include stdio h int strlen const char str
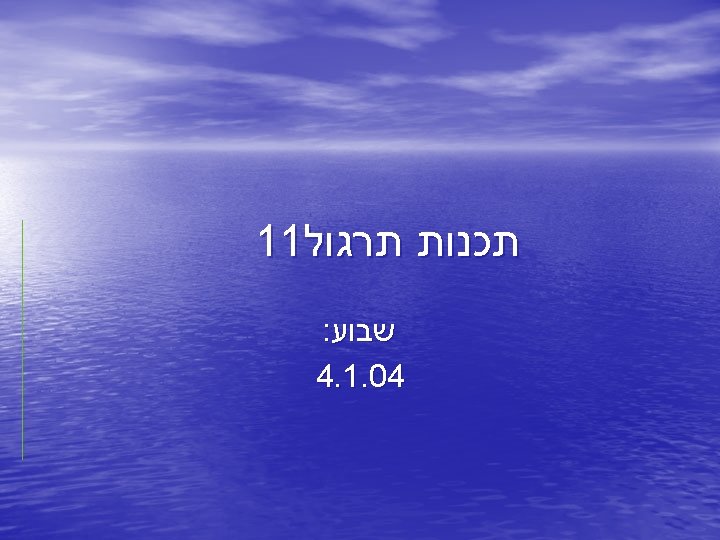
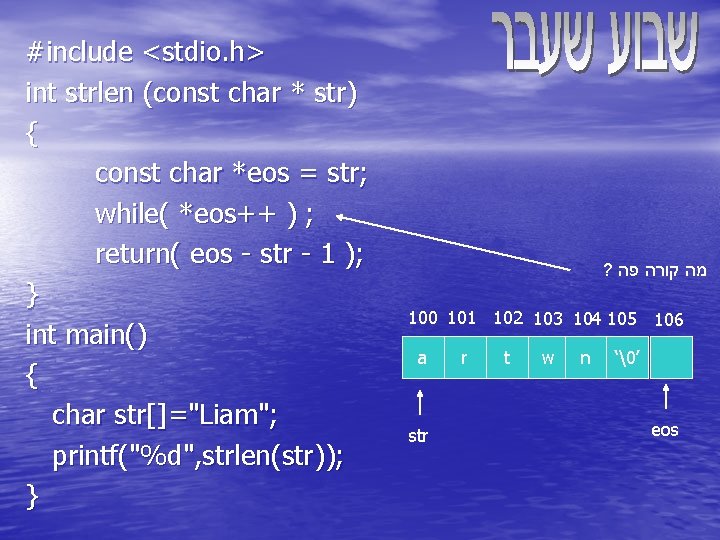
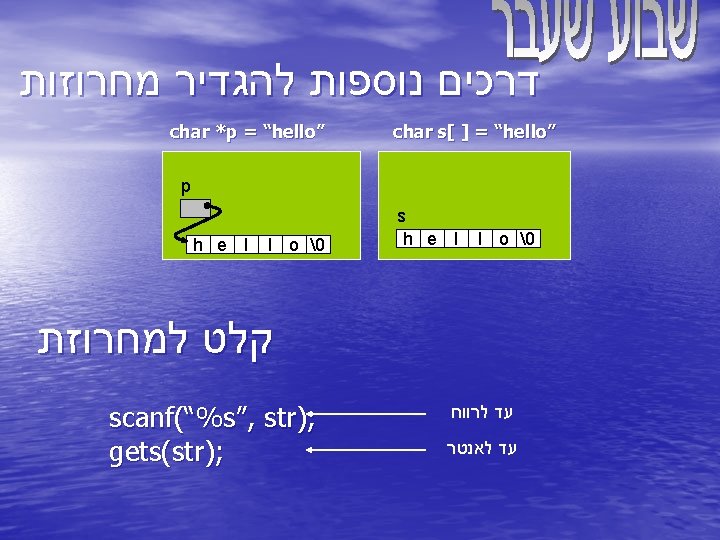
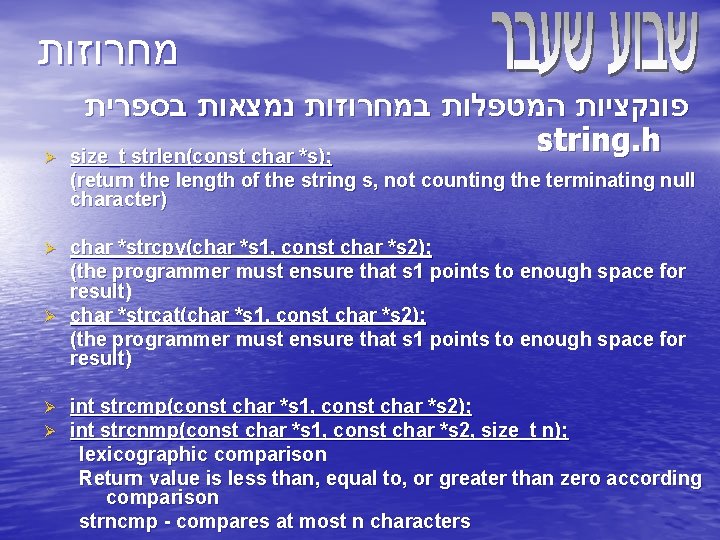
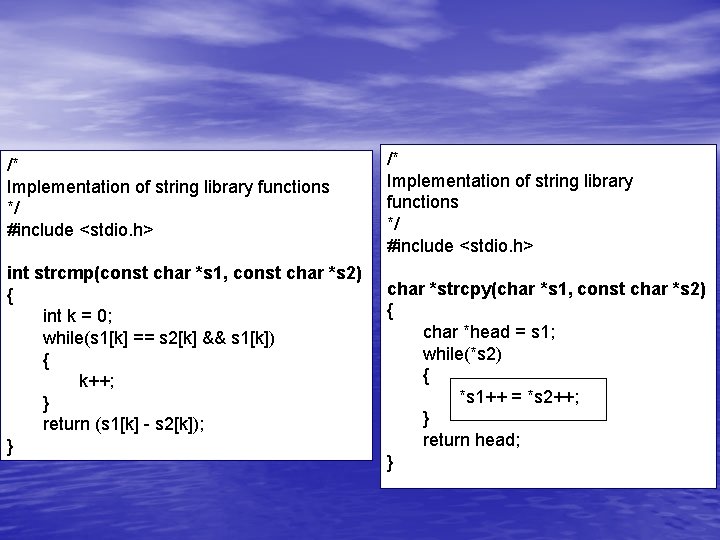
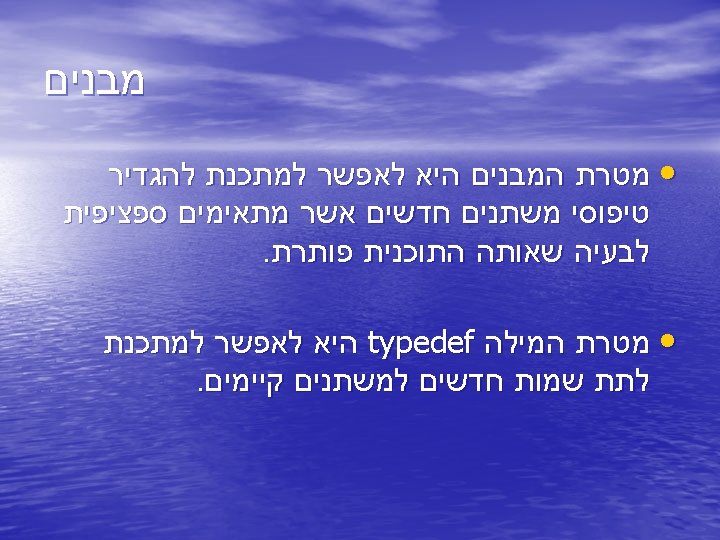
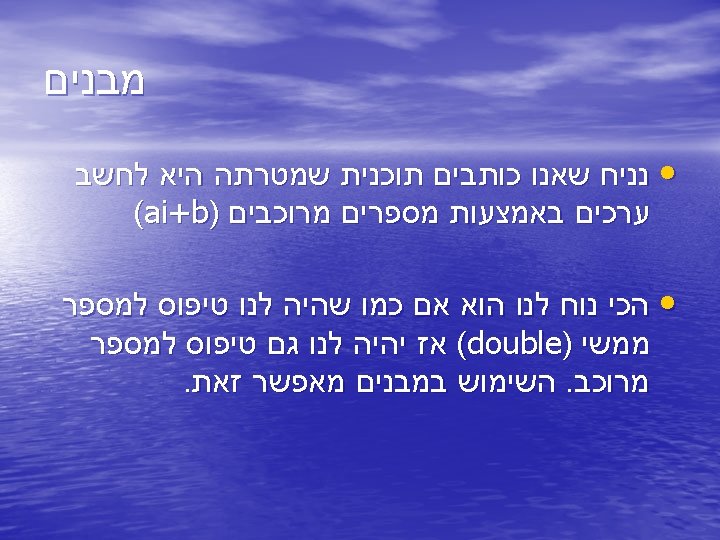
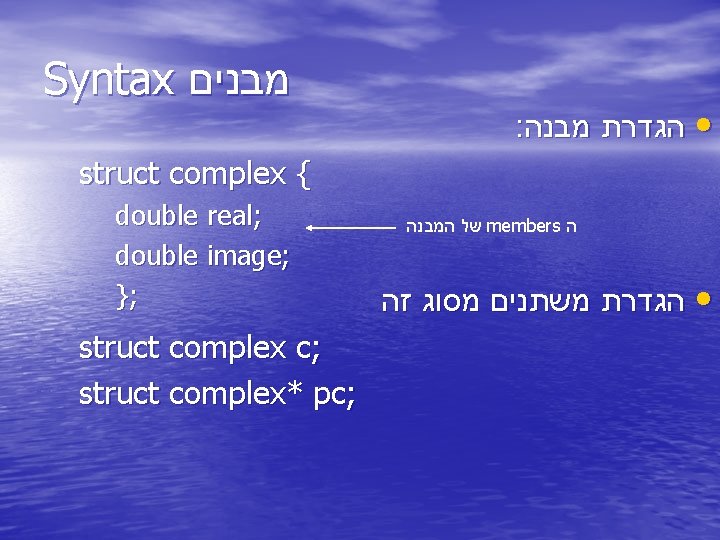
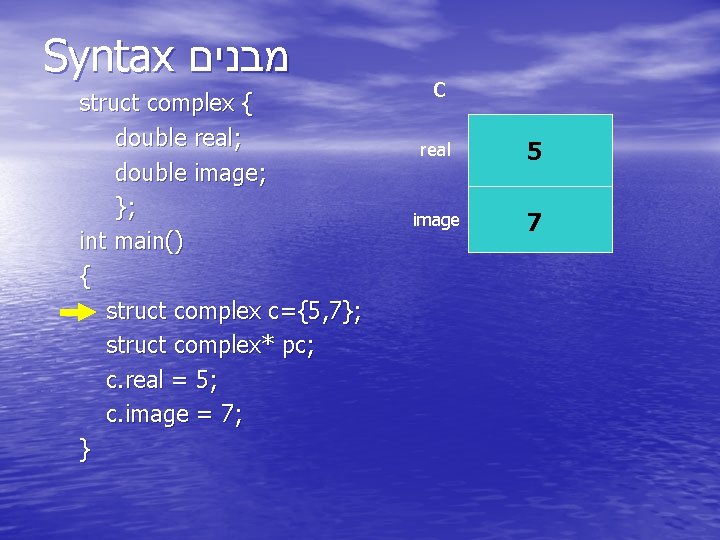
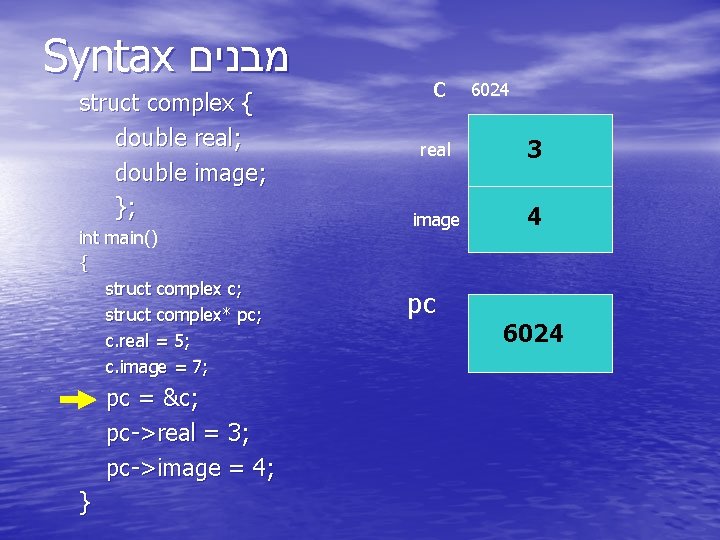
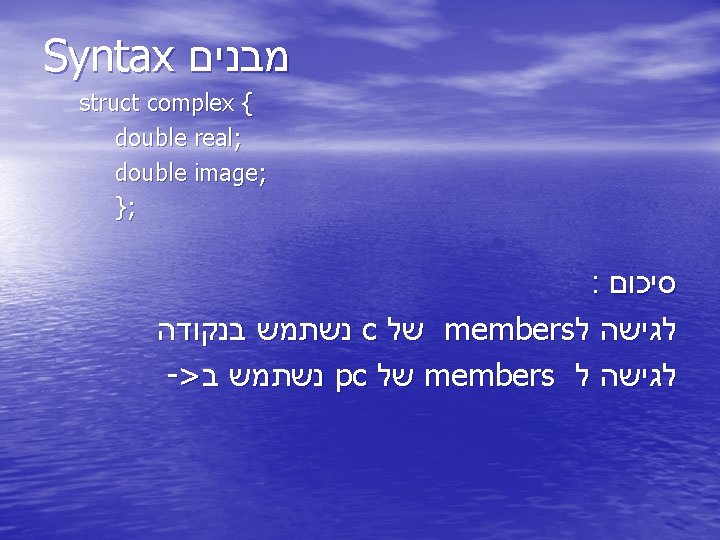
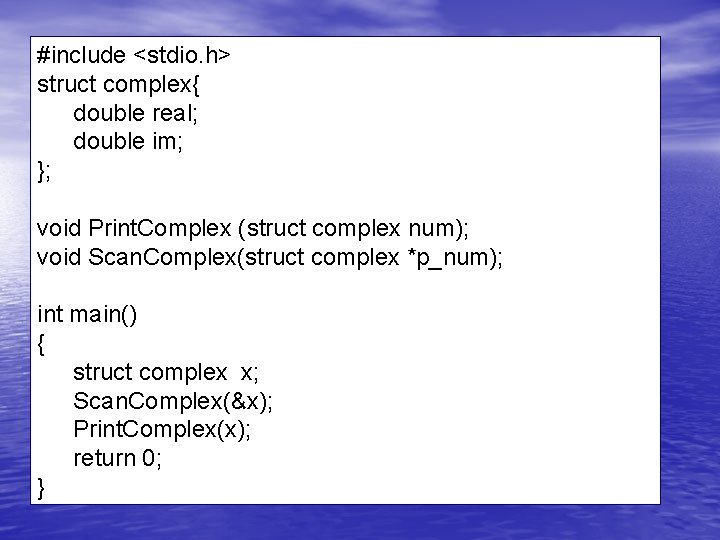
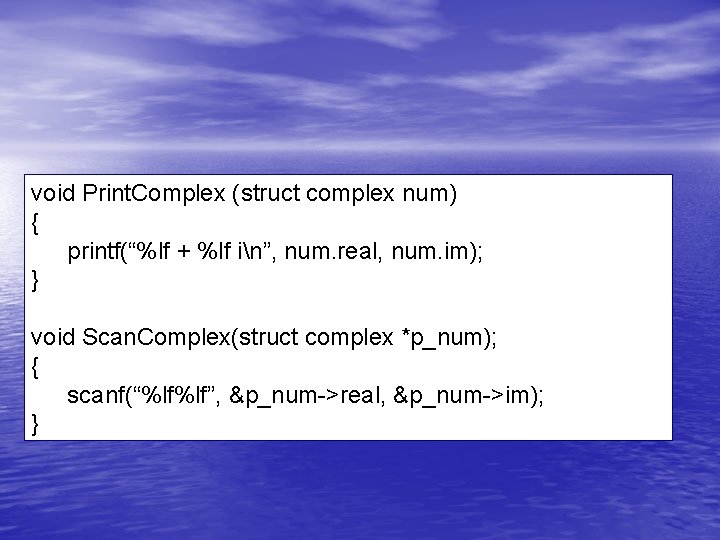
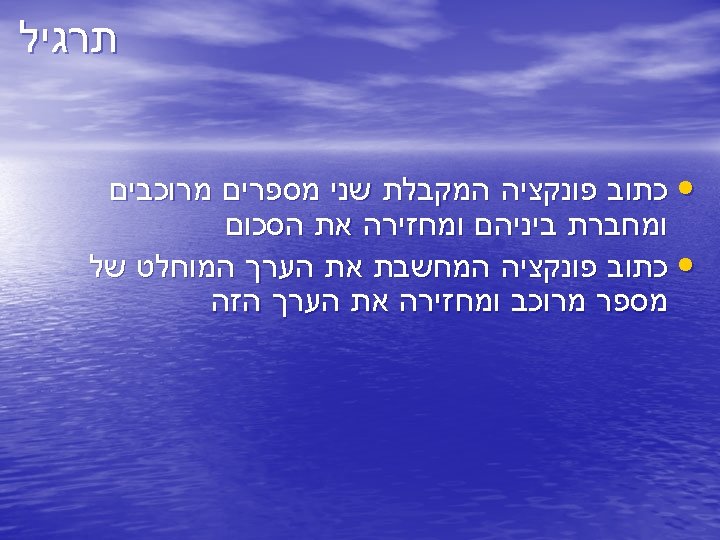
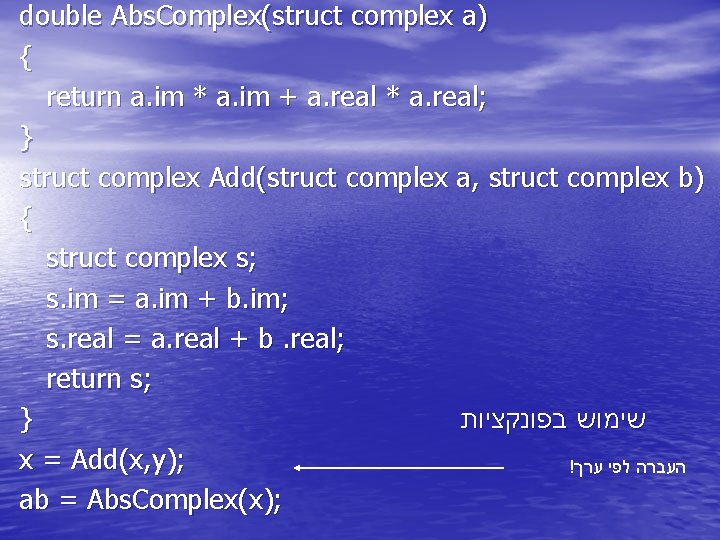
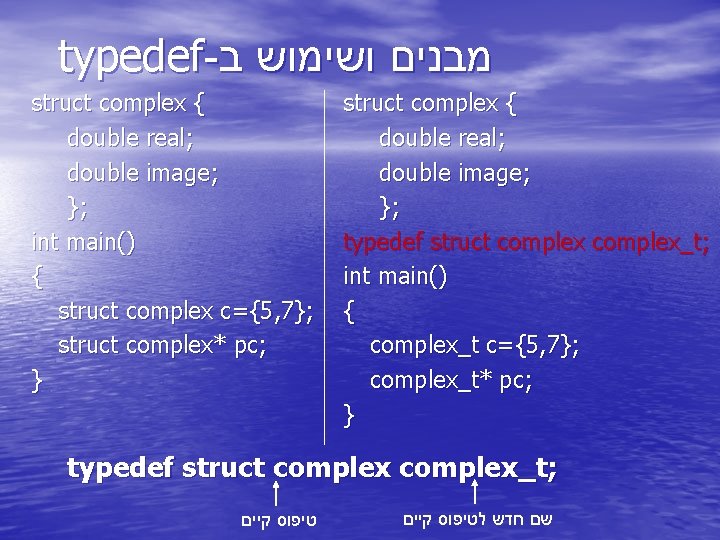
