IE Firefox TCP ClientServer Interaction TCP Server socket
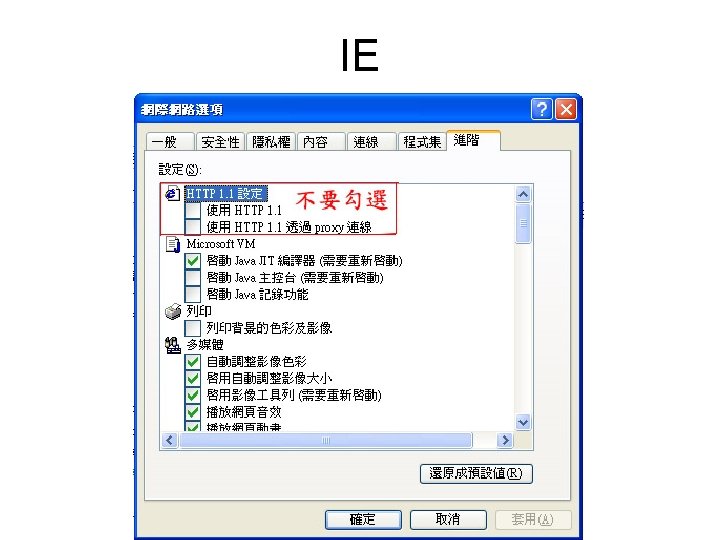
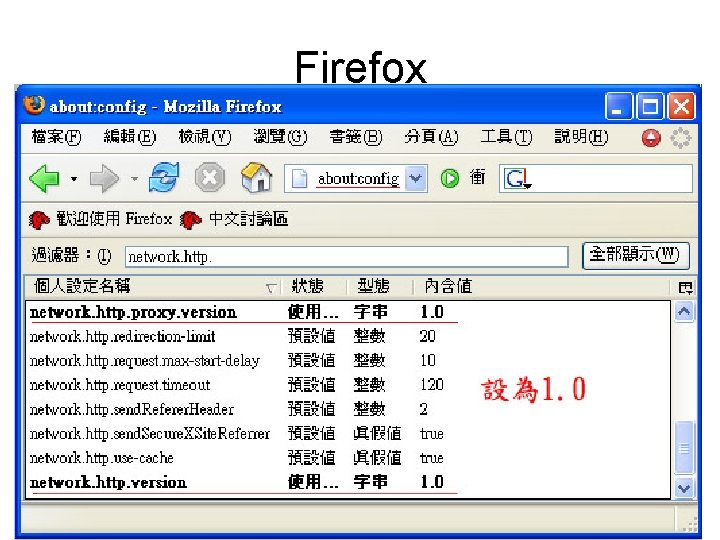
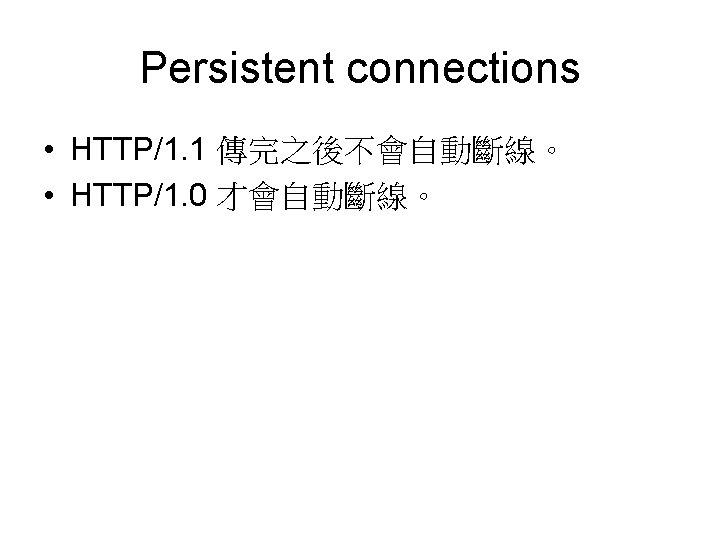
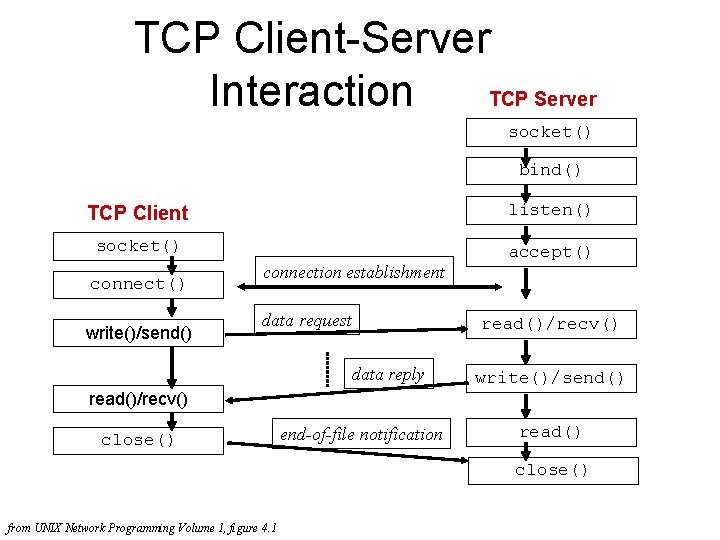
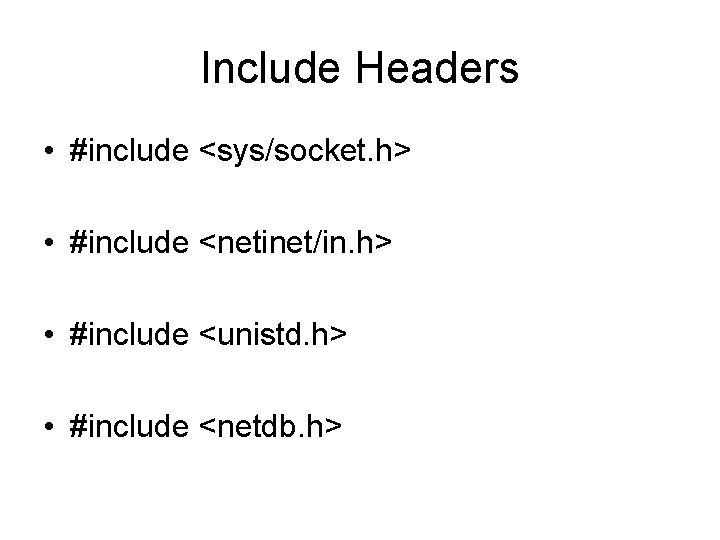
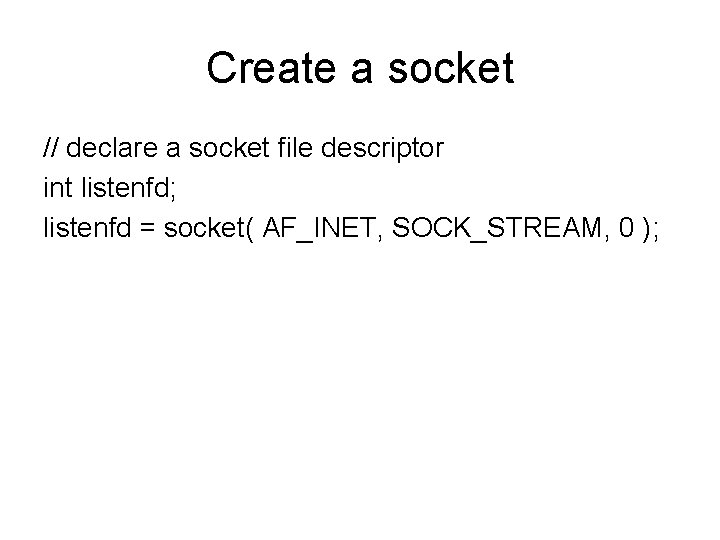
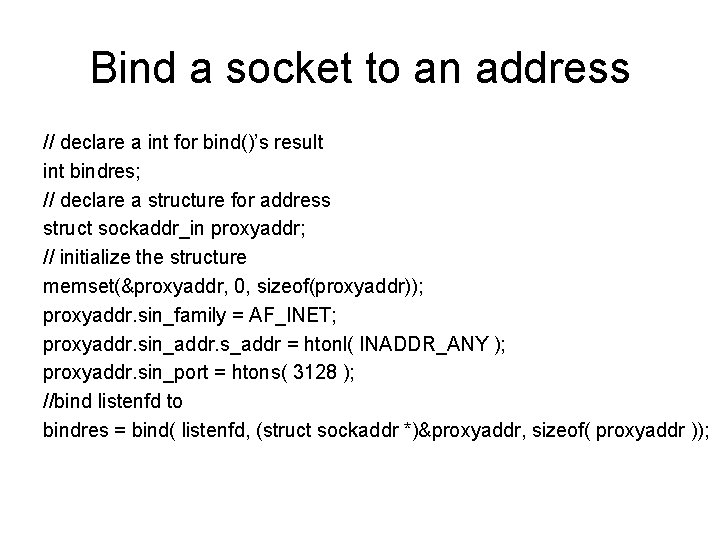
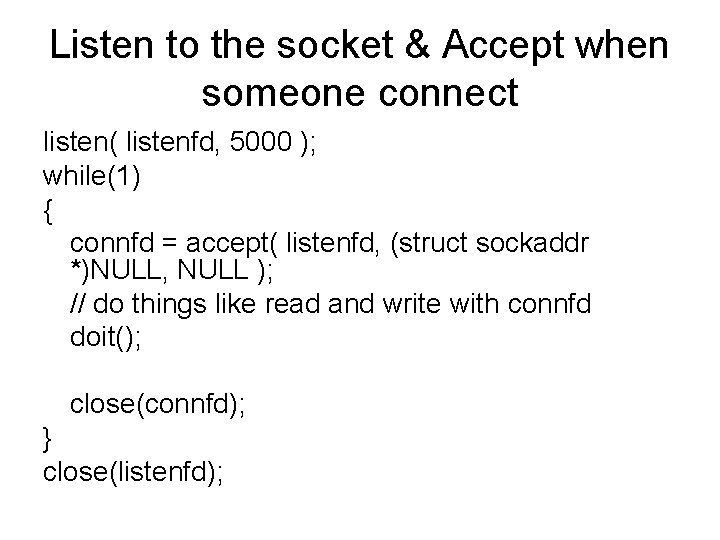
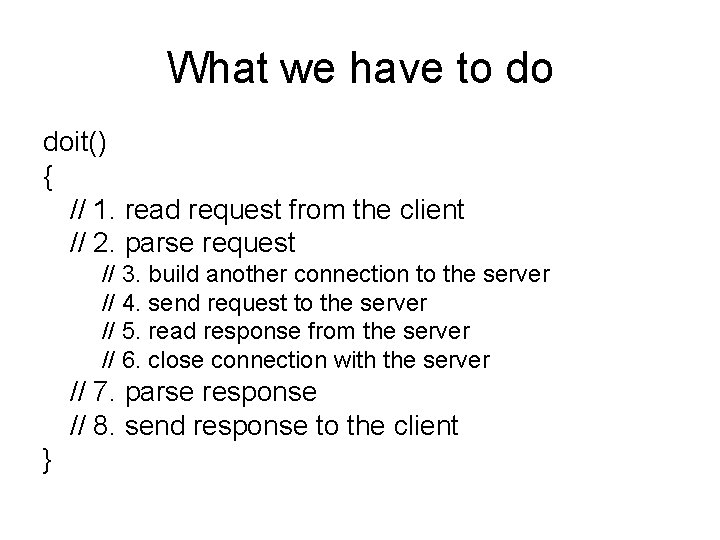
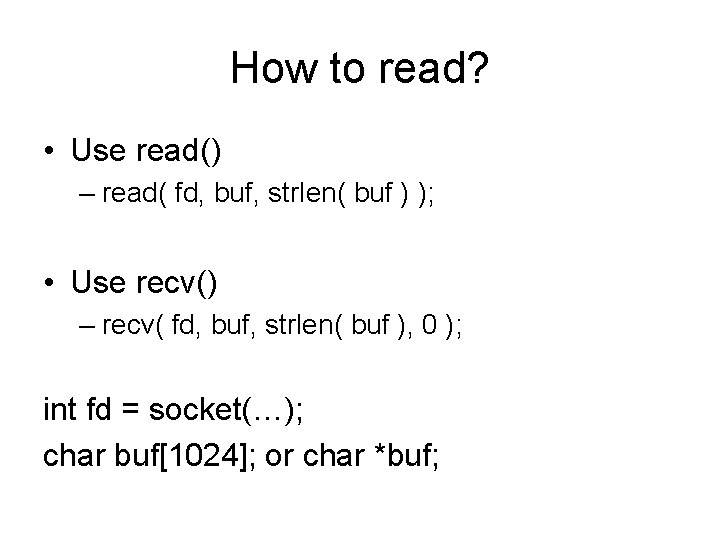
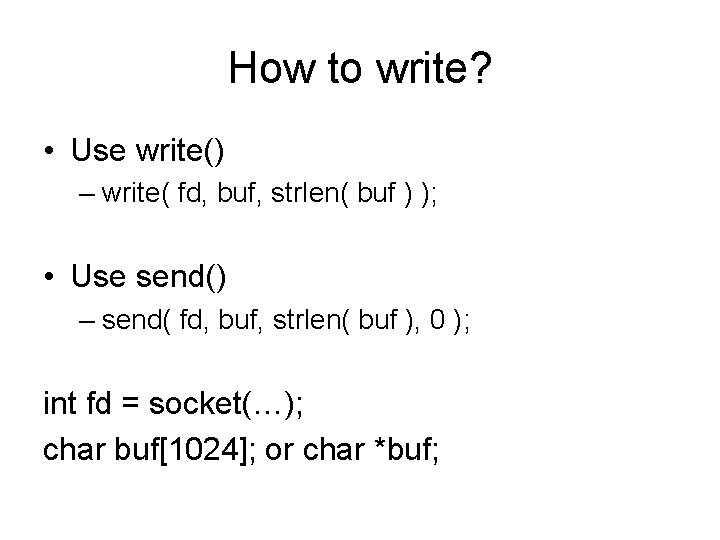
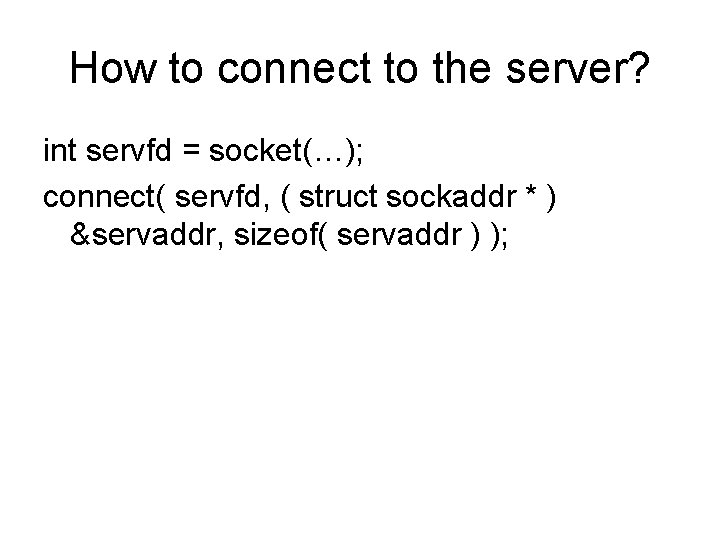
