Arrays Dr Jose Annunziato Arrays Up to this
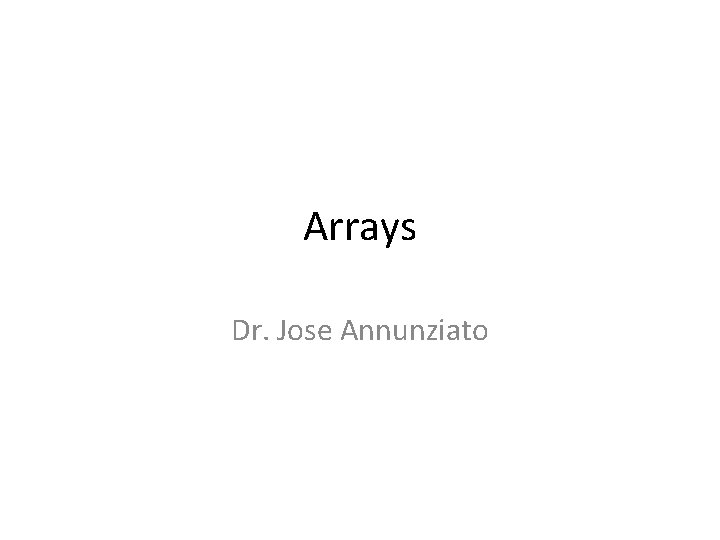
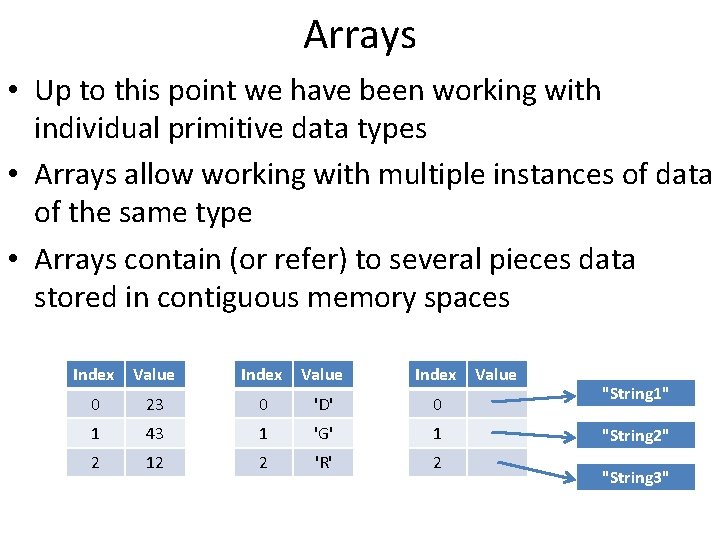
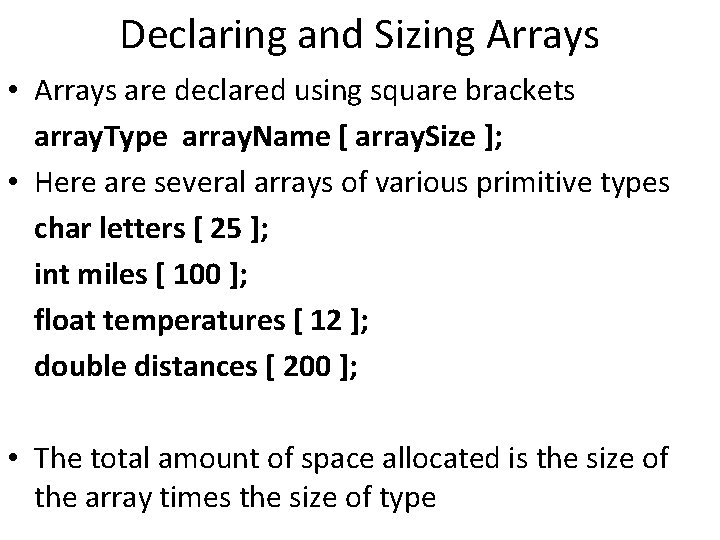
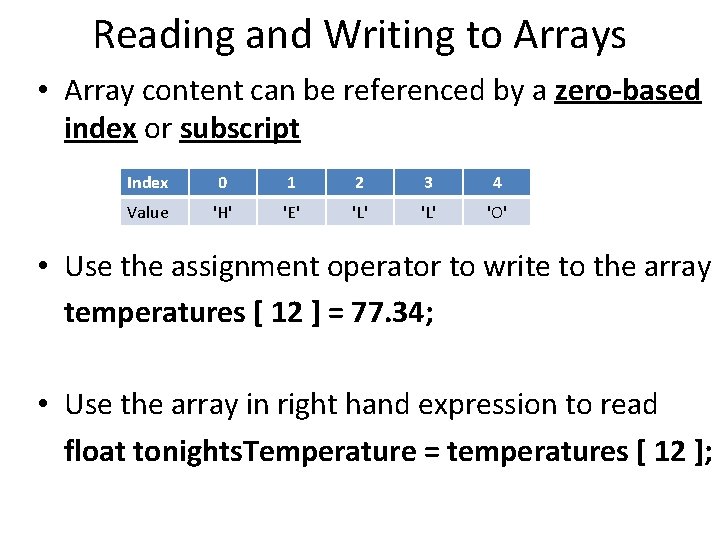
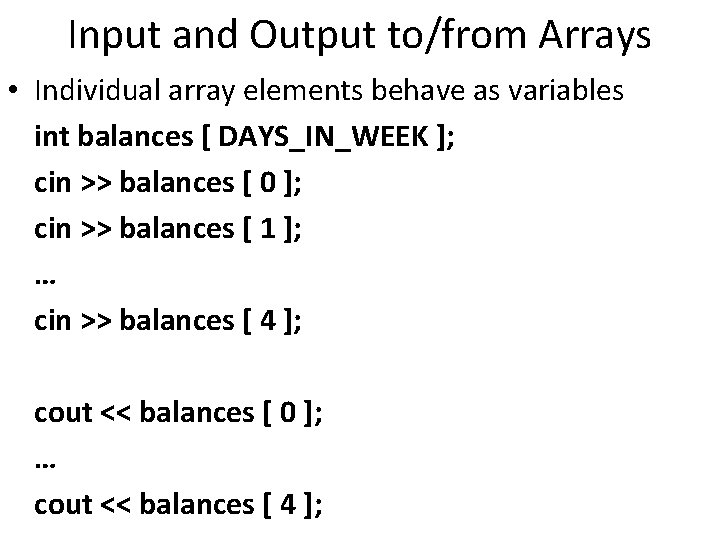
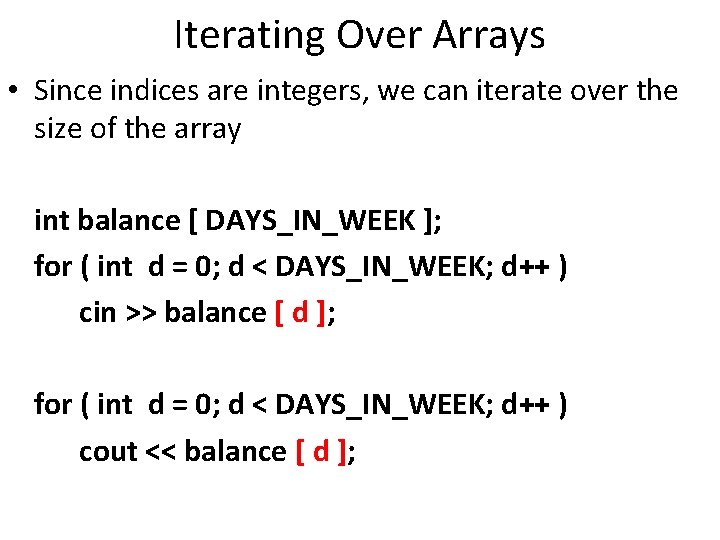
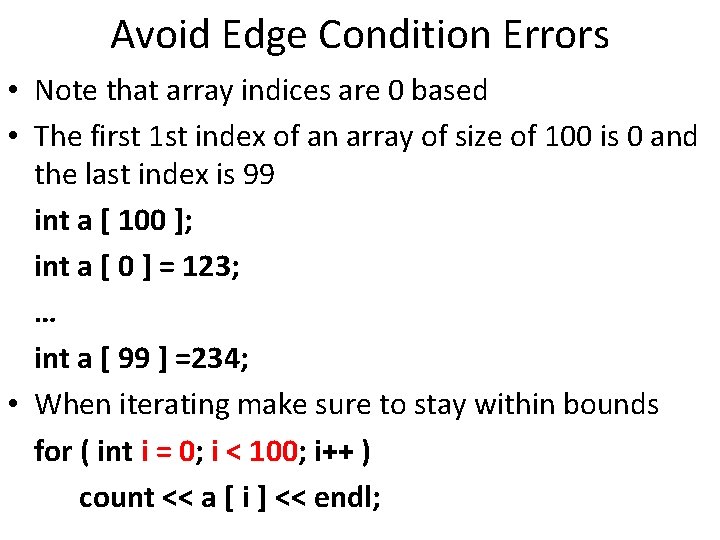
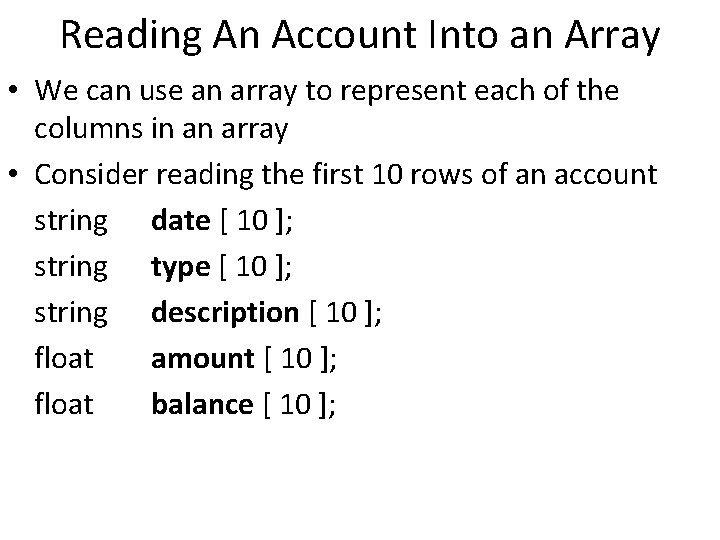
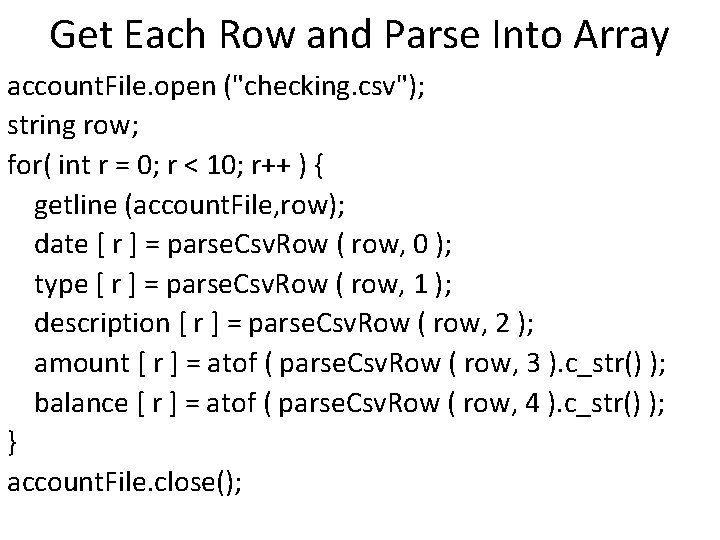
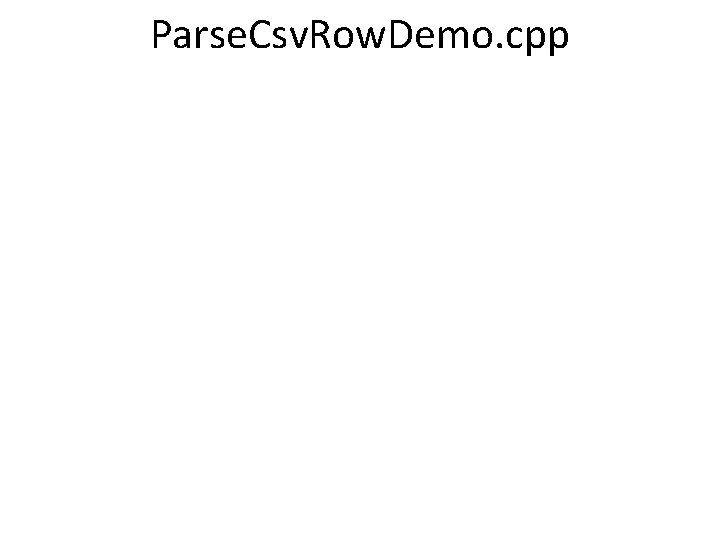
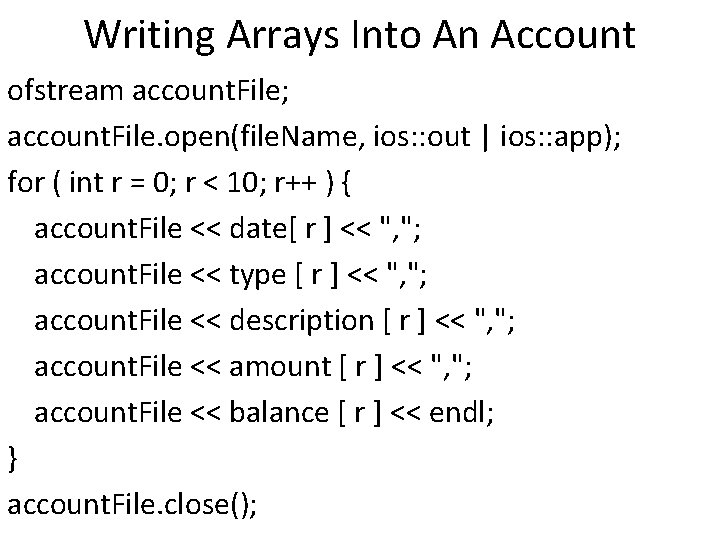
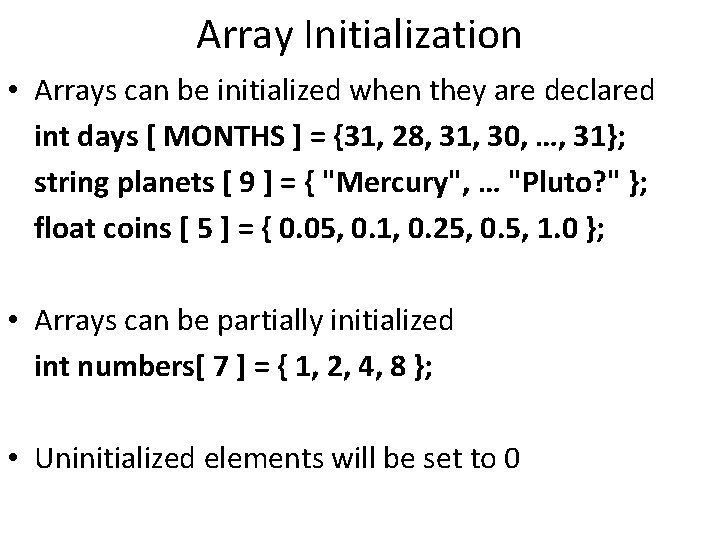
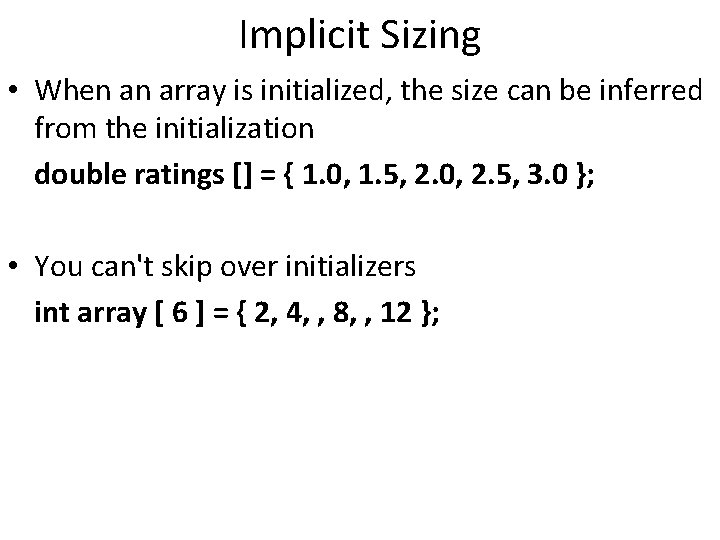
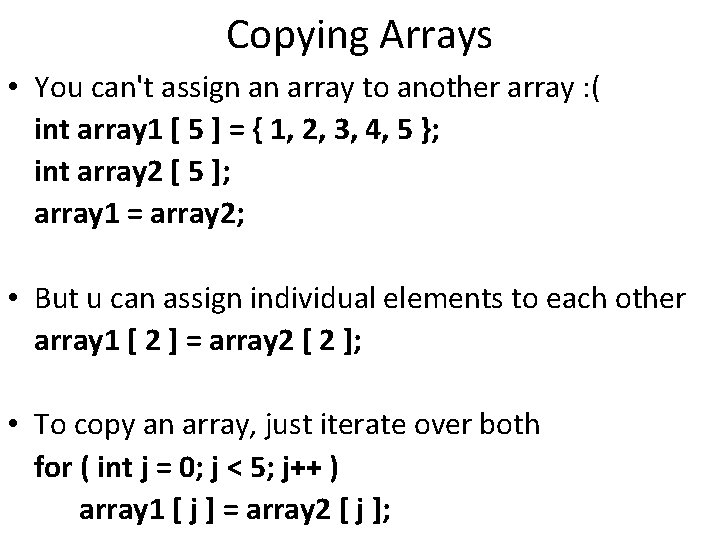
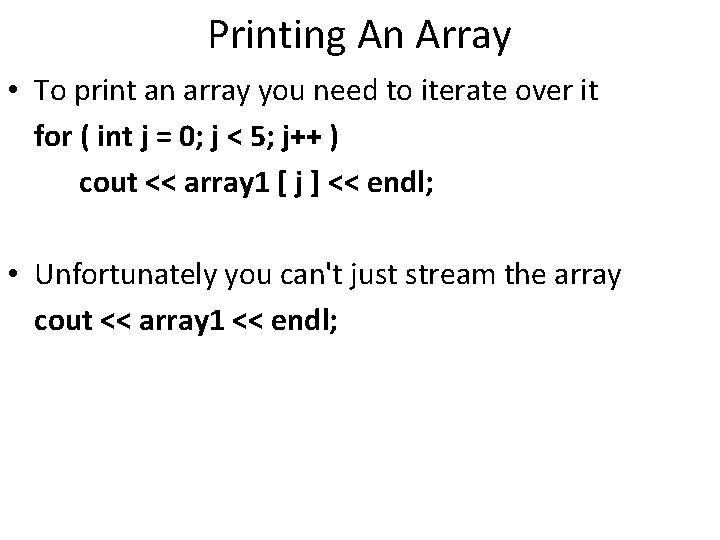
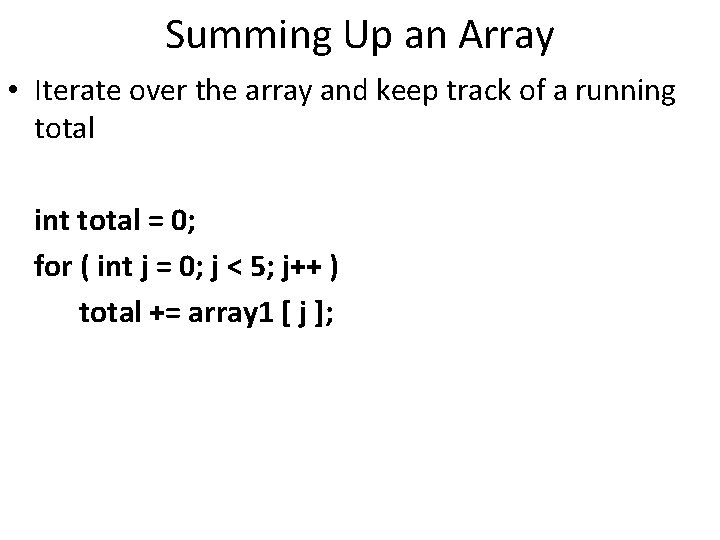
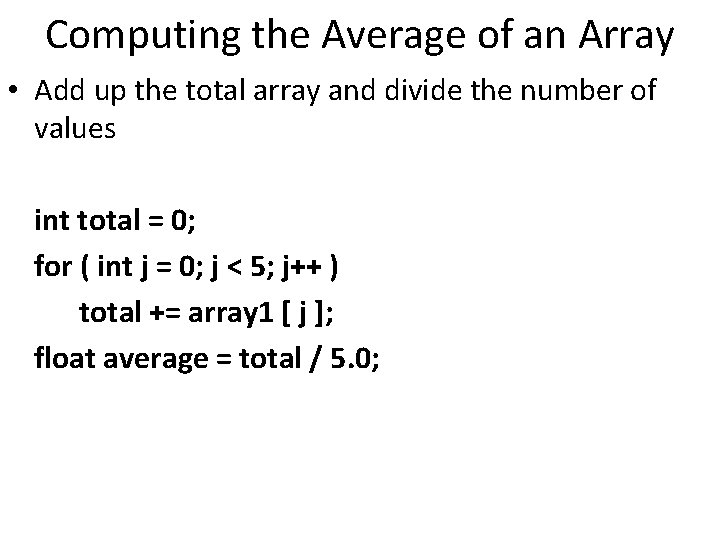
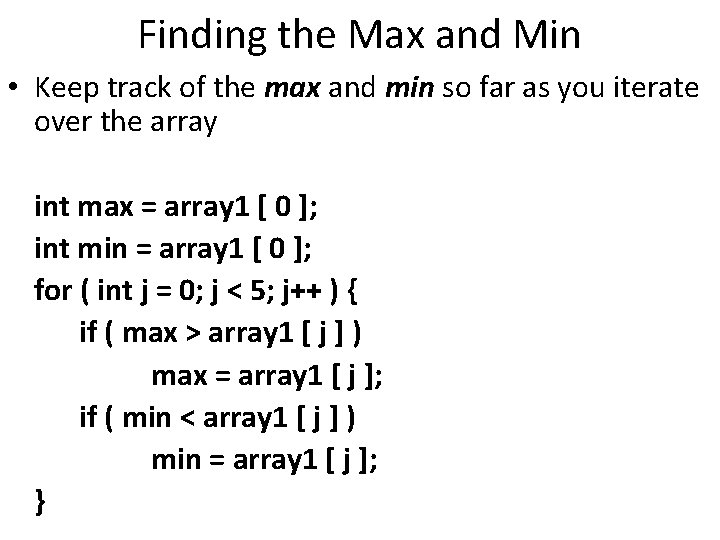
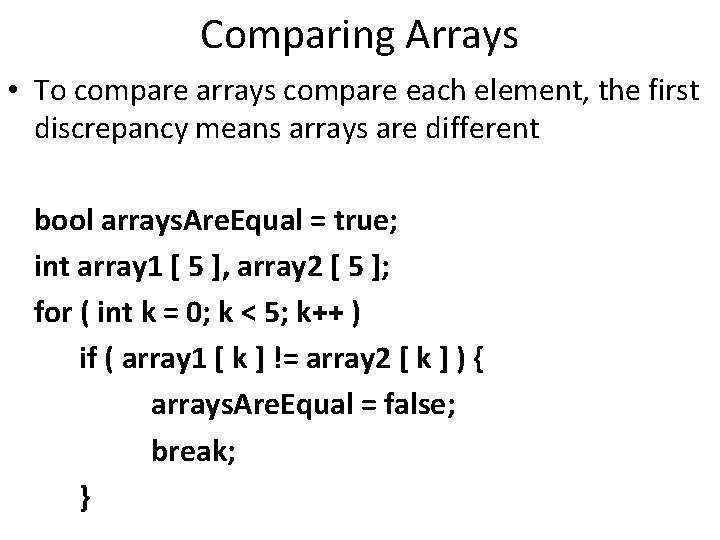
![Arrays as Function Arguments bool are. Arrays. Equal ( int a[], int b[], int Arrays as Function Arguments bool are. Arrays. Equal ( int a[], int b[], int](https://slidetodoc.com/presentation_image_h2/03444c1112b126da2a3b629aa19fb275/image-20.jpg)
![array. Max ( int [] ) int array. Max ( int array[], size ) array. Max ( int [] ) int array. Max ( int array[], size )](https://slidetodoc.com/presentation_image_h2/03444c1112b126da2a3b629aa19fb275/image-21.jpg)
