CMSC 341 Making Java GUIs Functional More on
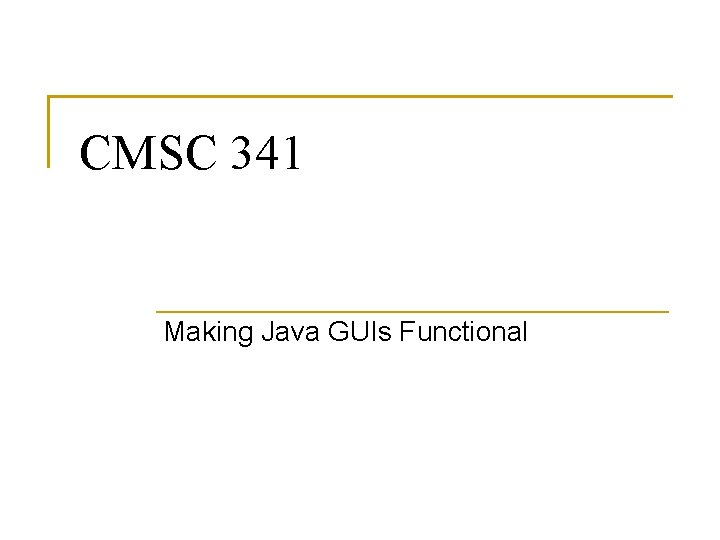
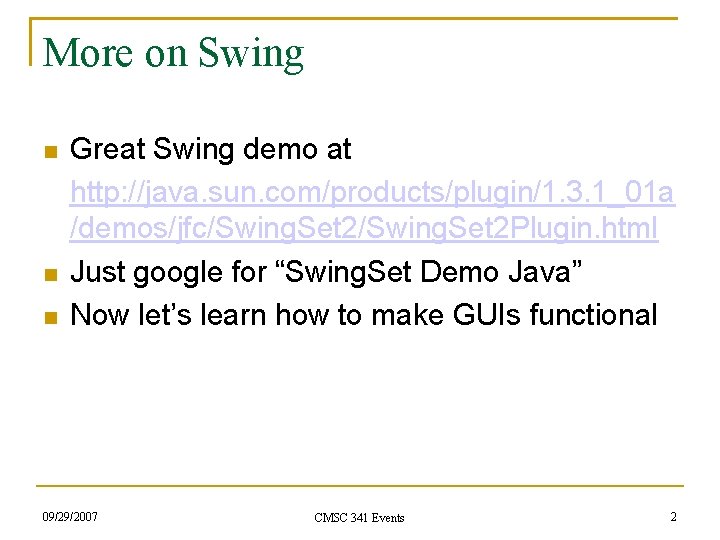
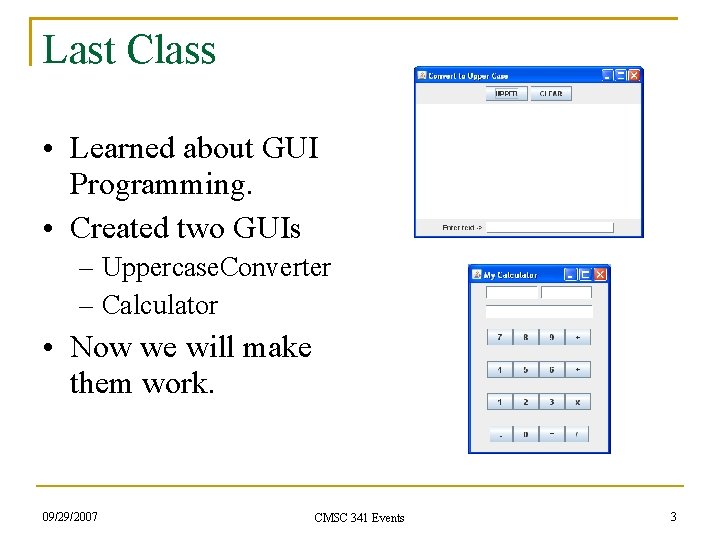
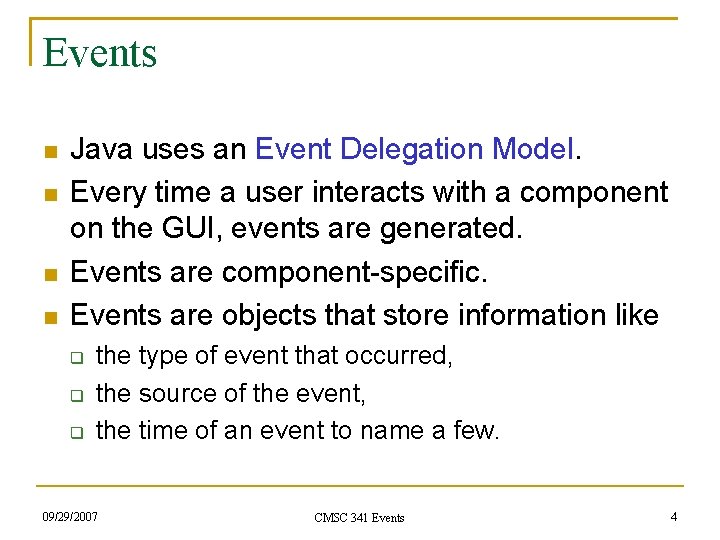
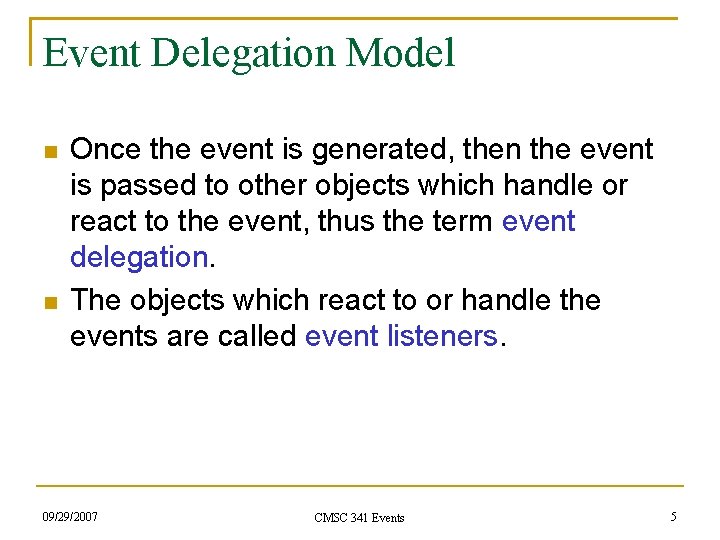
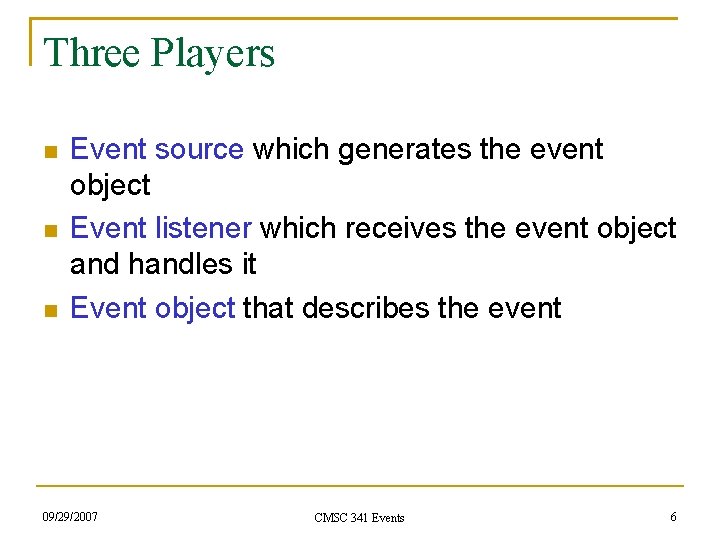
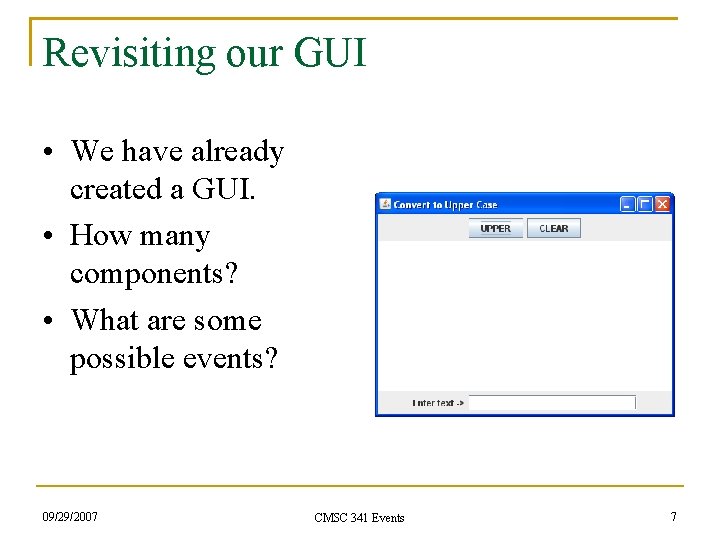
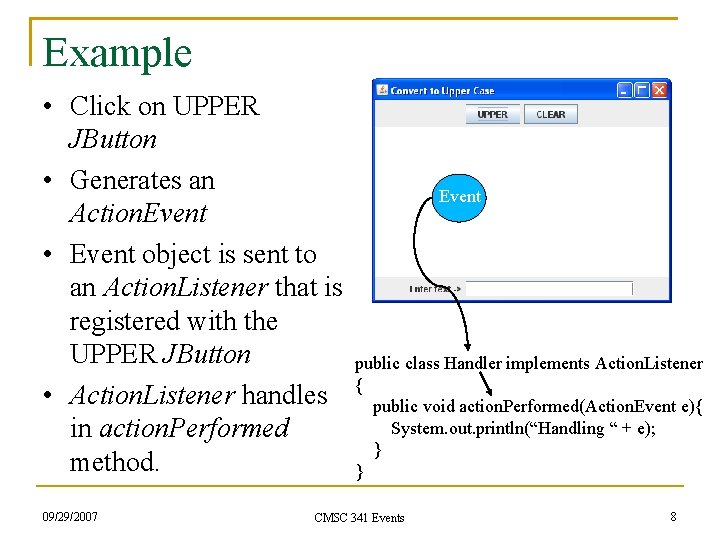
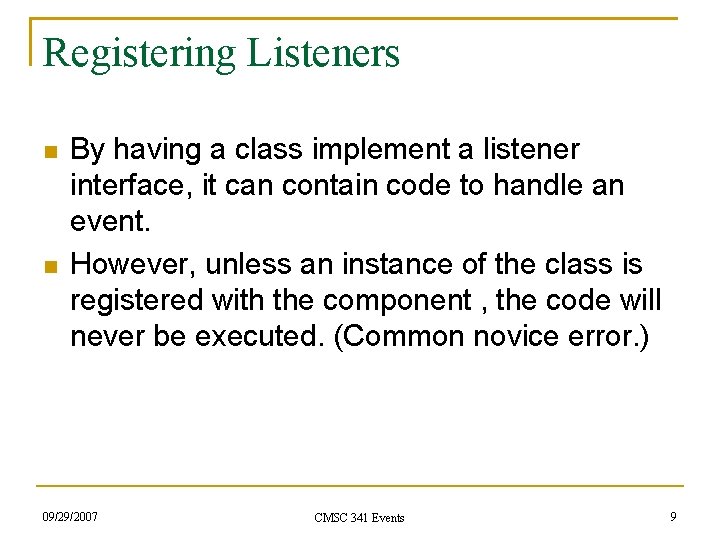
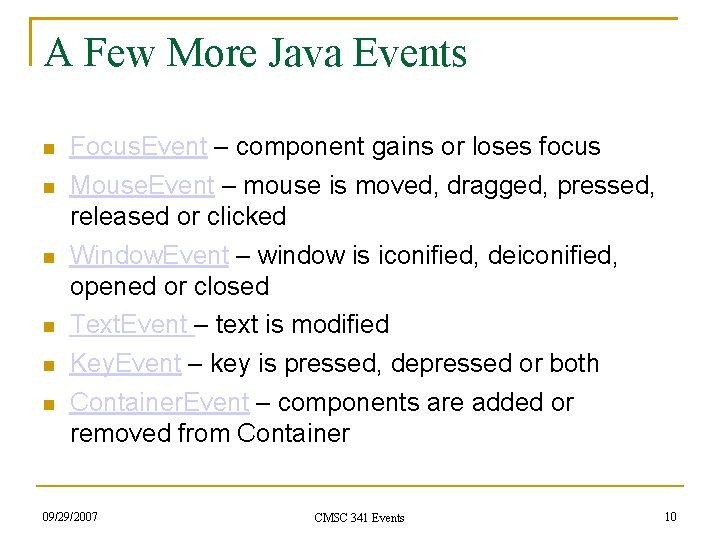
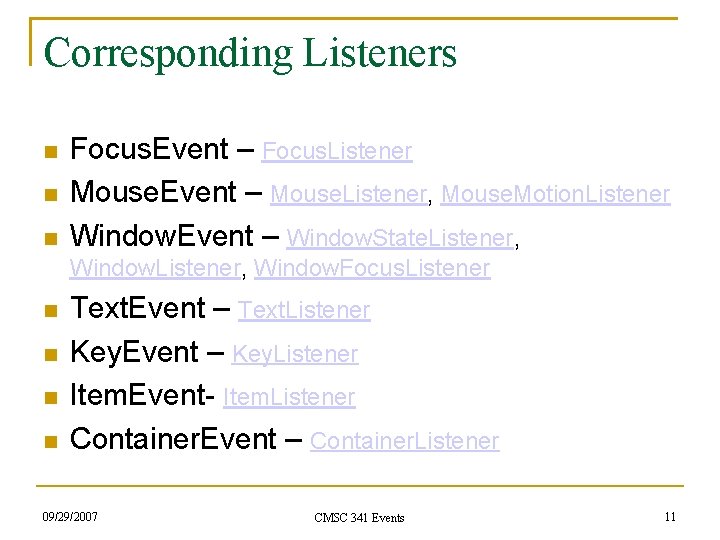
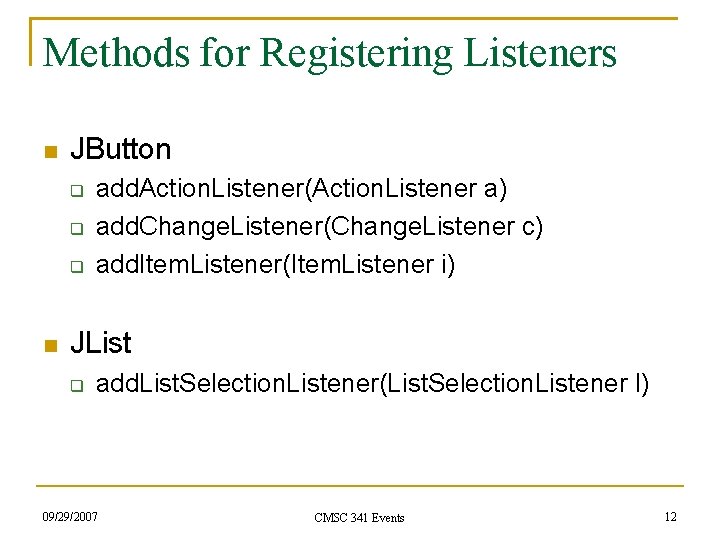
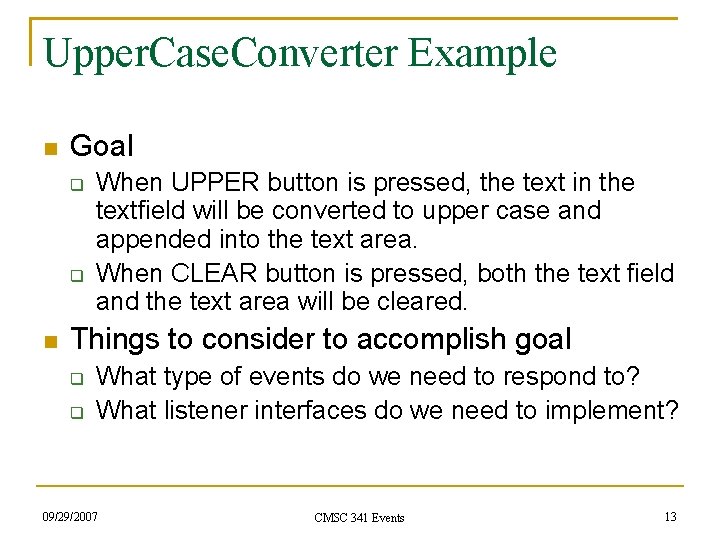
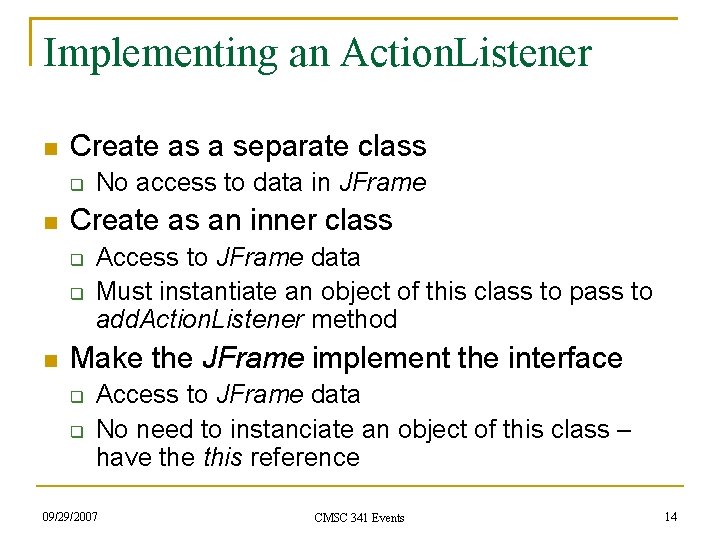
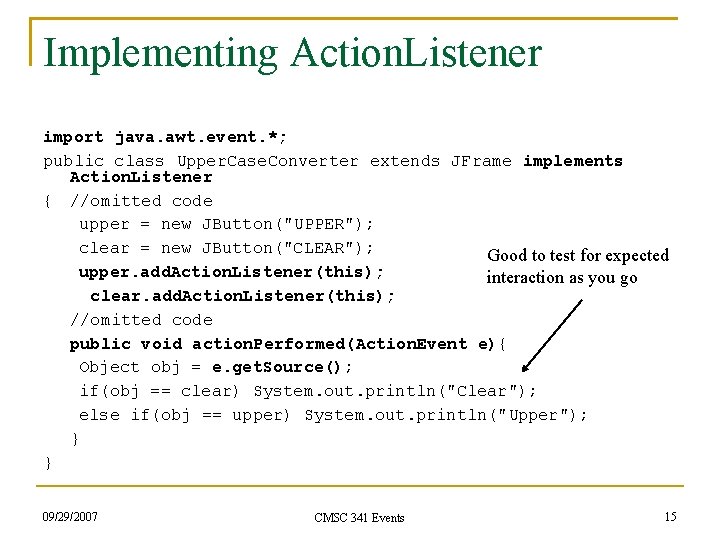
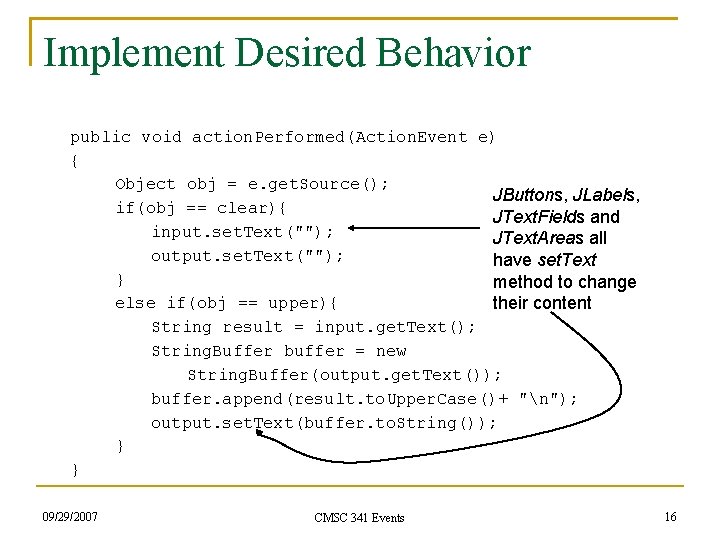
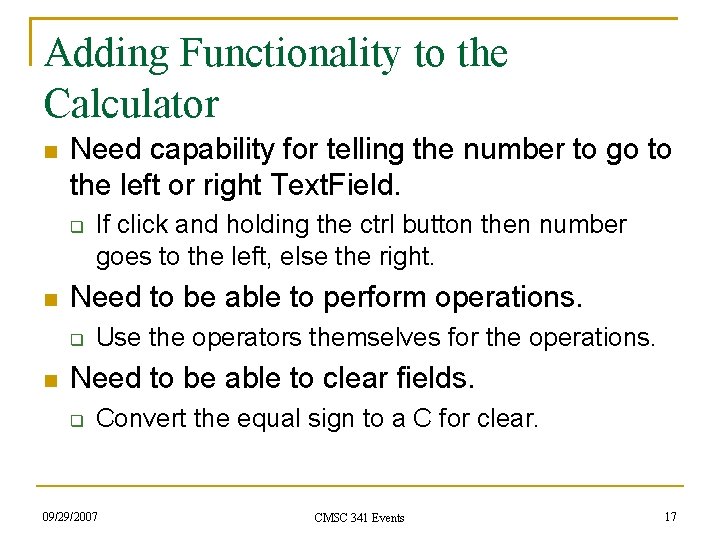
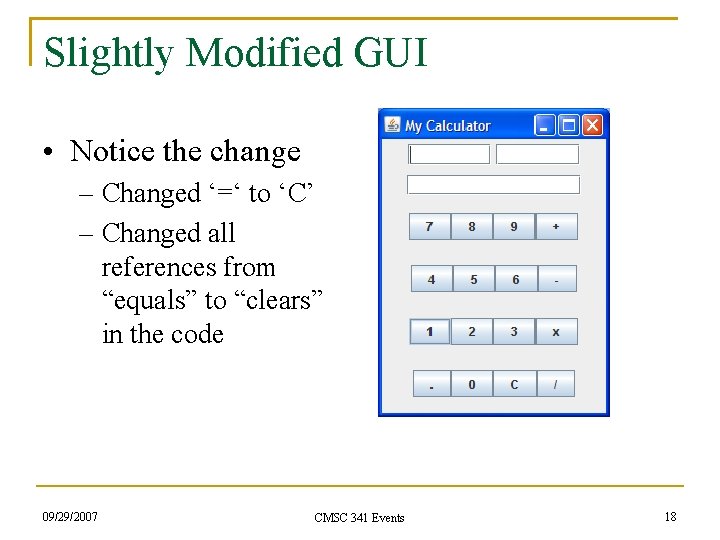
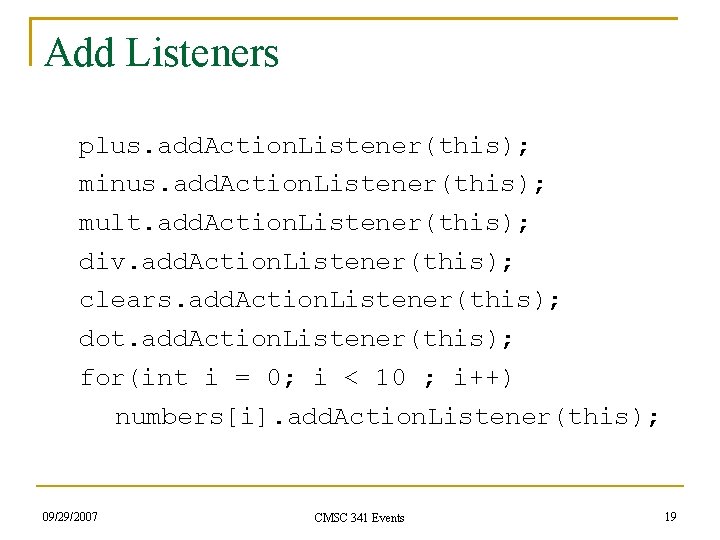
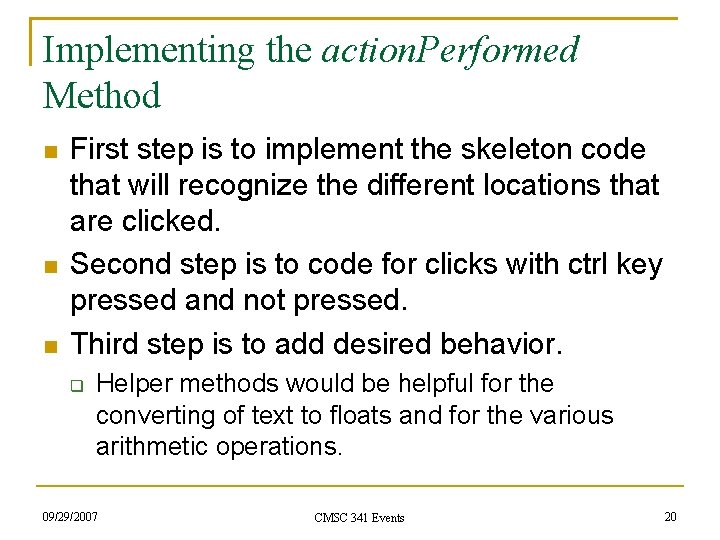
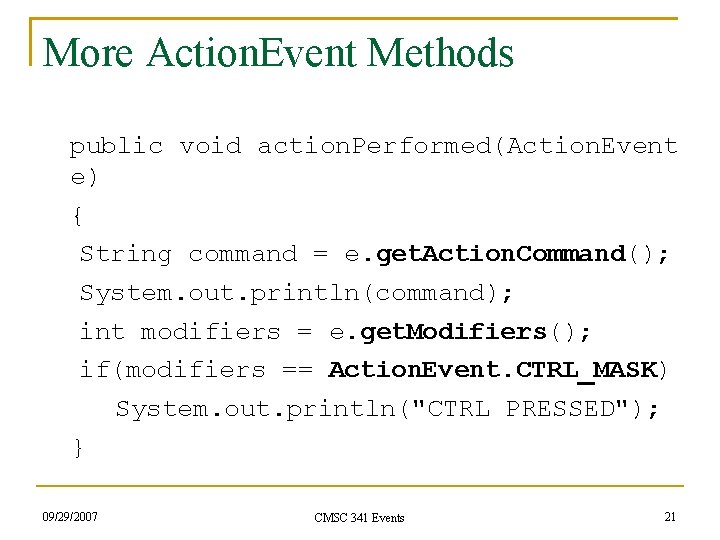
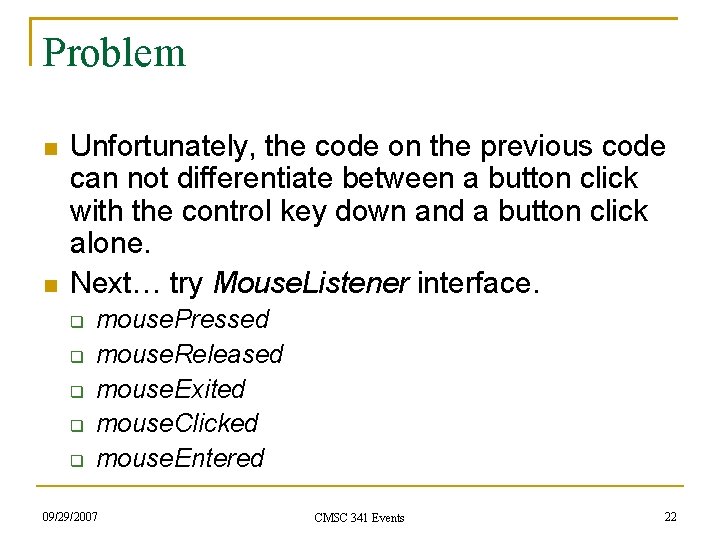
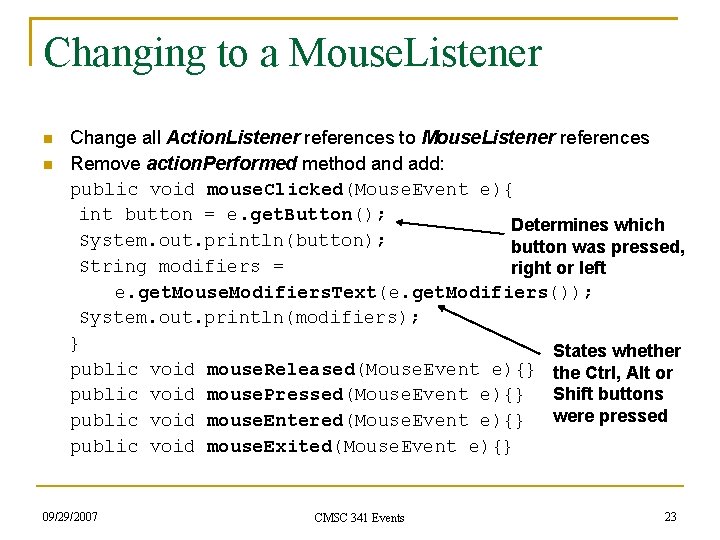
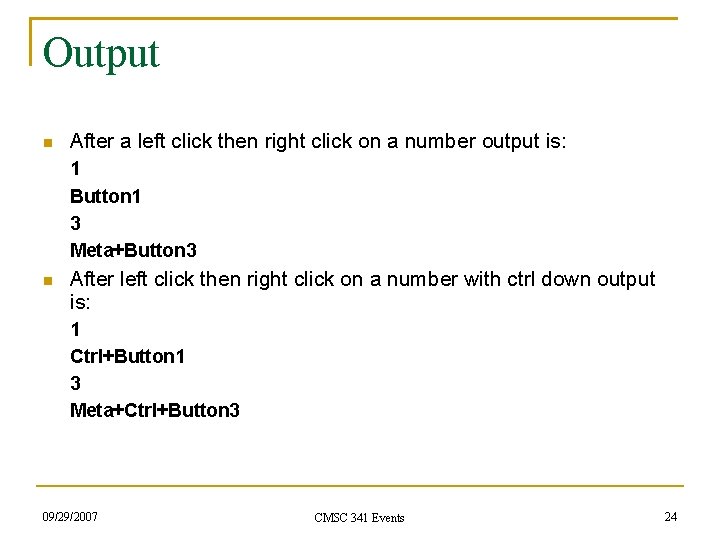
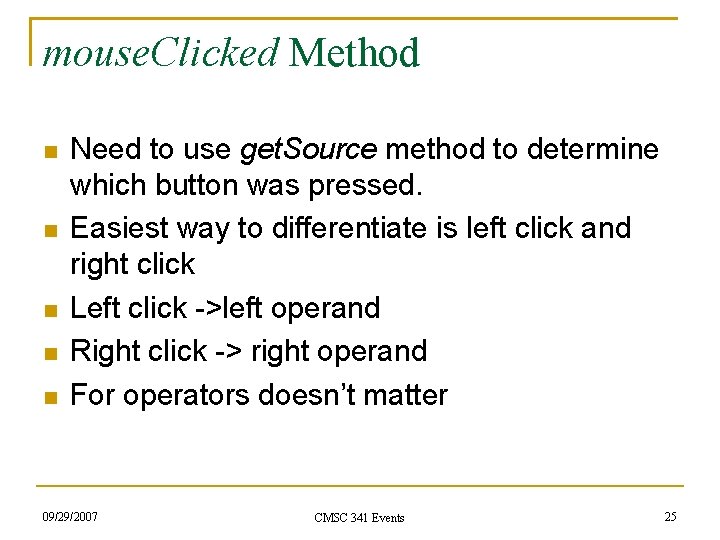
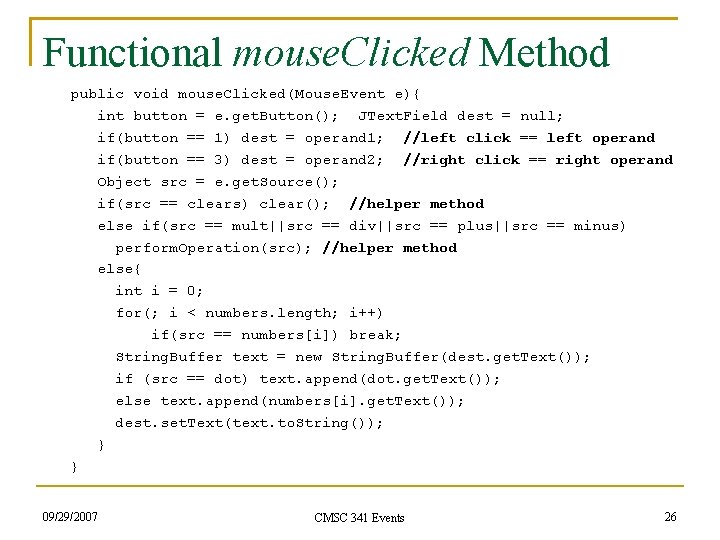
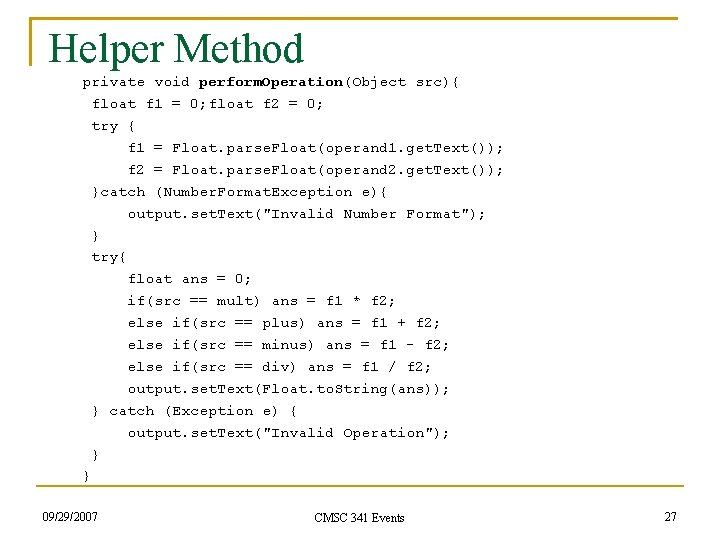
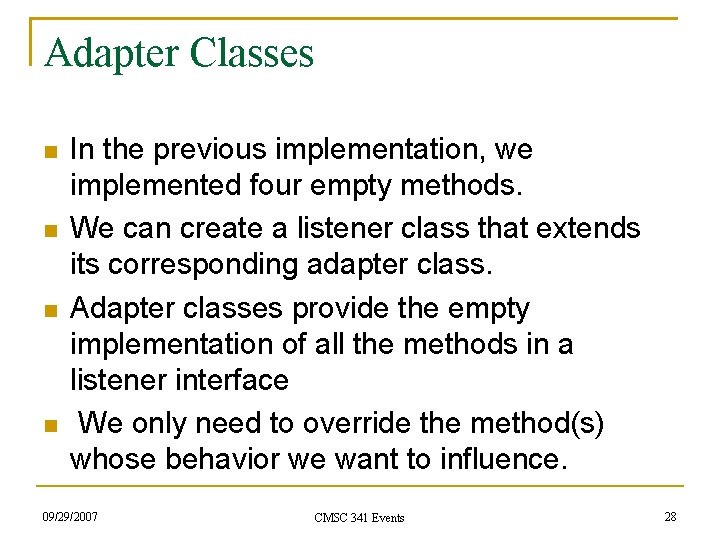
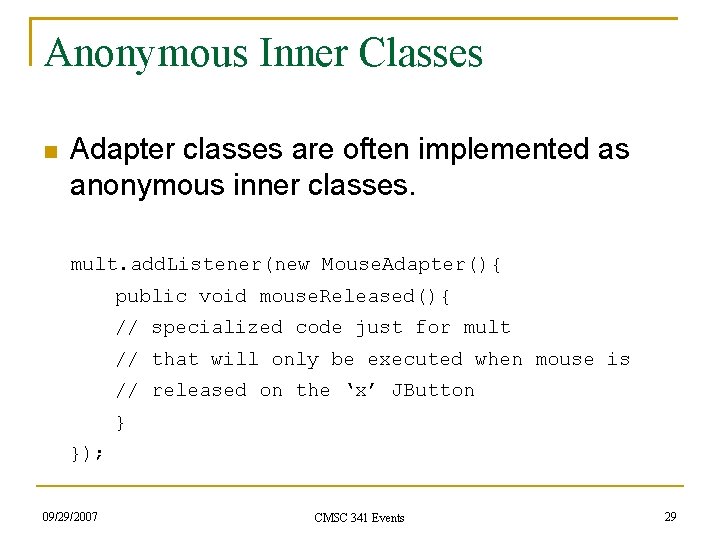
- Slides: 29
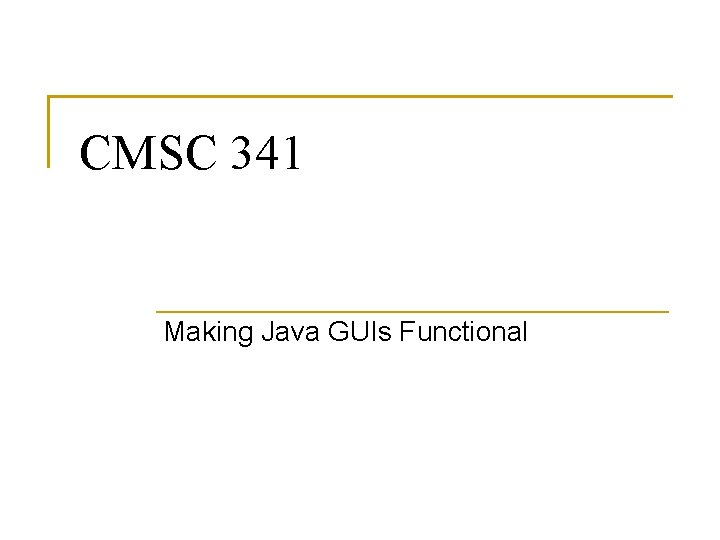
CMSC 341 Making Java GUIs Functional
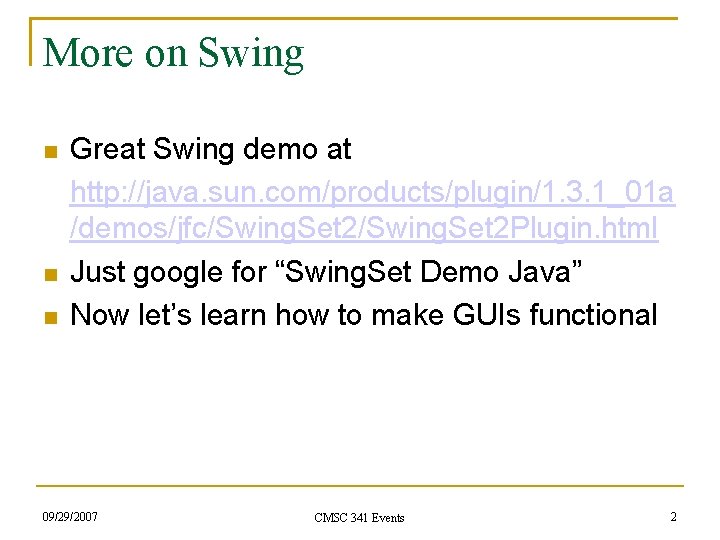
More on Swing Great Swing demo at http: //java. sun. com/products/plugin/1. 3. 1_01 a /demos/jfc/Swing. Set 2 Plugin. html Just google for “Swing. Set Demo Java” Now let’s learn how to make GUIs functional 09/29/2007 CMSC 341 Events 2
