Lecture 21 More On Django Django Projects and
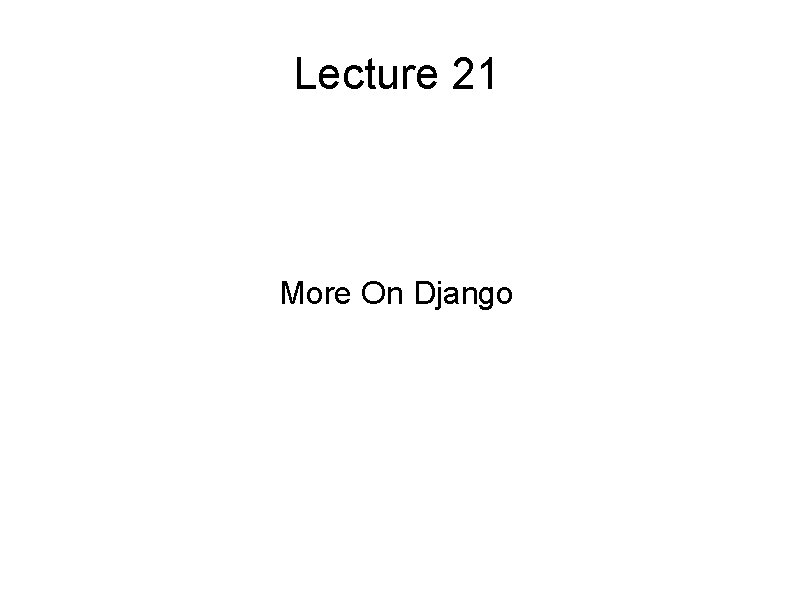
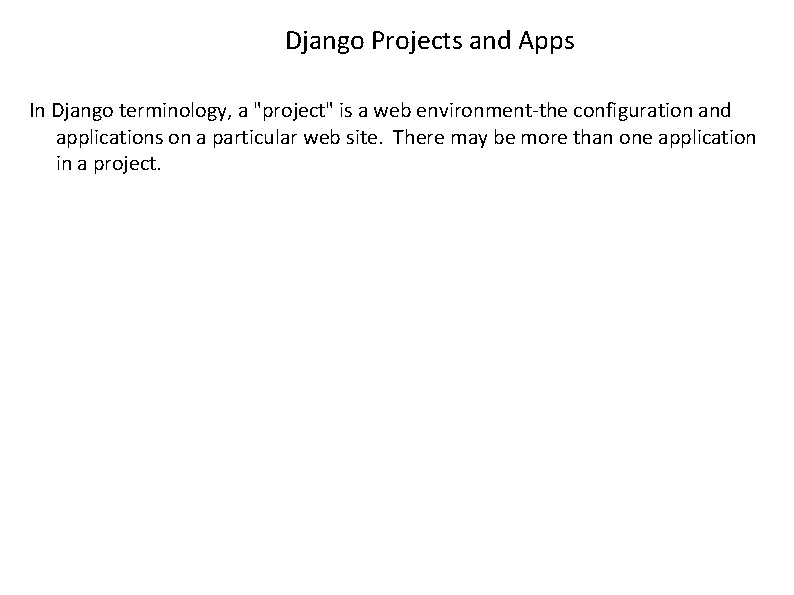
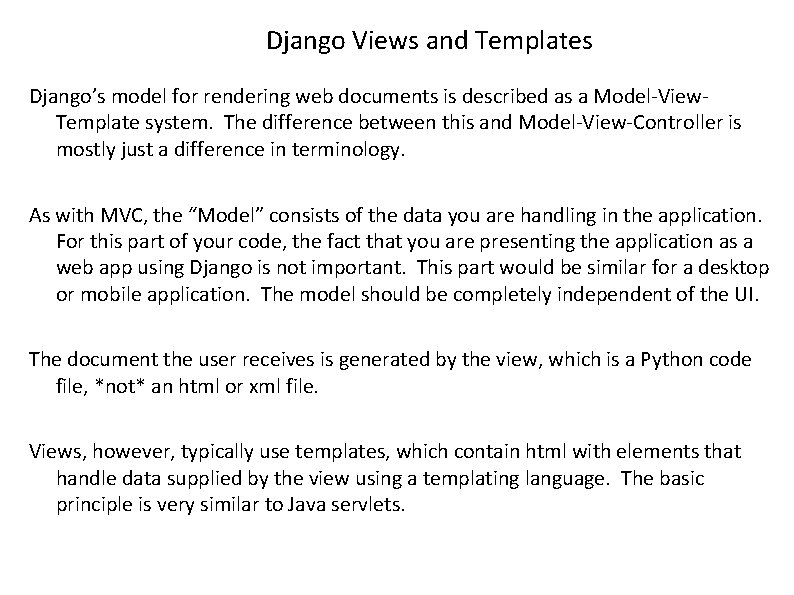
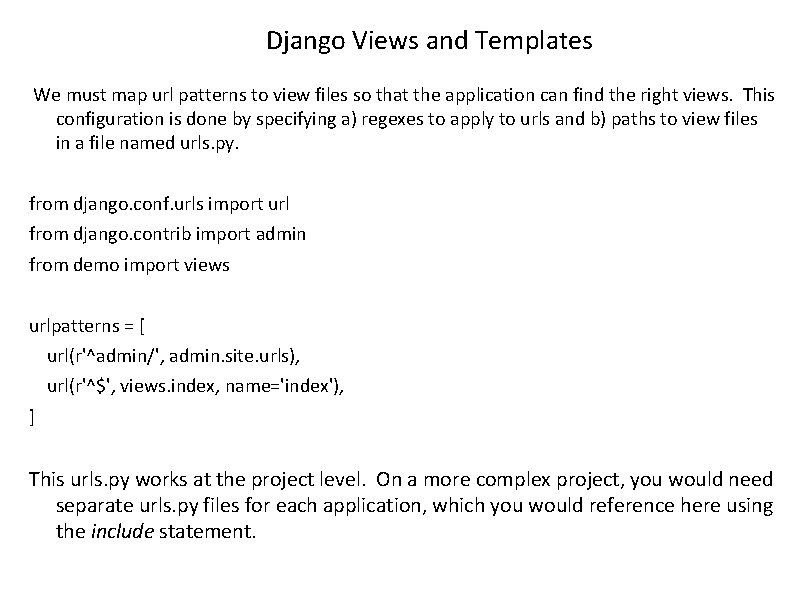
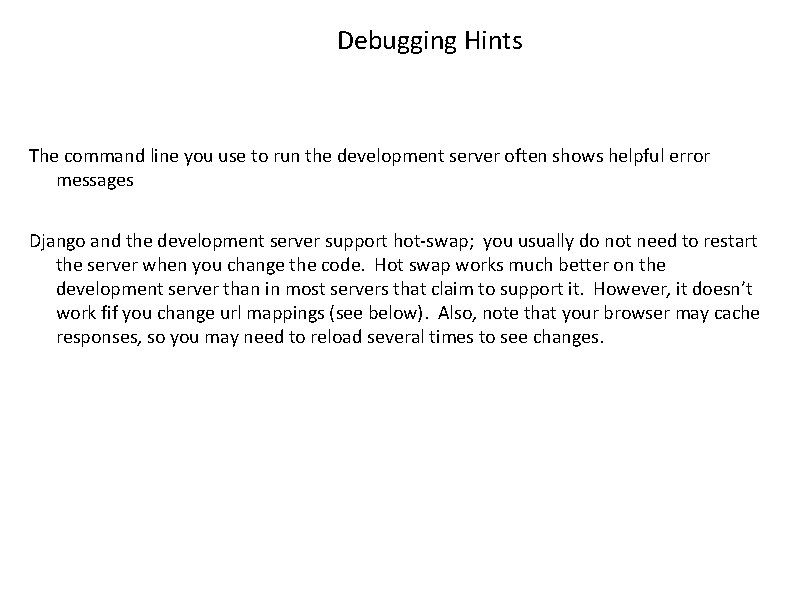
