21 Clang Tutorial CS 453 Automated Software Testing
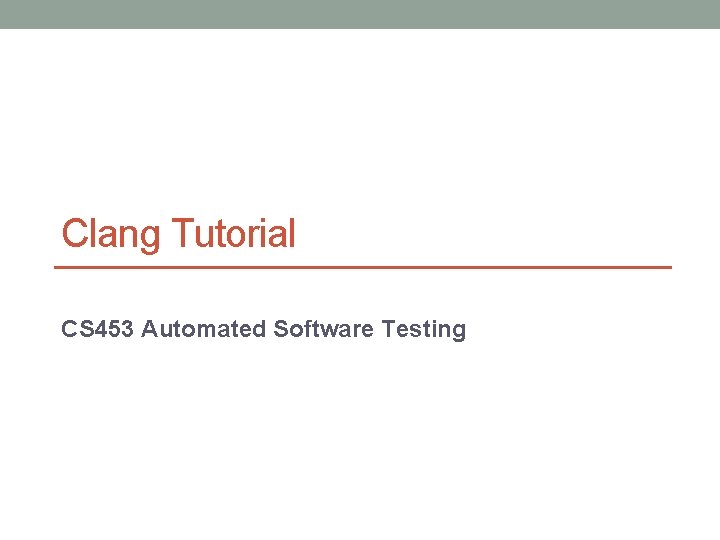
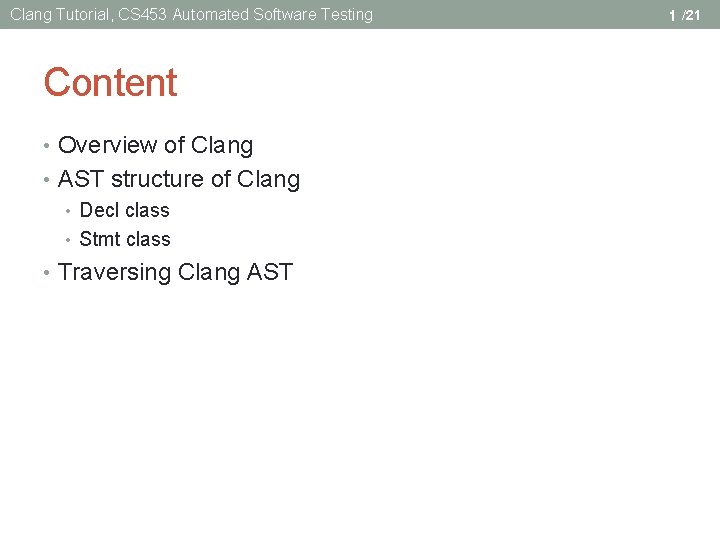
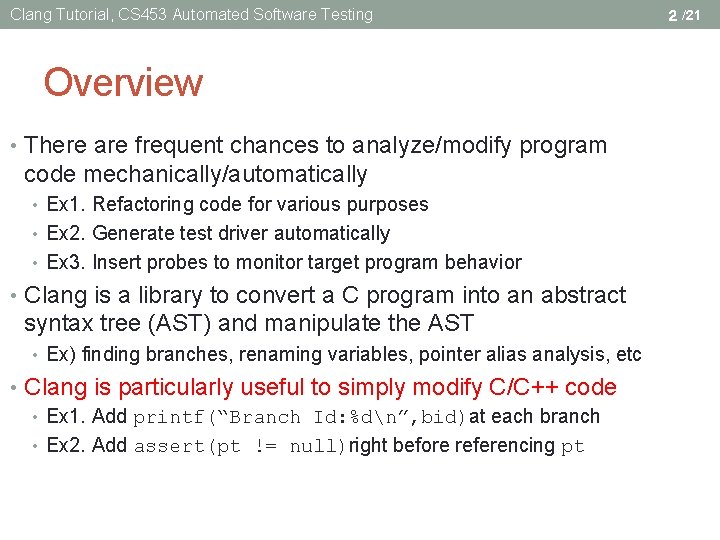
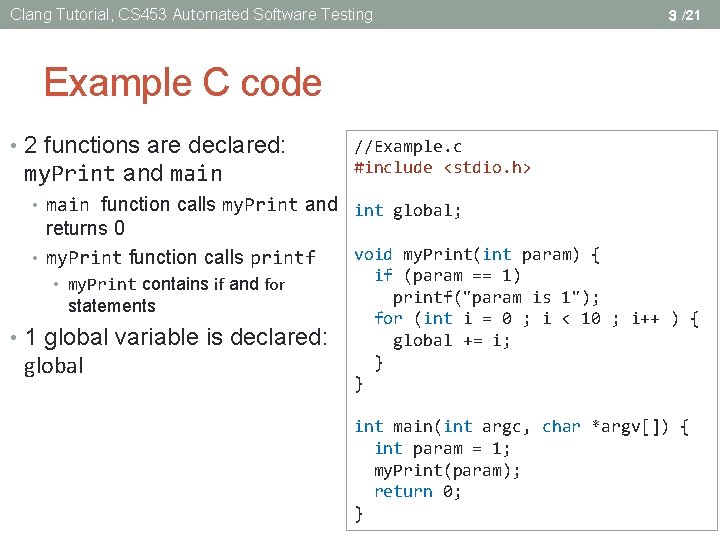
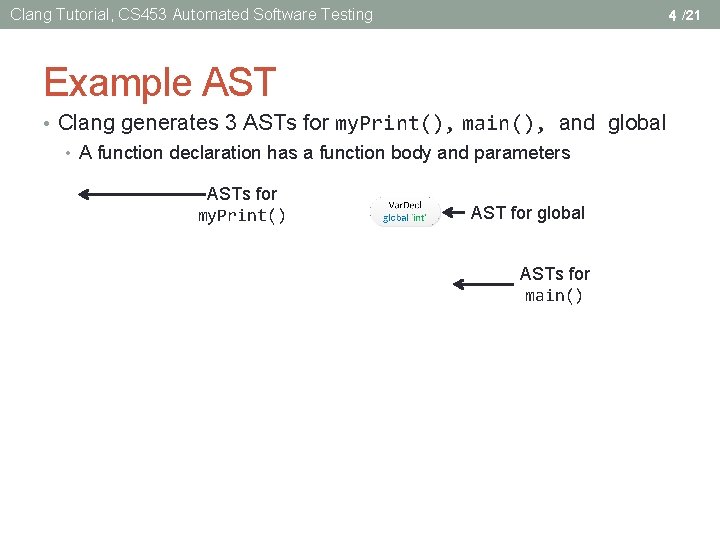
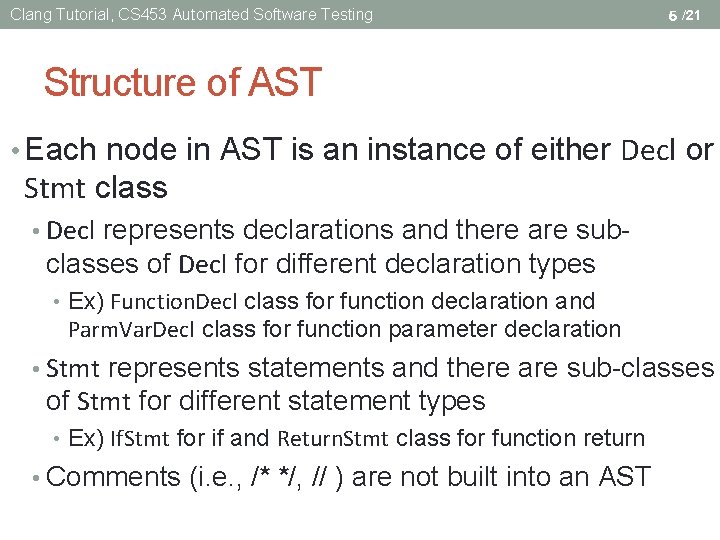
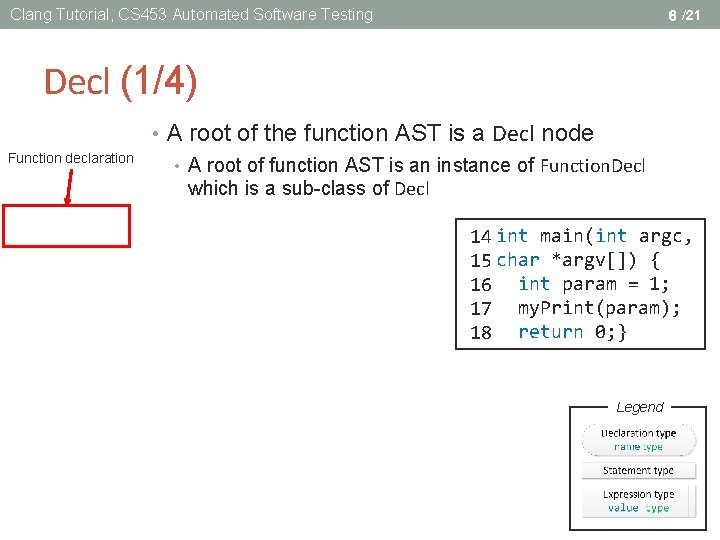
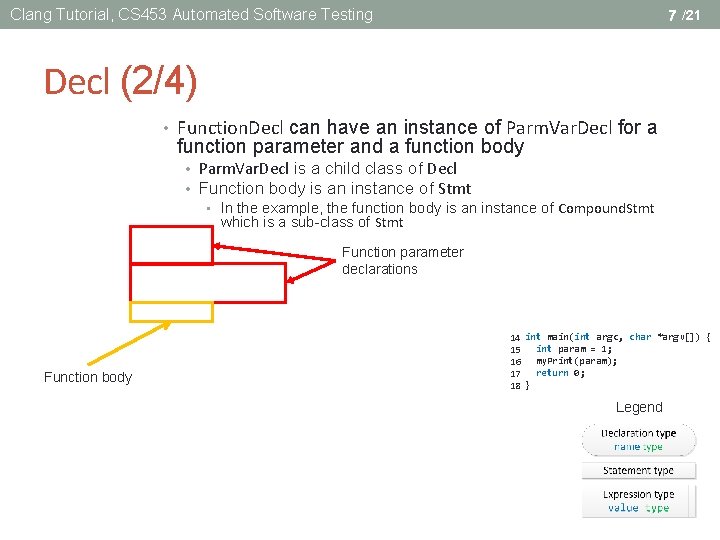
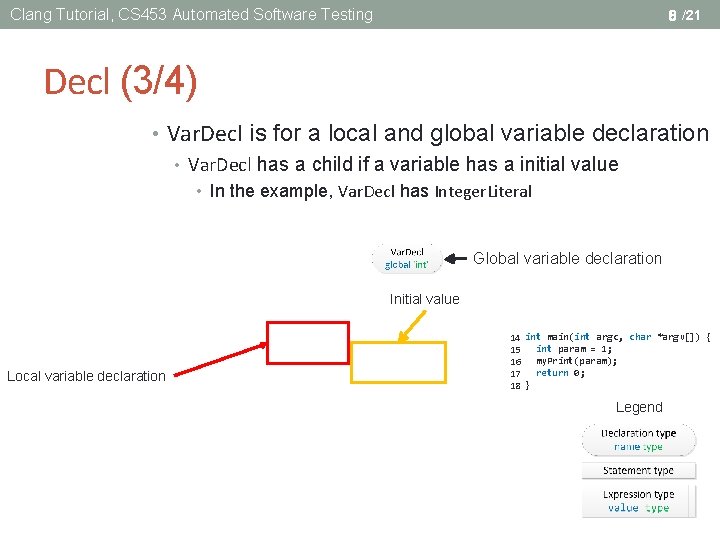
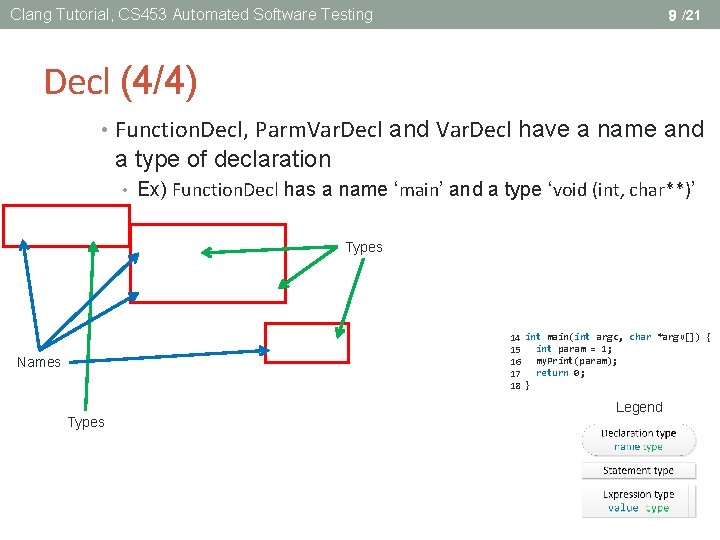
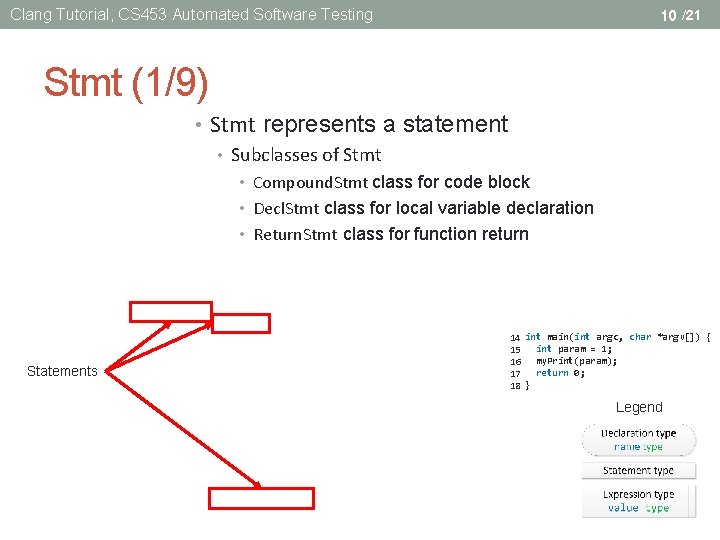
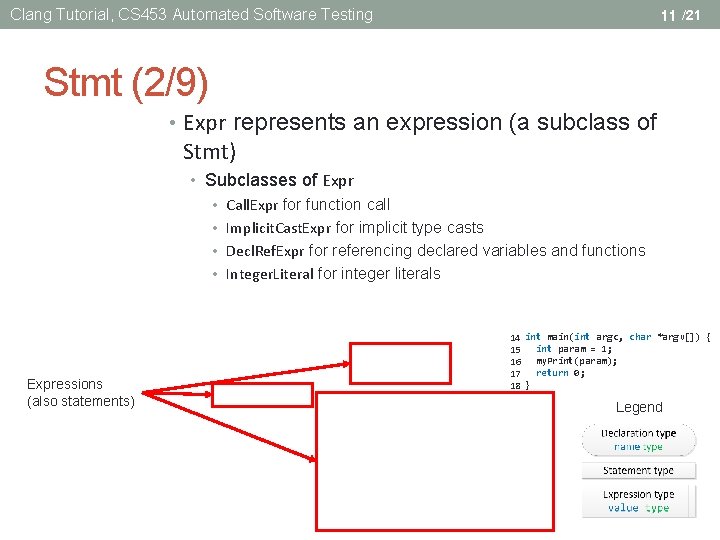
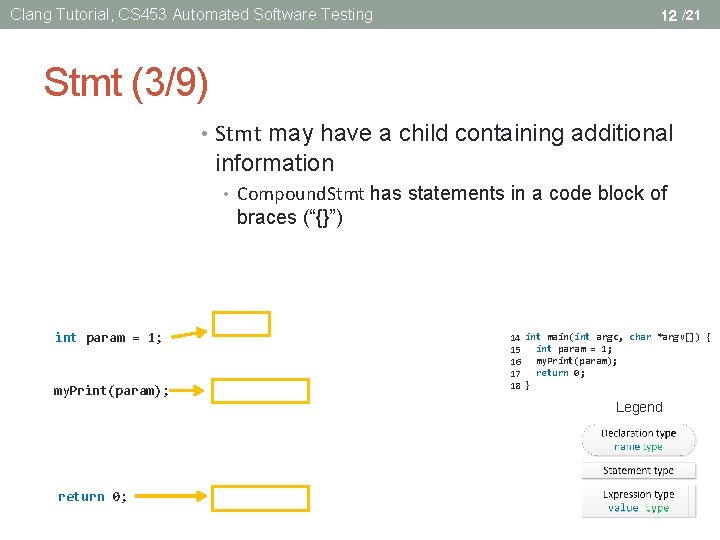
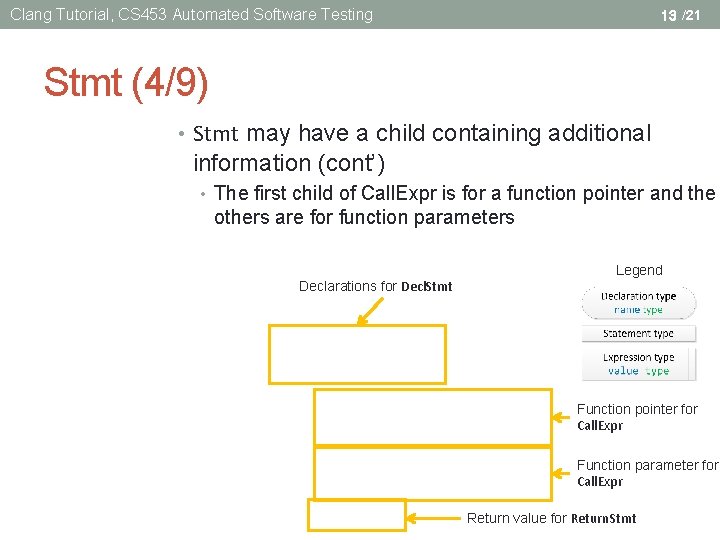
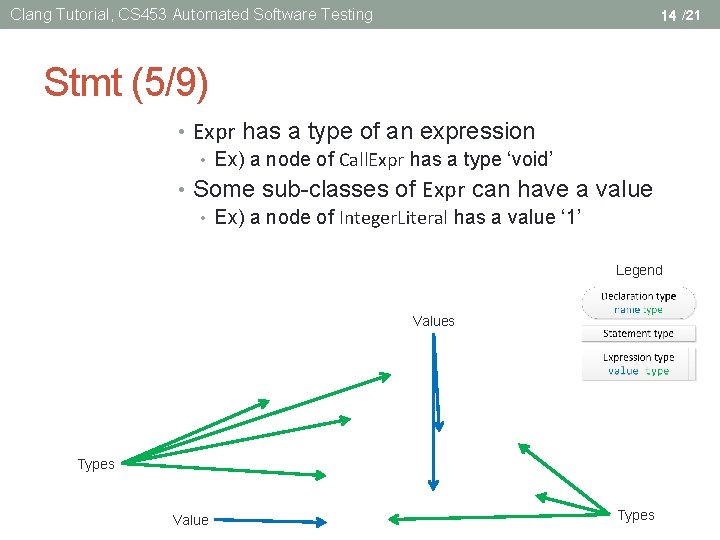
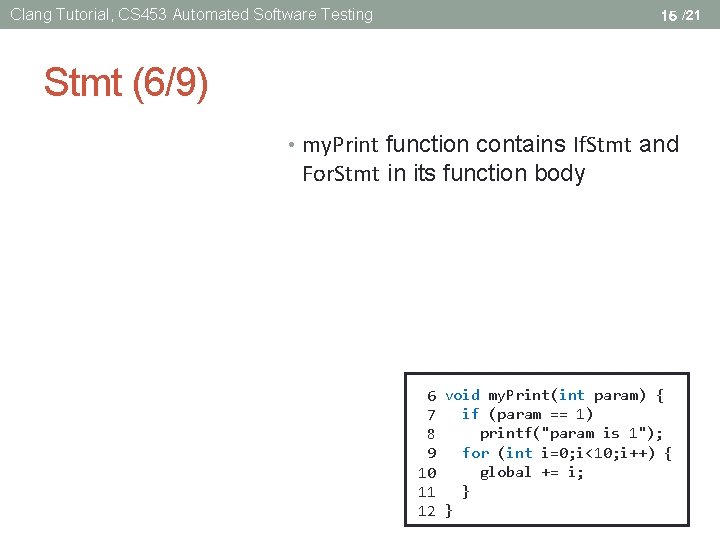
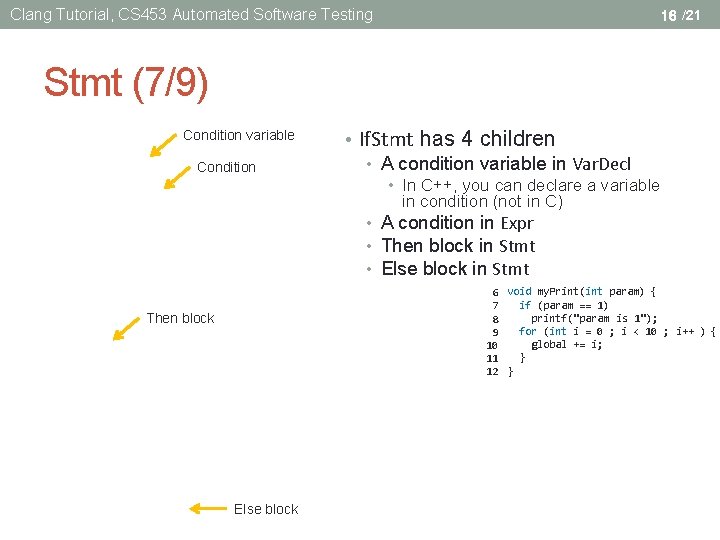
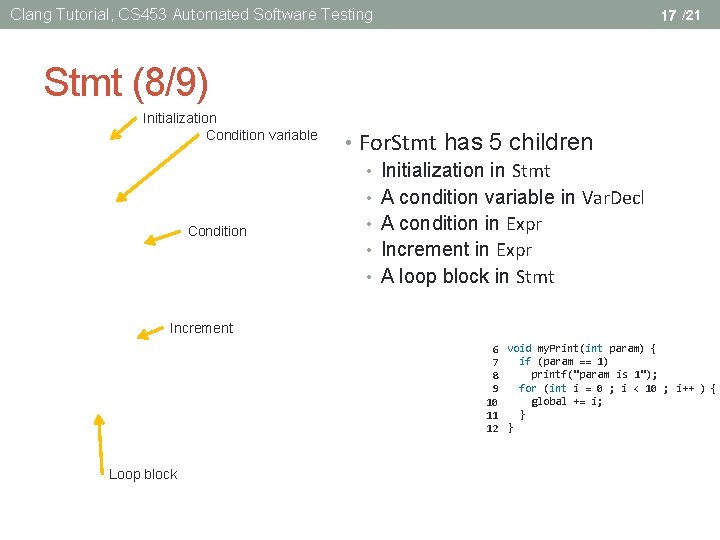
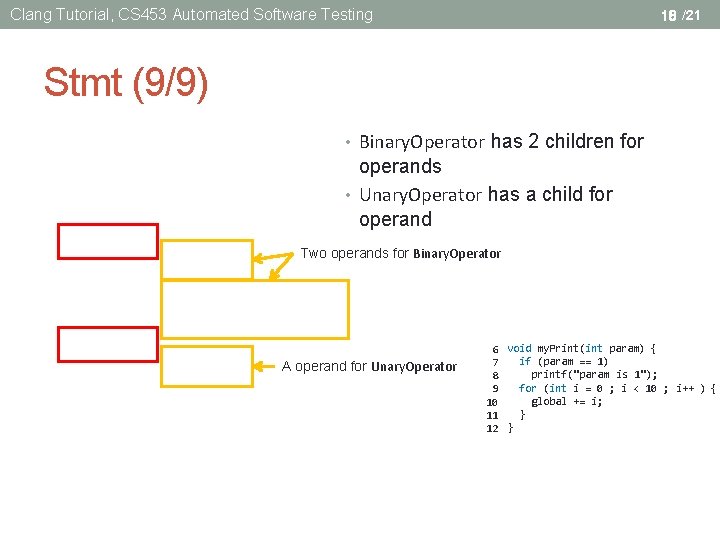
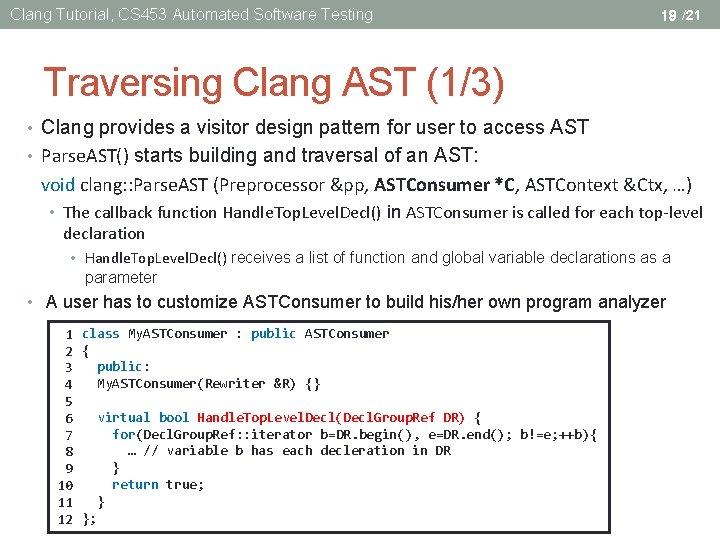
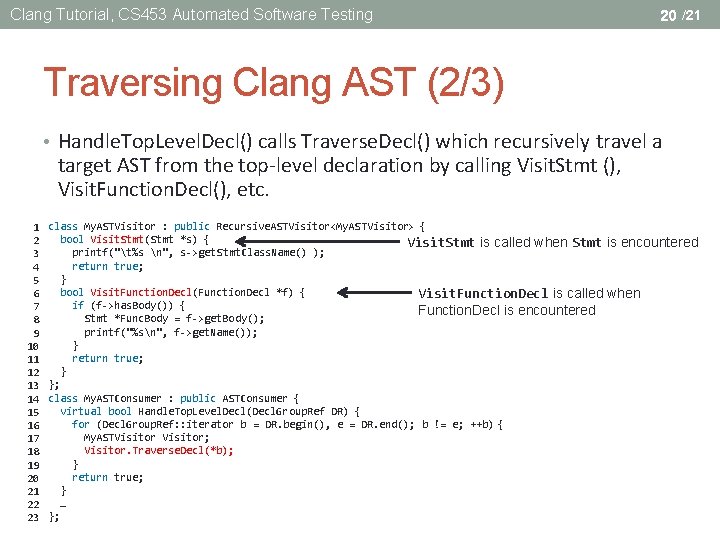
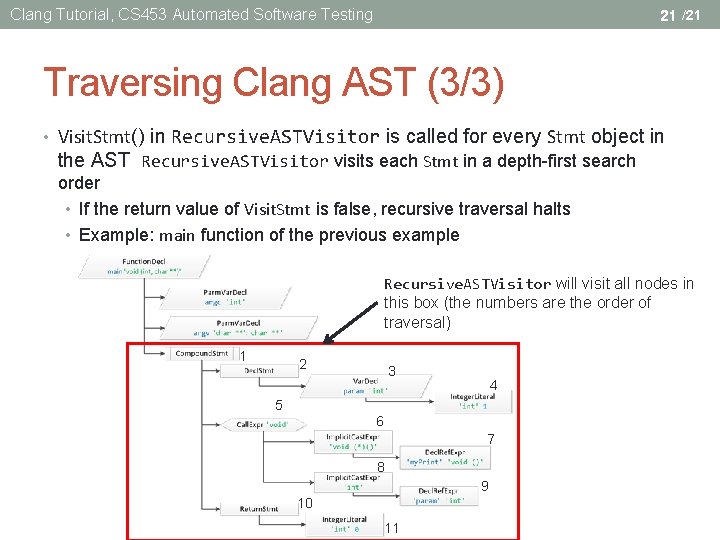
- Slides: 22
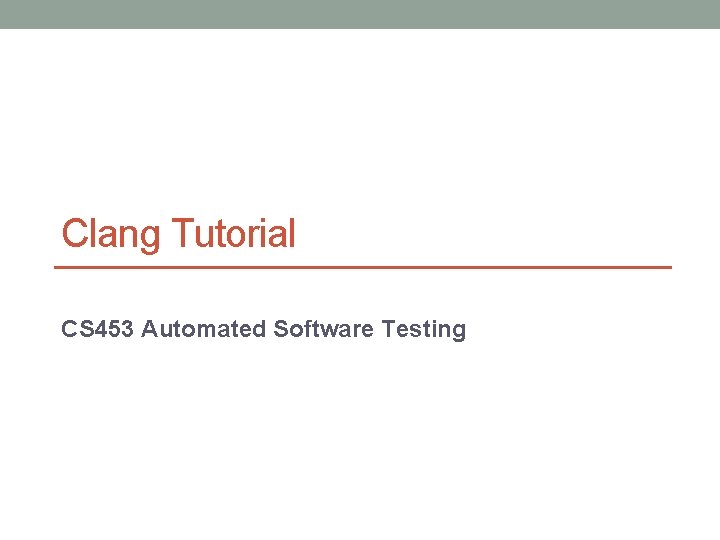
/21 Clang Tutorial CS 453 Automated Software Testing
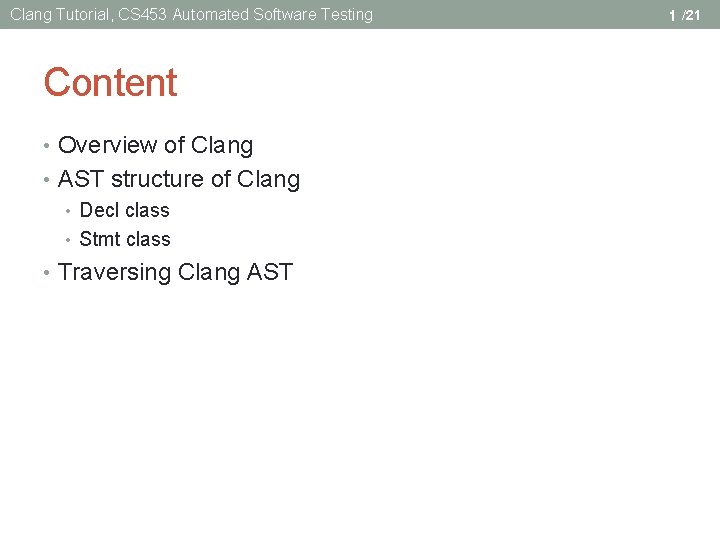
Clang Tutorial, CS 453 Automated Software Testing Content • Overview of Clang • AST structure of Clang • Decl class • Stmt class • Traversing Clang AST 1 /21
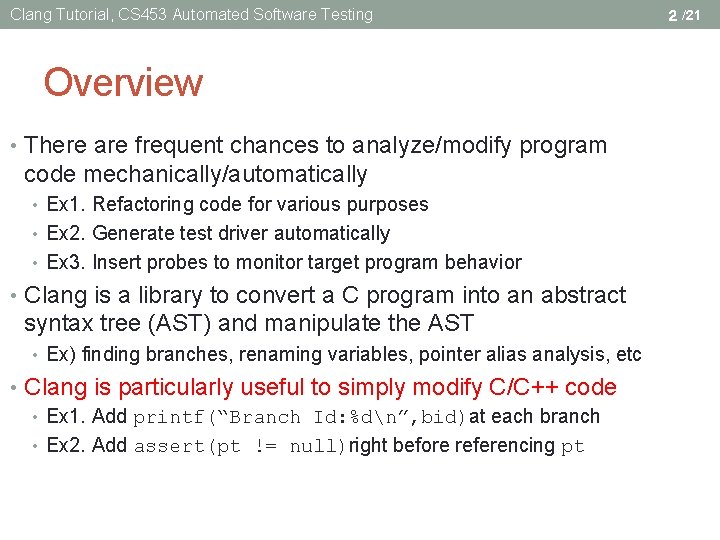
