Introduction to Flash Action Script Statements loops Conditional
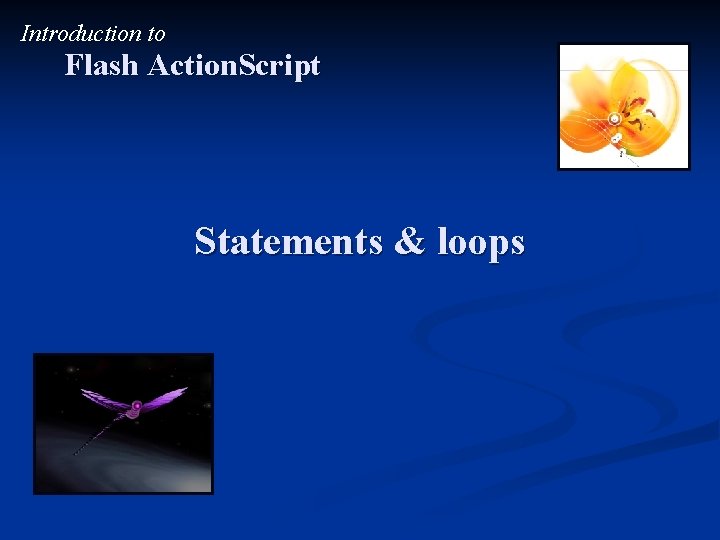
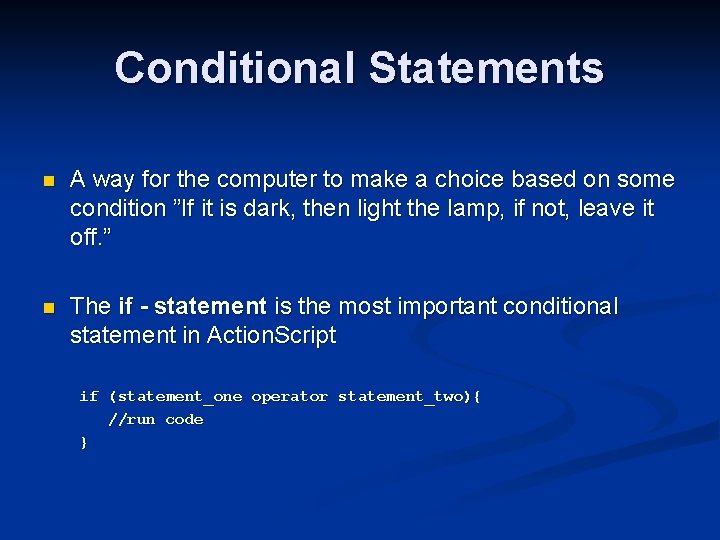
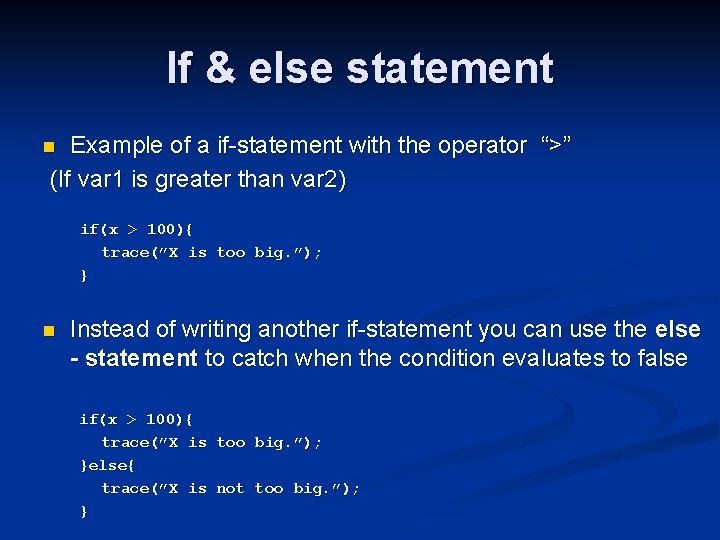
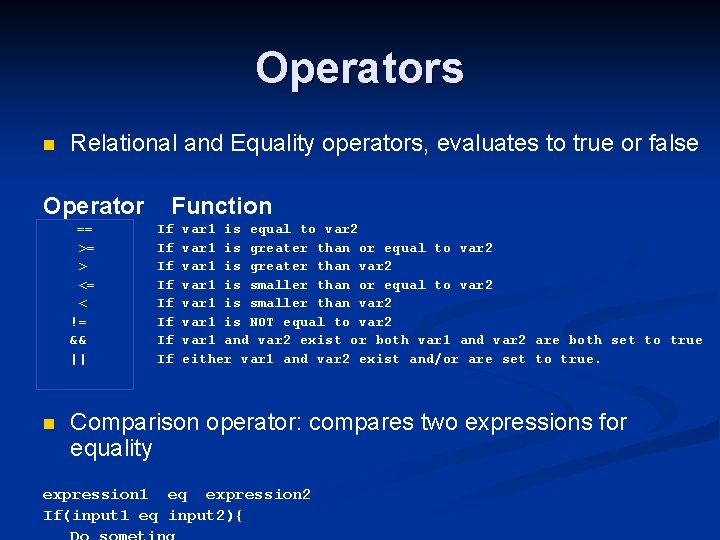
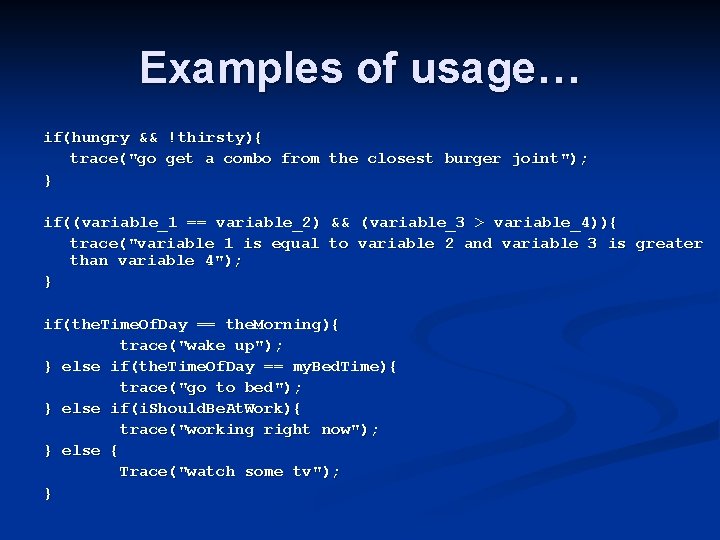
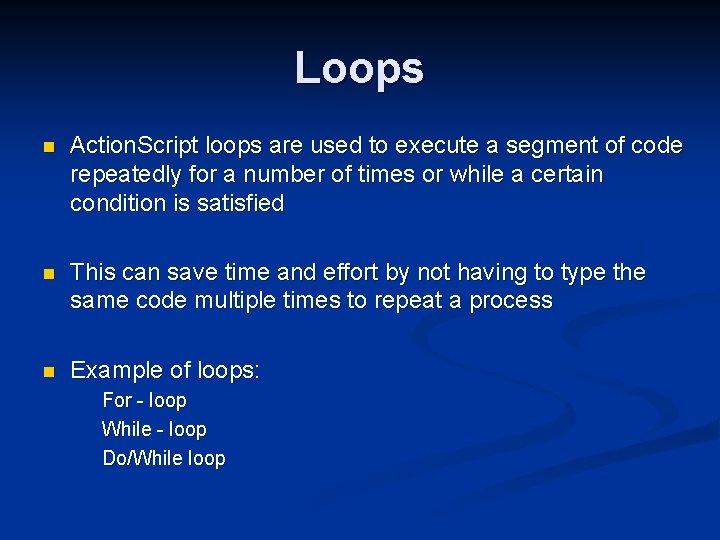
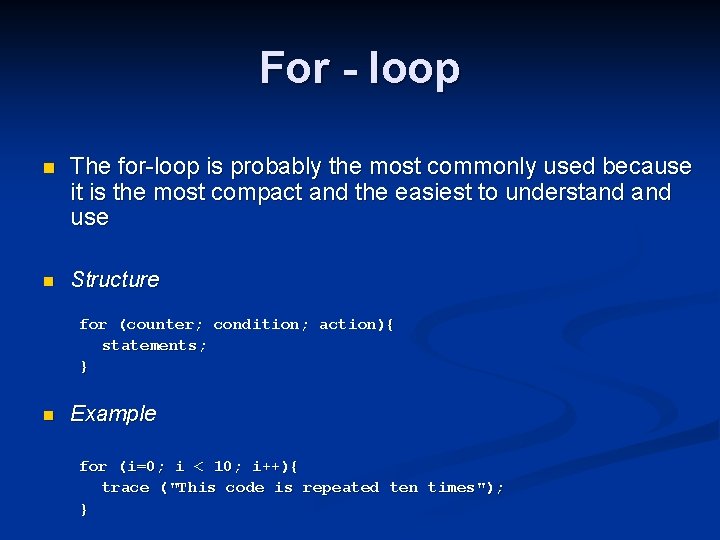
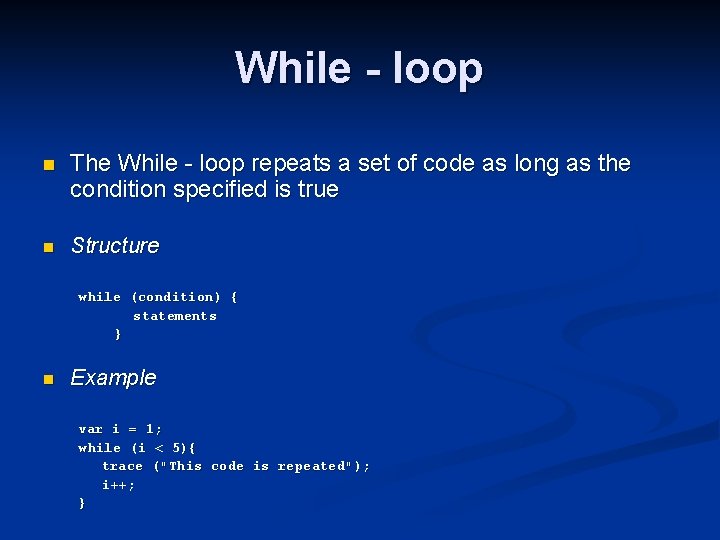
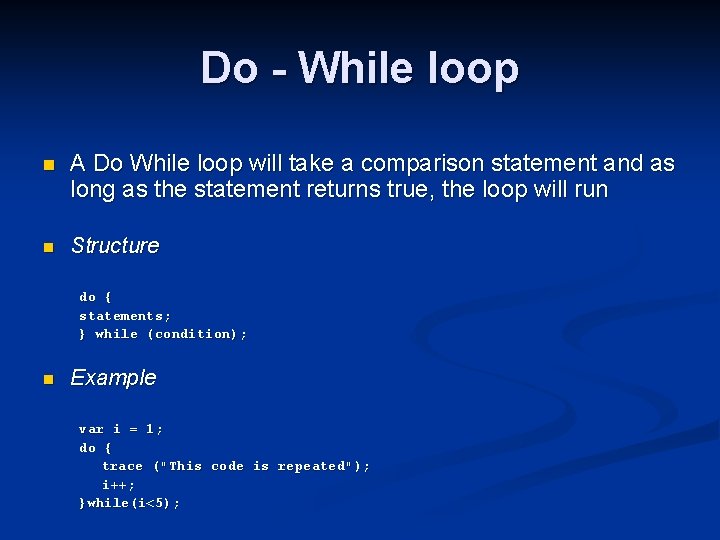
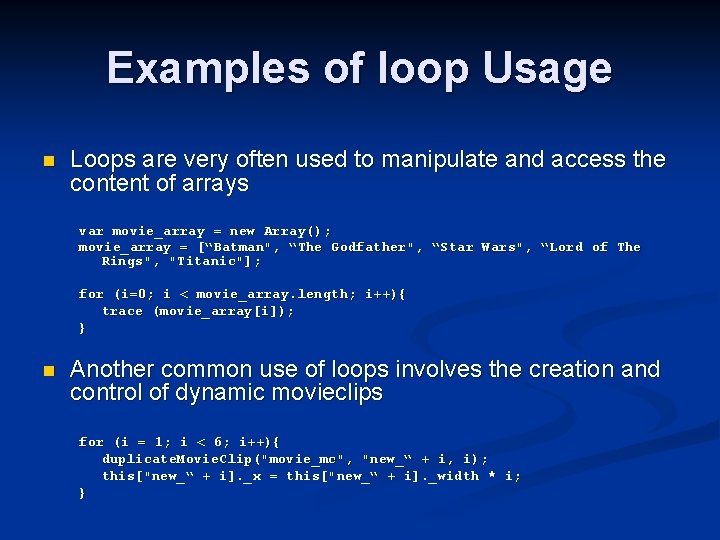
- Slides: 10
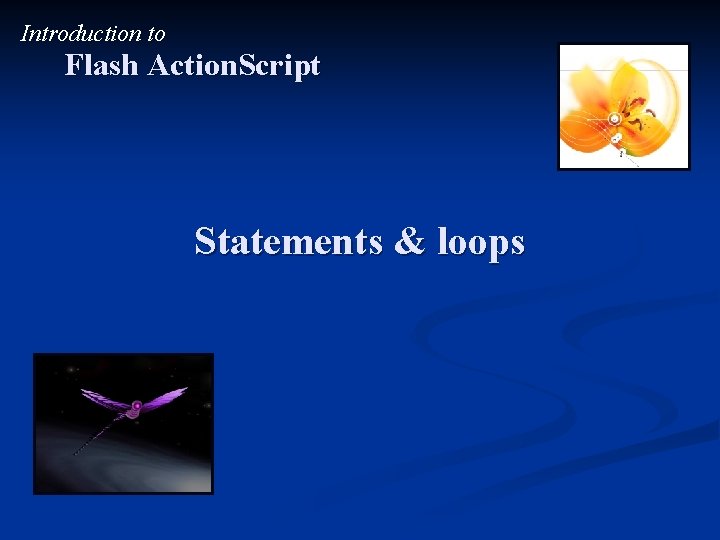
Introduction to Flash Action. Script Statements & loops
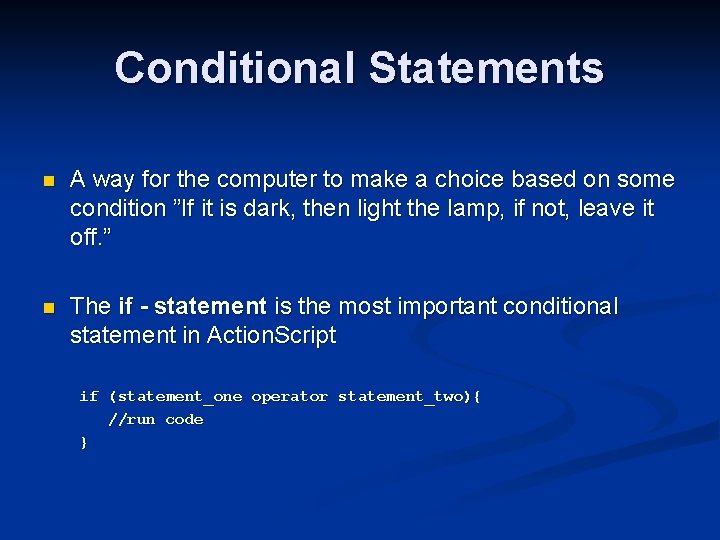
