5 include stdio h void mainvoid struct int
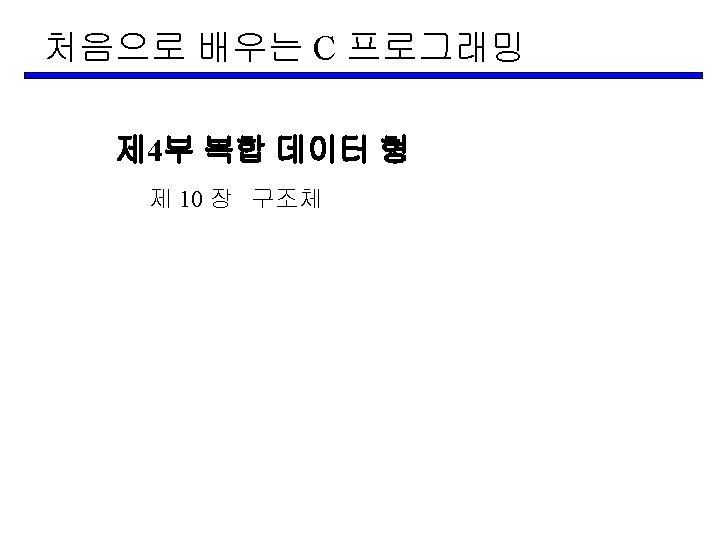
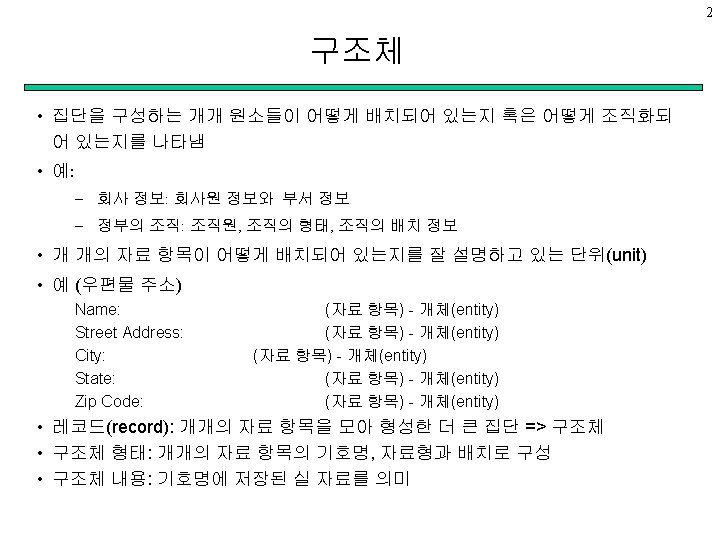
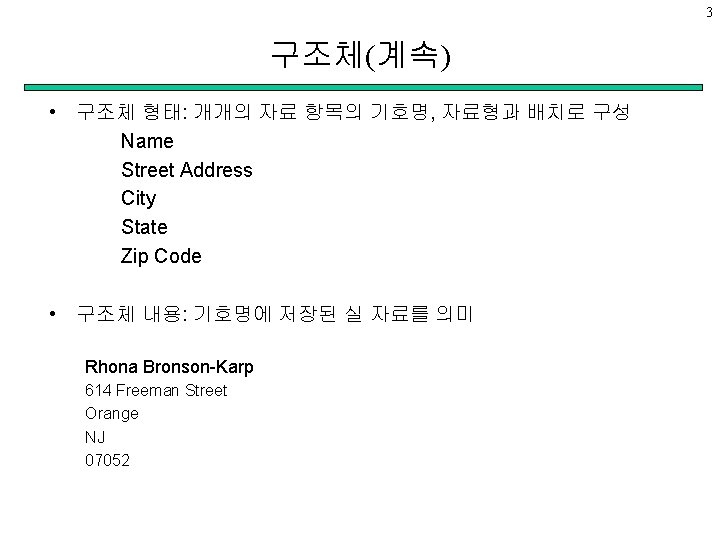
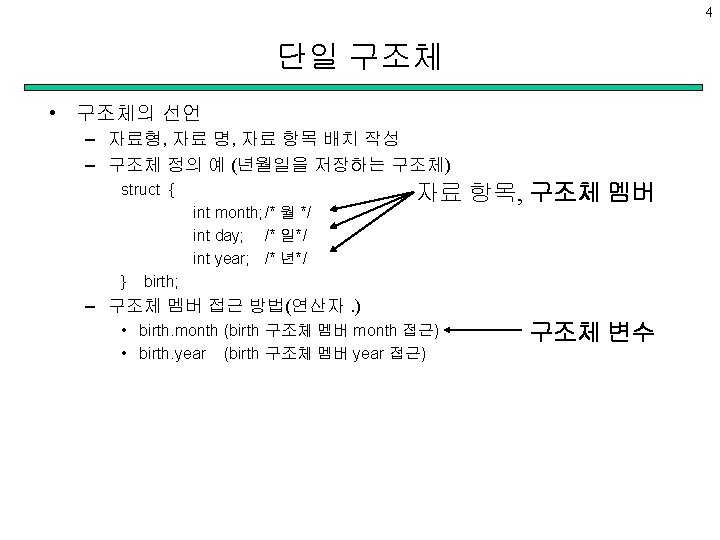
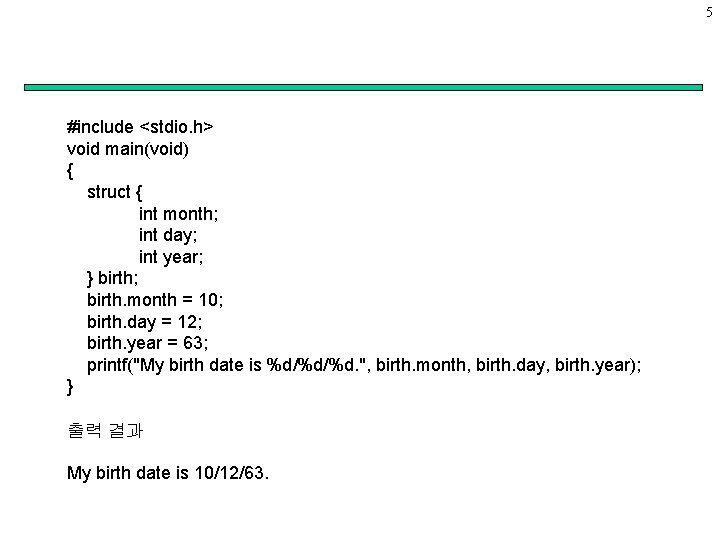
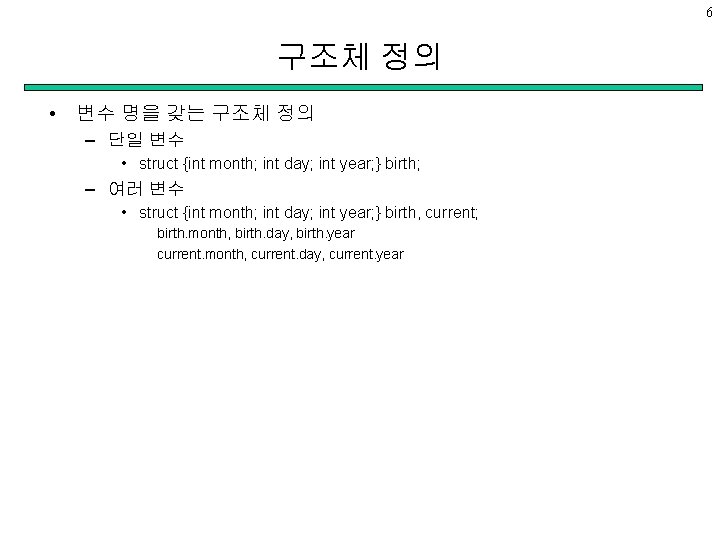
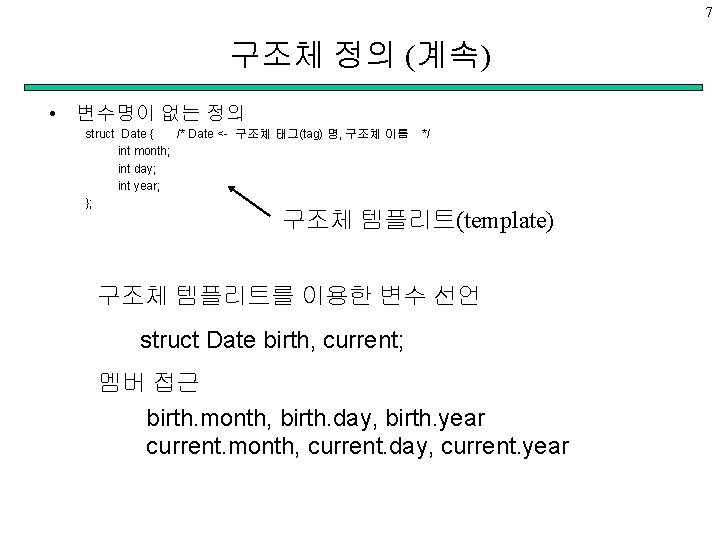
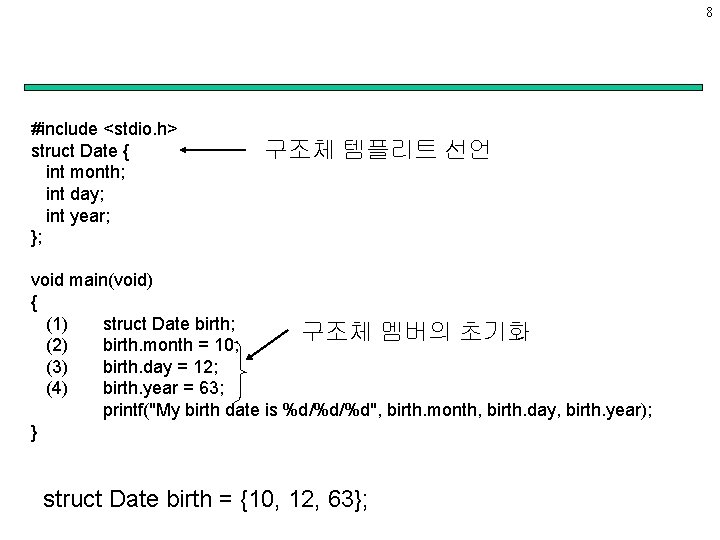
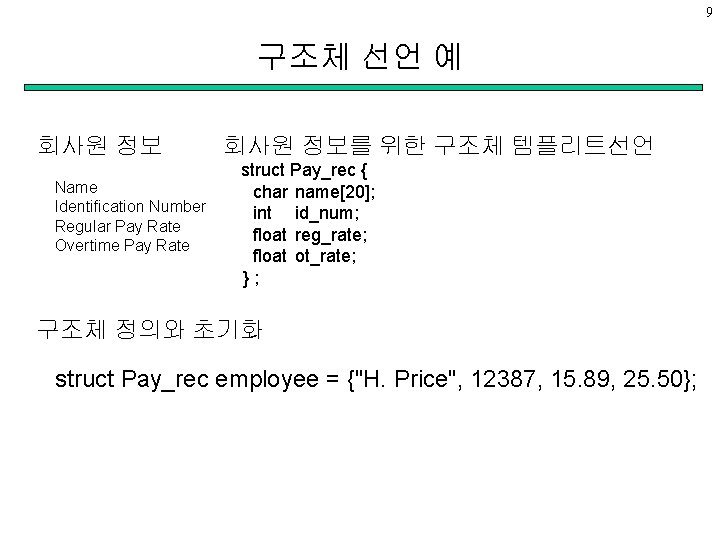
![10 • 사람의 생년월일을 위한 구조체 – Date 구조체 사용 struct { char name[20]; 10 • 사람의 생년월일을 위한 구조체 – Date 구조체 사용 struct { char name[20];](https://slidetodoc.com/presentation_image_h2/5f17dc378b5fbcaca400df444a61a038/image-10.jpg)
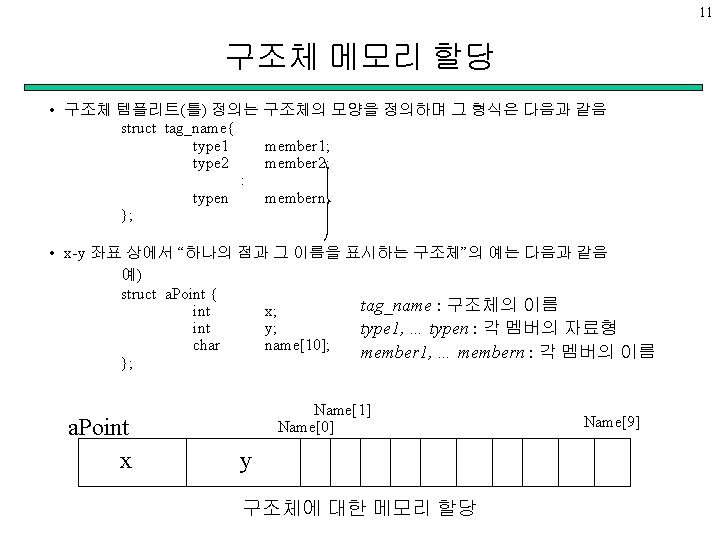
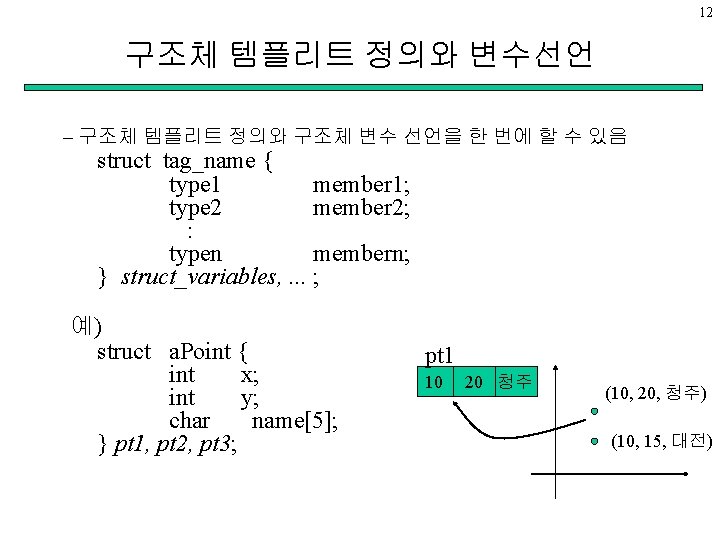
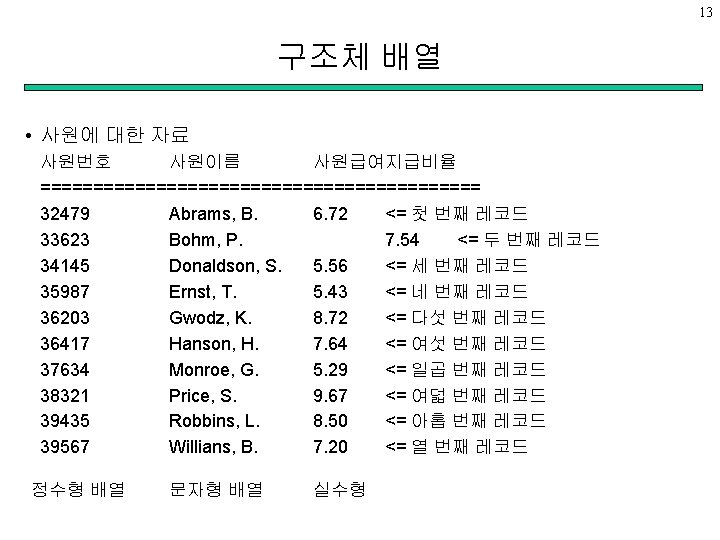
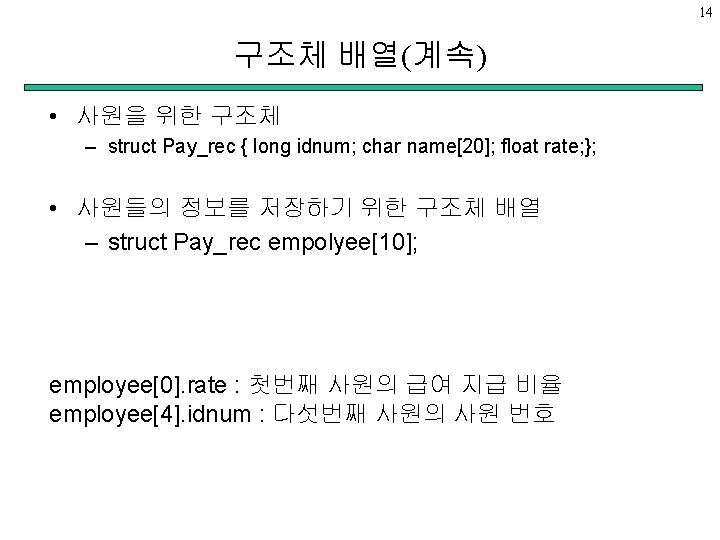
![15 #include <stdio. h> struct Pay_rec { long id; char name[20]; float rate; }; 15 #include <stdio. h> struct Pay_rec { long id; char name[20]; float rate; };](https://slidetodoc.com/presentation_image_h2/5f17dc378b5fbcaca400df444a61a038/image-15.jpg)