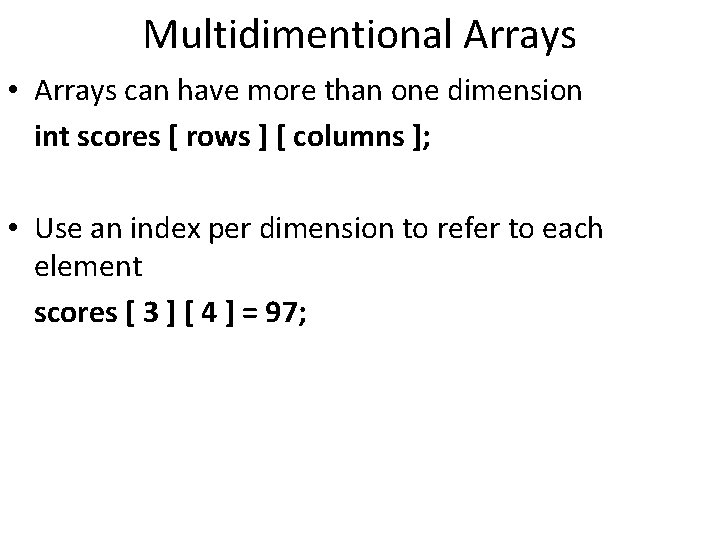
![Initializing Multidimensional Arrays • Multidimensional arrays are just arrays of arrays int scores[][] = Initializing Multidimensional Arrays • Multidimensional arrays are just arrays of arrays int scores[][] =](https://slidetodoc.com/presentation_image_h2/03444c1112b126da2a3b629aa19fb275/image-23.jpg)
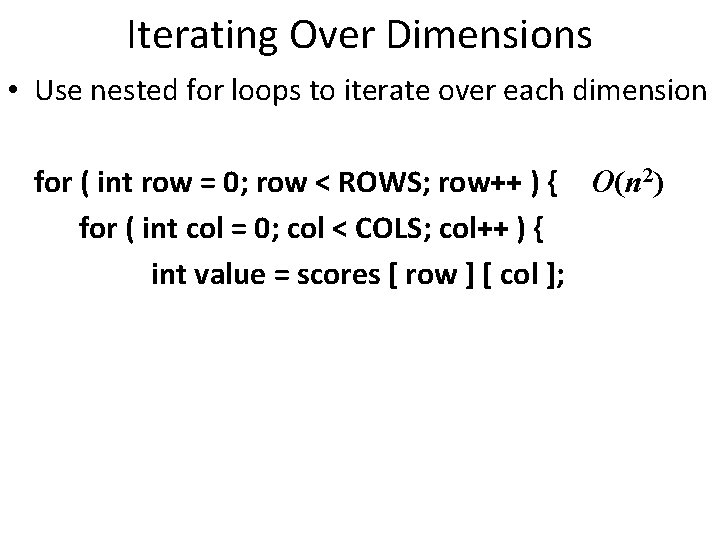
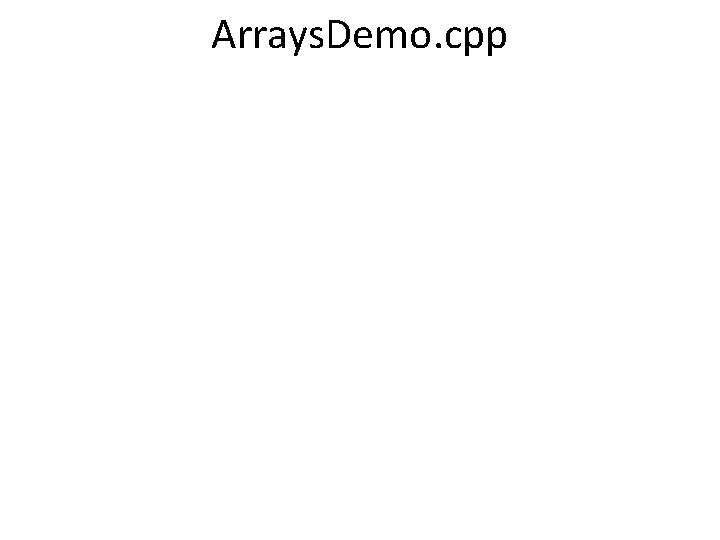
- Slides: 25
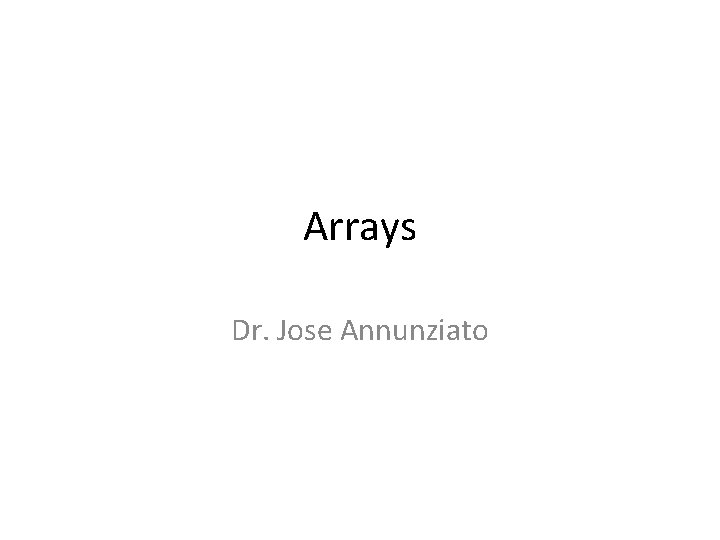
Arrays Dr. Jose Annunziato
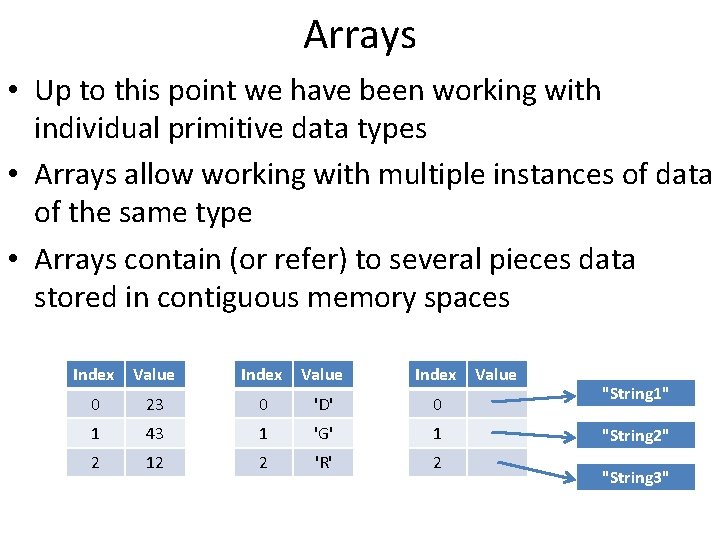
Arrays • Up to this point we have been working with individual primitive data types • Arrays allow working with multiple instances of data of the same type • Arrays contain (or refer) to several pieces data stored in contiguous memory spaces Index Value Index 0 23 0 'D' 0 1 43 1 'G' 1 2 12 2 'R' 2 Value "String 1" "String 2" "String 3"
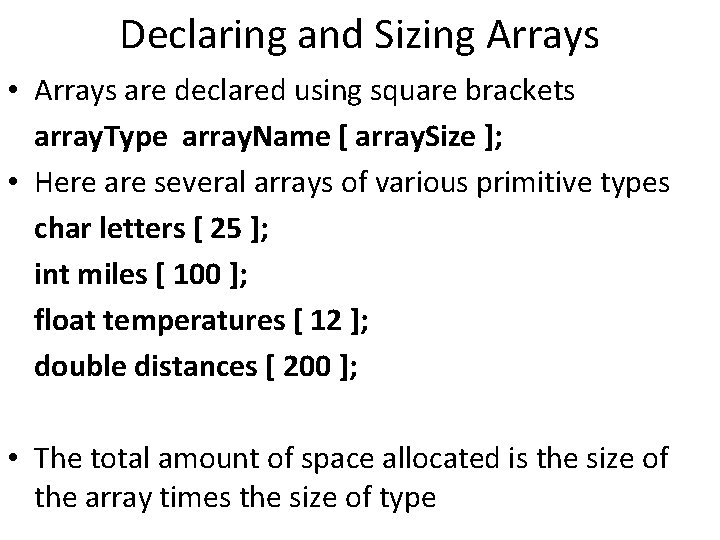
Declaring and Sizing Arrays • Arrays are declared using square brackets array. Type array. Name [ array. Size ]; • Here are several arrays of various primitive types char letters [ 25 ]; int miles [ 100 ]; float temperatures [ 12 ]; double distances [ 200 ]; • The total amount of space allocated is the size of the array times the size of type
