CH 2 INTRODUCTION TO PASCAL PROGRAMMING program Check
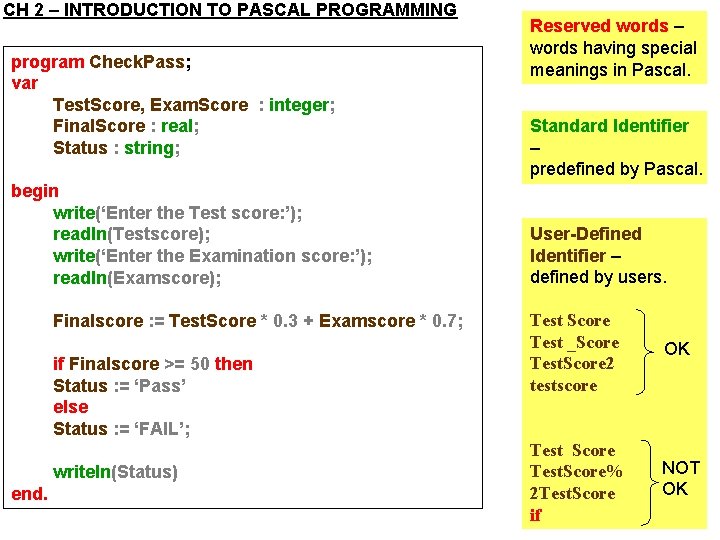
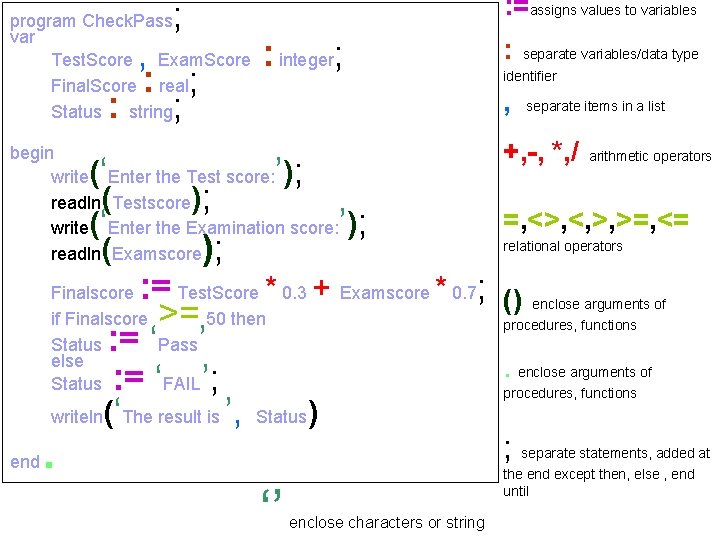
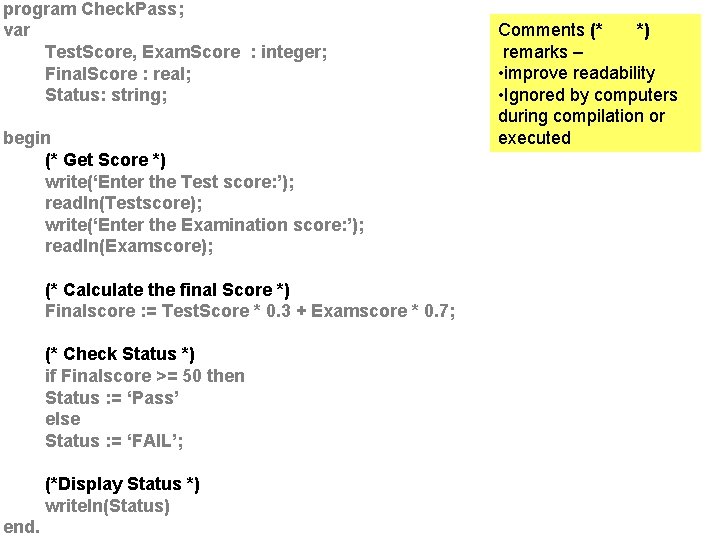
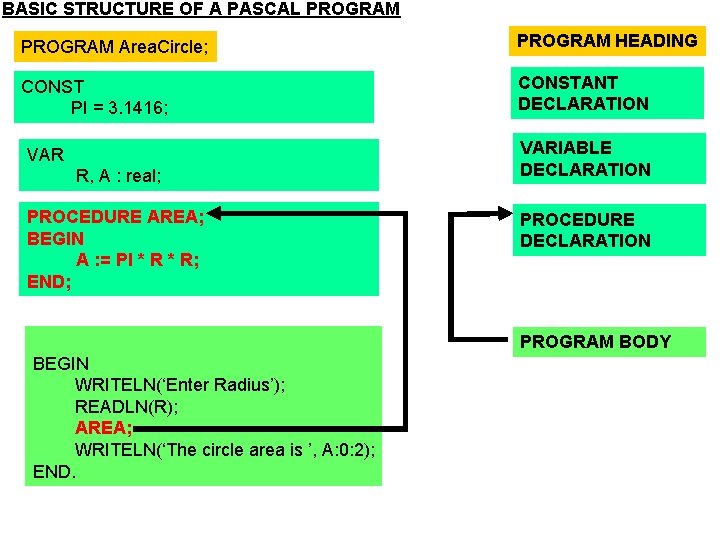
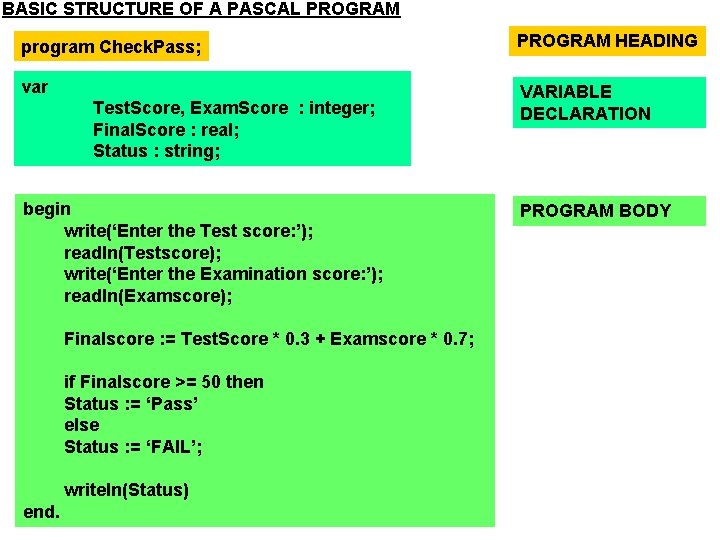
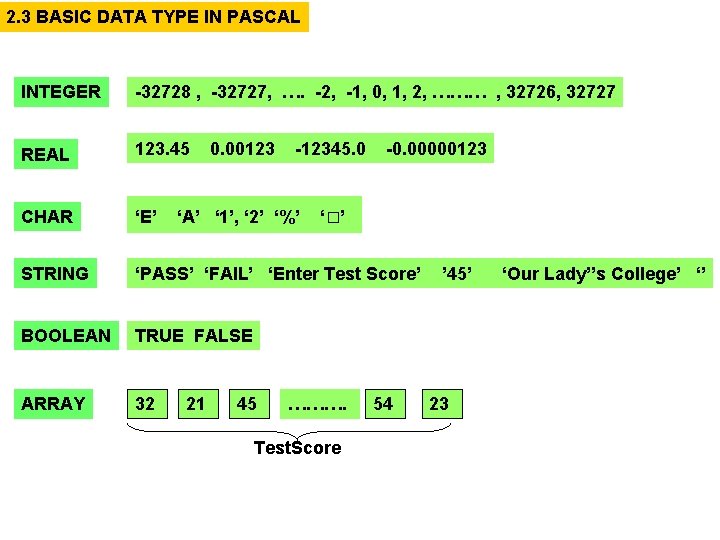
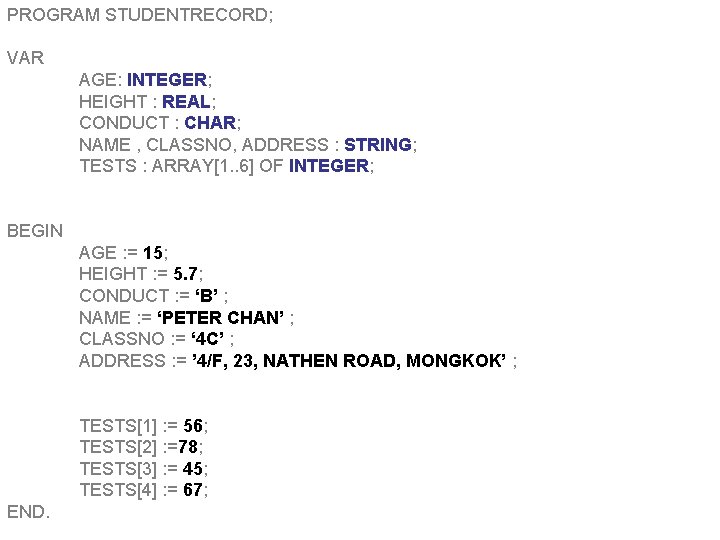
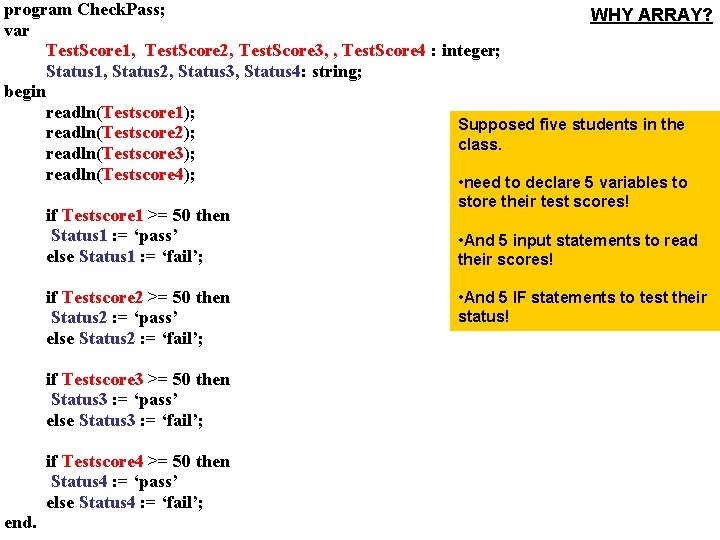
![program Check. Pass; var Test. Score: array[1. . 4] of integer; Status: array[1. . program Check. Pass; var Test. Score: array[1. . 4] of integer; Status: array[1. .](https://slidetodoc.com/presentation_image/15011cd6a6459fe2cabdac06a24ad9f4/image-9.jpg)