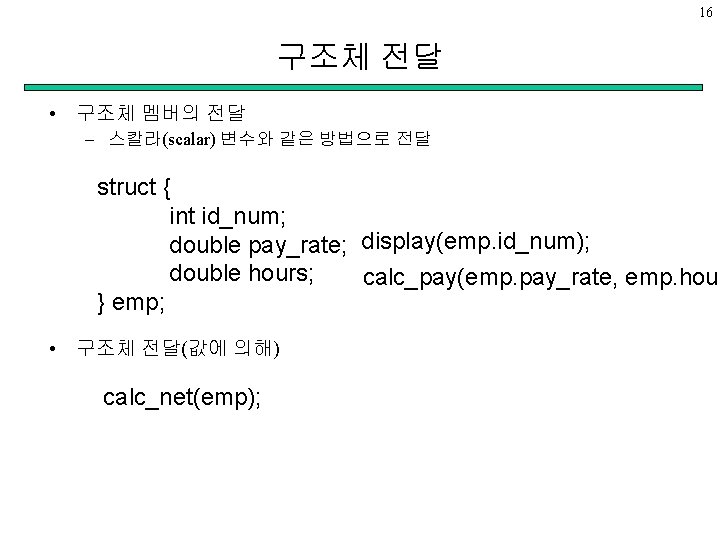
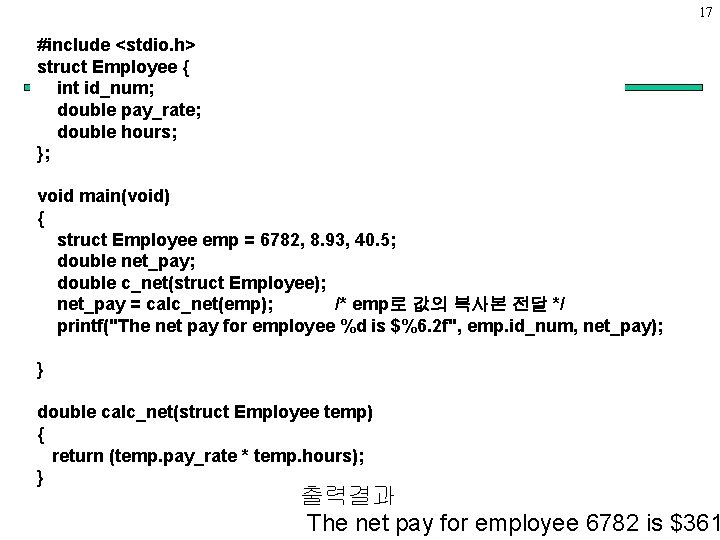
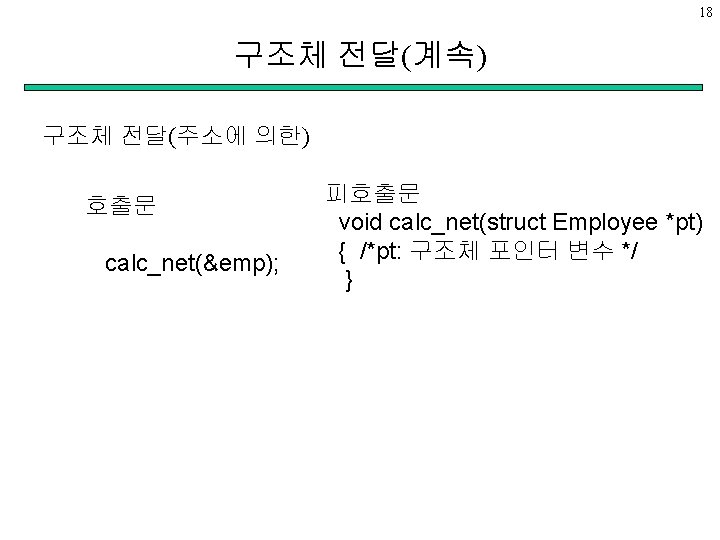
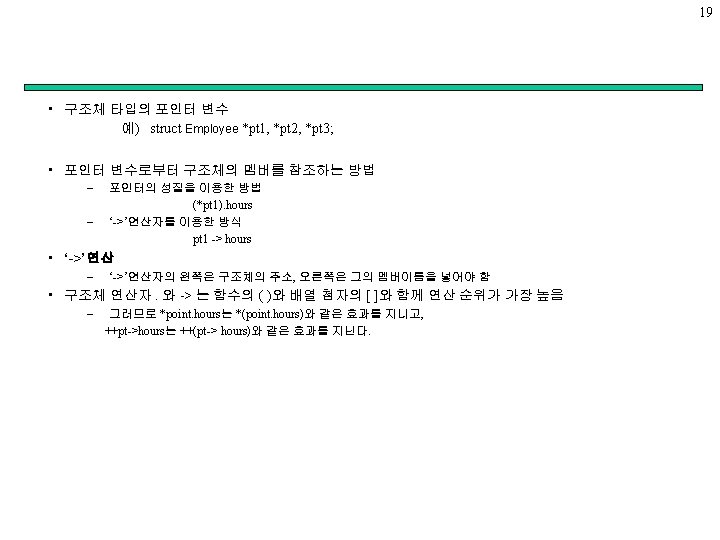
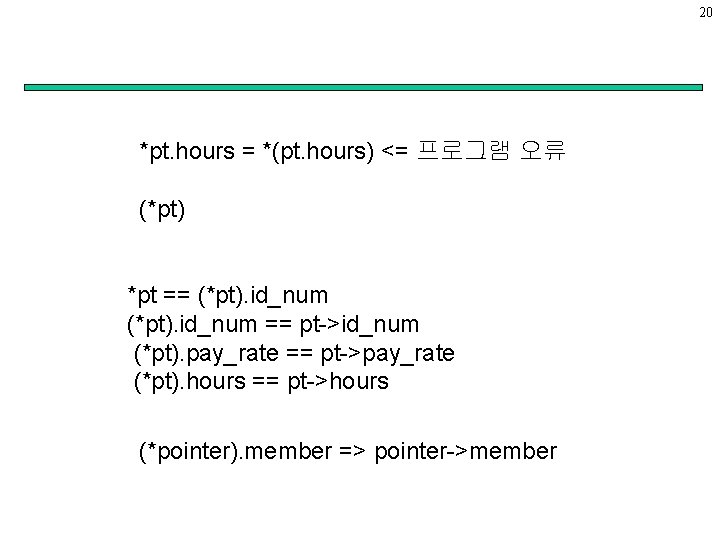
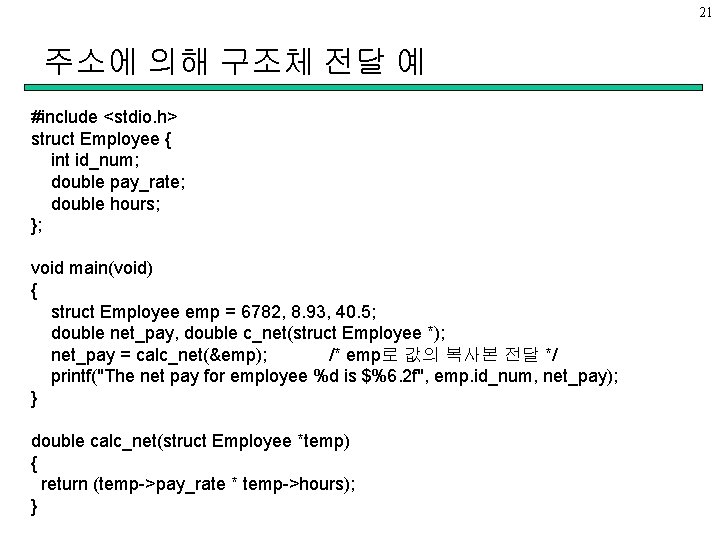
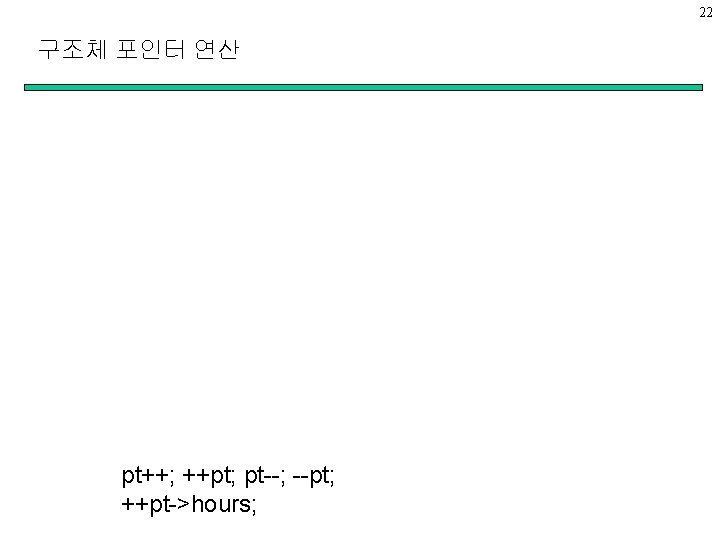
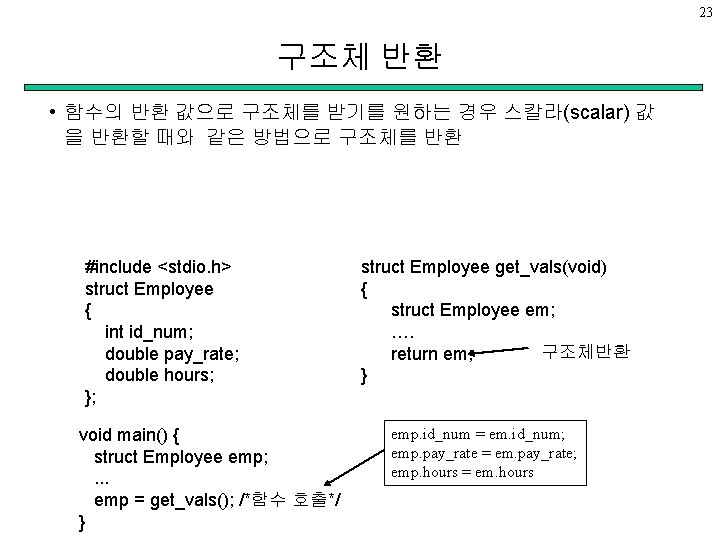
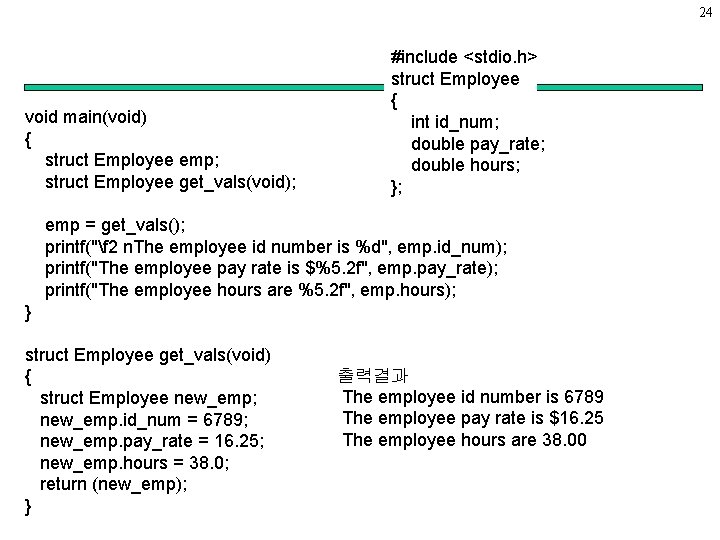
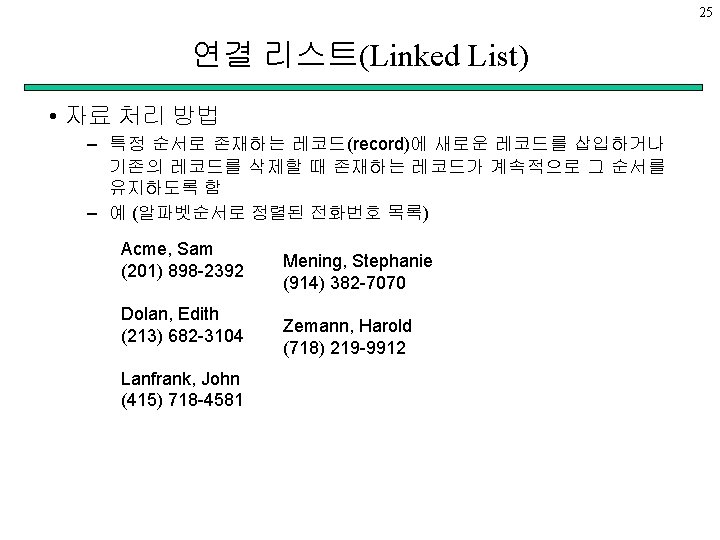
![26 연결리스트(계속) • 배열 구조를 사용하는 경우 struct person { char name[20]; char telnum[20]; 26 연결리스트(계속) • 배열 구조를 사용하는 경우 struct person { char name[20]; char telnum[20];](https://slidetodoc.com/presentation_image_h2/5f17dc378b5fbcaca400df444a61a038/image-26.jpg)
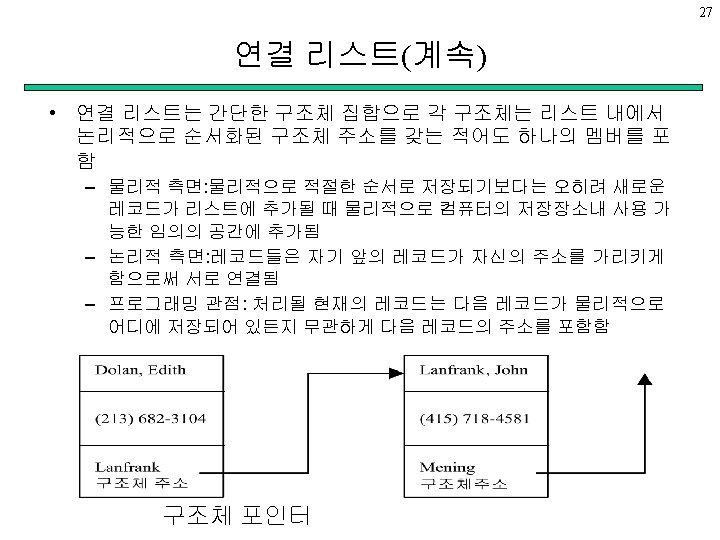
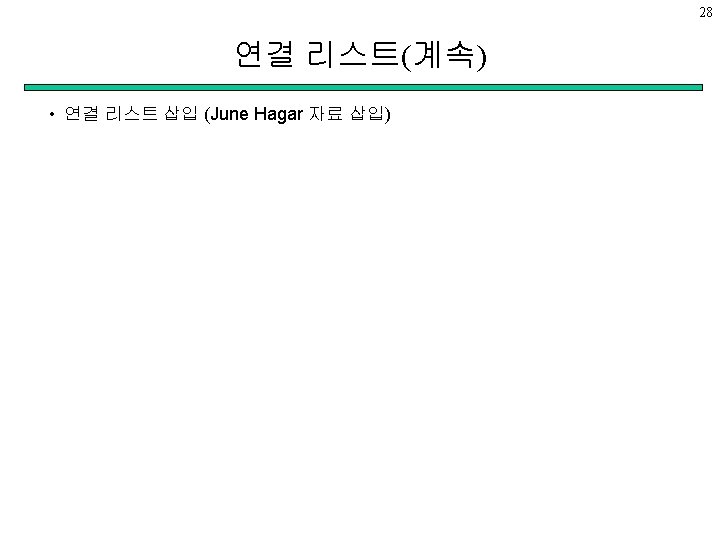
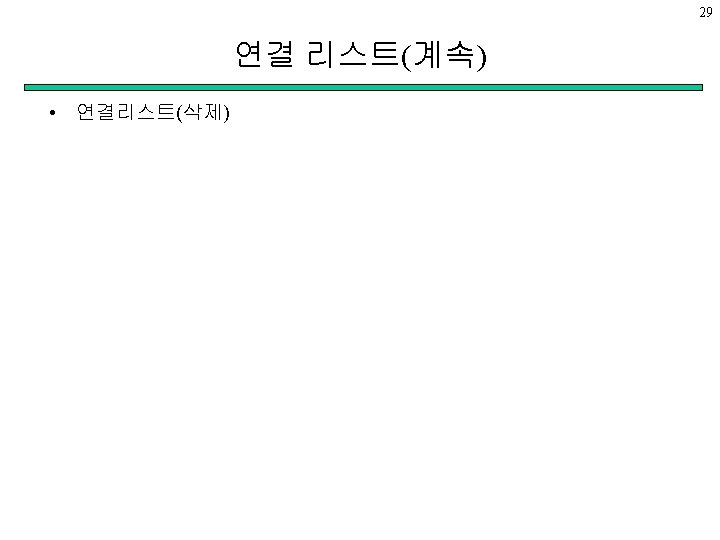
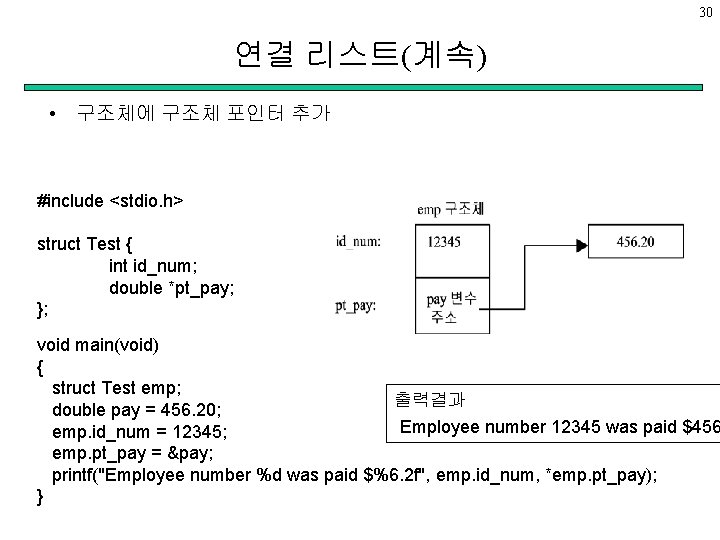