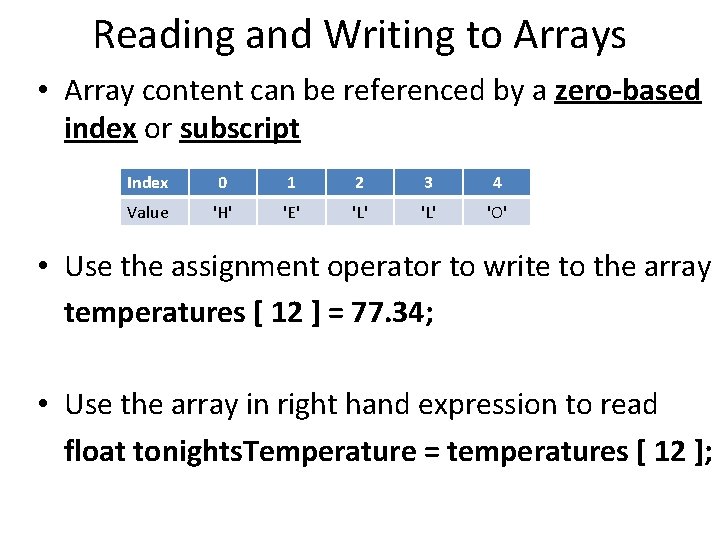
Reading and Writing to Arrays • Array content can be referenced by a zero-based index or subscript Index 0 1 2 3 4 Value 'H' 'E' 'L' 'O' • Use the assignment operator to write to the array temperatures [ 12 ] = 77. 34; • Use the array in right hand expression to read float tonights. Temperature = temperatures [ 12 ];
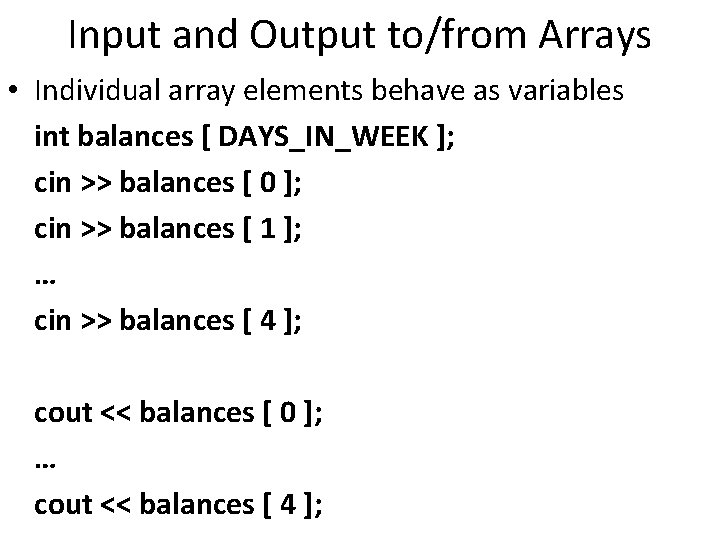
Input and Output to/from Arrays • Individual array elements behave as variables int balances [ DAYS_IN_WEEK ]; cin >> balances [ 0 ]; cin >> balances [ 1 ]; … cin >> balances [ 4 ]; cout << balances [ 0 ]; … cout << balances [ 4 ];
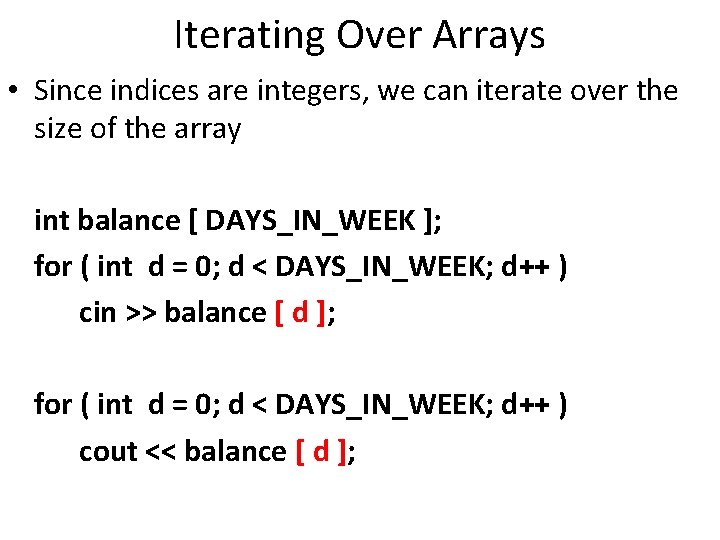
Iterating Over Arrays • Since indices are integers, we can iterate over the size of the array int balance [ DAYS_IN_WEEK ]; for ( int d = 0; d < DAYS_IN_WEEK; d++ ) cin >> balance [ d ]; for ( int d = 0; d < DAYS_IN_WEEK; d++ ) cout << balance [ d ];
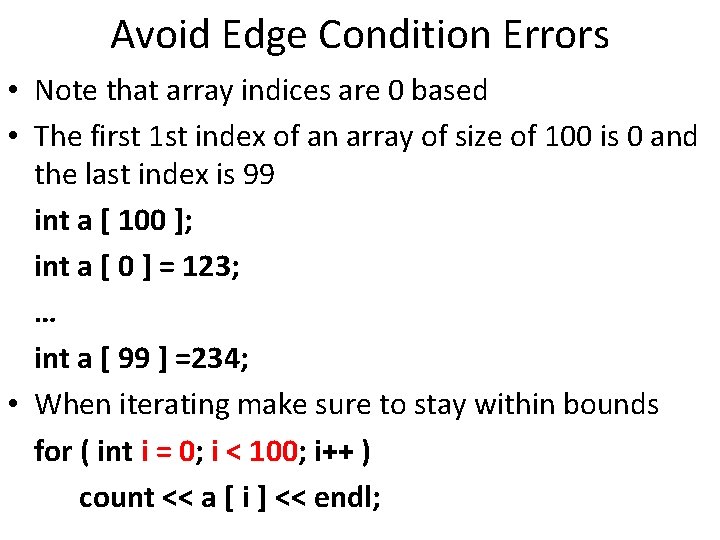
Avoid Edge Condition Errors • Note that array indices are 0 based • The first 1 st index of an array of size of 100 is 0 and the last index is 99 int a [ 100 ]; int a [ 0 ] = 123; … int a [ 99 ] =234; • When iterating make sure to stay within bounds for ( int i = 0; i < 100; i++ ) count << a [ i ] << endl;
