XNA An Introduction What XNA is Microsoft XNA
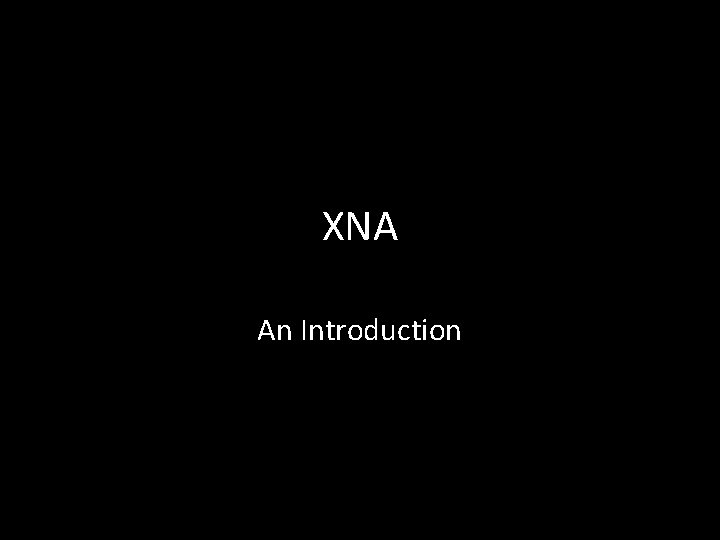
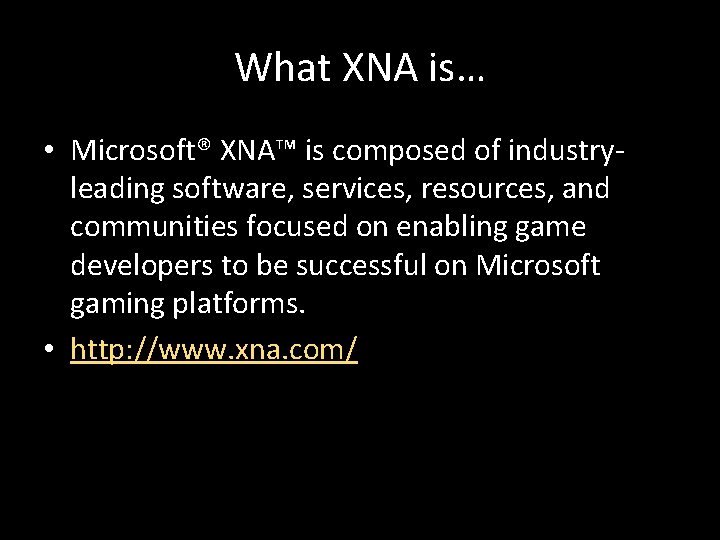
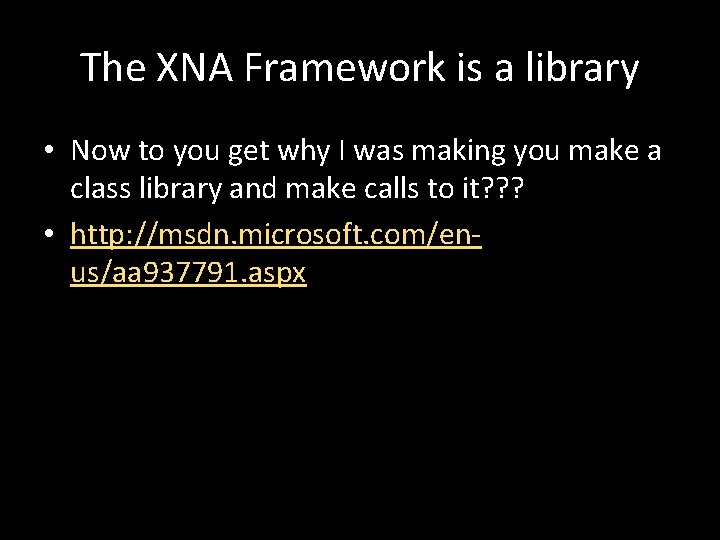
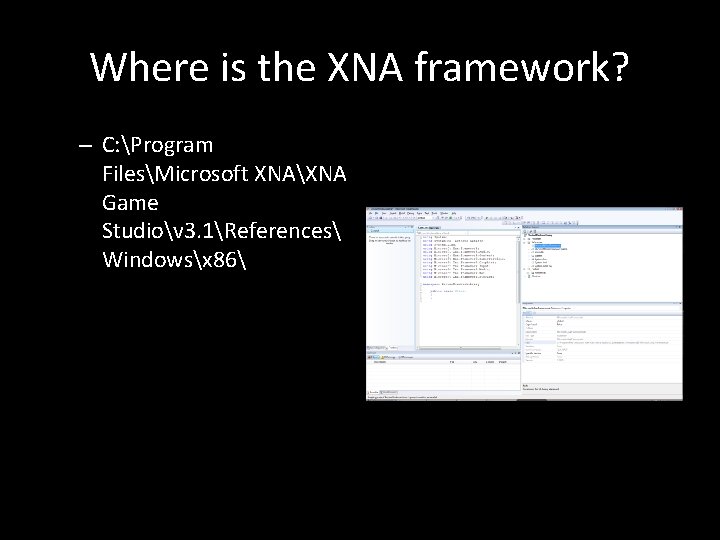
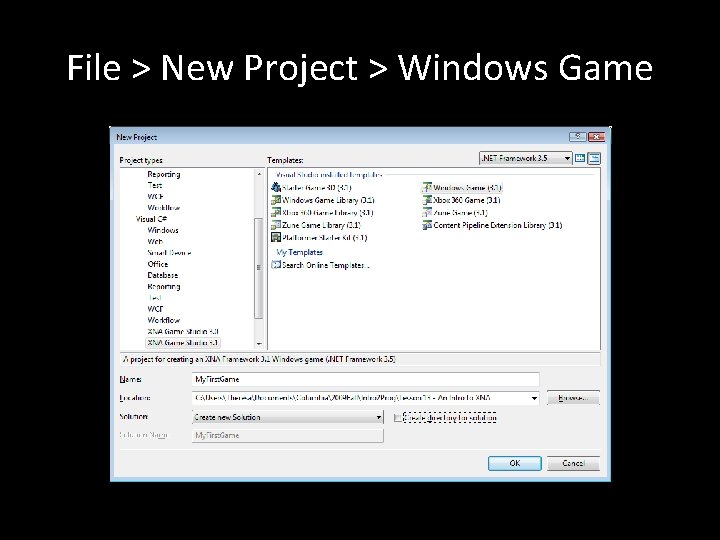
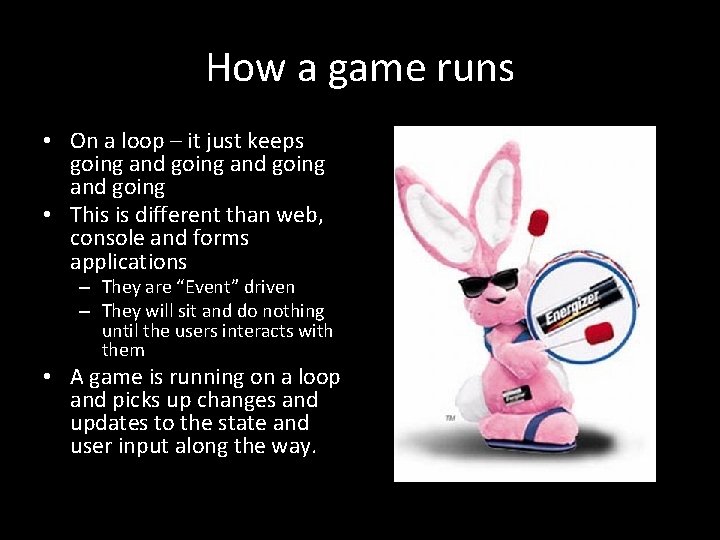
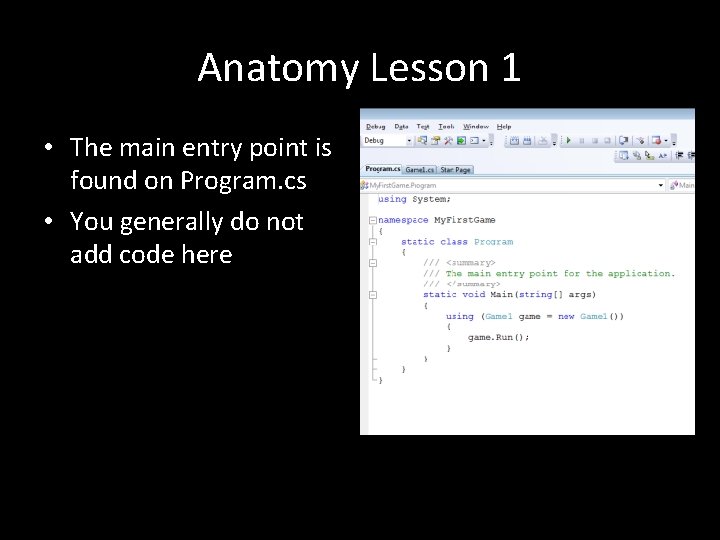
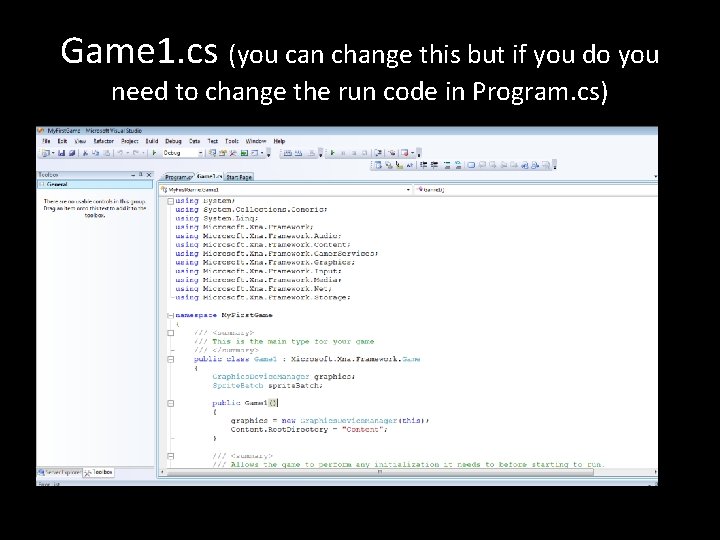
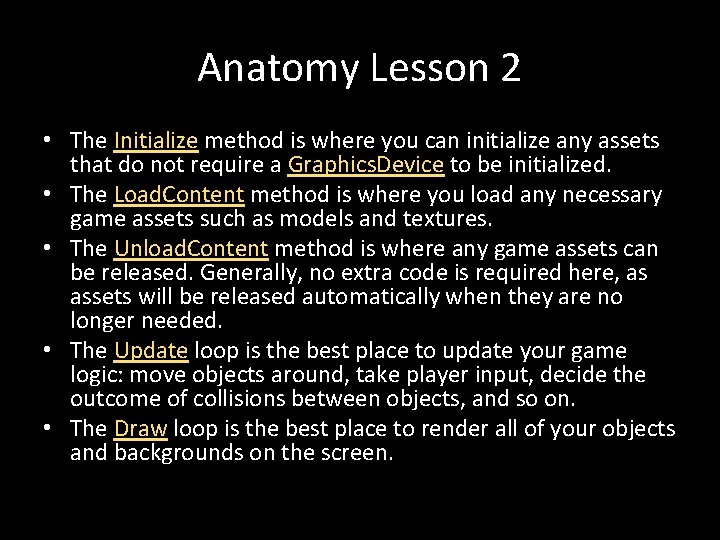
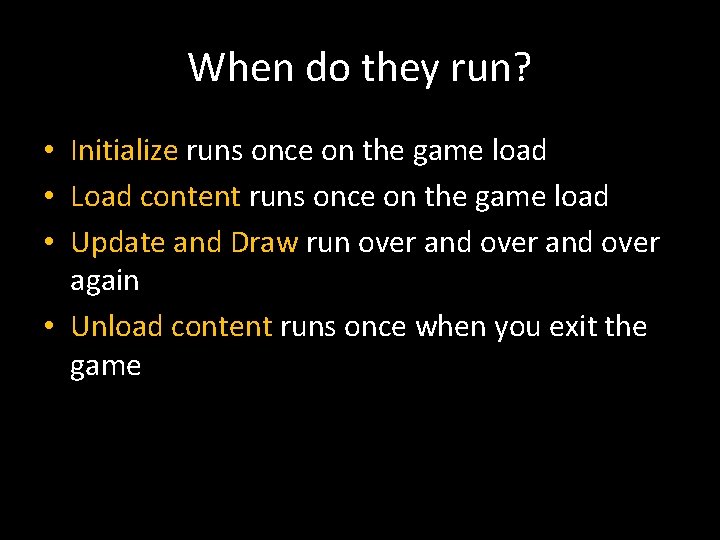
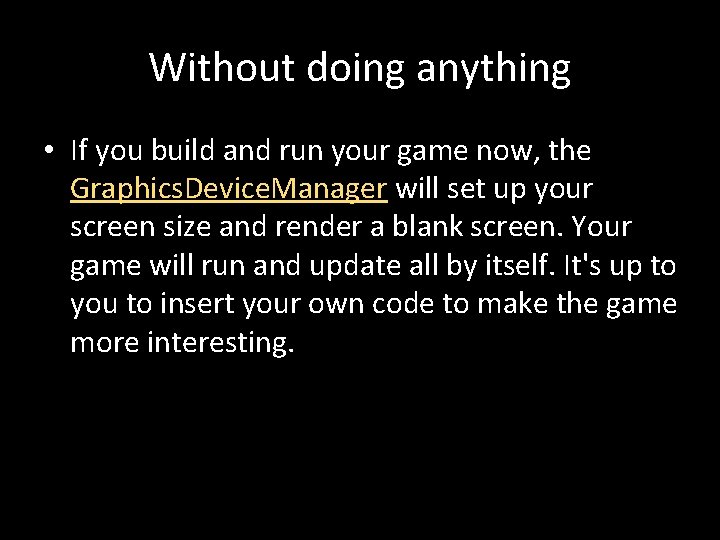
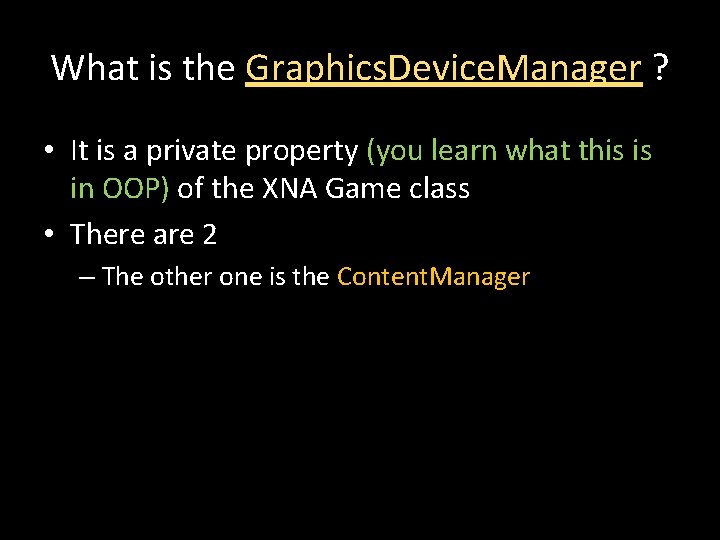
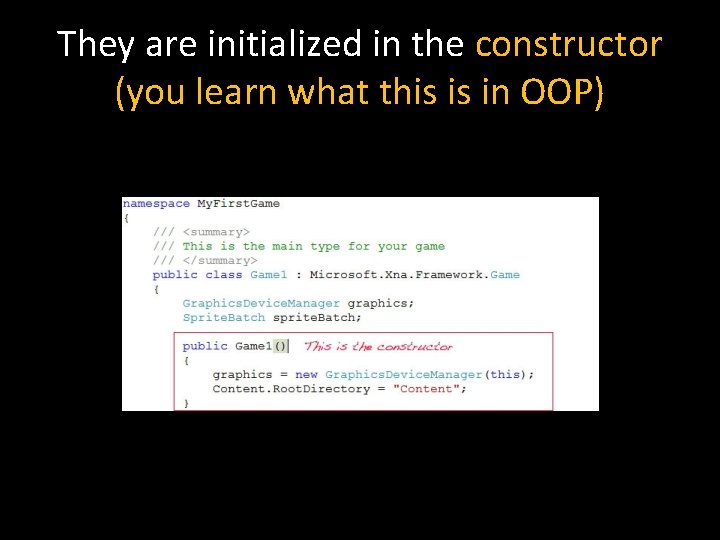
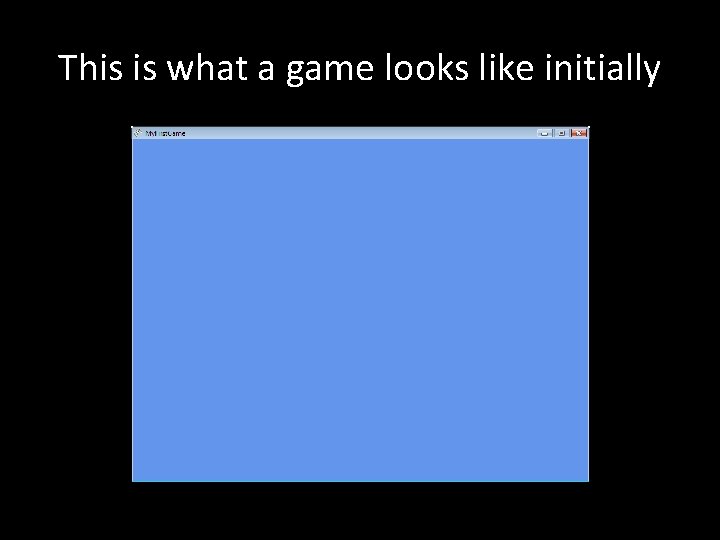
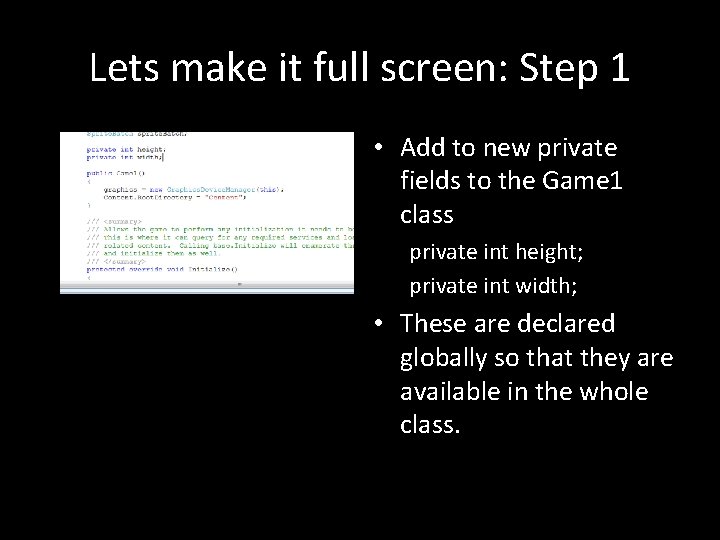
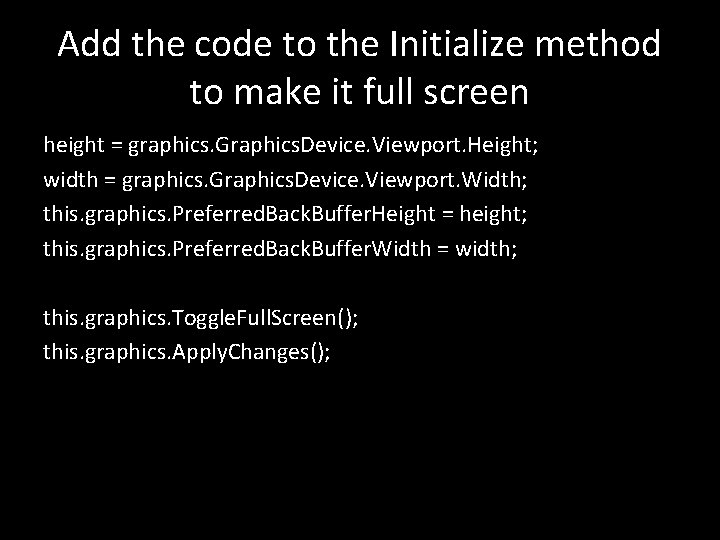
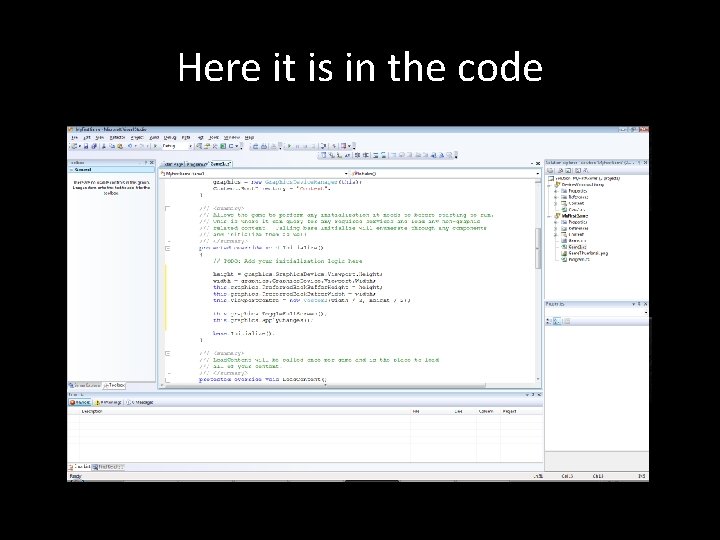
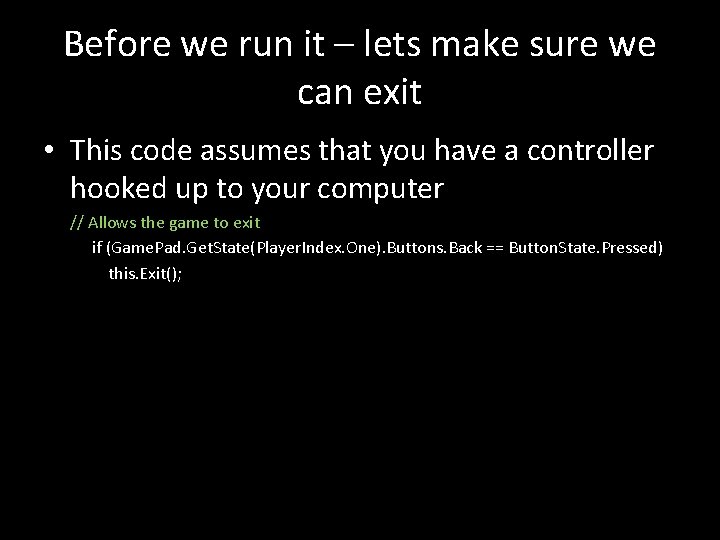
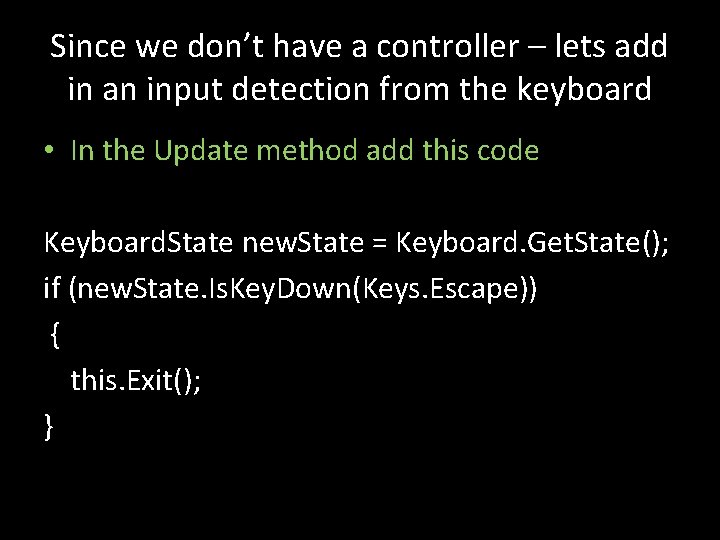
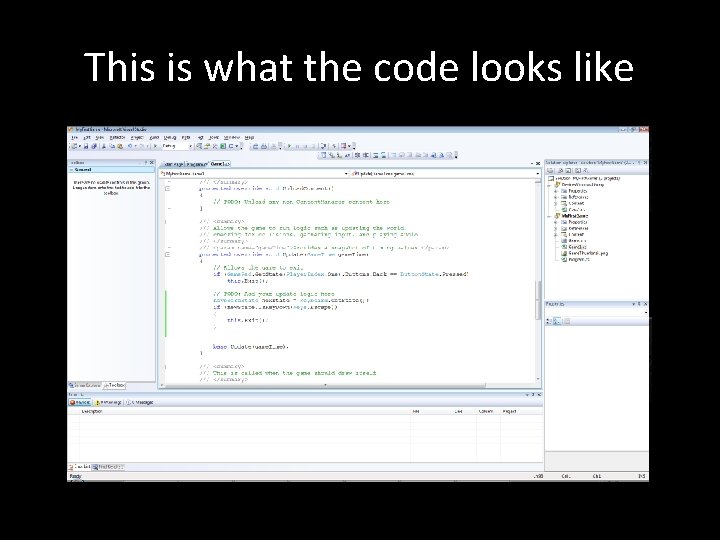
