Chapter 11 Detecting Termination and Deadlocks Motivation Diffusing
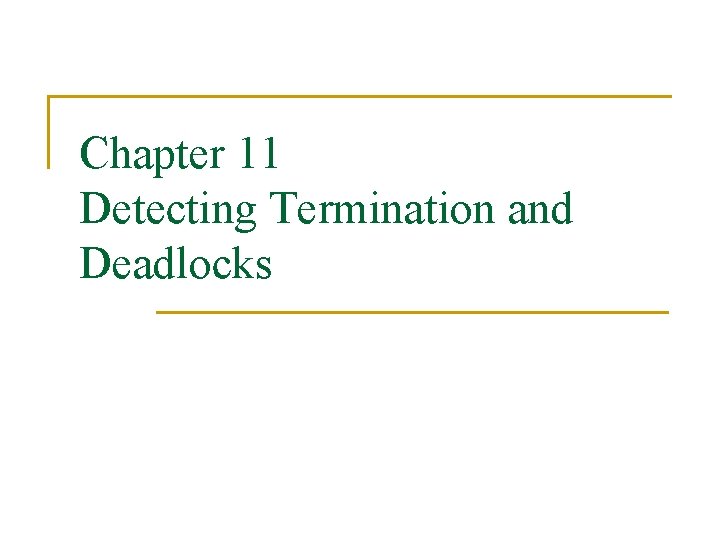
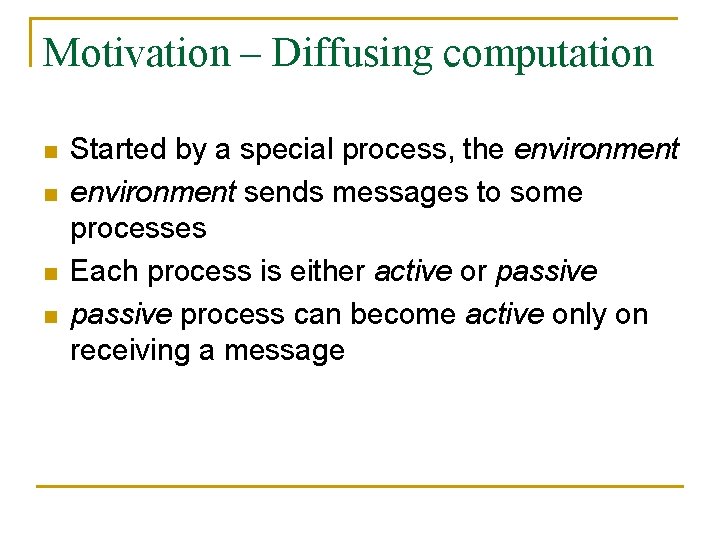
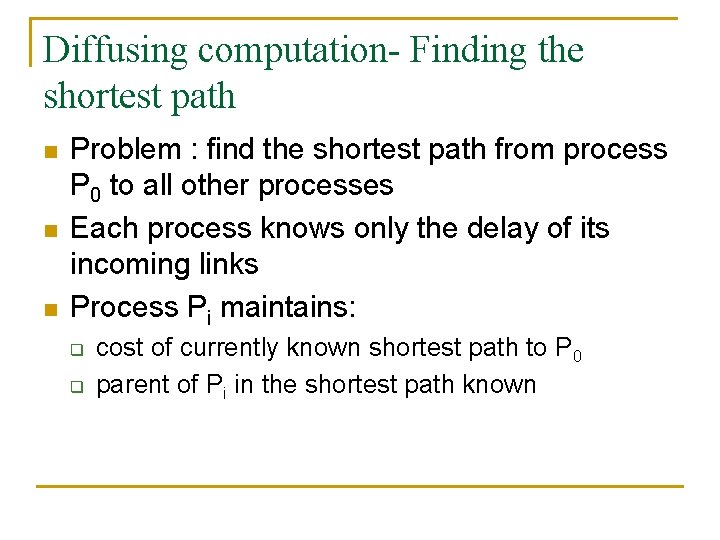
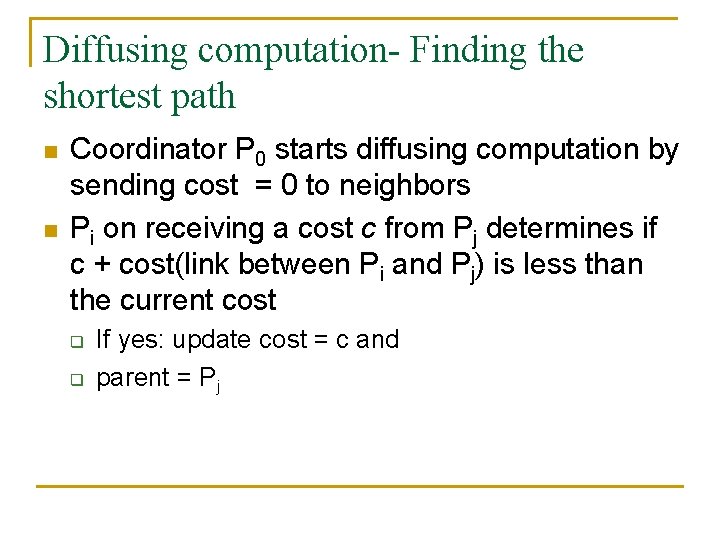
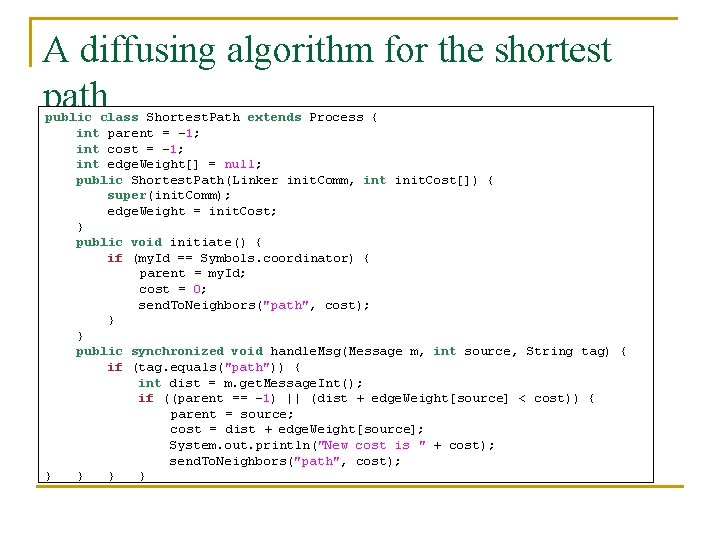
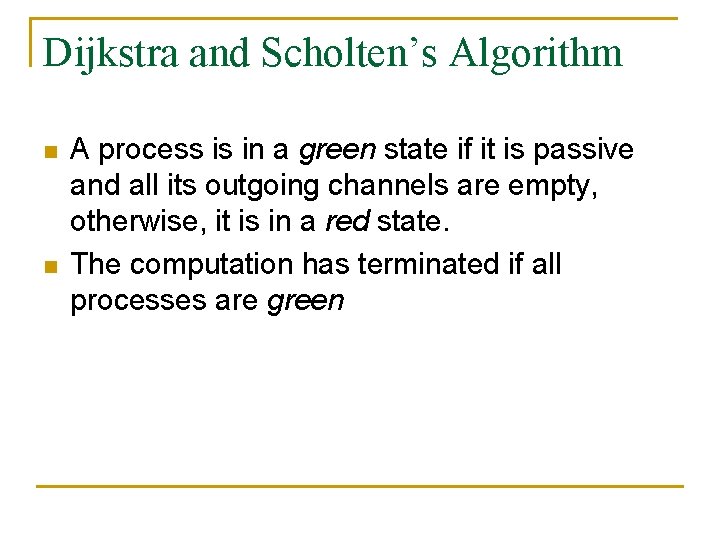
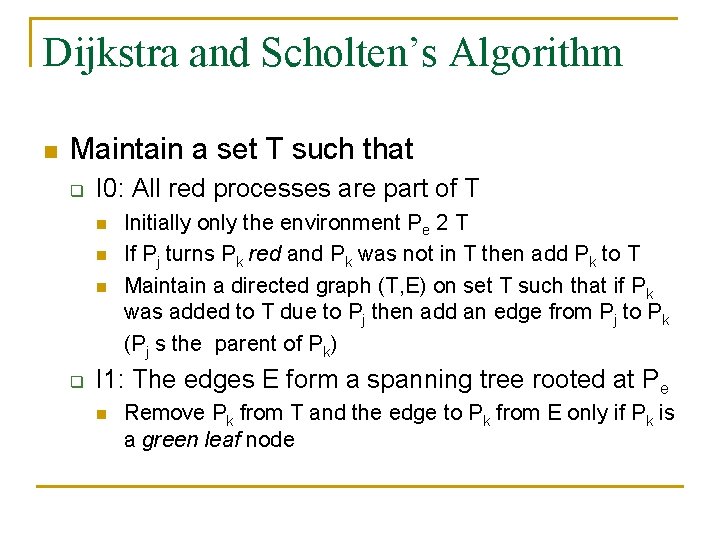
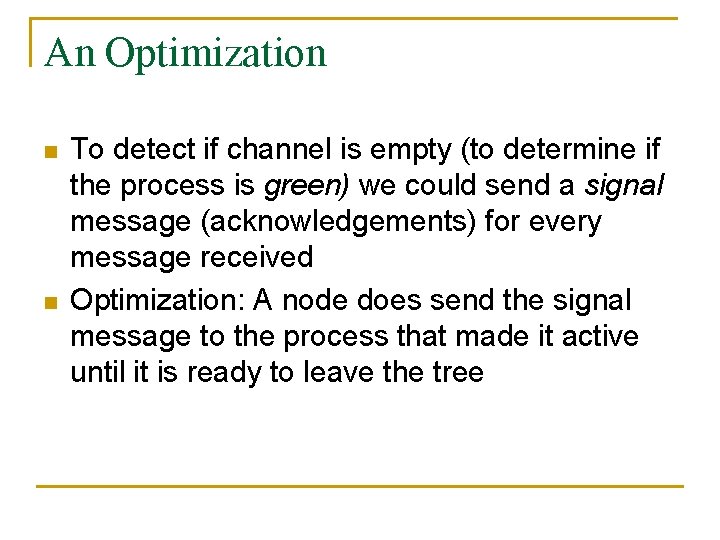
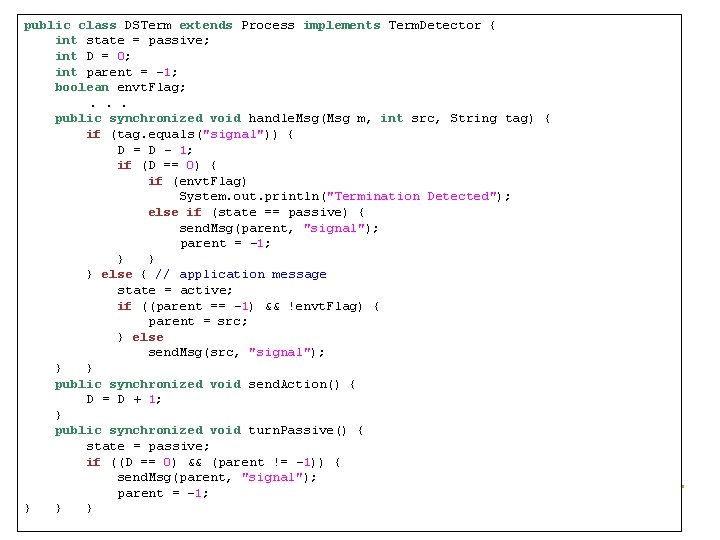
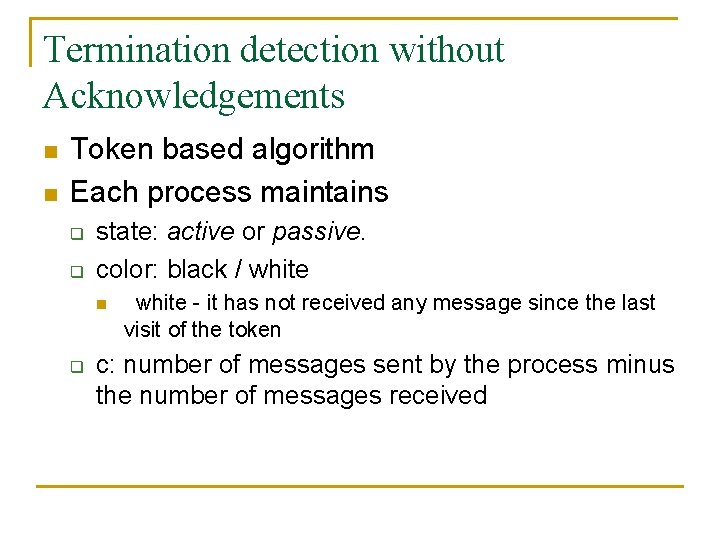
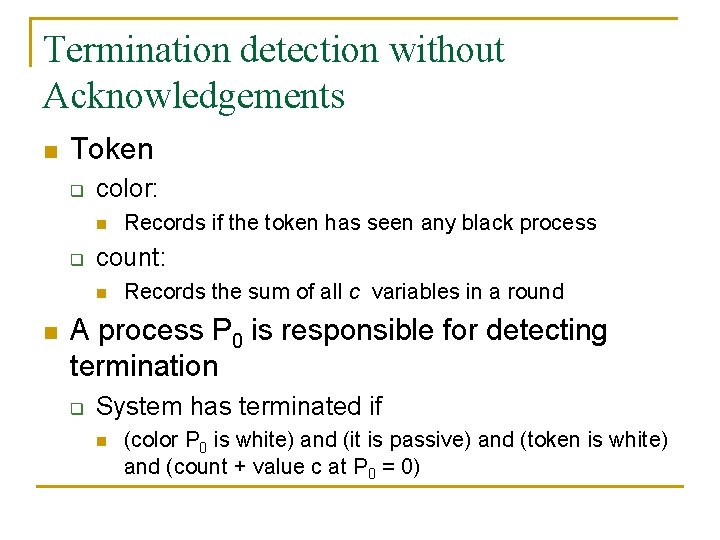
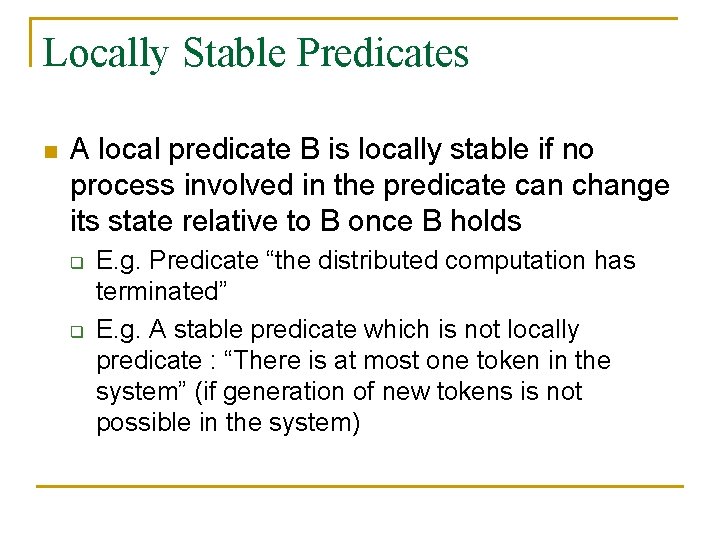
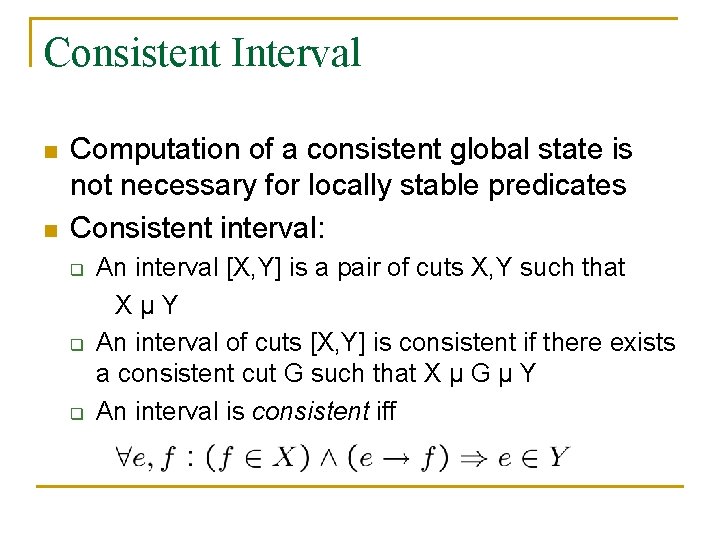
![Algorithm outline n Repeatedly compute consistent intervals [X, Y] q q If a predicate Algorithm outline n Repeatedly compute consistent intervals [X, Y] q q If a predicate](https://slidetodoc.com/presentation_image/38d3cbb60fd033a26dfee5b8c691f76e/image-14.jpg)
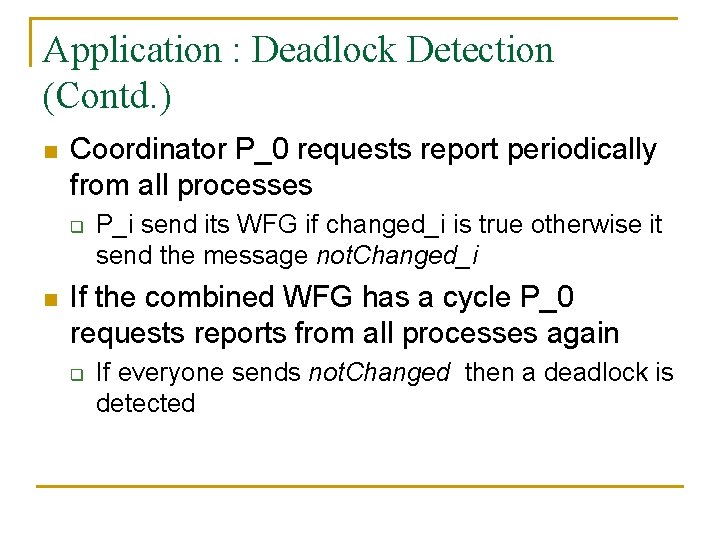
- Slides: 15
