Exception Handling An Exception is an indication of
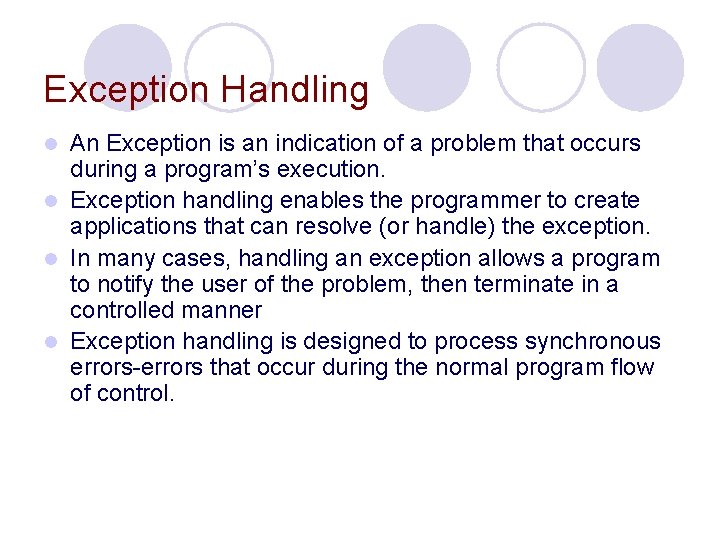
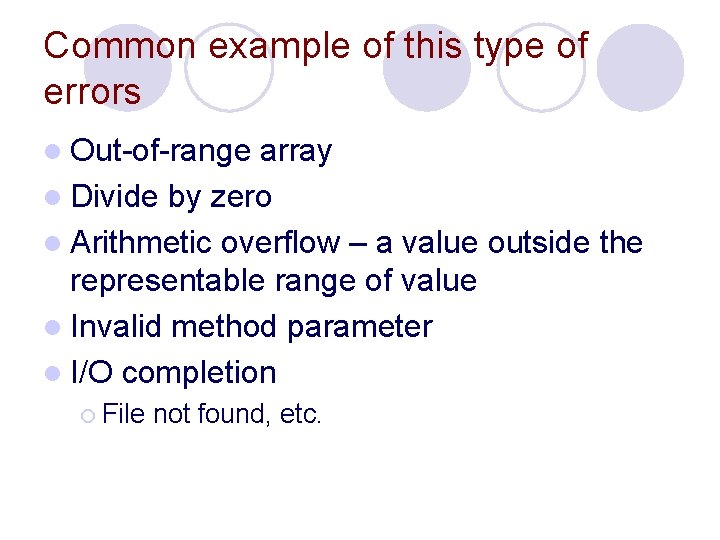
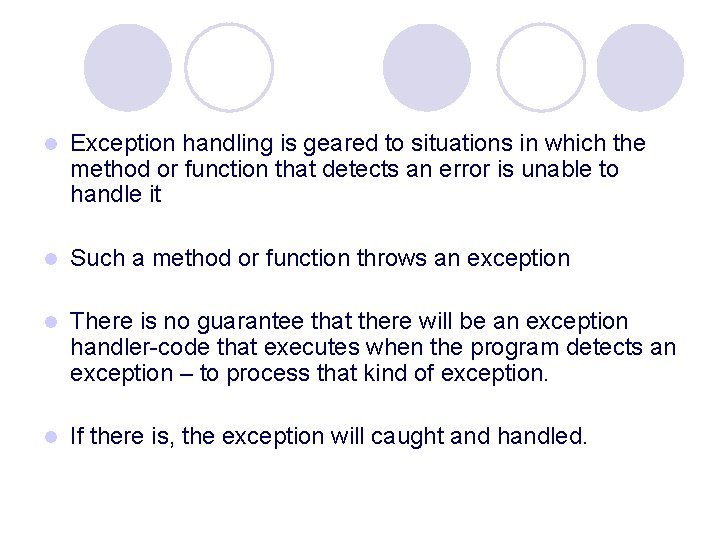
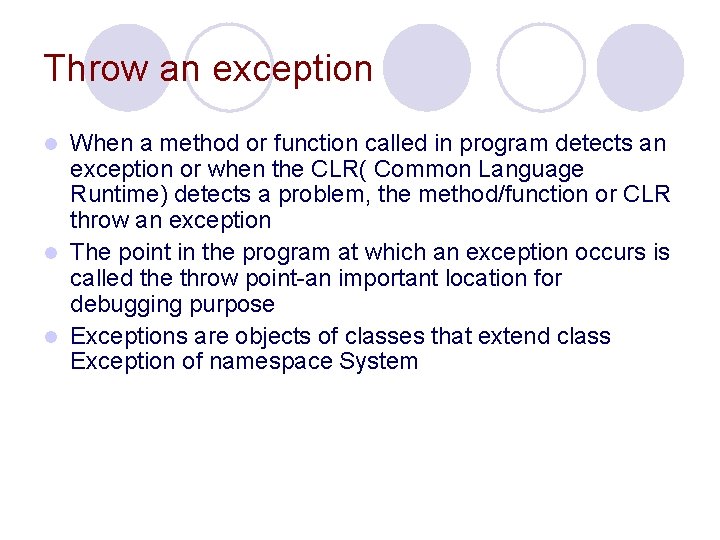
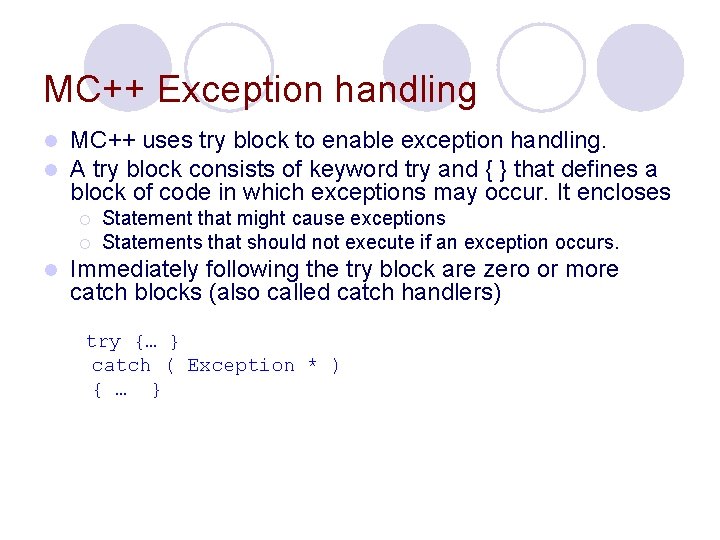
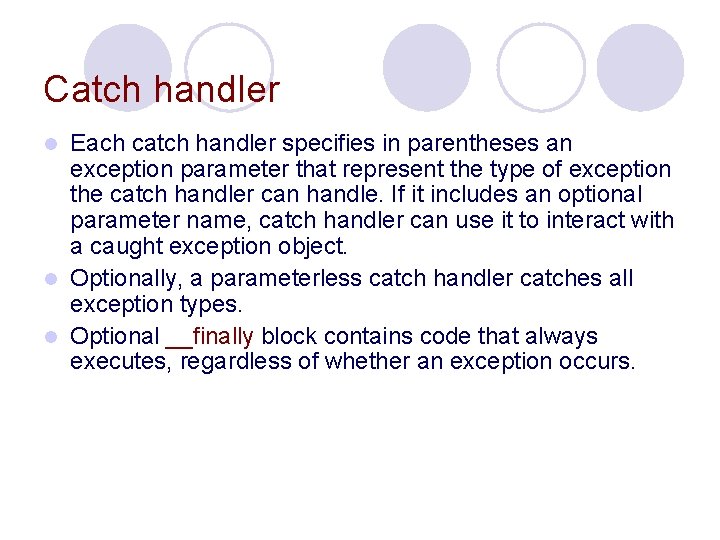
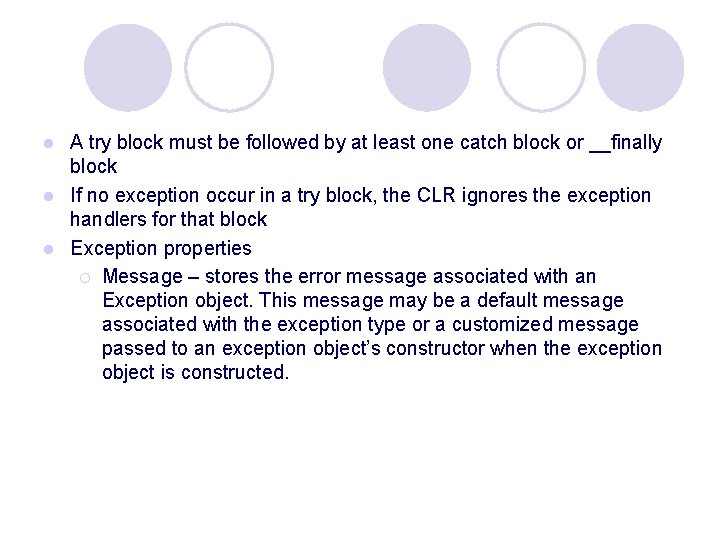
