Extract Subclass Extract Superclass and Extract Hierarchy BY
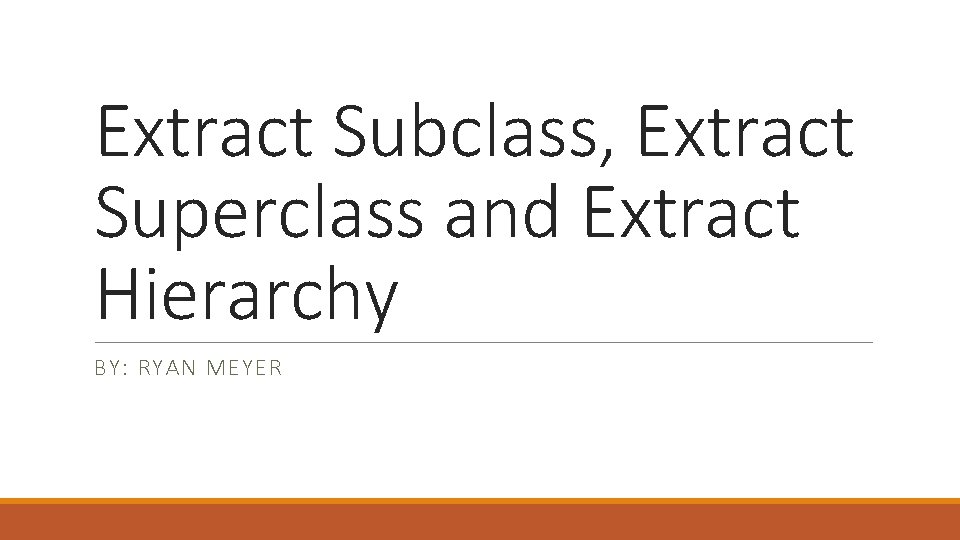
Extract Subclass, Extract Superclass and Extract Hierarchy BY: RYAN MEYER
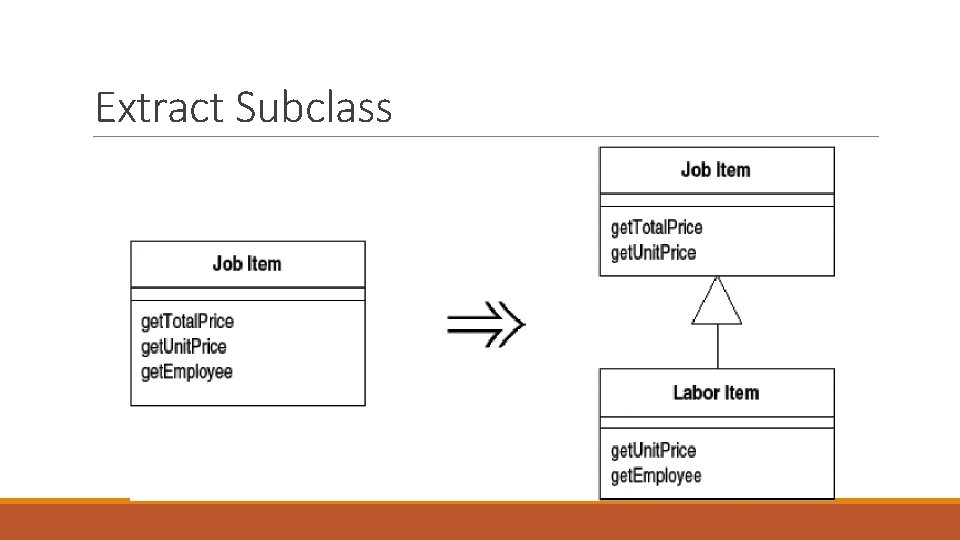
Extract Subclass
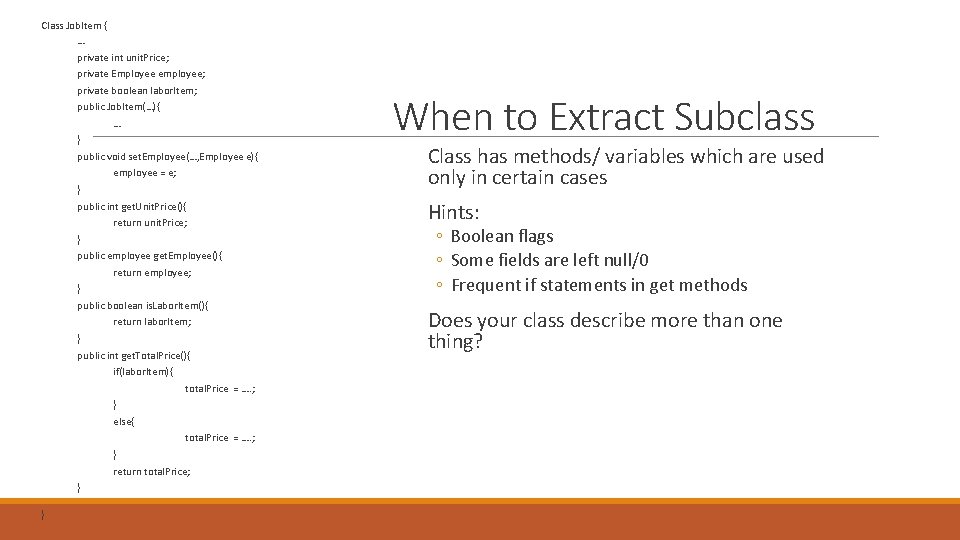
Class Job. Item { … private int unit. Price; private Employee employee; private boolean labor. Item; public Job. Item(…){ … } public void set. Employee(…, Employee e){ employee = e; } public int get. Unit. Price(){ return unit. Price; } public employee get. Employee(){ return employee; } public boolean is. Labor. Item(){ return labor. Item; } public int get. Total. Price(){ if(labor. Item){ total. Price = …. ; } else{ total. Price = …. ; } return total. Price; } } When to Extract Subclass Class has methods/ variables which are used only in certain cases Hints: ◦ Boolean flags ◦ Some fields are left null/0 ◦ Frequent if statements in get methods Does your class describe more than one thing?
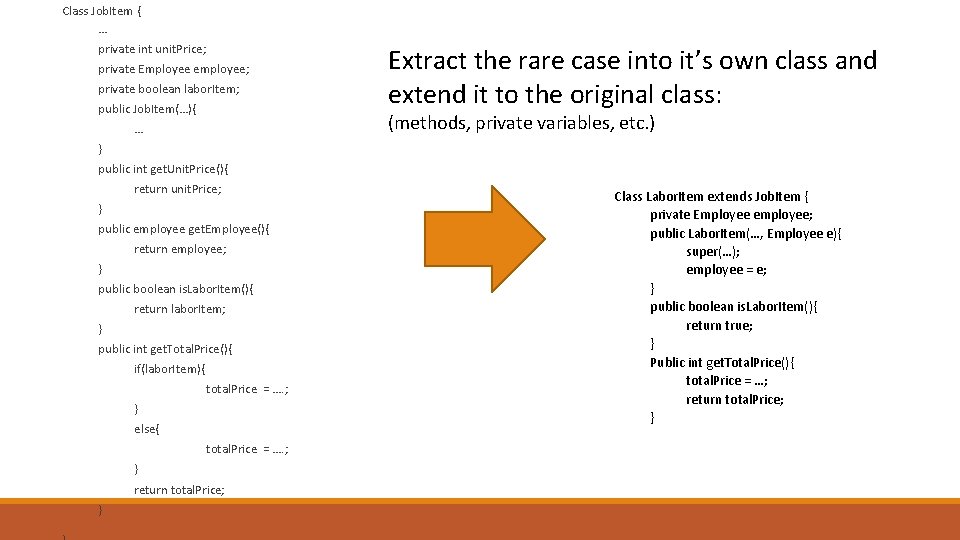
