Lecturers slides http www comp nus edu sgcs
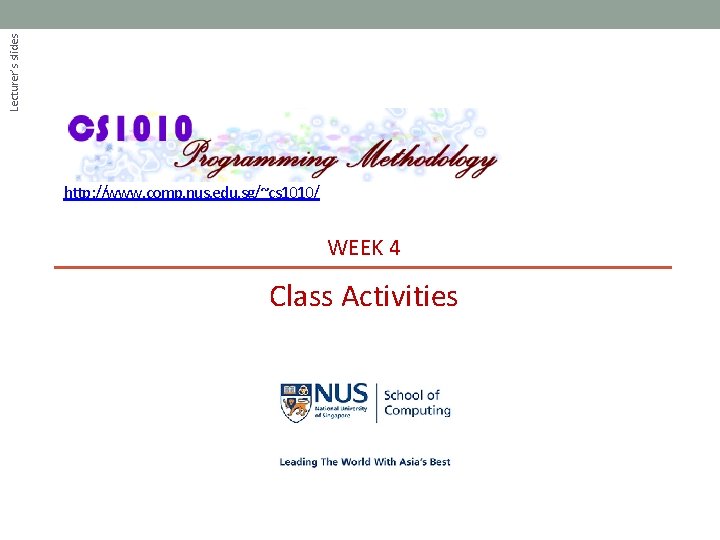
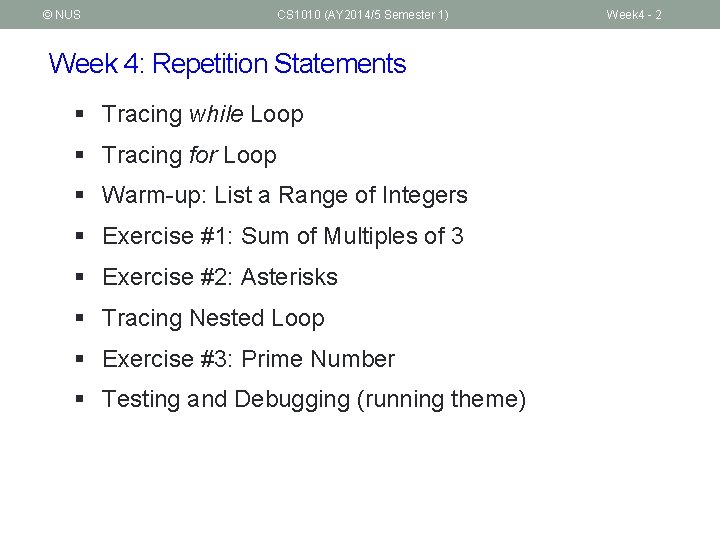
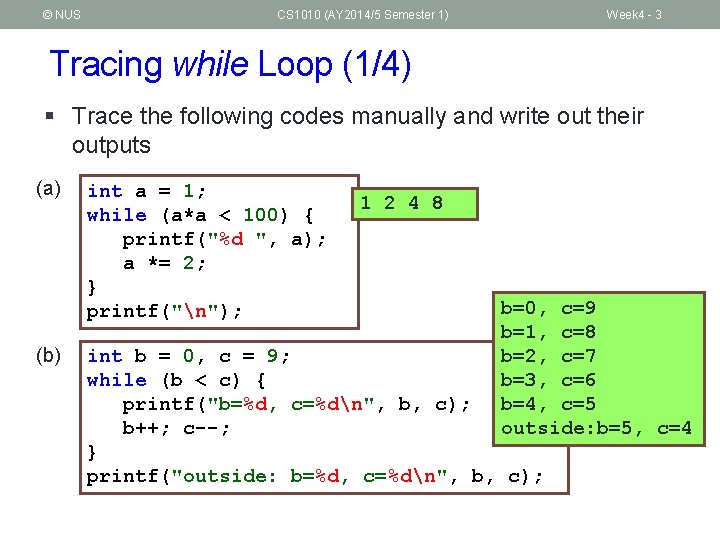
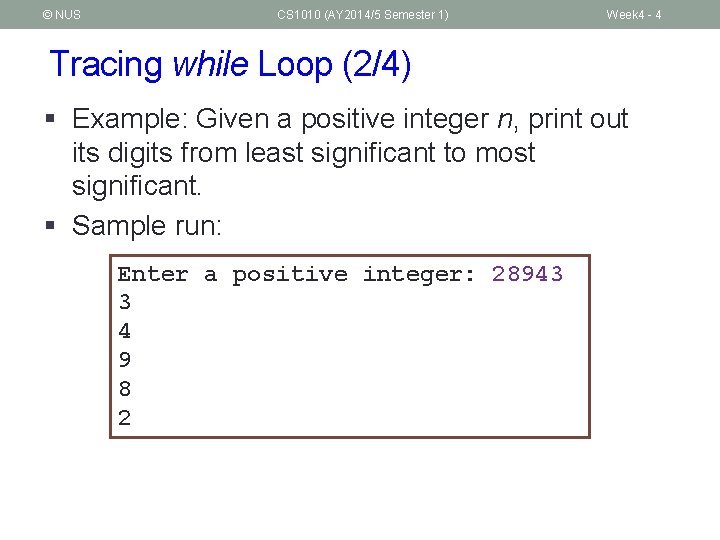
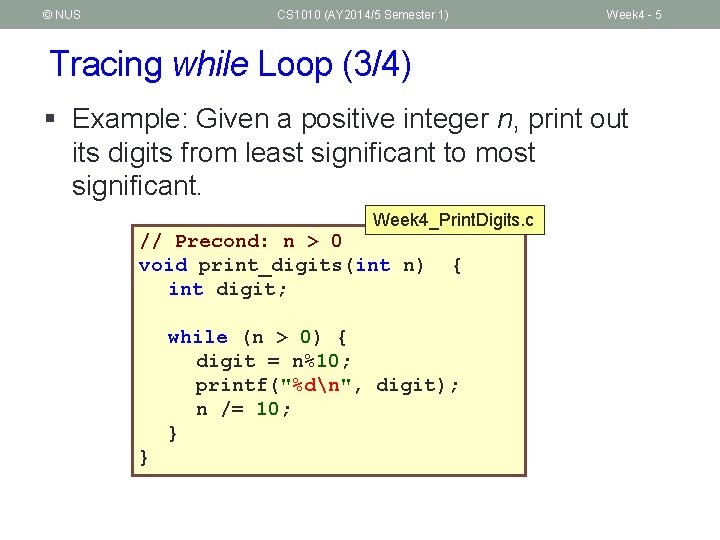
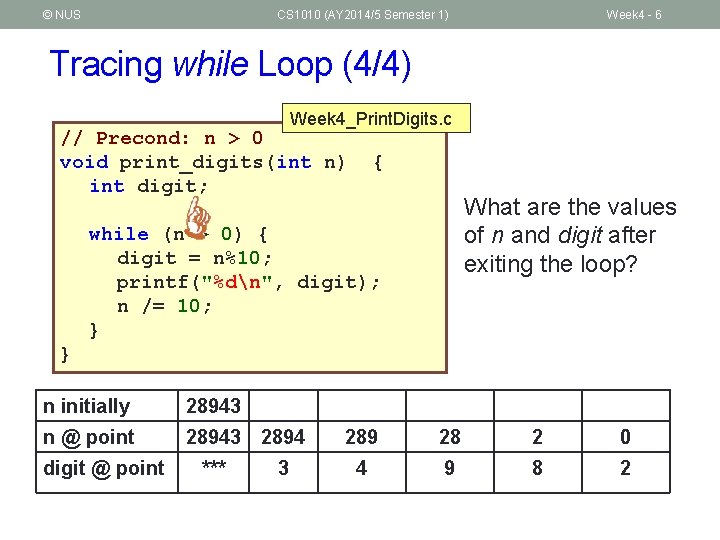
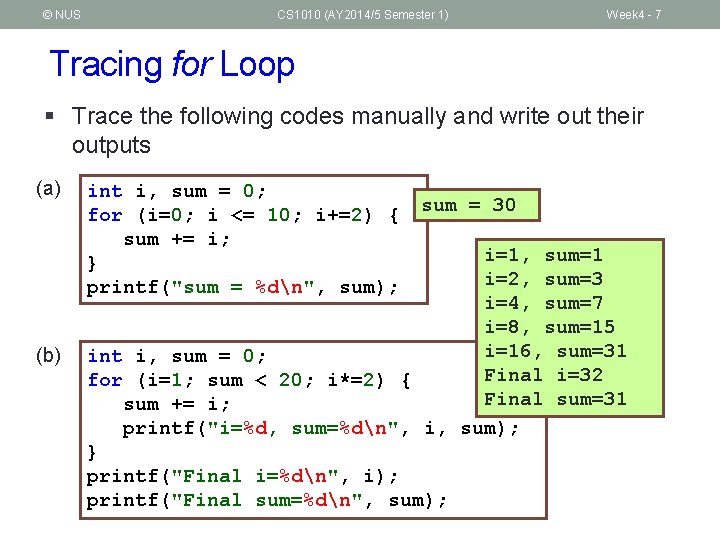
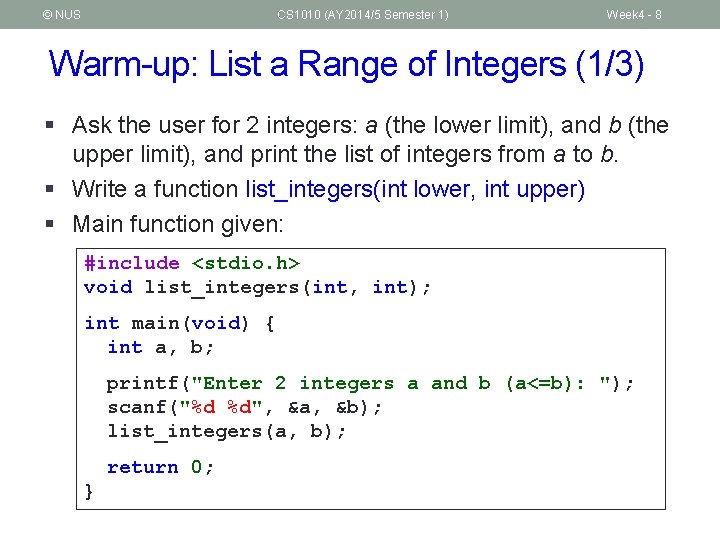
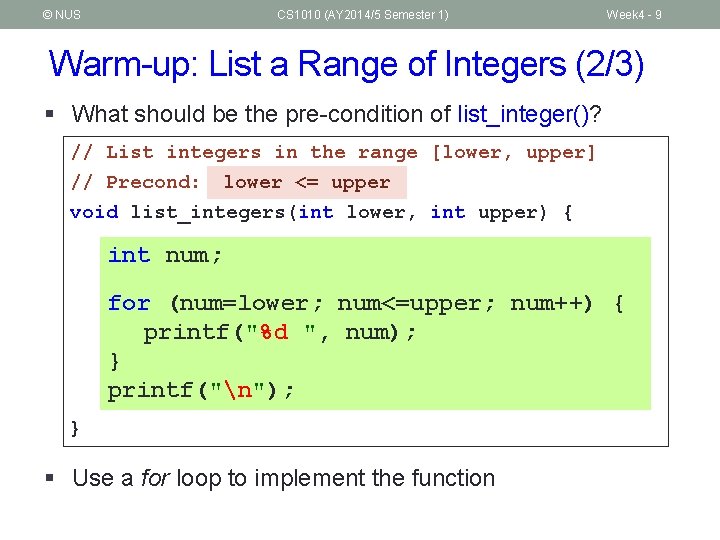
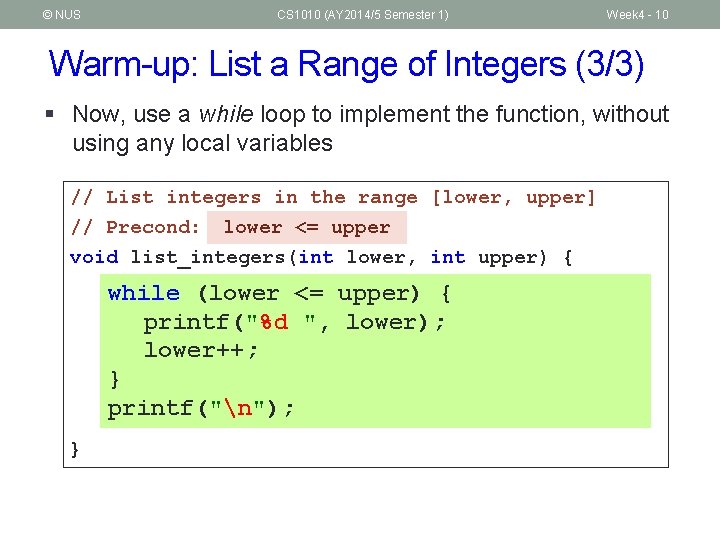
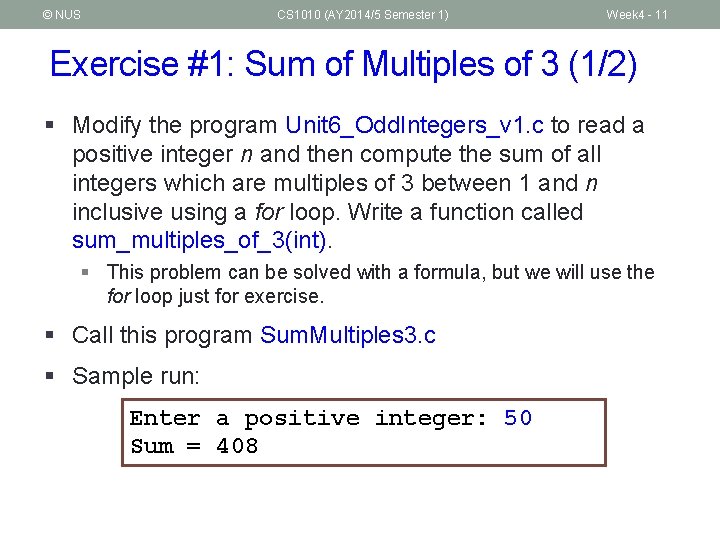
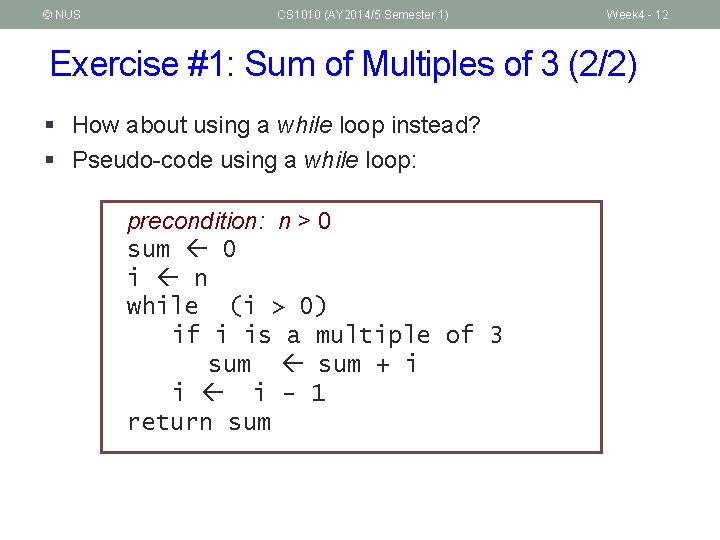
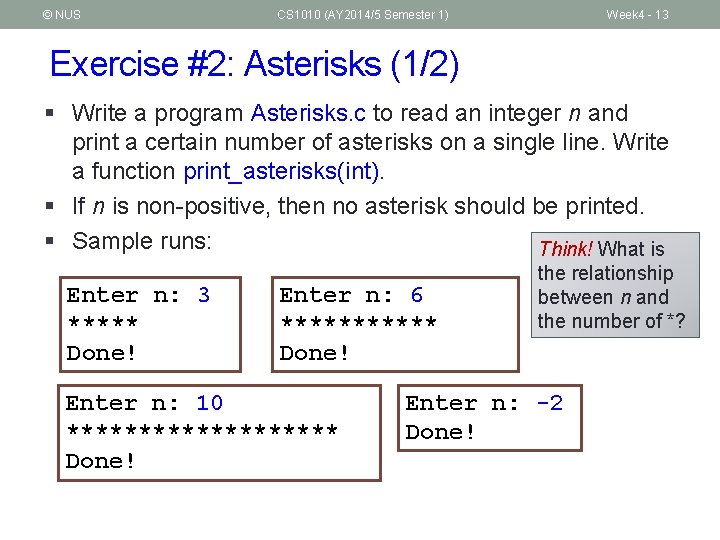
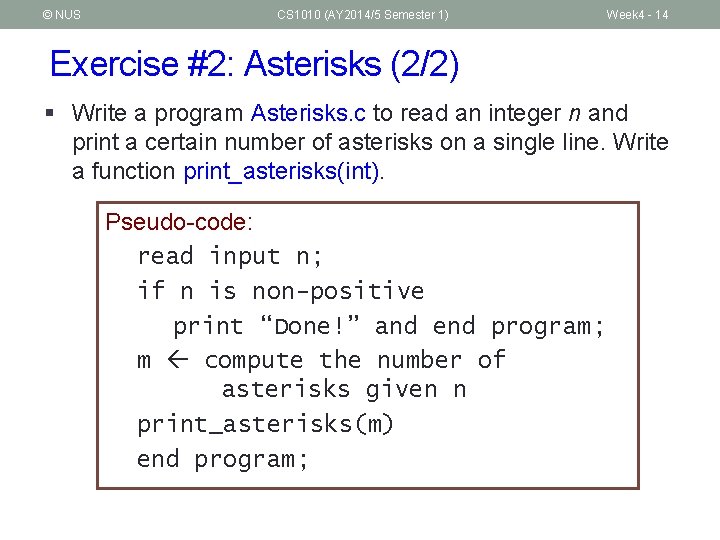
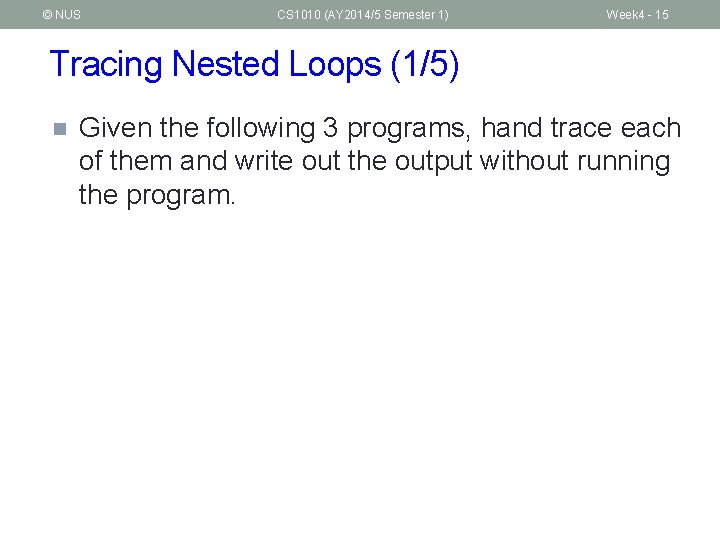
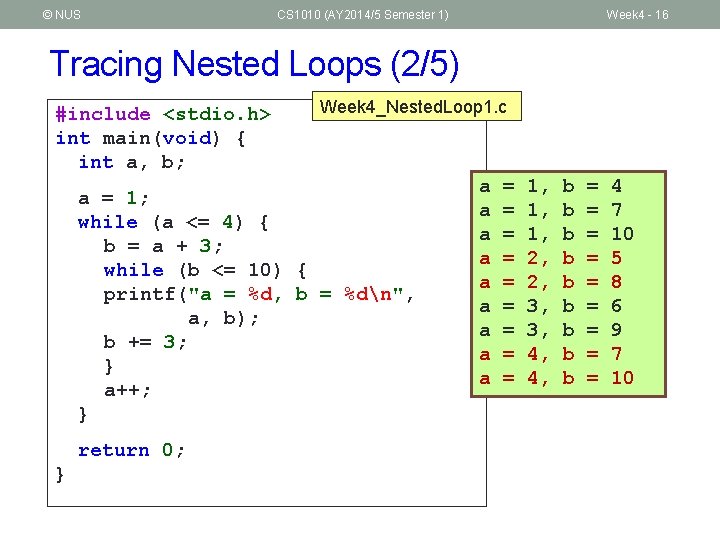
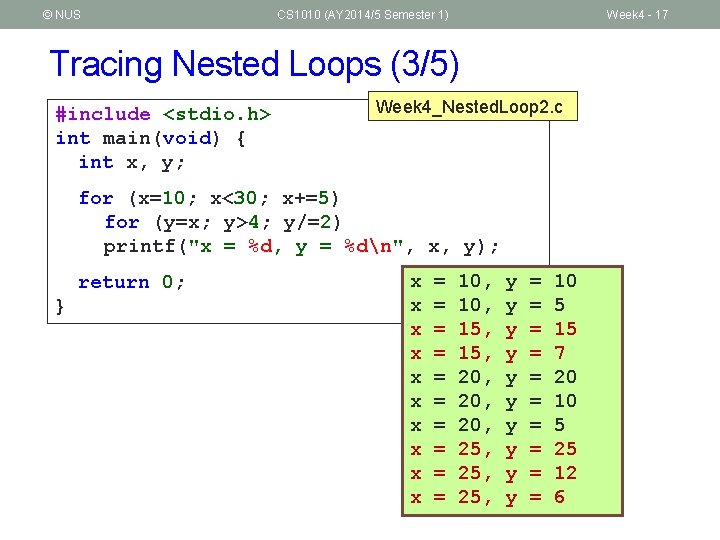
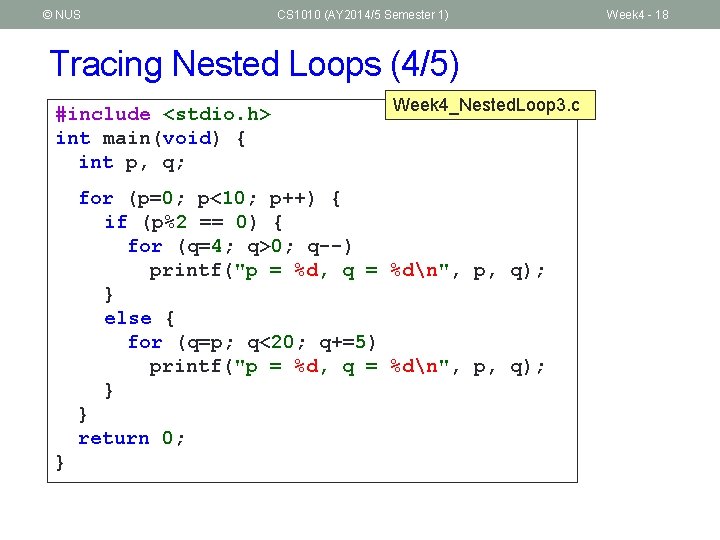
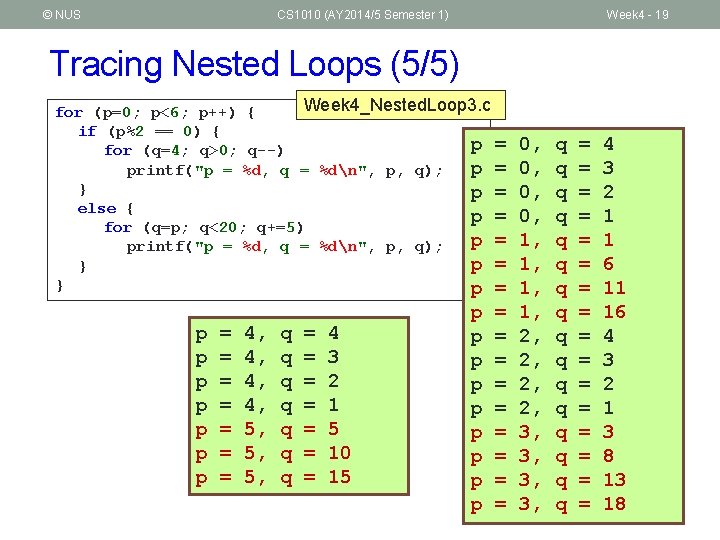
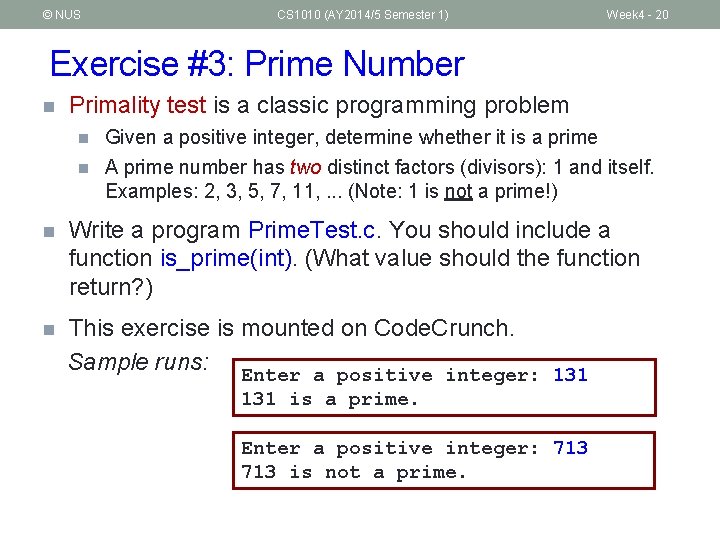
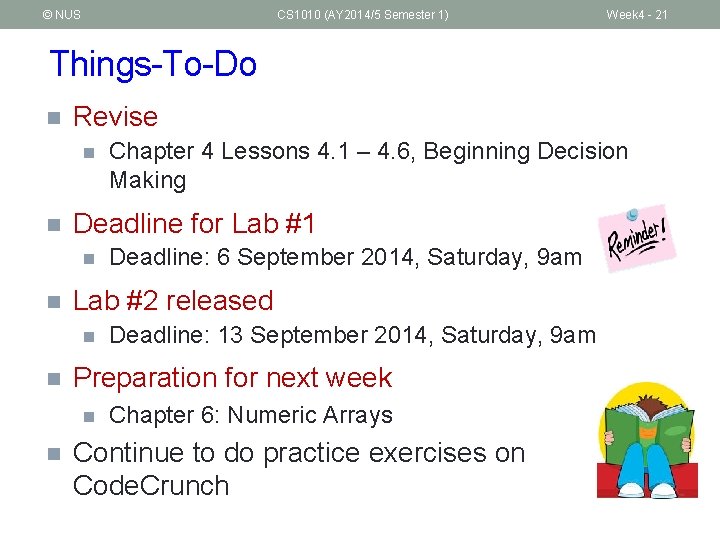
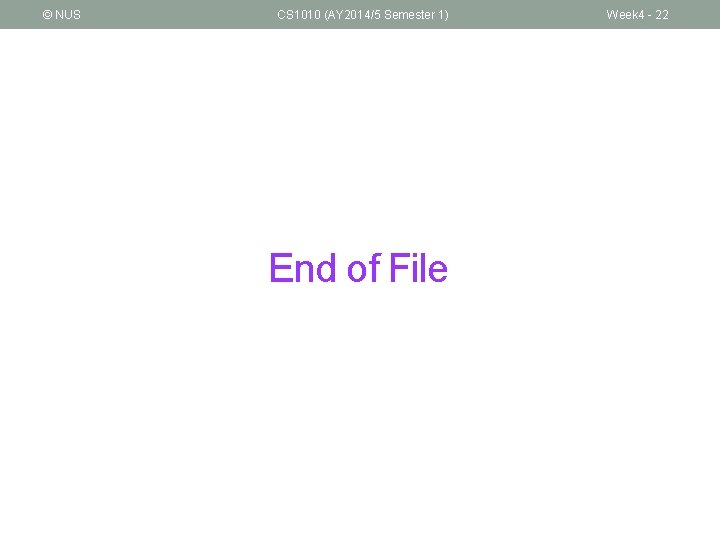
- Slides: 22
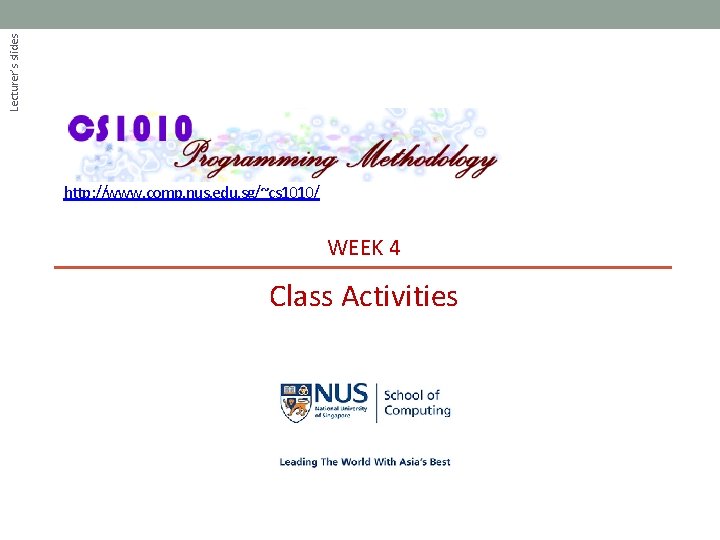
