www site uottawa caelsaddik CSI 1102 Introduction to
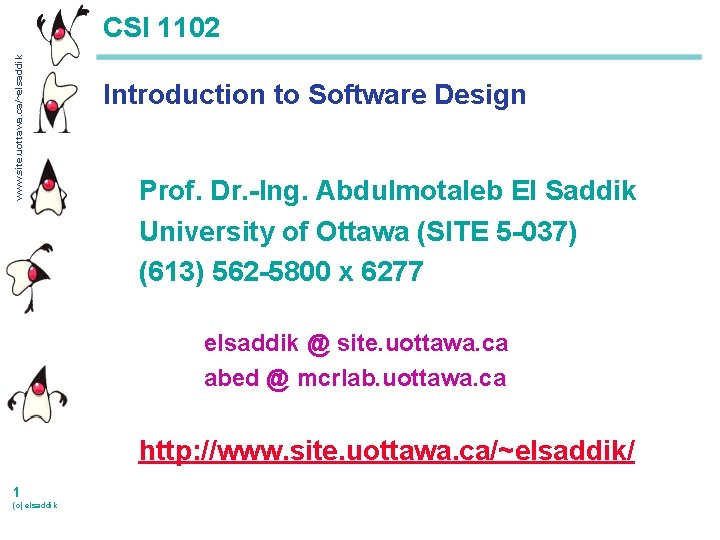
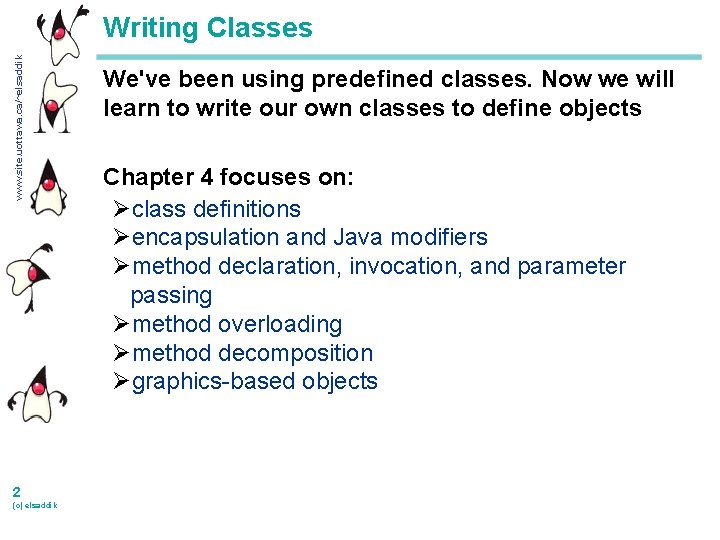
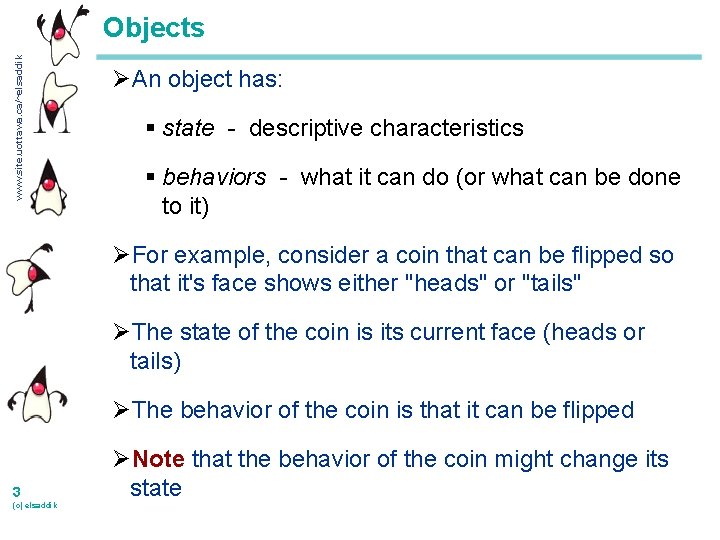
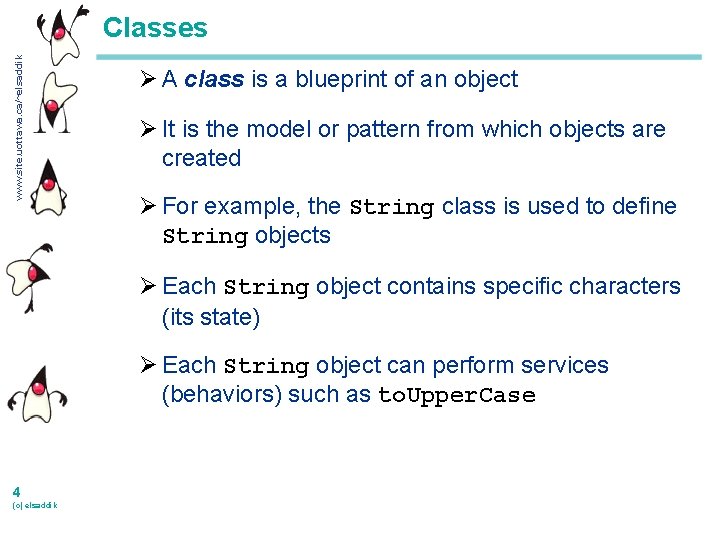
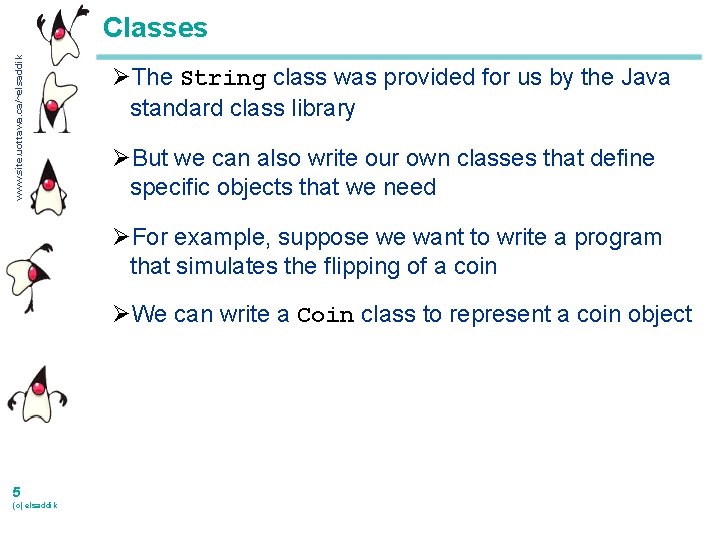
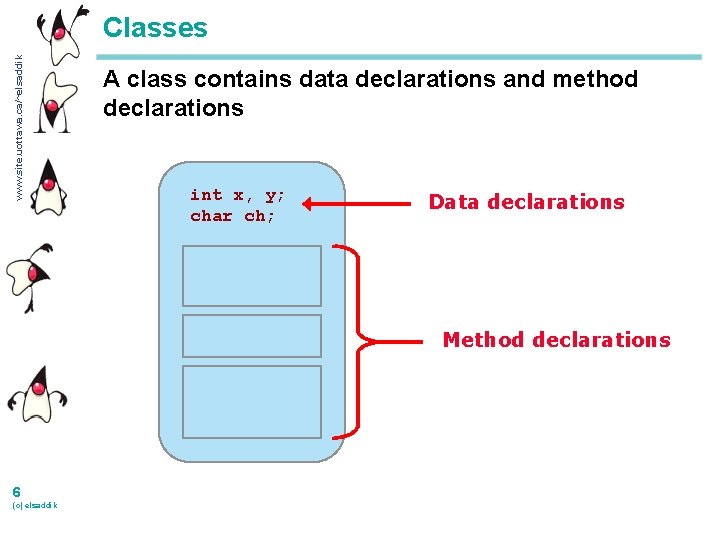
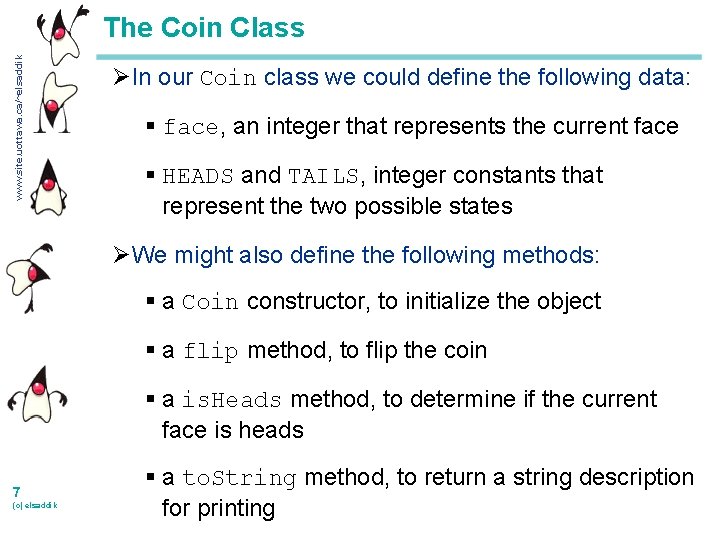
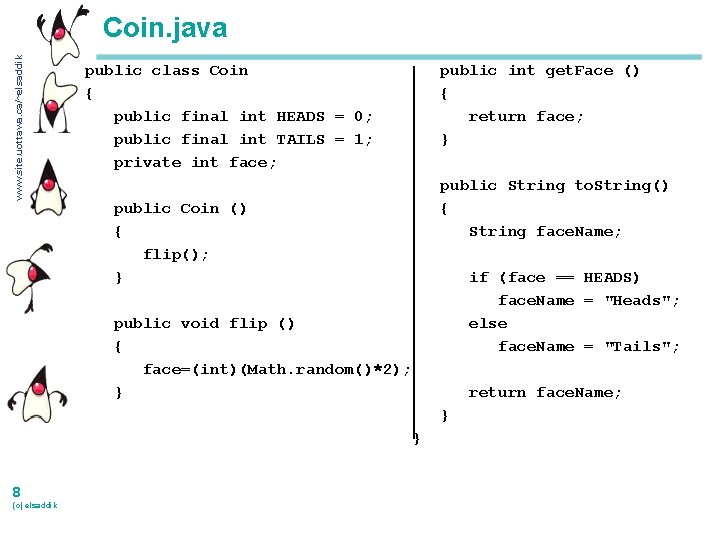
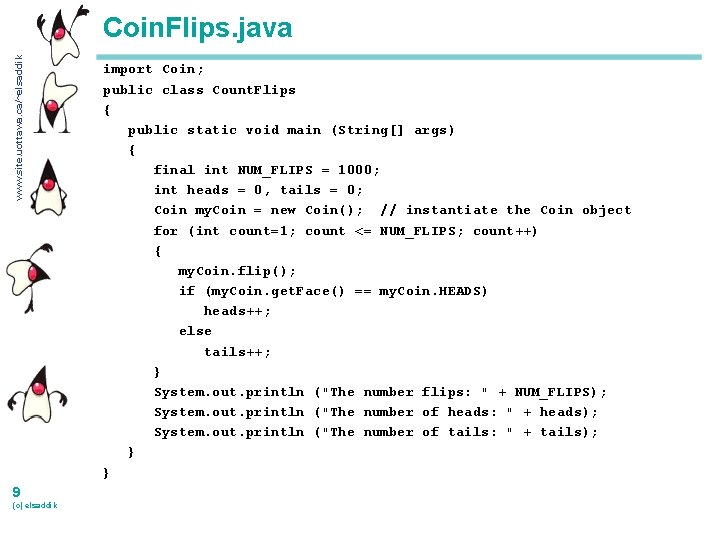
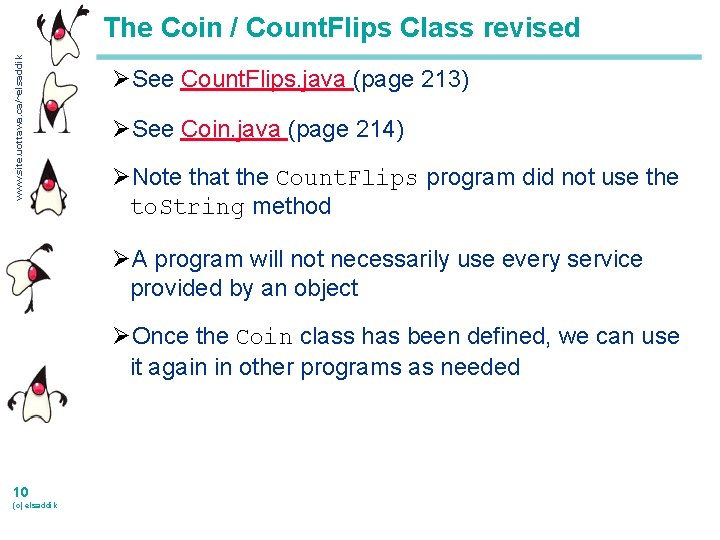
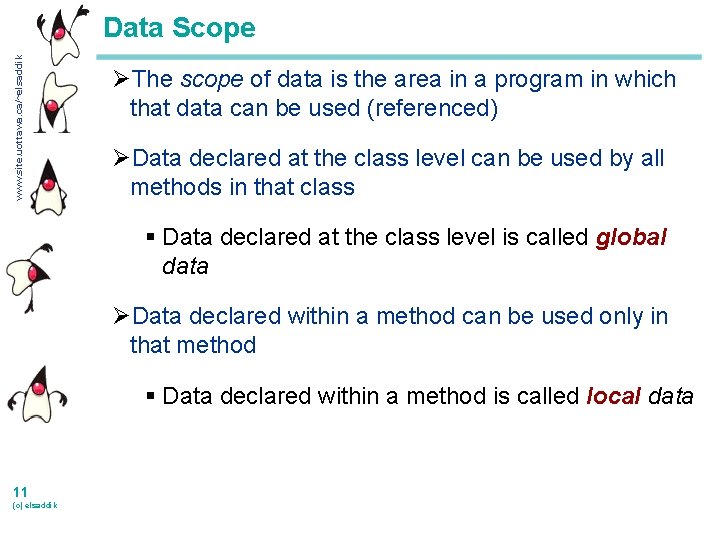
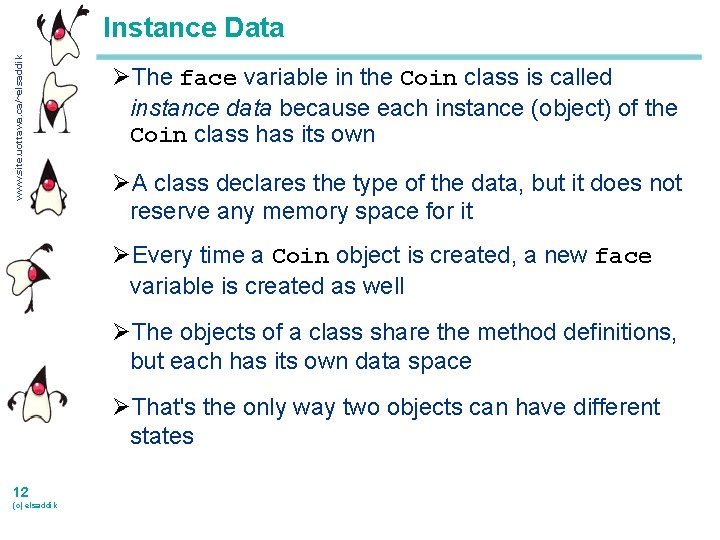
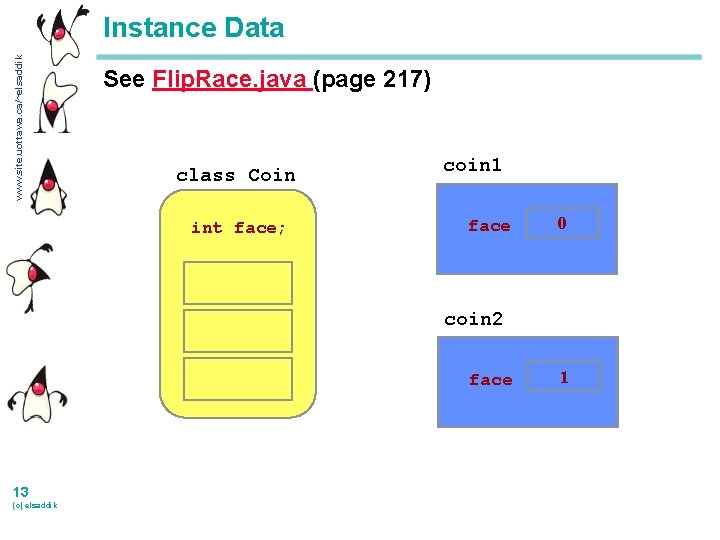
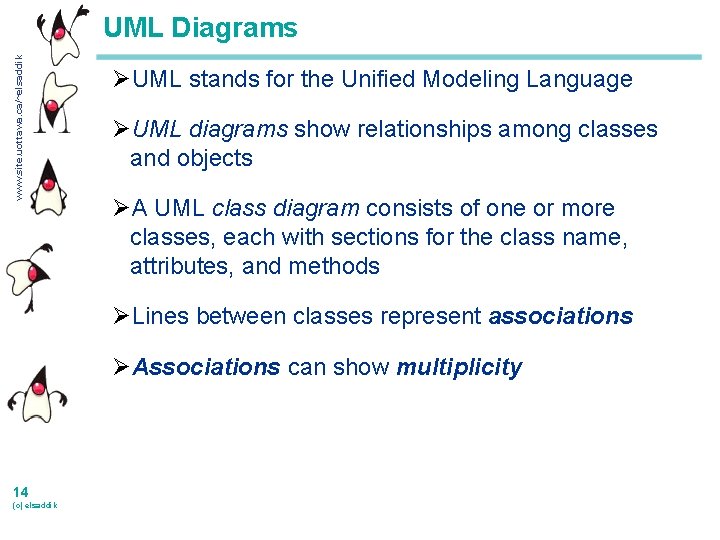
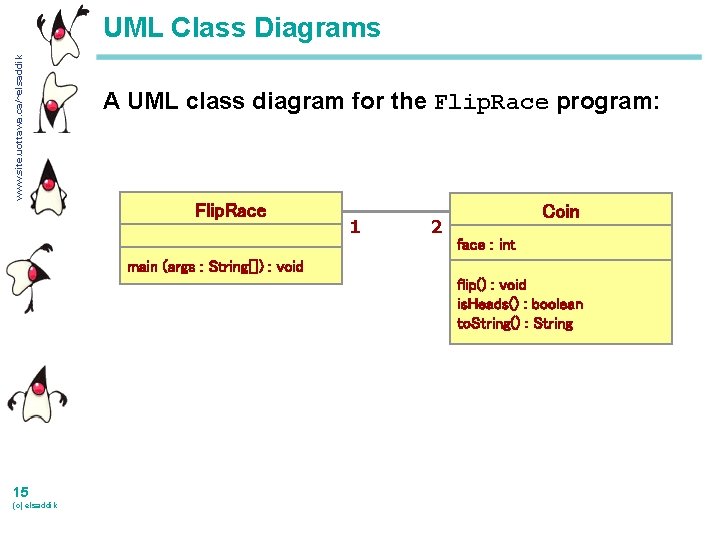
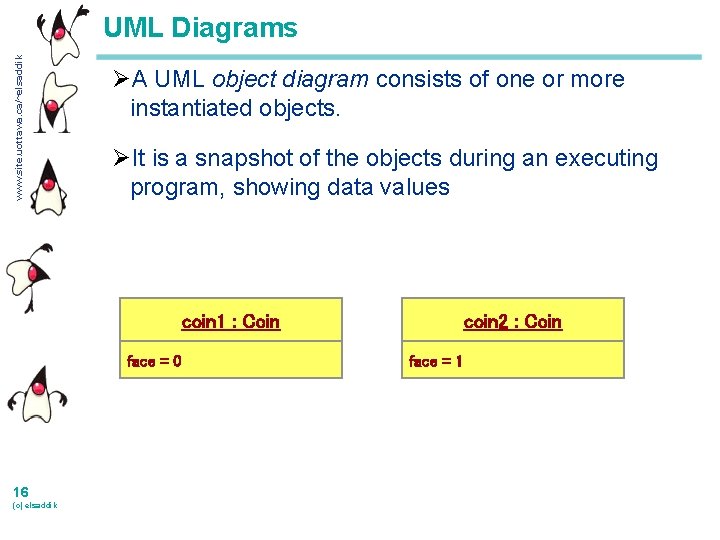
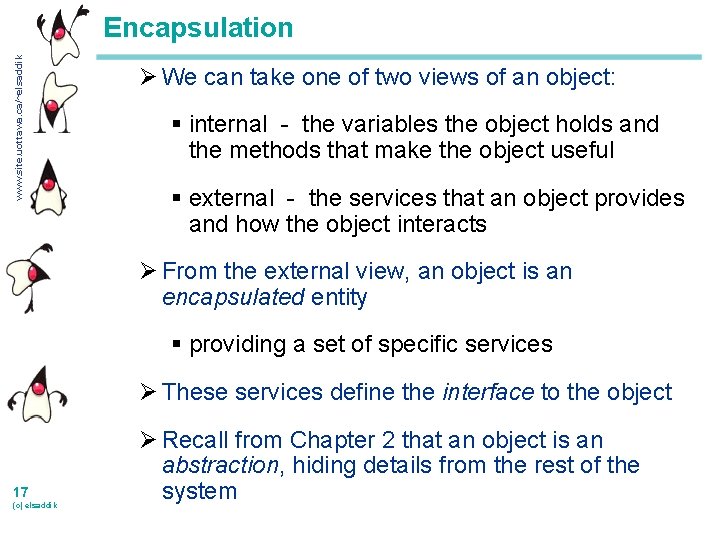
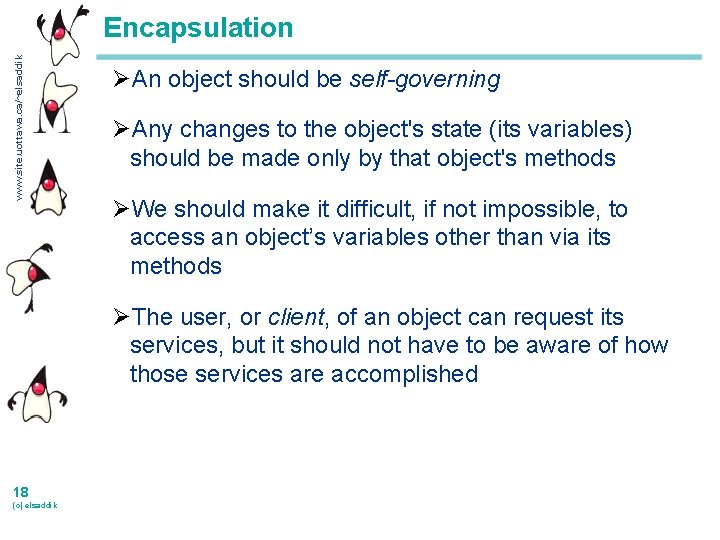
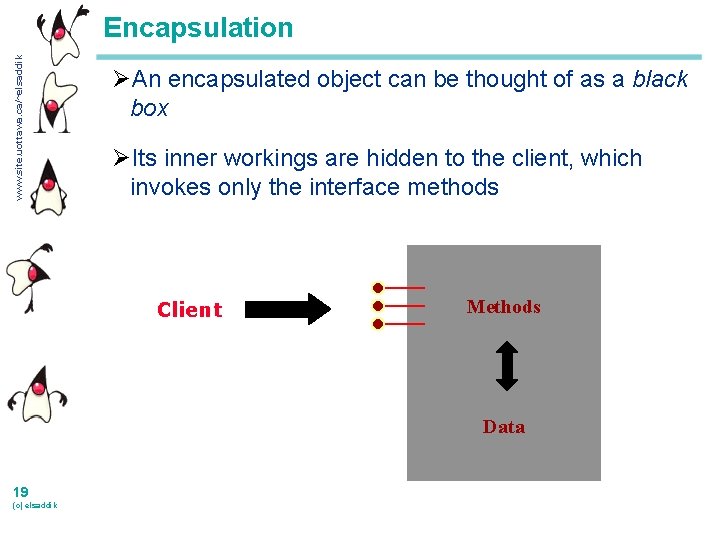
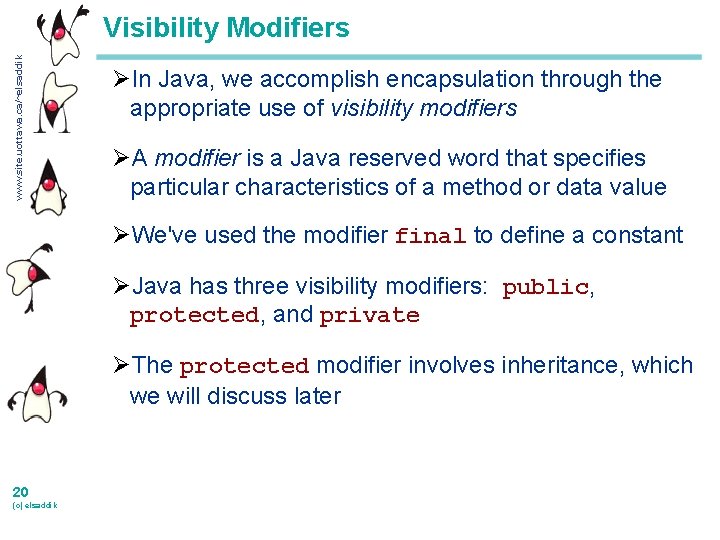
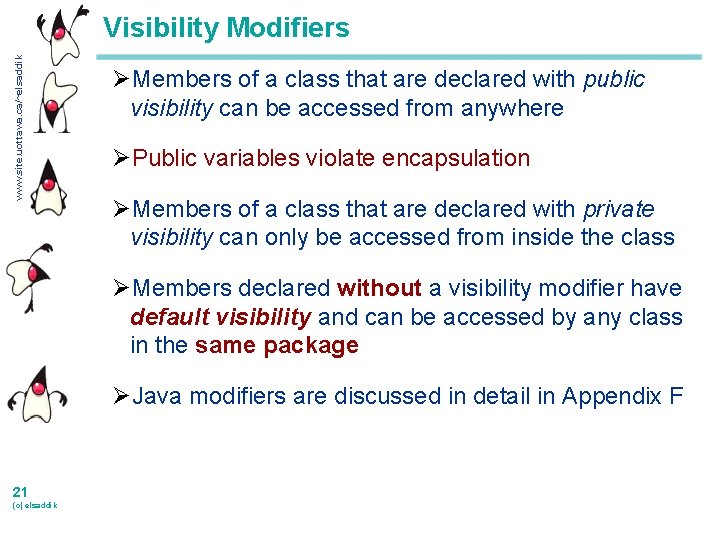
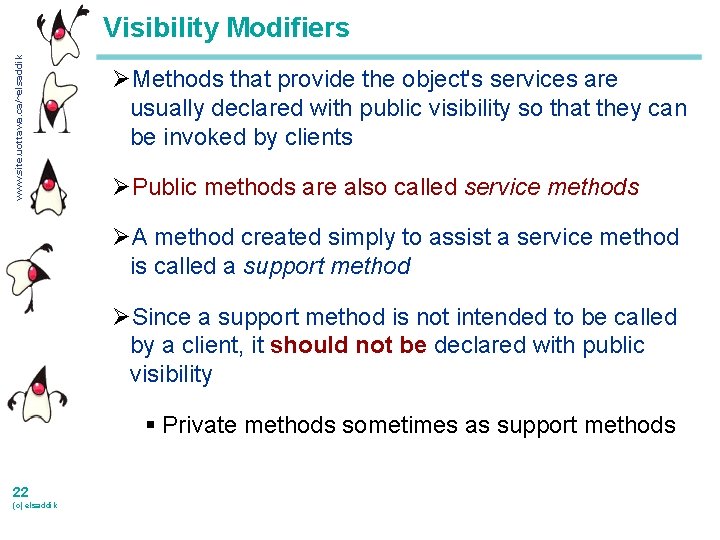
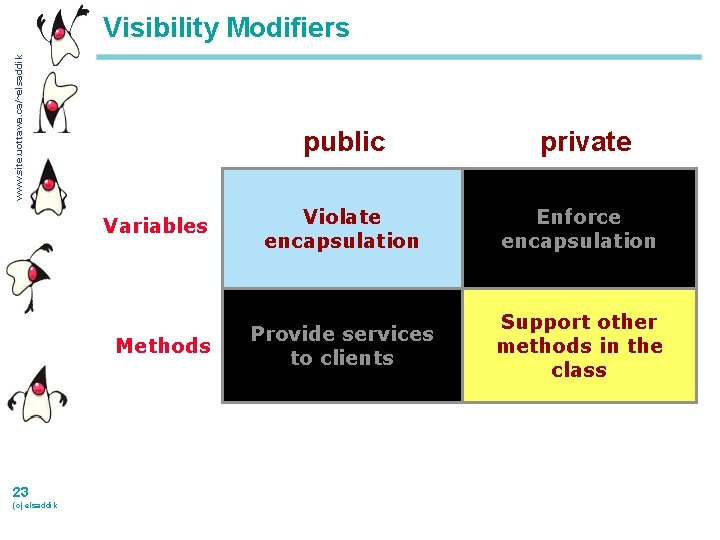
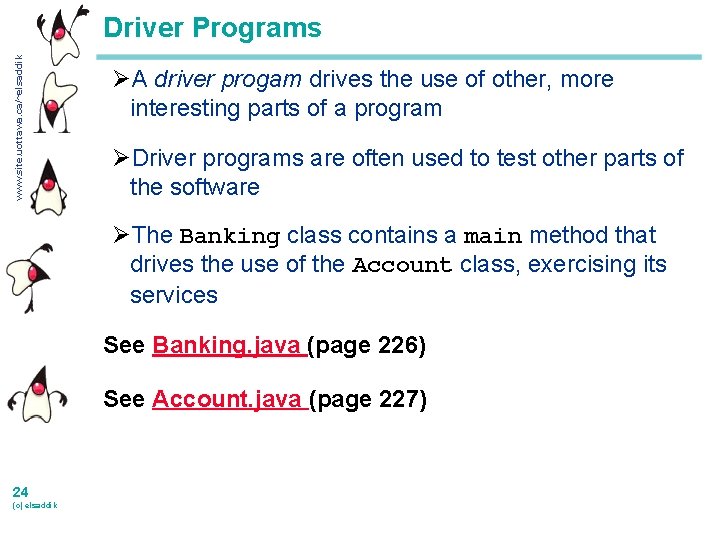
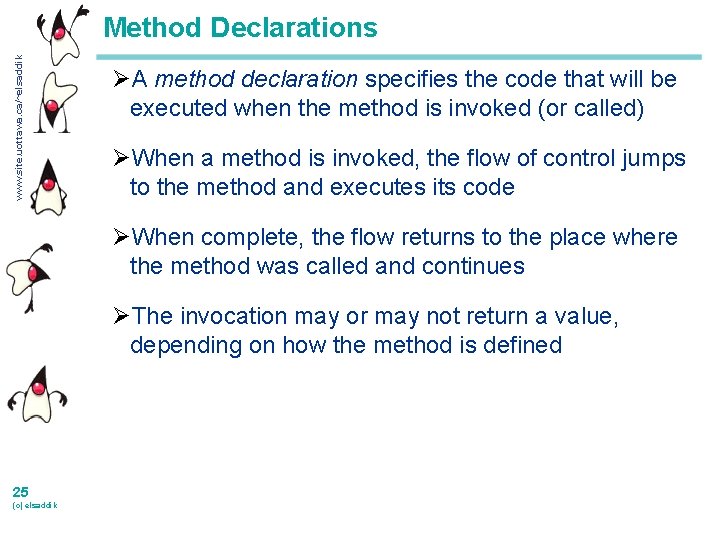
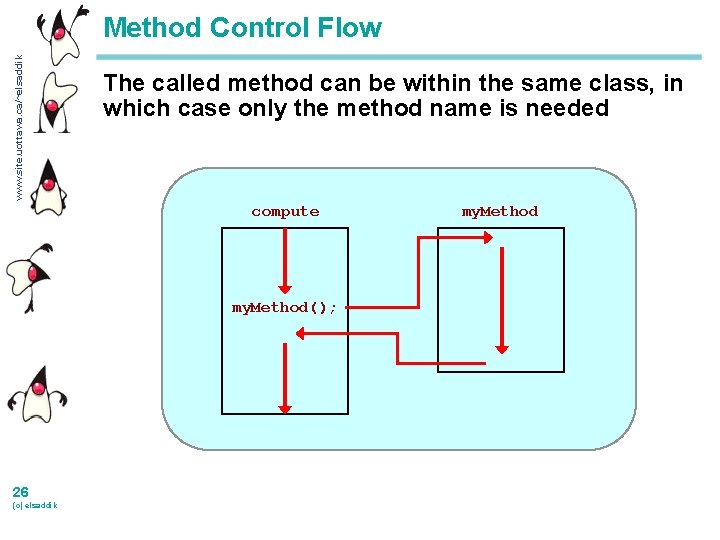
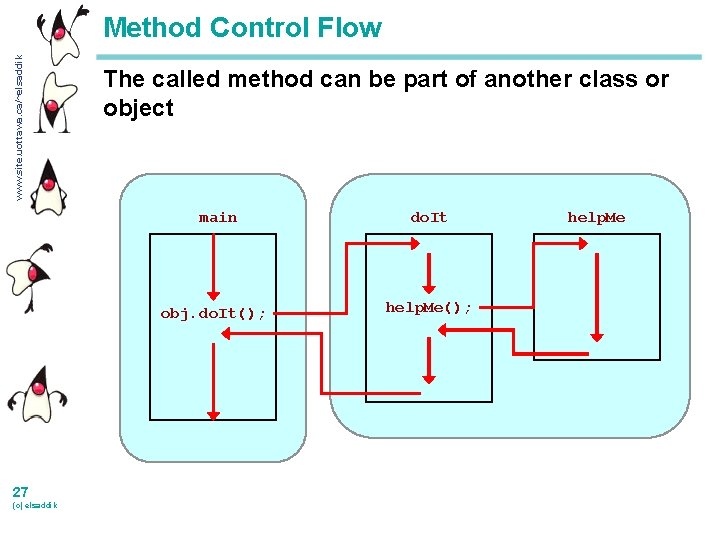
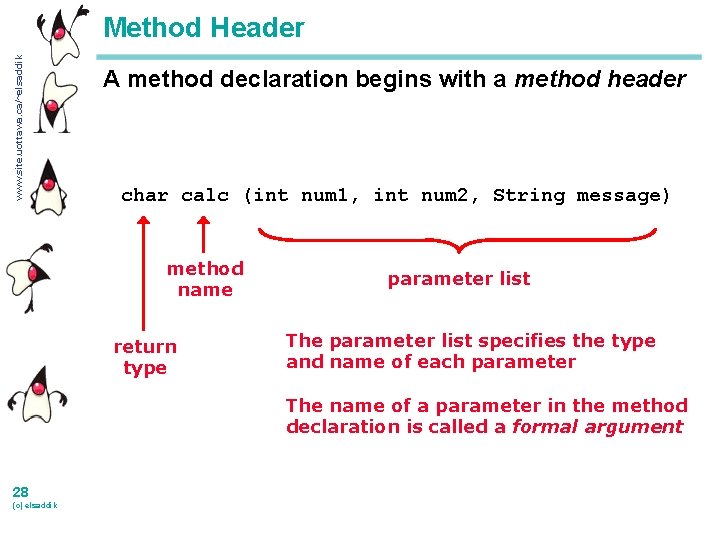
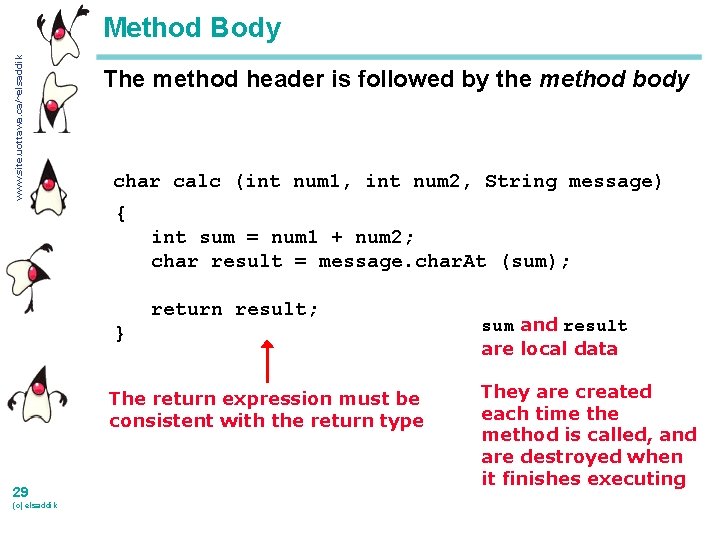
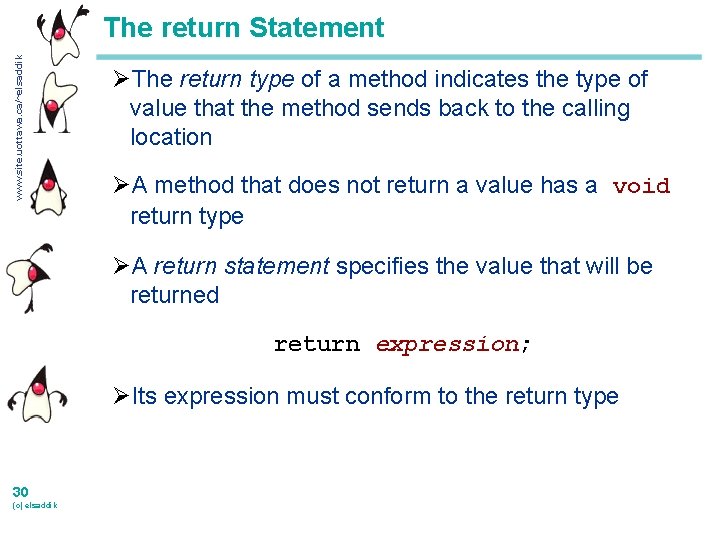
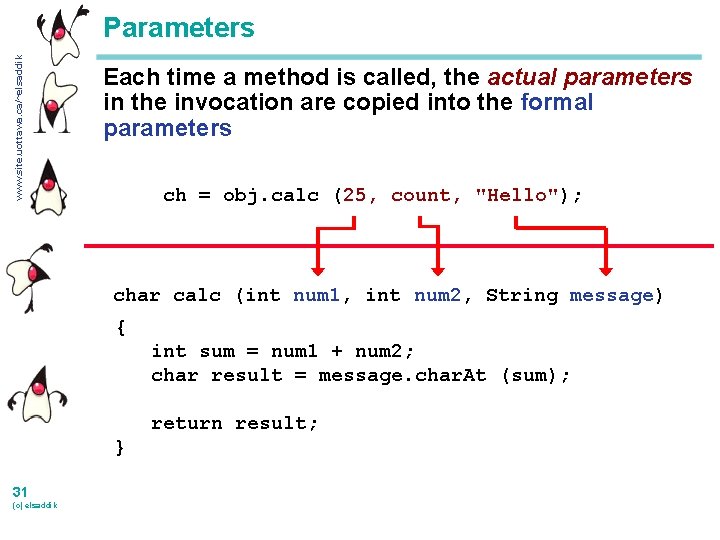
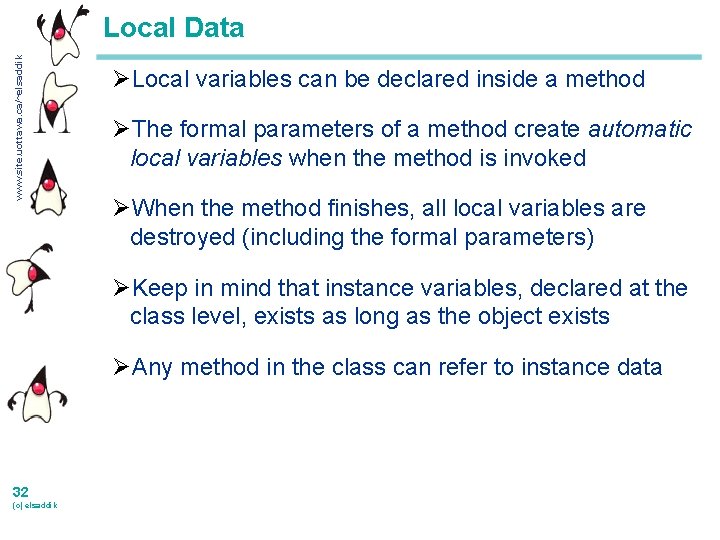
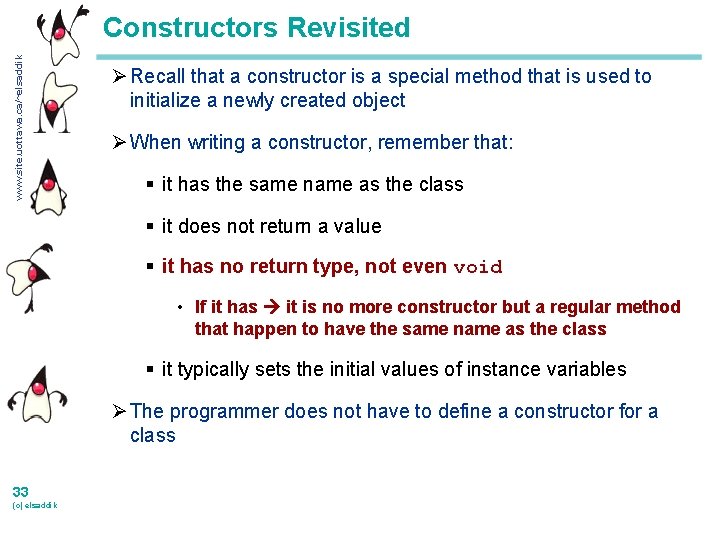
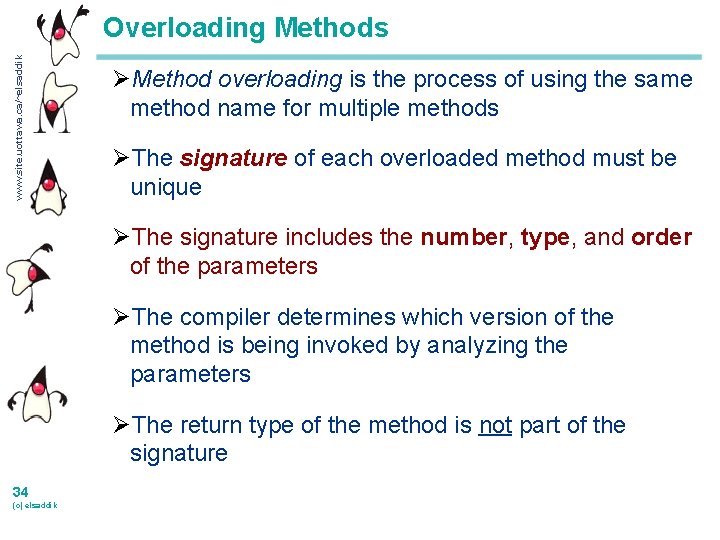
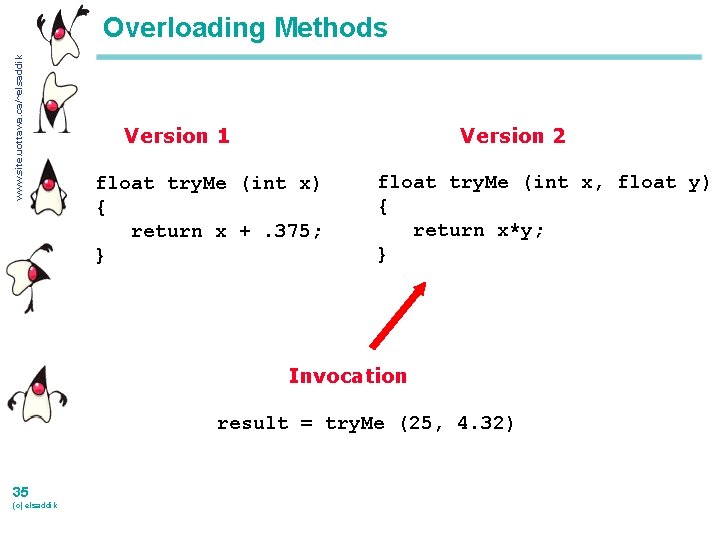
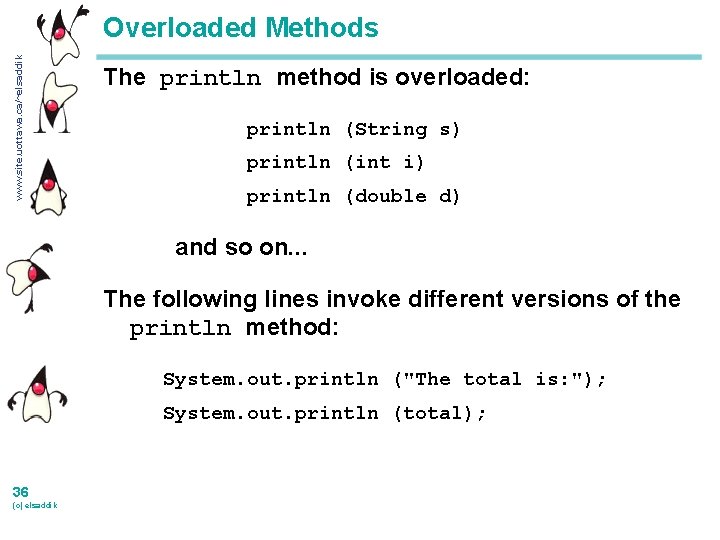
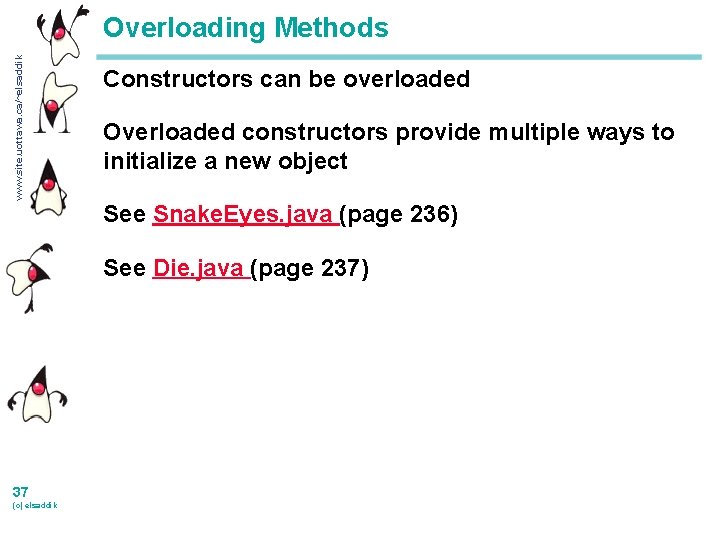
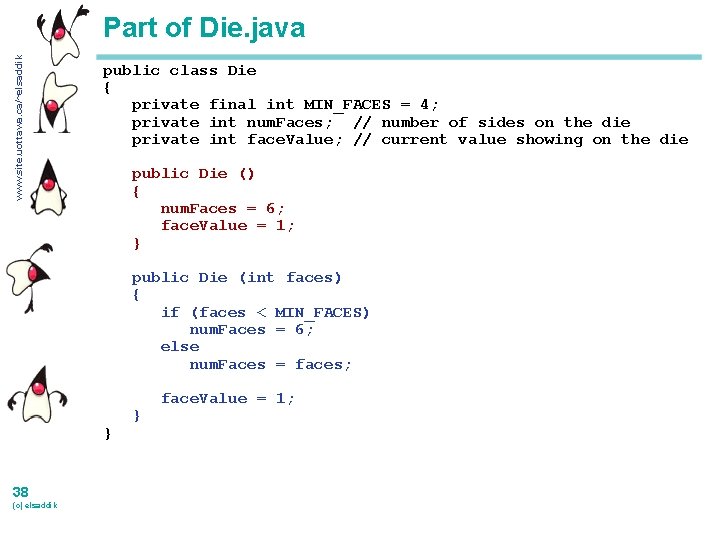
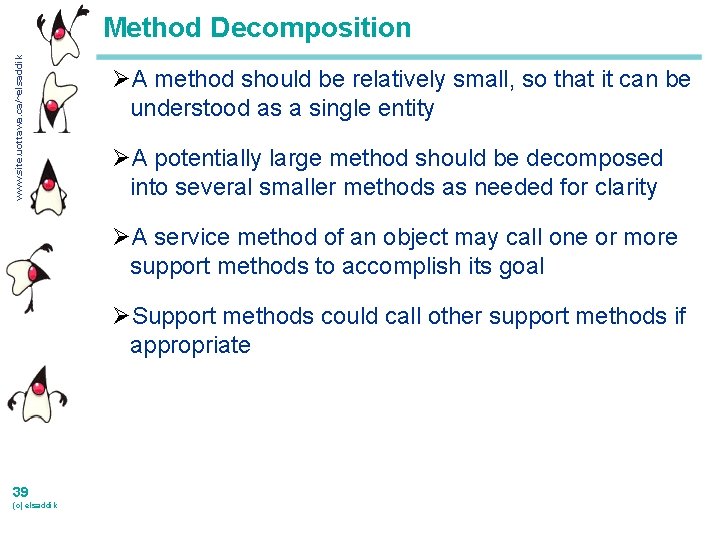
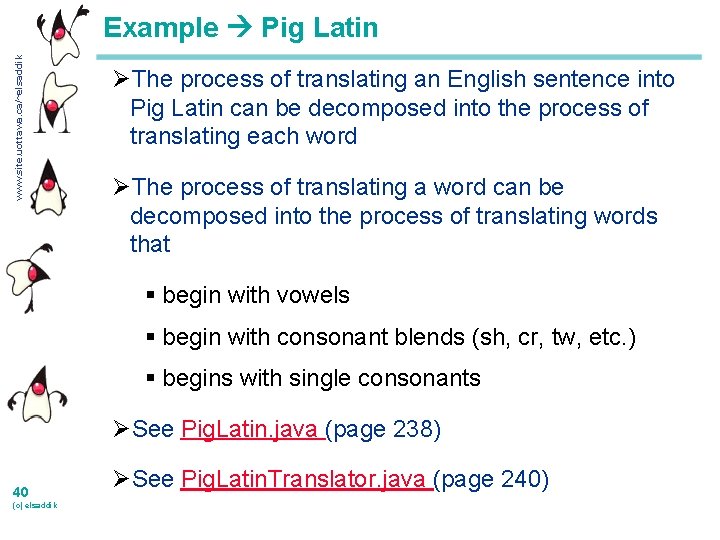
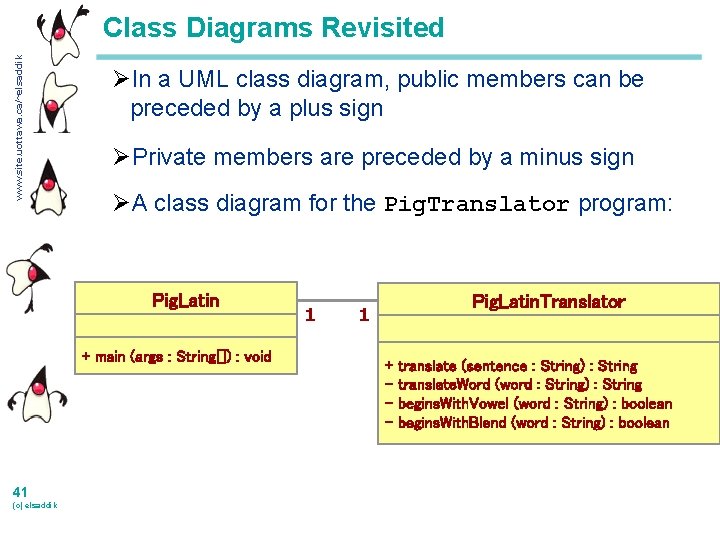
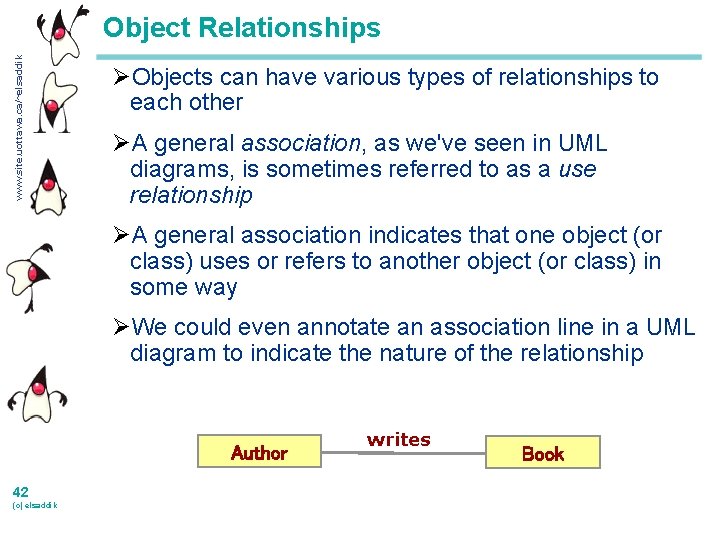
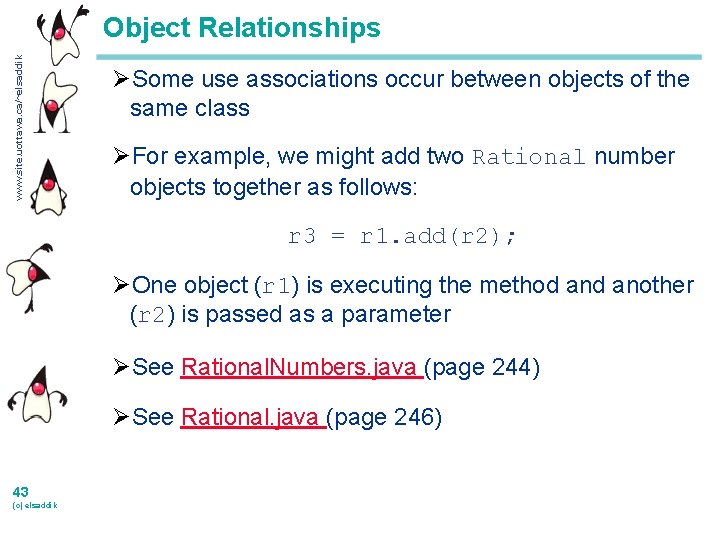
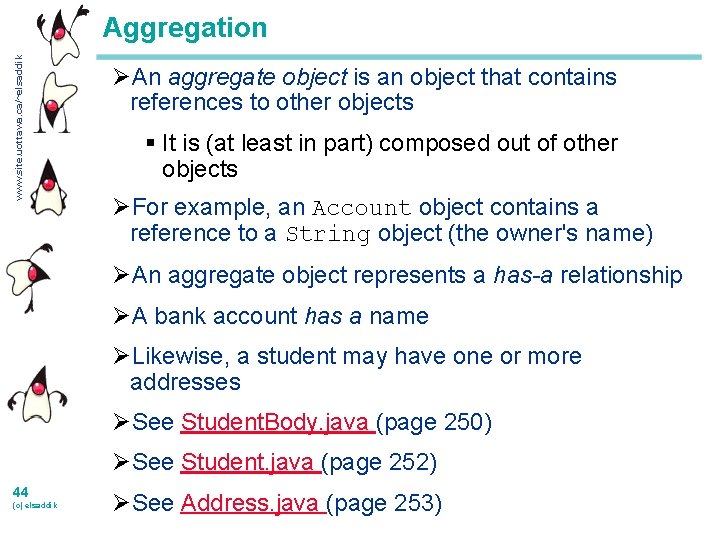
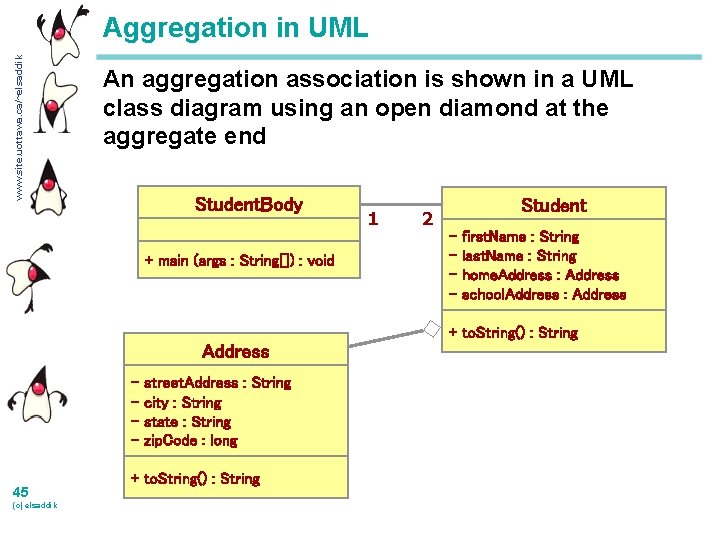
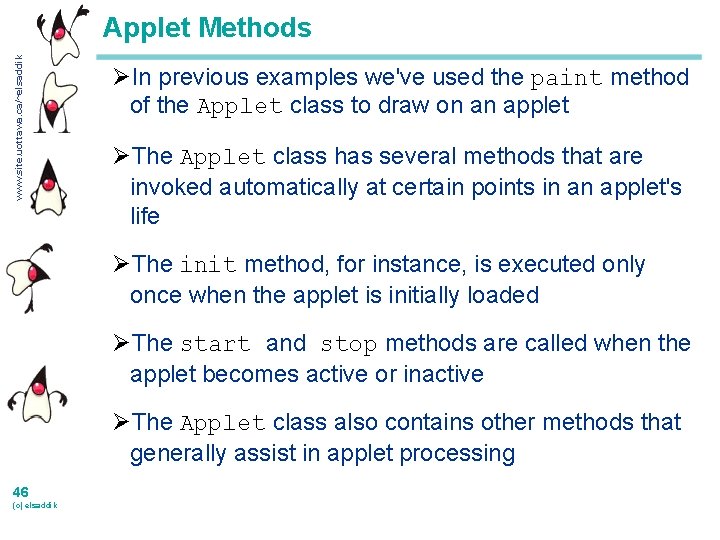
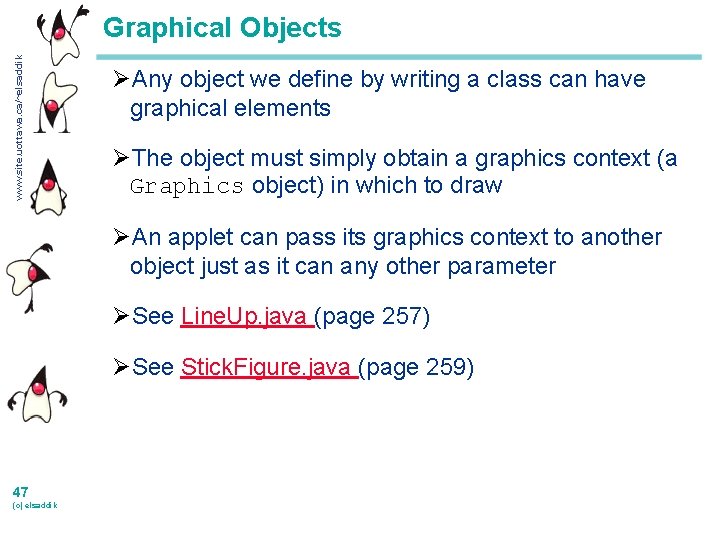
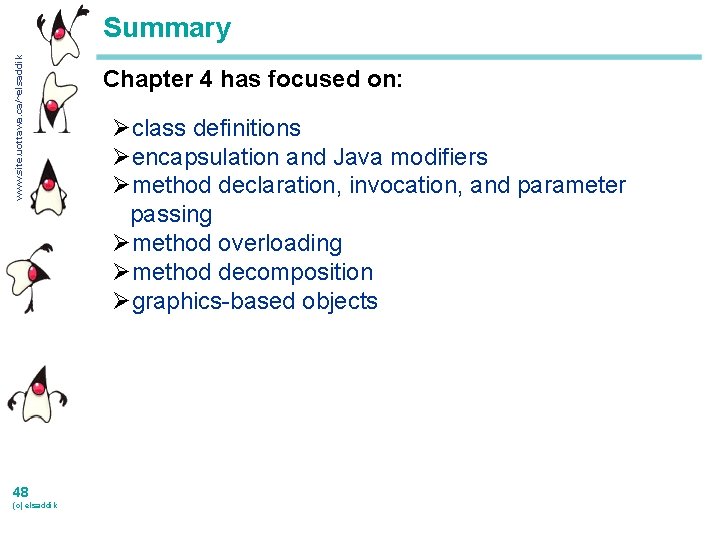
- Slides: 48
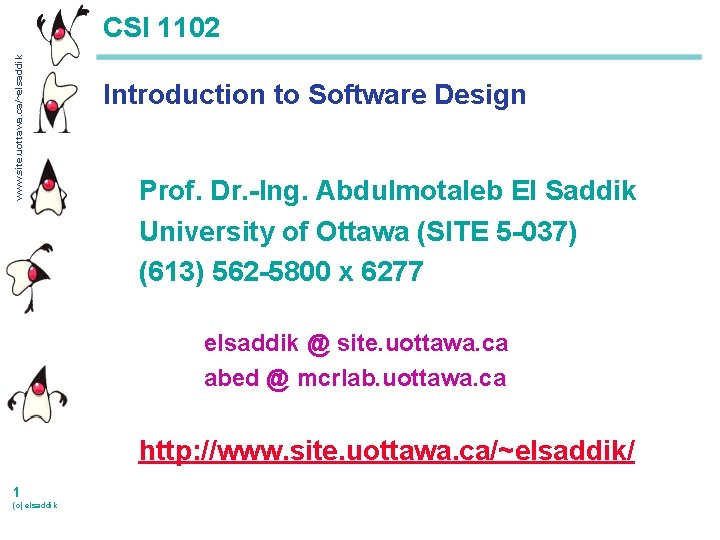
www. site. uottawa. ca/~elsaddik CSI 1102 Introduction to Software Design Prof. Dr. -Ing. Abdulmotaleb El Saddik University of Ottawa (SITE 5 -037) (613) 562 -5800 x 6277 elsaddik @ site. uottawa. ca abed @ mcrlab. uottawa. ca http: //www. site. uottawa. ca/~elsaddik/ 1 (c) elsaddik
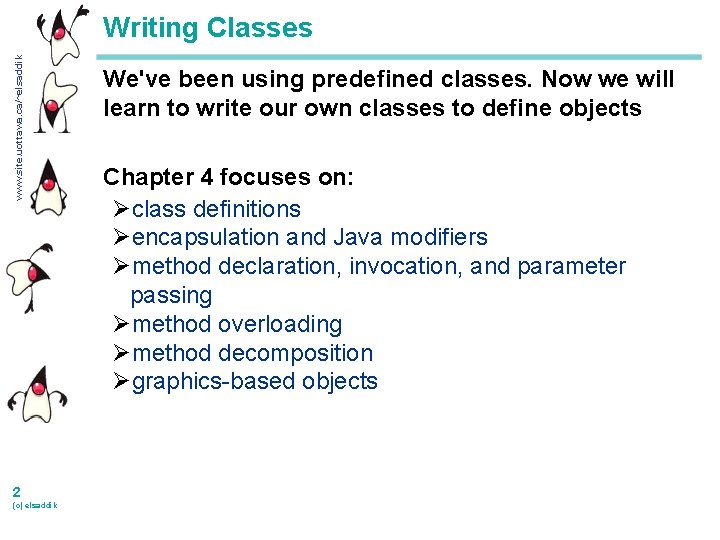
www. site. uottawa. ca/~elsaddik Writing Classes 2 (c) elsaddik We've been using predefined classes. Now we will learn to write our own classes to define objects Chapter 4 focuses on: Øclass definitions Øencapsulation and Java modifiers Ømethod declaration, invocation, and parameter passing Ømethod overloading Ømethod decomposition Øgraphics-based objects
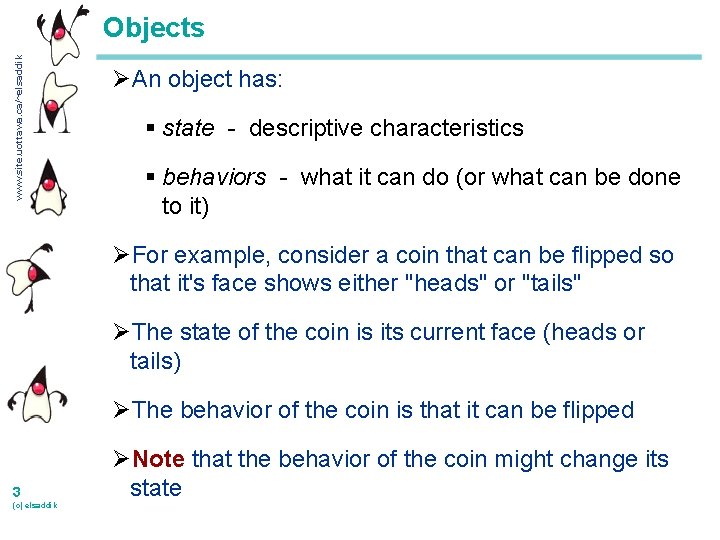