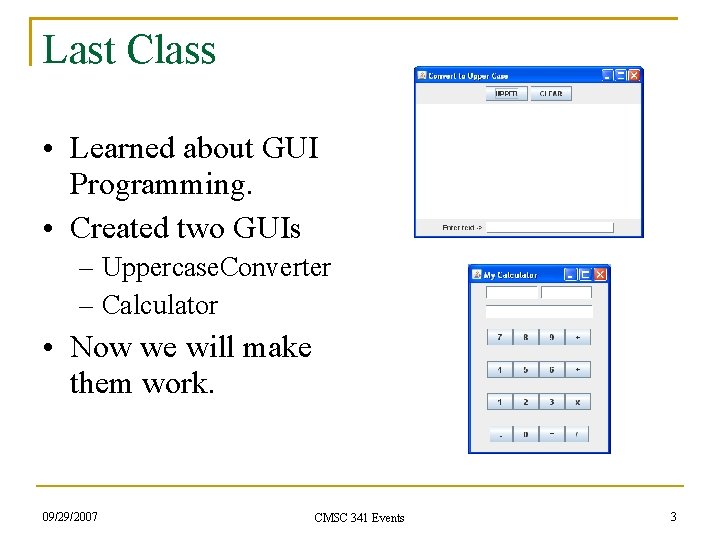
Last Class • Learned about GUI Programming. • Created two GUIs – Uppercase. Converter – Calculator • Now we will make them work. 09/29/2007 CMSC 341 Events 3
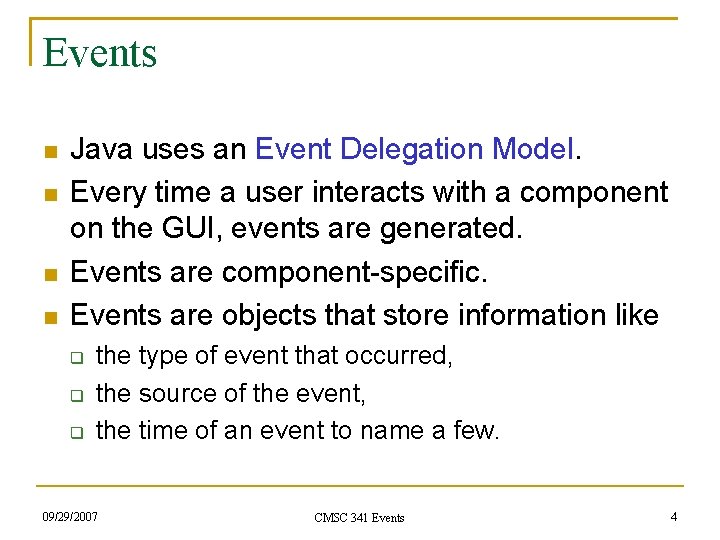
Events Java uses an Event Delegation Model. Every time a user interacts with a component on the GUI, events are generated. Events are component-specific. Events are objects that store information like the type of event that occurred, the source of the event, the time of an event to name a few. 09/29/2007 CMSC 341 Events 4
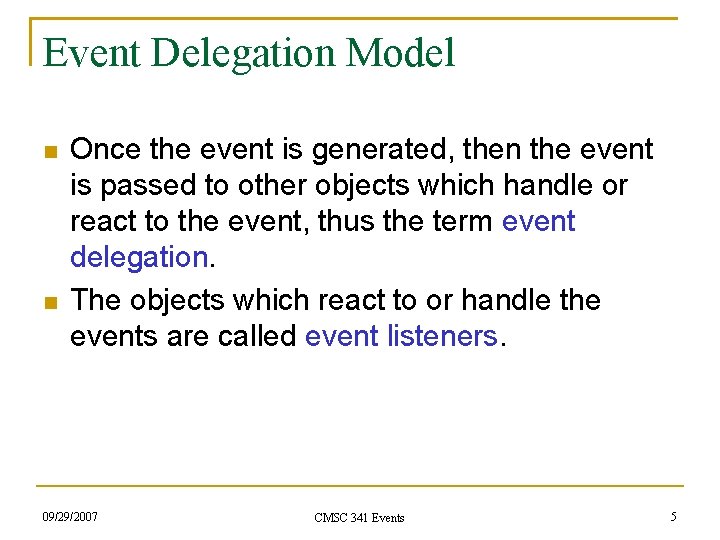
Event Delegation Model Once the event is generated, then the event is passed to other objects which handle or react to the event, thus the term event delegation. The objects which react to or handle the events are called event listeners. 09/29/2007 CMSC 341 Events 5
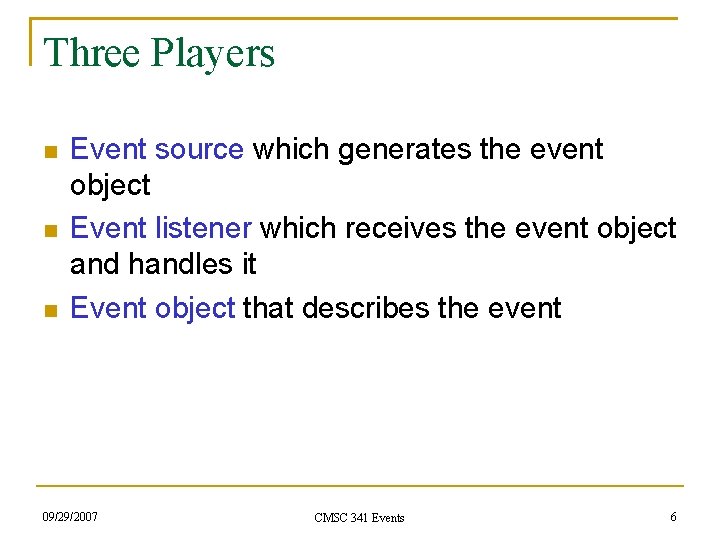
Three Players Event source which generates the event object Event listener which receives the event object and handles it Event object that describes the event 09/29/2007 CMSC 341 Events 6
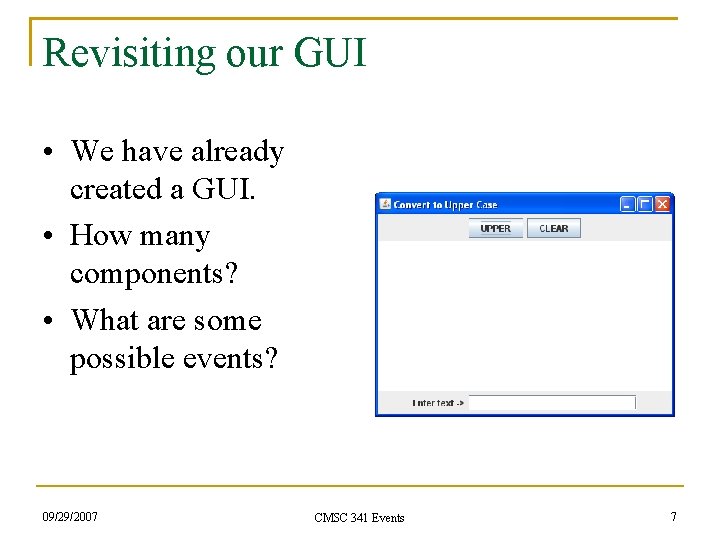
Revisiting our GUI • We have already created a GUI. • How many components? • What are some possible events? 09/29/2007 CMSC 341 Events 7
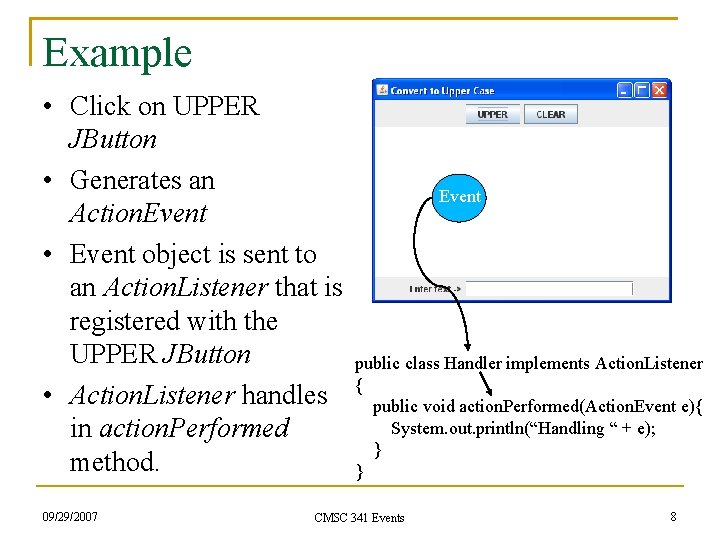
Example • Click on UPPER JButton • Generates an Action. Event • Event object is sent to an Action. Listener that is registered with the UPPER JButton • Action. Listener handles in action. Performed method. 09/29/2007 Event public class Handler implements Action. Listener { public void action. Performed(Action. Event e){ System. out. println(“Handling “ + e); } } CMSC 341 Events 8