Lecturer’s slides http: //www. comp. nus. edu. sg/~cs 1010/ WEEK 4 Class Activities
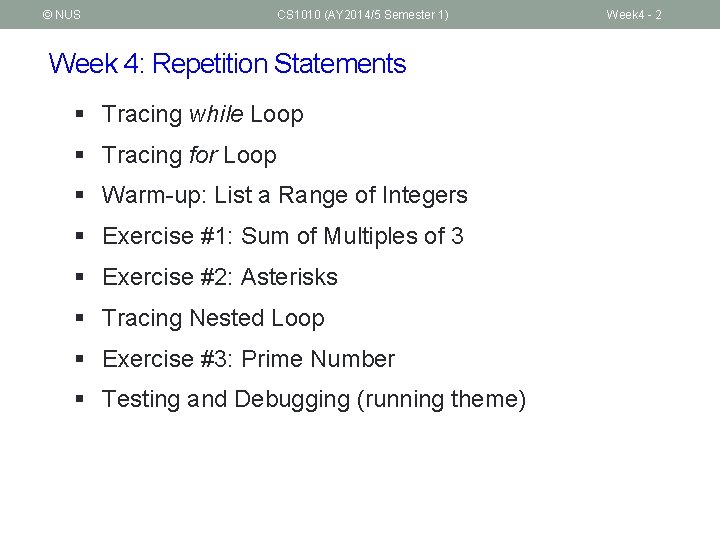
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4: Repetition Statements § Tracing while Loop § Tracing for Loop § Warm-up: List a Range of Integers § Exercise #1: Sum of Multiples of 3 § Exercise #2: Asterisks § Tracing Nested Loop § Exercise #3: Prime Number § Testing and Debugging (running theme) Week 4 - 2
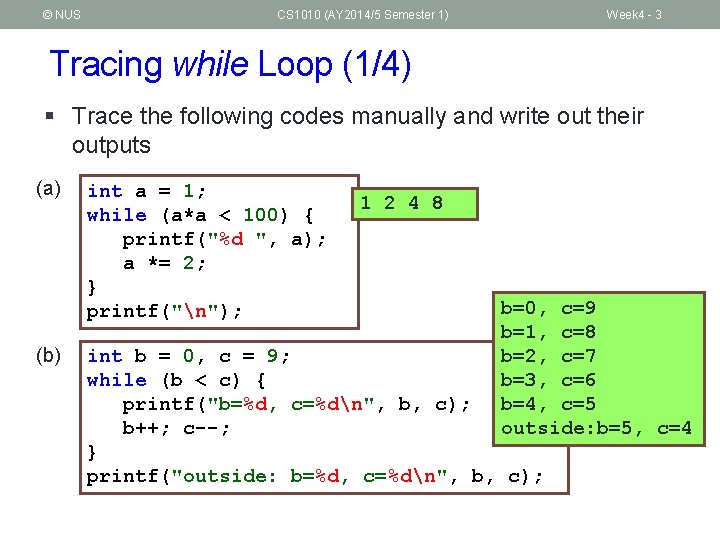
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 3 Tracing while Loop (1/4) § Trace the following codes manually and write out their outputs (a) (b) int a = 1; while (a*a < 100) { printf("%d ", a); a *= 2; } printf("n"); 1 2 4 8 b=0, c=9 b=1, c=8 b=2, c=7 b=3, c=6 b=4, c=5 outside: b=5, c=4 int b = 0, c = 9; while (b < c) { printf("b=%d, c=%dn", b, c); b++; c--; } printf("outside: b=%d, c=%dn", b, c);
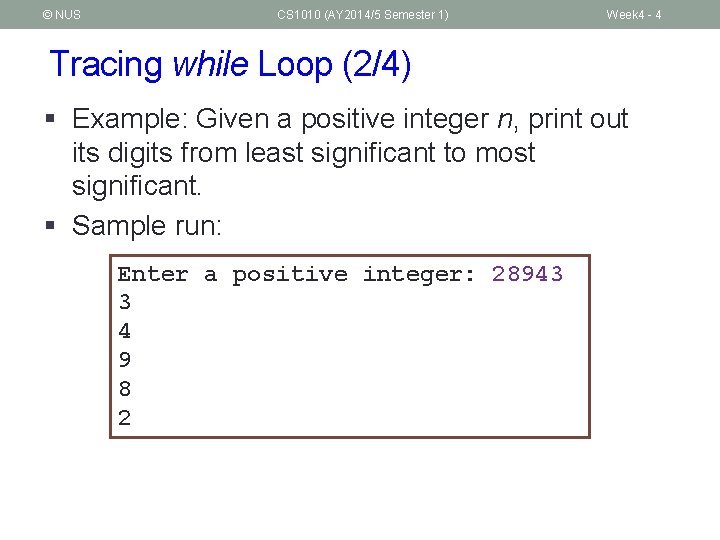
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 4 Tracing while Loop (2/4) § Example: Given a positive integer n, print out its digits from least significant to most significant. § Sample run: Enter a positive integer: 28943 3 4 9 8 2