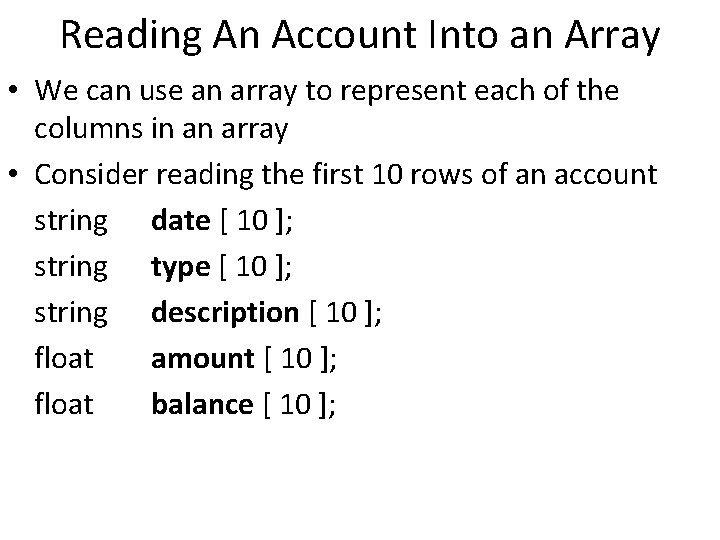
Reading An Account Into an Array • We can use an array to represent each of the columns in an array • Consider reading the first 10 rows of an account string date [ 10 ]; string type [ 10 ]; string description [ 10 ]; float amount [ 10 ]; float balance [ 10 ];
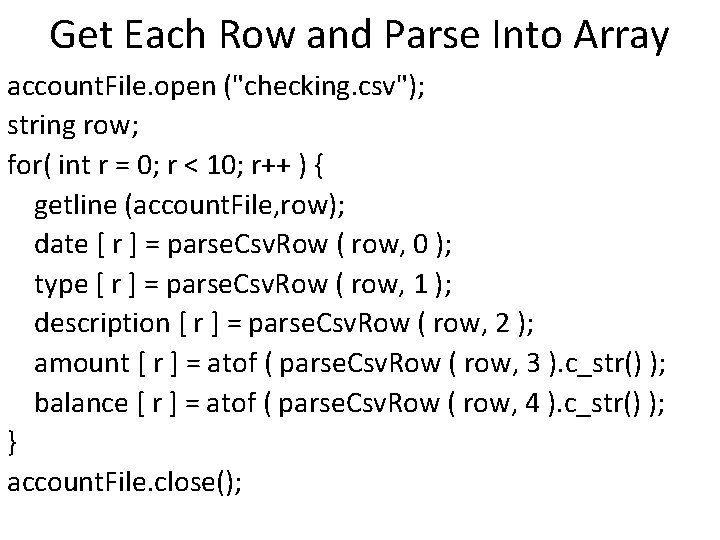
Get Each Row and Parse Into Array account. File. open ("checking. csv"); string row; for( int r = 0; r < 10; r++ ) { getline (account. File, row); date [ r ] = parse. Csv. Row ( row, 0 ); type [ r ] = parse. Csv. Row ( row, 1 ); description [ r ] = parse. Csv. Row ( row, 2 ); amount [ r ] = atof ( parse. Csv. Row ( row, 3 ). c_str() ); balance [ r ] = atof ( parse. Csv. Row ( row, 4 ). c_str() ); } account. File. close();
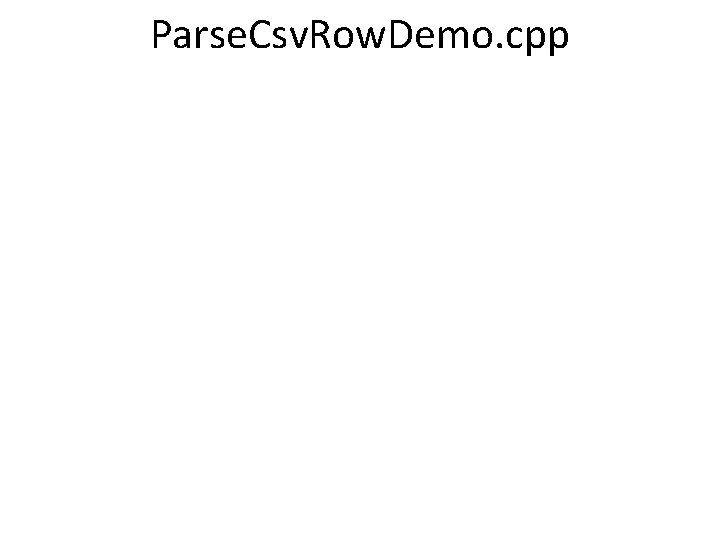
Parse. Csv. Row. Demo. cpp
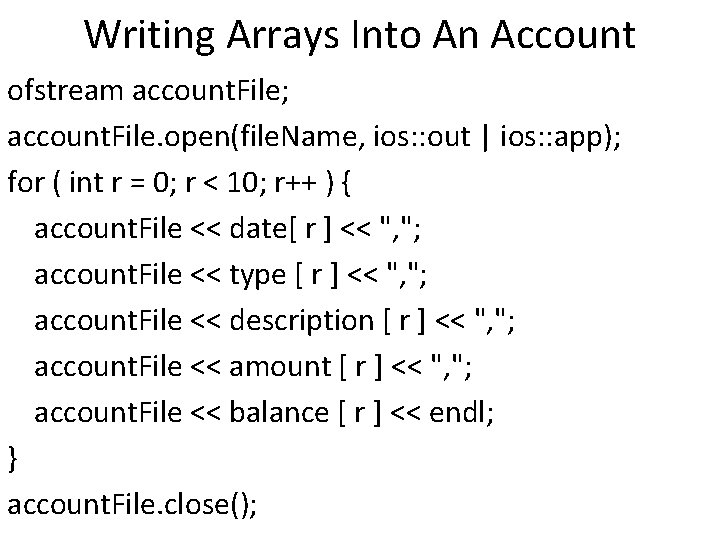
Writing Arrays Into An Account ofstream account. File; account. File. open(file. Name, ios: : out | ios: : app); for ( int r = 0; r < 10; r++ ) { account. File << date[ r ] << ", "; account. File << type [ r ] << ", "; account. File << description [ r ] << ", "; account. File << amount [ r ] << ", "; account. File << balance [ r ] << endl; } account. File. close();
