Partition sort Quicksort Pick a middle item and
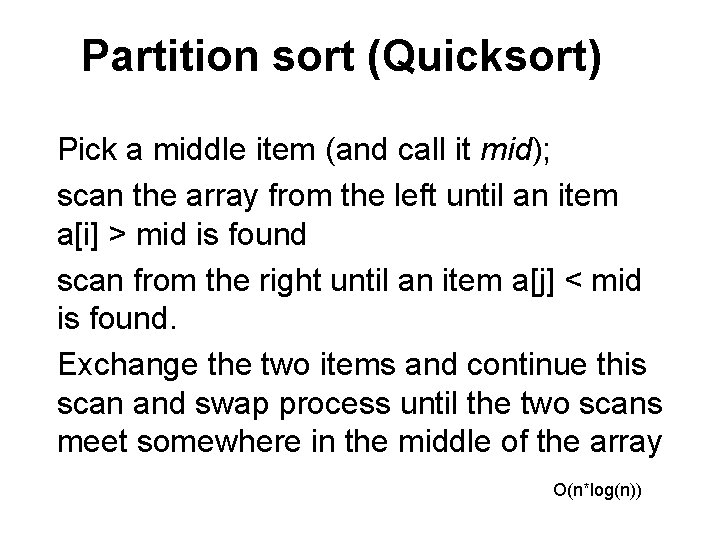
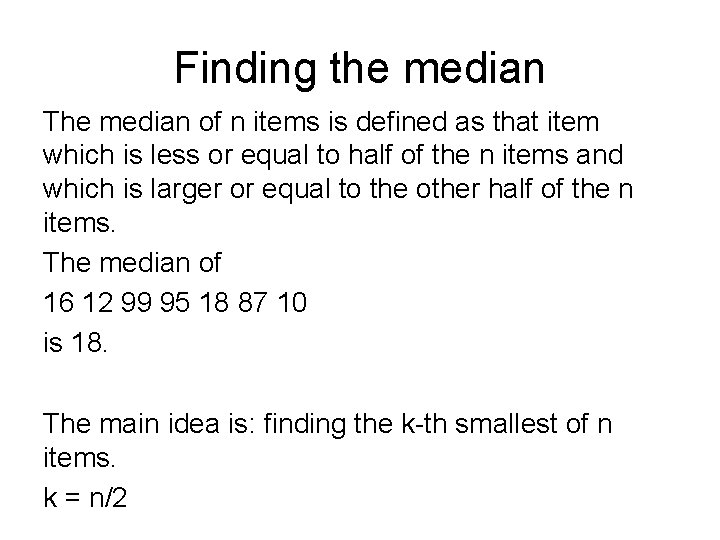
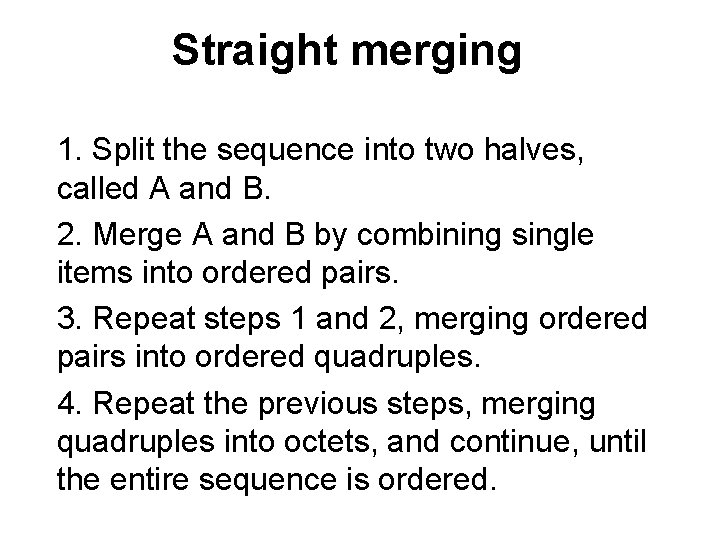
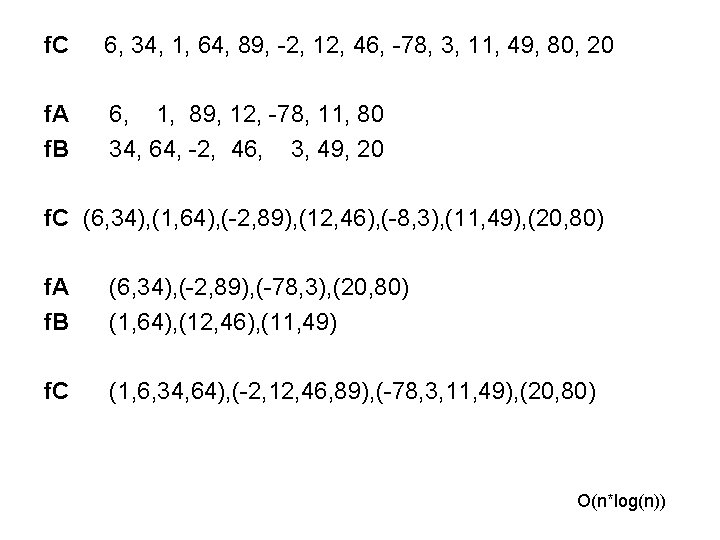
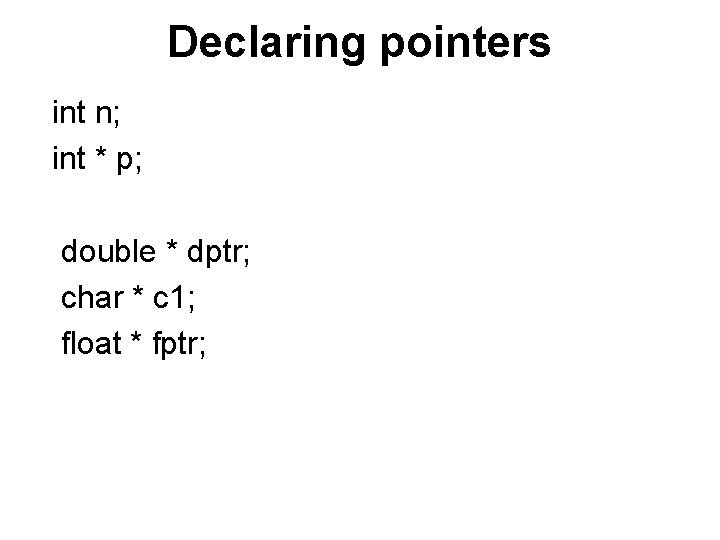
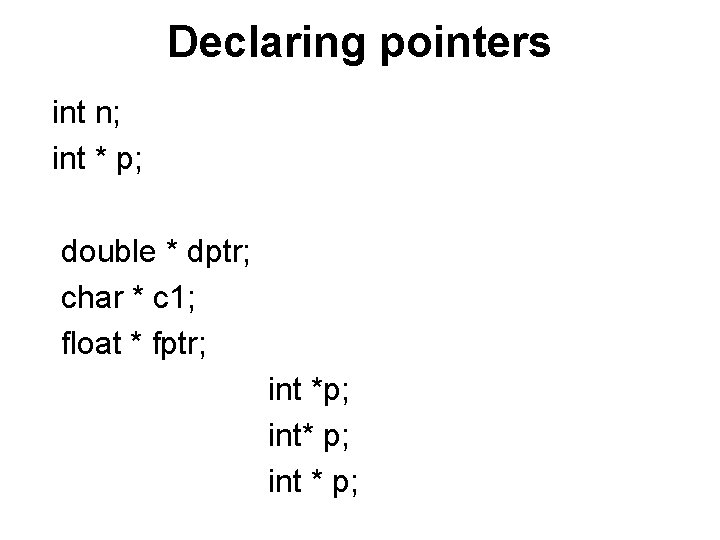
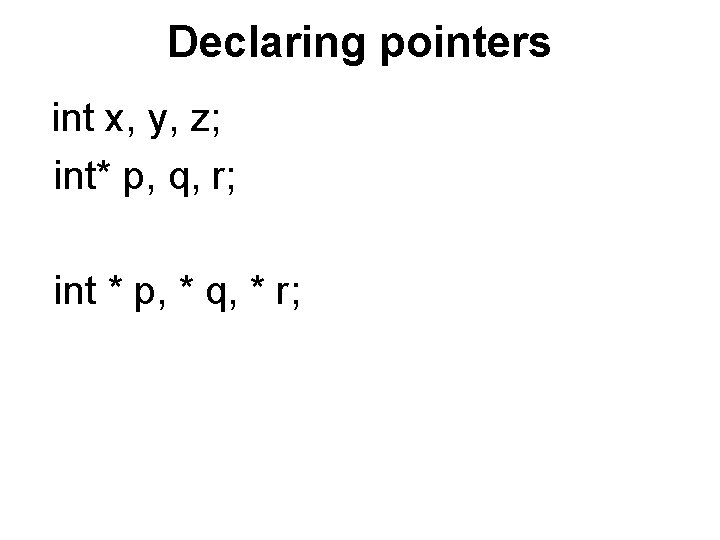
