CSC 243 Java Programming Spring 2014 March 2014
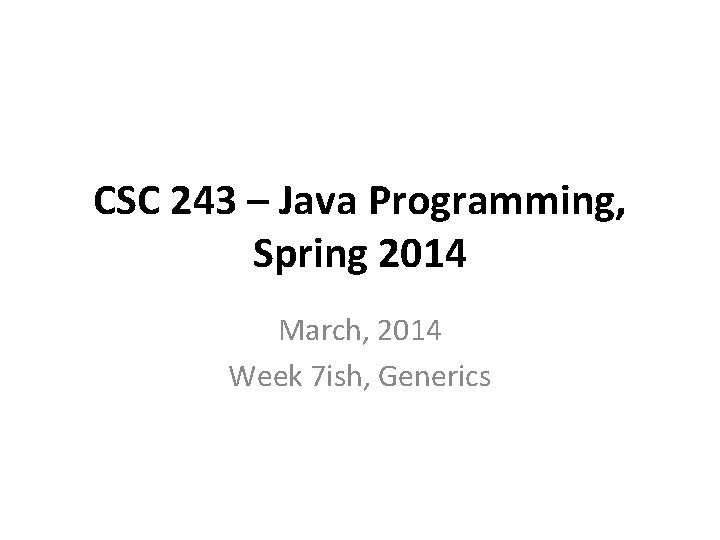
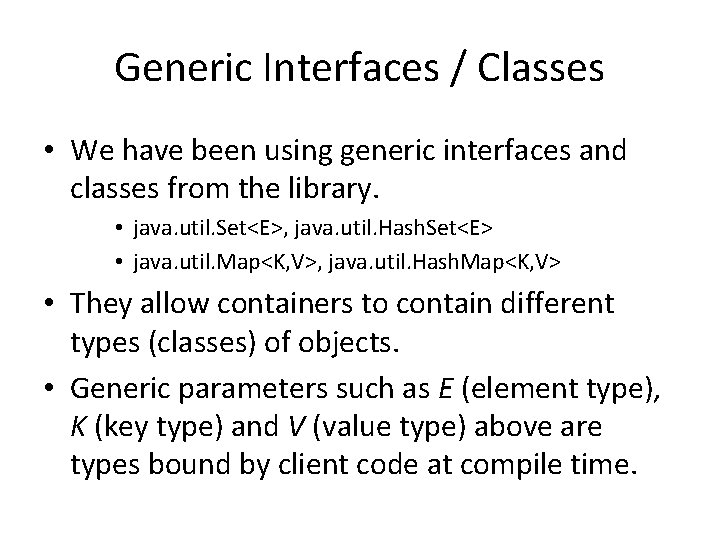
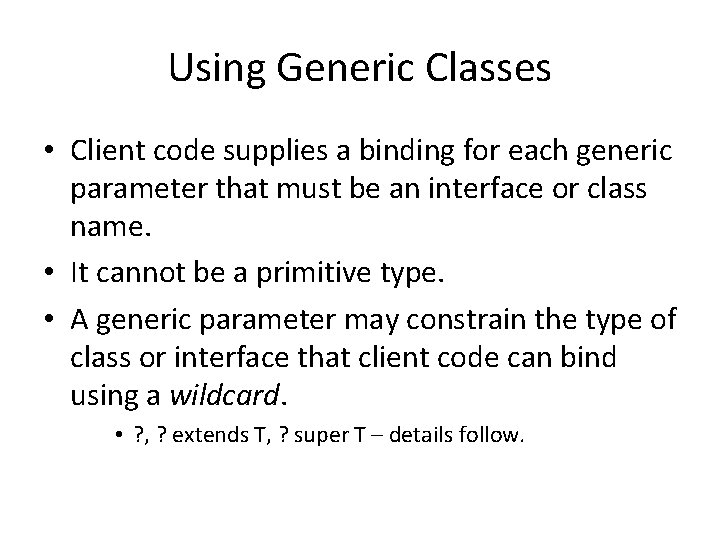
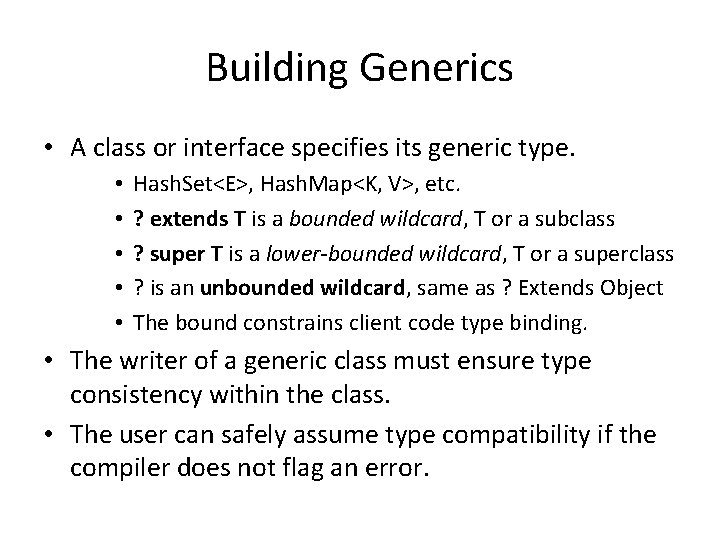
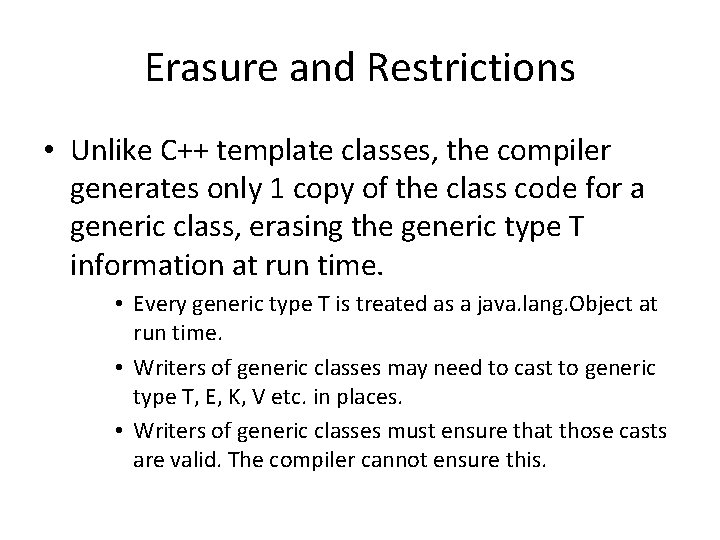
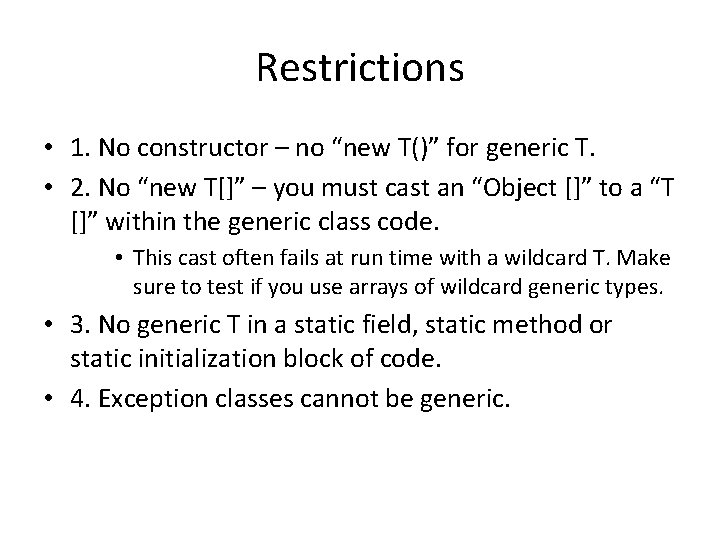
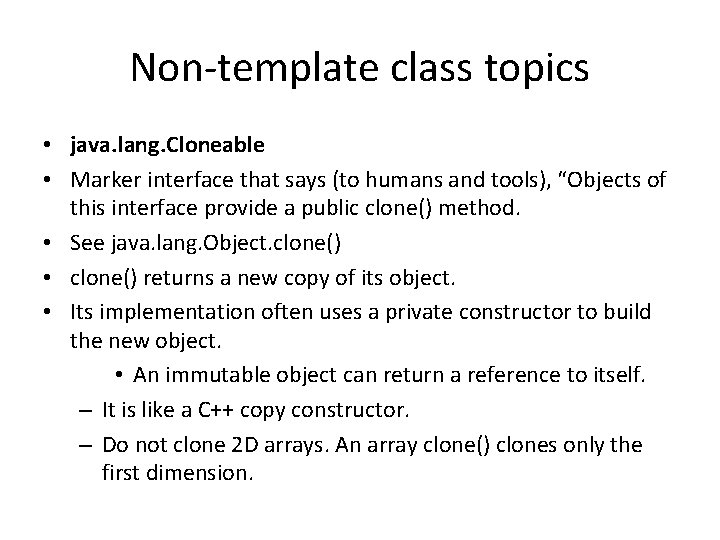