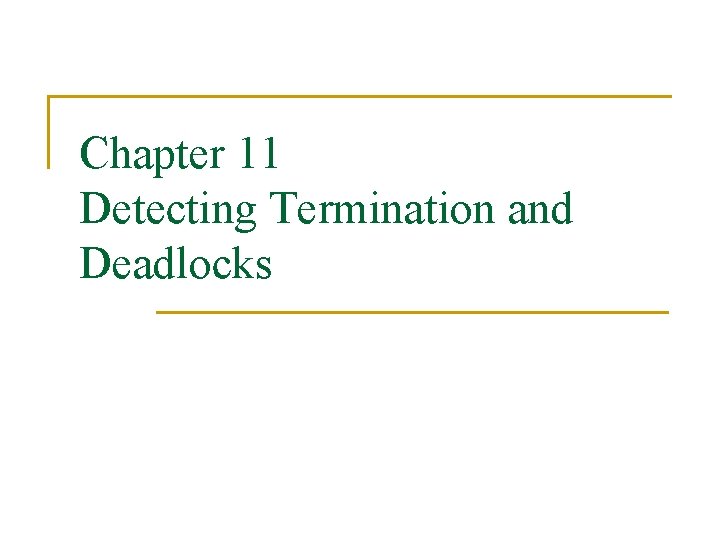
Chapter 11 Detecting Termination and Deadlocks
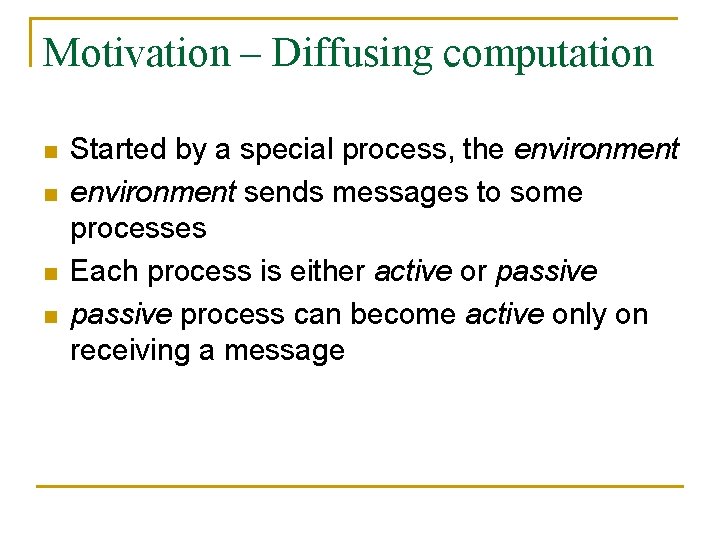
Motivation – Diffusing computation n n Started by a special process, the environment sends messages to some processes Each process is either active or passive process can become active only on receiving a message
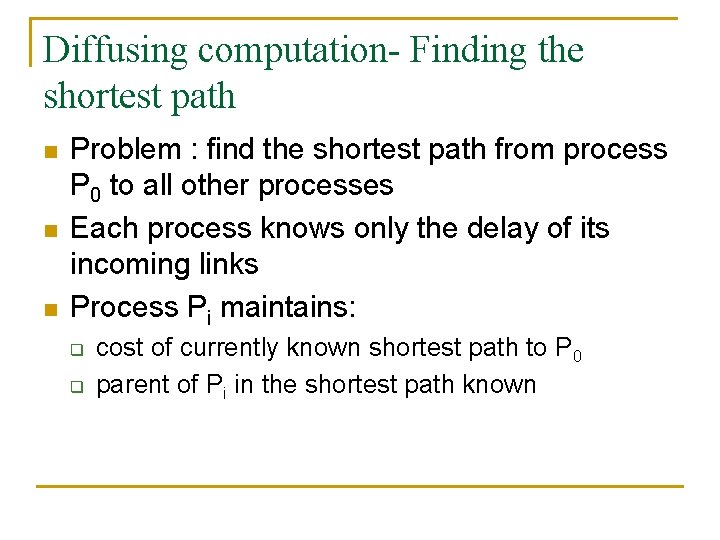
Diffusing computation- Finding the shortest path n n n Problem : find the shortest path from process P 0 to all other processes Each process knows only the delay of its incoming links Process Pi maintains: q q cost of currently known shortest path to P 0 parent of Pi in the shortest path known
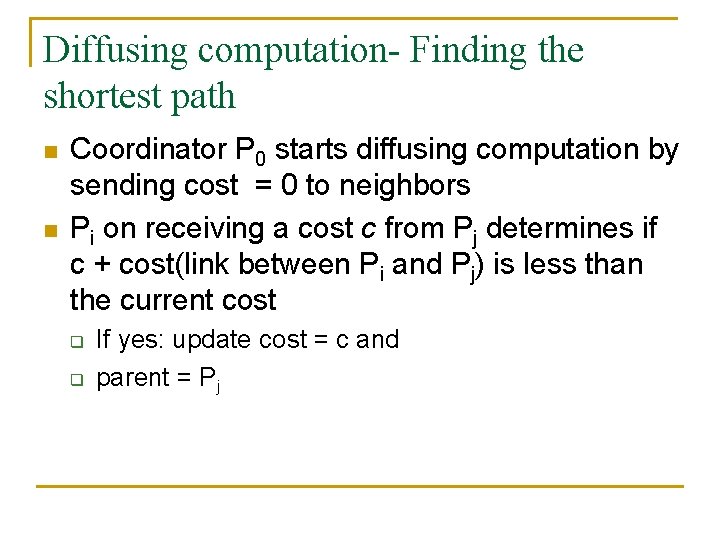
Diffusing computation- Finding the shortest path n n Coordinator P 0 starts diffusing computation by sending cost = 0 to neighbors Pi on receiving a cost c from Pj determines if c + cost(link between Pi and Pj) is less than the current cost q q If yes: update cost = c and parent = Pj