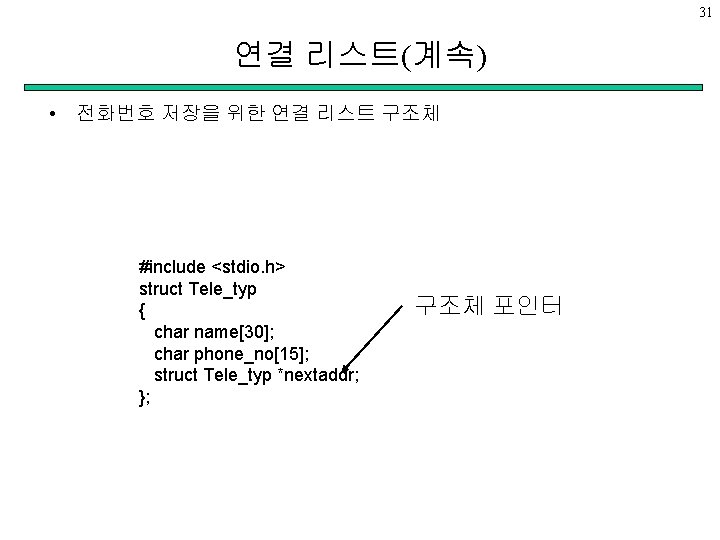
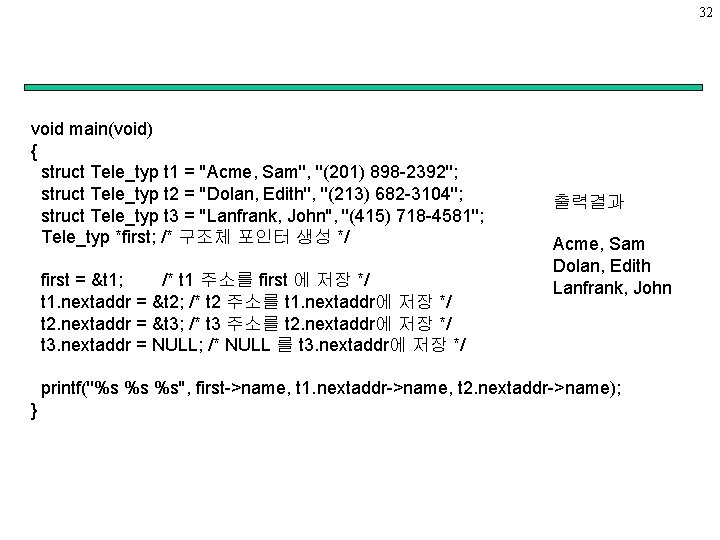
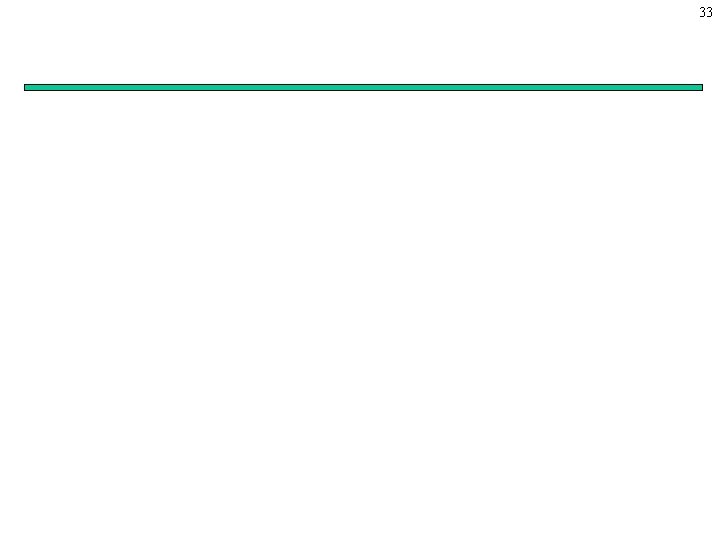
![34 구조체를 포인터로 전달 #include <stdio. h> struct Tele_typ { char name[30]; char phone_no[15]; 34 구조체를 포인터로 전달 #include <stdio. h> struct Tele_typ { char name[30]; char phone_no[15];](https://slidetodoc.com/presentation_image_h2/5f17dc378b5fbcaca400df444a61a038/image-34.jpg)
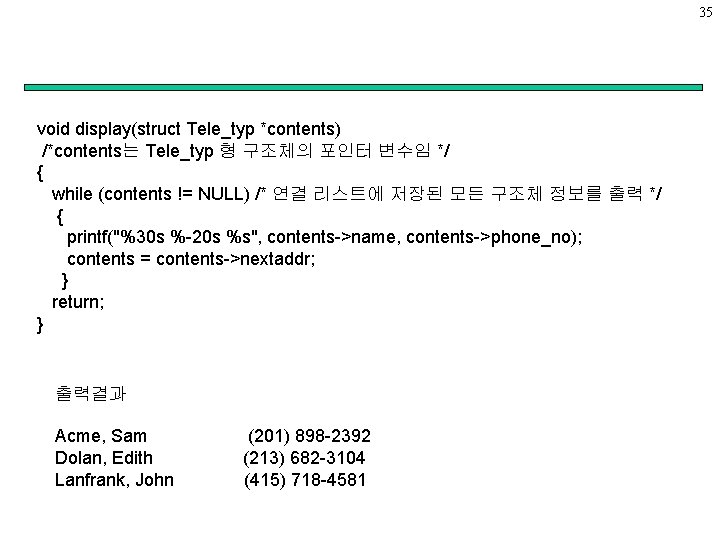
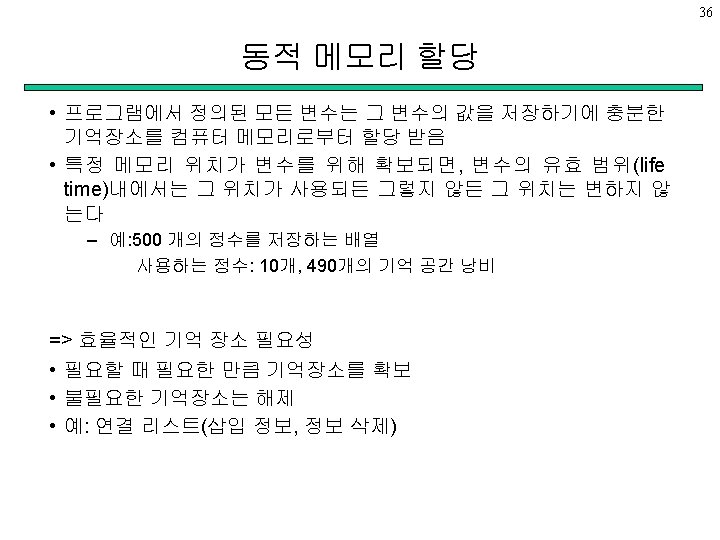
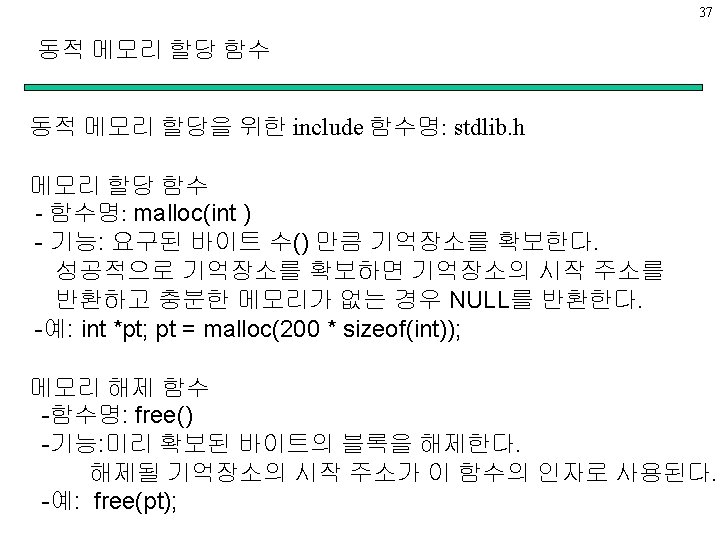
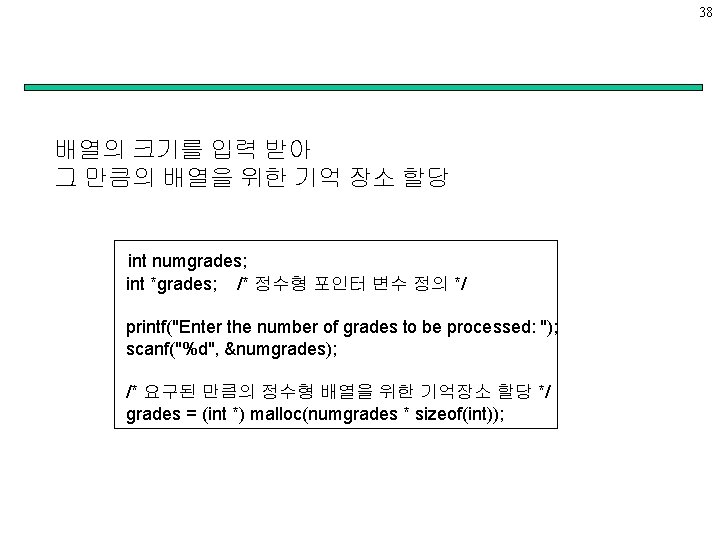
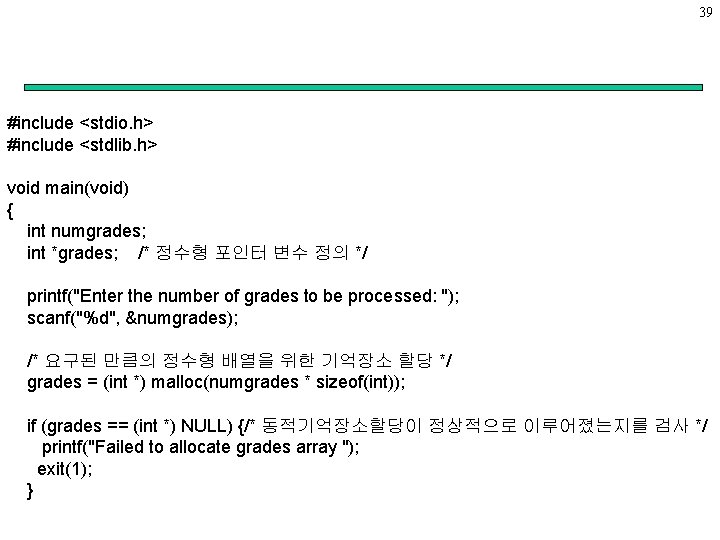
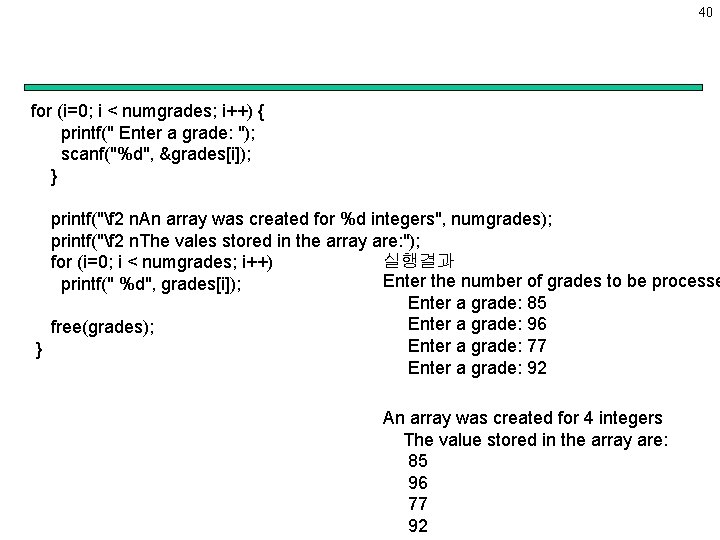
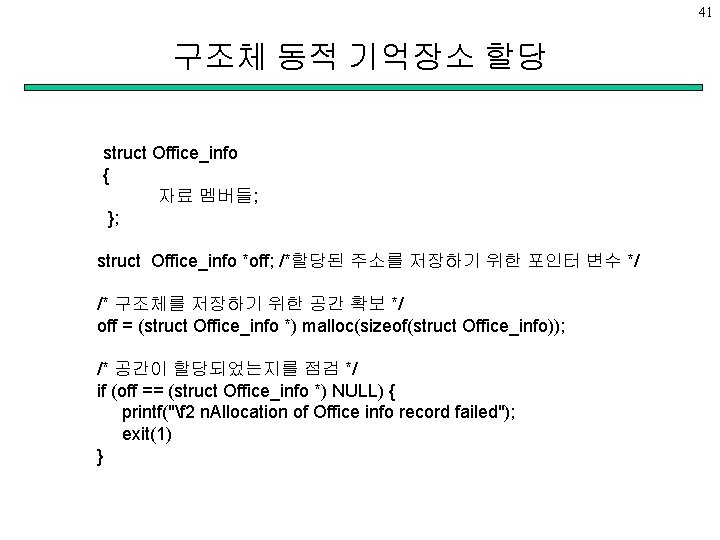
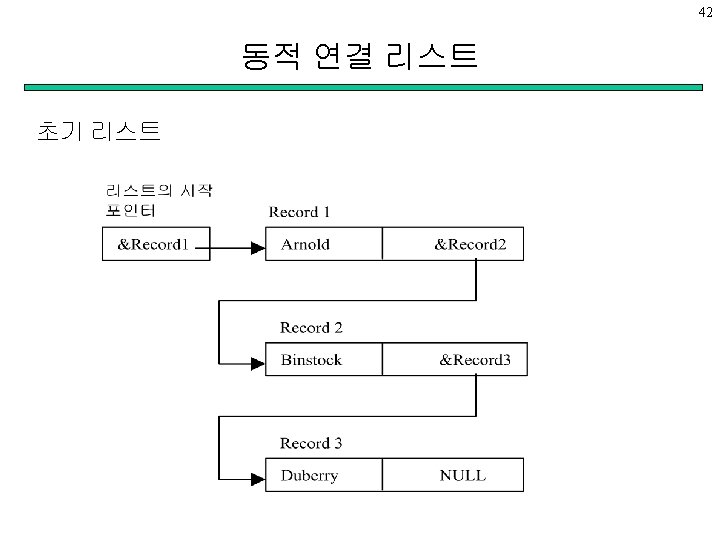
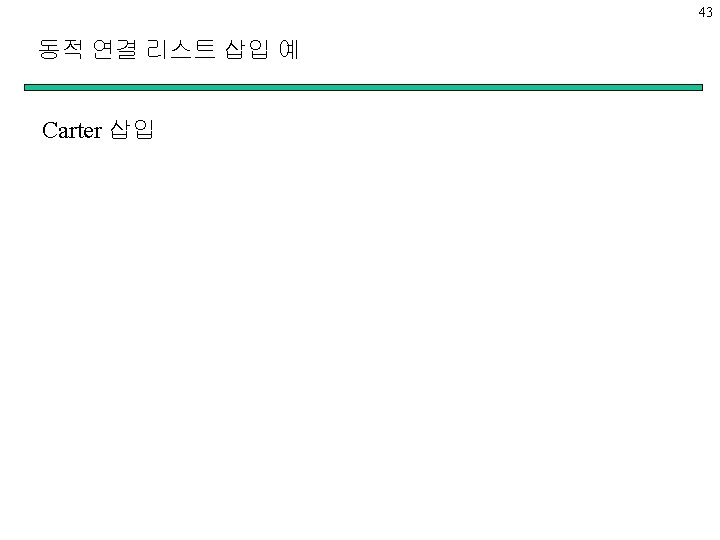
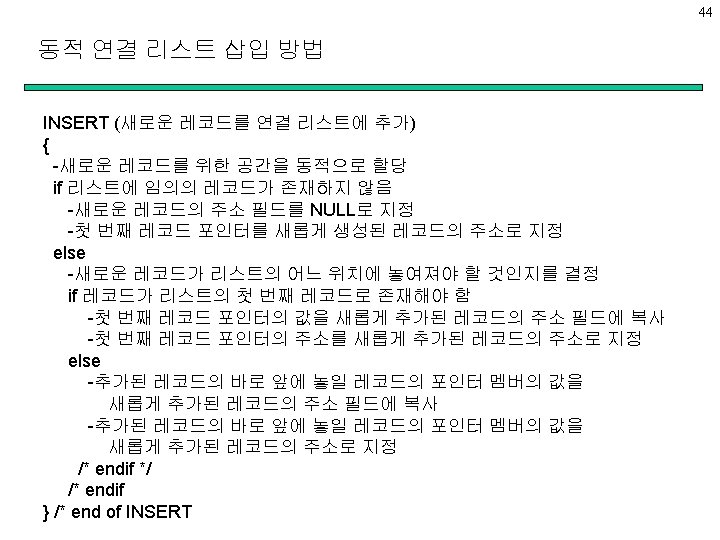
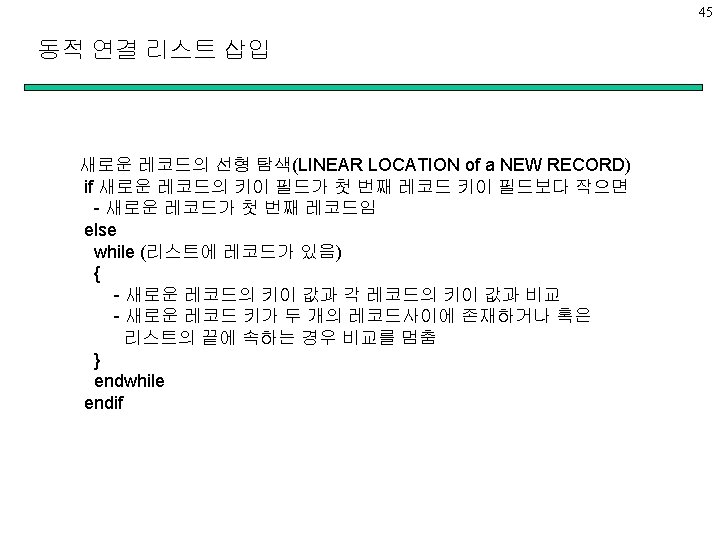
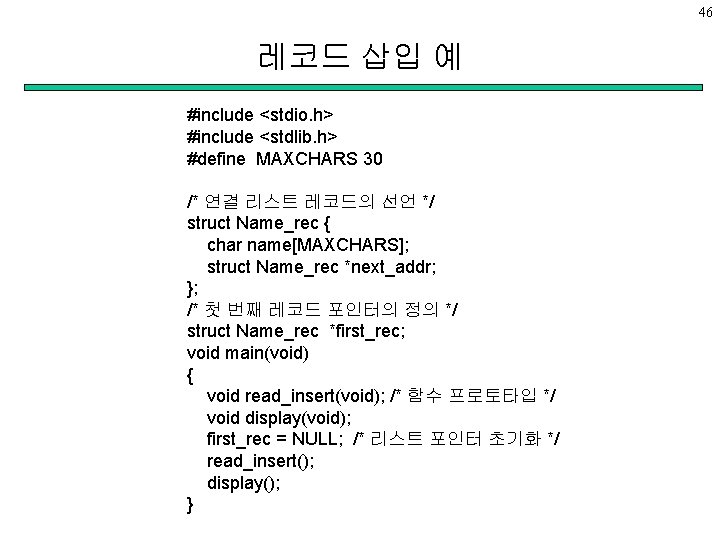
