XOM XOM Design Principles n n XOM XML
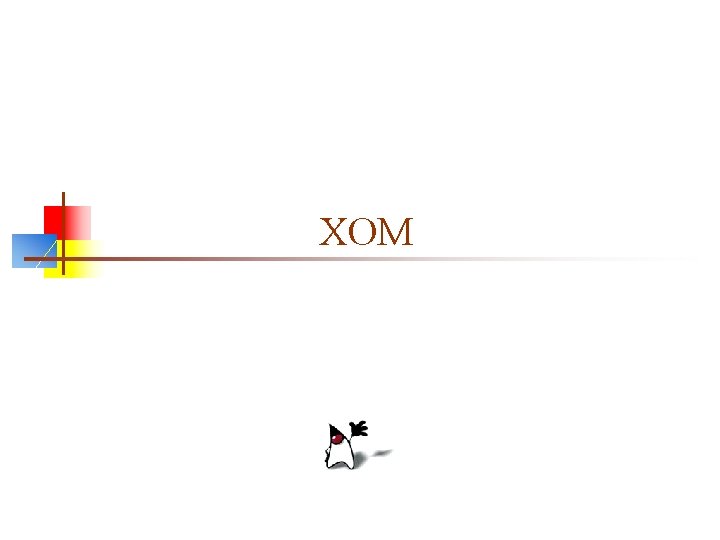
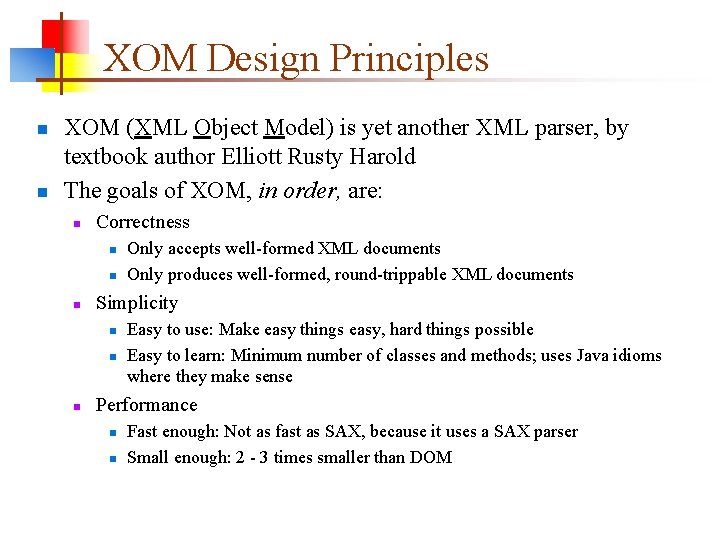
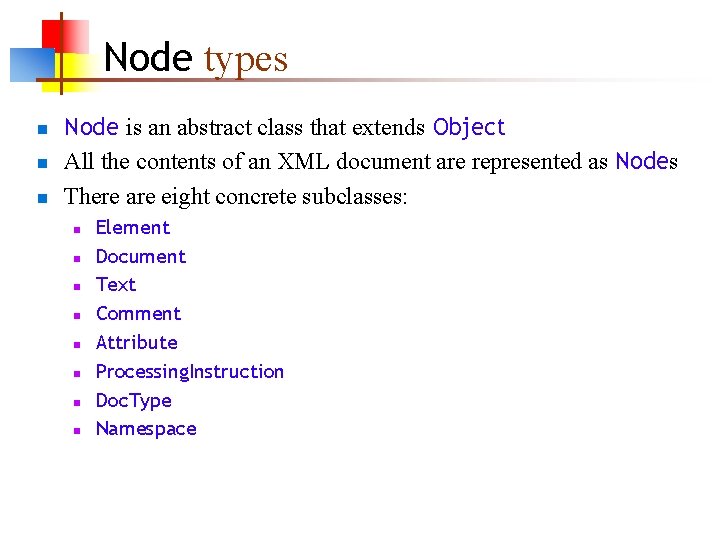
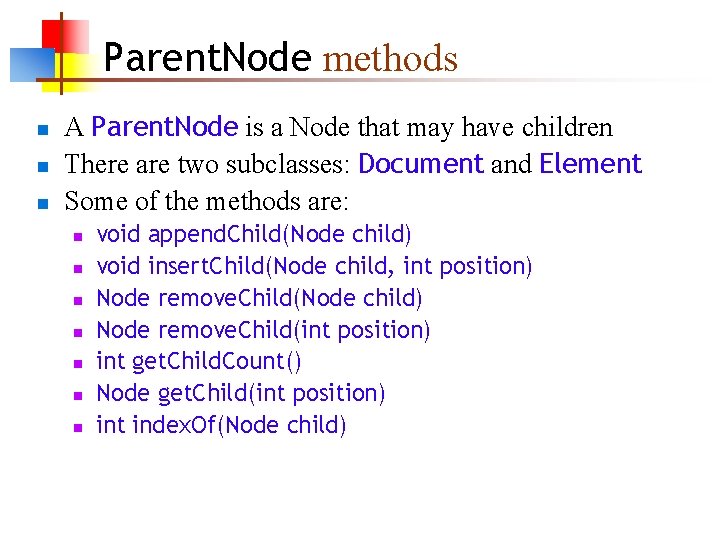
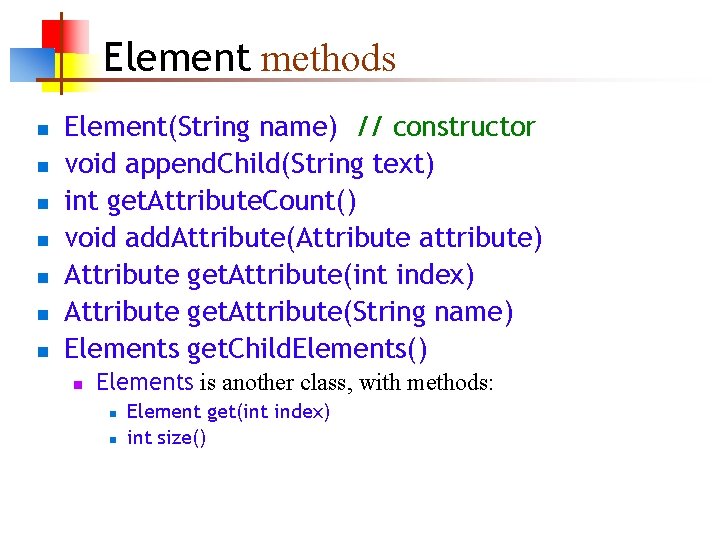
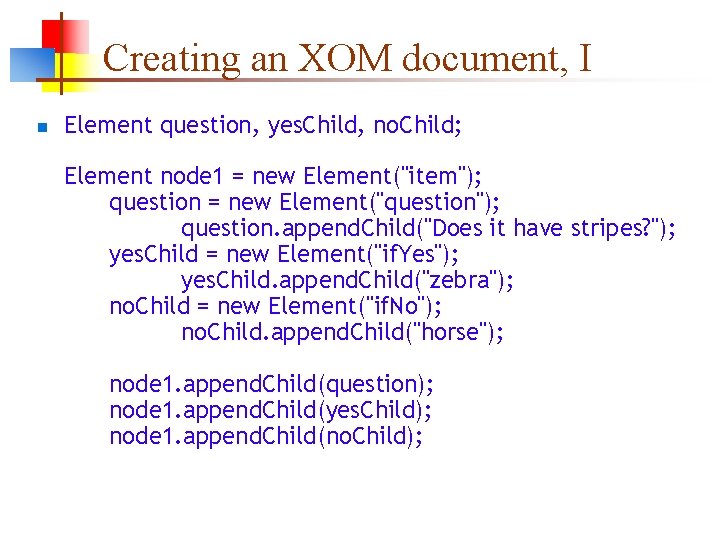
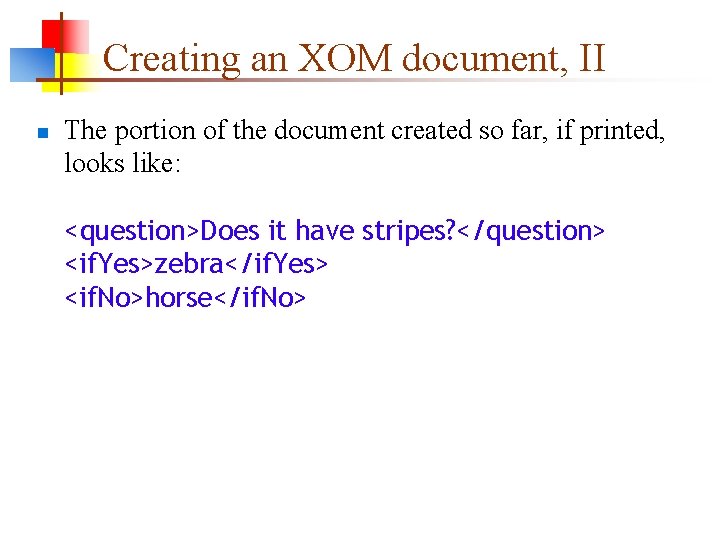
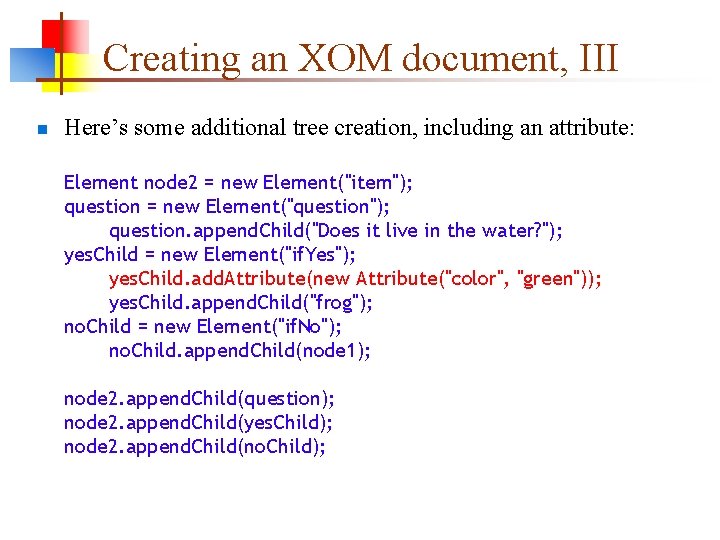
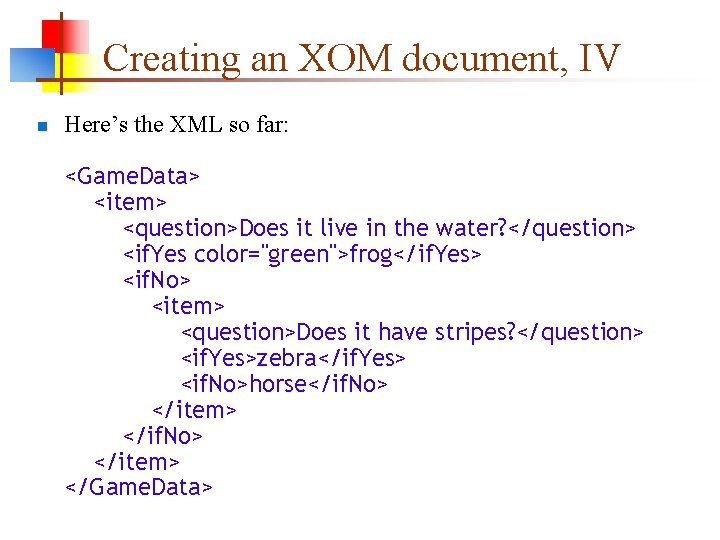
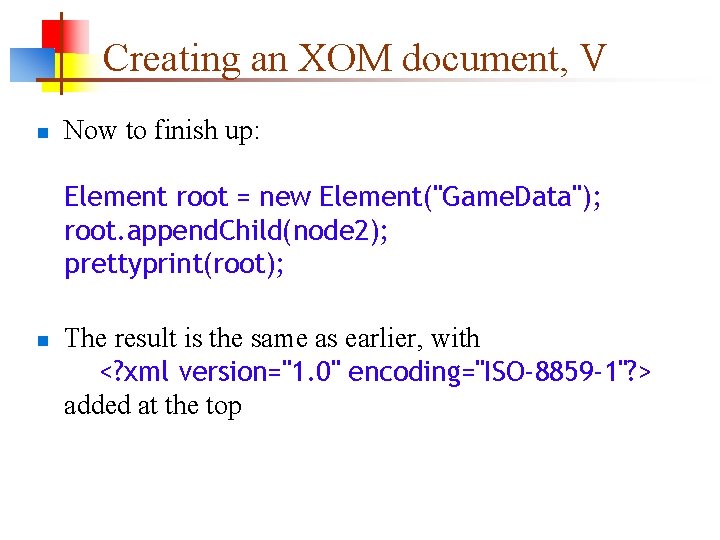
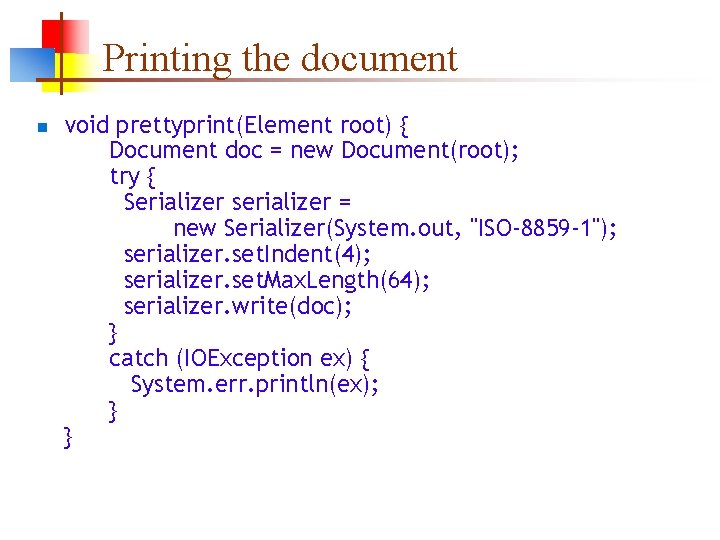
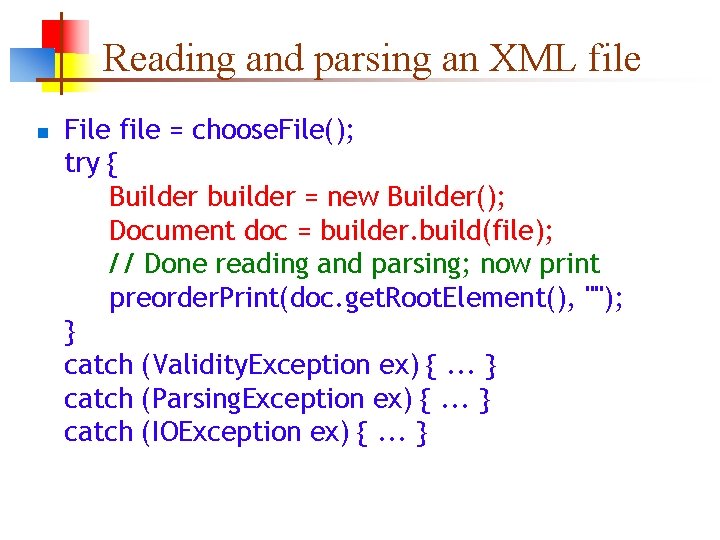
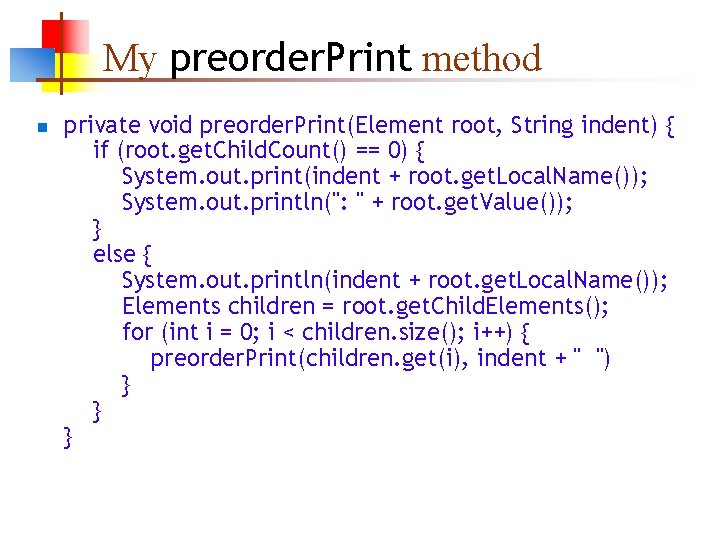
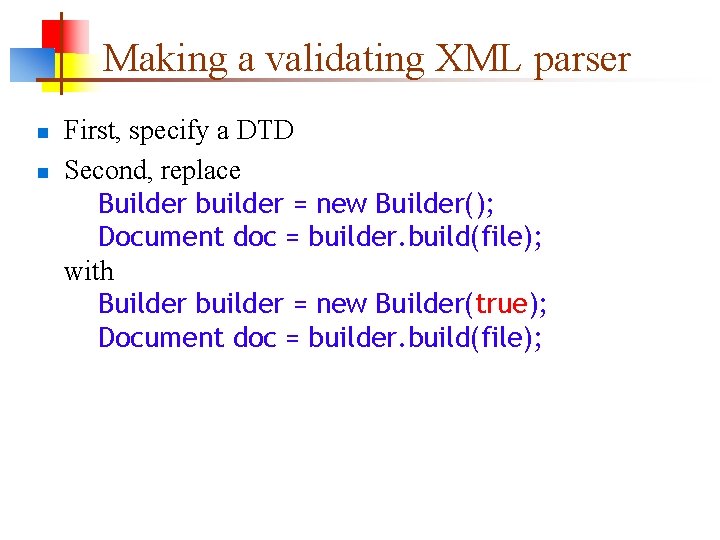
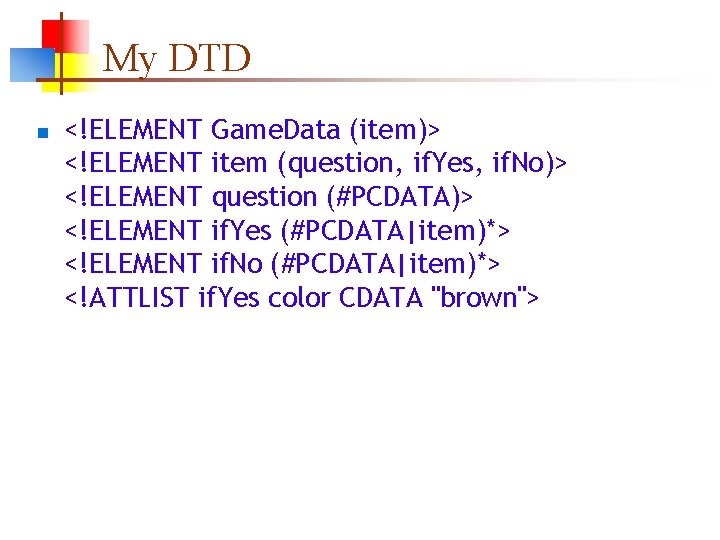
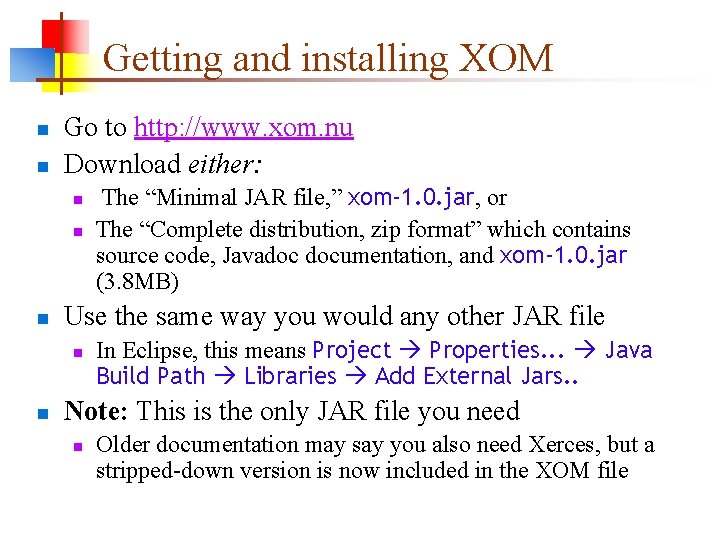
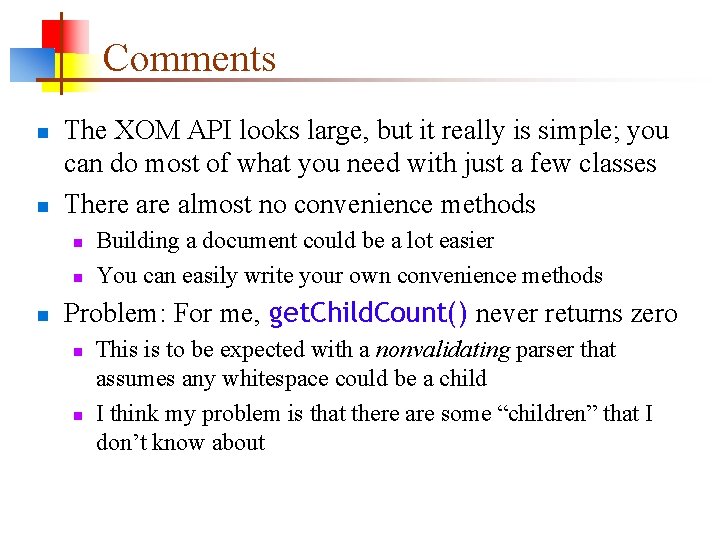
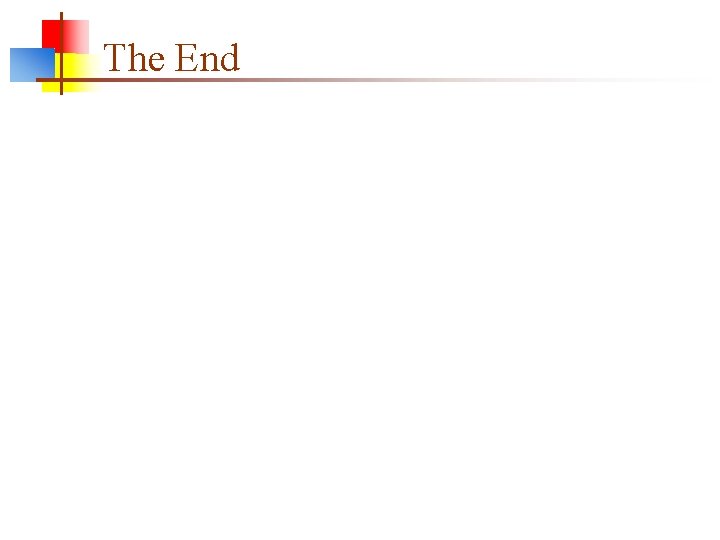
- Slides: 18
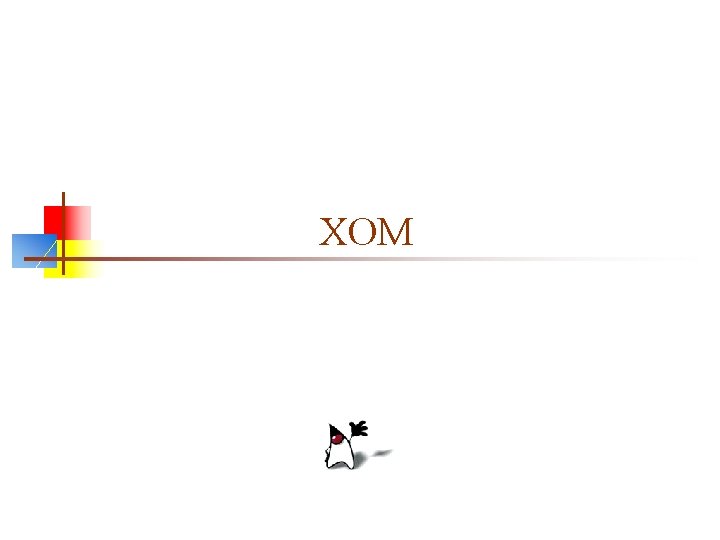
XOM
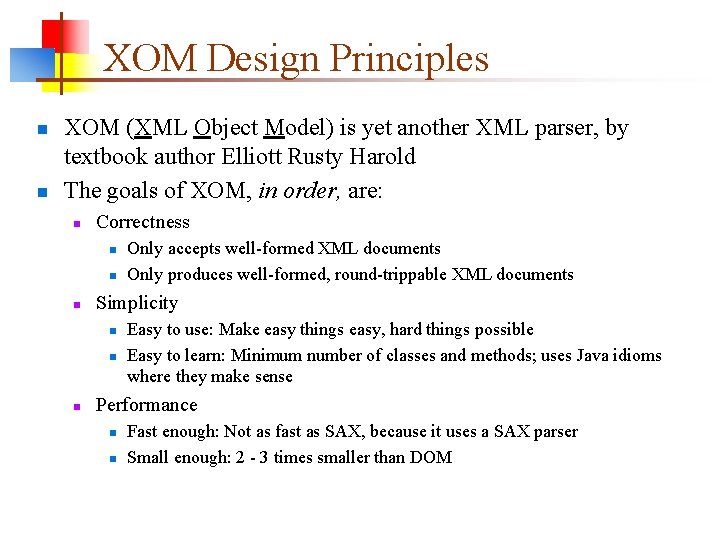
