WHAT ARE CODING STANDARDS Coding standards are guidelines
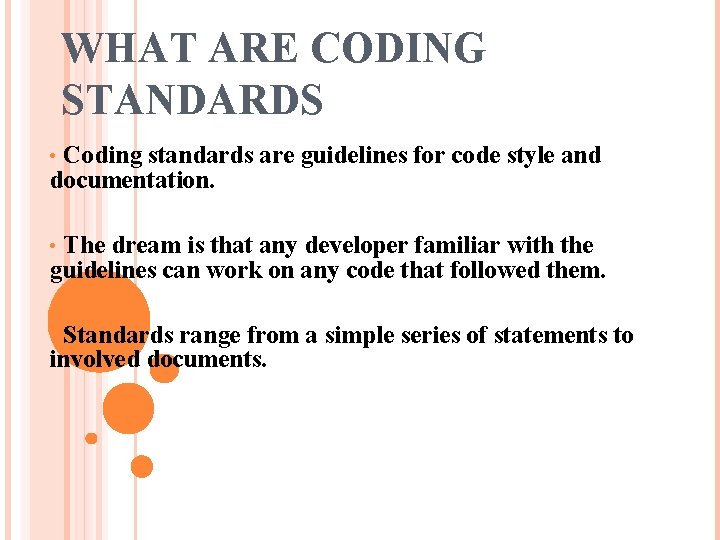
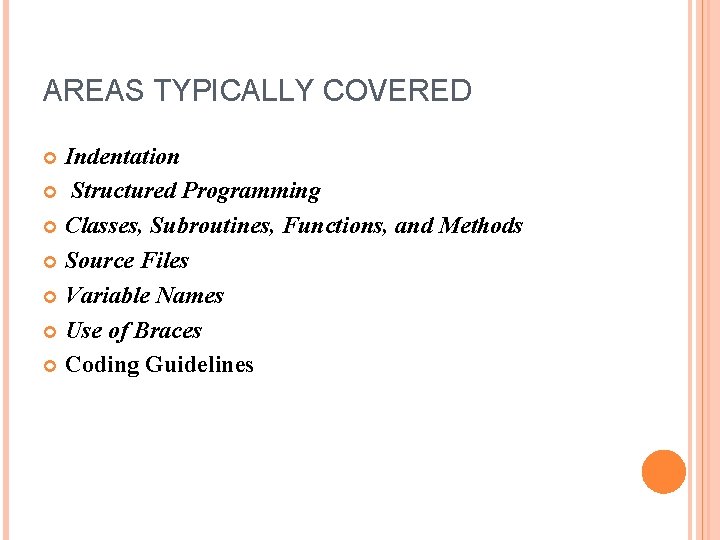
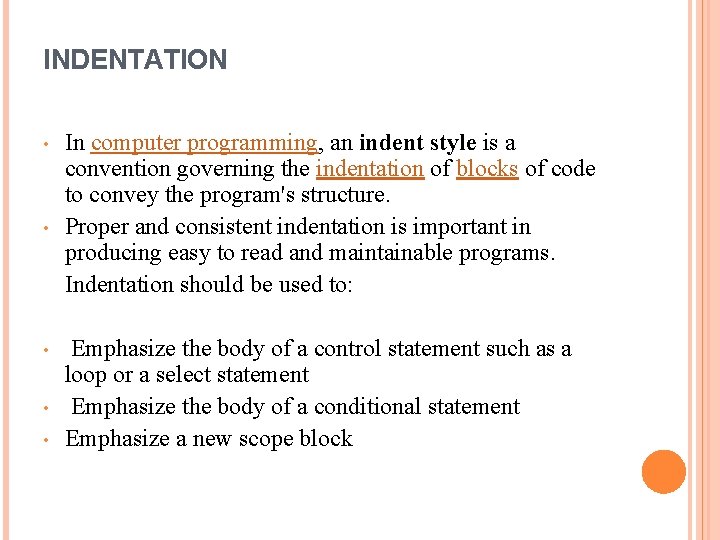
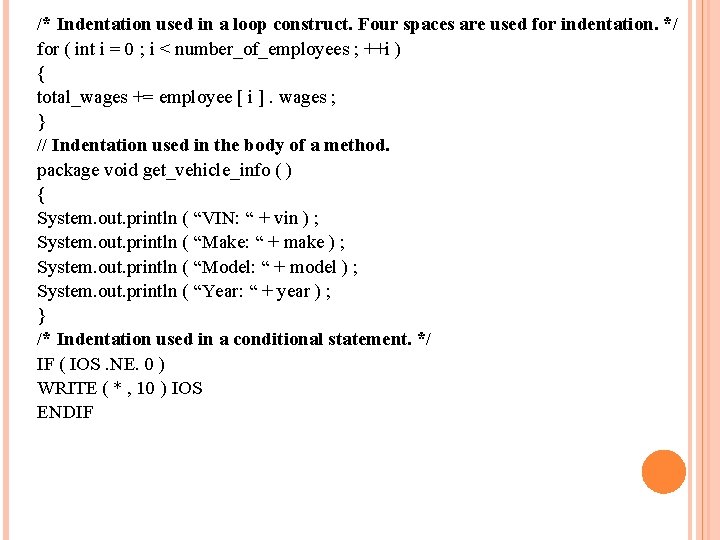
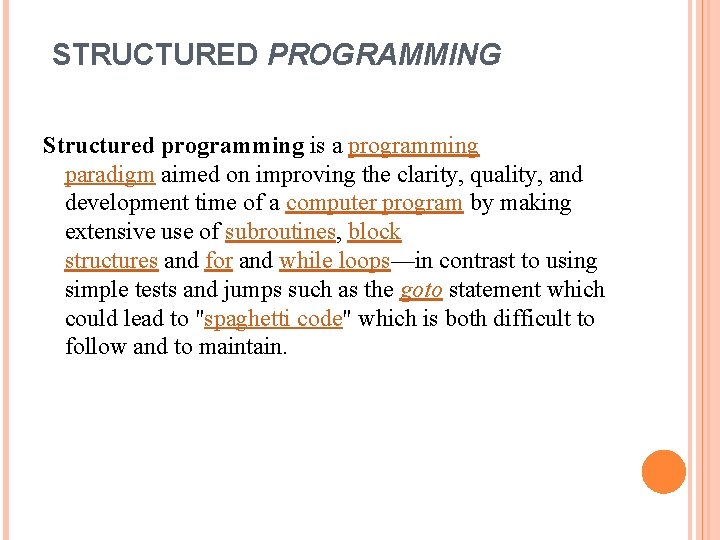
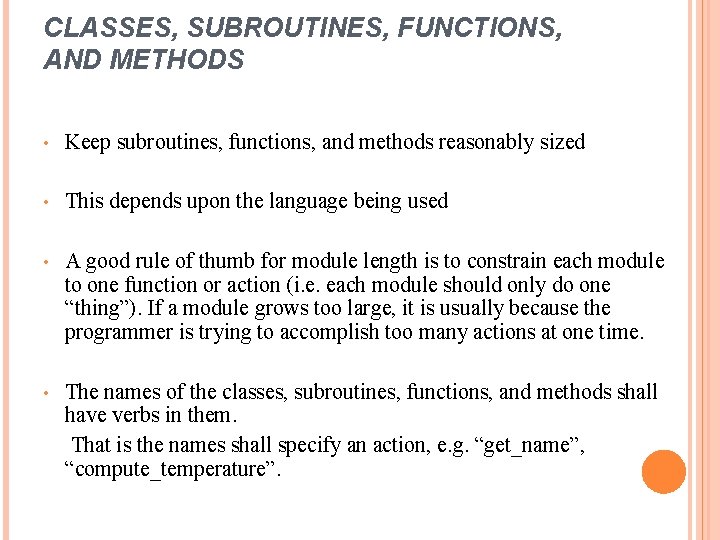
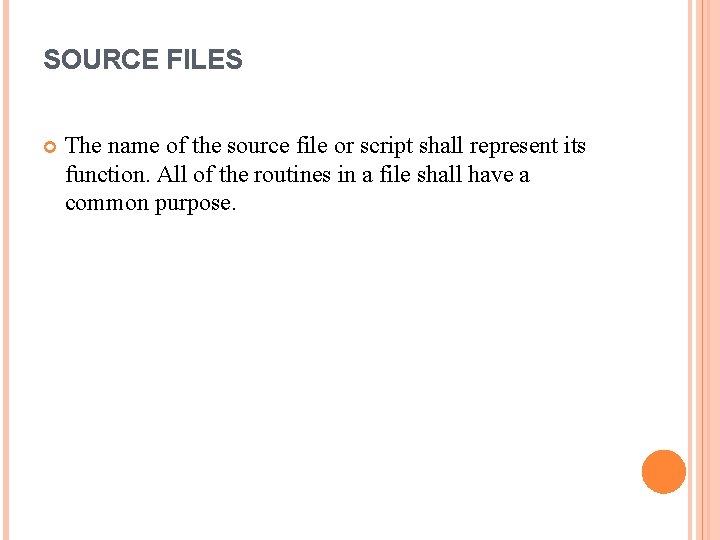
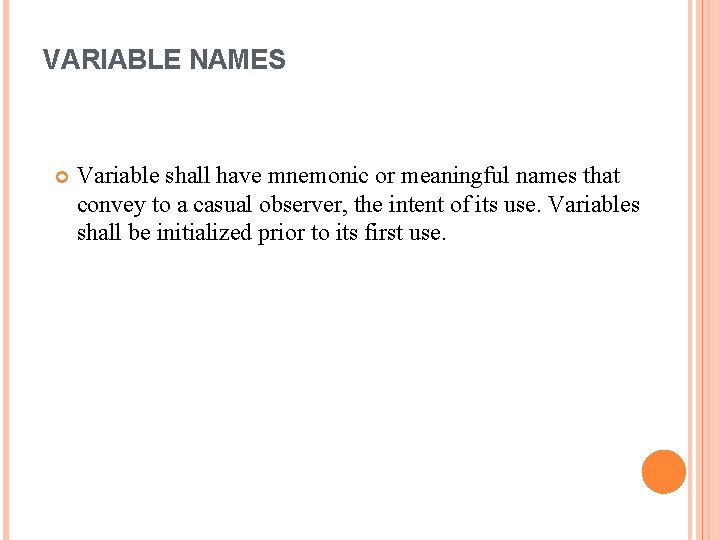
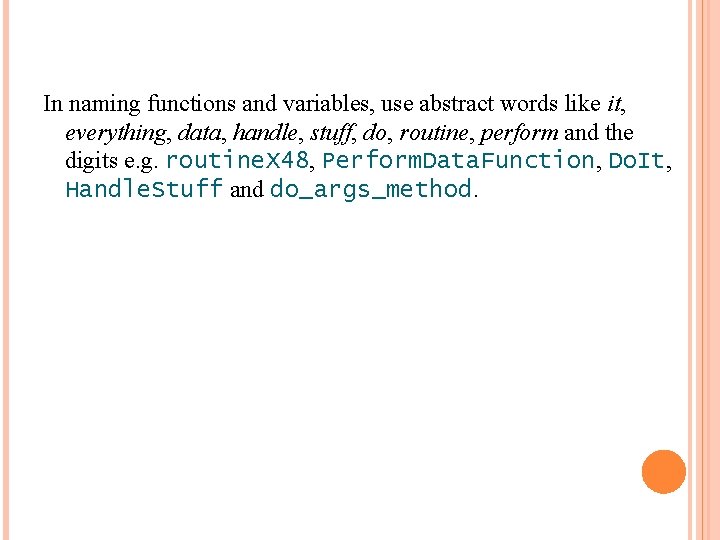
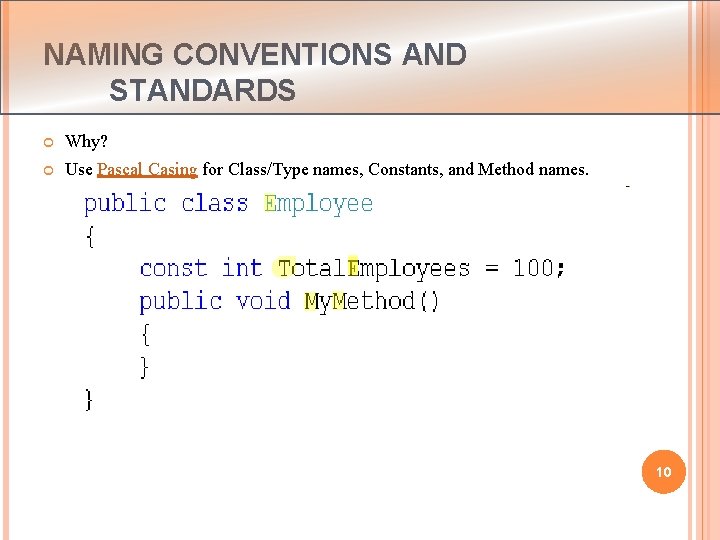
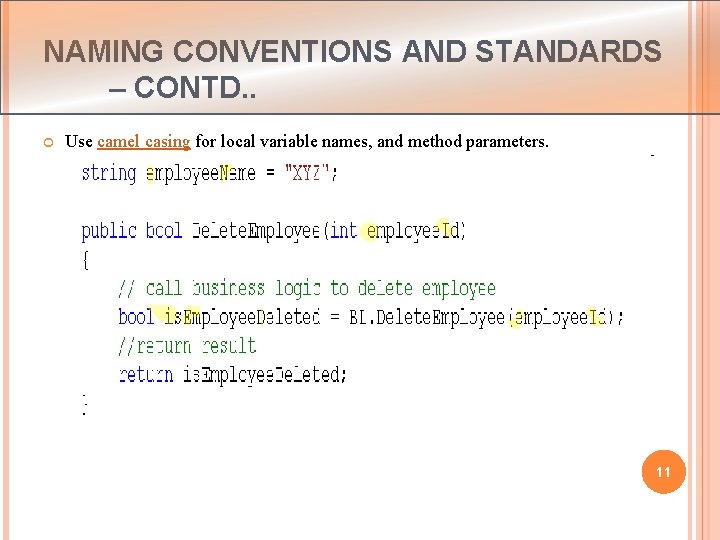
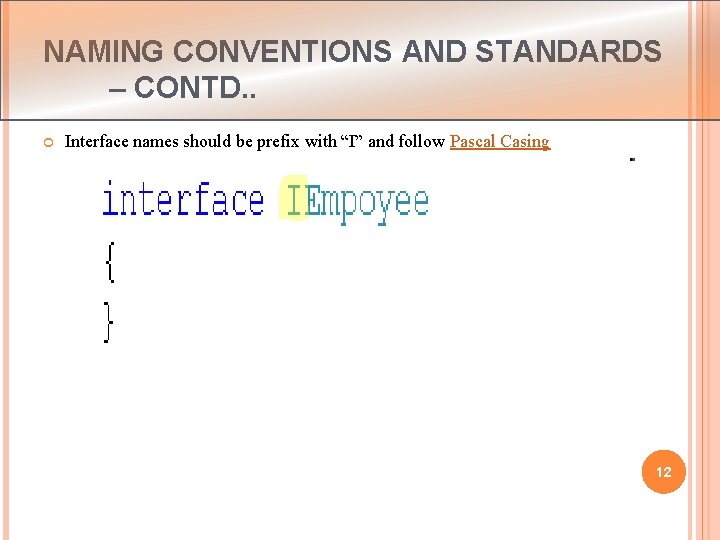
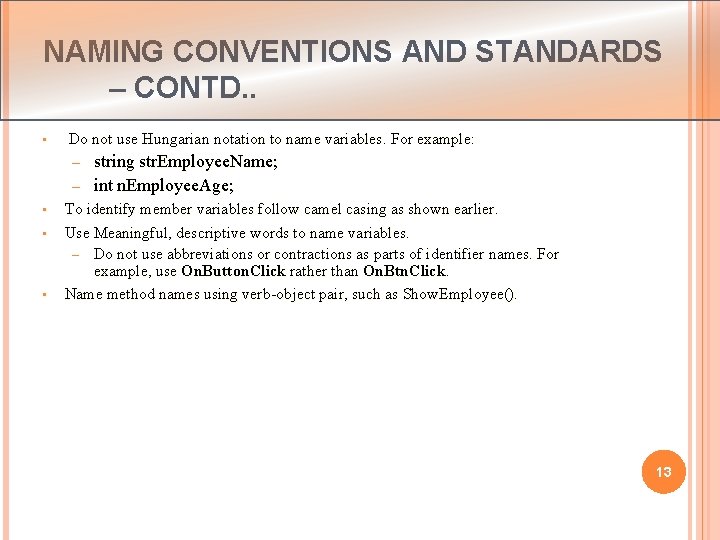
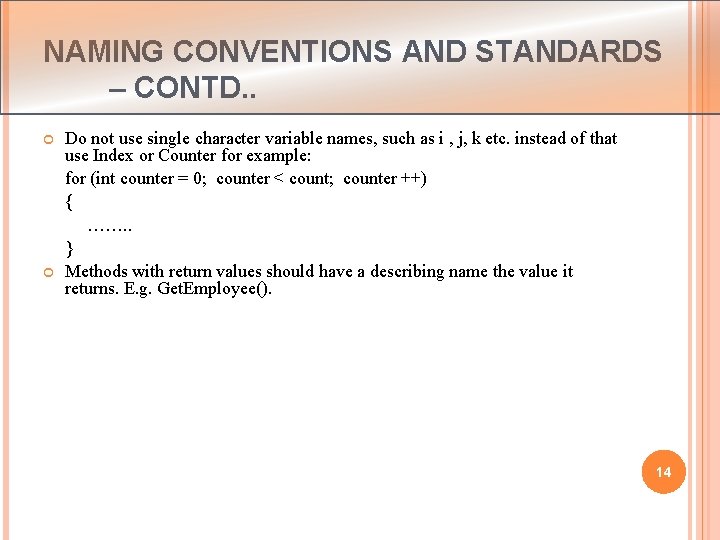
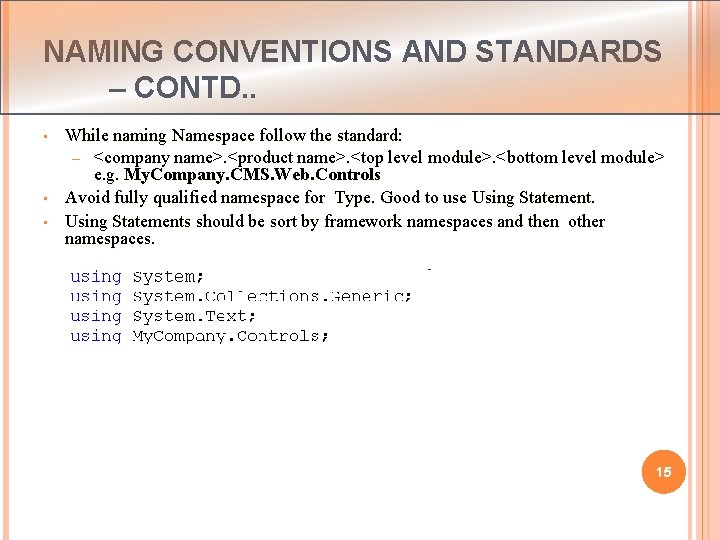
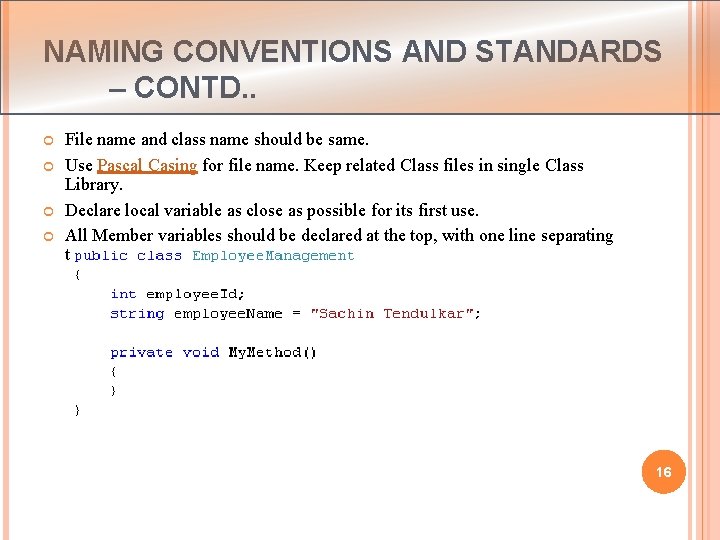
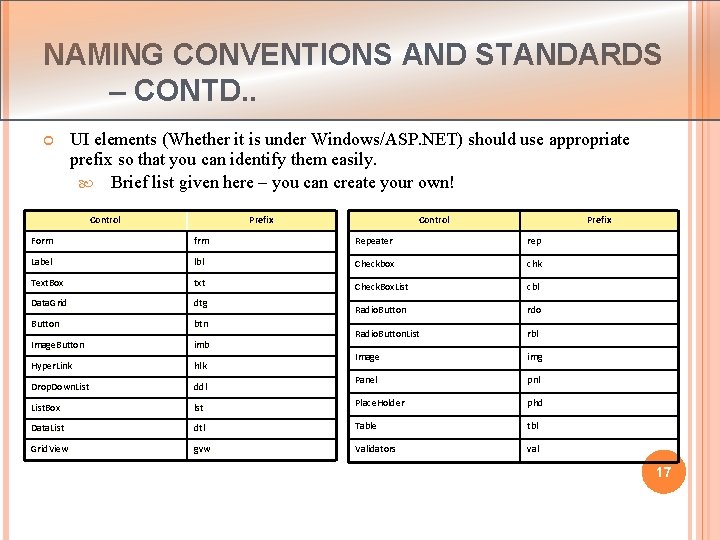
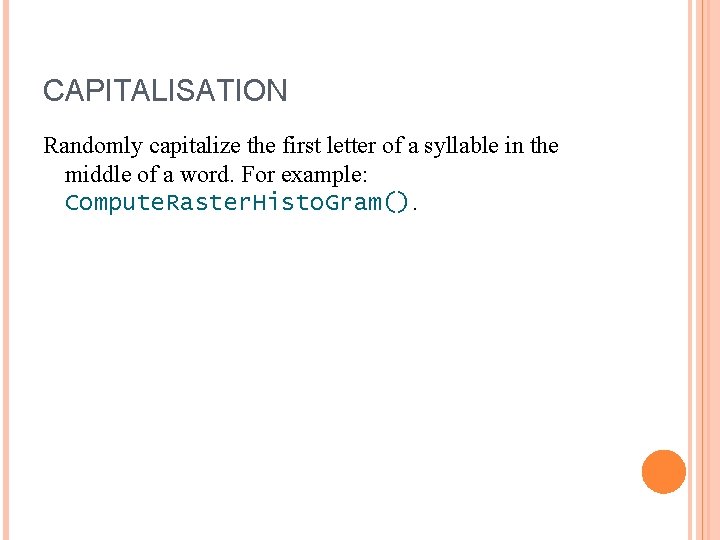
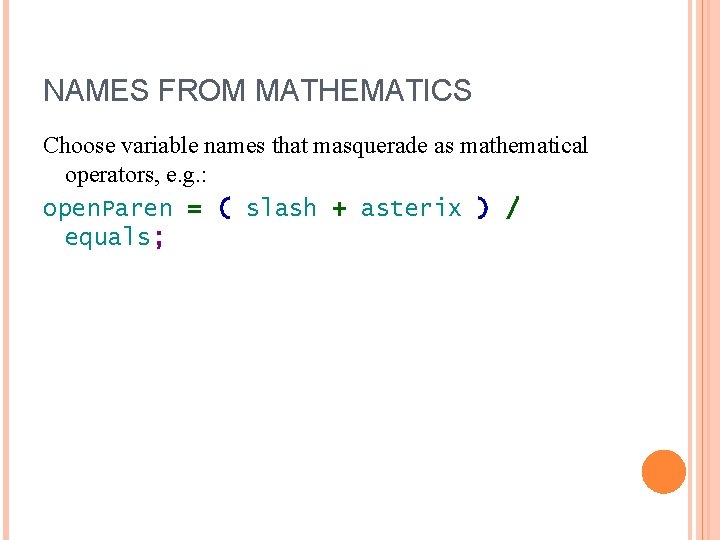
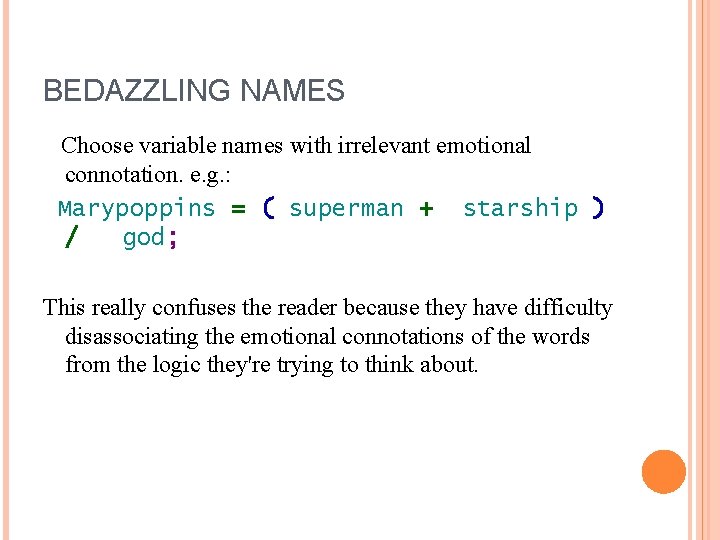
