Deadlock and Starvation Deadlock two or more processes
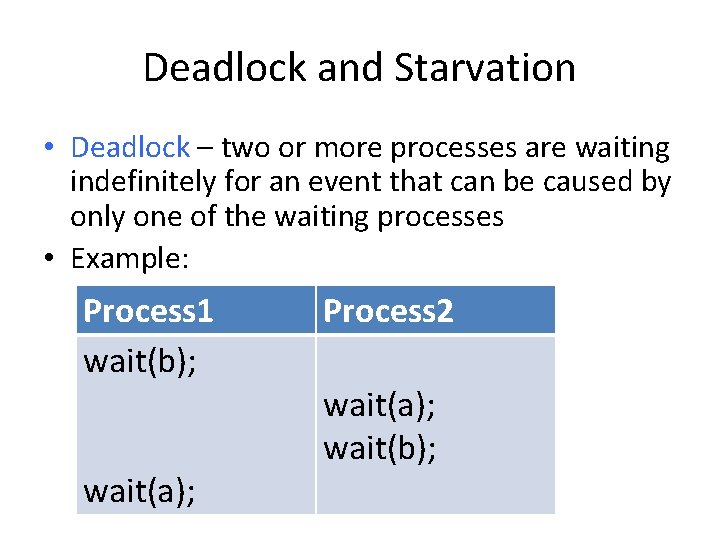
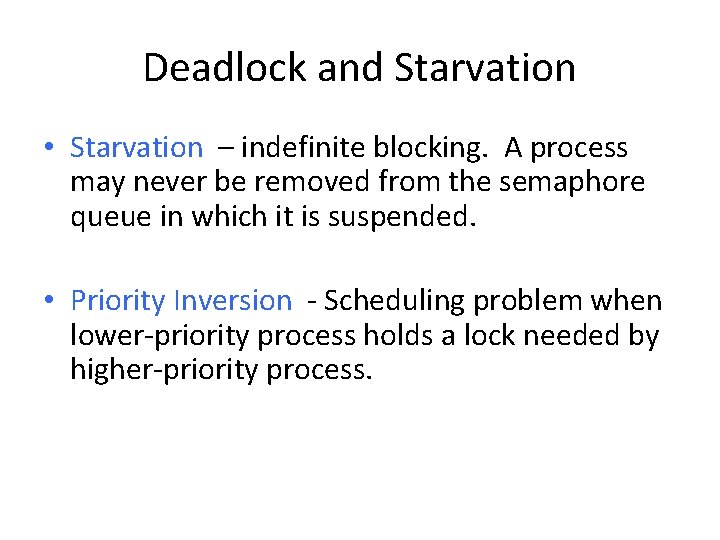
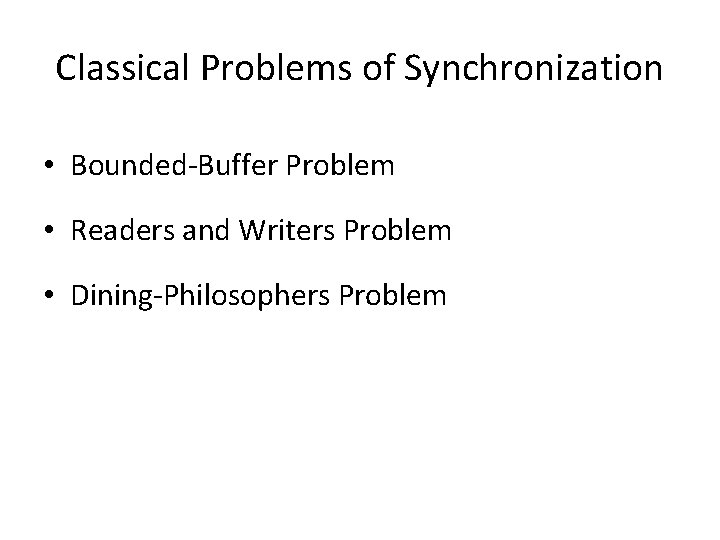
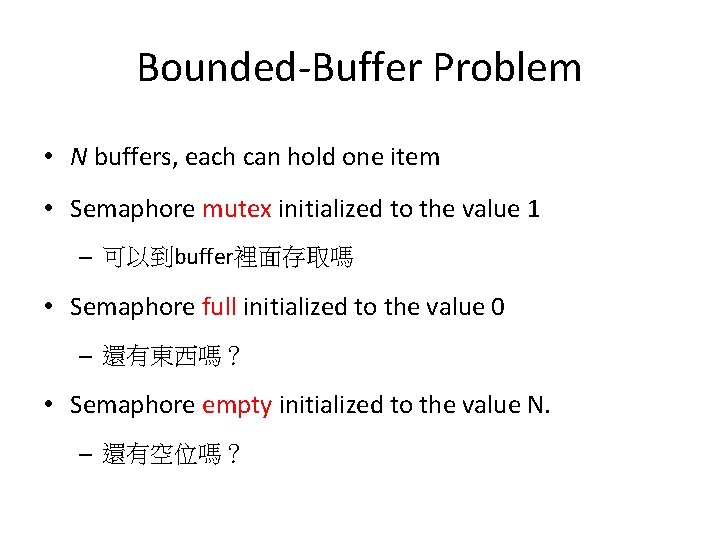
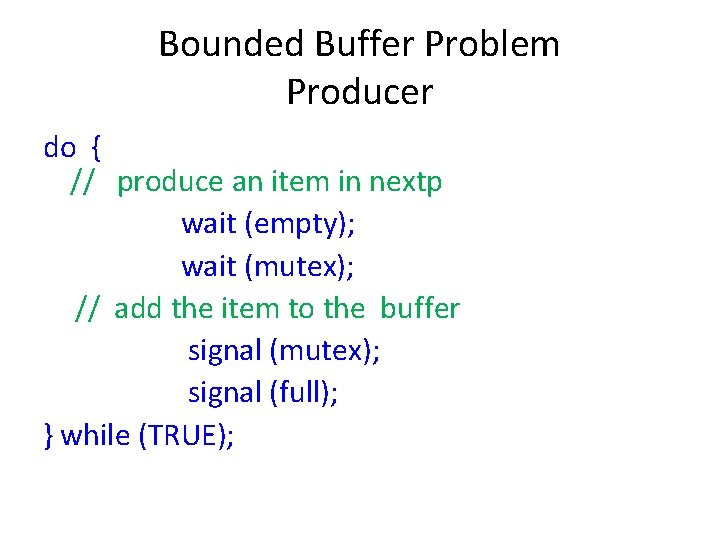
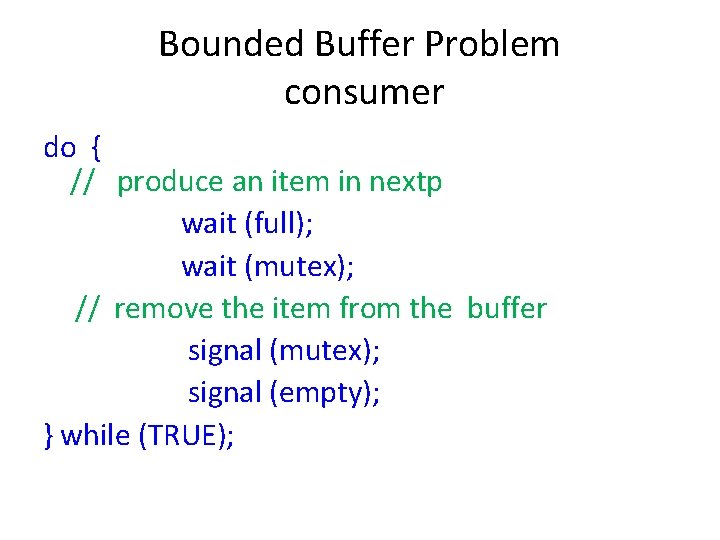
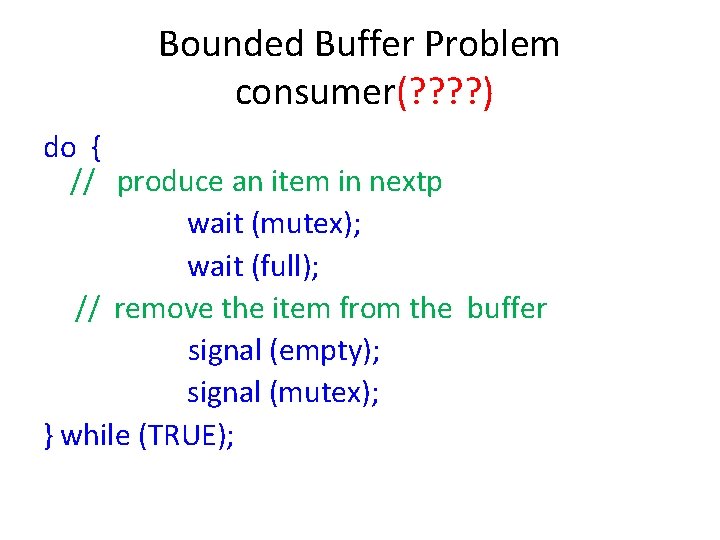
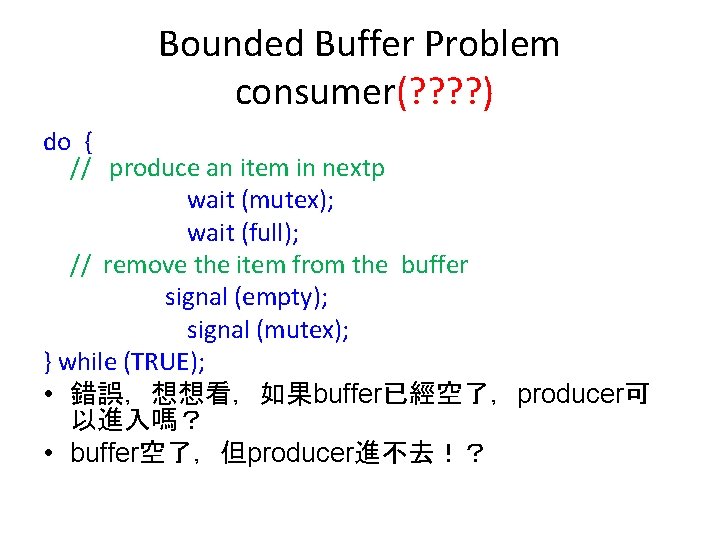
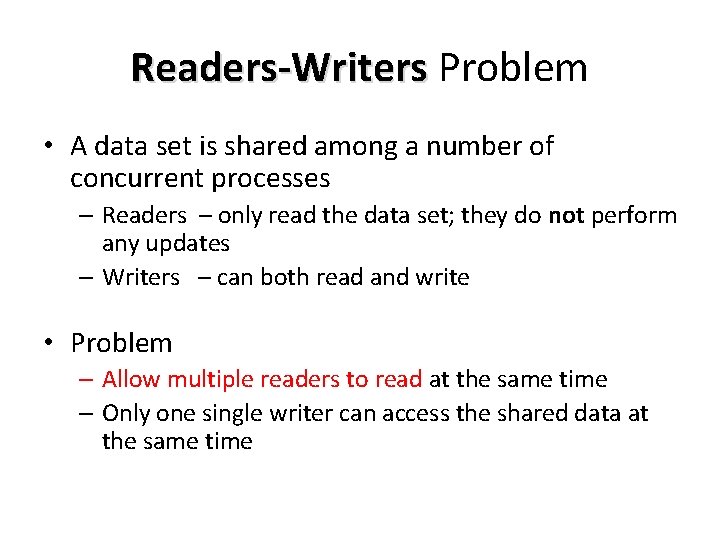
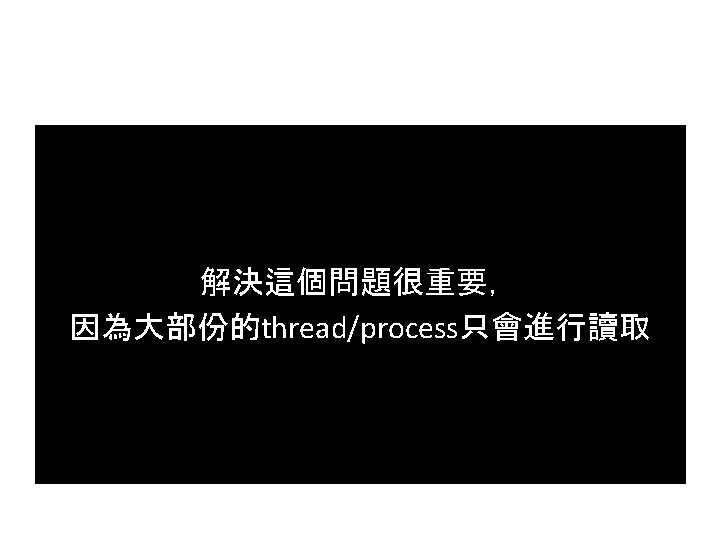
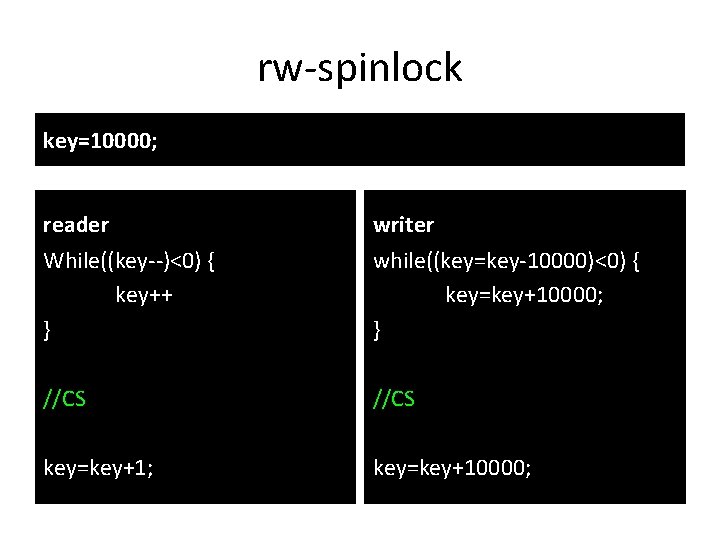
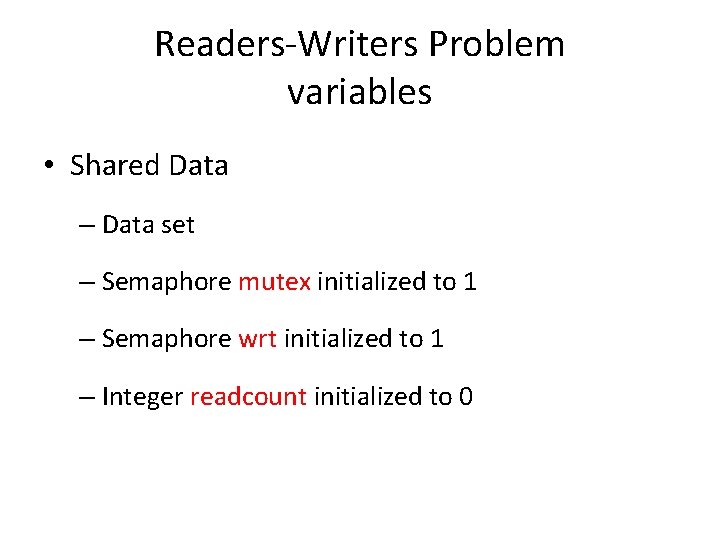