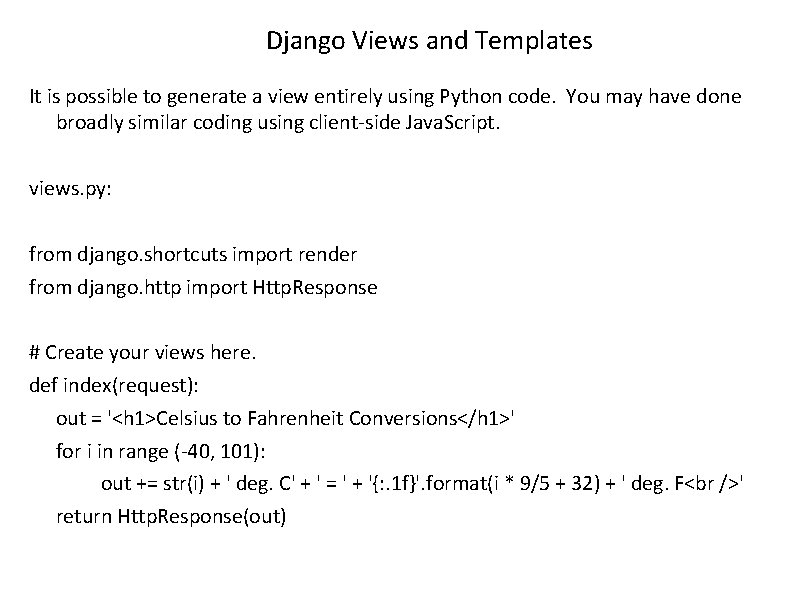
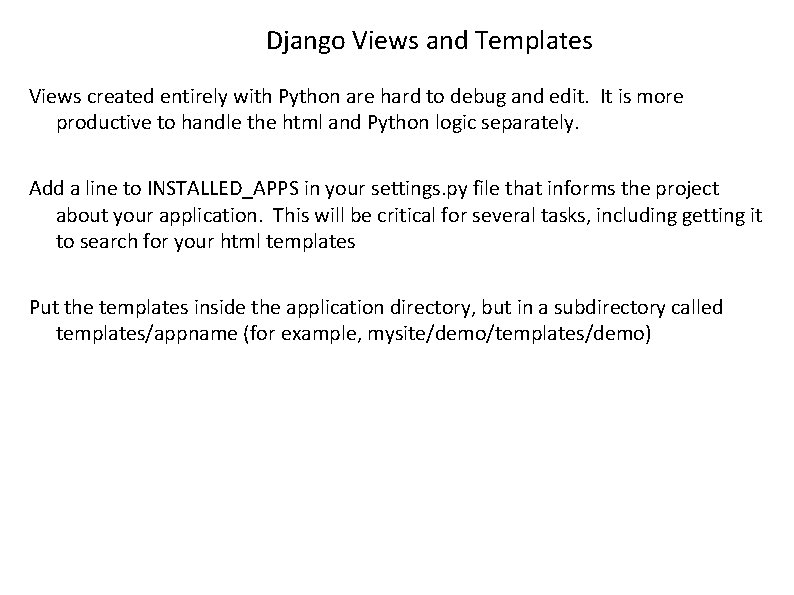
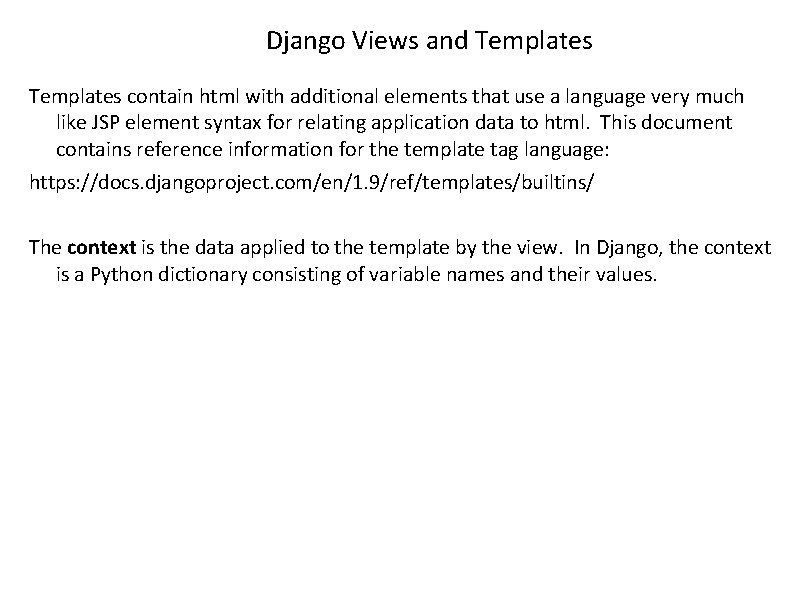
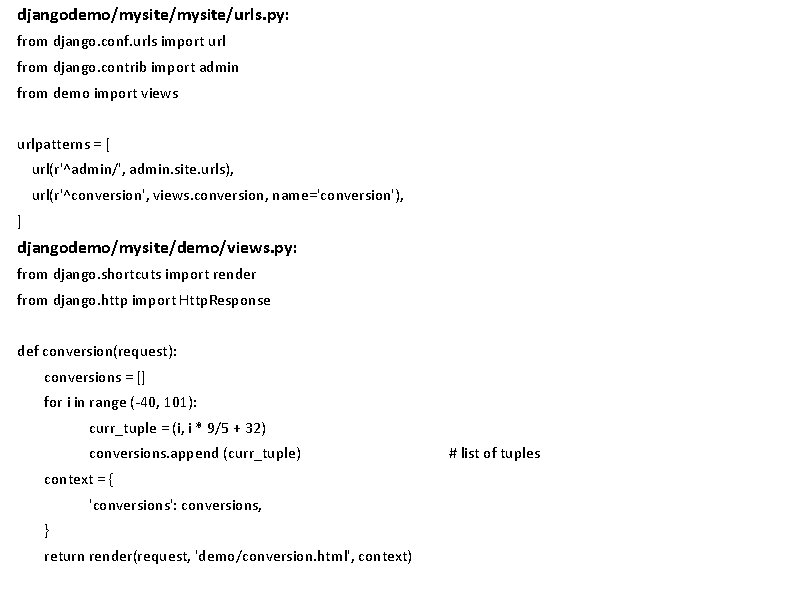
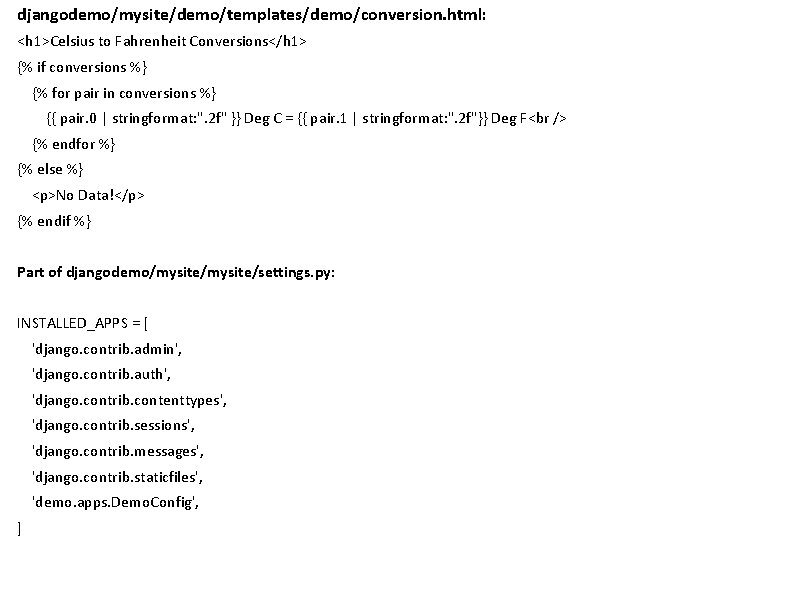
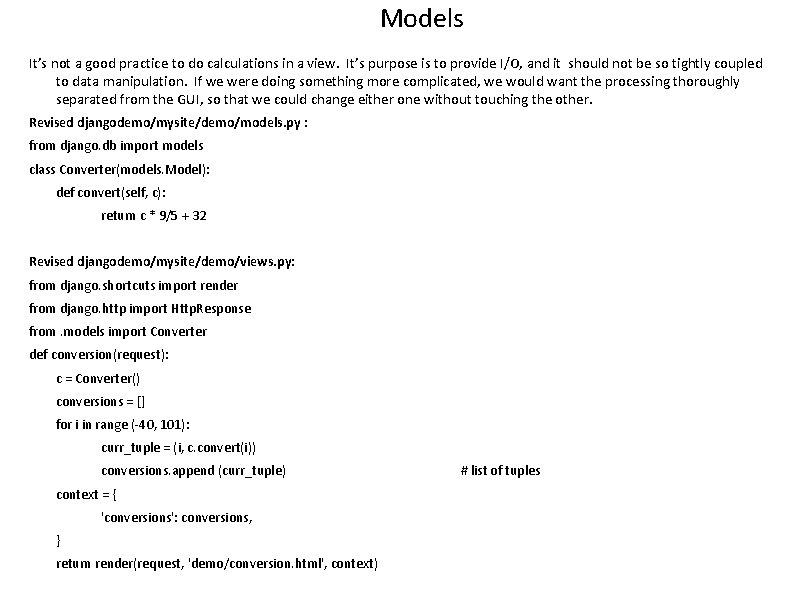
