Introduction to programming in java Lecture 21 Arrays
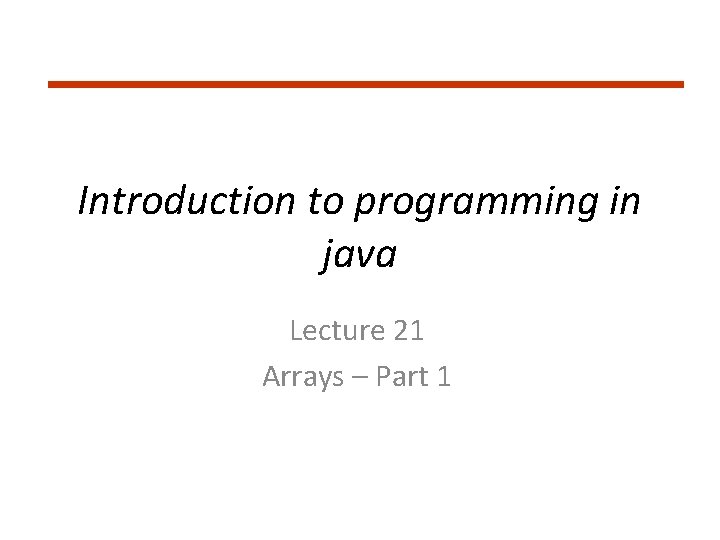
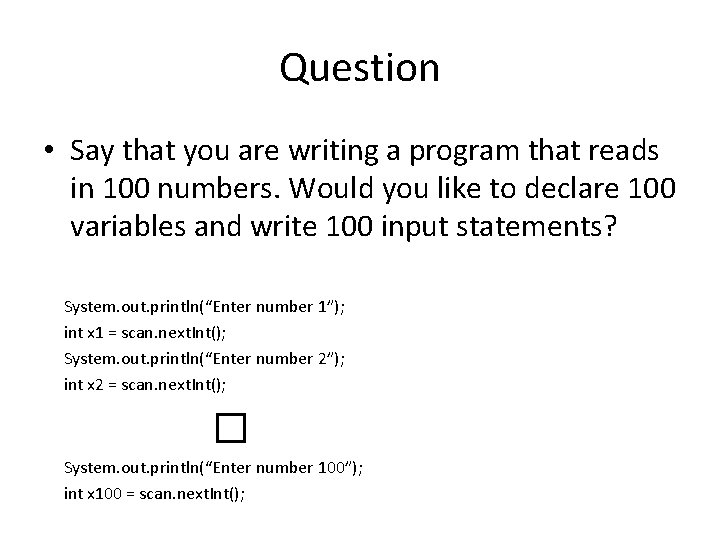
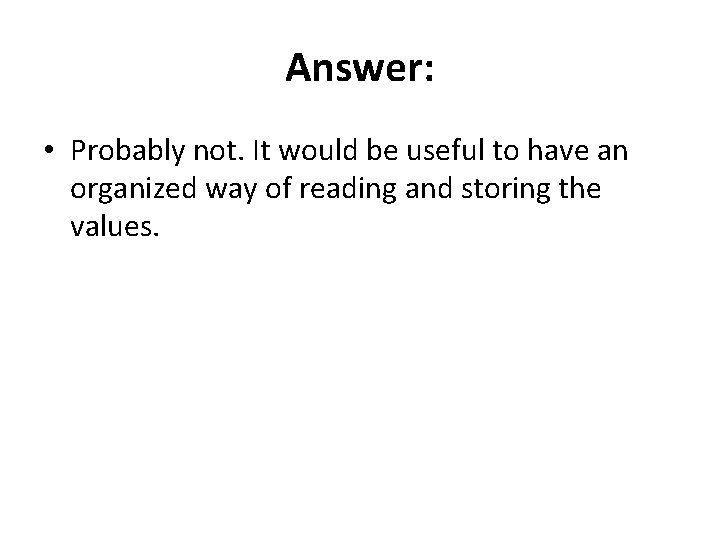
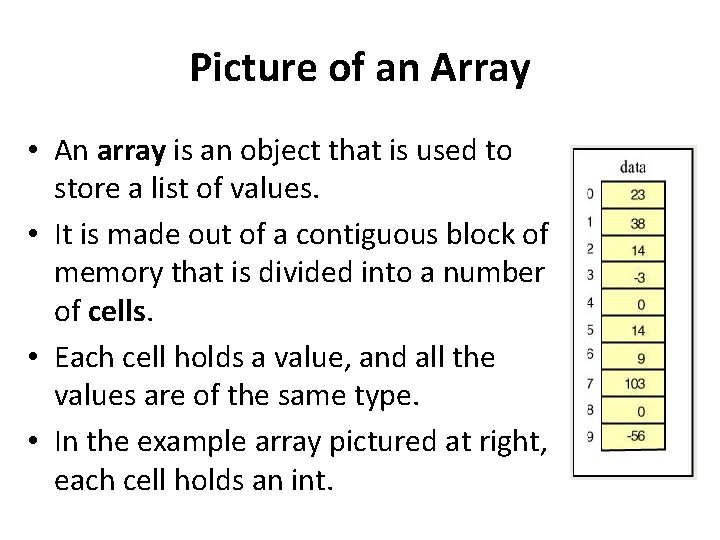
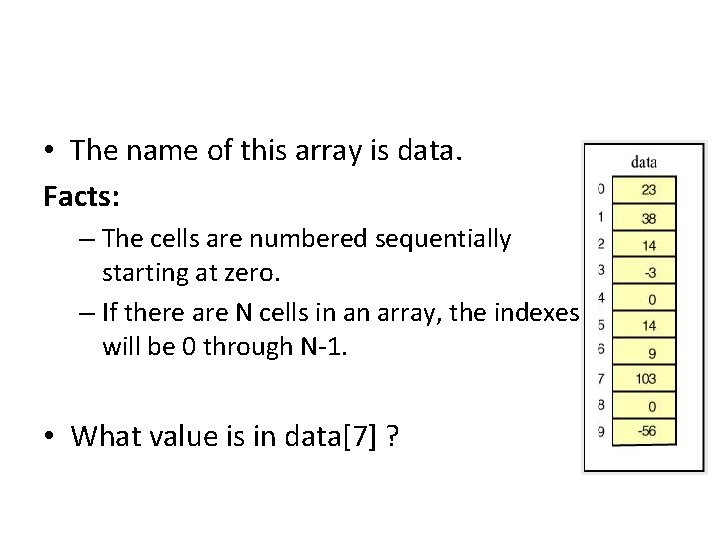
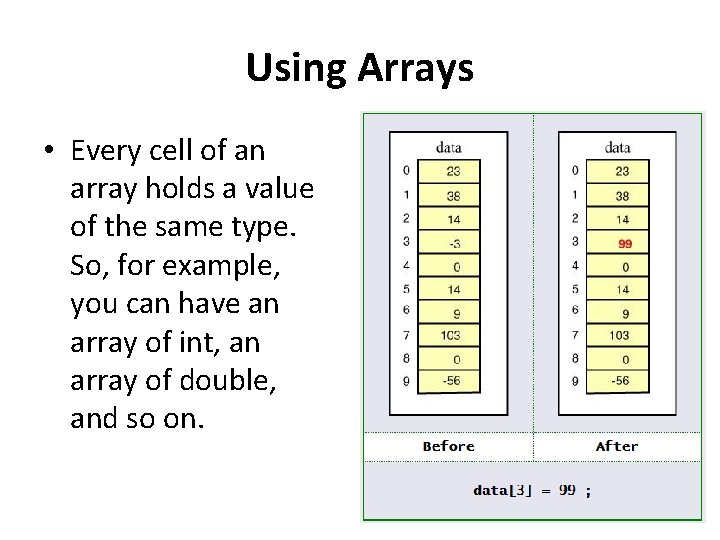
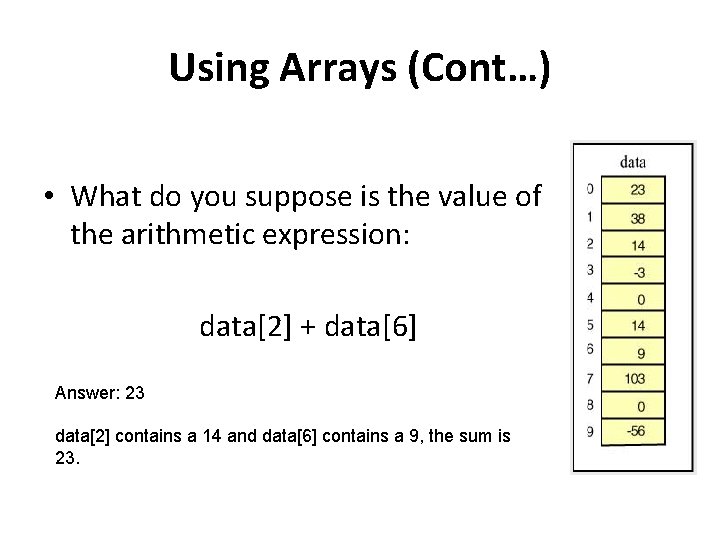
![How to declare Array • Syntax type[] array. Name = new type[ length ]; How to declare Array • Syntax type[] array. Name = new type[ length ];](https://slidetodoc.com/presentation_image_h/c54b8c72b14b23b25423ef80660ba280/image-8.jpg)
![Bounds Checking Example: int[] data = new int[10]; Bounds Checking Example: int[] data = new int[10];](https://slidetodoc.com/presentation_image_h/c54b8c72b14b23b25423ef80660ba280/image-9.jpg)
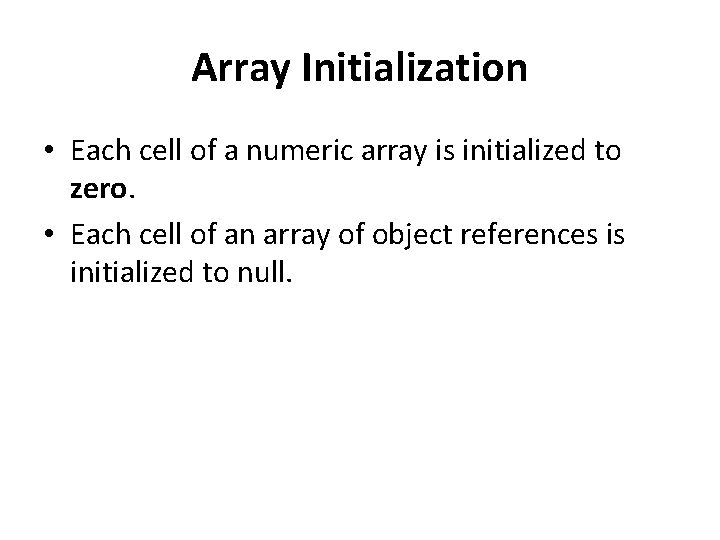
![What is the output of the following program? stuff[0] has 23 stuff[1] has 38 What is the output