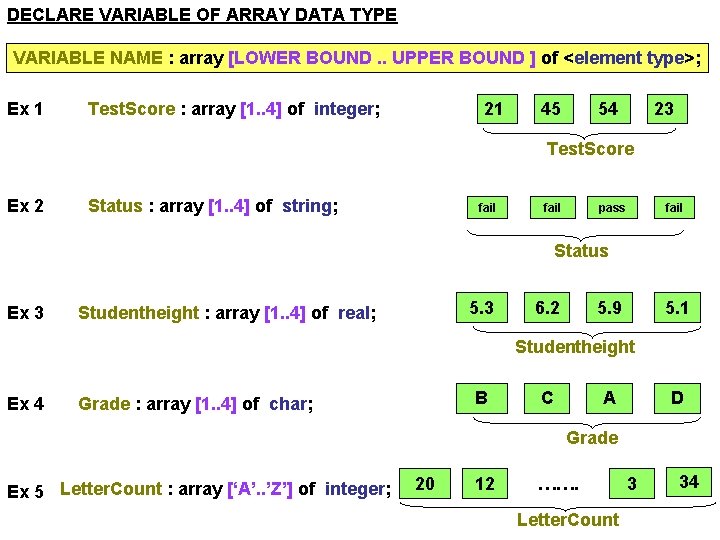
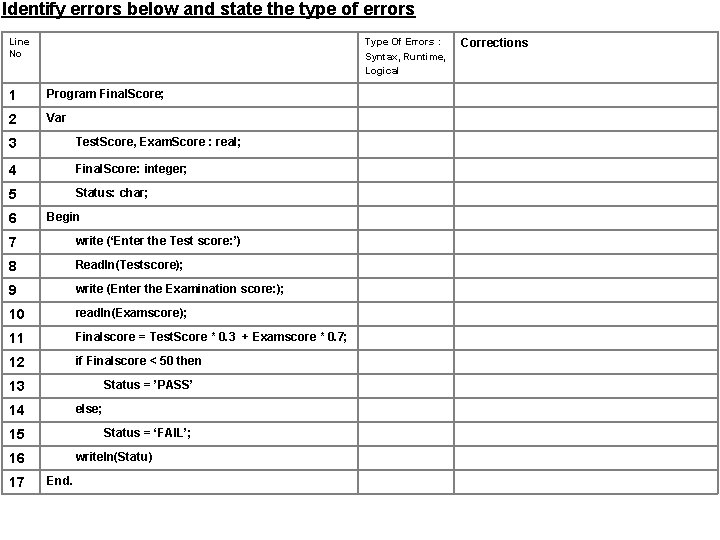
- Slides: 11
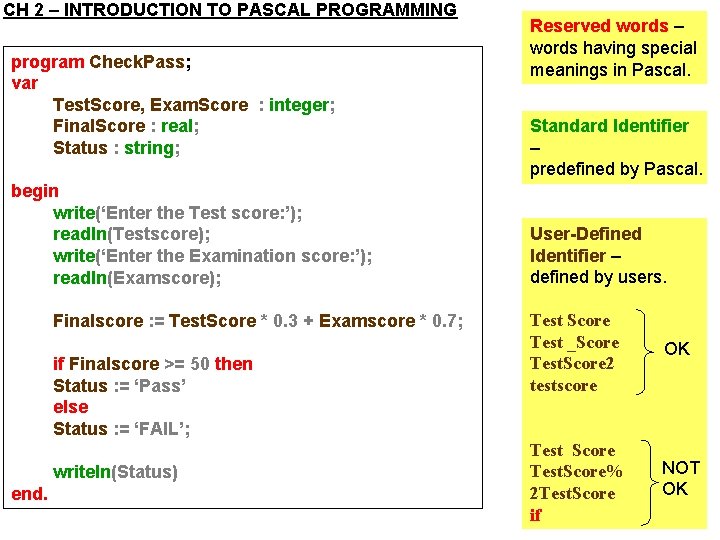
CH 2 – INTRODUCTION TO PASCAL PROGRAMMING program Check. Pass; var Test. Score, Exam. Score : integer; Final. Score : real; Status : string; begin write(‘Enter the Test score: ’); readln(Testscore); write(‘Enter the Examination score: ’); readln(Examscore); Finalscore : = Test. Score * 0. 3 + Examscore * 0. 7; if Finalscore >= 50 then Status : = ‘Pass’ else Status : = ‘FAIL’; writeln(Status) end. Reserved words – words having special meanings in Pascal. Standard Identifier – predefined by Pascal. User-Defined Identifier – defined by users. Test Score Test _Score Test. Score 2 testscore OK Test Score Test. Score% 2 Test. Score if NOT OK
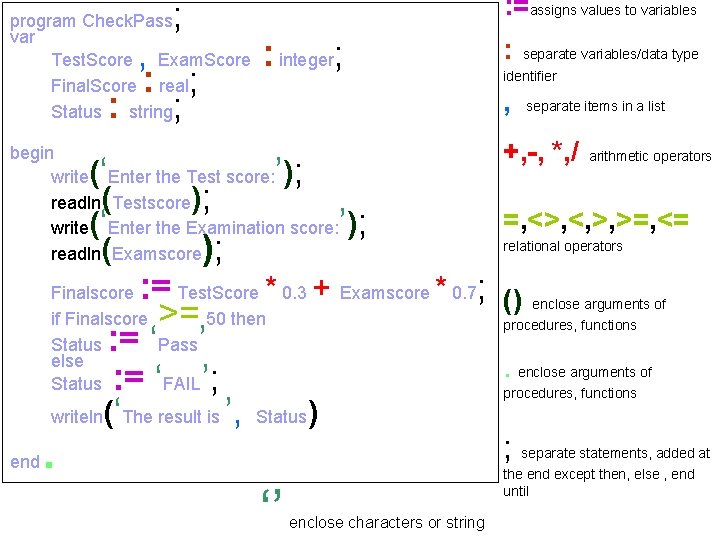
; program Check. Pass var Test. Score Exam. Score Final. Score real Status string , : ; : =assigns values to variables : integer; : separate variables/data type identifier , separate items in a list +, -, *, / begin write readln arithmetic operators (‘Enter the Test score: ’); (Testscore); =, <>, <, >, >=, <= (‘Enter the Examination score: ’); relational operators (Examscore); Finalscore : = Test. Score * 0. 