Class Job. Item { … private int unit. Price; private Employee employee; private boolean labor. Item; public Job. Item(…){ Extract the rare case into it’s own class and extend it to the original class: (methods, private variables, etc. ) … } public int get. Unit. Price(){ return unit. Price; } public employee get. Employee(){ return employee; } public boolean is. Labor. Item(){ return labor. Item; } public int get. Total. Price(){ if(labor. Item){ total. Price = …. ; } else{ total. Price = …. ; } return total. Price; } Class Labor. Item extends Job. Item { private Employee employee; public Labor. Item(…, Employee e){ super(…); employee = e; } public boolean is. Labor. Item(){ return true; } Public int get. Total. Price(){ total. Price = …; return total. Price; }
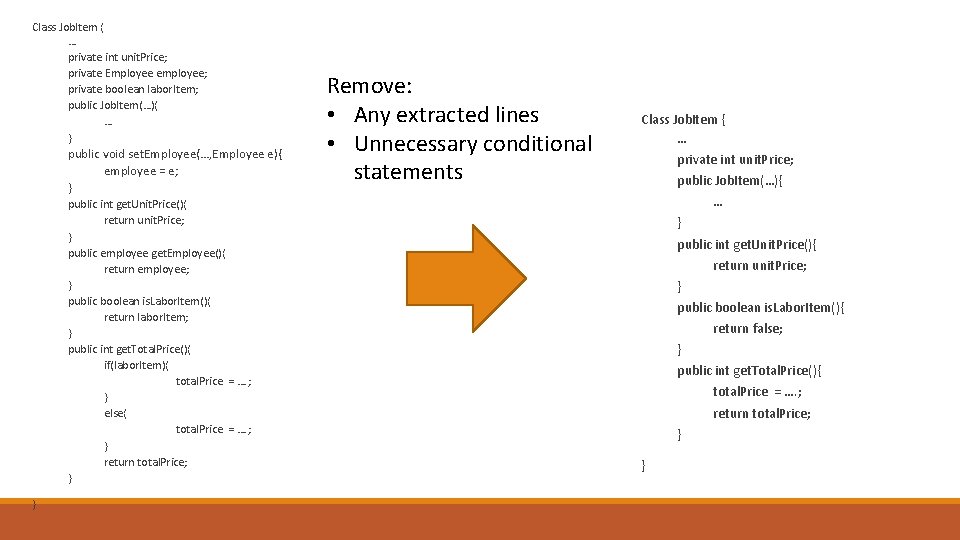
Class Job. Item { … private int unit. Price; private Employee employee; private boolean labor. Item; public Job. Item(…){ … } public void set. Employee(…, Employee e){ employee = e; } public int get. Unit. Price(){ return unit. Price; } public employee get. Employee(){ return employee; } public boolean is. Labor. Item(){ return labor. Item; } public int get. Total. Price(){ if(labor. Item){ total. Price = …. ; } else{ total. Price = …. ; } return total. Price; } } Remove: • Any extracted lines • Unnecessary conditional statements Class Job. Item { … private int unit. Price; public Job. Item(…){ … } public int get. Unit. Price(){ return unit. Price; } public boolean is. Labor. Item(){ return false; } public int get. Total. Price(){ total. Price = …. ; return total. Price; } }
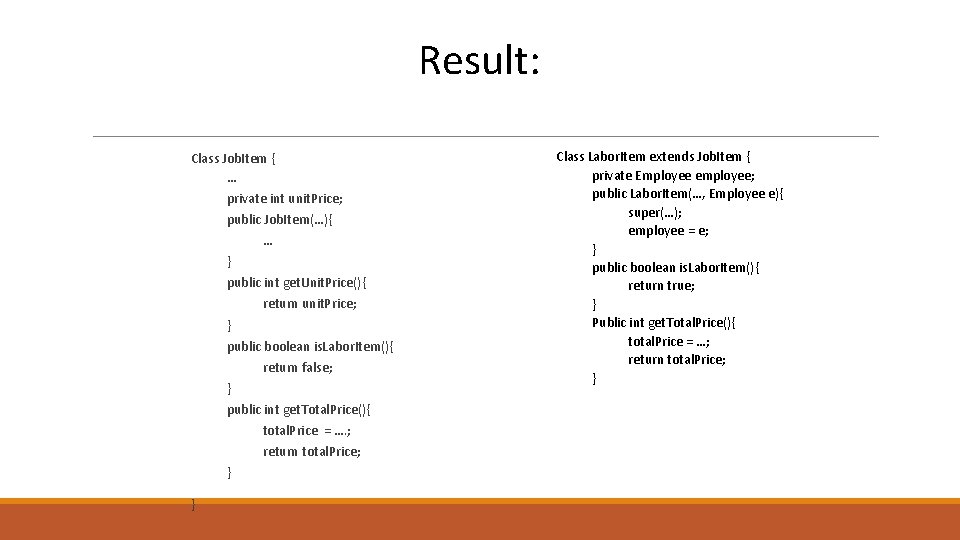
Result: Class Job. Item { … private int unit. Price; public Job. Item(…){ … } public int get. Unit. Price(){ return unit. Price; } public boolean is. Labor. Item(){ return false; } public int get. Total. Price(){ total. Price = …. ; return total. Price; } } Class Labor. Item extends Job. Item { private Employee employee; public Labor. Item(…, Employee