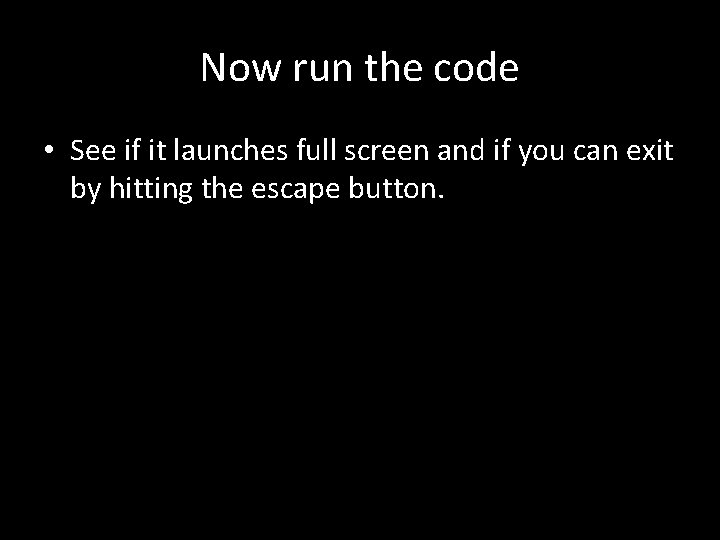
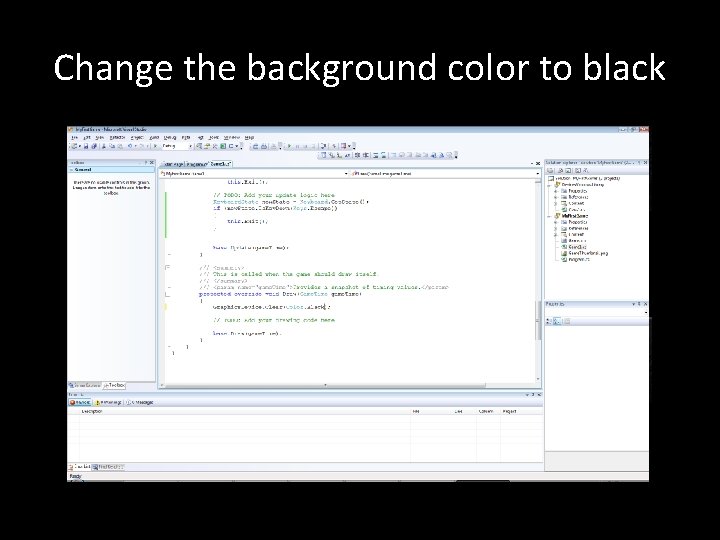
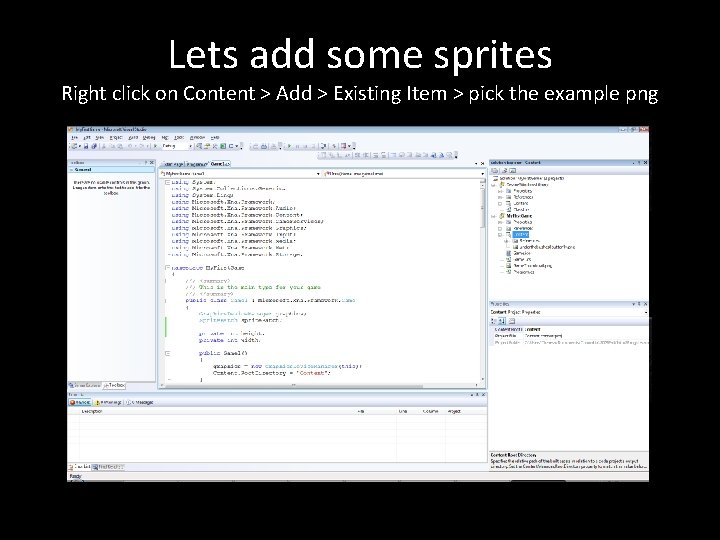
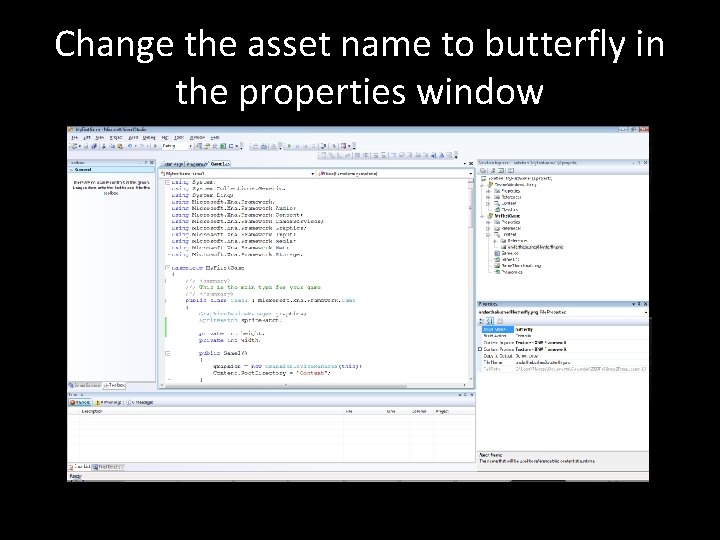
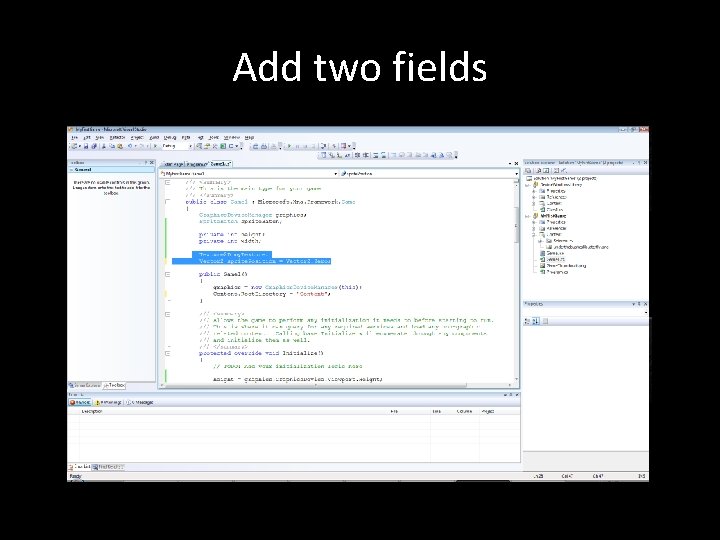
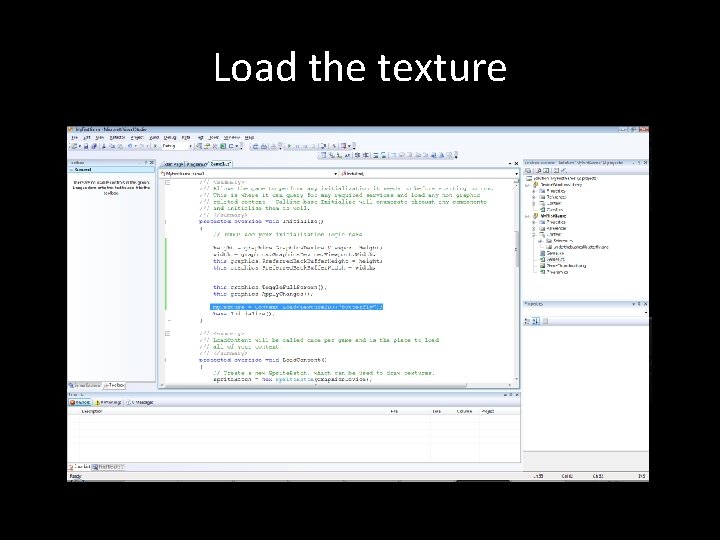
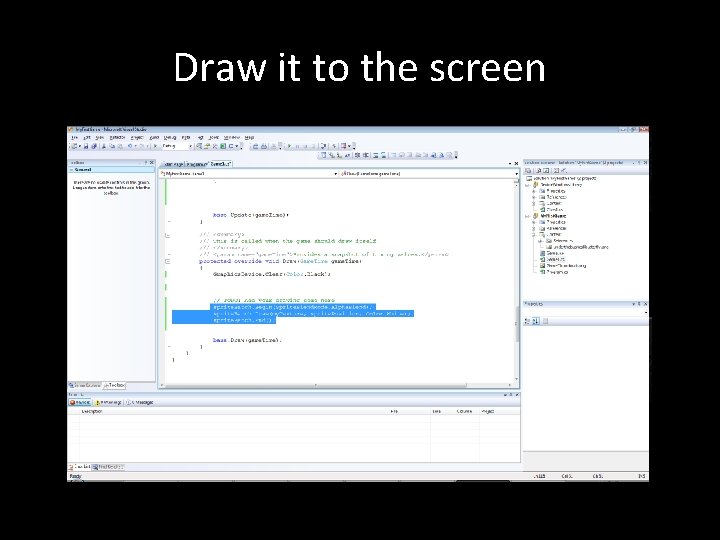
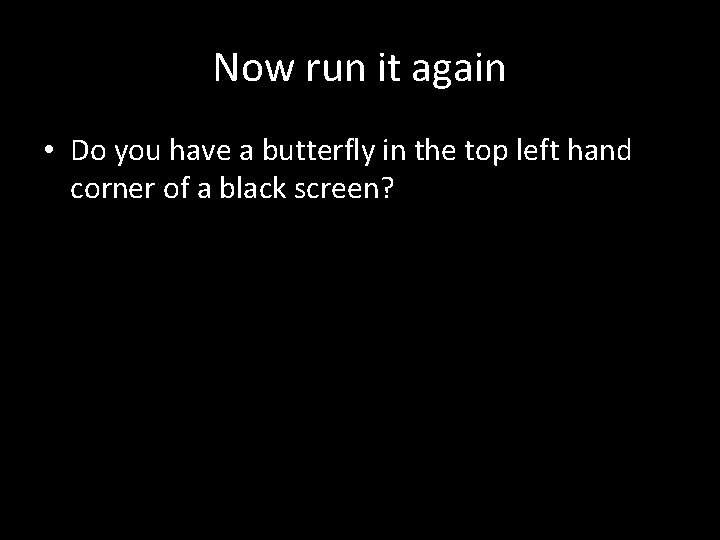
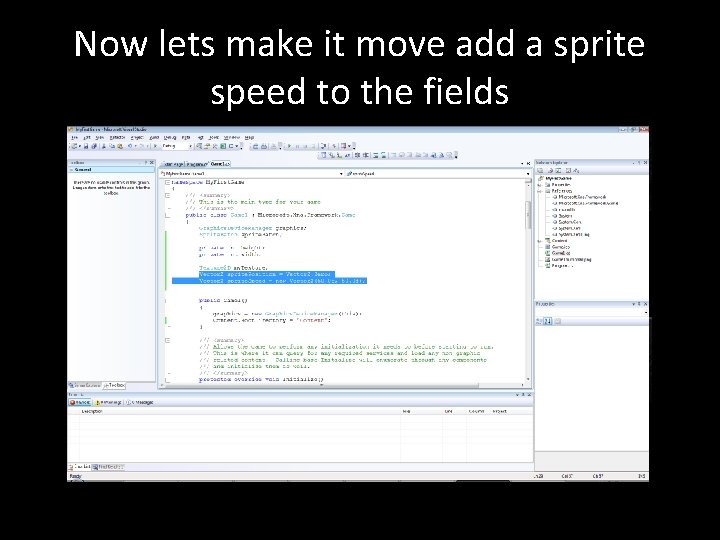
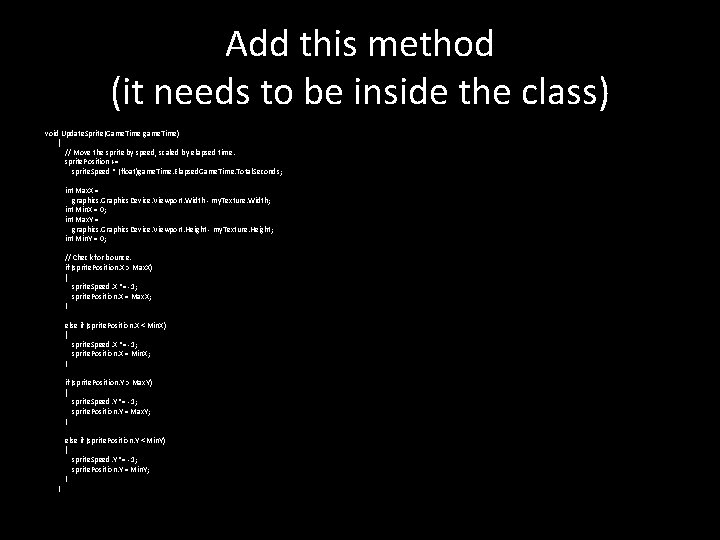
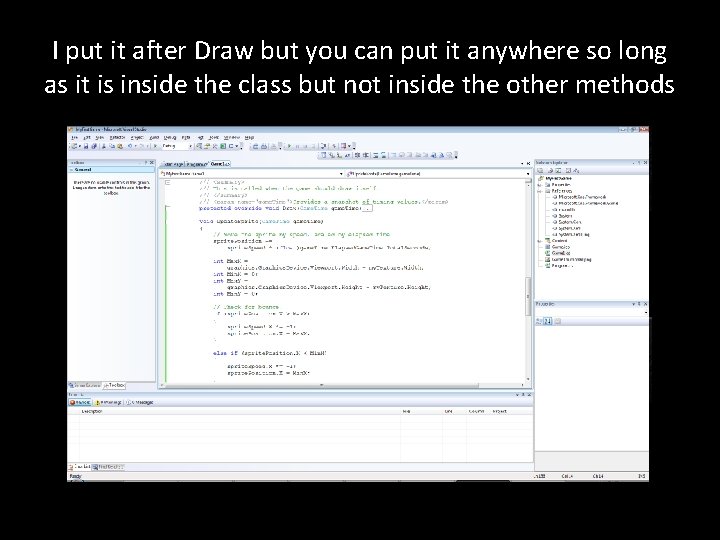
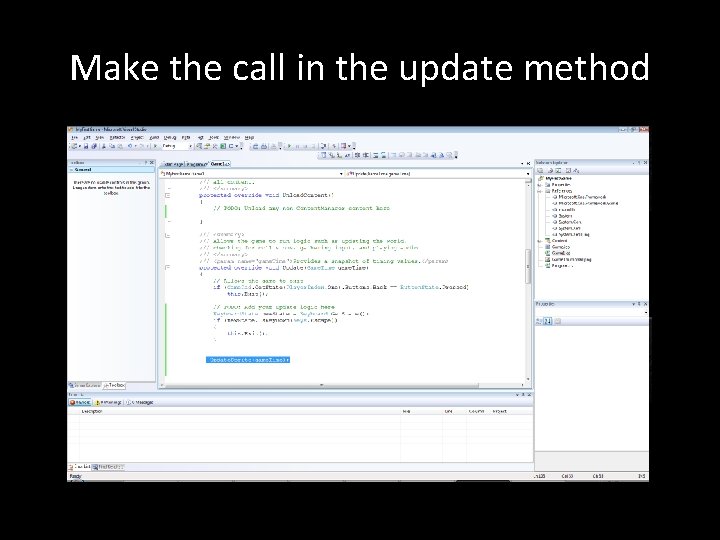
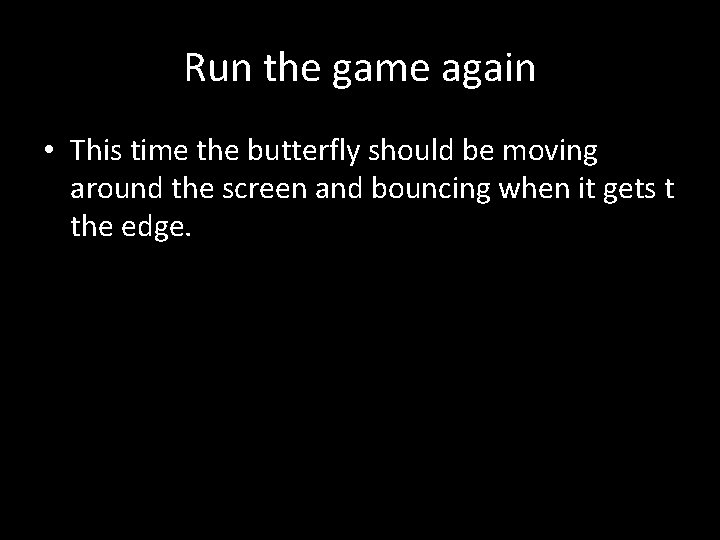
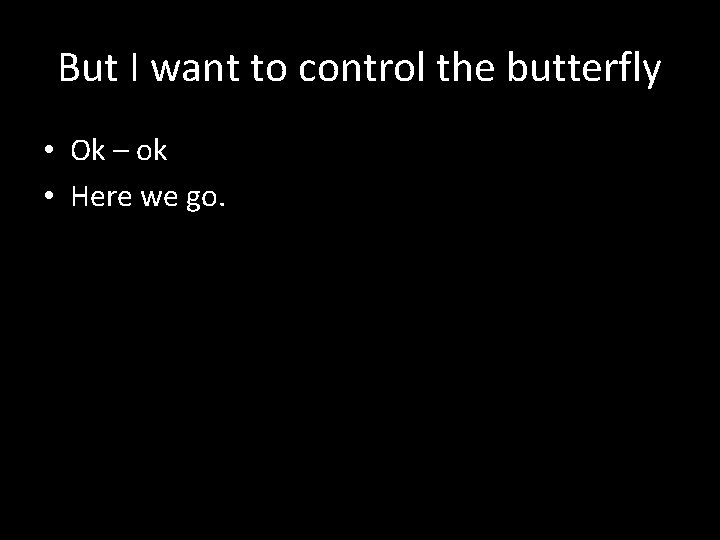
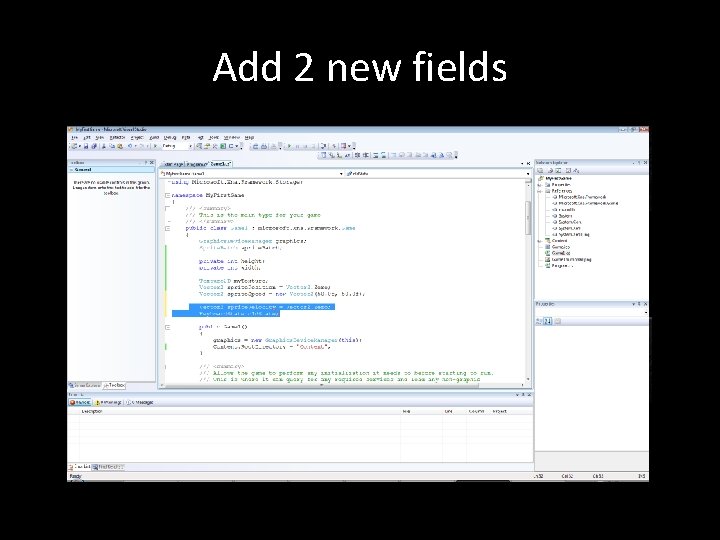
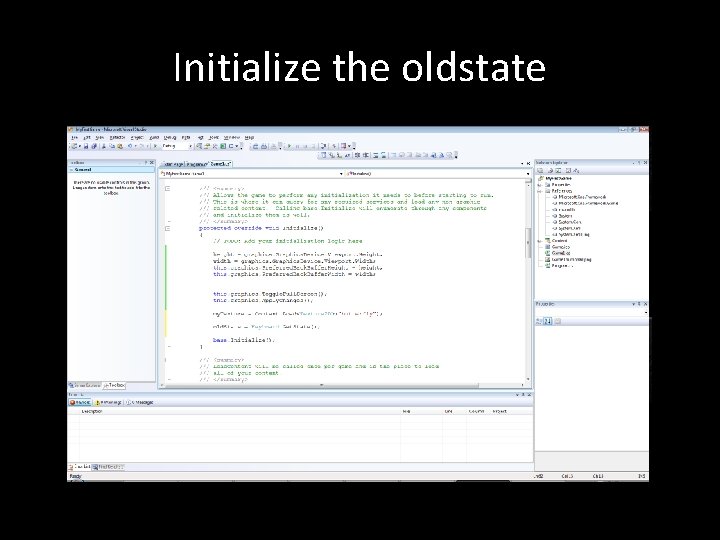
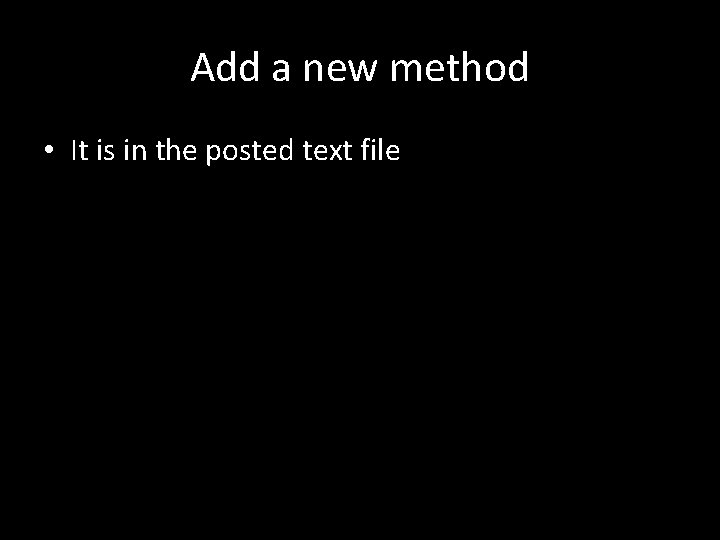
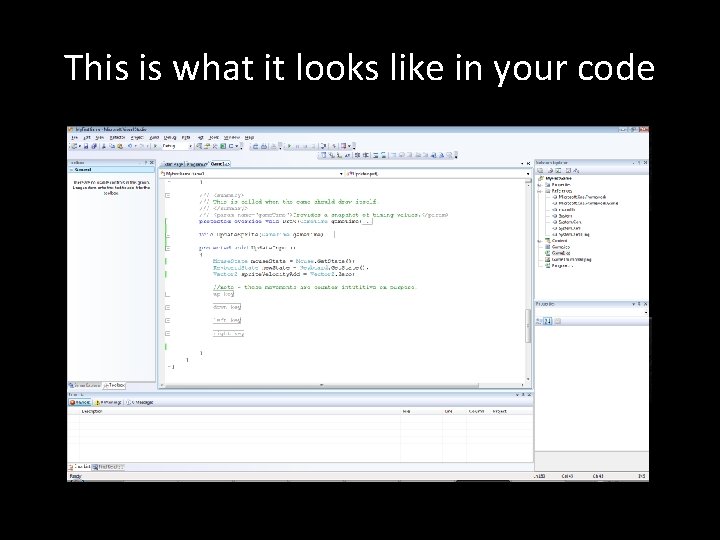
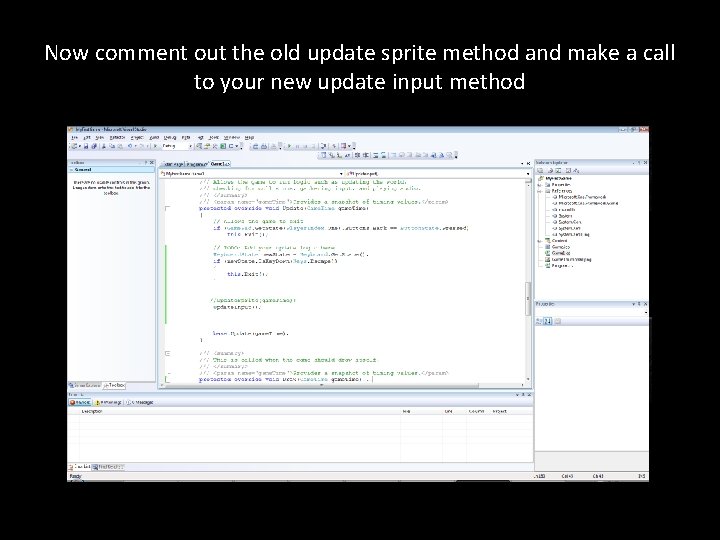
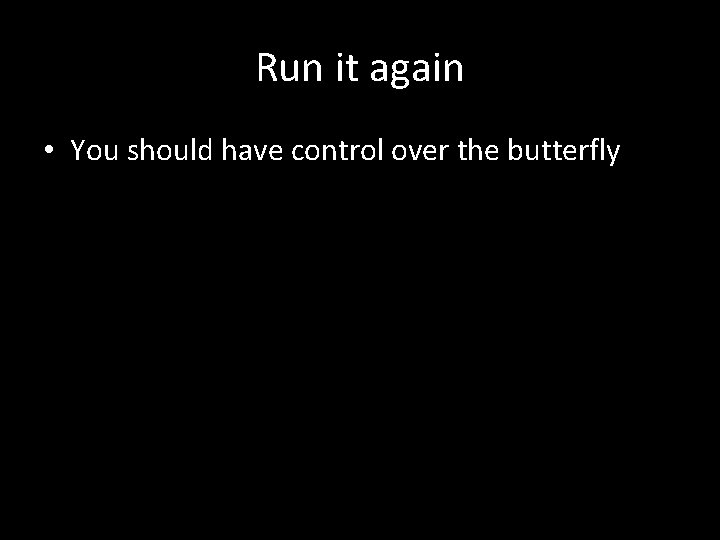
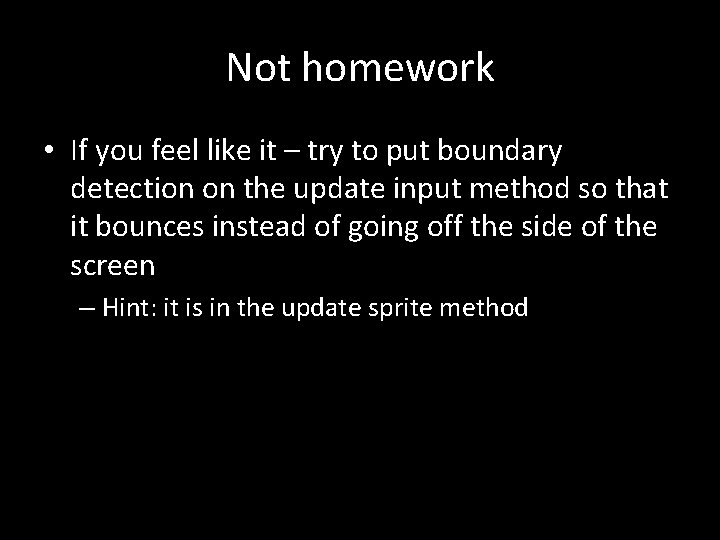
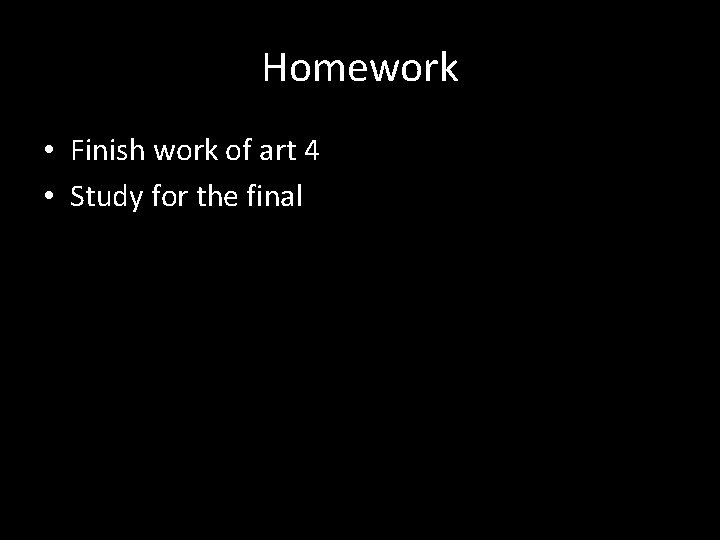