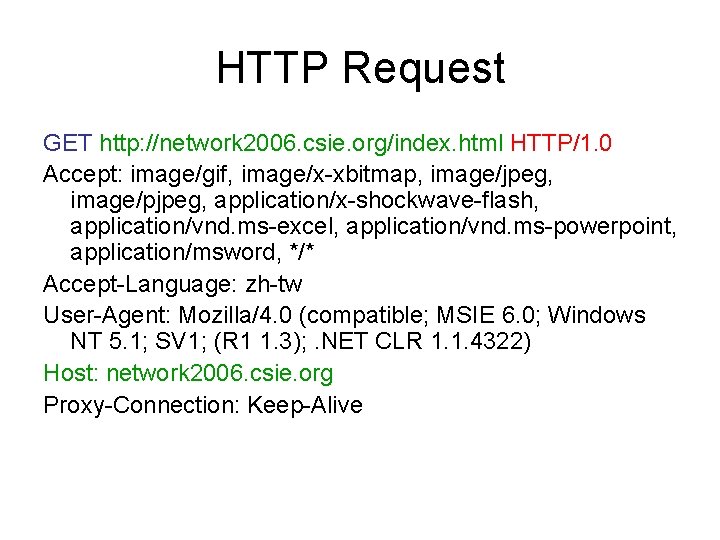
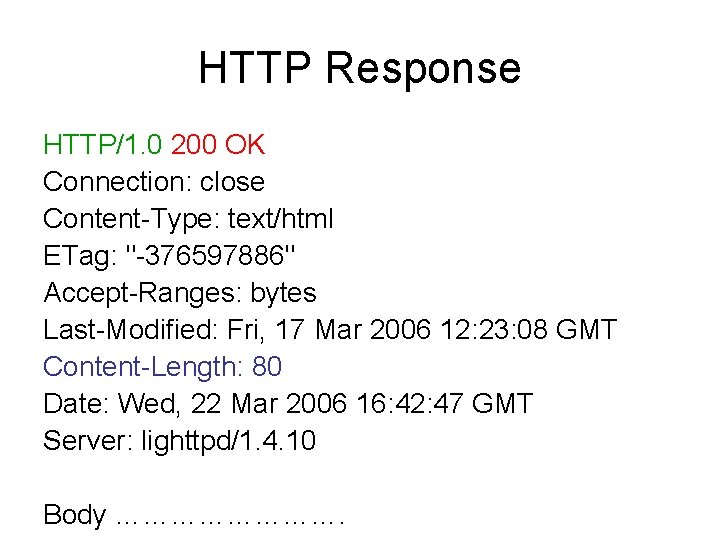
- Slides: 14
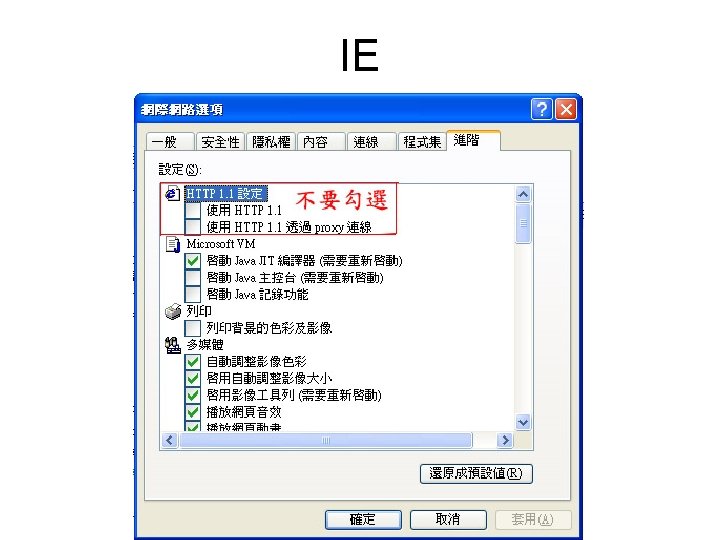
IE
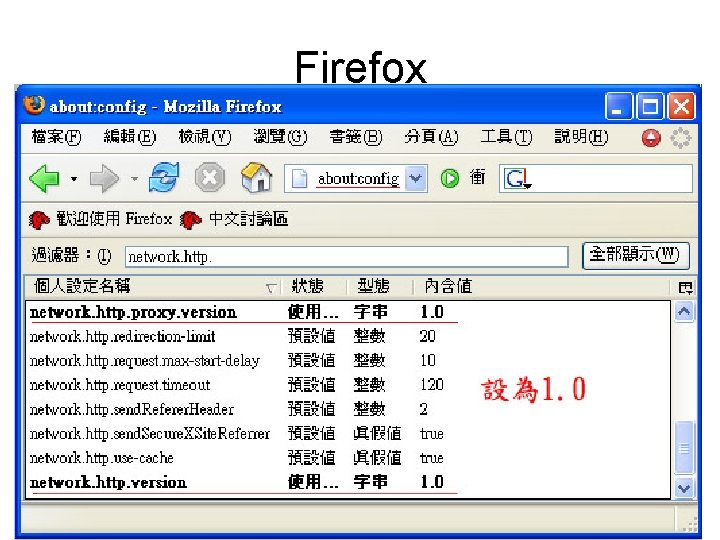
Firefox
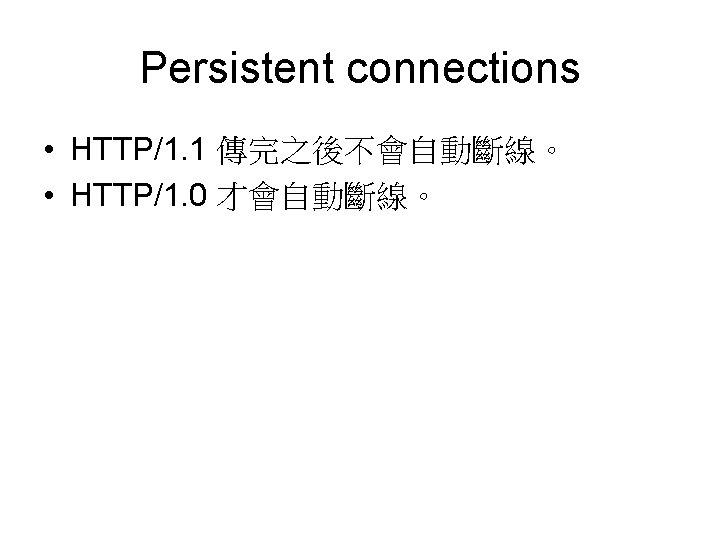
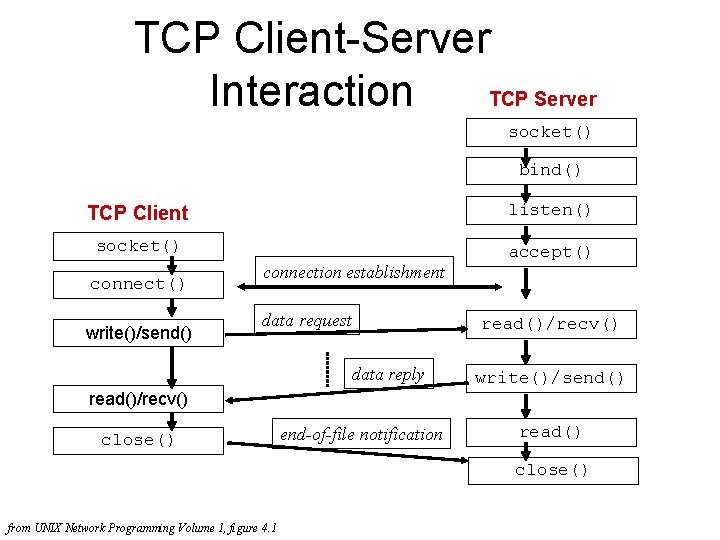
TCP Client-Server Interaction TCP Server socket() bind() TCP Client listen() socket() accept() connect() write()/send() connection establishment data request read()/recv() data reply write()/send() read()/recv() close() end-of-file notification read() close() from UNIX Network Programming Volume 1, figure 4. 1