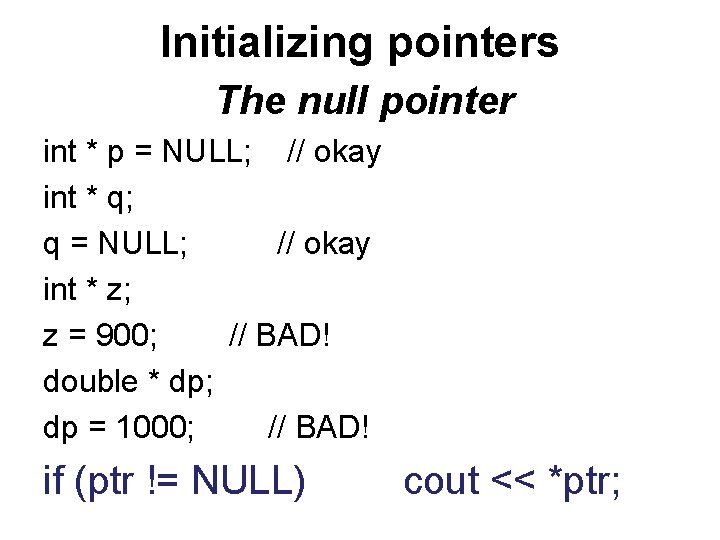
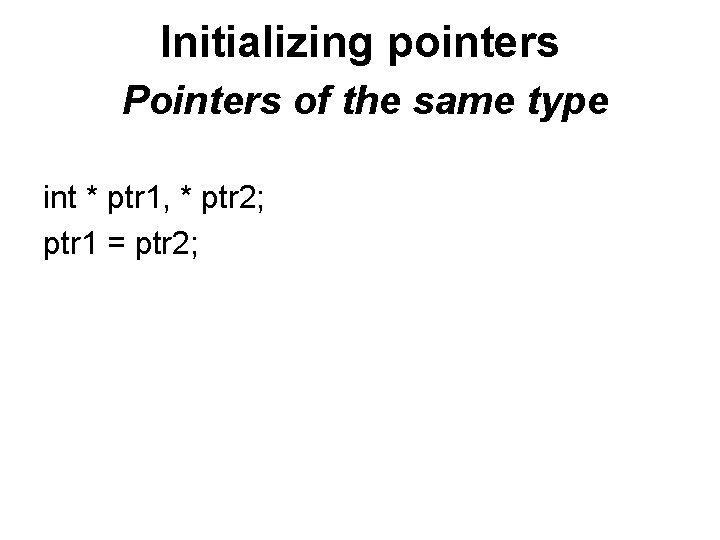
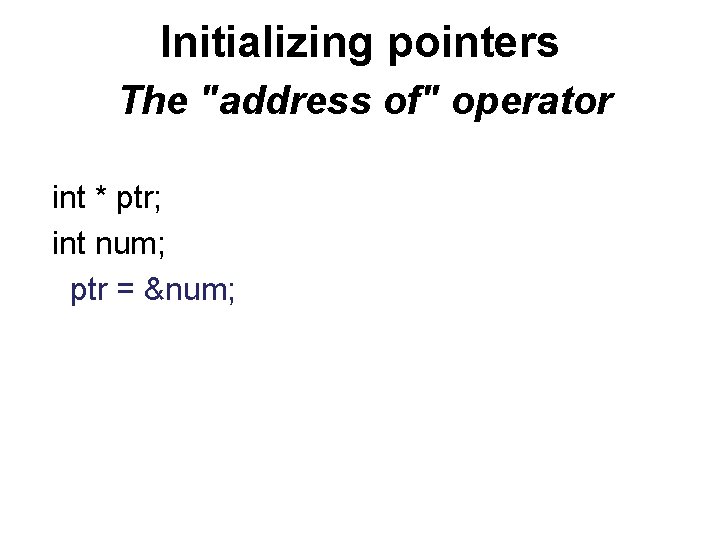
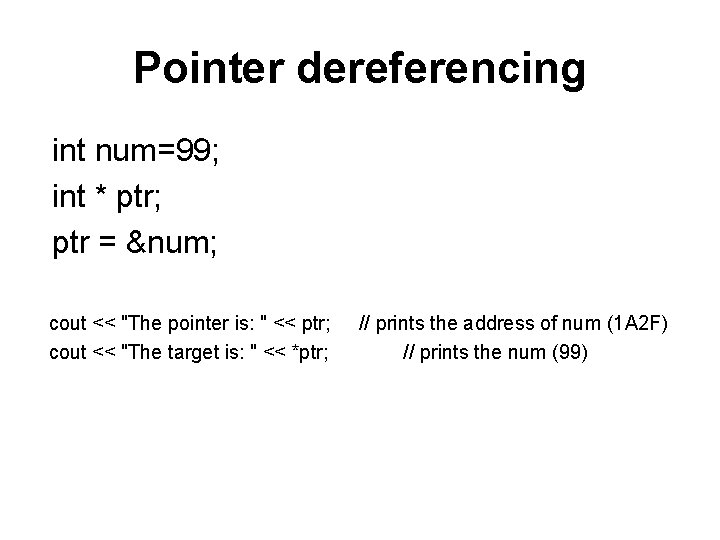
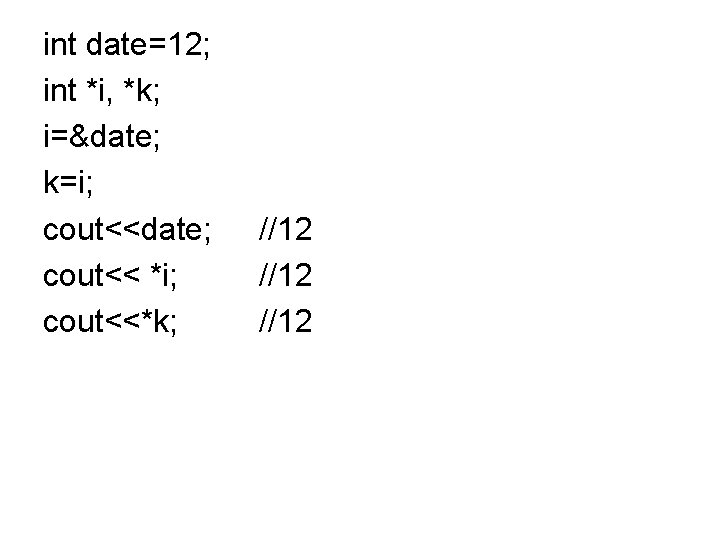
- Slides: 12
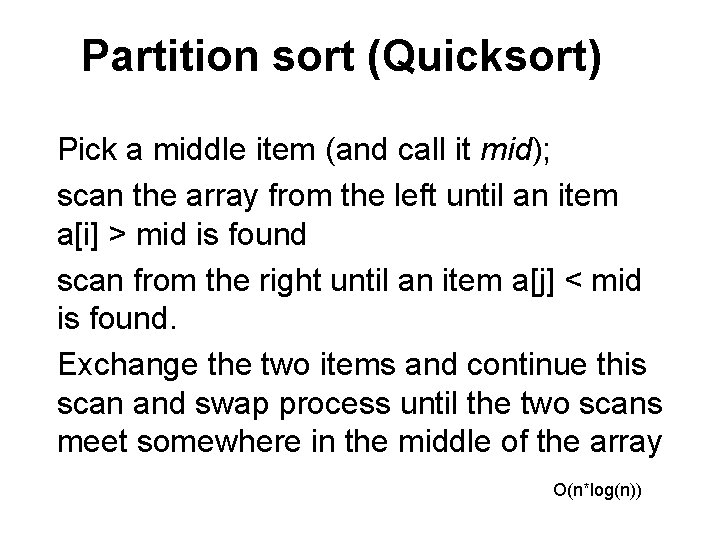