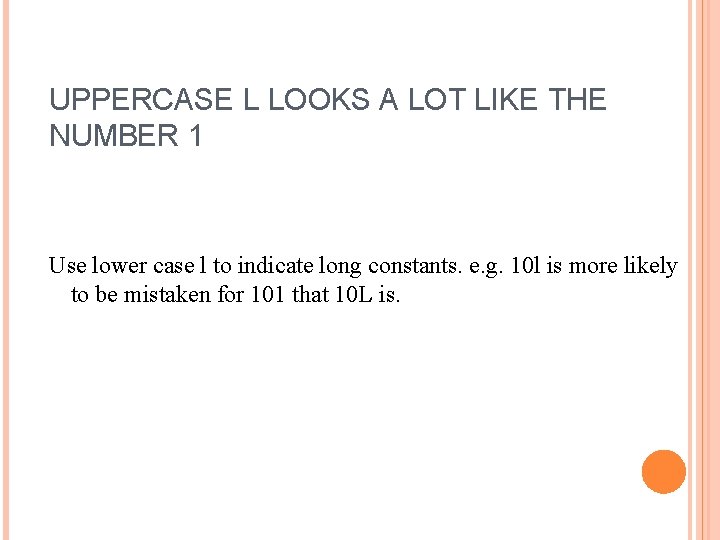
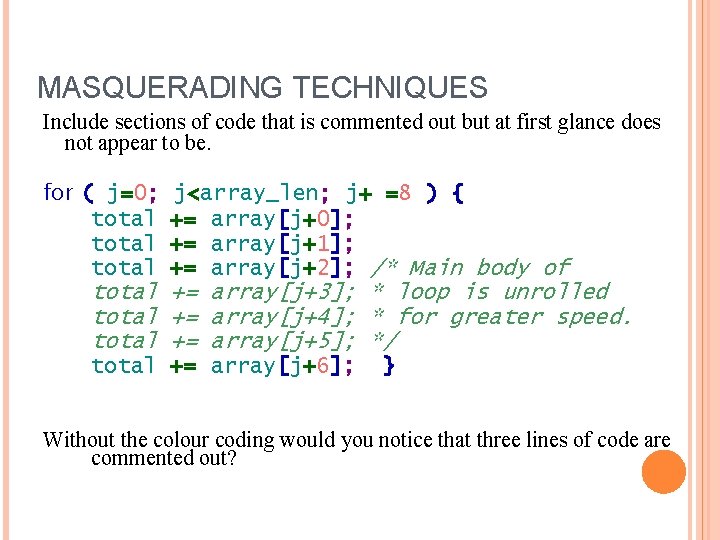
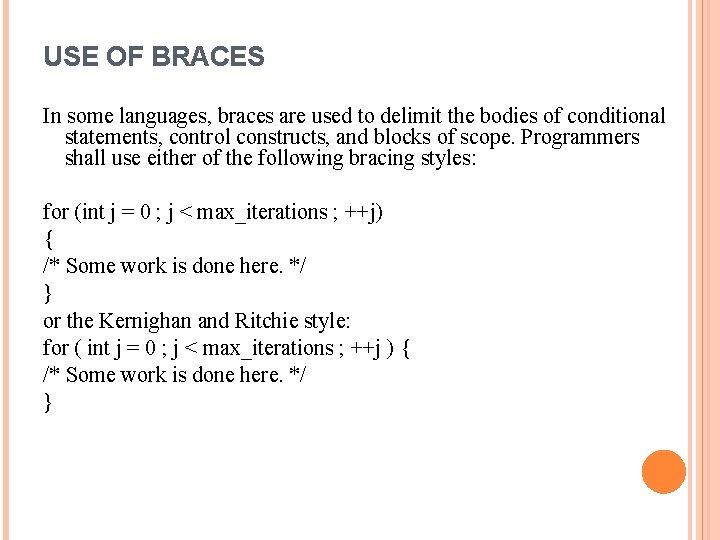
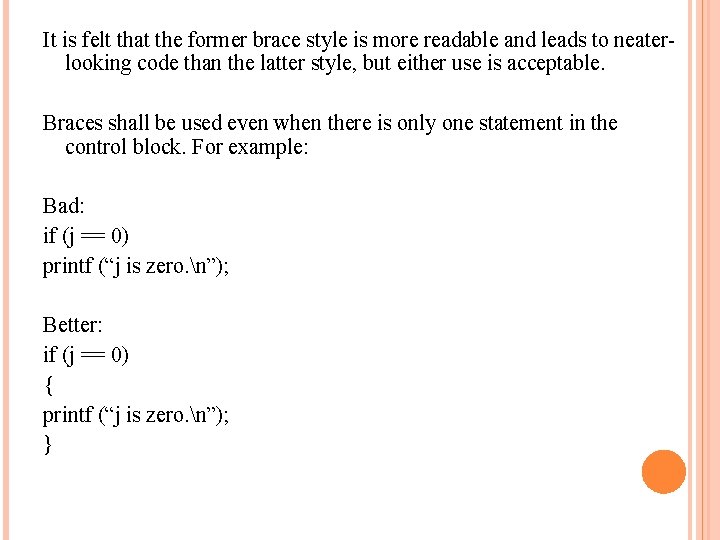
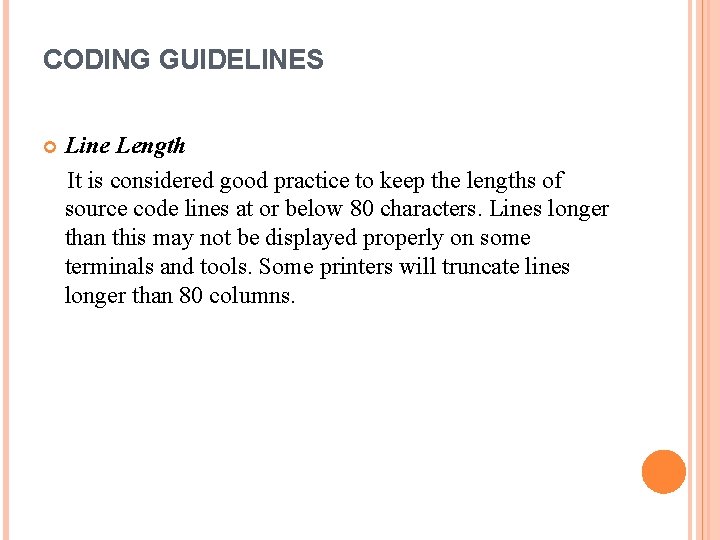
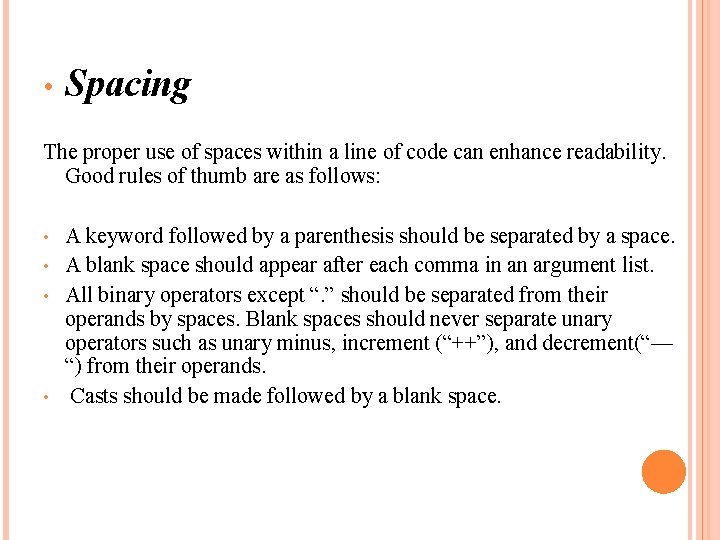
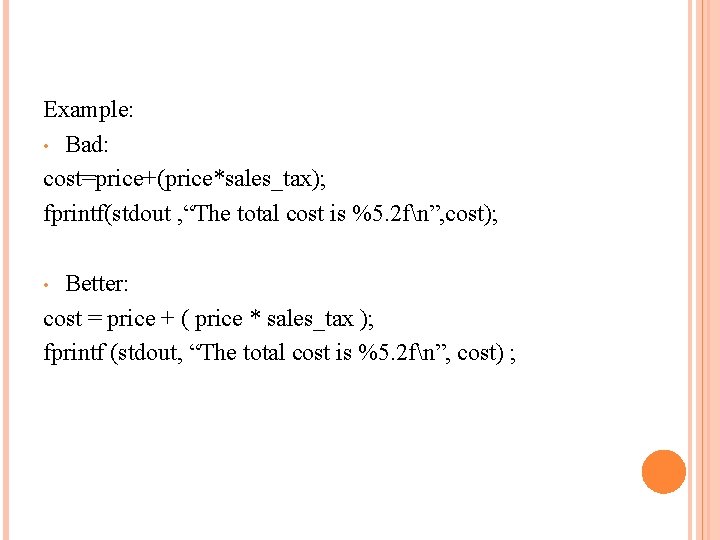
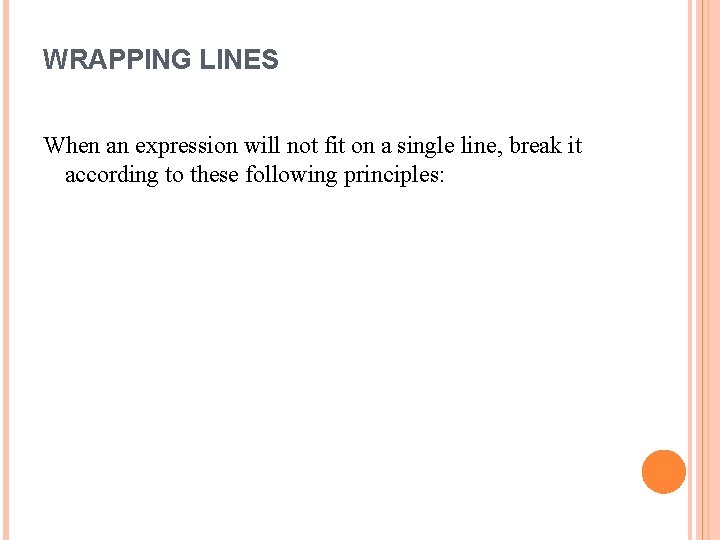
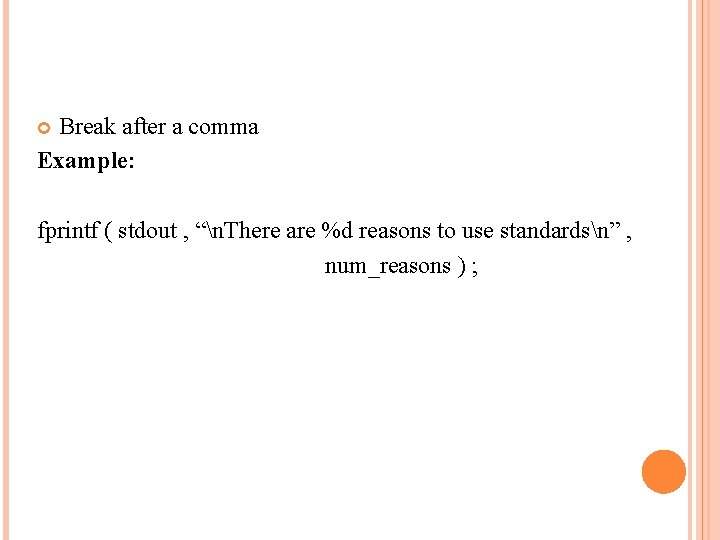
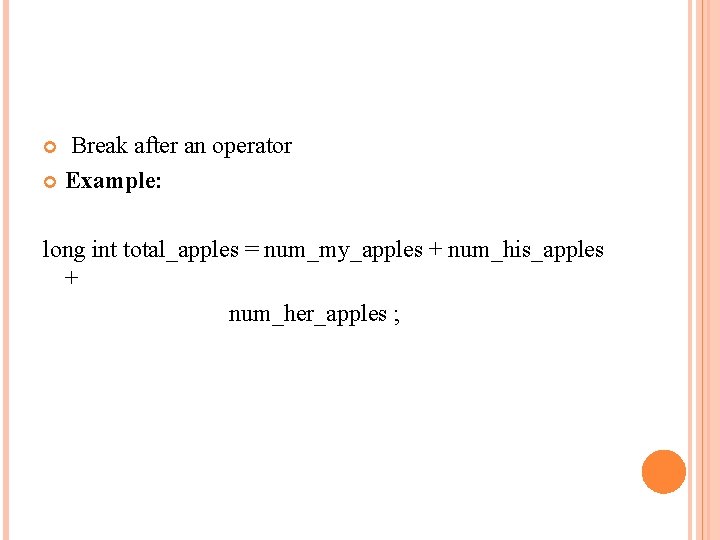
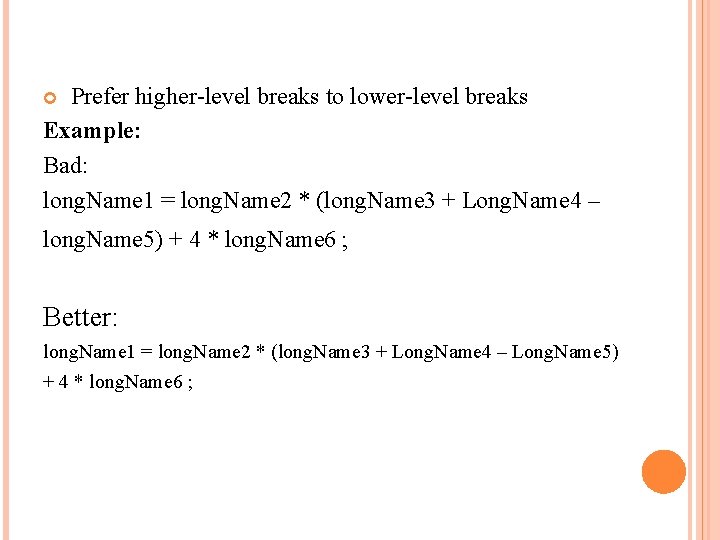
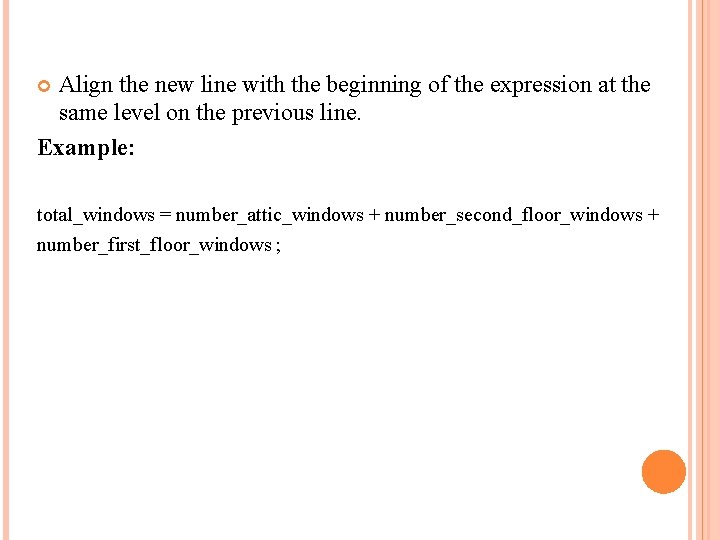
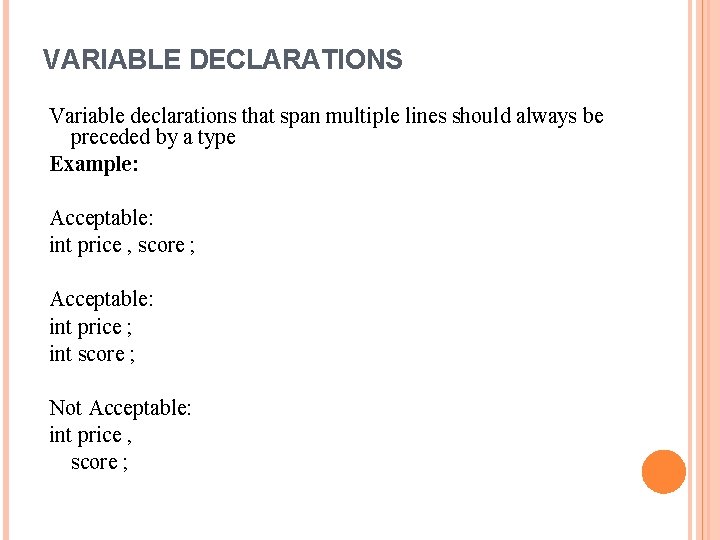
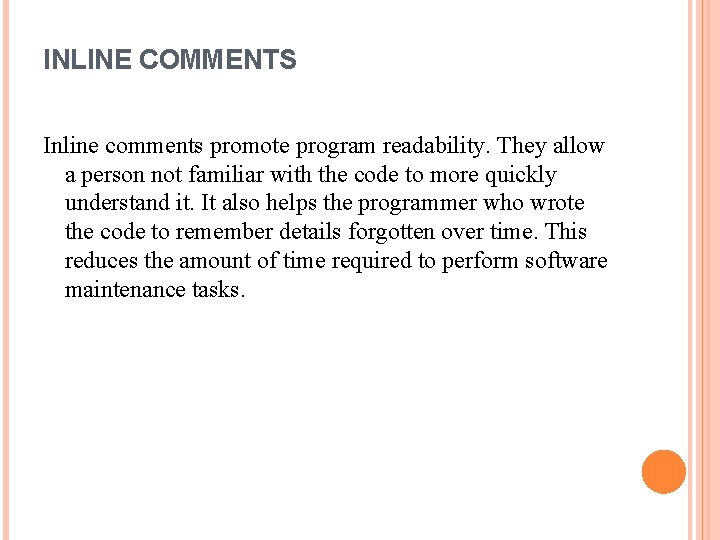
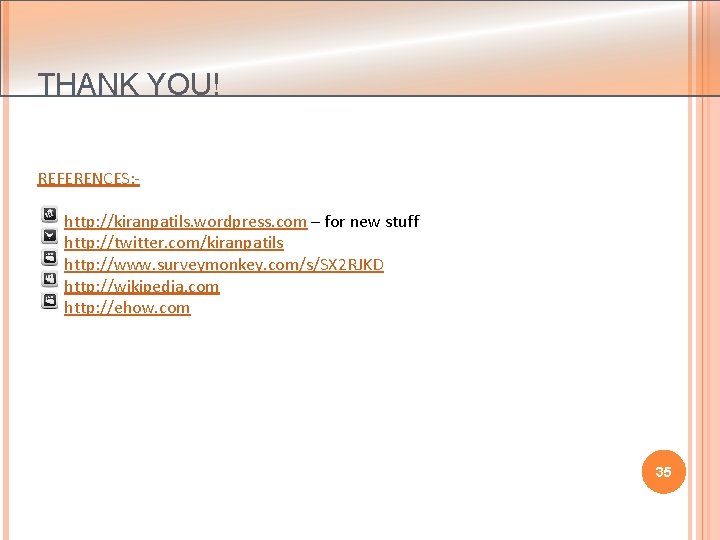
- Slides: 35
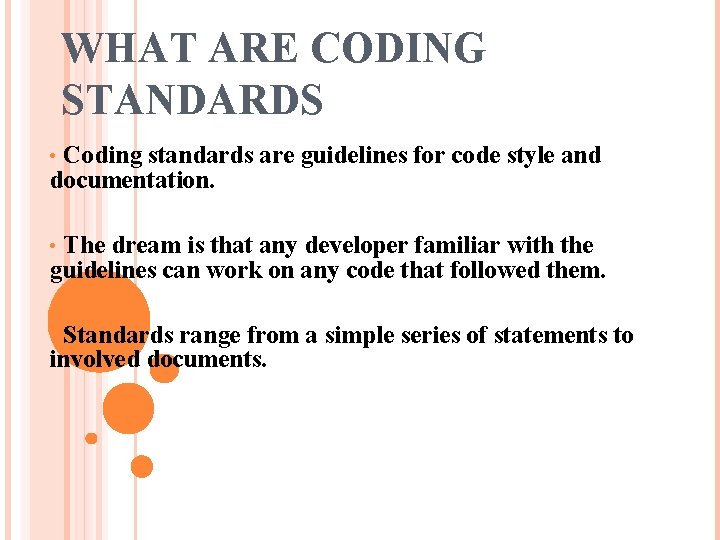
WHAT ARE CODING STANDARDS Coding standards are guidelines for code style and documentation. • The dream is that any developer familiar with the guidelines can work on any code that followed them. • Standards range from a simple series of statements to involved documents. •