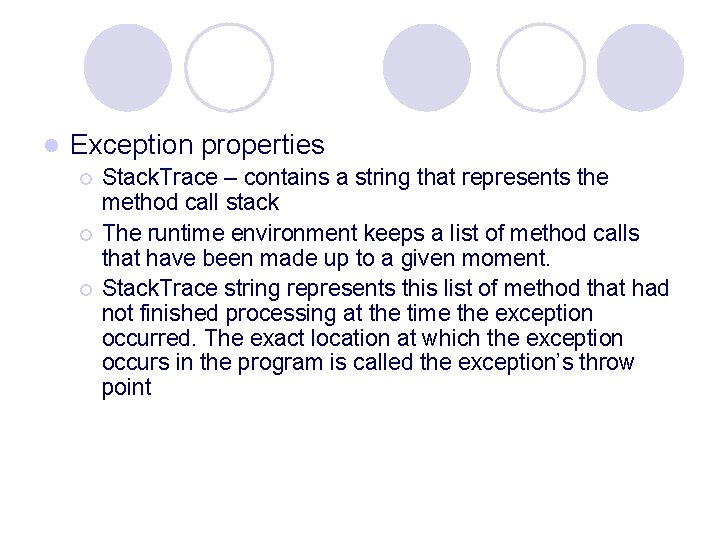
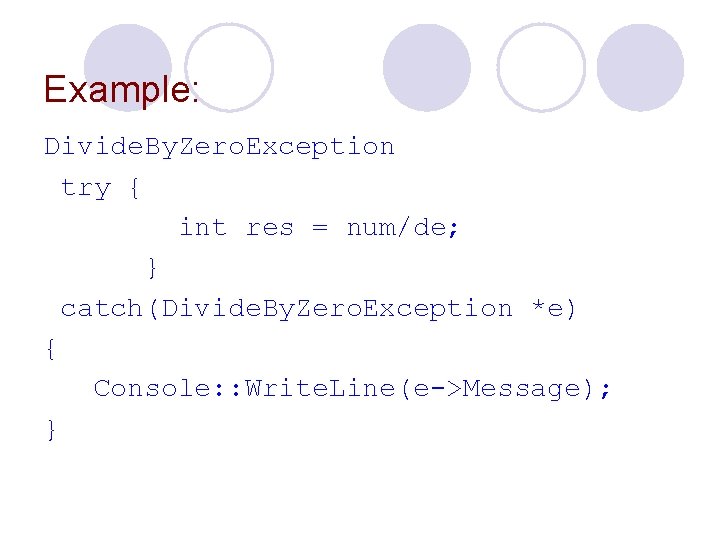
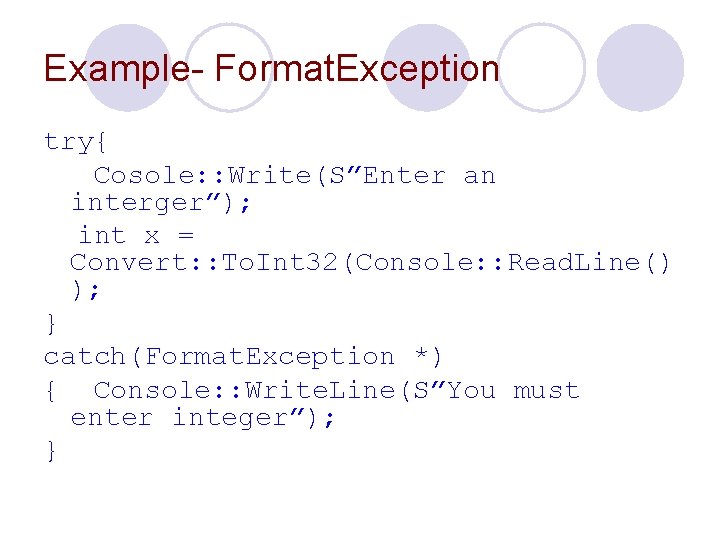
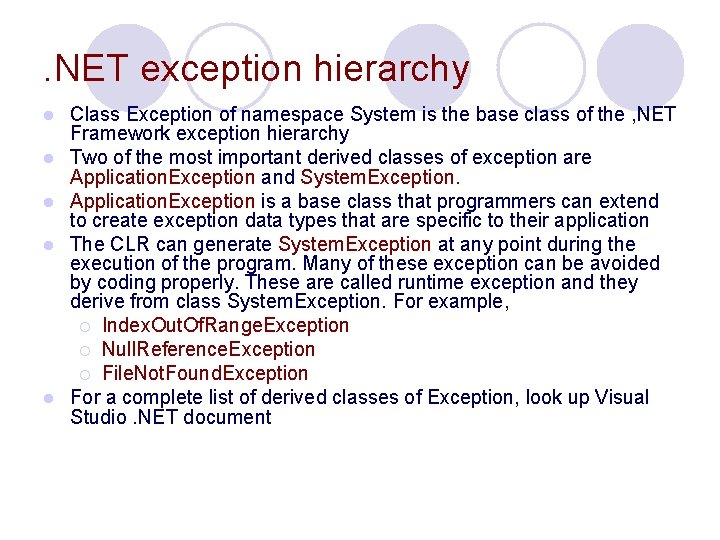
- Slides: 11
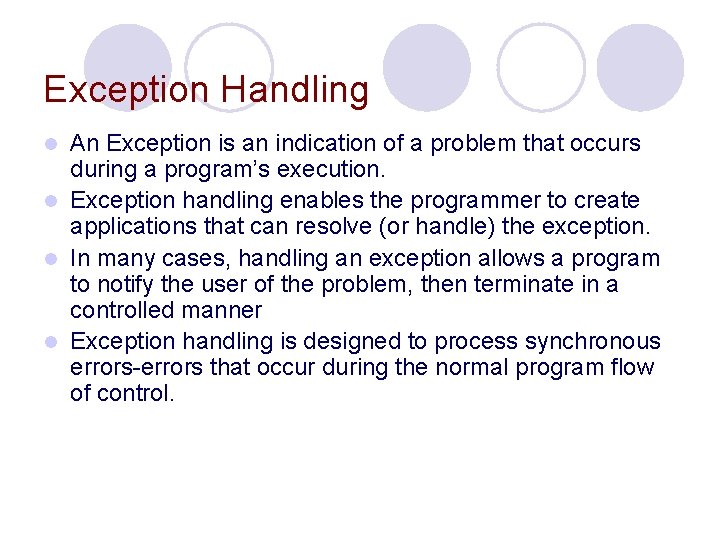
Exception Handling An Exception is an indication of a problem that occurs during a program’s execution. l Exception handling