CSC 215 Mathematical Functions math h Include mathematical
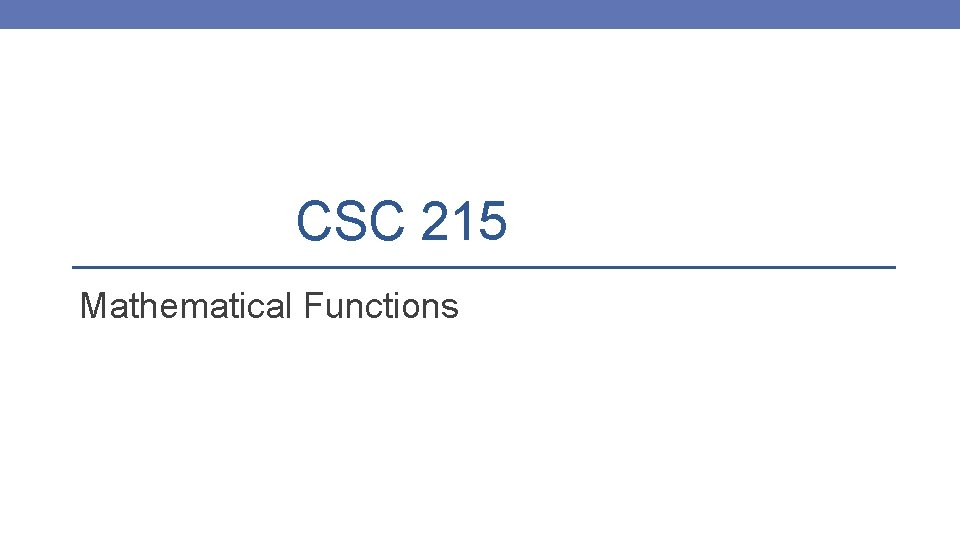
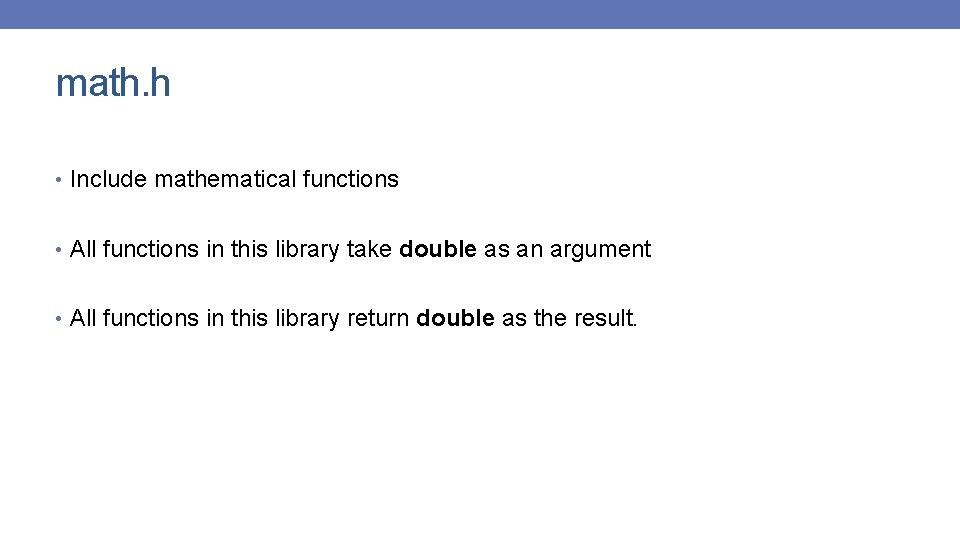
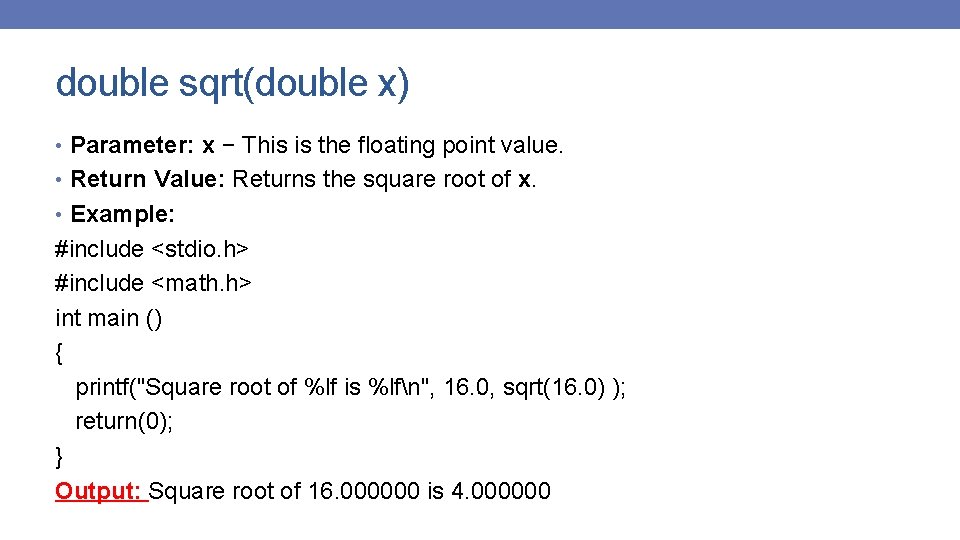