- Slides: 42
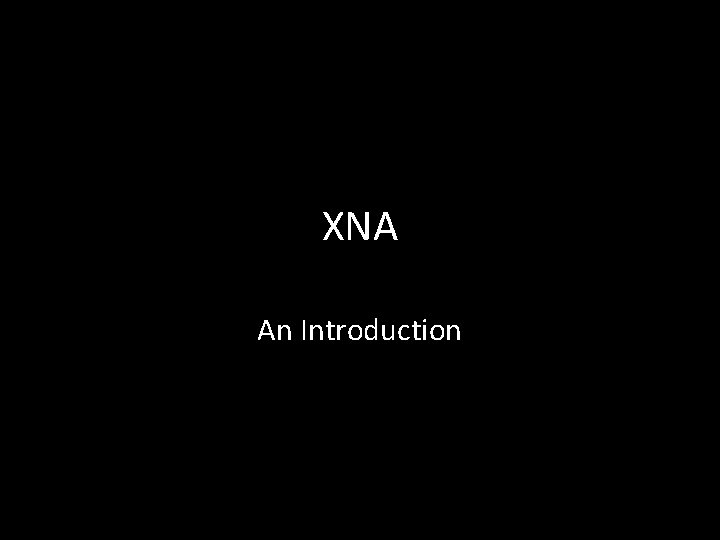
XNA An Introduction
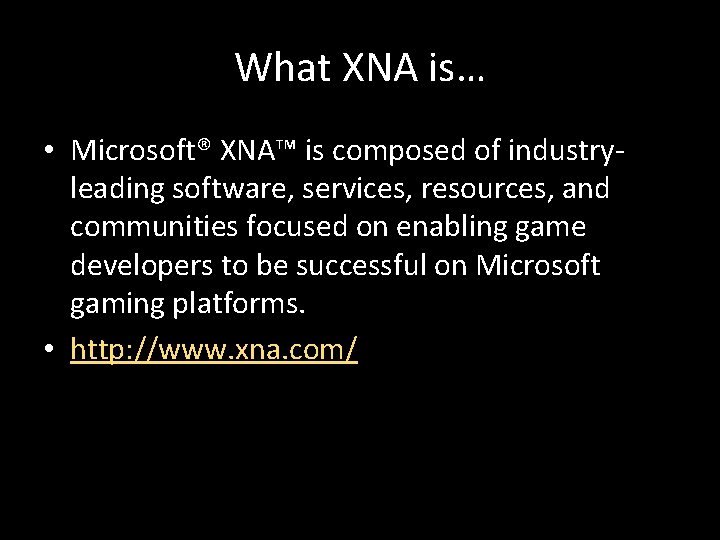
What XNA is… • Microsoft® XNA™ is composed of industryleading software, services, resources, and communities focused on enabling game developers to be successful on Microsoft gaming platforms. • http: //www. xna. com/
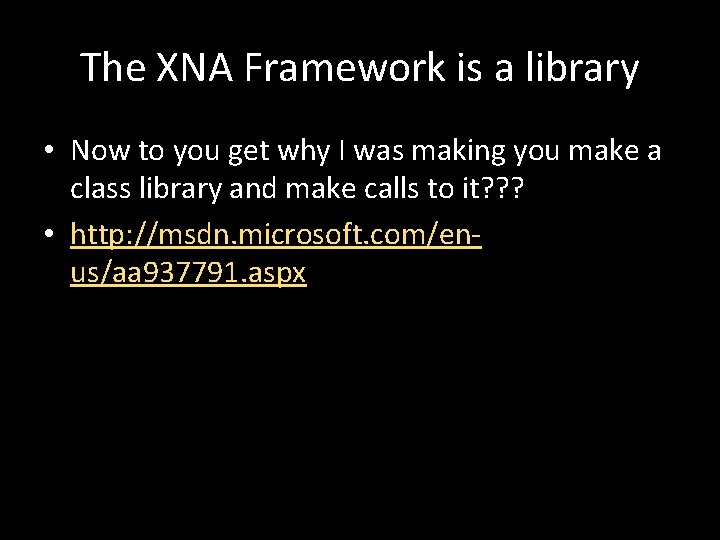
The XNA Framework is a library • Now to you get why I was making you make a class library and make calls to it? ? ? • http: //msdn. microsoft. com/enus/aa 937791. aspx
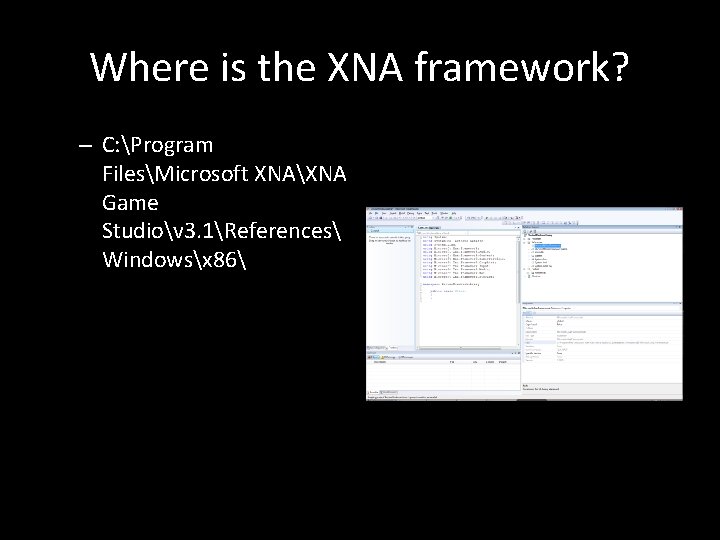
Where is the XNA framework? – C: Program FilesMicrosoft XNAXNA Game Studiov 3. 1References Windowsx 86
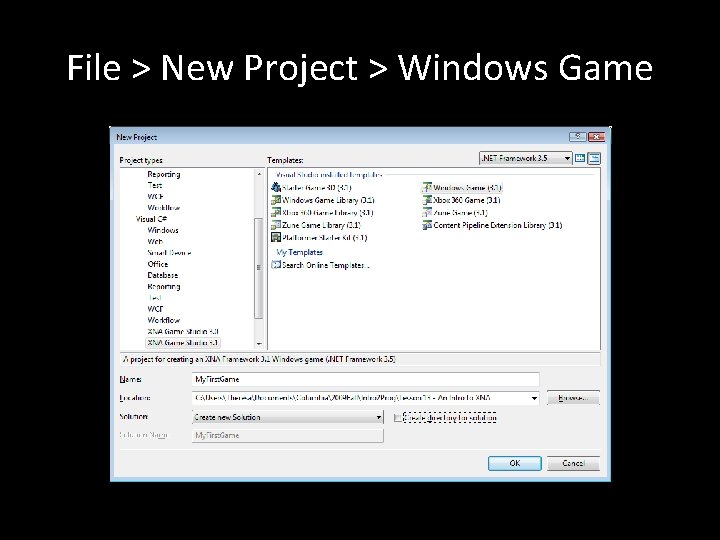
File > New Project > Windows Game
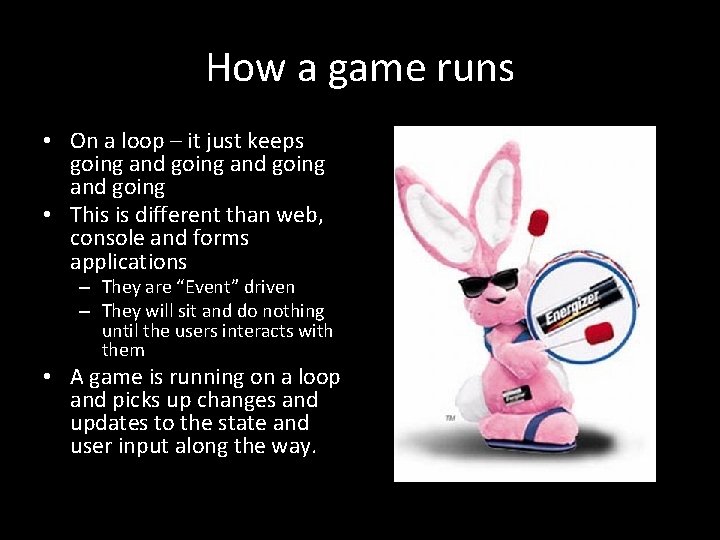
