Writing Classes You have already used classes String
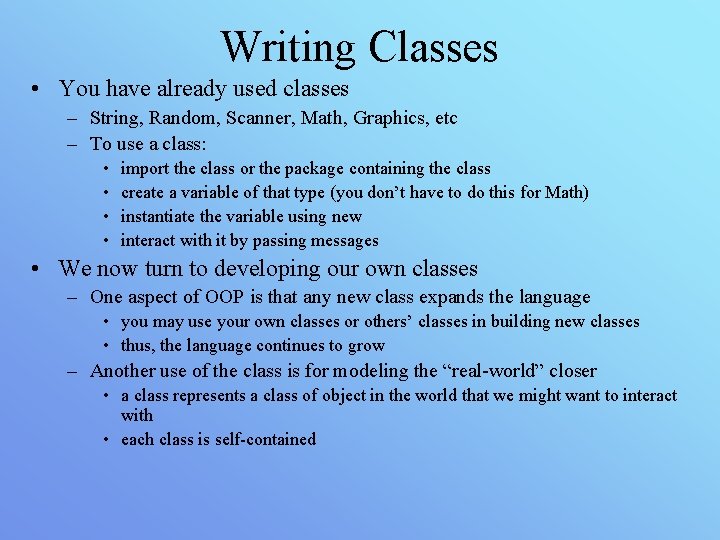
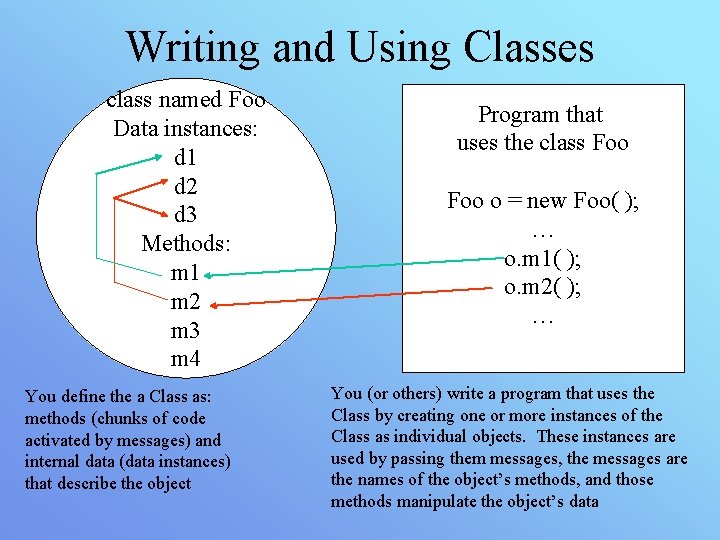
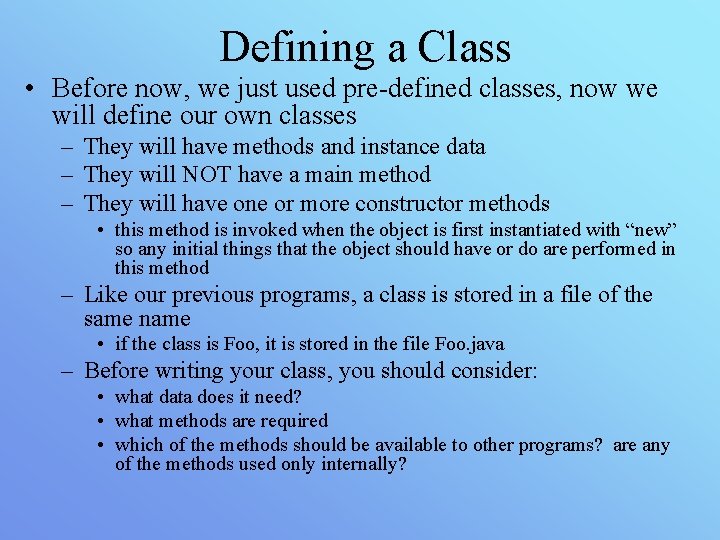
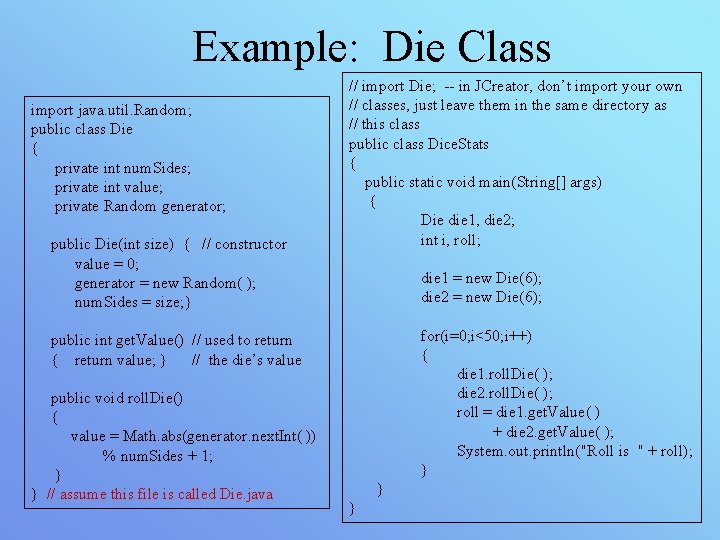
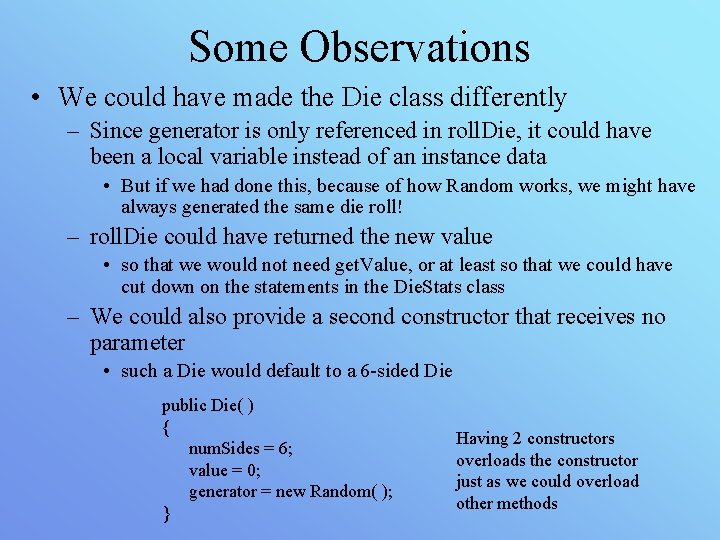
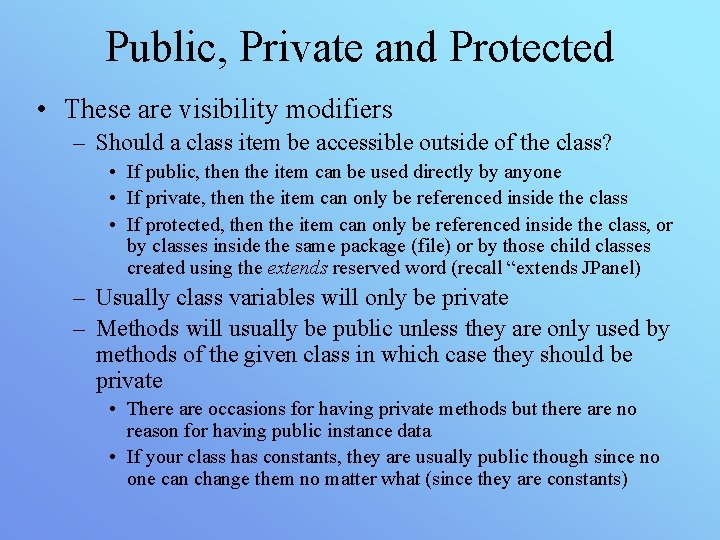
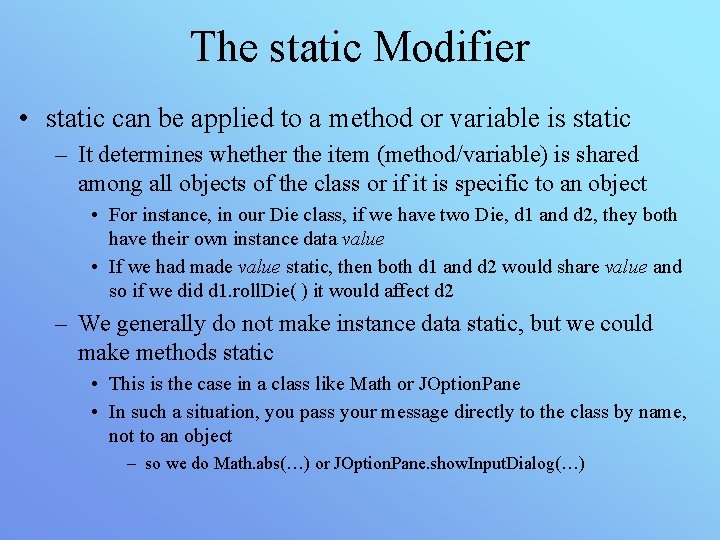
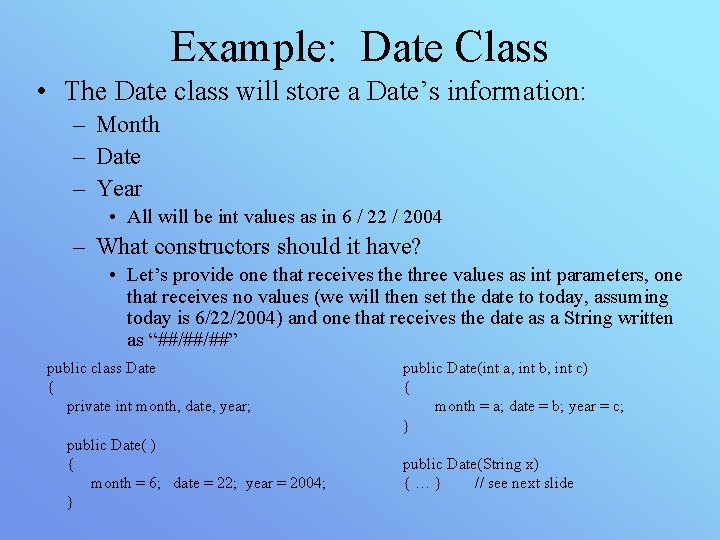
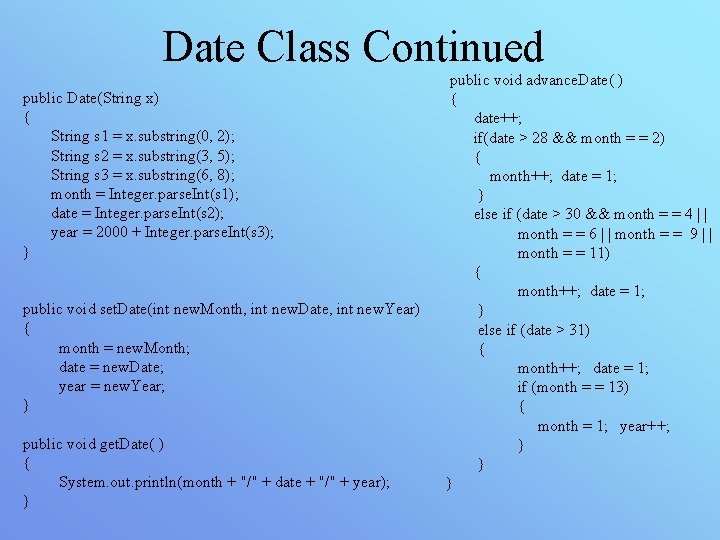
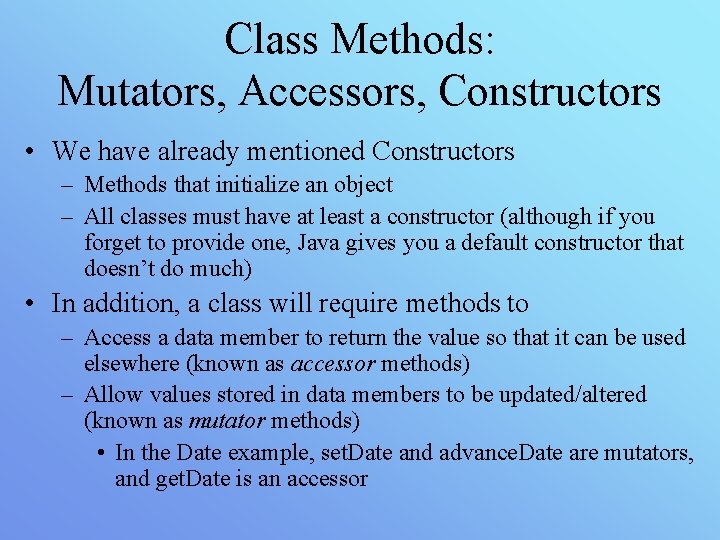
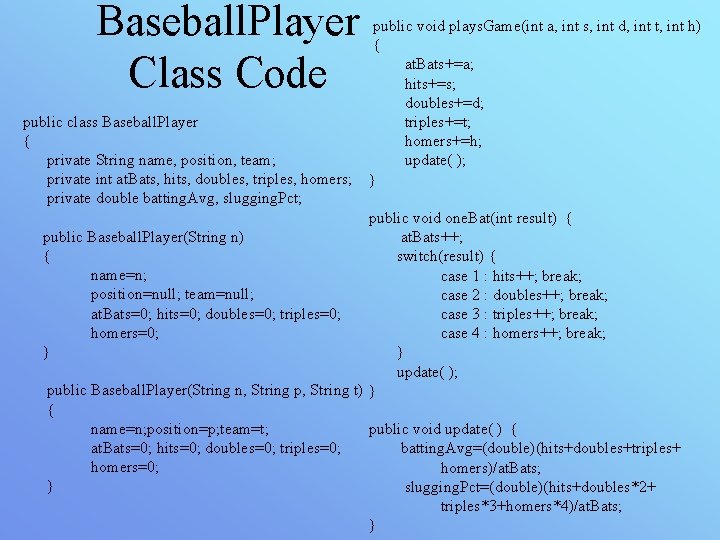
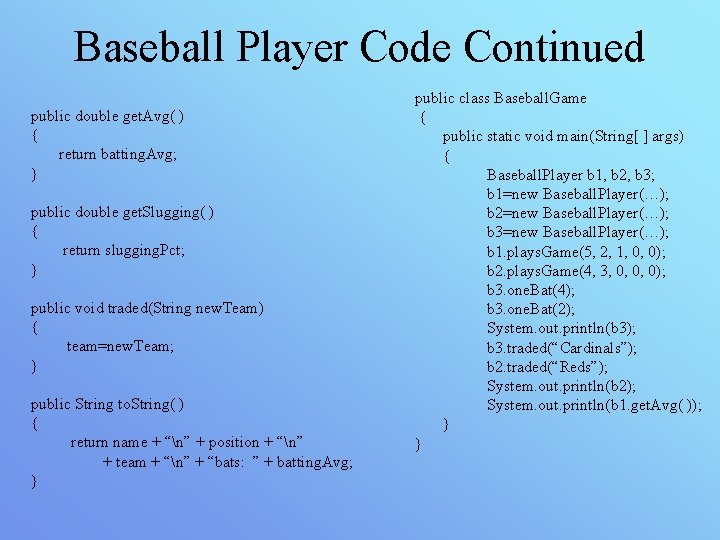
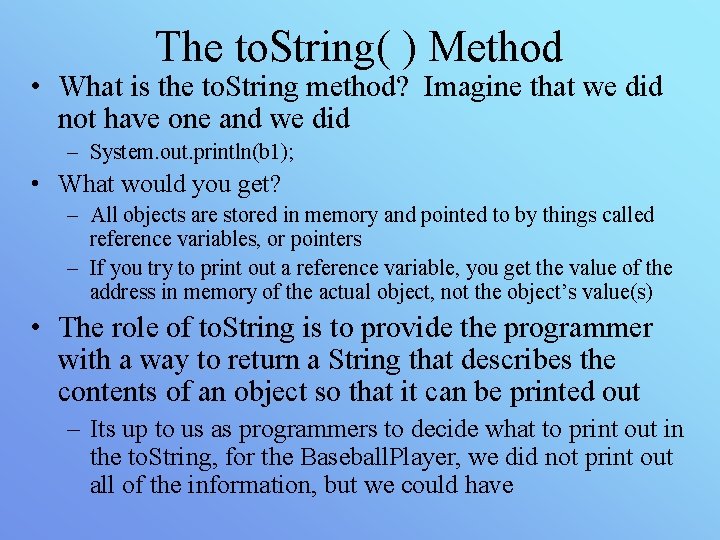
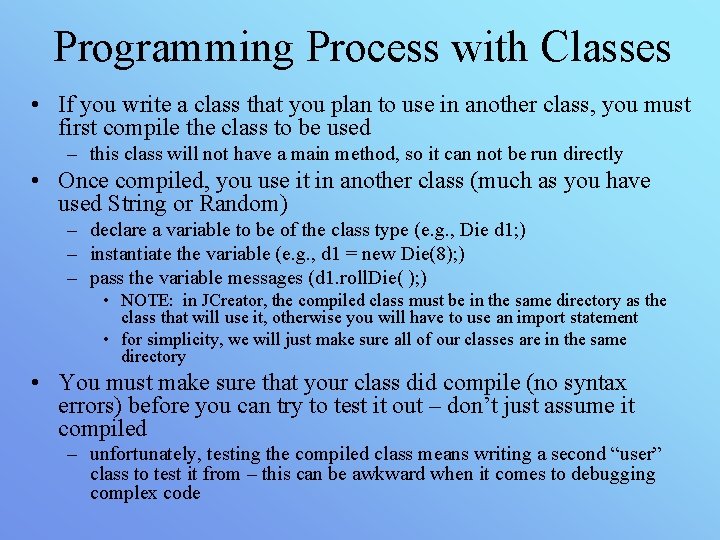
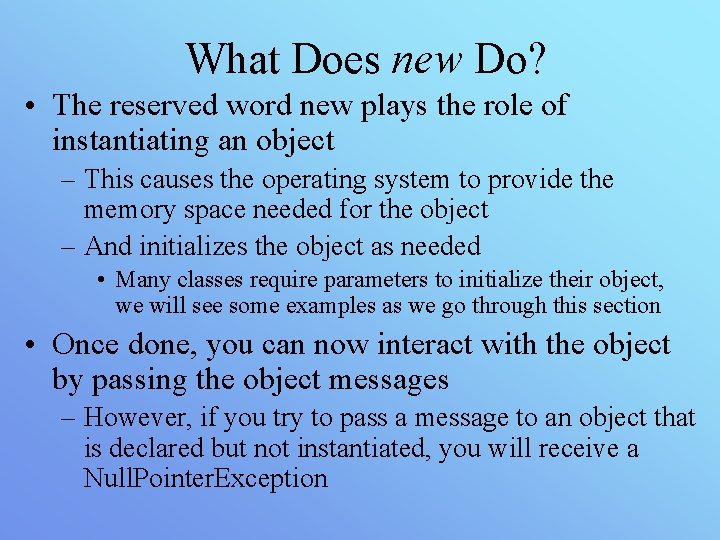
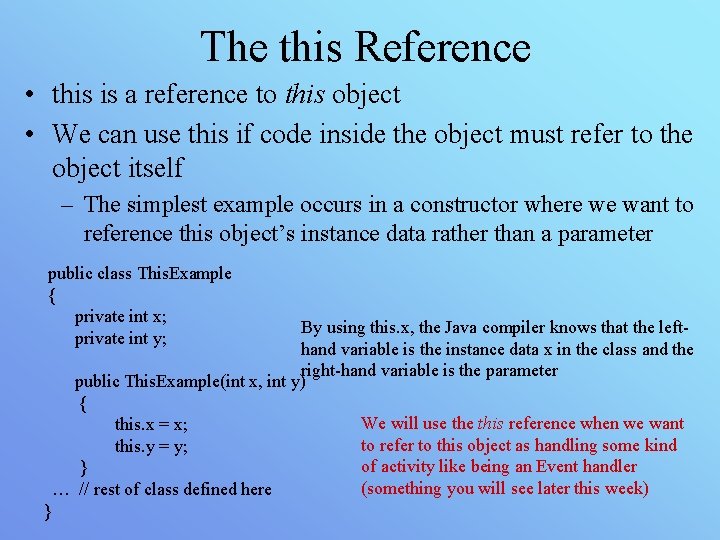
- Slides: 16
