7 7 Array Lists Limitations of Array Arrays
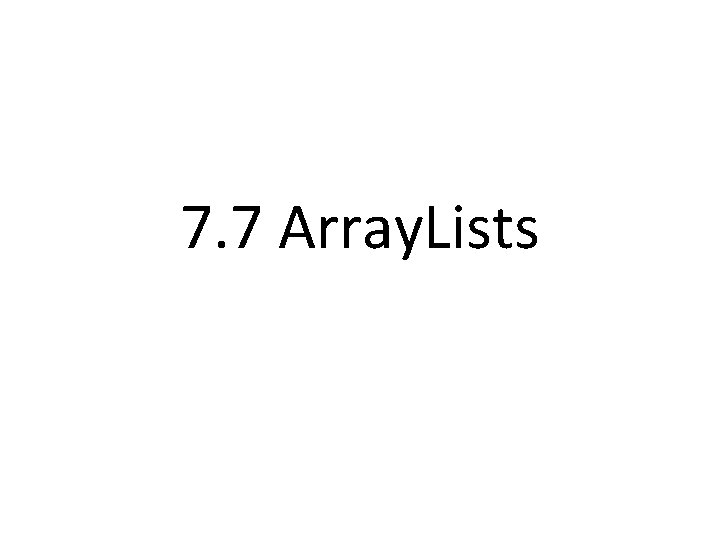
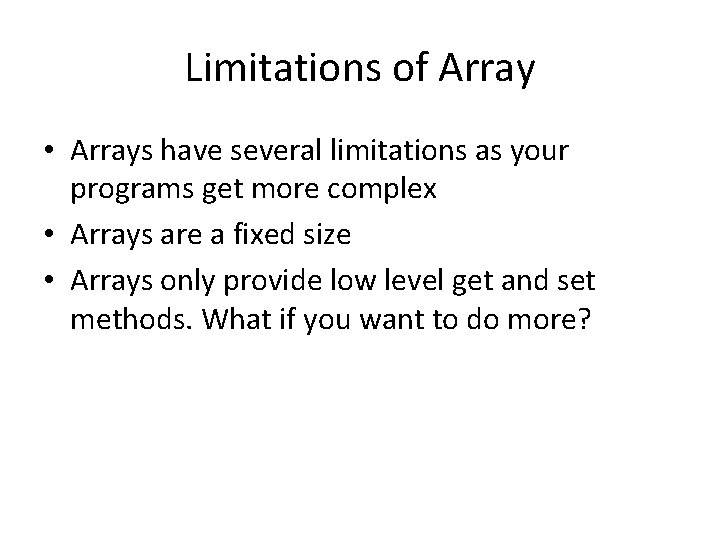
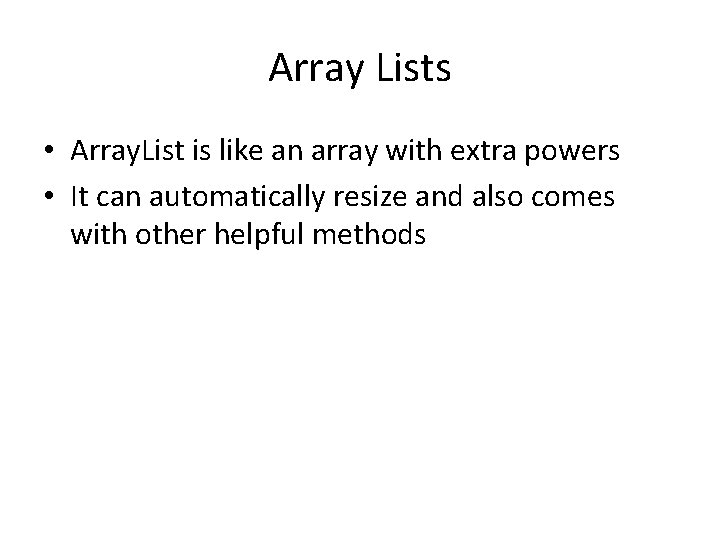
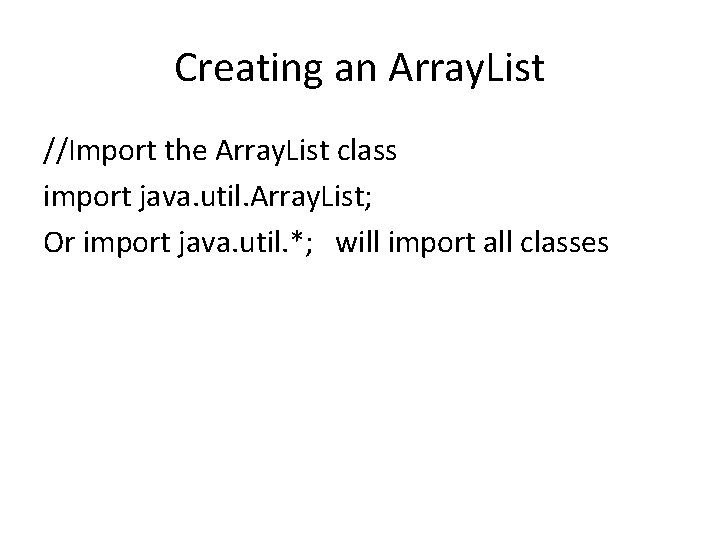
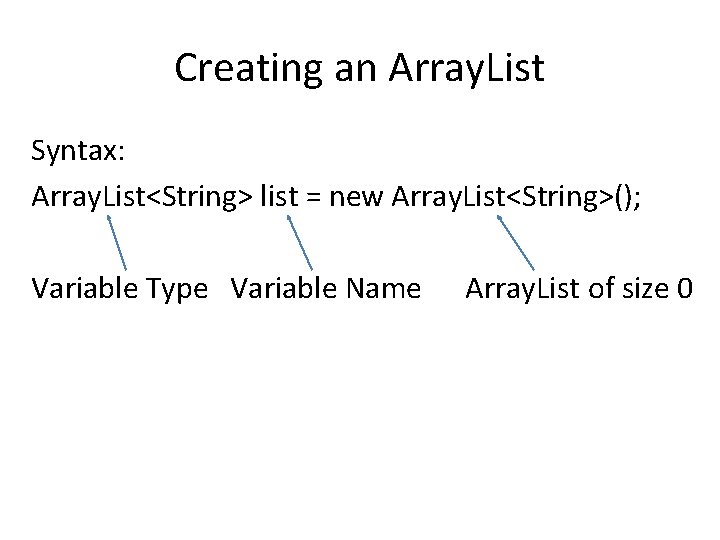
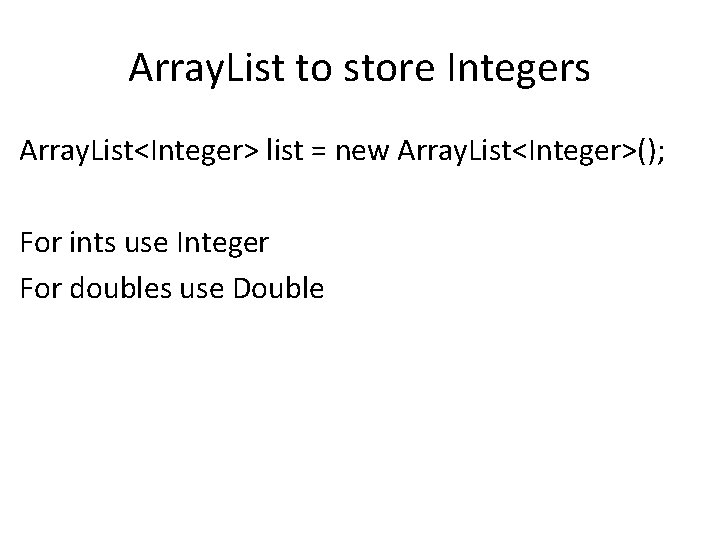
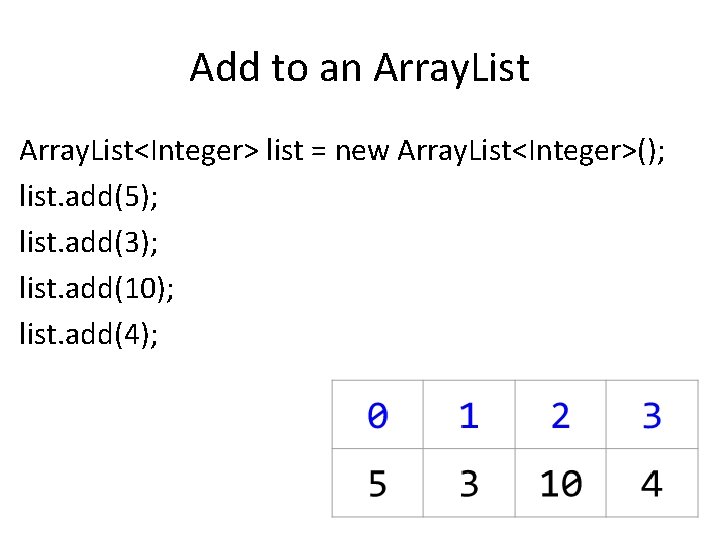