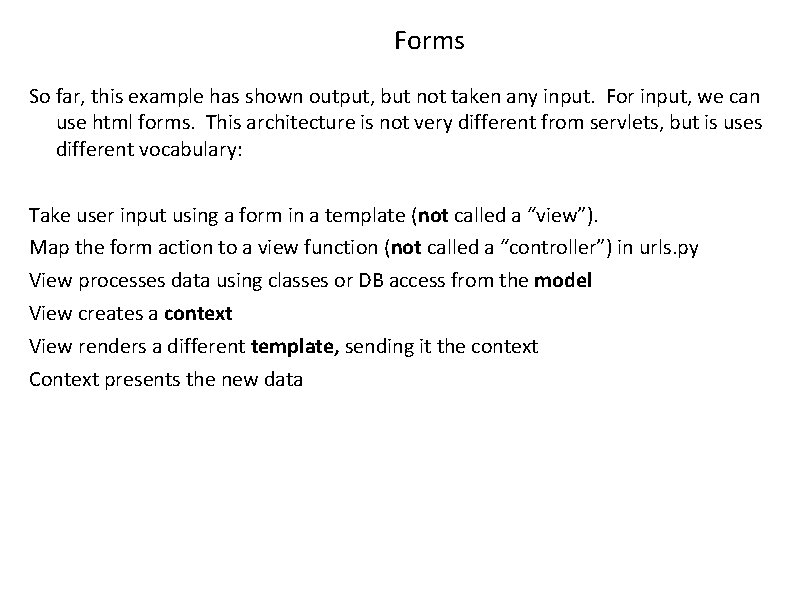
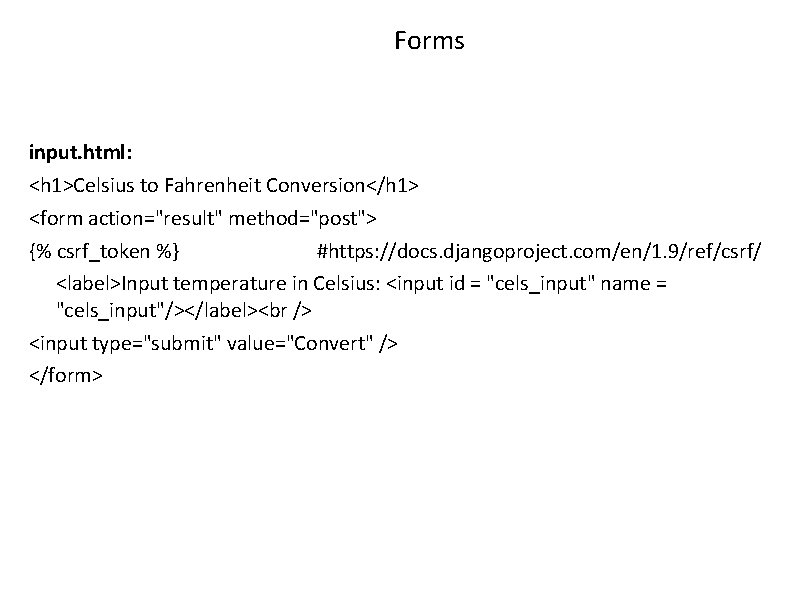
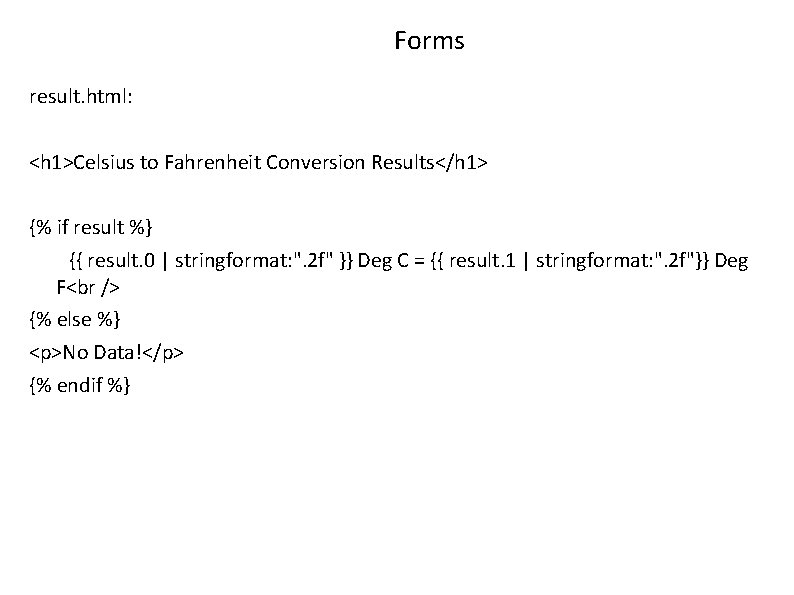
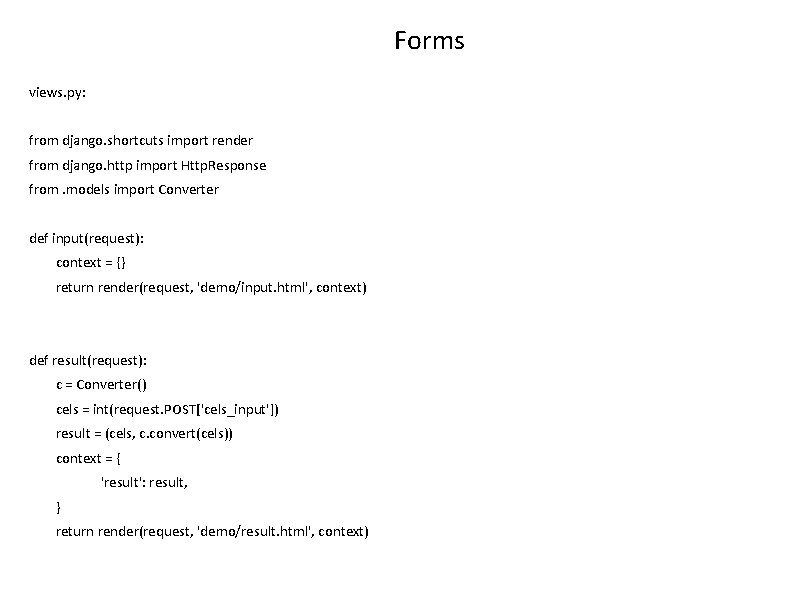
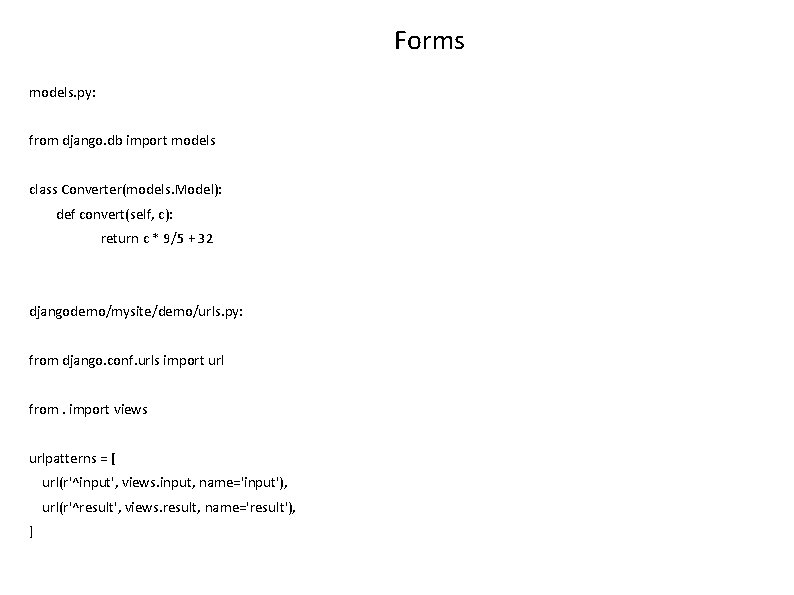
- Slides: 16
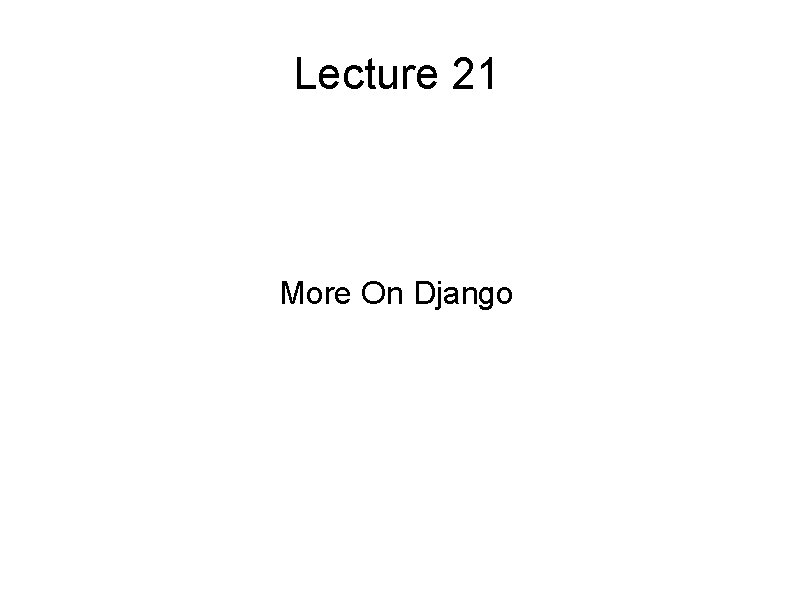
