Classes Class Definitions A class definition starts with
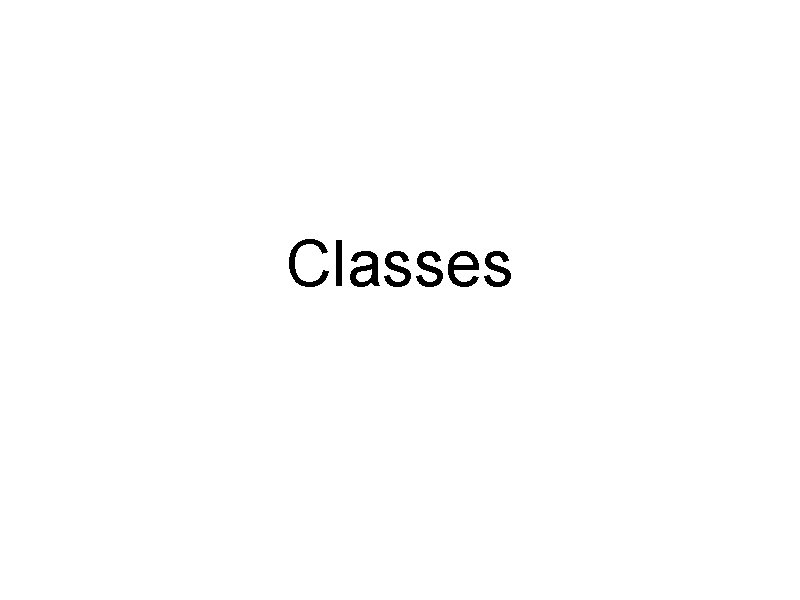
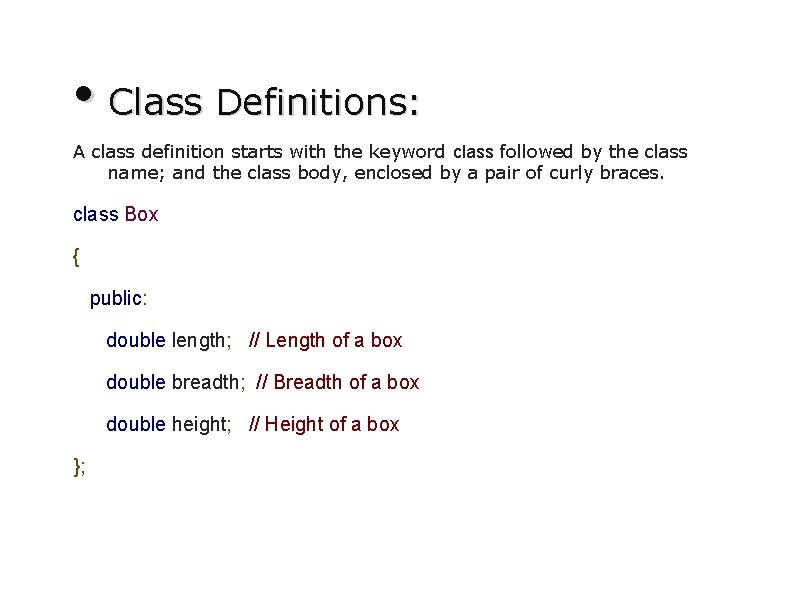
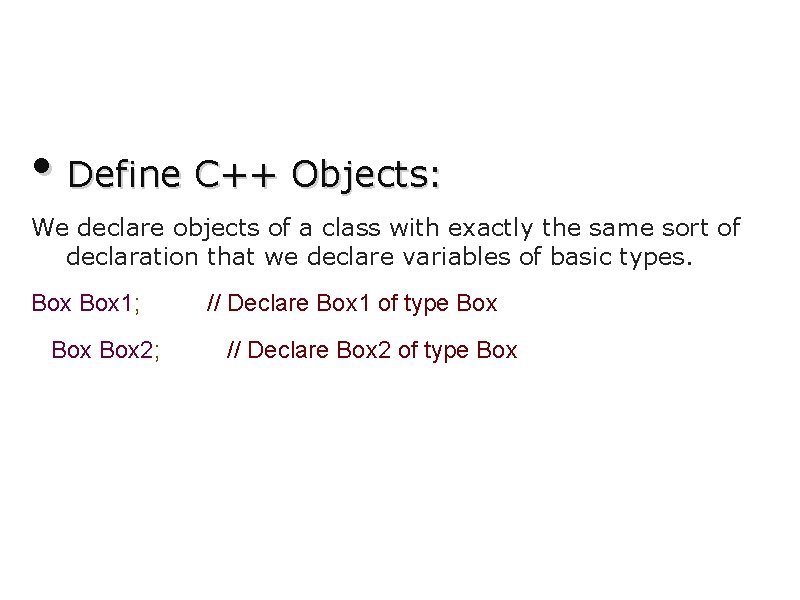
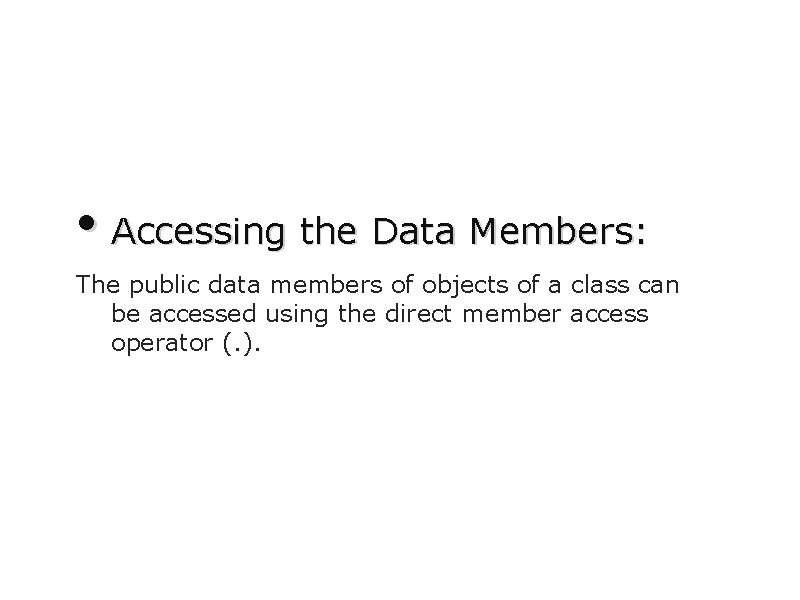
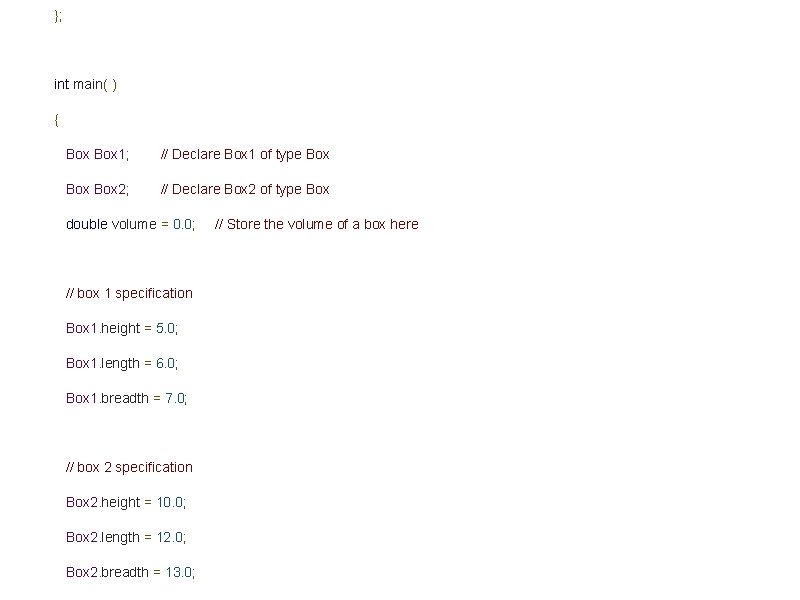
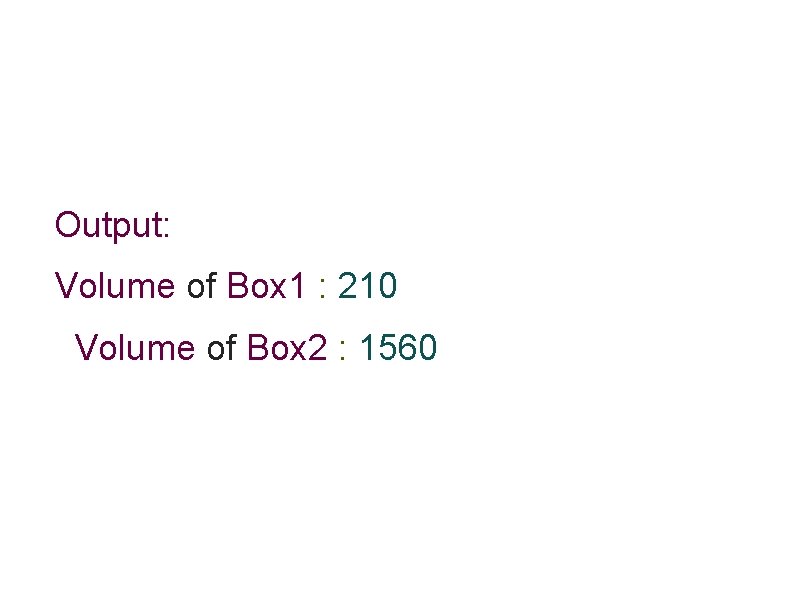
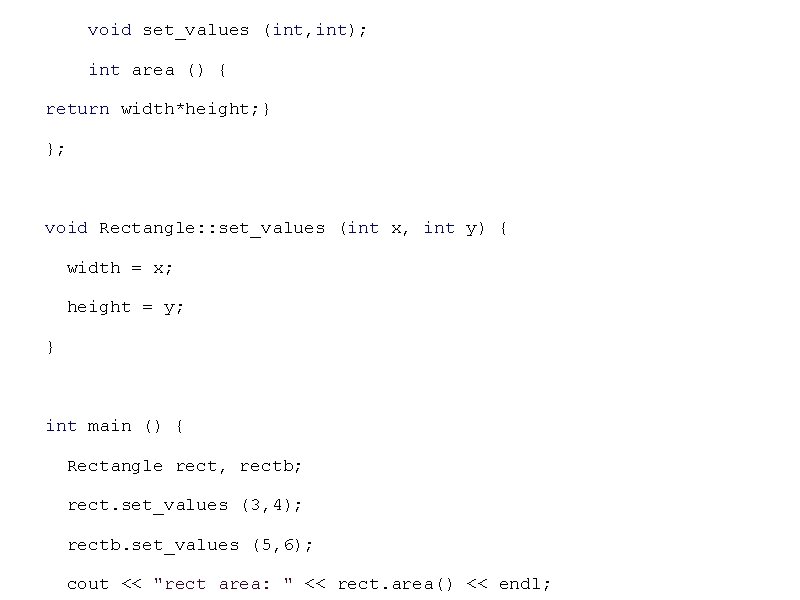
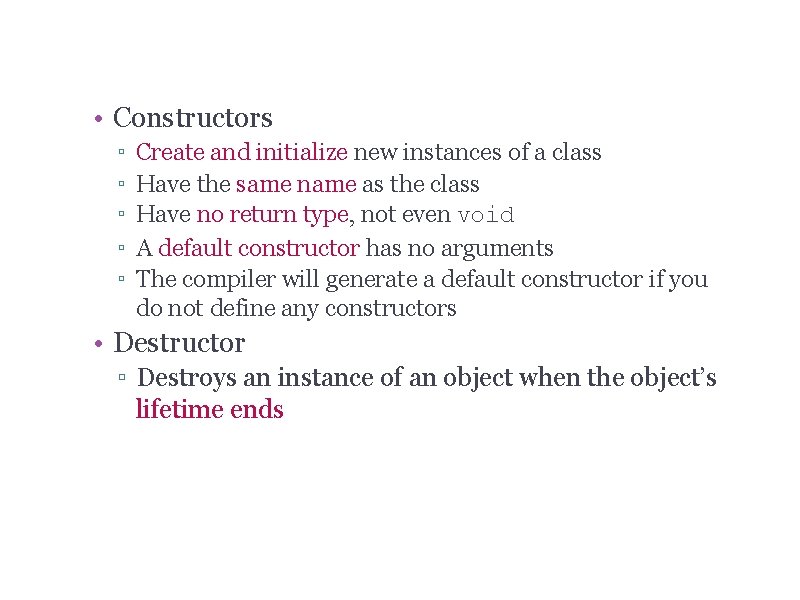
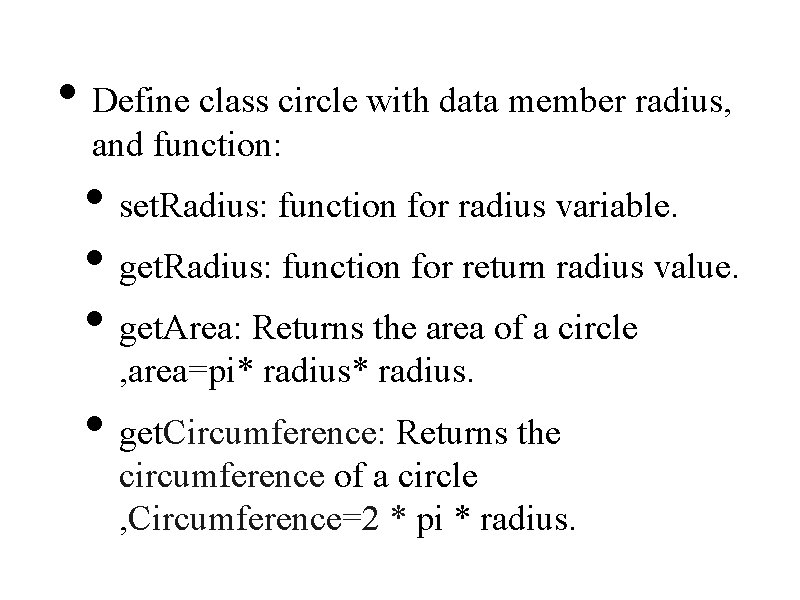