How a game runs • On a loop – it just keeps going and going • This is different than web, console and forms applications – They are “Event” driven – They will sit and do nothing until the users interacts with them • A game is running on a loop and picks up changes and updates to the state and user input along the way.
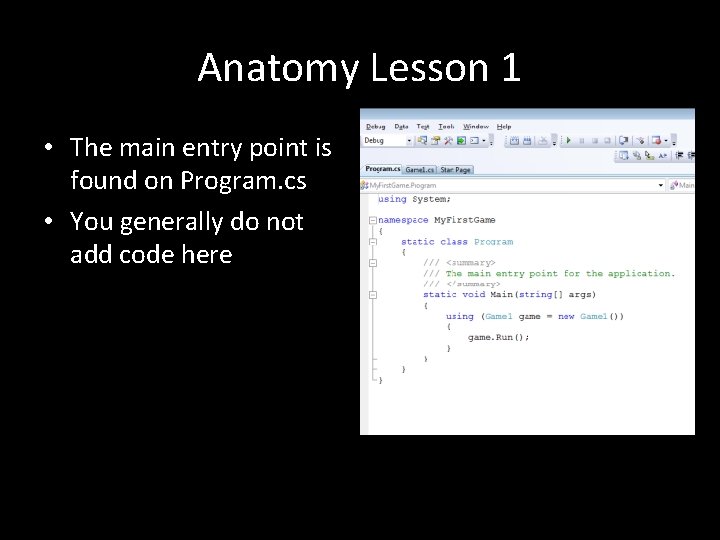
Anatomy Lesson 1 • The main entry point is found on Program. cs • You generally do not add code here
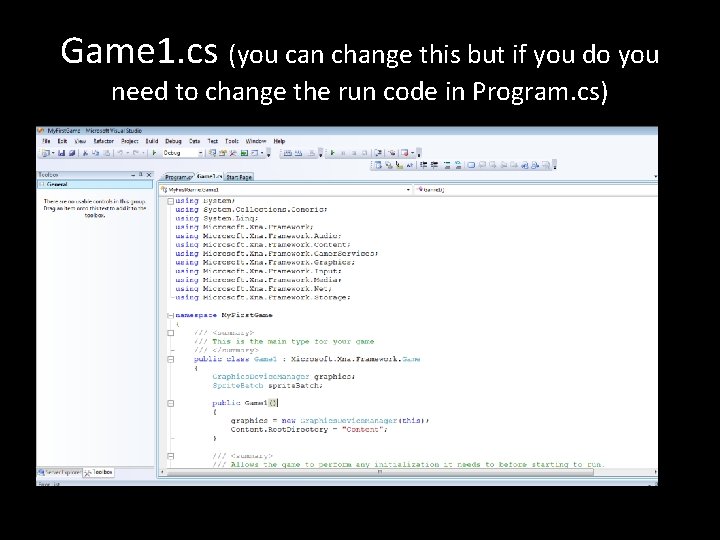
Game 1. cs (you can change this but if you do you need to change the run code in Program. cs)
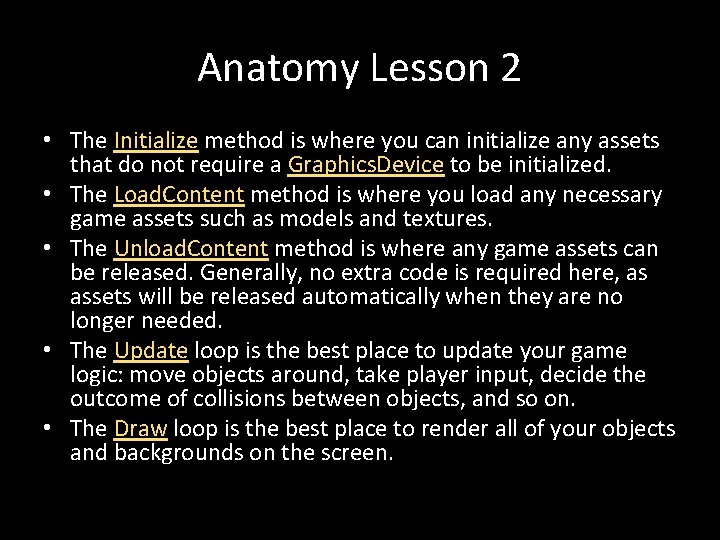
Anatomy Lesson 2 • The Initialize method is where you can initialize any assets that do not require a Graphics. Device to be initialized. • The Load. Content method is where you load any necessary game assets such as models and textures. • The Unload. Content method is where any game assets can be released. Generally, no extra code is