enables the programmer to create applications that can resolve (or handle) the exception. l In many cases, handling an exception allows a program to notify the user of the problem, then terminate in a controlled manner l Exception handling is designed to process synchronous errors-errors that occur during the normal program flow of control. l
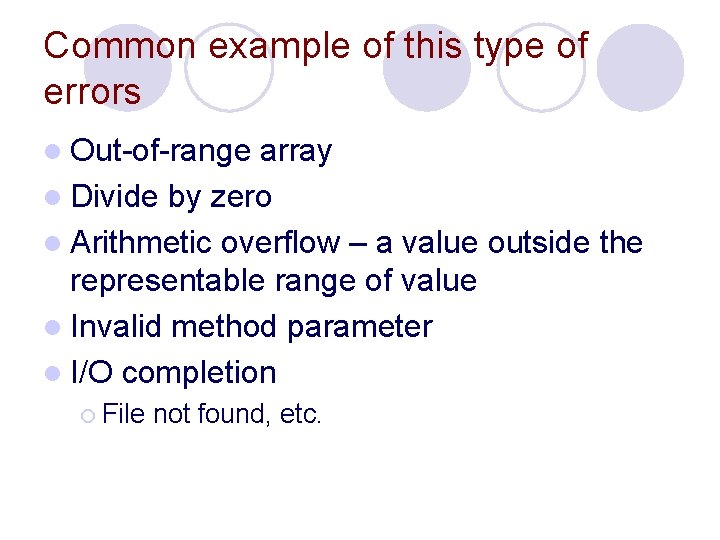
Common example of this type of errors l Out-of-range array l Divide by zero l Arithmetic overflow – a value outside the representable range of value l Invalid method parameter l I/O completion ¡ File not found, etc.
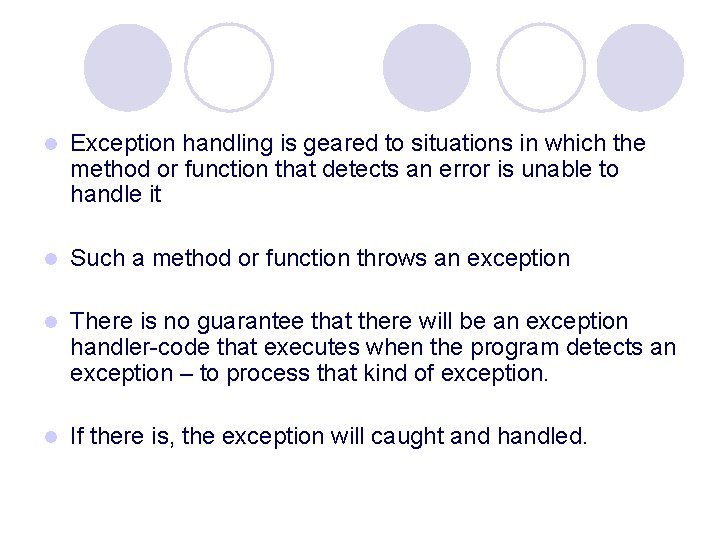
l Exception handling is geared to situations in which the method or function that detects an error is unable to handle it l Such a method or function throws an exception l There is no guarantee that there will be an exception handler-code that executes when the program detects an exception – to process that kind of exception. l If there is, the exception will caught and handled.