3 + Examscore * 0. 7; () enclose arguments of >= : = ‘ ’; writeln(‘The result is ’, if Finalscore 50 then Status Pass else Status FAIL end . procedures, functions . Status ‘’ ) enclose arguments of procedures, functions ; separate statements, added at the end except then, else , end until enclose characters or string
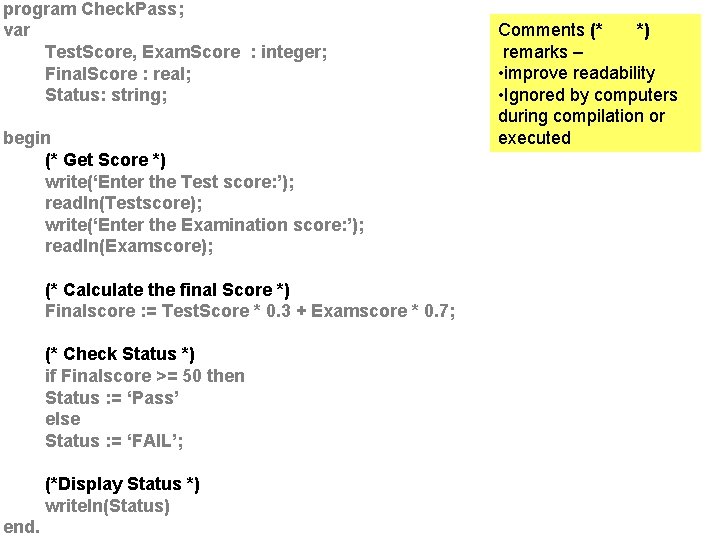
program Check. Pass; var Test. Score, Exam. Score : integer; Final. Score : real; Status: string; begin (* Get Score *) write(‘Enter the Test score: ’); readln(Testscore); write(‘Enter the Examination score: ’); readln(Examscore); (* Calculate the final Score *) Finalscore : = Test. Score * 0. 