Clang Tutorial, CS 453 Automated Software Testing Overview • There are frequent chances to analyze/modify program code mechanically/automatically • Ex 1. Refactoring code for various purposes • Ex 2. Generate test driver automatically • Ex 3. Insert probes to monitor target program behavior • Clang is a library to convert a C program into an abstract syntax tree (AST) and manipulate the AST • Ex) finding branches, renaming variables, pointer alias analysis, etc • Clang is particularly useful to simply modify C/C++ code • Ex 1. Add printf(“Branch Id: %dn”, bid)at each branch • Ex 2. Add assert(pt != null)right before referencing pt 2 /21
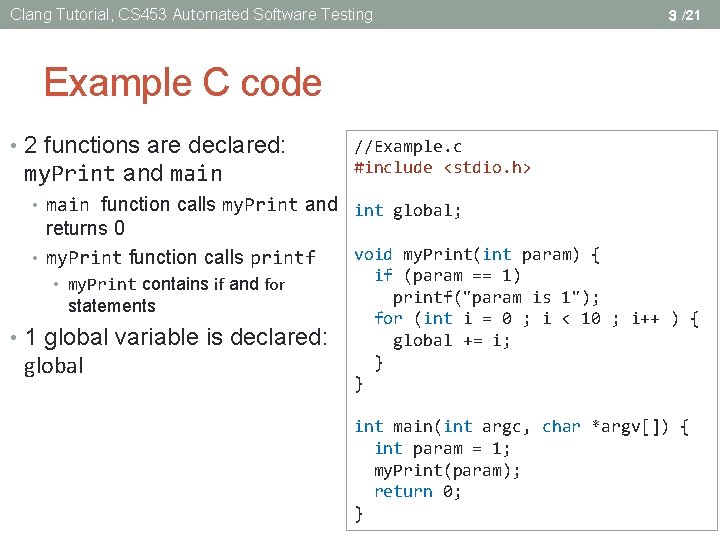
Clang Tutorial, CS 453 Automated Software Testing 3 /21 Example C code • 2 functions are declared: my. Print and main //Example. c #include <stdio. h> • main function calls my. Print and int global; returns 0 • my. Print function calls printf • my. Print contains if and for statements • 1 global variable is declared: global void my. Print(int param) { if (param == 1) printf("param is 1"); for (int i = 0 ; i < 10 ; i++ ) { global += i; } } int main(int argc, char *argv[]) { int param = 1; my. Print(param); return 0; }
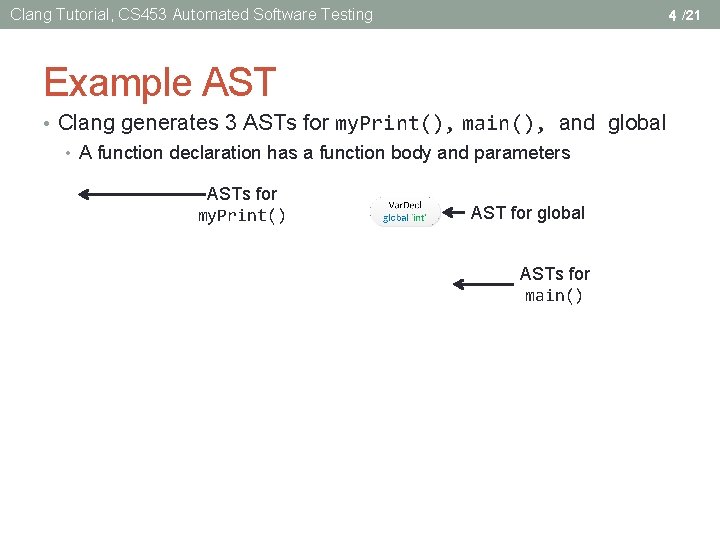
Clang Tutorial, CS 453 Automated Software Testing 4 /21 Example AST • Clang generates 3 ASTs for my. Print(), main(), and global • A function declaration has a function body and parameters ASTs for my. Print() AST for global ASTs for main()
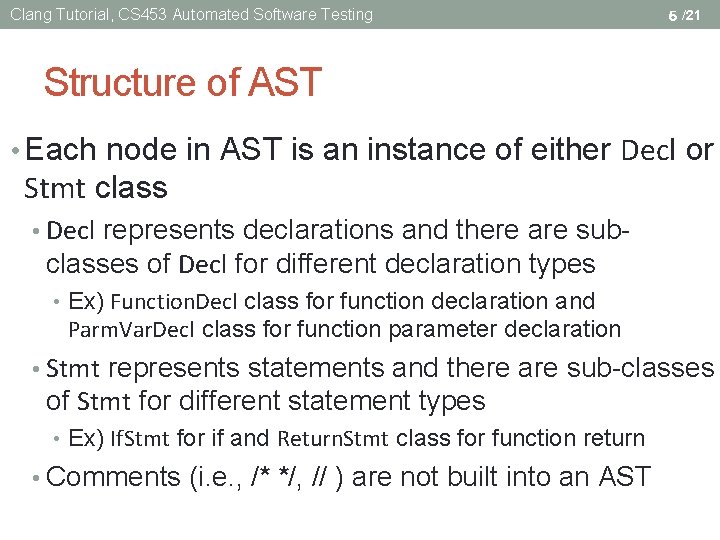