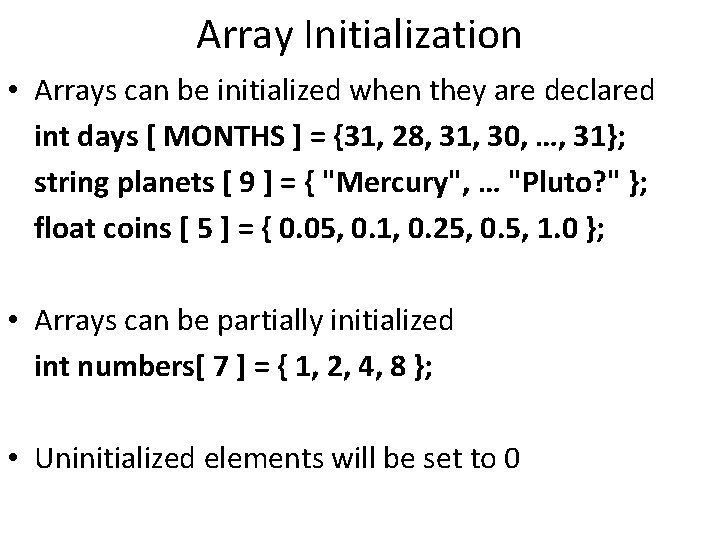
Array Initialization • Arrays can be initialized when they are declared int days [ MONTHS ] = {31, 28, 31, 30, …, 31}; string planets [ 9 ] = { "Mercury", … "Pluto? " }; float coins [ 5 ] = { 0. 05, 0. 1, 0. 25, 0. 5, 1. 0 }; • Arrays can be partially initialized int numbers[ 7 ] = { 1, 2, 4, 8 }; • Uninitialized elements will be set to 0
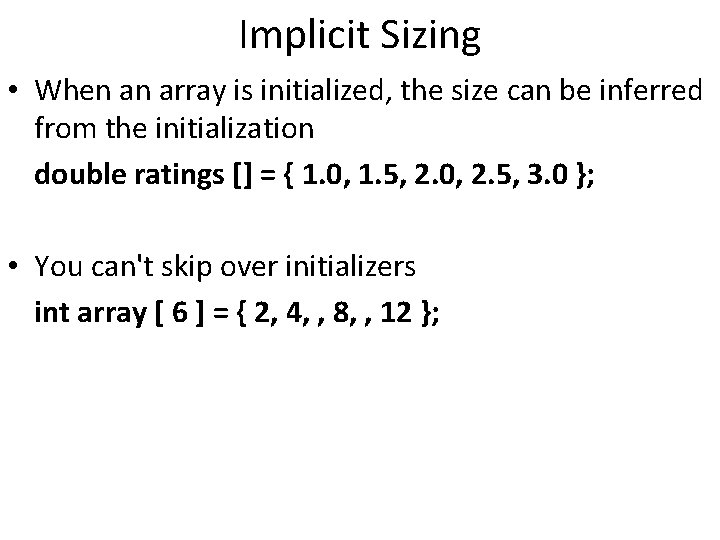
Implicit Sizing • When an array is initialized, the size can be inferred from the initialization double ratings [] = { 1. 0, 1. 5, 2. 0, 2. 5, 3. 0 }; • You can't skip over initializers int array [ 6 ] = { 2, 4, , 8, , 12 };
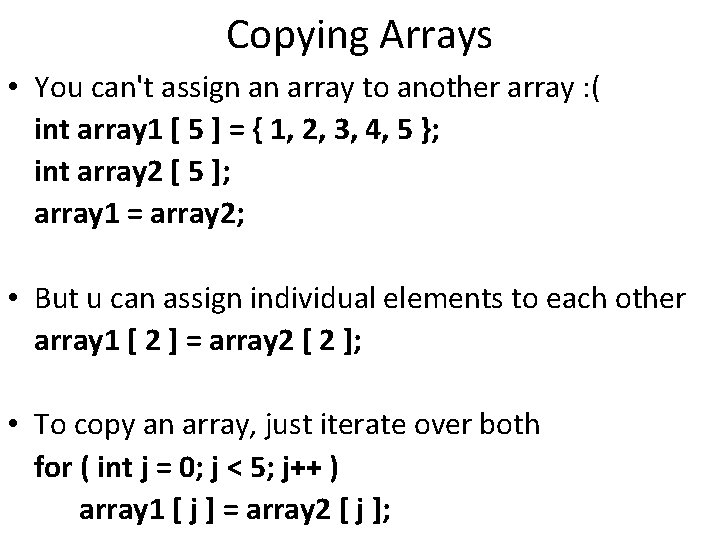
Copying Arrays • You can't assign an array to another array : ( int array 1 [ 5 ] = { 1, 2, 3, 4, 5 }; int array 2 [ 5 ]; array 1 = array 2; • But u can assign individual elements to each other array 1 [ 2 ] = array 2 [ 2 ]; • To copy an array, just iterate over both for ( int j = 0; j < 5; j++ ) array 1 [ j ] = array 2 [ j ];
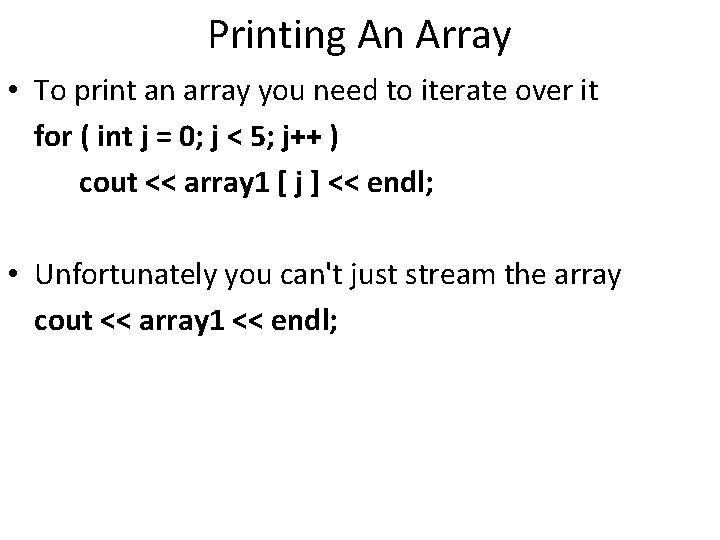