Partition sort (Quicksort) Pick a middle item (and call it mid); scan the array from the left until an item a[i] > mid is found scan from the right until an item a[j] < mid is found. Exchange the two items and continue this scan and swap process until the two scans meet somewhere in the middle of the array O(n*log(n))
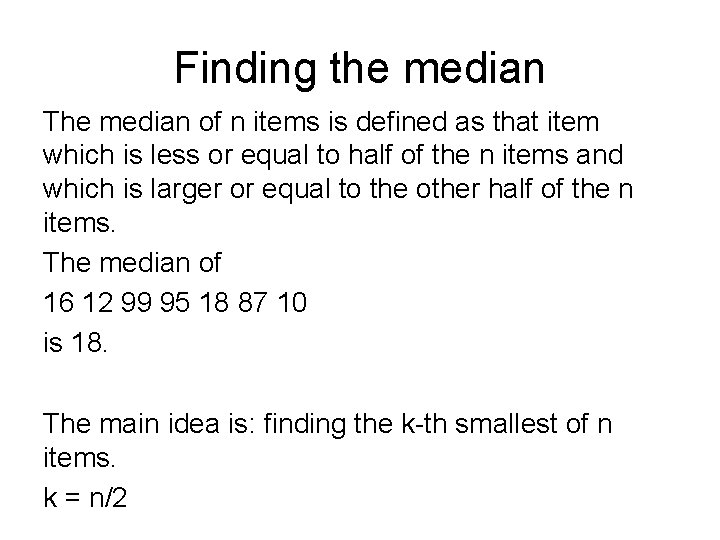