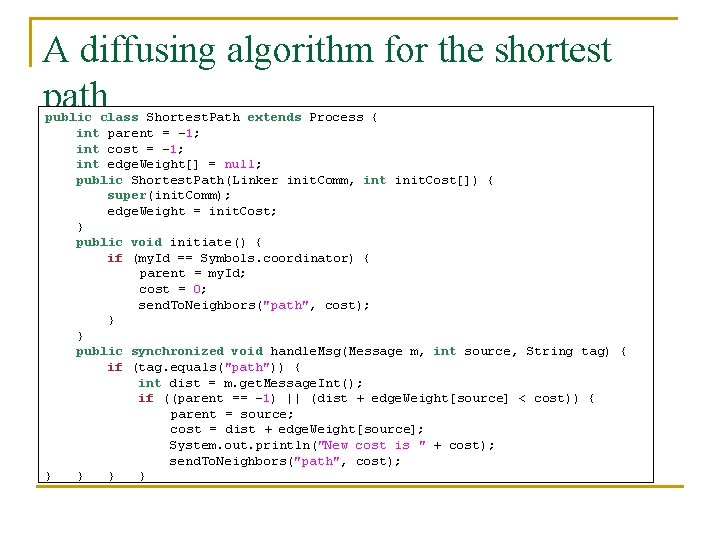
A diffusing algorithm for the shortest path public class Shortest. Path extends Process { int parent = -1; int cost = -1; int edge. Weight[] = null; public Shortest. Path(Linker init. Comm, int init. Cost[]) { super(init. Comm); edge. Weight = init. Cost; } public void initiate() { if (my. Id == Symbols. coordinator) { parent = my. Id; cost = 0; send. To. Neighbors("path", cost); } } public synchronized void handle. Msg(Message m, int source, String tag) { if (tag. equals("path")) { int dist = m. get. Message. Int(); if ((parent == -1) || (dist + edge. Weight[source] < cost)) { parent = source; cost = dist + edge. Weight[source]; System. out. println("New cost is " + cost); send. To. Neighbors("path", cost); } }
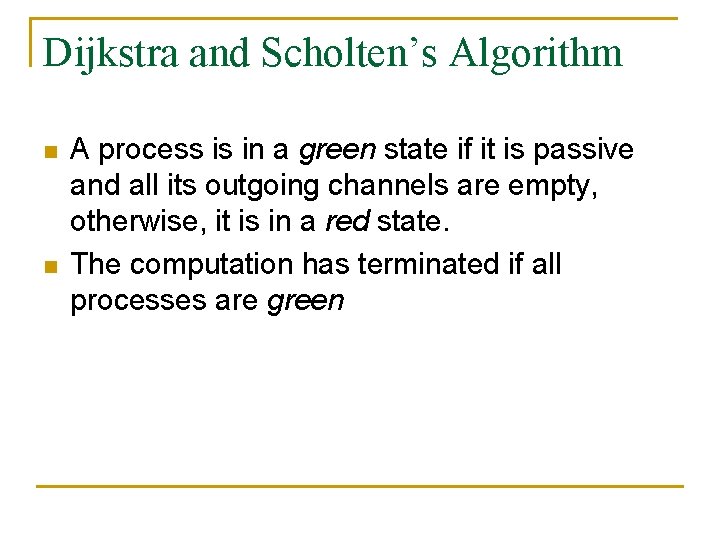
