NPRG 051 Advanced Programming in C Assignment 1
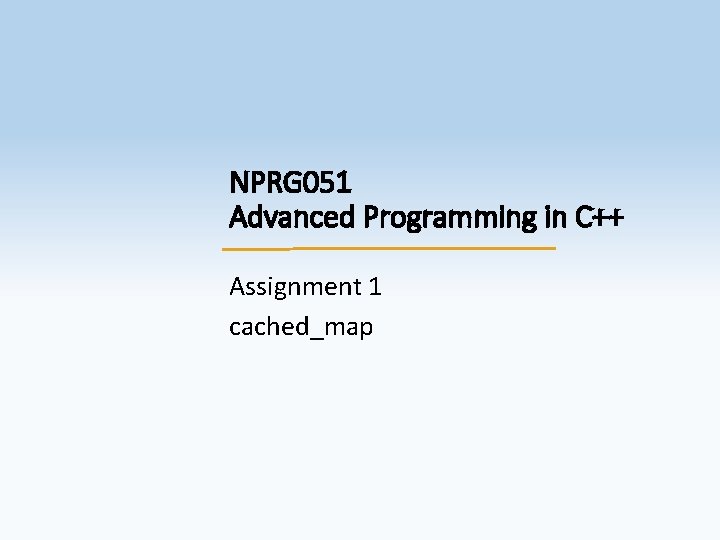
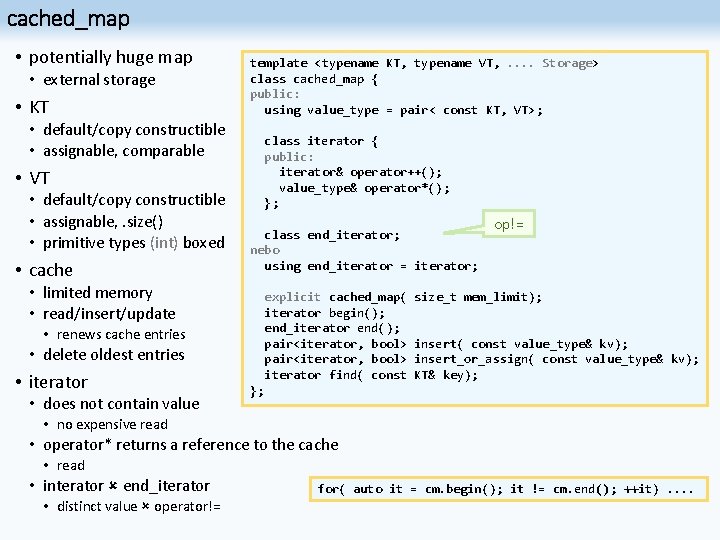
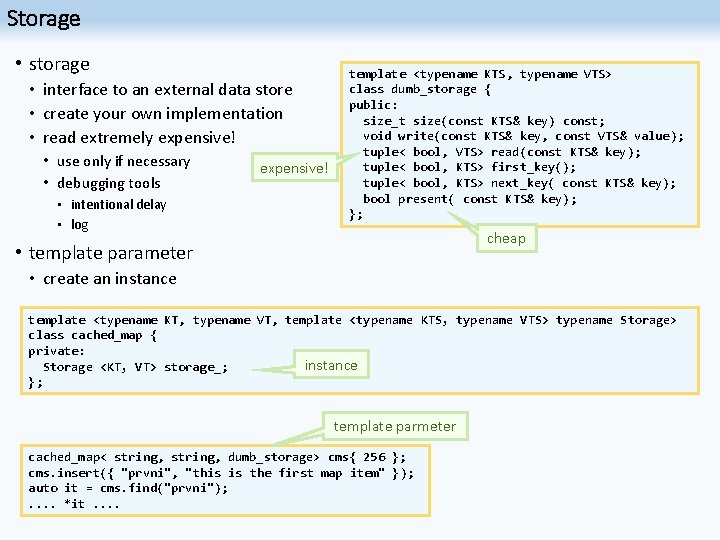
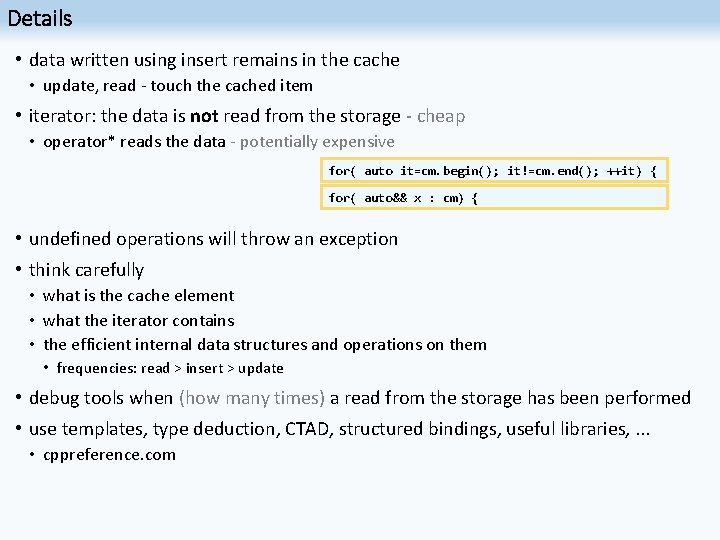
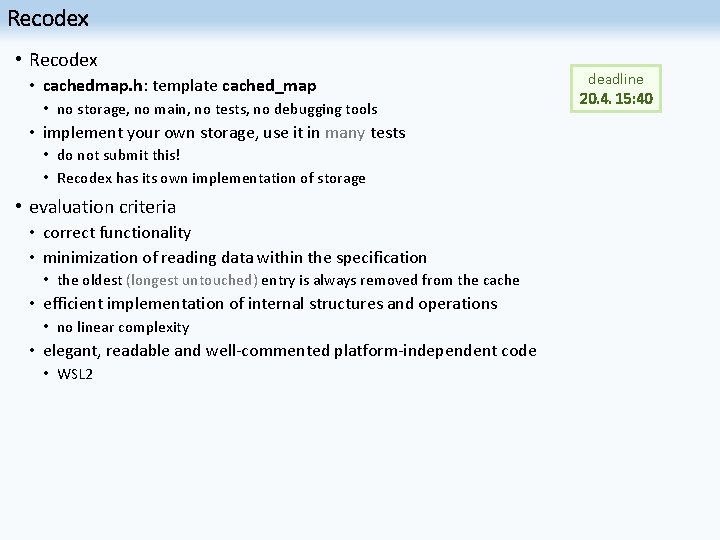
- Slides: 5
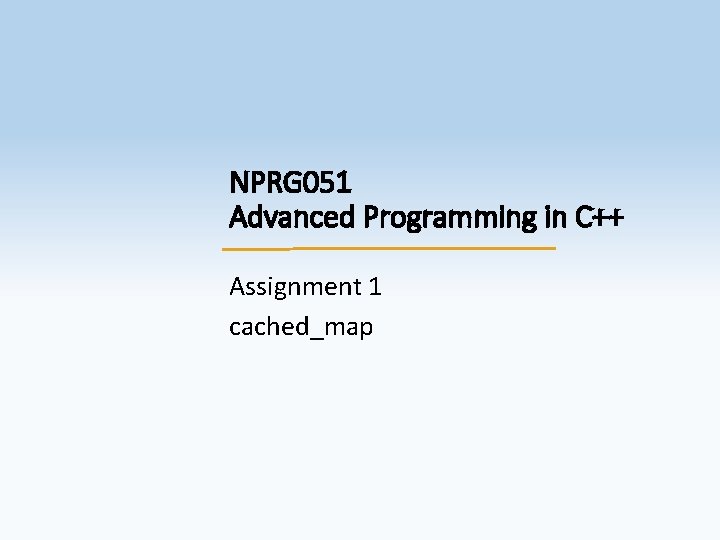
NPRG 051 Advanced Programming in C++ Assignment 1 cached_map
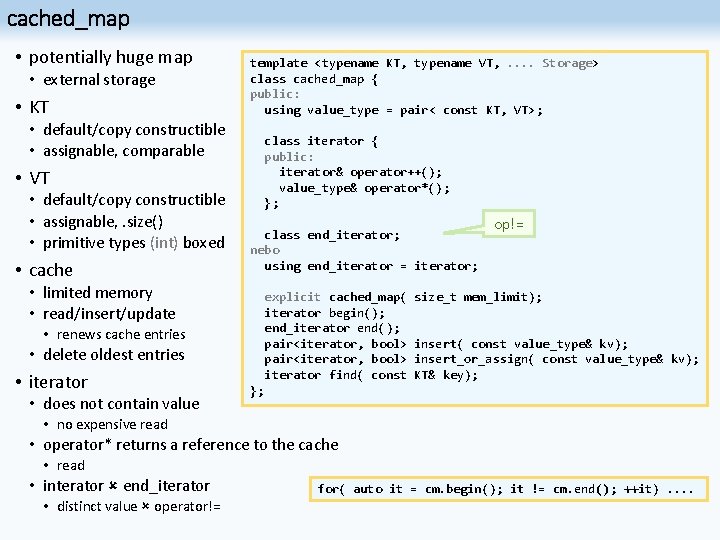
cached_map • potentially huge map • external storage • KT • default/copy constructible • assignable, comparable • VT • default/copy constructible • assignable, . size() • primitive types (int) boxed • cache • limited memory • read/insert/update • renews cache entries • delete oldest entries • iterator • does not contain value template <typename