![47 /* 이름을 입력받아 이를 연결 리스트에 삽입 */ void read_insert(void) { char name[MAXCHARS]; 47 /* 이름을 입력받아 이를 연결 리스트에 삽입 */ void read_insert(void) { char name[MAXCHARS];](https://slidetodoc.com/presentation_image_h2/5f17dc378b5fbcaca400df444a61a038/image-47.jpg)
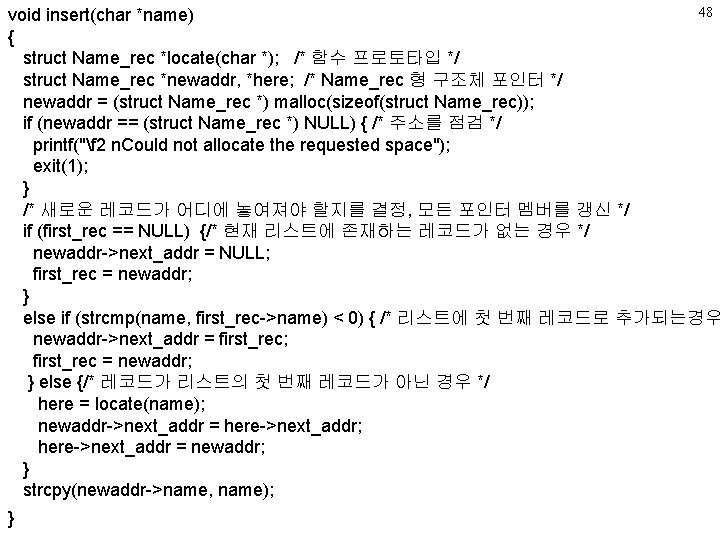
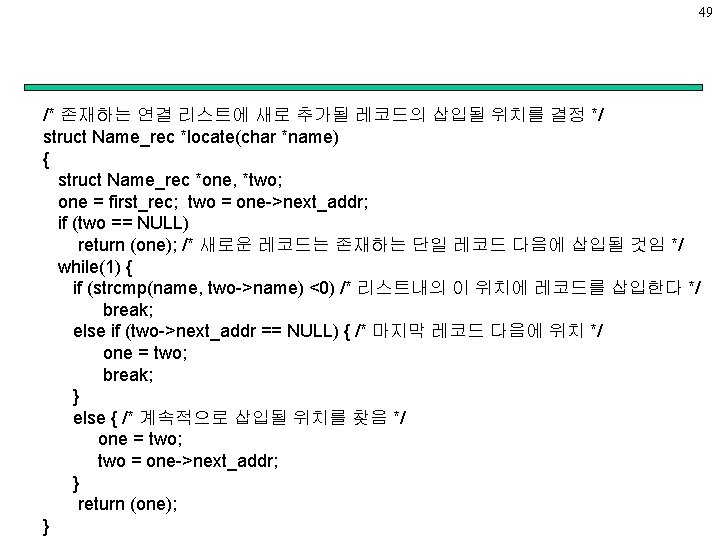
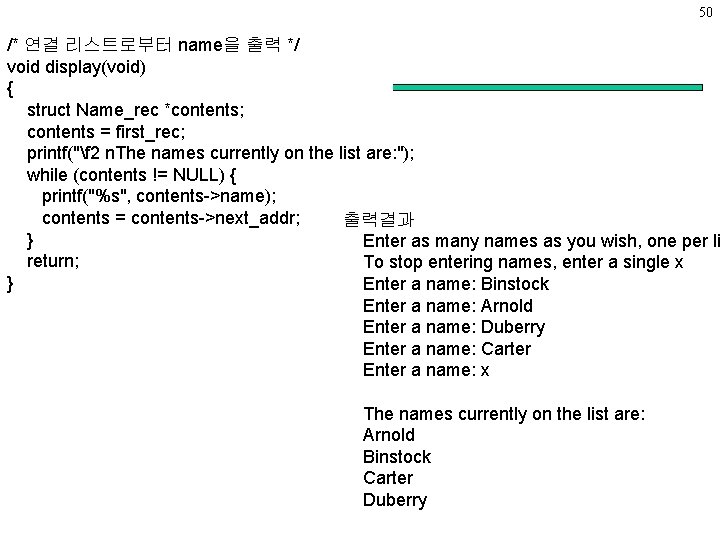
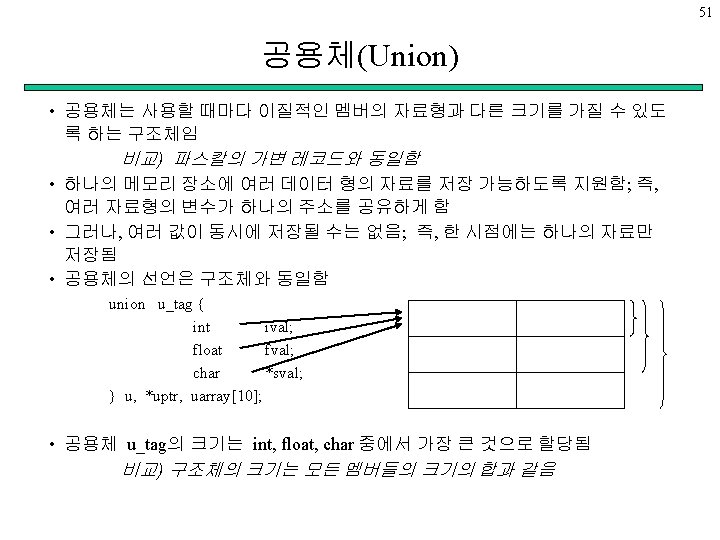
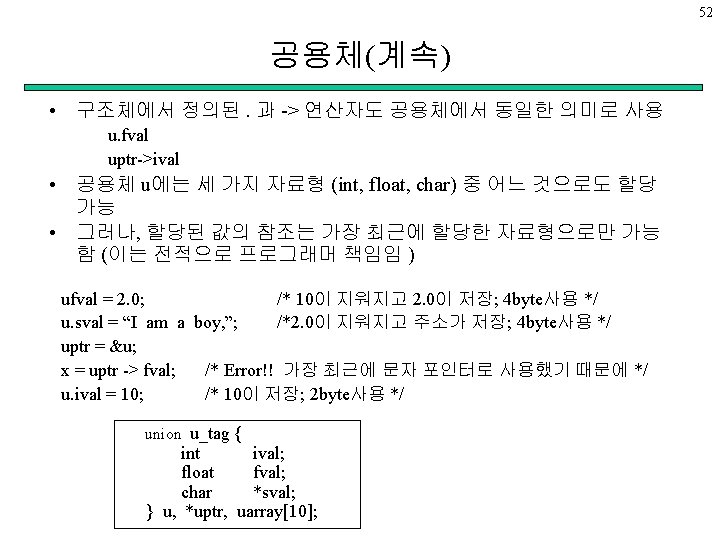
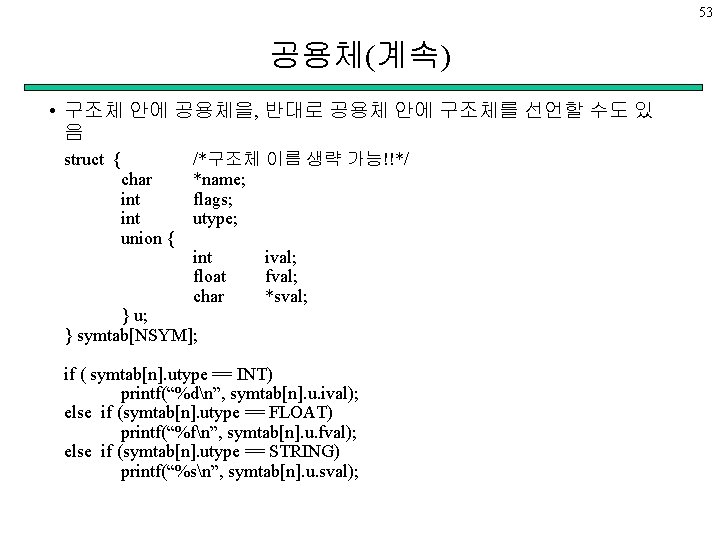
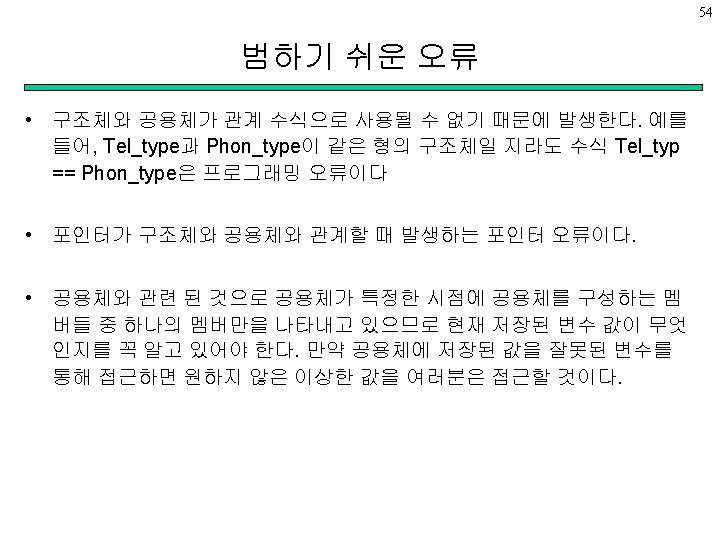
- Slides: 54
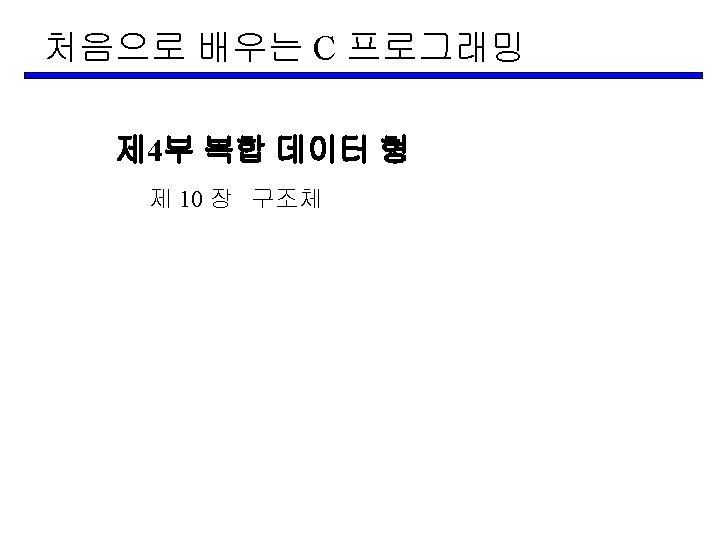
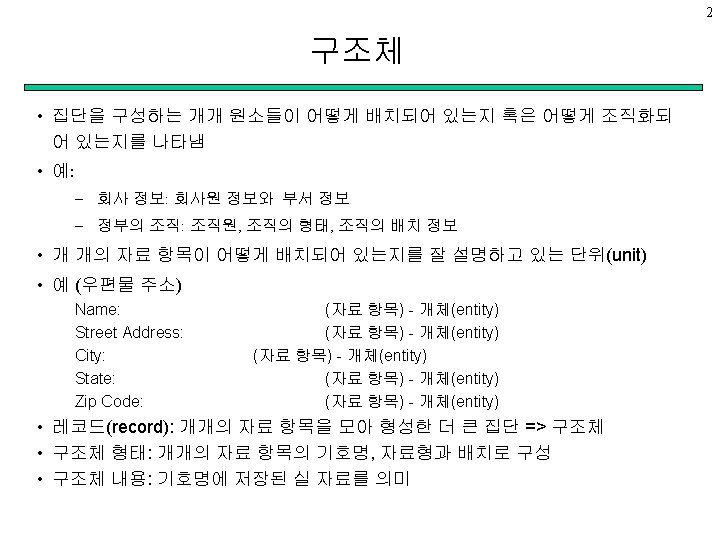
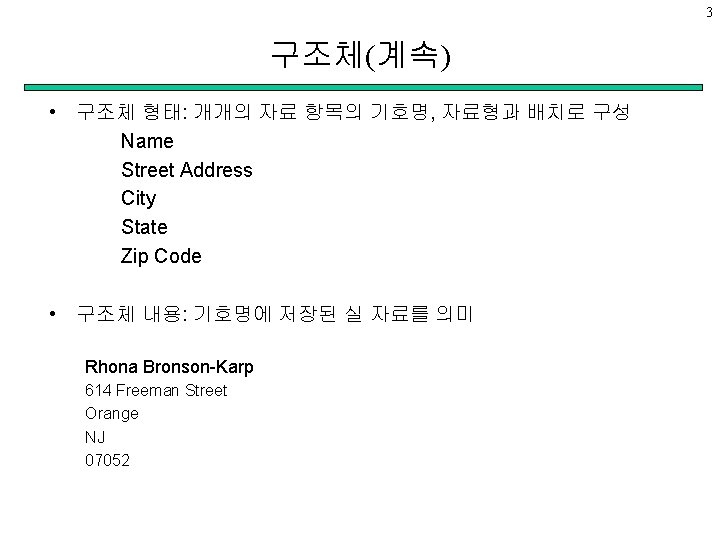
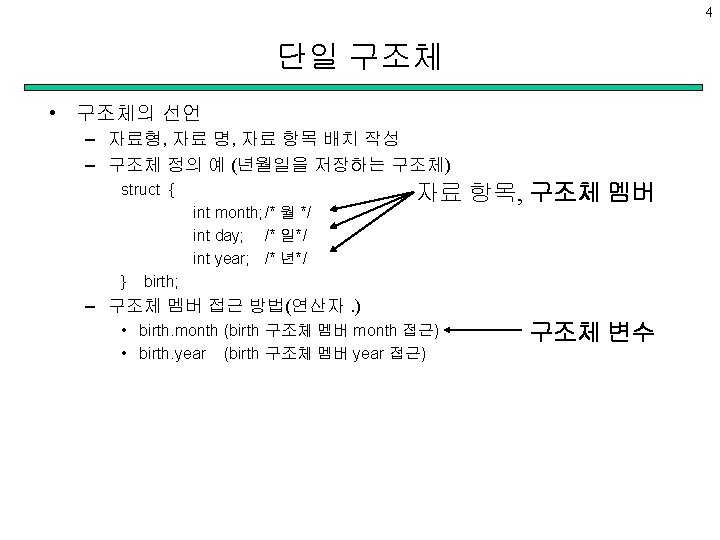
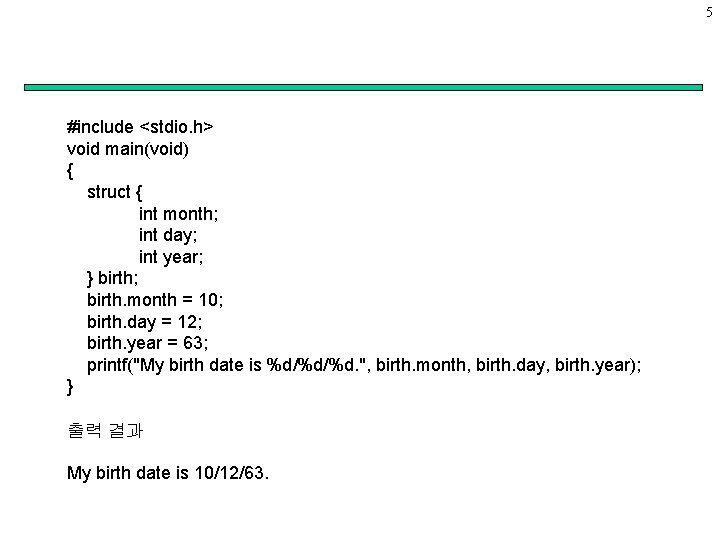
5 #include <stdio. h> void main(void) { struct { int month; int day; int year; } birth; birth. month = 10; birth. day = 12; birth. year = 63; printf("My birth date is %d/%d/%d. ", birth. month, birth. day, birth. year); } 출력 결과 My birth date is 10/12/63.