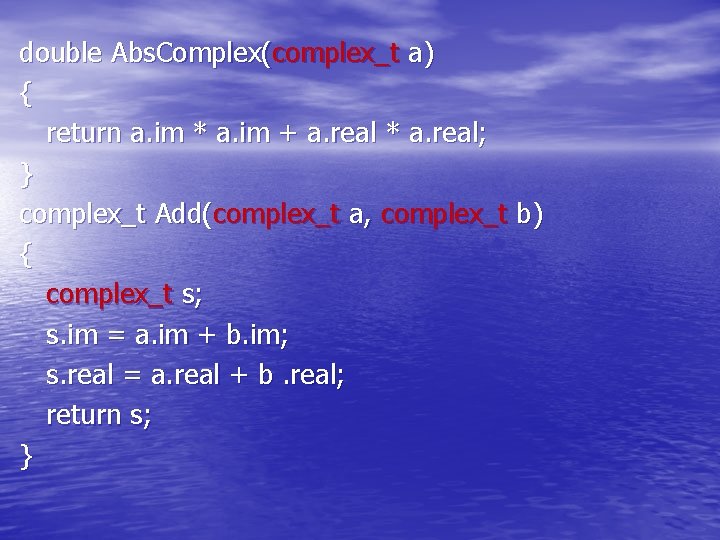
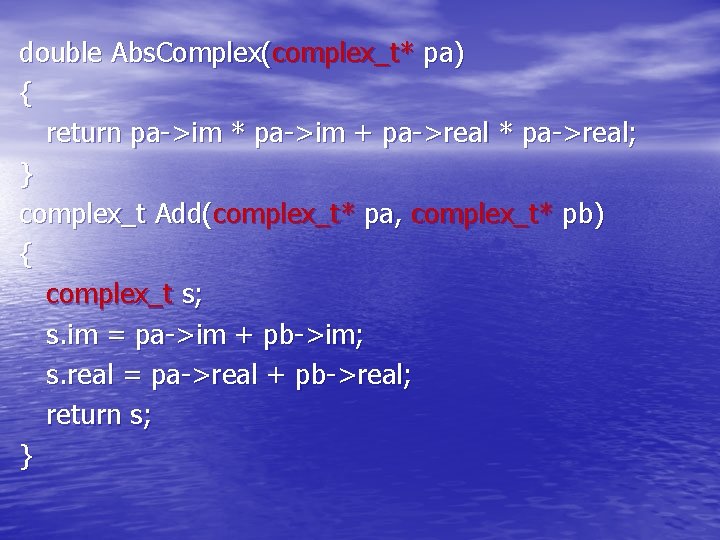
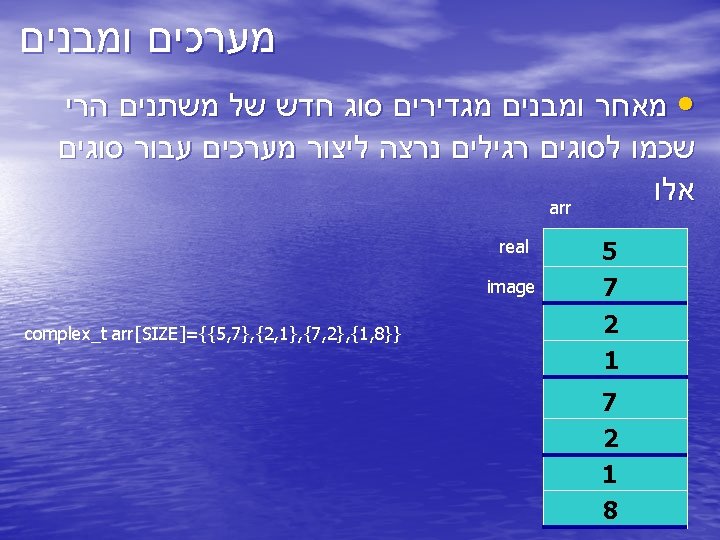
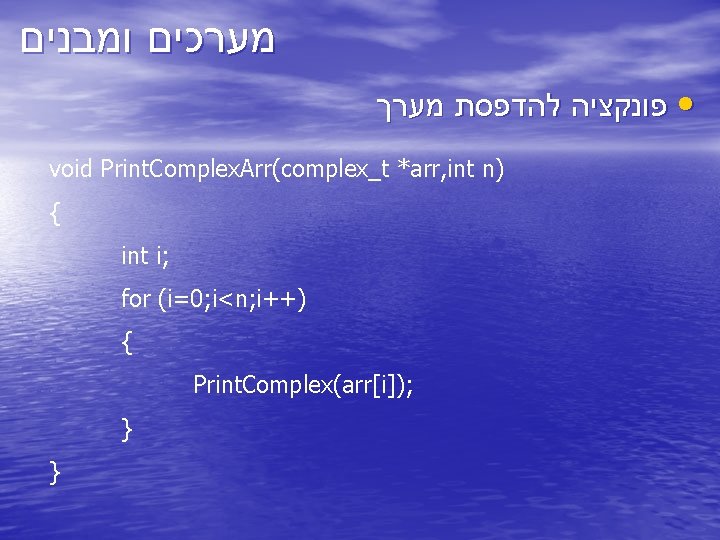
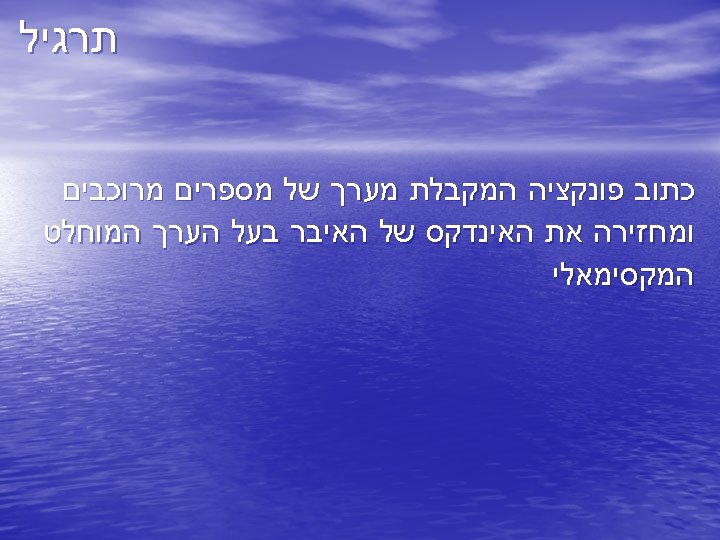
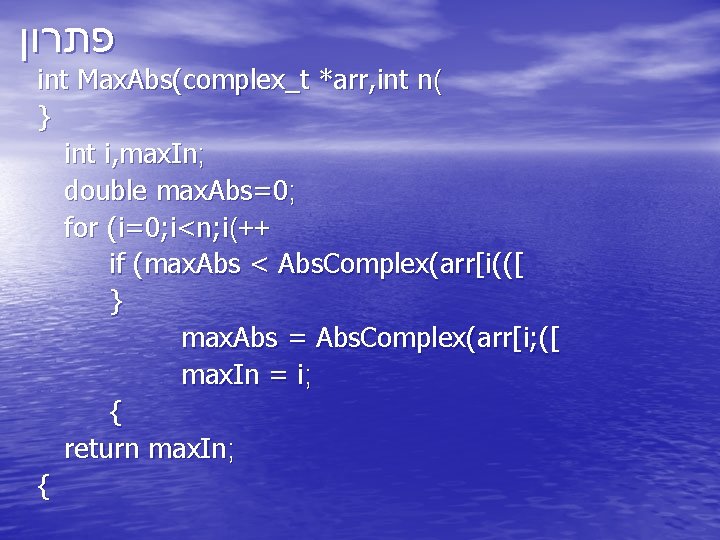
- Slides: 22
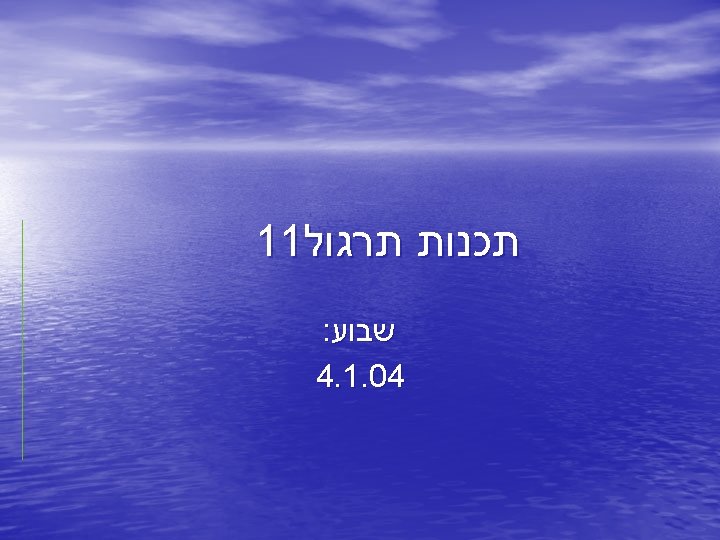
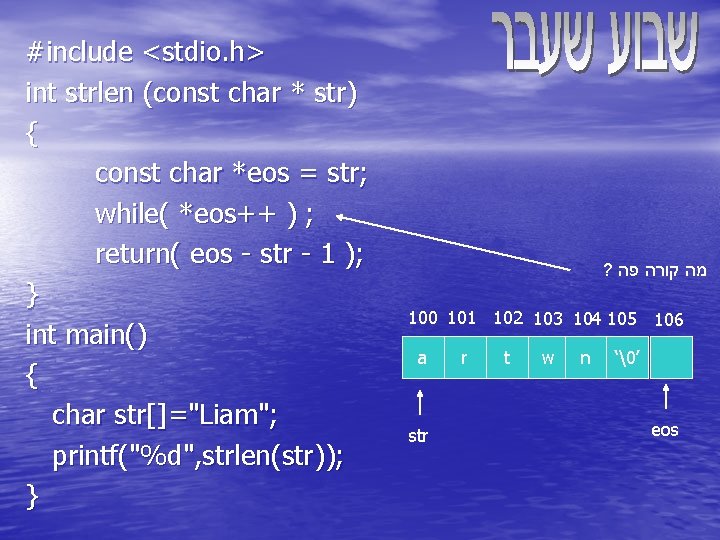
#include <stdio. h> int strlen (const char * str) { const char *eos = str; while( *eos++ ) ; return( eos - str - 1 ); } int main() { char str[]="Liam"; printf("%d", strlen(str)); } ? מה קורה פה 100 101 102 103 104 105 106 a str r t w n ‘ ’ eos
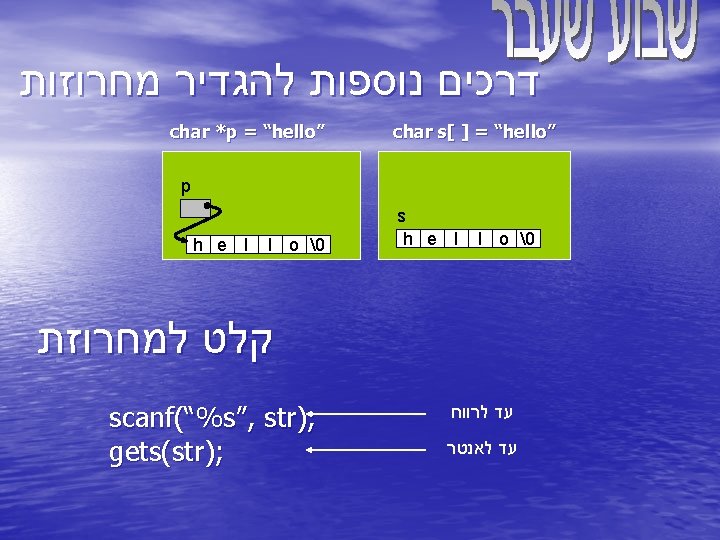
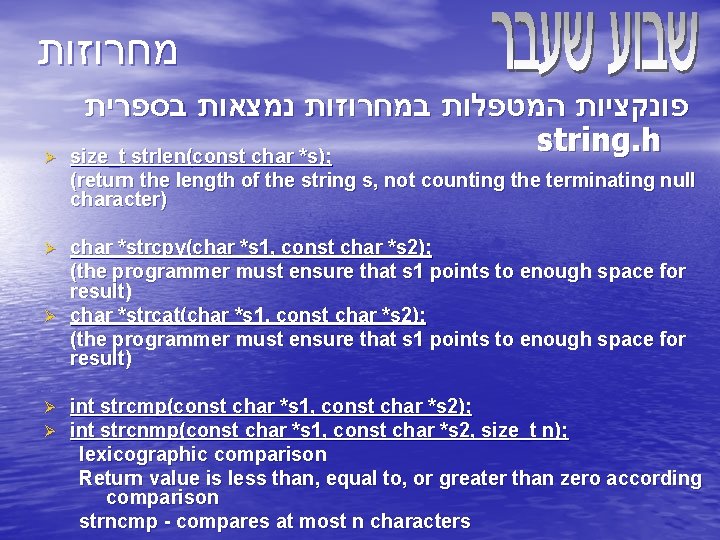