Finding the median The median of n items is defined as that item which is less or equal to half of the n items and which is larger or equal to the other half of the n items. The median of 16 12 99 95 18 87 10 is 18. The main idea is: finding the k-th smallest of n items. k = n/2
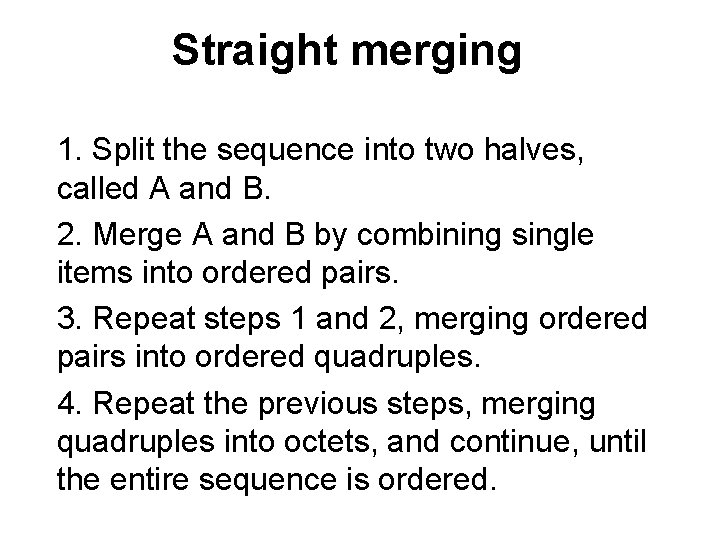
Straight merging 1. Split the sequence into two halves, called A and B. 2. Merge A and B by combining single items into ordered pairs. 3. Repeat steps 1 and 2, merging ordered pairs into ordered quadruples. 4. Repeat the previous steps, merging quadruples into octets, and continue, until the entire sequence is ordered.