KT, typename VT, . . Storage> class cached_map { public: using value_type = pair< const KT, VT>; class iterator { public: iterator& operator++(); value_type& operator*(); }; class end_iterator; nebo using end_iterator = iterator; explicit cached_map( iterator begin(); end_iterator end(); pair<iterator, bool> iterator find( const }; op!= size_t mem_limit); insert( const value_type& kv); insert_or_assign( const value_type& kv); KT& key); • no expensive read • operator* returns a reference to the cache • read • interator end_iterator • distinct value operator!= for( auto it = cm. begin(); it != cm. end(); ++it). .
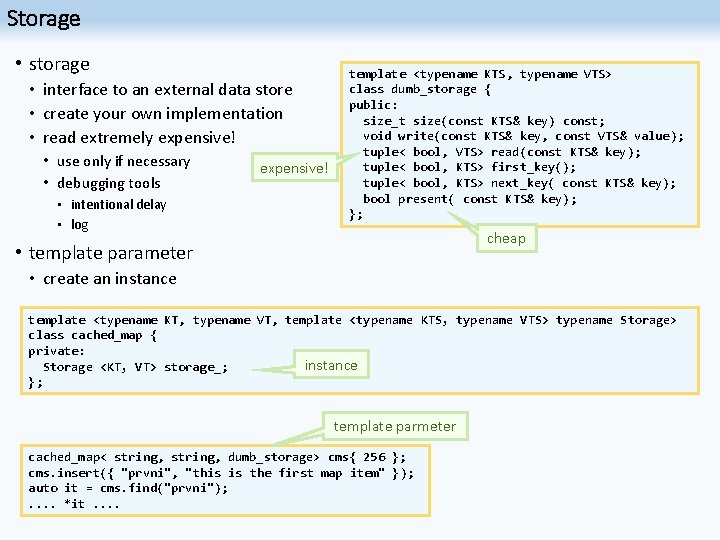
Storage • storage • interface to an external data store • create your own implementation • read extremely expensive! • use only if necessary • debugging tools • intentional delay • log expensive! template <typename KTS, typename VTS> class dumb_storage { public: size_t size(const KTS& key) const; void write(const KTS& key, const VTS& value); tuple< bool, VTS> read(const KTS& key); tuple< bool, KTS> first_key(); tuple< bool, KTS> next_key( const KTS& key); bool present( const KTS& key); }; cheap • template parameter • create an instance template <typename KT, typename VT, template <typename KTS, typename VTS> typename Storage> class cached_map { private: instance Storage <KT, VT> storage_; }; template parmeter cached_map< string, dumb_storage> cms{ 256 }; cms. insert({ "prvni", "this is the first map item" }); auto it = cms. find("prvni"); . . *it. .