מחרוזות Ø פונקציות המטפלות במחרוזות נמצאות בספרית string. h size_t strlen(const char *s); (return the length of the string s, not counting the terminating null character) char *strcpy(char *s 1, const char *s 2); (the programmer must ensure that s 1 points to enough space for result) Ø char *strcat(char *s 1, const char *s 2); (the programmer must ensure that s 1 points to enough space for result) Ø Ø Ø int strcmp(const char *s 1, const char *s 2); int strcnmp(const char *s 1, const char *s 2, size_t n); lexicographic comparison Return value is less than, equal to, or greater than zero according comparison strncmp - compares at most n characters
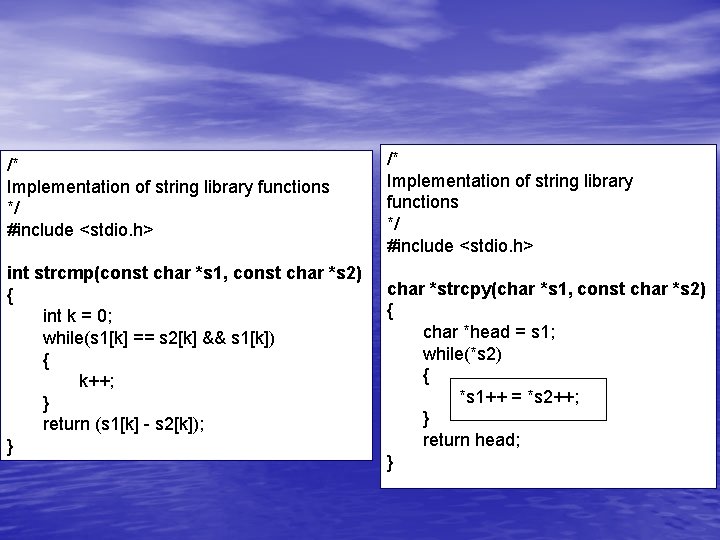