Lecture 21 More On Django
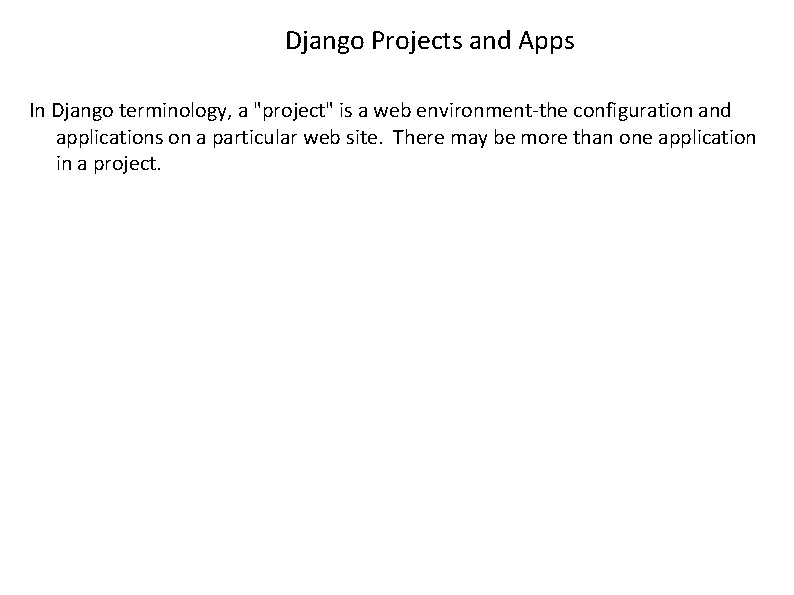
Django Projects and Apps In Django terminology, a "project" is a web environment-the configuration and applications on a particular web site. There may be more than one application in a project.