required here, as assets will be released automatically when they are no longer needed. • The Update loop is the best place to update your game logic: move objects around, take player input, decide the outcome of collisions between objects, and so on. • The Draw loop is the best place to render all of your objects and backgrounds on the screen.
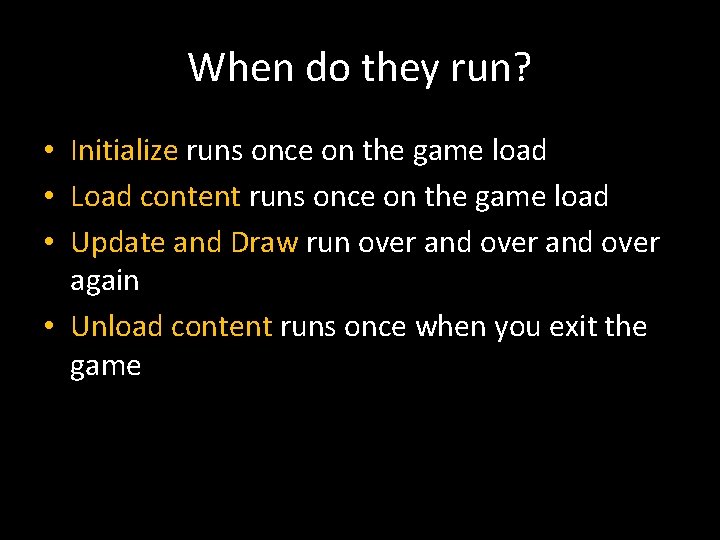
When do they run? • Initialize runs once on the game load • Load content runs once on the game load • Update and Draw run over and over again • Unload content runs once when you exit the game
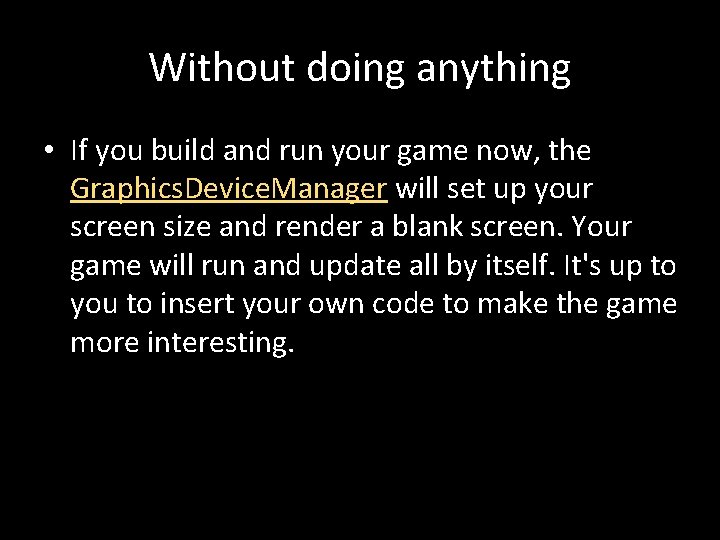
Without doing anything • If you build and run your game now, the Graphics. Device. Manager will set up your screen size and render a blank screen. Your game will run and update all by itself. It's up to you to insert your own code to make the game more interesting.