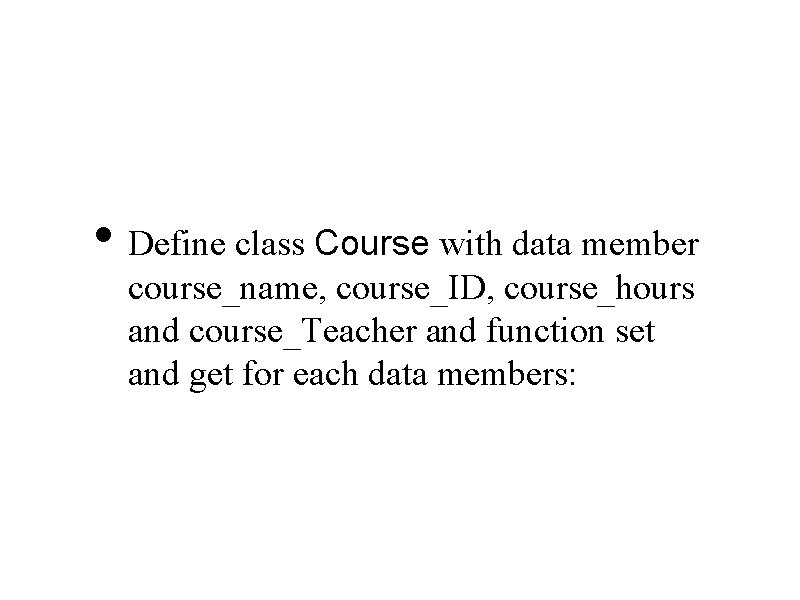
- Slides: 10
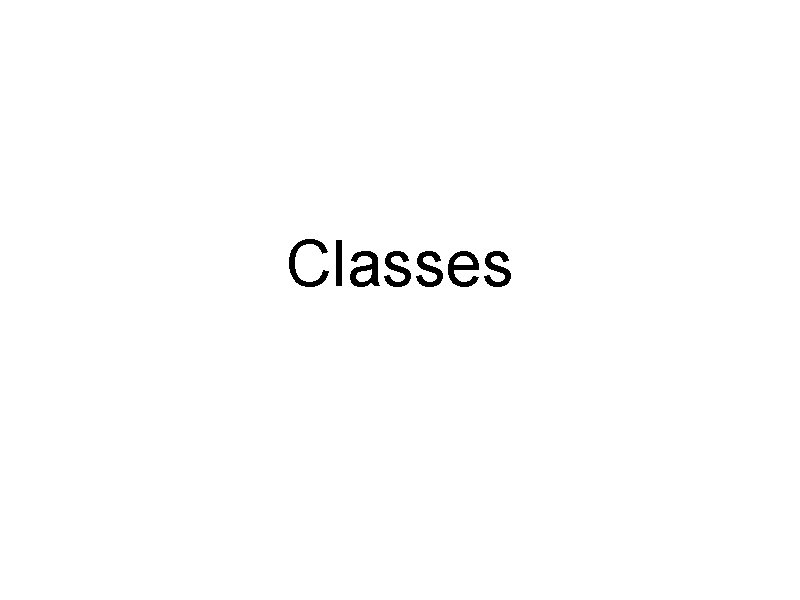
Classes
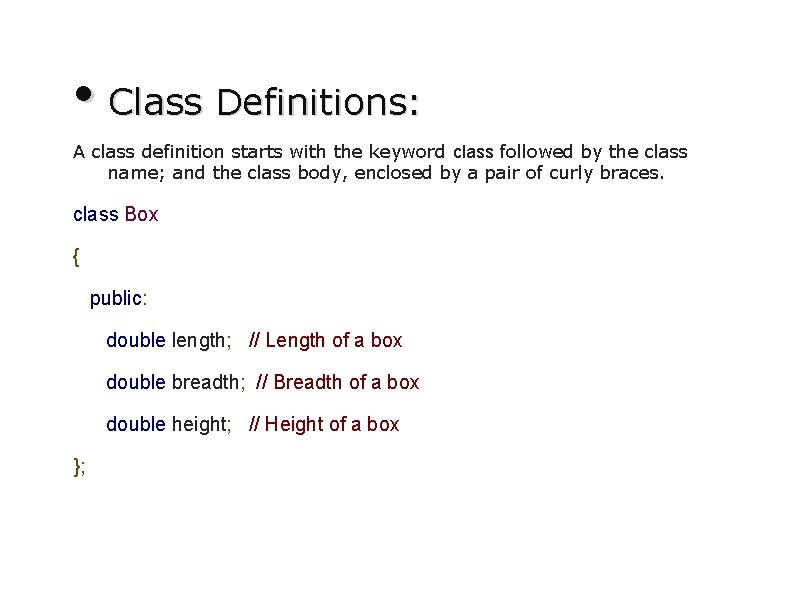
• Class Definitions: A class definition starts with the keyword class followed by the class name; and the class body, enclosed by a pair of curly braces. class Box { public: double length; // Length of a box double breadth; // Breadth of a box double height; // Height of a box };
