Week 1 Learn to program simple Java algos
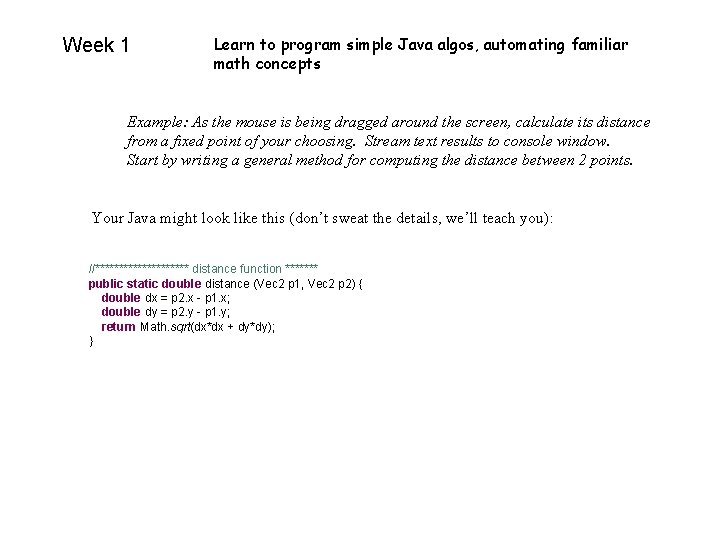
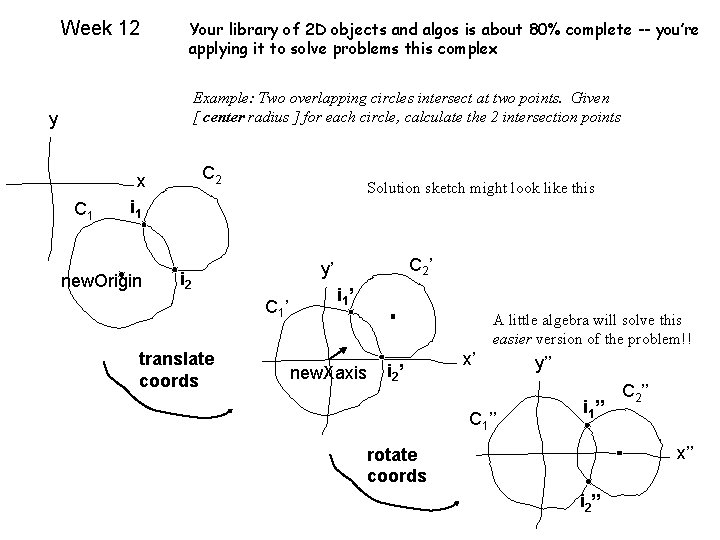
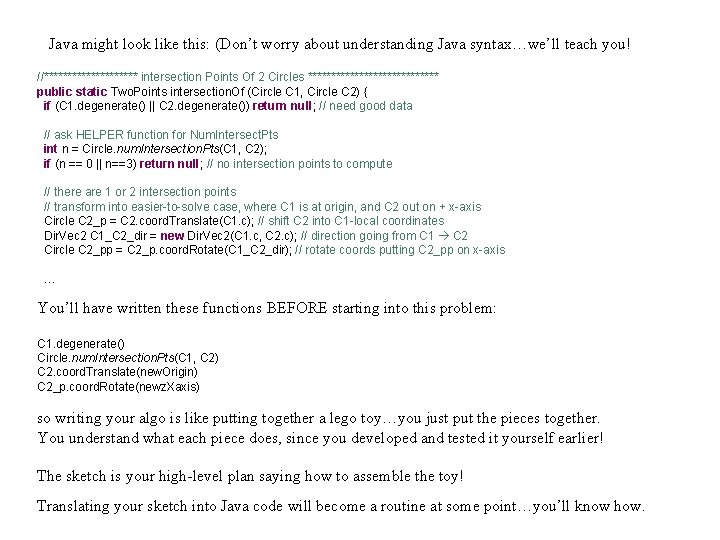
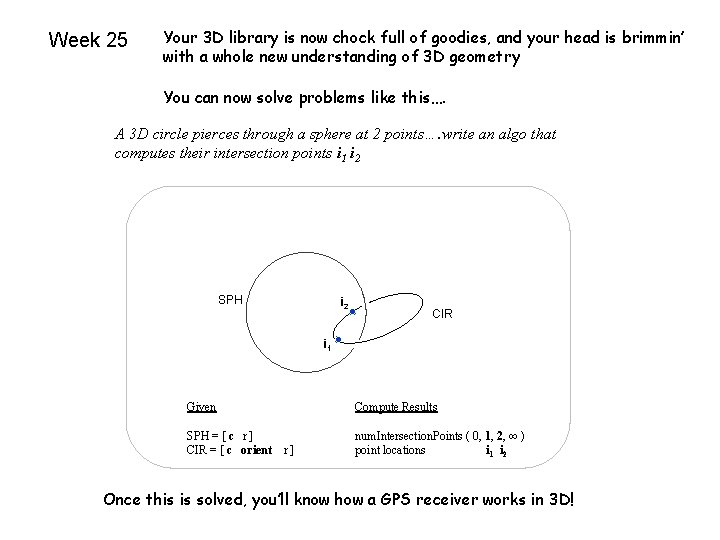
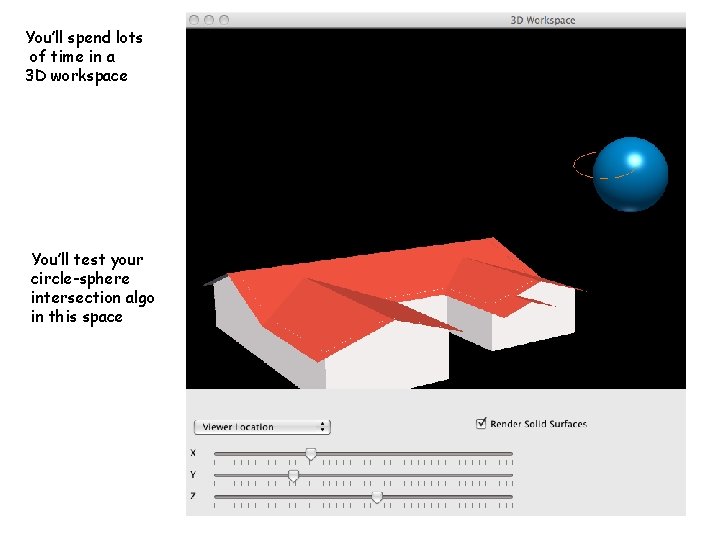
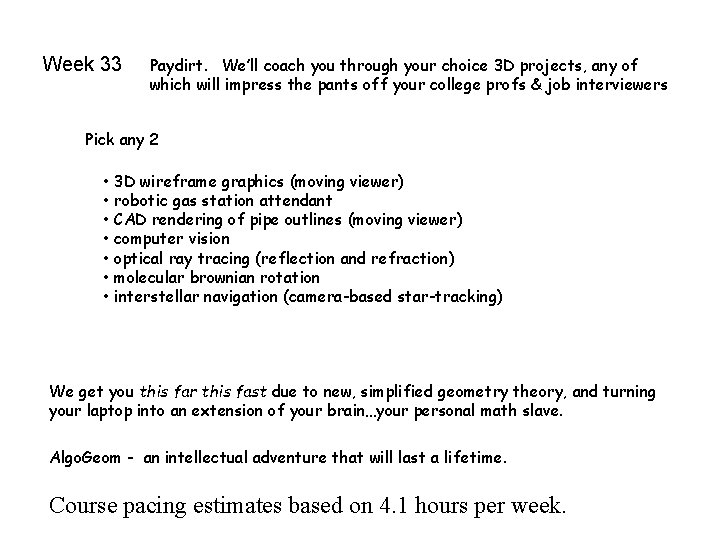
- Slides: 6
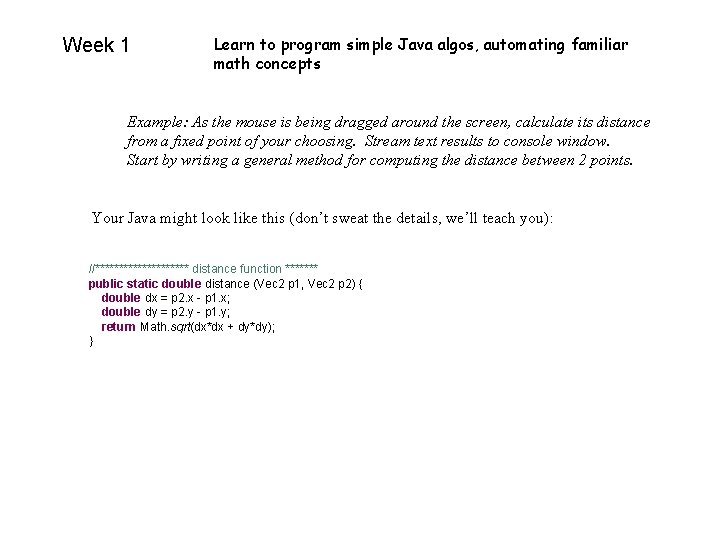
Week 1 Learn to program simple Java algos, automating familiar math concepts Example: As the mouse is being dragged around the screen, calculate its distance from a fixed point of your choosing. Stream text results to console window. Start by writing a general method for computing the distance between 2 points. Your Java might look like this (don’t sweat the details, we’ll teach you): //********** distance function ******* public static double distance (Vec 2 p 1, Vec 2 p 2) { double dx = p 2. x - p 1. x; double dy = p 2. y - p 1. y; return Math. sqrt(dx*dx + dy*dy); }
