Decorator Pattern Decorator pattern allows a user to
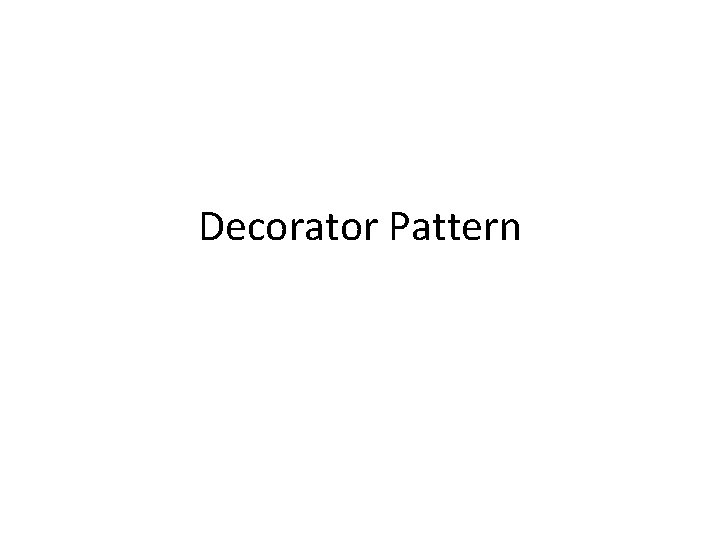
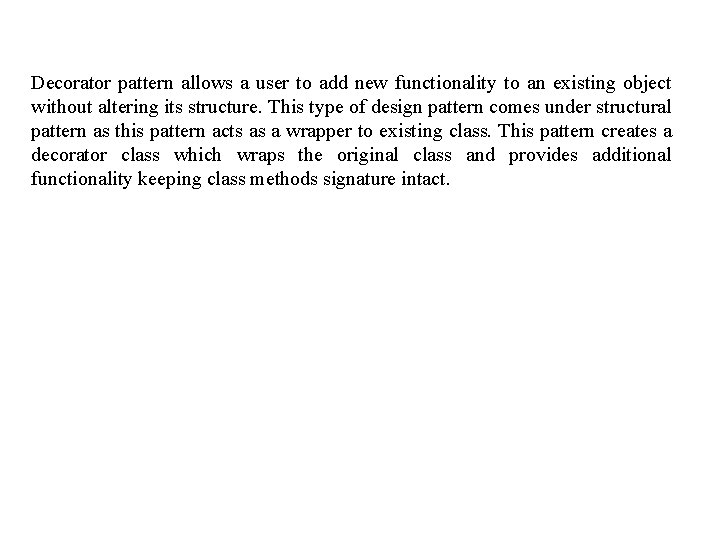
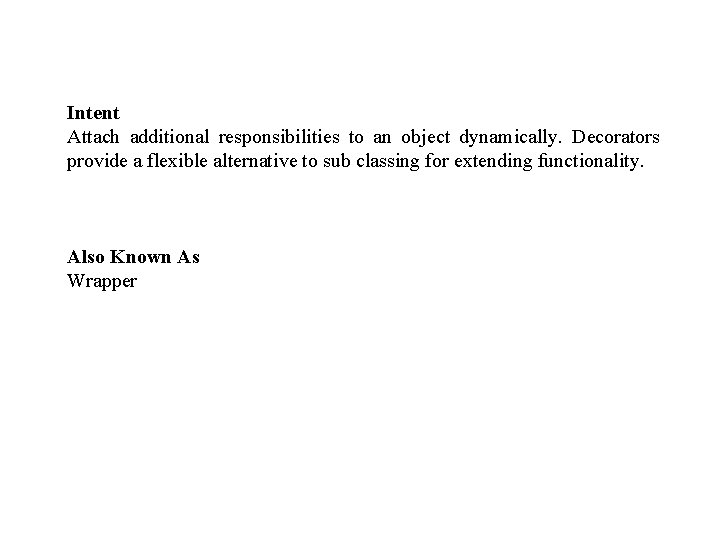
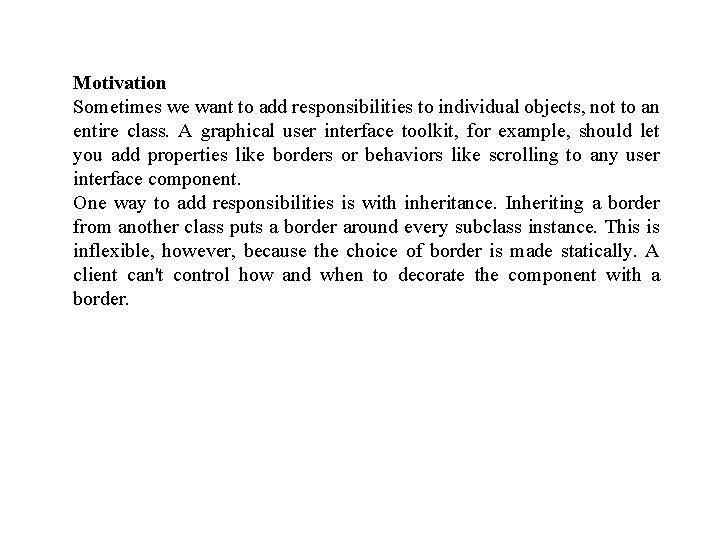
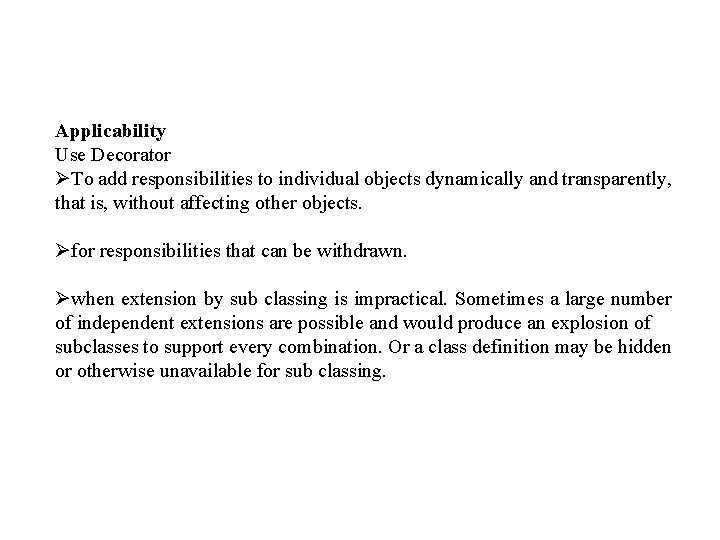
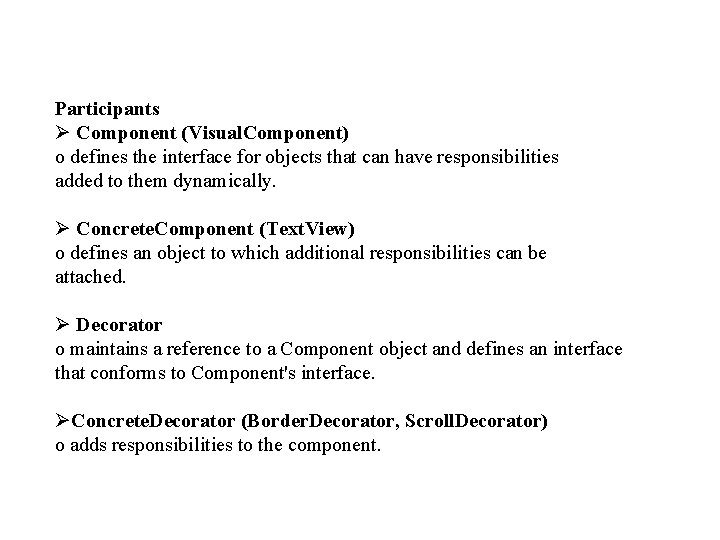