Conditional Statements n A way for the computer to make a choice based on some condition ”If it is dark, then light the lamp, if not, leave it off. ” n The if - statement is the most important conditional statement in Action. Script if (statement_one operator statement_two){ //run code }
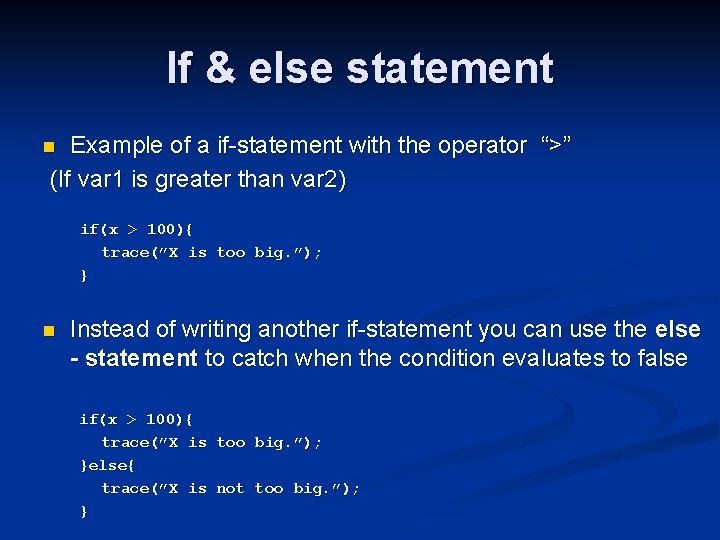
If & else statement Example of a if-statement with the operator “>” (If var 1 is greater than var 2) n if(x > 100){ trace(”X is too big. ”); } n Instead of writing another if-statement you can use the else - statement to catch when the condition evaluates to false if(x > 100){ trace(”X is too big. ”); }else{ trace(”X is not too big. ”); }