Dijkstra and Scholten’s Algorithm n n A process is in a green state if it is passive and all its outgoing channels are empty, otherwise, it is in a red state. The computation has terminated if all processes are green
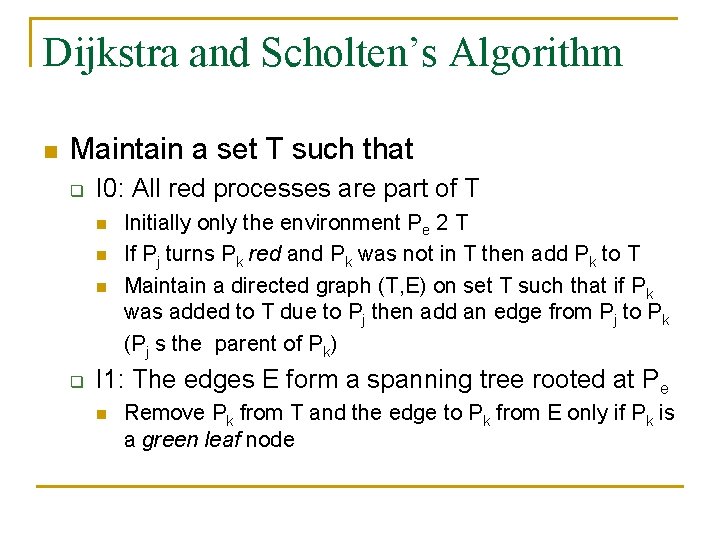
Dijkstra and Scholten’s Algorithm n Maintain a set T such that q I 0: All red processes are part of T n n n q Initially only the environment Pe 2 T If Pj turns Pk red and Pk was not in T then add Pk to T Maintain a directed graph (T, E) on set T such that if Pk was added to T due to Pj then add an edge from Pj to Pk (Pj s the parent of Pk) I 1: The edges E form a spanning tree rooted at Pe n Remove Pk from T and the edge to Pk from E only if Pk is a green leaf node
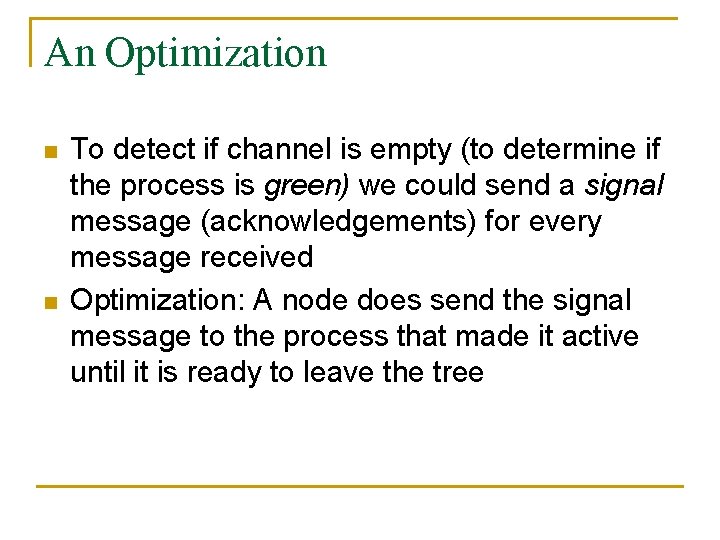
An Optimization n n To detect if channel is empty (to determine if the process is green) we could send a signal message (acknowledgements) for every message received Optimization: A node does send the signal message to the process that made it active until it is ready to leave the tree
