Python Input and Output Output Formatting Two methods
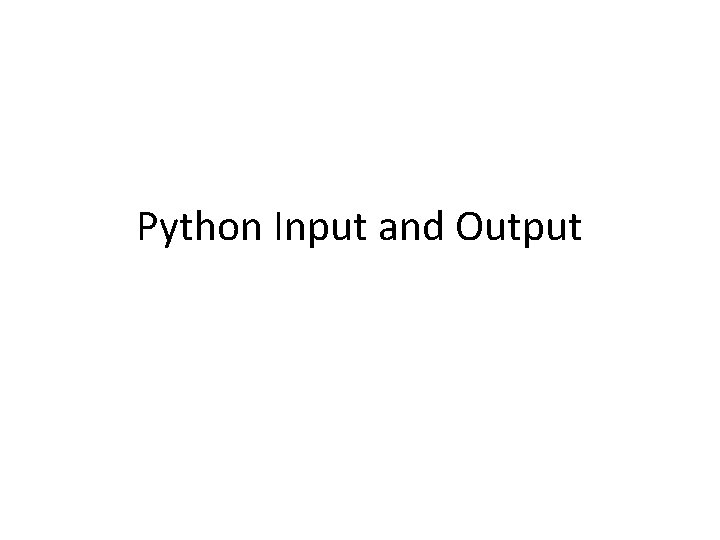
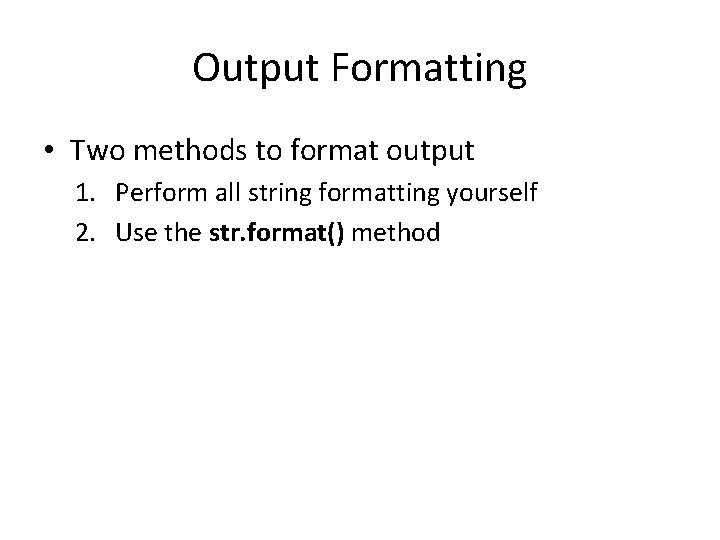
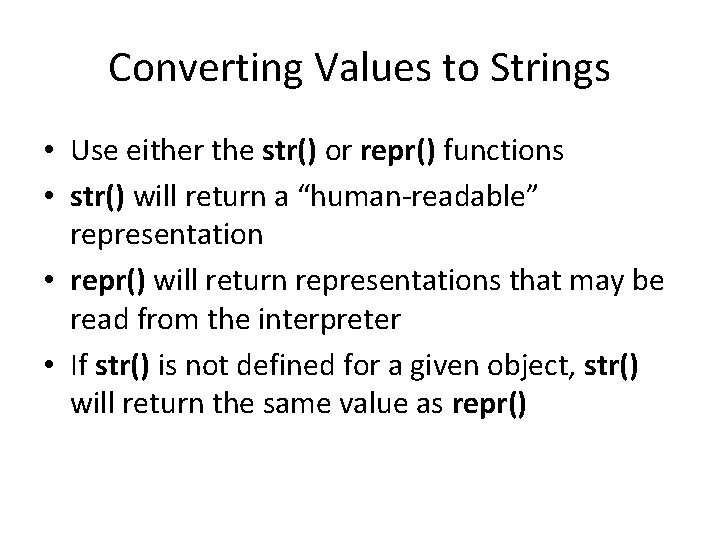
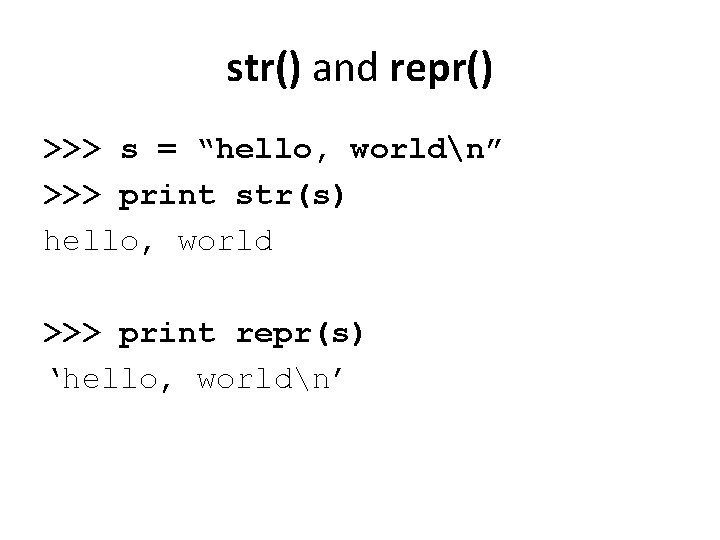
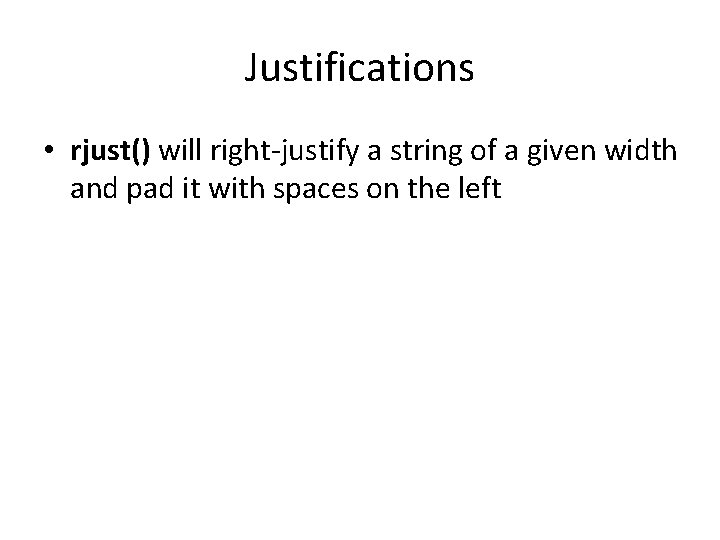
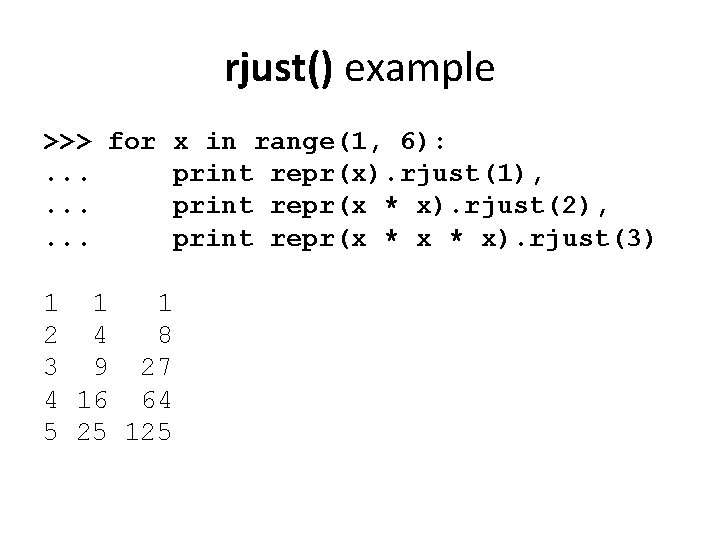
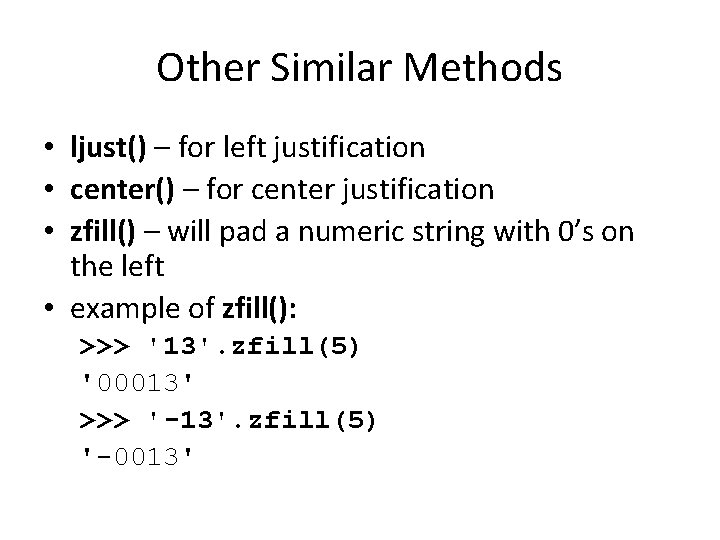
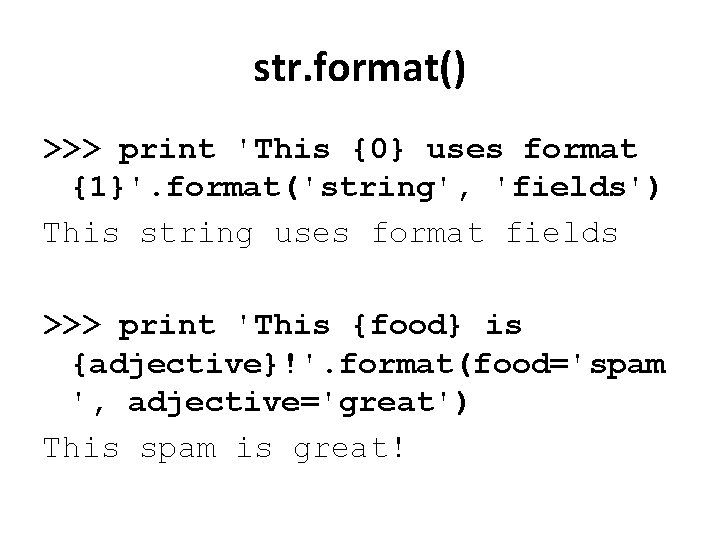
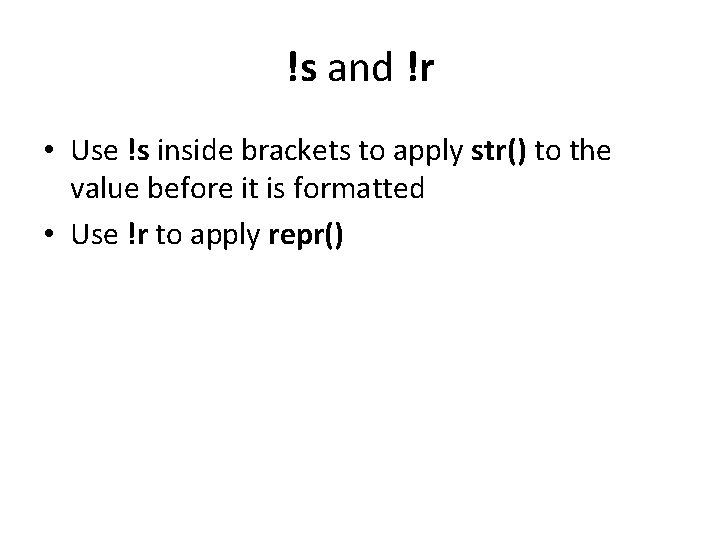
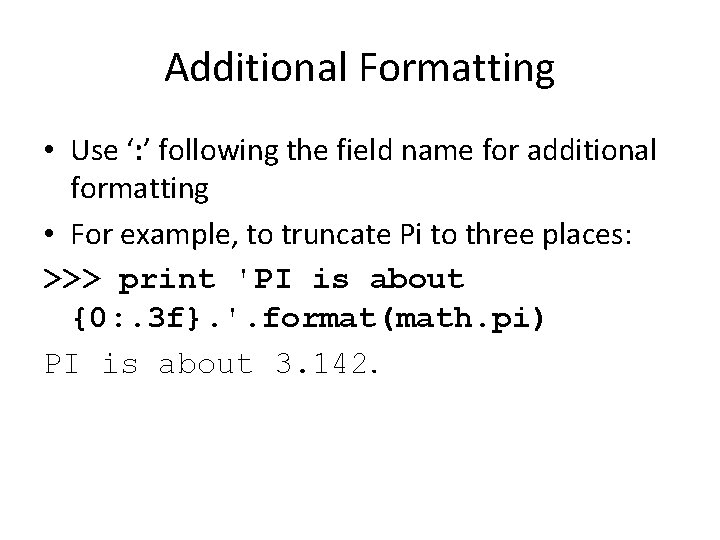