XOM Design Principles n n XOM (XML Object Model) is yet another XML parser, by textbook author Elliott Rusty Harold The goals of XOM, in order, are: n Correctness n n n Simplicity n n n Only accepts well-formed XML documents Only produces well-formed, round-trippable XML documents Easy to use: Make easy things easy, hard things possible Easy to learn: Minimum number of classes and methods; uses Java idioms where they make sense Performance n n Fast enough: Not as fast as SAX, because it uses a SAX parser Small enough: 2 - 3 times smaller than DOM
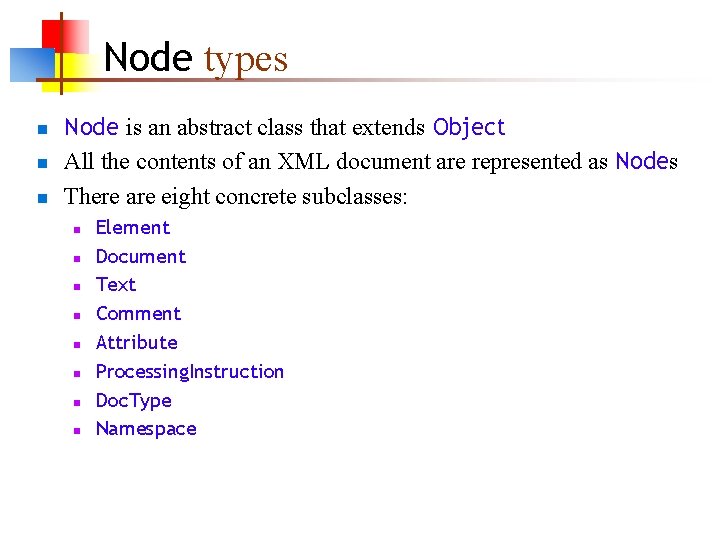
Node types n n n Node is an abstract class that extends Object All the contents of an XML document are represented as Nodes There are eight concrete subclasses: n n n n Element Document Text Comment Attribute Processing. Instruction Doc. Type Namespace
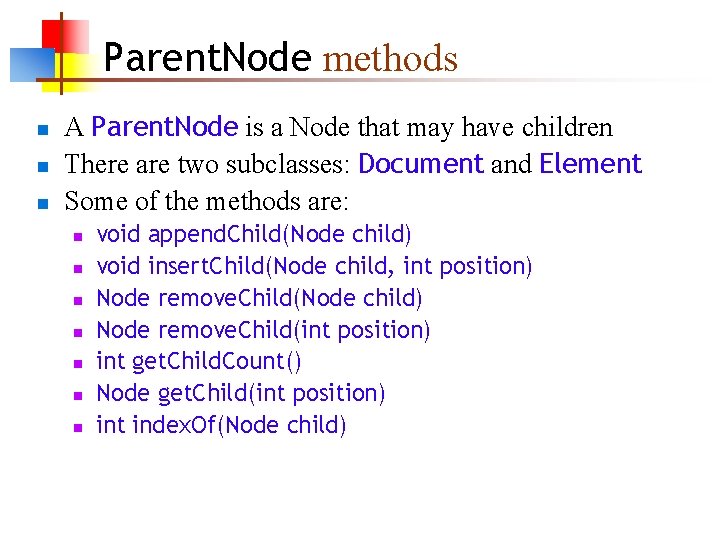