www. site. uottawa. ca/~elsaddik Objects ØAn object has: § state - descriptive characteristics § behaviors - what it can do (or what can be done to it) ØFor example, consider a coin that can be flipped so that it's face shows either "heads" or "tails" ØThe state of the coin is its current face (heads or tails) ØThe behavior of the coin is that it can be flipped 3 (c) elsaddik ØNote that the behavior of the coin might change its state
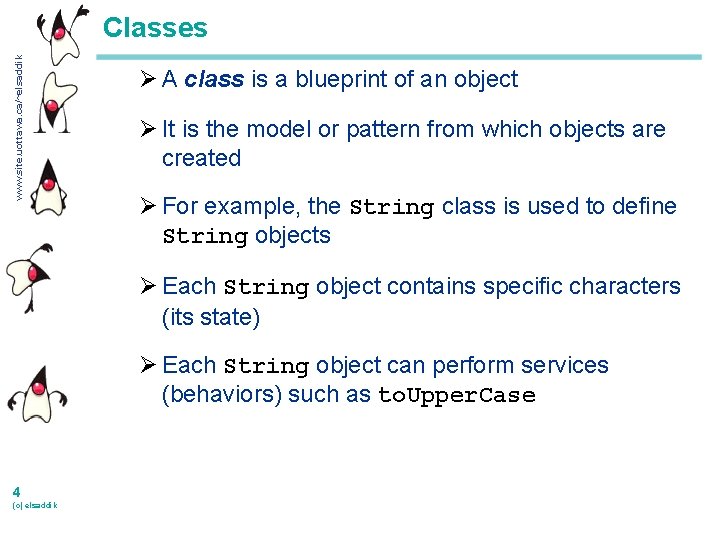
www. site. uottawa. ca/~elsaddik Classes Ø A class is a blueprint of an object Ø It is the model or pattern from which objects are created Ø For example, the String class is used to define String objects Ø Each String object contains specific characters (its state) Ø Each String object can perform services (behaviors) such as to. Upper. Case 4 (c) elsaddik
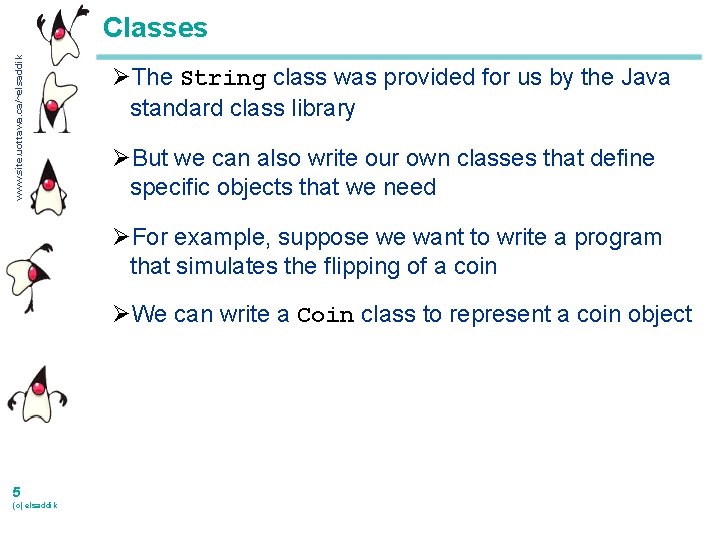
www. site. uottawa. ca/~elsaddik Classes ØThe String class was provided for us by the Java standard class library ØBut we can also write our own classes that define specific objects that we need ØFor example, suppose we want to write a program that simulates the flipping of a coin ØWe can write a Coin class to represent a coin object 5 (c) elsaddik
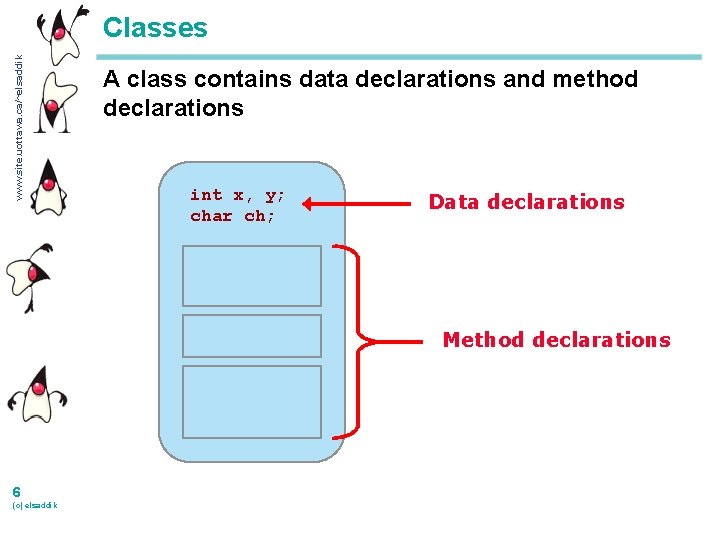
www. site. uottawa. ca/~elsaddik Classes A class contains data declarations and method declarations int x, y; char ch; Data declarations Method declarations 6 (c) elsaddik
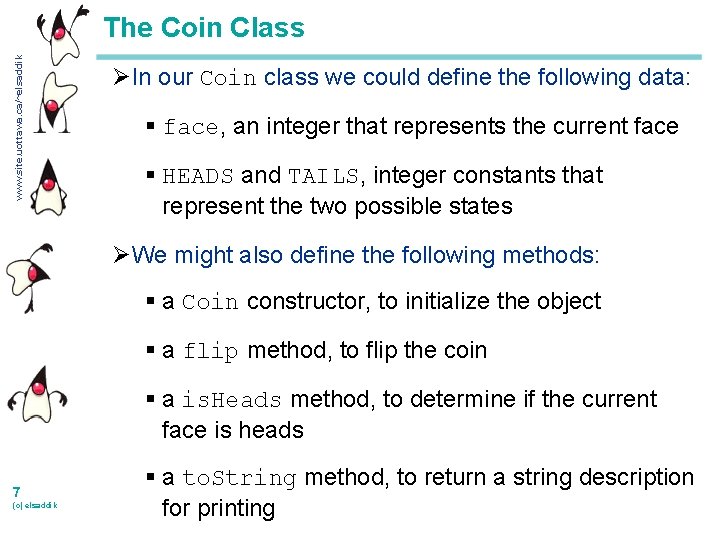
www. site. uottawa. ca/~elsaddik The Coin Class ØIn our Coin class we could define the following data: § face, an integer that represents the current face § HEADS and TAILS, integer constants that represent the two possible states ØWe might also define the following methods: § a Coin constructor, to initialize the object § a flip method, to flip the coin § a is. Heads method, to determine if the current face is heads 7 (c) elsaddik § a to. String method, to return a string description for printing
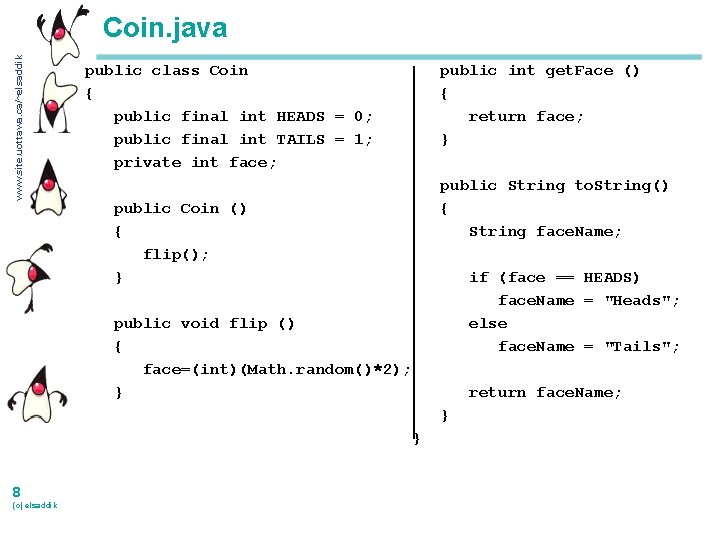
www. site. uottawa. ca/~elsaddik Coin. java public class Coin { public final int HEADS = 0; public final int TAILS = 1; private int face; public int get. Face () { return face; } public String to. String() { String face. Name; public Coin () { flip(); } if (face == HEADS) face. Name = "Heads"; else face. Name = "Tails"; public void flip () { face=(int)(Math. random()*2); } return face. Name; } } 8 (c) elsaddik
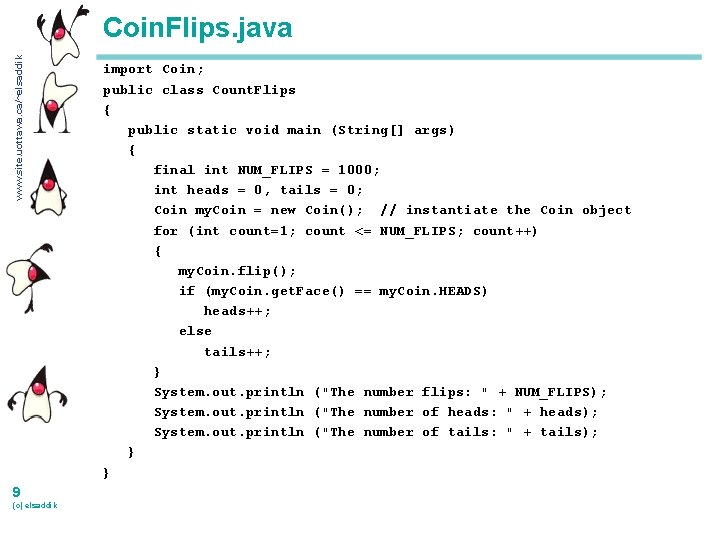
www. site. uottawa. ca/~elsaddik Coin. Flips. java 9 (c) elsaddik import Coin; public class Count. Flips { public static void main (String[] args) { final int NUM_FLIPS = 1000; int heads = 0, tails = 0; Coin my. Coin = new Coin(); // instantiate the Coin object for (int count=1; count <= NUM_FLIPS; count++) { my. Coin. flip(); if (my. Coin. get. Face() == my. Coin. HEADS) heads++; else tails++; } System. out. println ("The number flips: " + NUM_FLIPS); System. out. println ("The number of heads: " + heads); System. out. println ("The number of tails: " + tails); } }