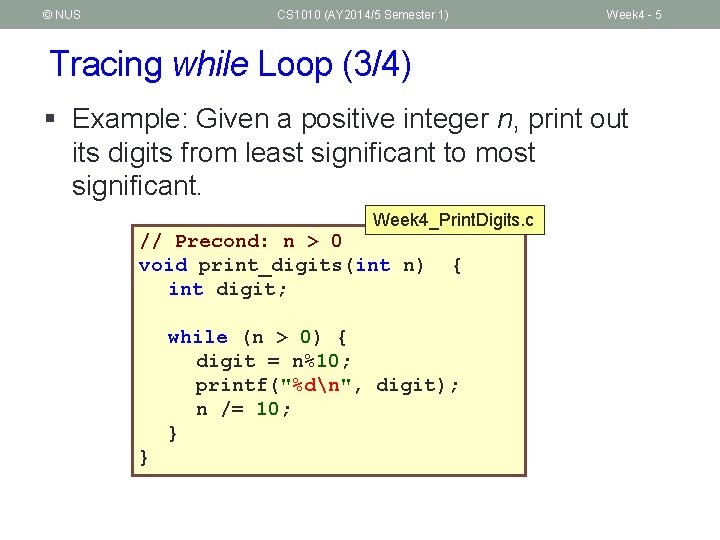
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 5 Tracing while Loop (3/4) § Example: Given a positive integer n, print out its digits from least significant to most significant. Week 4_Print. Digits. c // Precond: n > 0 void print_digits(int n) int digit; { while (n > 0) { digit = n%10; printf("%dn", digit); n /= 10; } }
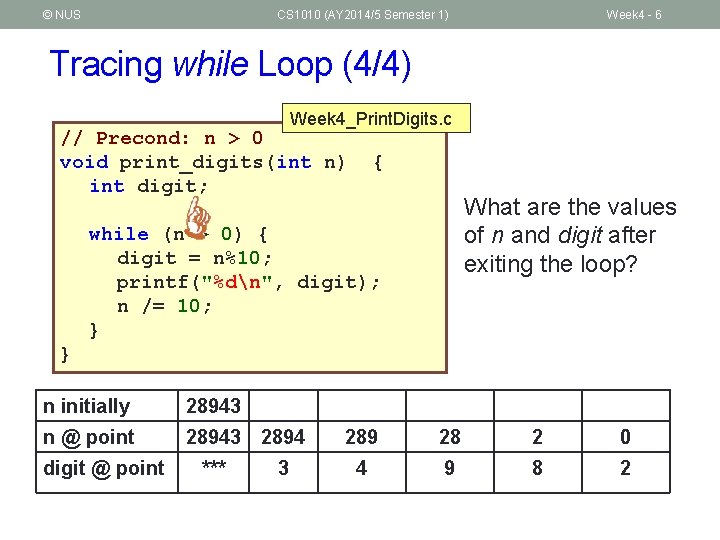
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 6 Tracing while Loop (4/4) Week 4_Print. Digits. c // Precond: n > 0 void print_digits(int n) int digit; { What are the values of n and digit after exiting the loop? while (n > 0) { digit = n%10; printf("%dn", digit); n /= 10; } } n initially 28943 n @ point 28943 2894 digit @ point *** 3 289 28 2 0 4 9 8 2
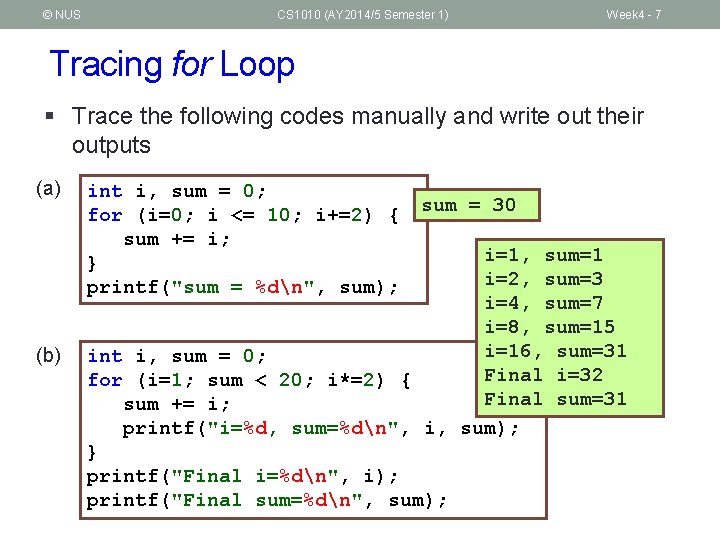