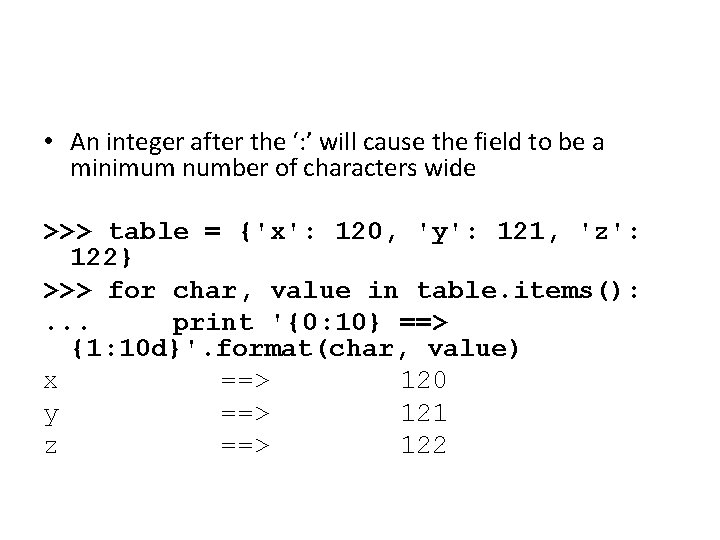
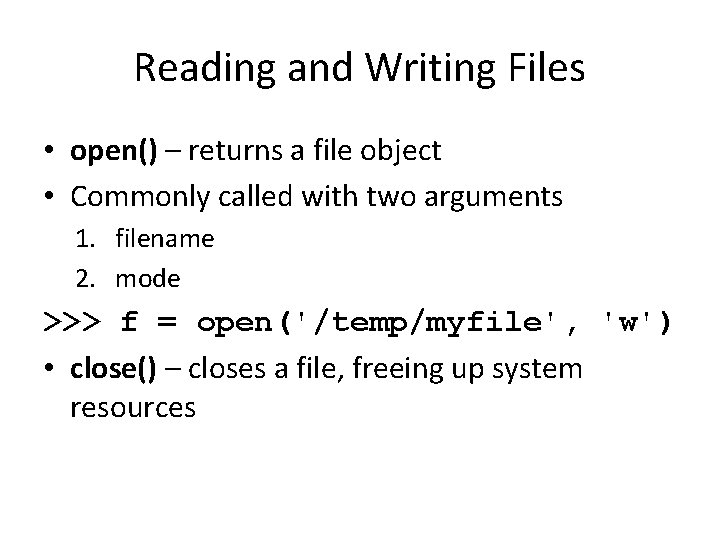
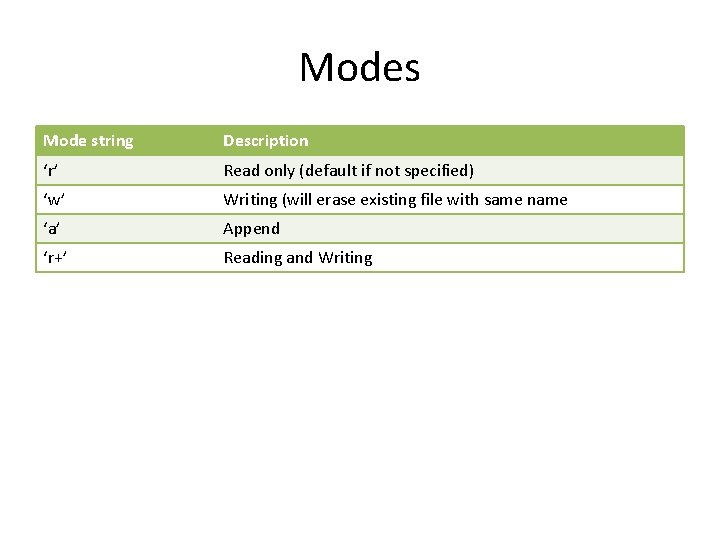
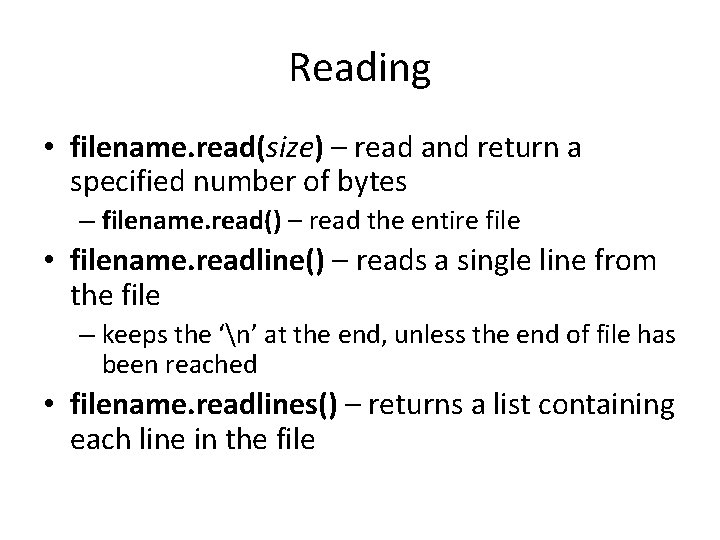
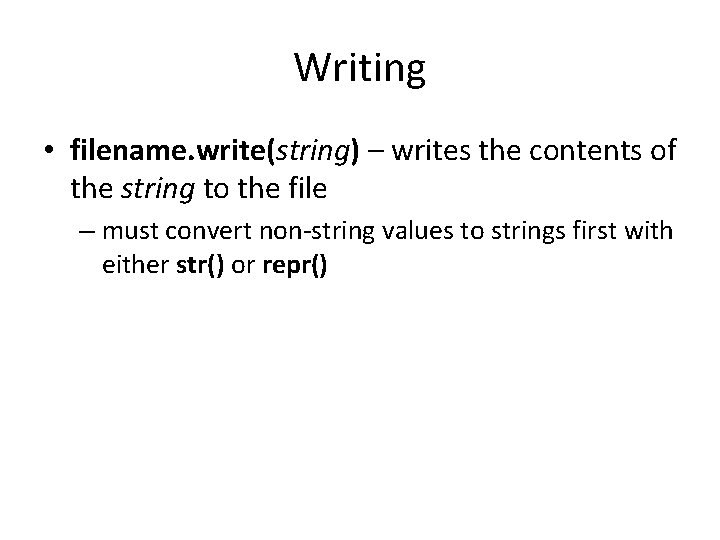
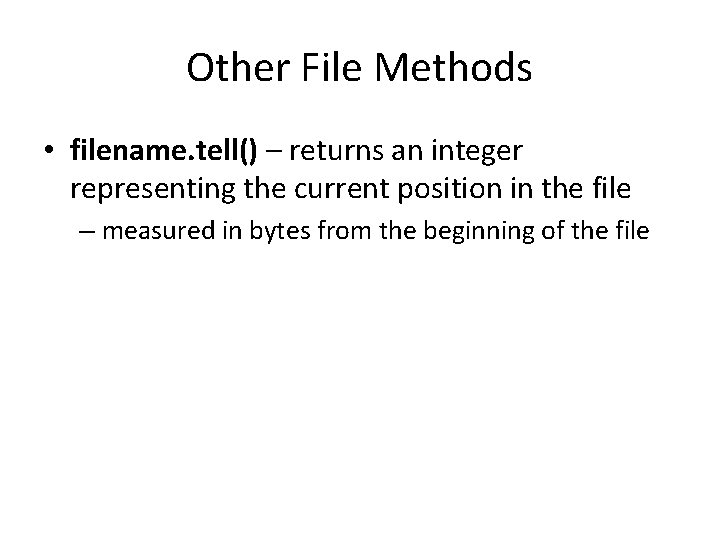
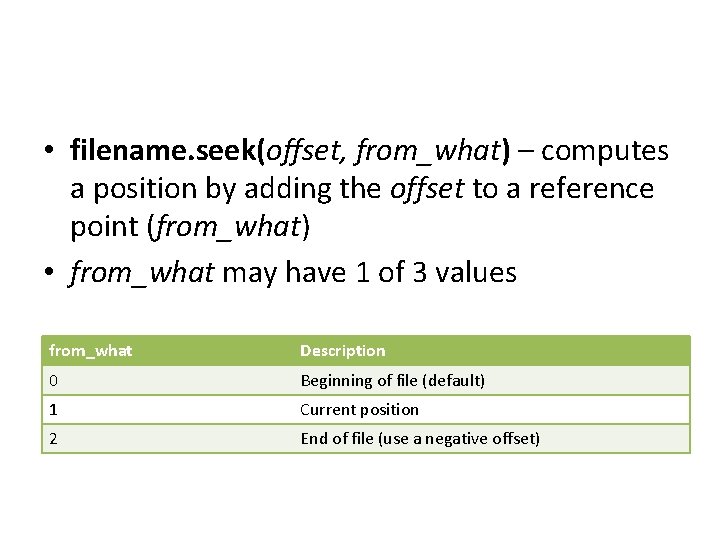
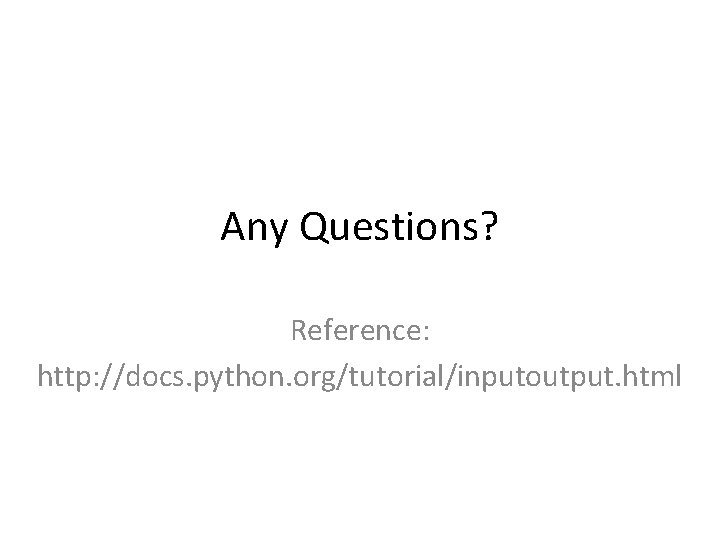
- Slides: 18
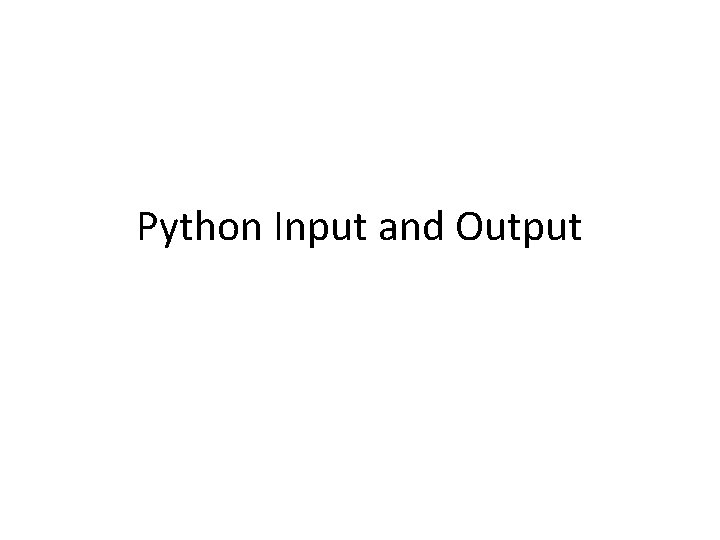
Python Input and Output
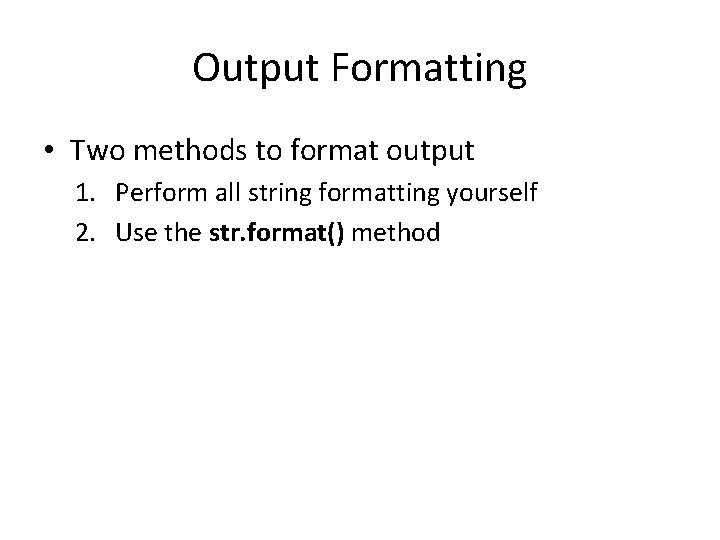