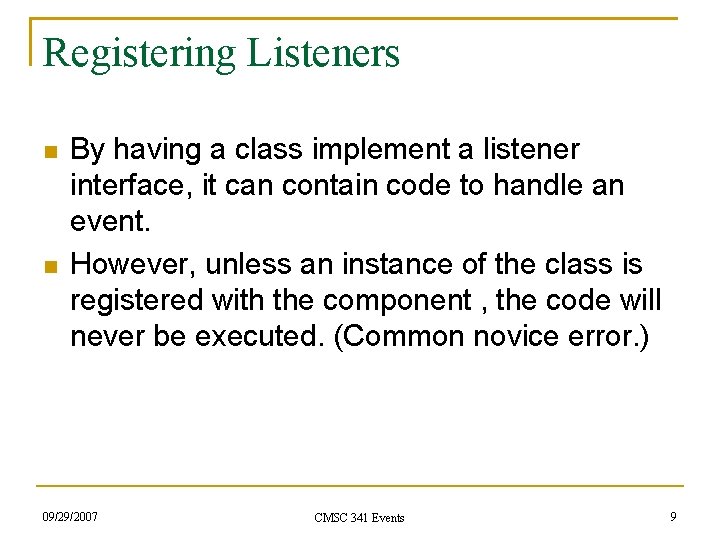
Registering Listeners By having a class implement a listener interface, it can contain code to handle an event. However, unless an instance of the class is registered with the component , the code will never be executed. (Common novice error. ) 09/29/2007 CMSC 341 Events 9
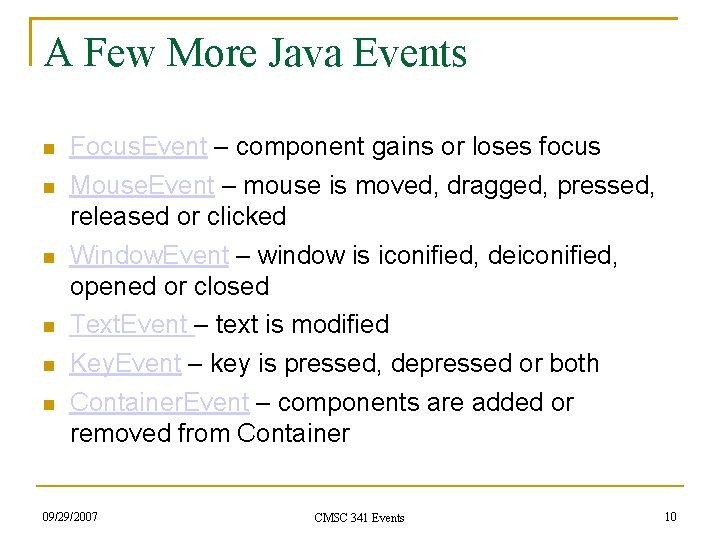
A Few More Java Events Focus. Event – component gains or loses focus Mouse. Event – mouse is moved, dragged, pressed, released or clicked Window. Event – window is iconified, deiconified, opened or closed Text. Event – text is modified Key. Event – key is pressed, depressed or both Container. Event – components are added or removed from Container 09/29/2007 CMSC 341 Events 10
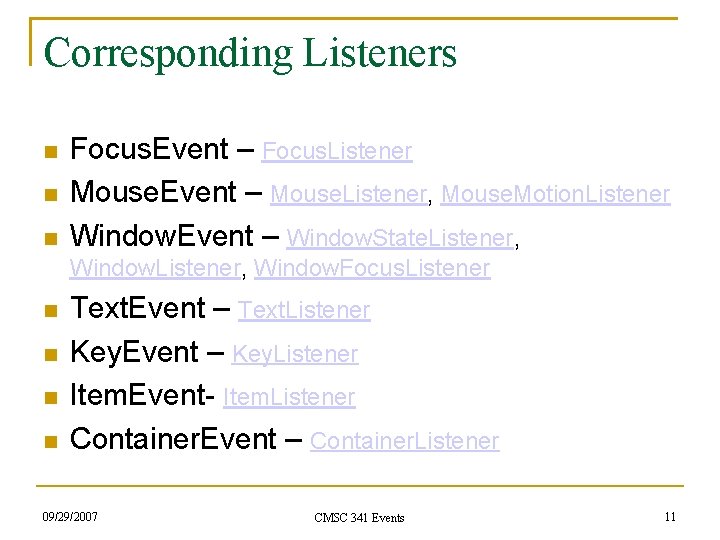
Corresponding Listeners Focus. Event – Focus. Listener Mouse. Event – Mouse. Listener, Mouse. Motion. Listener Window. Event – Window. State. Listener, Window. Focus. Listener Text. Event – Text. Listener Key. Event – Key. Listener Item. Event- Item. Listener Container. Event – Container. Listener 09/29/2007 CMSC 341 Events 11
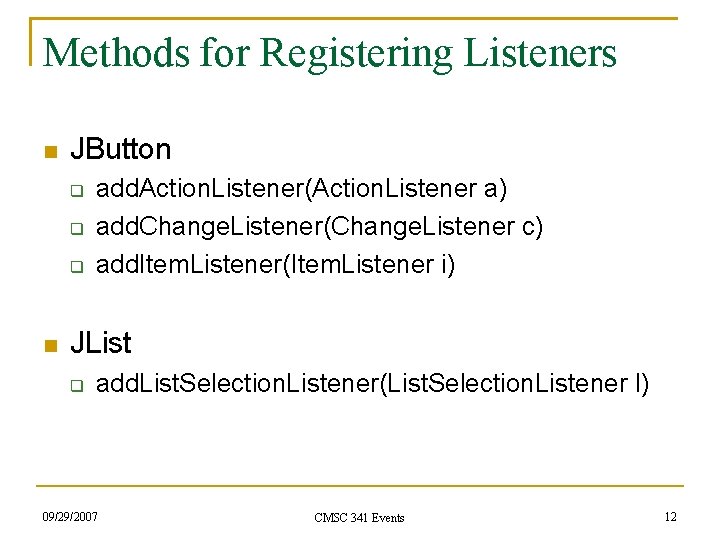
Methods for Registering Listeners JButton add. Action. Listener(Action. Listener a) add. Change. Listener(Change. Listener c) add. Item. Listener(Item. Listener i) JList add. List. Selection. Listener(List. Selection. Listener l) 09/29/2007 CMSC 341 Events 12
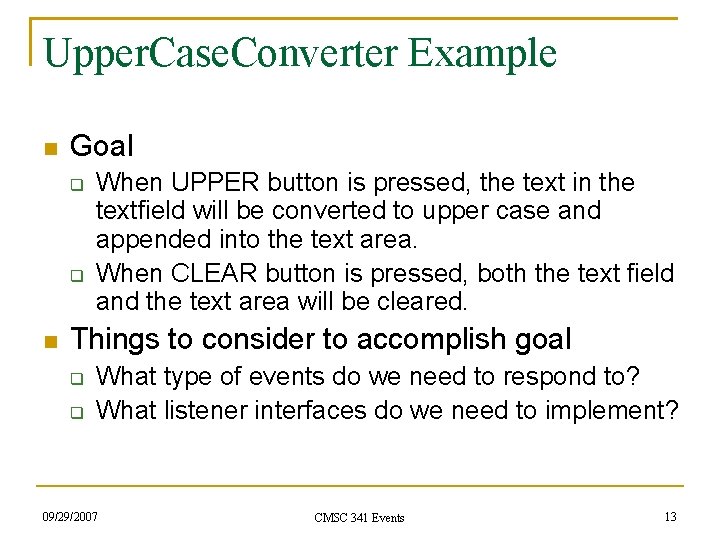