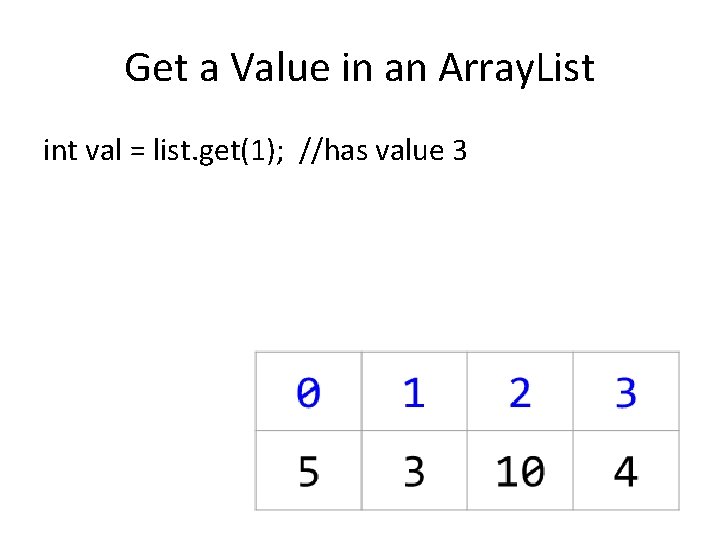
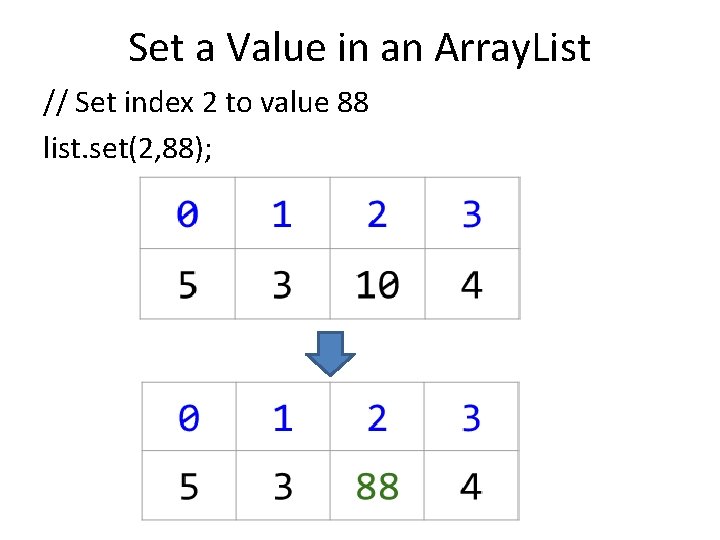
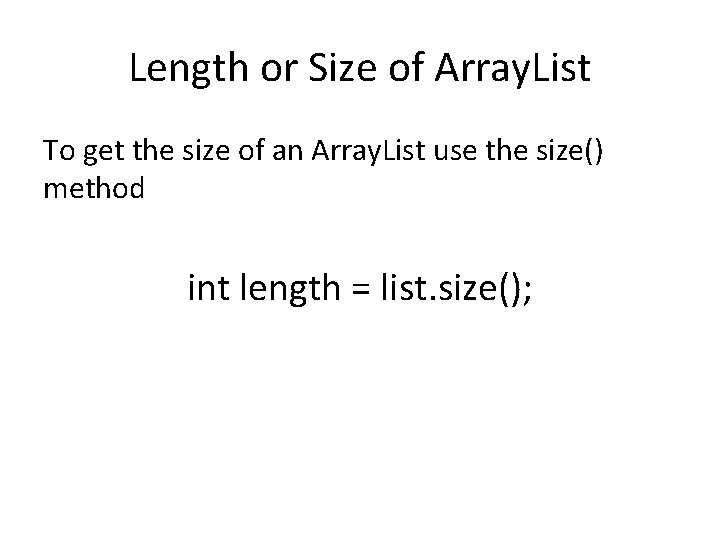
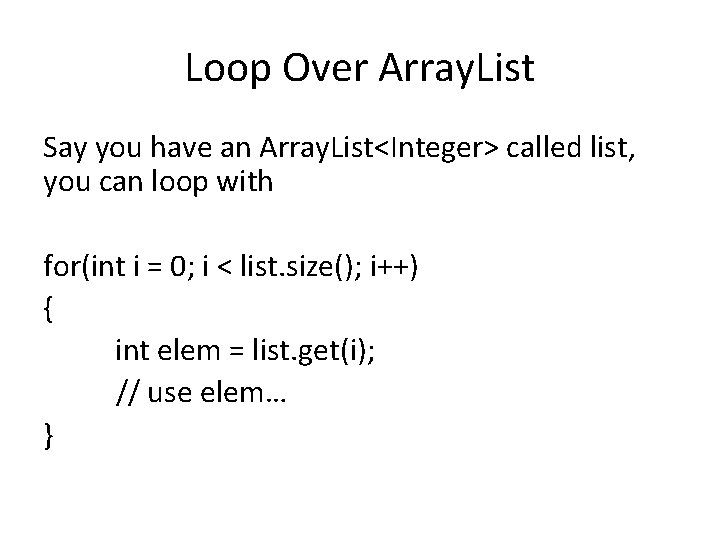
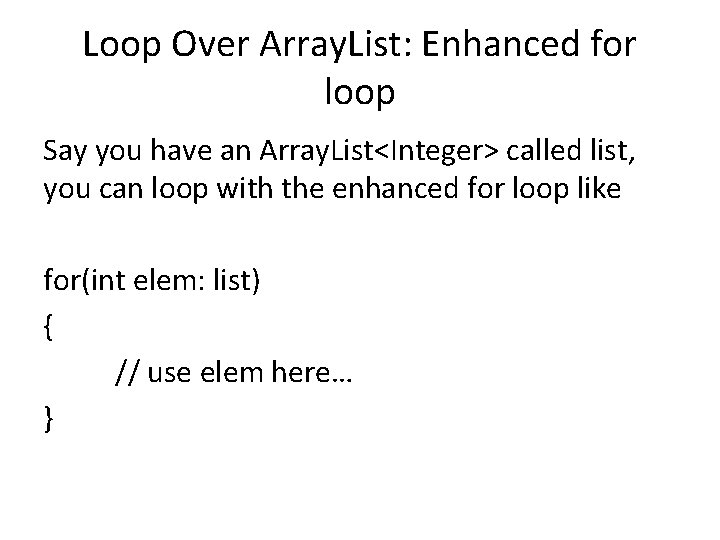
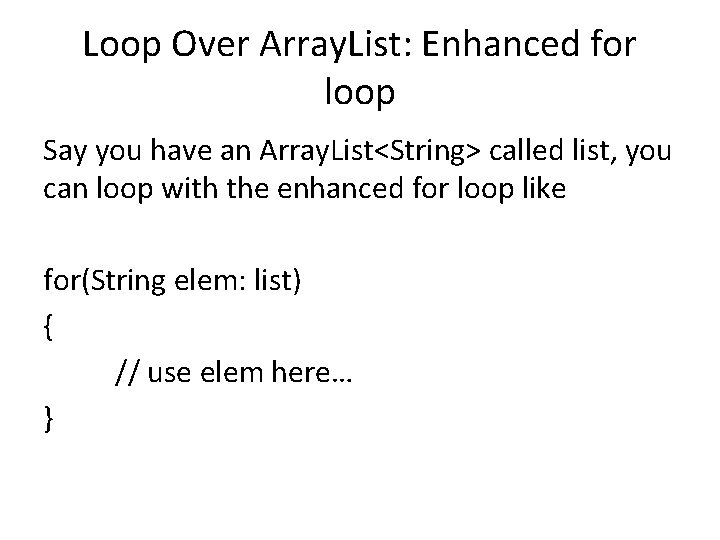
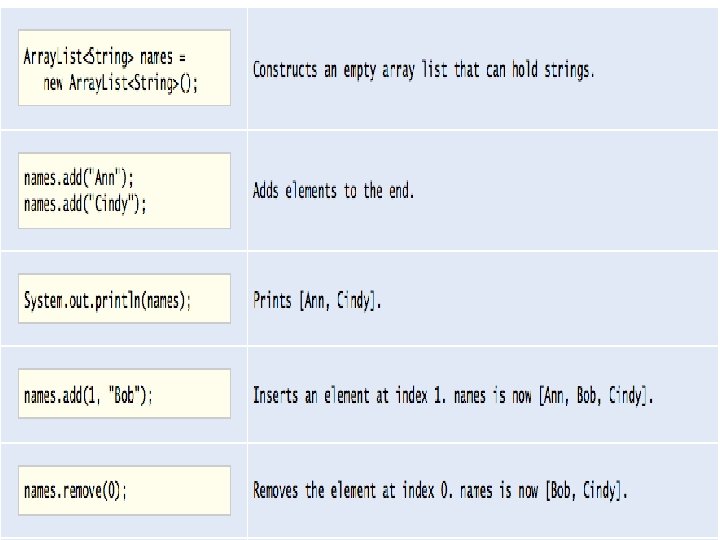
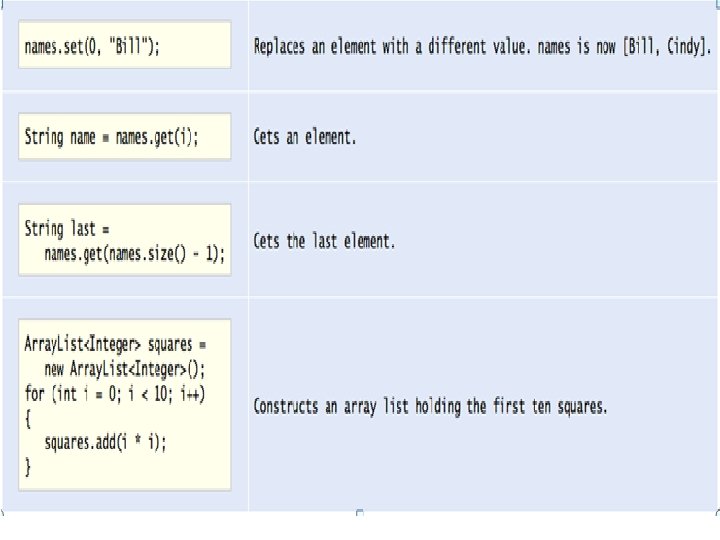
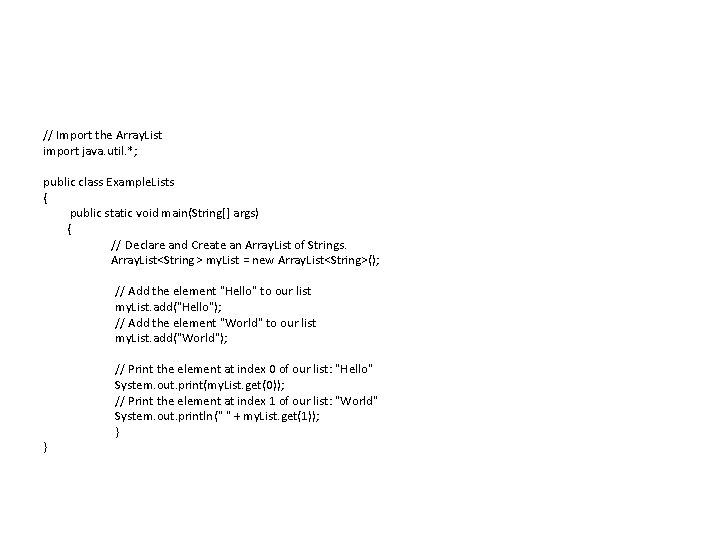