Clang Tutorial, CS 453 Automated Software Testing 5 /21 Structure of AST • Each node in AST is an instance of either Decl or Stmt class • Decl represents declarations and there are sub- classes of Decl for different declaration types • Ex) Function. Decl class for function declaration and Parm. Var. Decl class for function parameter declaration • Stmt represents statements and there are sub-classes of Stmt for different statement types • Ex) If. Stmt for if and Return. Stmt class for function return • Comments (i. e. , /* */, // ) are not built into an AST
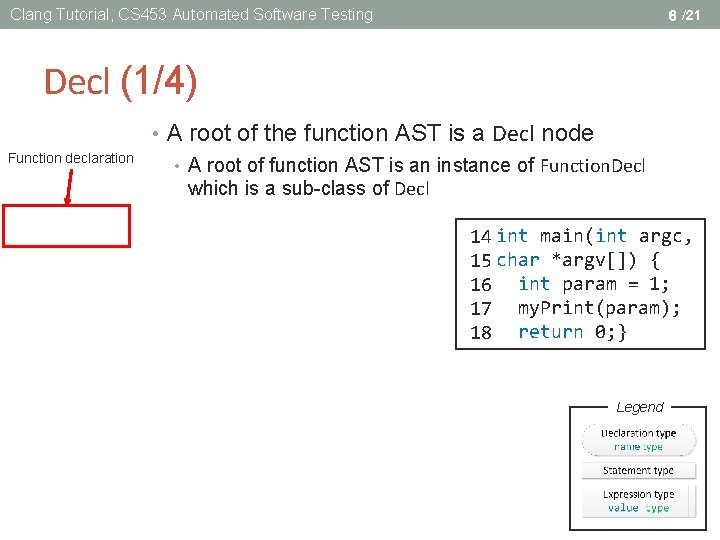
Clang Tutorial, CS 453 Automated Software Testing 6 /21 Decl (1/4) Function declaration • A root of the function AST is a Decl node • A root of function AST is an instance of Function. Decl which is a sub-class of Decl 14 int main(int argc, 15 char *argv[]) { 16 int param = 1; 17 my. Print(param); 18 return 0; } Legend
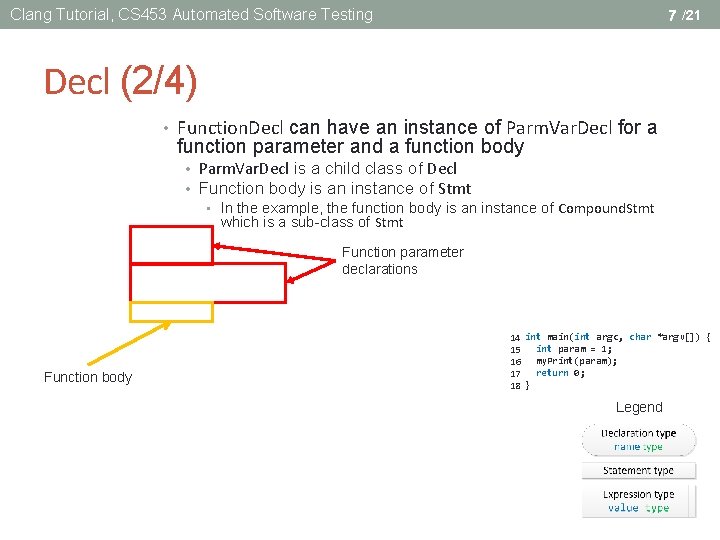
Clang Tutorial, CS 453 Automated Software Testing 7 /21 Decl (2/4) • Function. Decl can have an instance of Parm. Var. Decl for a function parameter and a function body • Parm. Var. Decl is a child class of Decl • Function body is an instance of Stmt • In the example, the function body is an instance of Compound. Stmt which is a sub-class of Stmt Function parameter declarations Function body 14 int main(int argc, char *argv[]) { 15 int param = 1; 16 my. Print(param); 17 return 0; 18 } Legend
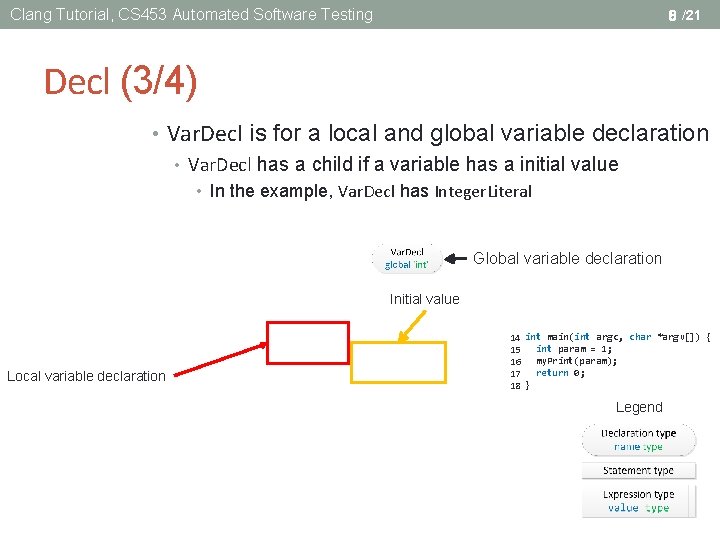
