RUNTIME MEMORY MANAGEMENT Keyword new int foo foo
![RUNTIME MEMORY MANAGEMENT // Keyword new int * foo; foo = new int [5]; RUNTIME MEMORY MANAGEMENT // Keyword new int * foo; foo = new int [5];](https://slidetodoc.com/presentation_image_h2/c47d7352b238d2860b5d5569695efc47/image-1.jpg)
![RUNTIME MEMORY MANAGEMENT // Keyword new int * foo; foo = new int [5]; RUNTIME MEMORY MANAGEMENT // Keyword new int * foo; foo = new int [5];](https://slidetodoc.com/presentation_image_h2/c47d7352b238d2860b5d5569695efc47/image-2.jpg)
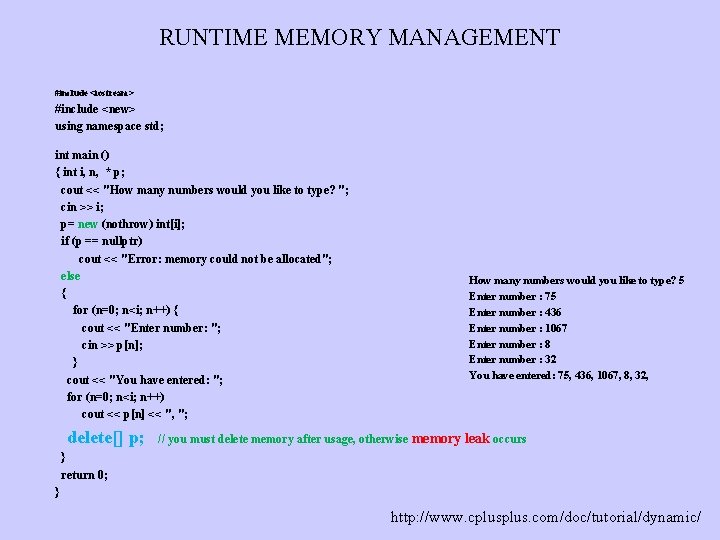
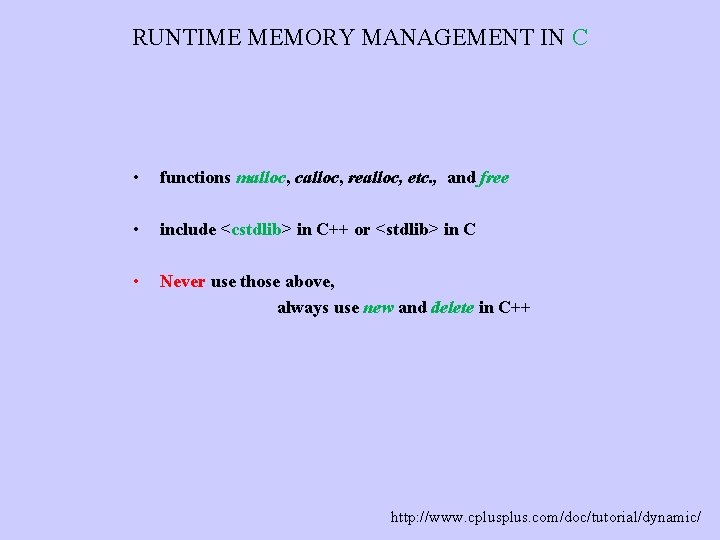
- Slides: 4
![RUNTIME MEMORY MANAGEMENT Keyword new int foo foo new int 5 RUNTIME MEMORY MANAGEMENT // Keyword new int * foo; foo = new int [5];](https://slidetodoc.com/presentation_image_h2/c47d7352b238d2860b5d5569695efc47/image-1.jpg)
RUNTIME MEMORY MANAGEMENT // Keyword new int * foo; foo = new int [5]; //dynamically creates five units of int pointed at by pointer foo // must use pointer http: //www. cplus. com/doc/tutorial/dynamic/
![RUNTIME MEMORY MANAGEMENT Keyword new int foo foo new int 5 RUNTIME MEMORY MANAGEMENT // Keyword new int * foo; foo = new int [5];](https://slidetodoc.com/presentation_image_h2/c47d7352b238d2860b5d5569695efc47/image-2.jpg)