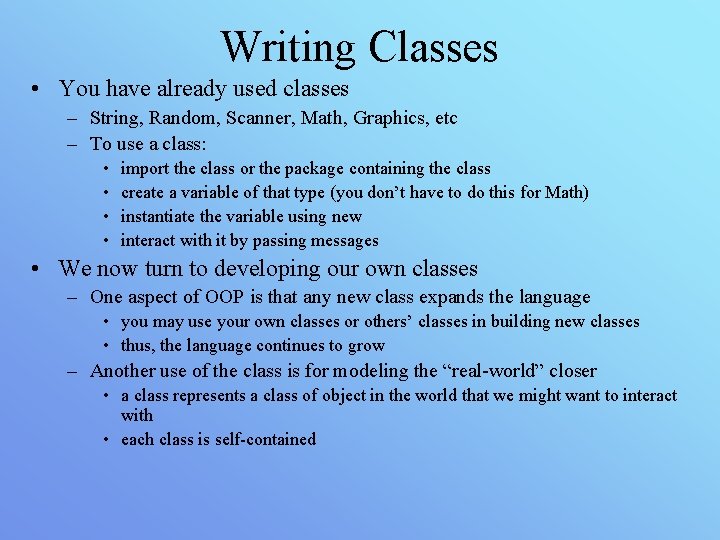
Writing Classes • You have already used classes – String, Random, Scanner, Math, Graphics, etc – To use a class: • • import the class or the package containing the class create a variable of that type (you don’t have to do this for Math) instantiate the variable using new interact with it by passing messages • We now turn to developing our own classes – One aspect of OOP is that any new class expands the language • you may use your own classes or others’ classes in building new classes • thus, the language continues to grow – Another use of the class is for modeling the “real-world” closer • a class represents a class of object in the world that we might want to interact with • each class is self-contained