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 7 Tracing for Loop § Trace the following codes manually and write out their outputs (a) (b) int i, sum = 0; for (i=0; i <= 10; i+=2) { sum += i; } printf("sum = %dn", sum); sum = 30 i=1, sum=1 i=2, sum=3 i=4, sum=7 i=8, sum=15 i=16, sum=31 int i, sum = 0; Final i=32 for (i=1; sum < 20; i*=2) { Final sum=31 sum += i; printf("i=%d, sum=%dn", i, sum); } printf("Final i=%dn", i); printf("Final sum=%dn", sum);
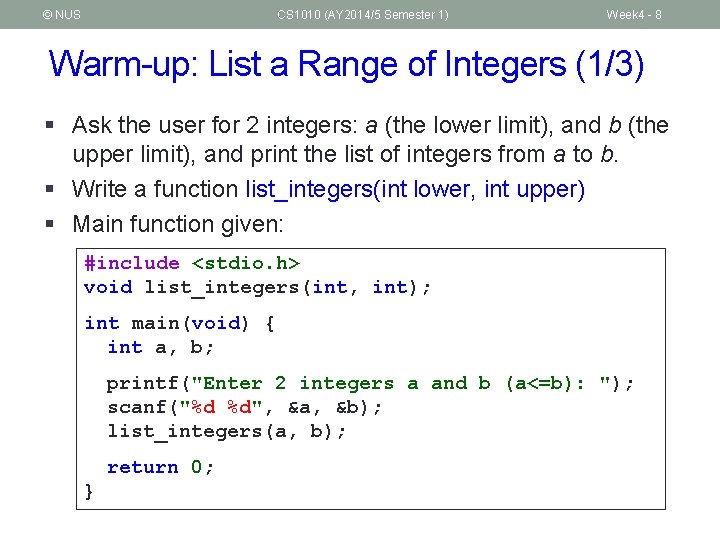
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 8 Warm-up: List a Range of Integers (1/3) § Ask the user for 2 integers: a (the lower limit), and b (the upper limit), and print the list of integers from a to b. § Write a function list_integers(int lower, int upper) § Main function given: #include <stdio. h> void list_integers(int, int); int main(void) { int a, b; printf("Enter 2 integers a and b (a<=b): "); scanf("%d %d", &a, &b); list_integers(a, b); return 0; }
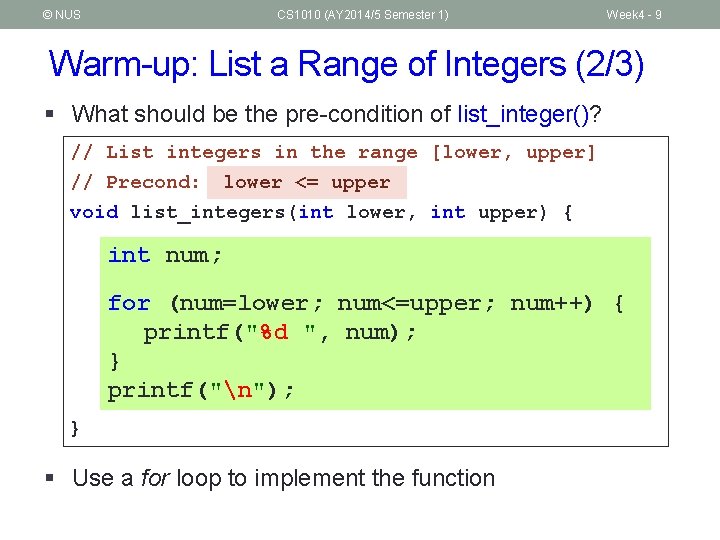