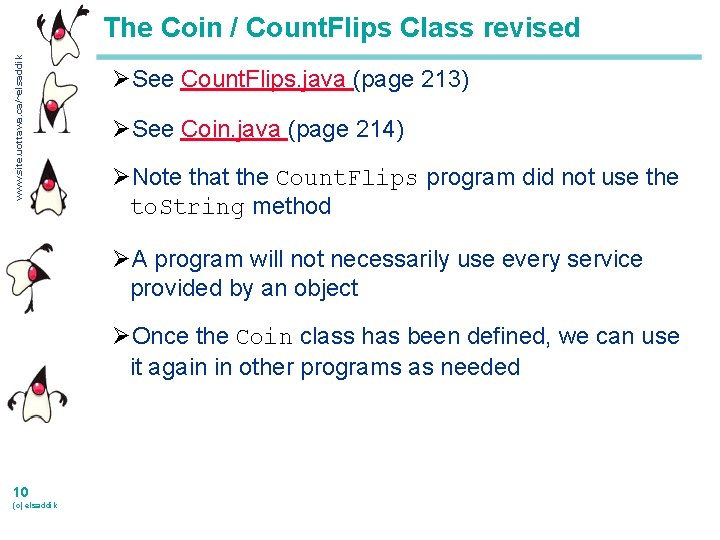
www. site. uottawa. ca/~elsaddik The Coin / Count. Flips Class revised ØSee Count. Flips. java (page 213) ØSee Coin. java (page 214) ØNote that the Count. Flips program did not use the to. String method ØA program will not necessarily use every service provided by an object ØOnce the Coin class has been defined, we can use it again in other programs as needed 10 (c) elsaddik
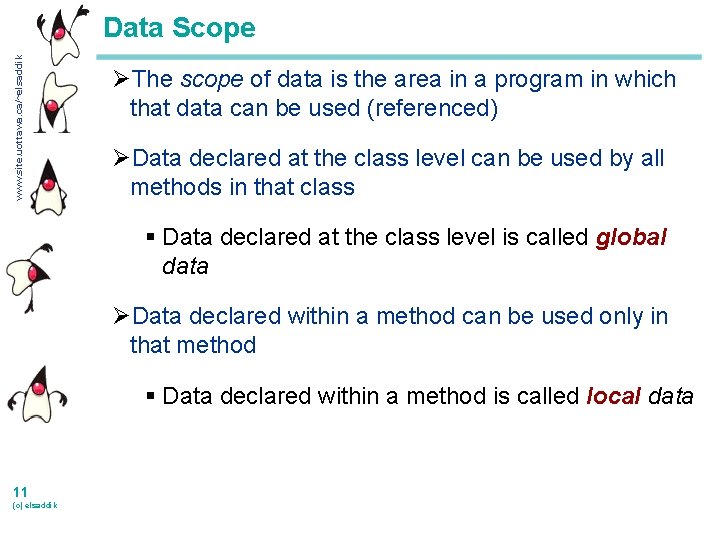
www. site. uottawa. ca/~elsaddik Data Scope ØThe scope of data is the area in a program in which that data can be used (referenced) ØData declared at the class level can be used by all methods in that class § Data declared at the class level is called global data ØData declared within a method can be used only in that method § Data declared within a method is called local data 11 (c) elsaddik
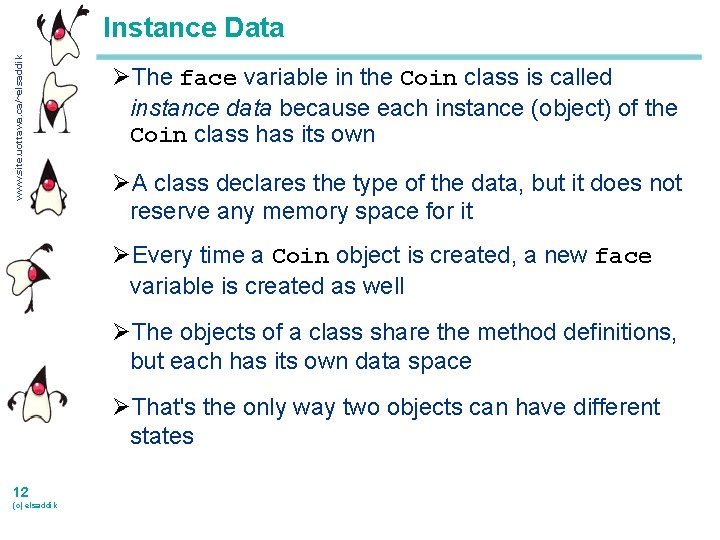
www. site. uottawa. ca/~elsaddik Instance Data ØThe face variable in the Coin class is called instance data because each instance (object) of the Coin class has its own ØA class declares the type of the data, but it does not reserve any memory space for it ØEvery time a Coin object is created, a new face variable is created as well ØThe objects of a class share the method definitions, but each has its own data space ØThat's the only way two objects can have different states 12 (c) elsaddik
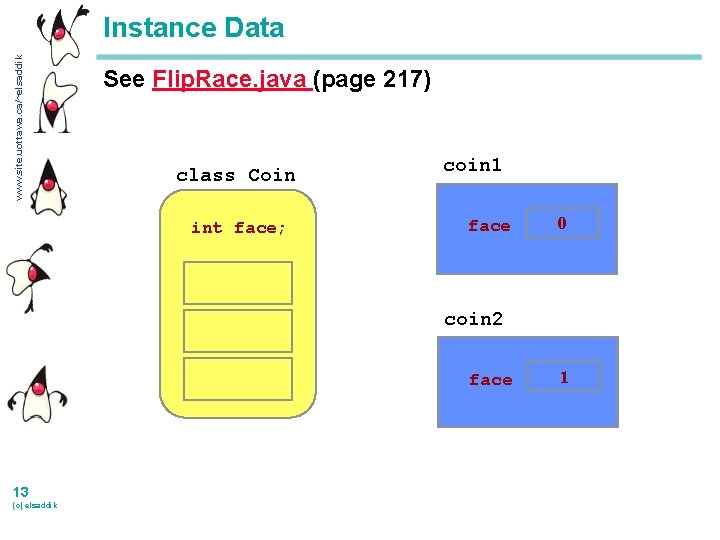
www. site. uottawa. ca/~elsaddik Instance Data See Flip. Race. java (page 217) class Coin int face; coin 1 face 0 coin 2 face 13 (c) elsaddik 1
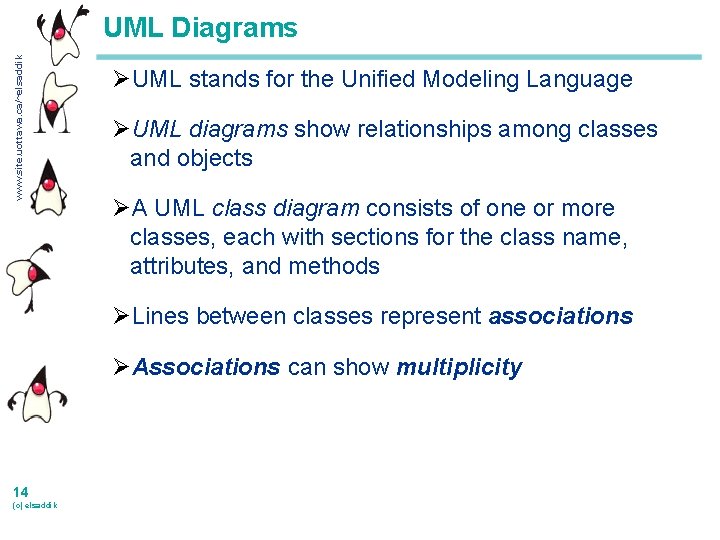
www. site. uottawa. ca/~elsaddik UML Diagrams ØUML stands for the Unified Modeling Language ØUML diagrams show relationships among classes and objects ØA UML class diagram consists of one or more classes, each with sections for the class name, attributes, and methods ØLines between classes represent associations ØAssociations can show multiplicity 14 (c) elsaddik
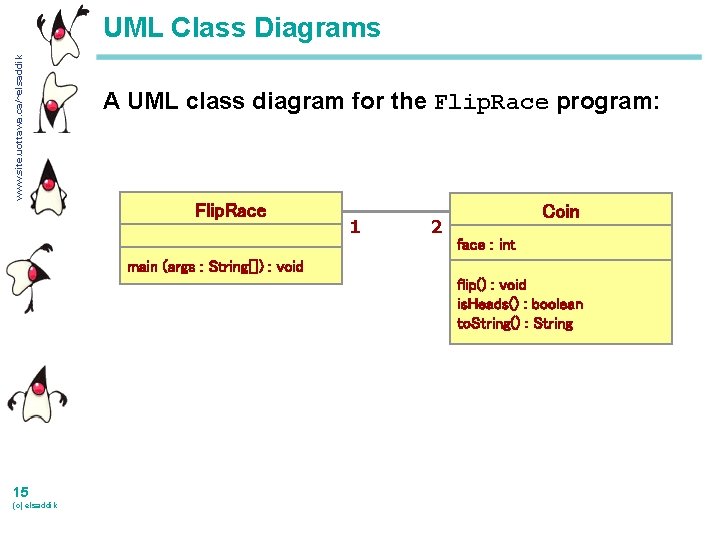
www. site. uottawa. ca/~elsaddik UML Class Diagrams A UML class diagram for the Flip. Race program: Flip. Race 1 2 Coin face : int main (args : String[]) : void flip() : void is. Heads() : boolean to. String() : String 15 (c) elsaddik
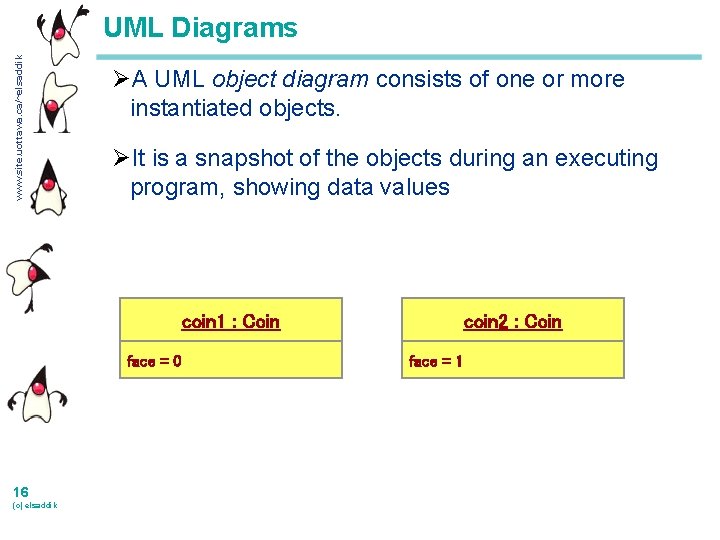
www. site. uottawa. ca/~elsaddik UML Diagrams ØA UML object diagram consists of one or more instantiated objects. ØIt is a snapshot of the objects during an executing program, showing data values coin 1 : Coin face = 0 16 (c) elsaddik coin 2 : Coin face = 1
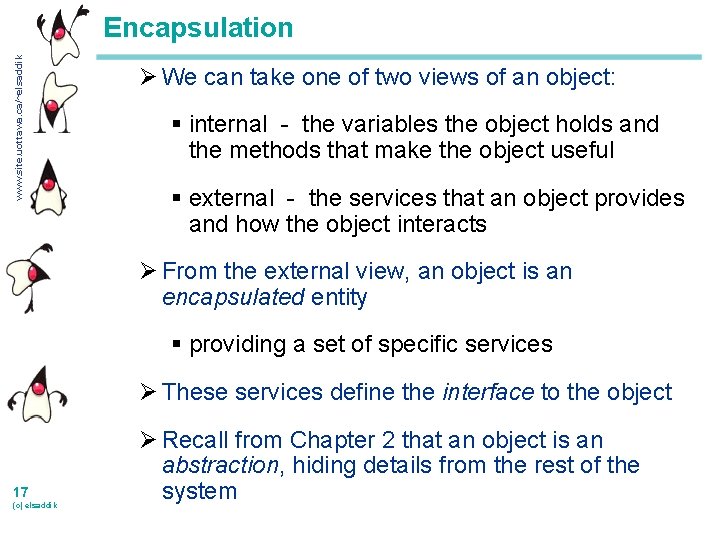