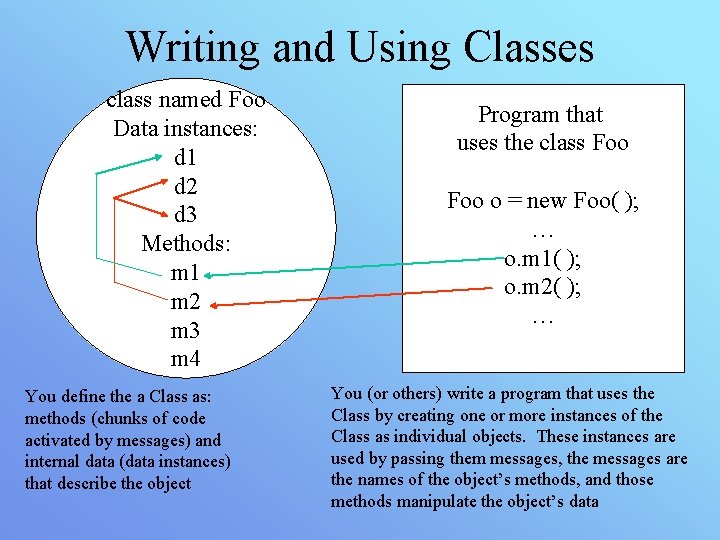
Writing and Using Classes class named Foo Data instances: d 1 d 2 d 3 Methods: m 1 m 2 m 3 m 4 You define the a Class as: methods (chunks of code activated by messages) and internal data (data instances) that describe the object Program that uses the class Foo o = new Foo( ); … o. m 1( ); o. m 2( ); … You (or others) write a program that uses the Class by creating one or more instances of the Class as individual objects. These instances are used by passing them messages, the messages are the names of the object’s methods, and those methods manipulate the object’s data
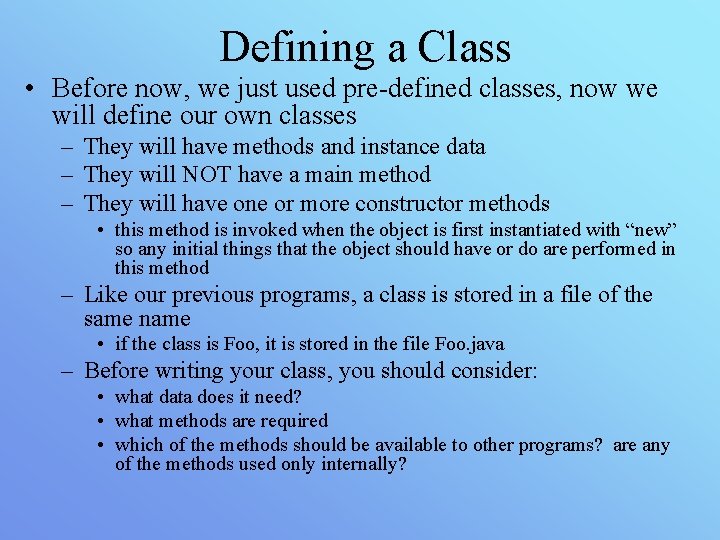
Defining a Class • Before now, we just used pre-defined classes, now we will define our own classes – They will have methods and instance data – They will NOT have a main method – They will have one or more constructor methods • this method is invoked when the object is first instantiated with “new” so any initial things that the object should have or do are performed in this method – Like our previous programs, a class is stored in a file of the same name • if the class is Foo, it is stored in the file Foo. java – Before writing your class, you should consider: • what data does it need? • what methods are required • which of the methods should be available to other programs? are any of the methods used only internally?