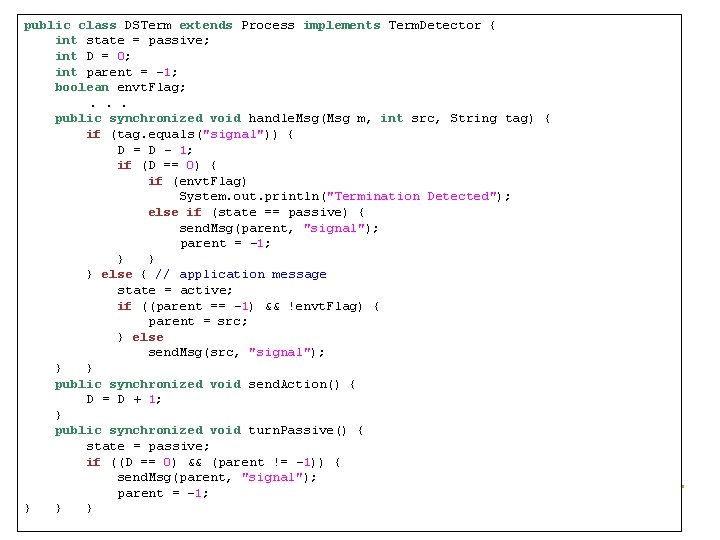
public class DSTerm extends Process implements Term. Detector { int state = passive; int D = 0; int parent = -1; boolean envt. Flag; . . . public synchronized void handle. Msg(Msg m, int src, String tag) { if (tag. equals("signal")) { D = D - 1; if (D == 0) { if (envt. Flag) System. out. println("Termination Detected"); else if (state == passive) { send. Msg(parent, "signal"); parent = -1; } } } else { // application message state = active; if ((parent == -1) && !envt. Flag) { parent = src; } else send. Msg(src, "signal"); } } public synchronized void send. Action() { D = D + 1; } public synchronized void turn. Passive() { state = passive; if ((D == 0) && (parent != -1)) { send. Msg(parent, "signal"); parent = -1; } } }
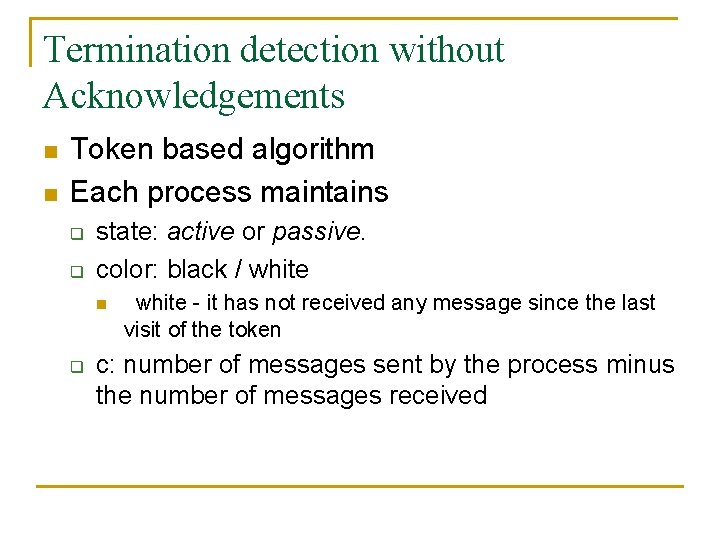