Parent. Node methods n n n A Parent. Node is a Node that may have children There are two subclasses: Document and Element Some of the methods are: n n n n void append. Child(Node child) void insert. Child(Node child, int position) Node remove. Child(Node child) Node remove. Child(int position) int get. Child. Count() Node get. Child(int position) int index. Of(Node child)
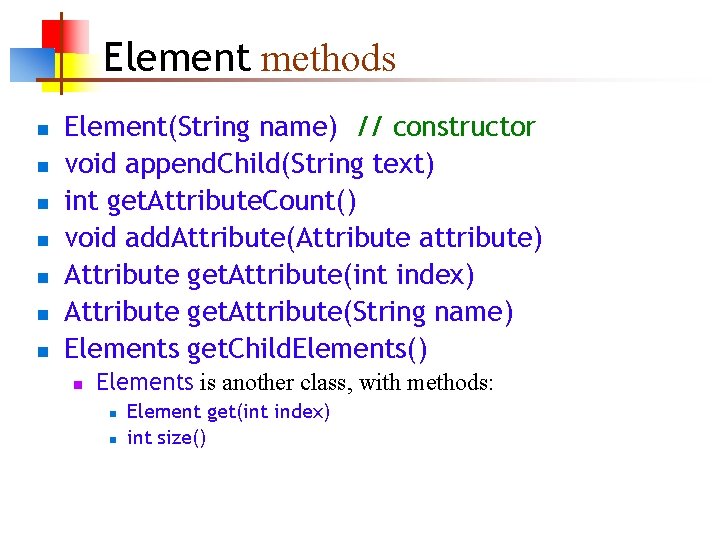
Element methods n n n n Element(String name) // constructor void append. Child(String text) int get. Attribute. Count() void add. Attribute(Attribute attribute) Attribute get. Attribute(int index) Attribute get. Attribute(String name) Elements get. Child. Elements() n Elements is another class, with methods: n n Element get(int index) int size()
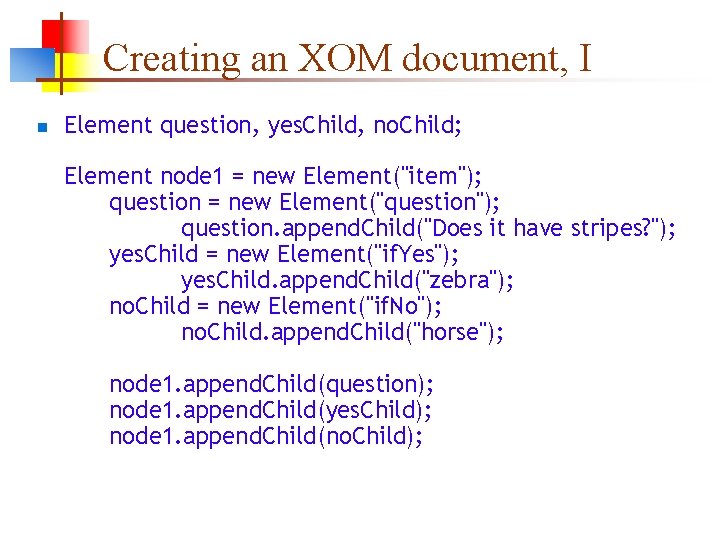
Creating an XOM document, I n Element question, yes. Child, no. Child; Element node 1 = new Element("item"); question = new Element("question"); question. append. Child("Does it