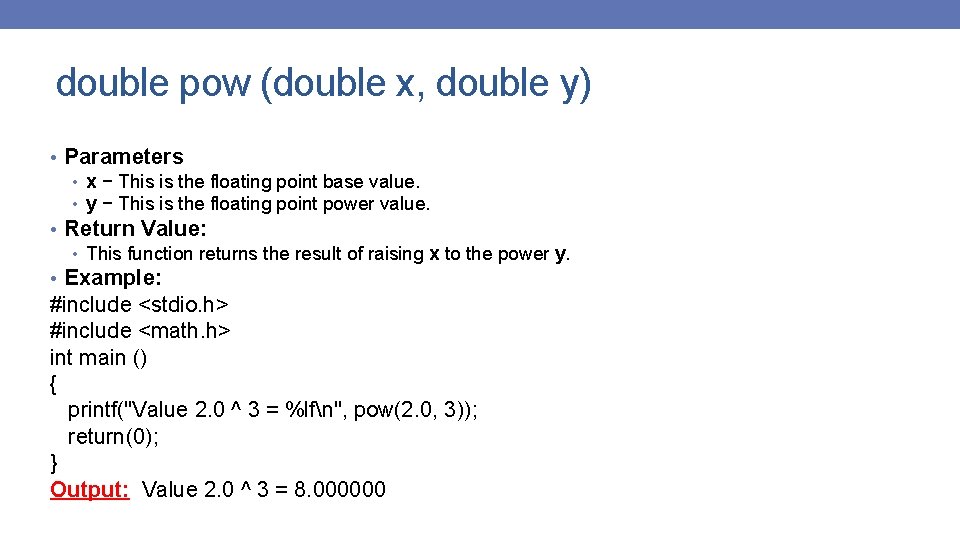
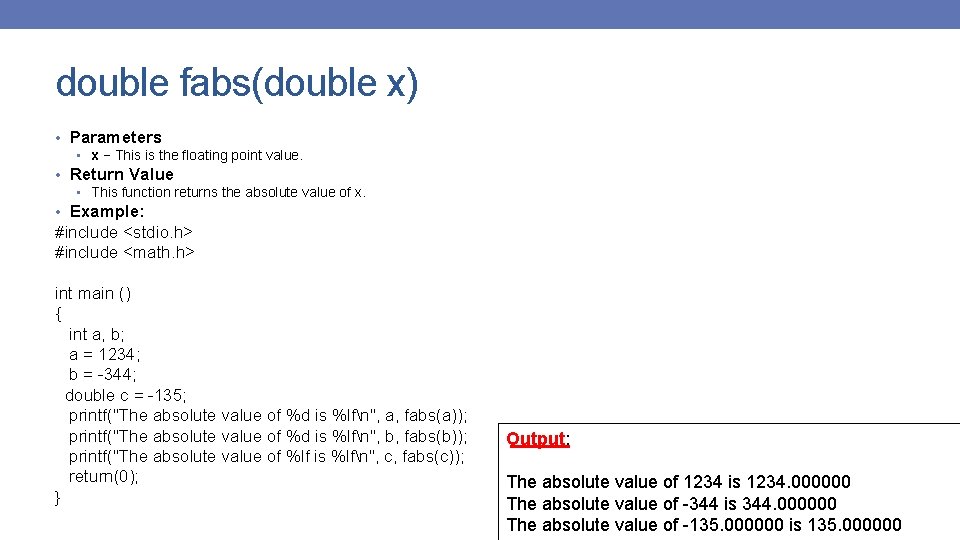
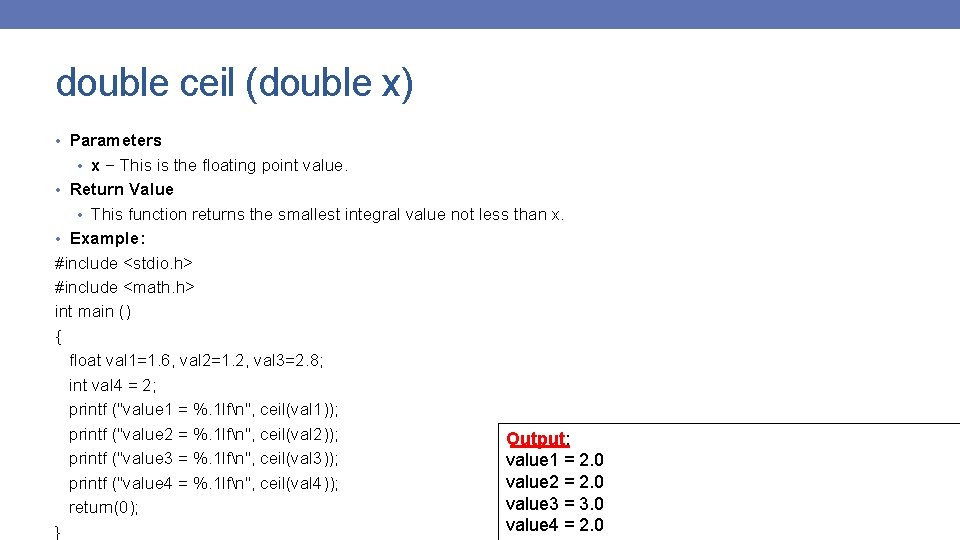
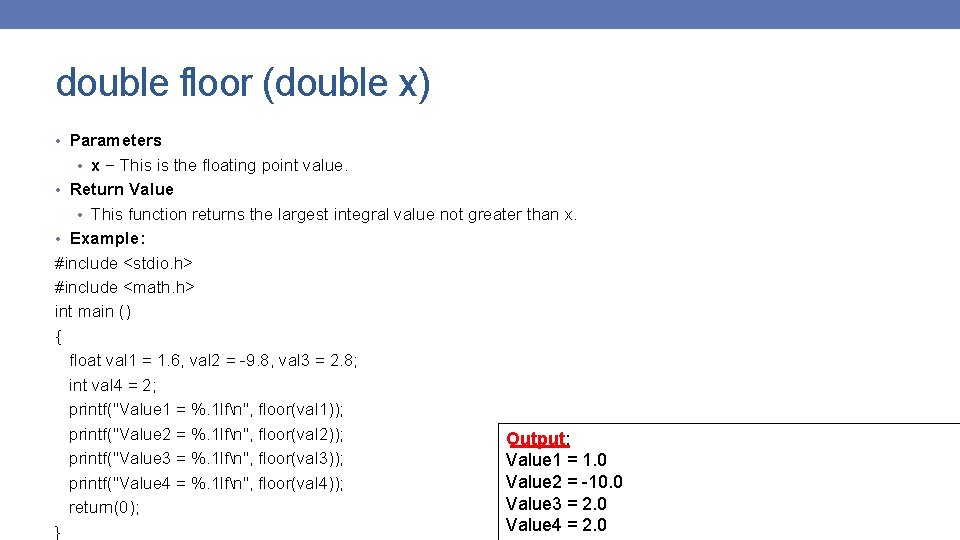
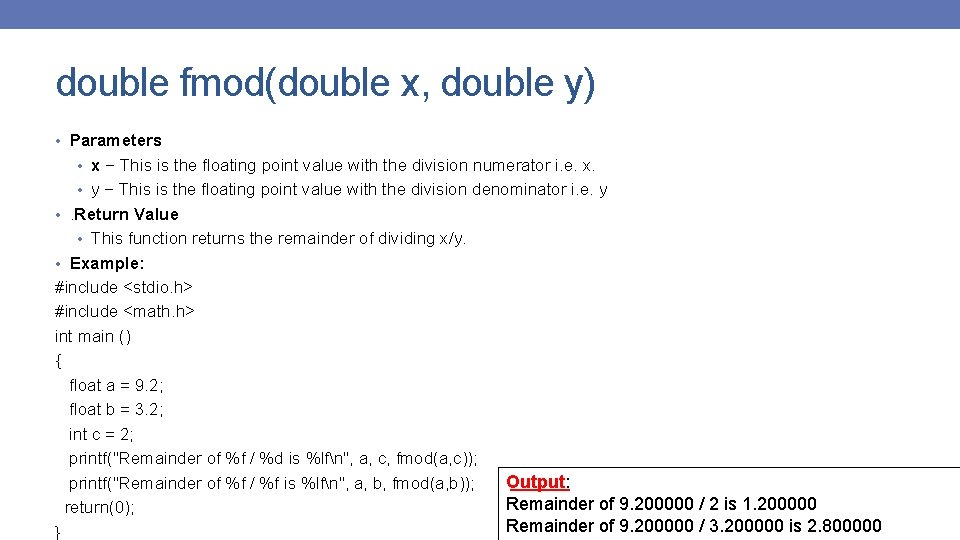