Termination detection without Acknowledgements n n Token based algorithm Each process maintains q q state: active or passive. color: black / white n q white - it has not received any message since the last visit of the token c: number of messages sent by the process minus the number of messages received
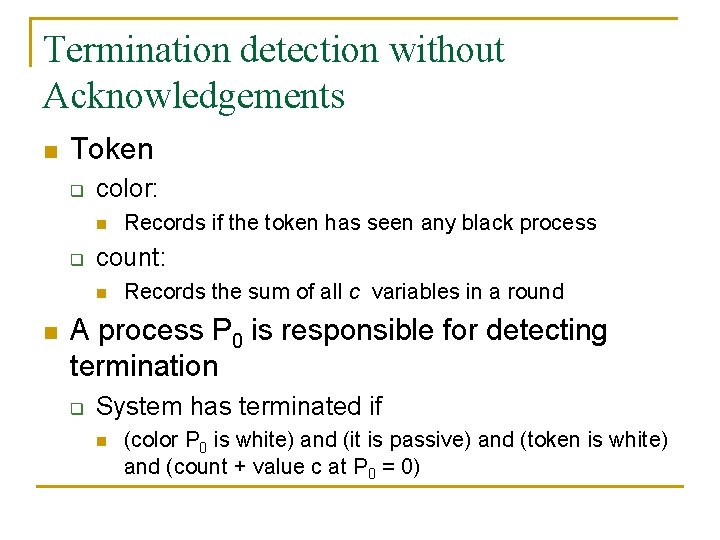
Termination detection without Acknowledgements n Token q color: n q count: n n Records if the token has seen any black process Records the sum of all c variables in a round A process P 0 is responsible for detecting termination q System has terminated if n (color P 0 is white) and (it is passive) and (token is white) and (count + value c at P 0 = 0)
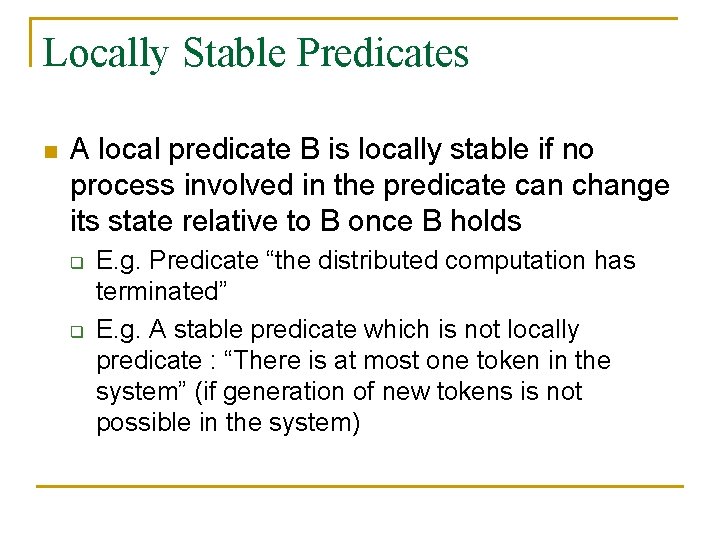
Locally Stable Predicates n A local predicate B is locally stable if no process involved in the predicate can change its state relative to B once B holds q q E. g. Predicate “the distributed computation has terminated” E. g. A stable