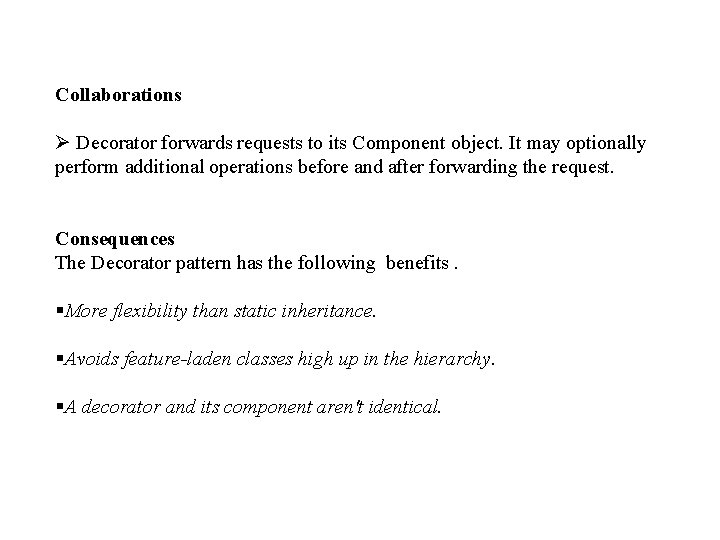
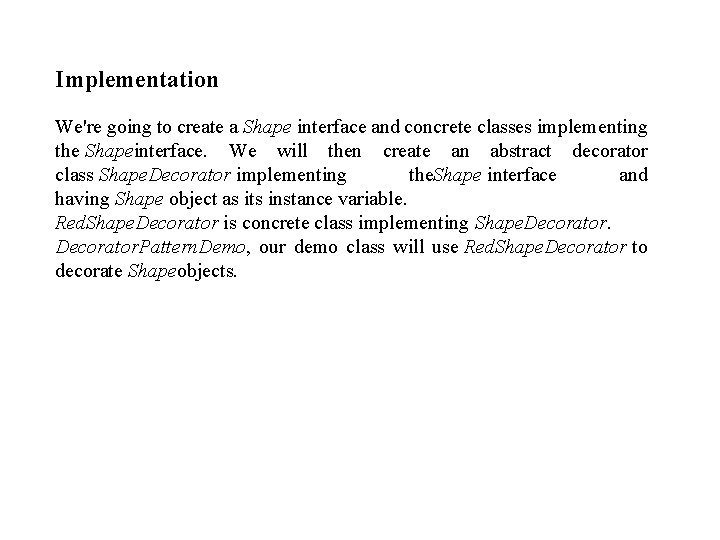
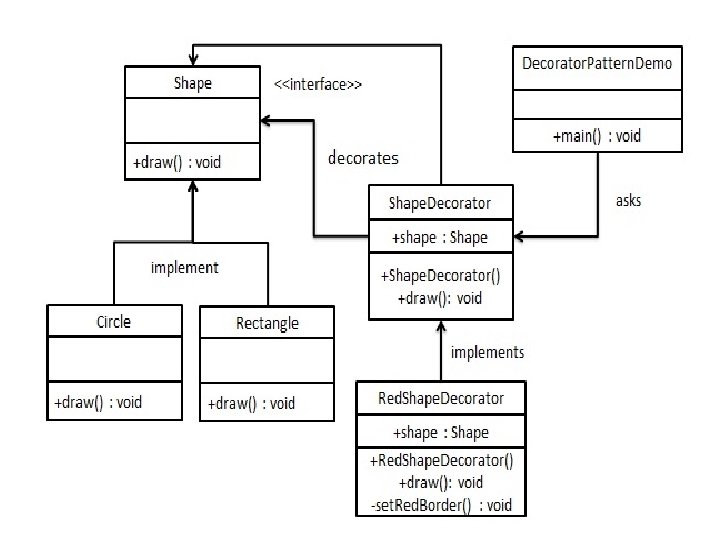
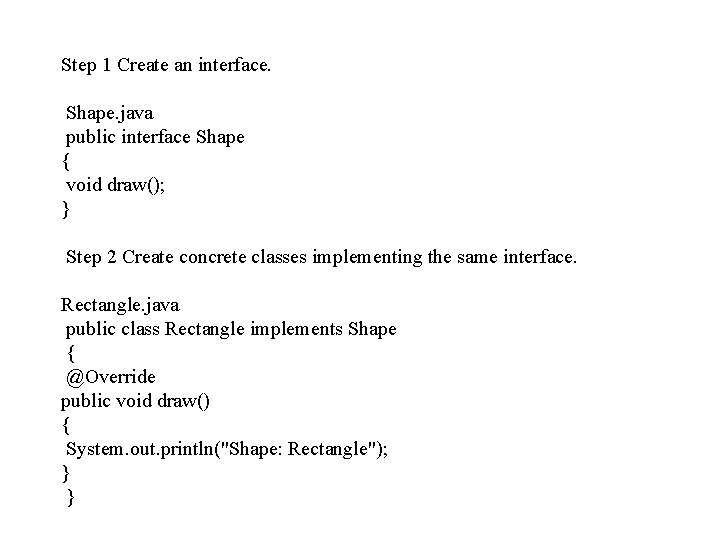
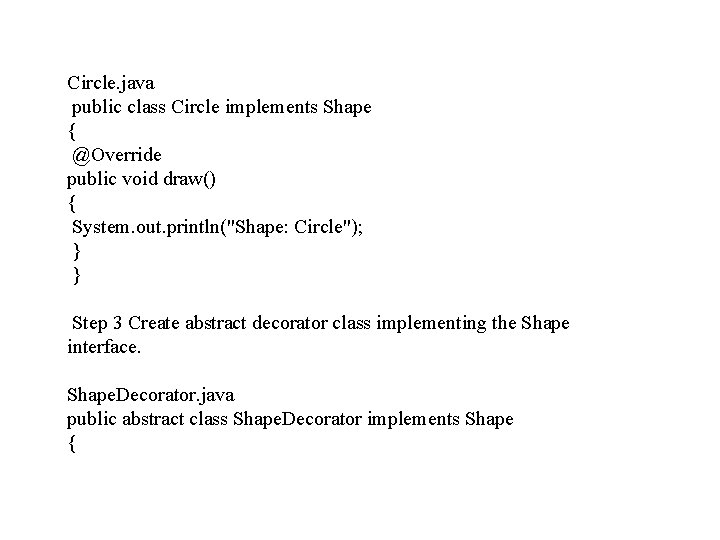
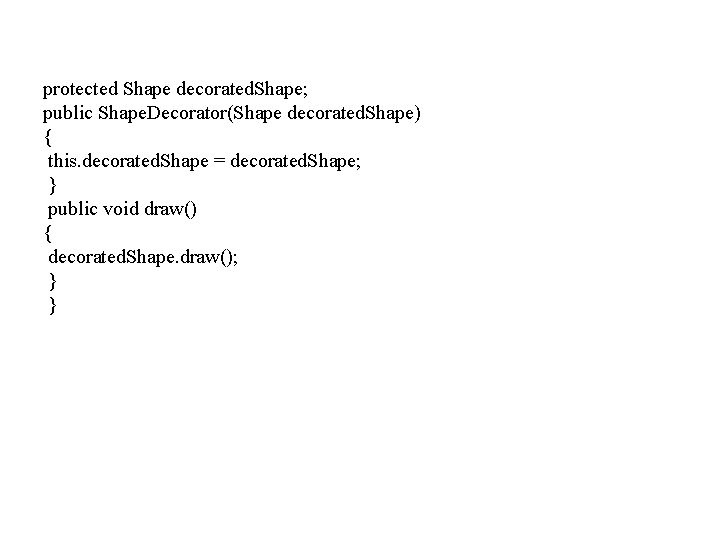
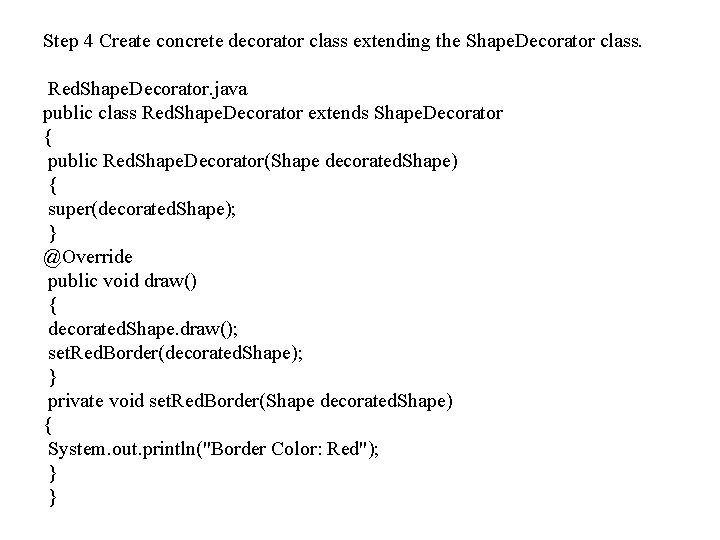