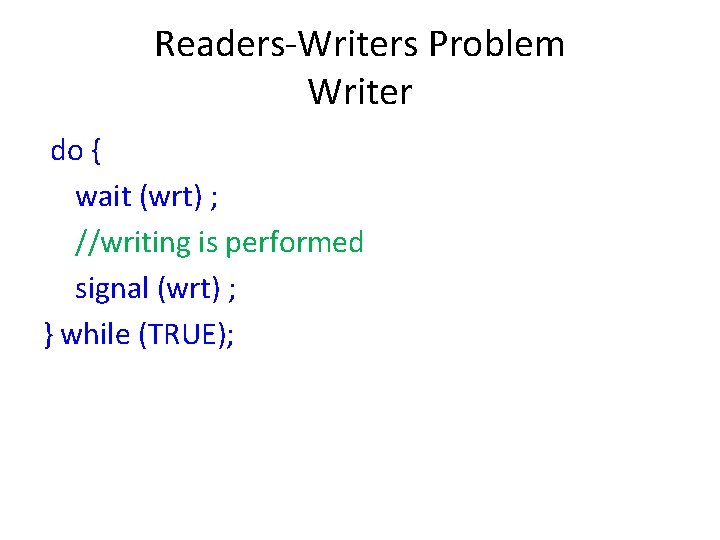
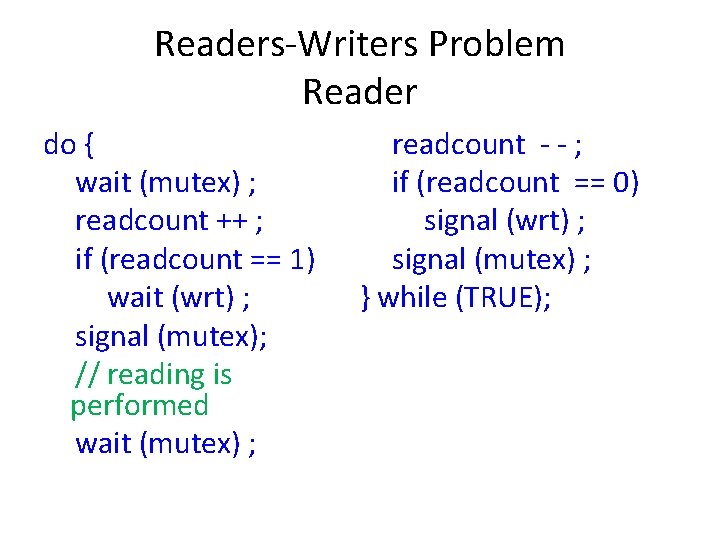
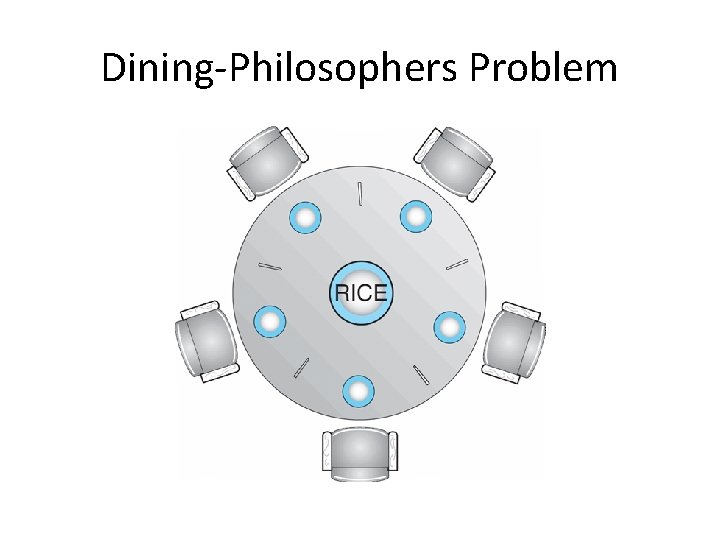
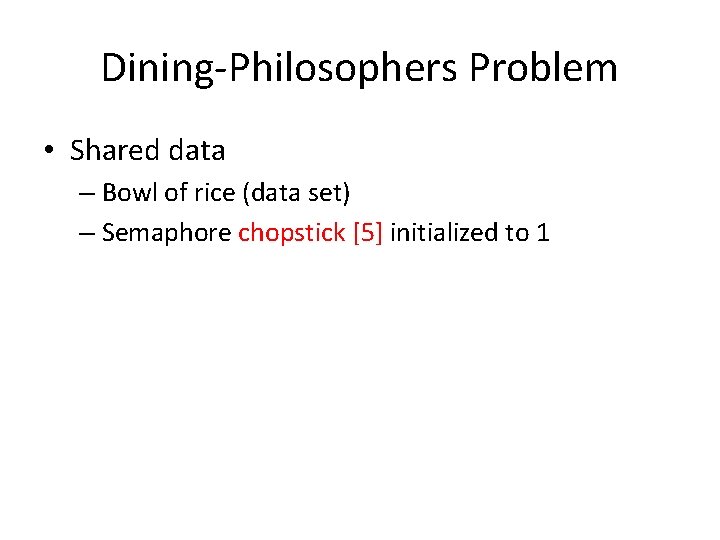
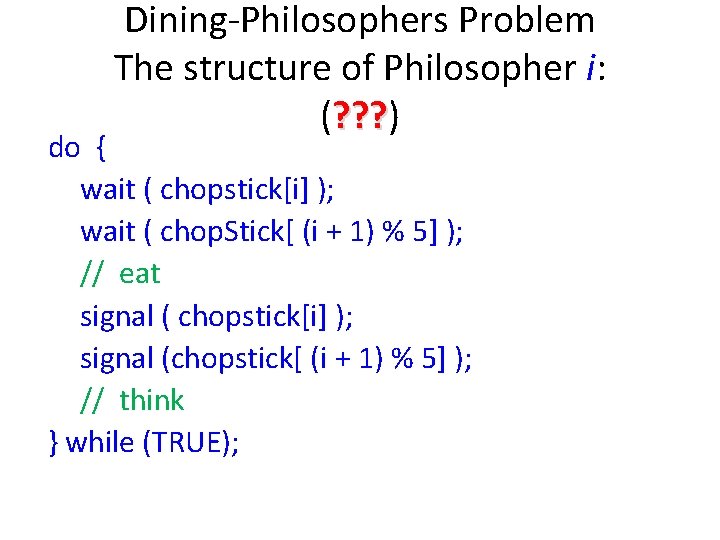
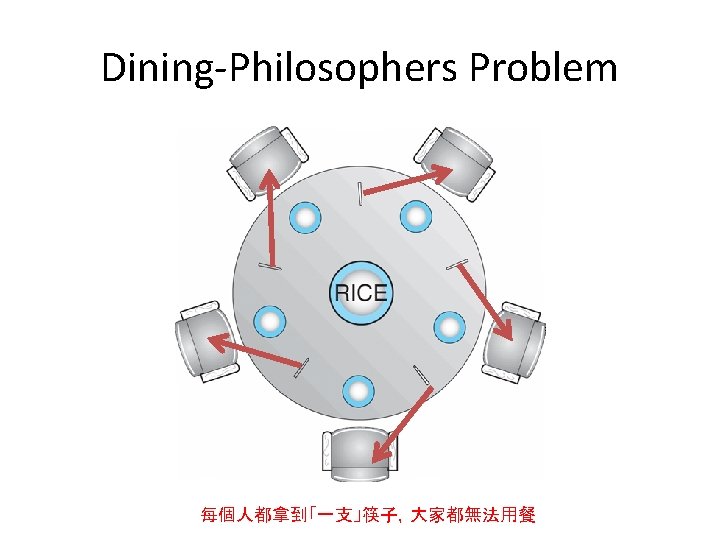
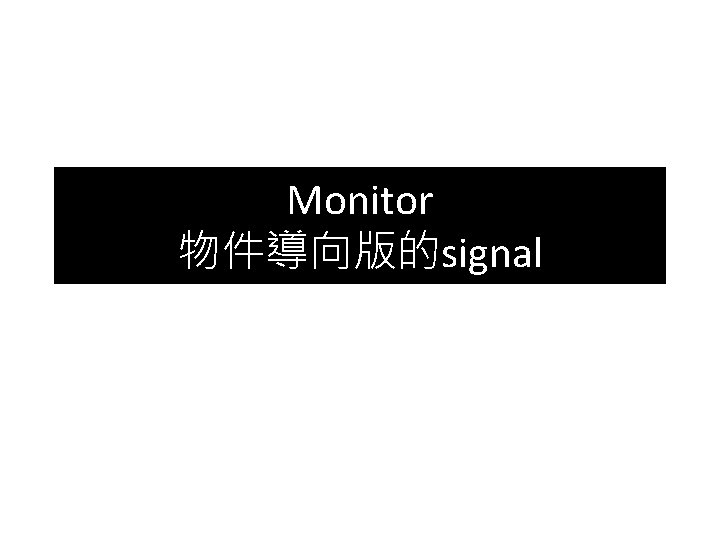
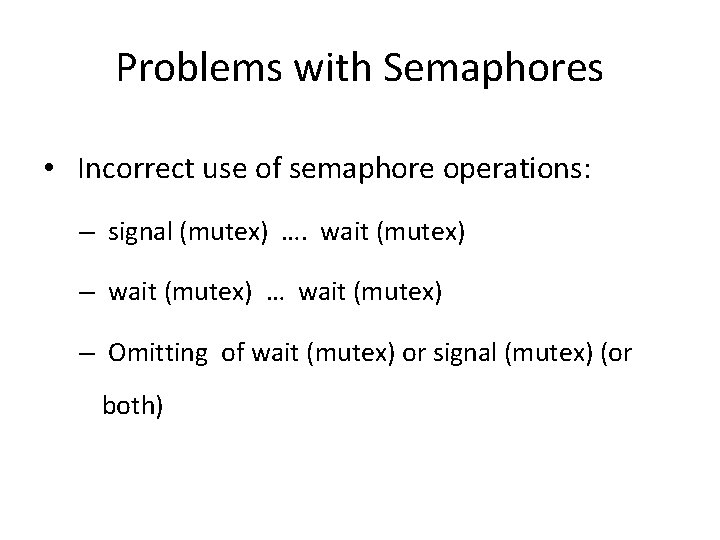
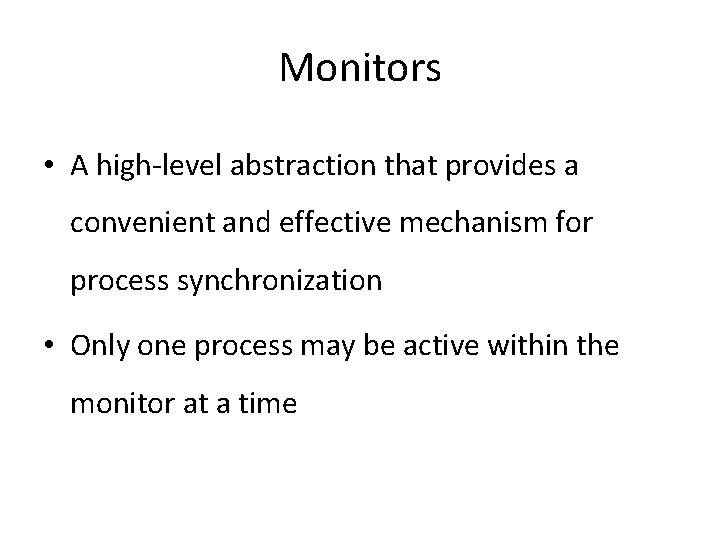
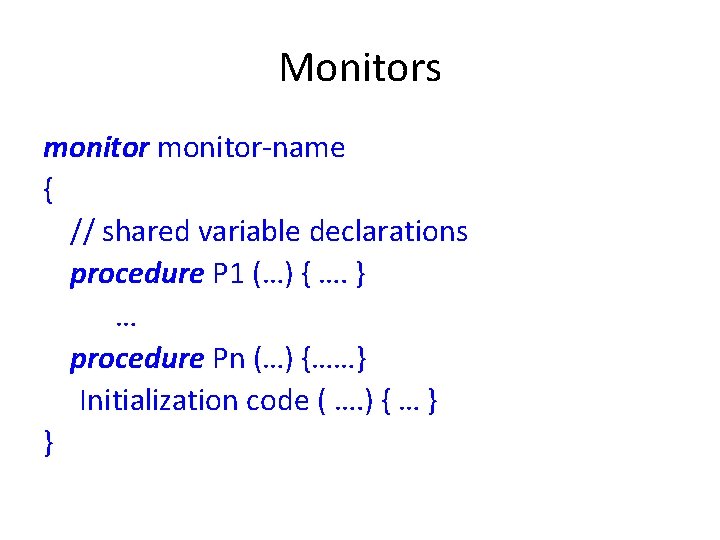
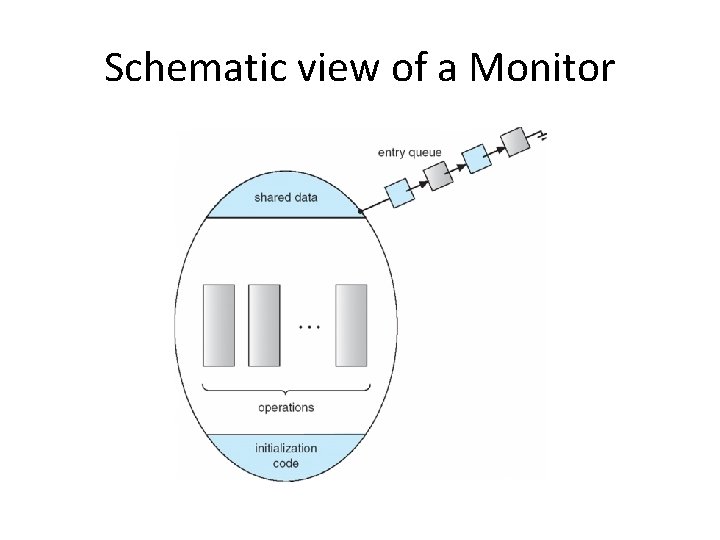
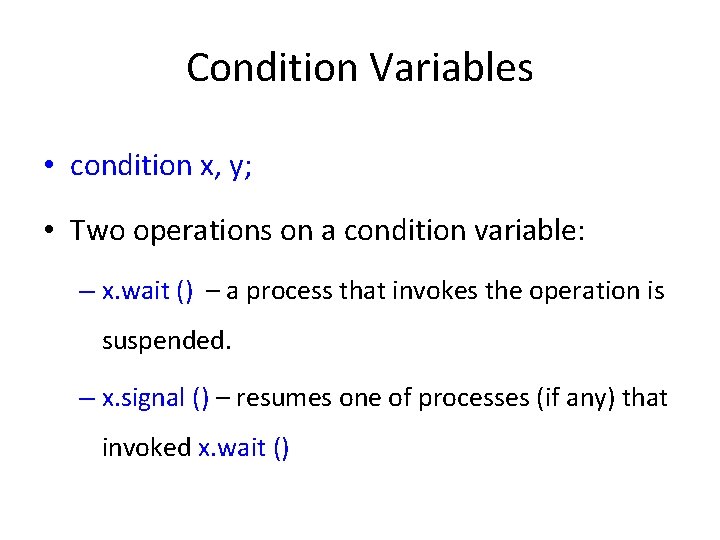
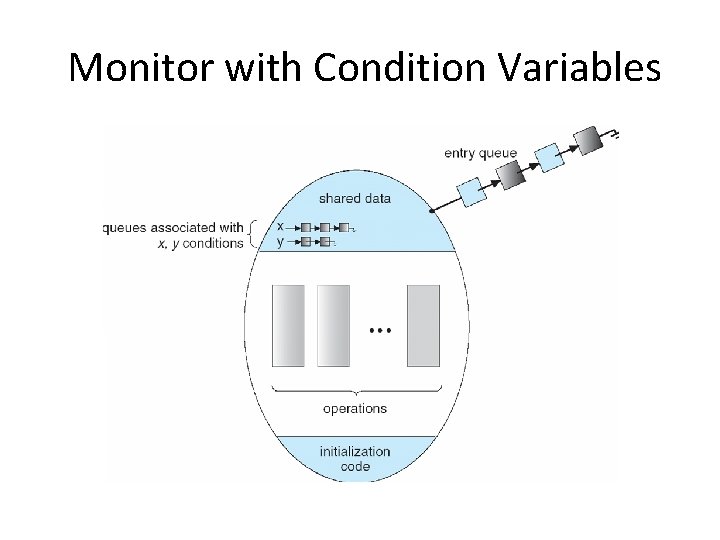
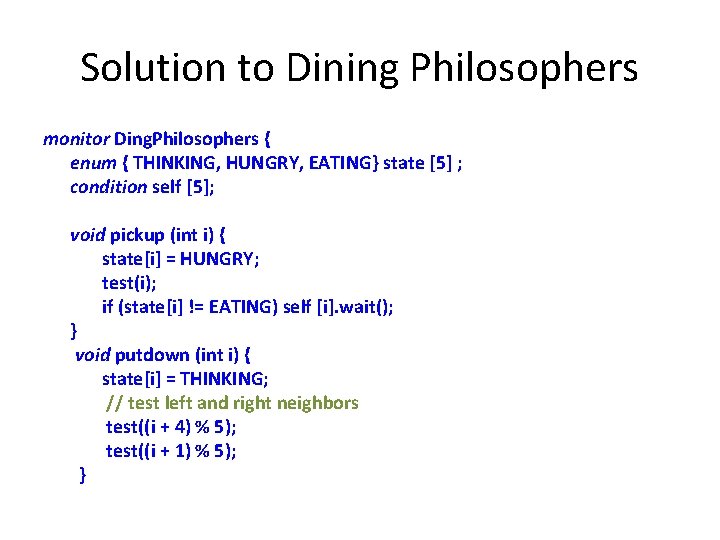