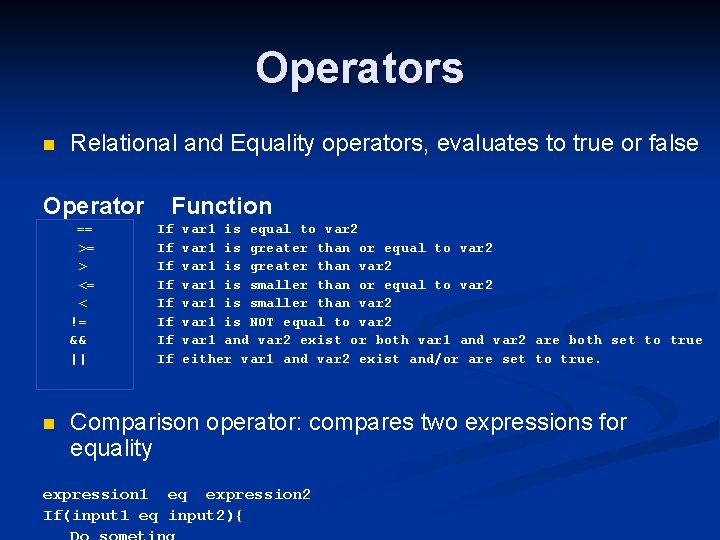
Operators n Relational and Equality operators, evaluates to true or false Operator == >= > <= < != && || n Function If If var 1 is equal to var 2 var 1 is greater than or equal to var 2 var 1 is greater than var 2 var 1 is smaller than or equal to var 2 var 1 is smaller than var 2 var 1 is NOT equal to var 2 var 1 and var 2 exist or both var 1 and var 2 are both set to true either var 1 and var 2 exist and/or are set to true. Comparison operator: compares two expressions for equality expression 1 eq expression 2 If(input 1 eq input 2){
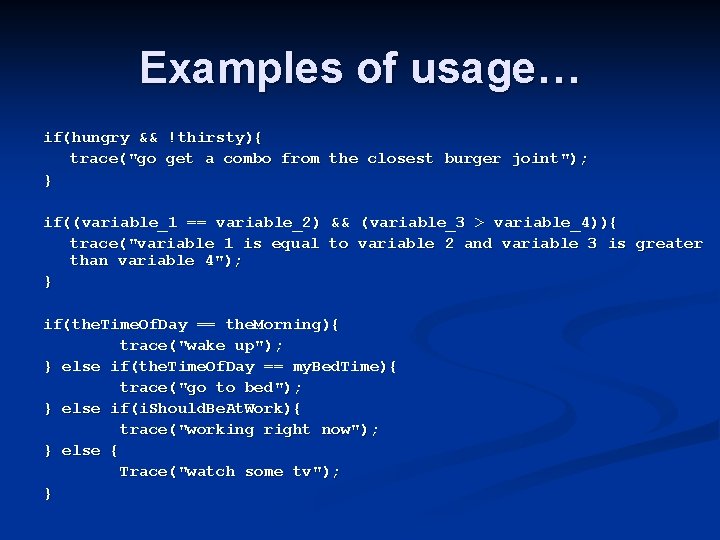