have stripes? "); yes. Child = new Element("if. Yes"); yes. Child. append. Child("zebra"); no. Child = new Element("if. No"); no. Child. append. Child("horse"); node 1. append. Child(question); node 1. append. Child(yes. Child); node 1. append. Child(no. Child);
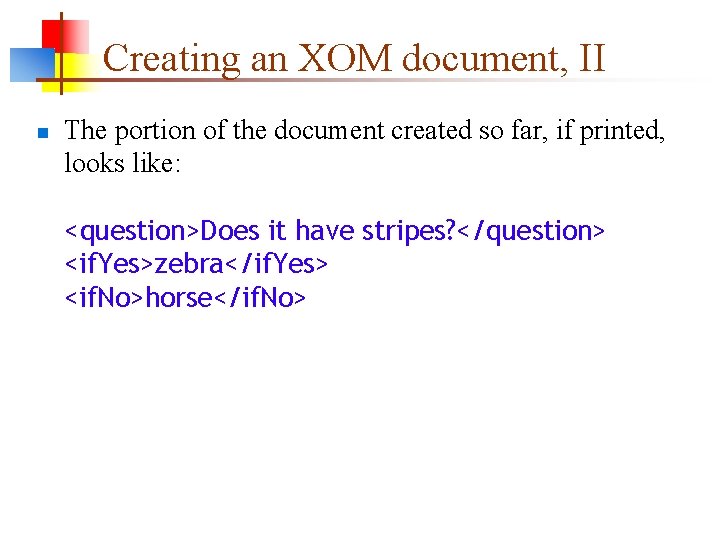
Creating an XOM document, II n The portion of the document created so far, if printed, looks like: <question>Does it have stripes? </question> <if. Yes>zebra</if. Yes> <if. No>horse</if. No>
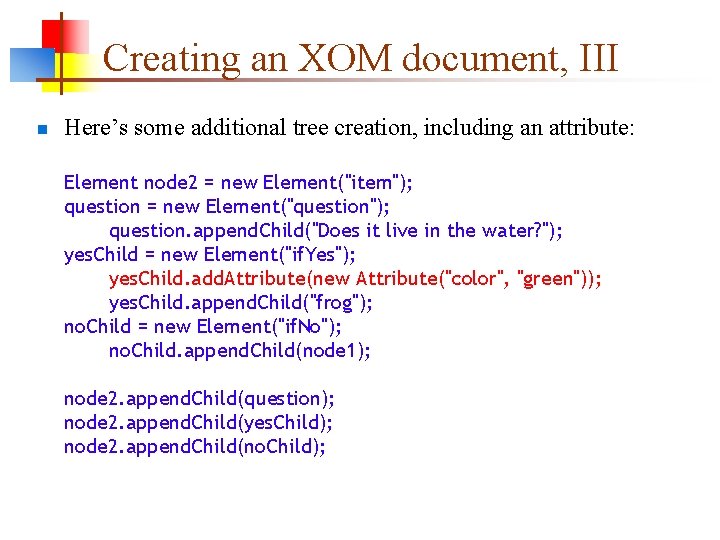
Creating an XOM document, III n Here’s some additional tree creation, including an attribute: Element node 2 = new Element("item"); question = new Element("question"); question. append. Child("Does it live in the water? "); yes. Child = new Element("if. Yes"); yes. Child. add. Attribute(new Attribute("color", "green")); yes. Child. append. Child("frog"); no. Child = new