Output Formatting • Two methods to format output 1. Perform all string formatting yourself 2. Use the str. format() method
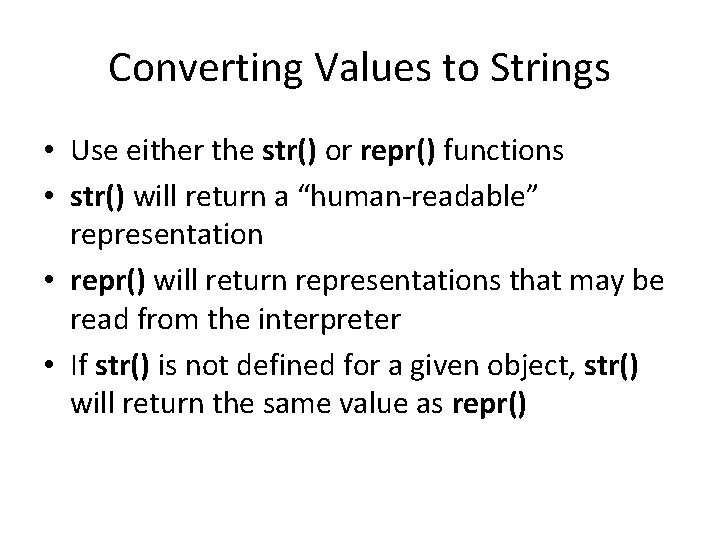
Converting Values to Strings • Use either the str() or repr() functions • str() will return a “human-readable” representation • repr() will return representations that may be read from the interpreter • If str() is not defined for a given object, str() will return the same value as repr()
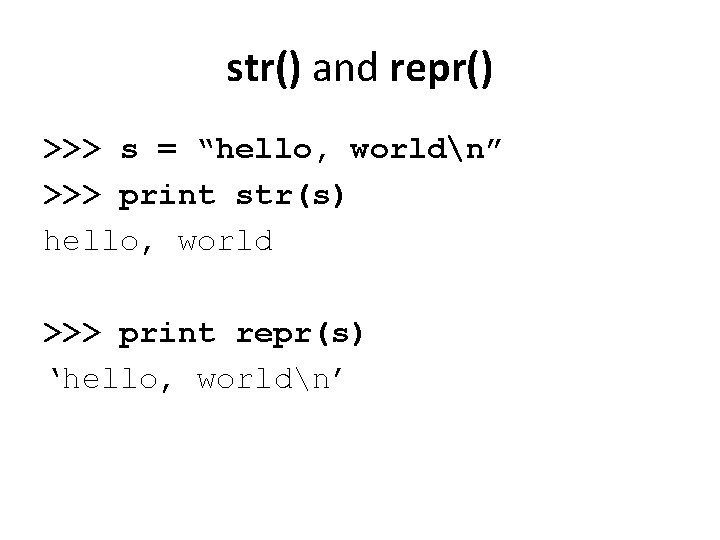