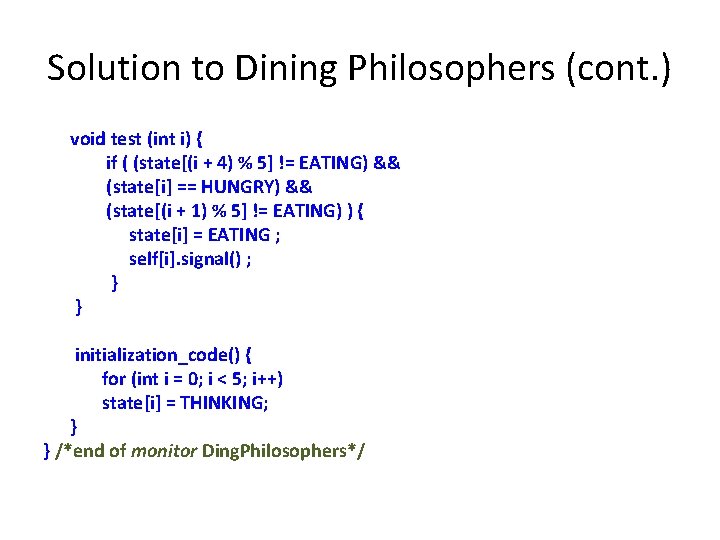
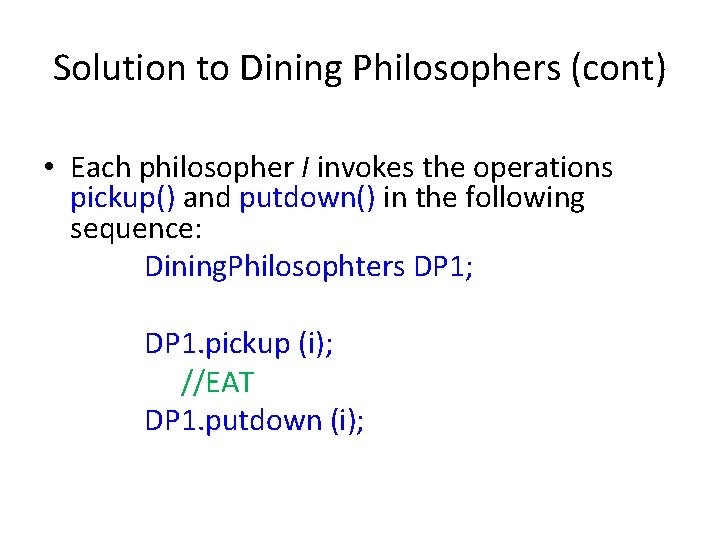
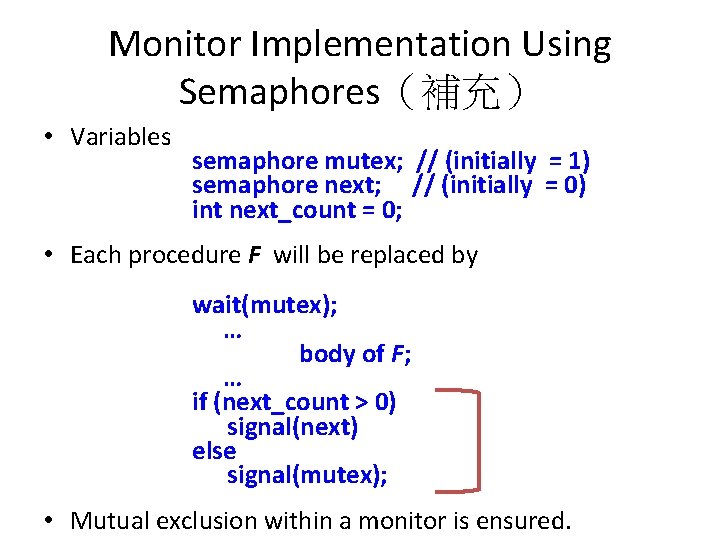
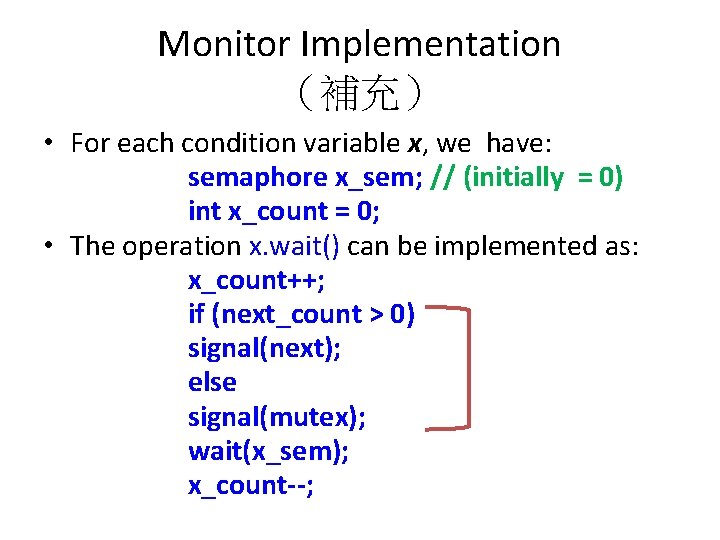
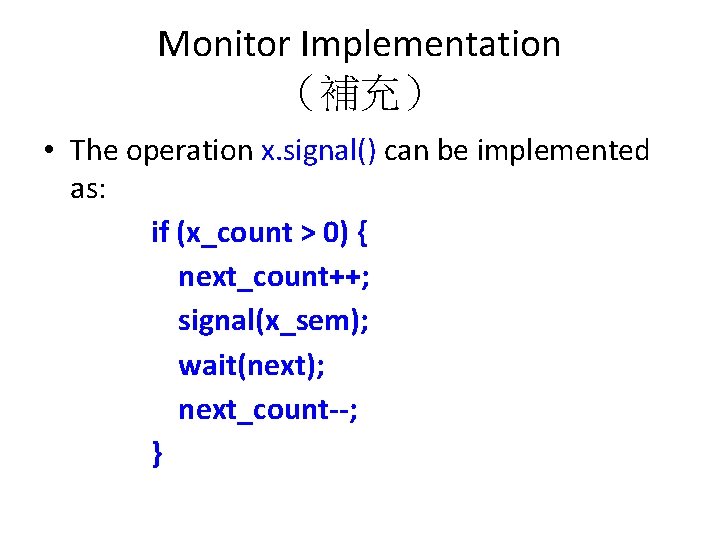
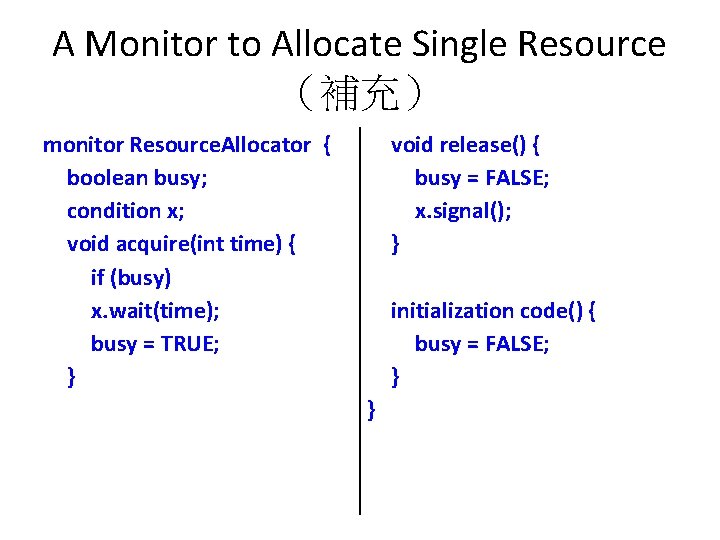
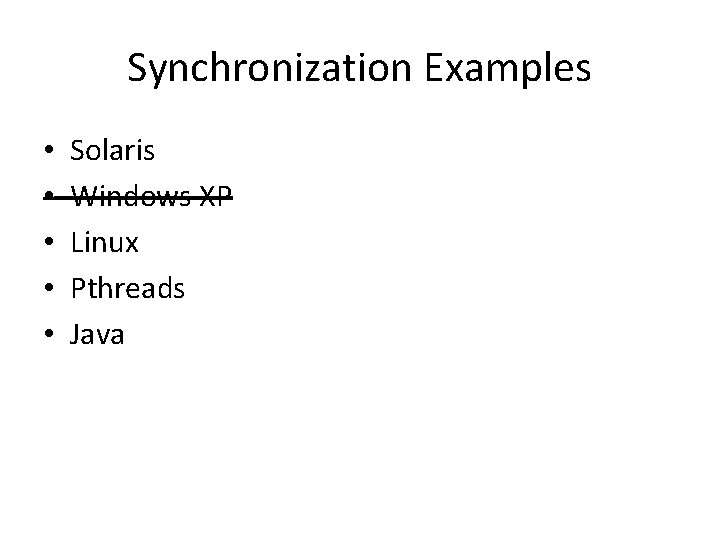
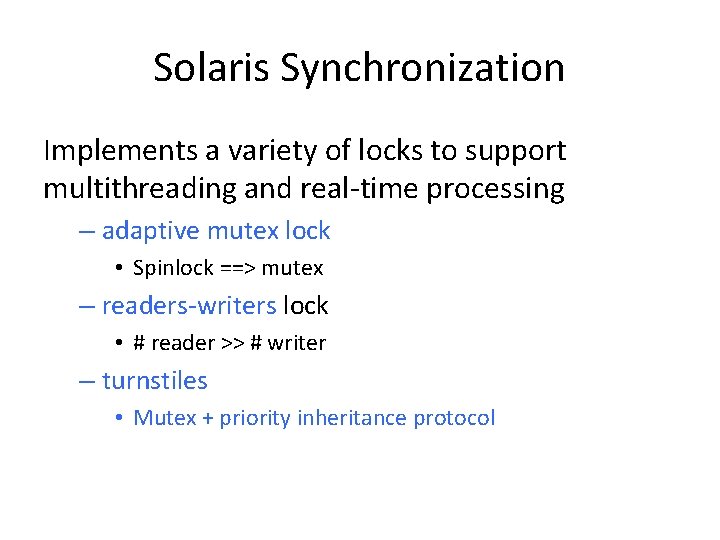
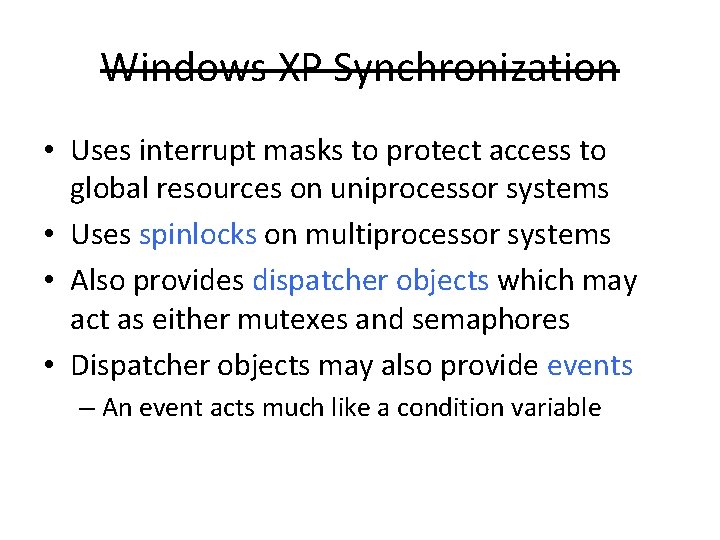
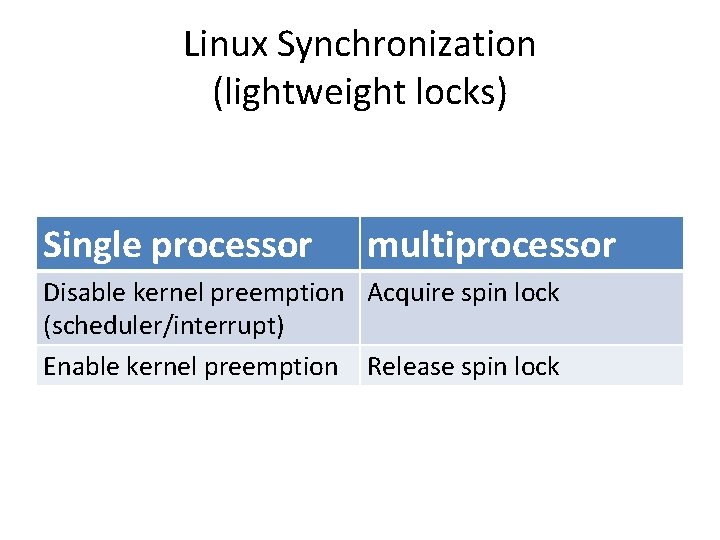
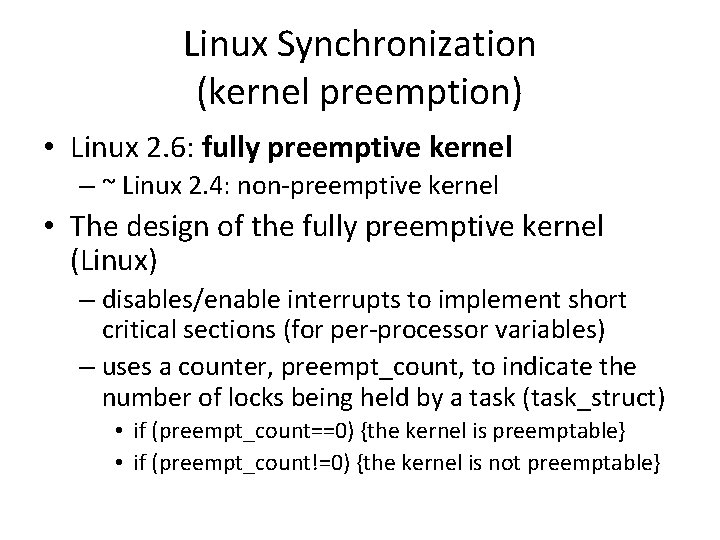
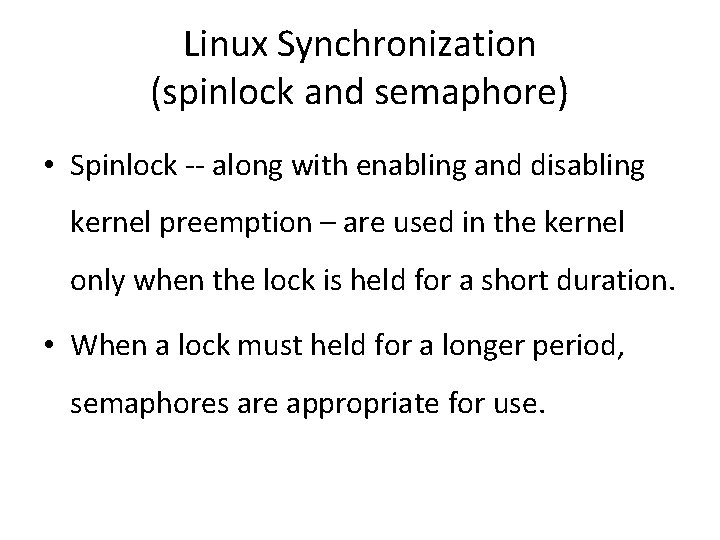
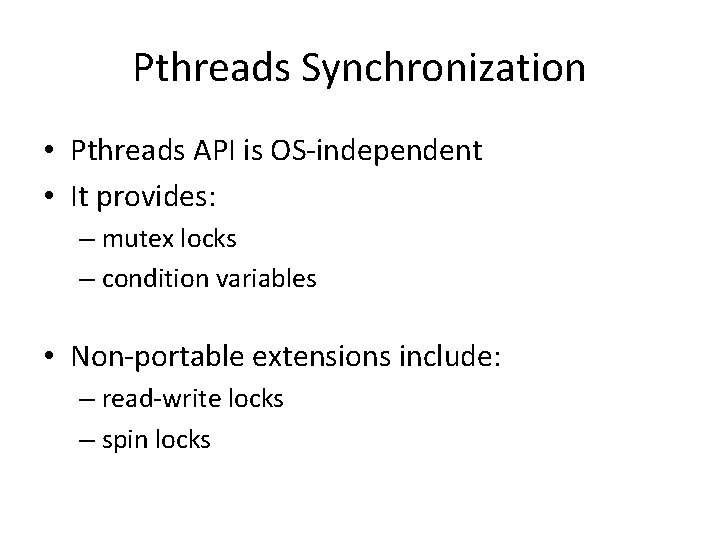
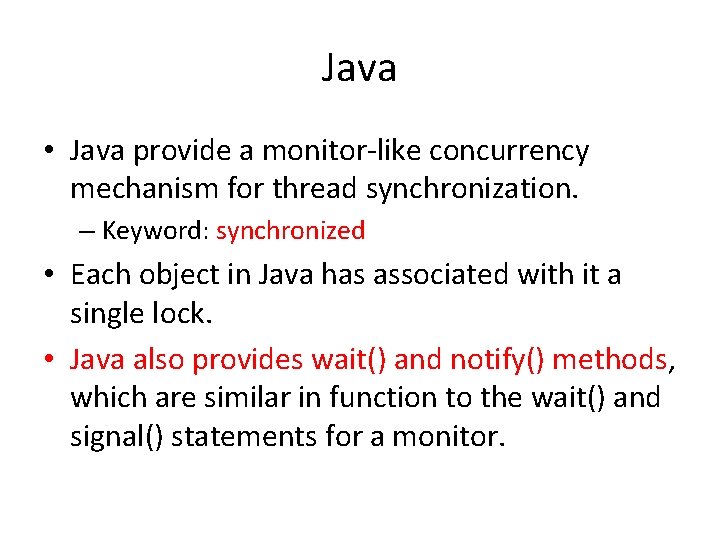
- Slides: 40
