GC 163011 Functional Programming Lecture 9 Higher Order
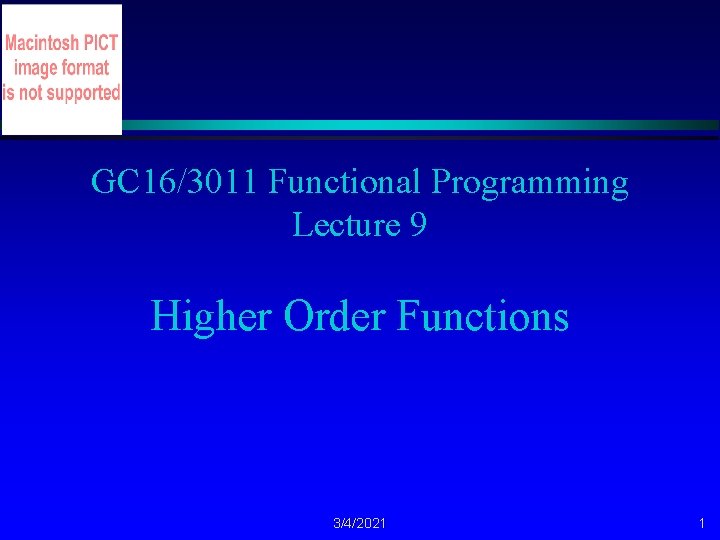
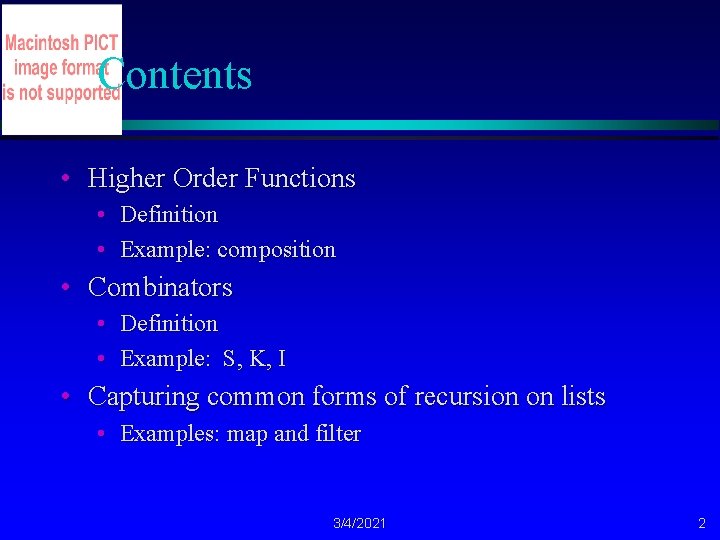
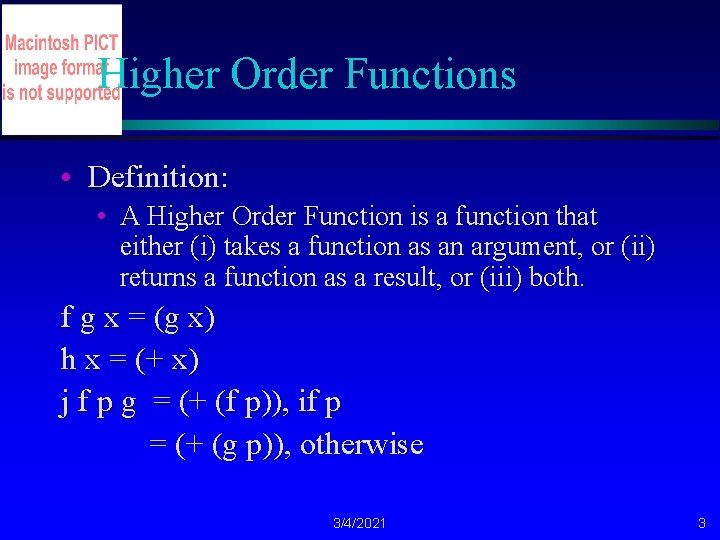
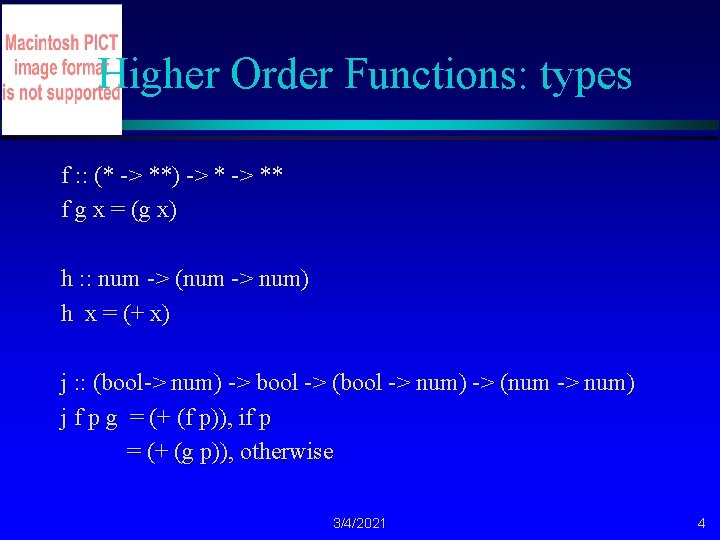
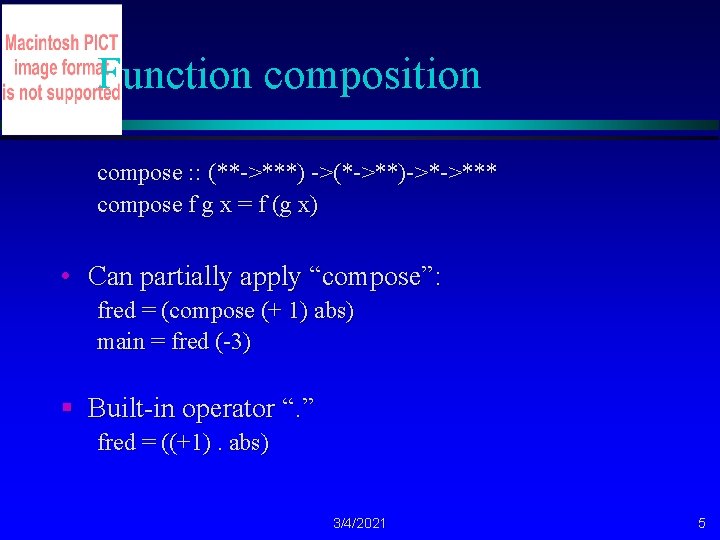
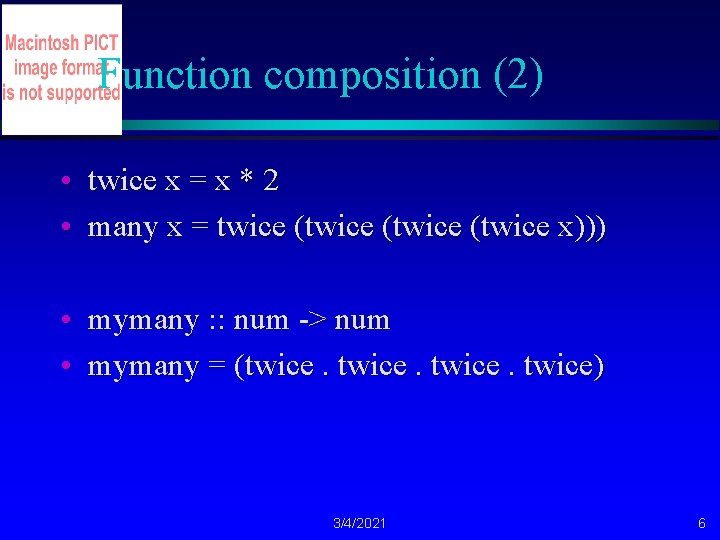
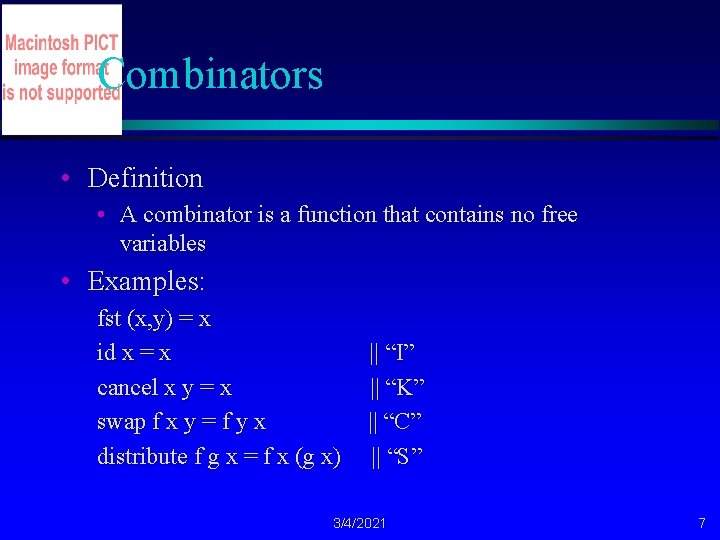
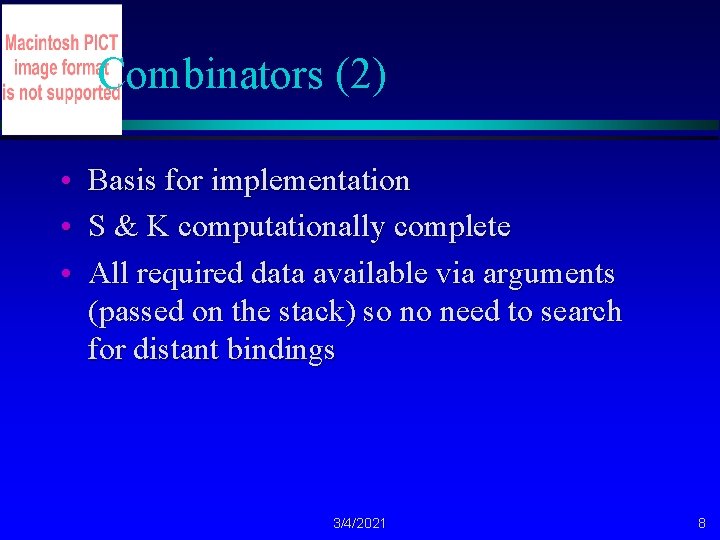
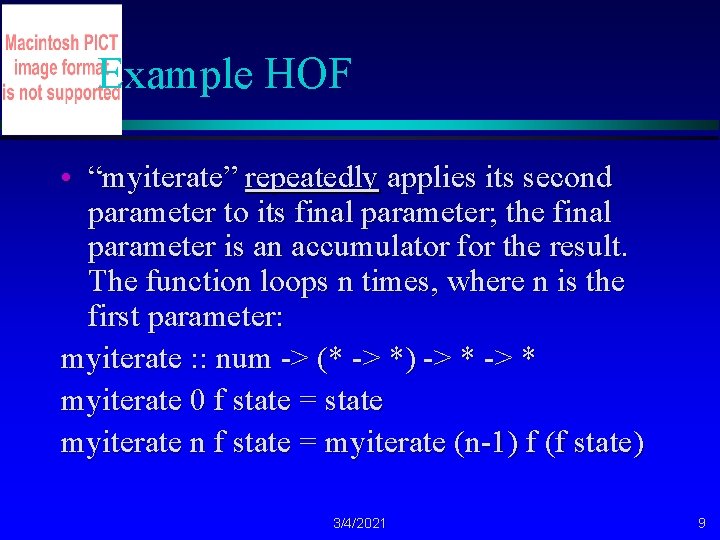
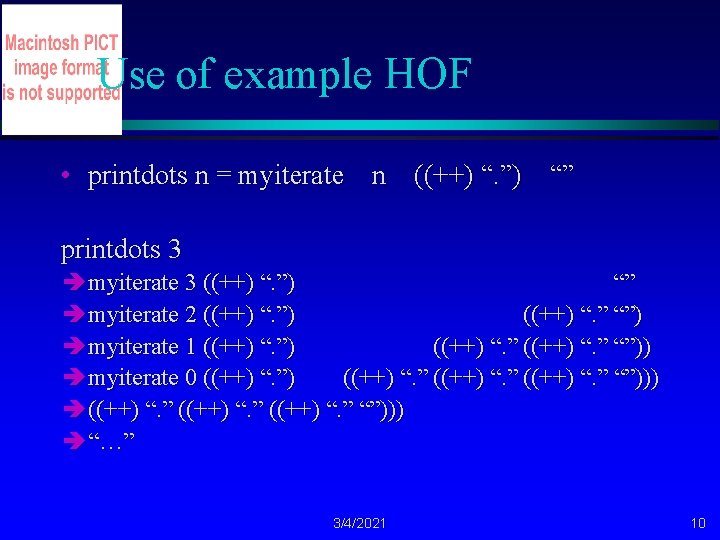
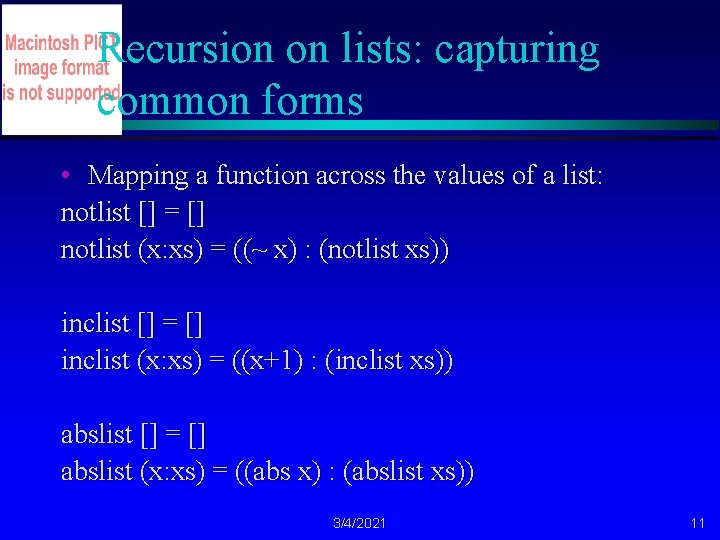
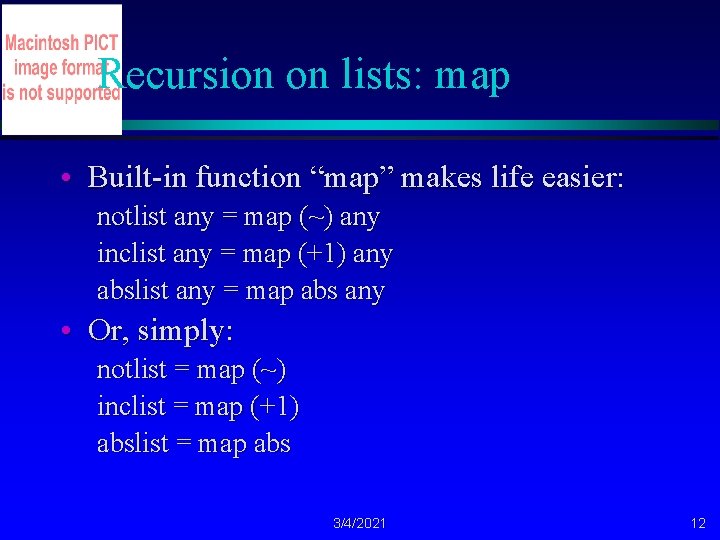
![Definition of map f [] = [] map f (x: xs) = ((f x): Definition of map f [] = [] map f (x: xs) = ((f x):](https://slidetodoc.com/presentation_image_h/24938c767264e590acda02080d0c5aa5/image-13.jpg)
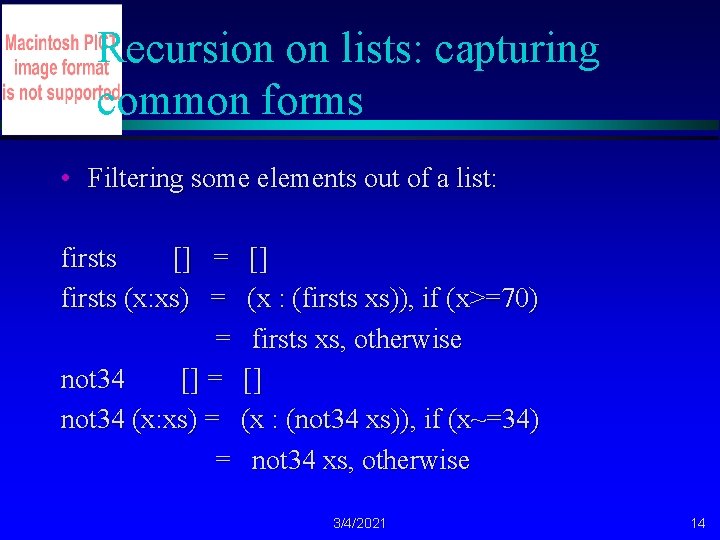
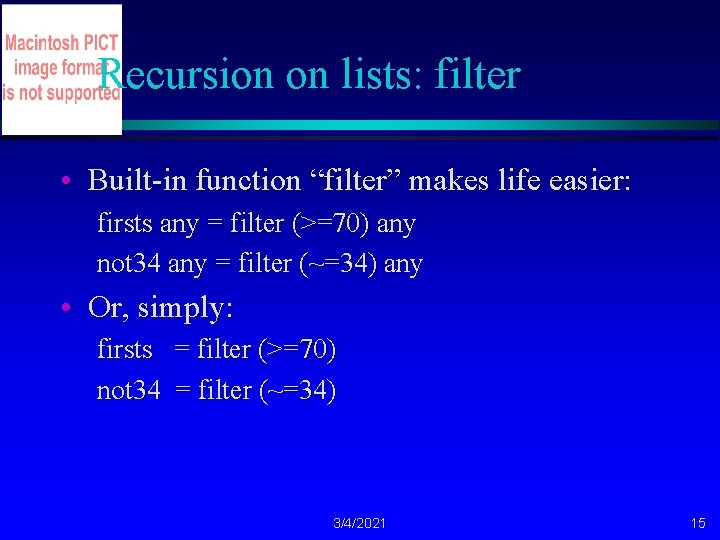
