Vectors 2004 Goodrich Tamassia Vectors 1 The Vector
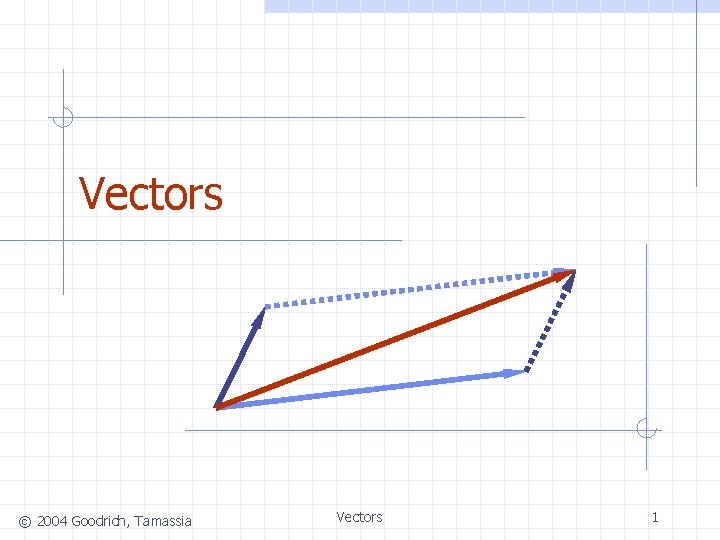
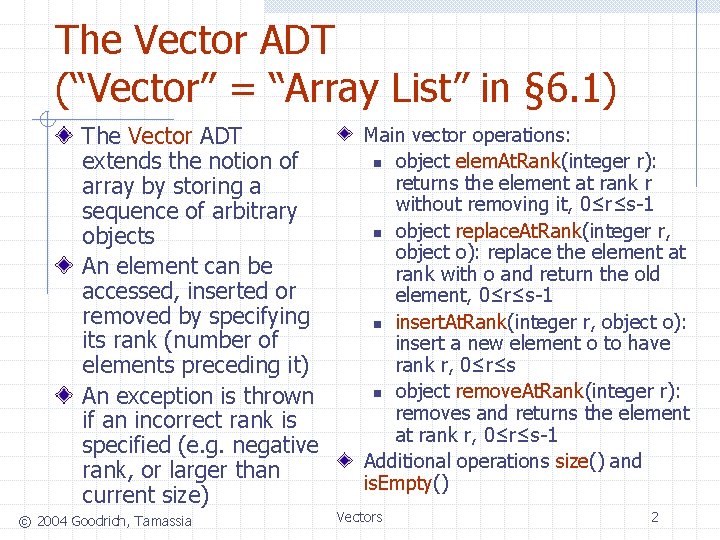
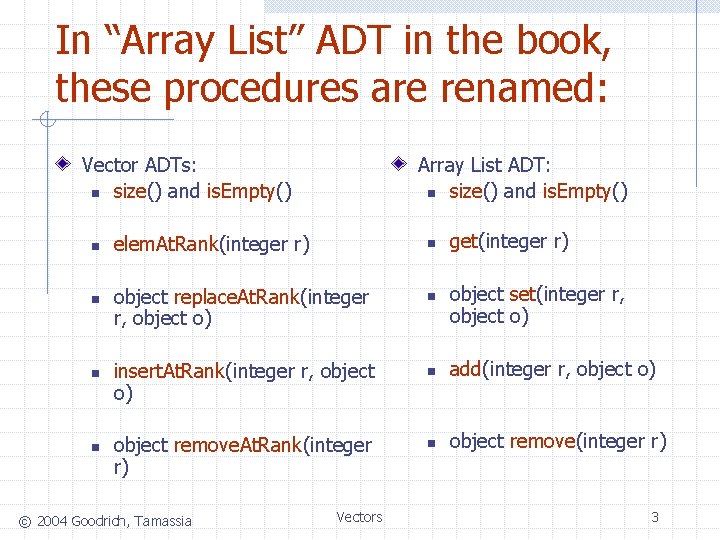