of the following program? stuff[0] has 23 stuff[1] has 38](https://slidetodoc.com/presentation_image_h/c54b8c72b14b23b25423ef80660ba280/image-11.jpg)
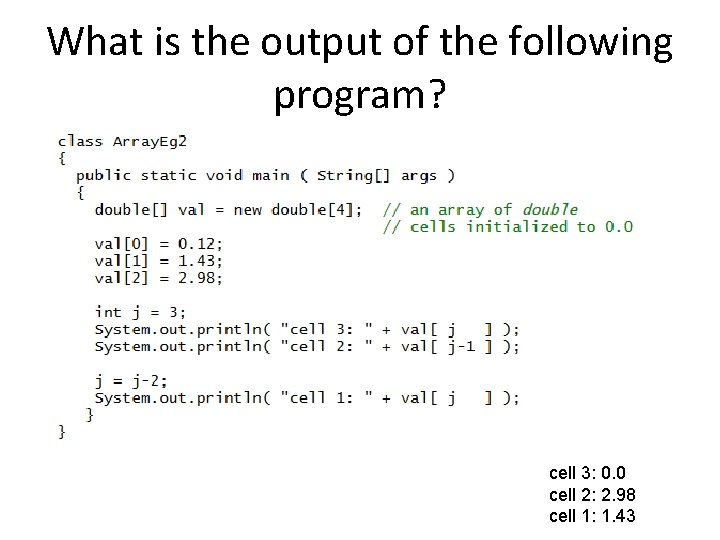
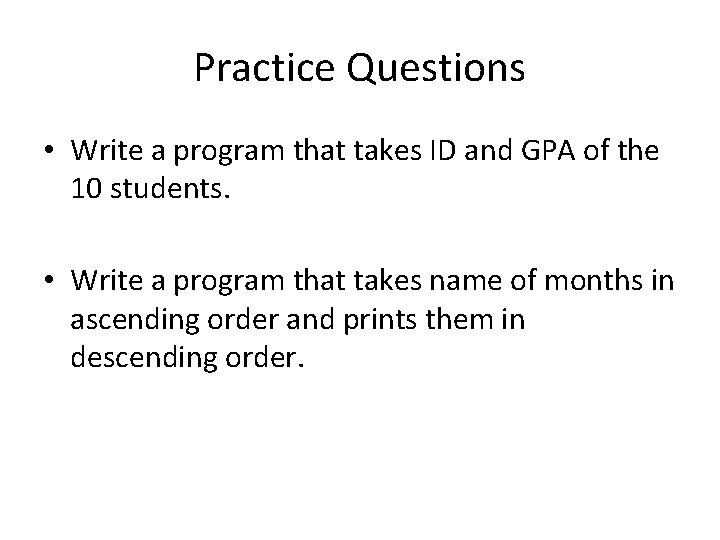
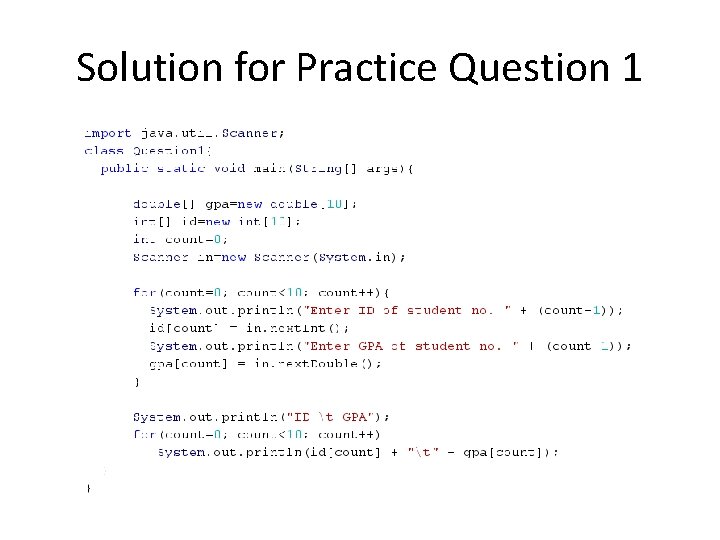
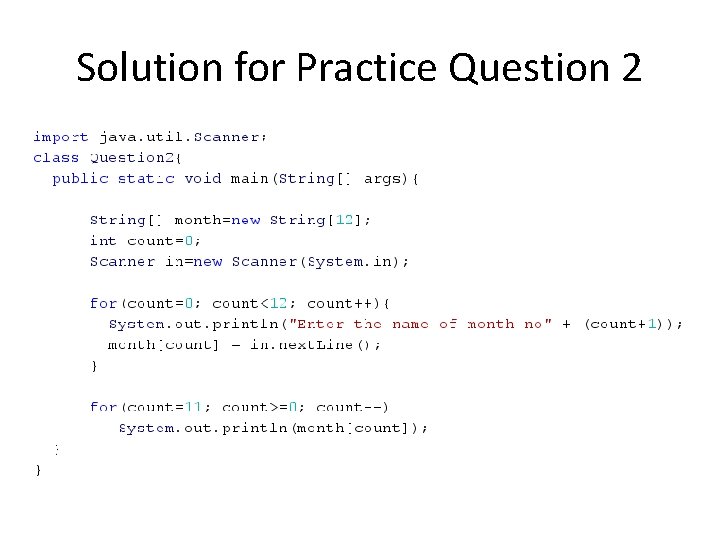
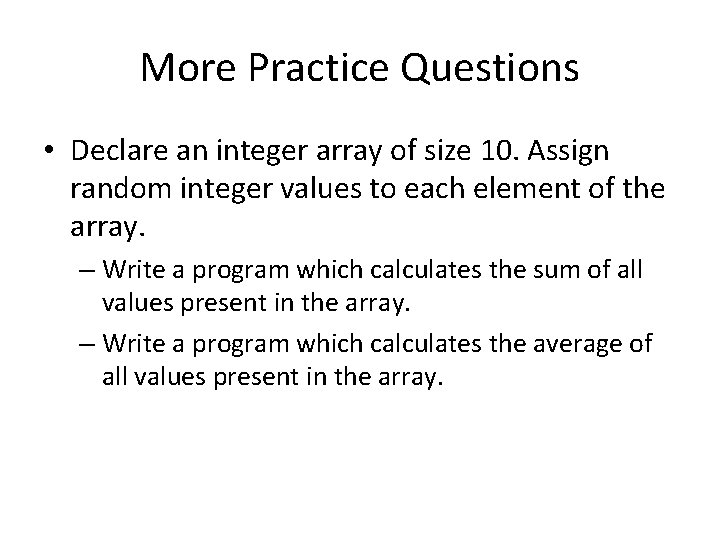
- Slides: 16
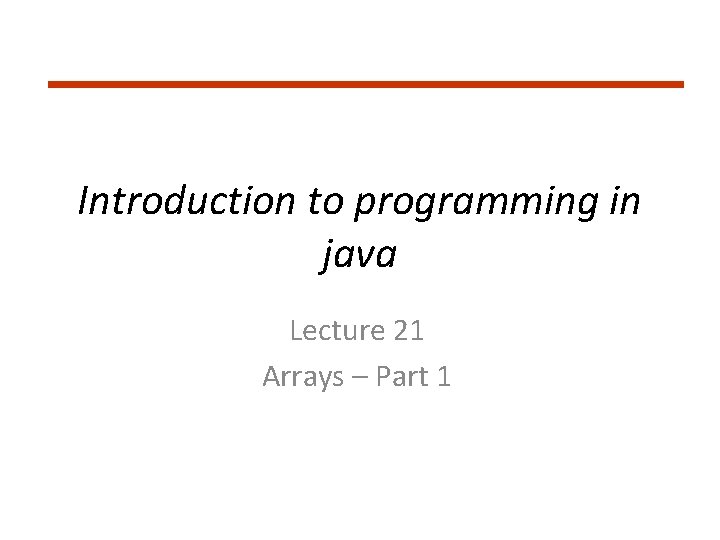
Introduction to programming in java Lecture 21 Arrays – Part 1