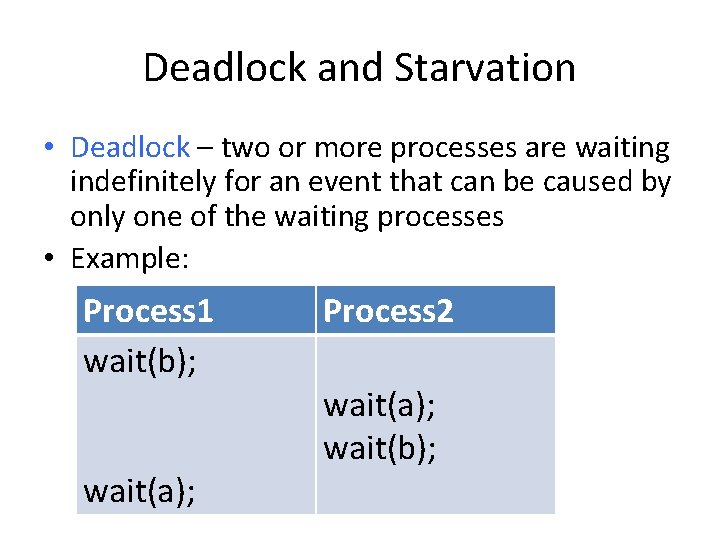
Deadlock and Starvation • Deadlock – two or more processes are waiting indefinitely for an event that can be caused by only one of the waiting processes • Example: Process 1 wait(b); wait(a); Process 2 wait(a); wait(b);
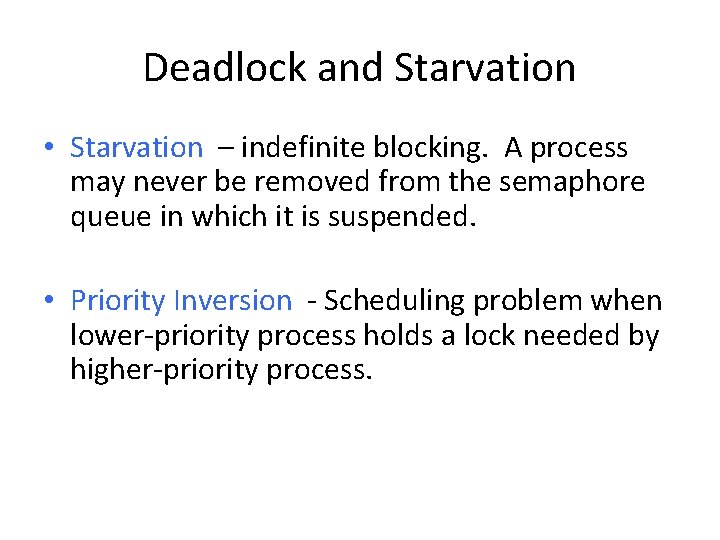
Deadlock and Starvation • Starvation – indefinite blocking. A process may never be removed from the semaphore queue in which it is suspended. • Priority Inversion - Scheduling problem when lower-priority process holds a lock needed by higher-priority process.