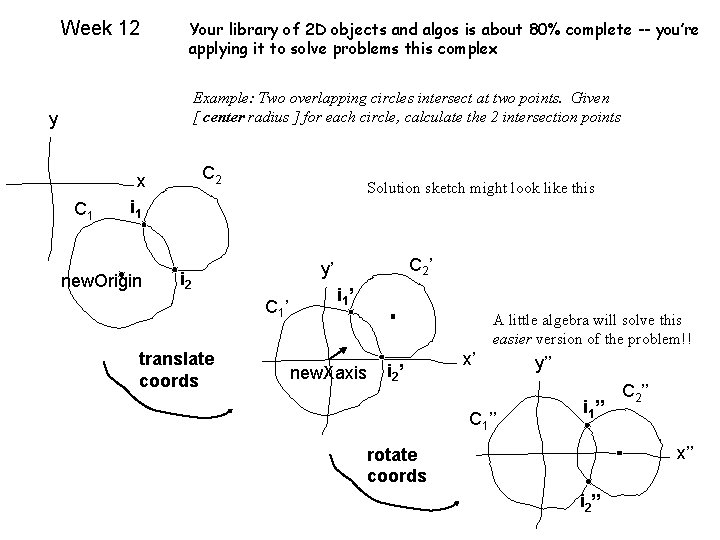
Week 12 Your library of 2 D objects and algos is about 80% complete -- you’re applying it to solve problems this complex Example: Two overlapping circles intersect at two points. Given [ center radius ] for each circle, calculate the 2 intersection points y C 2 x C 1 Solution sketch might look like this i 1 new. Origin i 2 C 1 ’ translate coords C 2 ’ y’ i 1 ’ A little algebra will solve this easier version of the problem!! new. Xaxis i 2 ’ x’ C 1’’ y’’ i 1’’ C 2’’ x’’ rotate coords i 2’’
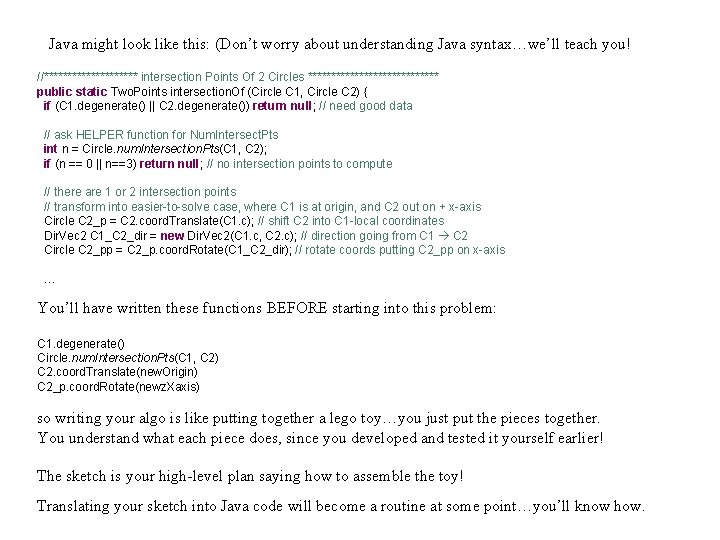
Java might look like this: (Don’t worry about understanding Java syntax…we’ll teach you! //********** intersection Points Of 2 Circles ************** public static Two. Points intersection. Of (Circle C 1, Circle C 