Element("if. No"); no. Child. append. Child(node 1); node 2. append. Child(question); node 2. append. Child(yes. Child); node 2. append. Child(no. Child);
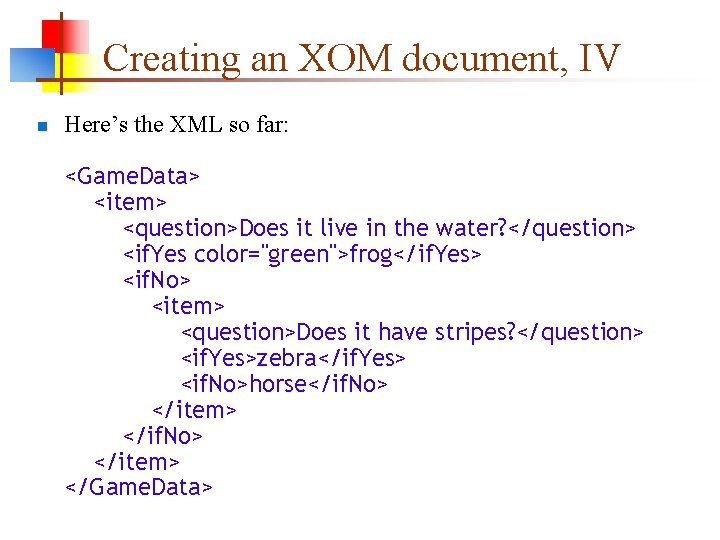
Creating an XOM document, IV n Here’s the XML so far: <Game. Data> <item> <question>Does it live in the water? </question> <if. Yes color="green">frog</if. Yes> <if. No> <item> <question>Does it have stripes? </question> <if. Yes>zebra</if. Yes> <if. No>horse</if. No> </item> </Game. Data>
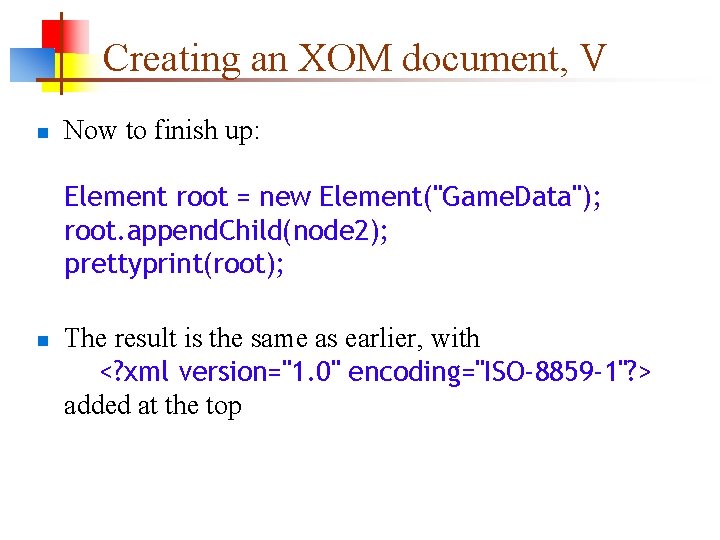
Creating an XOM document, V n Now to finish up: Element root = new Element("Game. Data"); root. append. Child(node 2); prettyprint(root); n The result is the same as earlier, with <? xml version="1. 0" encoding="ISO-8859 -1"? > added at the top
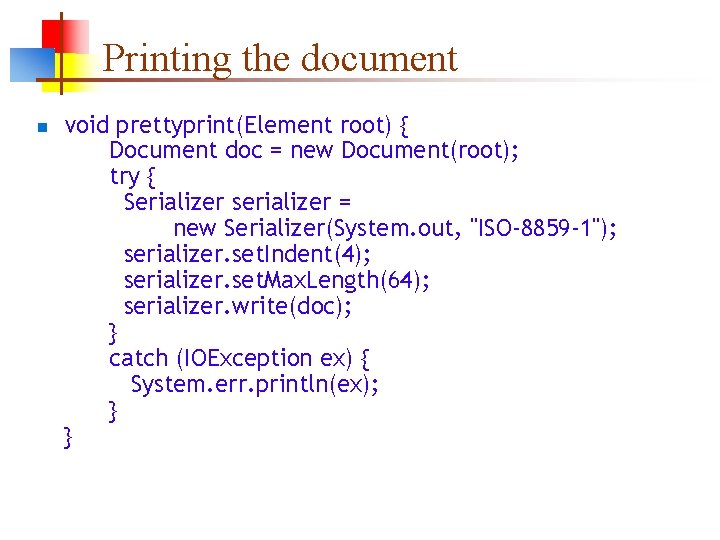
