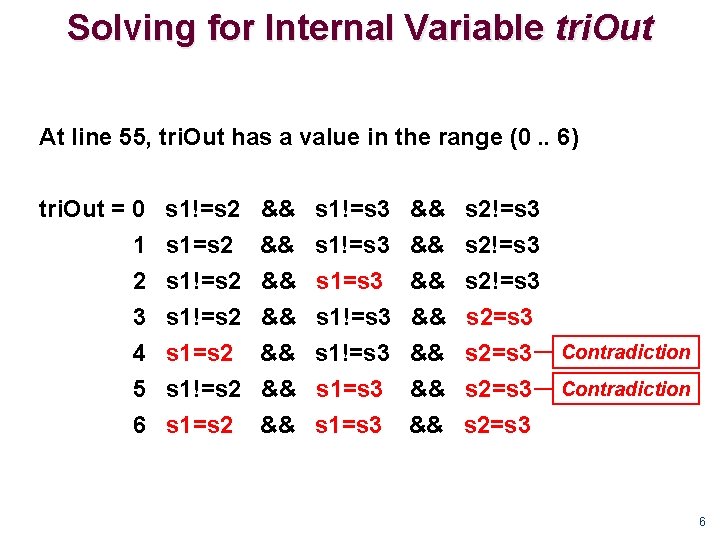
Solving for Internal Variable tri. Out At line 55, tri. Out has a value in the range (0. . 6) tri. Out = 0 1 2 3 4 5 6 s 1!=s 2 s 1=s 2 && && s 1!=s 3 s 1=s 3 && && s 2!=s 3 s 2=s 3 Contradiction s 2=s 3 6
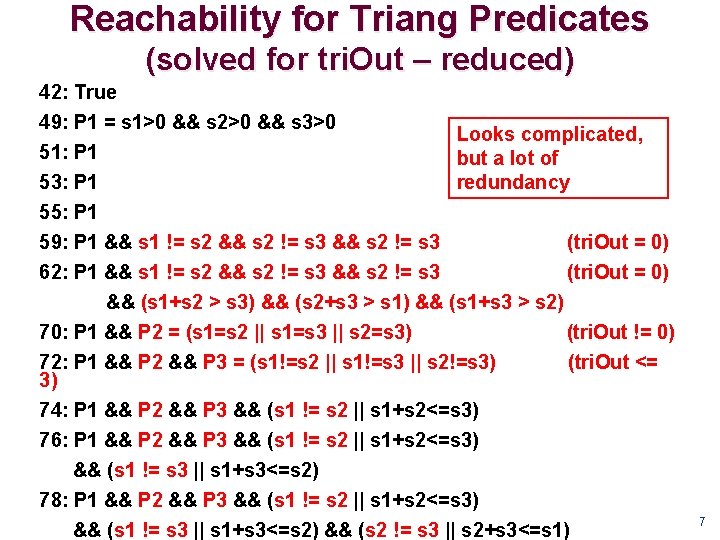
Reachability for Triang Predicates (solved for tri. Out – reduced) 42: True 49: P 1 = s 1>0 && s 2>0 && s 3>0 Looks complicated, 51: P 1 but a lot of redundancy 53: P 1 55: P 1 59: P 1 && s 1 != s 2 && s 2 != s 3 (tri. Out = 0) 62: P 1 && s 1 != s 2 && s 2 != s 3 (tri. Out = 0) && (s 1+s 2 > s 3) && (s 2+s 3 > s 1) && (s 1+s 3 > s 2) 70: P 1 && P 2 = (s 1=s 2 || s 1=s 3 || s 2=s 3) (tri. Out != 0) 72: P 1 && P 2 && P 3 = (s 1!=s 2 || s 1!=s 3 || s 2!=s 3) (tri. Out <= 3) 74: P 1 && P 2 && P 3 && (s 1 != s 2 || s 1+s 2<=s 3) 76: P 1 && P 2 && P 3 && (s 1 != s 2 || s 1+s 2<=s 3) && (s 1 != s 3 || s 1+s 3<=s 2) 78: P 1 && P 2 && P 3 && (s 1 != s 2 || s 1+s 2<=s 3) && (s 1 != s 3 || s 1+s 3<=s 2) && (s 2 != s 3 || s 2+s 3<=s 1) 7
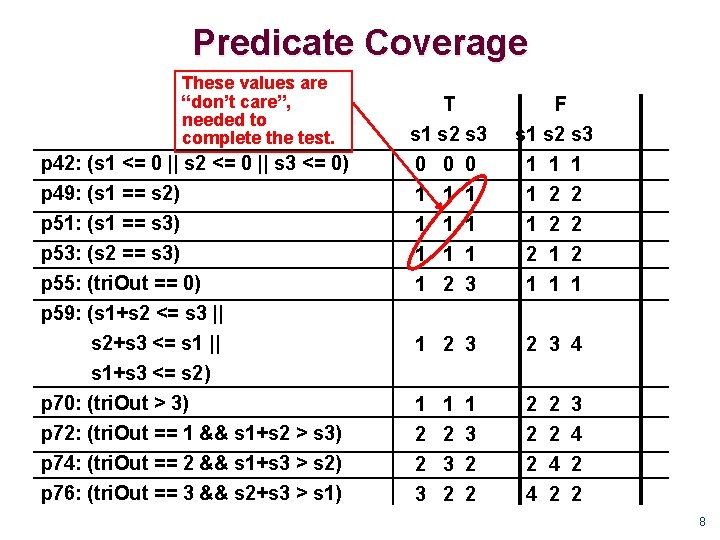