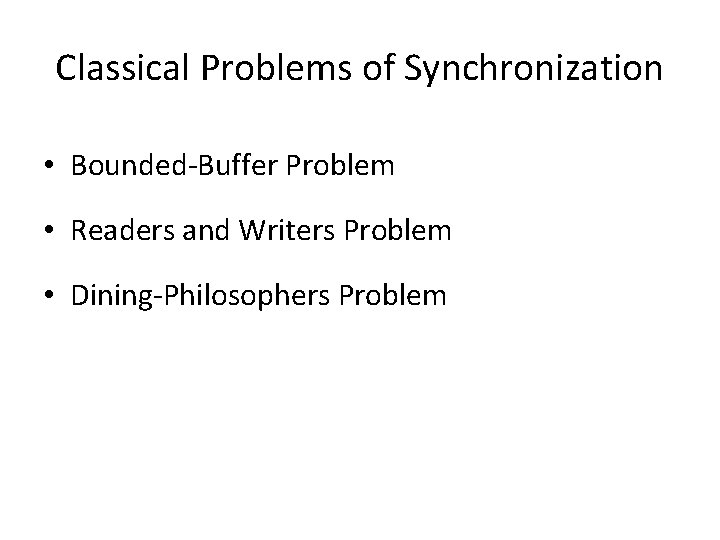
Classical Problems of Synchronization • Bounded-Buffer Problem • Readers and Writers Problem • Dining-Philosophers Problem
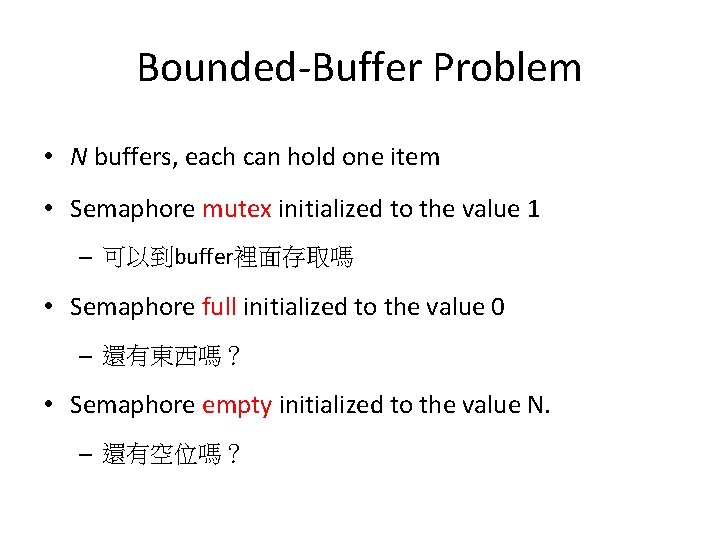
Bounded-Buffer Problem • N buffers, each can hold one item • Semaphore mutex initialized to the value 1 – 可以到buffer裡面存取嗎 • Semaphore full initialized to the value 0 – 還有東西嗎? • Semaphore empty initialized to the value N. – 還有空位嗎?
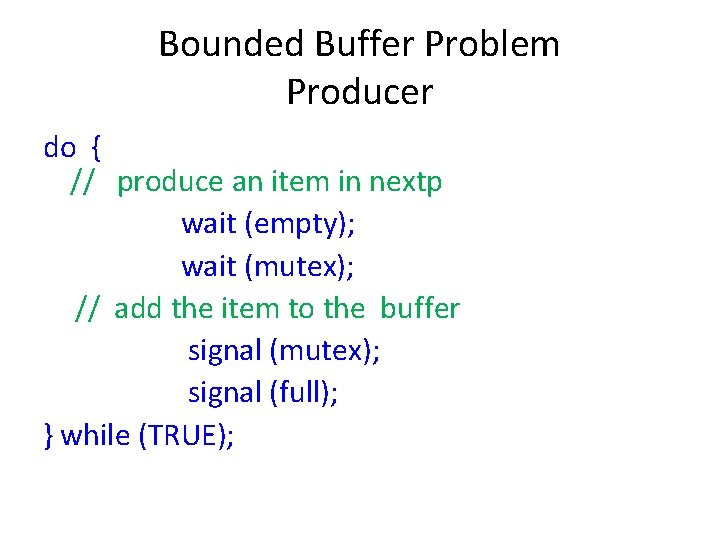
Bounded Buffer Problem Producer do { // produce an item in nextp wait (empty); wait (mutex); // add the item to the buffer signal (mutex); signal (full); } while (TRUE);
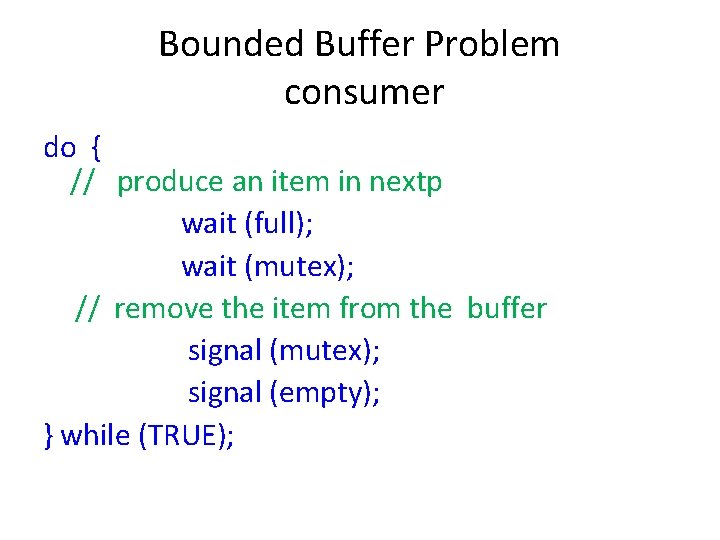
Bounded Buffer Problem consumer do { // produce an item in nextp wait (full); wait (mutex); // remove the item from the buffer signal (mutex); signal (empty); } while (TRUE);
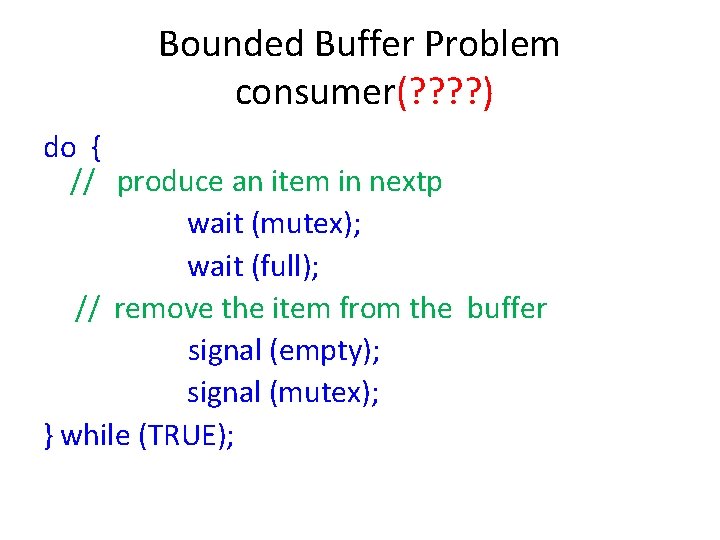
Bounded Buffer Problem consumer(? ? ) do { // produce an item in nextp wait (mutex); wait (full); // remove the item from the buffer signal (empty); signal (mutex); } while (TRUE);
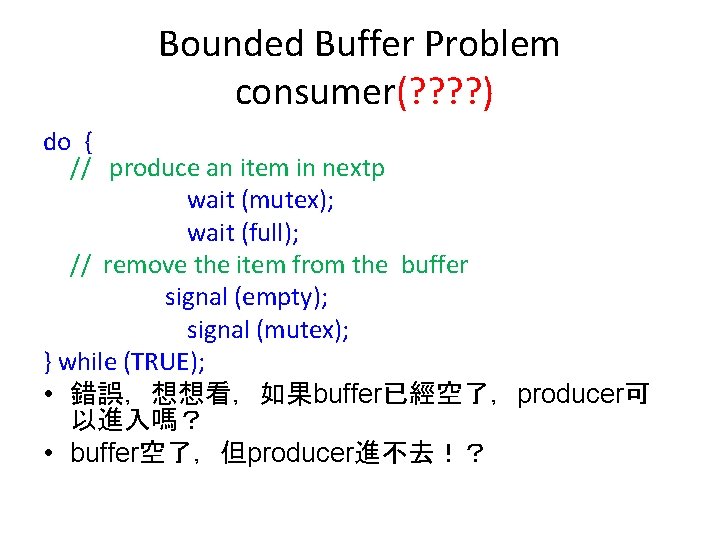
Bounded Buffer Problem consumer(? ? ) do { // produce an item in nextp wait (mutex); wait (full); // remove the item from the buffer signal (empty); signal (mutex); } while (TRUE); • 錯誤,想想看,如果buffer已經空了,producer可 以進入嗎? • buffer空了,但producer進不去!?
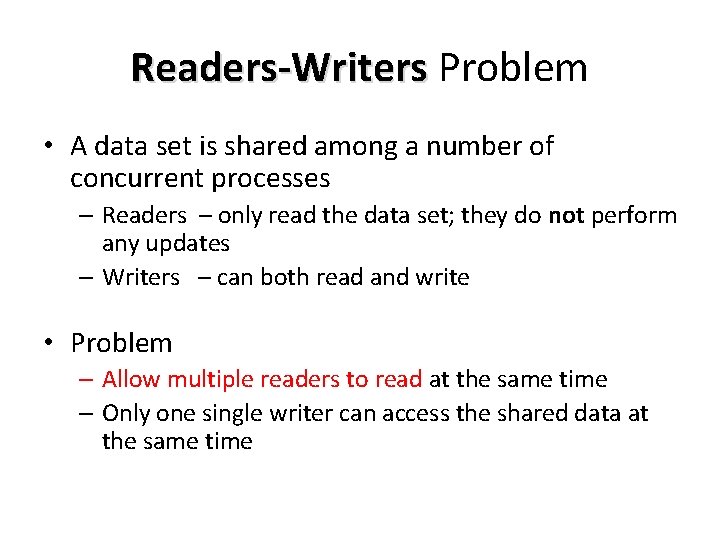
Readers-Writers Problem • A data set is shared among a number of concurrent processes – Readers – only read the data set; they do not perform any updates – Writers – can both read and write • Problem – Allow multiple readers to read at the same time – Only one single writer can access the shared data at the same time
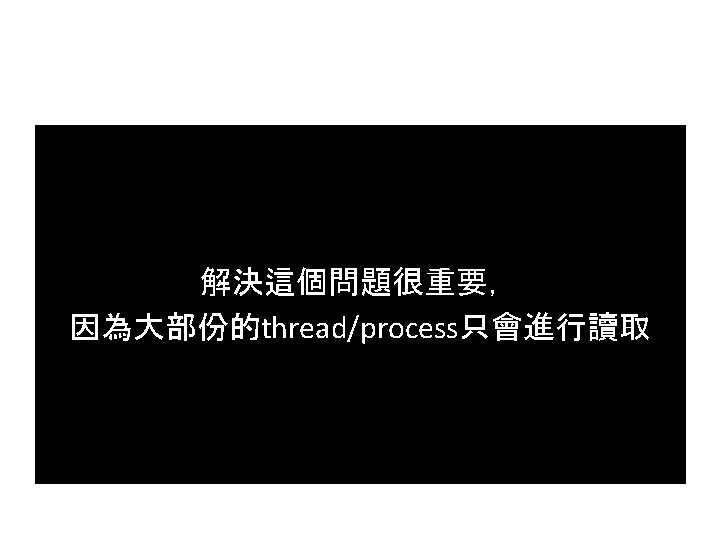
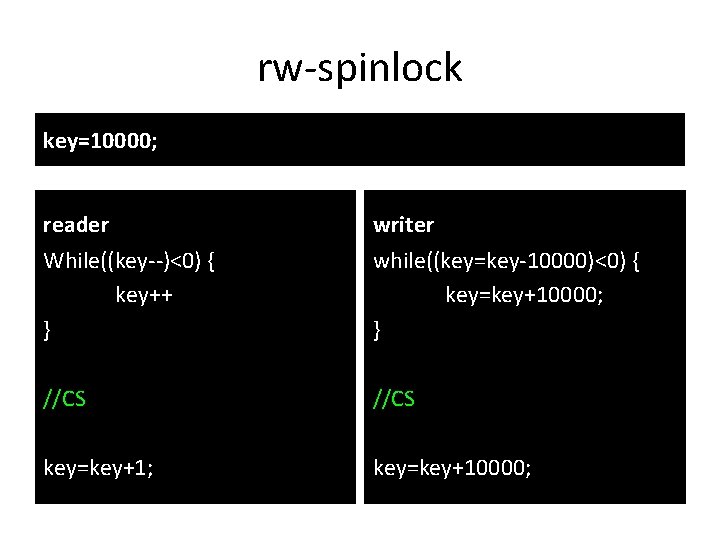