/* Implementation of string library functions */ #include <stdio. h> int strcmp(const char *s 1, const char *s 2) { int k = 0; while(s 1[k] == s 2[k] && s 1[k]) { k++; } return (s 1[k] - s 2[k]); } /* Implementation of string library functions */ #include <stdio. h> char *strcpy(char *s 1, const char *s 2) { char *head = s 1; while(*s 2) { *s 1++ = *s 2++; } return head; }
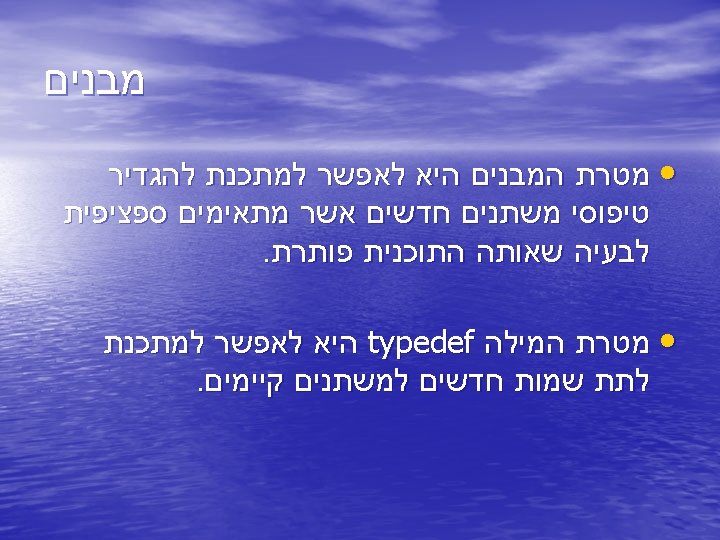
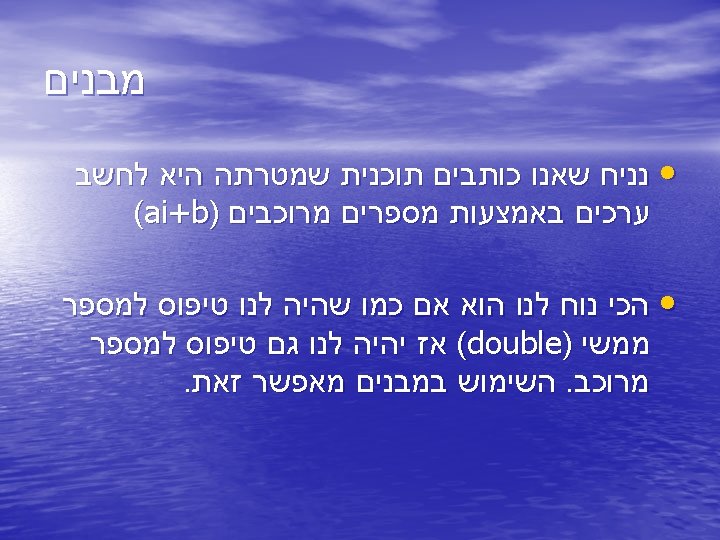
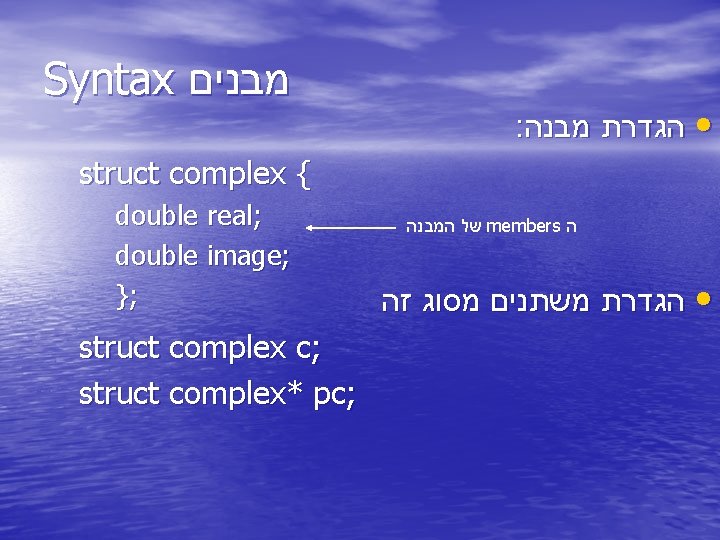
Syntax מבנים : • הגדרת מבנה struct complex { double real; double image; }; struct complex c; struct complex* pc; של המבנה members ה • הגדרת משתנים מסוג זה
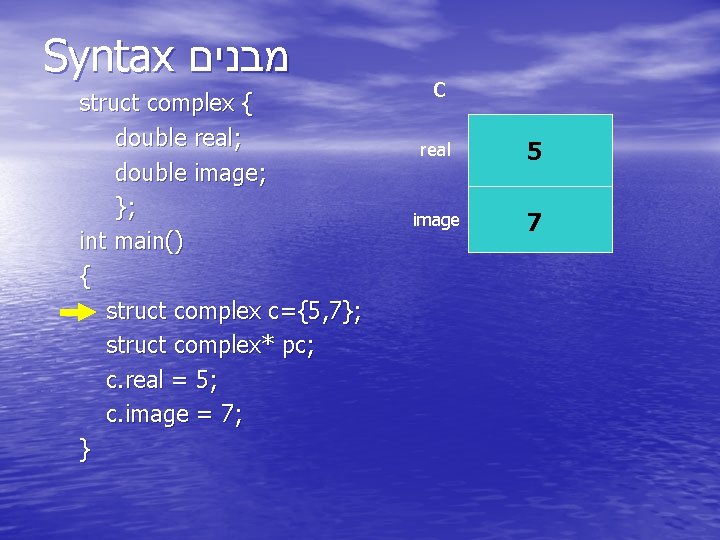