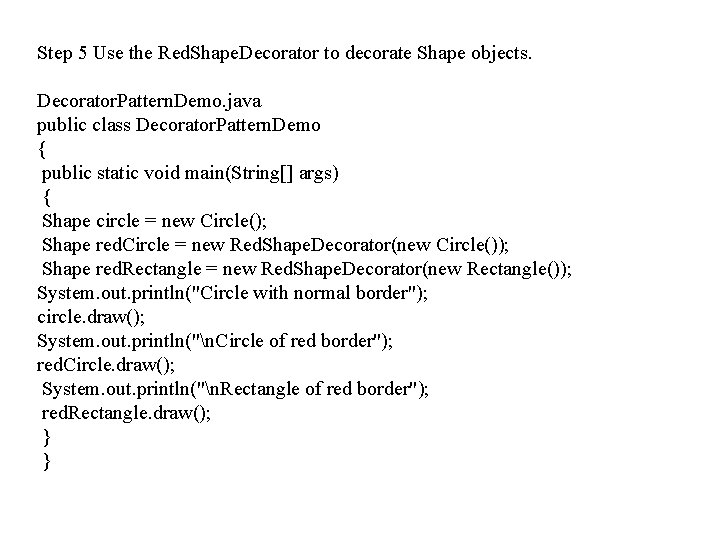
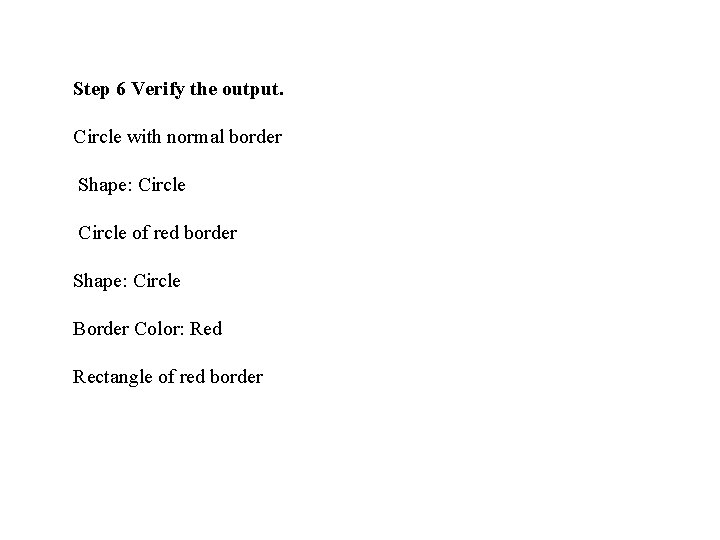
- Slides: 15
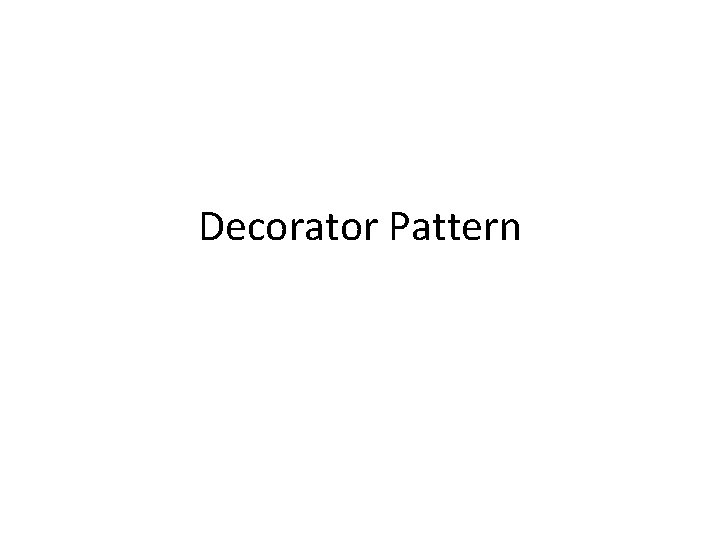
Decorator Pattern
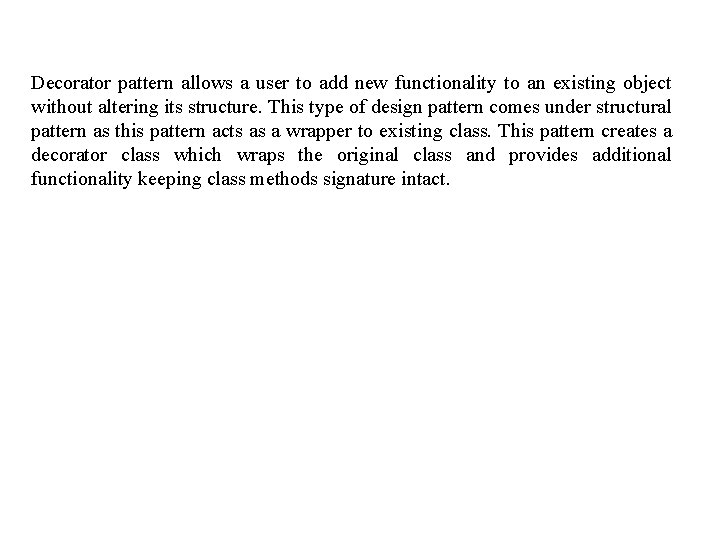
Decorator pattern allows a user to add new functionality to an existing object without altering its structure. This type of design pattern comes under structural pattern as this pattern acts as a wrapper to existing class. This pattern creates a decorator class which wraps the original class and provides additional functionality keeping class methods signature intact.
