Logical Operators and Precedence Mr Crone Logical Operators
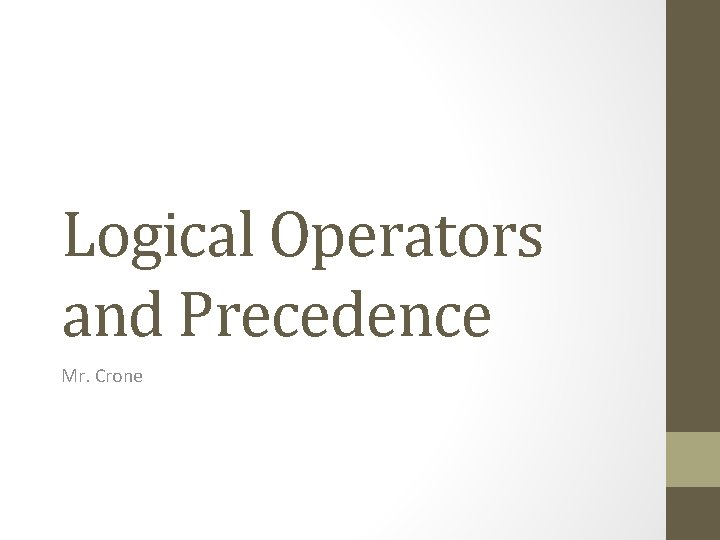
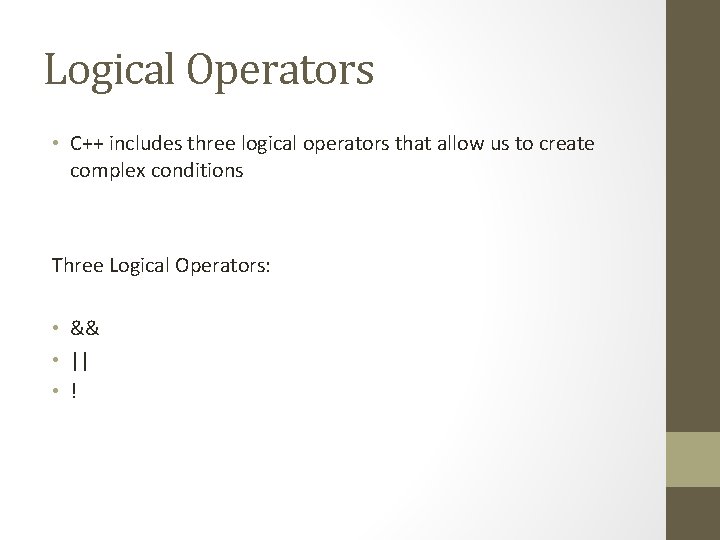
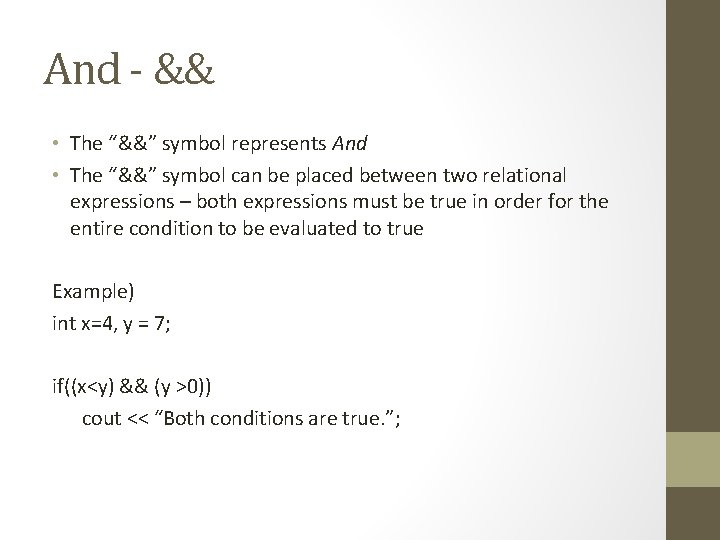
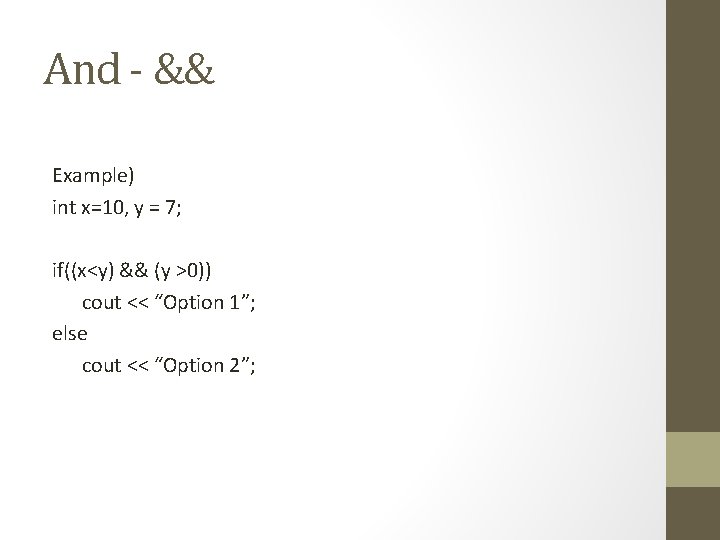
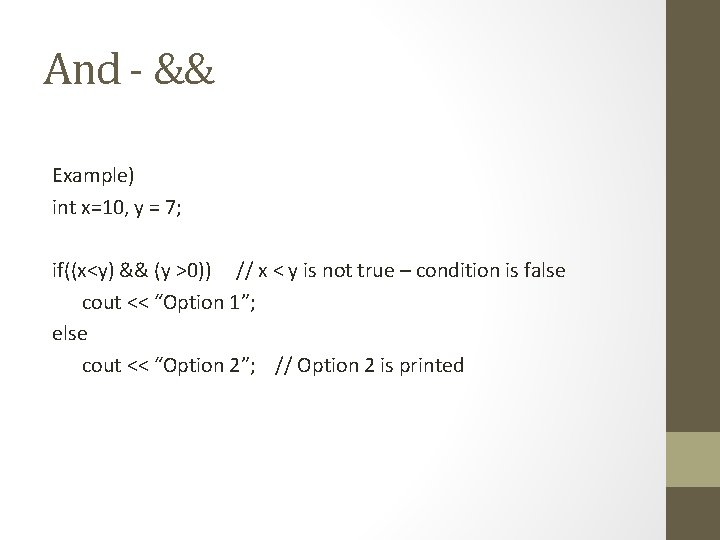