Clang Tutorial, CS 453 Automated Software Testing 8 /21 Decl (3/4) • Var. Decl is for a local and global variable declaration • Var. Decl has a child if a variable has a initial value • In the example, Var. Decl has Integer. Literal Global variable declaration Initial value Local variable declaration 14 int main(int argc, char *argv[]) { 15 int param = 1; 16 my. Print(param); 17 return 0; 18 } Legend
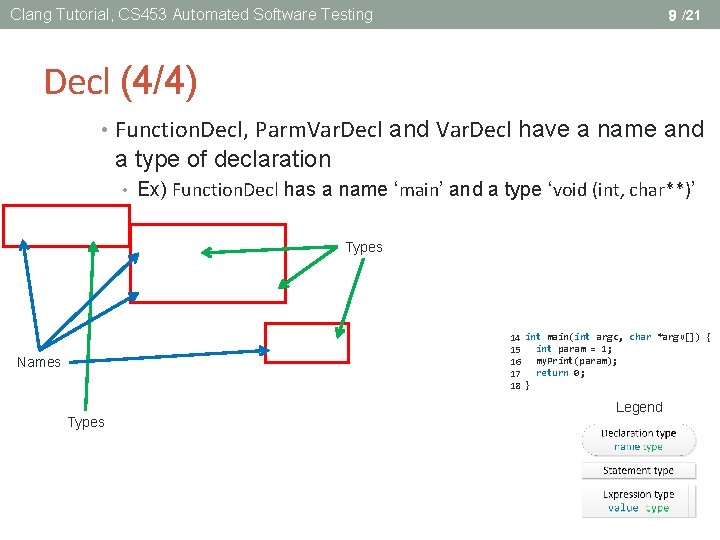
Clang Tutorial, CS 453 Automated Software Testing 9 /21 Decl (4/4) • Function. Decl, Parm. Var. Decl and Var. Decl have a name and a type of declaration • Ex) Function. Decl has a name ‘main’ and a type ‘void (int, char**)’ Types 14 int main(int argc, char *argv[]) { 15 int param = 1; 16 my. Print(param); 17 return 0; 18 } Names Types Legend
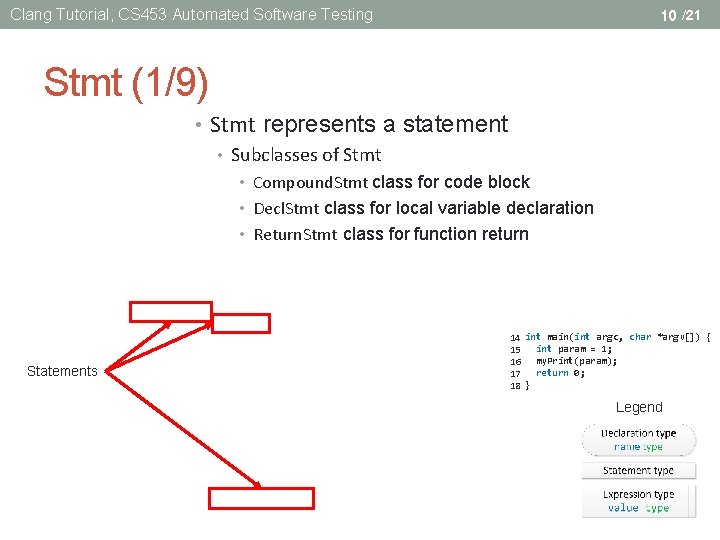
Clang Tutorial, CS 453 Automated Software Testing 10 /21 Stmt (1/9) • Stmt represents a statement • Subclasses of Stmt • Compound. Stmt class for code block • Decl. Stmt class for local variable declaration • Return. Stmt class for function return Statements 14 int main(int argc, char *argv[]) { 15 int param = 1; 16 my. Print(param); 17 return 0; 18 } Legend
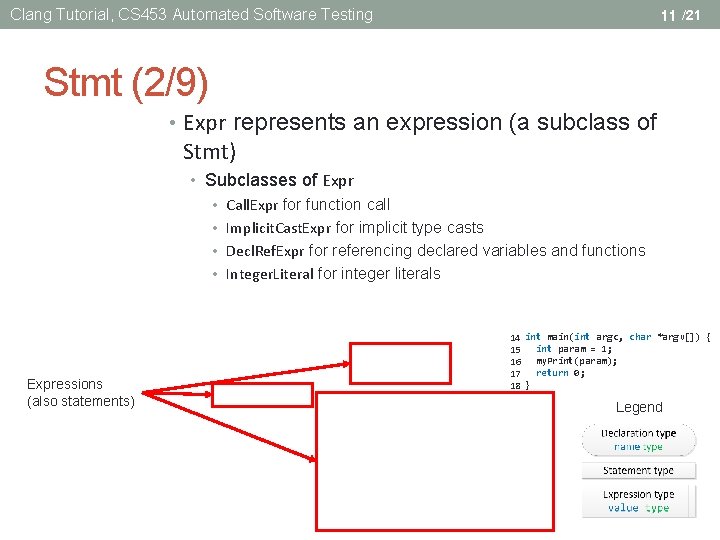
Clang Tutorial, CS 453 Automated Software Testing 11 /21 Stmt (2/9) • Expr represents an expression (a subclass of Stmt) • Subclasses of Expr • Call. Expr for function call • Implicit. Cast. Expr for implicit type casts • Decl. Ref. Expr for referencing declared variables and functions • Integer. Literal for integer literals Expressions (also statements) 14 int main(int argc, char *argv[]) { 15 int param = 1; 16 my. Print(param); 17 return 0; 18 } Legend