str() and repr() >>> s = “hello, worldn” >>> print str(s) hello, world >>> print repr(s) ‘hello, worldn’
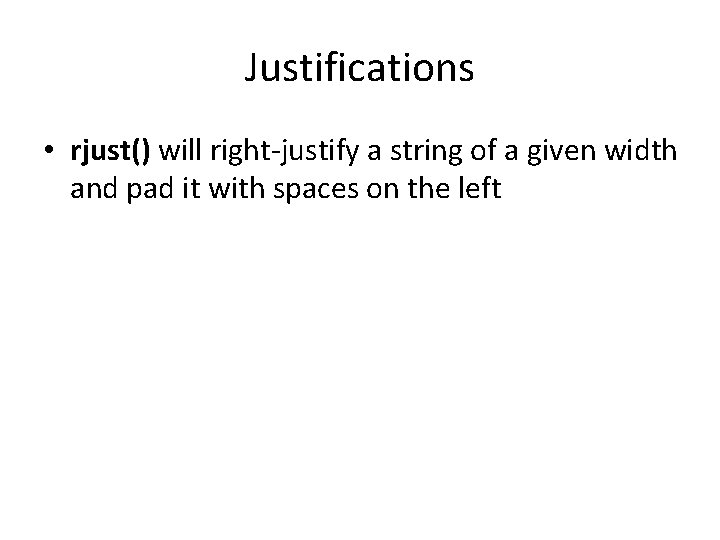
Justifications • rjust() will right-justify a string of a given width and pad it with spaces on the left
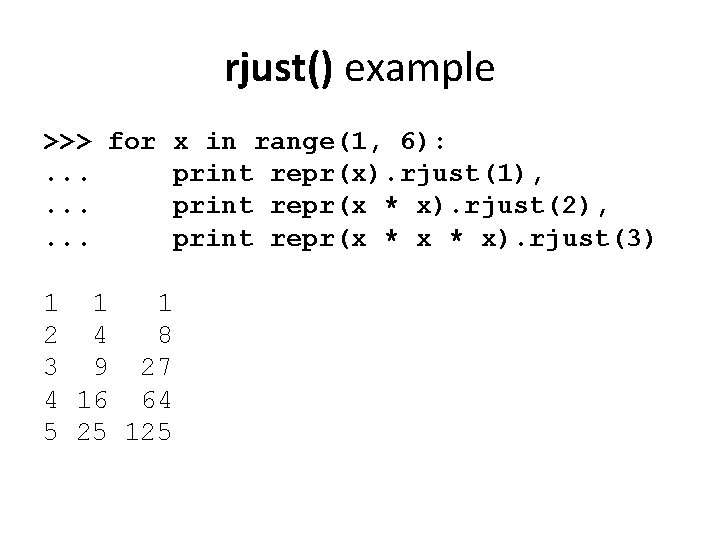
rjust() example >>> for x in range(1, 6): . . . print repr(x). rjust(1), . . . print repr(x * x). rjust(2), . . . print repr(x * x). rjust(3) 1 1 1 2 4 8 3 9 27 4 16 64 5 25 125