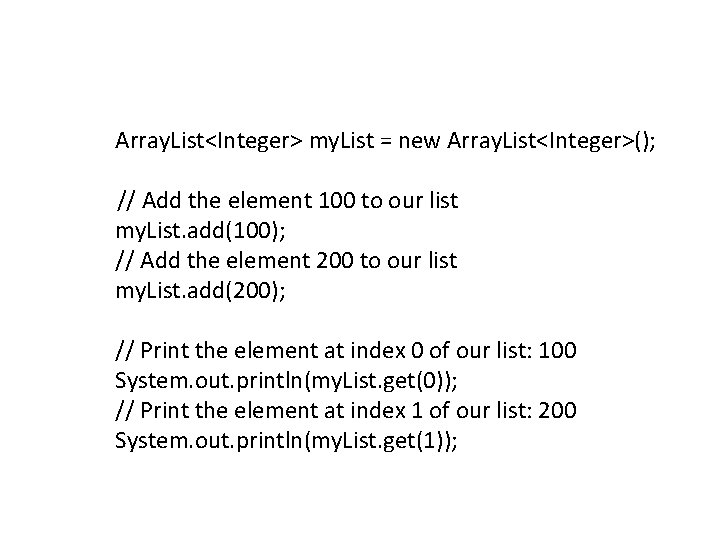
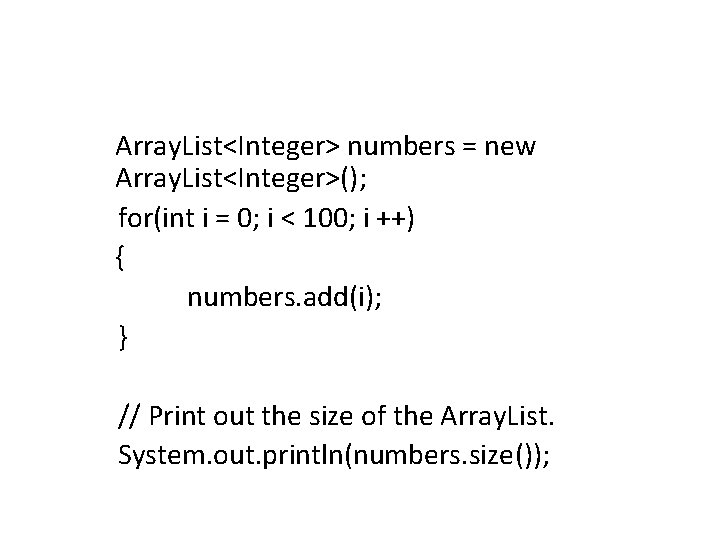
![public static void main(String[] args) { Array. List<Integer> numbers = new Array. List<Integer>(); // public static void main(String[] args) { Array. List<Integer> numbers = new Array. List<Integer>(); //](https://slidetodoc.com/presentation_image_h2/6fb8cc723c484592a3359864fc7af55e/image-19.jpg)