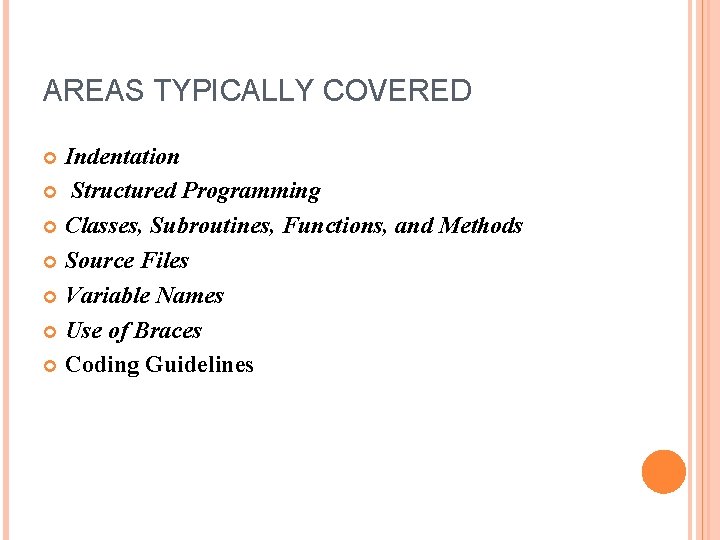
AREAS TYPICALLY COVERED Indentation Structured Programming Classes, Subroutines, Functions, and Methods Source Files Variable Names Use of Braces Coding Guidelines
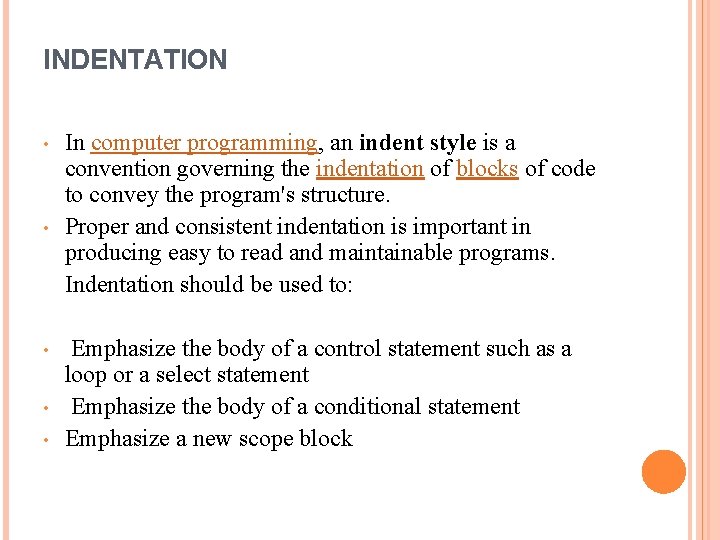
INDENTATION • • • In computer programming, an indent style is a convention governing the indentation of blocks of code to convey the program's structure. Proper and consistent indentation is important in producing easy to read and maintainable programs. Indentation should be used to: Emphasize the body of a control statement such as a loop or a select statement Emphasize the body of a conditional statement Emphasize a new scope block
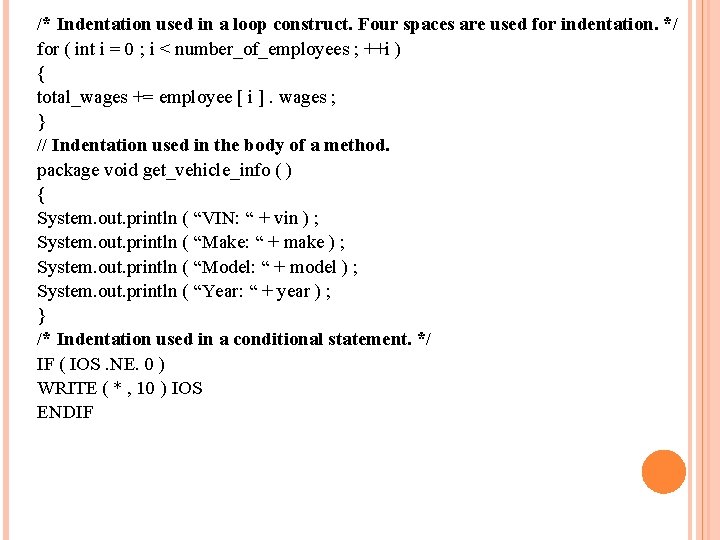