www. site. uottawa. ca/~elsaddik Encapsulation Ø We can take one of two views of an object: § internal - the variables the object holds and the methods that make the object useful § external - the services that an object provides and how the object interacts Ø From the external view, an object is an encapsulated entity § providing a set of specific services Ø These services define the interface to the object 17 (c) elsaddik Ø Recall from Chapter 2 that an object is an abstraction, hiding details from the rest of the system
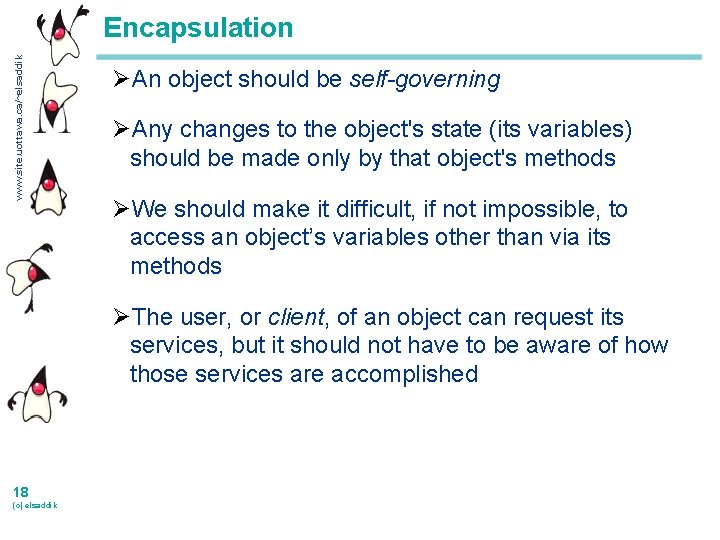
www. site. uottawa. ca/~elsaddik Encapsulation ØAn object should be self-governing ØAny changes to the object's state (its variables) should be made only by that object's methods ØWe should make it difficult, if not impossible, to access an object’s variables other than via its methods ØThe user, or client, of an object can request its services, but it should not have to be aware of how those services are accomplished 18 (c) elsaddik
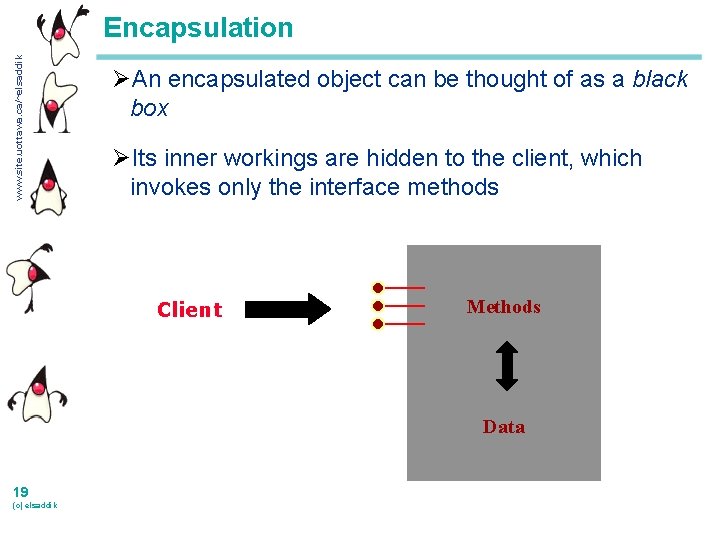
www. site. uottawa. ca/~elsaddik Encapsulation ØAn encapsulated object can be thought of as a black box ØIts inner workings are hidden to the client, which invokes only the interface methods Client Methods Data 19 (c) elsaddik
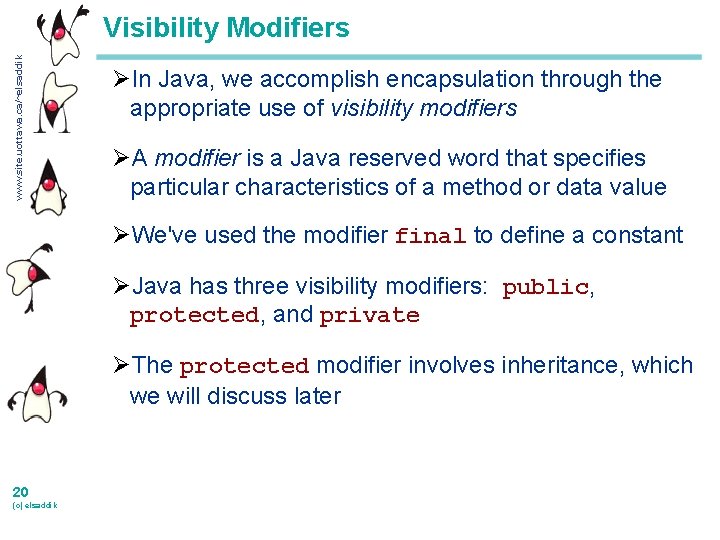
www. site. uottawa. ca/~elsaddik Visibility Modifiers ØIn Java, we accomplish encapsulation through the appropriate use of visibility modifiers ØA modifier is a Java reserved word that specifies particular characteristics of a method or data value ØWe've used the modifier final to define a constant ØJava has three visibility modifiers: public, protected, and private ØThe protected modifier involves inheritance, which we will discuss later 20 (c) elsaddik
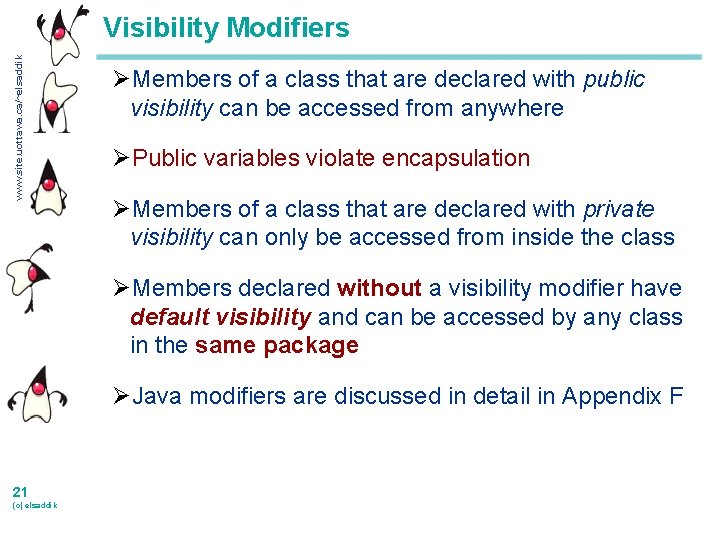
www. site. uottawa. ca/~elsaddik Visibility Modifiers ØMembers of a class that are declared with public visibility can be accessed from anywhere ØPublic variables violate encapsulation ØMembers of a class that are declared with private visibility can only be accessed from inside the class ØMembers declared without a visibility modifier have default visibility and can be accessed by any class in the same package ØJava modifiers are discussed in detail in Appendix F 21 (c) elsaddik
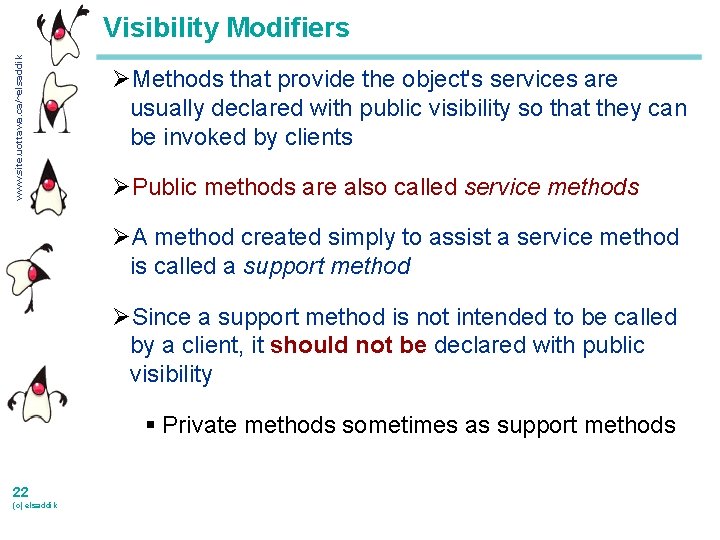
www. site. uottawa. ca/~elsaddik Visibility Modifiers ØMethods that provide the object's services are usually declared with public visibility so that they can be invoked by clients ØPublic methods are also called service methods ØA method created simply to assist a service method is called a support method ØSince a support method is not intended to be called by a client, it should not be declared with public visibility § Private methods sometimes as support methods 22 (c) elsaddik
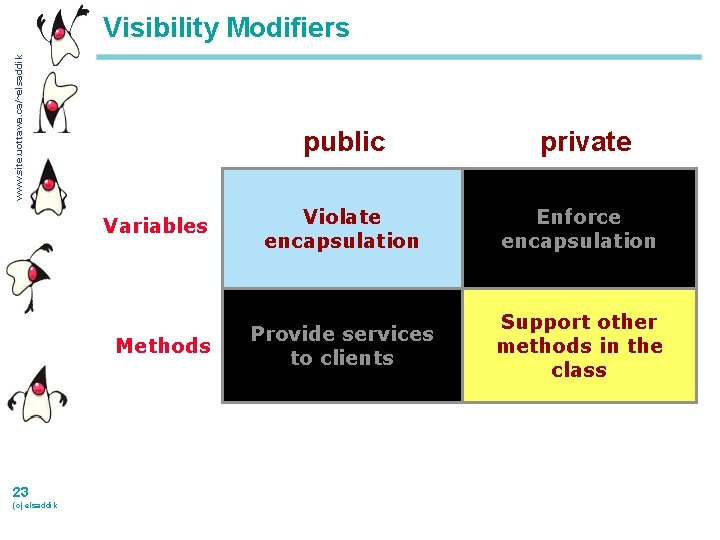
www. site. uottawa. ca/~elsaddik Visibility Modifiers public Variables Methods 23 (c) elsaddik private Violate encapsulation Enforce encapsulation Provide services to clients Support other methods in the class