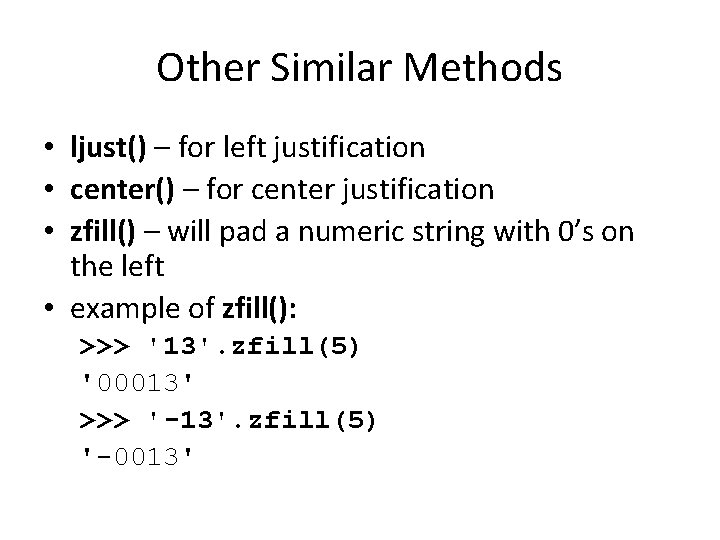
Other Similar Methods • ljust() – for left justification • center() – for center justification • zfill() – will pad a numeric string with 0’s on the left • example of zfill(): >>> '13'. zfill(5) '00013' >>> '-13'. zfill(5) '-0013'
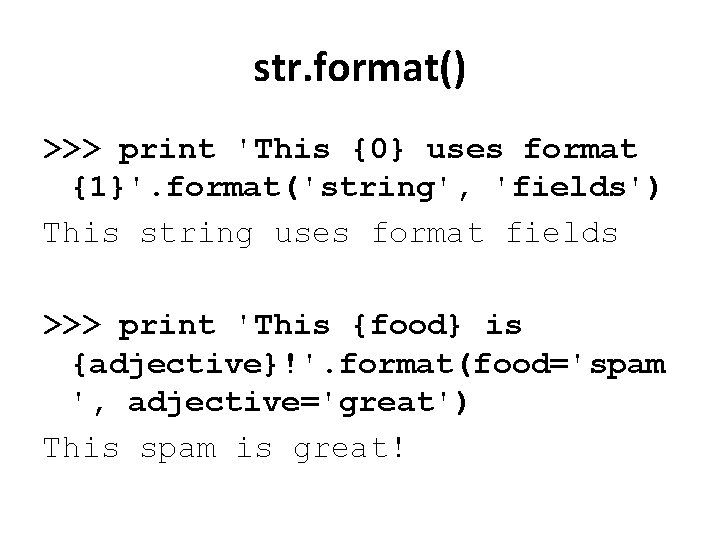
str. format() >>> print 'This {0} uses format {1}'. format('string', 'fields') This string uses format fields >>> print 'This {food} is {adjective}!'. format(food='spam ', adjective='great') This spam is great!