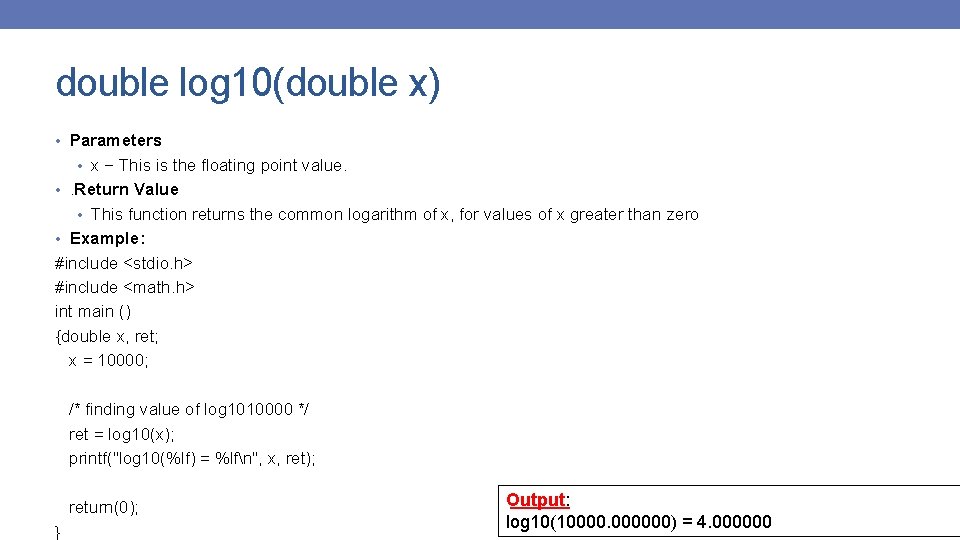
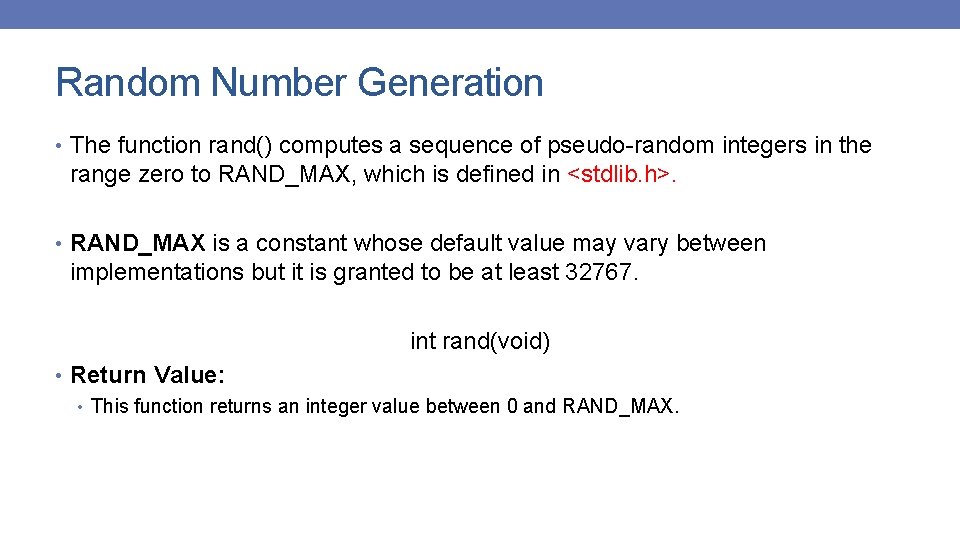
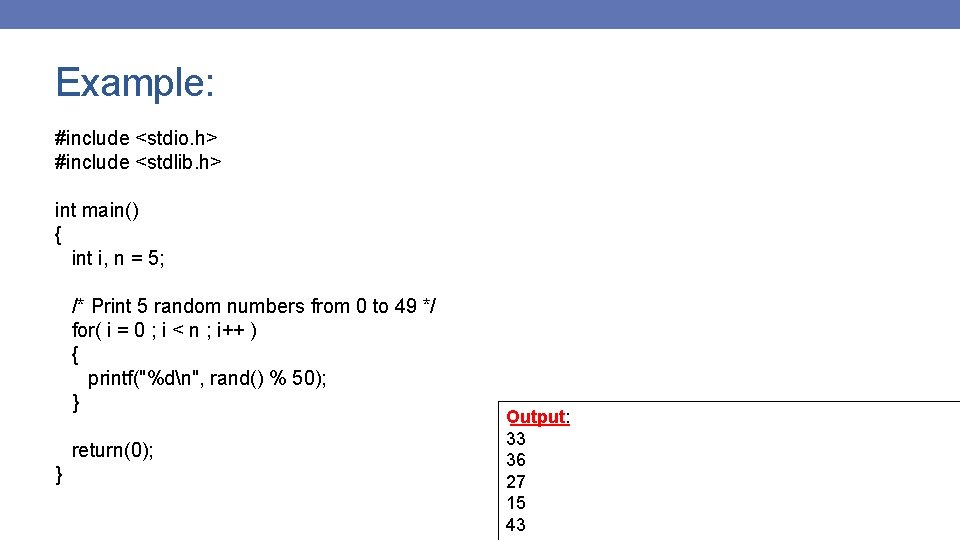
- Slides: 11
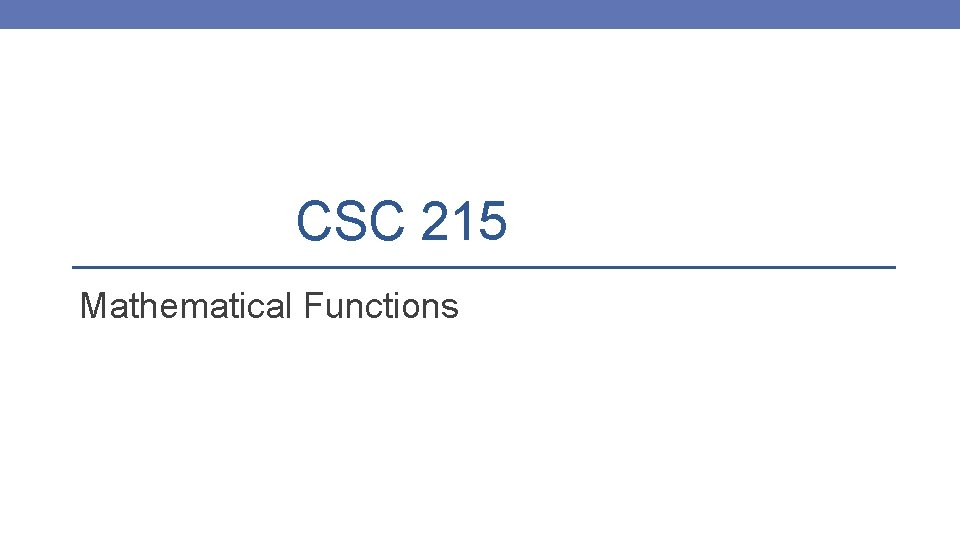
CSC 215 Mathematical Functions