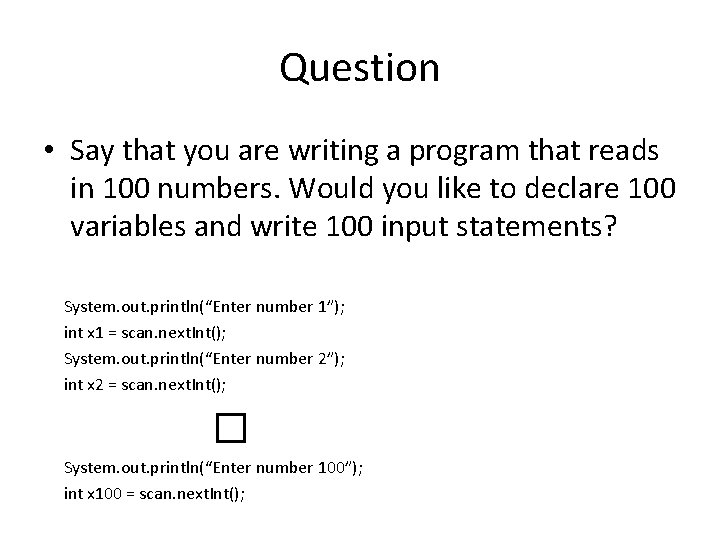
Question • Say that you are writing a program that reads in 100 numbers. Would you like to declare 100 variables and write 100 input statements? System. out. println(“Enter number 1”); int x 1 = scan. next. Int(); System. out. println(“Enter number 2”); int x 2 = scan. next. Int(); � System. out. println(“Enter number 100”); int x 100 = scan. next. Int();
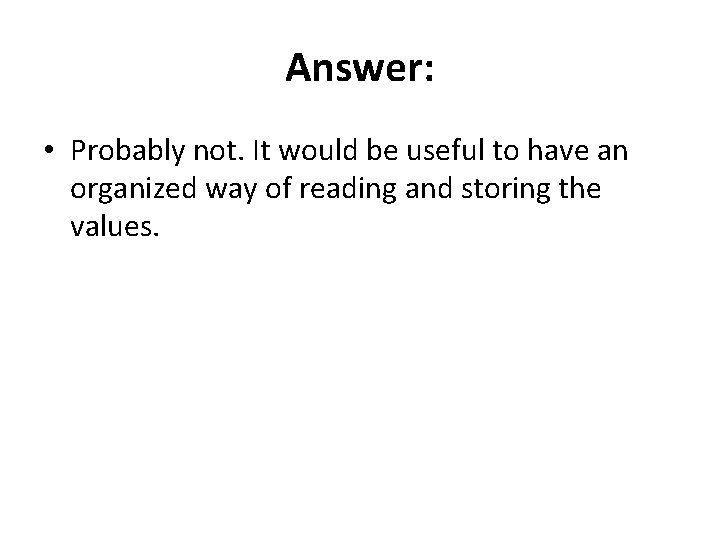