2) { if (C 1. degenerate() || C 2. degenerate()) return null; // need good data // ask HELPER function for Num. Intersect. Pts int n = Circle. num. Intersection. Pts(C 1, C 2); if (n == 0 || n==3) return null; // no intersection points to compute // there are 1 or 2 intersection points // transform into easier-to-solve case, where C 1 is at origin, and C 2 out on + x-axis Circle C 2_p = C 2. coord. Translate(C 1. c); // shift C 2 into C 1 -local coordinates Dir. Vec 2 C 1_C 2_dir = new Dir. Vec 2(C 1. c, C 2. c); // direction going from C 1 C 2 Circle C 2_pp = C 2_p. coord. Rotate(C 1_C 2_dir); // rotate coords putting C 2_pp on x-axis … You’ll have written these functions BEFORE starting into this problem: C 1. degenerate() Circle. num. Intersection. Pts(C 1, C 2) C 2. coord. Translate(new. Origin) C 2_p. coord. Rotate(newz. Xaxis) so writing your algo is like putting together a lego toy…you just put the pieces together. You understand what each piece does, since you developed and tested it yourself earlier! The sketch is your high-level plan saying how to assemble the toy! Translating your sketch into Java code will become a routine at some point…you’ll know how.
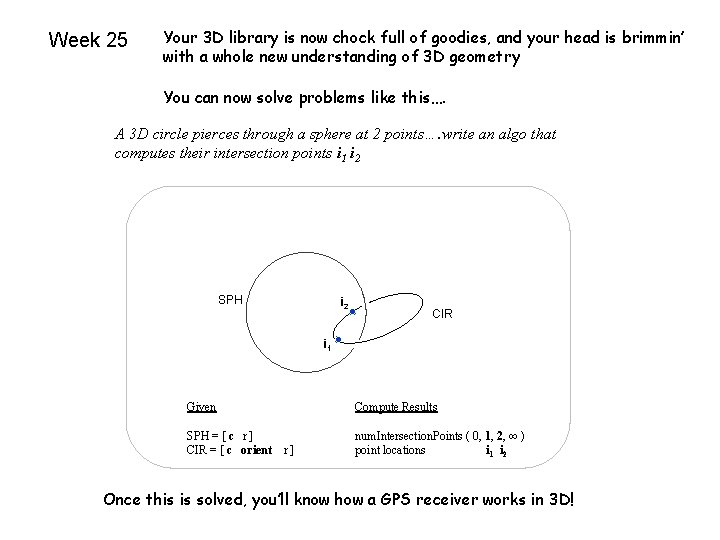
Week 25 Your 3 D library is now chock full of goodies, and your head is brimmin’ with a whole new understanding of 3 D geometry You can now solve problems like this…. A 3 D circle pierces through a sphere at 2 points…. write an algo that computes their intersection points i 1 i 2 SPH i 2 CIR i 1 Given Compute Results SPH = [ c r ] CIR = [ c orient num. Intersection. Points ( 0, 1, 2, ∞ ) point locations i 1 i 2 r] Once this is solved, you’ll know how a GPS receiver works in 3 D!