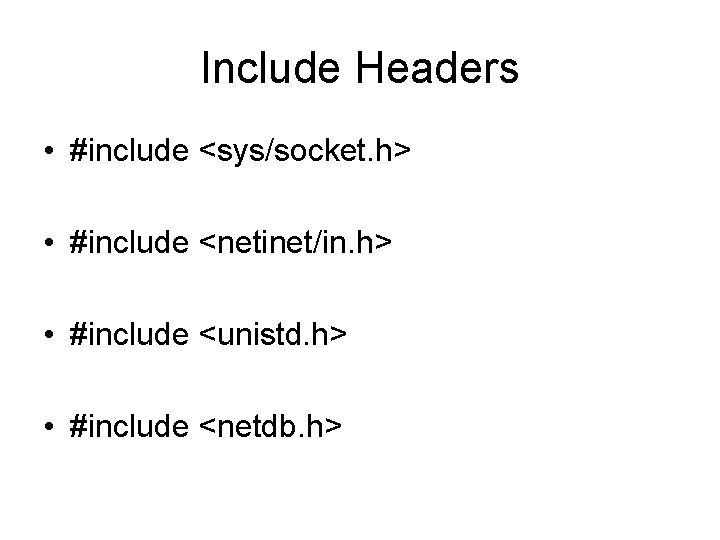
Include Headers • #include <sys/socket. h> • #include <netinet/in. h> • #include <unistd. h> • #include <netdb. h>
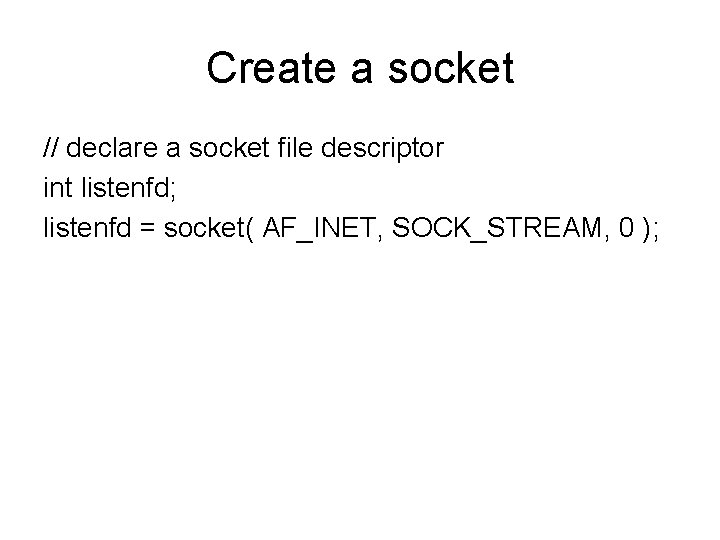
Create a socket // declare a socket file descriptor int listenfd; listenfd = socket( AF_INET, SOCK_STREAM, 0 );
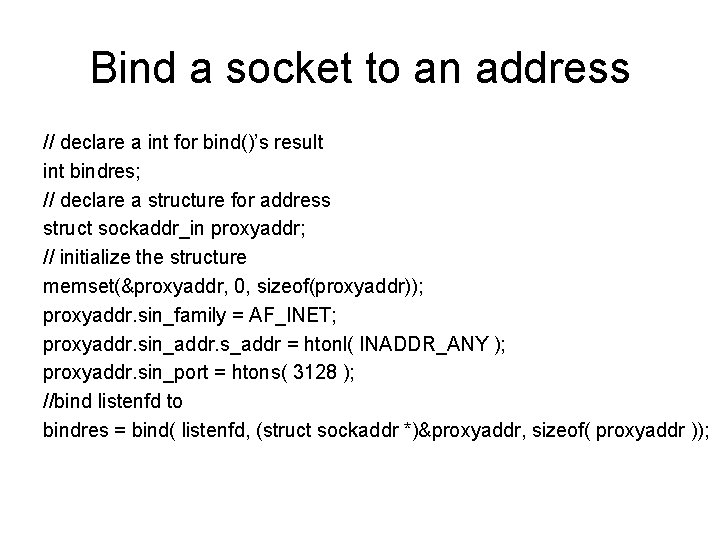
Bind a socket to an address // declare a int for bind()’s result int bindres; // declare a structure for address struct