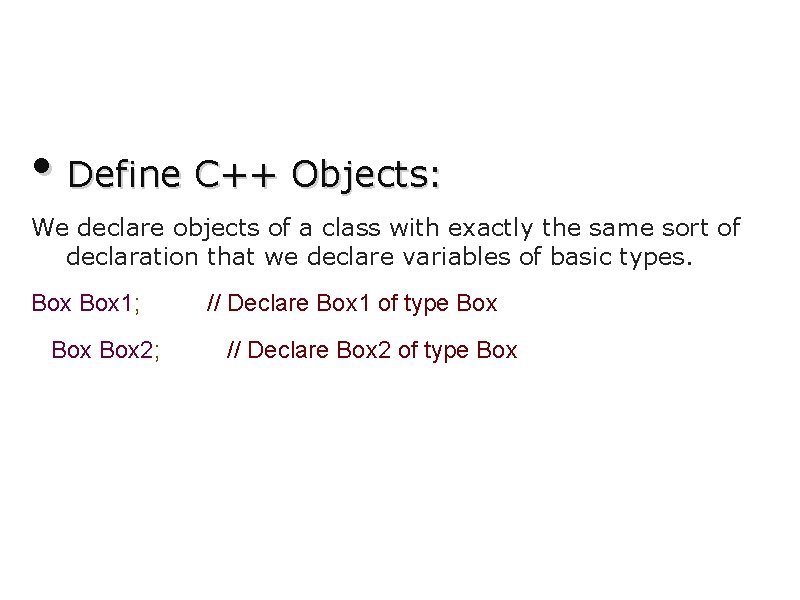
• Define C++ Objects: We declare objects of a class with exactly the same sort of declaration that we declare variables of basic types. Box 1; Box 2; // Declare Box 1 of type Box // Declare Box 2 of type Box
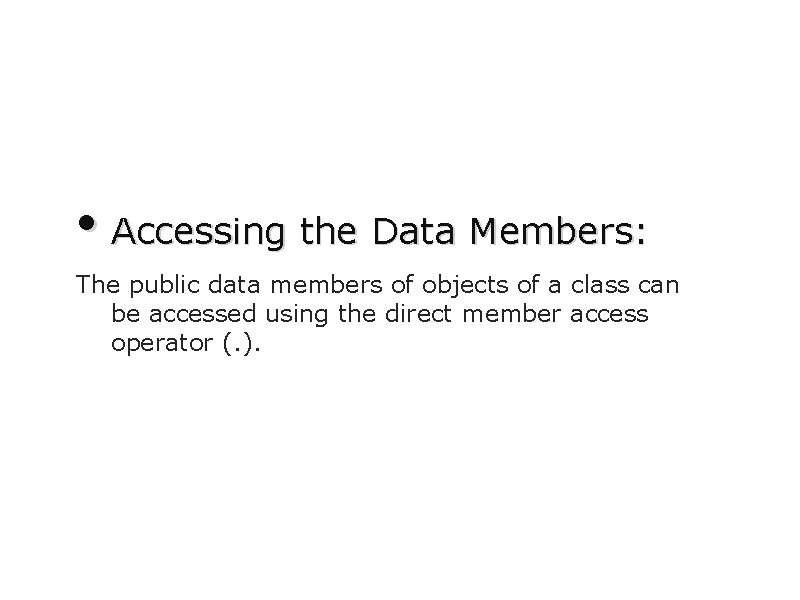
• Accessing the Data Members: The public data members of objects of a class can be accessed using the direct member access operator (. ).