Upper. Case. Converter Example Goal When UPPER button is pressed, the text in the textfield will be converted to upper case and appended into the text area. When CLEAR button is pressed, both the text field and the text area will be cleared. Things to consider to accomplish goal What type of events do we need to respond to? What listener interfaces do we need to implement? 09/29/2007 CMSC 341 Events 13
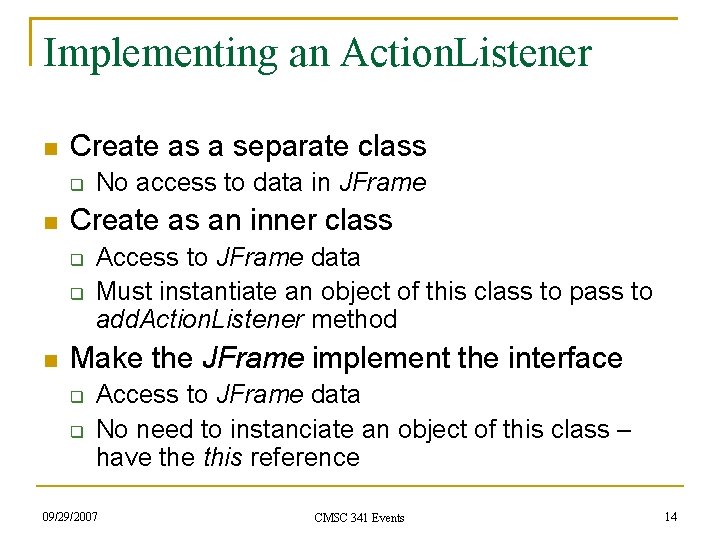
Implementing an Action. Listener Create as a separate class Create as an inner class No access to data in JFrame Access to JFrame data Must instantiate an object of this class to pass to add. Action. Listener method Make the JFrame implement the interface Access to JFrame data No need to instanciate an object of this class – have this reference 09/29/2007 CMSC 341 Events 14
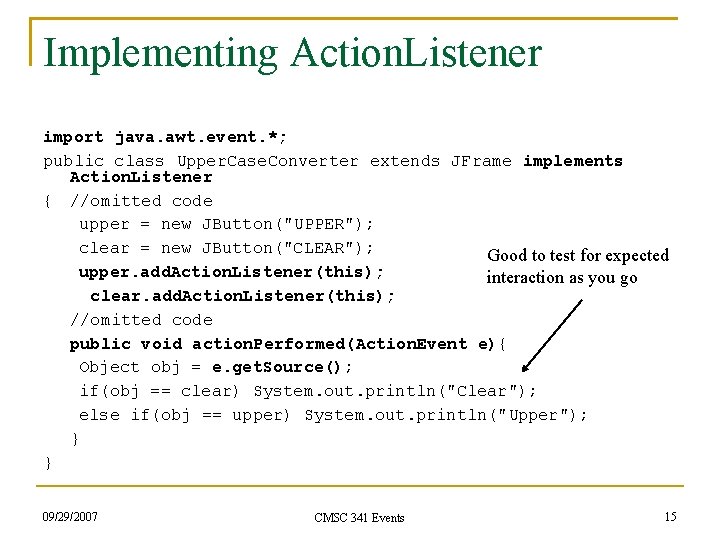
Implementing Action. Listener import java. awt. event. *; public class Upper. Case. Converter extends JFrame implements Action. Listener { //omitted code upper = new JButton("UPPER"); clear = new JButton("CLEAR"); Good to test for expected upper. add. Action. Listener(this); interaction as you go clear. add. Action. Listener(this); //omitted code public void action. Performed(Action. Event e){ Object obj = e. get. Source(); if(obj == clear) System. out. println("Clear"); else if(obj == upper) System. out. println("Upper"); } } 09/29/2007 CMSC 341 Events 15
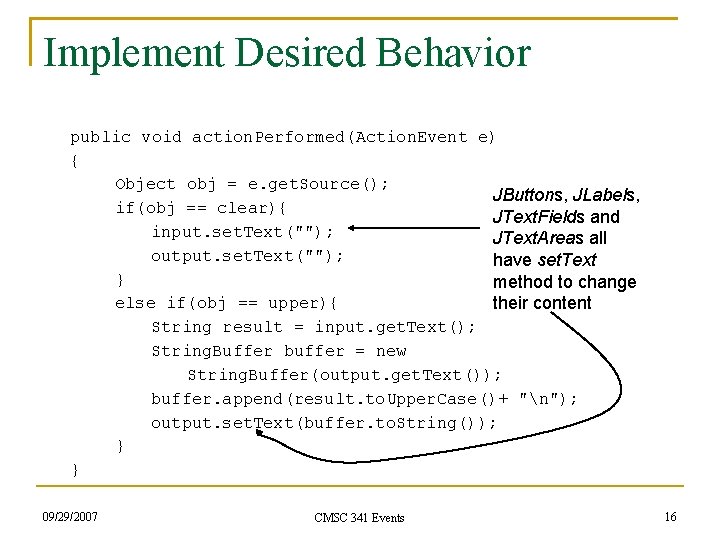