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 9 Warm-up: List a Range of Integers (2/3) § What should be the pre-condition of list_integer()? // List integers in the range [lower, upper] // Precond: lower <= upper void list_integers(int lower, int upper) { int num; . . . for (num=lower; num<=upper; num++) { printf("%d ", num); } printf("n"); } § Use a for loop to implement the function
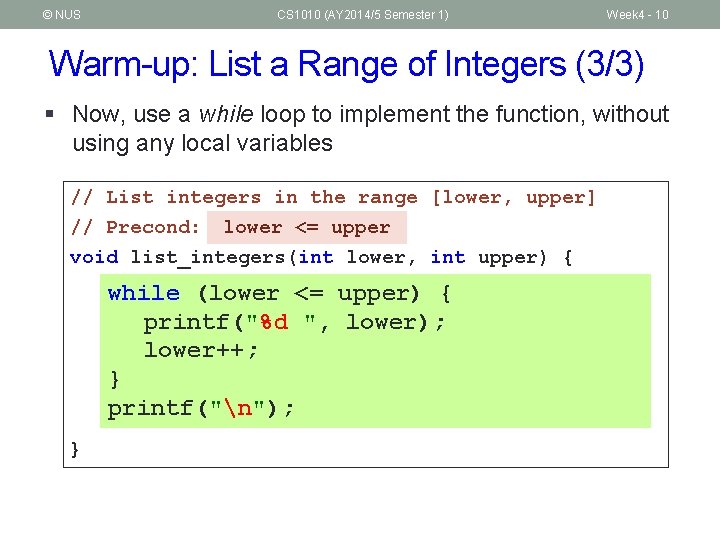
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 10 Warm-up: List a Range of Integers (3/3) § Now, use a while loop to implement the function, without using any local variables // List integers in the range [lower, upper] // Precond: lower <= upper void list_integers(int lower, int upper) { while (lower <= upper) {. . . printf("%d ", lower); lower++; } printf("n"); }
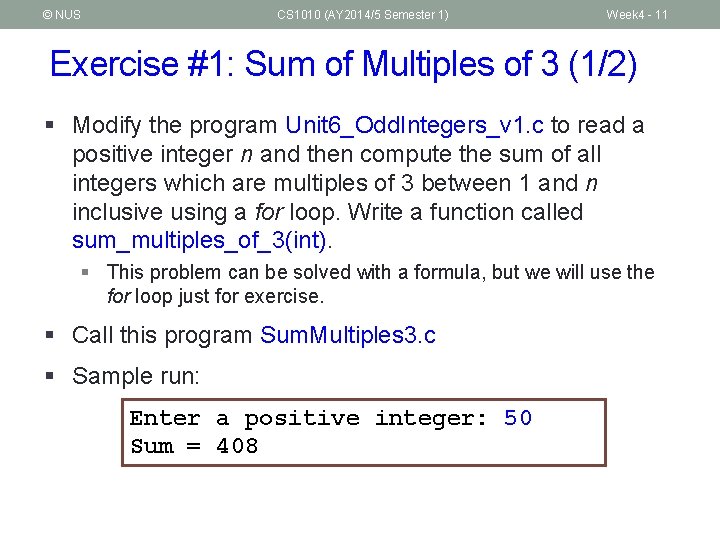
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 11 Exercise #1: Sum of Multiples of 3 (1/2) § Modify the program Unit 6_Odd. Integers_v 1. c to read a positive integer n and then compute the sum of all integers which are multiples of 3 between 1 and n inclusive using a for loop. Write a function