rw-spinlock key=10000; reader writer While((key--)<0) { key++ } while((key=key-10000)<0) { key=key+10000; } //CS key=key+1; key=key+10000;
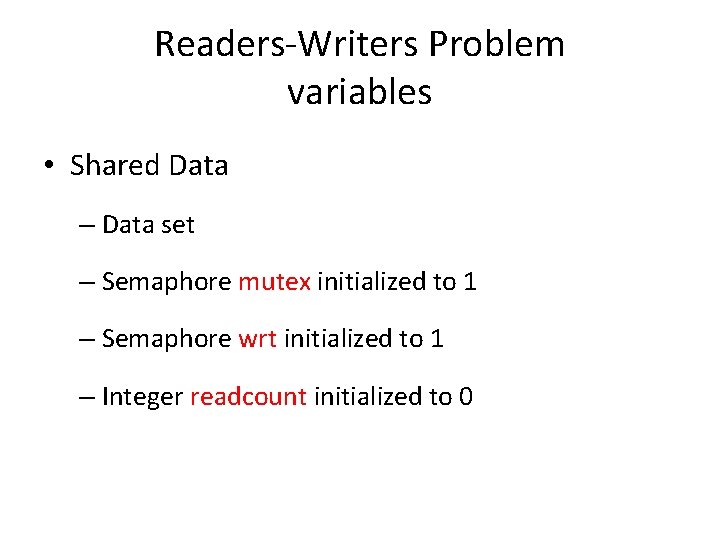
Readers-Writers Problem variables • Shared Data – Data set – Semaphore mutex initialized to 1 – Semaphore wrt initialized to 1 – Integer readcount initialized to 0
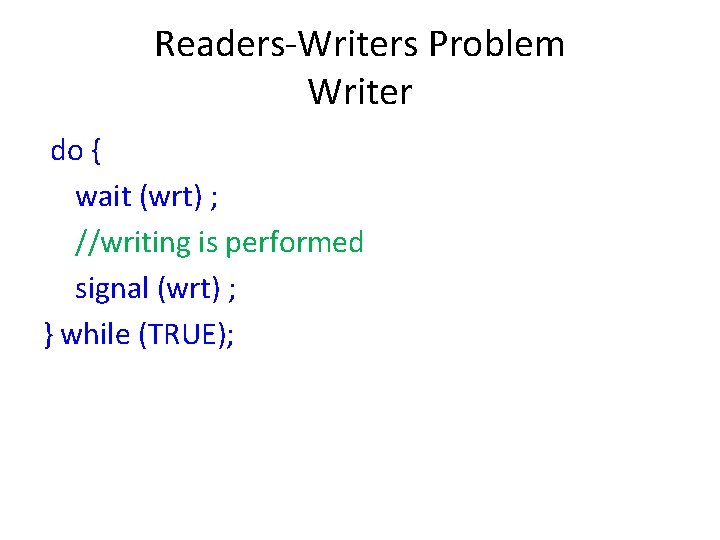
Readers-Writers Problem Writer do { wait (wrt) ; //writing is performed signal (wrt) ; } while (TRUE);
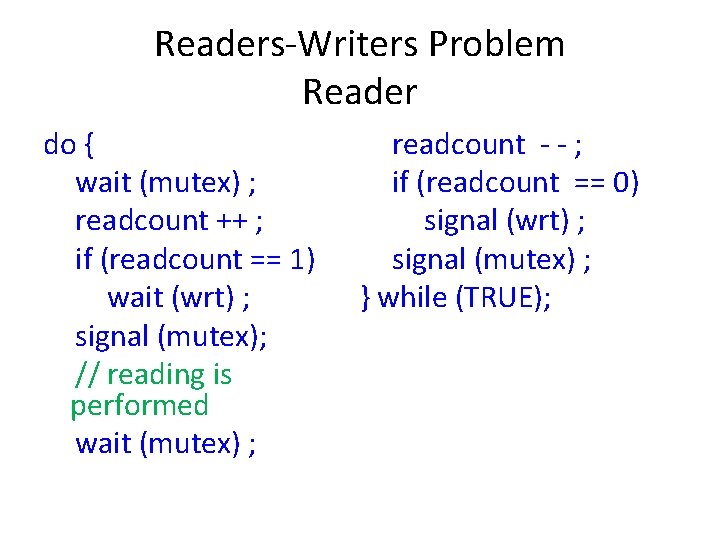
Readers-Writers Problem Reader do { wait (mutex) ; readcount ++ ; if (readcount == 1) wait (wrt) ; signal (mutex); // reading is performed wait (mutex) ; readcount - - ; if (readcount == 0) signal (wrt) ; signal (mutex) ; } while (TRUE);
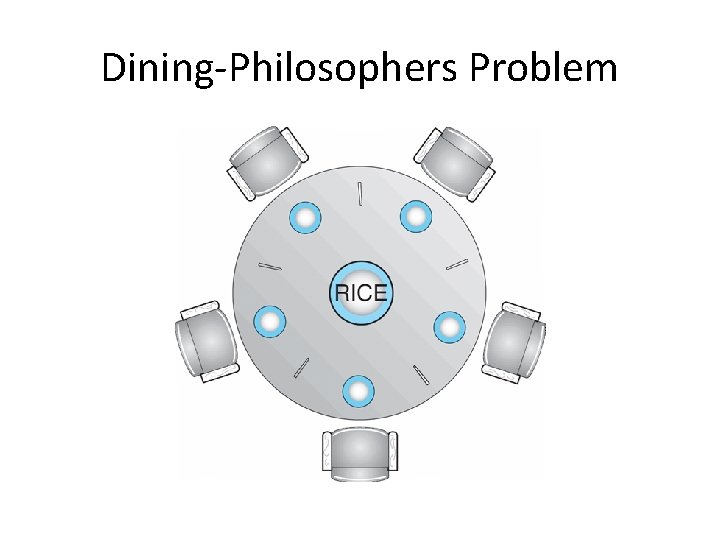
Dining-Philosophers Problem
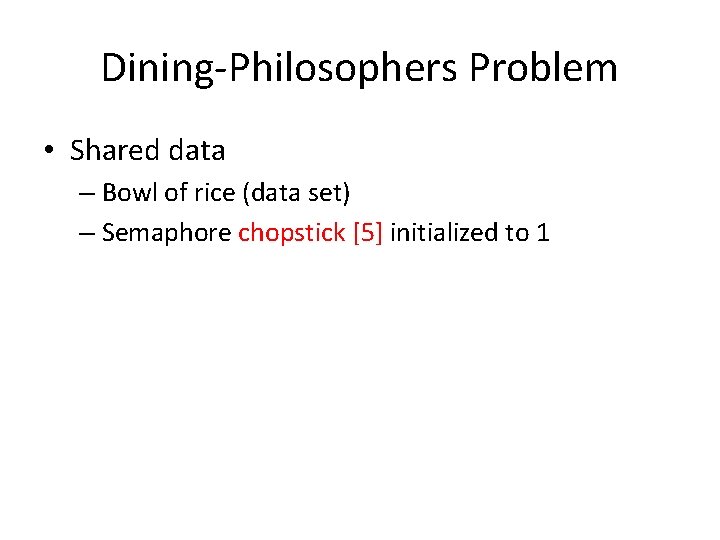
Dining-Philosophers Problem • Shared data – Bowl of rice (data set) – Semaphore chopstick [5] initialized to 1
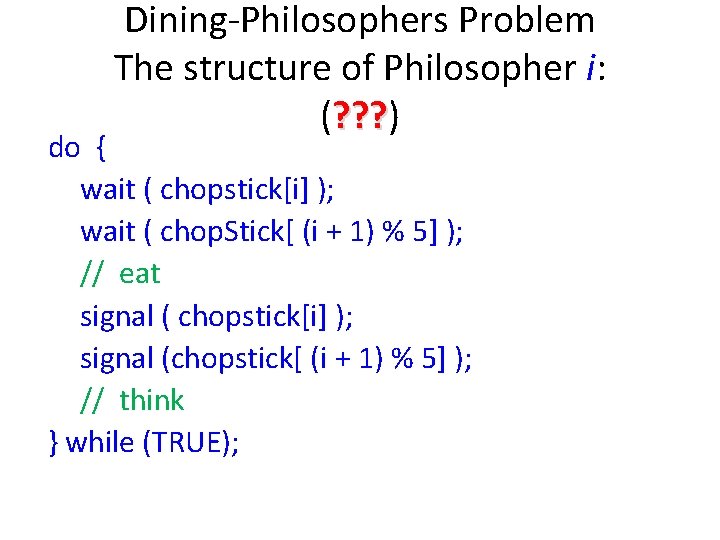
Dining-Philosophers Problem The structure of Philosopher i: (? ? ? ) ? ? ? do { wait ( chopstick[i] ); wait ( chop. Stick[ (i + 1) % 5] ); // eat signal ( chopstick[i] ); signal (chopstick[ (i + 1) % 5] ); // think } while (TRUE);
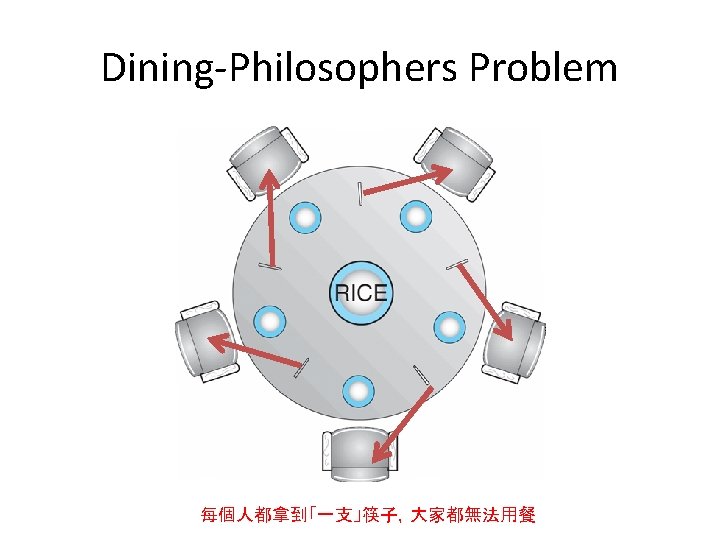
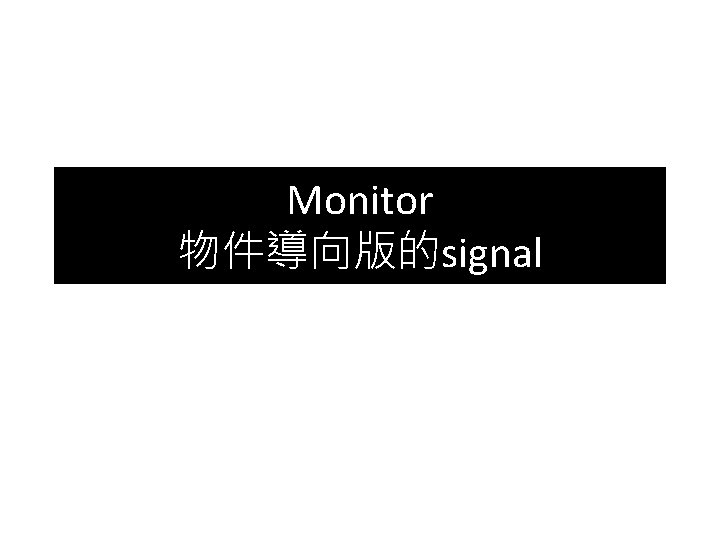
Monitor 物件導向版的signal
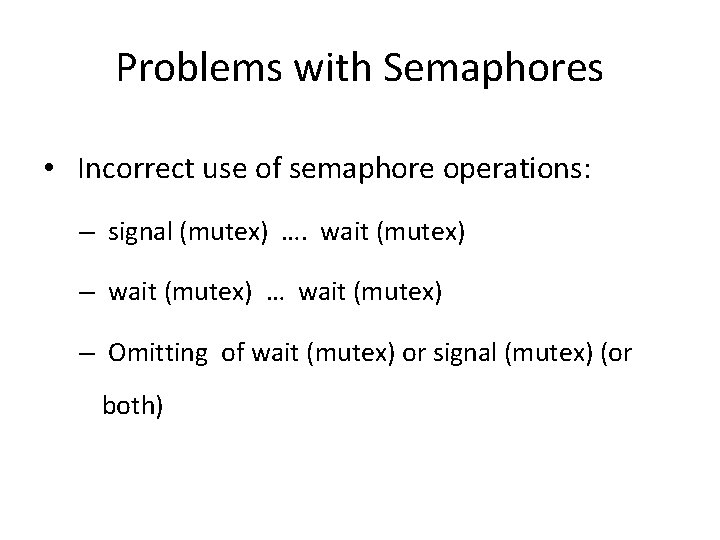
Problems with Semaphores • Incorrect use of semaphore operations: – signal (mutex) …. wait (mutex) – wait (mutex) … wait (mutex) – Omitting of wait (mutex) or signal (mutex) (or both)
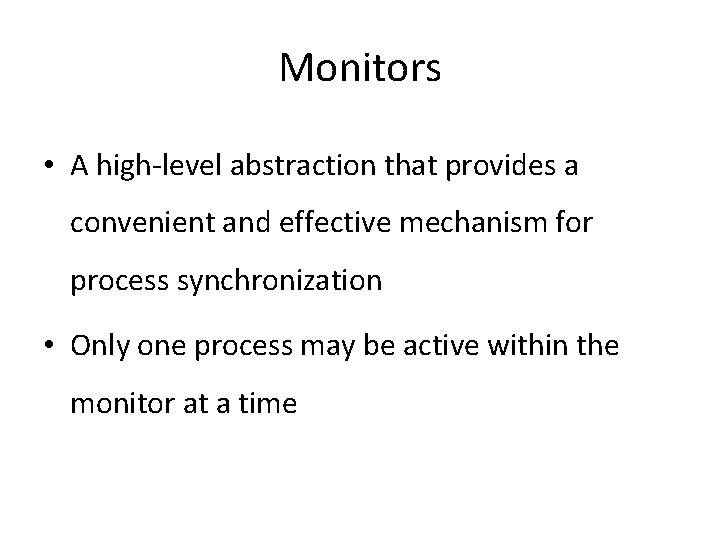
Monitors • A high-level abstraction that provides a convenient and effective mechanism for process synchronization • Only one process may be active within the monitor at a time
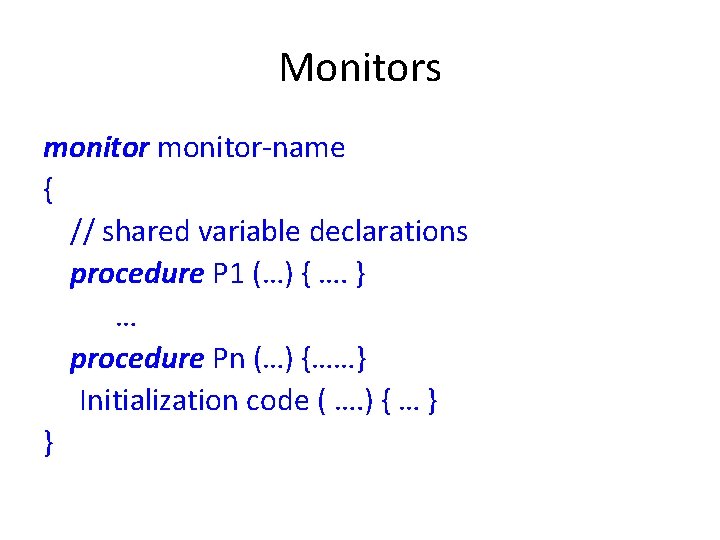