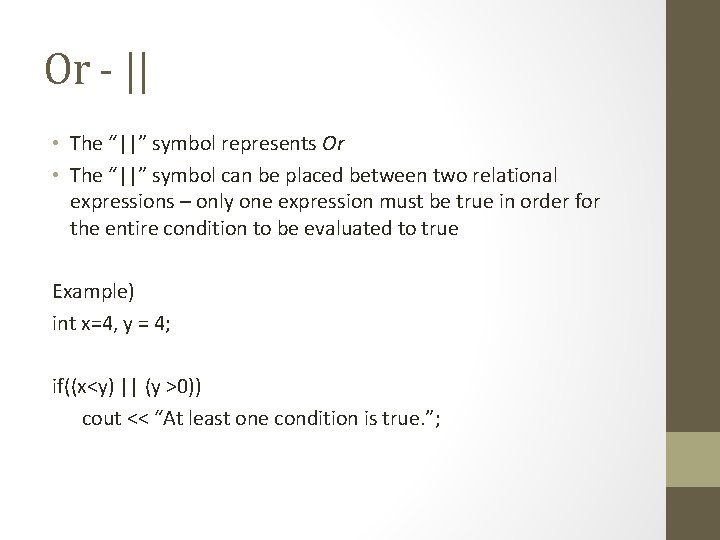
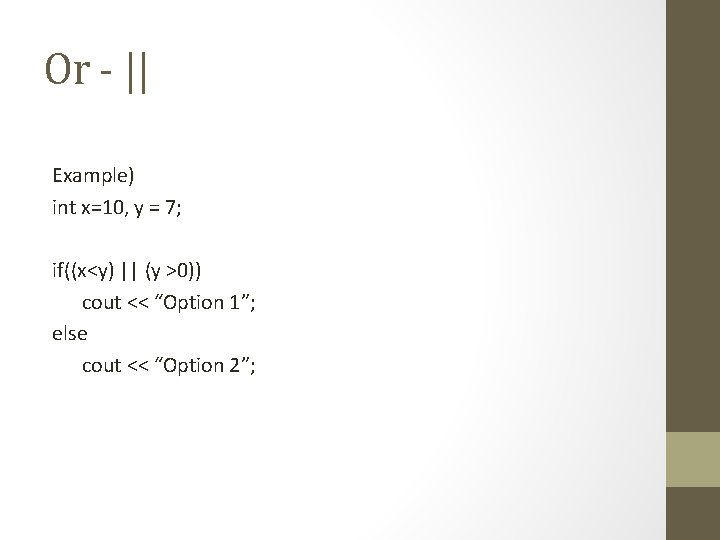
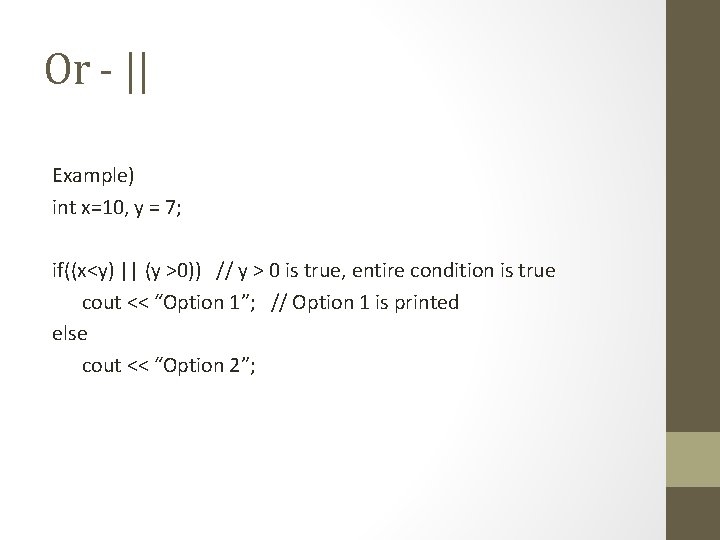
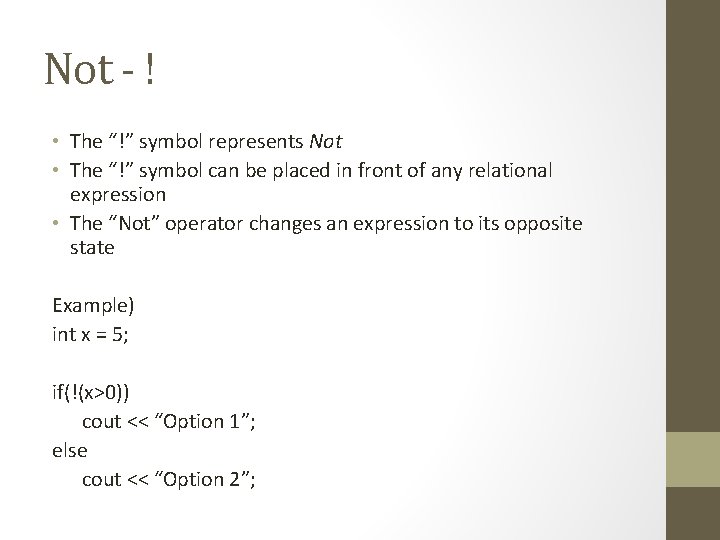
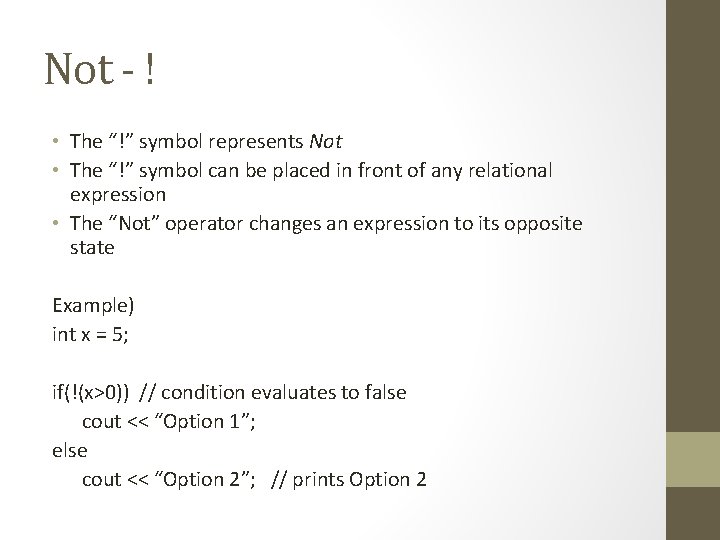
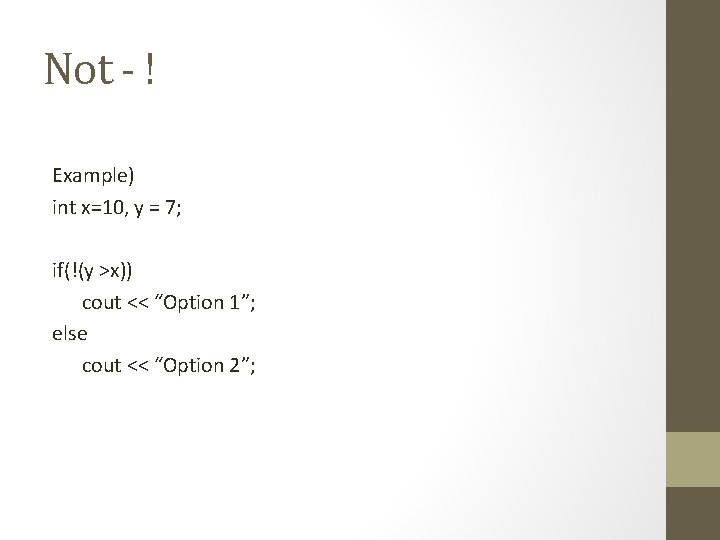