e){ super(…); employee = e; } public boolean is. Labor. Item(){ return true; } Public int get. Total. Price(){ total. Price = …; return total. Price; }
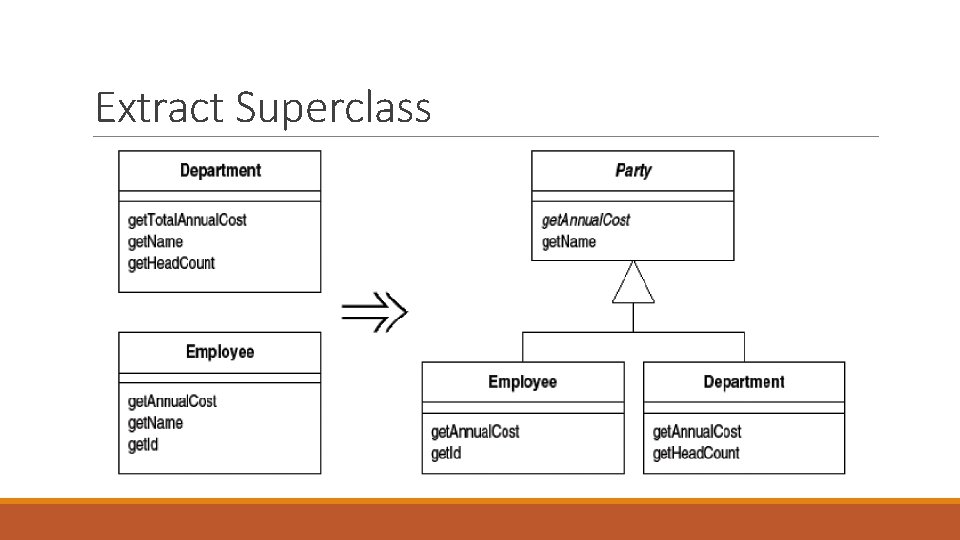
Extract Superclass
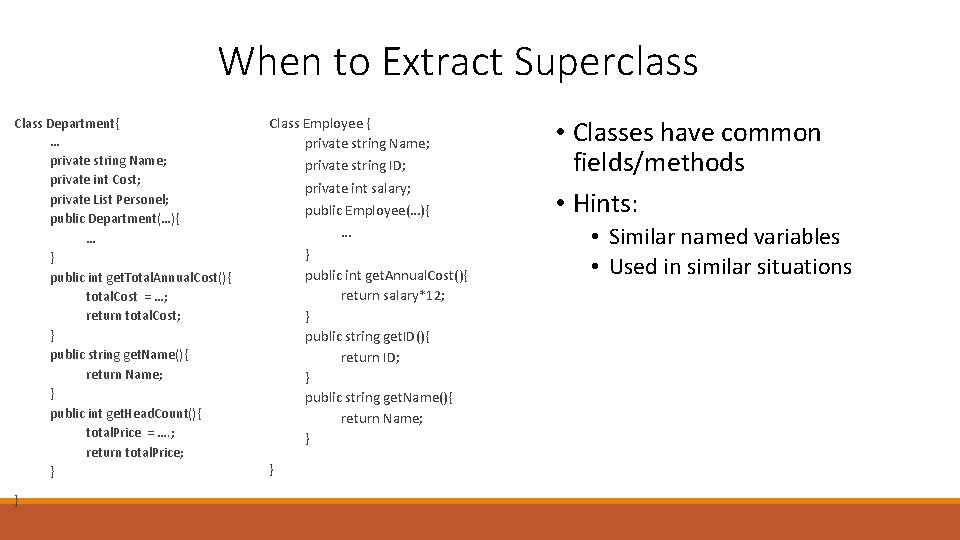
When to Extract Superclass Class Department{ … private string Name; private int Cost; private List Personel; public Department(…){ … } public int get. Total. Annual. Cost(){ total. Cost = …; return total. Cost; } public string get. Name(){ return Name; } public int get. Head. Count(){ total. Price = …. ; return total. Price; } } Class Employee { private string Name; private string ID; private int salary; public Employee(…){ … } public int get. Annual. Cost(){ return salary*12; } public string get. ID(){ return ID; } public string get. Name(){ return Name; } } • Classes have common fields/methods • Hints: • Similar named variables • Used in similar situations
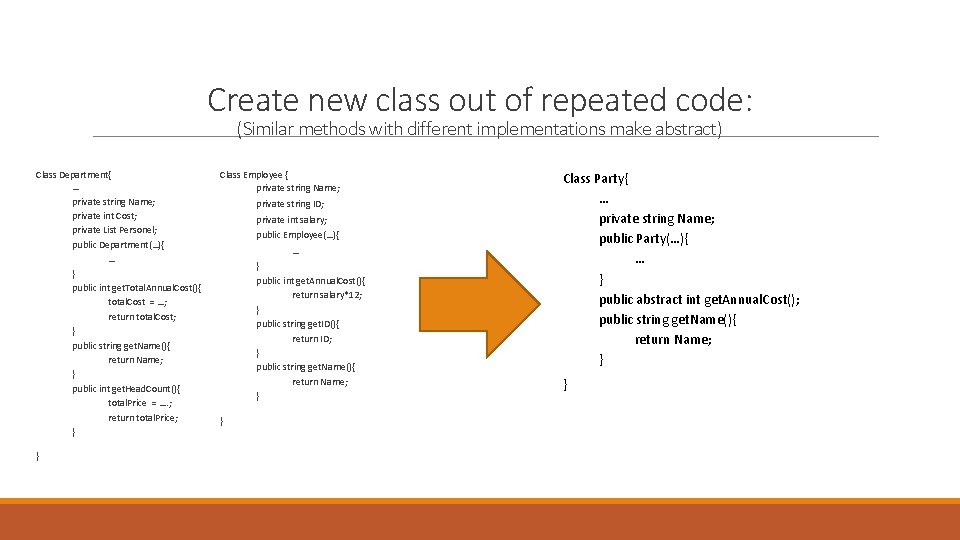