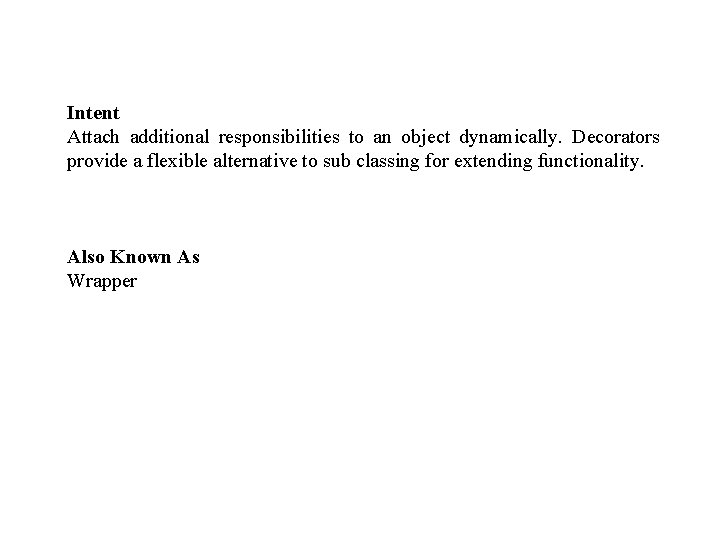
Intent Attach additional responsibilities to an object dynamically. Decorators provide a flexible alternative to sub classing for extending functionality. Also Known As Wrapper
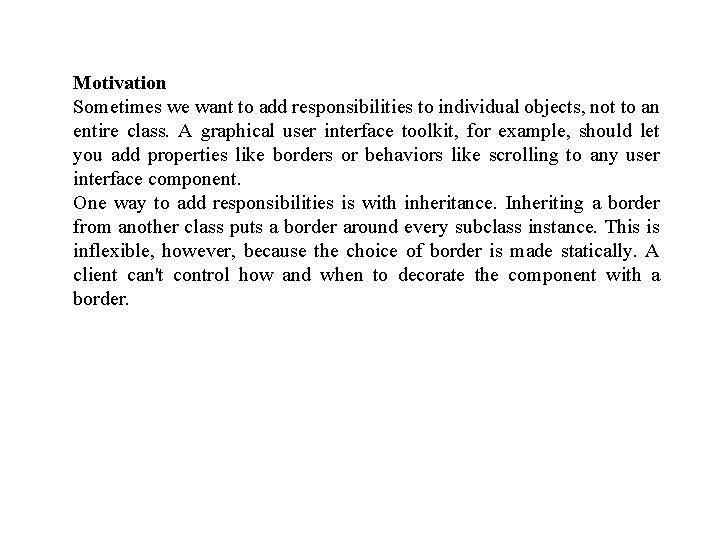
Motivation Sometimes we want to add responsibilities to individual objects, not to an entire class. A graphical user interface toolkit, for example, should let you add properties like borders or behaviors like scrolling to any user interface component. One way to add responsibilities is with inheritance. Inheriting a border from another class puts a border around every subclass instance. This is inflexible, however, because the choice of border is made statically. A client can't control how and when to decorate the component with a border.
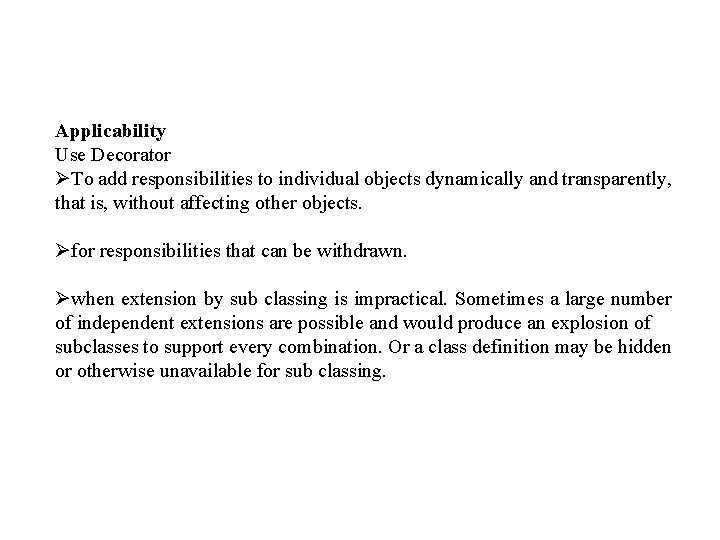
Applicability Use Decorator ØTo add responsibilities to individual objects dynamically and transparently, that is, without affecting other objects. Øfor responsibilities that can be withdrawn. Øwhen extension by sub classing is impractical. Sometimes a large number of independent extensions are possible and would produce an explosion of subclasses to support every combination. Or a class definition may be hidden or otherwise unavailable for sub classing.