Syntax מבנים struct complex { double real; double image; }; int main() { struct complex c={5, 7}; struct complex* pc; c. real = 5; c. image = 7; } c real 5 image 7
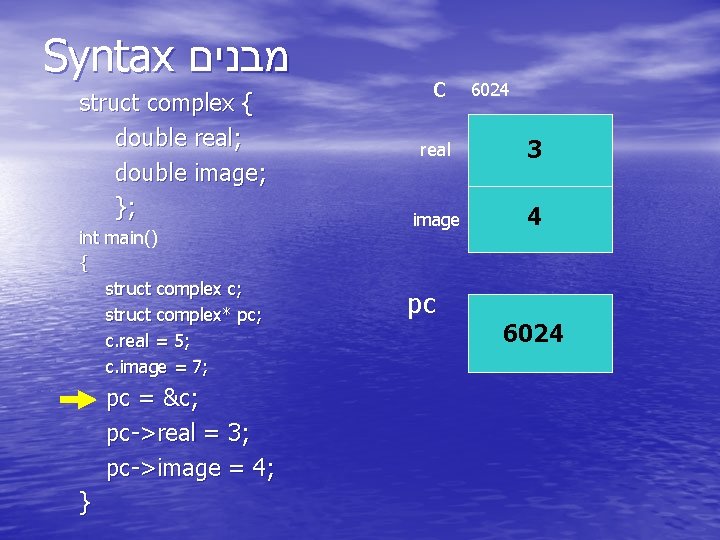
Syntax מבנים struct complex { double real; double image; }; int main() { struct complex c; struct complex* pc; c. real = 5; c. image = 7; pc = &c; pc->real = 3; pc->image = 4; } c 6024 real 3 5 image 4 7 pc 6024
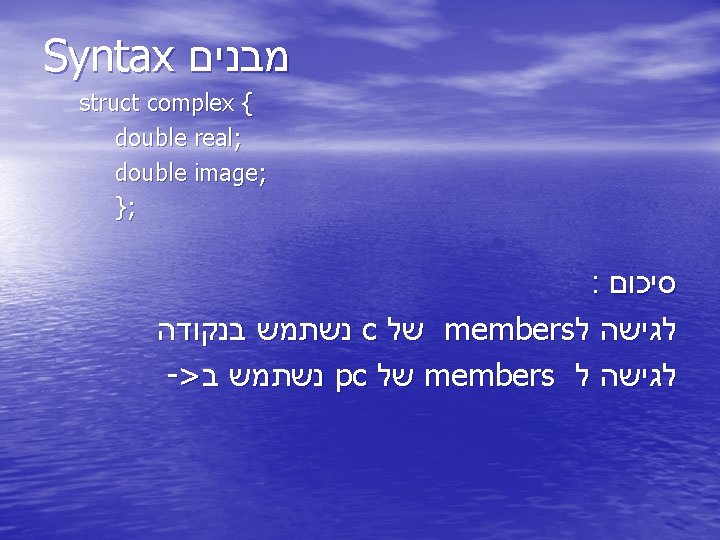
Syntax מבנים struct complex { double real; double image; }; : סיכום נשתמש בנקודה c של members לגישה ל -> נשתמש ב pc של members לגישה ל
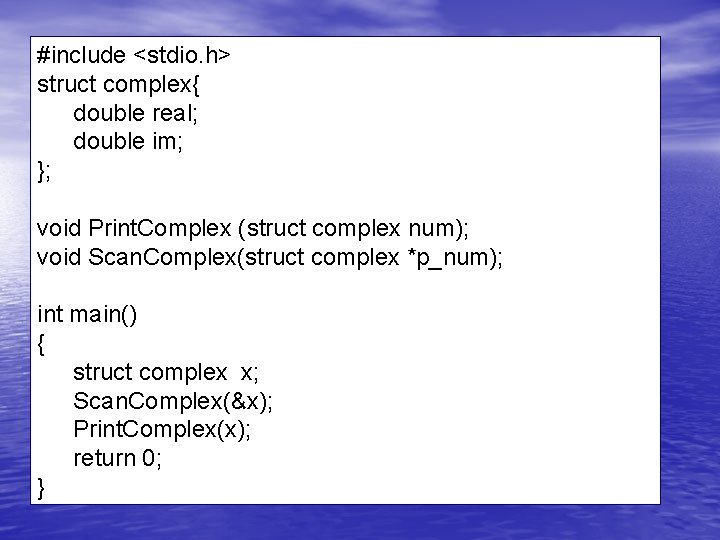