Implement Desired Behavior public void action. Performed(Action. Event e) { Object obj = e. get. Source(); JButtons, JLabels, if(obj == clear){ JText. Fields and input. set. Text(""); JText. Areas all output. set. Text(""); have set. Text } method to change else if(obj == upper){ their content String result = input. get. Text(); String. Buffer buffer = new String. Buffer(output. get. Text()); buffer. append(result. to. Upper. Case()+ "n"); output. set. Text(buffer. to. String()); } } 09/29/2007 CMSC 341 Events 16
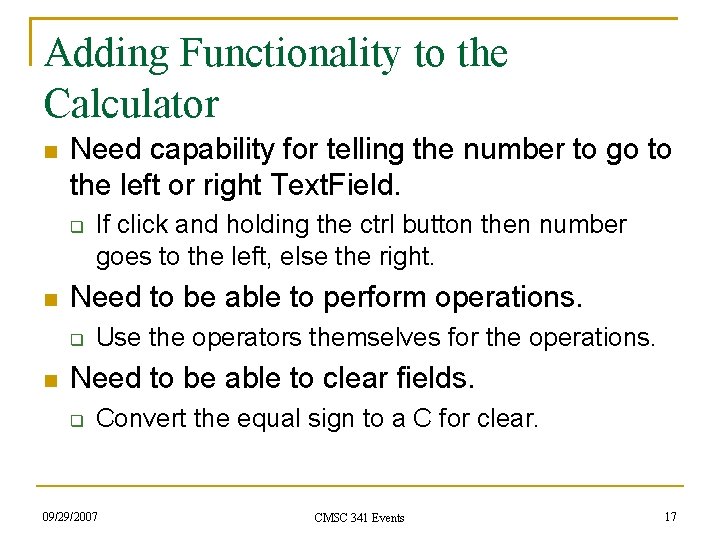
Adding Functionality to the Calculator Need capability for telling the number to go to the left or right Text. Field. Need to be able to perform operations. If click and holding the ctrl button then number goes to the left, else the right. Use the operators themselves for the operations. Need to be able to clear fields. Convert the equal sign to a C for clear. 09/29/2007 CMSC 341 Events 17
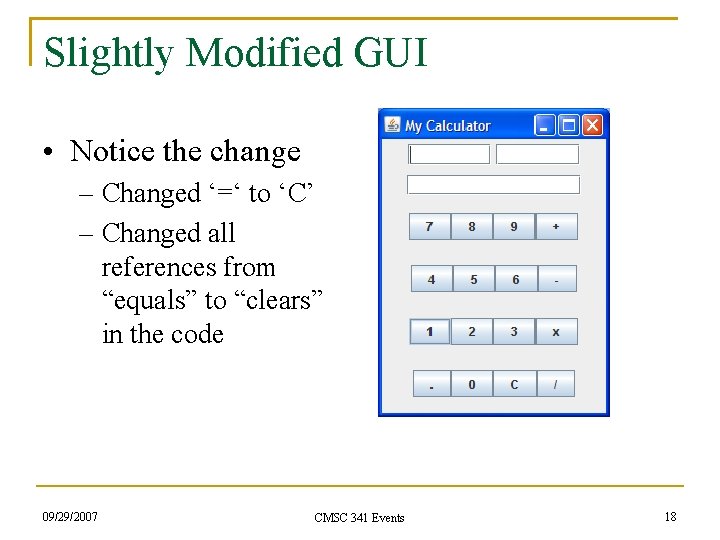
Slightly Modified GUI • Notice the change – Changed ‘=‘ to ‘C’ – Changed all references from “equals” to “clears” in the code 09/29/2007 CMSC 341 Events 18
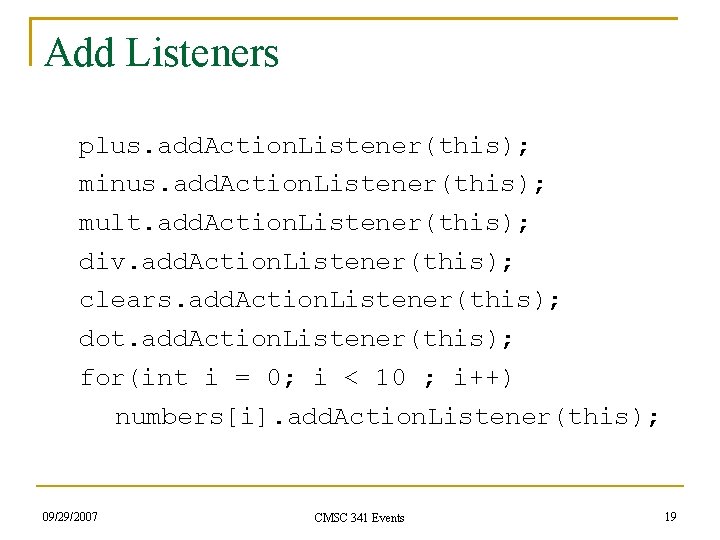
Add Listeners plus. add. Action. Listener(this); minus. add. Action. Listener(this); mult. add. Action. Listener(this); div. add. Action. Listener(this); clears. add. Action. Listener(this); dot. add. Action. Listener(this); for(int i = 0; i < 10 ; i++) numbers[i]. add. Action. Listener(this); 09/29/2007 CMSC 341 Events 19
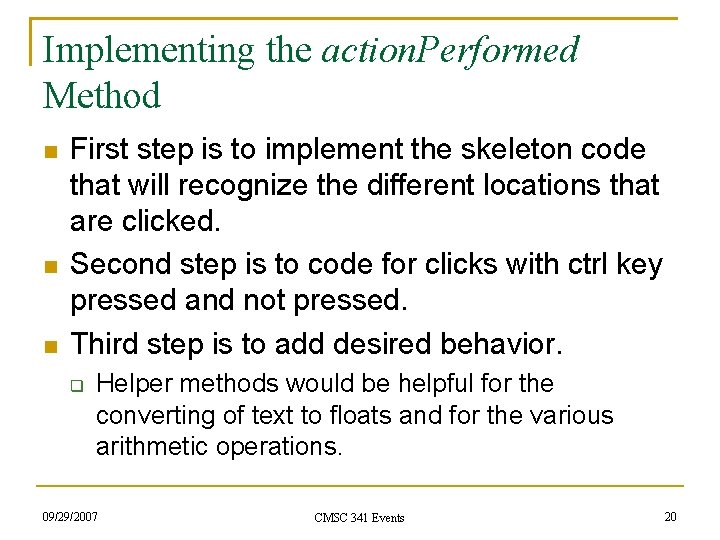
Implementing the action. Performed Method First step is to implement the skeleton code that will recognize the different locations that are clicked. Second step is to code for clicks with ctrl key pressed and not pressed. Third step is to add desired behavior. Helper methods would be helpful for the converting of text to floats and for the various arithmetic operations. 09/29/2007 CMSC 341 Events 20