Create new class out of repeated code: (Similar methods with different implementations make abstract) Class Department{ … private string Name; private int Cost; private List Personel; public Department(…){ … } public int get. Total. Annual. Cost(){ total. Cost = …; return total. Cost; } public string get. Name(){ return Name; } public int get. Head. Count(){ total. Price = …. ; return total. Price; } } Class Employee { private string Name; private string ID; private int salary; public Employee(…){ … } public int get. Annual. Cost(){ return salary*12; } public string get. ID(){ return ID; } public string get. Name(){ return Name; } } Class Party{ … private string Name; public Party(…){ … } public abstract int get. Annual. Cost(); public string get. Name(){ return Name; } }
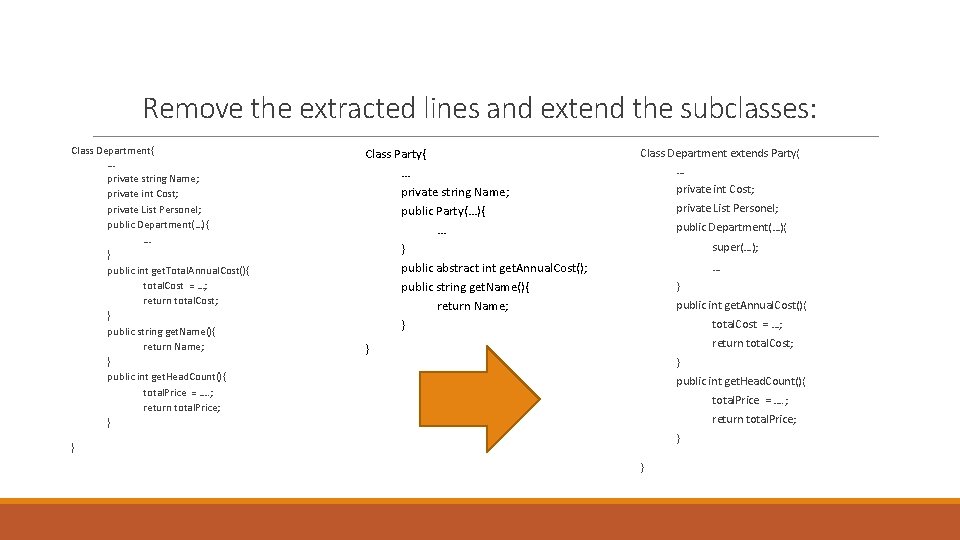
Remove the extracted lines and extend the subclasses: Class Department{ … private string Name; private int Cost; private List Personel; public Department(…){ … } public int get. Total. Annual. Cost(){ total. Cost = …; return total. Cost; } public string get. Name(){ return Name; } public int get. Head. Count(){ total. Price = …. ; return total. Price; } Class Party{ … private string Name; public Party(…){ … } public abstract int get. Annual. Cost(); public string get. Name(){ return Name; } Class Department extends Party{ … private int Cost; private List Personel; public Department(…){ super(…); … } public int get. Annual. Cost(){ total. Cost = …; return total. Cost; } } public int get. Head. Count(){ total. Price = …. ; return total. Price; } } }
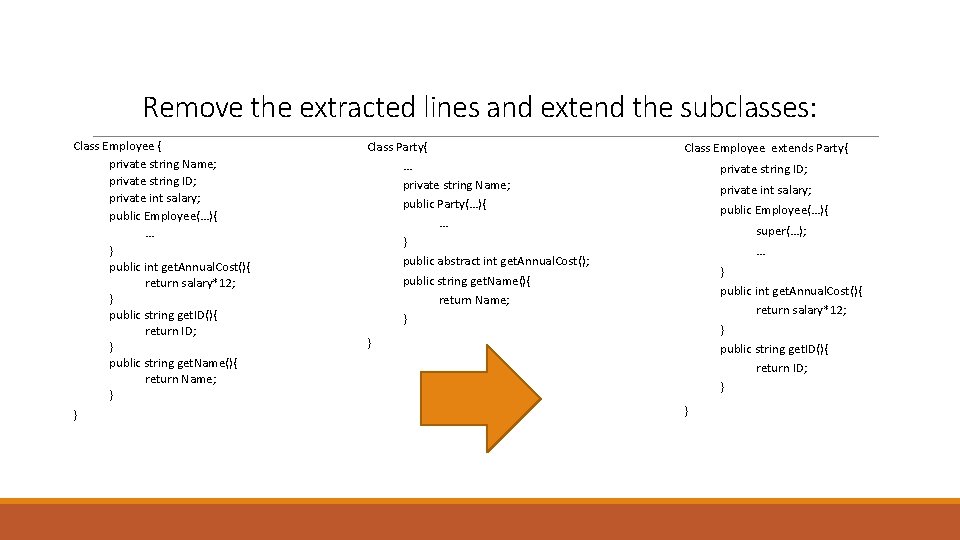
Remove the extracted lines and extend the subclasses: Class Employee { private string Name; private string ID; private int salary; public Employee(…){ … } public int get. Annual. Cost(){ return salary*12; } public string get. ID(){ return ID; } public string get. Name(){ return Name; } } Class Party{ … private string Name; public Party(…){ … } public abstract int get. Annual. Cost(); public string