Examples of usage… if(hungry && !thirsty){ trace("go get a combo from the closest burger joint"); } if((variable_1 == variable_2) && (variable_3 > variable_4)){ trace("variable 1 is equal to variable 2 and variable 3 is greater than variable 4"); } if(the. Time. Of. Day == the. Morning){ trace("wake up"); } else if(the. Time. Of. Day == my. Bed. Time){ trace("go to bed"); } else if(i. Should. Be. At. Work){ trace("working right now"); } else { Trace("watch some tv"); }
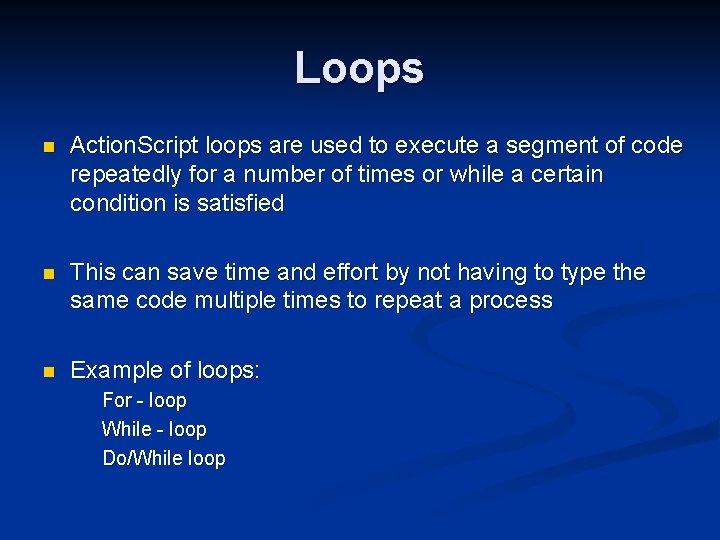
Loops n Action. Script loops are used to execute a segment of code repeatedly for a number of times or while a certain condition is