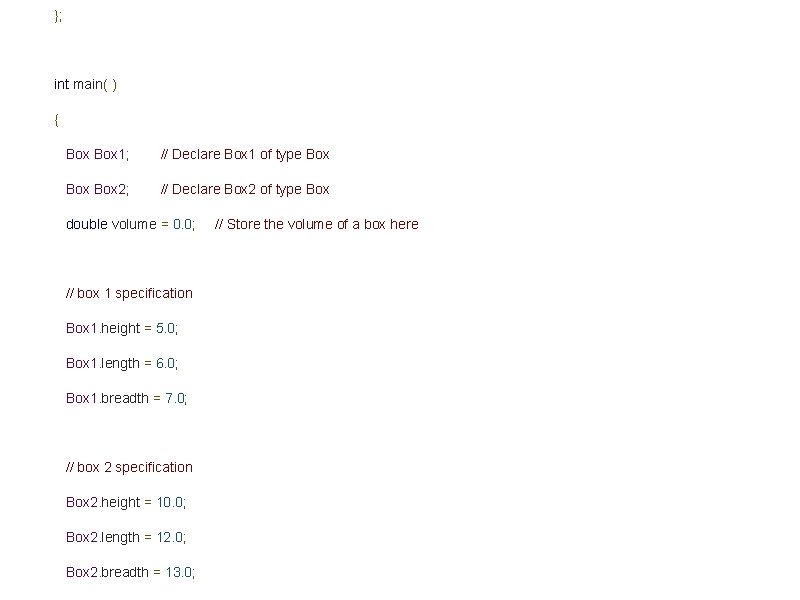
}; int main( ) { Box 1; // Declare Box 1 of type Box Box 2; // Declare Box 2 of type Box double volume = 0. 0; // box 1 specification Box 1. height = 5. 0; Box 1. length = 6. 0; Box 1. breadth = 7. 0; // box 2 specification Box 2. height = 10. 0; Box 2. length = 12. 0; Box 2. breadth = 13. 0; // Store the volume of a box here