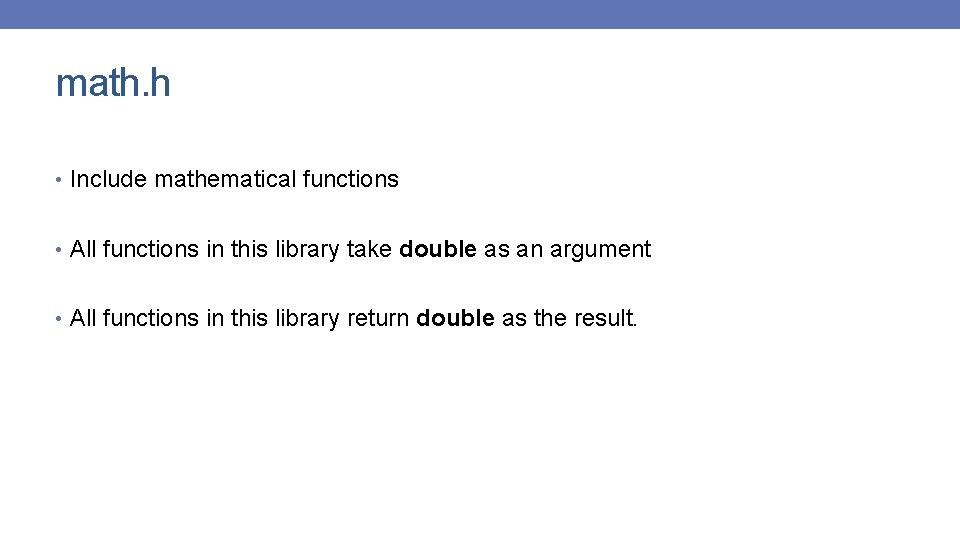
math. h • Include mathematical functions • All functions in this library take double as an argument • All functions in this library return double as the result.