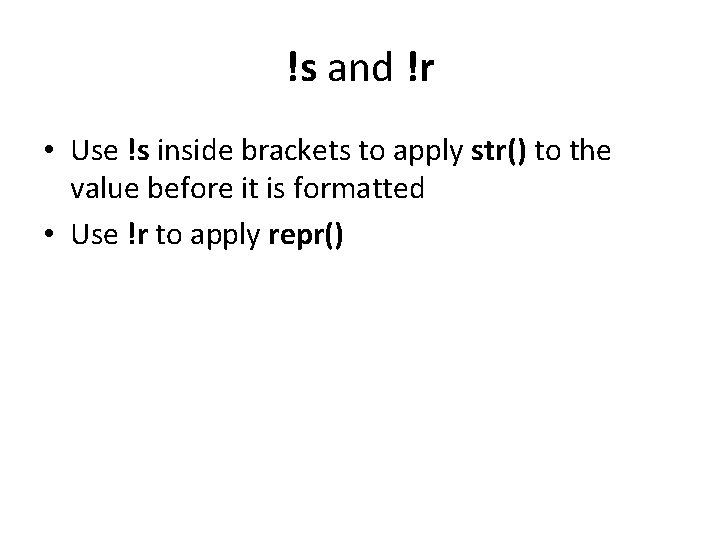
!s and !r • Use !s inside brackets to apply str() to the value before it is formatted • Use !r to apply repr()
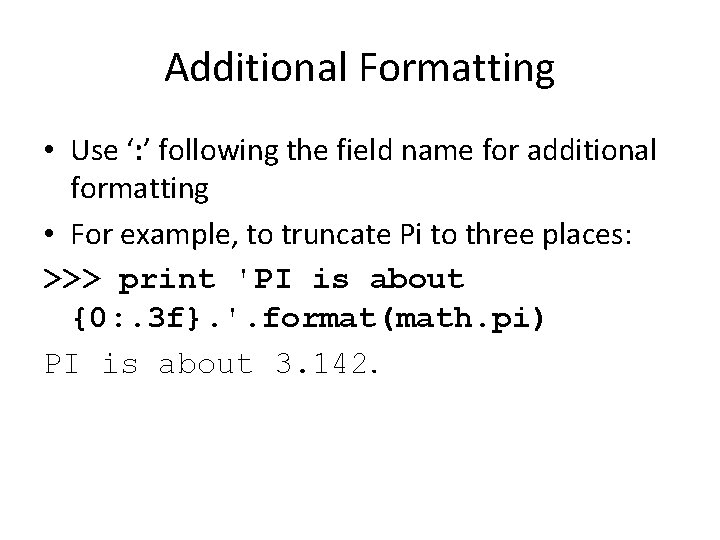
Additional Formatting • Use ‘: ’ following the field name for additional formatting • For example, to truncate Pi to three places: >>> print 'PI is about {0: . 3 f}. '. format(math. pi) PI is about 3. 142.