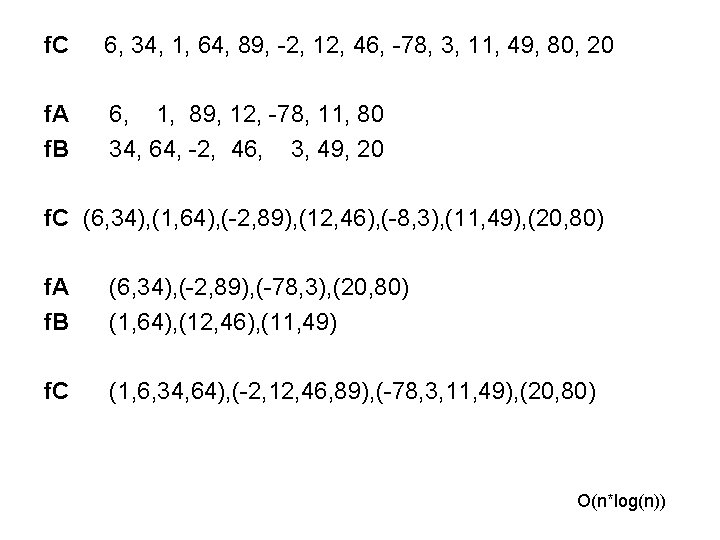
f. C 6, 34, 1, 64, 89, -2, 12, 46, -78, 3, 11, 49, 80, 20 f. A f. B 6, 1, 89, 12, -78, 11, 80 34, 64, -2, 46, 3, 49, 20 f. C (6, 34), (1, 64), (-2, 89), (12, 46), (-8, 3), (11, 49), (20, 80) f. A f. B (6, 34), (-2, 89), (-78, 3), (20, 80) (1, 64), (12, 46), (11, 49) f. C (1, 6, 34, 64), (-2, 12, 46, 89), (-78, 3, 11, 49), (20, 80) O(n*log(n))
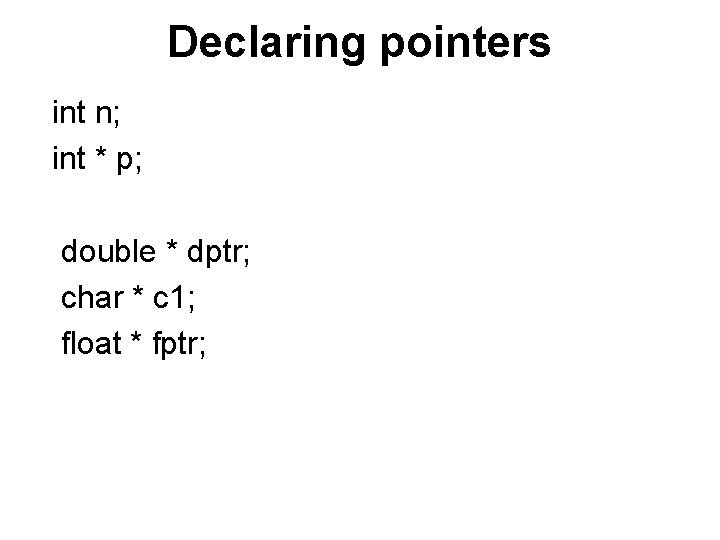
Declaring pointers int n; int * p; double * dptr; char * c 1; float * fptr;
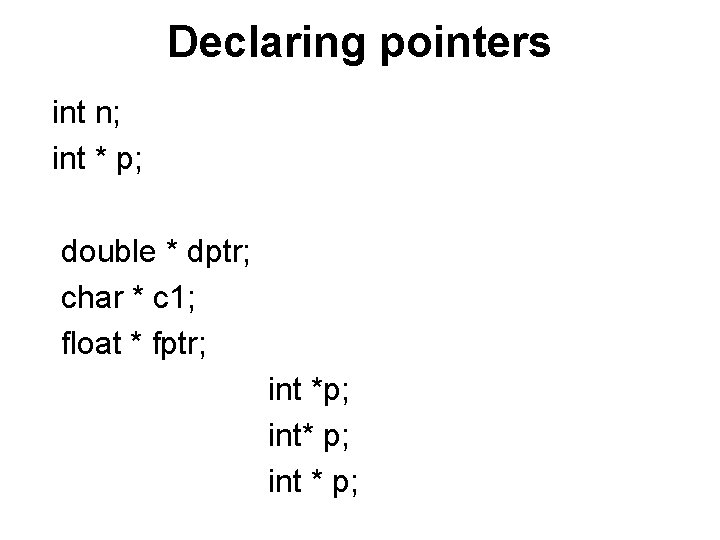
Declaring pointers int n; int * p; double * dptr; char * c 1; float * fptr; int *p; int* p; int * p;