![Definition of filter f [] = [] filter f (x: xs) = (x: (filter Definition of filter f [] = [] filter f (x: xs) = (x: (filter](https://slidetodoc.com/presentation_image_h/24938c767264e590acda02080d0c5aa5/image-16.jpg)
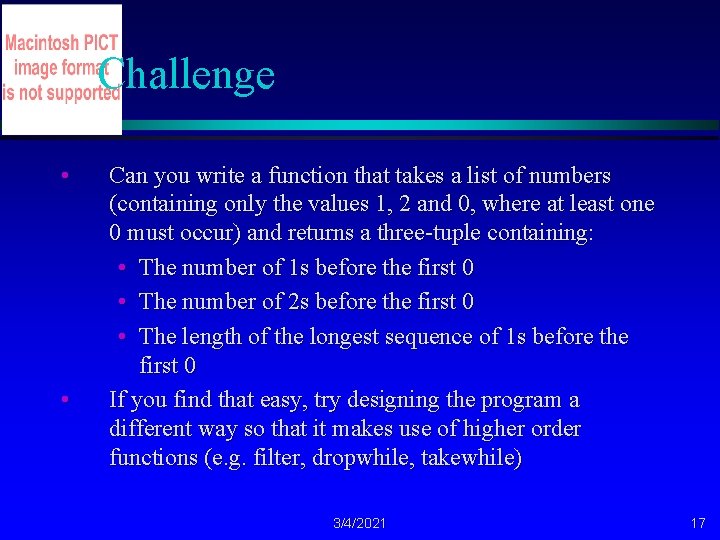
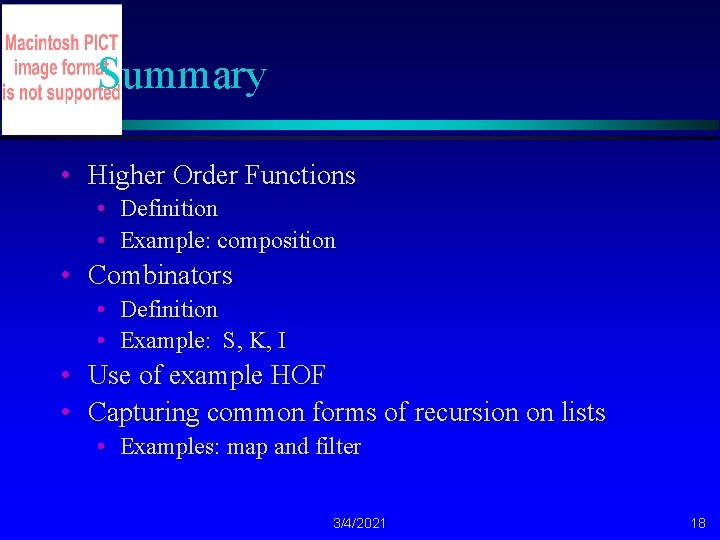
- Slides: 18
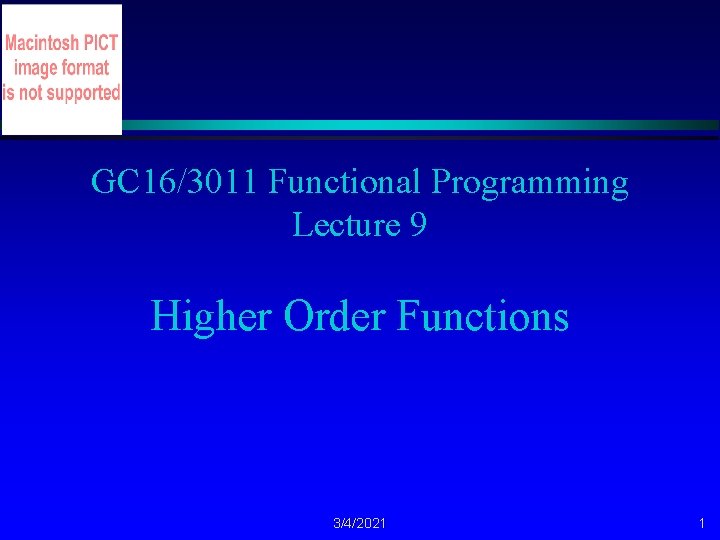
GC 16/3011 Functional Programming Lecture 9 Higher Order Functions 3/4/2021 1
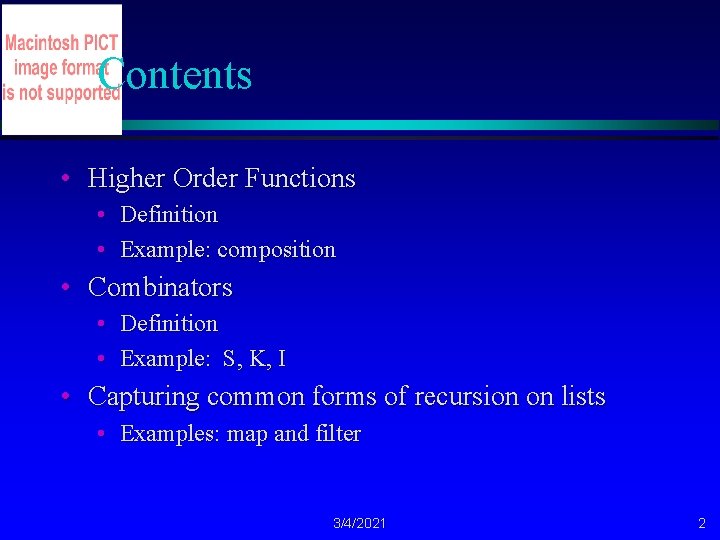
Contents • Higher Order Functions • Definition • Example: composition • Combinators • Definition • Example: S, K, I • Capturing common forms of recursion on lists • Examples: map and filter 3/4/2021 2
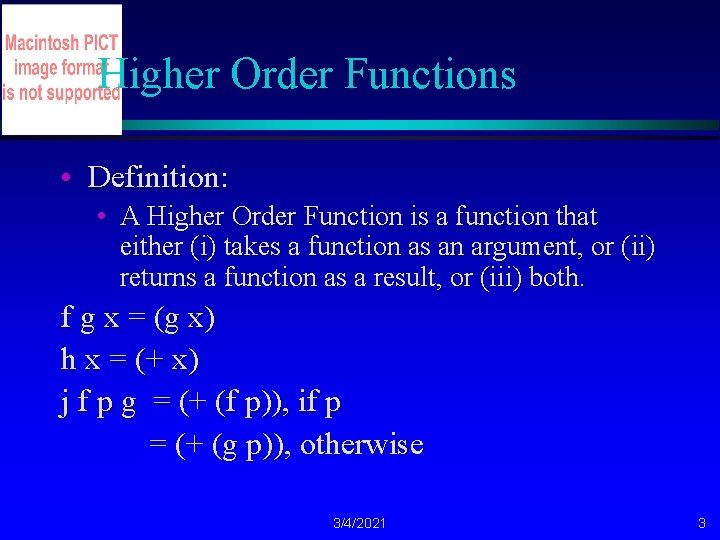