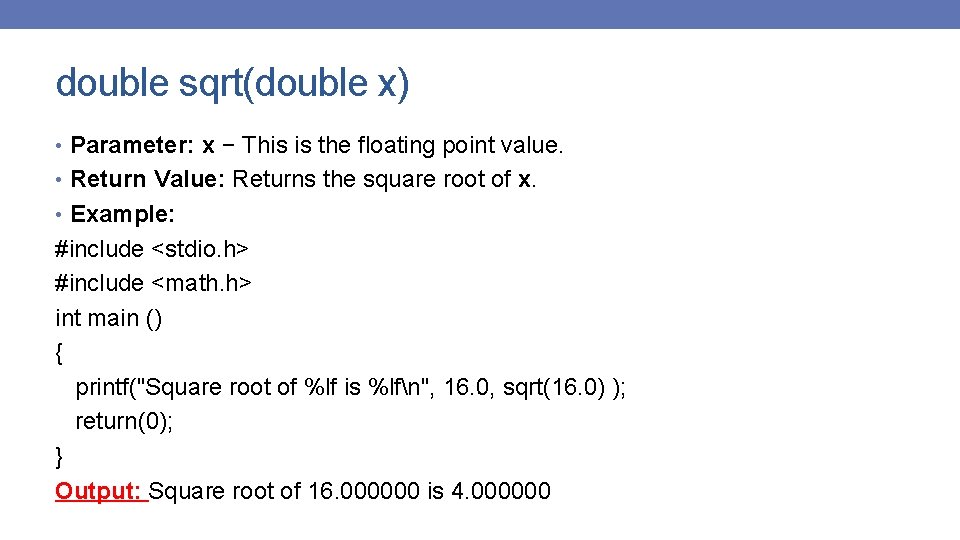
double sqrt(double x) • Parameter: x − This is the floating point value. • Return Value: Returns the square root of x. • Example: #include <stdio. h> #include <math. h> int main () { printf("Square root of %lf is %lfn", 16. 0, sqrt(16. 0) ); return(0); } Output: Square root of 16. 000000 is 4. 000000
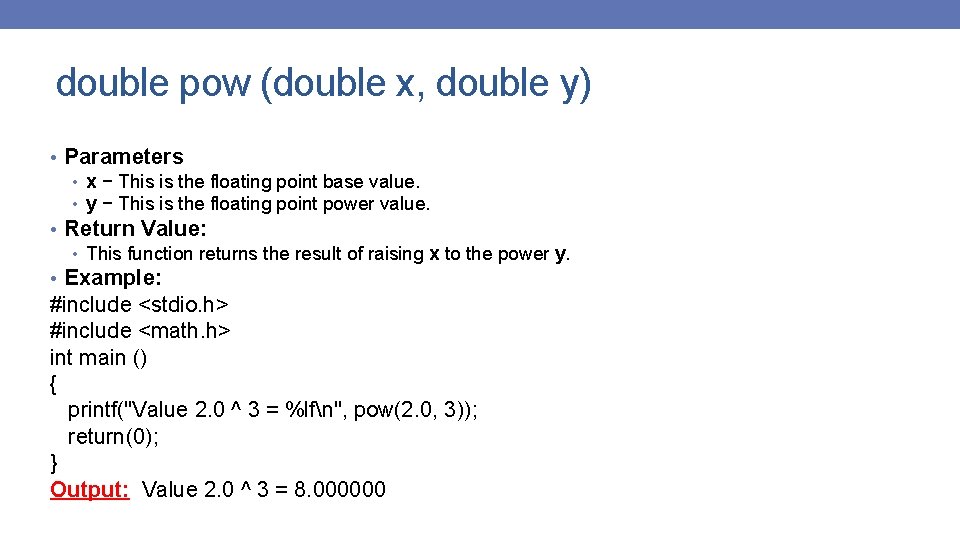
double pow (double x, double y) • Parameters • x − This is the floating point base value. • y − This is the floating point power value. • Return Value: • This function returns the result of raising x to the power y. • Example: #include <stdio. h> #include <math. h> int main () { printf("Value 2. 0 ^ 3 = %lfn", pow(2. 0, 3)); return(0); } Output: Value 2. 0 ^ 3 = 8. 000000
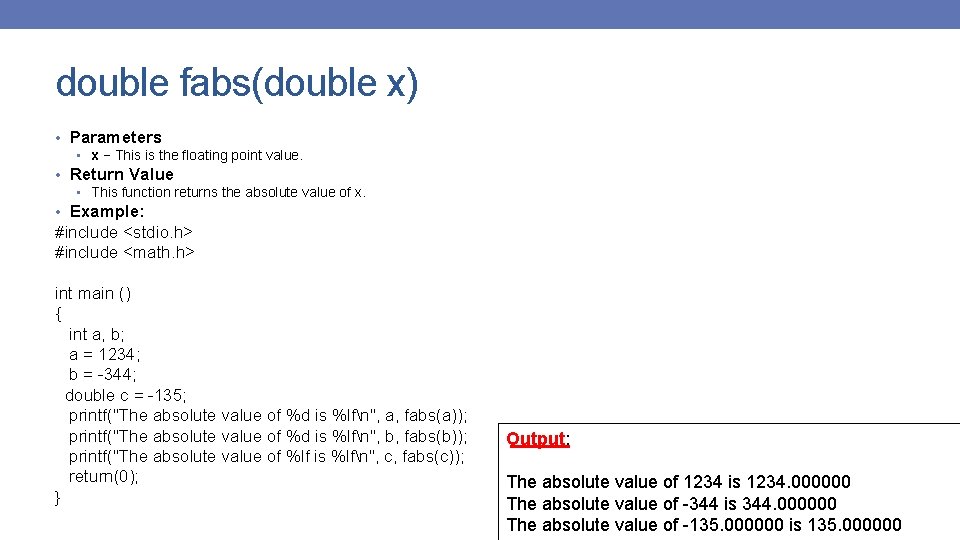
double fabs(double x) • Parameters • x − This is the floating point value. • Return Value • This function returns the absolute value of x. • Example: #include <stdio. h> #include <math. h> int main () { int a, b; a = 1234; b = -344; double c = -135; printf("The absolute value