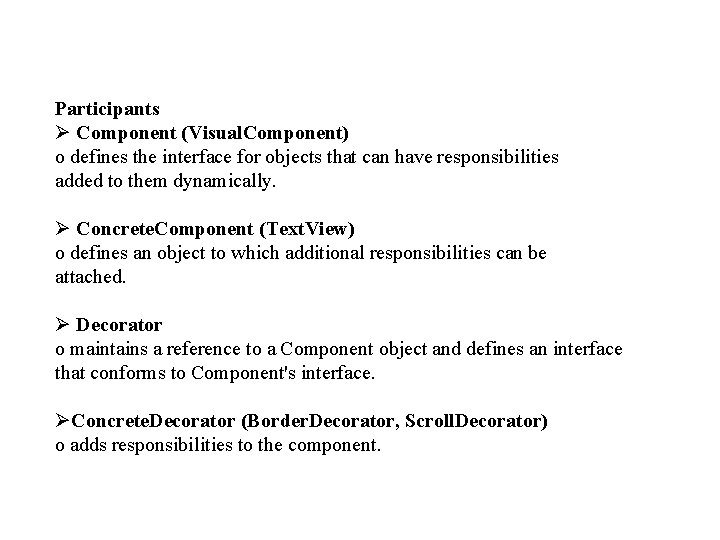
Participants Ø Component (Visual. Component) o defines the interface for objects that can have responsibilities added to them dynamically. Ø Concrete. Component (Text. View) o defines an object to which additional responsibilities can be attached. Ø Decorator o maintains a reference to a Component object and defines an interface that conforms to Component's interface. ØConcrete. Decorator (Border. Decorator, Scroll. Decorator) o adds responsibilities to the component.
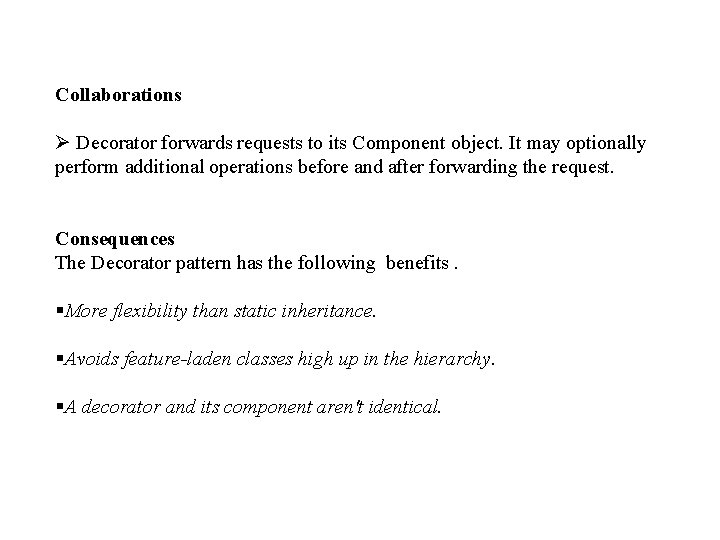
Collaborations Ø Decorator forwards requests to its Component object. It may optionally perform additional operations before and after forwarding the request. Consequences The Decorator pattern has the following benefits. §More flexibility than static inheritance. §Avoids feature-laden classes high up in the hierarchy. §A decorator and its component aren't identical.
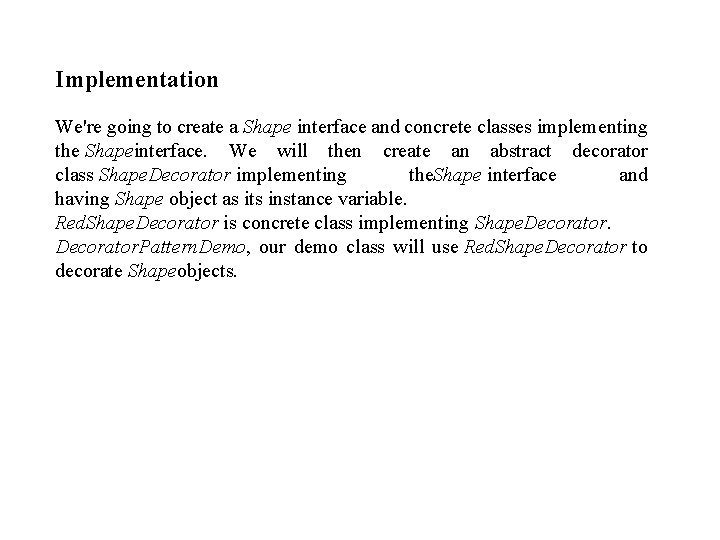
Implementation We're going to create a Shape interface and concrete classes implementing the Shapeinterface. We will then create an abstract decorator class Shape. Decorator implementing the. Shape interface and having Shape object as its instance variable. Red. Shape. Decorator is concrete class implementing Shape. Decorator. Pattern. Demo, our demo class will use Red. Shape. Decorator to decorate Shapeobjects.