/* Indentation used in a loop construct. Four spaces are used for indentation. */ for ( int i = 0 ; i < number_of_employees ; ++i ) { total_wages += employee [ i ]. wages ; } // Indentation used in the body of a method. package void get_vehicle_info ( ) { System. out. println ( “VIN: “ + vin ) ; System. out. println ( “Make: “ + make ) ; System. out. println ( “Model: “ + model ) ; System. out. println ( “Year: “ + year ) ; } /* Indentation used in a conditional statement. */ IF ( IOS. NE. 0 ) WRITE ( * , 10 ) IOS ENDIF
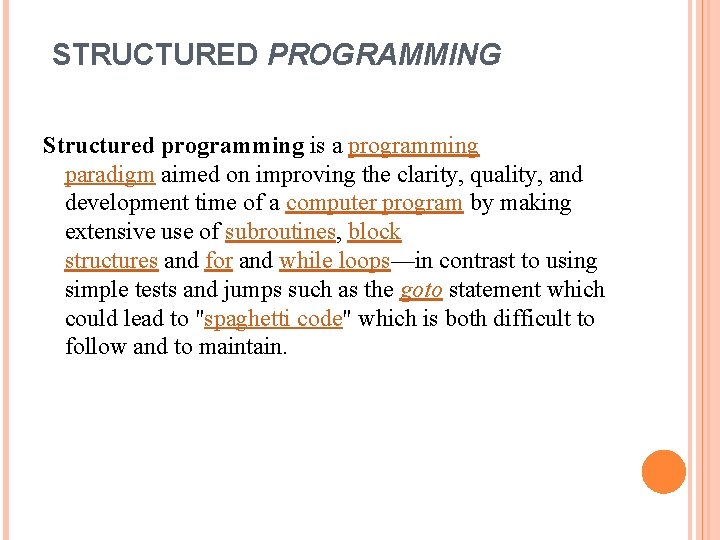
STRUCTURED PROGRAMMING Structured programming is a programming paradigm aimed on improving the clarity, quality, and development time of a computer program by making extensive use of subroutines, block structures and for and while loops—in contrast to using simple tests and jumps such as the goto statement which could lead to "spaghetti code" which is both difficult to follow and to maintain.