- Slides: 19
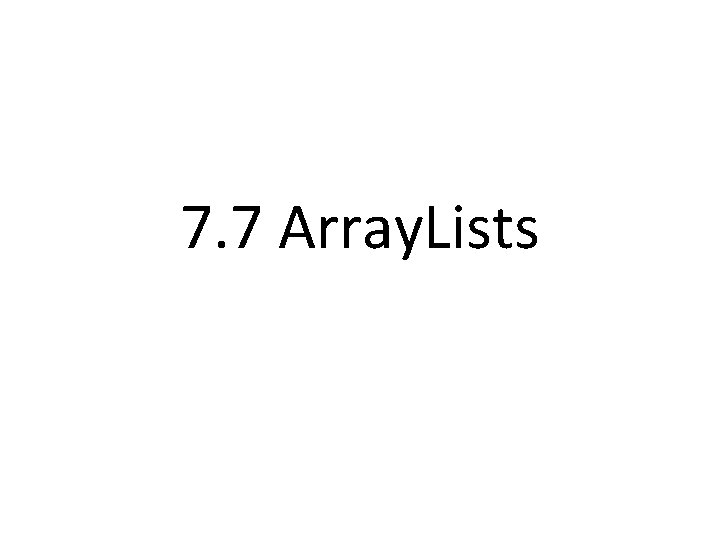
7. 7 Array. Lists
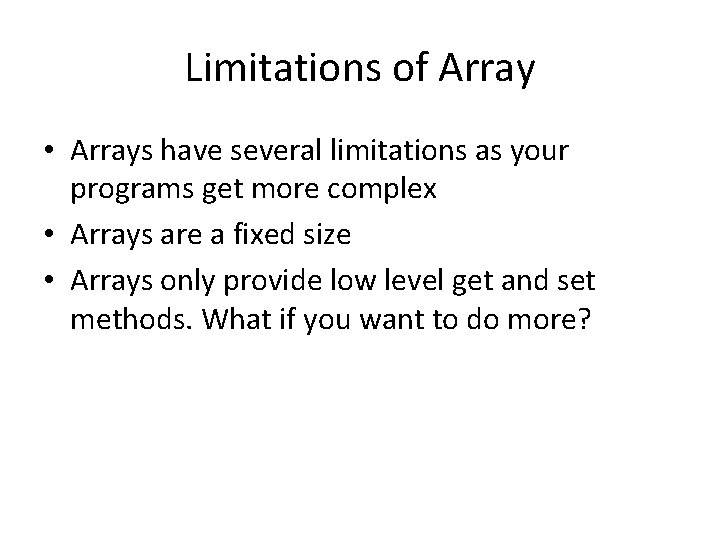
Limitations of Array • Arrays have several limitations as your programs get more complex • Arrays are a fixed size • Arrays only provide low level get and set methods. What if you want to do more?