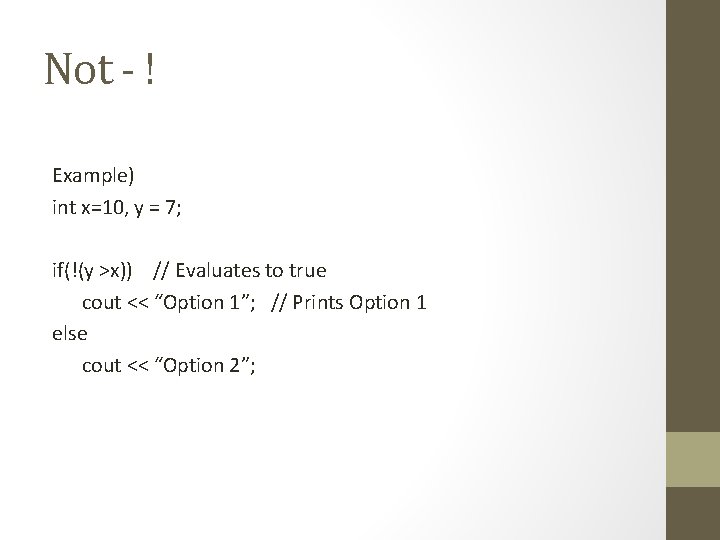
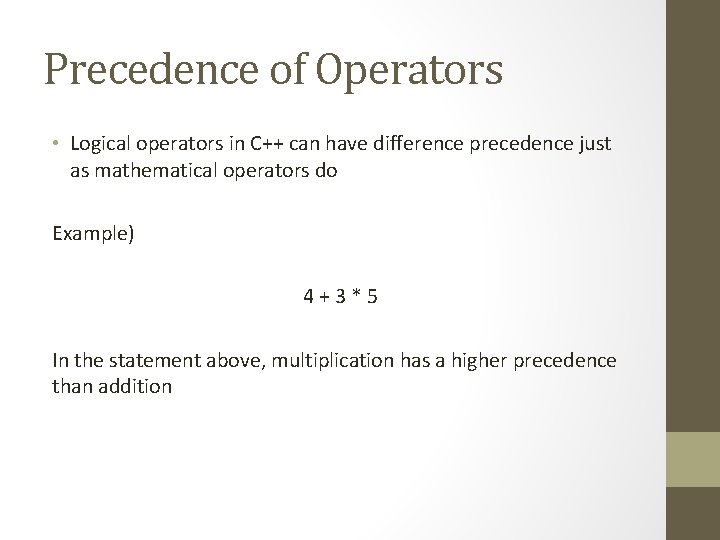
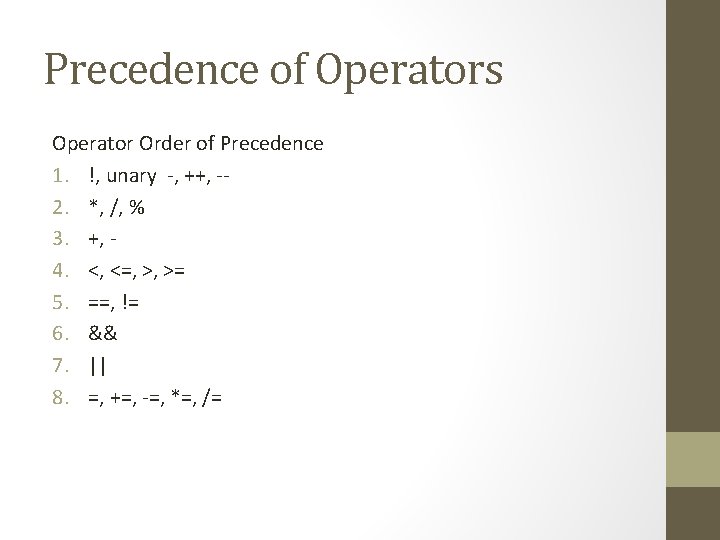
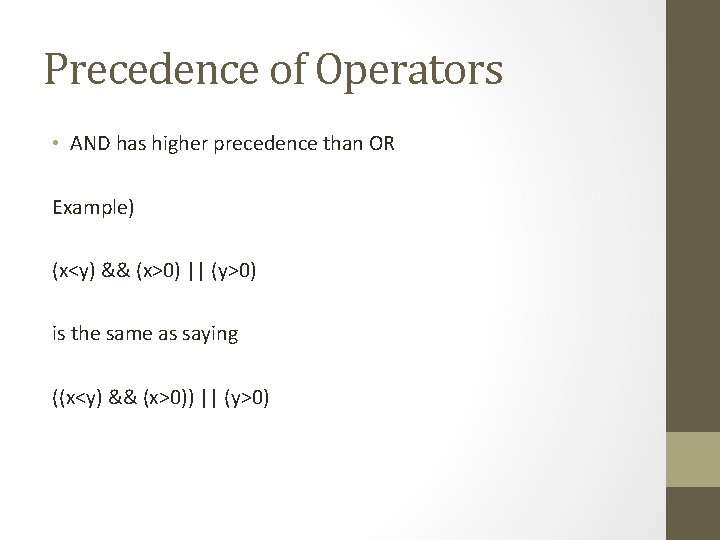
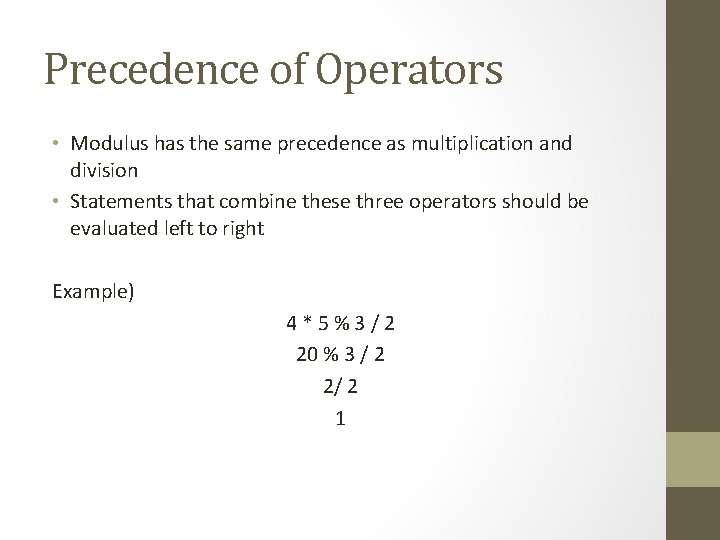
- Slides: 16