Answer: • Probably not. It would be useful to have an organized way of reading and storing the values.
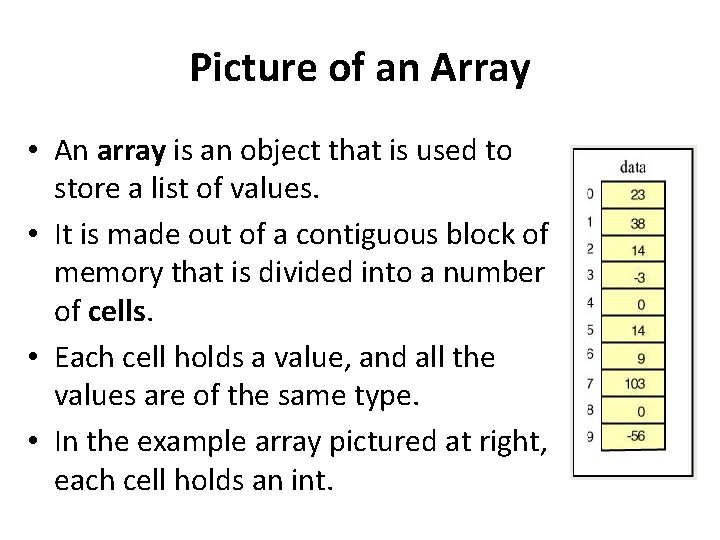
Picture of an Array • An array is an object that is used to store a list of values. • It is made out of a contiguous block of memory that is divided into a number of cells. • Each cell holds a value, and all the values are of the same type. • In the example array pictured at right, each cell holds an int.