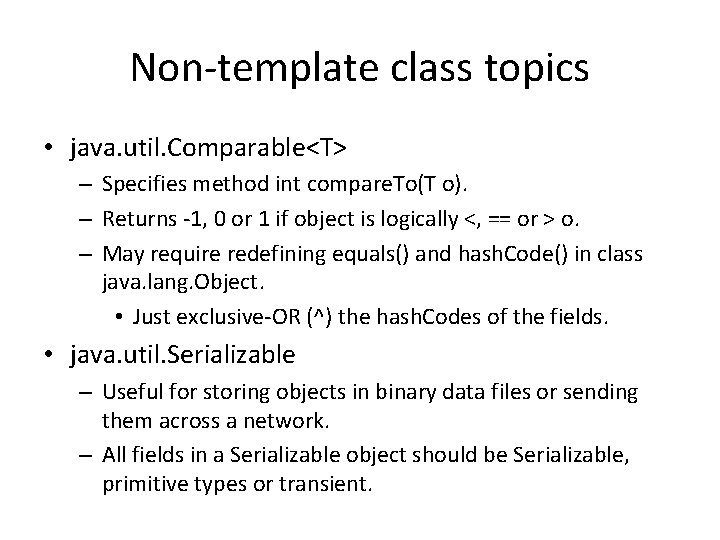
- Slides: 8
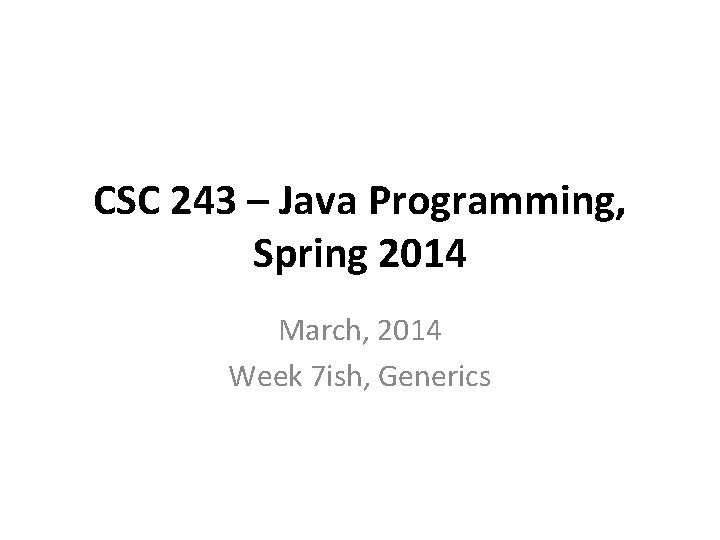
CSC 243 – Java Programming, Spring 2014 March, 2014 Week 7 ish, Generics
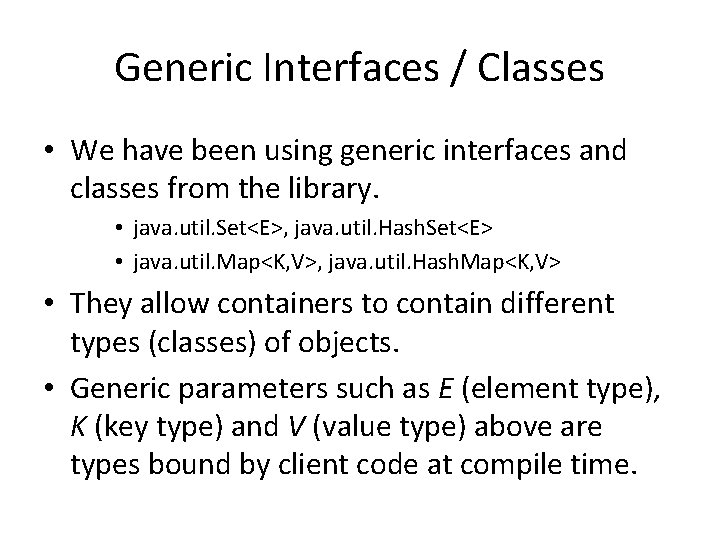
Generic Interfaces / Classes • We have been using generic interfaces and classes from the