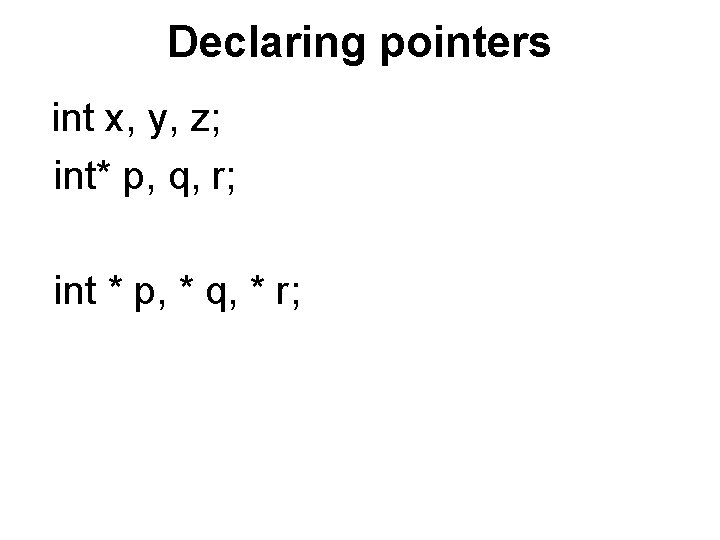
Declaring pointers int x, y, z; int* p, q, r; int * p, * q, * r;
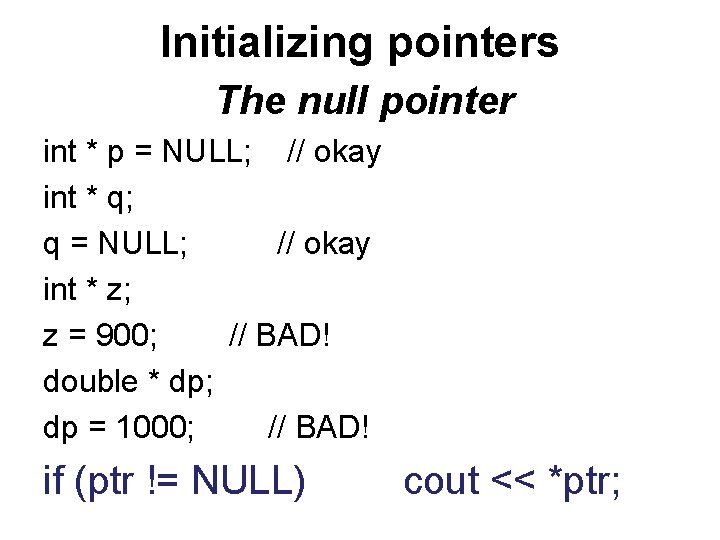
Initializing pointers The null pointer int * p = NULL; // okay int * q; q = NULL; // okay int * z; z = 900; // BAD! double * dp; dp = 1000; // BAD! if (ptr != NULL) cout << *ptr;
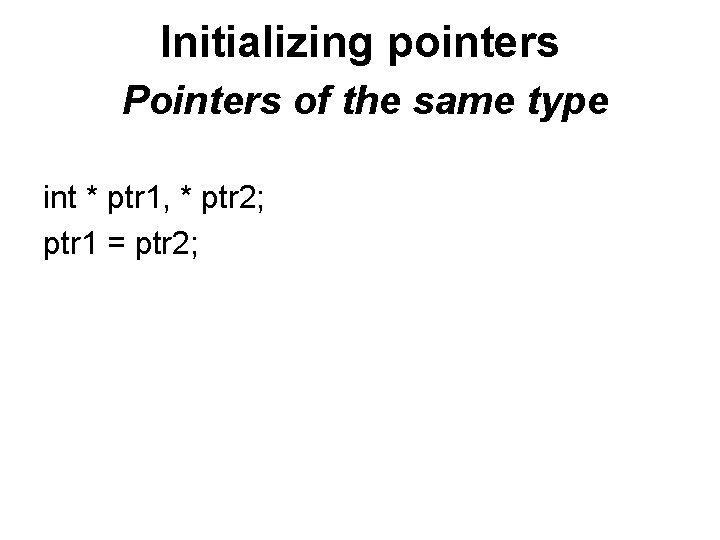
Initializing pointers Pointers of the same type int * ptr 1, * ptr 2; ptr 1 = ptr 2;
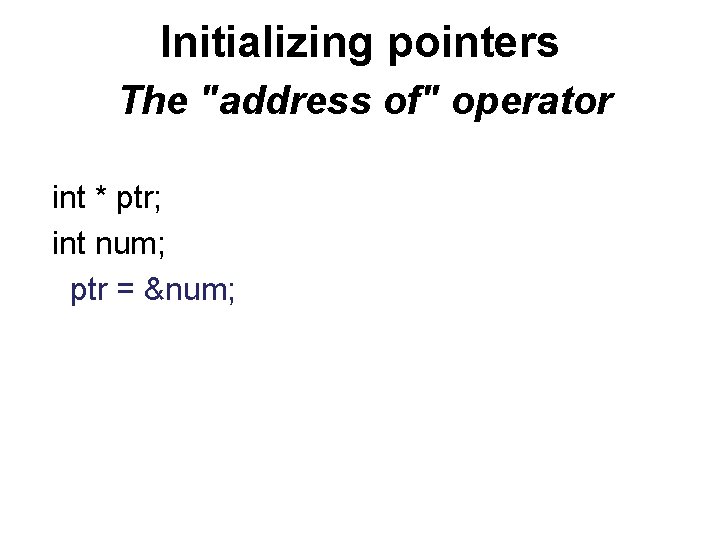
Initializing pointers The "address of" operator int * ptr; int num; ptr = #
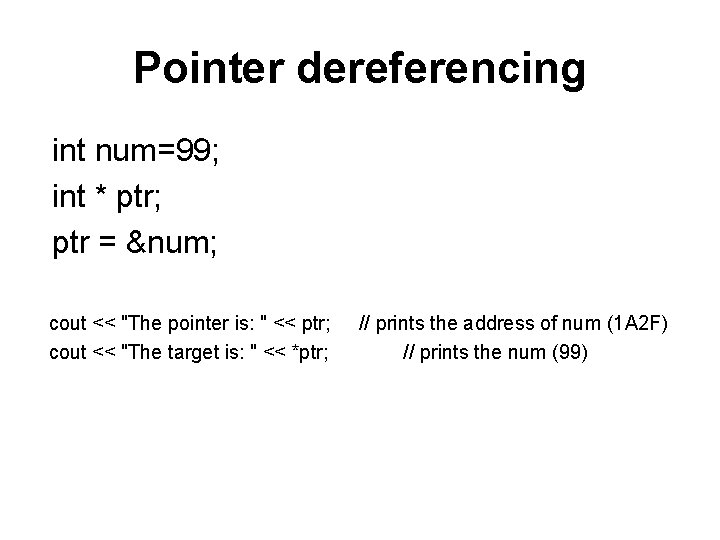