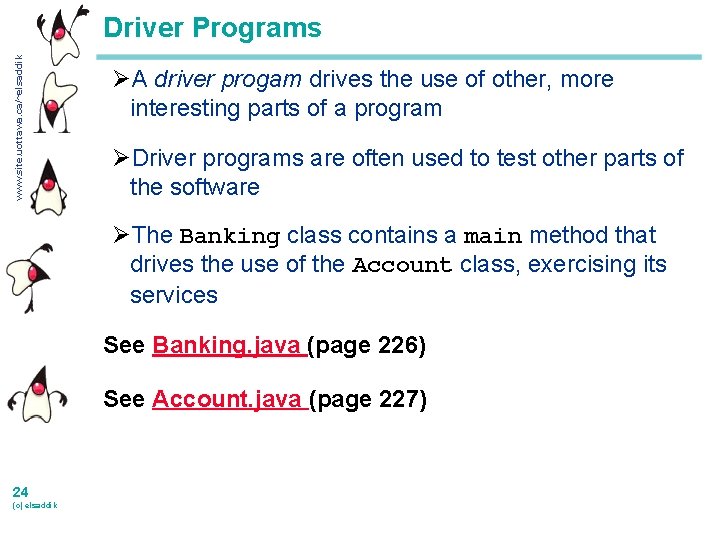
www. site. uottawa. ca/~elsaddik Driver Programs ØA driver progam drives the use of other, more interesting parts of a program ØDriver programs are often used to test other parts of the software ØThe Banking class contains a main method that drives the use of the Account class, exercising its services See Banking. java (page 226) See Account. java (page 227) 24 (c) elsaddik
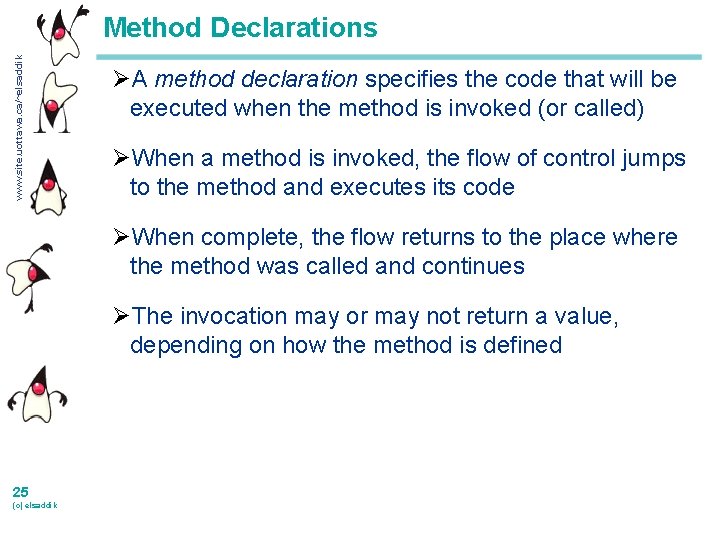
www. site. uottawa. ca/~elsaddik Method Declarations ØA method declaration specifies the code that will be executed when the method is invoked (or called) ØWhen a method is invoked, the flow of control jumps to the method and executes its code ØWhen complete, the flow returns to the place where the method was called and continues ØThe invocation may or may not return a value, depending on how the method is defined 25 (c) elsaddik
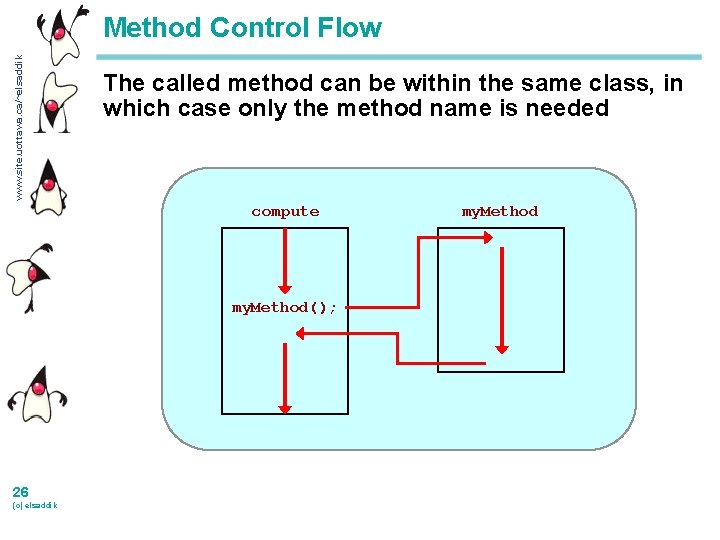
www. site. uottawa. ca/~elsaddik Method Control Flow The called method can be within the same class, in which case only the method name is needed compute my. Method(); 26 (c) elsaddik my. Method
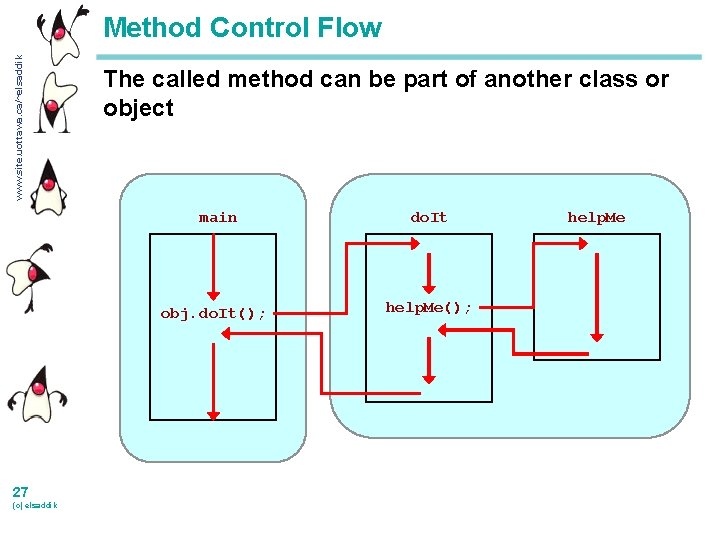
www. site. uottawa. ca/~elsaddik Method Control Flow The called method can be part of another class or object main obj. do. It(); 27 (c) elsaddik do. It help. Me(); help. Me
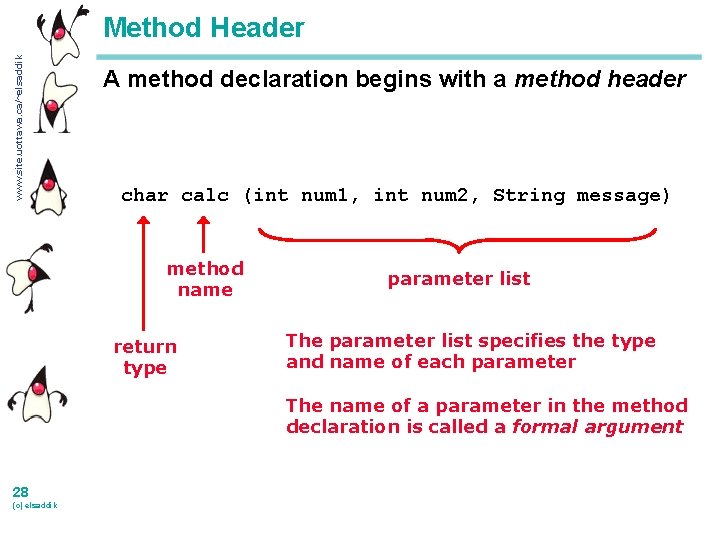
www. site. uottawa. ca/~elsaddik Method Header A method declaration begins with a method header char calc (int num 1, int num 2, String message) method name return type parameter list The parameter list specifies the type and name of each parameter The name of a parameter in the method declaration is called a formal argument 28 (c) elsaddik
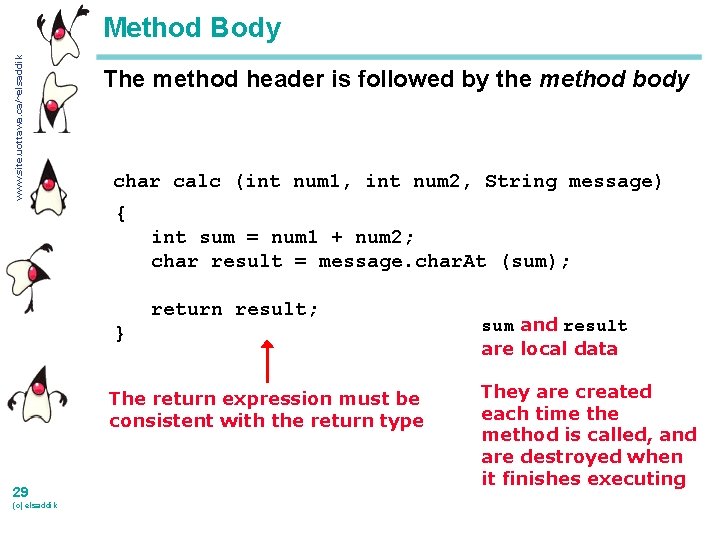
www. site. uottawa. ca/~elsaddik Method Body The method header is followed by the method body char calc (int num 1, int num 2, String message) { int sum = num 1 + num 2; char result = message. char. At (sum); return result; } The return expression must be consistent with the return type 29 (c) elsaddik sum and result are local data They are created each time the method is called, and are destroyed when it finishes executing
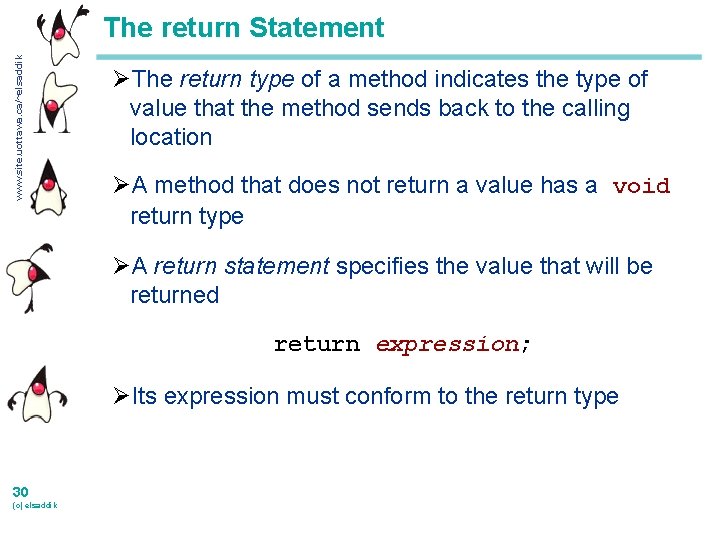
www. site. uottawa. ca/~elsaddik The return Statement ØThe return type of a method indicates the type of value that the method sends back to the calling location ØA method that does not return a value has a void return type ØA return statement specifies the value that will be returned return expression; ØIts expression must conform to the return type 30 (c) elsaddik
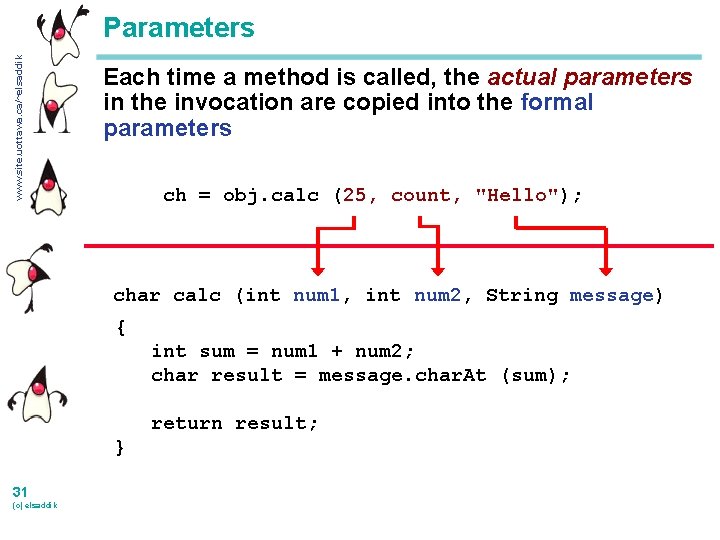
www. site. uottawa. ca/~elsaddik Parameters Each time a method is called, the actual parameters in the invocation are copied into the formal parameters ch = obj. calc (25, count, "Hello"); char calc (int num 1, int num 2, String message) { int sum = num 1 + num 2; char result = message. char. At (sum); return result; } 31 (c) elsaddik