Printing An Array • To print an array you need to iterate over it for ( int j = 0; j < 5; j++ ) cout << array 1 [ j ] << endl; • Unfortunately you can't just stream the array cout << array 1 << endl;
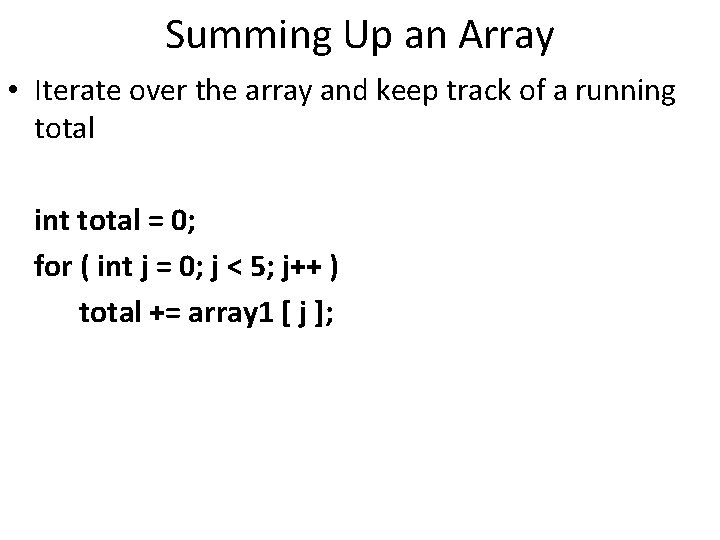
Summing Up an Array • Iterate over the array and keep track of a running total int total = 0; for ( int j = 0; j < 5; j++ ) total += array 1 [ j ];
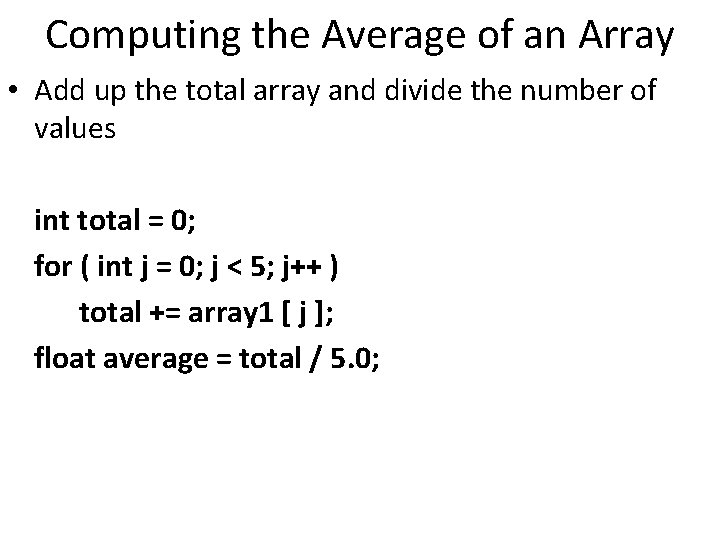
Computing the Average of an Array • Add up the total array and divide the number of values int total = 0; for ( int j = 0; j < 5; j++ ) total += array 1 [ j ]; float average = total / 5. 0;
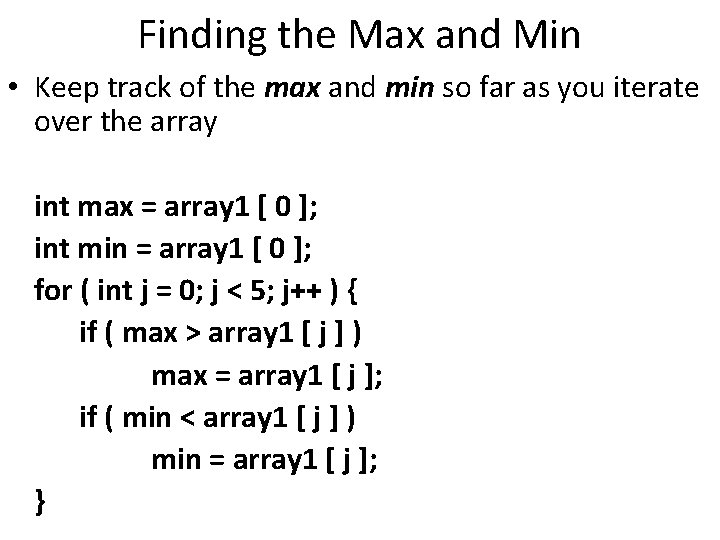
Finding the Max and Min • Keep track of the max and min so far as you iterate over the array int max = array 1 [ 0 ]; int min = array 1 [ 0 ]; for ( int j = 0; j < 5; j++ ) { if ( max > array 1 [ j ] ) max = array 1 [ j ]; if ( min < array 1 [ j ] ) min = array 1 [ j ]; }
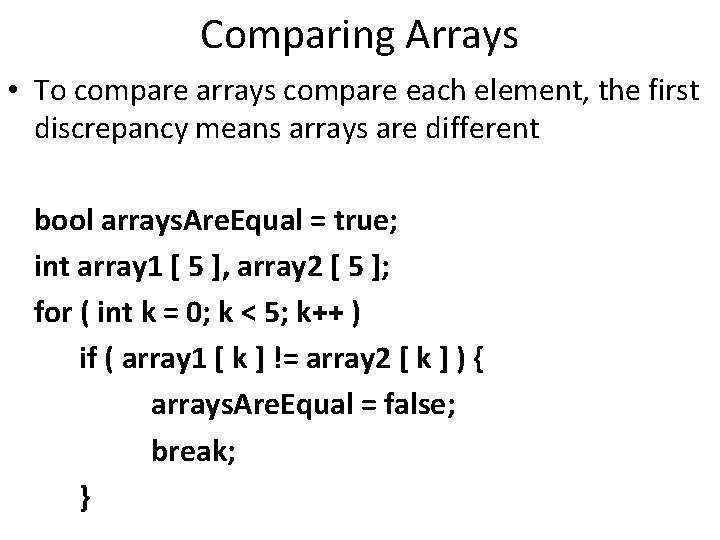
Comparing Arrays • To compare arrays compare each element, the first discrepancy means arrays are different bool arrays. Are. Equal = true; int array 1 [ 5 ], array 2 [ 5 ]; for ( int k = 0; k < 5; k++ ) if ( array 1 [ k ] != array 2 [ k ] ) { arrays. Are. Equal = false; break; }