Monitors monitor-name { // shared variable declarations procedure P 1 (…) { …. } … procedure Pn (…) {……} Initialization code ( …. ) { … } }
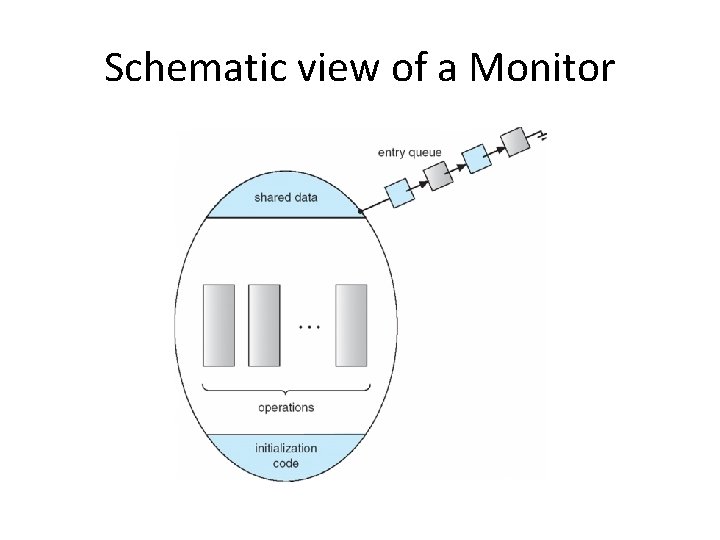
Schematic view of a Monitor
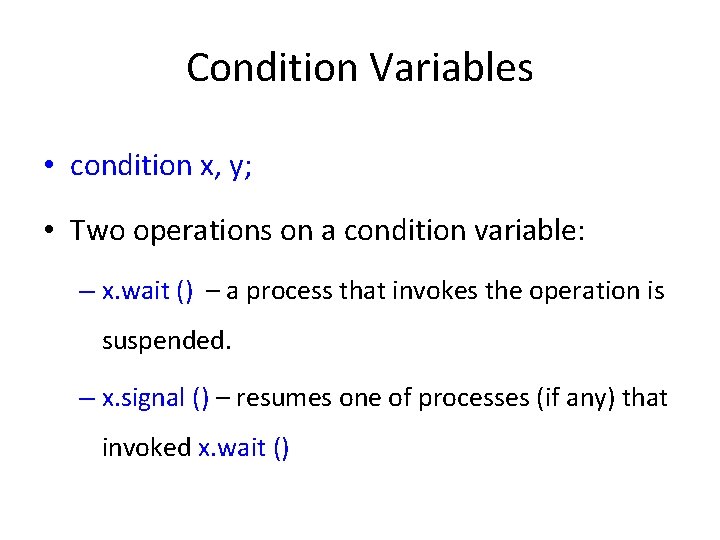
Condition Variables • condition x, y; • Two operations on a condition variable: – x. wait () – a process that invokes the operation is suspended. – x. signal () – resumes one of processes (if any) that invoked x. wait ()
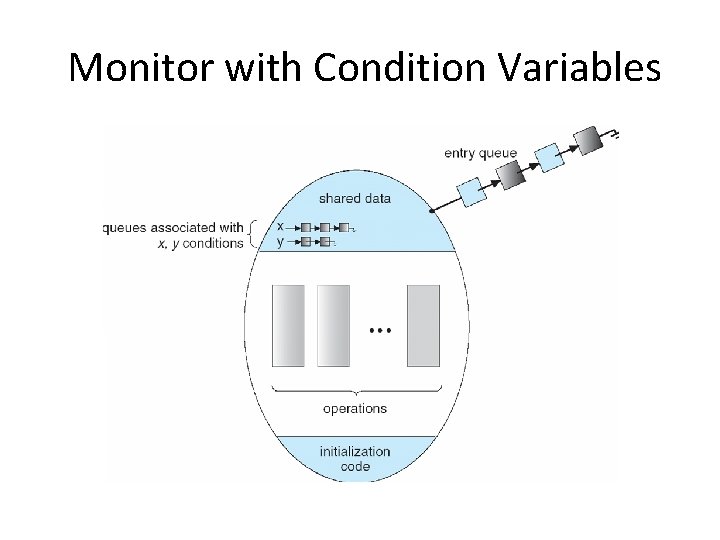
Monitor with Condition Variables
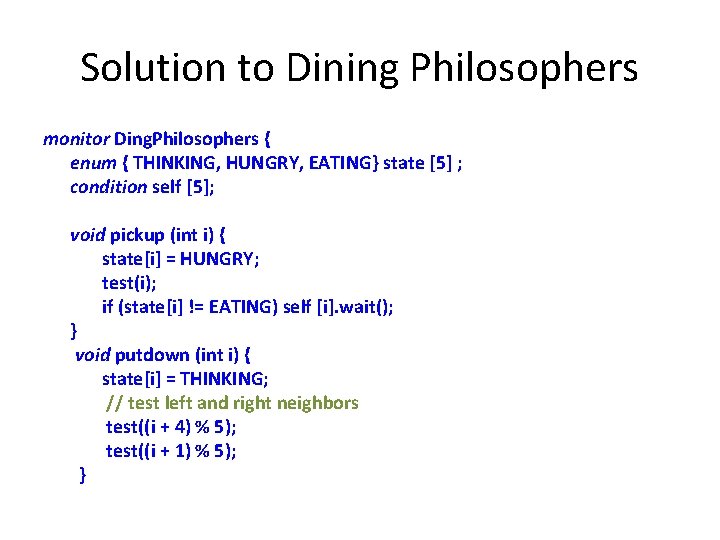
Solution to Dining Philosophers monitor Ding. Philosophers { enum { THINKING, HUNGRY, EATING} state [5] ; condition self [5]; void pickup (int i) { state[i] = HUNGRY; test(i); if (state[i] != EATING) self [i]. wait(); } void putdown (int i) { state[i] = THINKING; // test left and right neighbors test((i + 4) % 5); test((i + 1) % 5); }
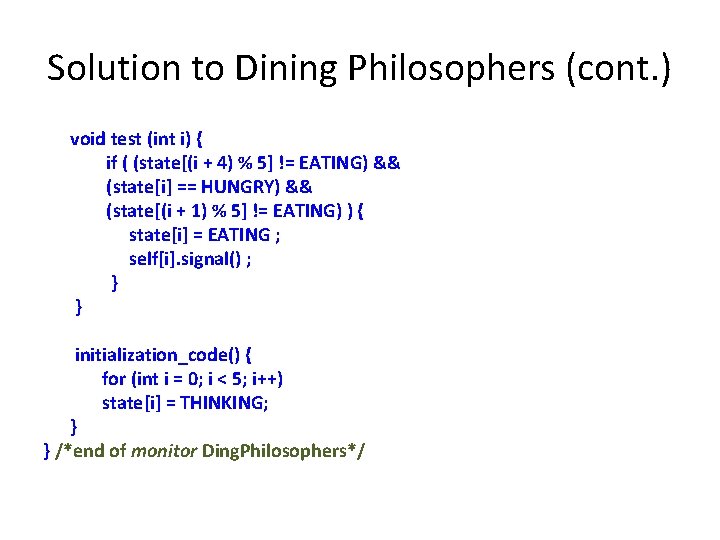
Solution to Dining Philosophers (cont. ) void test (int i) { if ( (state[(i + 4) % 5] != EATING) && (state[i] == HUNGRY) && (state[(i + 1) % 5] != EATING) ) { state[i] = EATING ; self[i]. signal() ; } } initialization_code() { for (int i = 0; i < 5; i++) state[i] = THINKING; } } /*end of monitor Ding. Philosophers*/
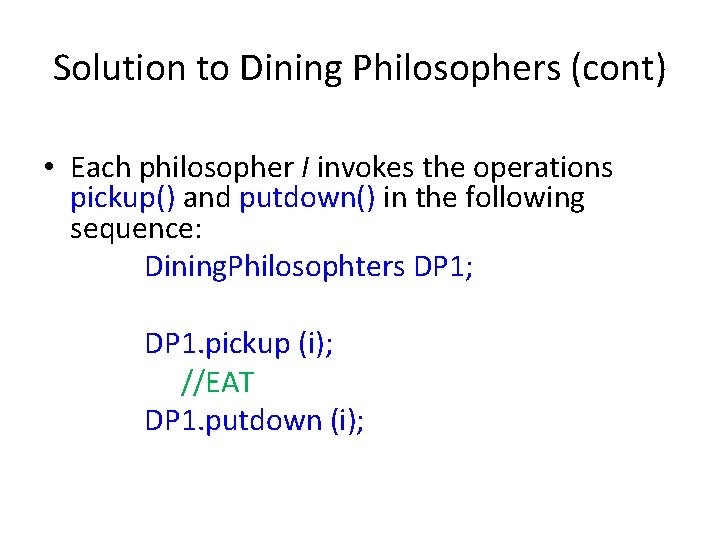
Solution to Dining Philosophers (cont) • Each philosopher I invokes the operations pickup() and putdown() in the following sequence: Dining. Philosophters DP 1; DP 1. pickup (i); //EAT DP 1. putdown (i);
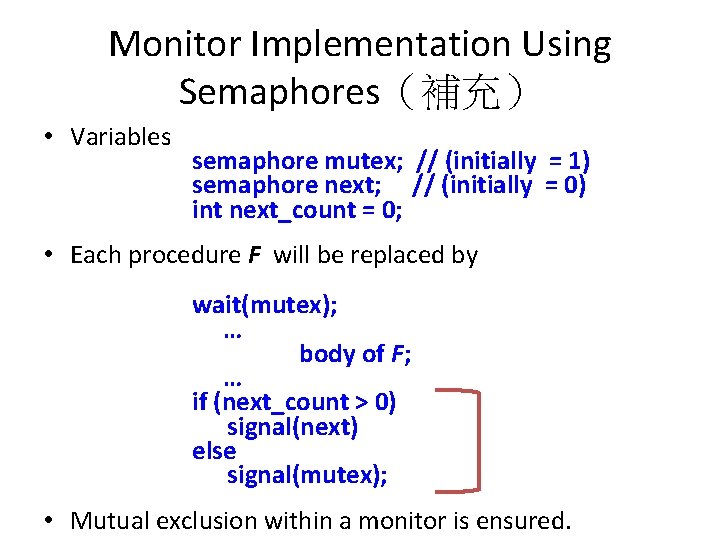
Monitor Implementation Using Semaphores(補充) • Variables semaphore mutex; // (initially = 1) semaphore next; // (initially = 0) int next_count = 0; • Each procedure F will be replaced by wait(mutex); … body of F; … if (next_count > 0) signal(next) else signal(mutex); • Mutual exclusion within a monitor is ensured.