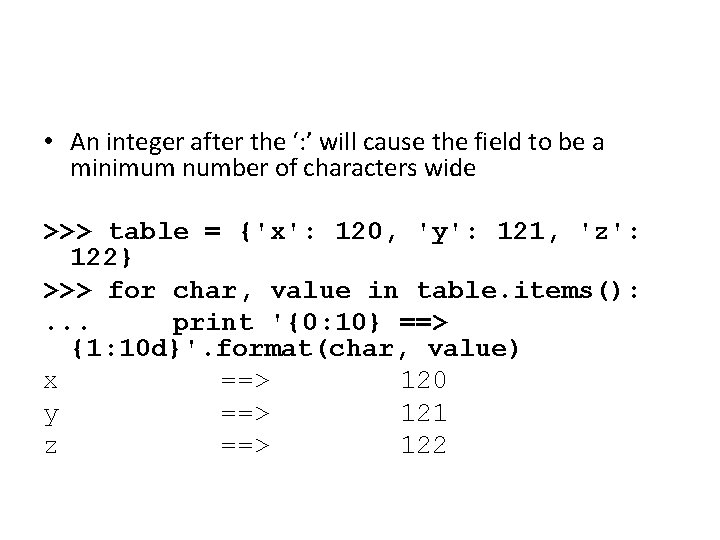
• An integer after the ‘: ’ will cause the field to be a minimum number of characters wide >>> table = {'x': 120, 'y': 121, 'z': 122} >>> for char, value in table. items(): . . . print '{0: 10} ==> {1: 10 d}'. format(char, value) x ==> 120 y ==> 121 z ==> 122
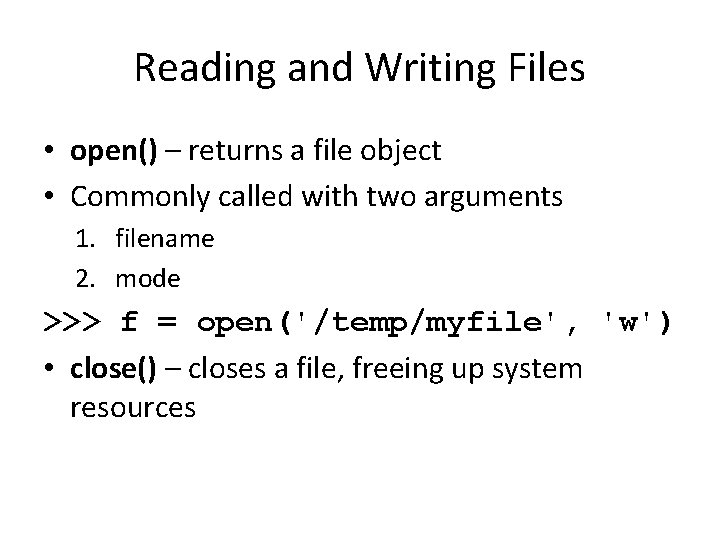
Reading and Writing Files • open() – returns a file object • Commonly called with two arguments 1. filename 2. mode >>> f = open('/temp/myfile', 'w') • close() – closes a file, freeing up system resources
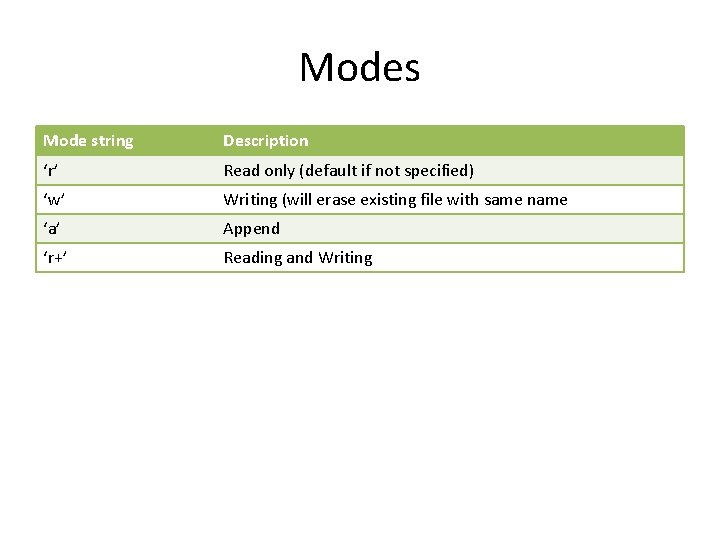
Modes Mode string Description ‘r’ Read only (default if not specified) ‘w’ Writing (will erase existing file with same name ‘a’ Append ‘r+’ Reading and Writing
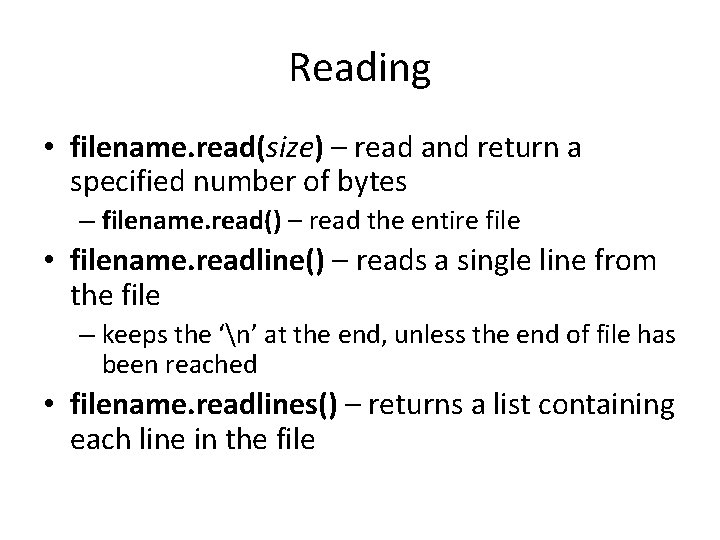
Reading • filename. read(size) – read and return a specified number of bytes – filename. read() – read the entire file • filename. readline() – reads a single line from the file – keeps the ‘n’ at the end, unless the end of file has been reached • filename. readlines() – returns a list containing each line in the file
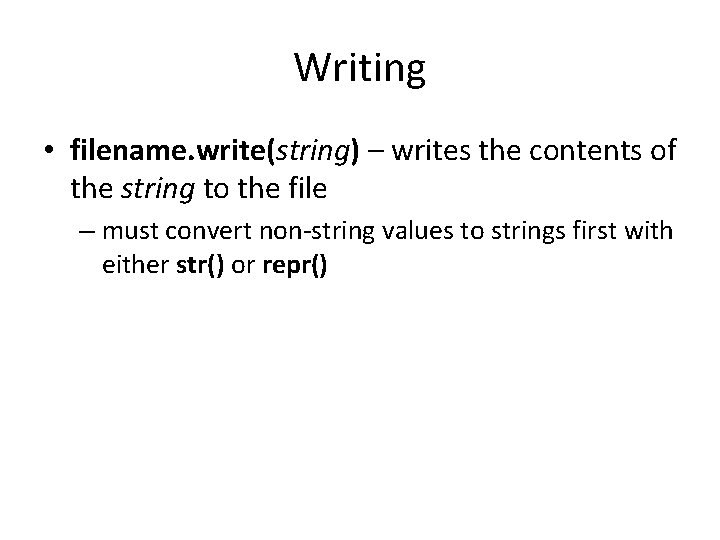