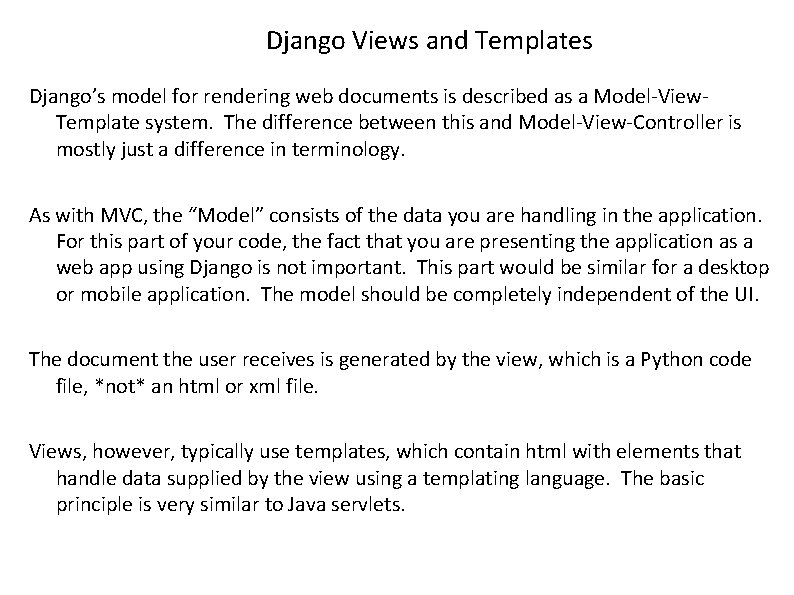
Django Views and Templates Django’s model for rendering web documents is described as a Model-View. Template system. The difference between this and Model-View-Controller is mostly just a difference in terminology. As with MVC, the “Model” consists of the data you are handling in the application. For this part of your code, the fact that you are presenting the application as a web app using Django is not important. This part would be similar for a desktop or mobile application. The model should be completely independent of the UI. The document the user receives is generated by the view, which is a Python code file, *not* an html or xml file. Views, however, typically use templates, which contain html with elements that handle data supplied by the view using a templating language. The basic principle is very similar to Java servlets.
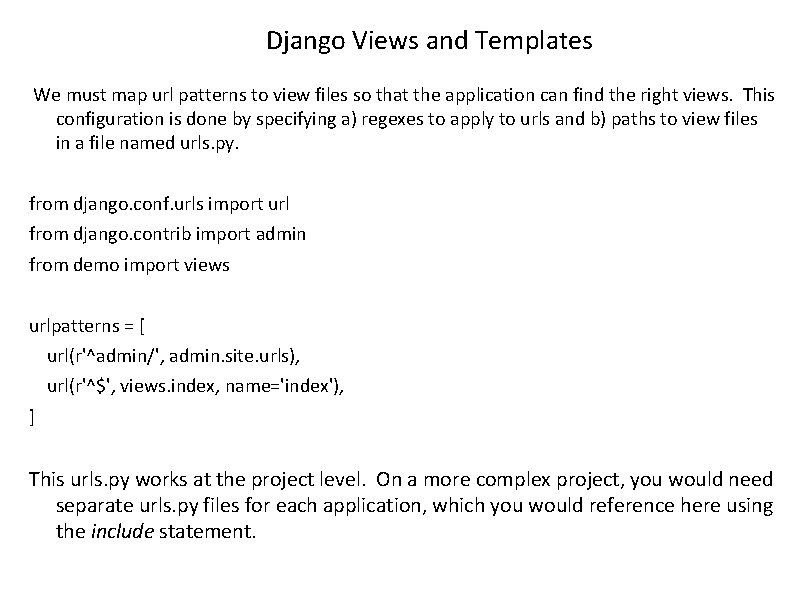
Django Views and Templates We must map url patterns to view files so that the application can find the right views. This configuration is done by specifying a) regexes to apply to urls and b) paths to view files in a file named urls. py. from django. conf. urls import url from django. contrib import admin from demo import views urlpatterns = [ url(r'^admin/', admin. site. urls), url(r'^$', views. index, name='index'), ] This urls. py works at the project level. On a more complex project, you would need separate urls. py files for each application, which you would reference here using the include statement.
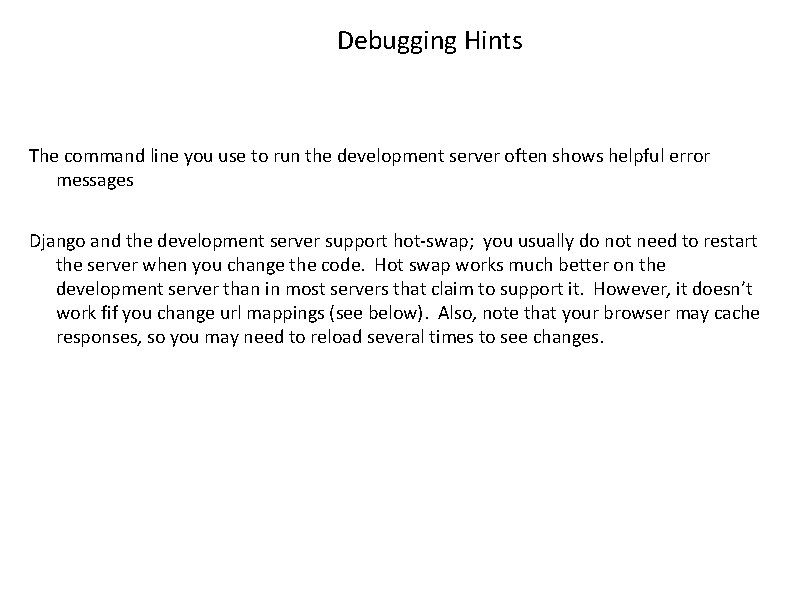
Debugging Hints The command line you use to run the development server often shows helpful error messages Django and the development server support hot-swap; you usually do not need to restart the server when you change the code. Hot swap works much better on the development server than in most servers that claim to support it. However, it doesn’t work fif you change url mappings (see below). Also, note that your browser may cache responses, so you may need to reload several times to see changes.