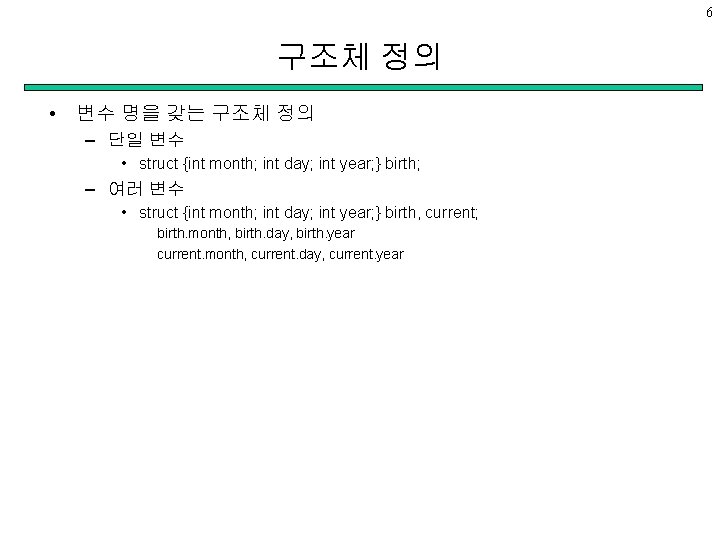
6 구조체 정의 • 변수 명을 갖는 구조체 정의 – 단일 변수 • struct {int month; int day; int year; } birth; – 여러 변수 • struct {int month; int day; int year; } birth, current; birth. month, birth. day, birth. year current. month, current. day, current. year
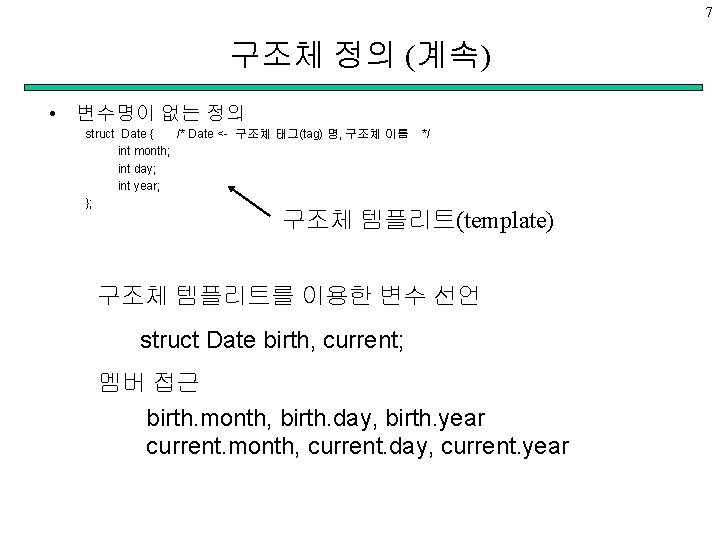
7 구조체 정의 (계속) • 변수명이 없는 정의 struct Date { /* Date <- 구조체 태그(tag) 명, 구조체 이름 int month; int day; int year; }; */ 구조체 템플리트(template) 구조체 템플리트를 이용한 변수 선언 struct Date birth, current; 멤버 접근 birth. month, birth. day, birth. year current. month, current. day, current. year
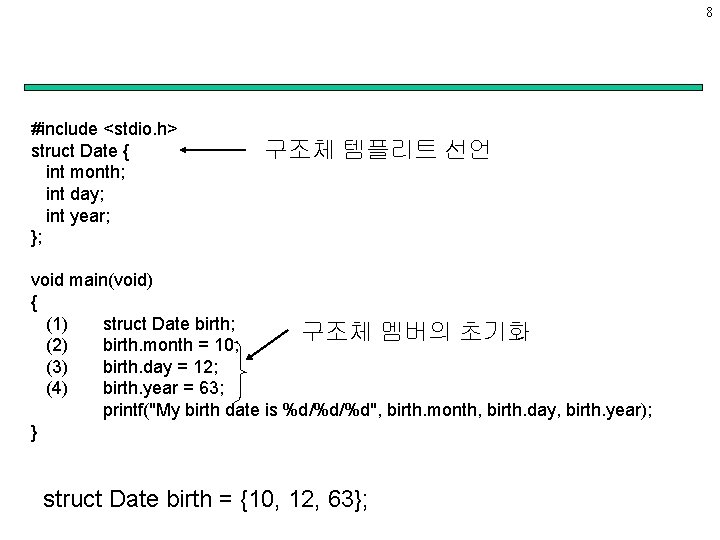
8 #include <stdio. h> struct Date { int month; int day; int year; }; 구조체 템플리트 선언 void main(void) { (1) struct Date birth; 구조체 멤버의 초기화 (2) birth. month = 10; (3) birth. day = 12; (4) birth. year = 63; printf("My birth date is %d/%d/%d", birth. month, birth. day, birth. year); } struct Date birth = {10, 12, 63};
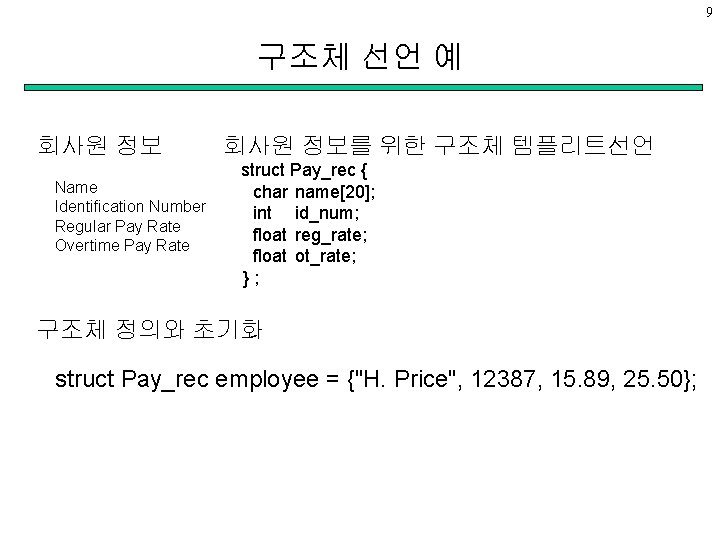
9 구조체 선언 예 회사원 정보 Name Identification Number Regular Pay Rate Overtime Pay Rate 회사원 정보를 위한 구조체 템플리트선언 struct Pay_rec { char name[20]; int id_num; float reg_rate; float ot_rate; }; 구조체 정의와 초기화 struct Pay_rec employee = {"H. Price", 12387, 15. 89, 25. 50};
![10 사람의 생년월일을 위한 구조체 Date 구조체 사용 struct char name20 10 • 사람의 생년월일을 위한 구조체 – Date 구조체 사용 struct { char name[20];](https://slidetodoc.com/presentation_image_h2/5f17dc378b5fbcaca400df444a61a038/image-10.jpg)
10 • 사람의 생년월일을 위한 구조체 – Date 구조체 사용 struct { char name[20]; struct Date birth; } person; • 멤버 접근 방법 – – person. name person. birth. month person. birth. day person. birth. year /* 이름을 저장하는 변수 */ /* 생년월일을 저장하는 변수 */
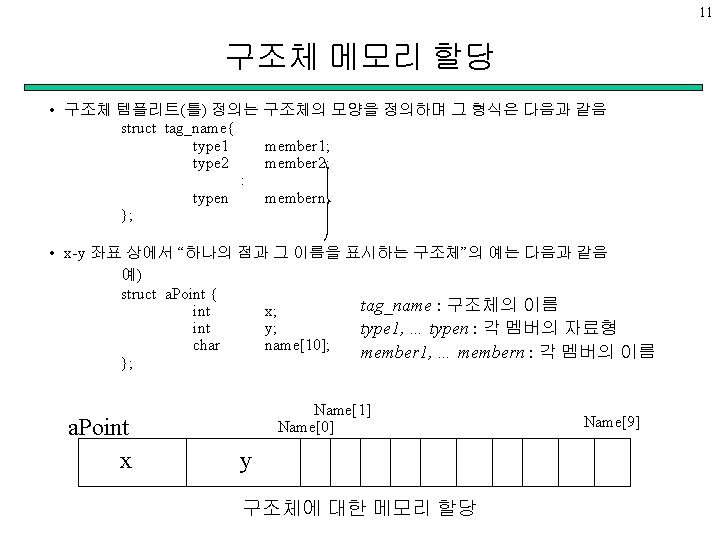
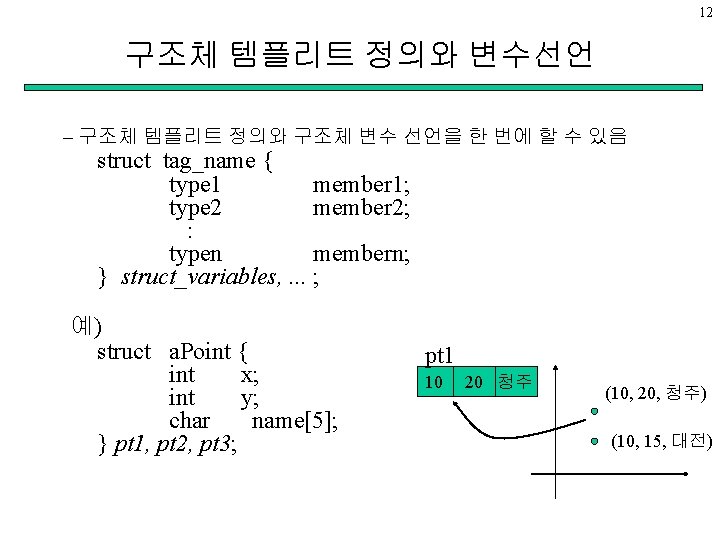
12 구조체 템플리트 정의와 변수선언 – 구조체 템플리트 정의와 구조체 변수 선언을 한 번에 할 수 있음 struct tag_name { type 1 member 1; type 2 member 2; : typen membern; } struct_variables, . . . ; 예) struct a. Point { int x; int y; char name[5]; } pt 1, pt 2, pt 3; pt 1 10 20 청주 (10, 20, 청주) (10, 15, 대전)
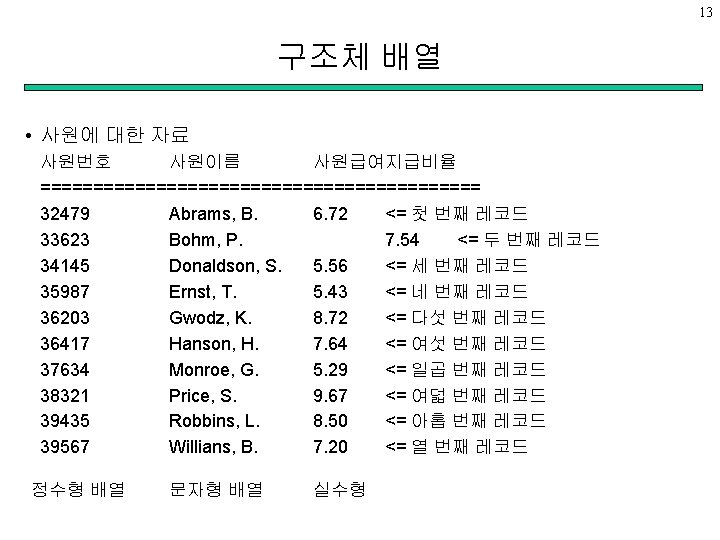
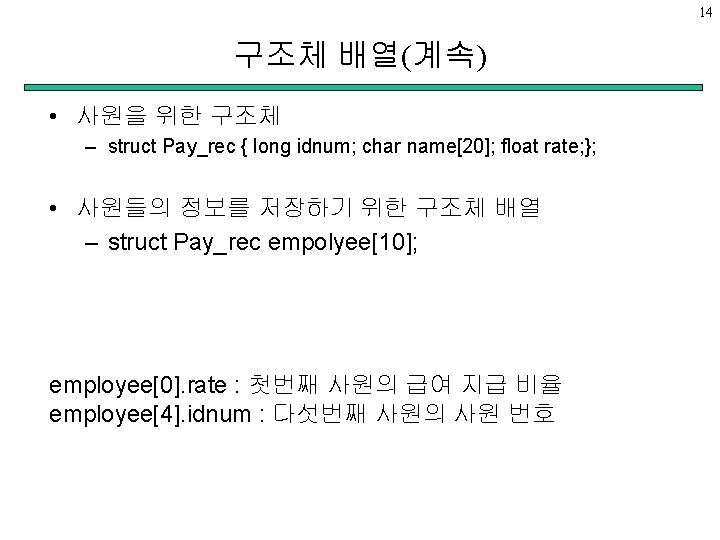
![15 include stdio h struct Payrec long id char name20 float rate 15 #include <stdio. h> struct Pay_rec { long id; char name[20]; float rate; };](https://slidetodoc.com/presentation_image_h2/5f17dc378b5fbcaca400df444a61a038/image-15.jpg)
15 #include <stdio. h> struct Pay_rec { long id; char name[20]; float rate; }; /* 전역 템플리트 구성 */ 출력결과 void main(void) 32479 { 33623 int i; 34145 struct Pay_rec employee[10] = { 35987 32479, "Abrams, B. ", 6. 72, 36203 33623, "Bohm, P. ", 7. 54, 34145, "Donaldson, S. ", 5. 56, 35987, "Ernst, T. ", 5. 43, 36203, "Gwodz, K. ", 8. 72, 36417, "Hanson, H. ", 7. 64, 37634, "Monroe, G. ", 5. 29, 38321, "Price, S. ", 9. 67, 39435, "Robbins, L. ", 8. 50, 39567, "Willians, B. ", 7. 20 }; for (i=0; i<5; i++) printf("f 2 nld %-20 s %4. 2 f", employee[i]. id, employee[i]. name, employee[i]. rate); } /* end of main */ Abrams, B. Bohm, P. Donaldson, S. Ernst, T. Gwodz, K. 6. 72 7. 54 5. 56 5. 43 8. 72
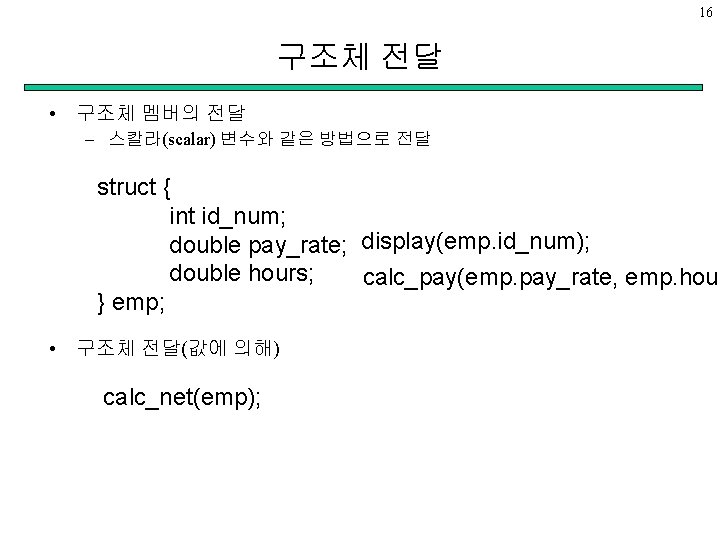
16 구조체 전달 • 구조체 멤버의 전달 – 스칼라(scalar) 변수와 같은 방법으로 전달 struct { int id_num; double pay_rate; display(emp. id_num); double hours; calc_pay(emp. pay_rate, emp. hour } emp; • 구조체 전달(값에 의해) calc_net(emp);
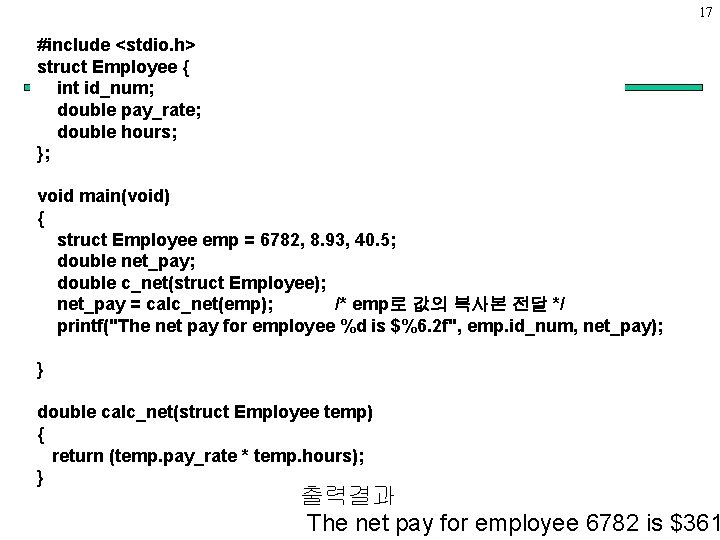
17 #include <stdio. h> struct Employee { int id_num; double pay_rate; double hours; }; void main(void) { struct Employee emp = 6782, 8. 93, 40. 5; double net_pay; double c_net(struct Employee); net_pay = calc_net(emp); /* emp로 값의 복사본 전달 */ printf("The net pay for employee %d is $%6. 2 f", emp. id_num, net_pay); } double calc_net(struct Employee temp) { return (temp. pay_rate * temp. hours); } 출력결과 The net pay for employee 6782 is $361