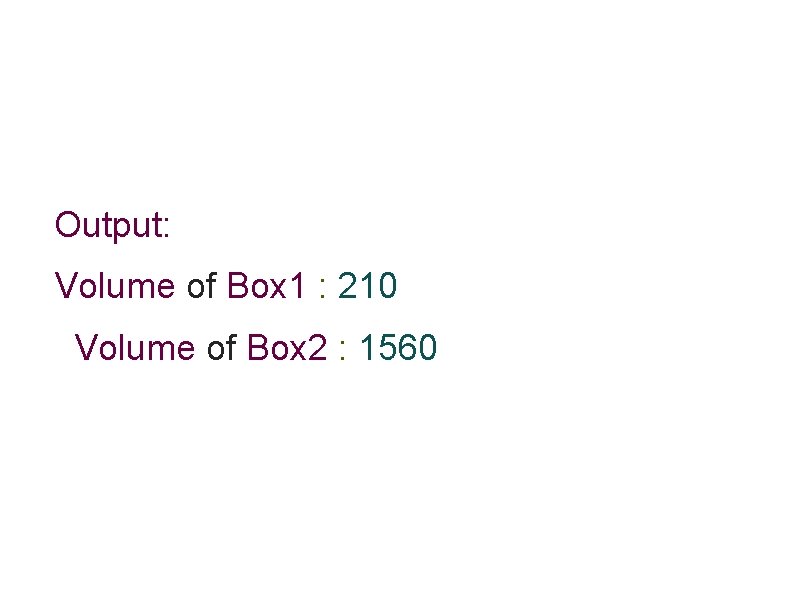
Output: Volume of Box 1 : 210 Volume of Box 2 : 1560
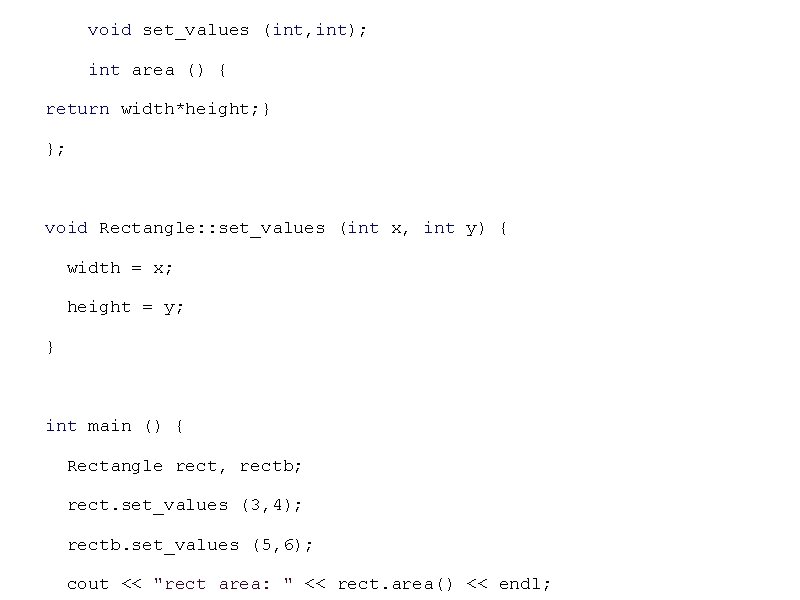
void set_values (int, int); int area () { return width*height; } }; void Rectangle: : set_values (int x, int y) { width = x; height = y; } int main () { Rectangle rect, rectb; rect. set_values (3, 4); rectb. set_values (5, 6); cout << "rect area: " << rect. area() << endl;