3 + Examscore * 0. 7; (* Check Status *) if Finalscore >= 50 then Status : = ‘Pass’ else Status : = ‘FAIL’; (*Display Status *) writeln(Status) end. Comments (* *) remarks – • improve readability • Ignored by computers during compilation or executed
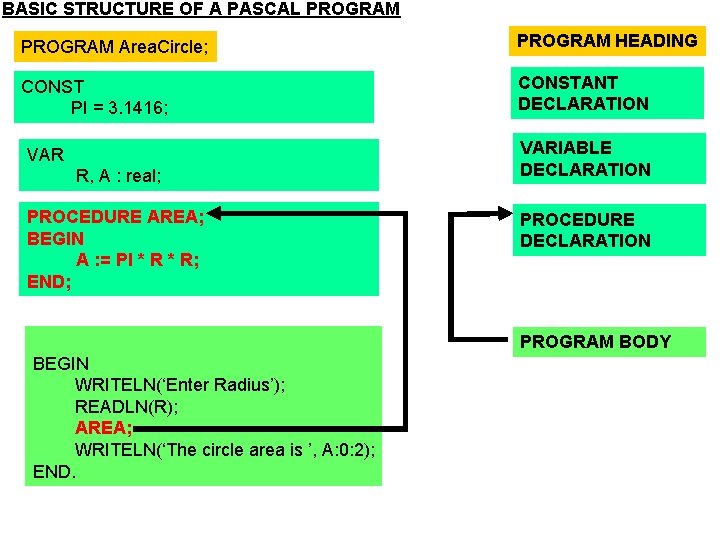
BASIC STRUCTURE OF A PASCAL PROGRAM Area. Circle; PROGRAM HEADING CONST PI = 3. 1416; CONSTANT DECLARATION VAR R, A : real; PROCEDURE AREA; BEGIN A : = PI * R; END; VARIABLE DECLARATION PROCEDURE DECLARATION PROGRAM BODY BEGIN WRITELN(‘Enter Radius’); READLN(R); AREA; WRITELN(‘The circle area is ’, A: 0: 2); END.