Higher Order Functions • Definition: • A Higher Order Function is a function that either (i) takes a function as an argument, or (ii) returns a function as a result, or (iii) both. f g x = (g x) h x = (+ x) j f p g = (+ (f p)), if p = (+ (g p)), otherwise 3/4/2021 3
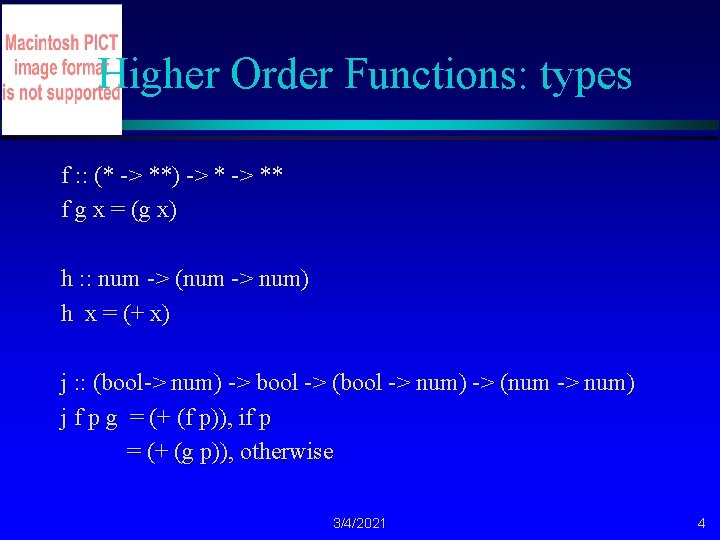
Higher Order Functions: types f : : (* -> **) -> ** f g x = (g x) h : : num -> (num -> num) h x = (+ x) j : : (bool-> num) -> bool -> (bool -> num) -> (num -> num) j f p g = (+ (f p)), if p = (+ (g p)), otherwise 3/4/2021 4
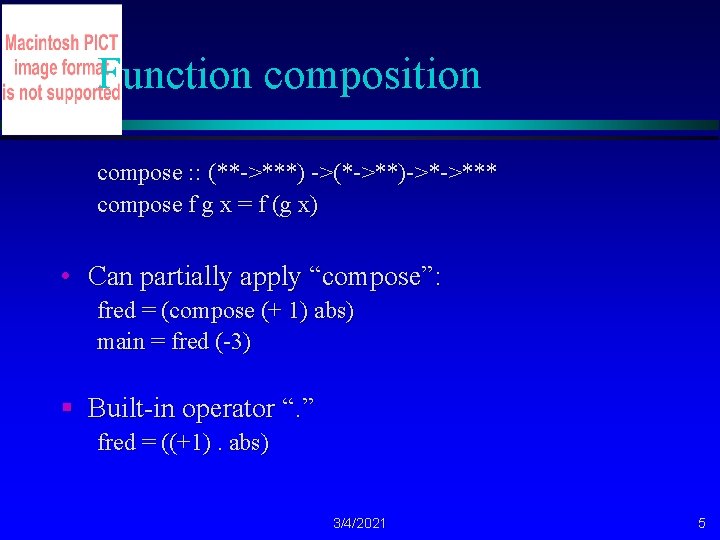
Function composition compose : : (**->***) ->(*->**)->*->*** compose f g x = f (g x) • Can partially apply “compose”: fred = (compose (+ 1) abs) main = fred (-3) § Built-in operator “. ” fred = ((+1). abs) 3/4/2021 5
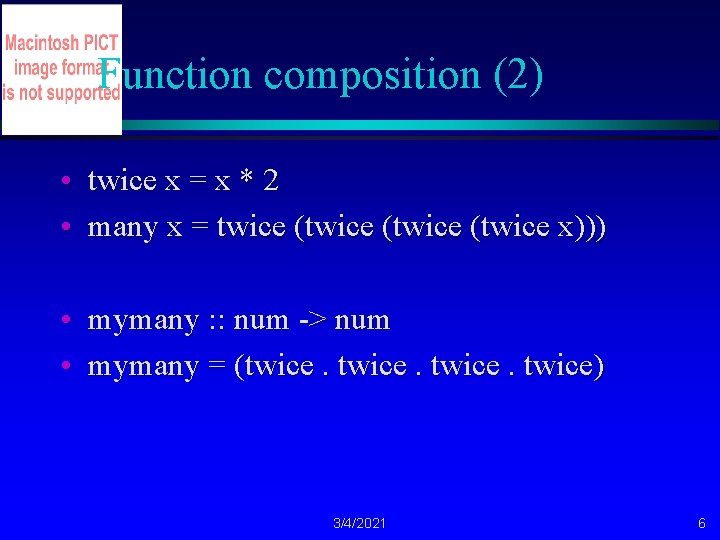
Function composition (2) • twice x = x * 2 • many x = twice (twice x))) • mymany : : num -> num • mymany = (twice. twice) 3/4/2021 6