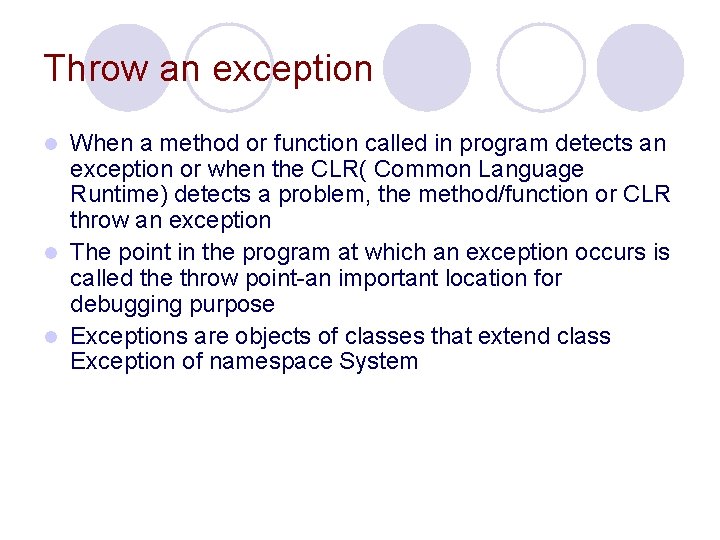
Throw an exception When a method or function called in program detects an exception or when the CLR( Common Language Runtime) detects a problem, the method/function or CLR throw an exception l The point in the program at which an exception occurs is called the throw point-an important location for debugging purpose l Exceptions are objects of classes that extend class Exception of namespace System l
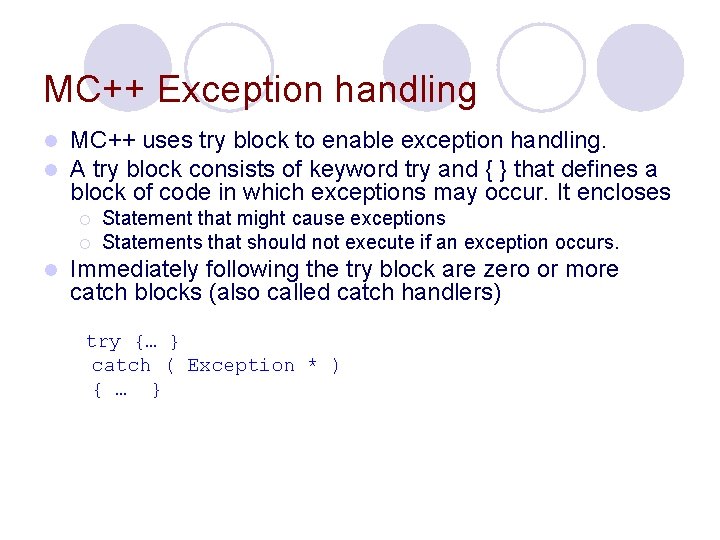
MC++ Exception handling l l MC++ uses try block to enable exception handling. A try block consists of keyword try and { } that defines a block of code in which exceptions may occur. It encloses ¡ ¡ l Statement that might cause exceptions Statements that should not execute if an exception occurs. Immediately following the try block are zero or more catch blocks (also called catch handlers) try {… } catch ( Exception * ) { … }
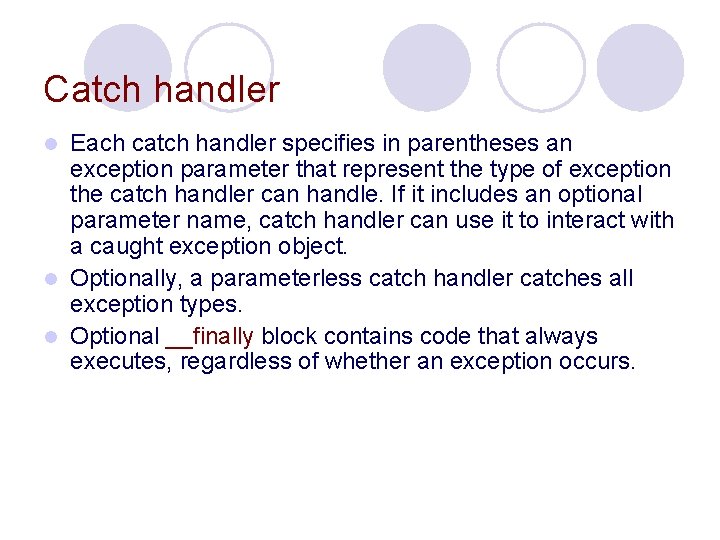
Catch handler Each catch handler specifies in parentheses an exception parameter that represent the type of exception the catch handler can handle. If it includes an optional parameter name, catch handler can use it to interact with a caught exception object. l Optionally, a parameterless catch handler catches all exception types. l Optional __finally block contains code that always executes, regardless of whether an exception occurs. l