Writing • filename. write(string) – writes the contents of the string to the file – must convert non-string values to strings first with either str() or repr()
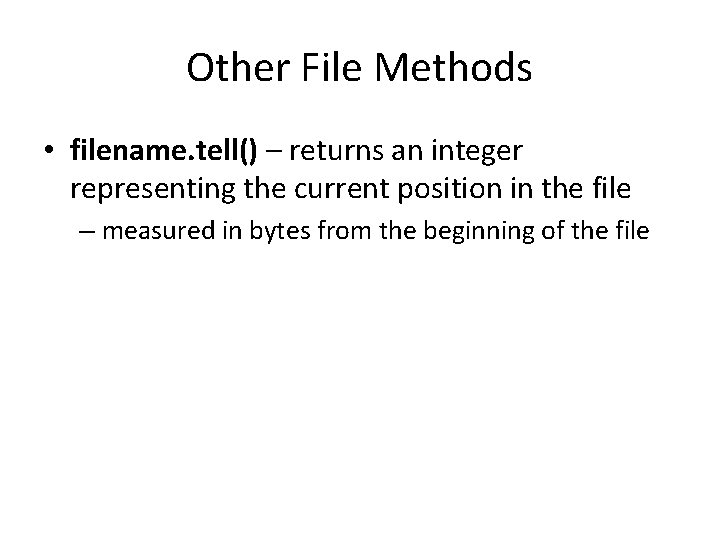
Other File Methods • filename. tell() – returns an integer representing the current position in the file – measured in bytes from the beginning of the file
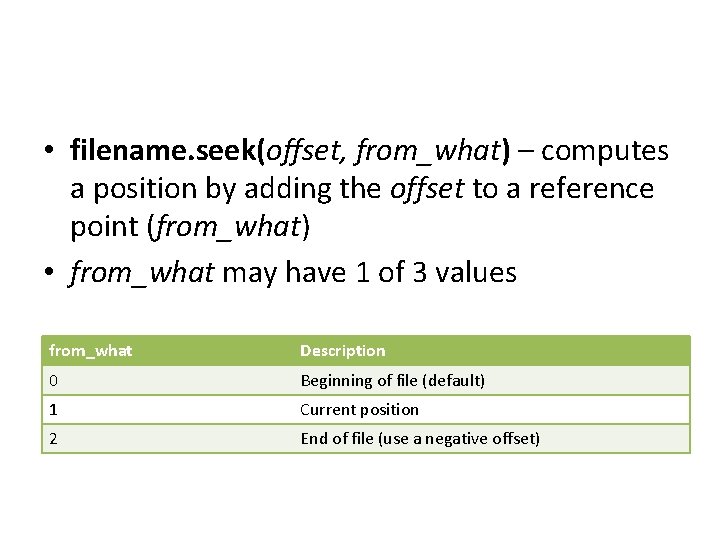
• filename. seek(offset, from_what) – computes a position by adding the offset to a reference point (from_what) • from_what may have 1 of 3 values from_what Description 0 Beginning of file (default) 1 Current position 2 End of file (use a negative offset)
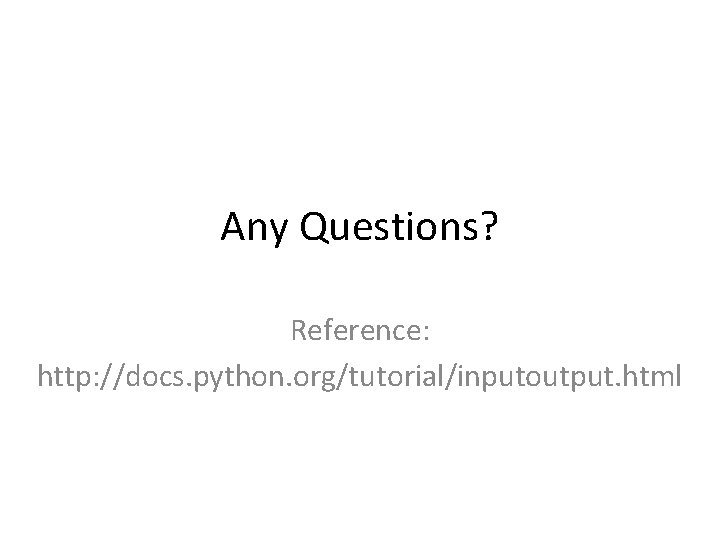
Any Questions? Reference: http: //docs. python. org/tutorial/inputoutput. html
Input and output unit
Define output design
Manual and automatic input devices
Finely tuned input and roughly tuned input
Editing and formatting text
Magnetic disk read and write mechanism
Punctuation marks quotes
Python methods vs functions
Functions list
Wax pattern fabrication
Input format python
Reading an empty input is an example of unobvious errors.
Direct input python
What are the interactive input methods?
Data input methods
Output devices used in virtual reality
Input vat and output vat
Input vs output vat
Input vat