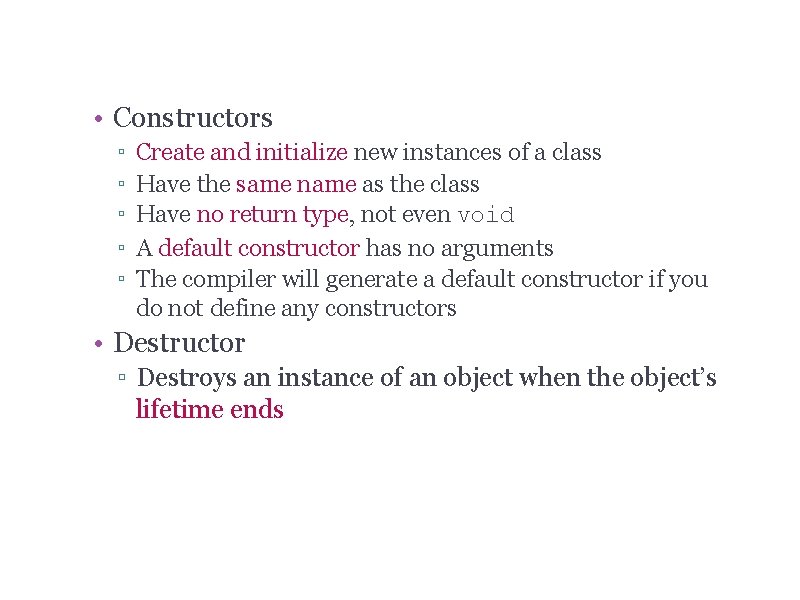
• Constructors ▫ ▫ ▫ Create and initialize new instances of a class Have the same name as the class Have no return type, not even void A default constructor has no arguments The compiler will generate a default constructor if you do not define any constructors • Destructor ▫ Destroys an instance of an object when the object’s lifetime ends
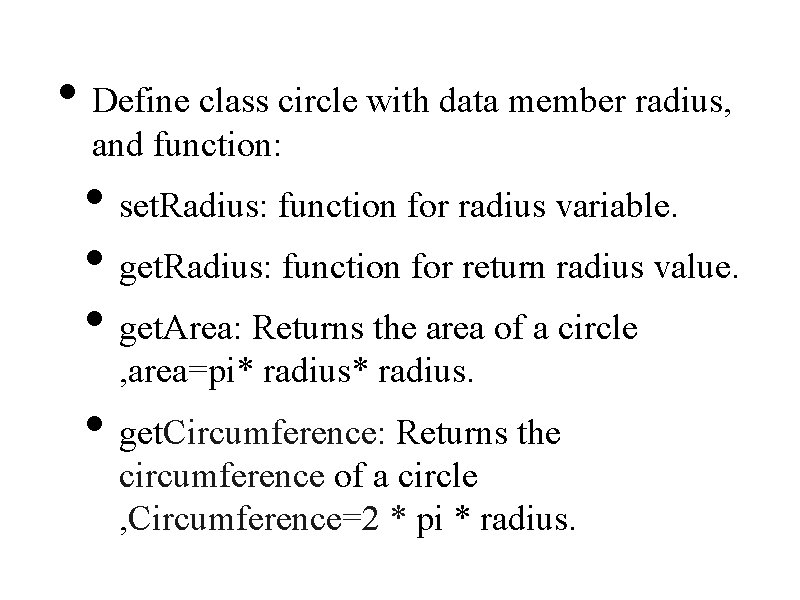