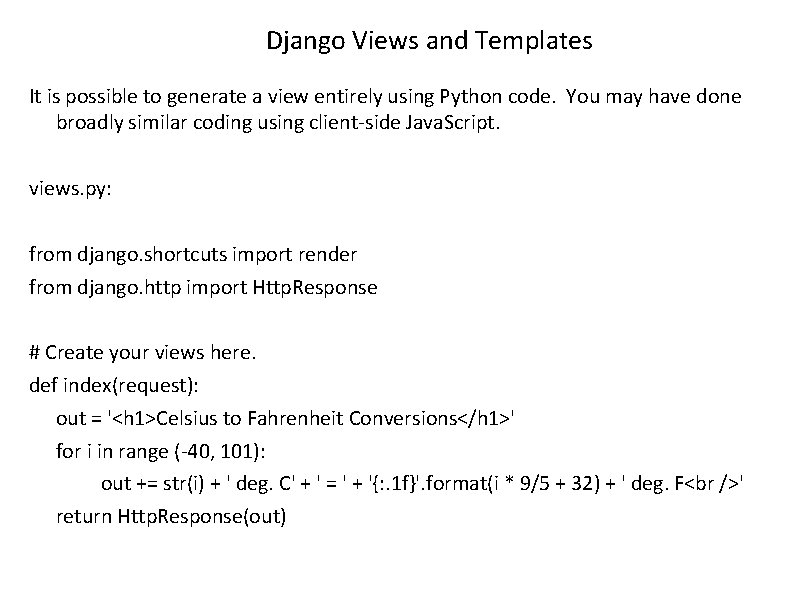
Django Views and Templates It is possible to generate a view entirely using Python code. You may have done broadly similar coding using client-side Java. Script. views. py: from django. shortcuts import render from django. http import Http. Response # Create your views here. def index(request): out = '<h 1>Celsius to Fahrenheit Conversions</h 1>' for i in range (-40, 101): out += str(i) + ' deg. C' + ' = ' + '{: . 1 f}'. format(i * 9/5 + 32) + ' deg. F ' return Http. Response(out)
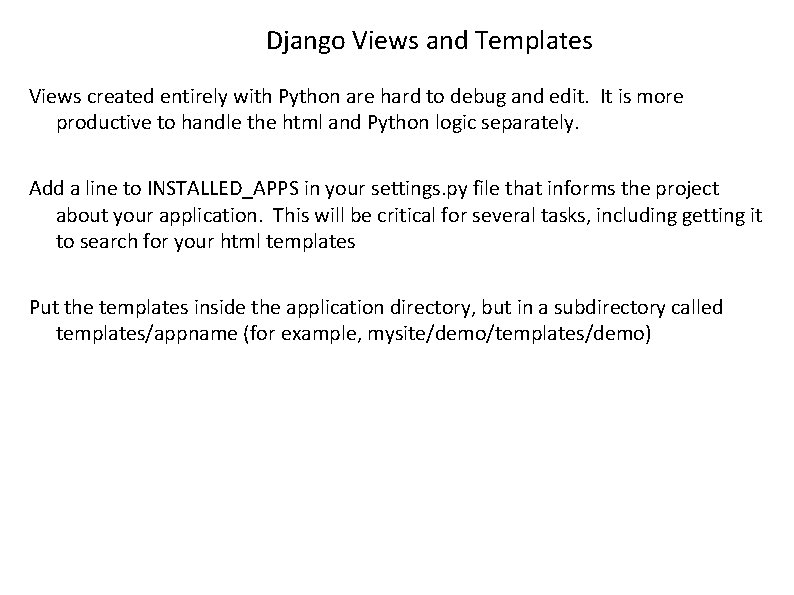
Django Views and Templates Views created entirely with Python are hard to debug and edit. It is more productive to handle the html and Python logic separately. Add a line to INSTALLED_APPS in your settings. py file that informs the project about your application. This will be critical for several tasks, including getting it to search for your html templates Put the templates inside the application directory, but in a subdirectory called templates/appname (for example, mysite/demo/templates/demo)
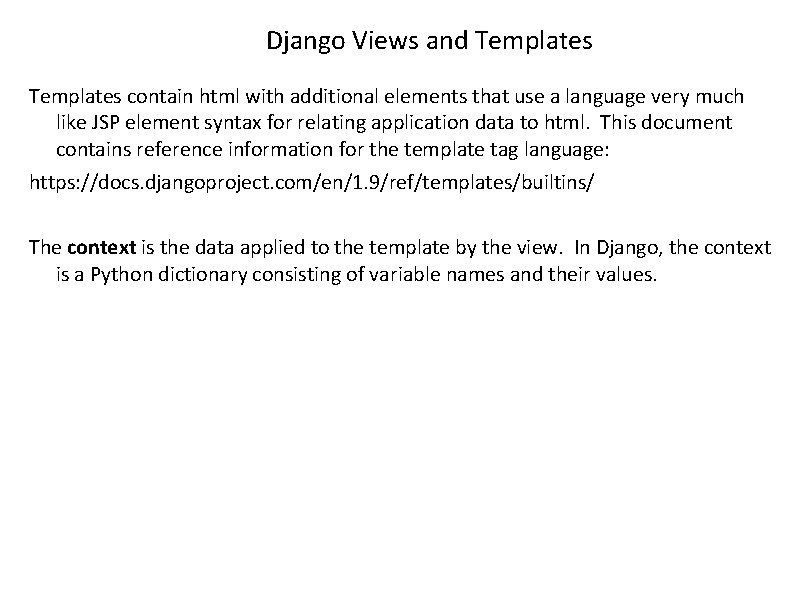
Django Views and Templates contain html with additional elements that use a language very much like JSP element syntax for relating application data to html. This document contains reference information for the template tag language: https: //docs. djangoproject. com/en/1. 9/ref/templates/builtins/ The context is the data applied to the template by the view. In Django, the context is a Python dictionary consisting of variable names and their values.
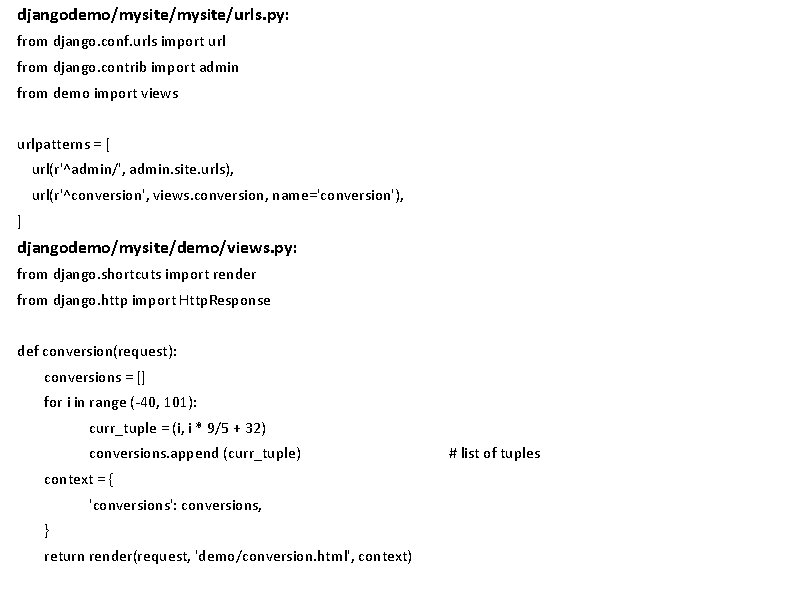
djangodemo/mysite/urls. py: from django. conf. urls import url from django. contrib import admin from demo import views urlpatterns = [ url(r'^admin/', admin. site. urls), url(r'^conversion', views. conversion, name='conversion'), ] djangodemo/mysite/demo/views. py: from django. shortcuts import render from django. http import Http. Response def conversion(request): conversions = [] for i in range (-40, 101): curr_tuple = (i, i * 9/5 + 32) conversions. append (curr_tuple) context = { 'conversions': conversions, } return render(request, 'demo/conversion. html', context) # list of tuples