#include <stdio. h> struct complex{ double real; double im; }; void Print. Complex (struct complex num); void Scan. Complex(struct complex *p_num); int main() { struct complex x; Scan. Complex(&x); Print. Complex(x); return 0; }
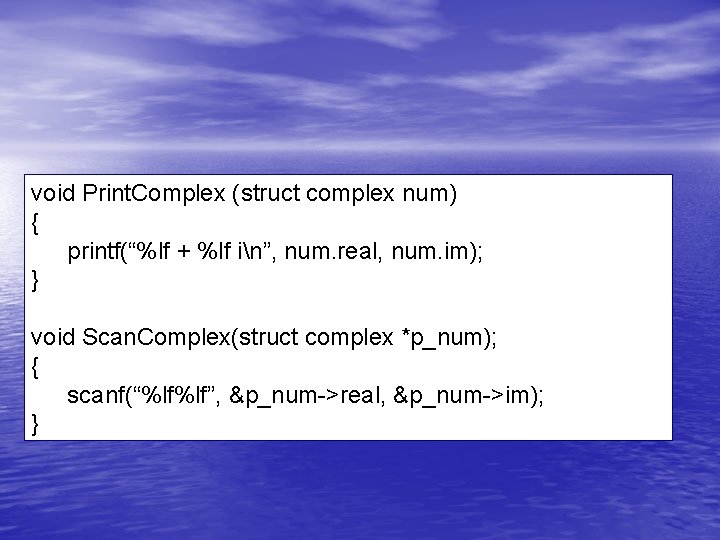
void Print. Complex (struct complex num) { printf(“%lf + %lf in”, num. real, num. im); } void Scan. Complex(struct complex *p_num); { scanf(“%lf%lf”, &p_num->real, &p_num->im); }
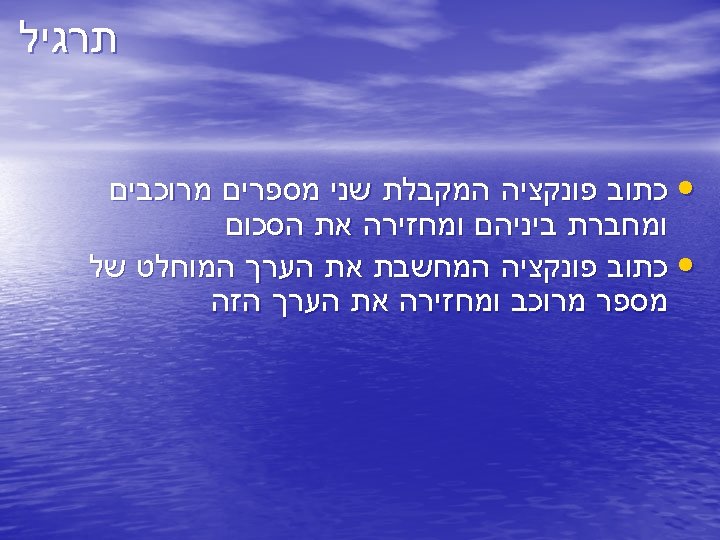
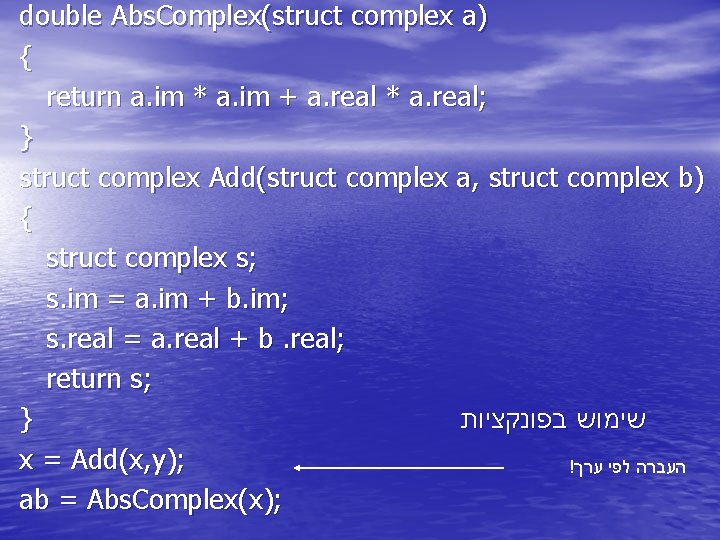
double Abs. Complex(struct complex a) { return a. im * a. im + a. real * a. real; } struct complex Add(struct complex a, struct complex b) { struct complex s; s. im = a. im + b. im; s. real = a. real + b. real; return s; } שימוש בפונקציות x = Add(x, y); ! העברה לפי ערך ab = Abs. Complex(x);