Printing the document n void prettyprint(Element root) { Document doc = new Document(root); try { Serializer serializer = new Serializer(System. out, "ISO-8859 -1"); serializer. set. Indent(4); serializer. set. Max. Length(64); serializer. write(doc); } catch (IOException ex) { System. err. println(ex); } }
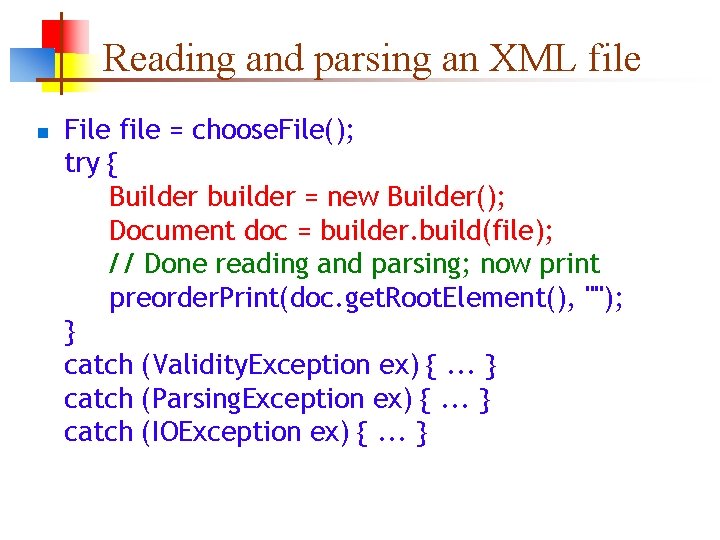
Reading and parsing an XML file n File file = choose. File(); try { Builder builder = new Builder(); Document doc = builder. build(file); // Done reading and parsing; now print preorder. Print(doc. get. Root. Element(), ""); } catch (Validity. Exception ex) {. . . } catch (Parsing. Exception ex) {. . . } catch (IOException ex) {. . . }
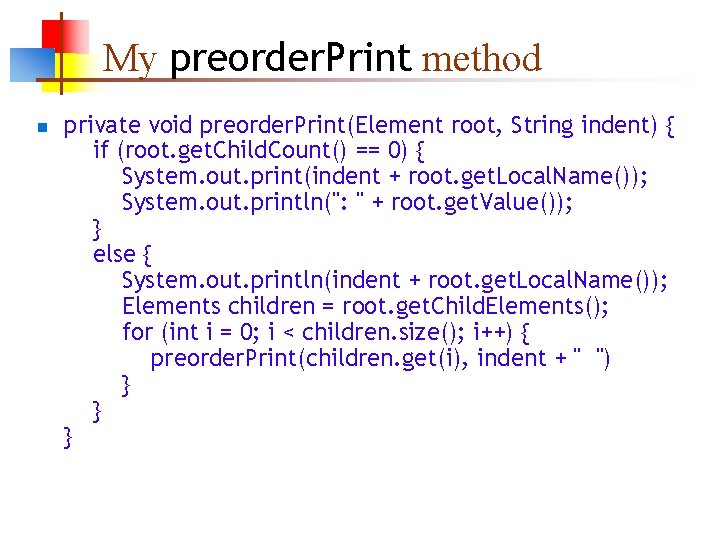
My preorder. Print method n private void preorder. Print(Element root, String indent) { if (root. get. Child. Count() == 0) { System. out. print(indent + root. get. Local. Name()); System. out. println(": " + root. get. Value()); } else { System. out. println(indent + root. get. Local. Name()); Elements