library. • java. util. Set<E>, java. util. Hash. Set<E> • java. util. Map<K, V>, java. util. Hash. Map<K, V> • They allow containers to contain different types (classes) of objects. • Generic parameters such as E (element type), K (key type) and V (value type) above are types bound by client code at compile time.
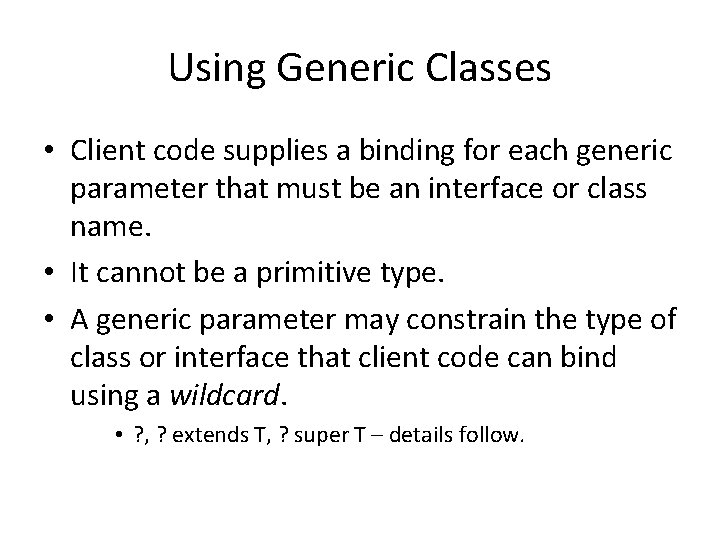
Using Generic Classes • Client code supplies a binding for each generic parameter that must be an interface or class name. • It cannot be a primitive type. • A generic parameter may constrain the type of class or interface that client code can bind using a wildcard. • ? , ? extends T, ? super T – details follow.