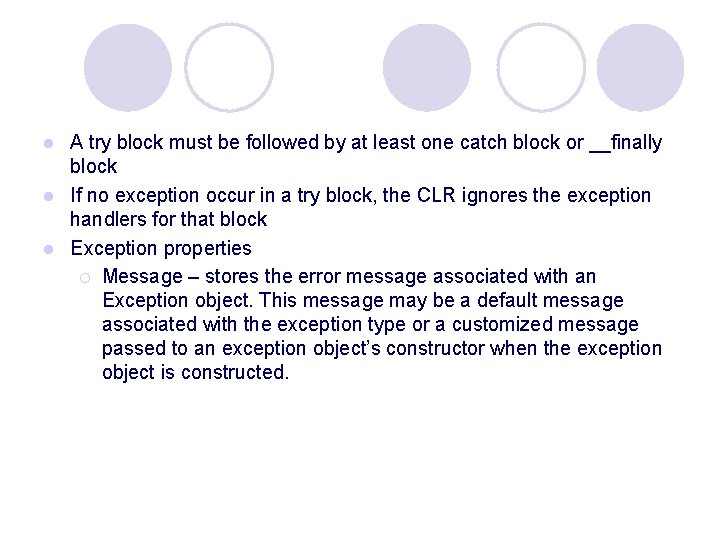
A try block must be followed by at least one catch block or __finally block l If no exception occur in a try block, the CLR ignores the exception handlers for that block l Exception properties ¡ Message – stores the error message associated with an Exception object. This message may be a default message associated with the exception type or a customized message passed to an exception object’s constructor when the exception object is constructed. l
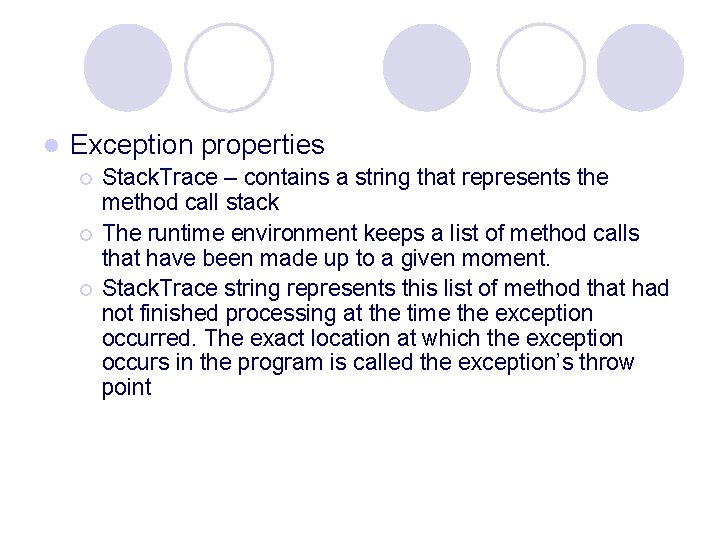
l Exception properties ¡ ¡ ¡ Stack. Trace – contains a string that represents the method call stack The runtime environment keeps a list of method calls that have been made up to a given moment. Stack. Trace string represents this list of method that had not finished processing at the time the exception occurred. The exact location at which the exception occurs in the program is called the exception’s throw point
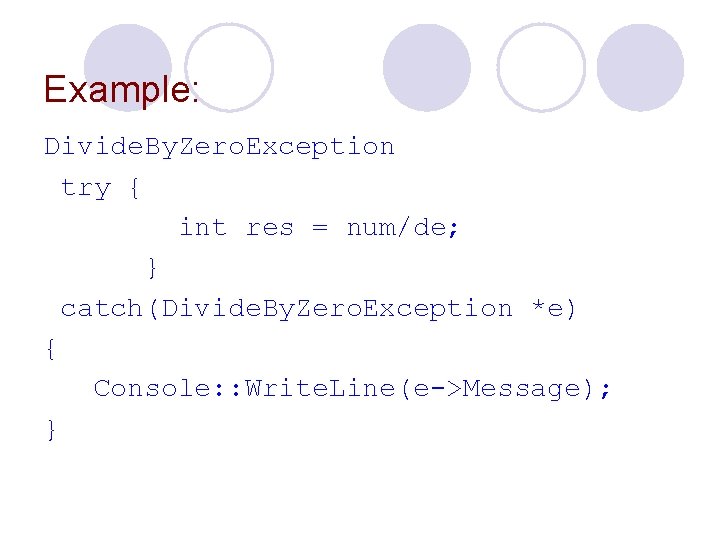
Example: Divide. By. Zero. Exception try { int res = num/de; } catch(Divide. By. Zero. Exception *e) { Console: : Write. Line(e->Message); }
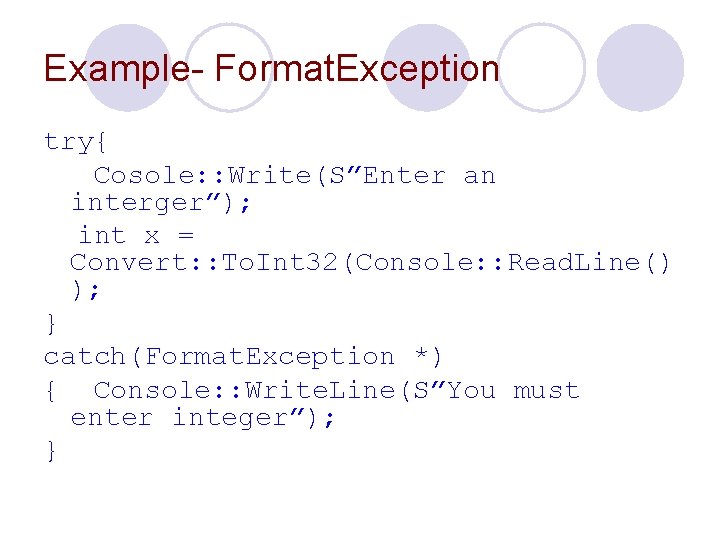