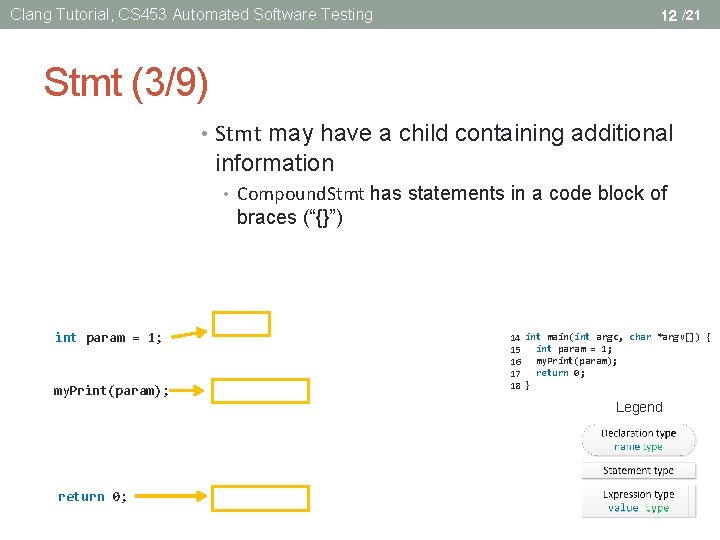
Clang Tutorial, CS 453 Automated Software Testing 12 /21 Stmt (3/9) • Stmt may have a child containing additional information • Compound. Stmt has statements in a code block of braces (“{}”) int param = 1; my. Print(param); 14 int main(int argc, char *argv[]) { 15 int param = 1; 16 my. Print(param); 17 return 0; 18 } Legend return 0;
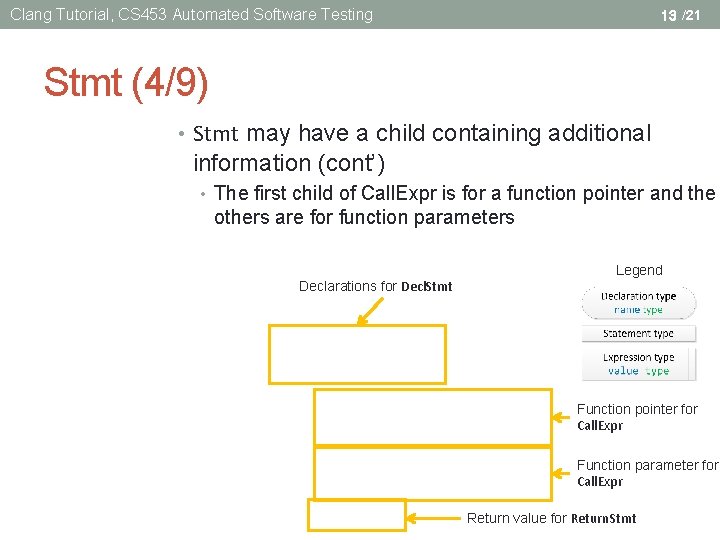
Clang Tutorial, CS 453 Automated Software Testing 13 /21 Stmt (4/9) • Stmt may have a child containing additional information (cont’) • The first child of Call. Expr is for a function pointer and the others are for function parameters Declarations for Decl. Stmt Legend Function pointer for Call. Expr Function parameter for Call. Expr Return value for Return. Stmt
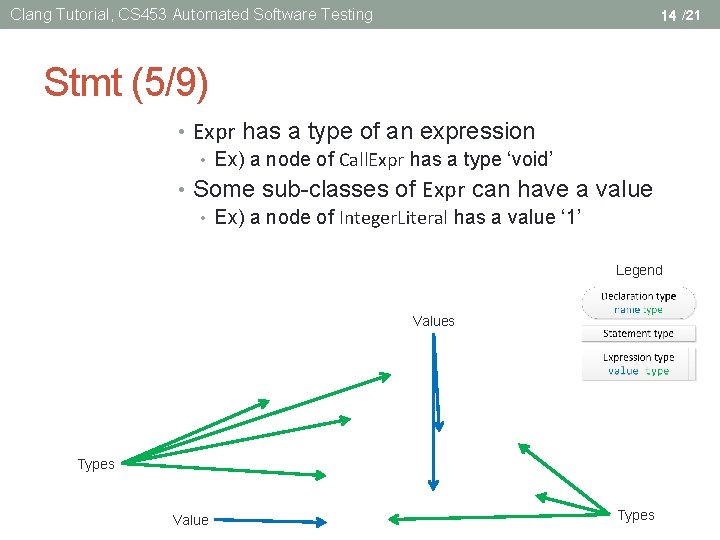
Clang Tutorial, CS 453 Automated Software Testing 14 /21 Stmt (5/9) • Expr has a type of an expression • Ex) a node of Call. Expr has a type ‘void’ • Some sub-classes of Expr can have a value • Ex) a node of Integer. Literal has a value ‘ 1’ Legend Values Types Value Types
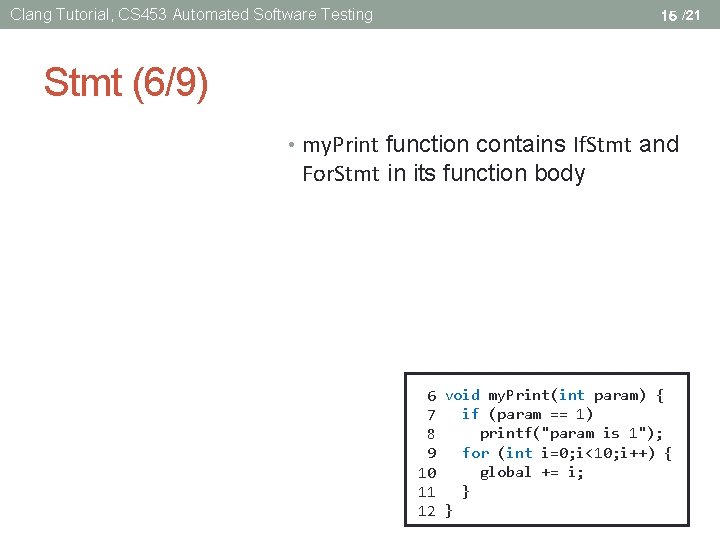
Clang Tutorial, CS 453 Automated Software Testing 15 /21 Stmt (6/9) • my. Print function contains If. Stmt and For. Stmt in its function body 6 void my. Print(int param) { if (param == 1) 7 printf("param is 1"); 8 for (int i=0; i<10; i++) { 9 global += i; 10 } 11 12 }
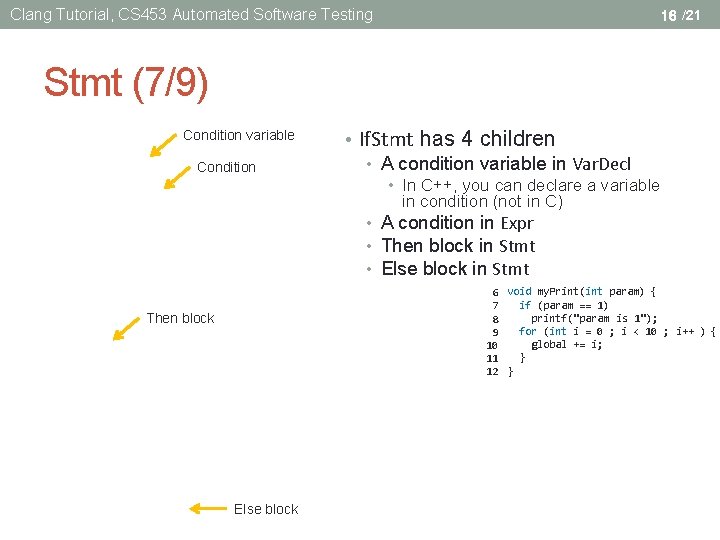