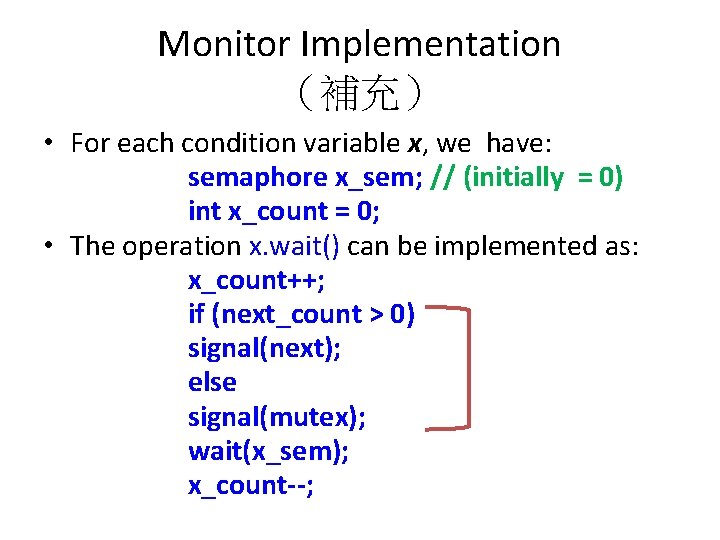
Monitor Implementation (補充) • For each condition variable x, we have: semaphore x_sem; // (initially = 0) int x_count = 0; • The operation x. wait() can be implemented as: x_count++; if (next_count > 0) signal(next); else signal(mutex); wait(x_sem); x_count--;
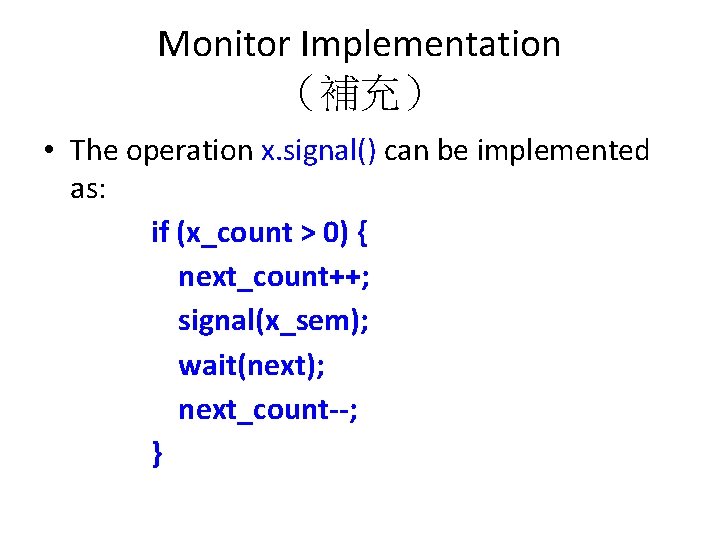
Monitor Implementation (補充) • The operation x. signal() can be implemented as: if (x_count > 0) { next_count++; signal(x_sem); wait(next); next_count--; }
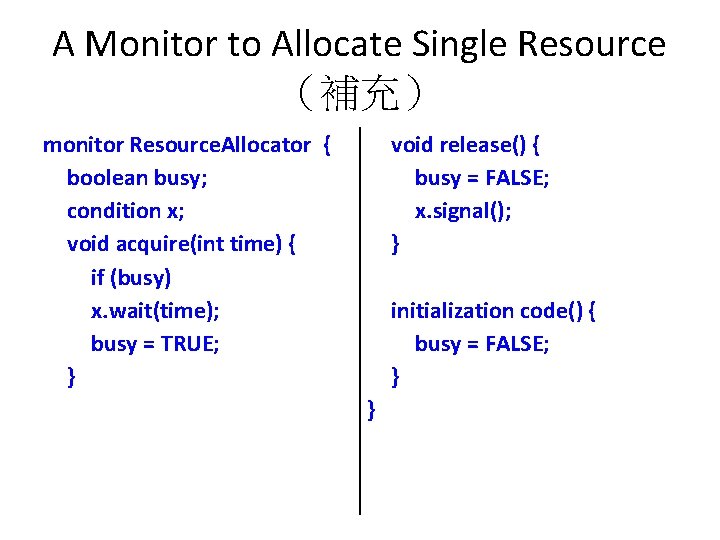
A Monitor to Allocate Single Resource (補充) monitor Resource. Allocator { boolean busy; condition x; void acquire(int time) { if (busy) x. wait(time); busy = TRUE; } void release() { busy = FALSE; x. signal(); } initialization code() { busy = FALSE; } }
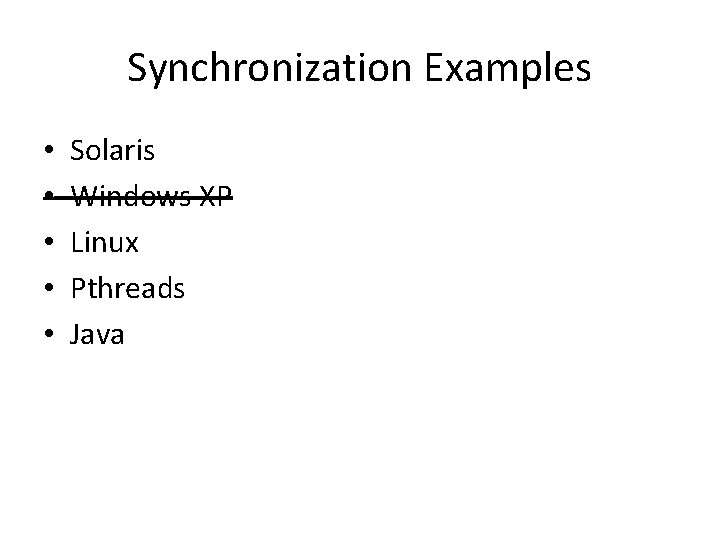
Synchronization Examples • • • Solaris Windows XP Linux Pthreads Java
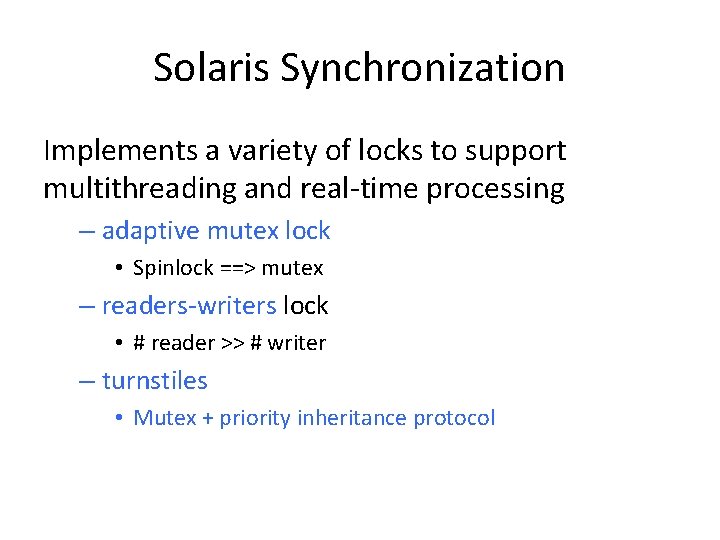
Solaris Synchronization Implements a variety of locks to support multithreading and real-time processing – adaptive mutex lock • Spinlock ==> mutex – readers-writers lock • # reader >> # writer – turnstiles • Mutex + priority inheritance protocol
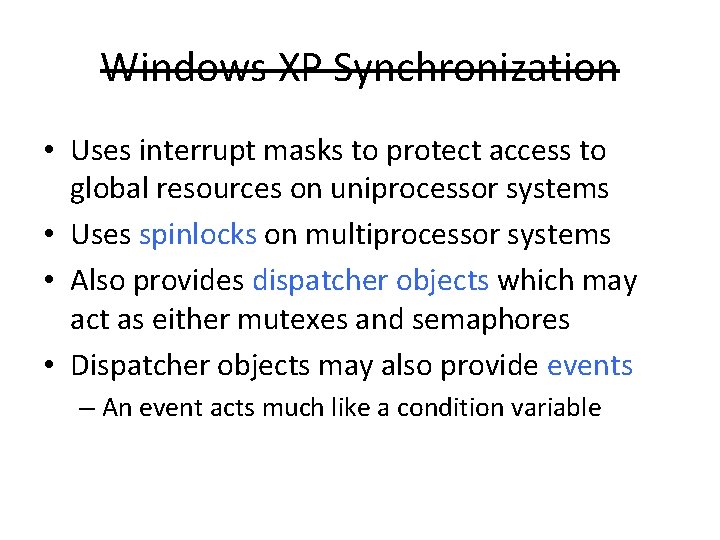
Windows XP Synchronization • Uses interrupt masks to protect access to global resources on uniprocessor systems • Uses spinlocks on multiprocessor systems • Also provides dispatcher objects which may act as either mutexes and semaphores • Dispatcher objects may also provide events – An event acts much like a condition variable
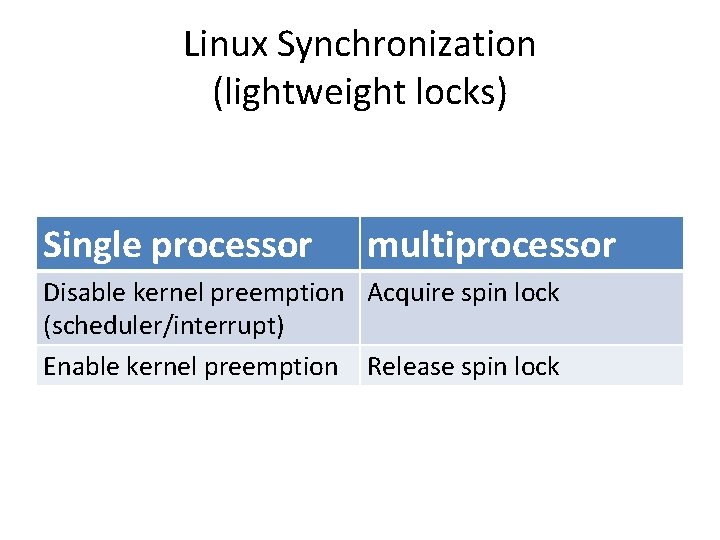
Linux Synchronization (lightweight locks) Single processor multiprocessor Disable kernel preemption Acquire spin lock (scheduler/interrupt) Enable kernel preemption Release spin lock
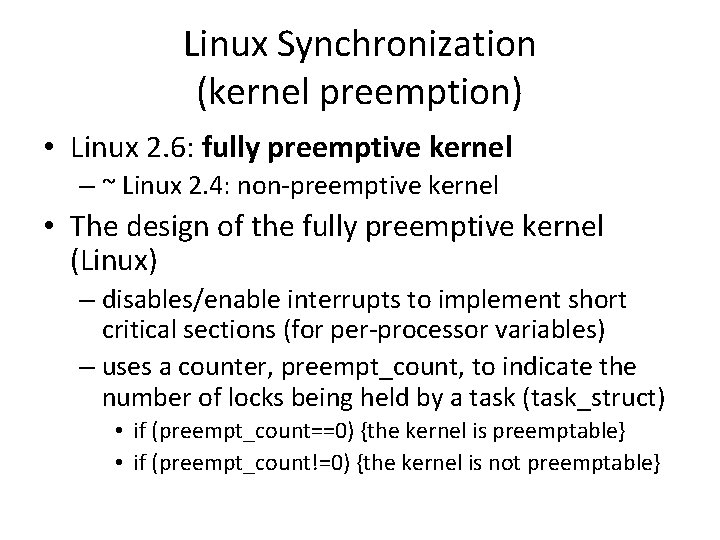
Linux Synchronization (kernel preemption) • Linux 2. 6: fully preemptive kernel – ~ Linux 2. 4: non-preemptive kernel • The design of the fully preemptive kernel (Linux) – disables/enable interrupts to implement short critical sections (for per-processor variables) – uses a counter, preempt_count, to indicate the number of locks being held by a task (task_struct) • if (preempt_count==0) {the kernel is preemptable} • if (preempt_count!=0) {the kernel is not preemptable}
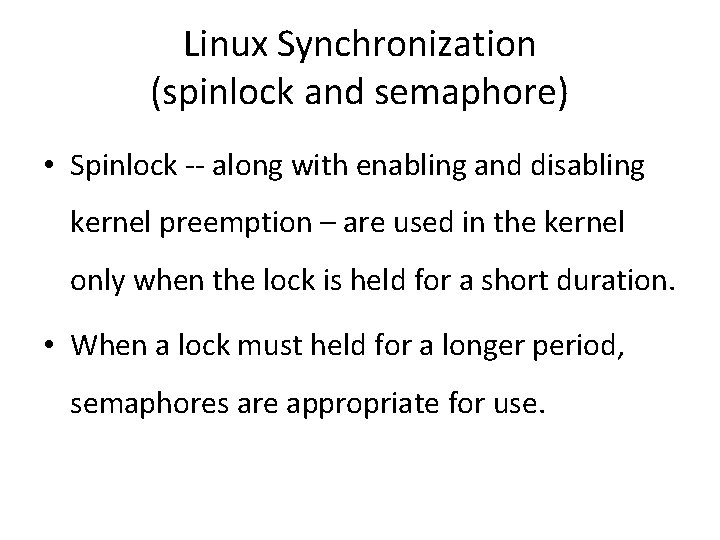
Linux Synchronization (spinlock and semaphore) • Spinlock -- along with enabling and disabling kernel preemption – are used in the kernel only when the lock is held for a short duration. • When a lock must held for a longer period, semaphores are appropriate for use.
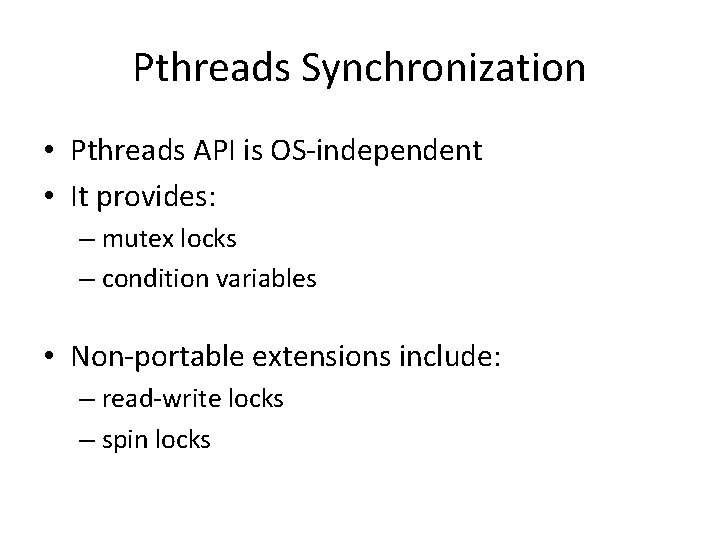
Pthreads Synchronization • Pthreads API is OS-independent • It provides: – mutex locks – condition variables • Non-portable extensions include: – read-write locks – spin locks
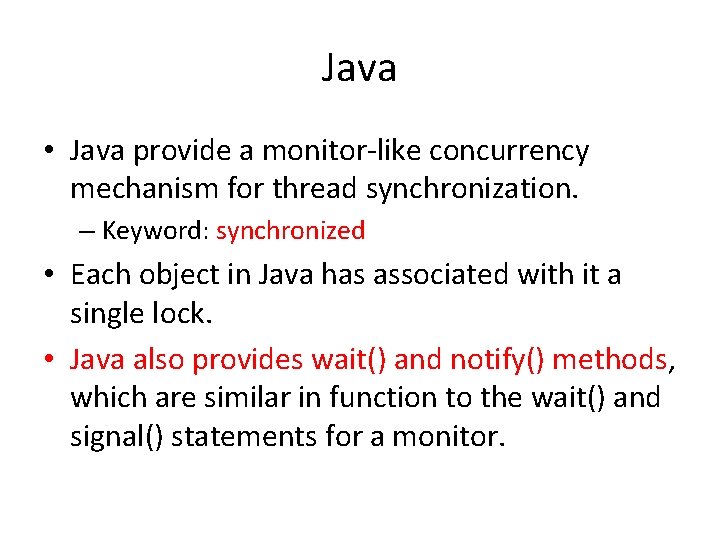
Java • Java provide a monitor-like concurrency mechanism for thread synchronization. – Keyword: synchronized • Each object in Java has associated with it a single lock. • Java also provides wait() and notify() methods, which are similar in function to the wait() and signal() statements for a monitor.
Lirik lagu more more more we praise you
More more more i want more more more more we praise you
Deadlock and starvation
Deadlock and starvation
Deadlock vs starvation
Starvation vs deadlock
Deadlock prevention and avoidance
Deadlock vs livelock
Starvation deadlock
Concurrent in os
Circular wait in operating system
What is starvation in fire fighting
Ventilator waveform analysis
Chapter 5 nutrition guidelines tools for healthful eating
Beyonce bmi
Starvation diabetes mellitus
Starvation binging analogy
Human history becomes more and more a race
Two opposing processes occur at equal rates
Ipc in os
First law bar chart
The more you take the more you leave behind
The more you study the more you learn
Aspire not to
Newtons first law example
Knowing more remembering more
More love to thee o lord
More choices more chances
Ability of two or more waves to combine and form a new wave
Deadlock detection and recovery
Deadlock prevention or avoidance
Safe and unsafe state in deadlock
Deadlock solution in os
What is banner in newspaper
7 elements of music
Specialized cloud mechanisms
I think you should be more specific here in step two
Ladybug food web
Crc binary division
Who says let two more summers wither in their pride
Comparative and superlative of l