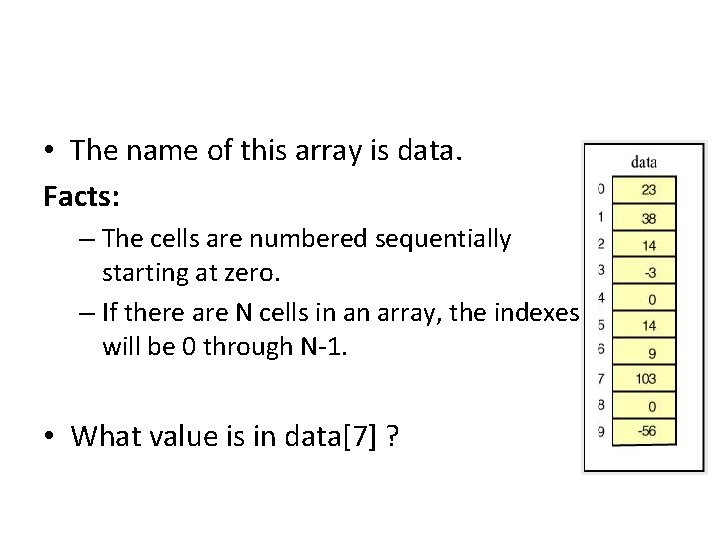
• The name of this array is data. Facts: – The cells are numbered sequentially starting at zero. – If there are N cells in an array, the indexes will be 0 through N-1. • What value is in data[7] ?
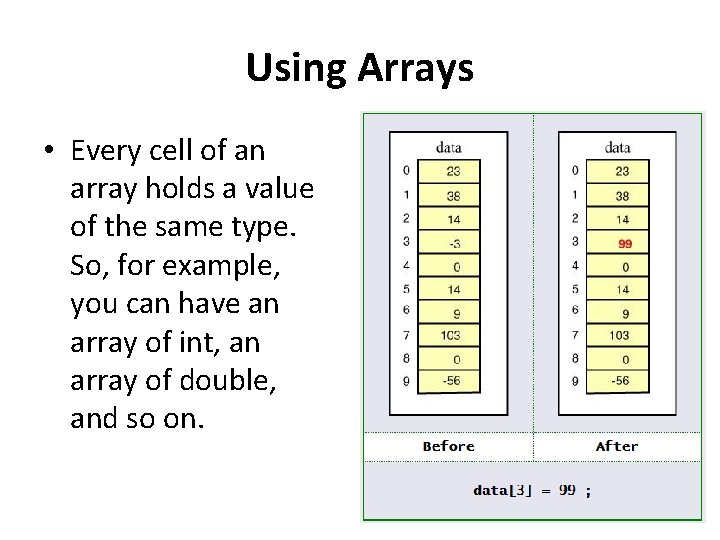
Using Arrays • Every cell of an array holds a value of the same type. So, for example, you can have an array of int, an array of double, and so on.
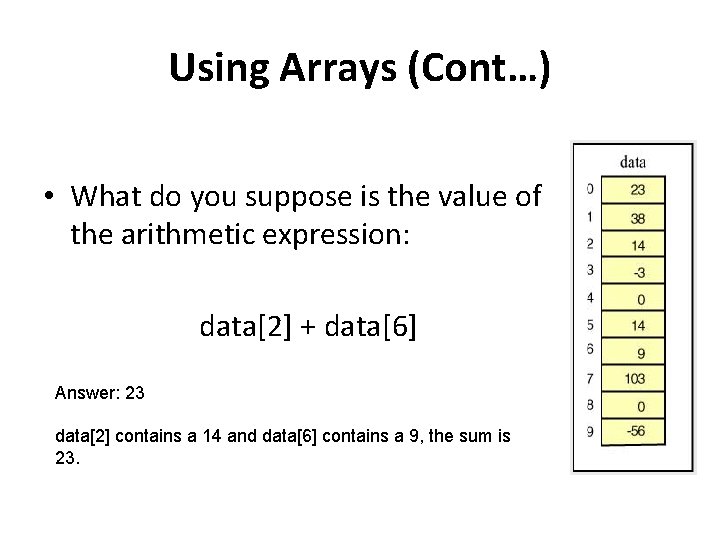
Using Arrays (Cont…) • What do you suppose is the value of the arithmetic expression: data[2] + data[6] Answer: 23 data[2] contains a 14 and data[6] contains a 9, the sum is 23.