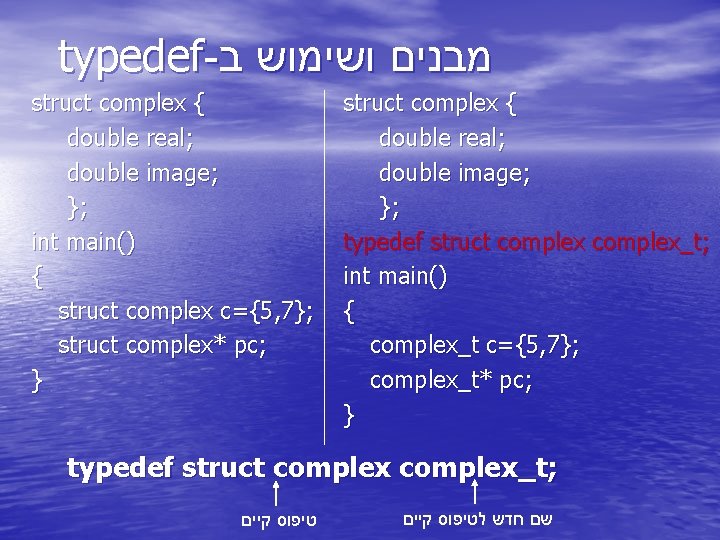
typedef- מבנים ושימוש ב struct complex { double real; double image; }; int main() { struct complex c={5, 7}; struct complex* pc; } struct complex { double real; double image; }; typedef struct complex_t; int main() { complex_t c={5, 7}; complex_t* pc; } typedef struct complex_t; טיפוס קיים שם חדש לטיפוס קיים
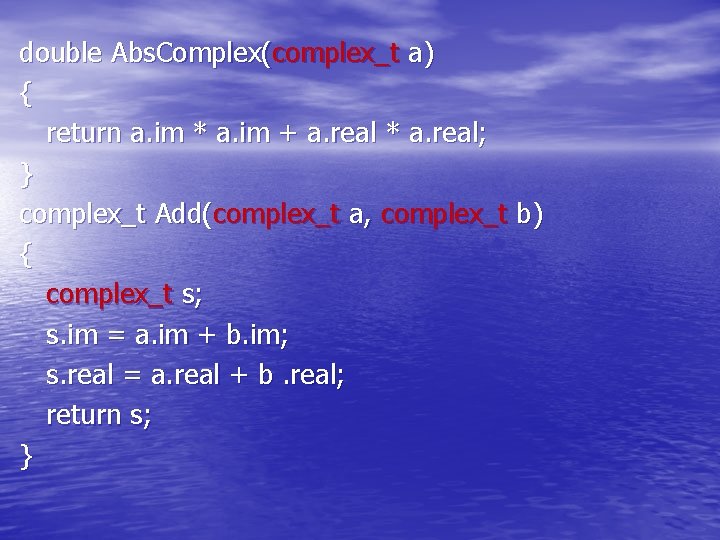
double Abs. Complex(complex_t a) { return a. im * a. im + a. real * a. real; } complex_t Add(complex_t a, complex_t b) { complex_t s; s. im = a. im + b. im; s. real = a. real + b. real; return s; }
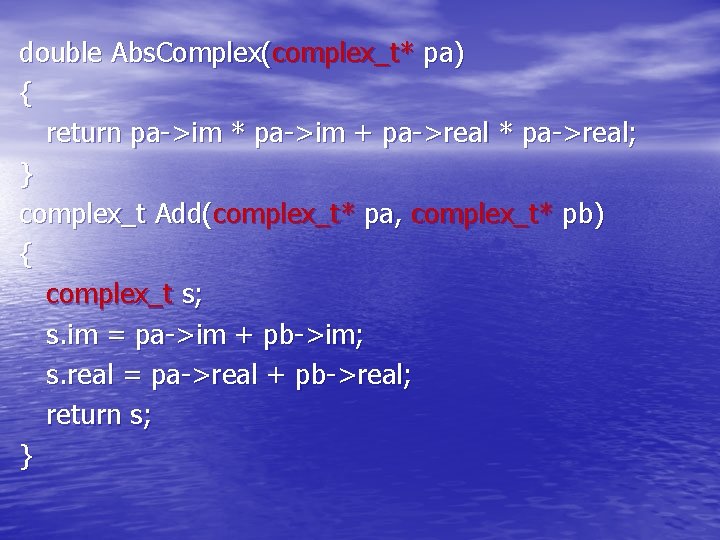