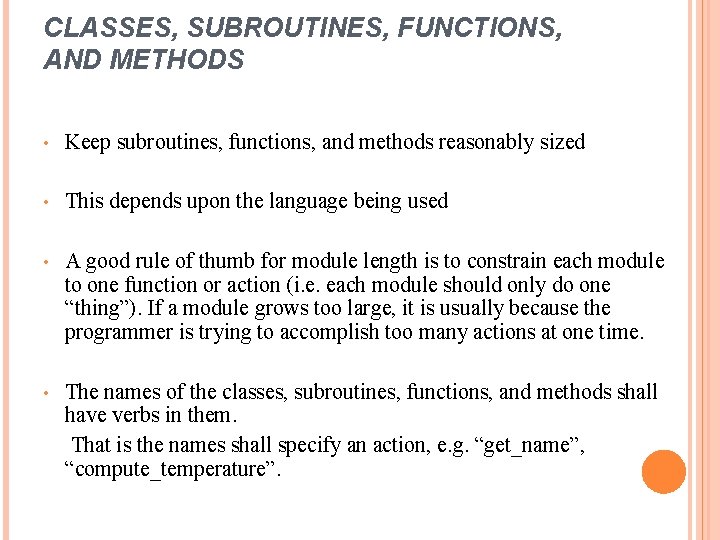
CLASSES, SUBROUTINES, FUNCTIONS, AND METHODS • Keep subroutines, functions, and methods reasonably sized • This depends upon the language being used • A good rule of thumb for module length is to constrain each module to one function or action (i. e. each module should only do one “thing”). If a module grows too large, it is usually because the programmer is trying to accomplish too many actions at one time. The names of the classes, subroutines, functions, and methods shall have verbs in them. That is the names shall specify an action, e. g. “get_name”, “compute_temperature”. •
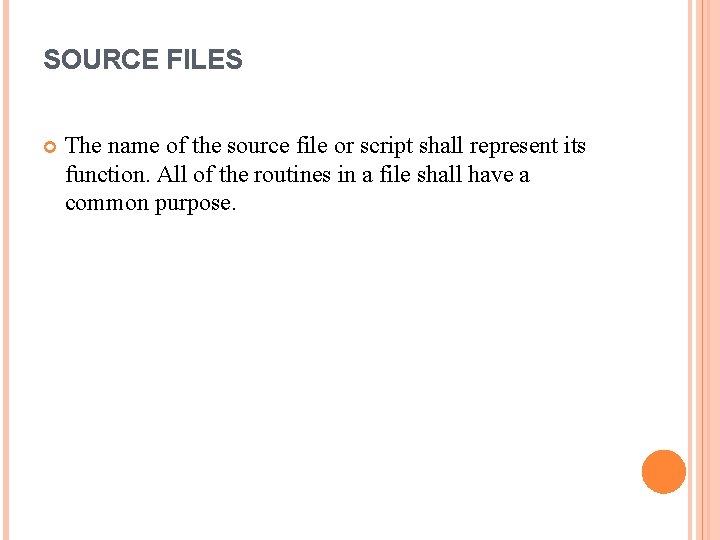
SOURCE FILES The name of the source file or script shall represent its function. All of the routines in a file shall have a common purpose.
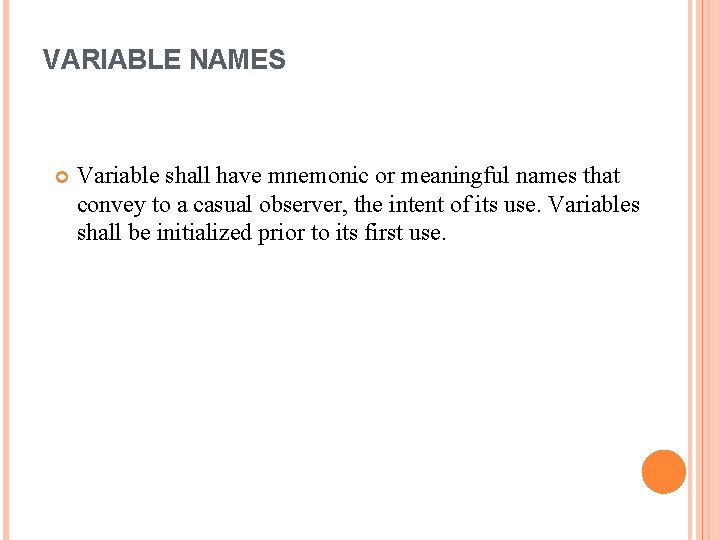
VARIABLE NAMES Variable shall have mnemonic or meaningful names that convey to a casual observer, the intent of its use. Variables shall be initialized prior to its first use.
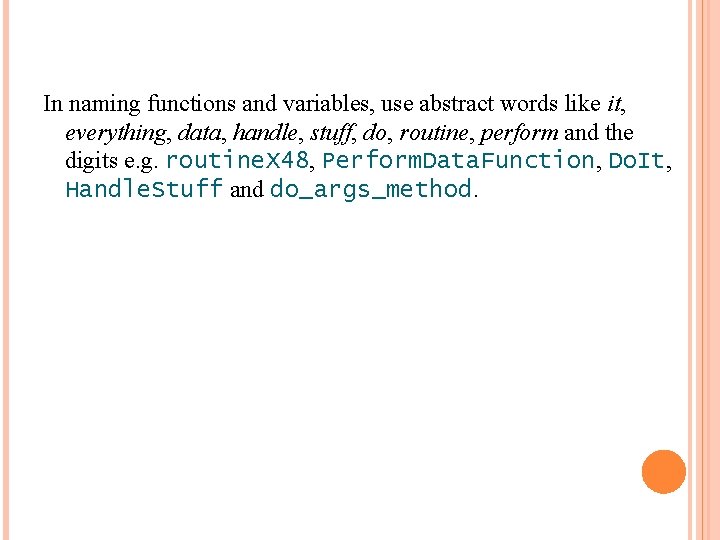
In naming functions and variables, use abstract words like it, everything, data, handle, stuff, do, routine, perform and the digits e. g. routine. X 48, Perform. Data. Function, Do. It, Handle. Stuff and do_args_method.