of %d is %lfn", a, fabs(a)); printf("The absolute value of %d is %lfn", b, fabs(b)); printf("The absolute value of %lf is %lfn", c, fabs(c)); return(0); } Output: The absolute value of 1234 is 1234. 000000 The absolute value of -344 is 344. 000000 The absolute value of -135. 000000 is 135. 000000
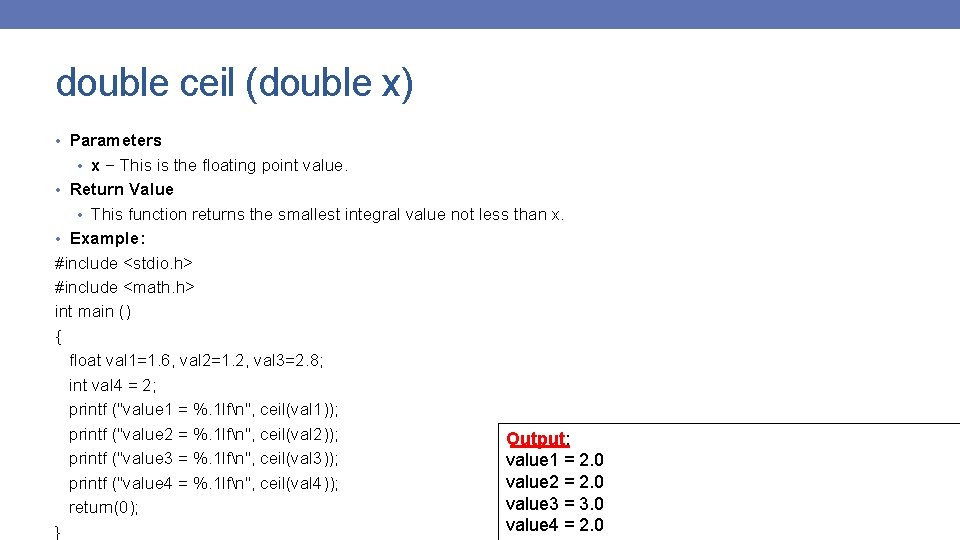
double ceil (double x) • Parameters • x − This is the floating point value. • Return Value • This function returns the smallest integral value not less than x. • Example: #include <stdio. h> #include <math. h> int main () { float val 1=1. 6, val 2=1. 2, val 3=2. 8; int val 4 = 2; printf ("value 1 = %. 1 lfn", ceil(val 1)); printf ("value 2 = %. 1 lfn", ceil(val 2)); printf ("value 3 = %. 1 lfn", ceil(val 3)); printf ("value 4 = %. 1 lfn", ceil(val 4)); return(0); } Output: value 1 = 2. 0 value 2 = 2. 0 value 3 = 3. 0 value 4 = 2. 0
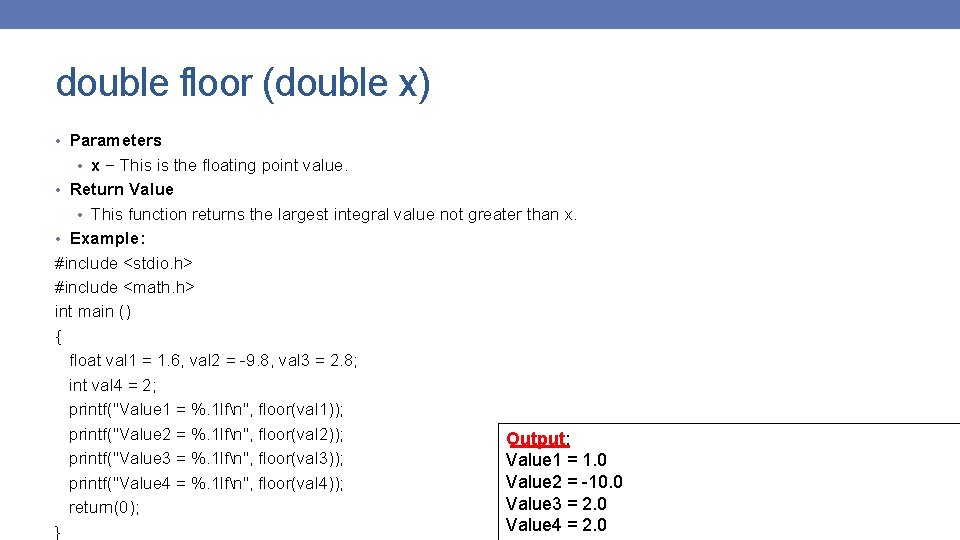