get. Name(){ return Name; } Class Employee extends Party{ private string ID; private int salary; public Employee(…){ super(…); … } public int get. Annual. Cost(){ return salary*12; } public string get. ID(){ return ID; } } }
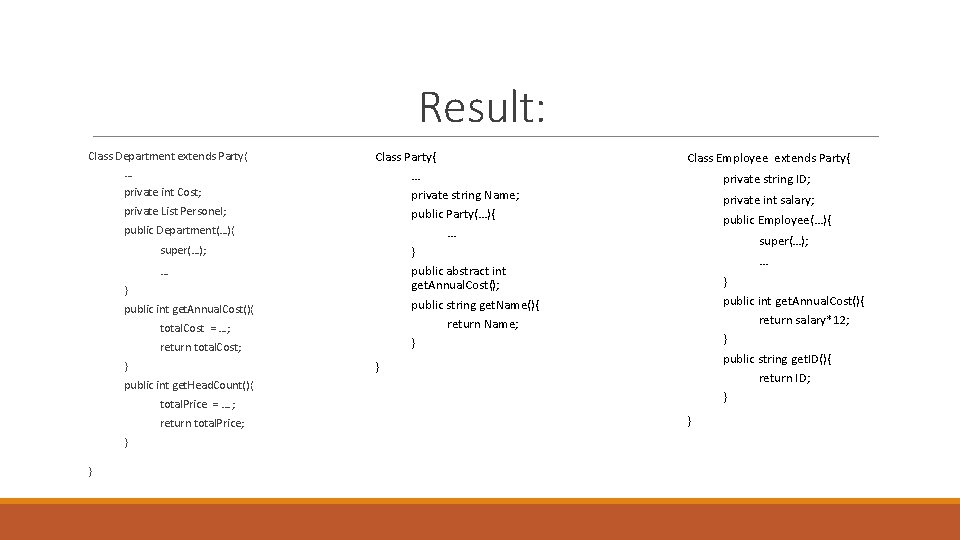
Result: Class Department extends Party{ … private int Cost; private List Personel; public Department(…){ super(…); … } public int get. Annual. Cost(){ total. Cost = …; return total. Cost; Class Party{ … private string Name; public Party(…){ … } public abstract int get. Annual. Cost(); public string get. Name(){ return Name; } Class Employee extends Party{ private string ID; private int salary; public Employee(…){ super(…); … } public int get. Annual. Cost(){ return salary*12; } public string get. ID(){ return ID; } } } public int get. Head. Count(){ total. Price = …. ; return total. Price; } } }
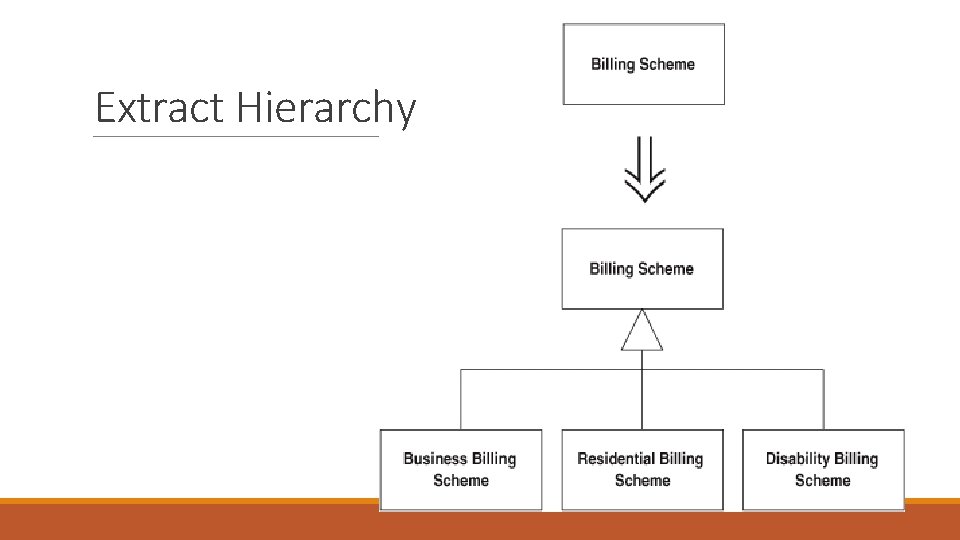
Extract Hierarchy
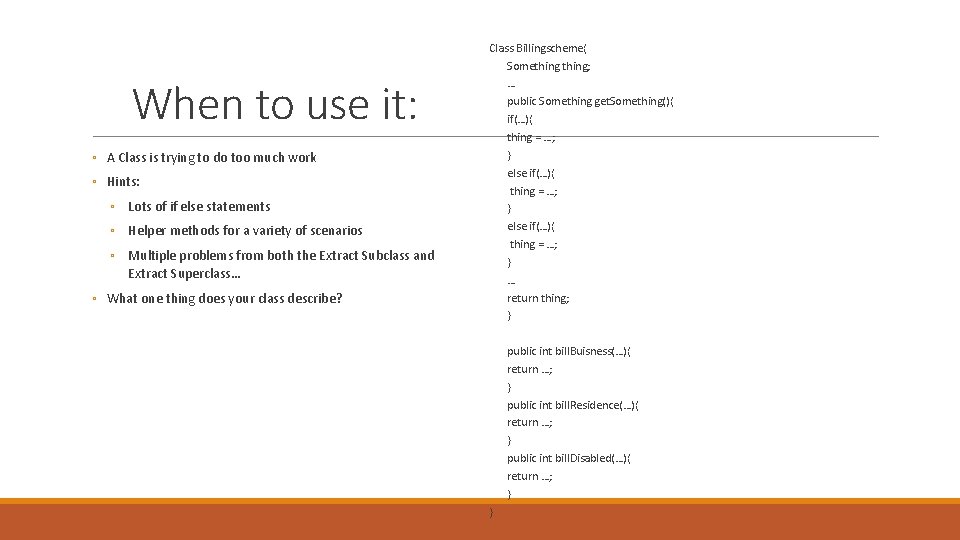