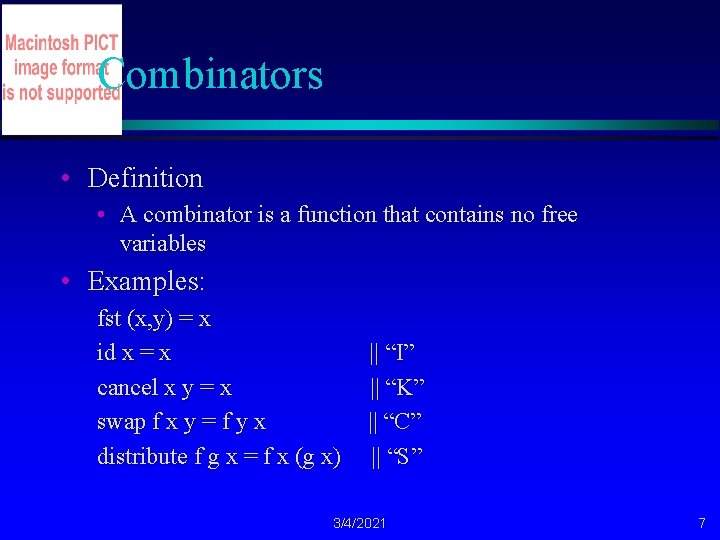
Combinators • Definition • A combinator is a function that contains no free variables • Examples: fst (x, y) = x id x = x cancel x y = x swap f x y = f y x distribute f g x = f x (g x) || “I” || “K” || “C” || “S” 3/4/2021 7
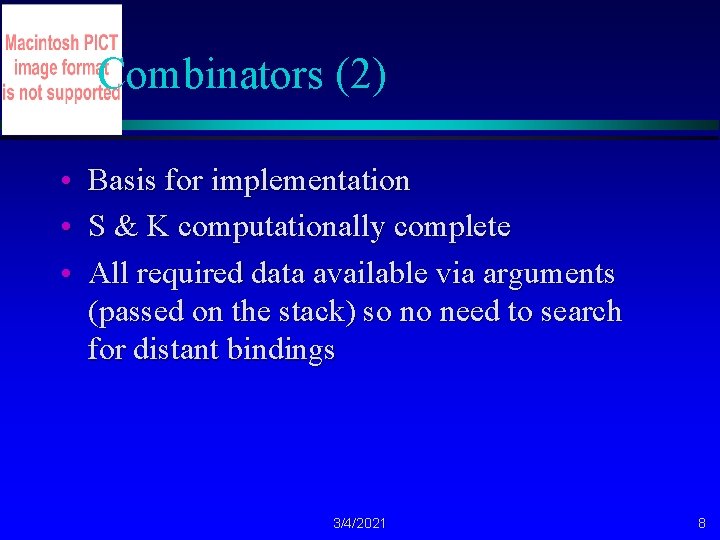
Combinators (2) • Basis for implementation • S & K computationally complete • All required data available via arguments (passed on the stack) so no need to search for distant bindings 3/4/2021 8
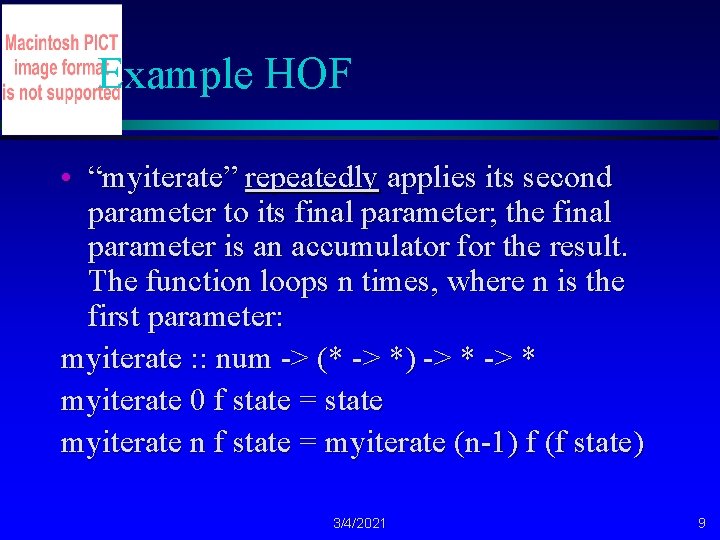
Example HOF • “myiterate” repeatedly applies its second parameter to its final parameter; the final parameter is an accumulator for the result. The function loops n times, where n is the first parameter: myiterate : : num -> (* -> *) -> * myiterate 0 f state = state myiterate n f state = myiterate (n-1) f (f state) 3/4/2021 9