double Abs. Complex(complex_t* pa) { return pa->im * pa->im + pa->real * pa->real; } complex_t Add(complex_t* pa, complex_t* pb) { complex_t s; s. im = pa->im + pb->im; s. real = pa->real + pb->real; return s; }
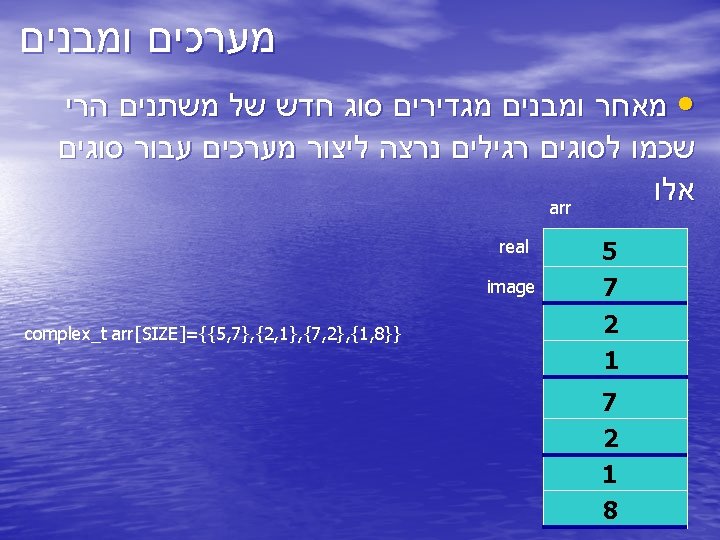
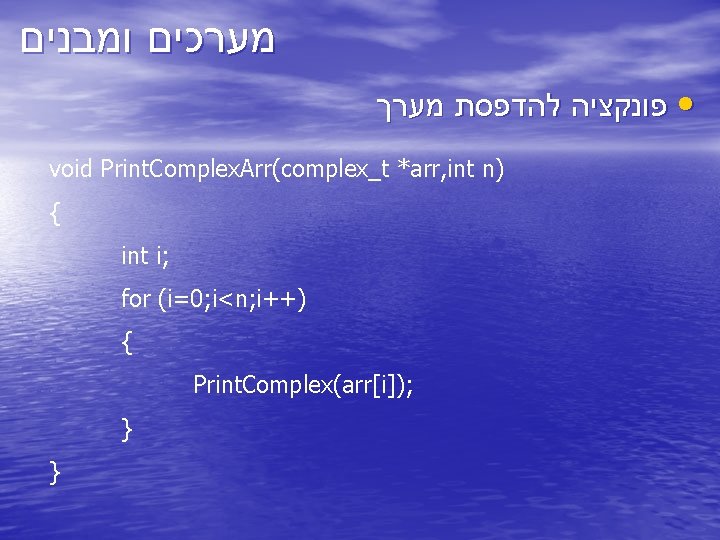
מערכים ומבנים • פונקציה להדפסת מערך void Print. Complex. Arr(complex_t *arr, int n) { int i; for (i=0; i<n; i++) { Print. Complex(arr[i]); } }
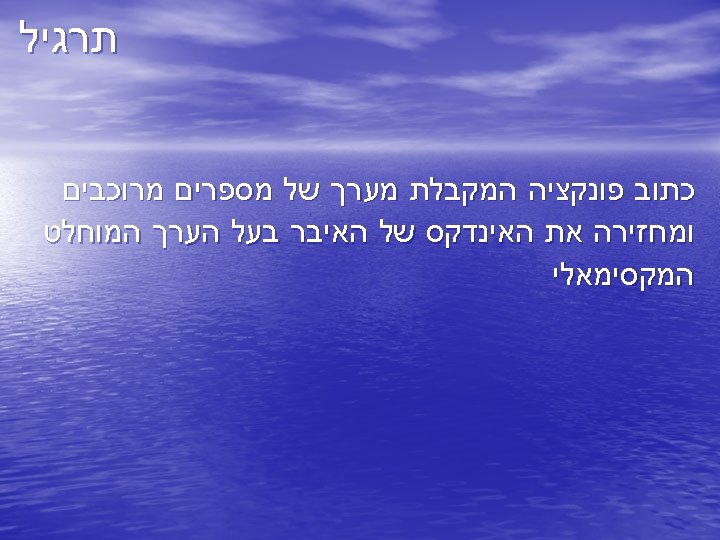
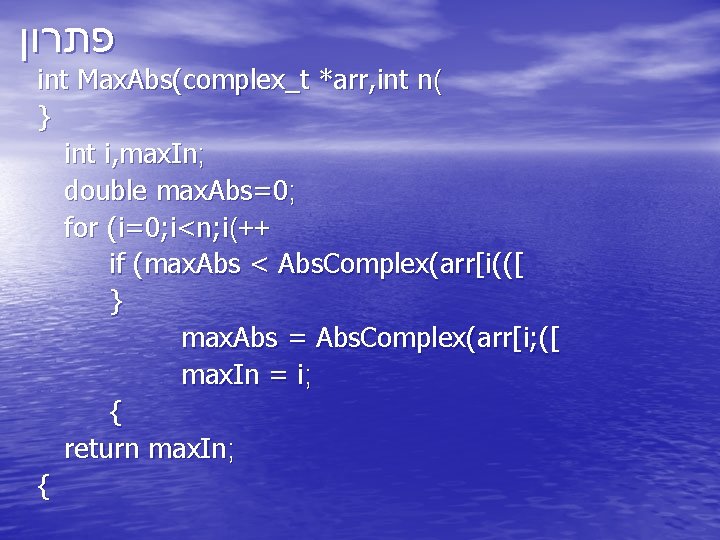
פתרון int Max. Abs(complex_t *arr, int n( } int i, max. In; double max. Abs=0; for (i=0; i<n; i(++ if (max. Abs < Abs. Complex(arr[i(([ } max. Abs = Abs. Complex(arr[i; ([ max. In = i; { return max. In; {