called sum_multiples_of_3(int). § This problem can be solved with a formula, but we will use the for loop just for exercise. § Call this program Sum. Multiples 3. c § Sample run: Enter a positive integer: 50 Sum = 408
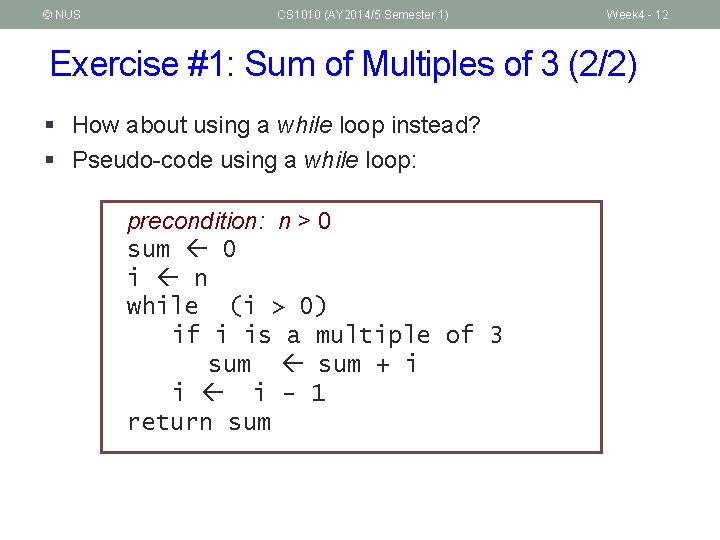
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 12 Exercise #1: Sum of Multiples of 3 (2/2) § How about using a while loop instead? § Pseudo-code using a while loop: precondition: n > 0 sum 0 i n while (i > 0) if i is a multiple of 3 sum + i i i - 1 return sum
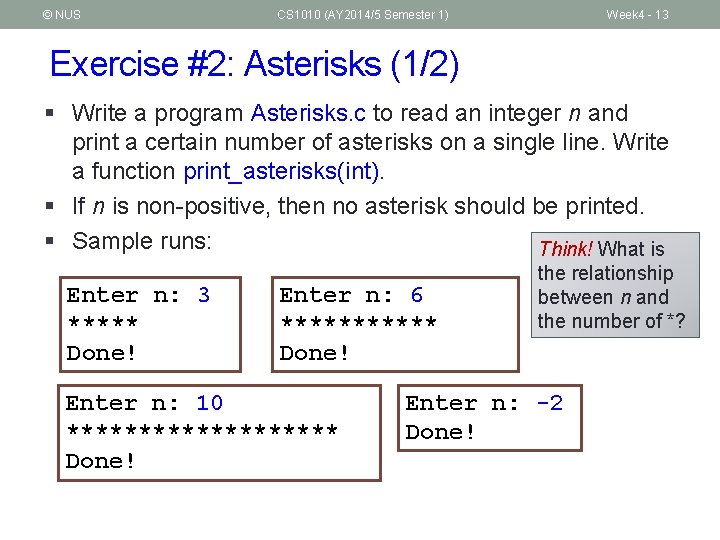
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 13 Exercise #2: Asterisks (1/2) § Write a program Asterisks. c to read an integer n and print a certain number of asterisks on a single line. Write a function print_asterisks(int). § If n is non-positive, then no asterisk should be printed. § Sample runs: Think! What is Enter n: 3 ***** Done! Enter n: 6 ****** Done! Enter n: 10 ********** Done! the relationship between n and the number of *? Enter n: -2 Done!