Example- Format. Exception try{ Cosole: : Write(S”Enter an interger”); int x = Convert: : To. Int 32(Console: : Read. Line() ); } catch(Format. Exception *) { Console: : Write. Line(S”You must enter integer”); }
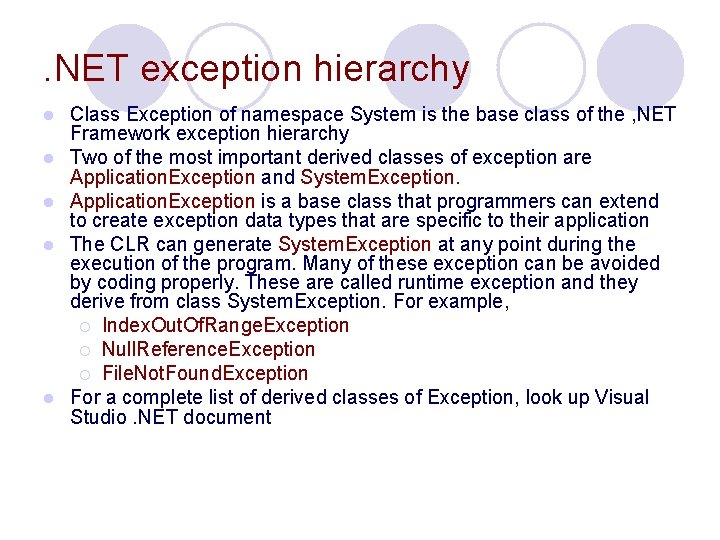
. NET exception hierarchy l l l Class Exception of namespace System is the base class of the , NET Framework exception hierarchy Two of the most important derived classes of exception are Application. Exception and System. Exception. Application. Exception is a base class that programmers can extend to create exception data types that are specific to their application The CLR can generate System. Exception at any point during the execution of the program. Many of these exception can be avoided by coding properly. These are called runtime exception and they derive from class System. Exception. For example, ¡ Index. Out. Of. Range. Exception ¡ Null. Reference. Exception ¡ File. Not. Found. Exception For a complete list of derived classes of Exception, look up Visual Studio. NET document
No irq handler for vector
Apa itu exception
Exception handling in ada
Exception handling pl/sql
Php exception handler
Php global exception handler
Vb.net exception handling
Exception handling pada java
Exception handling in vb net
Exception handling in java
Systemic contraindications of extraction
Resuscitation of newborn procedure