double floor (double x) • Parameters • x − This is the floating point value. • Return Value • This function returns the largest integral value not greater than x. • Example: #include <stdio. h> #include <math. h> int main () { float val 1 = 1. 6, val 2 = -9. 8, val 3 = 2. 8; int val 4 = 2; printf("Value 1 = %. 1 lfn", floor(val 1)); printf("Value 2 = %. 1 lfn", floor(val 2)); printf("Value 3 = %. 1 lfn", floor(val 3)); printf("Value 4 = %. 1 lfn", floor(val 4)); return(0); } Output: Value 1 = 1. 0 Value 2 = -10. 0 Value 3 = 2. 0 Value 4 = 2. 0
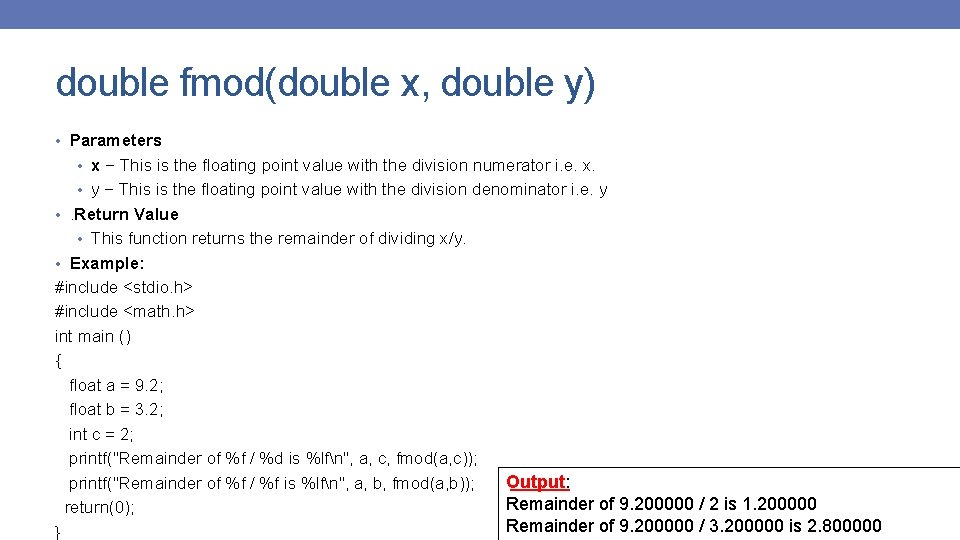
double fmod(double x, double y) • Parameters • x − This is the floating point value with the division numerator i. e. x. • y − This is the floating point value with the division denominator i. e. y • . Return Value • This function returns the remainder of dividing x/y. • Example: #include <stdio. h> #include <math. h> int main () { float a = 9. 2; float b = 3. 2; int c = 2; printf("Remainder of %f / %d is %lfn", a, c, fmod(a, c)); printf("Remainder of %f / %f is %lfn", a, b, fmod(a, b)); return(0); } Output: Remainder of 9. 200000 / 2 is 1. 200000 Remainder of 9. 200000 / 3. 200000 is 2. 800000
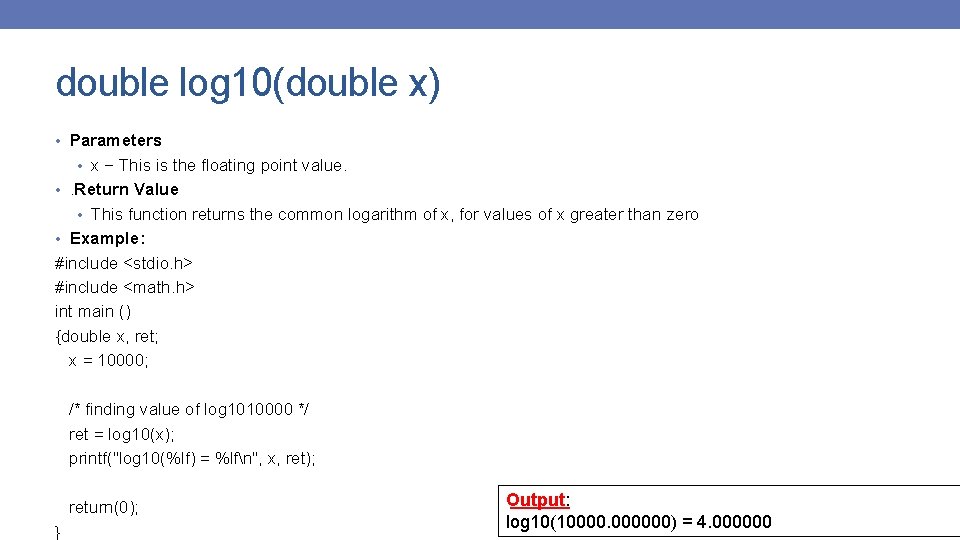