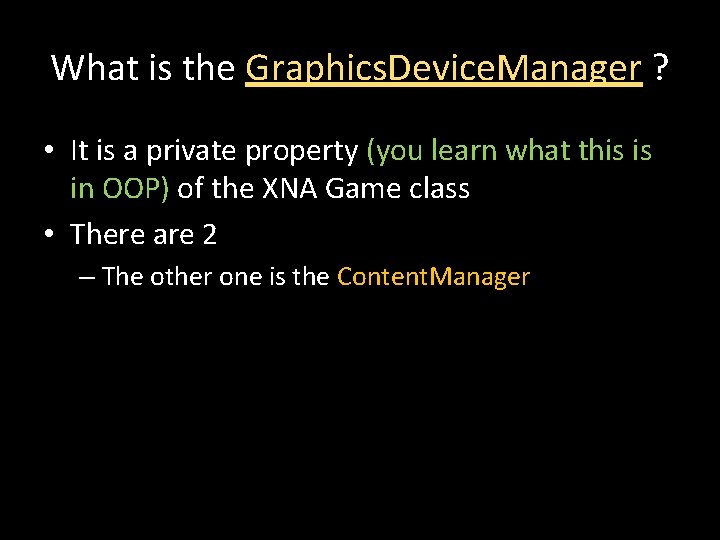
What is the Graphics. Device. Manager ? • It is a private property (you learn what this is in OOP) of the XNA Game class • There are 2 – The other one is the Content. Manager
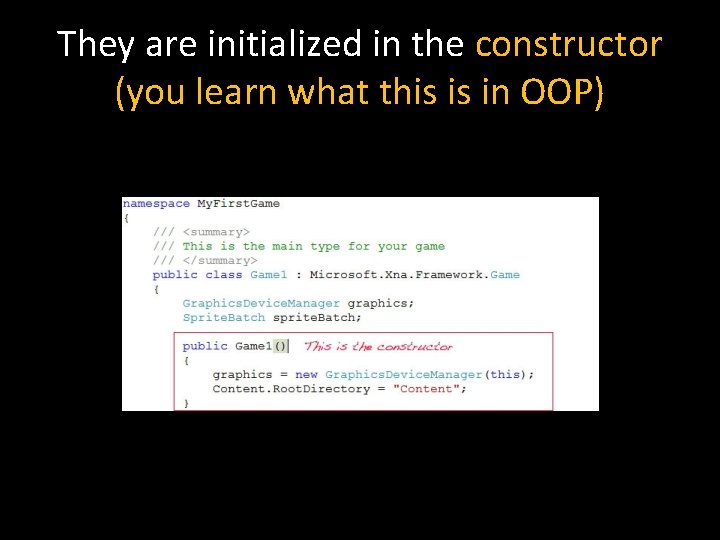
They are initialized in the constructor (you learn what this is in OOP)
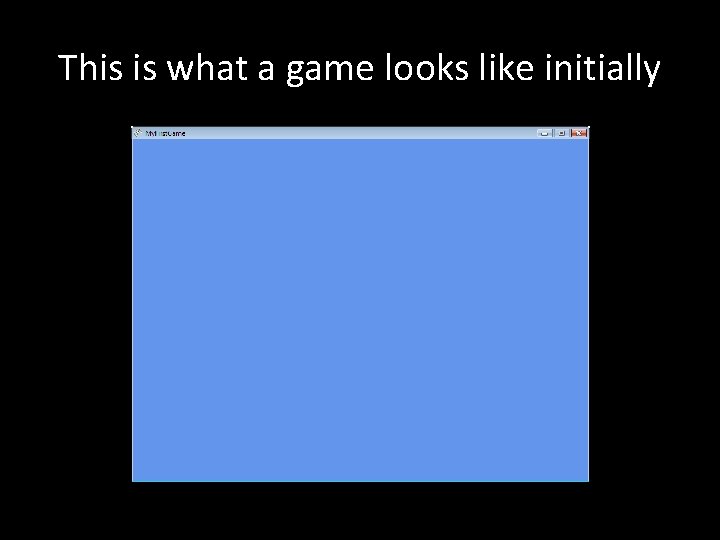
This is what a game looks like initially
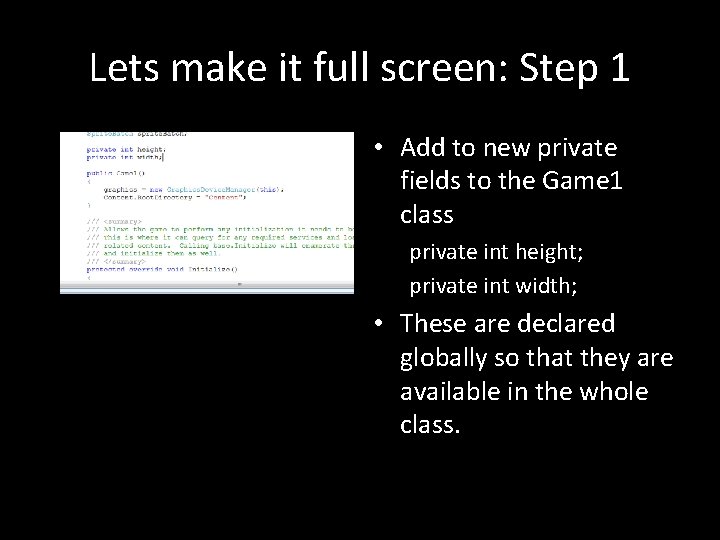
Lets make it full screen: Step 1 • Add to new private fields to the Game 1 class private int height; private int width; • These are declared globally so that they are available in the whole class.
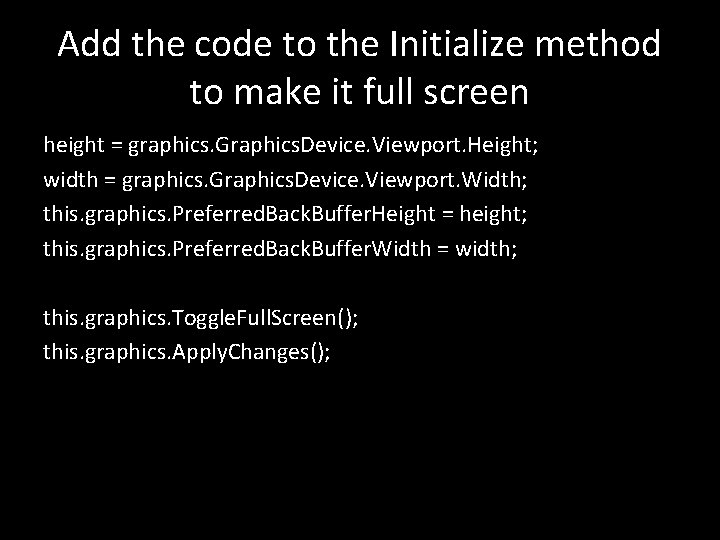
Add the code to the Initialize method to make it full screen height = graphics. Graphics. Device. Viewport. Height; width = graphics. Graphics. Device. Viewport. Width; this. graphics. Preferred. Back. Buffer. Height = height; this. graphics. Preferred. Back. Buffer. Width = width; this. graphics. Toggle. Full. Screen(); this. graphics. Apply. Changes();
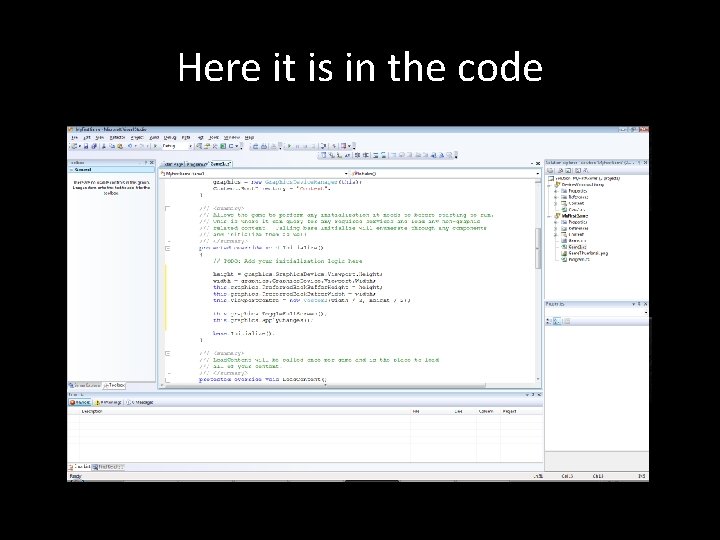
Here it is in the code
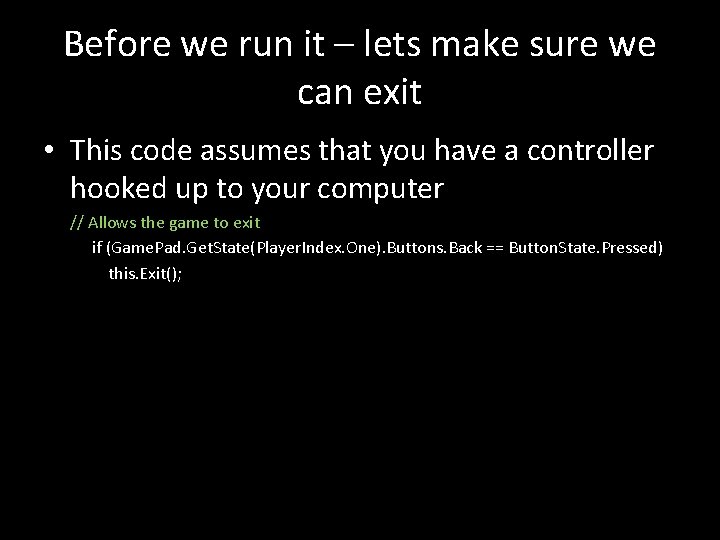
Before we run it – lets make sure we can exit • This code assumes that you have a controller hooked up to your computer // Allows the game to exit if (Game. Pad. Get. State(Player. Index. One). Buttons. Back == Button. State. Pressed) this. Exit();
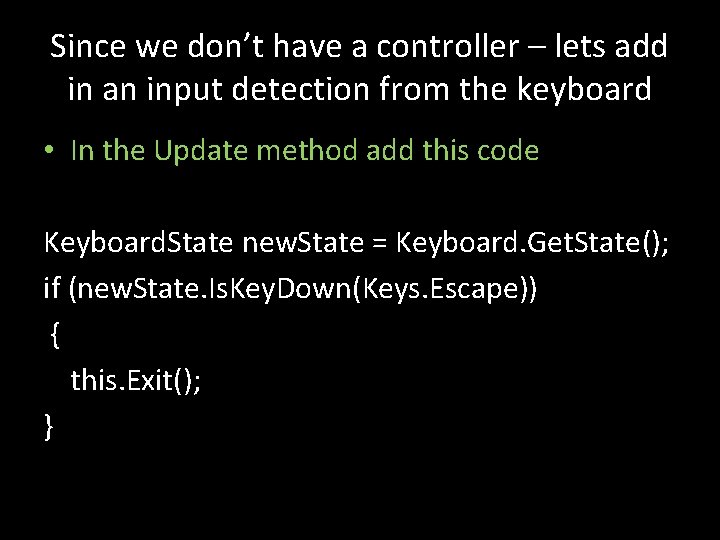
Since we don’t have a controller – lets add in an input detection from the keyboard • In the Update method add this code Keyboard. State new. State = Keyboard. Get. State(); if (new. State. Is. Key. Down(Keys. Escape)) { this. Exit(); }
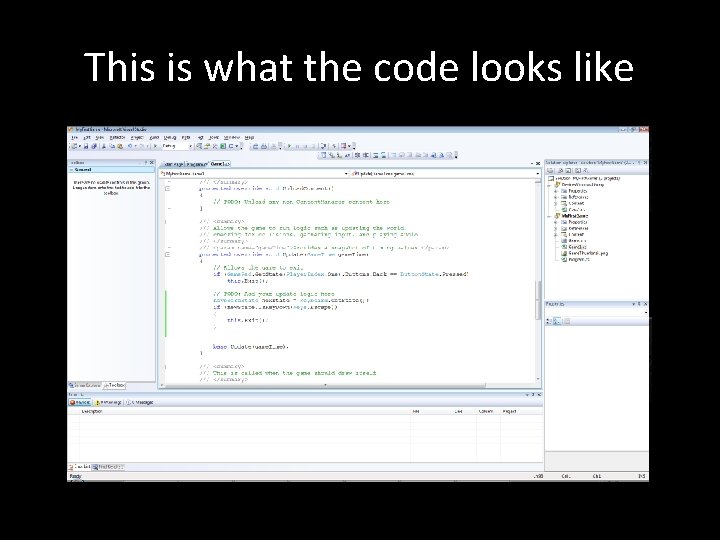
This is what the code looks like
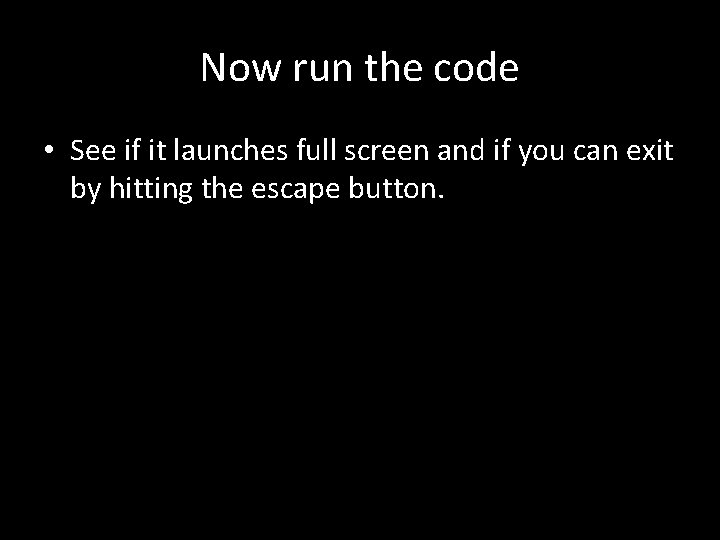
Now run the code • See if it launches full screen and if you can exit by hitting the escape button.