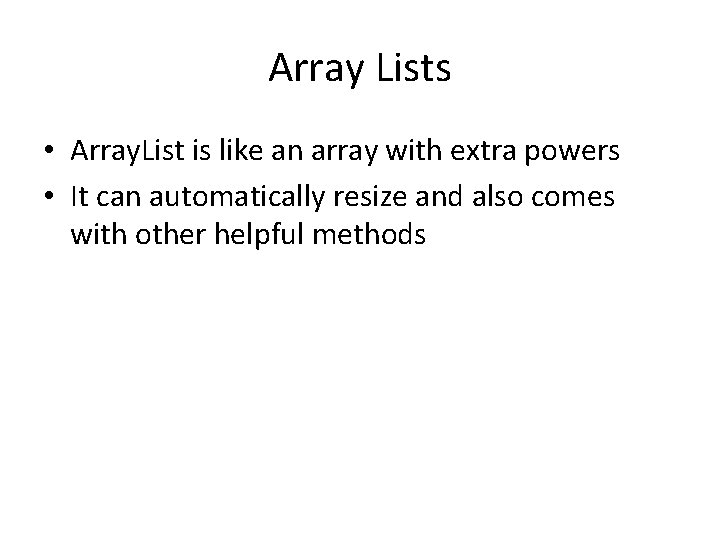
Array Lists • Array. List is like an array with extra powers • It can automatically resize and also comes with other helpful methods
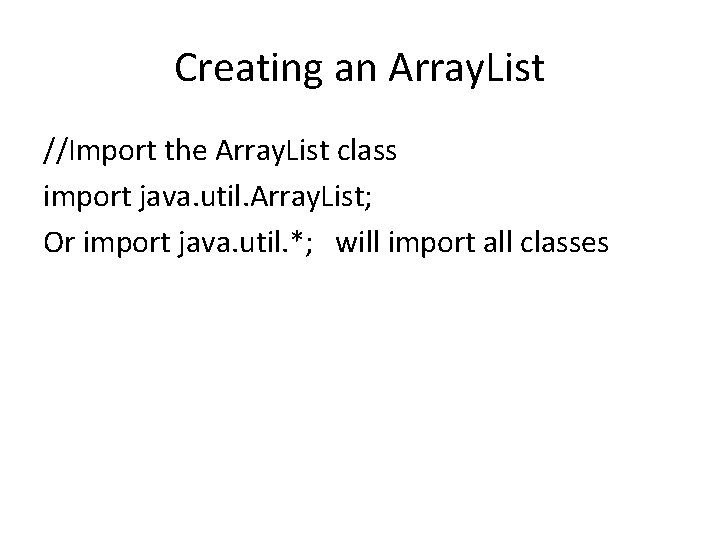
Creating an Array. List //Import the Array. List class import java. util. Array. List; Or import java. util. *; will import all classes
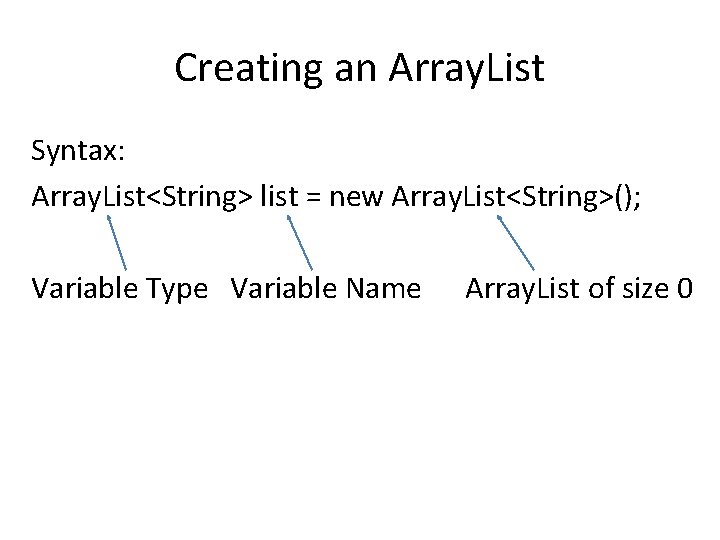
Creating an Array. List Syntax: Array. List<String> list = new Array. List<String>(); Variable Type Variable Name Array. List of size 0
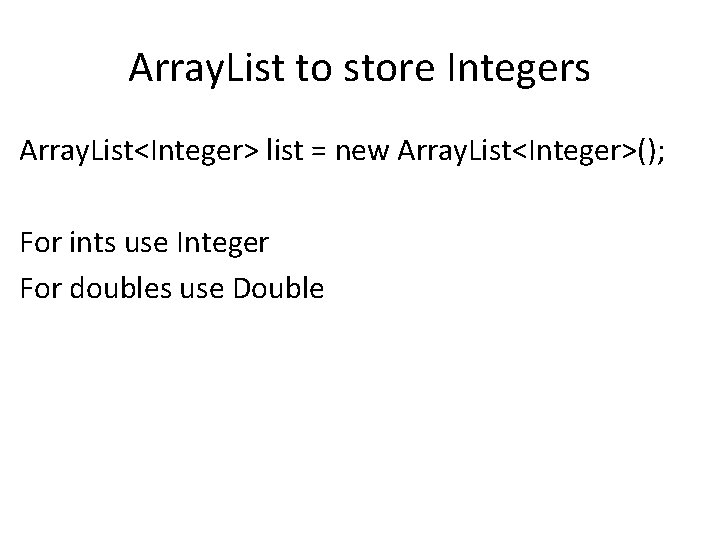
Array. List to store Integers Array. List<Integer> list = new Array. List<Integer>(); For ints use Integer For doubles use Double
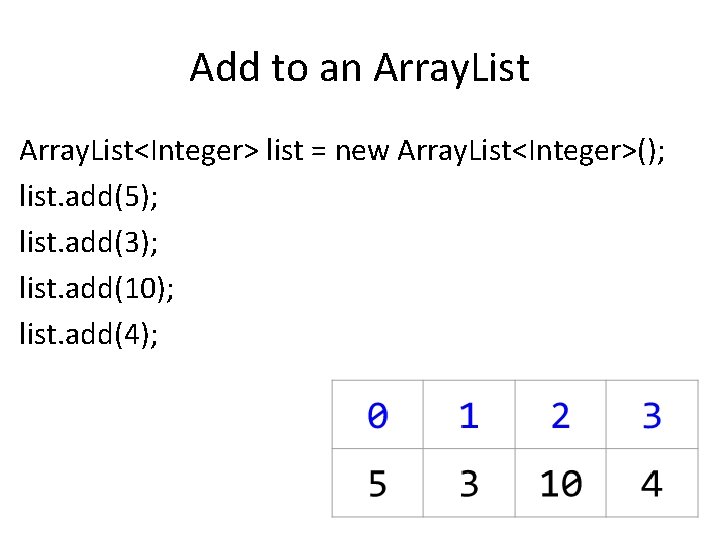
Add to an Array. List<Integer> list = new Array. List<Integer>(); list. add(5); list. add(3); list. add(10); list. add(4);
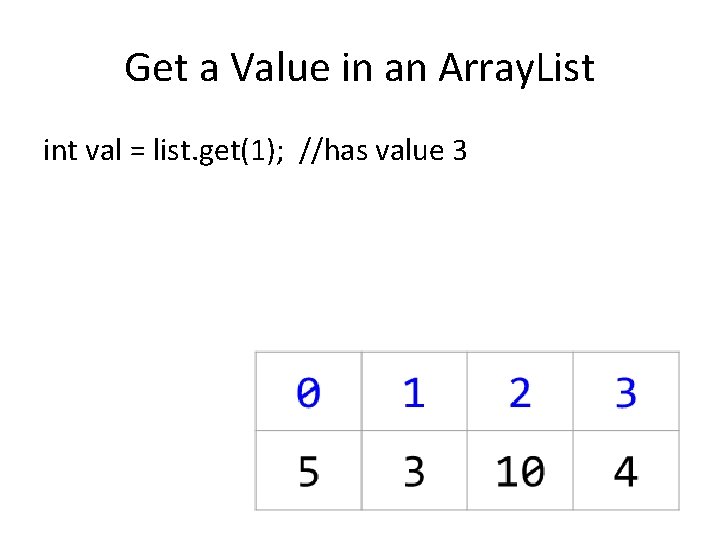
Get a Value in an Array. List int val = list. get(1); //has value 3
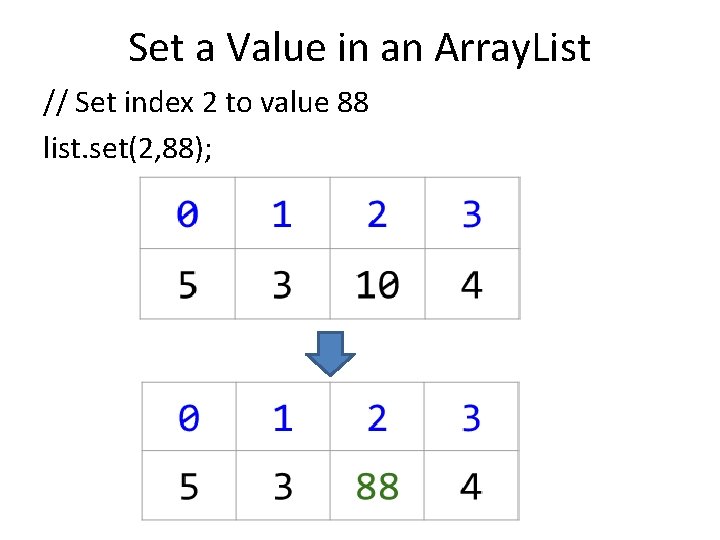
Set a Value in an Array. List // Set index 2 to value 88 list. set(2, 88);