sockaddr_in proxyaddr; // initialize the structure memset(&proxyaddr, 0, sizeof(proxyaddr)); proxyaddr. sin_family = AF_INET; proxyaddr. sin_addr. s_addr = htonl( INADDR_ANY ); proxyaddr. sin_port = htons( 3128 ); //bind listenfd to bindres = bind( listenfd, (struct sockaddr *)&proxyaddr, sizeof( proxyaddr ));
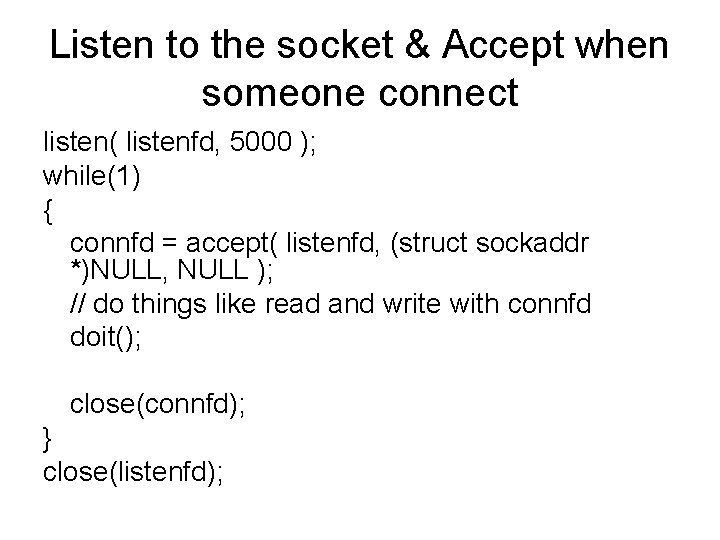
Listen to the socket & Accept when someone connect listen( listenfd, 5000 ); while(1) { connfd = accept( listenfd, (struct sockaddr *)NULL, NULL ); // do things like read and write with connfd doit(); close(connfd); } close(listenfd);