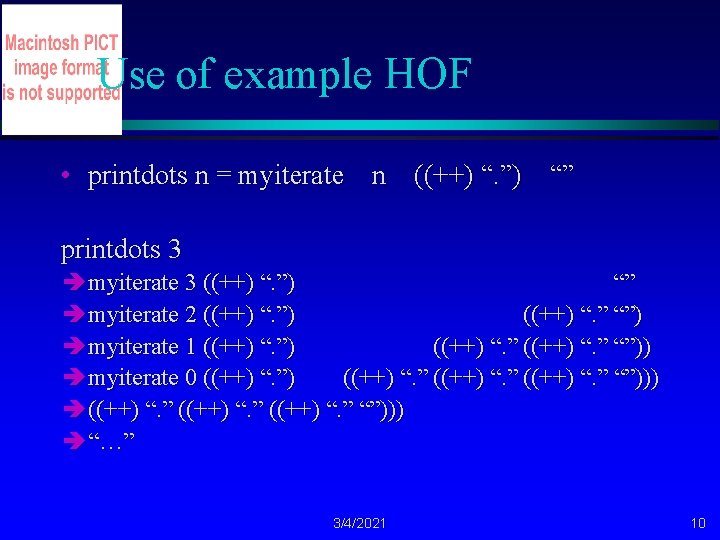
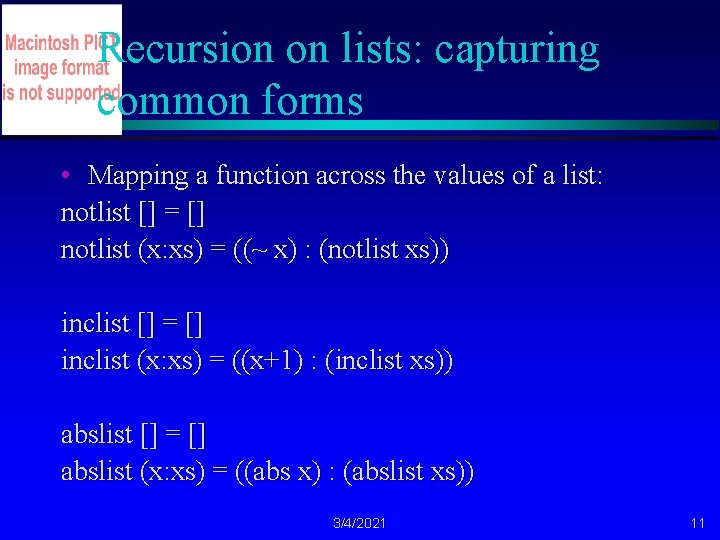
Recursion on lists: capturing common forms • Mapping a function across the values of a list: notlist [] = [] notlist (x: xs) = ((~ x) : (notlist xs)) inclist [] = [] inclist (x: xs) = ((x+1) : (inclist xs)) abslist [] = [] abslist (x: xs) = ((abs x) : (abslist xs)) 3/4/2021 11
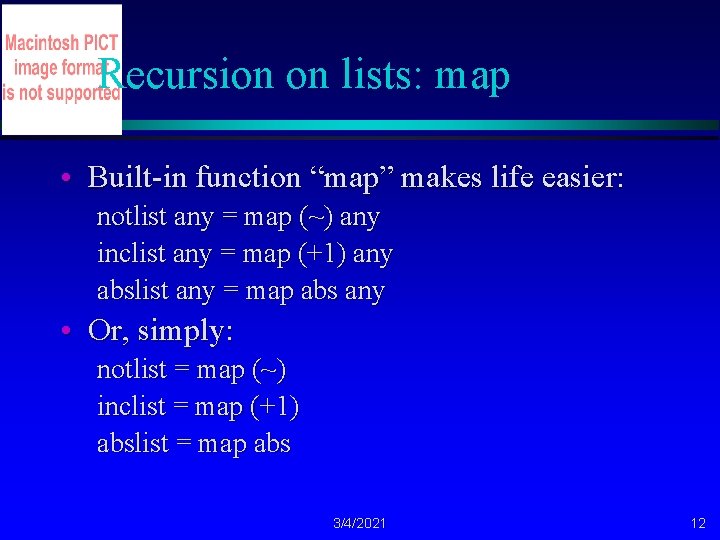
Recursion on lists: map • Built-in function “map” makes life easier: notlist any = map (~) any inclist any = map (+1) any abslist any = map abs any • Or, simply: notlist = map (~) inclist = map (+1) abslist = map abs 3/4/2021 12
![Definition of map f map f x xs f x Definition of map f [] = [] map f (x: xs) = ((f x):](https://slidetodoc.com/presentation_image_h/24938c767264e590acda02080d0c5aa5/image-13.jpg)