children = root. get. Child. Elements(); for (int i = 0; i < children. size(); i++) { preorder. Print(children. get(i), indent + " ") } } }
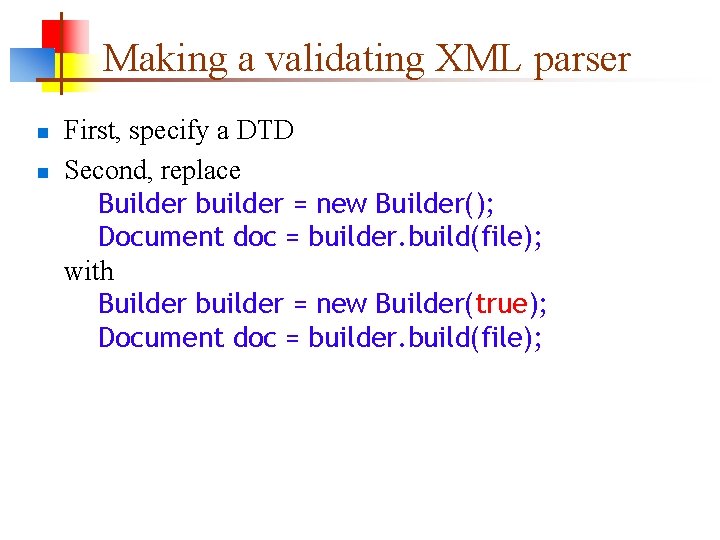
Making a validating XML parser n n First, specify a DTD Second, replace Builder builder = new Builder(); Document doc = builder. build(file); with Builder builder = new Builder(true); Document doc = builder. build(file);
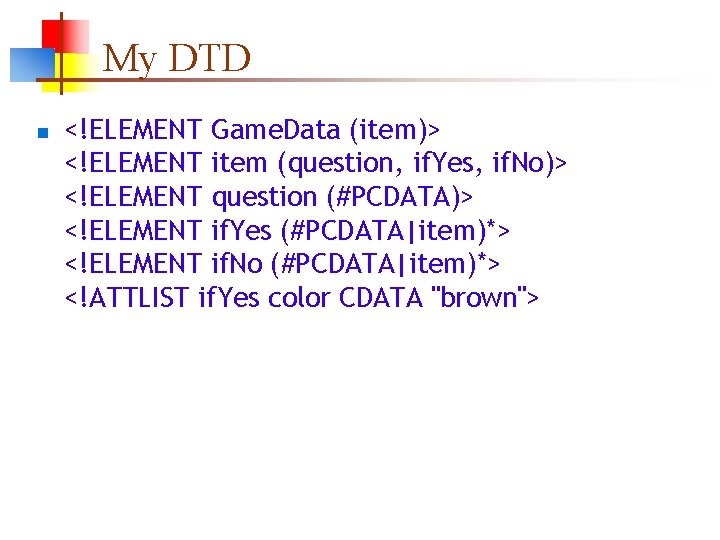
My DTD n <!ELEMENT Game. Data (item)> <!ELEMENT item (question, if. Yes, if. No)> <!ELEMENT question (#PCDATA)> <!ELEMENT if. Yes (#PCDATA|item)*> <!ELEMENT if. No (#PCDATA|item)*> <!ATTLIST if. Yes color CDATA "brown">
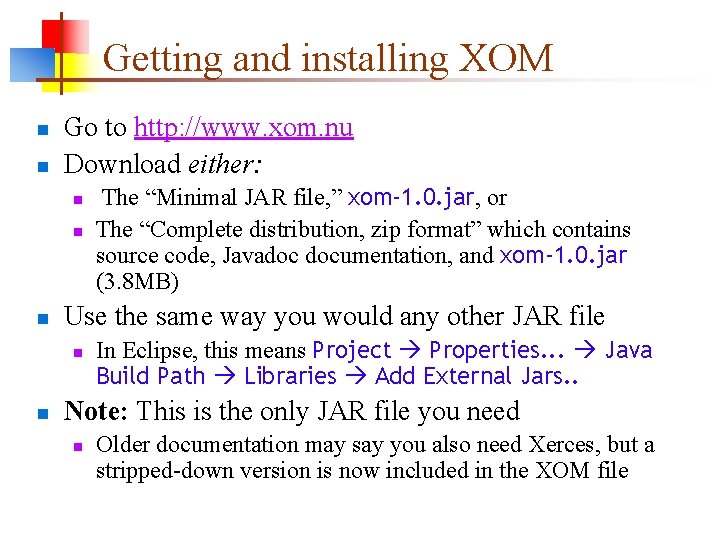
Getting and installing XOM n n Go to http: //www. xom. nu Download either: n n n Use the same way you would any other JAR file n n The “Minimal JAR file, ” xom-1. 