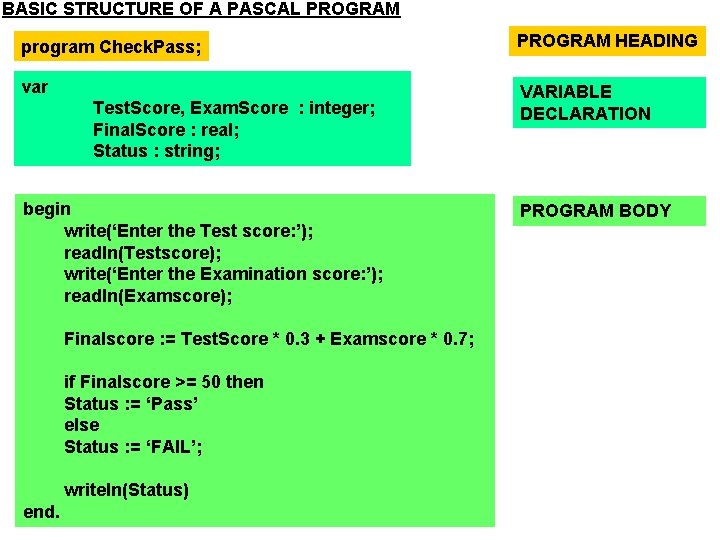
BASIC STRUCTURE OF A PASCAL PROGRAM program Check. Pass; PROGRAM HEADING var VARIABLE DECLARATION Test. Score, Exam. Score : integer; Final. Score : real; Status : string; begin write(‘Enter the Test score: ’); readln(Testscore); write(‘Enter the Examination score: ’); readln(Examscore); Finalscore : = Test. Score * 0. 3 + Examscore * 0. 7; if Finalscore >= 50 then Status : = ‘Pass’ else Status : = ‘FAIL’; writeln(Status) end. PROGRAM BODY
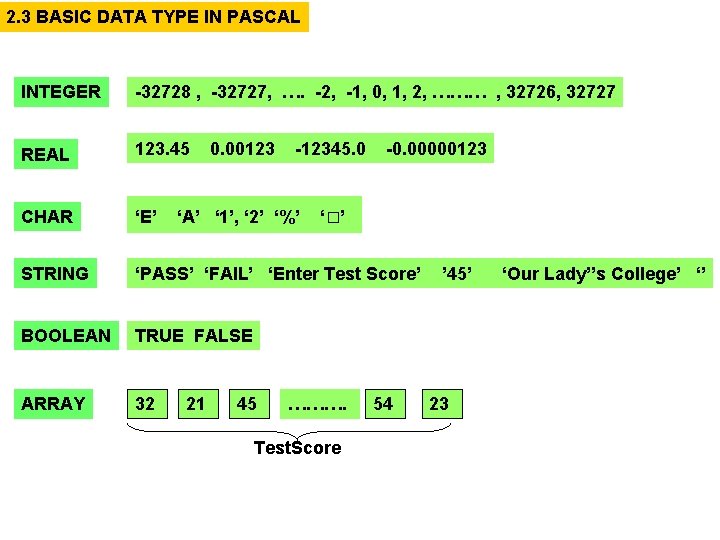
2. 3 BASIC DATA TYPE IN PASCAL INTEGER -32728 , -32727, …. -2, -1, 0, 1, 2, ……… , 32726, 32727 REAL 123. 45 CHAR ‘E’ STRING ‘PASS’ ‘FAIL’ ‘Enter Test Score’ BOOLEAN TRUE FALSE ARRAY 32 0. 00123 -12345. 0 ‘A’ ‘ 1’, ‘ 2’ ‘%’ 21 45 -0. 00000123 ‘ □’ ………. Test. Score 54 ’ 45’ 23 ‘Our Lady’’s College’ ‘’
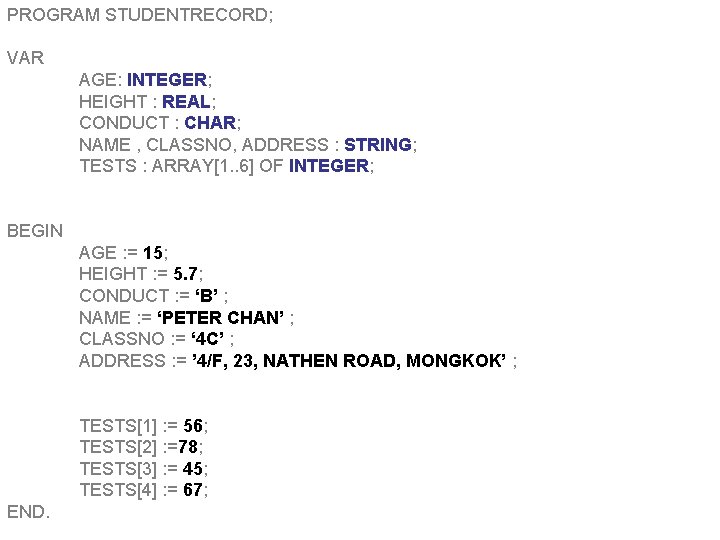