Clang Tutorial, CS 453 Automated Software Testing 16 /21 Stmt (7/9) Condition variable Condition • If. Stmt has 4 children • A condition variable in Var. Decl • In C++, you can declare a variable in condition (not in C) • A condition in Expr • Then block in Stmt • Else block in Stmt 6 void my. Print(int param) { if (param == 1) 7 printf("param is 1"); 8 for (int i = 0 ; i < 10 ; i++ ) { 9 global += i; 10 } 11 12 } Then block Else block
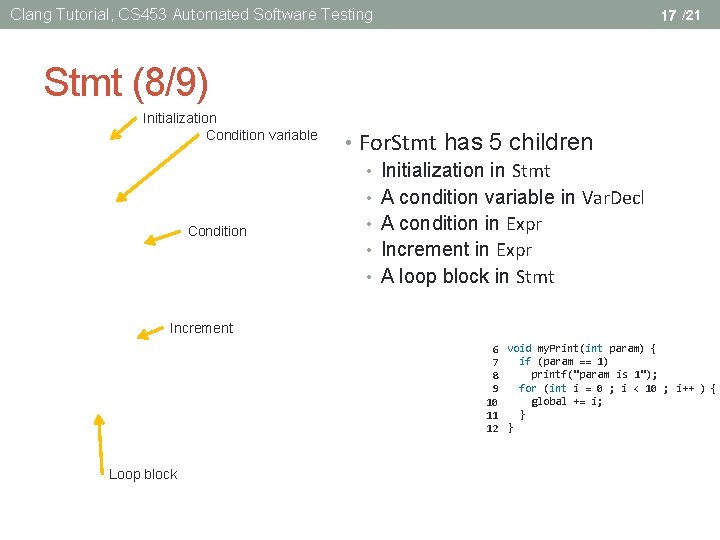
Clang Tutorial, CS 453 Automated Software Testing 17 /21 Stmt (8/9) Initialization Condition variable Condition • For. Stmt has 5 children • Initialization in Stmt • A condition variable in Var. Decl • A condition in Expr • Increment in Expr • A loop block in Stmt Increment 6 void my. Print(int param) { if (param == 1) 7 printf("param is 1"); 8 for (int i = 0 ; i < 10 ; i++ ) { 9 global += i; 10 } 11 12 } Loop block
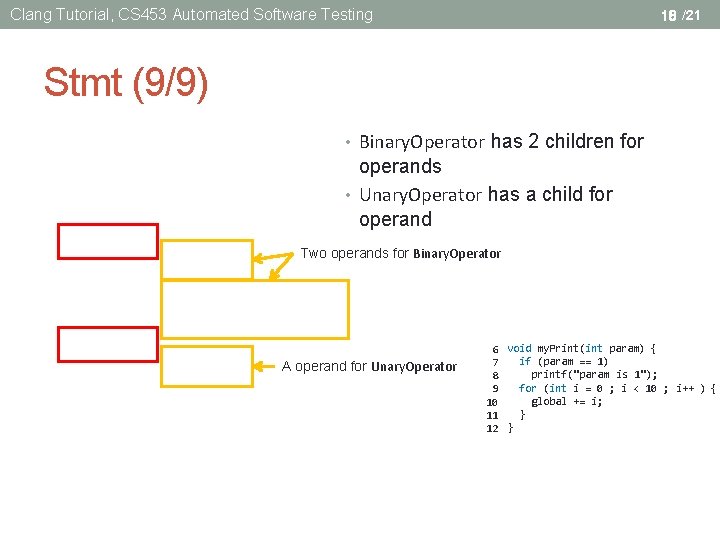
Clang Tutorial, CS 453 Automated Software Testing 18 /21 Stmt (9/9) • Binary. Operator has 2 children for operands • Unary. Operator has a child for operand Two operands for Binary. Operator A operand for Unary. Operator 6 void my. Print(int param) { if (param == 1) 7 printf("param is 1"); 8 for (int i = 0 ; i < 10 ; i++ ) { 9 global += i; 10 } 11 12 }
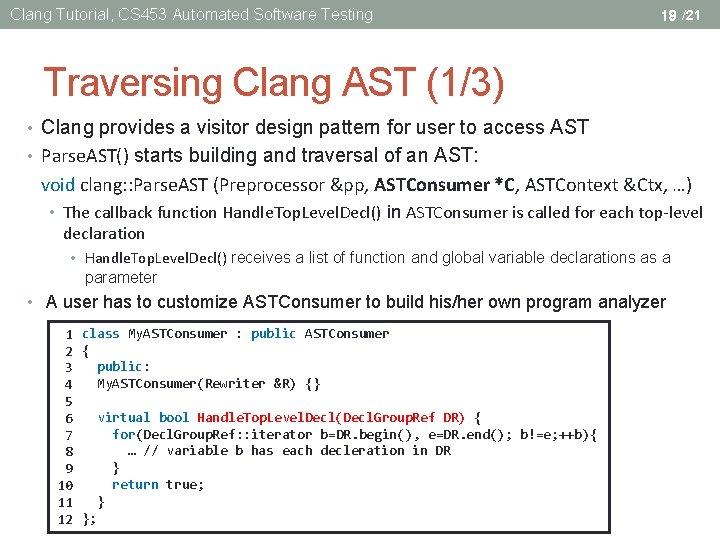