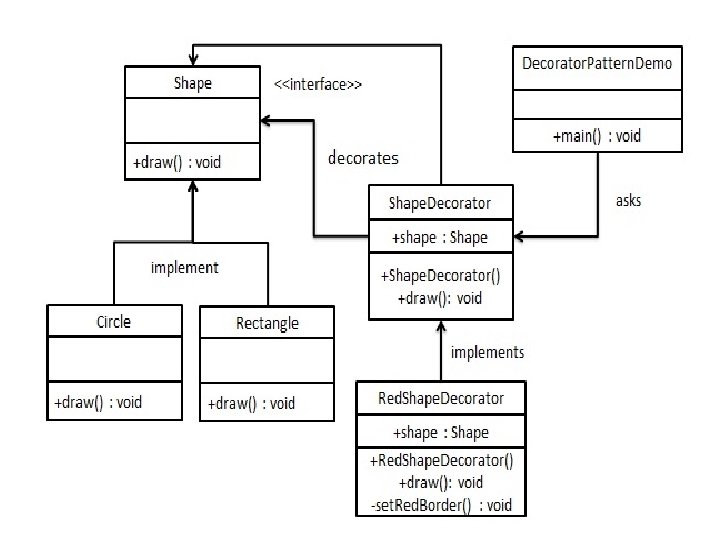
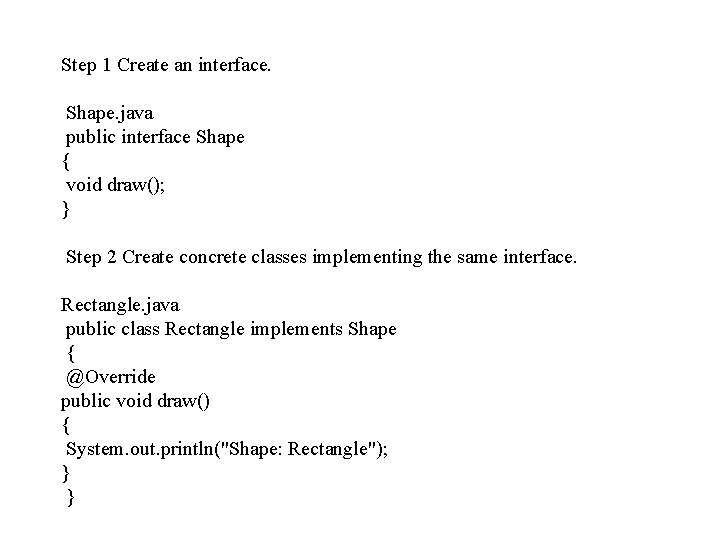
Step 1 Create an interface. Shape. java public interface Shape { void draw(); } Step 2 Create concrete classes implementing the same interface. Rectangle. java public class Rectangle implements Shape { @Override public void draw() { System. out. println("Shape: Rectangle"); } }
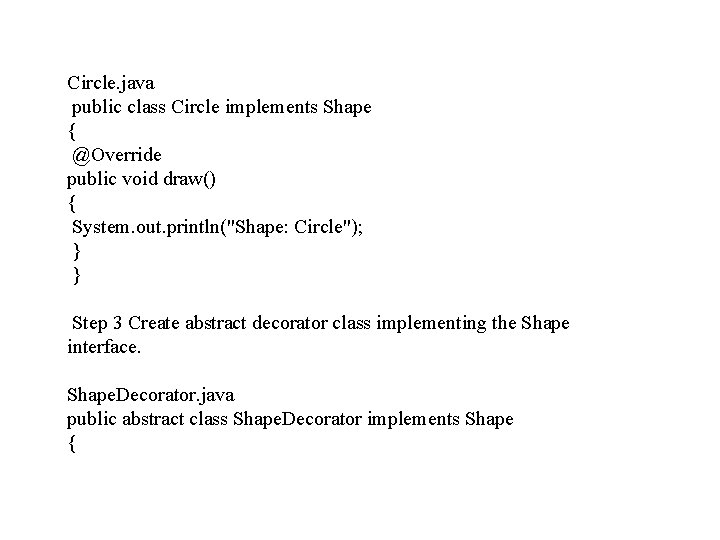
Circle. java public class Circle implements Shape { @Override public void draw() { System. out. println("Shape: Circle"); } } Step 3 Create abstract decorator class implementing the Shape interface. Shape. Decorator. java public abstract class Shape. Decorator implements Shape {
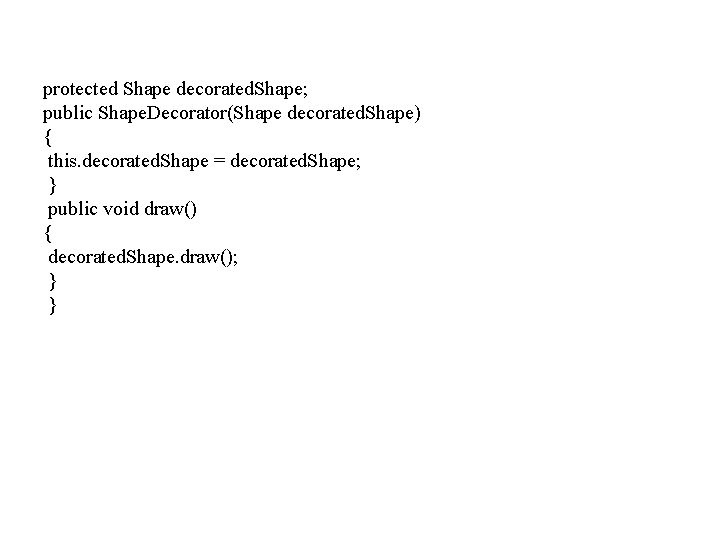
protected Shape decorated. Shape; public Shape. Decorator(Shape decorated. Shape) { this. decorated. Shape = decorated. Shape; } public void draw() { decorated. Shape. draw(); } }
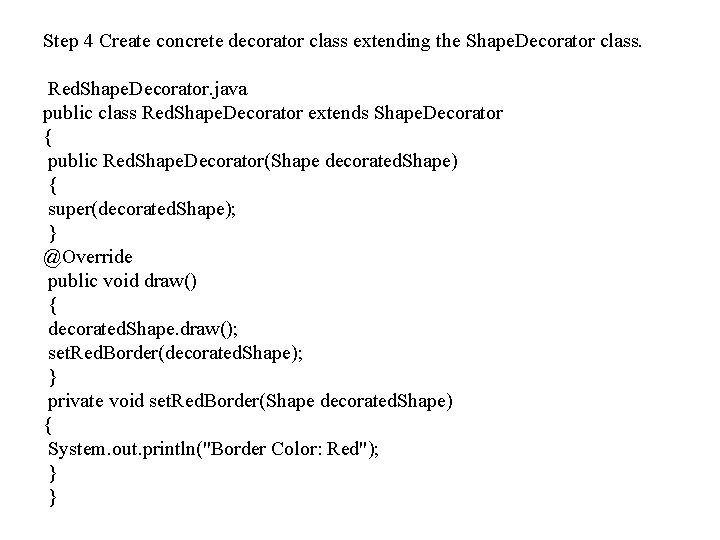
Step 4 Create concrete decorator class extending the Shape. Decorator class. Red. Shape. Decorator. java public class Red. Shape. Decorator extends Shape. Decorator { public Red. Shape. Decorator(Shape decorated. Shape) { super(decorated. Shape); } @Override public void draw() { decorated. Shape. draw(); set. Red. Border(decorated. Shape); } private void set. Red. Border(Shape decorated. Shape) { System. out. println("Border Color: Red"); } }