RUNTIME MEMORY MANAGEMENT // Keyword new int * foo; foo = new int [5]; //create five units of int pointed at by pointer foo // must use pointer // It is possible to ask for so large a memory that it is not available on RAM, system responds then // with Error: memory could not be allocated int * foo; foo = new (nothrow) int [5]; // to avoid a termination without program crashing if (foo = = nullptr) { // error assigning memory. Take measures. } http: //www. cplus. com/doc/tutorial/dynamic/
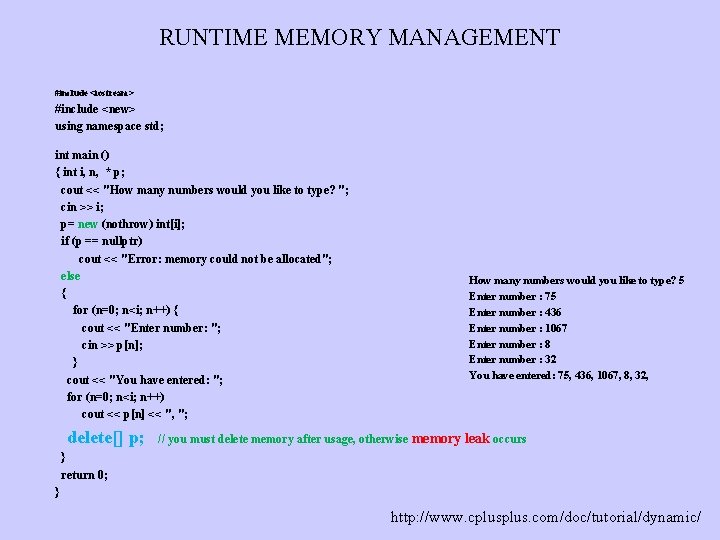
RUNTIME MEMORY MANAGEMENT #include <iostream> #include <new> using namespace std; int main () { int i, n, * p; cout << "How many numbers would you like to type? "; cin >> i; p= new (nothrow) int[i]; if (p == nullptr) cout << "Error: memory could not be allocated"; else { for (n=0; n<i; n++) { cout << "Enter number: "; cin >> p[n]; } cout << "You have entered: "; for (n=0; n<i; n++) cout << p[n] << ", "; delete[] p; How many numbers would you like to type? 5 Enter number : 75 Enter number : 436 Enter number : 1067 Enter number : 8 Enter number : 32 You have entered: 75, 436, 1067, 8, 32, // you must delete memory after usage, otherwise memory leak occurs } return 0; } http: //www. cplus. com/doc/tutorial/dynamic/
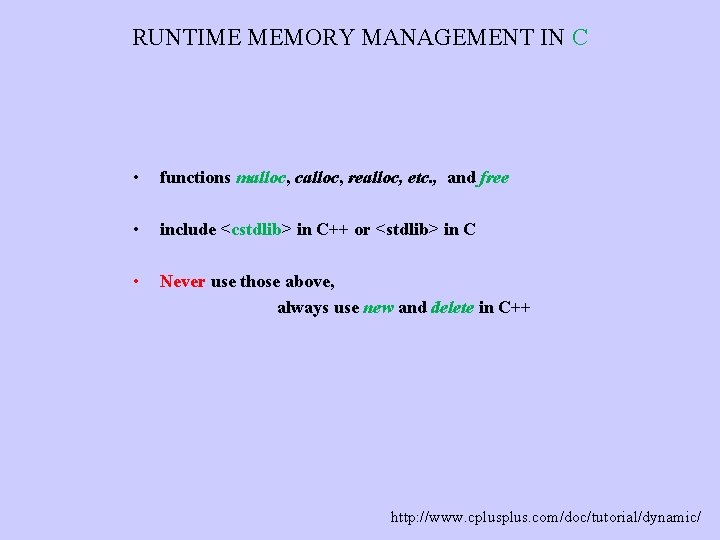
RUNTIME MEMORY MANAGEMENT IN C • functions malloc, calloc, realloc, etc. , and free • include <cstdlib> in C++ or <stdlib> in C • Never use those above, always use new and delete in C++ http: //www. cplus. com/doc/tutorial/dynamic/