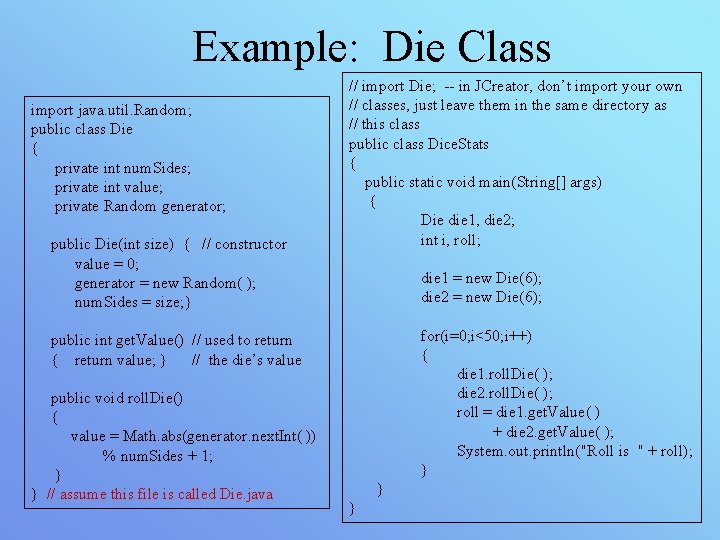
Example: Die Class import java. util. Random; public class Die { private int num. Sides; private int value; private Random generator; public Die(int size) { // constructor value = 0; generator = new Random( ); num. Sides = size; } // import Die; -- in JCreator, don’t import your own // classes, just leave them in the same directory as // this class public class Dice. Stats { public static void main(String[] args) { Die die 1, die 2; int i, roll; die 1 = new Die(6); die 2 = new Die(6); for(i=0; i<50; i++) { die 1. roll. Die( ); die 2. roll. Die( ); roll = die 1. get. Value( ) + die 2. get. Value( ); System. out. println("Roll is " + roll); } public int get. Value() // used to return { return value; } // the die’s value public void roll. Die() { value = Math. abs(generator. next. Int( )) % num. Sides + 1; } } // assume this file is called Die. java } }
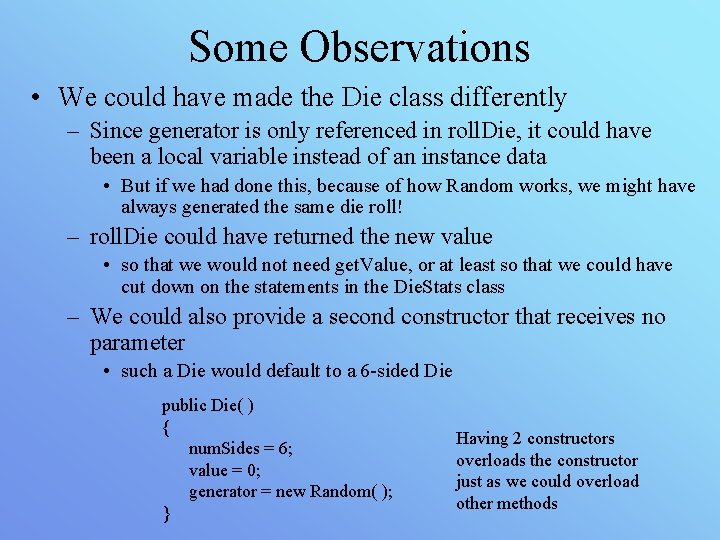
Some Observations • We could have made the Die class differently – Since generator is only referenced in roll. Die, it could have been a local variable instead of an instance data • But if we had done this, because of how Random works, we might have always generated the same die roll! – roll. Die could have returned the new value • so that we would not need get. Value, or at least so that we could have cut down on the statements in the Die. Stats class – We could also provide a second constructor that receives no parameter • such a Die would default to a 6 -sided Die public Die( ) { num. Sides = 6; value = 0; generator = new Random( ); } Having 2 constructors overloads the constructor just as we could overload other methods
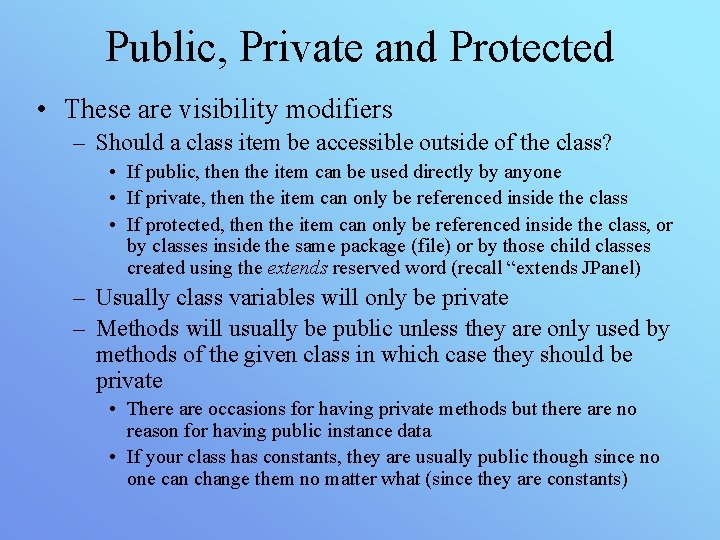
Public, Private and Protected • These are visibility modifiers – Should a class item be accessible outside of the class? • If public, then the item can be used directly by anyone • If private, then the item can only be referenced inside the class • If protected, then the item can only be referenced inside the class, or by classes inside the same package (file) or by those child classes created using the extends reserved word (recall “extends JPanel) – Usually class variables will only be private – Methods will usually be public unless they are only used by methods of the given class in which case they should be private • There are occasions for having private methods but there are no reason for having public instance data • If your class has constants, they are usually public though since no one can change them no matter what (since they are constants)