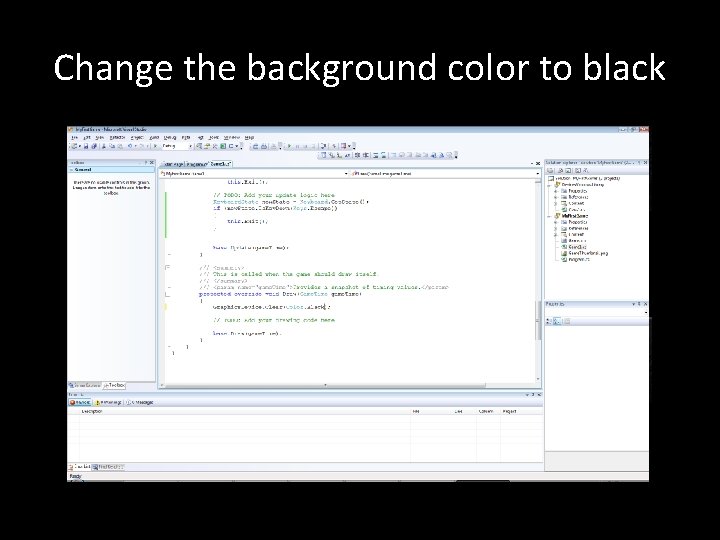
Change the background color to black
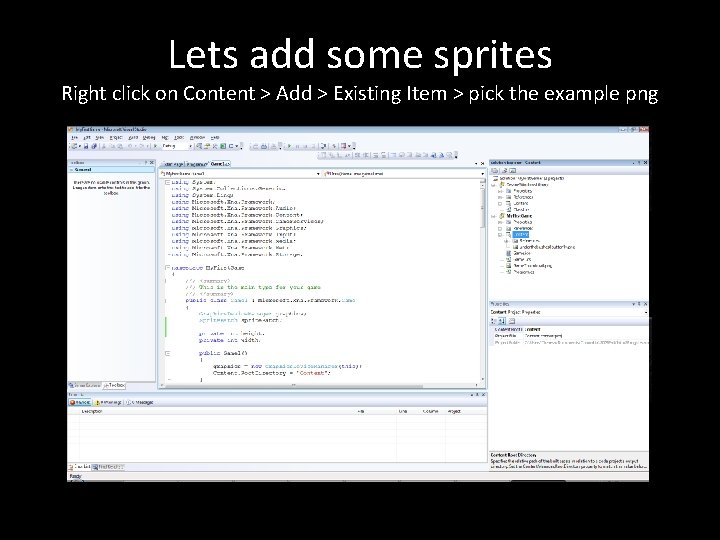
Lets add some sprites Right click on Content > Add > Existing Item > pick the example png
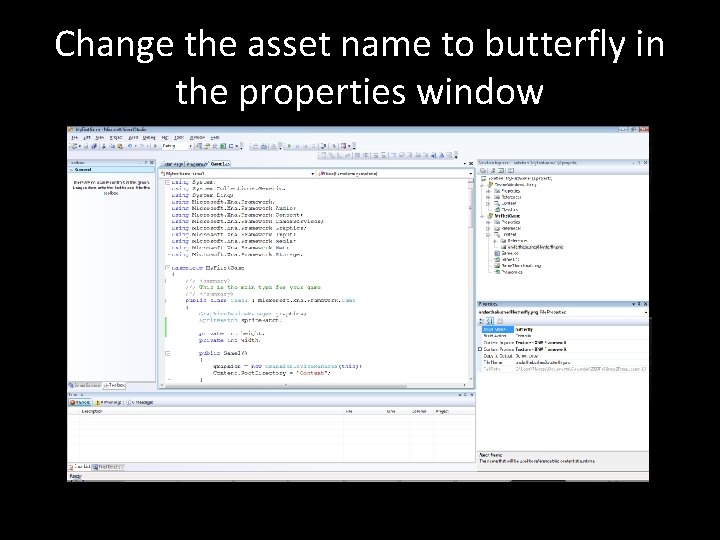
Change the asset name to butterfly in the properties window
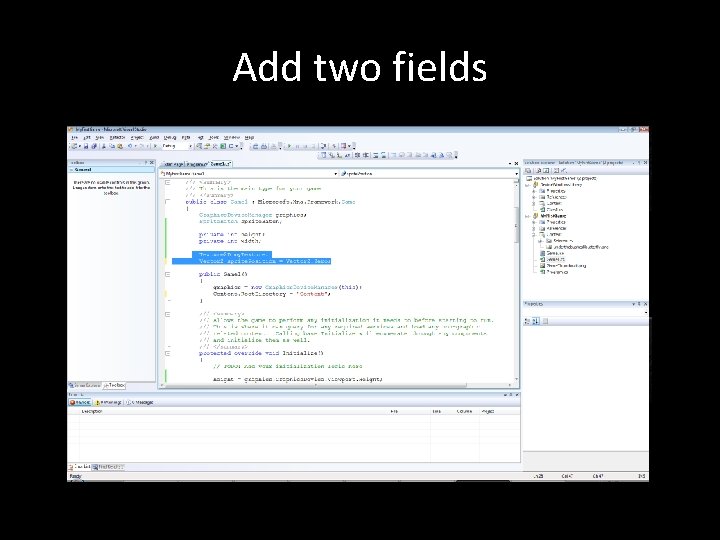
Add two fields
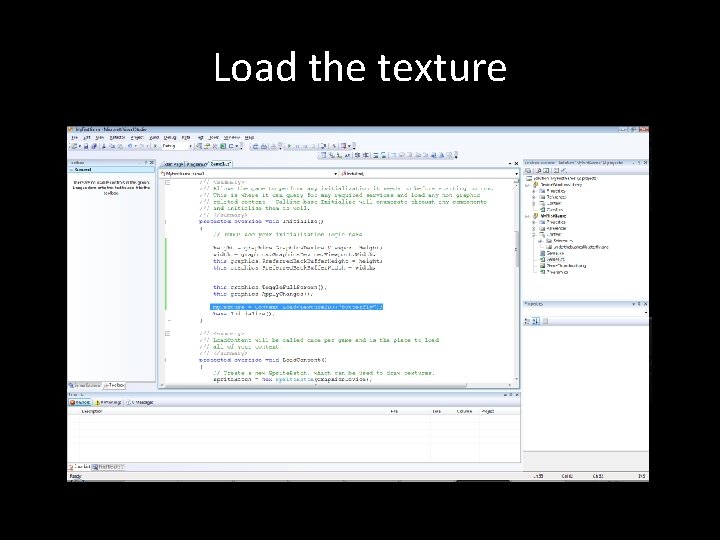
Load the texture
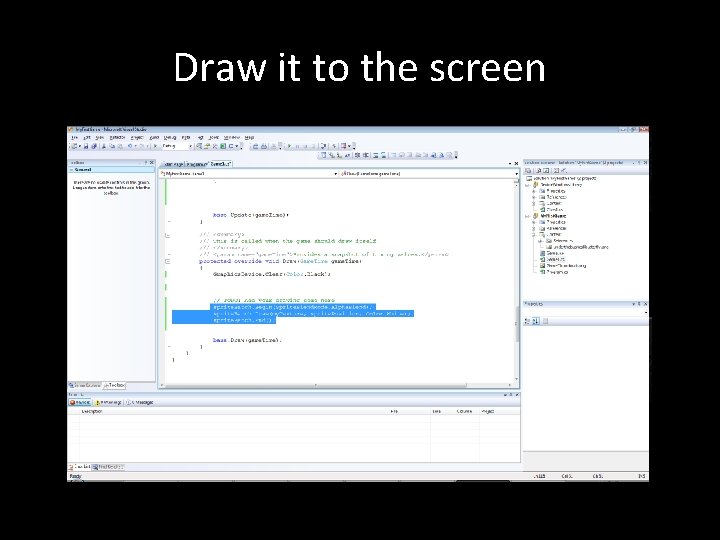
Draw it to the screen
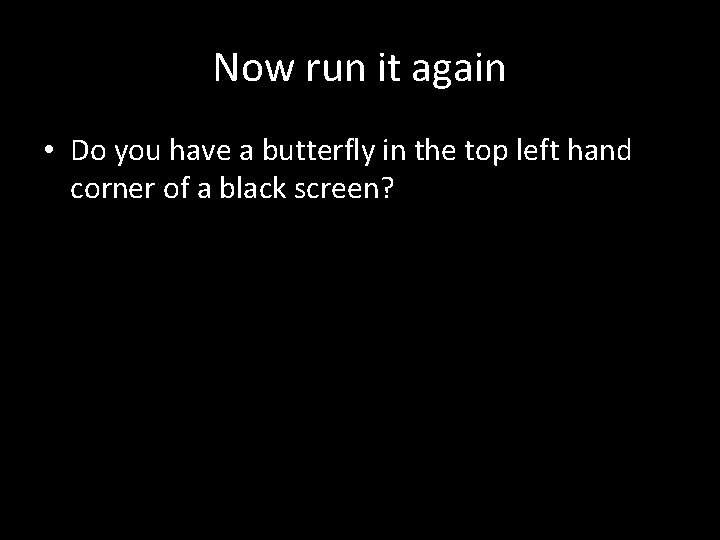
Now run it again • Do you have a butterfly in the top left hand corner of a black screen?
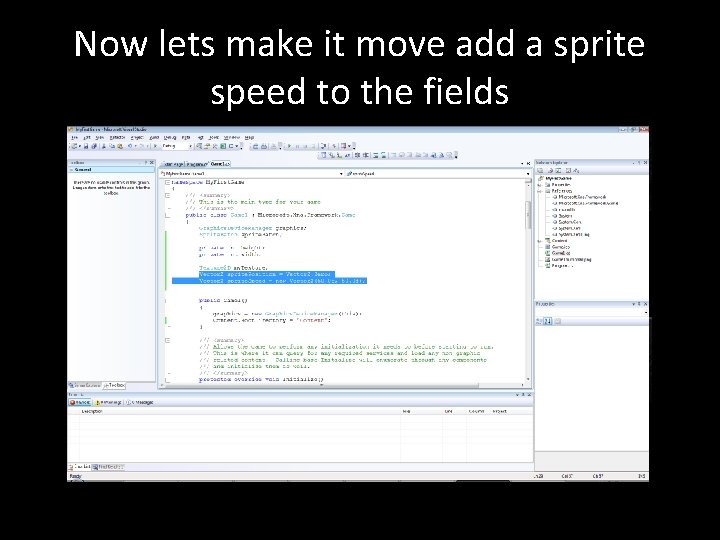
Now lets make it move add a sprite speed to the fields
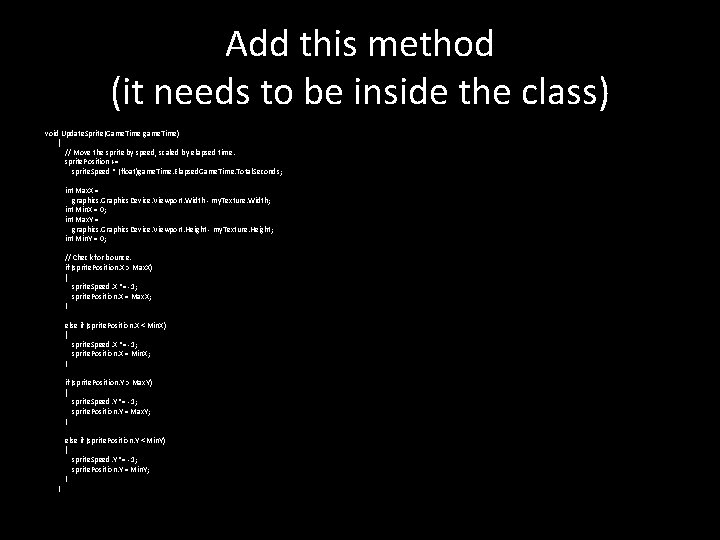
Add this method (it needs to be inside the class) void Update. Sprite(Game. Time game. Time) { // Move the sprite by speed, scaled by elapsed time. sprite. Position += sprite. Speed * (float)game. Time. Elapsed. Game. Time. Total. Seconds; int Max. X = graphics. Graphics. Device. Viewport. Width - my. Texture. Width; int Min. X = 0; int Max. Y = graphics. Graphics. Device. Viewport. Height - my. Texture. Height; int Min. Y = 0; // Check for bounce. if (sprite. Position. X > Max. X) { sprite. Speed. X *= -1; sprite. Position. X = Max. X; } else if (sprite. Position. X < Min. X) { sprite. Speed. X *= -1; sprite. Position. X = Min. X; } if (sprite. Position. Y > Max. Y) { sprite. Speed. Y *= -1; sprite. Position. Y = Max. Y; } } else if (sprite. Position. Y < Min. Y) { sprite. Speed. Y *= -1; sprite. Position. Y = Min. Y; }
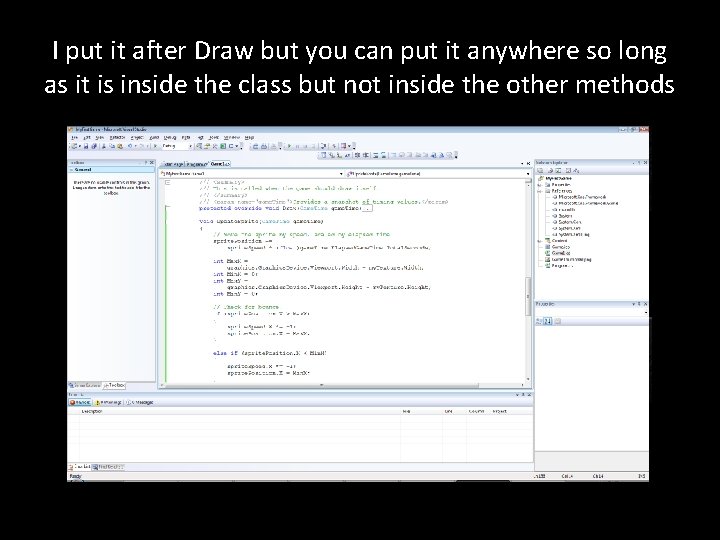
I put it after Draw but you can put it anywhere so long as it is inside the class but not inside the other methods
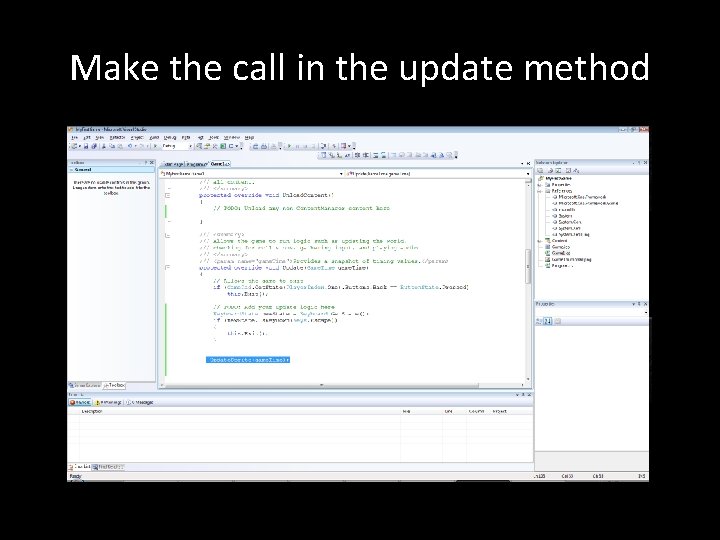
Make the call in the update method
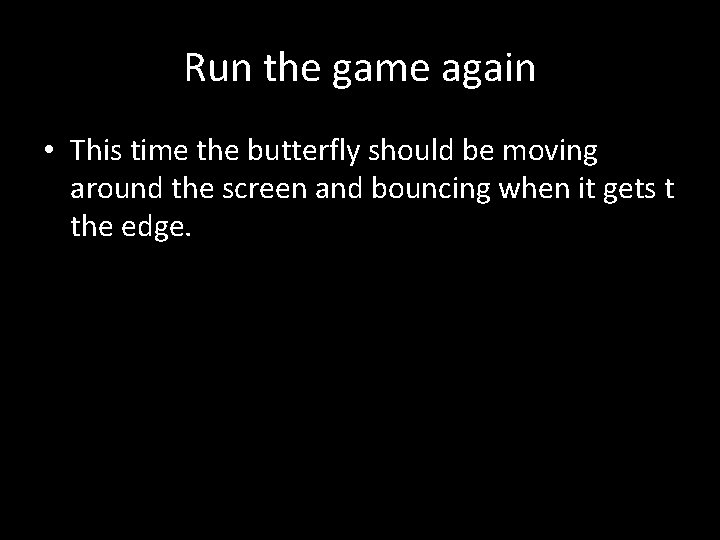
Run the game again • This time the butterfly should be moving around the screen and bouncing when it gets t the edge.