Definition of map f [] = [] map f (x: xs) = ((f x): (map f xs)) • So, what’s the type of map? • Write it here: 3/4/2021 13
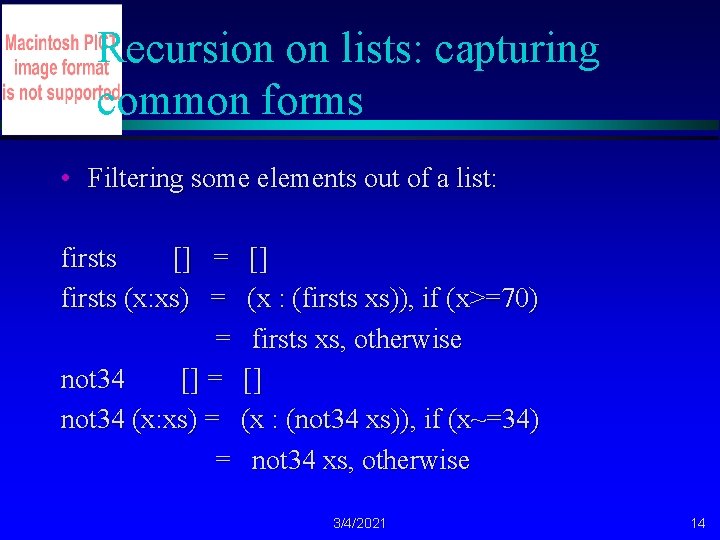
Recursion on lists: capturing common forms • Filtering some elements out of a list: firsts [] firsts (x: xs) = = = not 34 [] = not 34 (x: xs) = = [] (x : (firsts xs)), if (x>=70) firsts xs, otherwise [] (x : (not 34 xs)), if (x~=34) not 34 xs, otherwise 3/4/2021 14
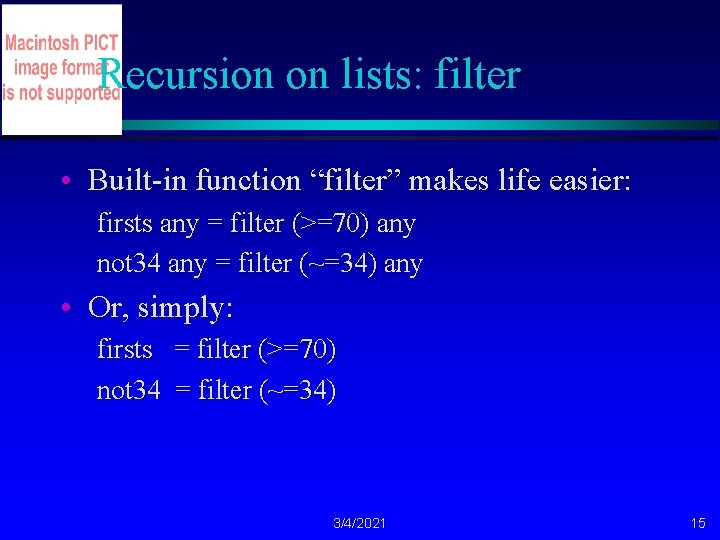
Recursion on lists: filter • Built-in function “filter” makes life easier: firsts any = filter (>=70) any not 34 any = filter (~=34) any • Or, simply: firsts = filter (>=70) not 34 = filter (~=34) 3/4/2021 15