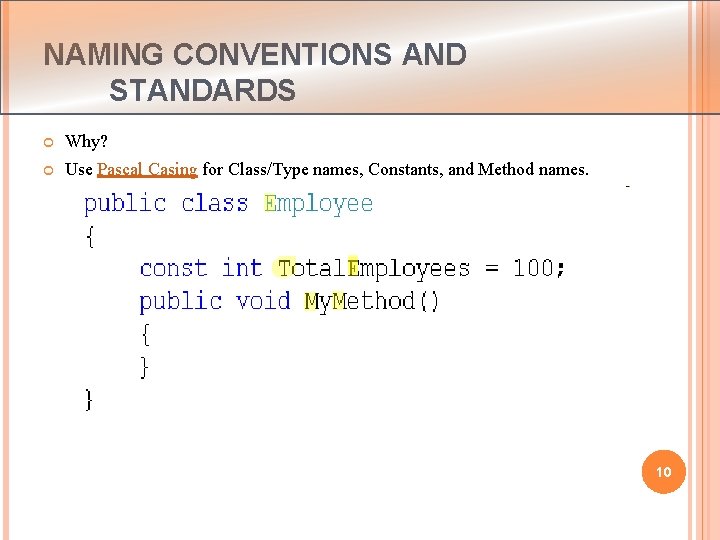
NAMING CONVENTIONS AND STANDARDS Why? Use Pascal Casing for Class/Type names, Constants, and Method names. 10
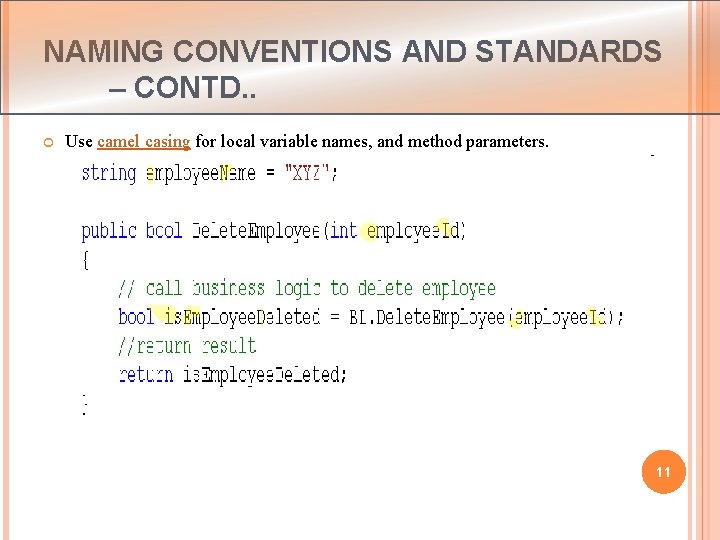
NAMING CONVENTIONS AND STANDARDS – CONTD. . Use camel casing for local variable names, and method parameters. 11
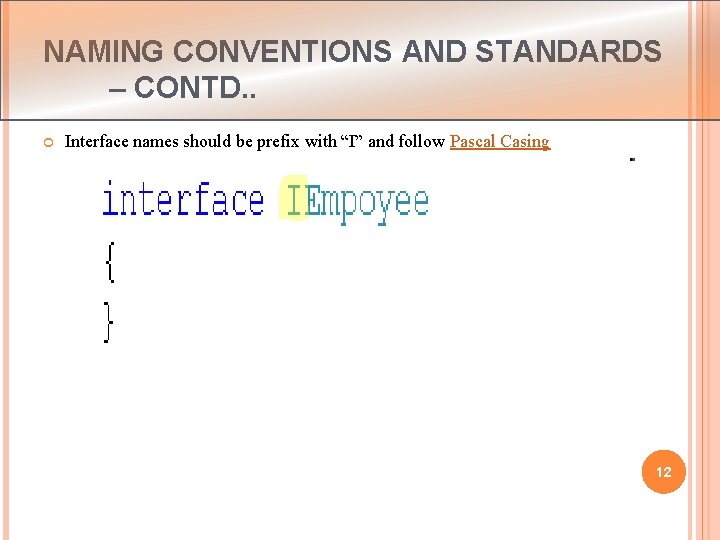
NAMING CONVENTIONS AND STANDARDS – CONTD. . Interface names should be prefix with “I” and follow Pascal Casing 12
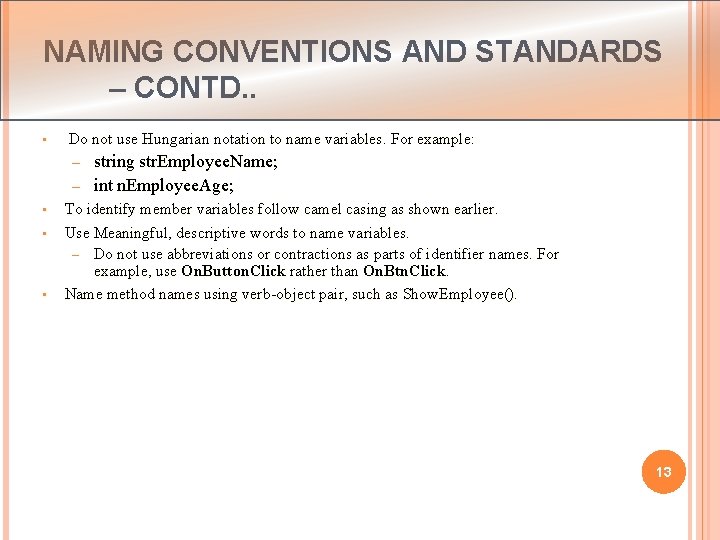
NAMING CONVENTIONS AND STANDARDS – CONTD. . • Do not use Hungarian notation to name variables. For example: string str. Employee. Name; – int n. Employee. Age; – • • • To identify member variables follow camel casing as shown earlier. Use Meaningful, descriptive words to name variables. – Do not use abbreviations or contractions as parts of identifier names. For example, use On. Button. Click rather than On. Btn. Click. Name method names using verb-object pair, such as Show. Employee(). 13
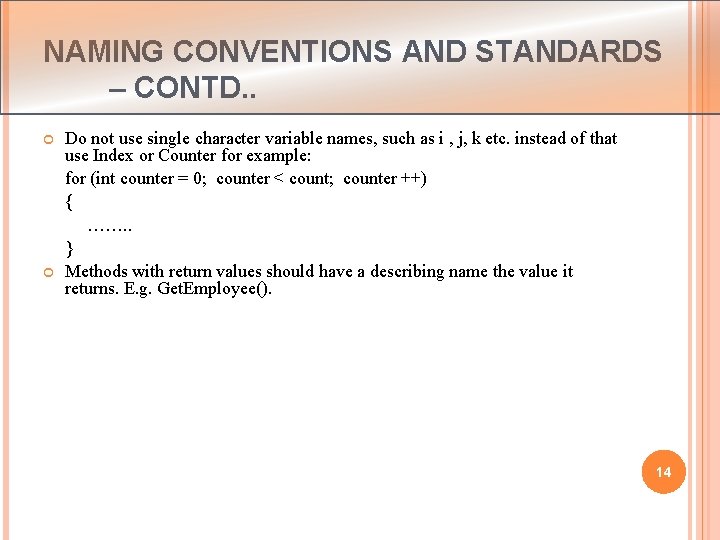
NAMING CONVENTIONS AND STANDARDS – CONTD. . Do not use single character variable names, such as i , j, k etc. instead of that use Index or Counter for example: for (int counter = 0; counter < count; counter ++) { ……. . } Methods with return values should have a describing name the value it returns. E. g. Get. Employee(). 14
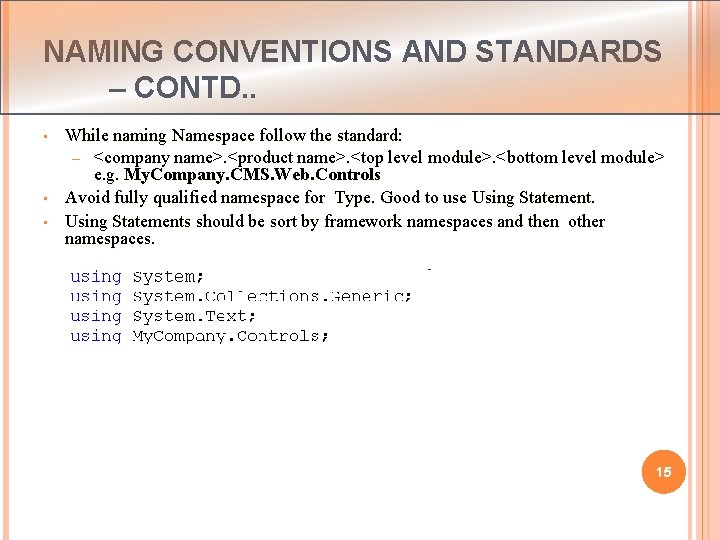
NAMING CONVENTIONS AND STANDARDS – CONTD. . • • • While naming Namespace follow the standard: – <company name>. <product name>. <top level module>. <bottom level module> e. g. My. Company. CMS. Web. Controls Avoid fully qualified namespace for Type. Good to use Using Statements should be sort by framework namespaces and then other namespaces. 15
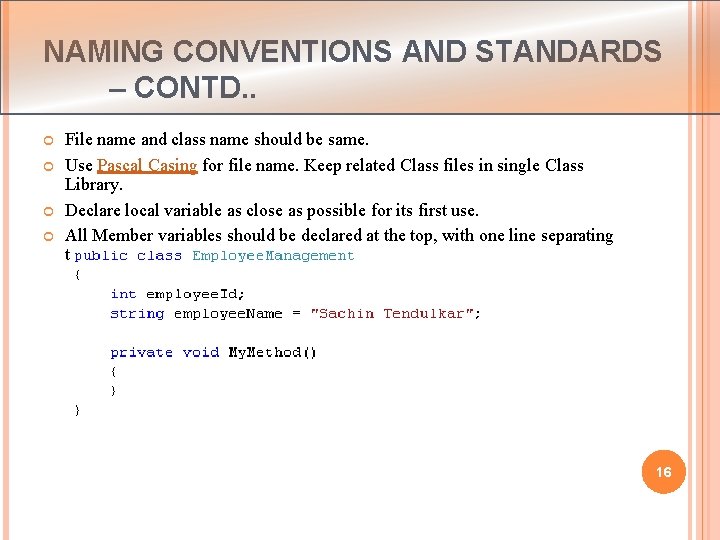
NAMING CONVENTIONS AND STANDARDS – CONTD. . File name and class name should be same. Use Pascal Casing for file name. Keep related Class files in single Class Library. Declare local variable as close as possible for its first use. All Member variables should be declared at the top, with one line separating them from Properties and Methods. 16
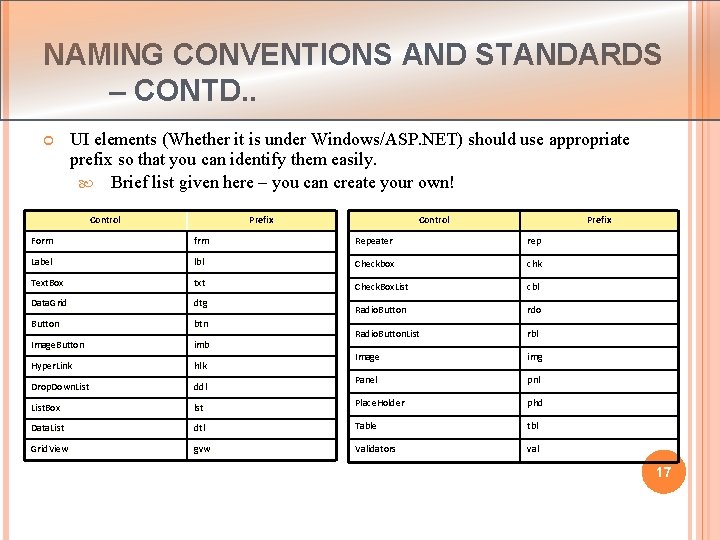
NAMING CONVENTIONS AND STANDARDS – CONTD. . UI elements (Whether it is under Windows/ASP. NET) should use appropriate prefix so that you can identify them easily. Brief list given here – you can create your own! Control Prefix Form frm Repeater rep Label lbl Checkbox chk Text. Box txt Check. Box. List cbl Data. Grid dtg Radio. Button rdo Button btn Image. Button imb Radio. Button. List rbl Hyper. Link hlk Image img Drop. Down. List ddl Panel pnl List. Box lst Place. Holder phd Data. List dtl Table tbl Grid. View gvw Validators val 17
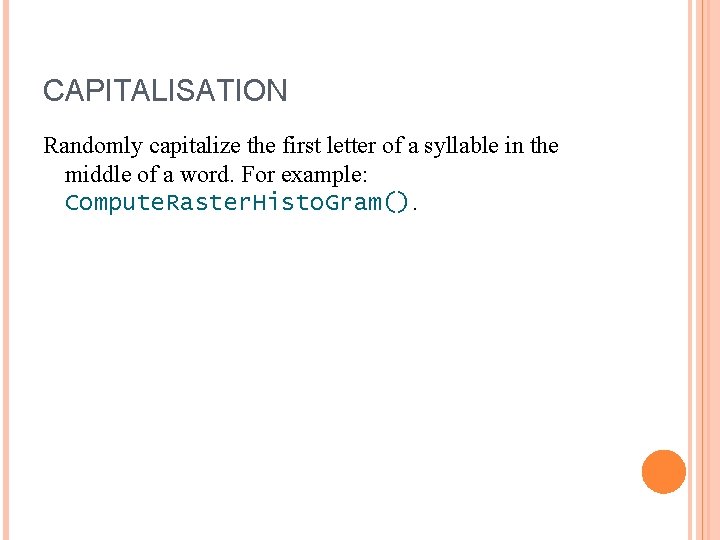
CAPITALISATION Randomly capitalize the first letter of a syllable in the middle of a word. For example: Compute. Raster. Histo. Gram().