predicate which is not locally predicate : “There is at most one token in the system” (if generation of new tokens is not possible in the system)
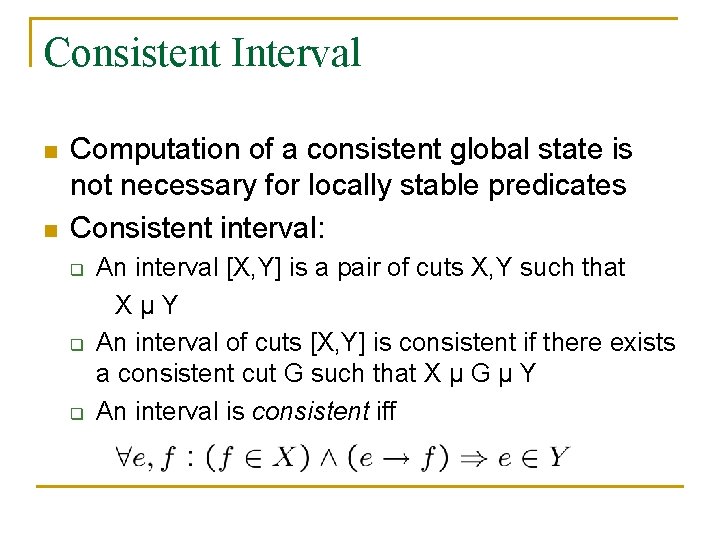
Consistent Interval n n Computation of a consistent global state is not necessary for locally stable predicates Consistent interval: q q q An interval [X, Y] is a pair of cuts X, Y such that XµY An interval of cuts [X, Y] is consistent if there exists a consistent cut G such that X µ G µ Y An interval is consistent iff
![Algorithm outline n Repeatedly compute consistent intervals X Y q q If a predicate Algorithm outline n Repeatedly compute consistent intervals [X, Y] q q If a predicate](https://slidetodoc.com/presentation_image/38d3cbb60fd033a26dfee5b8c691f76e/image-14.jpg)
Algorithm outline n Repeatedly compute consistent intervals [X, Y] q q If a predicate is true in cut Y and The values of the variables in predicate B have not changed during the interval Then B is true (though X, Y may be inconsistent cuts)