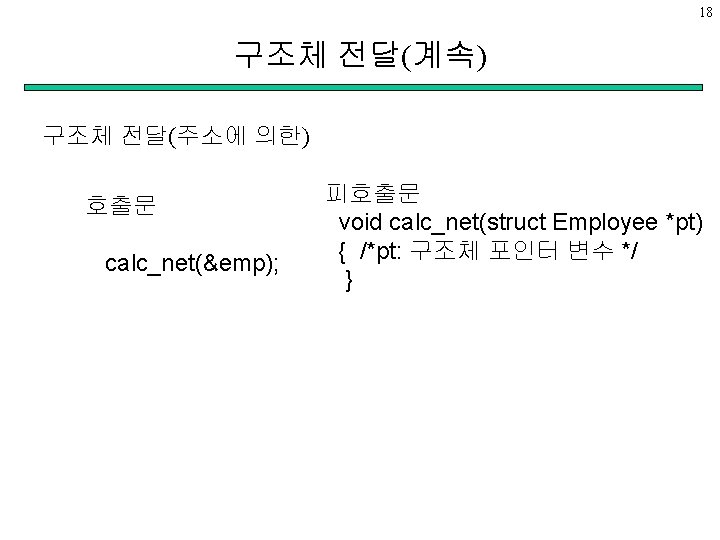
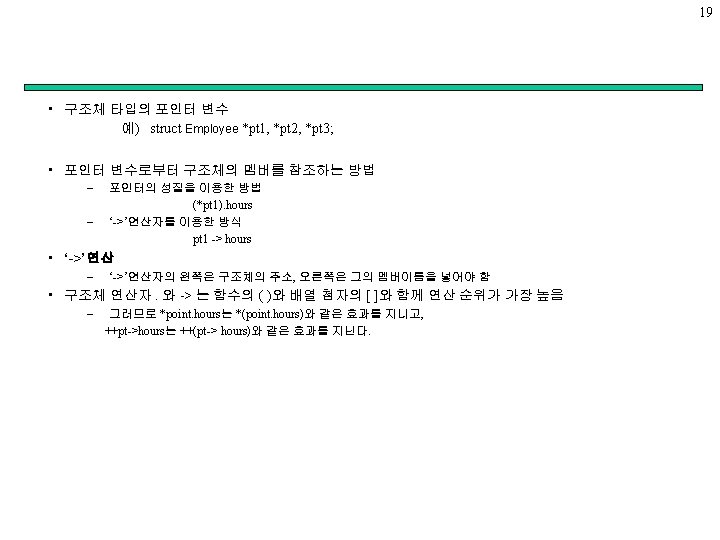
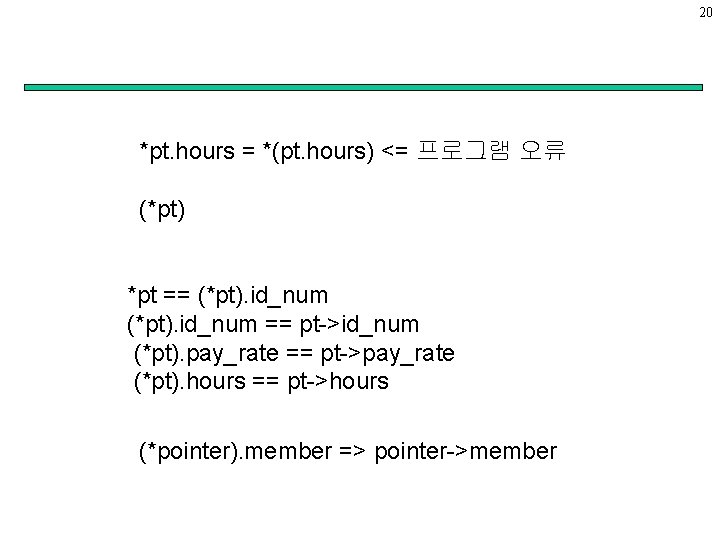
20 *pt. hours = *(pt. hours) <= 프로그램 오류 (*pt) *pt == (*pt). id_num == pt->id_num (*pt). pay_rate == pt->pay_rate (*pt). hours == pt->hours (*pointer). member => pointer->member
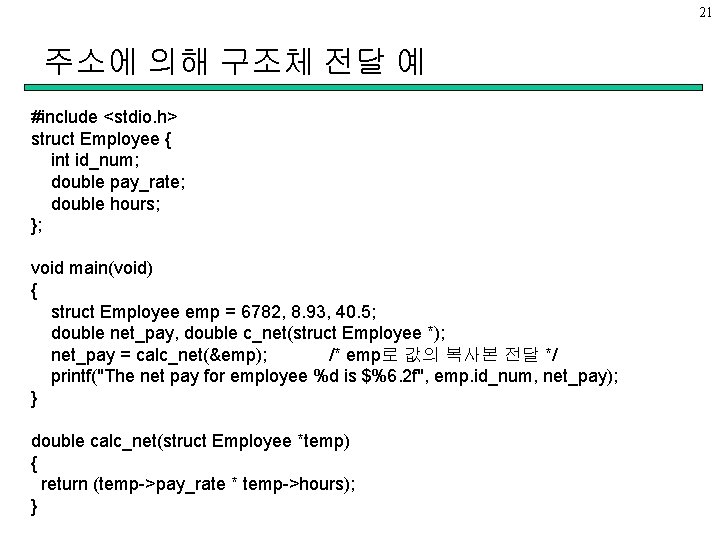
21 주소에 의해 구조체 전달 예 #include <stdio. h> struct Employee { int id_num; double pay_rate; double hours; }; void main(void) { struct Employee emp = 6782, 8. 93, 40. 5; double net_pay, double c_net(struct Employee *); net_pay = calc_net(&emp); /* emp로 값의 복사본 전달 */ printf("The net pay for employee %d is $%6. 2 f", emp. id_num, net_pay); } double calc_net(struct Employee *temp) { return (temp->pay_rate * temp->hours); }
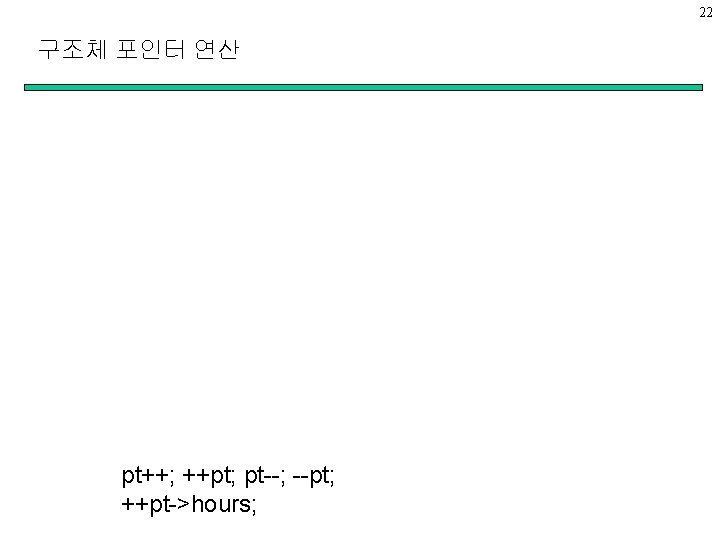
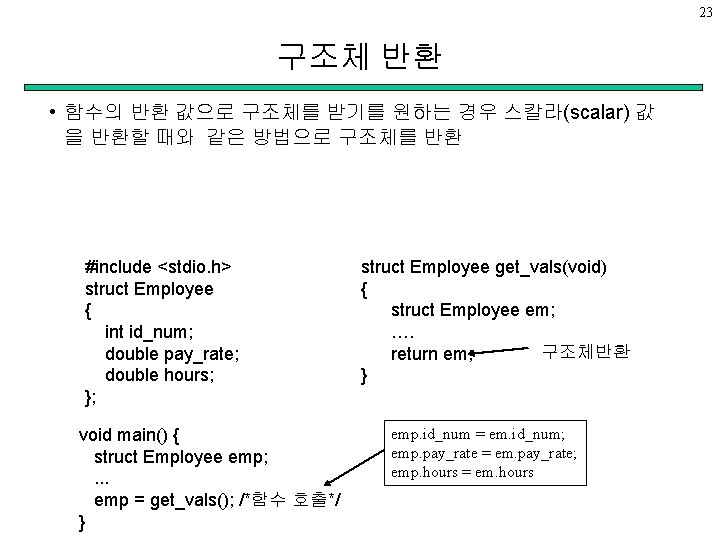
23 구조체 반환 • 함수의 반환 값으로 구조체를 받기를 원하는 경우 스칼라(scalar) 값 을 반환할 때와 같은 방법으로 구조체를 반환 #include <stdio. h> struct Employee { int id_num; double pay_rate; double hours; }; void main() { struct Employee emp; . . . emp = get_vals(); /*함수 호출*/ } struct Employee get_vals(void) { struct Employee em; …. 구조체반환 return em; } emp. id_num = em. id_num; emp. pay_rate = em. pay_rate; emp. hours = em. hours
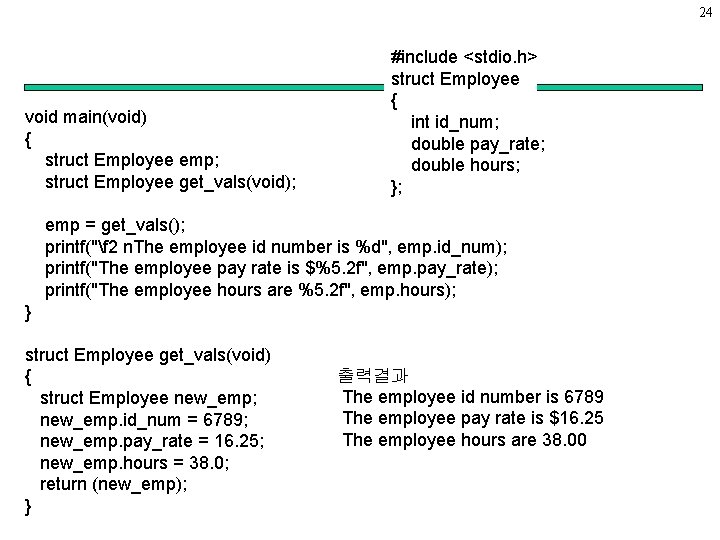
24 void main(void) { struct Employee emp; struct Employee get_vals(void); #include <stdio. h> struct Employee { int id_num; double pay_rate; double hours; }; emp = get_vals(); printf("f 2 n. The employee id number is %d", emp. id_num); printf("The employee pay rate is $%5. 2 f", emp. pay_rate); printf("The employee hours are %5. 2 f", emp. hours); } struct Employee get_vals(void) { struct Employee new_emp; new_emp. id_num = 6789; new_emp. pay_rate = 16. 25; new_emp. hours = 38. 0; return (new_emp); } 출력결과 The employee id number is 6789 The employee pay rate is $16. 25 The employee hours are 38. 00
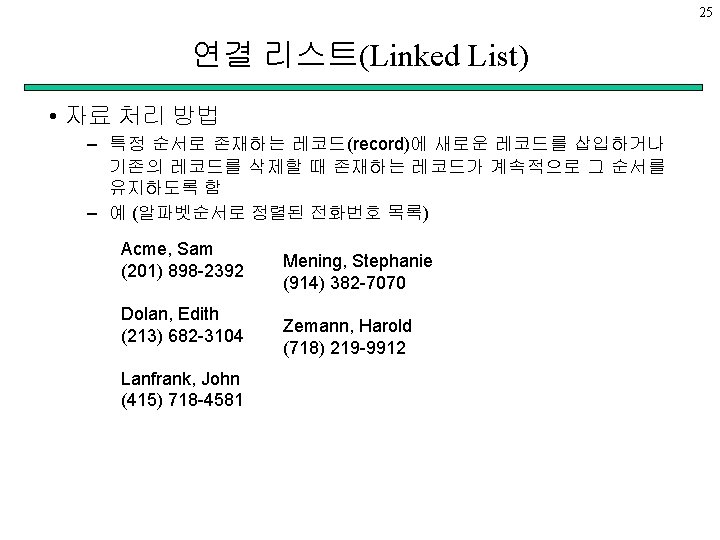
![26 연결리스트계속 배열 구조를 사용하는 경우 struct person char name20 char telnum20 26 연결리스트(계속) • 배열 구조를 사용하는 경우 struct person { char name[20]; char telnum[20];](https://slidetodoc.com/presentation_image_h2/5f17dc378b5fbcaca400df444a61a038/image-26.jpg)
26 연결리스트(계속) • 배열 구조를 사용하는 경우 struct person { char name[20]; char telnum[20]; }; 삽입과 삭제의 문제점 발생 struct person hb[10]; Acme, Sam Lanfrank, John Mening, Stephanie. Zemann, Harold Dolan, Edith (201) 898 -2392 (213) 682 -3104 (415) 718 -4581(914) 382 -7070 (718) 219 -9912 hb[0] hb[1] hb[2] hb[3] hb[4]
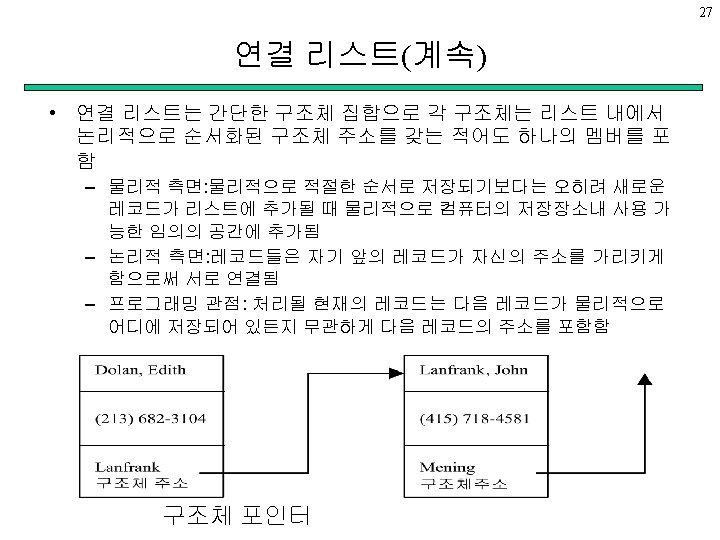
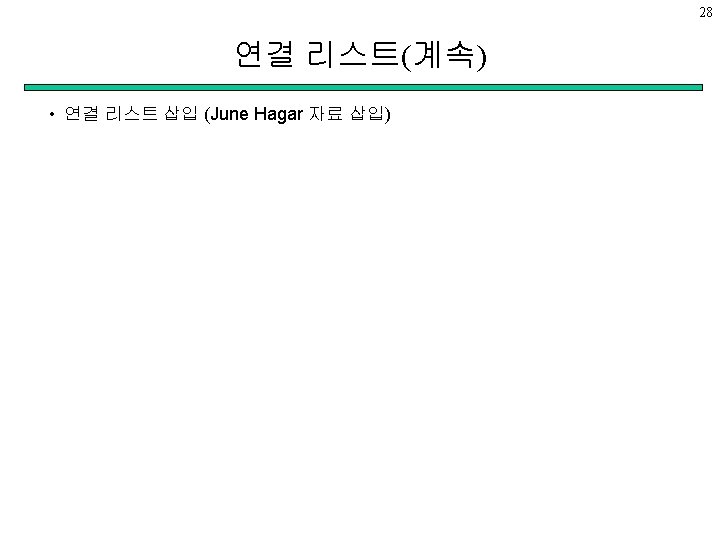
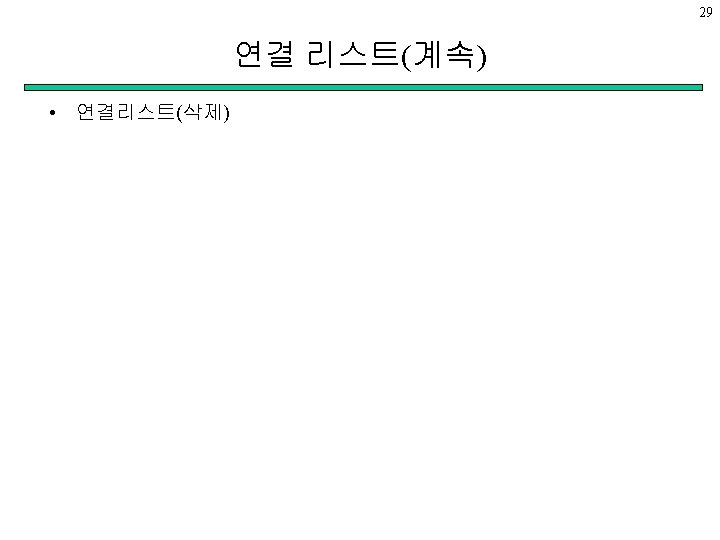
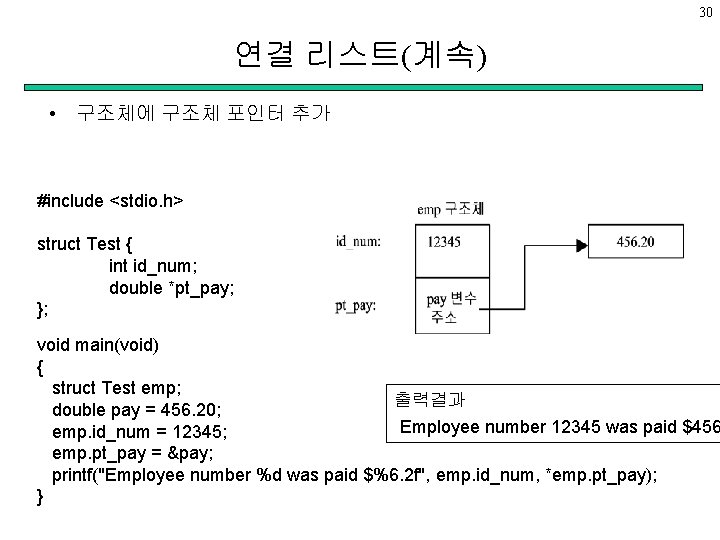
30 연결 리스트(계속) • 구조체에 구조체 포인터 추가 #include <stdio. h> struct Test { int id_num; double *pt_pay; }; void main(void) { struct Test emp; 출력결과 double pay = 456. 20; Employee number 12345 was paid $456 emp. id_num = 12345; emp. pt_pay = &pay; printf("Employee number %d was paid $%6. 2 f'', emp. id_num, *emp. pt_pay); }
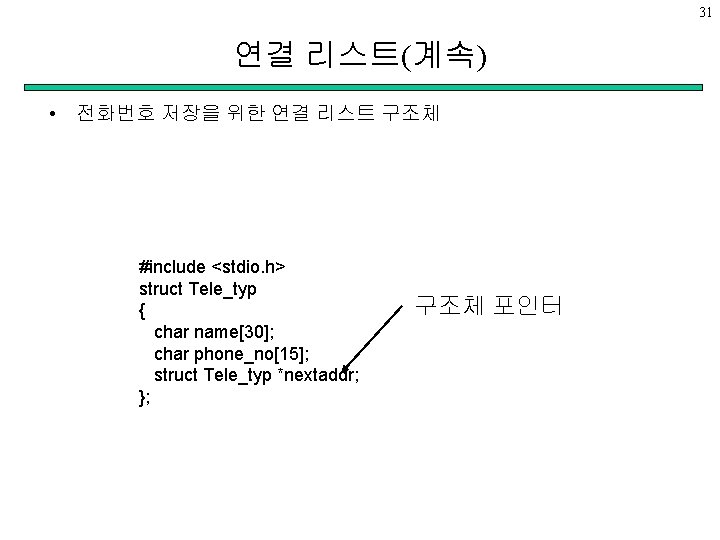
31 연결 리스트(계속) • 전화번호 저장을 위한 연결 리스트 구조체 #include <stdio. h> struct Tele_typ { char name[30]; char phone_no[15]; struct Tele_typ *nextaddr; }; 구조체 포인터
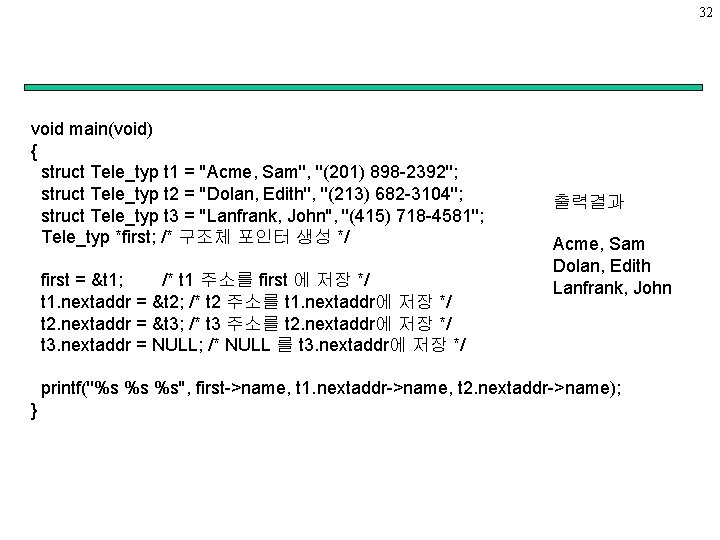