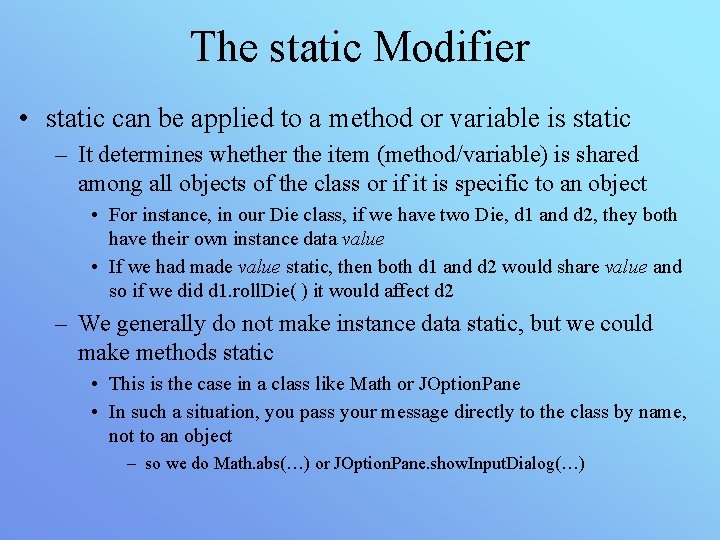
The static Modifier • static can be applied to a method or variable is static – It determines whether the item (method/variable) is shared among all objects of the class or if it is specific to an object • For instance, in our Die class, if we have two Die, d 1 and d 2, they both have their own instance data value • If we had made value static, then both d 1 and d 2 would share value and so if we did d 1. roll. Die( ) it would affect d 2 – We generally do not make instance data static, but we could make methods static • This is the case in a class like Math or JOption. Pane • In such a situation, you pass your message directly to the class by name, not to an object – so we do Math. abs(…) or JOption. Pane. show. Input. Dialog(…)
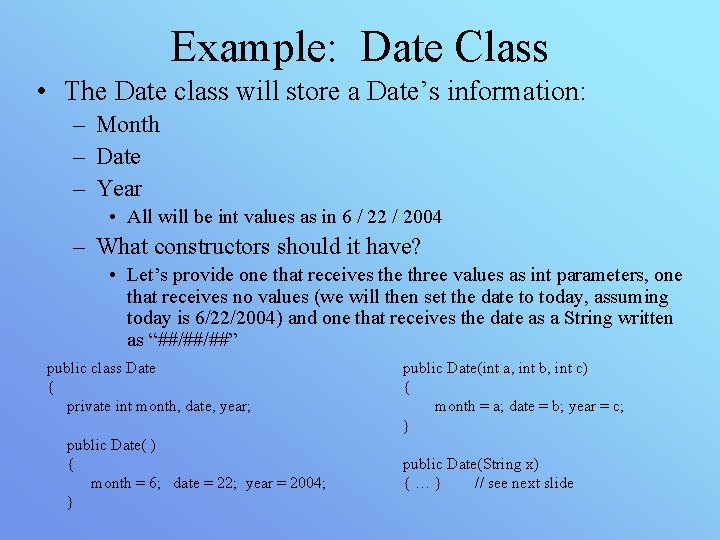
Example: Date Class • The Date class will store a Date’s information: – Month – Date – Year • All will be int values as in 6 / 22 / 2004 – What constructors should it have? • Let’s provide one that receives the three values as int parameters, one that receives no values (we will then set the date to today, assuming today is 6/22/2004) and one that receives the date as a String written as “##/##/##” public class Date { private int month, date, year; public Date( ) { month = 6; date = 22; year = 2004; } public Date(int a, int b, int c) { month = a; date = b; year = c; } public Date(String x) {…} // see next slide
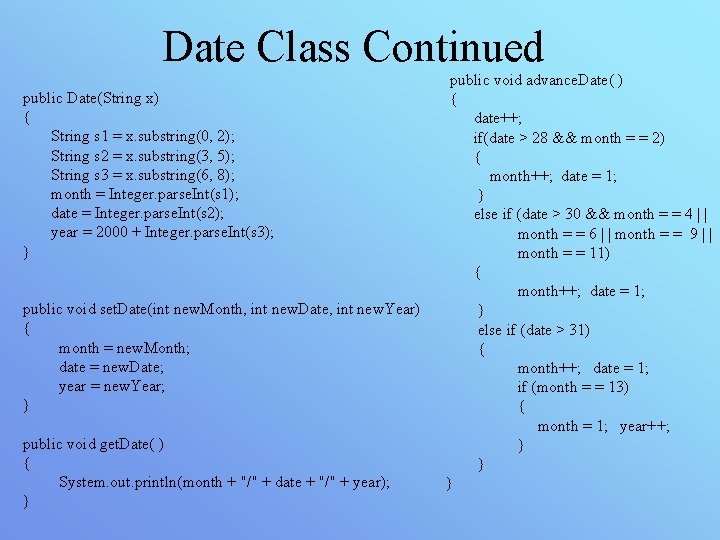
Date Class Continued public Date(String x) { String s 1 = x. substring(0, 2); String s 2 = x. substring(3, 5); String s 3 = x. substring(6, 8); month = Integer. parse. Int(s 1); date = Integer. parse. Int(s 2); year = 2000 + Integer. parse. Int(s 3); } public void set. Date(int new. Month, int new. Date, int new. Year) { month = new. Month; date = new. Date; year = new. Year; } public void get. Date( ) { System. out. println(month + "/" + date + "/" + year); } public void advance. Date( ) { date++; if(date > 28 && month = = 2) { month++; date = 1; } else if (date > 30 && month = = 4 | | month = = 6 | | month = = 9 | | month = = 11) { month++; date = 1; } else if (date > 31) { month++; date = 1; if (month = = 13) { month = 1; year++; } } }
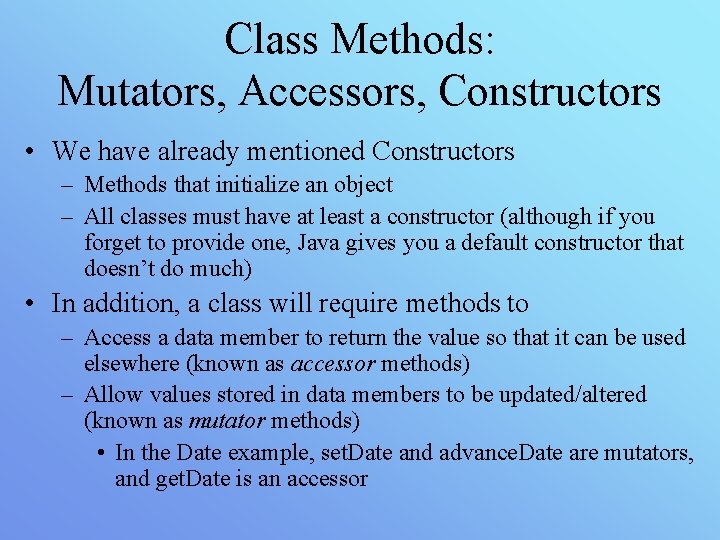
Class Methods: Mutators, Accessors, Constructors • We have already mentioned Constructors – Methods that initialize an object – All classes must have at least a constructor (although if you forget to provide one, Java gives you a default constructor that doesn’t do much) • In addition, a class will require methods to – Access a data member to return the value so that it can be used elsewhere (known as accessor methods) – Allow values stored in data members to be updated/altered (known as mutator methods) • In the Date example, set. Date and advance. Date are mutators, and get. Date is an accessor