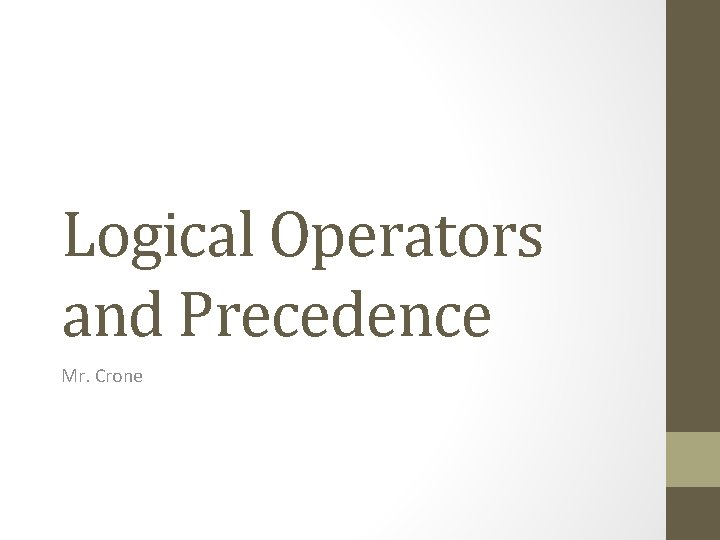
Logical Operators and Precedence Mr. Crone
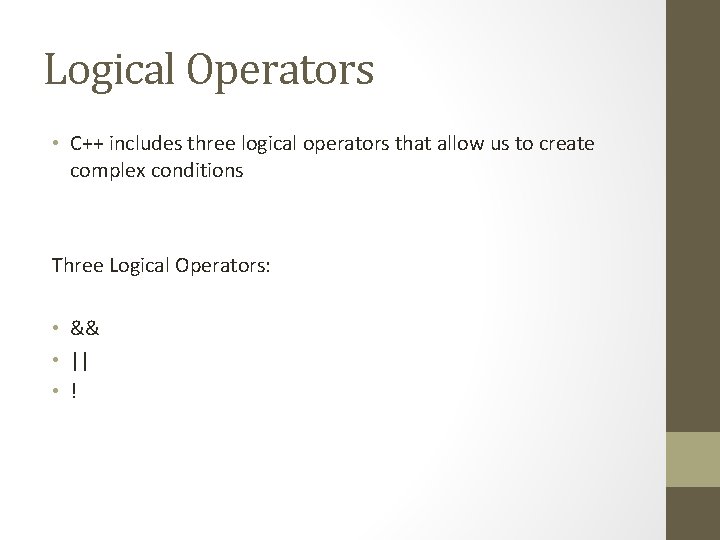
Logical Operators • C++ includes three logical operators that allow us to create complex conditions Three Logical Operators: • && • || • !