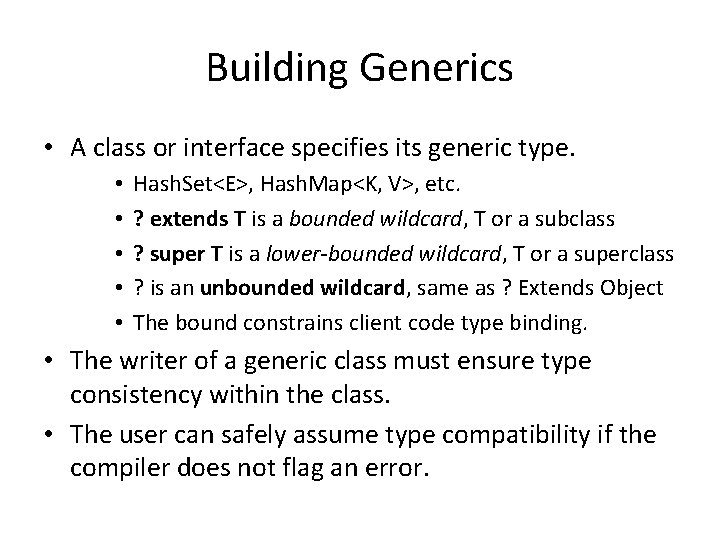
Building Generics • A class or interface specifies its generic type. • • • Hash. Set<E>, Hash. Map<K, V>, etc. ? extends T is a bounded wildcard, T or a subclass ? super T is a lower-bounded wildcard, T or a superclass ? is an unbounded wildcard, same as ? Extends Object The bound constrains client code type binding. • The writer of a generic class must ensure type consistency within the class. • The user can safely assume type compatibility if the compiler does not flag an error.
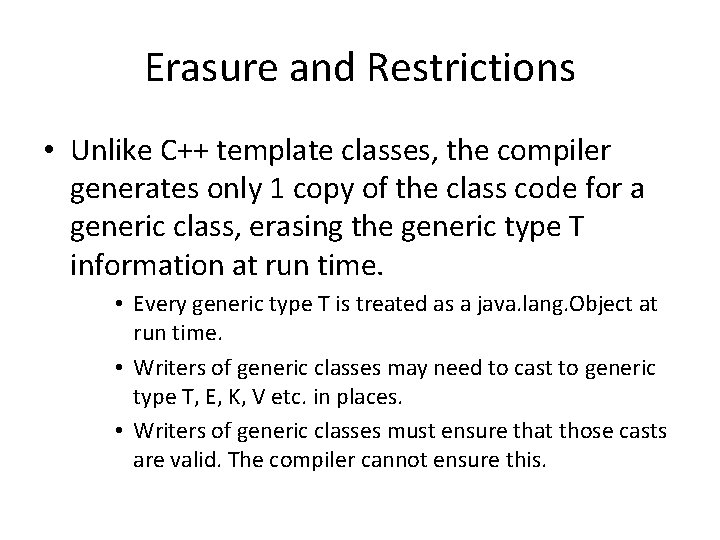
Erasure and Restrictions • Unlike C++ template classes, the compiler generates only 1 copy of the class code for a generic class, erasing the generic type T information at run time. • Every generic type T is treated as a java. lang. Object at run time. • Writers of generic classes may need to cast to generic type T, E, K, V etc. in places. • Writers of generic classes must ensure that those casts are valid. The compiler cannot ensure this.
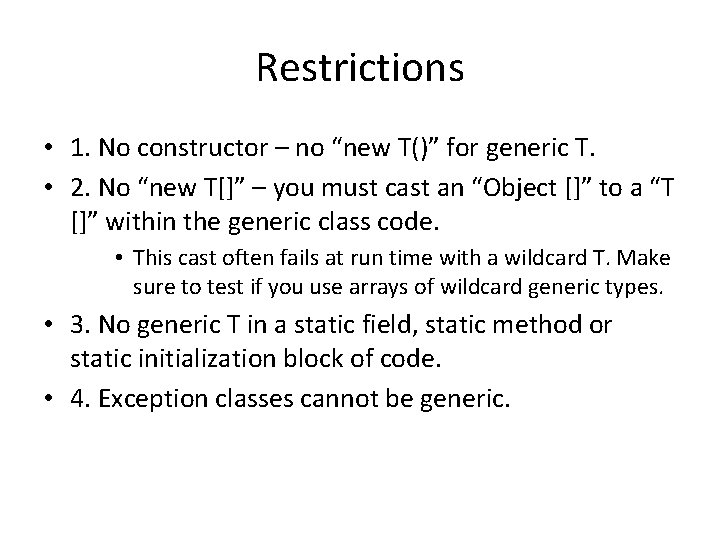
Restrictions • 1. No constructor – no “new T()” for generic T. • 2. No “new T[]” – you must cast an “Object []” to a “T []” within the generic class code. • This cast often fails at run time with a wildcard T. Make sure to test if you use arrays of wildcard generic types. • 3. No generic T in a static field, static method or static initialization block of code. • 4. Exception classes cannot be generic.