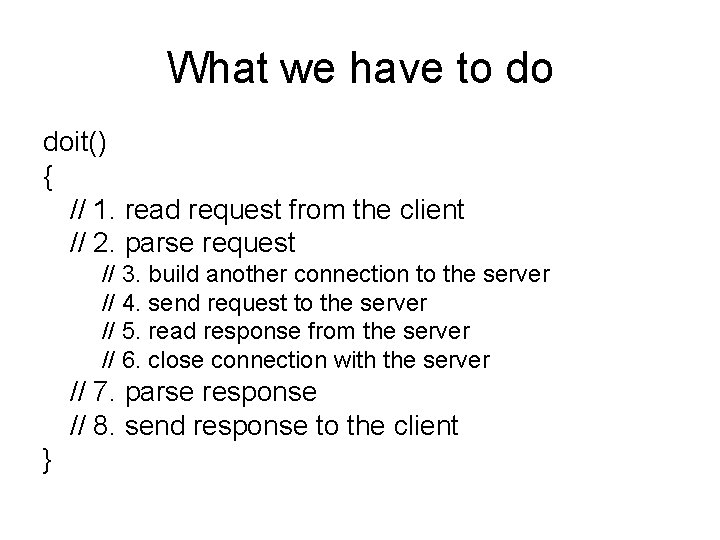
What we have to do doit() { // 1. read request from the client // 2. parse request // 3. build another connection to the server // 4. send request to the server // 5. read response from the server // 6. close connection with the server // 7. parse response // 8. send response to the client }
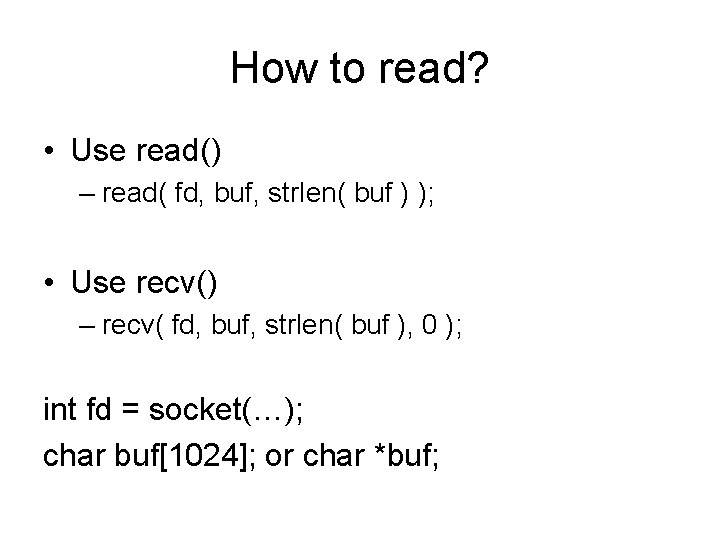
How to read? • Use read() – read( fd, buf, strlen( buf ) ); • Use recv() – recv( fd, buf, strlen( buf ), 0 ); int fd = socket(…); char buf[1024]; or char *buf;