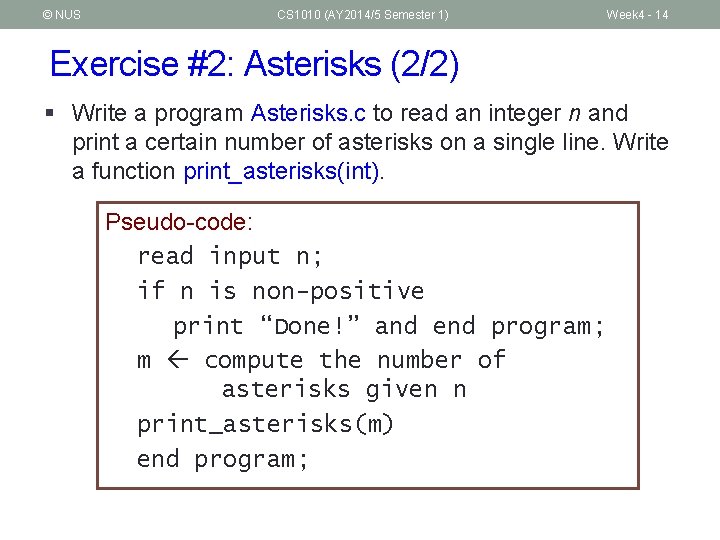
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 14 Exercise #2: Asterisks (2/2) § Write a program Asterisks. c to read an integer n and print a certain number of asterisks on a single line. Write a function print_asterisks(int). Pseudo-code: read input n; if n is non-positive print “Done!” and end program; m compute the number of asterisks given n print_asterisks(m) end program;
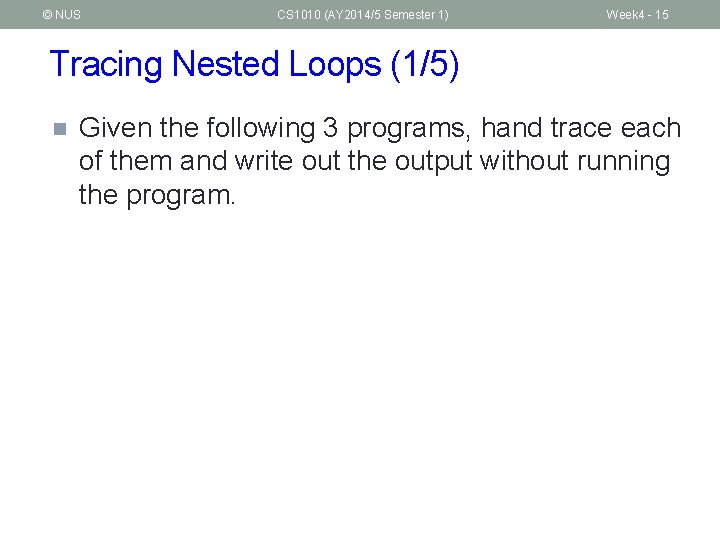
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 15 Tracing Nested Loops (1/5) n Given the following 3 programs, hand trace each of them and write out the output without running the program.
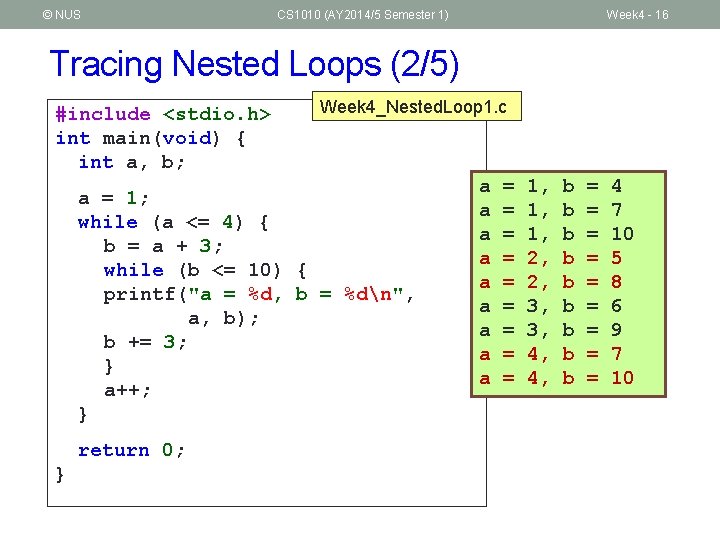
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 16 Tracing Nested Loops (2/5) #include <stdio. h> int main(void) { int a, b; Week 4_Nested. Loop 1. c a = 1; while (a <= 4) { b = a + 3; while (b <= 10) { printf("a = %d, b = %dn", a, b); b += 3; } a++; } return 0; } a a a a a = = = = = 1, 1, 1, 2, 2, 3, 3, 4, 4, b b b b b = = = = = 4 7 10 5 8 6 9 7 10
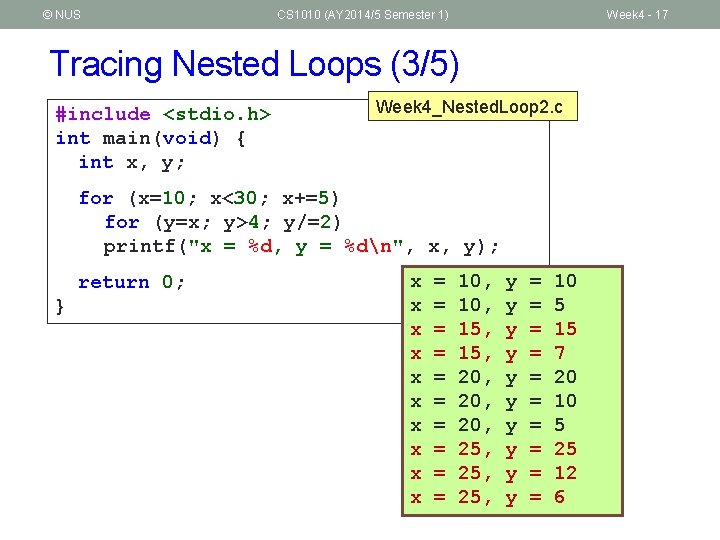
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 17 Tracing Nested Loops (3/5) #include <stdio. h> int main(void) { int x, y; Week 4_Nested. Loop 2. c for (x=10; x<30; x+=5) for (y=x; y>4; y/=2) printf("x = %d, y = %dn", x, y); return 0; } x x x x x = = = = = 10, 15, 20, 20, 25, 25, y y y y y = = = = = 10 5 15 7 20 10 5 25 12 6
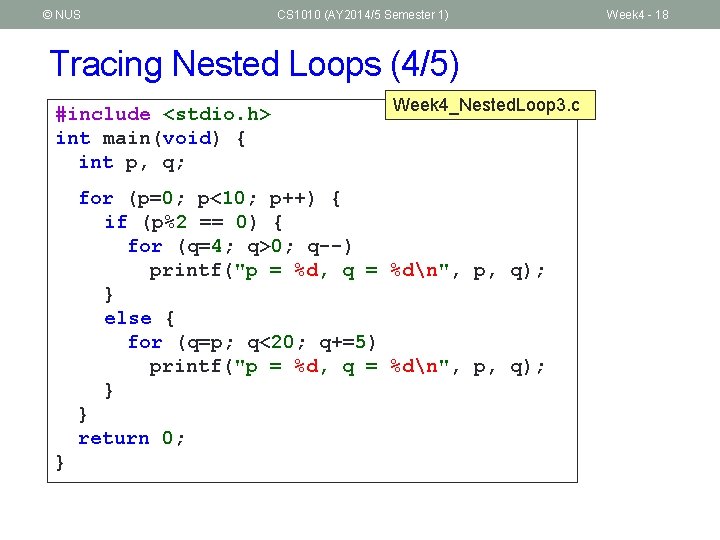
© NUS CS 1010 (AY 2014/5 Semester 1) Tracing Nested Loops (4/5) #include <stdio. h> int main(void) { int p, q; Week 4_Nested. Loop 3. c for (p=0; p<10; p++) { if (p%2 == 0) { for (q=4; q>0; q--) printf("p = %d, q = %dn", p, q); } else { for (q=p; q<20; q+=5) printf("p = %d, q = %dn", p, q); } } return 0; } Week 4 - 18
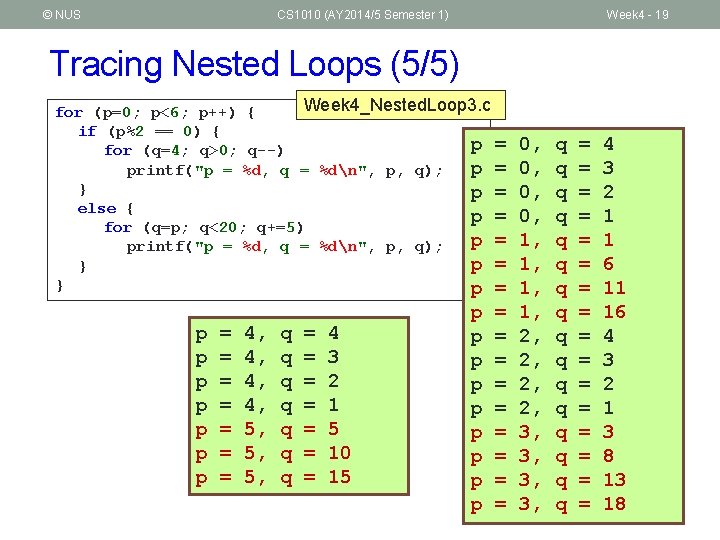