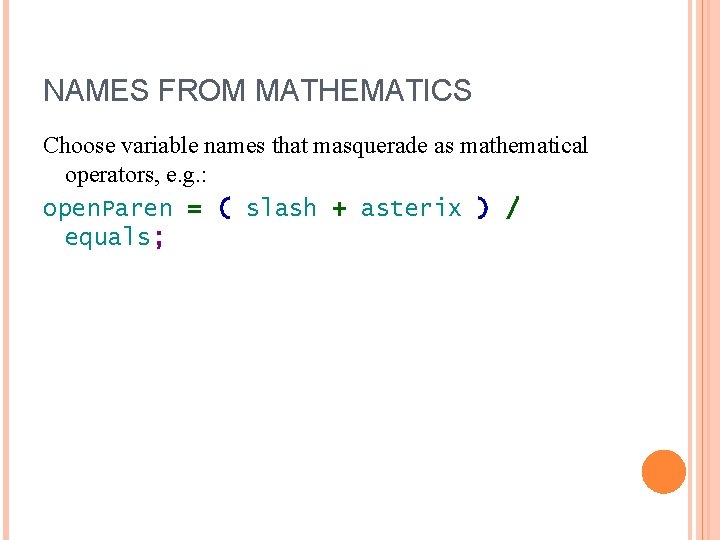
NAMES FROM MATHEMATICS Choose variable names that masquerade as mathematical operators, e. g. : open. Paren = ( slash + asterix ) / equals;
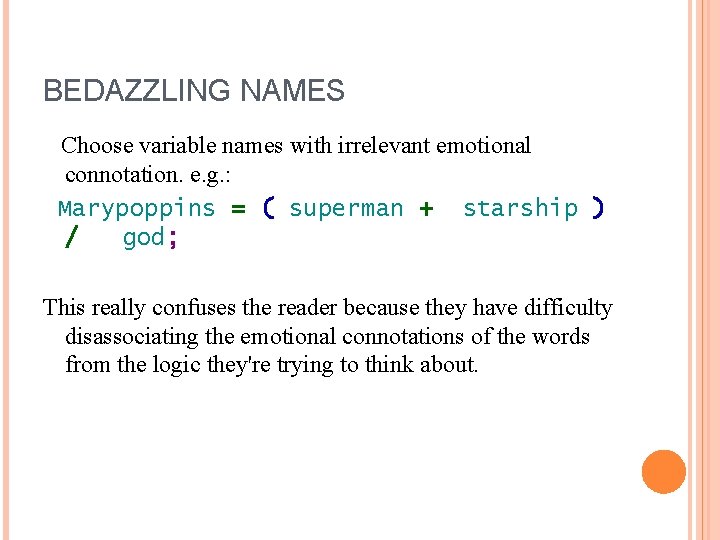
BEDAZZLING NAMES Choose variable names with irrelevant emotional connotation. e. g. : Marypoppins = ( superman + starship ) / god; This really confuses the reader because they have difficulty disassociating the emotional connotations of the words from the logic they're trying to think about.
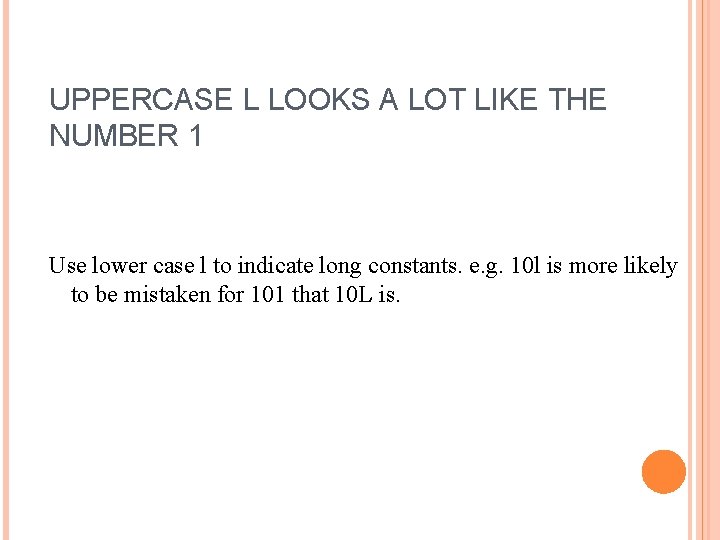
UPPERCASE L LOOKS A LOT LIKE THE NUMBER 1 Use lower case l to indicate long constants. e. g. 10 l is more likely to be mistaken for 101 that 10 L is.
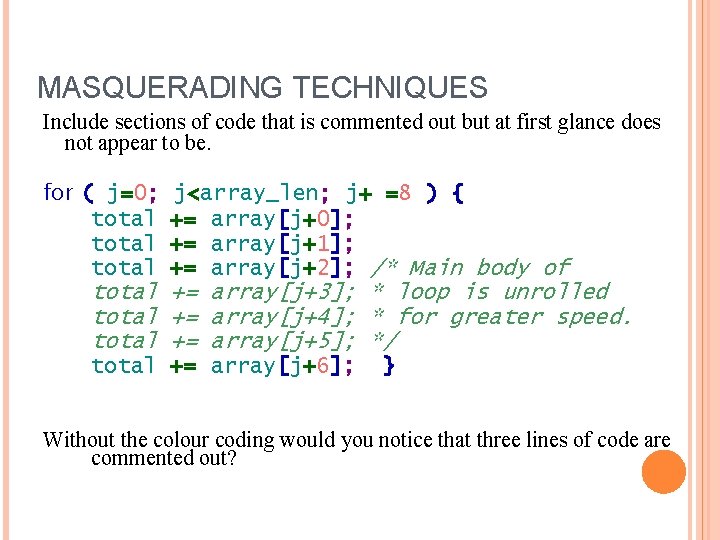
MASQUERADING TECHNIQUES Include sections of code that is commented out but at first glance does not appear to be. for ( j=0; total j<array_len; j+ =8 ) { += array[j+0]; += array[j+1]; += array[j+2]; /* Main body of total += array[j+3]; * loop is unrolled total += array[j+4]; * for greater speed. total += array[j+5]; */ total += array[j+6]; } Without the colour coding would you notice that three lines of code are commented out?
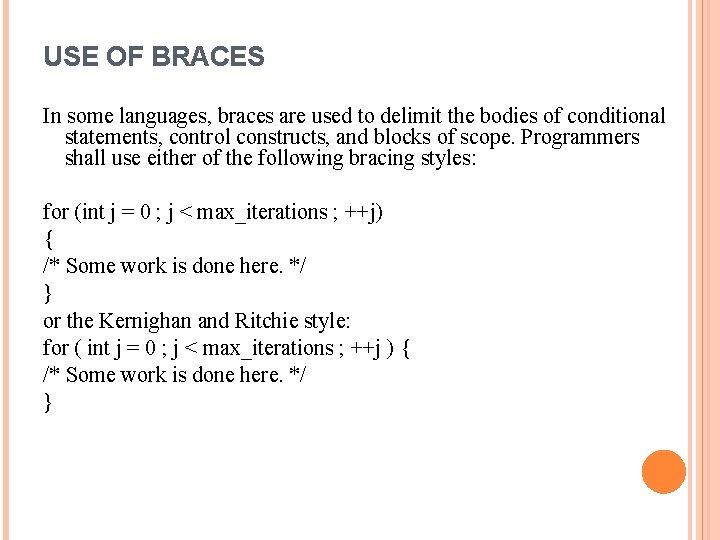
USE OF BRACES In some languages, braces are used to delimit the bodies of conditional statements, control constructs, and blocks of scope. Programmers shall use either of the following bracing styles: for (int j = 0 ; j < max_iterations ; ++j) { /* Some work is done here. */ } or the Kernighan and Ritchie style: for ( int j = 0 ; j < max_iterations ; ++j ) { /* Some work is done here. */ }
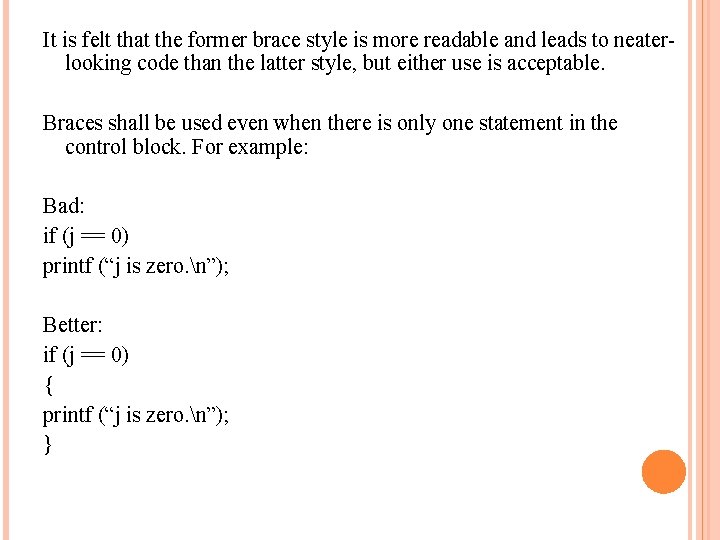
It is felt that the former brace style is more readable and leads to neaterlooking code than the latter style, but either use is acceptable. Braces shall be used even when there is only one statement in the control block. For example: Bad: if (j == 0) printf (“j is zero. n”); Better: if (j == 0) { printf (“j is zero. n”); }
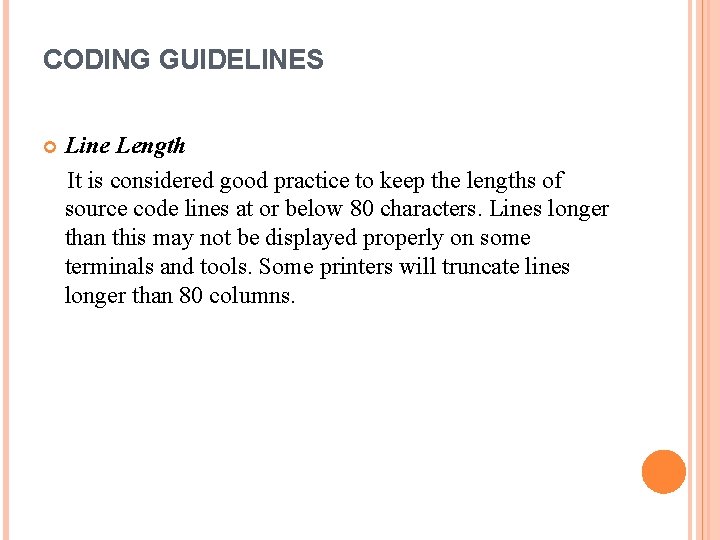
CODING GUIDELINES Line Length It is considered good practice to keep the lengths of source code lines at or below 80 characters. Lines longer than this may not be displayed properly on some terminals and tools. Some printers will truncate lines longer than 80 columns.
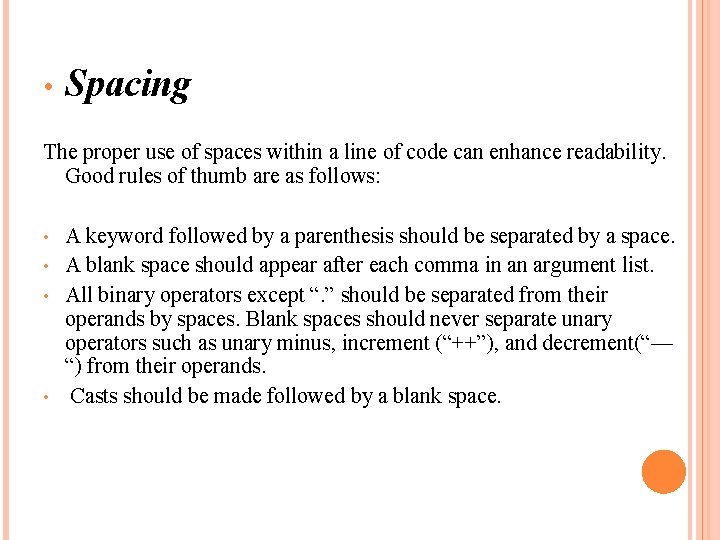
• Spacing The proper use of spaces within a line of code can enhance readability. Good rules of thumb are as follows: • • A keyword followed by a parenthesis should be separated by a space. A blank space should appear after each comma in an argument list. All binary operators except “. ” should be separated from their operands by spaces. Blank spaces should never separate unary operators such as unary minus, increment (“++”), and decrement(“— “) from their operands. Casts should be made followed by a blank space.