satisfied n This can save time and effort by not having to type the same code multiple times to repeat a process n Example of loops: For - loop While - loop Do/While loop
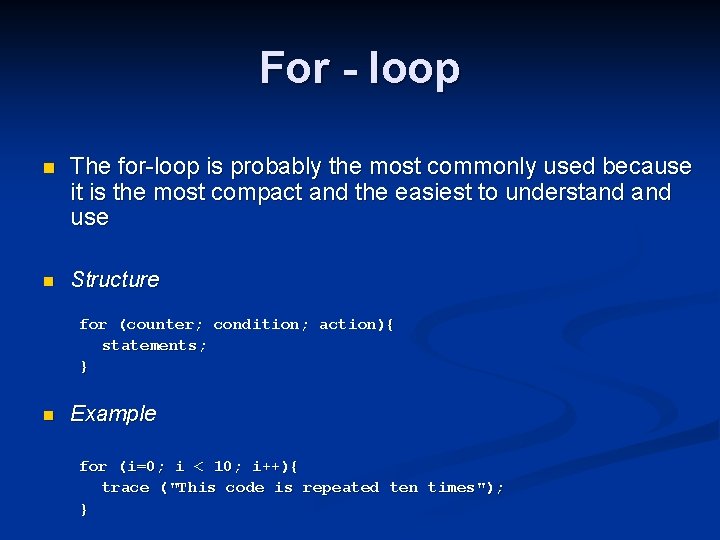
For - loop n The for-loop is probably the most commonly used because it is the most compact and the easiest to understand use n Structure for (counter; condition; action){ statements; } n Example for (i=0; i < 10; i++){ trace ("This code is repeated ten times"); }
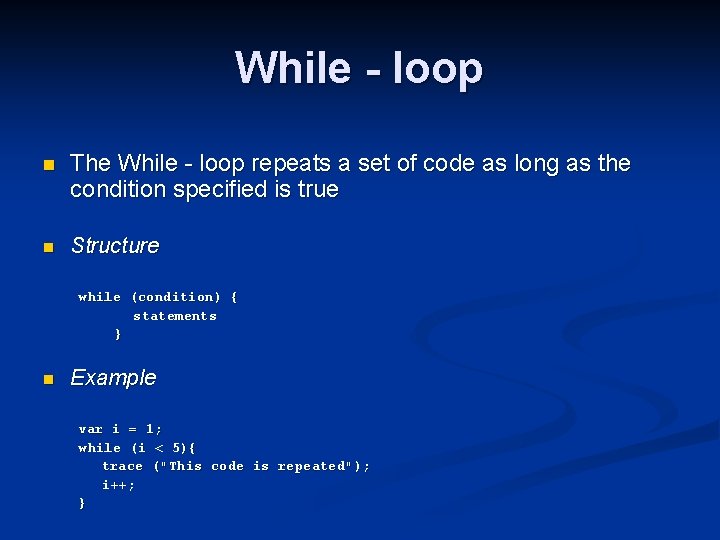
While - loop n The While - loop repeats a set of code as long as the condition specified is true n Structure while (condition) { statements } n Example var i = 1; while (i < 5){ trace ("This code is repeated"); i++; }