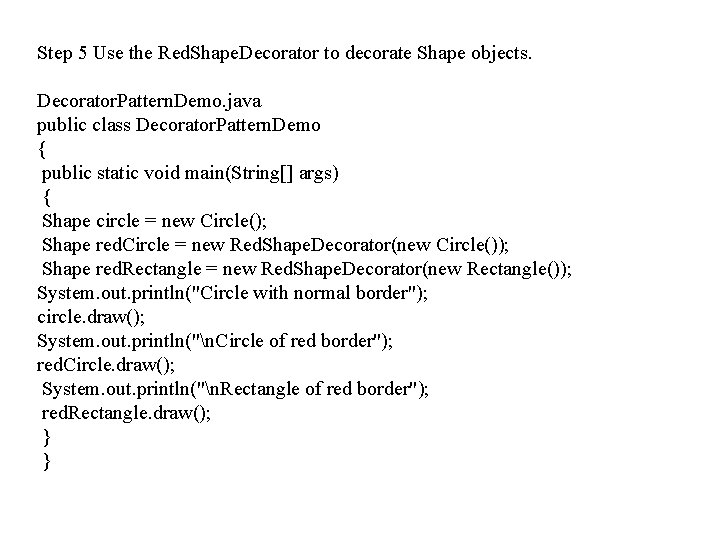
Step 5 Use the Red. Shape. Decorator to decorate Shape objects. Decorator. Pattern. Demo. java public class Decorator. Pattern. Demo { public static void main(String[] args) { Shape circle = new Circle(); Shape red. Circle = new Red. Shape. Decorator(new Circle()); Shape red. Rectangle = new Red. Shape. Decorator(new Rectangle()); System. out. println("Circle with normal border"); circle. draw(); System. out. println("n. Circle of red border"); red. Circle. draw(); System. out. println("n. Rectangle of red border"); red. Rectangle. draw(); } }
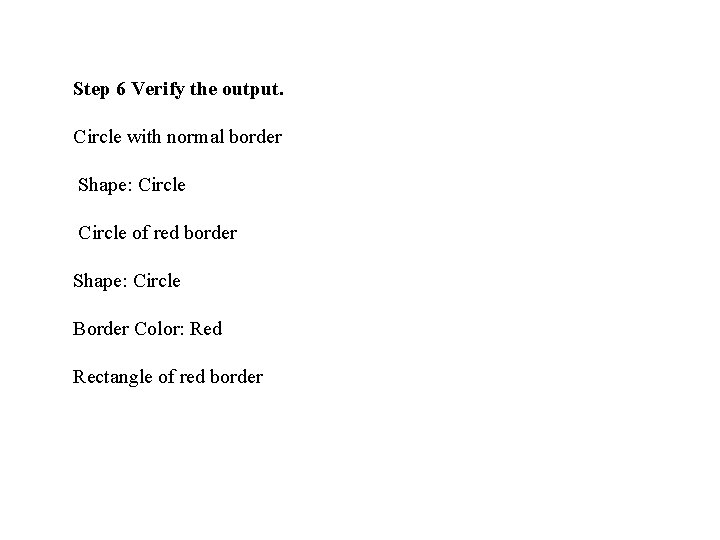
Step 6 Verify the output. Circle with normal border Shape: Circle of red border Shape: Circle Border Color: Red Rectangle of red border
Java io decorator pattern
Slidetodoc.com
Multimedia software uses
Sharing of diverse information through universal web access
Single user and multi user operating system
Operating systems
Hashing
Hammock carry drawing
Inheritance allows
The first trial of a controlled experiment allows
A lan allows several lans to be connected
Identify trust boundaries
The locus of points idea allows
Distributed file systems
Direct mapping
Trackball and thumbwheels