![Arrays as Function Arguments bool are Arrays Equal int a int b int Arrays as Function Arguments bool are. Arrays. Equal ( int a[], int b[], int](https://slidetodoc.com/presentation_image_h2/03444c1112b126da2a3b629aa19fb275/image-20.jpg)
Arrays as Function Arguments bool are. Arrays. Equal ( int a[], int b[], int size ) { for ( int k = 0; k < size; k++ ) O(n) if ( a [ k ] != b [ k ] ) return false; return true; } int main () { int x[ 4 ] = {1, 2, 3, 4}; int y[ 4 ] = {1, 2, 3, 4}; cout << are. Arrays. Equal ( x, y, 4 ) << endl; }
![array Max int int array Max int array size array. Max ( int [] ) int array. Max ( int array[], size )](https://slidetodoc.com/presentation_image_h2/03444c1112b126da2a3b629aa19fb275/image-21.jpg)
array. Max ( int [] ) int array. Max ( int array[], size ) { int max = array [ 0 ]; for ( int j = 0; j < 5; j++ ) { if ( max > array [ j ] ) max = array [ j ]; return max; } int main() { int[] a = {1, 2, 3, 4, 5}; cout << array. Max ( a, 5 ) << endl; } O(n)
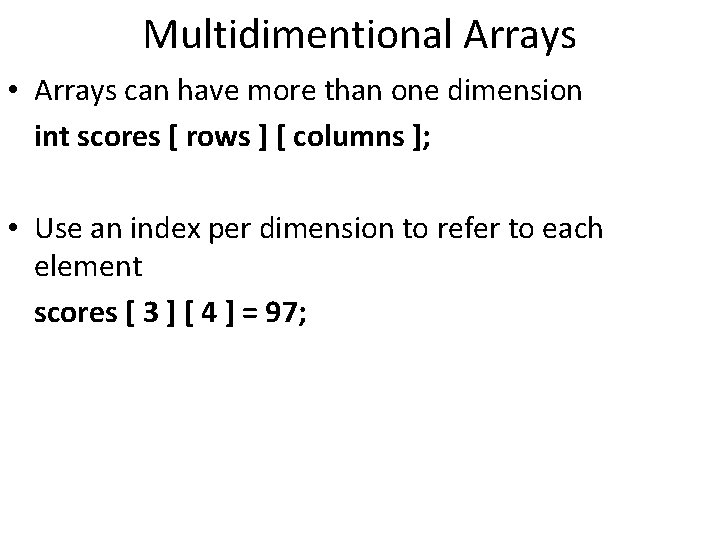
Multidimentional Arrays • Arrays can have more than one dimension int scores [ rows ] [ columns ]; • Use an index per dimension to refer to each element scores [ 3 ] [ 4 ] = 97;