PROGRAM STUDENTRECORD; VAR AGE: INTEGER; HEIGHT : REAL; CONDUCT : CHAR; NAME , CLASSNO, ADDRESS : STRING; TESTS : ARRAY[1. . 6] OF INTEGER; BEGIN AGE : = 15; HEIGHT : = 5. 7; CONDUCT : = ‘B’ ; NAME : = ‘PETER CHAN’ ; CLASSNO : = ‘ 4 C’ ; ADDRESS : = ’ 4/F, 23, NATHEN ROAD, MONGKOK’ ; TESTS[1] : = 56; TESTS[2] : =78; TESTS[3] : = 45; TESTS[4] : = 67; END.
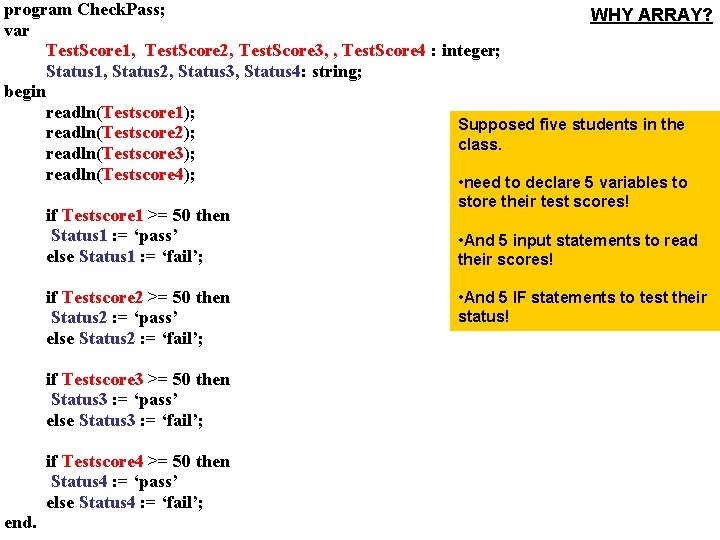
program Check. Pass; WHY ARRAY? var Test. Score 1, Test. Score 2, Test. Score 3, , Test. Score 4 : integer; Status 1, Status 2, Status 3, Status 4: string; begin readln(Testscore 1); Supposed five students in the readln(Testscore 2); class. readln(Testscore 3); readln(Testscore 4); • need to declare 5 variables to if Testscore 1 >= 50 then Status 1 : = ‘pass’ else Status 1 : = ‘fail’; if Testscore 2 >= 50 then Status 2 : = ‘pass’ else Status 2 : = ‘fail’; if Testscore 3 >= 50 then Status 3 : = ‘pass’ else Status 3 : = ‘fail’; if Testscore 4 >= 50 then Status 4 : = ‘pass’ else Status 4 : = ‘fail’; end. store their test scores! • And 5 input statements to read their scores! • And 5 IF statements to test their status!