• Define class circle with data member radius, and function: • set. Radius: function for radius variable. • get. Radius: function for return radius value. • get. Area: Returns the area of a circle , area=pi* radius. • get. Circumference: Returns the circumference of a circle , Circumference=2 * pi * radius.
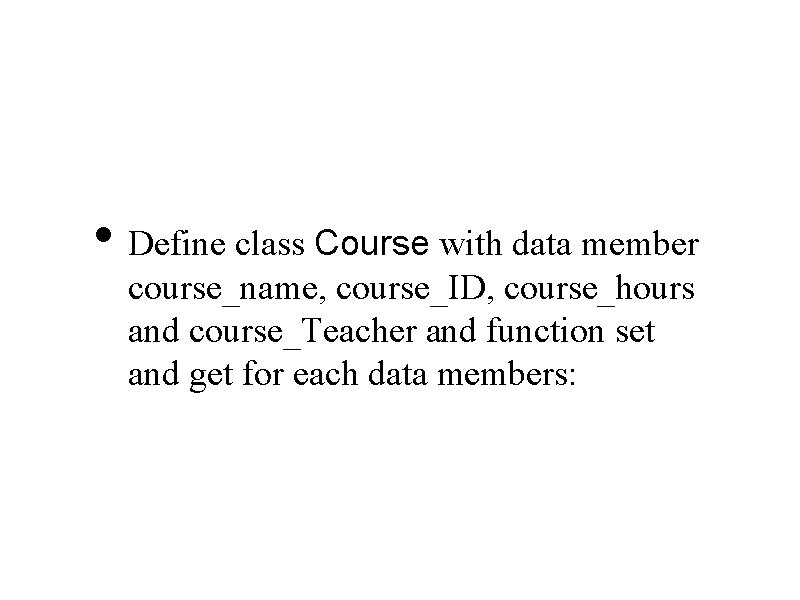
• Define class Course with data member course_name, course_ID, course_hours and course_Teacher and function set and get for each data members:
Classe e subclasse de aquele
Pre ap classes vs regular classes
Before class starts
Class starts today
Tollgate review example
What part of the sewing machine where feet are stationed
Letter starts with o
Luther starts the reformation
Life of a plant poem by risa jordan
The global trade starts here
Kelvin scale starts from