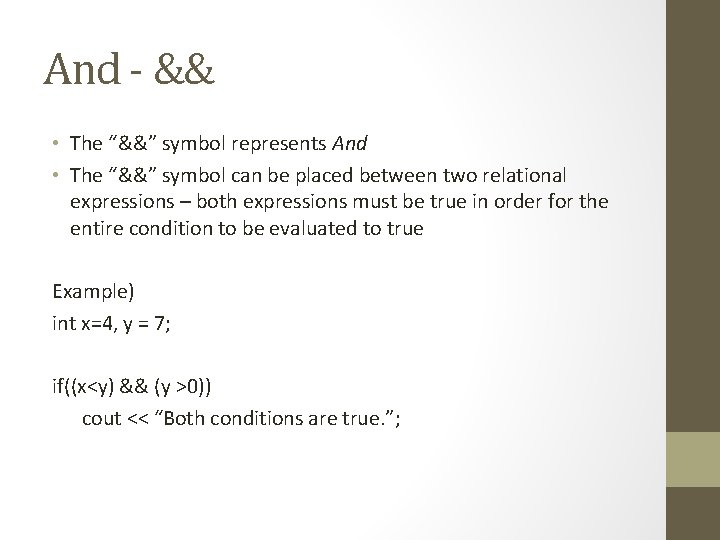
And - && • The “&&” symbol represents And • The “&&” symbol can be placed between two relational expressions – both expressions must be true in order for the entire condition to be evaluated to true Example) int x=4, y = 7; if((x<y) && (y >0)) cout << “Both conditions are true. ”;
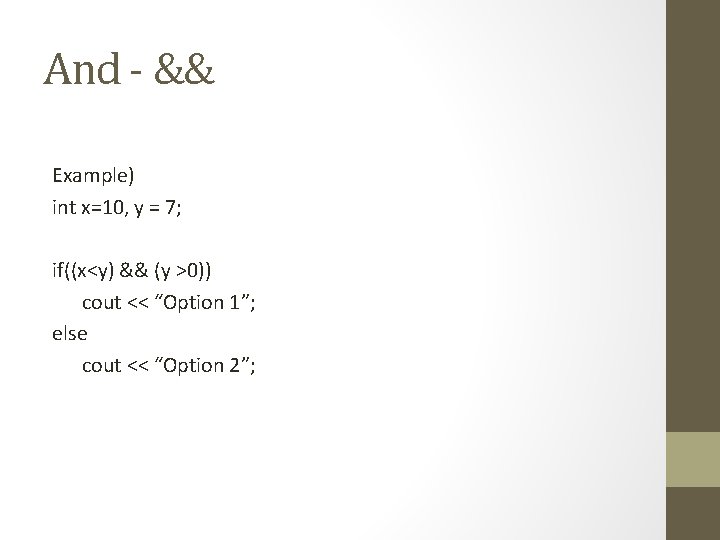
And - && Example) int x=10, y = 7; if((x<y) && (y >0)) cout << “Option 1”; else cout << “Option 2”;
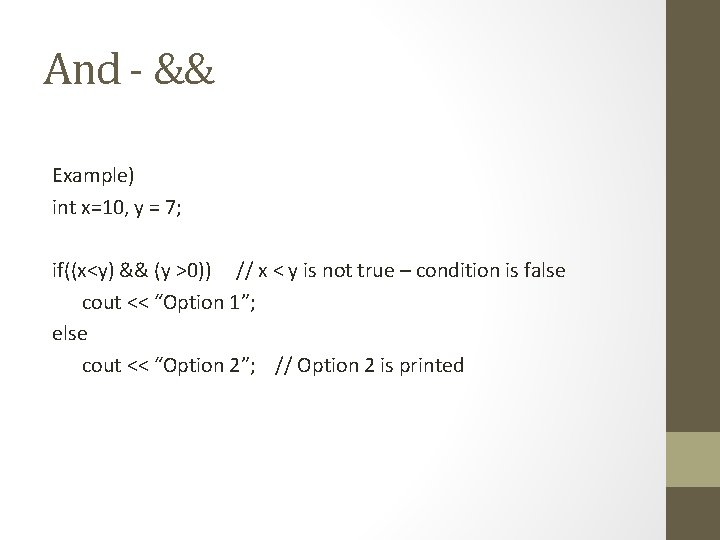
And - && Example) int x=10, y = 7; if((x<y) && (y >0)) // x < y is not true – condition is false cout << “Option 1”; else cout << “Option 2”; // Option 2 is printed
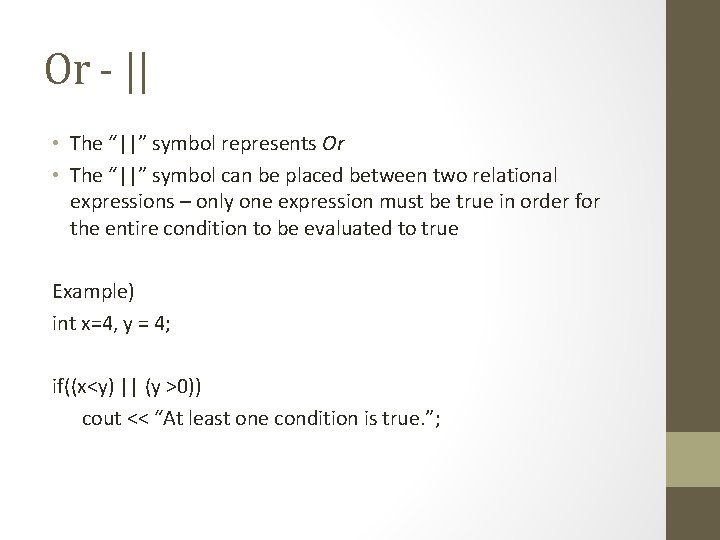
Or - || • The “||” symbol represents Or • The “||” symbol can be placed between two relational expressions – only one expression must be true in order for the entire condition to be evaluated to true Example) int x=4, y = 4; if((x<y) || (y >0)) cout << “At least one condition is true. ”;