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 19 Tracing Nested Loops (5/5) Week 4_Nested. Loop 3. c for (p=0; p<6; p++) { if (p%2 == 0) { p = for (q=4; q>0; q--) p = printf("p = %d, q = %dn", p, q); } p = else { p = for (q=p; q<20; q+=5) p = printf("p = %d, q = %dn", p, q); p = } } p = p p p p = = = = 4, 4, 5, 5, 5, q q q q = = = = 4 3 2 1 5 10 15 p p p p p = = = = = 0, 0, 1, 1, 2, 2, 3, 3, q q q q = = = = 4 3 2 1 1 6 11 16 4 3 2 1 3 8 13 18
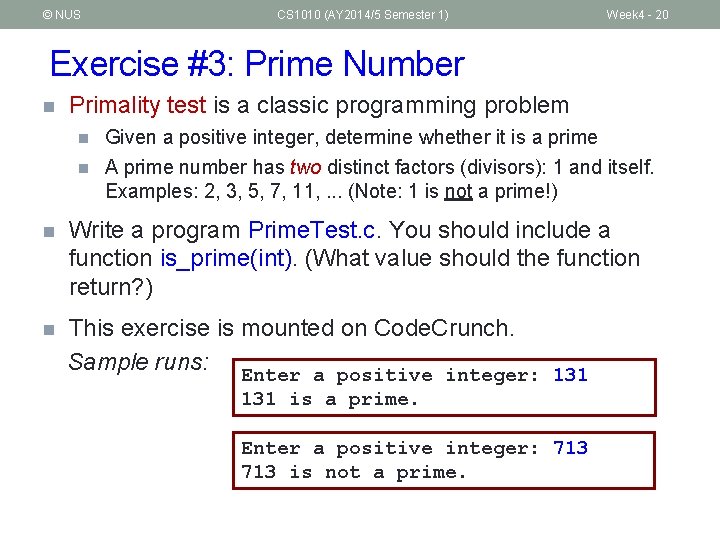
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 20 Exercise #3: Prime Number n Primality test is a classic programming problem n Given a positive integer, determine whether it is a prime n A prime number has two distinct factors (divisors): 1 and itself. Examples: 2, 3, 5, 7, 11, . . . (Note: 1 is not a prime!) n Write a program Prime. Test. c. You should include a function is_prime(int). (What value should the function return? ) n This exercise is mounted on Code. Crunch. Sample runs: Enter a positive integer: 131 is a prime. Enter a positive integer: 713 is not a prime.
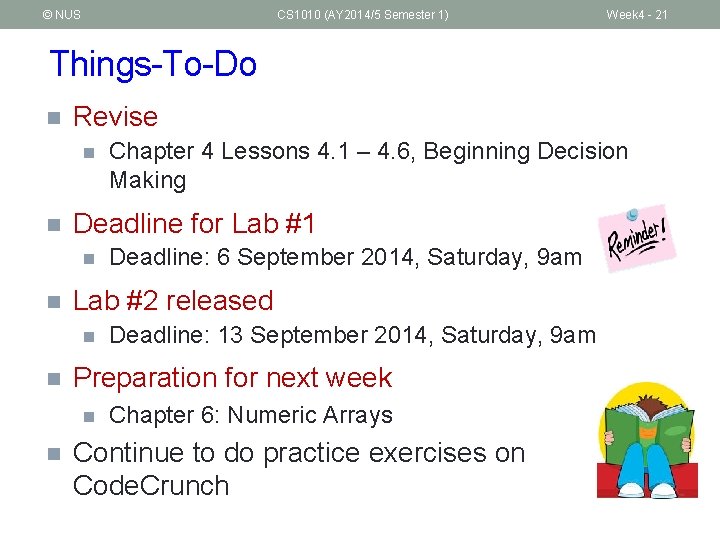
© NUS CS 1010 (AY 2014/5 Semester 1) Week 4 - 21 Things-To-Do n Revise n n Deadline for Lab #1 n n Deadline: 13 September 2014, Saturday, 9 am Preparation for next week n n Deadline: 6 September 2014, Saturday, 9 am Lab #2 released n n Chapter 4 Lessons 4. 1 – 4. 6, Beginning Decision Making Chapter 6: Numeric Arrays Continue to do practice exercises on Code. Crunch
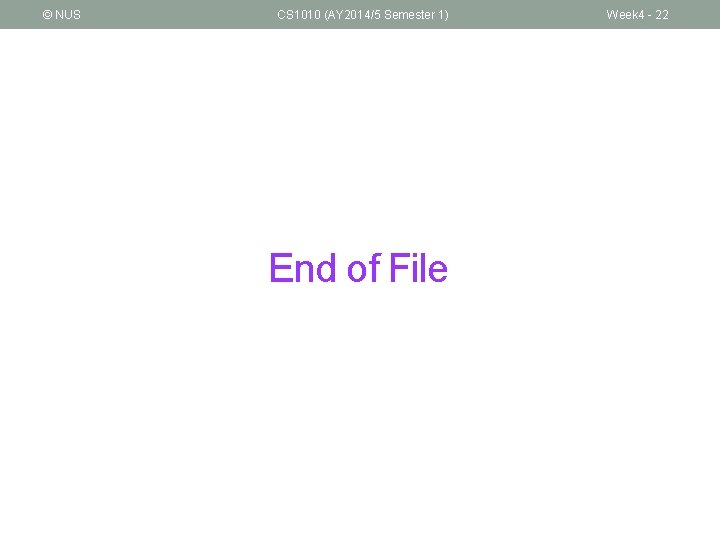
© NUS CS 1010 (AY 2014/5 Semester 1) End of File Week 4 - 22
Ebm n5 notes
Lecturers without borders
List of lecturers at icu zambia
Raquel anido
Nus modreg round 0
Dig comp
Energy conservation quick check
A small child slides down the four frictionless slides
Edu.sharif.edu
Http //scratch.mit.edu/
Regulationssynonym
Wave optics topics
Space shuttle discovery
Naplan coordinator handbook
Dlib.nyu.edu/aco/
้https //scratch.mit.edu
Egif umich
Http://learn.genetics.utah.edu/content/addiction/
Http://www.openclass.chc.edu.tw
Http://scratch.mit.edu/
Http://evolution.berkeley.edu
Scratch https //scratch.mit.edu/
Pde