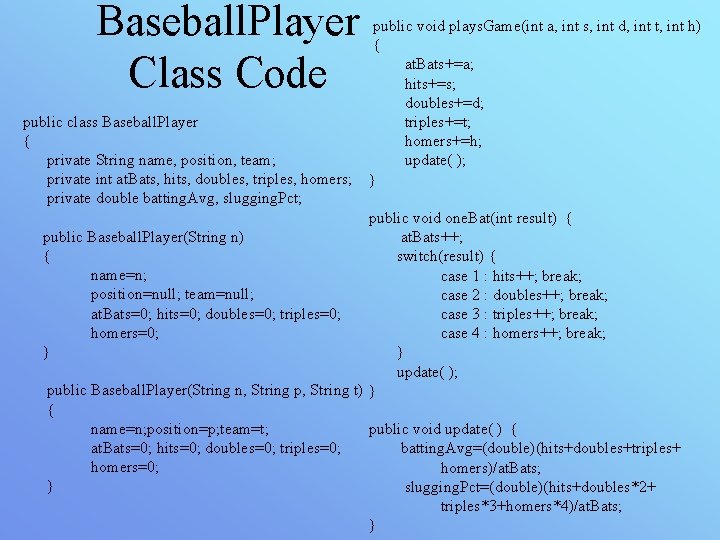
Baseball. Player Class Code public class Baseball. Player { private String name, position, team; private int at. Bats, hits, doubles, triples, homers; private double batting. Avg, slugging. Pct; public void plays. Game(int a, int s, int d, int t, int h) { at. Bats+=a; hits+=s; doubles+=d; triples+=t; homers+=h; update( ); } public void one. Bat(int result) { public Baseball. Player(String n) at. Bats++; { switch(result) { name=n; case 1 : hits++; break; position=null; team=null; case 2 : doubles++; break; at. Bats=0; hits=0; doubles=0; triples=0; case 3 : triples++; break; homers=0; case 4 : homers++; break; } } update( ); public Baseball. Player(String n, String p, String t) } { name=n; position=p; team=t; public void update( ) { at. Bats=0; hits=0; doubles=0; triples=0; batting. Avg=(double)(hits+doubles+triples+ homers=0; homers)/at. Bats; } slugging. Pct=(double)(hits+doubles*2+ triples*3+homers*4)/at. Bats; }
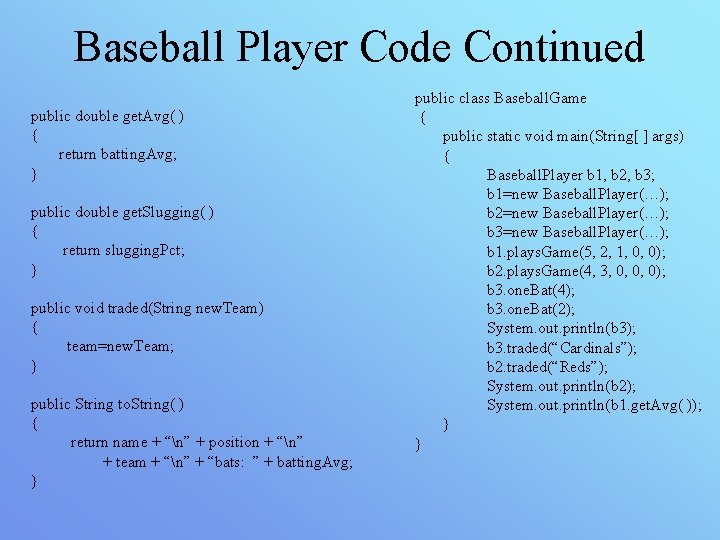
Baseball Player Code Continued public double get. Avg( ) { return batting. Avg; } public double get. Slugging( ) { return slugging. Pct; } public void traded(String new. Team) { team=new. Team; } public String to. String( ) { return name + “n” + position + “n” + team + “n” + “bats: ” + batting. Avg; } public class Baseball. Game { public static void main(String[ ] args) { Baseball. Player b 1, b 2, b 3; b 1=new Baseball. Player(…); b 2=new Baseball. Player(…); b 3=new Baseball. Player(…); b 1. plays. Game(5, 2, 1, 0, 0); b 2. plays. Game(4, 3, 0, 0, 0); b 3. one. Bat(4); b 3. one. Bat(2); System. out. println(b 3); b 3. traded(“Cardinals”); b 2. traded(“Reds”); System. out. println(b 2); System. out. println(b 1. get. Avg( )); } }
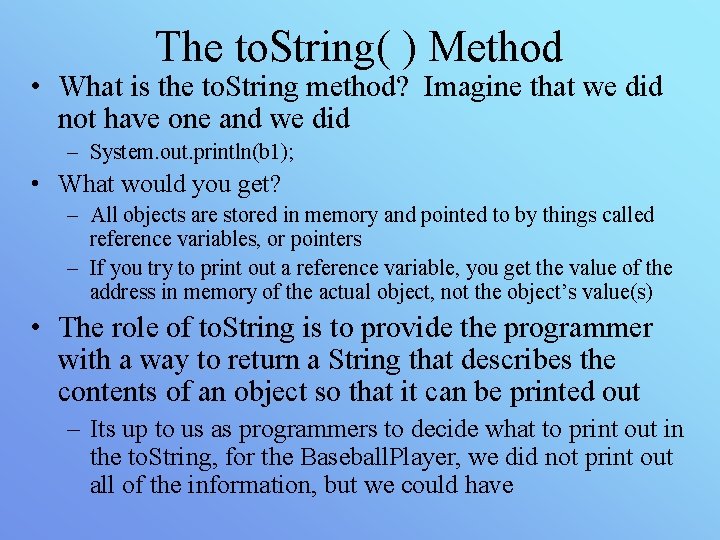
The to. String( ) Method • What is the to. String method? Imagine that we did not have one and we did – System. out. println(b 1); • What would you get? – All objects are stored in memory and pointed to by things called reference variables, or pointers – If you try to print out a reference variable, you get the value of the address in memory of the actual object, not the object’s value(s) • The role of to. String is to provide the programmer with a way to return a String that describes the contents of an object so that it can be printed out – Its up to us as programmers to decide what to print out in the to. String, for the Baseball. Player, we did not print out all of the information, but we could have