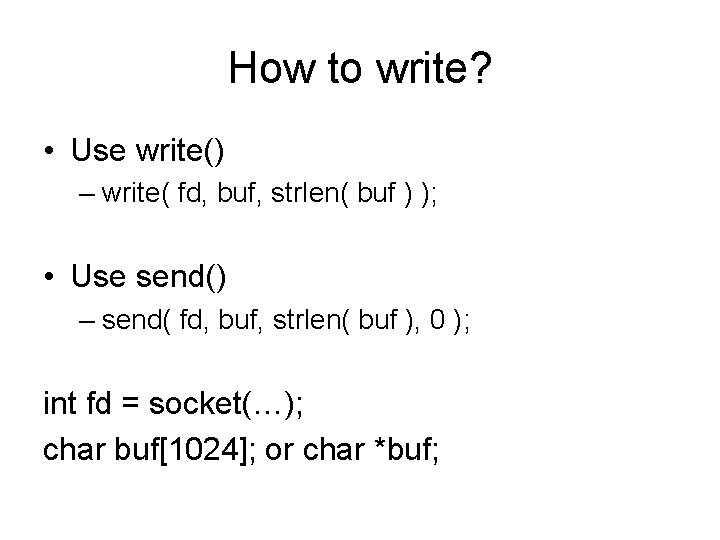
How to write? • Use write() – write( fd, buf, strlen( buf ) ); • Use send() – send( fd, buf, strlen( buf ), 0 ); int fd = socket(…); char buf[1024]; or char *buf;
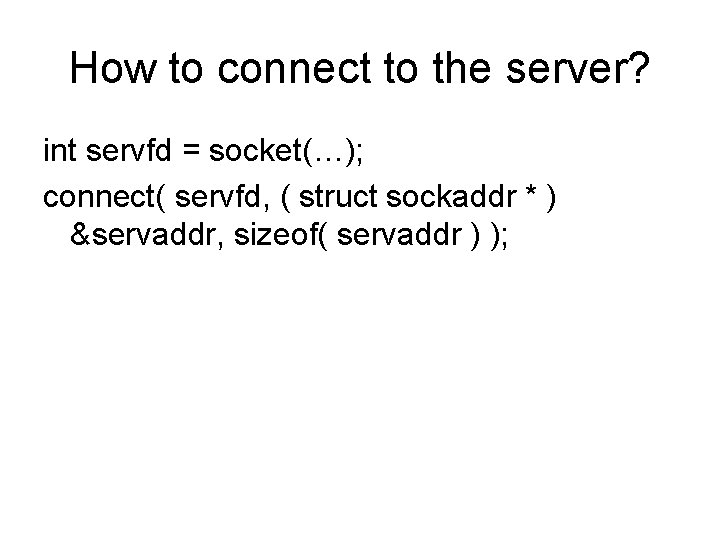
How to connect to the server? int servfd = socket(…); connect( servfd, ( struct sockaddr * ) &servaddr, sizeof( servaddr ) );
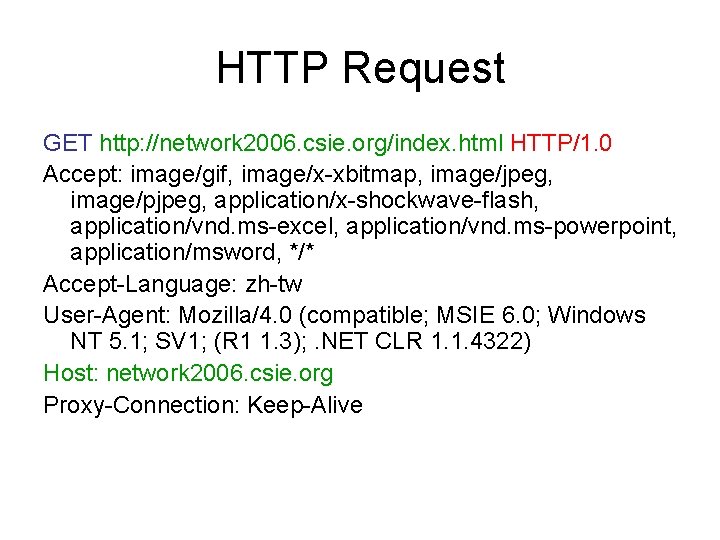