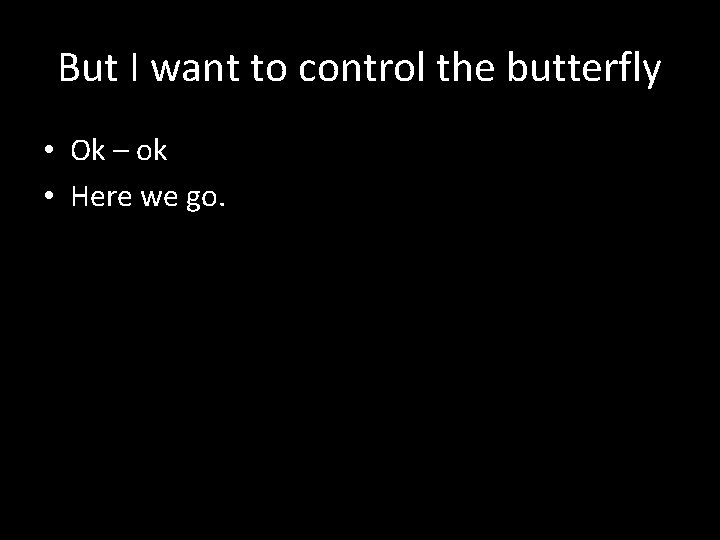
But I want to control the butterfly • Ok – ok • Here we go.
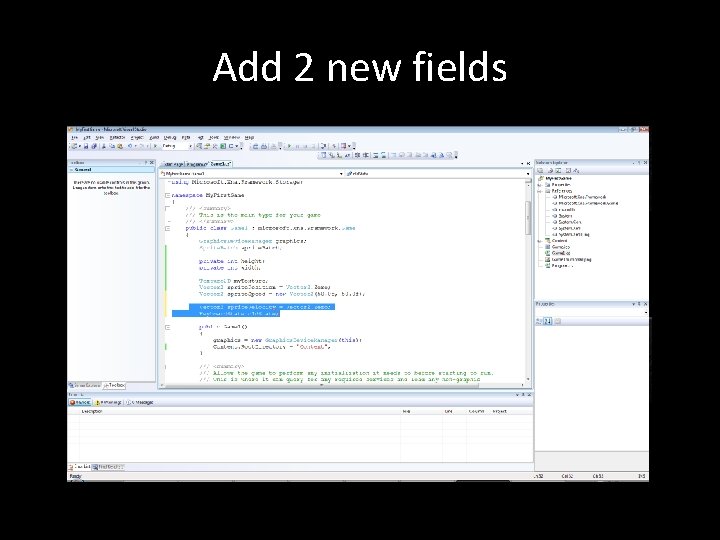
Add 2 new fields
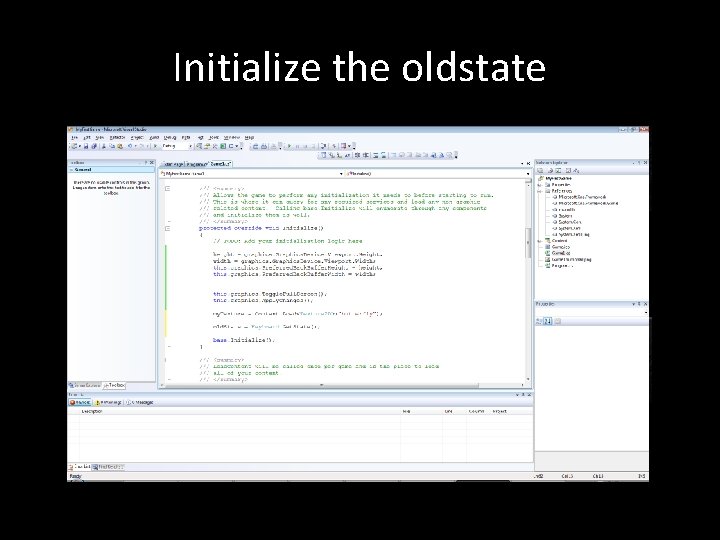
Initialize the oldstate
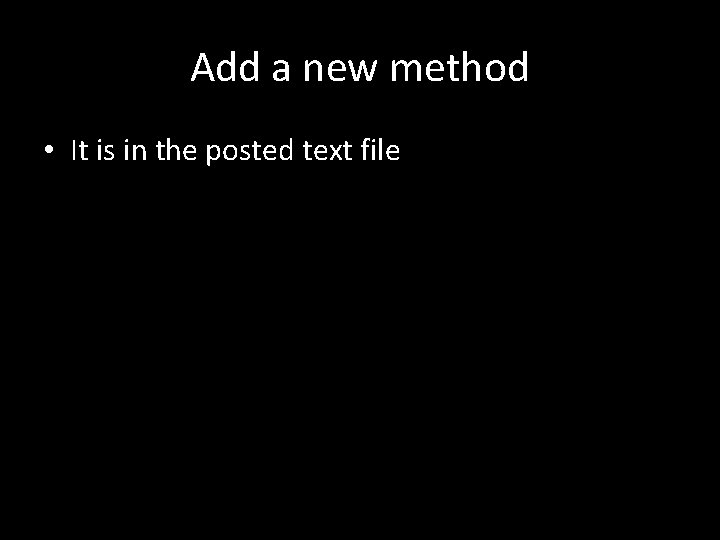
Add a new method • It is in the posted text file
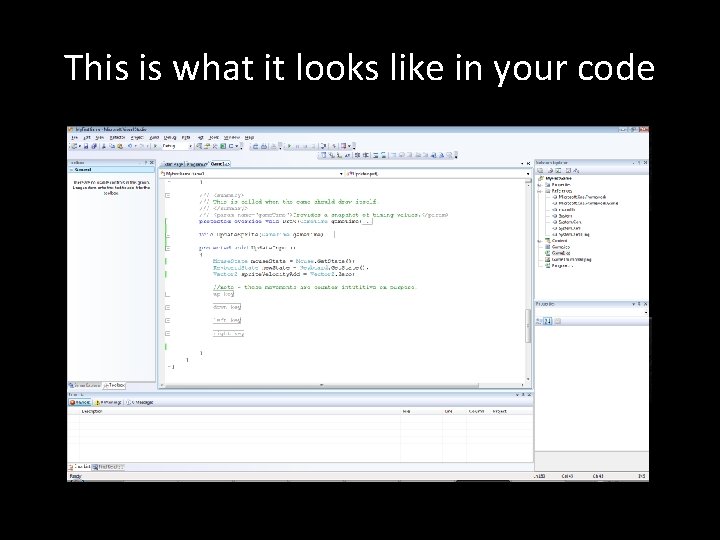
This is what it looks like in your code
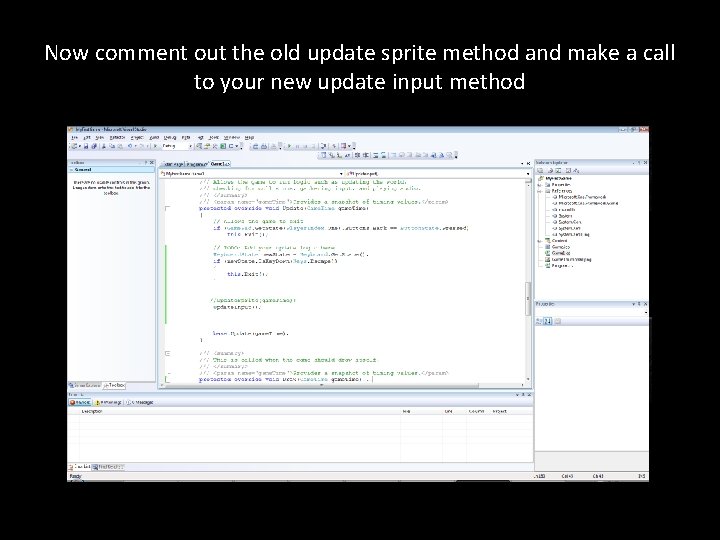
Now comment out the old update sprite method and make a call to your new update input method
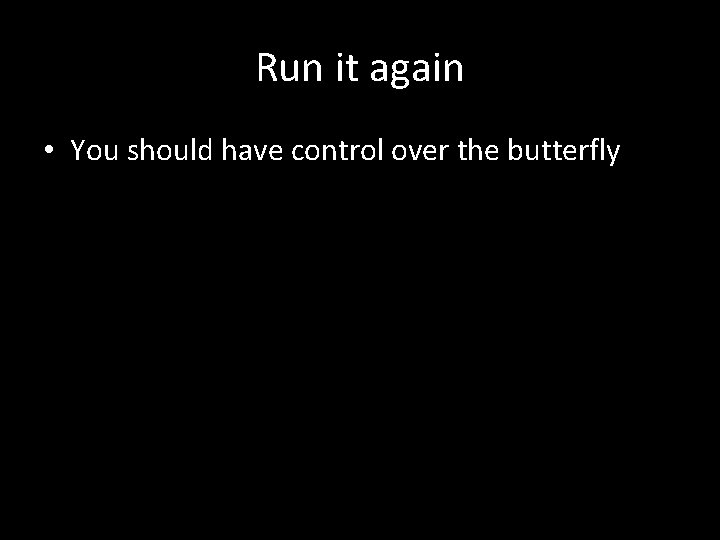
Run it again • You should have control over the butterfly
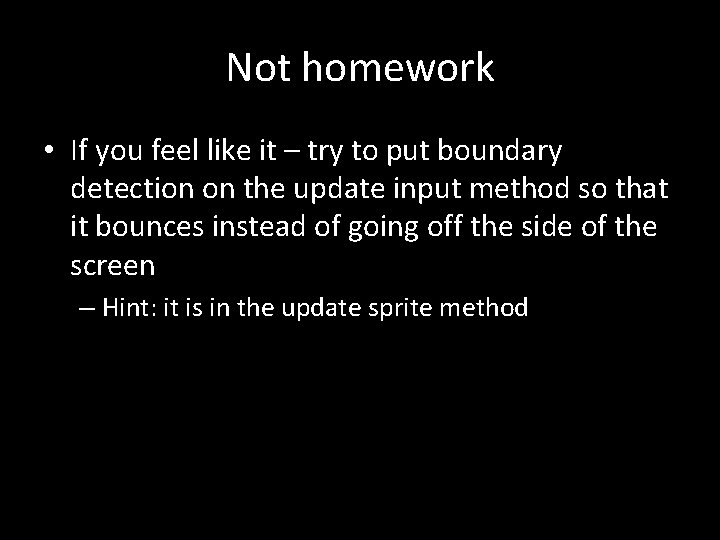
Not homework • If you feel like it – try to put boundary detection on the update input method so that it bounces instead of going off the side of the screen – Hint: it is in the update sprite method
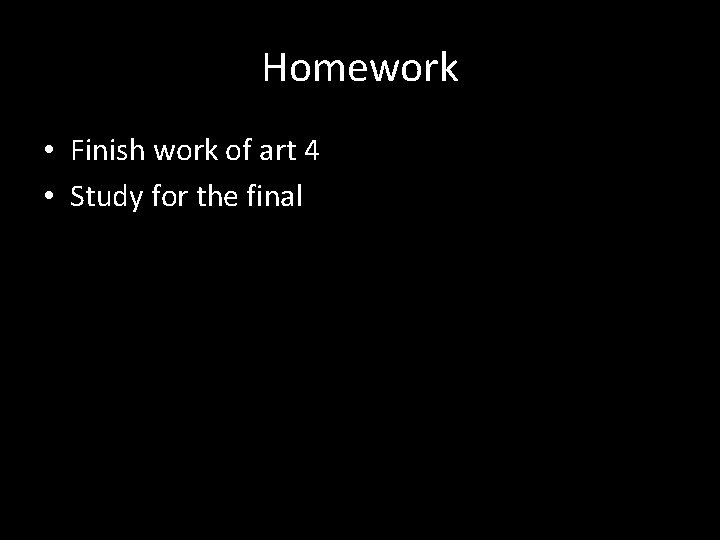
Homework • Finish work of art 4 • Study for the final