0. jar, or The “Complete distribution, zip format” which contains source code, Javadoc documentation, and xom-1. 0. jar (3. 8 MB) In Eclipse, this means Project Properties. . . Java Build Path Libraries Add External Jars. . Note: This is the only JAR file you need n Older documentation may say you also need Xerces, but a stripped-down version is now included in the XOM file
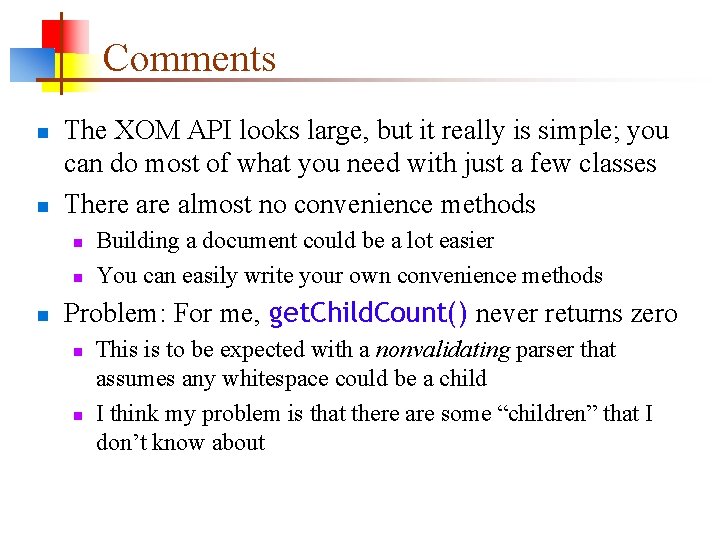
Comments n n The XOM API looks large, but it really is simple; you can do most of what you need with just a few classes There almost no convenience methods n n n Building a document could be a lot easier You can easily write your own convenience methods Problem: For me, get. Child. Count() never returns zero n n This is to be expected with a nonvalidating parser that assumes any whitespace could be a child I think my problem is that there are some “children” that I don’t know about
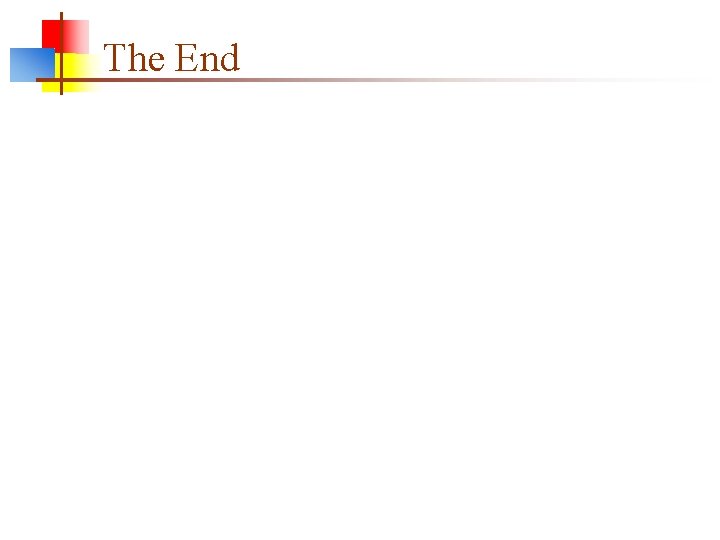
The End