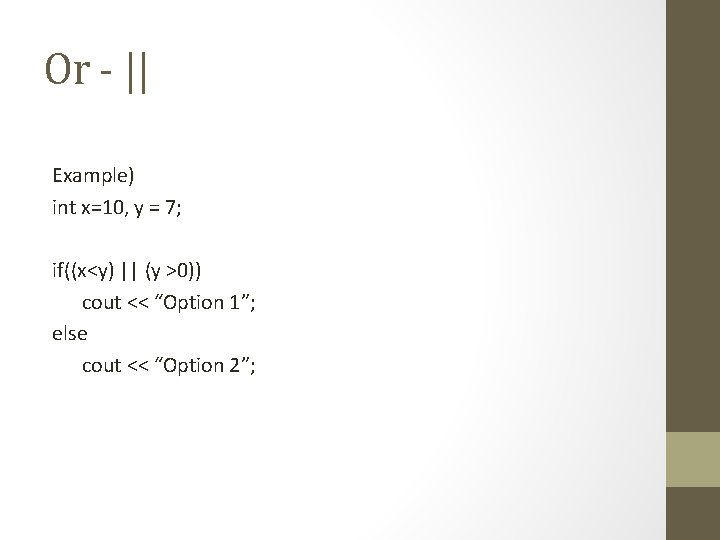
Or - || Example) int x=10, y = 7; if((x<y) || (y >0)) cout << “Option 1”; else cout << “Option 2”;
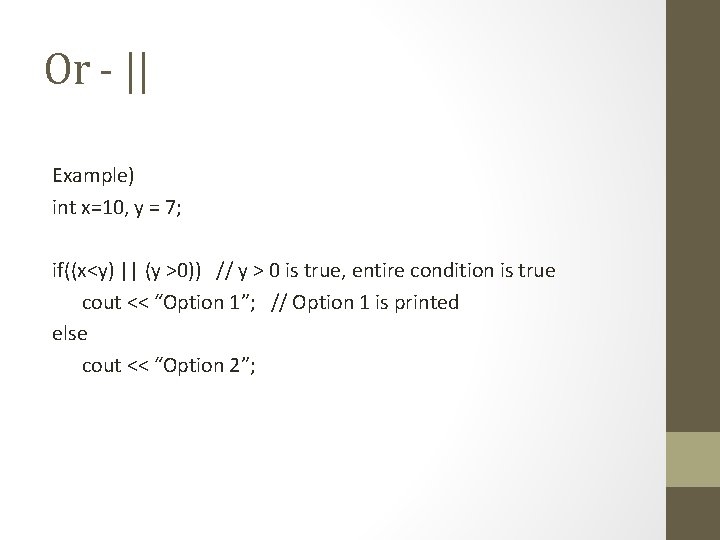
Or - || Example) int x=10, y = 7; if((x<y) || (y >0)) // y > 0 is true, entire condition is true cout << “Option 1”; // Option 1 is printed else cout << “Option 2”;
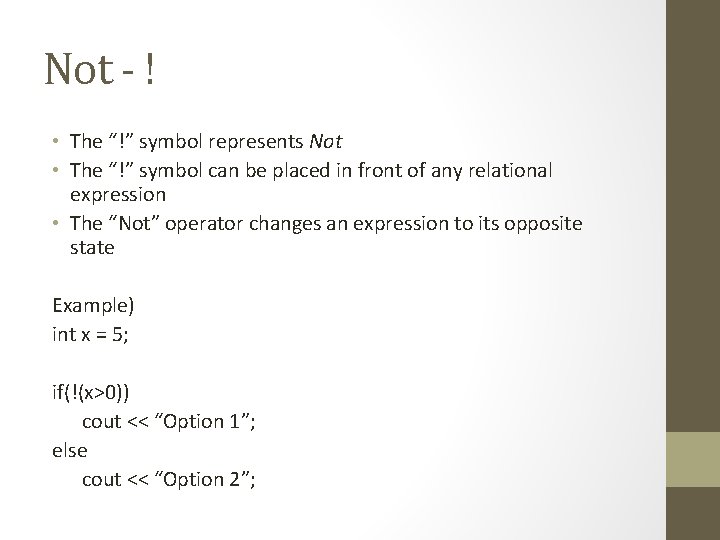
Not - ! • The “!” symbol represents Not • The “!” symbol can be placed in front of any relational expression • The “Not” operator changes an expression to its opposite state Example) int x = 5; if(!(x>0)) cout << “Option 1”; else cout << “Option 2”;
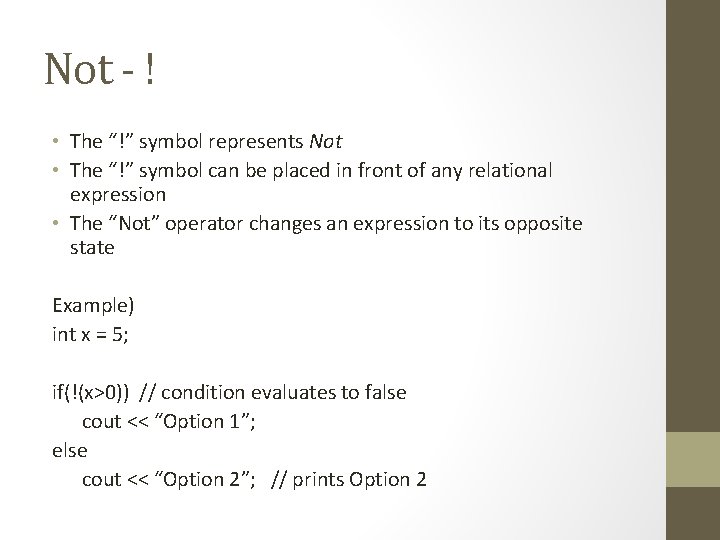