Pointer dereferencing int num=99; int * ptr; ptr = # cout << "The pointer is: " << ptr; cout << "The target is: " << *ptr; // prints the address of num (1 A 2 F) // prints the num (99)
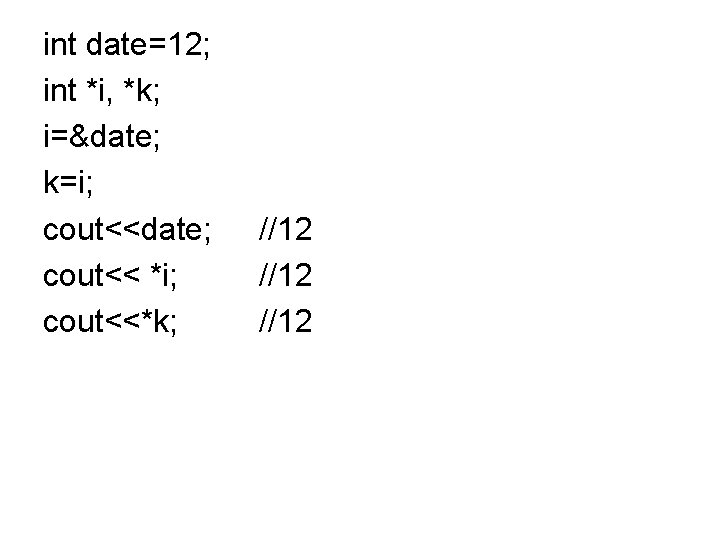
int date=12; int *i, *k; i=&date; k=i; cout<<date; cout<< *i; cout<<*k; //12
Quicksort vs merge sort
Difference between selection sort and bubble sort
Insertion sort vs selection sort
Bubble sort and selection sort
Difference between bubble sort and selection sort
Selection sort vs heap sort
Bubble sort vs selection sort
Bubblesort pseudocode
Quick sort merge sort
Quick sort merge sort
Radix sort animation
Radix bucket sort
Metaphor adalah