![How to declare Array Syntax type array Name new type length How to declare Array • Syntax type[] array. Name = new type[ length ];](https://slidetodoc.com/presentation_image_h/c54b8c72b14b23b25423ef80660ba280/image-8.jpg)
How to declare Array • Syntax type[] array. Name = new type[ length ]; Examples: – int[ ] data = new int[10]; – double[] scores=new double[100]; – String[] names = new String[20]; – char[] alphabets = new char[24];
![Bounds Checking Example int data new int10 Bounds Checking Example: int[] data = new int[10];](https://slidetodoc.com/presentation_image_h/c54b8c72b14b23b25423ef80660ba280/image-9.jpg)
Bounds Checking Example: int[] data = new int[10];
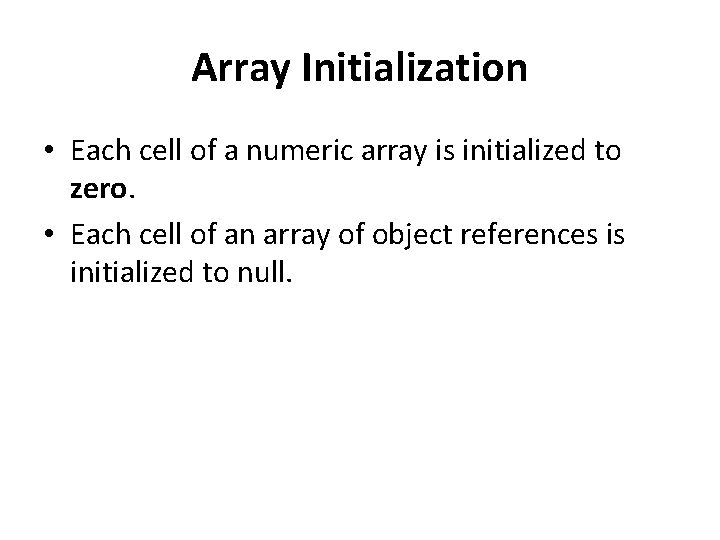
Array Initialization • Each cell of a numeric array is initialized to zero. • Each cell of an array of object references is initialized to null.
![What is the output of the following program stuff0 has 23 stuff1 has 38 What is the output of the following program? stuff[0] has 23 stuff[1] has 38](https://slidetodoc.com/presentation_image_h/c54b8c72b14b23b25423ef80660ba280/image-11.jpg)
What is the output of the following program? stuff[0] has 23 stuff[1] has 38 stuff[2] has 14 stuff[3] has 0 stuff[4] has 0
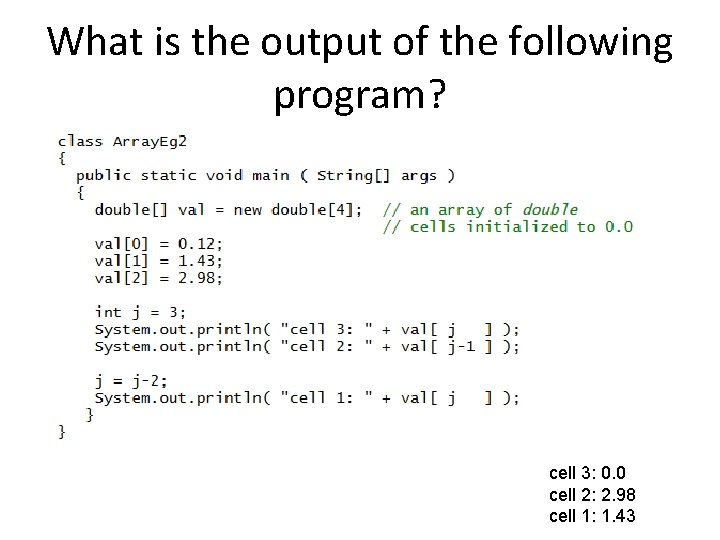
What is the output of the following program? cell 3: 0. 0 cell 2: 2. 98 cell 1: 1. 43
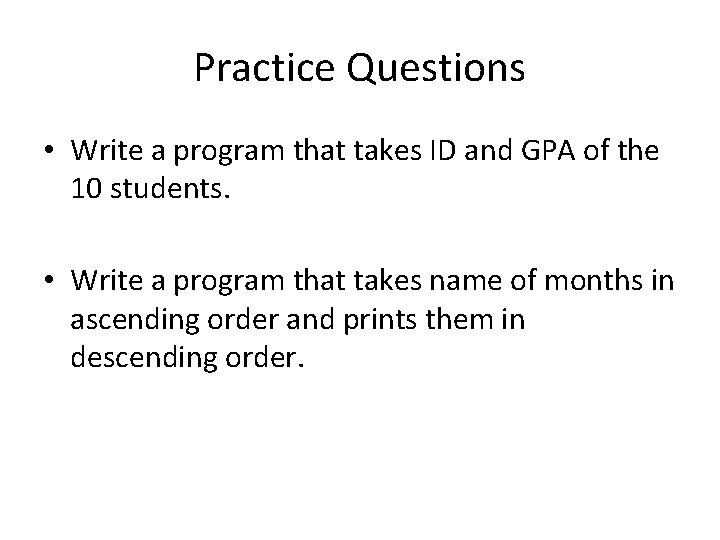
Practice Questions • Write a program that takes ID and GPA of the 10 students. • Write a program that takes name of months in ascending order and prints them in descending order.