![Initializing Multidimensional Arrays Multidimensional arrays are just arrays of arrays int scores Initializing Multidimensional Arrays • Multidimensional arrays are just arrays of arrays int scores[][] =](https://slidetodoc.com/presentation_image_h2/03444c1112b126da2a3b629aa19fb275/image-23.jpg)
Initializing Multidimensional Arrays • Multidimensional arrays are just arrays of arrays int scores[][] = { { 89, 78, 67, 78, 87 }, { 98, 87, 78, 90 }, {76, 87, 89 } }
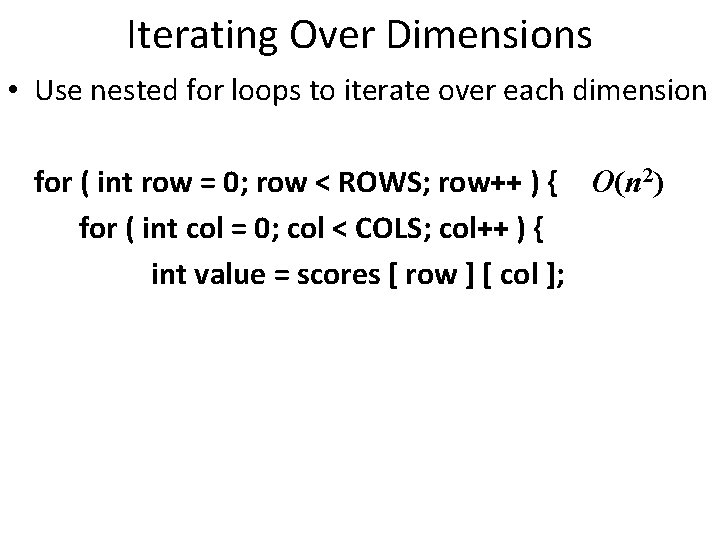
Iterating Over Dimensions • Use nested for loops to iterate over each dimension for ( int row = 0; row < ROWS; row++ ) { O(n 2) for ( int col = 0; col < COLS; col++ ) { int value = scores [ row ] [ col ];
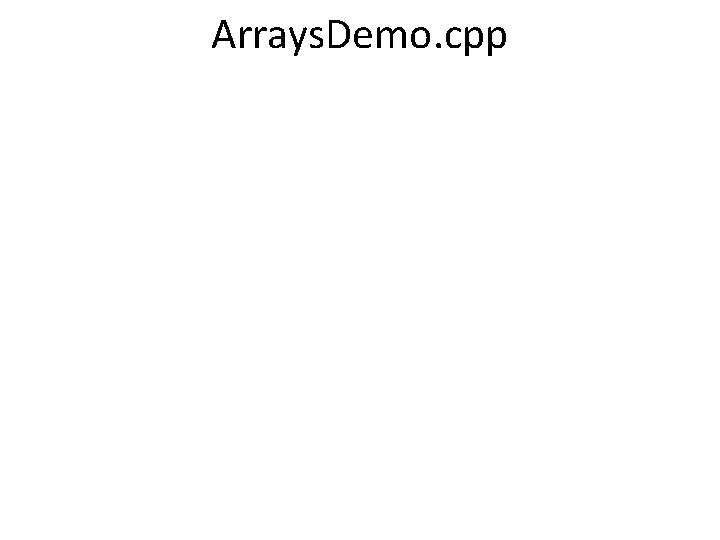
Arrays. Demo. cpp
Jose annunziato
Jose annunziato
"augusto branco" -josé -jose
Arrays as adt
Partially filled arrays
Arrays visual basic
Small basic array
Arrays
Arreglos unidimensionales en java ejemplos
Parallel arrays java
2d array pascal
Global arrays in c
Array of strings assembly
Facts about arrays
Partially filled arrays
C++ parallel arrays
Python parallel arrays
Arrays bidimensionales java
Disadvantages of arrays
Array of arrays c++
Declare array in mips
Computer science arrays
Redundant arrays of independent disks
Why do we need arrays?
Day 3: arrays
What are the disadvantages of arrays