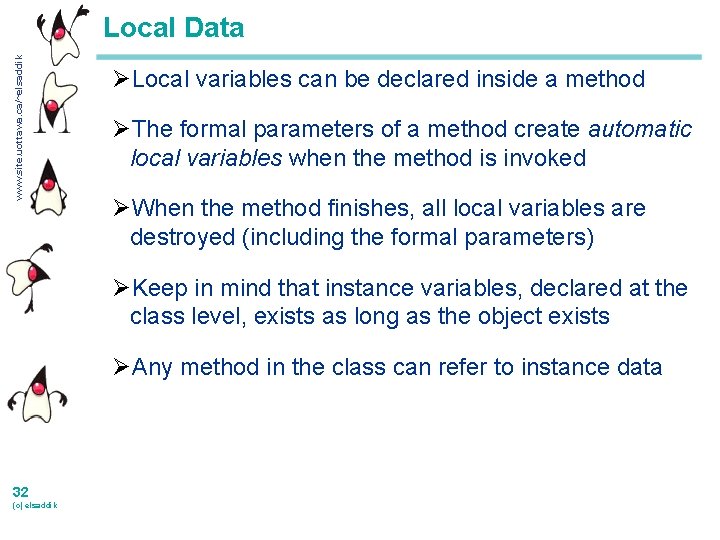
www. site. uottawa. ca/~elsaddik Local Data ØLocal variables can be declared inside a method ØThe formal parameters of a method create automatic local variables when the method is invoked ØWhen the method finishes, all local variables are destroyed (including the formal parameters) ØKeep in mind that instance variables, declared at the class level, exists as long as the object exists ØAny method in the class can refer to instance data 32 (c) elsaddik
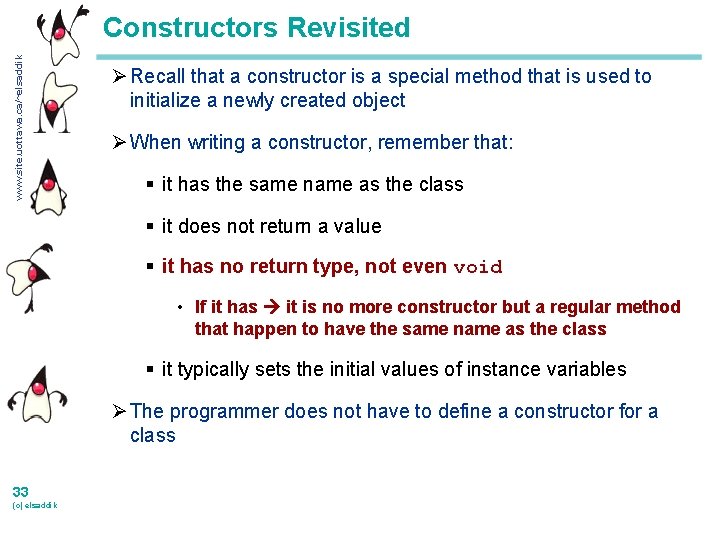
www. site. uottawa. ca/~elsaddik Constructors Revisited Ø Recall that a constructor is a special method that is used to initialize a newly created object Ø When writing a constructor, remember that: § it has the same name as the class § it does not return a value § it has no return type, not even void • If it has it is no more constructor but a regular method that happen to have the same name as the class § it typically sets the initial values of instance variables Ø The programmer does not have to define a constructor for a class 33 (c) elsaddik
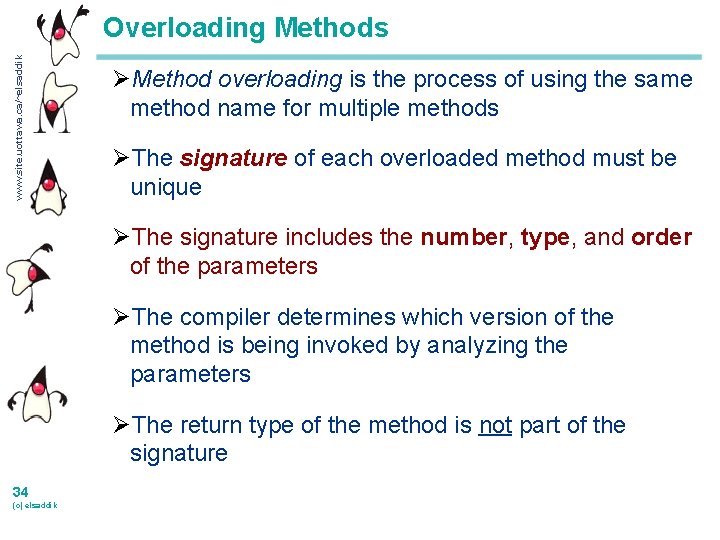
www. site. uottawa. ca/~elsaddik Overloading Methods ØMethod overloading is the process of using the same method name for multiple methods ØThe signature of each overloaded method must be unique ØThe signature includes the number, type, and order of the parameters ØThe compiler determines which version of the method is being invoked by analyzing the parameters ØThe return type of the method is not part of the signature 34 (c) elsaddik
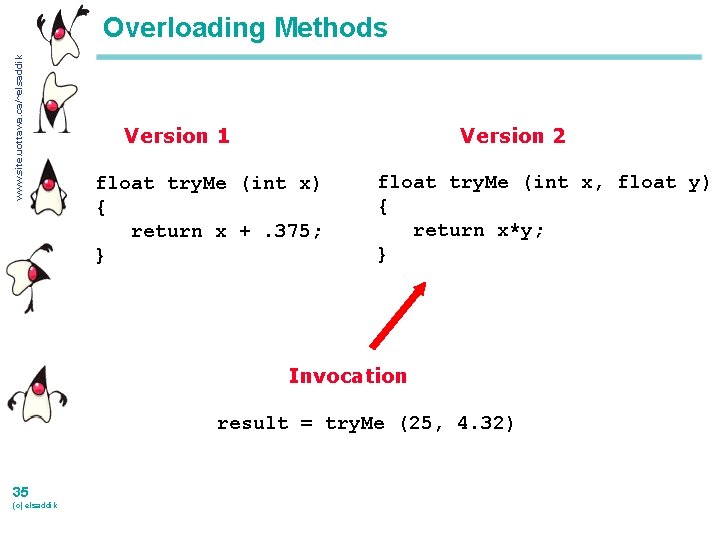
www. site. uottawa. ca/~elsaddik Overloading Methods Version 1 Version 2 float try. Me (int x) { return x +. 375; } float try. Me (int x, float y) { return x*y; } Invocation result = try. Me (25, 4. 32) 35 (c) elsaddik
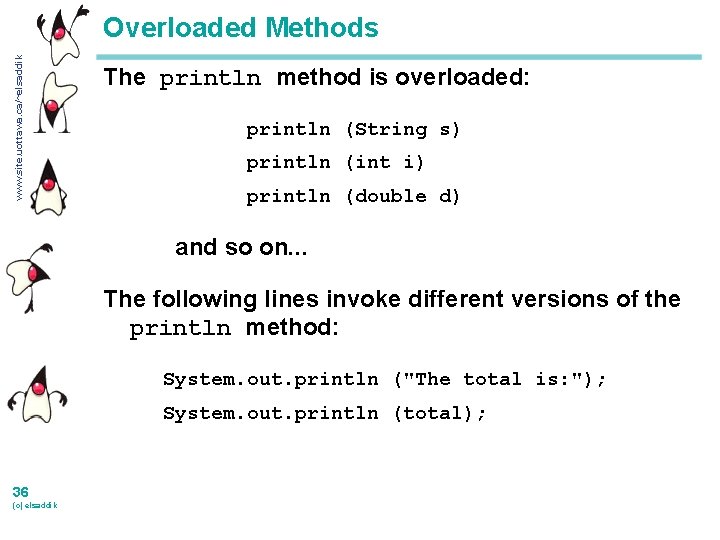
www. site. uottawa. ca/~elsaddik Overloaded Methods The println method is overloaded: println (String s) println (int i) println (double d) and so on. . . The following lines invoke different versions of the println method: System. out. println ("The total is: "); System. out. println (total); 36 (c) elsaddik
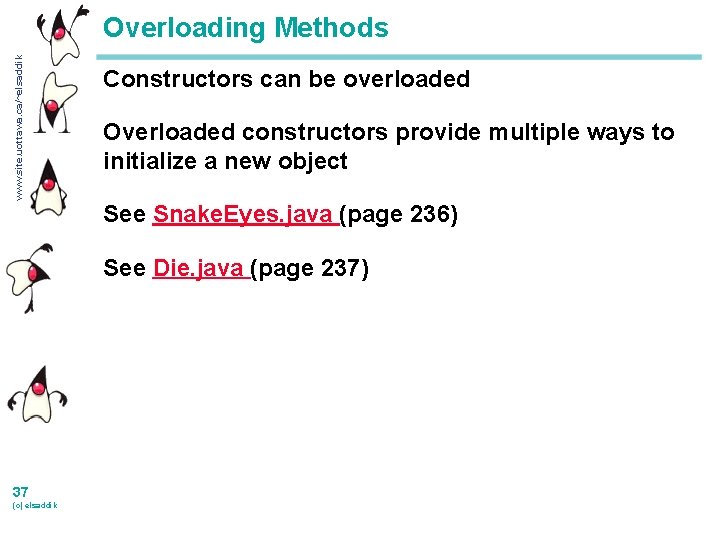
www. site. uottawa. ca/~elsaddik Overloading Methods Constructors can be overloaded Overloaded constructors provide multiple ways to initialize a new object See Snake. Eyes. java (page 236) See Die. java (page 237) 37 (c) elsaddik
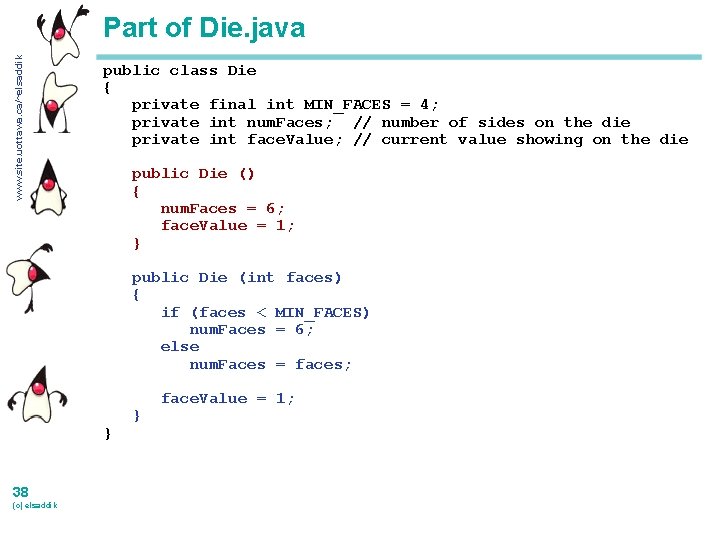
www. site. uottawa. ca/~elsaddik Part of Die. java public class Die { private final int MIN_FACES = 4; private int num. Faces; // number of sides on the die private int face. Value; // current value showing on the die public Die () { num. Faces = 6; face. Value = 1; } public Die (int faces) { if (faces < MIN_FACES) num. Faces = 6; else num. Faces = faces; } 38 (c) elsaddik } face. Value = 1;