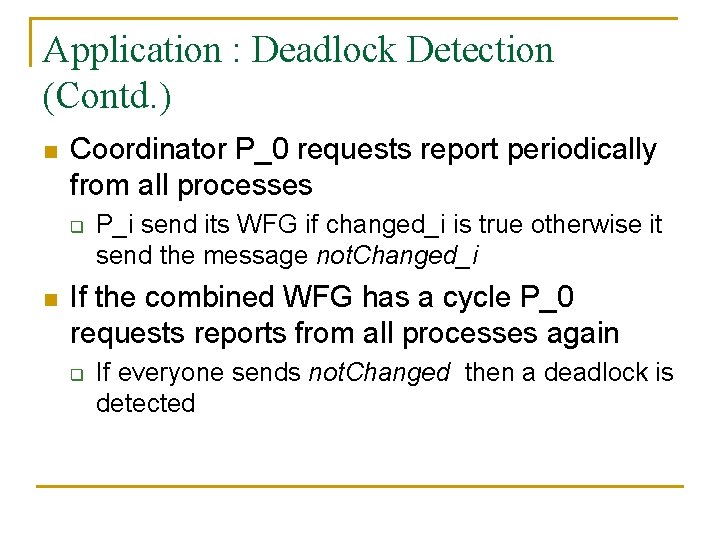
Application : Deadlock Detection (Contd. ) n Coordinator P_0 requests report periodically from all processes q n P_i send its WFG if changed_i is true otherwise it send the message not. Changed_i If the combined WFG has a cycle P_0 requests reports from all processes again q If everyone sends not. Changed then a deadlock is detected
What is diffused responsibility
Deadlocks can be described more precisely
All deadlocks involve conflicting needs for
Deadlocks can be described more precisely
Rag deadlock
Coffman deadlock
No preemption
Deadlocks
Deadlock
Sniffer for detecting lost mobiles
Detecting evolutionary forces in language change
Silver tickets adsecurity spn
How do fraud symptoms help in detecting fraud
Havex
Tcp connection diagram
In file organization a fixed format is used for records