32 void main(void) { struct Tele_typ t 1 = ''Acme, Sam'', ''(201) 898 -2392''; struct Tele_typ t 2 = ''Dolan, Edith'', ''(213) 682 -3104''; struct Tele_typ t 3 = ''Lanfrank, John'', ''(415) 718 -4581''; Tele_typ *first; /* 구조체 포인터 생성 */ first = &t 1; /* t 1 주소를 first 에 저장 */ t 1. nextaddr = &t 2; /* t 2 주소를 t 1. nextaddr에 저장 */ t 2. nextaddr = &t 3; /* t 3 주소를 t 2. nextaddr에 저장 */ t 3. nextaddr = NULL; /* NULL 를 t 3. nextaddr에 저장 */ 출력결과 Acme, Sam Dolan, Edith Lanfrank, John printf(''%s %s %s'', first->name, t 1. nextaddr->name, t 2. nextaddr->name); }
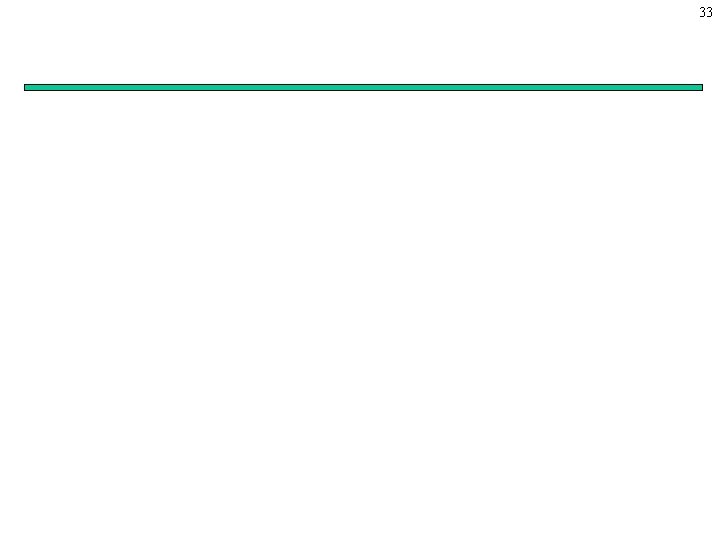
33
![34 구조체를 포인터로 전달 include stdio h struct Teletyp char name30 char phoneno15 34 구조체를 포인터로 전달 #include <stdio. h> struct Tele_typ { char name[30]; char phone_no[15];](https://slidetodoc.com/presentation_image_h2/5f17dc378b5fbcaca400df444a61a038/image-34.jpg)
34 구조체를 포인터로 전달 #include <stdio. h> struct Tele_typ { char name[30]; char phone_no[15]; struct Tele_typ *nextaddr; }; void main(void) { struct Tele_typ t 1 = "Acme, Sam", "(201) 898 -2392"; struct Tele_typ t 2 = "Dolan, Edith", "(213) 682 -3104"; struct Tele_typ t 3 = "Lanfrank, John", "(415) 718 -4581" Tele_typ *first; /* 구조체 포인터 생성 */ first = &t 1; /* t 1 주소를 first 에 저장 */ t 1. nextaddr = &t 2; /* t 2 주소를 t 1. nextaddr에 저장 */ t 2. nextaddr = &t 3; /* t 3 주소를 t 2. nextaddr에 저장 */ t 3. nextaddr = NULL; /* NULL 를 t 3. nextaddr에 저장 */ display(first); } /* 구조체 first의 주소를 전달 */
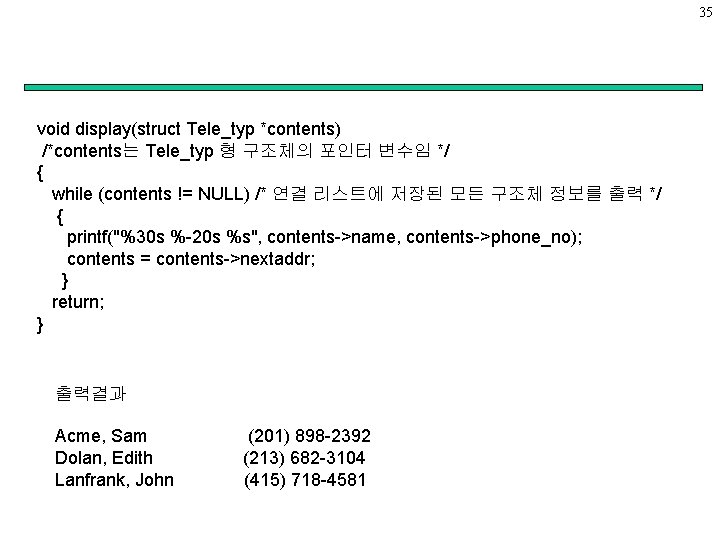
35 void display(struct Tele_typ *contents) /*contents는 Tele_typ 형 구조체의 포인터 변수임 */ { while (contents != NULL) /* 연결 리스트에 저장된 모든 구조체 정보를 출력 */ { printf("%30 s %-20 s %s", contents->name, contents->phone_no); contents = contents->nextaddr; } return; } 출력결과 Acme, Sam Dolan, Edith Lanfrank, John (201) 898 -2392 (213) 682 -3104 (415) 718 -4581
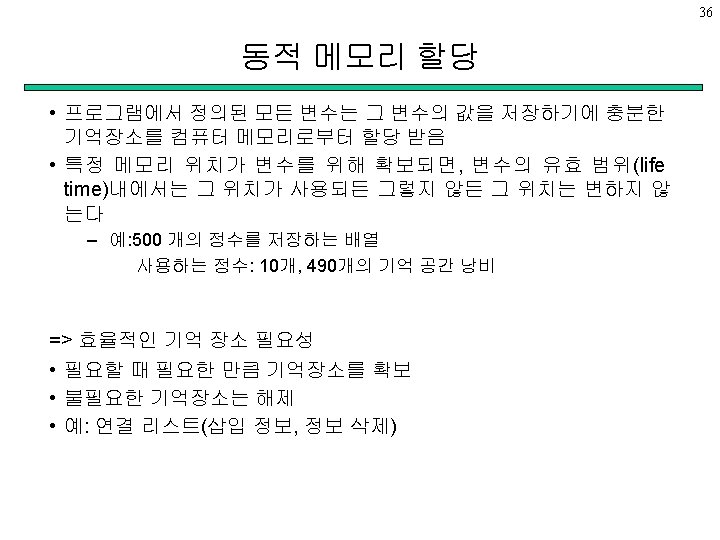
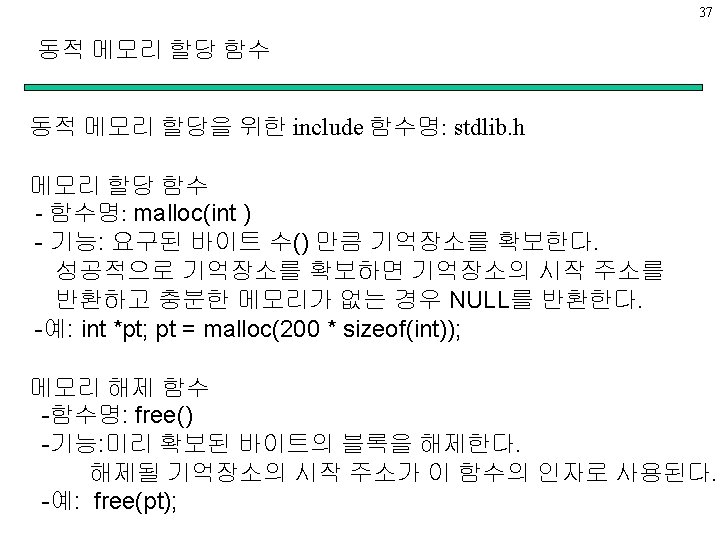
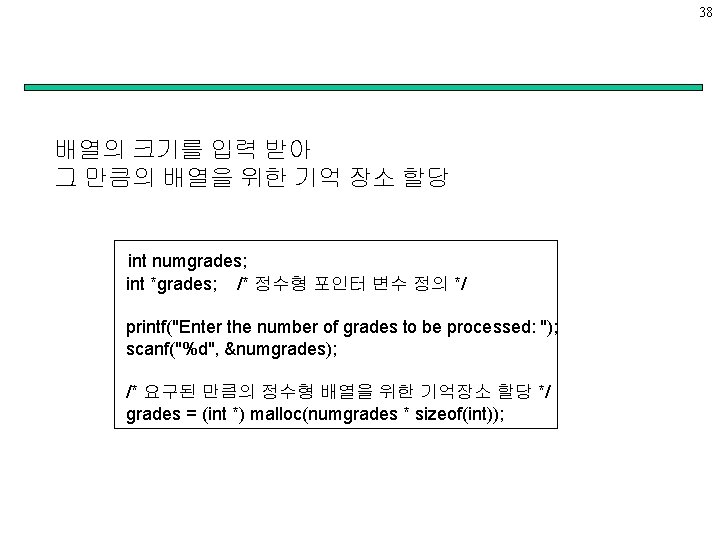
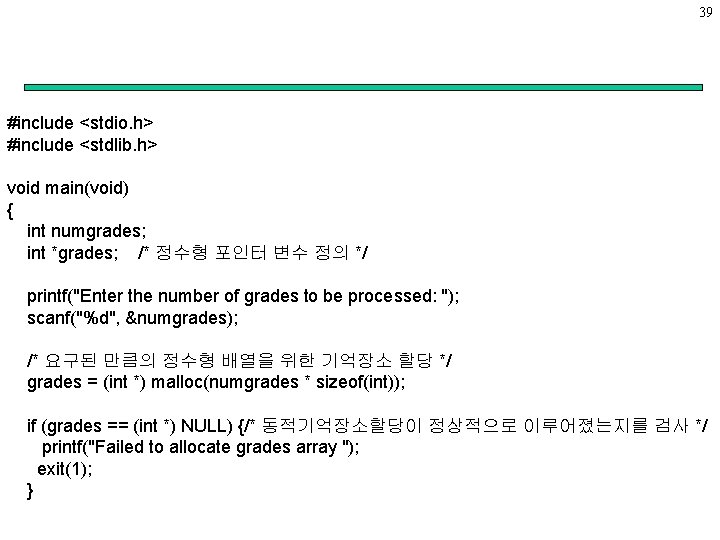
39 #include <stdio. h> #include <stdlib. h> void main(void) { int numgrades; int *grades; /* 정수형 포인터 변수 정의 */ printf("Enter the number of grades to be processed: "); scanf("%d", &numgrades); /* 요구된 만큼의 정수형 배열을 위한 기억장소 할당 */ grades = (int *) malloc(numgrades * sizeof(int)); if (grades == (int *) NULL) {/* 동적기억장소할당이 정상적으로 이루어졌는지를 검사 */ printf("Failed to allocate grades array "); exit(1); }
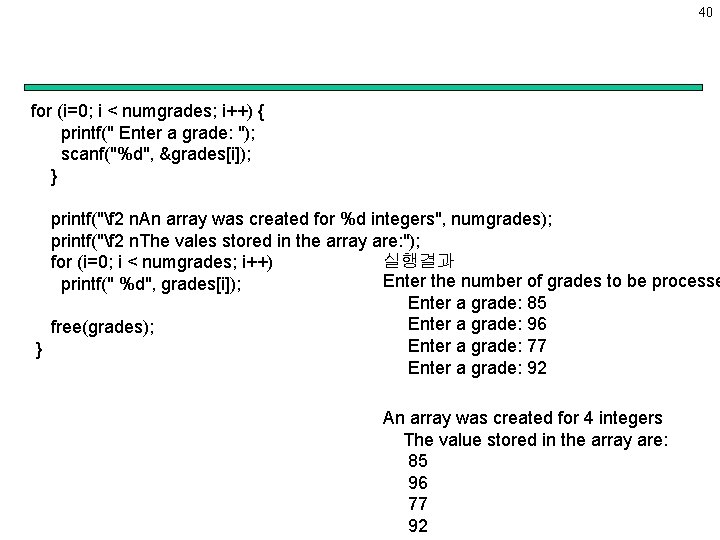
40 for (i=0; i < numgrades; i++) { printf(" Enter a grade: "); scanf("%d", &grades[i]); } printf("f 2 n. An array was created for %d integers", numgrades); printf("f 2 n. The vales stored in the array are: "); 실행결과 for (i=0; i < numgrades; i++) Enter the number of grades to be processe printf(" %d", grades[i]); Enter a grade: 85 Enter a grade: 96 free(grades); Enter a grade: 77 } Enter a grade: 92 An array was created for 4 integers The value stored in the array are: 85 96 77 92
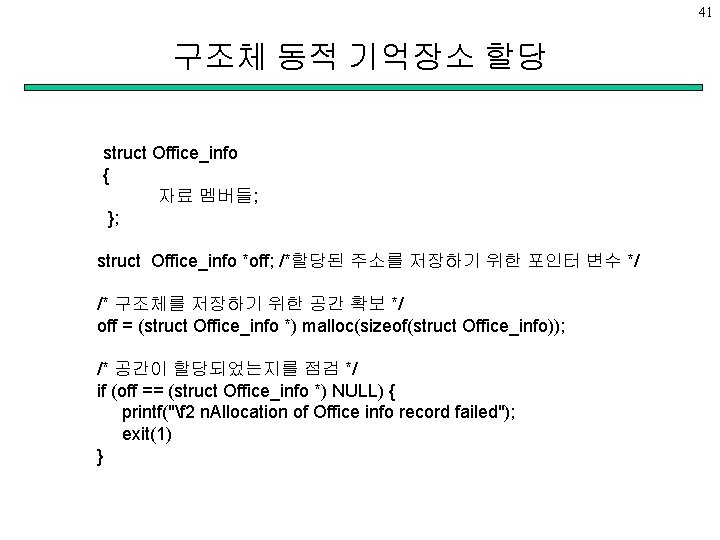
41 구조체 동적 기억장소 할당 struct Office_info { 자료 멤버들; }; struct Office_info *off; /*할당된 주소를 저장하기 위한 포인터 변수 */ /* 구조체를 저장하기 위한 공간 확보 */ off = (struct Office_info *) malloc(sizeof(struct Office_info)); /* 공간이 할당되었는지를 점검 */ if (off == (struct Office_info *) NULL) { printf("f 2 n. Allocation of Office info record failed"); exit(1) }
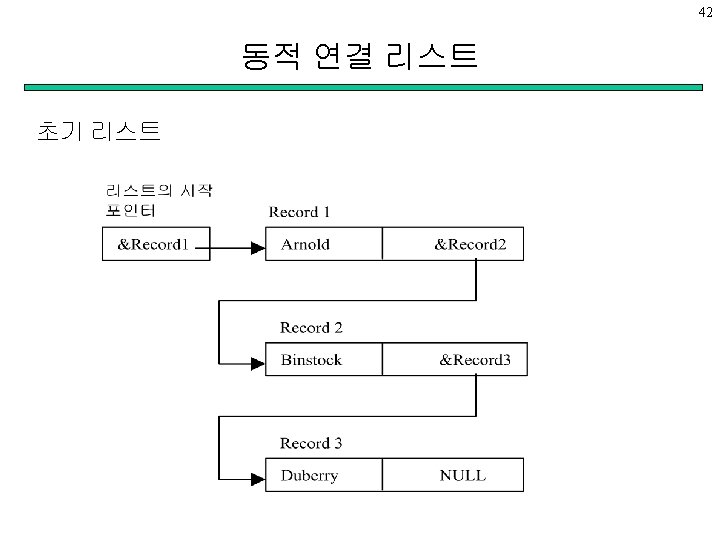
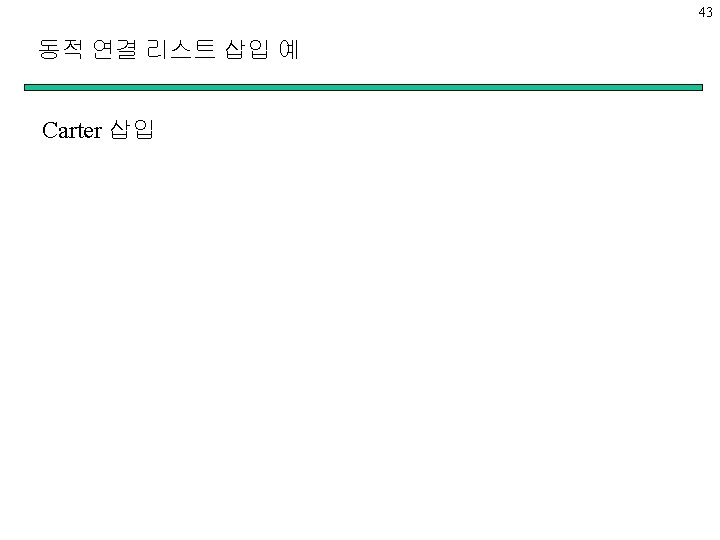
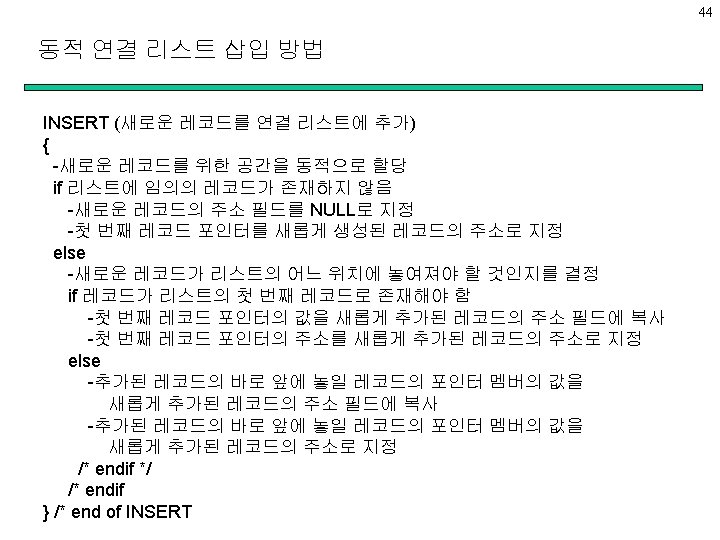
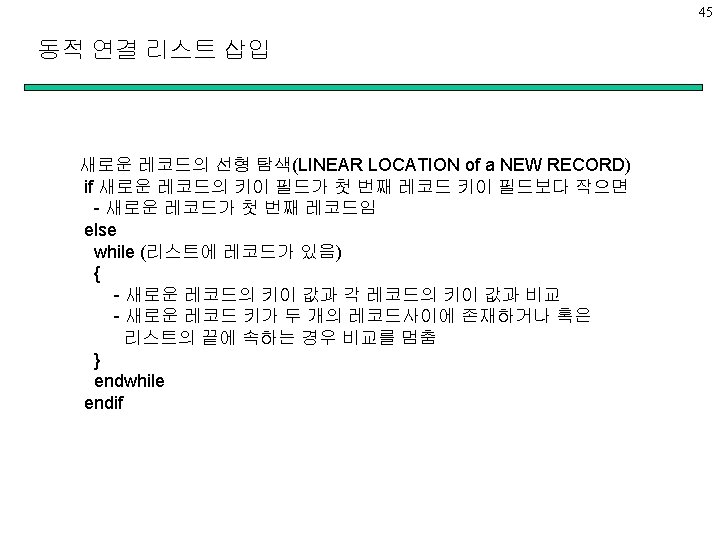
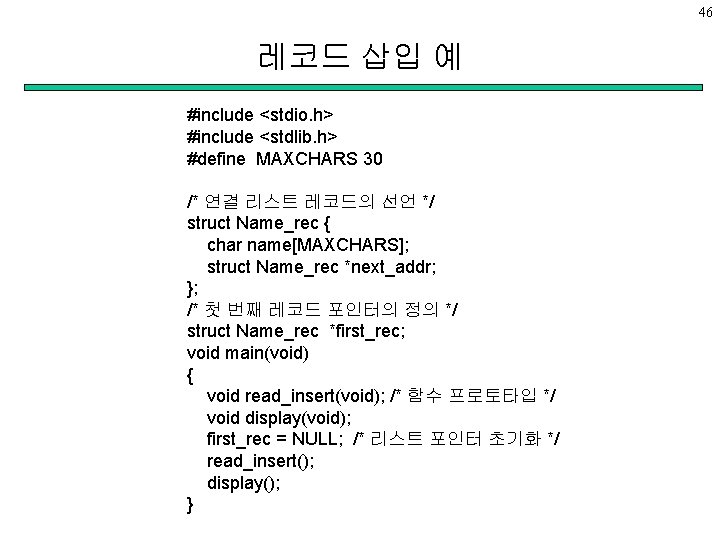
46 레코드 삽입 예 #include <stdio. h> #include <stdlib. h> #define MAXCHARS 30 /* 연결 리스트 레코드의 선언 */ struct Name_rec { char name[MAXCHARS]; struct Name_rec *next_addr; }; /* 첫 번째 레코드 포인터의 정의 */ struct Name_rec *first_rec; void main(void) { void read_insert(void); /* 함수 프로토타입 */ void display(void); first_rec = NULL; /* 리스트 포인터 초기화 */ read_insert(); display(); }