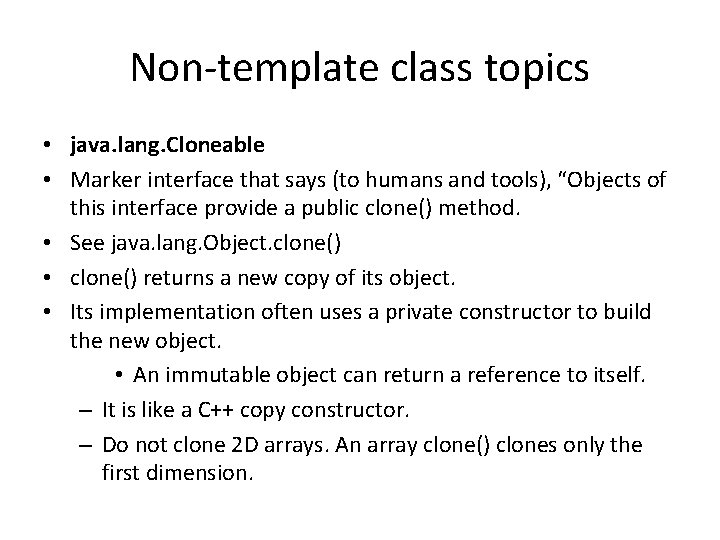
Non-template class topics • java. lang. Cloneable • Marker interface that says (to humans and tools), “Objects of this interface provide a public clone() method. • See java. lang. Object. clone() • clone() returns a new copy of its object. • Its implementation often uses a private constructor to build the new object. • An immutable object can return a reference to itself. – It is like a C++ copy constructor. – Do not clone 2 D arrays. An array clone() clones only the first dimension.
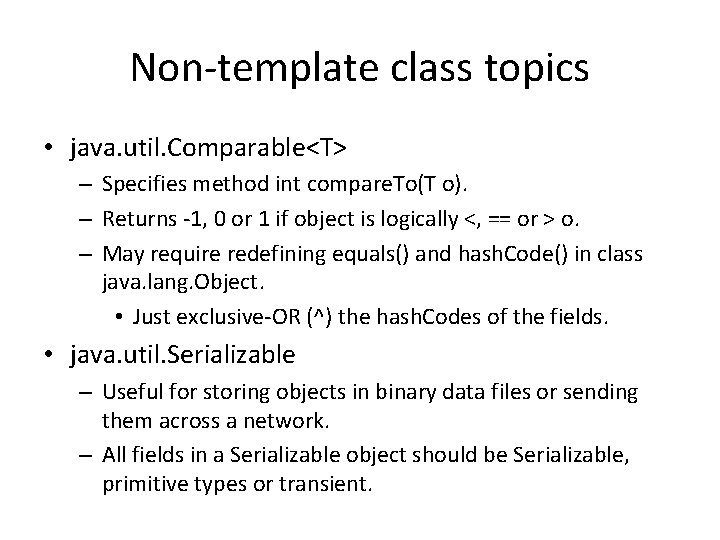
Non-template class topics • java. util. Comparable<T> – Specifies method int compare. To(T o). – Returns -1, 0 or 1 if object is logically <, == or > o. – May require redefining equals() and hash. Code() in class java. lang. Object. • Just exclusive-OR (^) the hash. Codes of the fields. • java. util. Serializable – Useful for storing objects in binary data files or sending them across a network. – All fields in a Serializable object should be Serializable, primitive types or transient.