Not - ! • The “!” symbol represents Not • The “!” symbol can be placed in front of any relational expression • The “Not” operator changes an expression to its opposite state Example) int x = 5; if(!(x>0)) // condition evaluates to false cout << “Option 1”; else cout << “Option 2”; // prints Option 2
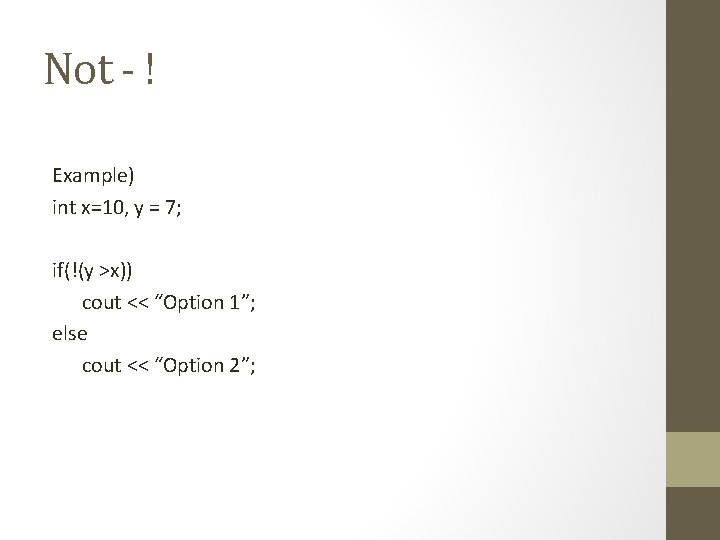
Not - ! Example) int x=10, y = 7; if(!(y >x)) cout << “Option 1”; else cout << “Option 2”;
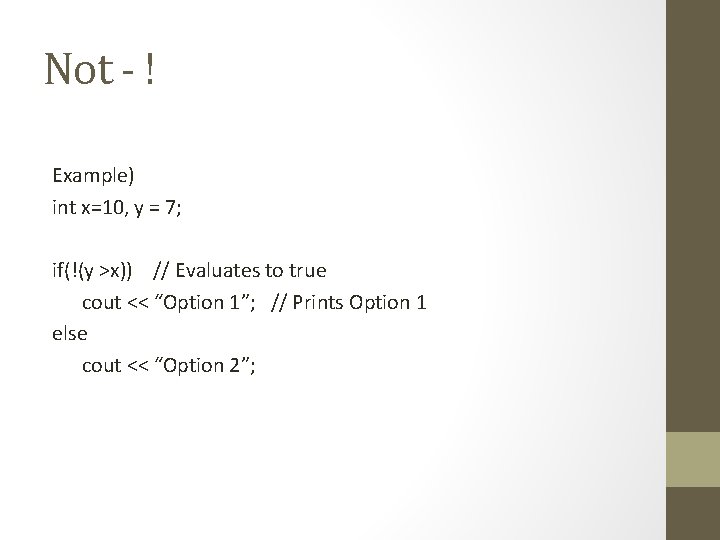
Not - ! Example) int x=10, y = 7; if(!(y >x)) // Evaluates to true cout << “Option 1”; // Prints Option 1 else cout << “Option 2”;
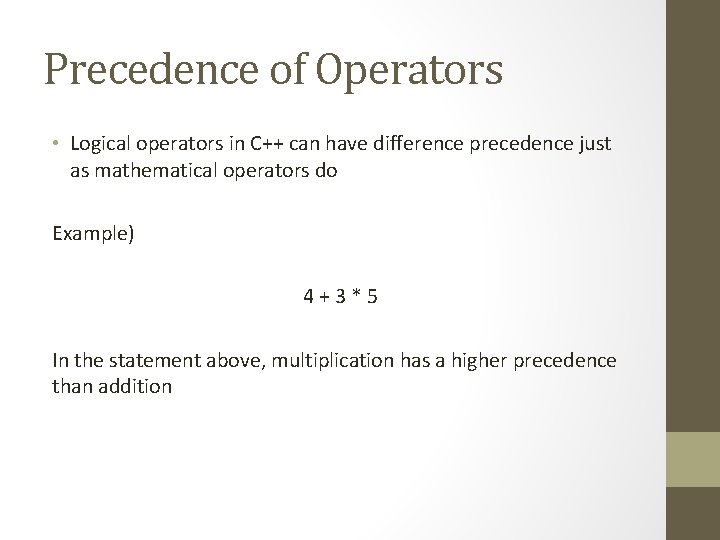
Precedence of Operators • Logical operators in C++ can have difference precedence just as mathematical operators do Example) 4+3*5 In the statement above, multiplication has a higher precedence than addition
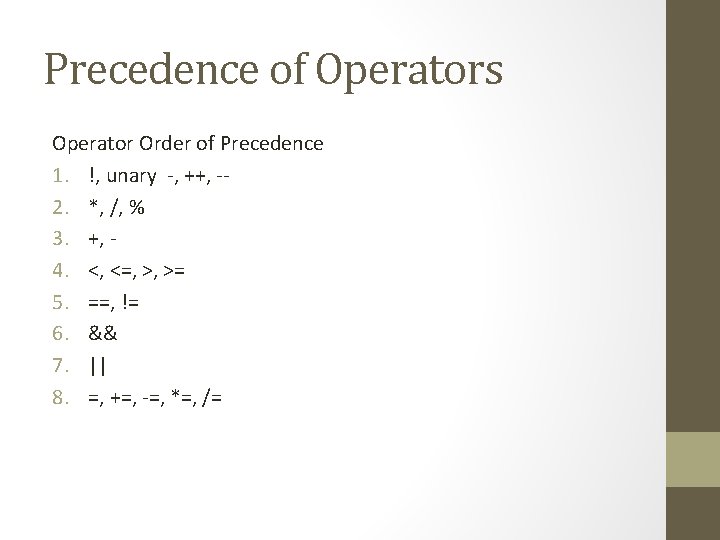
Precedence of Operators Operator Order of Precedence 1. !, unary -, ++, -2. *, /, % 3. +, 4. <, <=, >, >= 5. ==, != 6. && 7. || 8. =, +=, -=, *=, /=