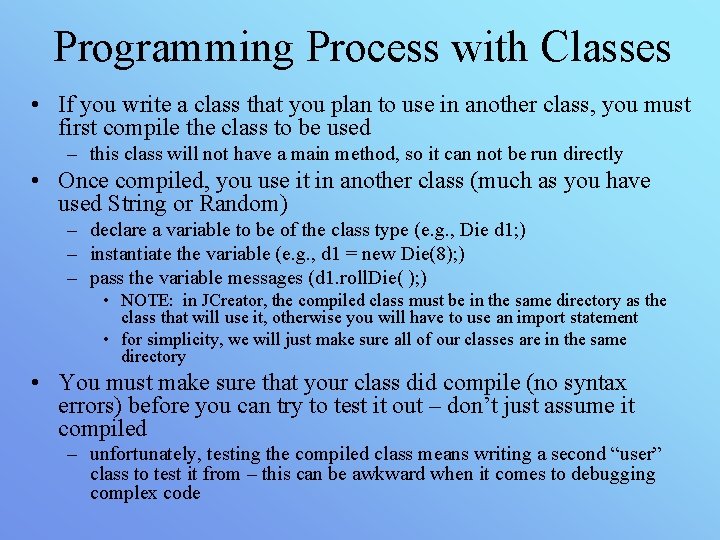
Programming Process with Classes • If you write a class that you plan to use in another class, you must first compile the class to be used – this class will not have a main method, so it can not be run directly • Once compiled, you use it in another class (much as you have used String or Random) – declare a variable to be of the class type (e. g. , Die d 1; ) – instantiate the variable (e. g. , d 1 = new Die(8); ) – pass the variable messages (d 1. roll. Die( ); ) • NOTE: in JCreator, the compiled class must be in the same directory as the class that will use it, otherwise you will have to use an import statement • for simplicity, we will just make sure all of our classes are in the same directory • You must make sure that your class did compile (no syntax errors) before you can try to test it out – don’t just assume it compiled – unfortunately, testing the compiled class means writing a second “user” class to test it from – this can be awkward when it comes to debugging complex code
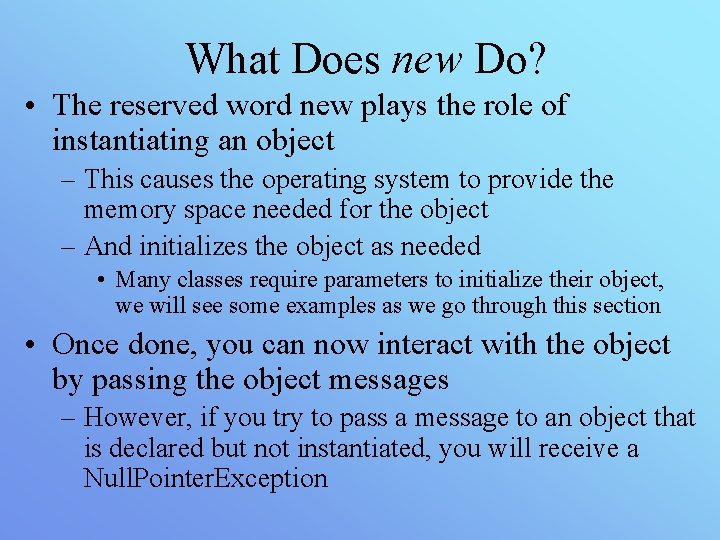
What Does new Do? • The reserved word new plays the role of instantiating an object – This causes the operating system to provide the memory space needed for the object – And initializes the object as needed • Many classes require parameters to initialize their object, we will see some examples as we go through this section • Once done, you can now interact with the object by passing the object messages – However, if you try to pass a message to an object that is declared but not instantiated, you will receive a Null. Pointer. Exception
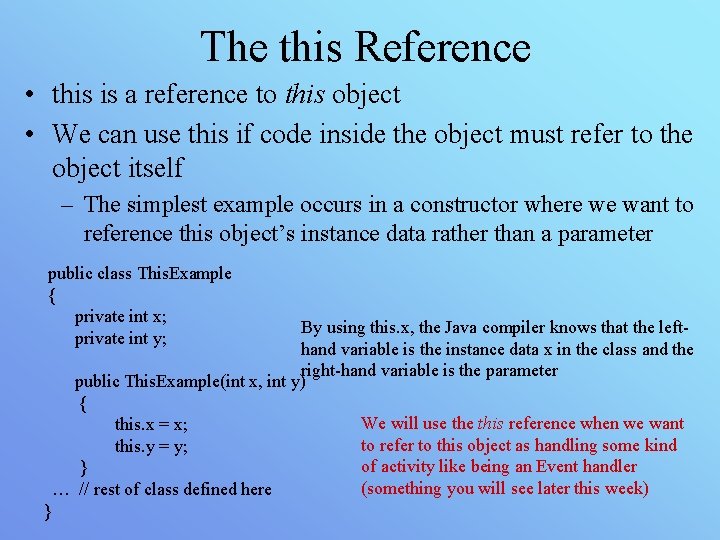
The this Reference • this is a reference to this object • We can use this if code inside the object must refer to the object itself – The simplest example occurs in a constructor where we want to reference this object’s instance data rather than a parameter public class This. Example { private int x; private int y; By using this. x, the Java compiler knows that the lefthand variable is the instance data x in the class and the right-hand variable is the parameter public This. Example(int x, int y) { We will use this reference when we want this. x = x; to refer to this object as handling some kind this. y = y; of activity like being an Event handler } (something you will see later this week) … // rest of class defined here }