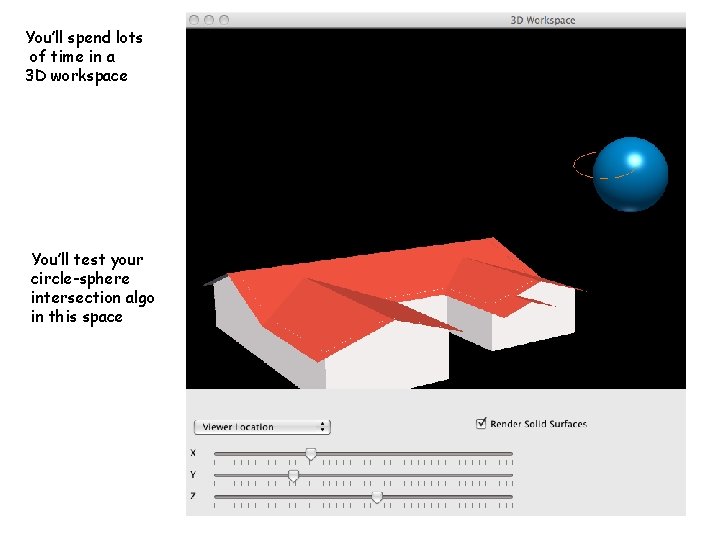
You’ll spend lots of time in a 3 D workspace You’ll test your circle-sphere intersection algo in this space
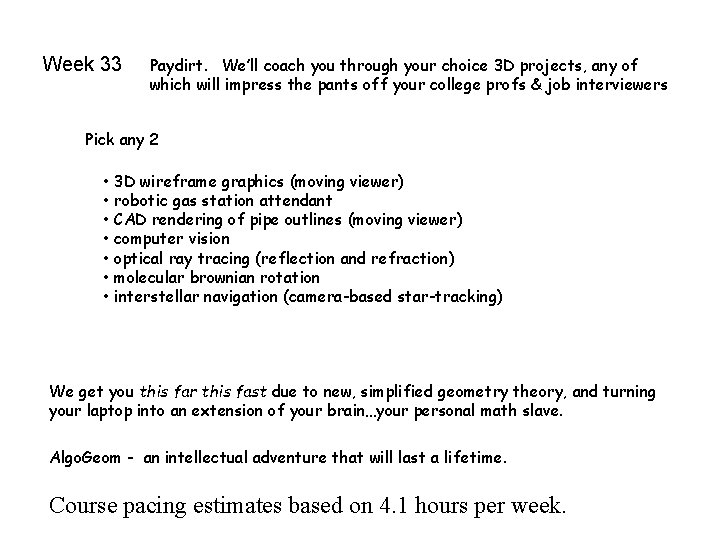
Week 33 Paydirt. We’ll coach you through your choice 3 D projects, any of which will impress the pants off your college profs & job interviewers Pick any 2 • 3 D wireframe graphics (moving viewer) • robotic gas station attendant • CAD rendering of pipe outlines (moving viewer) • computer vision • optical ray tracing (reflection and refraction) • molecular brownian rotation • interstellar navigation (camera-based star-tracking) We get you this far this fast due to new, simplified geometry theory, and turning your laptop into an extension of your brain…your personal math slave. Algo. Geom - an intellectual adventure that will last a lifetime. Course pacing estimates based on 4. 1 hours per week.