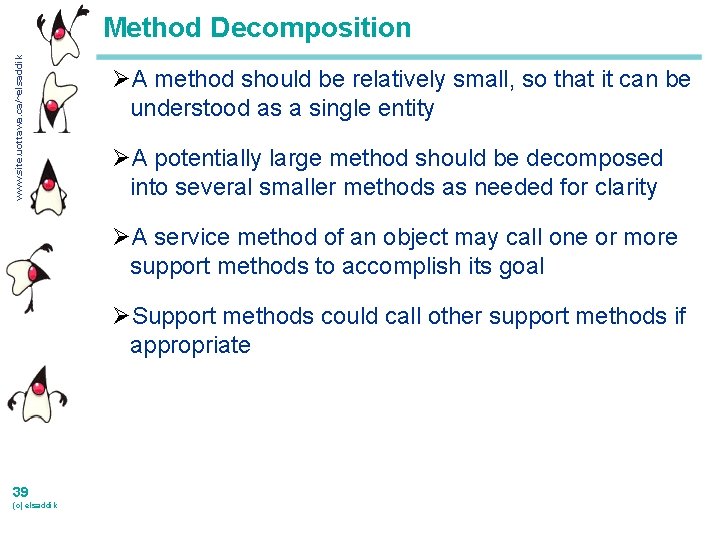
www. site. uottawa. ca/~elsaddik Method Decomposition ØA method should be relatively small, so that it can be understood as a single entity ØA potentially large method should be decomposed into several smaller methods as needed for clarity ØA service method of an object may call one or more support methods to accomplish its goal ØSupport methods could call other support methods if appropriate 39 (c) elsaddik
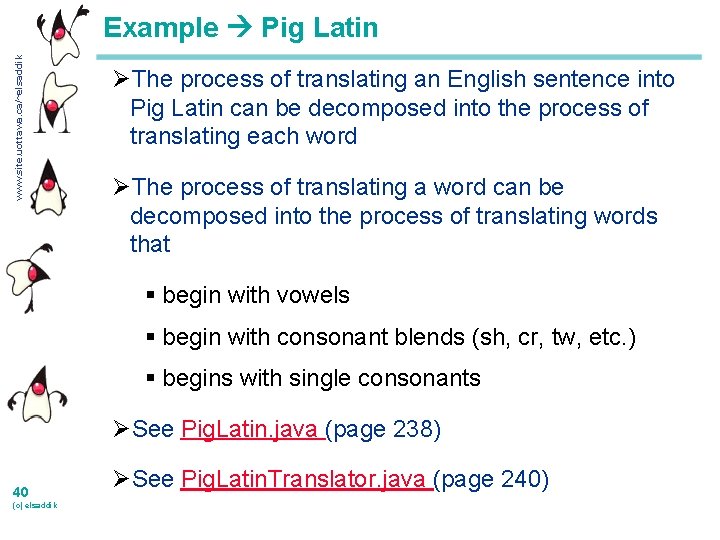
www. site. uottawa. ca/~elsaddik Example Pig Latin ØThe process of translating an English sentence into Pig Latin can be decomposed into the process of translating each word ØThe process of translating a word can be decomposed into the process of translating words that § begin with vowels § begin with consonant blends (sh, cr, tw, etc. ) § begins with single consonants ØSee Pig. Latin. java (page 238) 40 (c) elsaddik ØSee Pig. Latin. Translator. java (page 240)
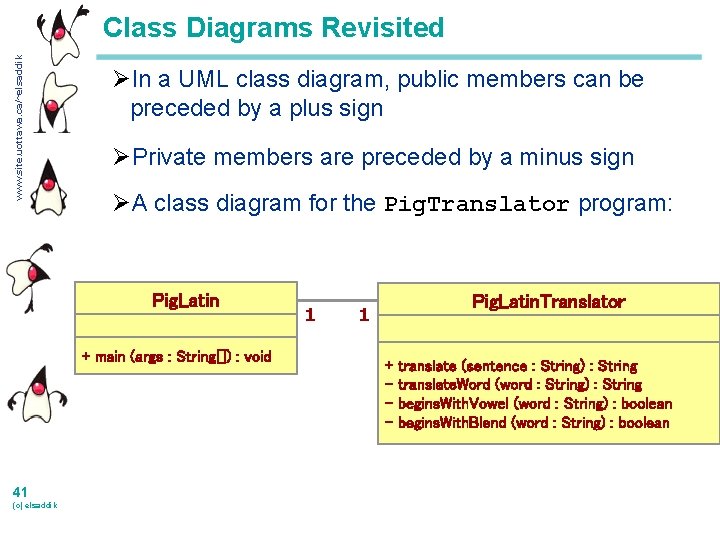
www. site. uottawa. ca/~elsaddik Class Diagrams Revisited ØIn a UML class diagram, public members can be preceded by a plus sign ØPrivate members are preceded by a minus sign ØA class diagram for the Pig. Translator program: Pig. Latin + main (args : String[]) : void 41 (c) elsaddik 1 Pig. Latin. Translator 1 + - translate (sentence : String) : String translate. Word (word : String) : String begins. With. Vowel (word : String) : boolean begins. With. Blend (word : String) : boolean
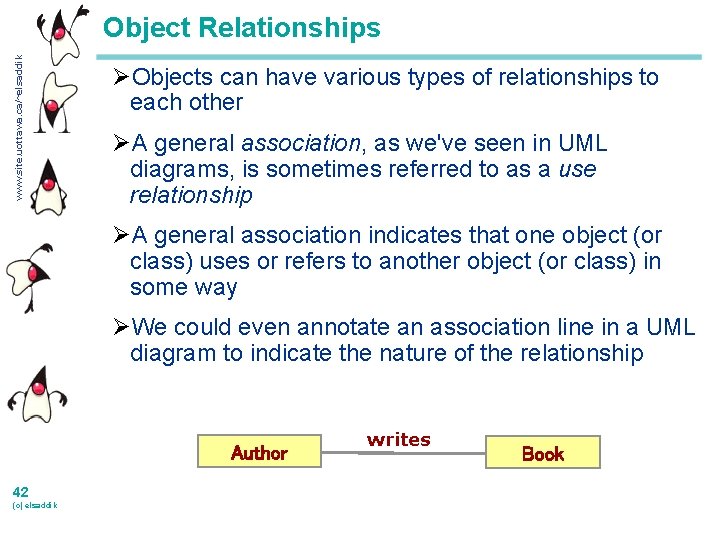
www. site. uottawa. ca/~elsaddik Object Relationships ØObjects can have various types of relationships to each other ØA general association, as we've seen in UML diagrams, is sometimes referred to as a use relationship ØA general association indicates that one object (or class) uses or refers to another object (or class) in some way ØWe could even annotate an association line in a UML diagram to indicate the nature of the relationship Author 42 (c) elsaddik writes Book
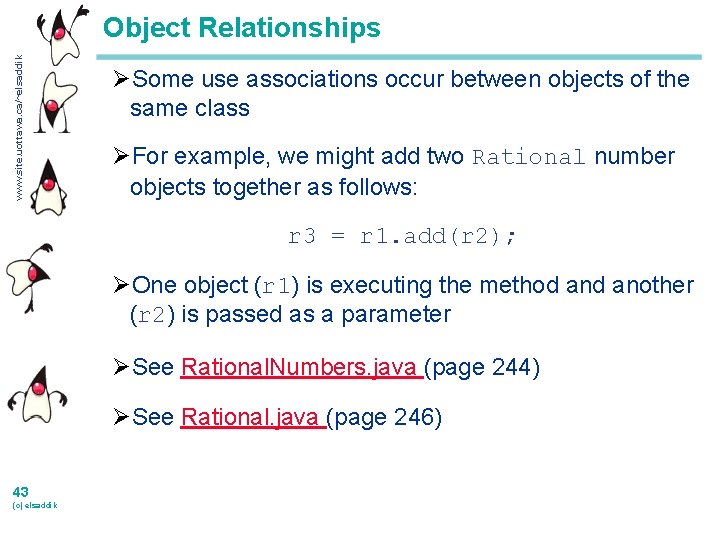
www. site. uottawa. ca/~elsaddik Object Relationships ØSome use associations occur between objects of the same class ØFor example, we might add two Rational number objects together as follows: r 3 = r 1. add(r 2); ØOne object (r 1) is executing the method another (r 2) is passed as a parameter ØSee Rational. Numbers. java (page 244) ØSee Rational. java (page 246) 43 (c) elsaddik
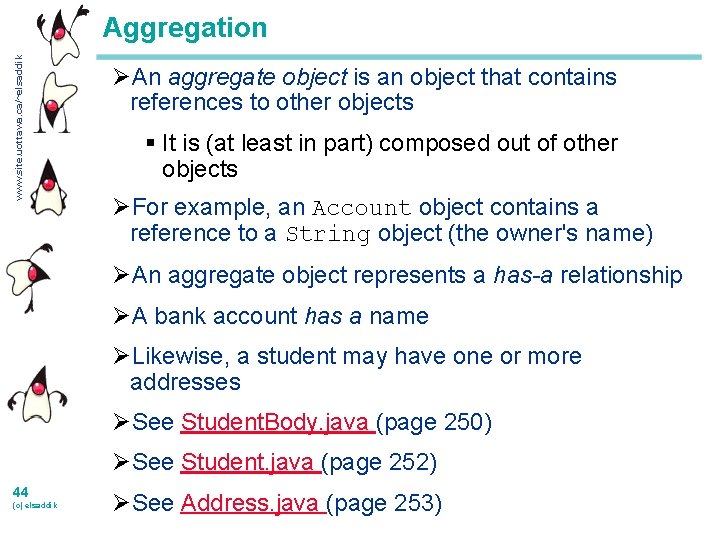
www. site. uottawa. ca/~elsaddik Aggregation ØAn aggregate object is an object that contains references to other objects § It is (at least in part) composed out of other objects ØFor example, an Account object contains a reference to a String object (the owner's name) ØAn aggregate object represents a has-a relationship ØA bank account has a name ØLikewise, a student may have one or more addresses ØSee Student. Body. java (page 250) ØSee Student. java (page 252) 44 (c) elsaddik ØSee Address. java (page 253)