Clang Tutorial, CS 453 Automated Software Testing 19 /21 Traversing Clang AST (1/3) • Clang provides a visitor design pattern for user to access AST • Parse. AST() starts building and traversal of an AST: void clang: : Parse. AST (Preprocessor &pp, ASTConsumer *C, ASTContext &Ctx, …) • The callback function Handle. Top. Level. Decl() in ASTConsumer is called for each top-level declaration • Handle. Top. Level. Decl() receives a list of function and global variable declarations as a parameter • A user has to customize ASTConsumer to build his/her own program analyzer 1 class My. ASTConsumer : public ASTConsumer 2 { public: 3 My. ASTConsumer(Rewriter &R) {} 4 5 virtual bool Handle. Top. Level. Decl(Decl. Group. Ref DR) { 6 for(Decl. Group. Ref: : iterator b=DR. begin(), e=DR. end(); b!=e; ++b){ 7 … // variable b has each decleration in DR 8 } 9 return true; 10 } 11 12 };
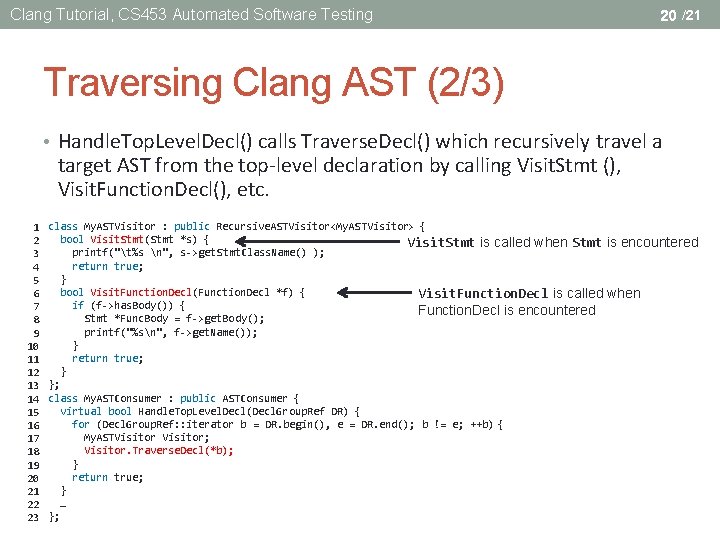
Clang Tutorial, CS 453 Automated Software Testing 20 /21 Traversing Clang AST (2/3) • Handle. Top. Level. Decl() calls Traverse. Decl() which recursively travel a target AST from the top-level declaration by calling Visit. Stmt (), Visit. Function. Decl(), etc. 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 class My. ASTVisitor : public Recursive. ASTVisitor<My. ASTVisitor> { bool Visit. Stmt(Stmt *s) { Visit. Stmt is called when Stmt is encountered printf("t%s n", s->get. Stmt. Class. Name() ); return true; } bool Visit. Function. Decl(Function. Decl *f) { Visit. Function. Decl is called when if (f->has. Body()) { Function. Decl is encountered Stmt *Func. Body = f->get. Body(); printf("%sn", f->get. Name()); } return true; } }; class My. ASTConsumer : public ASTConsumer { virtual bool Handle. Top. Level. Decl(Decl. Group. Ref DR) { for (Decl. Group. Ref: : iterator b = DR. begin(), e = DR. end(); b != e; ++b) { My. ASTVisitor; Visitor. Traverse. Decl(*b); } return true; } … };
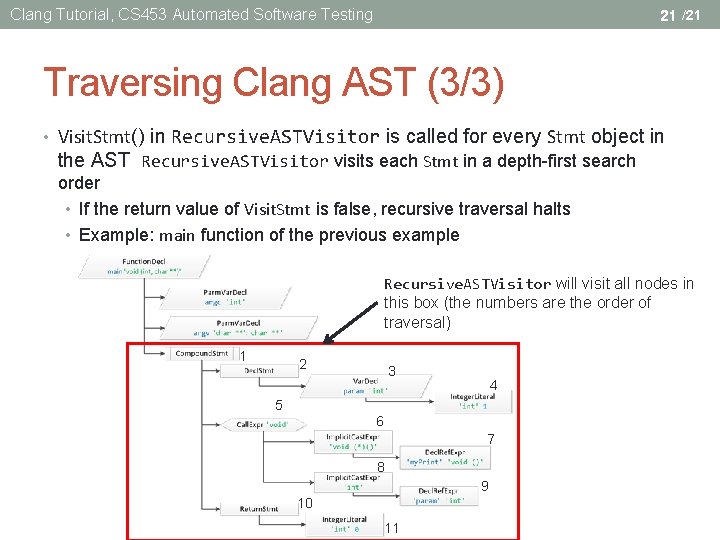
Clang Tutorial, CS 453 Automated Software Testing 21 /21 Traversing Clang AST (3/3) • Visit. Stmt() in Recursive. ASTVisitor is called for every Stmt object in the AST Recursive. ASTVisitor visits each Stmt in a depth-first search order • If the return value of Visit. Stmt is false, recursive traversal halts • Example: main function of the previous example Recursive. ASTVisitor will visit all nodes in this box (the numbers are the order of traversal) 1 2 5 3 4 6 7 8 9 10 11
Cs 453
Cs 453
Onomatopoeia definition poetry
David poliakoff
Build linux kernel with clang
Clang wasm
Domain test means
Motivational overview of logic based testing
Du path testing
Globalization testing in software testing
Decision table testing in software testing
Control structure testing in software testing
Decision table testing in software testing
What is decision table testing
Decision table based testing in software testing
Rigorous testing in software testing
Testing blindness in software testing
Domain testing in software testing
Directed automated random testing
Sitecore test automation
Creating an automated testing framework with selenium
Which process requires automated builds and testing
Sap solution manager automated testing