Predicate Coverage These values are “don’t care”, needed to complete the test. p 42: (s 1 <= 0 || s 2 <= 0 || s 3 <= 0) p 49: (s 1 == s 2) p 51: (s 1 == s 3) p 53: (s 2 == s 3) p 55: (tri. Out == 0) p 59: (s 1+s 2 <= s 3 || s 2+s 3 <= s 1 || s 1+s 3 <= s 2) p 70: (tri. Out > 3) p 72: (tri. Out == 1 && s 1+s 2 > s 3) p 74: (tri. Out == 2 && s 1+s 3 > s 2) p 76: (tri. Out == 3 && s 2+s 3 > s 1) T s 1 s 2 s 3 0 0 0 1 1 1 1 1 2 3 F s 1 s 2 s 3 1 1 2 2 1 2 1 1 2 3 4 1 2 2 3 2 2 2 4 1 2 3 2 1 3 2 2 4 2 3 4 2 2 8
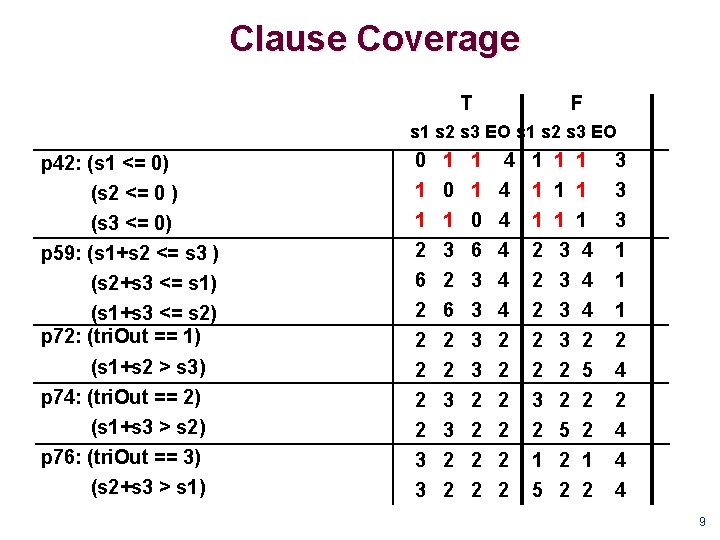
Clause Coverage T F s 1 s 2 s 3 EO p 42: (s 1 <= 0) (s 2 <= 0 ) (s 3 <= 0) p 59: (s 1+s 2 <= s 3 ) (s 2+s 3 <= s 1) (s 1+s 3 <= s 2) p 72: (tri. Out == 1) (s 1+s 2 > s 3) p 74: (tri. Out == 2) (s 1+s 3 > s 2) p 76: (tri. Out == 3) (s 2+s 3 > s 1) 0 1 1 2 6 2 2 2 3 3 1 0 1 3 2 6 2 2 3 3 2 2 1 1 0 6 3 3 2 2 4 4 4 2 2 2 1 1 1 2 2 2 3 2 1 5 1 1 1 3 4 3 4 3 2 2 5 2 2 1 2 2 3 3 3 1 1 1 2 4 4 4 9
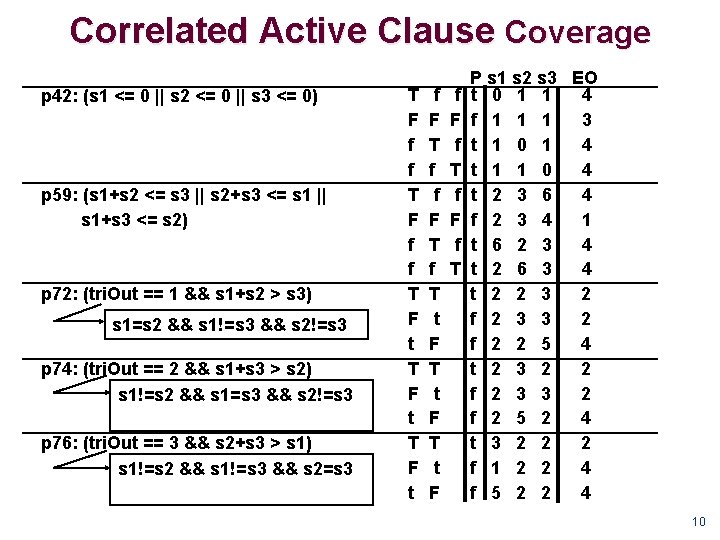
Correlated Active Clause Coverage p 42: (s 1 <= 0 || s 2 <= 0 || s 3 <= 0) p 59: (s 1+s 2 <= s 3 || s 2+s 3 <= s 1 || s 1+s 3 <= s 2) p 72: (tri. Out == 1 && s 1+s 2 > s 3) s 1=s 2 && s 1!=s 3 && s 2!=s 3 p 74: (tri. Out == 2 && s 1+s 3 > s 2) s 1!=s 2 && s 1=s 3 && s 2!=s 3 p 76: (tri. Out == 3 && s 2+s 3 > s 1) s 1!=s 2 && s 1!