When to use it: ◦ A Class is trying to do too much work ◦ Hints: ◦ Lots of if else statements ◦ Helper methods for a variety of scenarios ◦ Multiple problems from both the Extract Subclass and Extract Superclass… ◦ What one thing does your class describe? Class Billingscheme{ Something; … public Something get. Something(){ if(…){ thing = …; } else if(…){ thing = …; } … return thing; } public int bill. Buisness(…){ return …; } public int bill. Residence(…){ return …; } public int bill. Disabled(…){ return …; } }
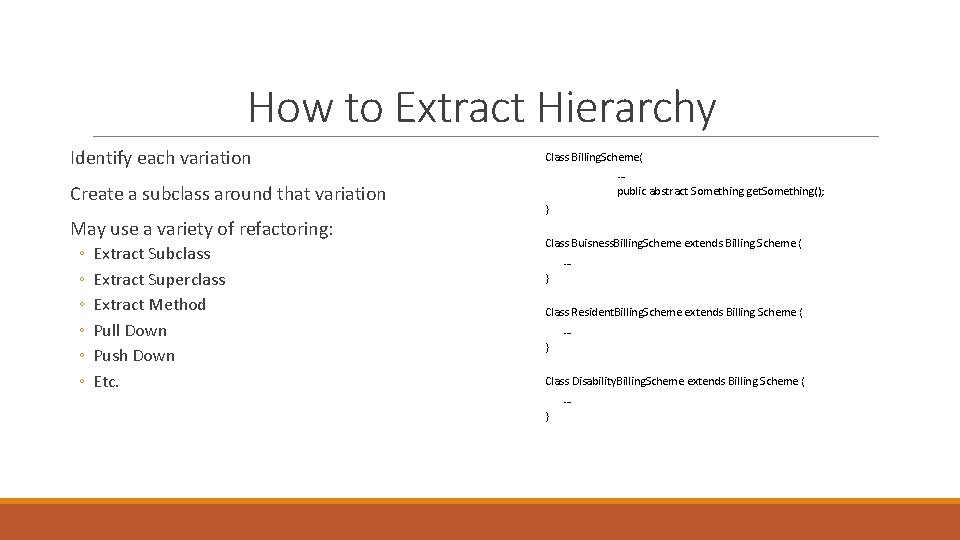
How to Extract Hierarchy Identify each variation Create a subclass around that variation May use a variety of refactoring: ◦ ◦ ◦ Extract Subclass Extract Superclass Extract Method Pull Down Push Down Etc. Class Billing. Scheme{ … public abstract Something get. Something(); } Class Buisness. Billing. Scheme extends Billing Scheme { … } Class Resident. Billing. Scheme extends Billing Scheme { … } Class Disability. Billing. Scheme extends Billing Scheme { … }
- Slides: 15