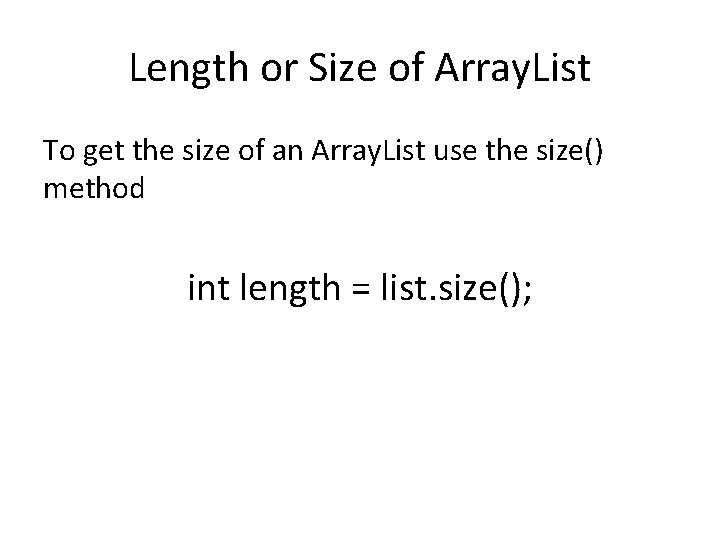
Length or Size of Array. List To get the size of an Array. List use the size() method int length = list. size();
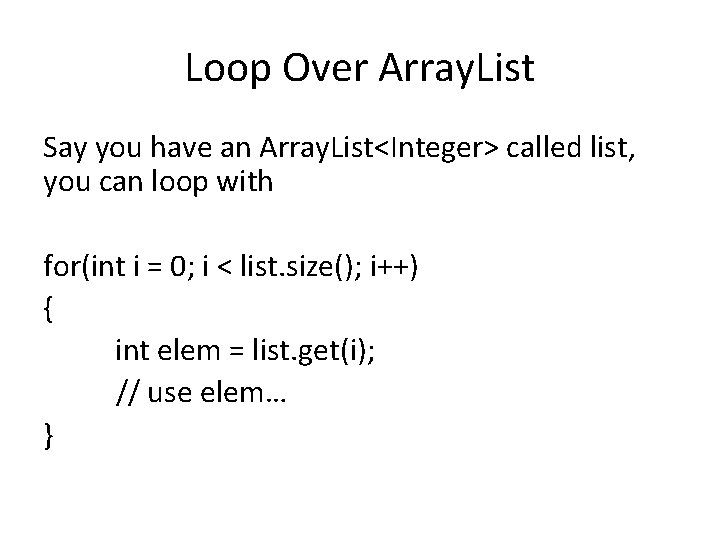
Loop Over Array. List Say you have an Array. List<Integer> called list, you can loop with for(int i = 0; i < list. size(); i++) { int elem = list. get(i); // use elem… }
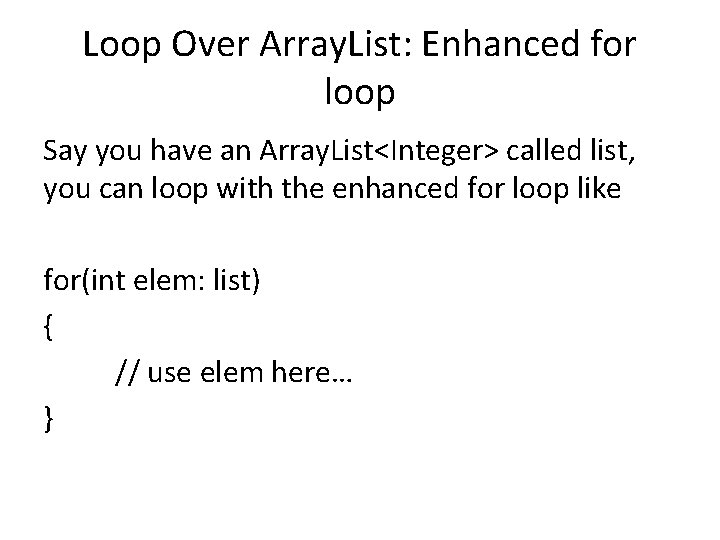
Loop Over Array. List: Enhanced for loop Say you have an Array. List<Integer> called list, you can loop with the enhanced for loop like for(int elem: list) { // use elem here… }
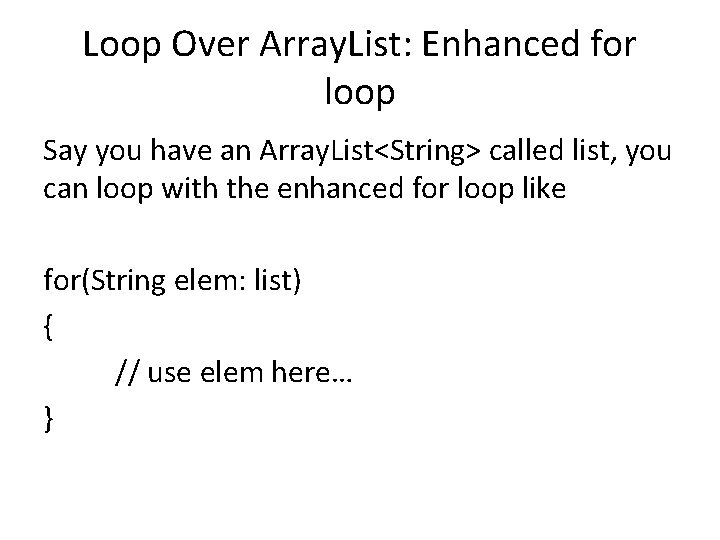
Loop Over Array. List: Enhanced for loop Say you have an Array. List<String> called list, you can loop with the enhanced for loop like for(String elem: list) { // use elem here… }
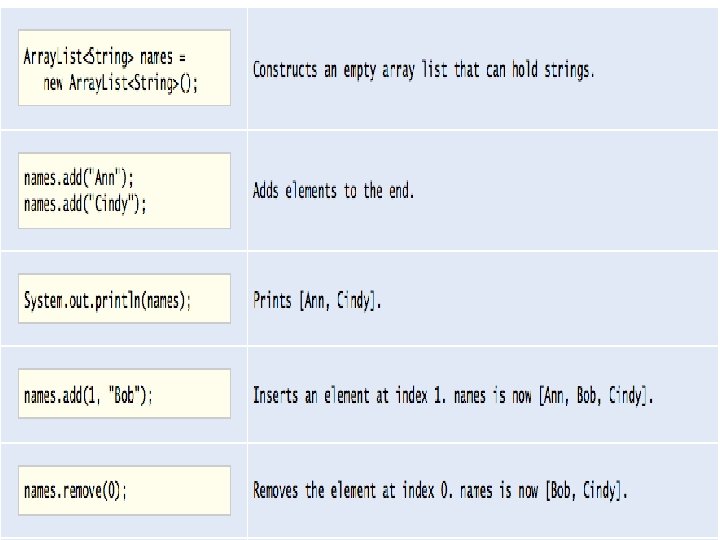
Array. List Quick Reference
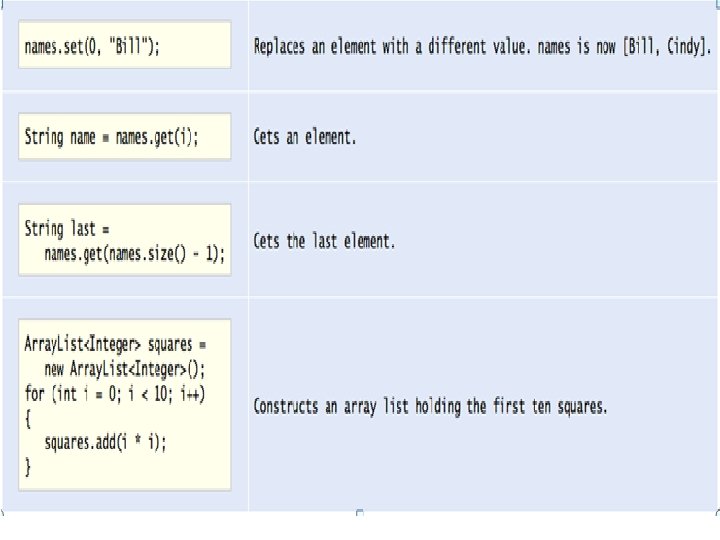
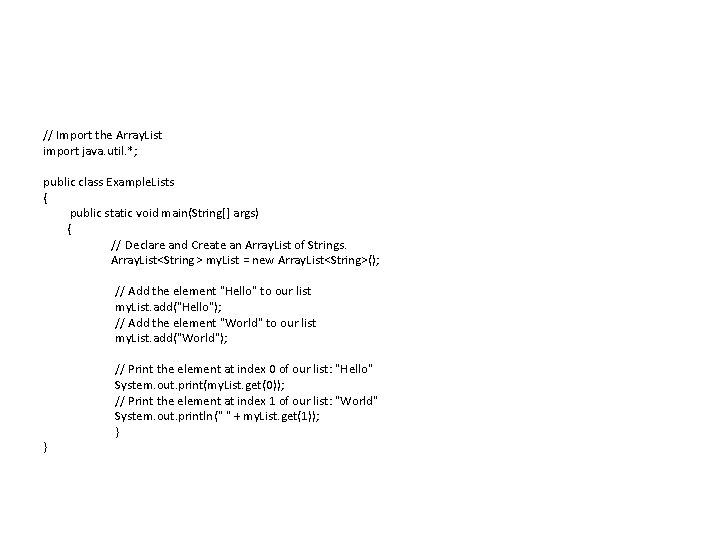