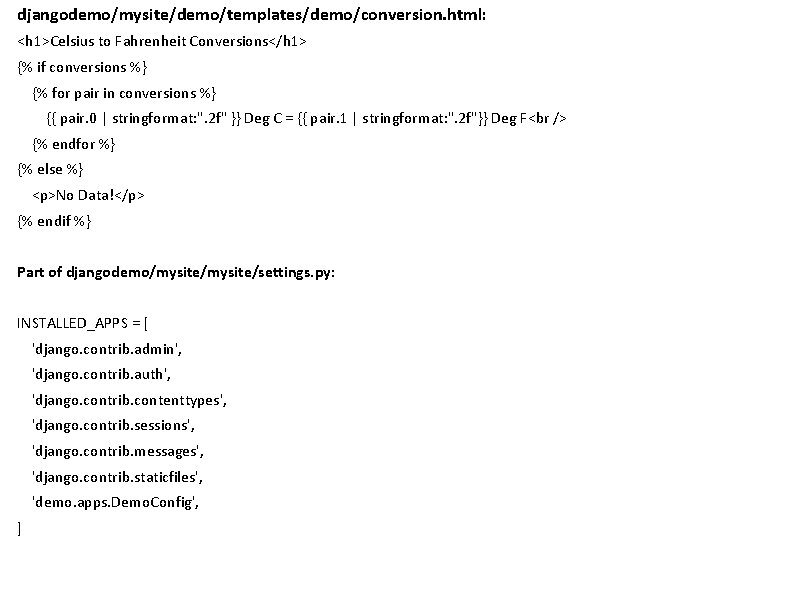
djangodemo/mysite/demo/templates/demo/conversion. html: <h 1>Celsius to Fahrenheit Conversions</h 1> {% if conversions %} {% for pair in conversions %} {{ pair. 0 | stringformat: ". 2 f" }} Deg C = {{ pair. 1 | stringformat: ". 2 f"}} Deg F {% endfor %} {% else %} <p>No Data!</p> {% endif %} Part of djangodemo/mysite/settings. py: INSTALLED_APPS = [ 'django. contrib. admin', 'django. contrib. auth', 'django. contrib. contenttypes', 'django. contrib. sessions', 'django. contrib. messages', 'django. contrib. staticfiles', 'demo. apps. Demo. Config', ]
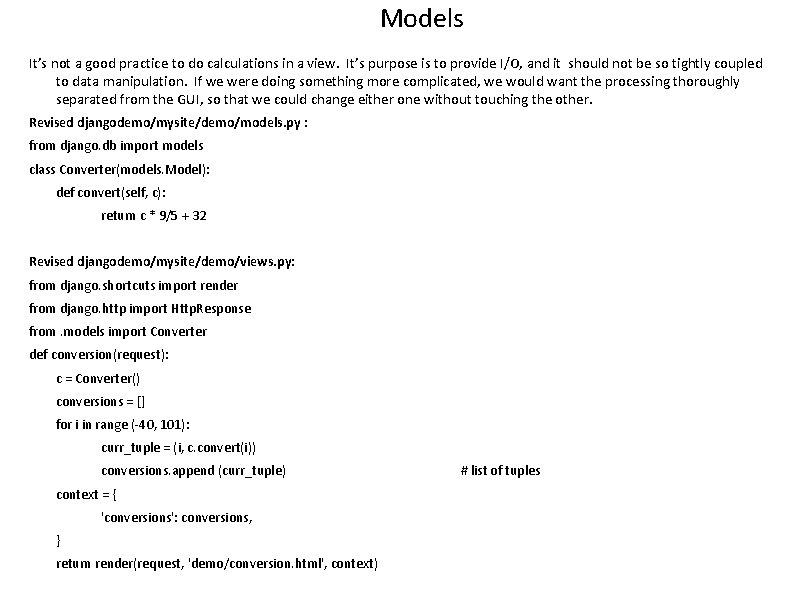
Models It’s not a good practice to do calculations in a view. It’s purpose is to provide I/O, and it should not be so tightly coupled to data manipulation. If we were doing something more complicated, we would want the processing thoroughly separated from the GUI, so that we could change either one without touching the other. Revised djangodemo/mysite/demo/models. py : from django. db import models class Converter(models. Model): def convert(self, c): return c * 9/5 + 32 Revised djangodemo/mysite/demo/views. py: from django. shortcuts import render from django. http import Http. Response from. models import Converter def conversion(request): c = Converter() conversions = [] for i in range (-40, 101): curr_tuple = (i, c. convert(i)) conversions. append (curr_tuple) context = { 'conversions': conversions, } return render(request, 'demo/conversion. html', context) # list of tuples
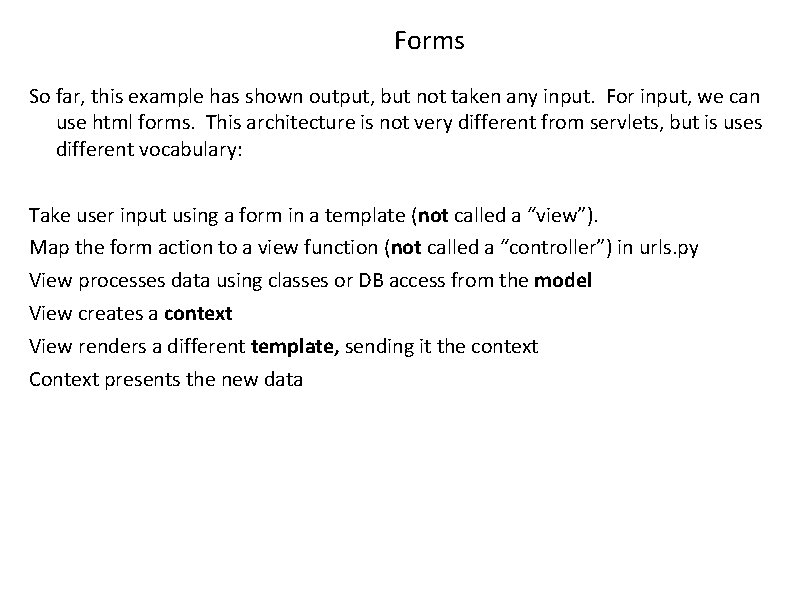
Forms So far, this example has shown output, but not taken any input. For input, we can use html forms. This architecture is not very different from servlets, but is uses different vocabulary: Take user input using a form in a template (not called a “view”). Map the form action to a view function (not called a “controller”) in urls. py View processes data using classes or DB access from the model View creates a context View renders a different template, sending it the context Context presents the new data