![47 이름을 입력받아 이를 연결 리스트에 삽입 void readinsertvoid char nameMAXCHARS 47 /* 이름을 입력받아 이를 연결 리스트에 삽입 */ void read_insert(void) { char name[MAXCHARS];](https://slidetodoc.com/presentation_image_h2/5f17dc378b5fbcaca400df444a61a038/image-47.jpg)
47 /* 이름을 입력받아 이를 연결 리스트에 삽입 */ void read_insert(void) { char name[MAXCHARS]; void insert(char []); printf("f 2 n. Enter as many names as you wish, one per line"); printf("f 2 n. To stop entering names, enter a single x "); while(1) { printf("Enter a name: "); gets(name); if (strcmp(name, "x") == 0) break; insert(name); } }
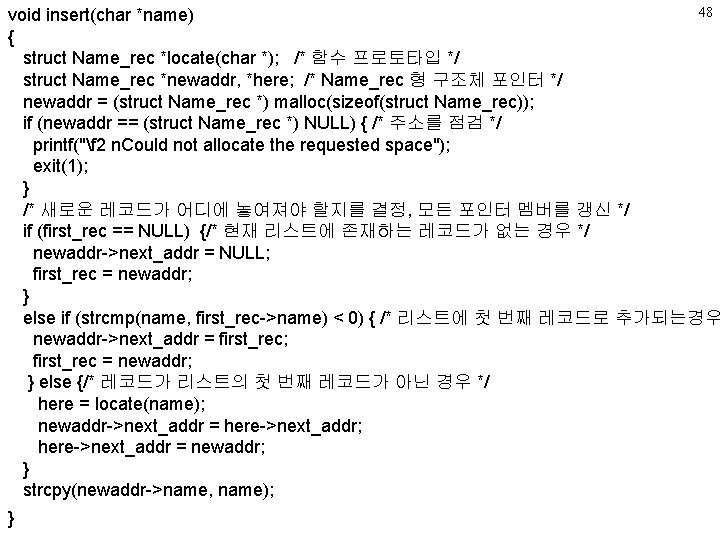
48 void insert(char *name) { struct Name_rec *locate(char *); /* 함수 프로토타입 */ struct Name_rec *newaddr, *here; /* Name_rec 형 구조체 포인터 */ newaddr = (struct Name_rec *) malloc(sizeof(struct Name_rec)); if (newaddr == (struct Name_rec *) NULL) { /* 주소를 점검 */ printf("f 2 n. Could not allocate the requested space"); exit(1); } /* 새로운 레코드가 어디에 놓여져야 할지를 결정, 모든 포인터 멤버를 갱신 */ if (first_rec == NULL) {/* 현재 리스트에 존재하는 레코드가 없는 경우 */ newaddr->next_addr = NULL; first_rec = newaddr; } else if (strcmp(name, first_rec->name) < 0) { /* 리스트에 첫 번째 레코드로 추가되는경우 newaddr->next_addr = first_rec; first_rec = newaddr; } else {/* 레코드가 리스트의 첫 번째 레코드가 아닌 경우 */ here = locate(name); newaddr->next_addr = here->next_addr; here->next_addr = newaddr; } strcpy(newaddr->name, name); }
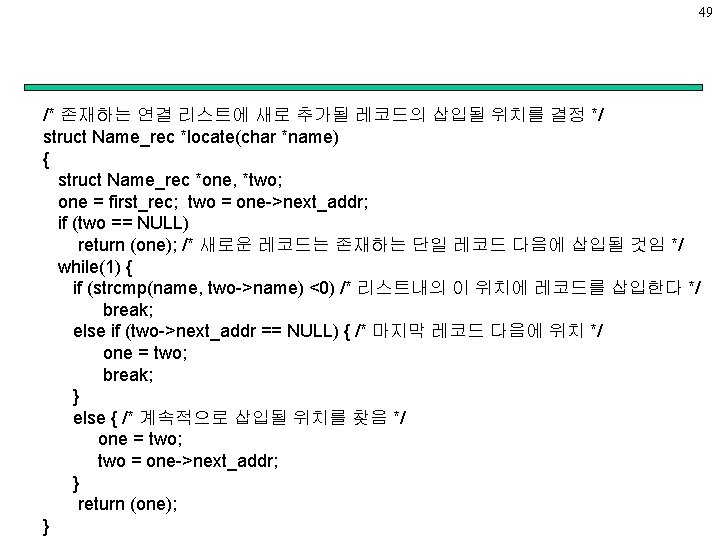
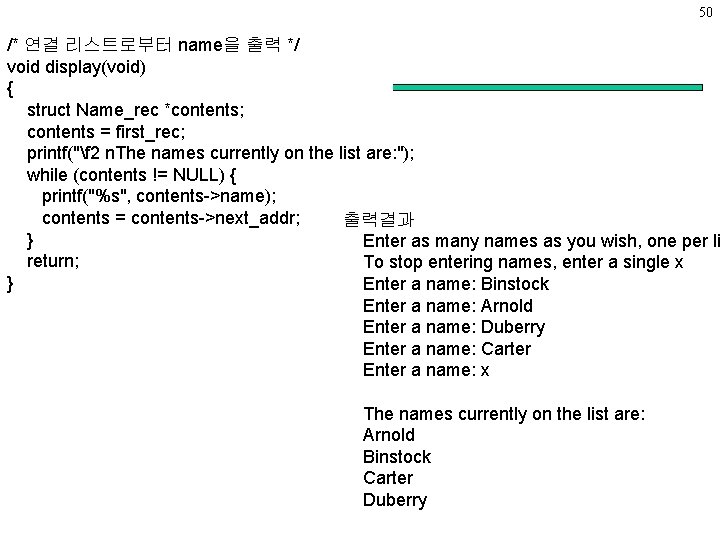
50 /* 연결 리스트로부터 name을 출력 */ void display(void) { struct Name_rec *contents; contents = first_rec; printf("f 2 n. The names currently on the list are: "); while (contents != NULL) { printf("%s", contents->name); contents = contents->next_addr; 출력결과 } Enter as many names as you wish, one per li return; To stop entering names, enter a single x } Enter a name: Binstock Enter a name: Arnold Enter a name: Duberry Enter a name: Carter Enter a name: x The names currently on the list are: Arnold Binstock Carter Duberry
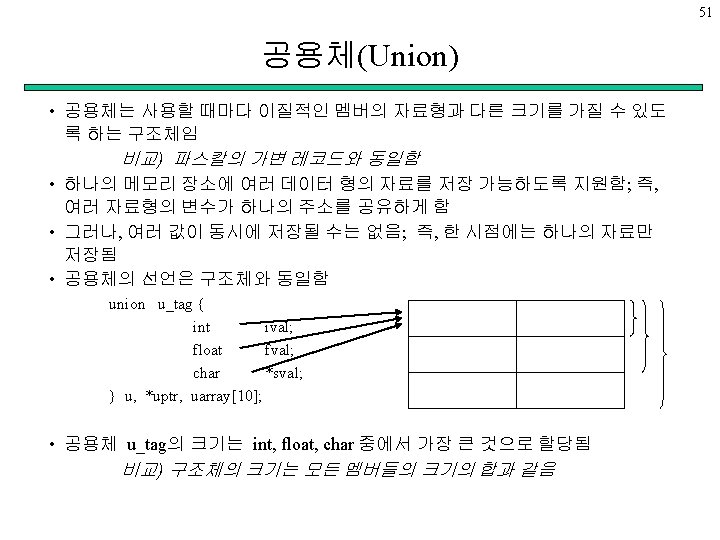
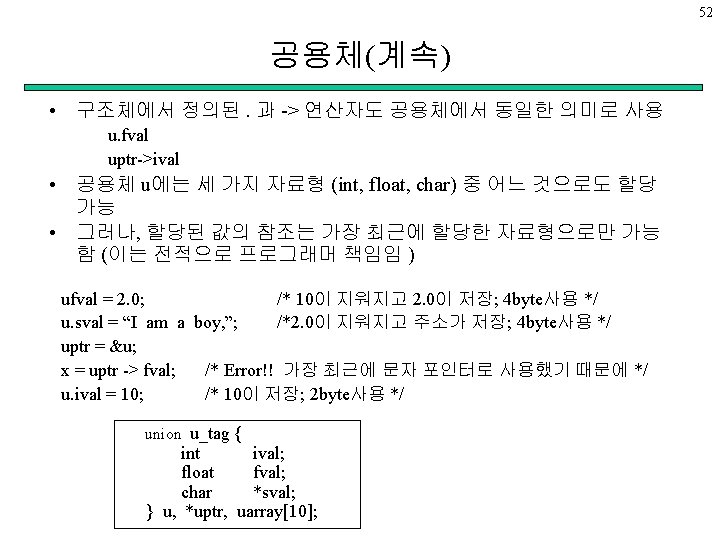
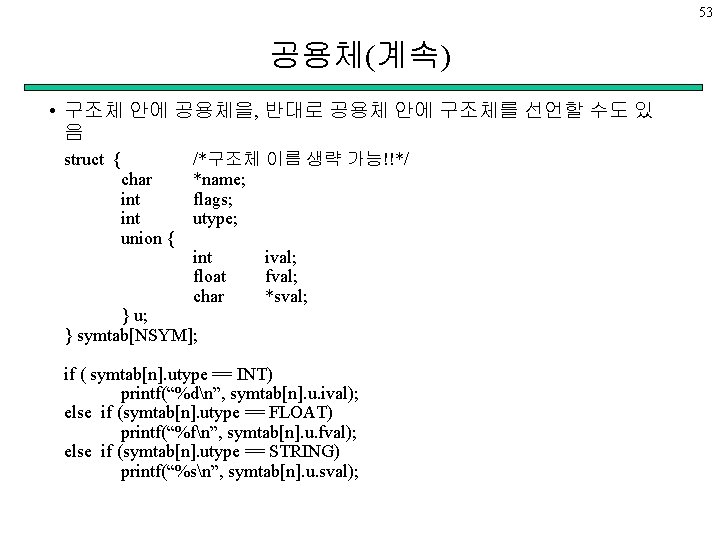
53 공용체(계속) • 구조체 안에 공용체을, 반대로 공용체 안에 구조체를 선언할 수도 있 음 struct { char int union { /*구조체 이름 생략 가능!!*/ *name; flags; utype; int float char } u; } symtab[NSYM]; ival; fval; *sval; if ( symtab[n]. utype == INT) printf(“%dn”, symtab[n]. u. ival); else if (symtab[n]. utype == FLOAT) printf(“%fn”, symtab[n]. u. fval); else if (symtab[n]. utype == STRING) printf(“%sn”, symtab[n]. u. sval);
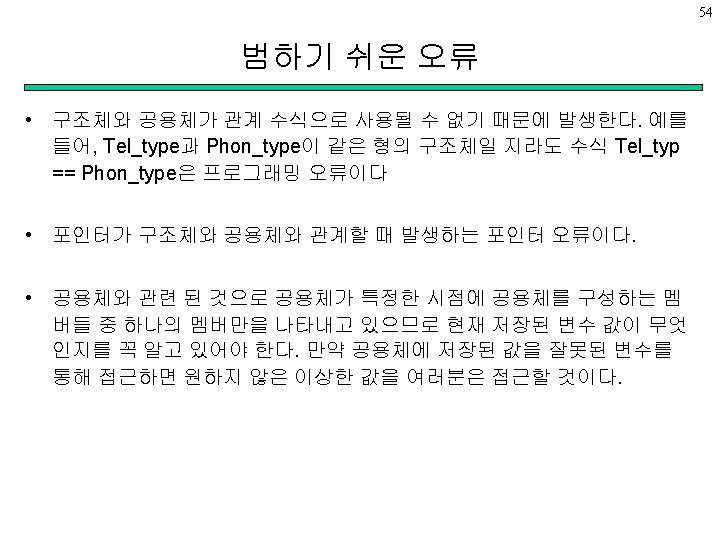
I^2
#include stdio.h void main()
Public void drawsquare(int x, int y, int len)
Int sum(int a int n) int sum=0 i
#include stdio h
Include stdio.h
#include stdio.h #include stdlib.h #include string.h
#include stdio.h #include conio.h #include stdlib.h
Typedef struct tree int info struct *left
Typedef struct node
Int tree
Struct node int data struct node* next
Struct sample int a
#include stdio.h void main()
#include stdio.h void main()
Num=printf( 2 )
#include stdio.h void main()
#include stdio.h void main()
#include stdio.h void main()
#include stdio.h int main()
Void swap(int a int b)
Void
Inheritance calculator
Public int divide(int a int b)
Int max(int x int y)
Stdlib h
How to copy a struct in c
Struct node int data
Nodenext
Typedef struct node int value
Struct node int i float j
Typedef struct node
What are structure
Struct point int x y
Struct point int x y
Void main(void)
Void setup(void)
Void main(void)
#include stdio.h
#include stdio.h
H
##include stdio.h
Pthread h
Stdio