// Import the Array. List import java. util. *; public class Example. Lists { public static void main(String[] args) { // Declare and Create an Array. List of Strings. Array. List<String > my. List = new Array. List<String>(); // Add the element "Hello" to our list my. List. add("Hello"); // Add the element "World" to our list my. List. add("World"); } // Print the element at index 0 of our list: "Hello" System. out. print(my. List. get(0)); // Print the element at index 1 of our list: "World" System. out. println(" " + my. List. get(1)); }
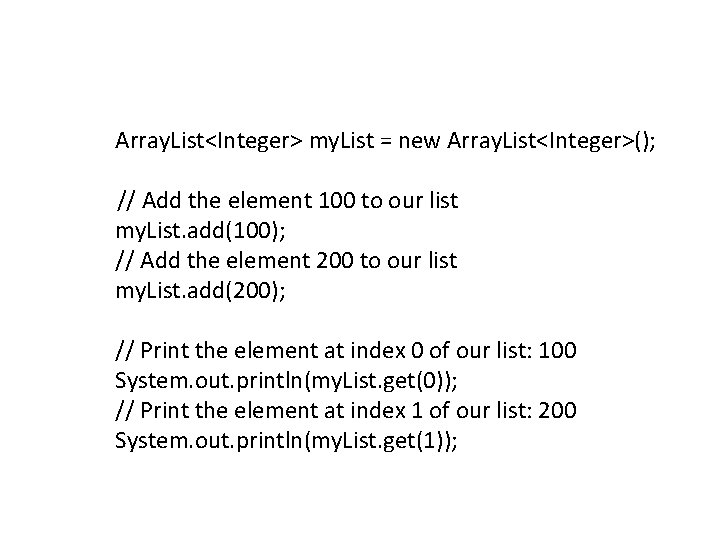
Array. List<Integer> my. List = new Array. List<Integer>(); // Add the element 100 to our list my. List. add(100); // Add the element 200 to our list my. List. add(200); // Print the element at index 0 of our list: 100 System. out. println(my. List. get(0)); // Print the element at index 1 of our list: 200 System. out. println(my. List. get(1));
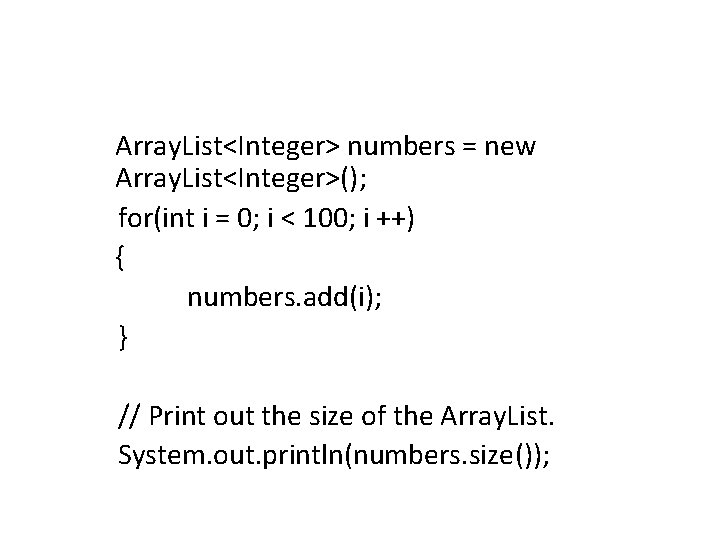
Array. List<Integer> numbers = new Array. List<Integer>(); for(int i = 0; i < 100; i ++) { numbers. add(i); } // Print out the size of the Array. List. System. out. println(numbers. size());
![public static void mainString args Array ListInteger numbers new Array ListInteger public static void main(String[] args) { Array. List<Integer> numbers = new Array. List<Integer>(); //](https://slidetodoc.com/presentation_image_h2/6fb8cc723c484592a3359864fc7af55e/image-19.jpg)
public static void main(String[] args) { Array. List<Integer> numbers = new Array. List<Integer>(); // Add 5 numbers to `numbers` numbers. add(1); numbers. add(2); numbers. add(3); numbers. add(4); numbers. add(5); numbers. add(3, 10); // Print out the first element in `numbers` System. out. println(numbers. get(0)); }
Array of arrays c++
Are vectors dynamic arrays
Small basic array
Polynomial representation using arrays
Arrays as adt
Veteork
Arrays visual basic
Searching and sorting arrays in c++
Arrays
Vectores unidimensionales ejemplos
Pascal 2d array
Assembly array of strings
Facts about arrays
C++ parallel arrays
Java partially filled array
Python find index of max
Day 3: arrays
Arreglo java
Disadvantages of static memory allocation
Dynamic array mips