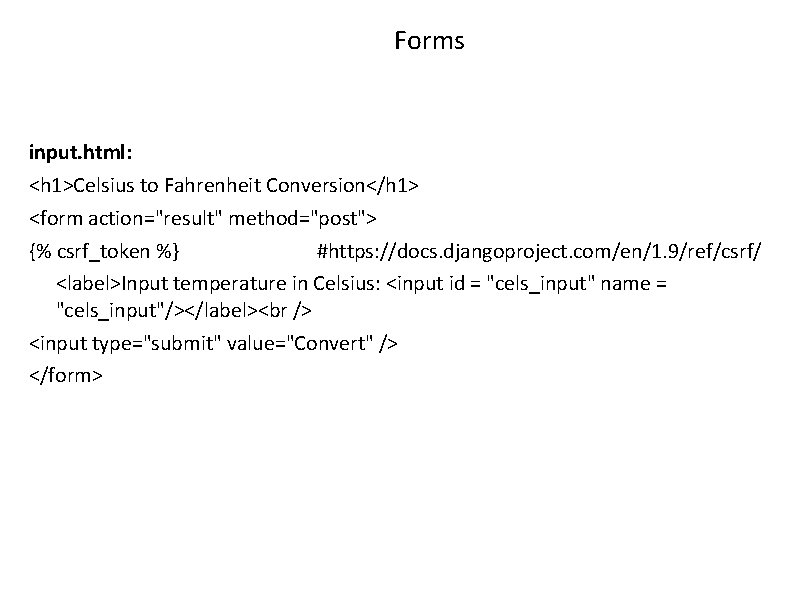
Forms input. html: <h 1>Celsius to Fahrenheit Conversion</h 1> <form action="result" method="post"> {% csrf_token %} #https: //docs. djangoproject. com/en/1. 9/ref/csrf/ <label>Input temperature in Celsius: <input id = "cels_input" name = "cels_input"/></label> <input type="submit" value="Convert" /> </form>
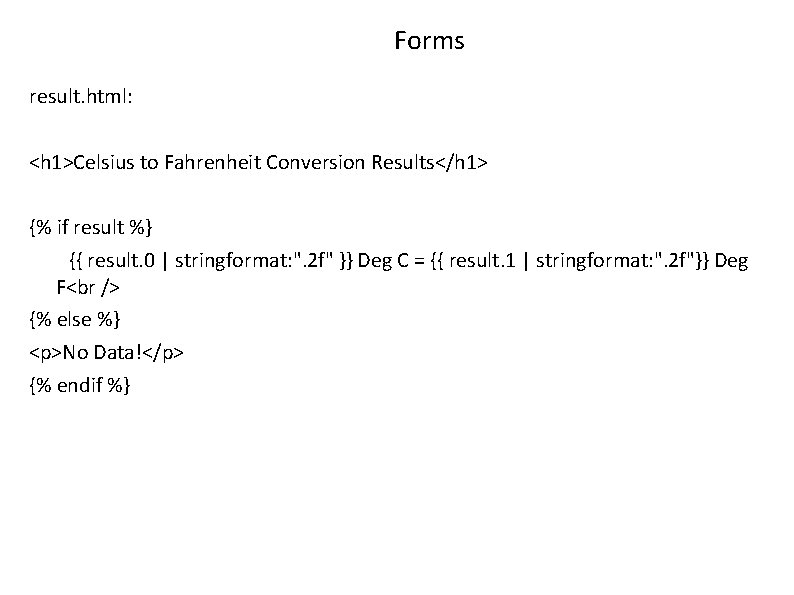
Forms result. html: <h 1>Celsius to Fahrenheit Conversion Results</h 1> {% if result %} {{ result. 0 | stringformat: ". 2 f" }} Deg C = {{ result. 1 | stringformat: ". 2 f"}} Deg F {% else %} <p>No Data!</p> {% endif %}
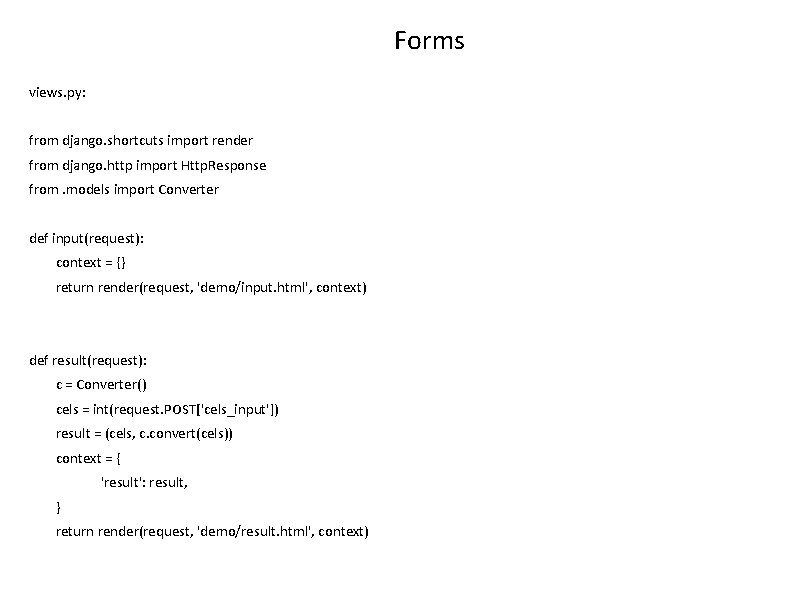
Forms views. py: from django. shortcuts import render from django. http import Http. Response from. models import Converter def input(request): context = {} return render(request, 'demo/input. html', context) def result(request): c = Converter() cels = int(request. POST['cels_input']) result = (cels, c. convert(cels)) context = { 'result': result, } return render(request, 'demo/result. html', context)
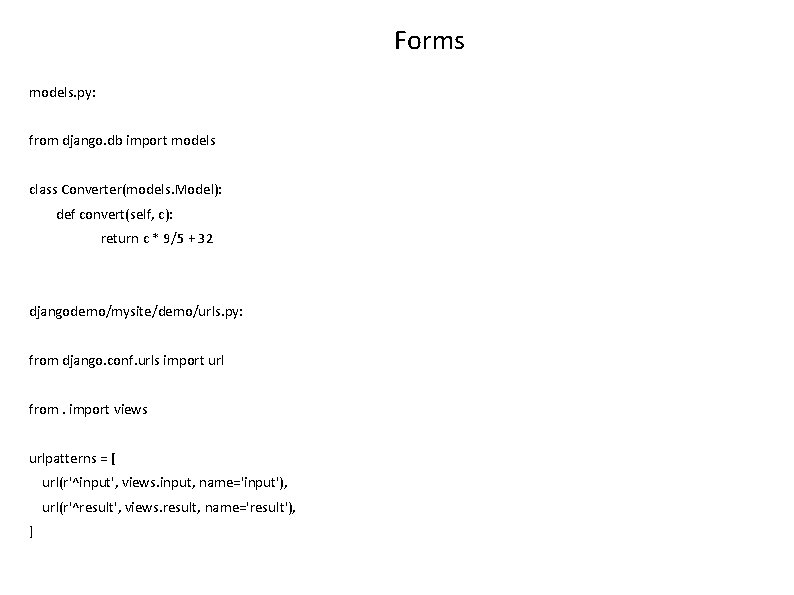
Forms models. py: from django. db import models class Converter(models. Model): def convert(self, c): return c * 9/5 + 32 djangodemo/mysite/demo/urls. py: from django. conf. urls import url from. import views urlpatterns = [ url(r'^input', views. input, name='input'), url(r'^result', views. result, name='result'), ]
More more more i want more more more more we praise you
More more more i want more more more more we praise you
01:640:244 lecture notes - lecture 15: plat, idah, farad
Django mtv
Yves reinhardt
Little sussie
Load balancer django
Social network with django
Active record vs data mapper
Django graph visualization
Django web socket
Pythonclassroomdiary
Django reinhardt documentary
Python django template
Human history becomes more and more a race
5 apples in a basket riddle
The more you study the more you learn