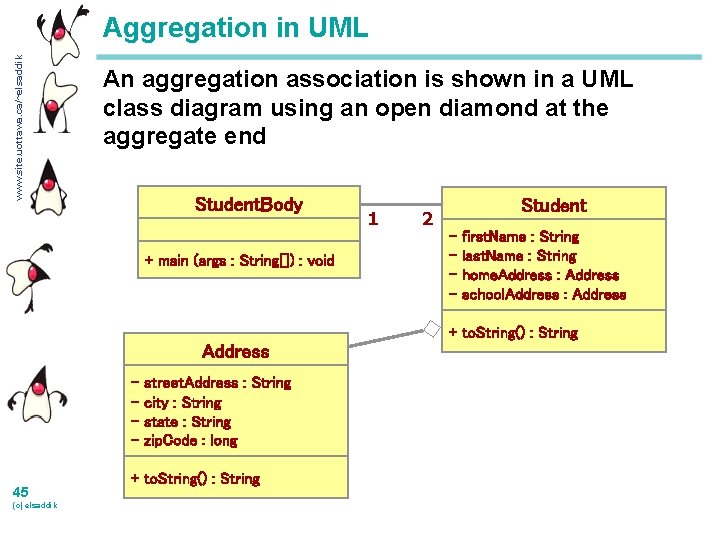
www. site. uottawa. ca/~elsaddik Aggregation in UML An aggregation association is shown in a UML class diagram using an open diamond at the aggregate end Student. Body + main (args : String[]) : void 1 2 Student - first. Name : String last. Name : String home. Address : Address school. Address : Address + to. String() : String Address 45 (c) elsaddik street. Address : String city : String state : String zip. Code : long + to. String() : String
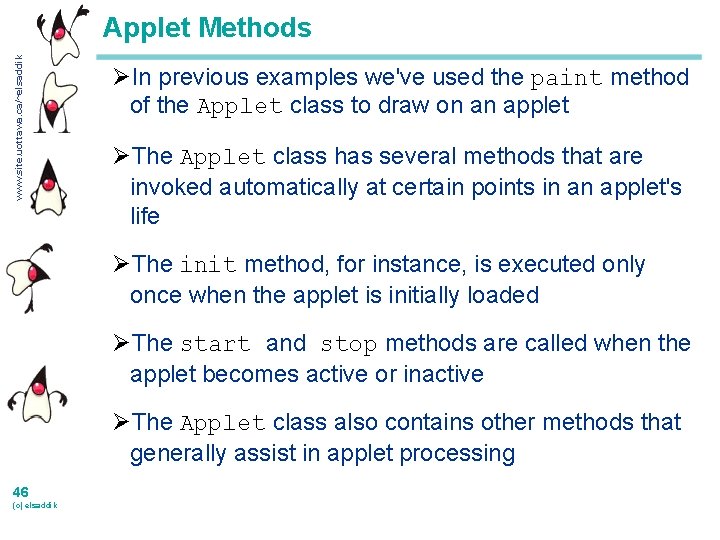
www. site. uottawa. ca/~elsaddik Applet Methods ØIn previous examples we've used the paint method of the Applet class to draw on an applet ØThe Applet class has several methods that are invoked automatically at certain points in an applet's life ØThe init method, for instance, is executed only once when the applet is initially loaded ØThe start and stop methods are called when the applet becomes active or inactive ØThe Applet class also contains other methods that generally assist in applet processing 46 (c) elsaddik
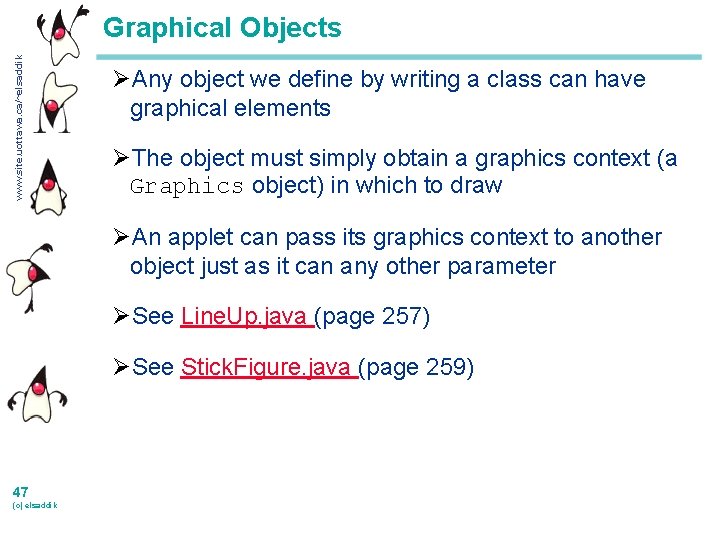
www. site. uottawa. ca/~elsaddik Graphical Objects ØAny object we define by writing a class can have graphical elements ØThe object must simply obtain a graphics context (a Graphics object) in which to draw ØAn applet can pass its graphics context to another object just as it can any other parameter ØSee Line. Up. java (page 257) ØSee Stick. Figure. java (page 259) 47 (c) elsaddik
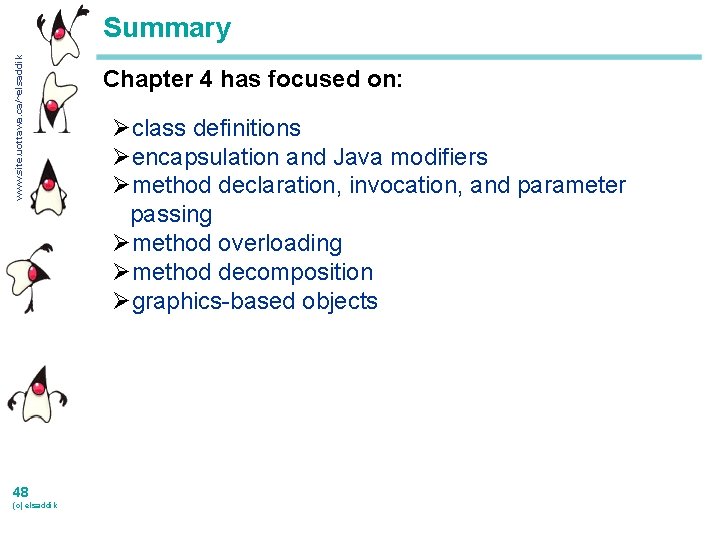
www. site. uottawa. ca/~elsaddik Summary 48 (c) elsaddik Chapter 4 has focused on: Øclass definitions Øencapsulation and Java modifiers Ømethod declaration, invocation, and parameter passing Ømethod overloading Ømethod decomposition Øgraphics-based objects
Csi uottawa
Csi uottawa
Hacer clic en ingreso al sistema 1102-404
Comp sci 1102
Chemsheets
Phil 1102
Komplemen 9 dari 458
Bps4104
Dr daniel dubois
Uottawa ste
Uottawa notation
Scopus uottawa
Dgd uottawa
Software engineering uottawa course sequence
Uottawa seg course sequence
Premed101 uottawa
Genie uottawa
Verena kantere uottawa
Genie uottawa
Csi-321 introduction to computing applications
Hot site cold site warm site disaster recovery
Csi 3120
Csi3120
Csi 321
Csi in project management
Csi handwriting analysis
Csi varese
Csi framework
Csi-2 to usb
Csi 3140
Csi 3140
Csi 3140
Csi 3140
Csi 3131
Elg 2911
Elg2911
While the csi team is searching the crime scene, _____.
Csi great lakes region
Bnl csi
Corporate spending innovations (csi)
1 penny challenge
Csi 2132
Project scheduling in software engineering
Master csi
Law & order: criminal mind
Cara menghitung csi
Csi xcad
Csi como 7
Csi 3531