double log 10(double x) • Parameters • x − This is the floating point value. • . Return Value • This function returns the common logarithm of x, for values of x greater than zero • Example: #include <stdio. h> #include <math. h> int main () {double x, ret; x = 10000; /* finding value of log 1010000 */ ret = log 10(x); printf("log 10(%lf) = %lfn", x, ret); return(0); } Output: log 10(1000000) = 4. 000000
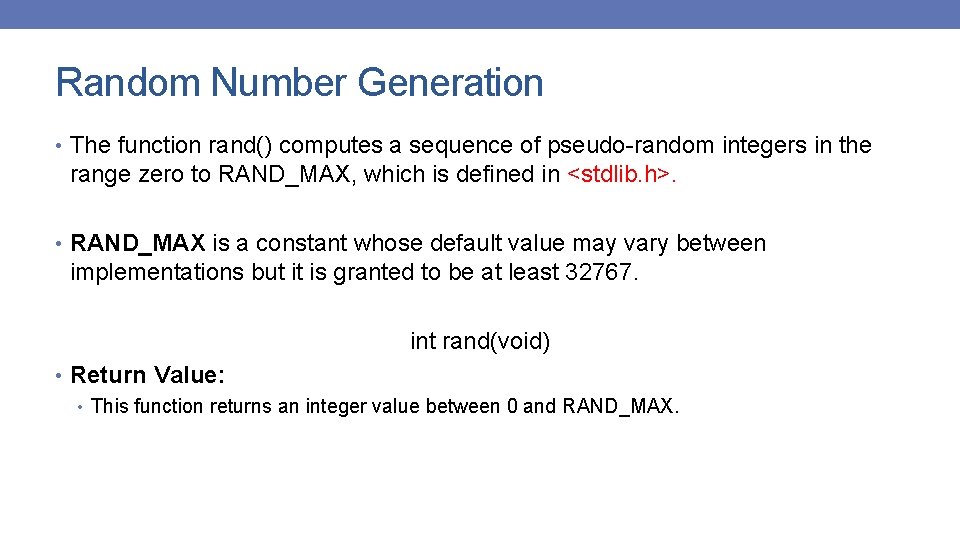
Random Number Generation • The function rand() computes a sequence of pseudo-random integers in the range zero to RAND_MAX, which is defined in <stdlib. h>. • RAND_MAX is a constant whose default value may vary between implementations but it is granted to be at least 32767. int rand(void) • Return Value: • This function returns an integer value between 0 and RAND_MAX.
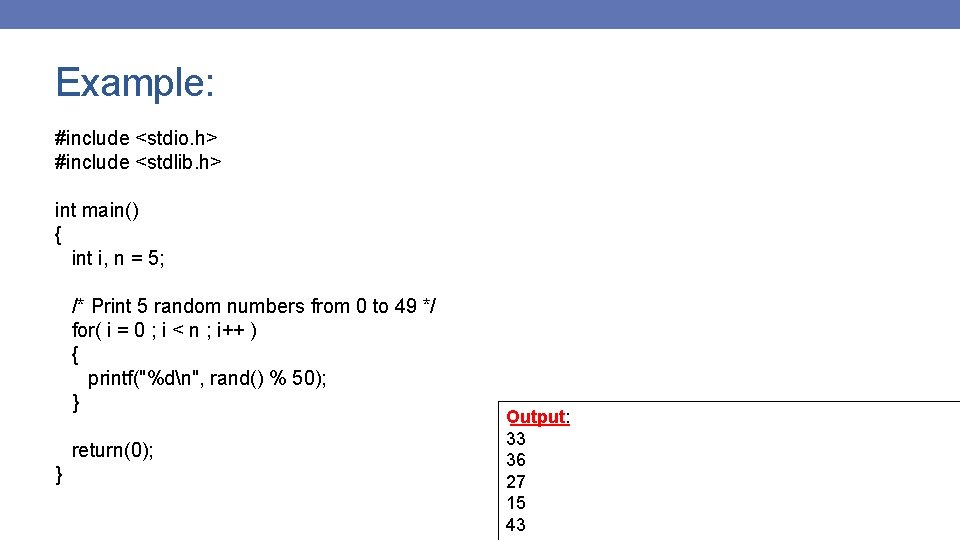
Example: #include <stdio. h> #include <stdlib. h> int main() { int i, n = 5; /* Print 5 random numbers from 0 to 49 */ for( i = 0 ; i < n ; i++ ) { printf("%dn", rand() % 50); } return(0); } Output: 33 36 27 15 43