=s 3 && s 2=s 3 T F f f T F t f F T f T t F f T f F f T P s 1 s 2 s 3 EO t 0 1 1 4 f 1 1 1 3 t 1 0 1 4 t 1 1 0 4 t 2 3 6 4 f 2 3 4 1 t 6 2 3 4 t 2 6 3 4 t 2 2 3 2 f 2 3 3 2 f 2 2 5 4 t 2 3 2 2 f 2 3 3 2 f 2 5 2 4 t 3 2 2 2 f 1 2 2 4 f 5 2 2 4 10
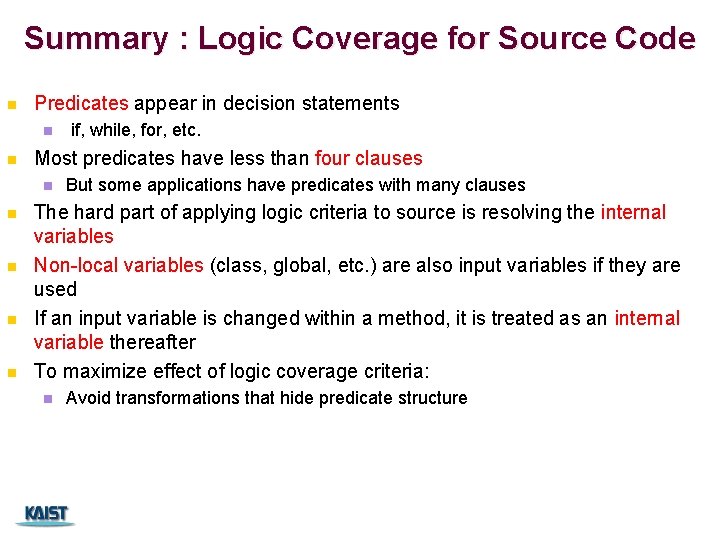
Summary : Logic Coverage for Source Code n Predicates appear in decision statements n n Most predicates have less than four clauses n n n if, while, for, etc. But some applications have predicates with many clauses The hard part of applying logic criteria to source is resolving the internal variables Non-local variables (class, global, etc. ) are also input variables if they are used If an input variable is changed within a method, it is treated as an internal variable thereafter To maximize effect of logic coverage criteria: n Avoid transformations that hide predicate structure 11
Logic based testing
Domain closure in software testing
Du path testing
Globalization testing in software testing
Language testing
Control structure testing in software engineering
Decision table testing in software testing
Decision table testing in software testing
Limited entry decision table
Rigorous testing in software testing
Testing blindness in software testing
Software domain examples