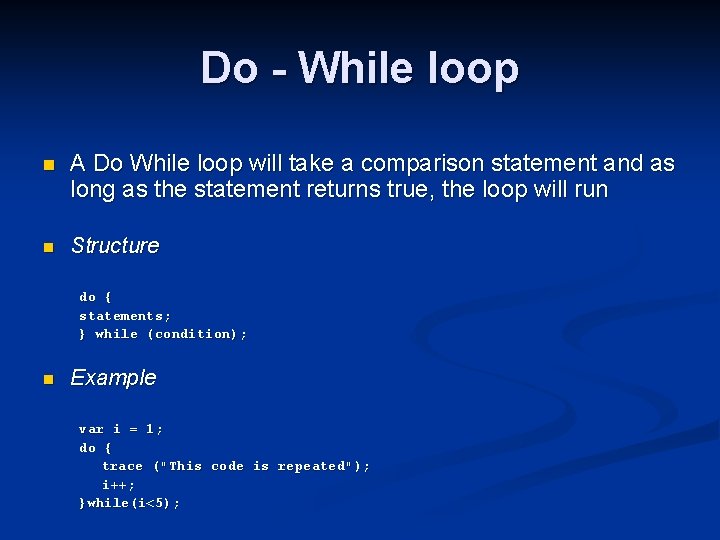
Do - While loop n A Do While loop will take a comparison statement and as long as the statement returns true, the loop will run n Structure do { statements; } while (condition); n Example var i = 1; do { trace ("This code is repeated"); i++; }while(i<5);
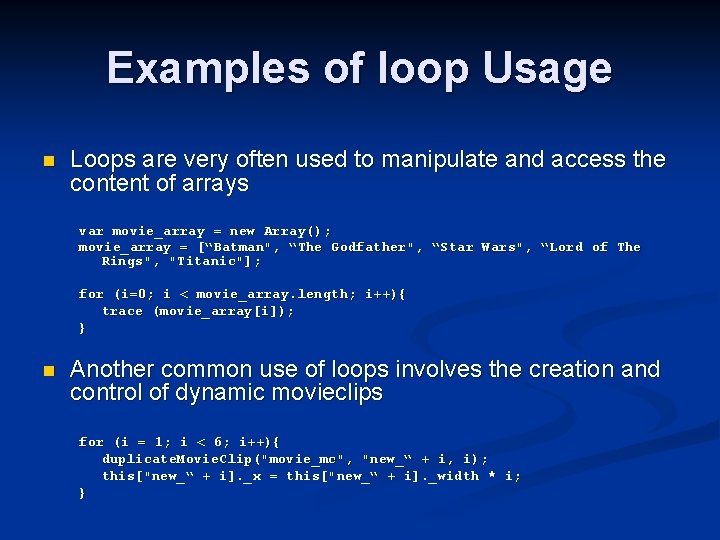
Examples of loop Usage n Loops are very often used to manipulate and access the content of arrays var movie_array = new Array(); movie_array = [“Batman", “The Godfather", “Star Wars", “Lord of The Rings", "Titanic"]; for (i=0; i < movie_array. length; i++){ trace (movie_array[i]); } n Another common use of loops involves the creation and control of dynamic movieclips for (i = 1; i < 6; i++){ duplicate. Movie. Clip("movie_mc", "new_“ + i, i); this["new_“ + i]. _x = this["new_“ + i]. _width * i; }
Digital photography with flash and no-flash image pairs
Conditional statment
Conditional definition geometry
English grammar first conditional
Conditional symbolic notation
2-2 practice conditional statements
Sequential conditional and iterative
Symbol of conditional statement
Bioconditional statement
2-2 conditional statements
What is past conditional