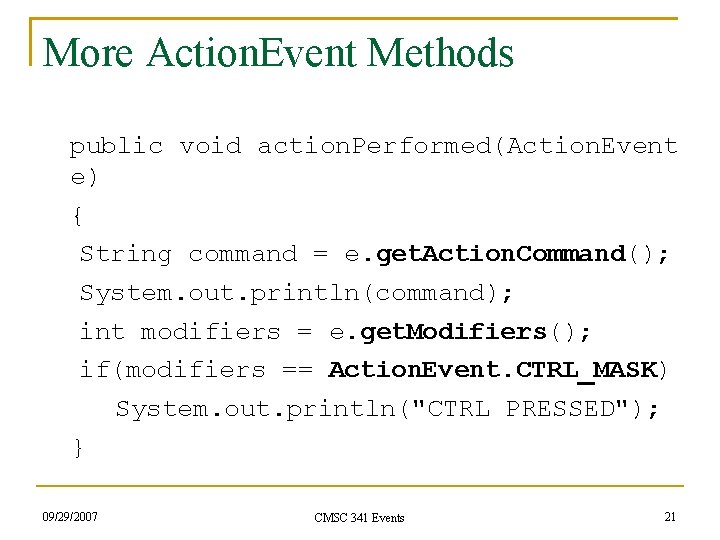
More Action. Event Methods public void action. Performed(Action. Event e) { String command = e. get. Action. Command(); System. out. println(command); int modifiers = e. get. Modifiers(); if(modifiers == Action. Event. CTRL_MASK) System. out. println("CTRL PRESSED"); } 09/29/2007 CMSC 341 Events 21
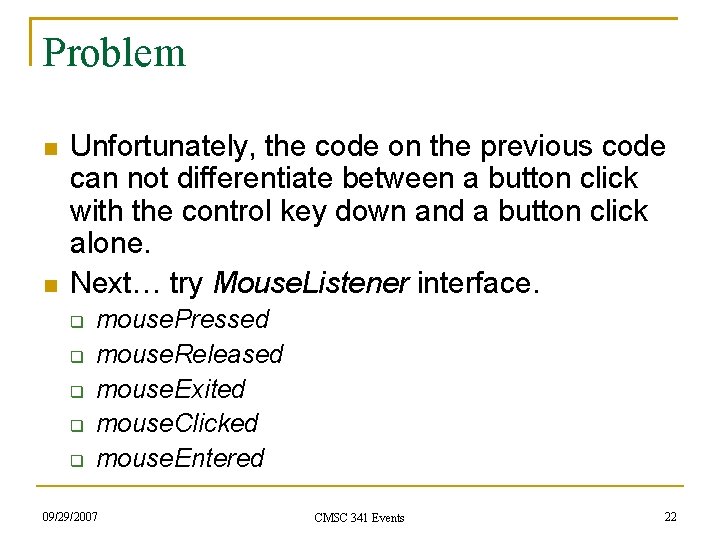
Problem Unfortunately, the code on the previous code can not differentiate between a button click with the control key down and a button click alone. Next… try Mouse. Listener interface. mouse. Pressed mouse. Released mouse. Exited mouse. Clicked mouse. Entered 09/29/2007 CMSC 341 Events 22
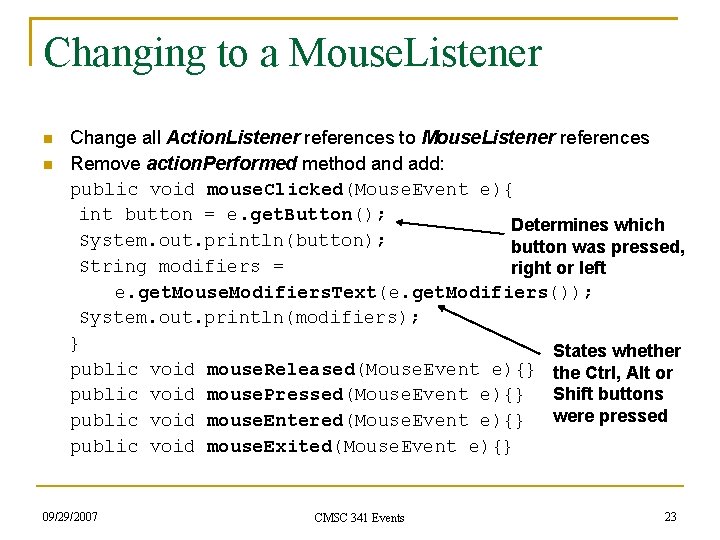
Changing to a Mouse. Listener Change all Action. Listener references to Mouse. Listener references Remove action. Performed method and add: public void mouse. Clicked(Mouse. Event e){ int button = e. get. Button(); Determines which System. out. println(button); button was pressed, String modifiers = right or left e. get. Mouse. Modifiers. Text(e. get. Modifiers()); System. out. println(modifiers); } States whether public void mouse. Released(Mouse. Event e){} the Ctrl, Alt or public void mouse. Pressed(Mouse. Event e){} Shift buttons public void mouse. Entered(Mouse. Event e){} were pressed public void mouse. Exited(Mouse. Event e){} 09/29/2007 CMSC 341 Events 23
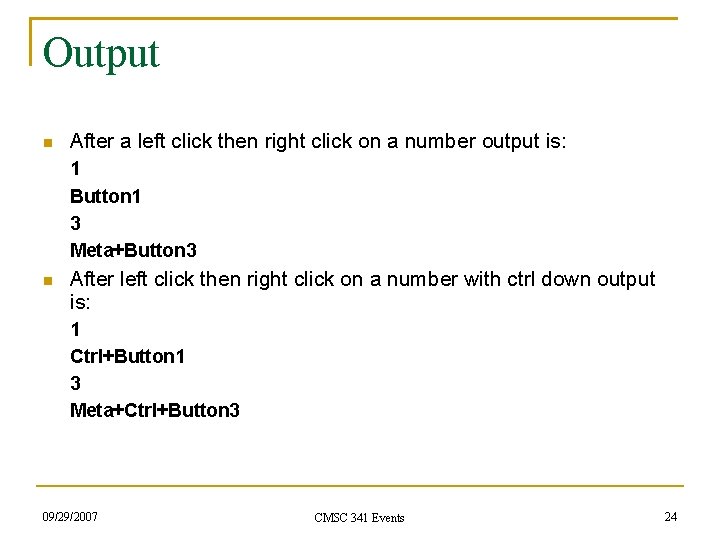