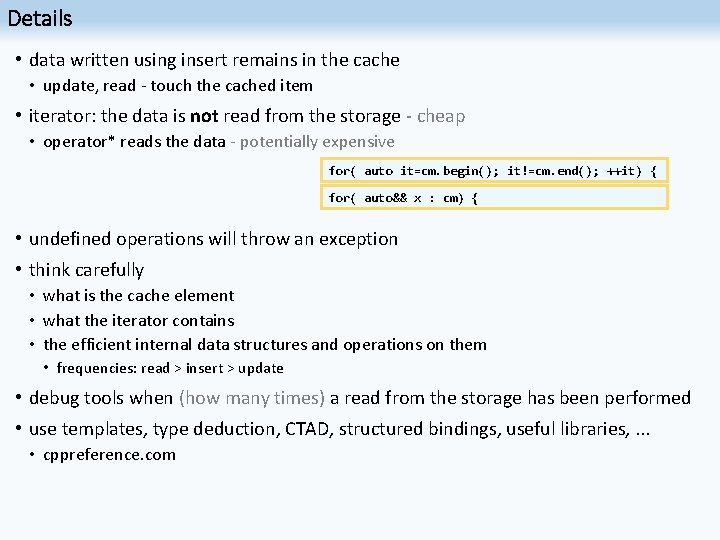
Details • data written using insert remains in the cache • update, read - touch the cached item • iterator: the data is not read from the storage - cheap • operator* reads the data - potentially expensive for( auto it=cm. begin(); it!=cm. end(); ++it) { for( auto&& x : cm) { • undefined operations will throw an exception • think carefully • what is the cache element • what the iterator contains • the efficient internal data structures and operations on them • frequencies: read > insert > update • debug tools when (how many times) a read from the storage has been performed • use templates, type deduction, CTAD, structured bindings, useful libraries, . . . • cppreference. com
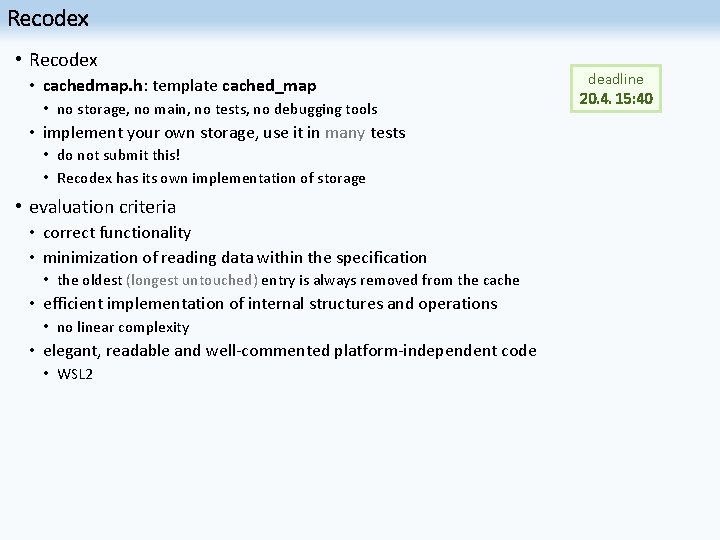
Recodex • cachedmap. h: template cached_map • no storage, no main, no tests, no debugging tools • implement your own storage, use it in many tests • do not submit this! • Recodex has its own implementation of storage • evaluation criteria • correct functionality • minimization of reading data within the specification • the oldest (longest untouched) entry is always removed from the cache • efficient implementation of internal structures and operations • no linear complexity • elegant, readable and well-commented platform-independent code • WSL 2 deadline 20. 4. 15: 40