![program Check Pass var Test Score array1 4 of integer Status array1 program Check. Pass; var Test. Score: array[1. . 4] of integer; Status: array[1. .](https://slidetodoc.com/presentation_image/15011cd6a6459fe2cabdac06a24ad9f4/image-9.jpg)
program Check. Pass; var Test. Score: array[1. . 4] of integer; Status: array[1. . 4] of string; I : integer; begin for I : = 1 to 4 do readln(Testscore[I]) for I : = 1 to 4 do if Testscore[I]>= 50 then Status[I] : = ‘pass’ else Status[I] : = ‘fail’; end. 21 45 54 23 Test. Score fail pass Status fail
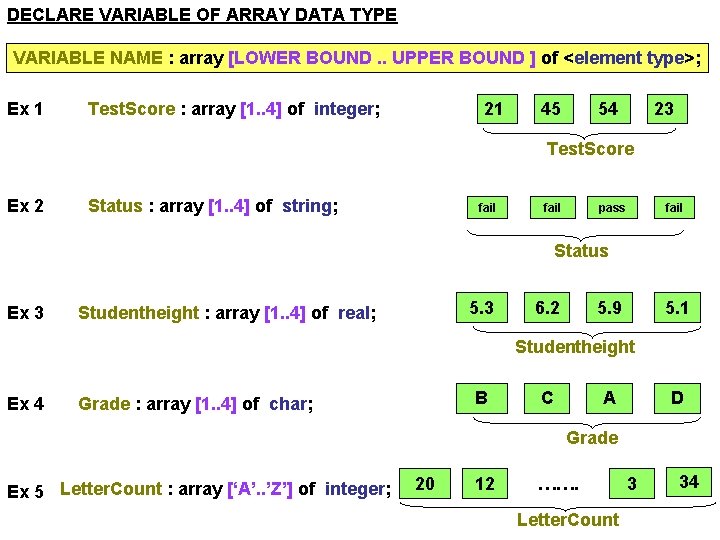
DECLARE VARIABLE OF ARRAY DATA TYPE VARIABLE NAME : array [LOWER BOUND. . UPPER BOUND ] of <element type>; Ex 1 Test. Score : array [1. . 4] of integer; 21 45 54 23 Test. Score Ex 2 Status : array [1. . 4] of string; fail pass fail Status Ex 3 5. 3 Studentheight : array [1. . 4] of real; 6. 2 5. 9 5. 1 Studentheight Ex 4 B Grade : array [1. . 4] of char; C A D Grade Ex 5 Letter. Count : array [‘A’. . ’Z’] of integer; 20 12 ……. Letter. Count 3 34
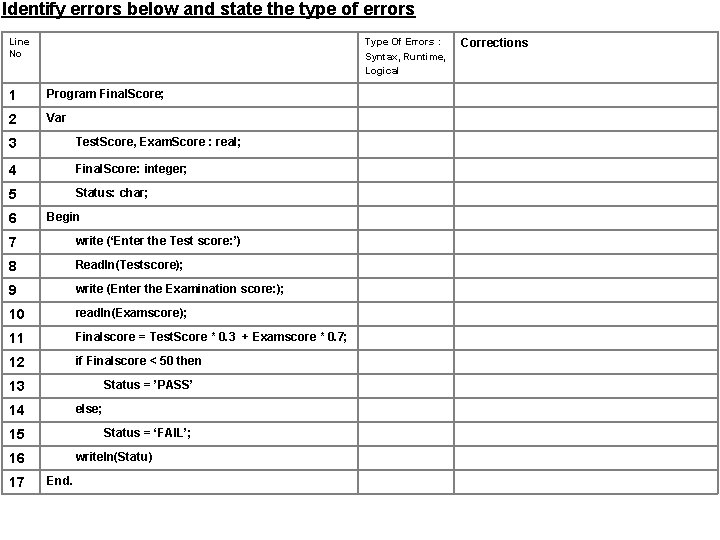
Identify errors below and state the type of errors Line No Type Of Errors : Syntax, Runtime, Logical 1 Program Final. Score; 2 Var 3 Test. Score, Exam. Score : real; 4 Final. Score: integer; 5 Status: char; 6 Begin 7 write (‘Enter the Test score: ’) 8 Readln(Testscore); 9 write (Enter the Examination score: ); 10 readln(Examscore); 11 Finalscore = Test. Score * 0. 3 + Examscore * 0. 7; 12 if Finalscore < 50 then Status = ’PASS’ 13 else; 14 Status = ‘FAIL’; 15 writeln(Statu) 16 17 End. Corrections
Pascal programs examples
Behavior check in check out sheet
Check in check out forms
Check in check out behavior intervention
Check in check out system for students
Cashier check
Dda algorithm advantages and disadvantages
Behavior check-in
Check in check out intervention
Collision forces quick check
How to sign a check over
Check your progress 1