Output After a left click then right click on a number output is: 1 Button 1 3 Meta+Button 3 After left click then right click on a number with ctrl down output is: 1 Ctrl+Button 1 3 Meta+Ctrl+Button 3 09/29/2007 CMSC 341 Events 24
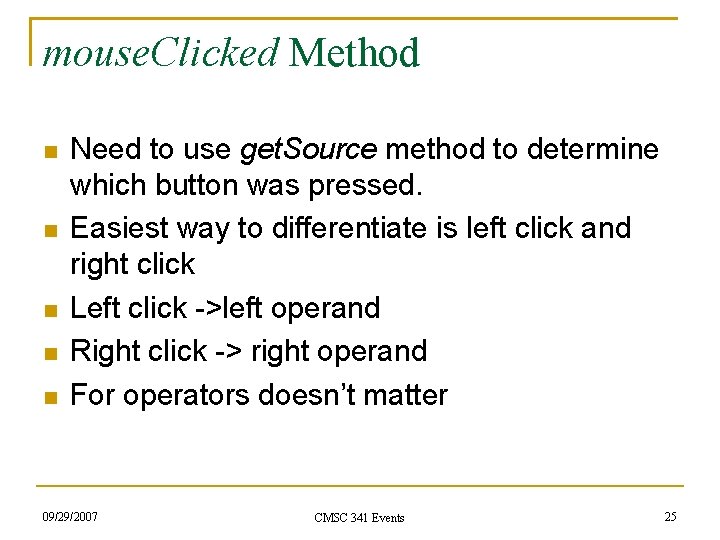
mouse. Clicked Method Need to use get. Source method to determine which button was pressed. Easiest way to differentiate is left click and right click Left click ->left operand Right click -> right operand For operators doesn’t matter 09/29/2007 CMSC 341 Events 25
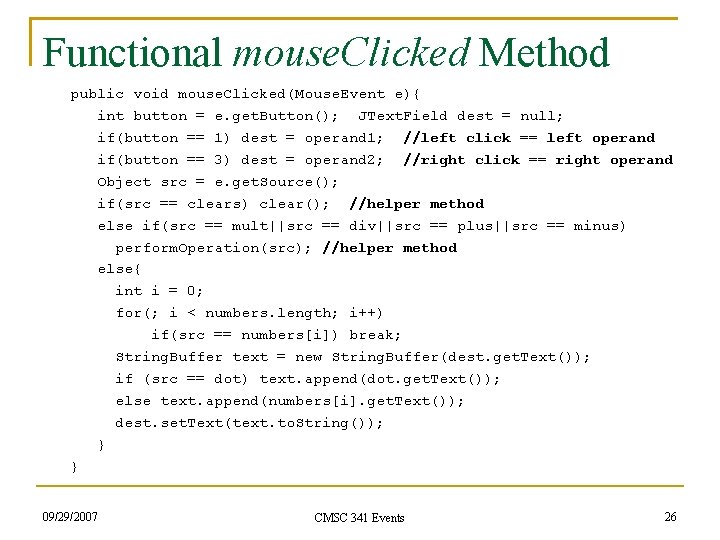
Functional mouse. Clicked Method public void mouse. Clicked(Mouse. Event e){ int button = e. get. Button(); JText. Field dest = null; if(button == 1) dest = operand 1; //left click == left operand if(button == 3) dest = operand 2; //right click == right operand Object src = e. get. Source(); if(src == clears) clear(); //helper method else if(src == mult||src == div||src == plus||src == minus) perform. Operation(src); //helper method else{ int i = 0; for(; i < numbers. length; i++) if(src == numbers[i]) break; String. Buffer text = new String. Buffer(dest. get. Text()); if (src == dot) text. append(dot. get. Text()); else text. append(numbers[i]. get. Text()); dest. set. Text(text. to. String()); } } 09/29/2007 CMSC 341 Events 26
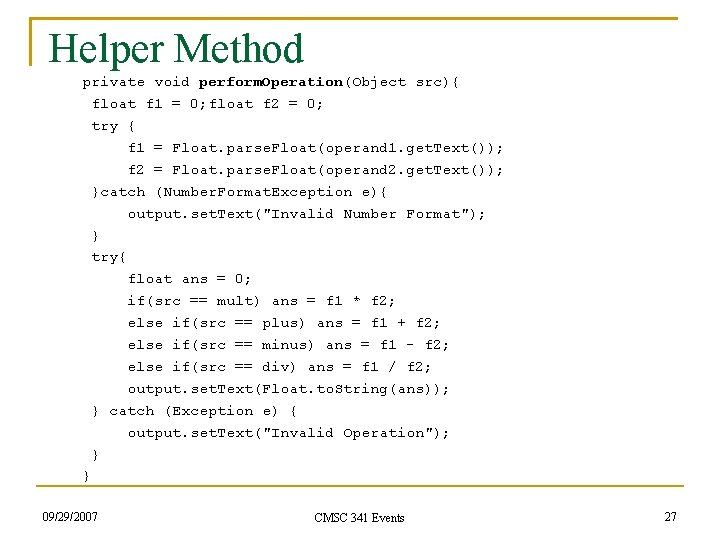
Helper Method private void perform. Operation(Object src){ float f 1 = 0; float f 2 = 0; try { f 1 = Float. parse. Float(operand 1. get. Text()); f 2 = Float. parse. Float(operand 2. get. Text()); }catch (Number. Format. Exception e){ output. set. Text("Invalid Number Format"); } try{ float ans = 0; if(src == mult) ans = f 1 * f 2; else if(src == plus) ans = f 1 + f 2; else if(src == minus) ans = f 1 - f 2; else if(src == div) ans = f 1 / f 2; output. set. Text(Float. to. String(ans)); } catch (Exception e) { output. set. Text("Invalid Operation"); } } 09/29/2007 CMSC 341 Events 27
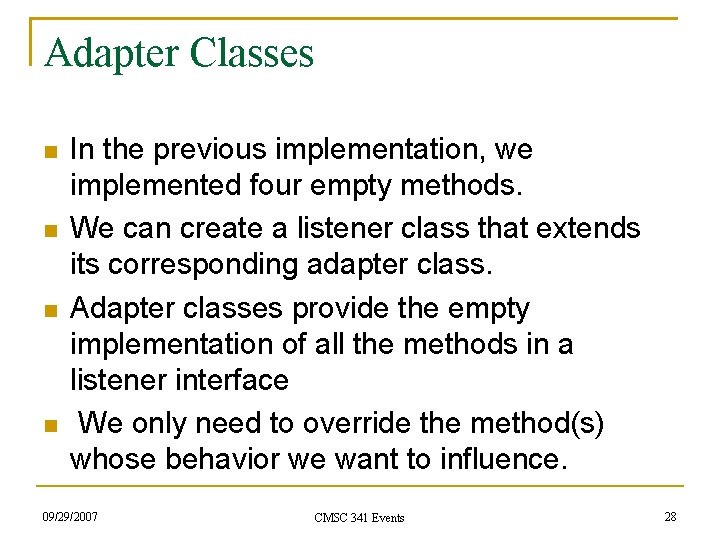
Adapter Classes In the previous implementation, we implemented four empty methods. We can create a listener class that extends its corresponding adapter class. Adapter classes provide the empty implementation of all the methods in a listener interface We only need to override the method(s) whose behavior we want to influence. 09/29/2007 CMSC 341 Events 28
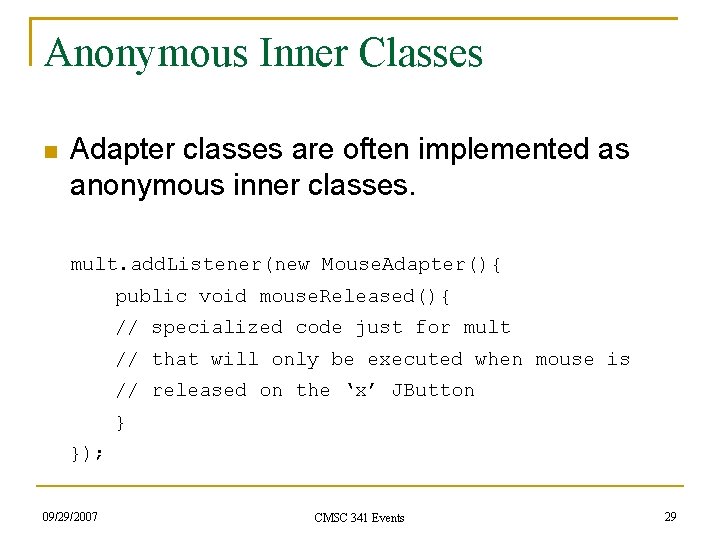
Anonymous Inner Classes Adapter classes are often implemented as anonymous inner classes. mult. add. Listener(new Mouse. Adapter(){ public void mouse. Released(){ // specialized code just for mult // that will only be executed when mouse is // released on the ‘x’ JButton } }); 09/29/2007 CMSC 341 Events 29
More more more i want more more more more we praise you
More more more i want more more more more we praise you
Cmsc 341
Cmsc 341 umbc
Cmsc 341
Umbc cmsc 341
Umbc cmsc 341
Cmsc 341
Cmsc 341
Umbc cmsc 202
Umbc cmsc 341
Cmsc 341
Cmsc 341
Gui graphics
Characteristics of web user interface
Band and loop space maintainer advantages
Functional and non functional plasma enzymes
Enzymes
Functional and non functional
Sda hymn 341
Ecma-341
631 to the nearest 10
Komax bc
Cse 341
341 ces
Xr-341
Oh mon sauveur a toi seul je veux etre
Mgmt 341
Ncg 341
Cs341 uwaterloo