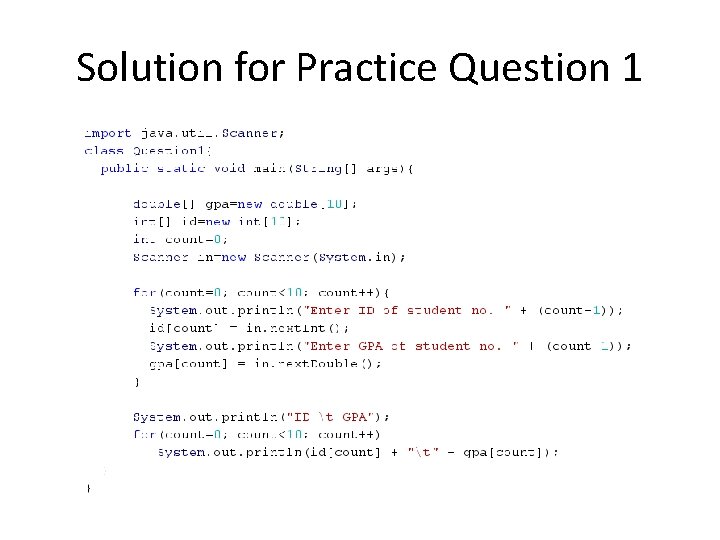
Solution for Practice Question 1
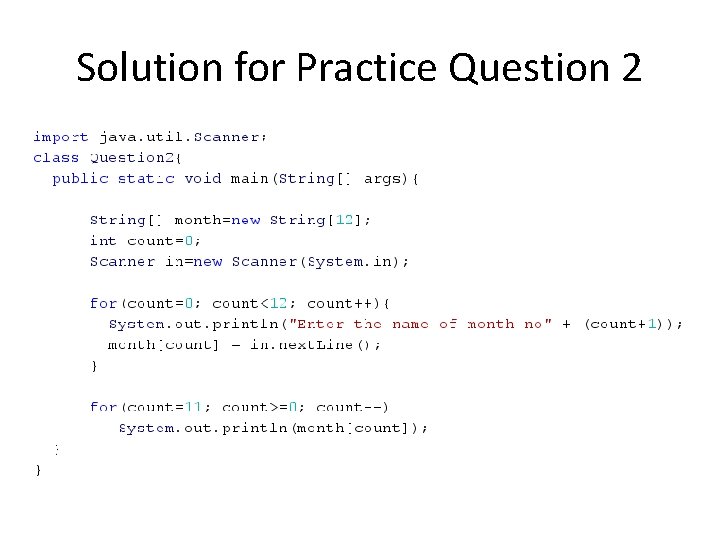
Solution for Practice Question 2
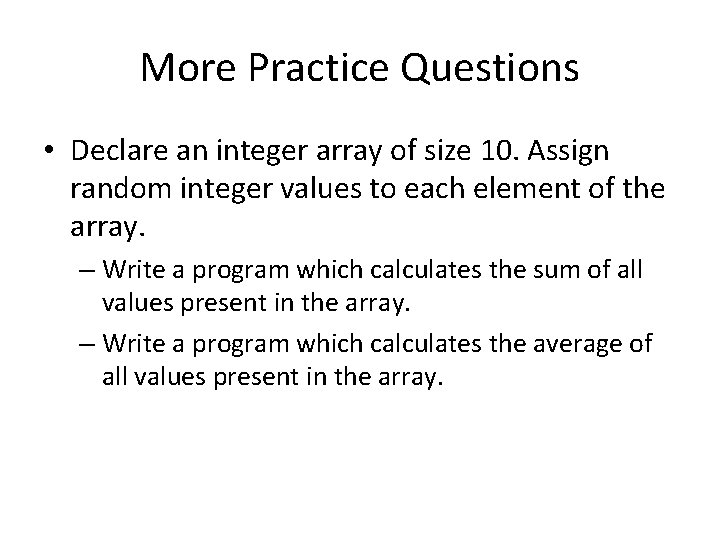
More Practice Questions • Declare an integer array of size 10. Assign random integer values to each element of the array. – Write a program which calculates the sum of all values present in the array. – Write a program which calculates the average of all values present in the array.
Parallel arrays examples
Arreglos unidimensionales y bidimensionales en java
Arrays bidimensionales java
Problem solving
Elementary programming in java
Java introduction to problem solving and programming
Java introduction to problem solving and programming
Introduction to java programming 10th edition quizzes
01:640:244 lecture notes - lecture 15: plat, idah, farad
C data types with examples
Java lecture
Array of arrays c++
Ragged array
潘仁義
Parallel arrays
Why do we need arrays?
Dynamic arrays and amortized analysis