HTTP Request GET http: //network 2006. csie. org/index. html HTTP/1. 0 Accept: image/gif, image/x-xbitmap, image/jpeg, image/pjpeg, application/x-shockwave-flash, application/vnd. ms-excel, application/vnd. ms-powerpoint, application/msword, */* Accept-Language: zh-tw User-Agent: Mozilla/4. 0 (compatible; MSIE 6. 0; Windows NT 5. 1; SV 1; (R 1 1. 3); . NET CLR 1. 1. 4322) Host: network 2006. csie. org Proxy-Connection: Keep-Alive
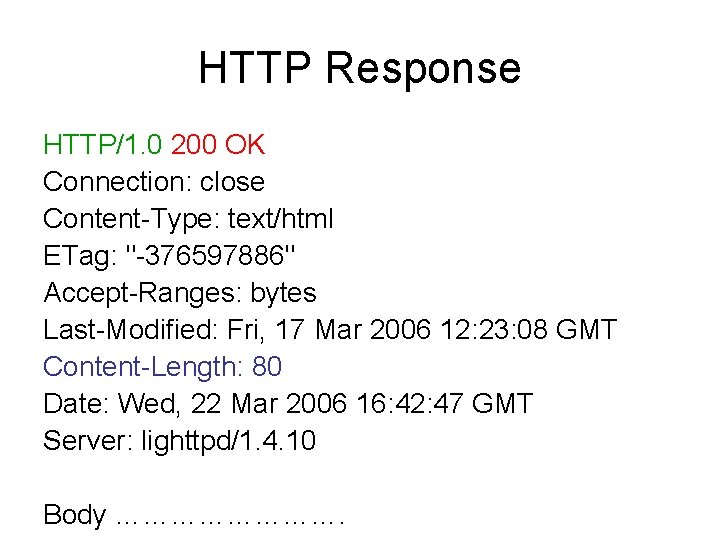
HTTP Response HTTP/1. 0 200 OK Connection: close Content-Type: text/html ETag: "-376597886" Accept-Ranges: bytes Last-Modified: Fri, 17 Mar 2006 12: 23: 08 GMT Content-Length: 80 Date: Wed, 22 Mar 2006 16: 42: 47 GMT Server: lighttpd/1. 4. 10 Body ………….
Lập trình socket giao tiếp tcp client/server java
Clientserver model
Basics of client server model and its applications
Clientserver model
What are the features of network operating system
Clientserver network
Java client server tutorial
Tcp socket options
Socket primitives of tcp
Socket server
Thread socket python
Tcp echo server
Modbus tcp server
The server program tells its tcp
Firefox o opera