![Definition of filter f filter f x xs x filter Definition of filter f [] = [] filter f (x: xs) = (x: (filter](https://slidetodoc.com/presentation_image_h/24938c767264e590acda02080d0c5aa5/image-16.jpg)
Definition of filter f [] = [] filter f (x: xs) = (x: (filter f xs)), if (f x) = filter f xs, otherwise • So, what’s the type of filter? • Write it here: 3/4/2021 16
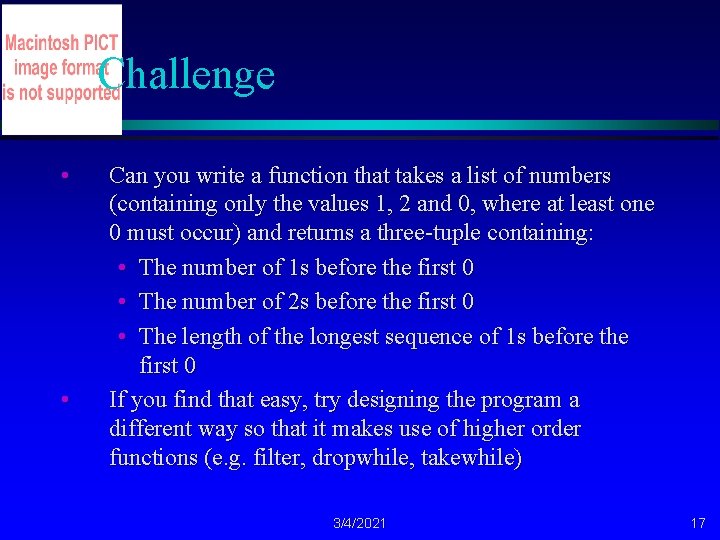
Challenge • • Can you write a function that takes a list of numbers (containing only the values 1, 2 and 0, where at least one 0 must occur) and returns a three-tuple containing: • The number of 1 s before the first 0 • The number of 2 s before the first 0 • The length of the longest sequence of 1 s before the first 0 If you find that easy, try designing the program a different way so that it makes use of higher order functions (e. g. filter, dropwhile, takewhile) 3/4/2021 17
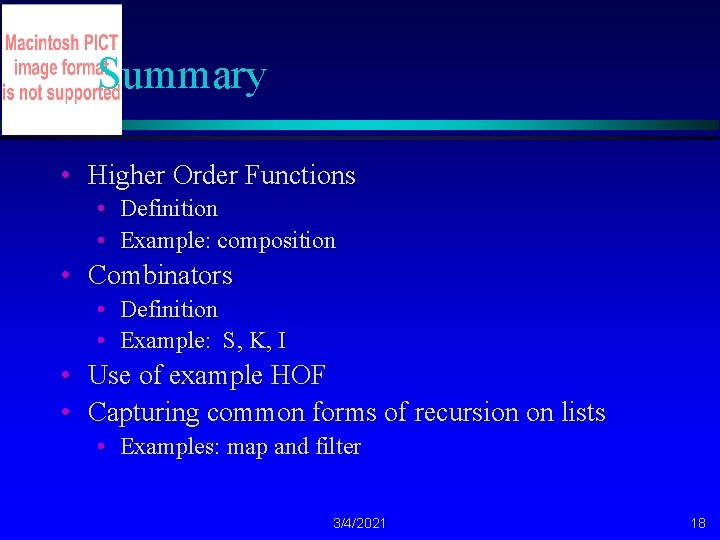
Summary • Higher Order Functions • Definition • Example: composition • Combinators • Definition • Example: S, K, I • Use of example HOF • Capturing common forms of recursion on lists • Examples: map and filter 3/4/2021 18
Via optica
01:640:244 lecture notes - lecture 15: plat, idah, farad
C data types with examples
Space maintainer types
Non functional plasma enzyme
Plasma enzyme
Functional and non functional
Functional programming vs object oriented
Fundamentals of functional programming
Scheme functional programming
Elm programming language
Functional programming roadmap
Lisp functional programming
Typed functional programming
Fundamentals of functional programming language
Xkcd programming language
Adt functional programming
Is lisp a functional programming language
Functional decomposition programming