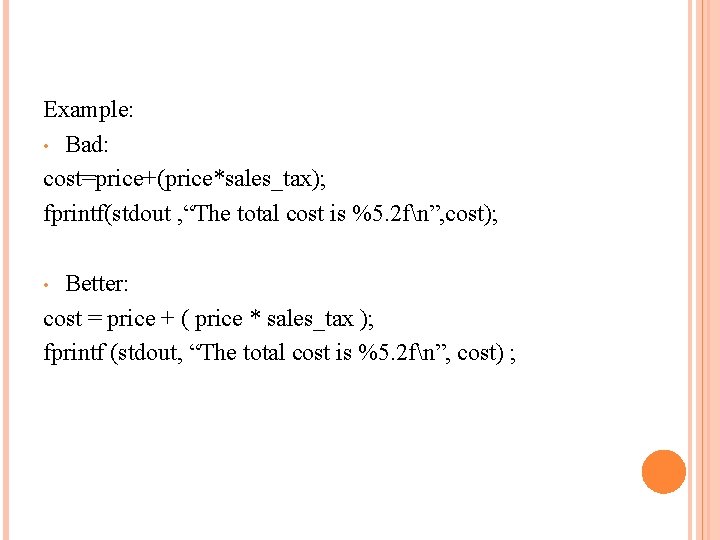
Example: • Bad: cost=price+(price*sales_tax); fprintf(stdout , “The total cost is %5. 2 fn”, cost); Better: cost = price + ( price * sales_tax ); fprintf (stdout, “The total cost is %5. 2 fn”, cost) ; •
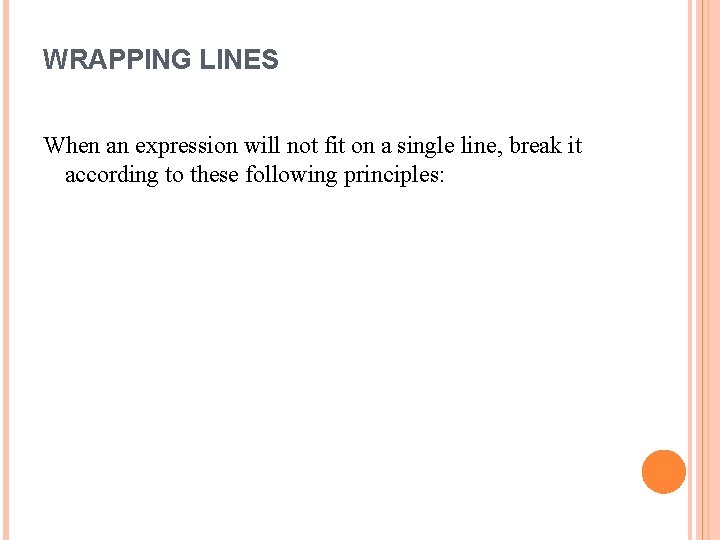
WRAPPING LINES When an expression will not fit on a single line, break it according to these following principles:
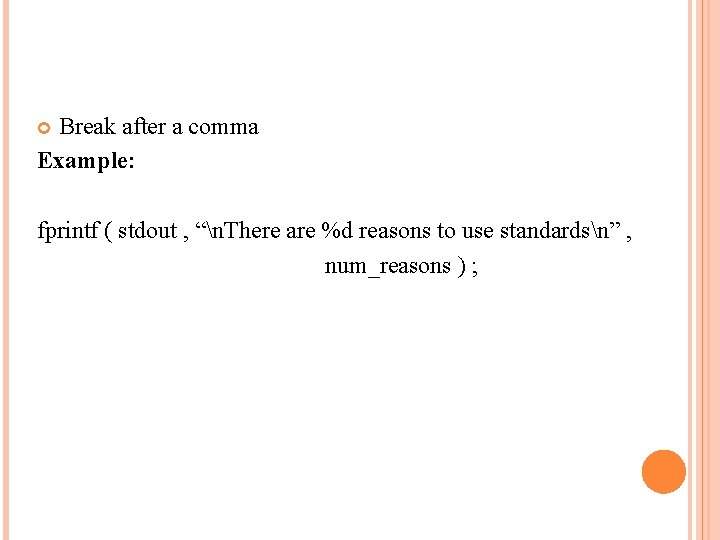
Break after a comma Example: fprintf ( stdout , “n. There are %d reasons to use standardsn” , num_reasons ) ;
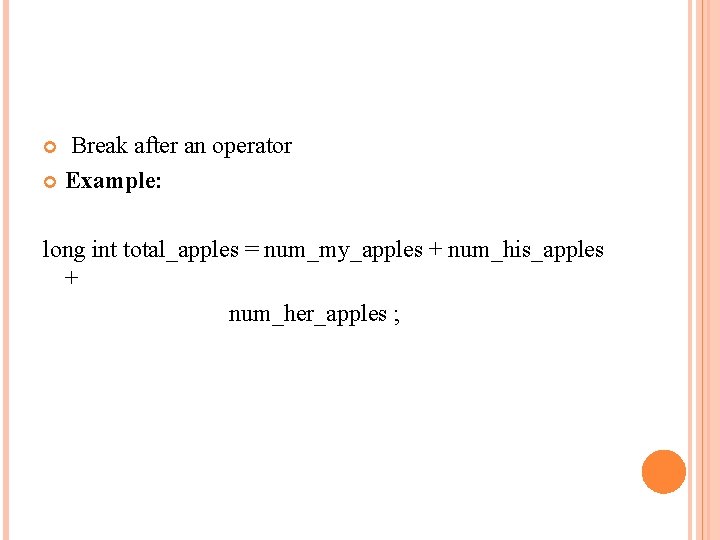
Break after an operator Example: long int total_apples = num_my_apples + num_his_apples + num_her_apples ;
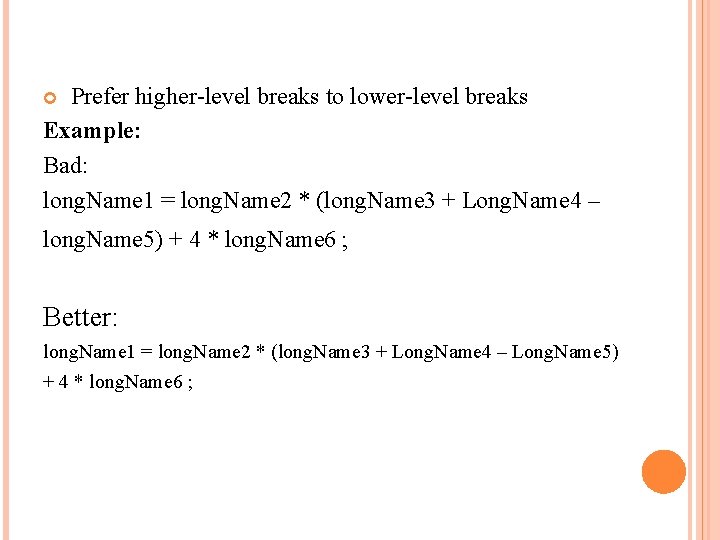
Prefer higher-level breaks to lower-level breaks Example: Bad: long. Name 1 = long. Name 2 * (long. Name 3 + Long. Name 4 – long. Name 5) + 4 * long. Name 6 ; Better: long. Name 1 = long. Name 2 * (long. Name 3 + Long. Name 4 – Long. Name 5) + 4 * long. Name 6 ;
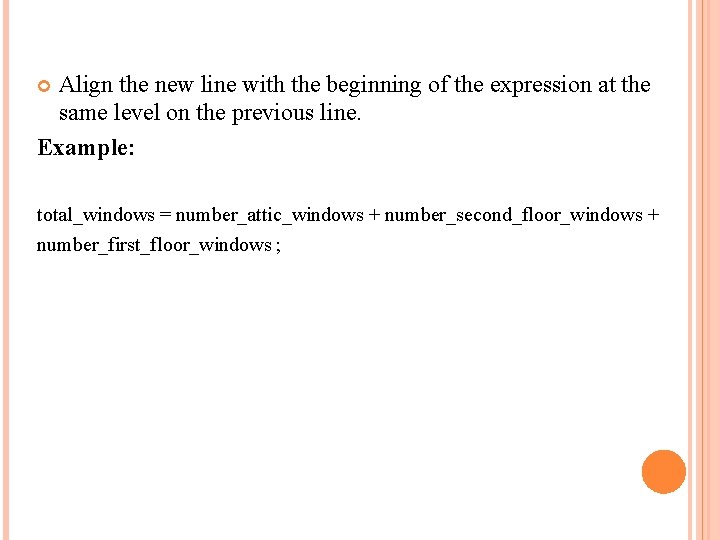
Align the new line with the beginning of the expression at the same level on the previous line. Example: total_windows = number_attic_windows + number_second_floor_windows + number_first_floor_windows ;
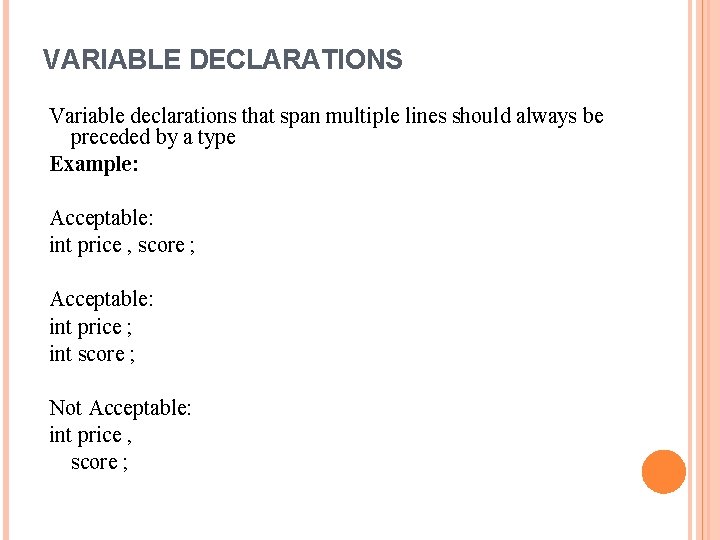
VARIABLE DECLARATIONS Variable declarations that span multiple lines should always be preceded by a type Example: Acceptable: int price , score ; Acceptable: int price ; int score ; Not Acceptable: int price , score ;
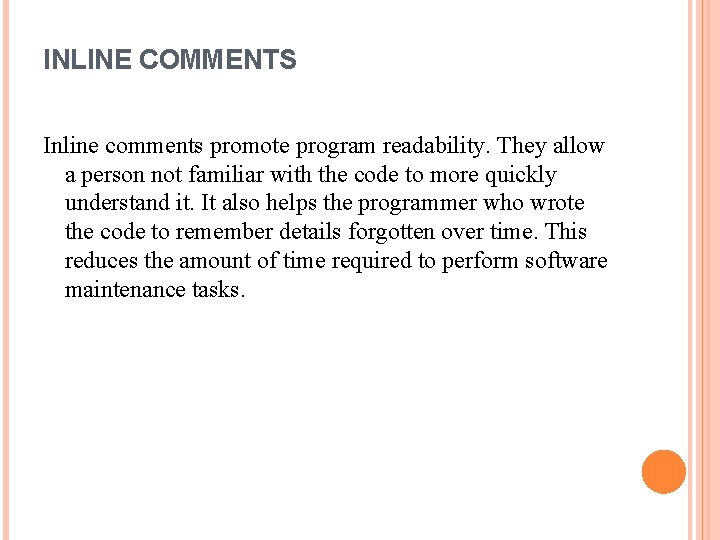
INLINE COMMENTS Inline comments promote program readability. They allow a person not familiar with the code to more quickly understand it. It also helps the programmer who wrote the code to remember details forgotten over time. This reduces the amount of time required to perform software maintenance tasks.
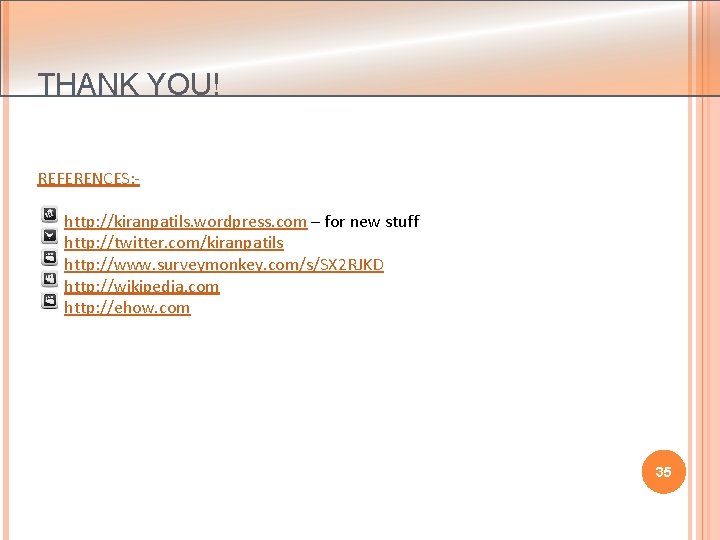
THANK YOU! REFERENCES: http: //kiranpatils. wordpress. com – for new stuff http: //twitter. com/kiranpatils http: //www. surveymonkey. com/s/SX 2 RJKD http: //wikipedia. com http: //ehow. com 35