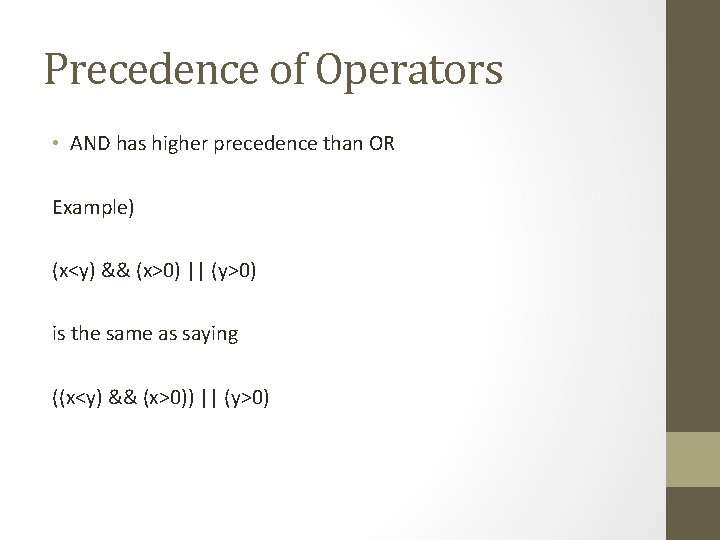
Precedence of Operators • AND has higher precedence than OR Example) (x<y) && (x>0) || (y>0) is the same as saying ((x<y) && (x>0)) || (y>0)
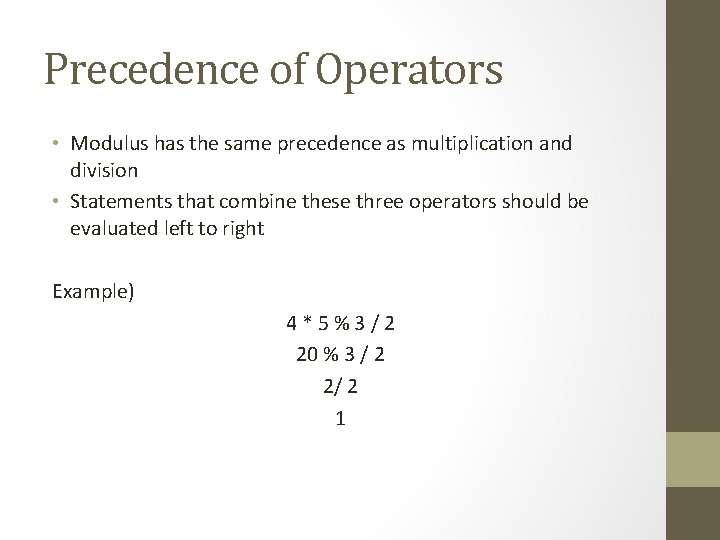
Precedence of Operators • Modulus has the same precedence as multiplication and division • Statements that combine these three operators should be evaluated left to right Example) 4*5%3/2 20 % 3 / 2 2/ 2 1
Precedence of operators in python
Precedence of operators
Andy crone
Patricia crone hagarism
Ssvf university webinars
Rational and logical operators
Matlab logical operators
Logical operators truth table
Logical operators quiz
Logical operators calculator
Pl sql operators
Prolog and or operators
Logical operators exercises
Logical operators priority in c
What are the boolean operators
Logical form examples
Google operands