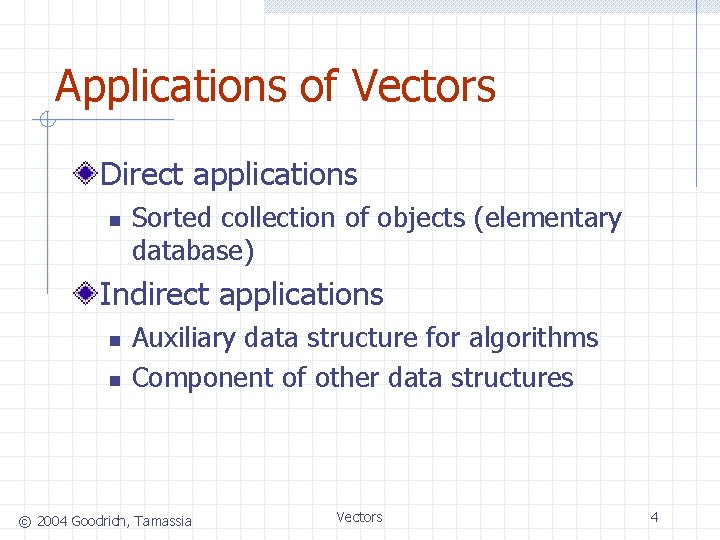
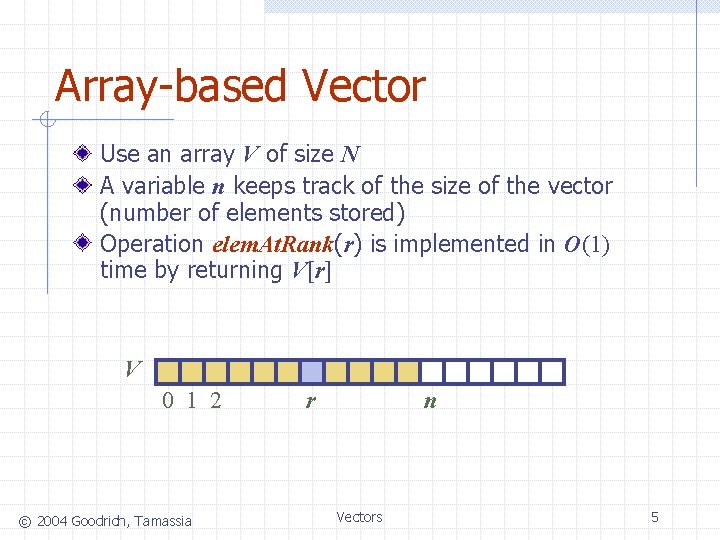
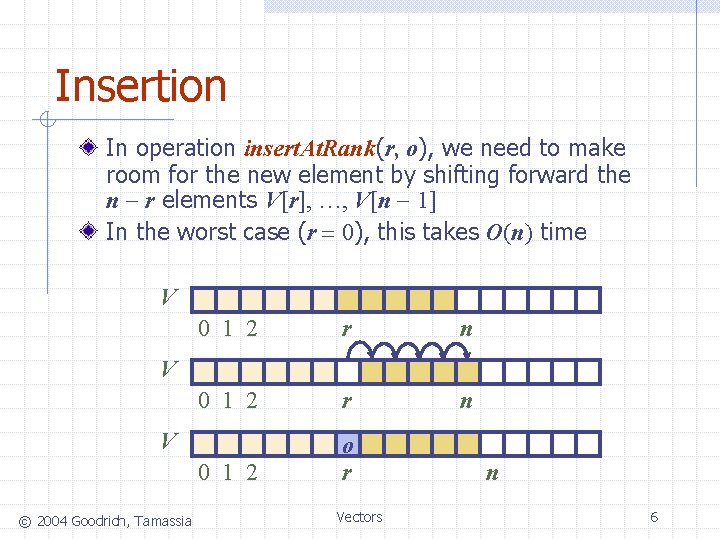
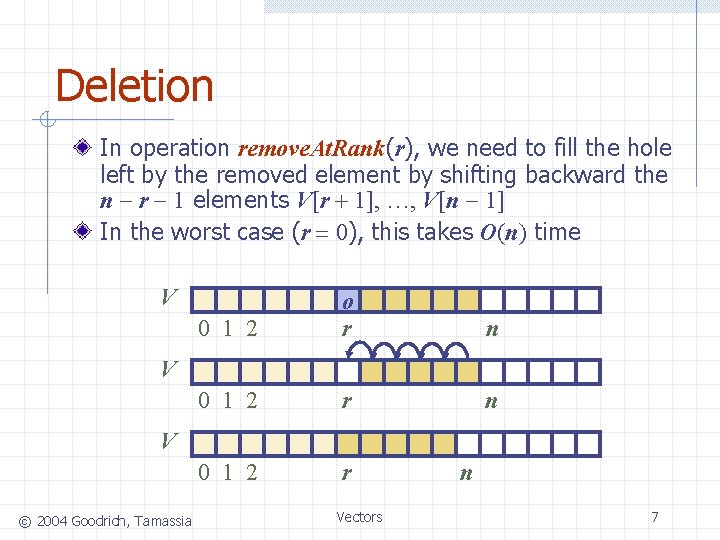
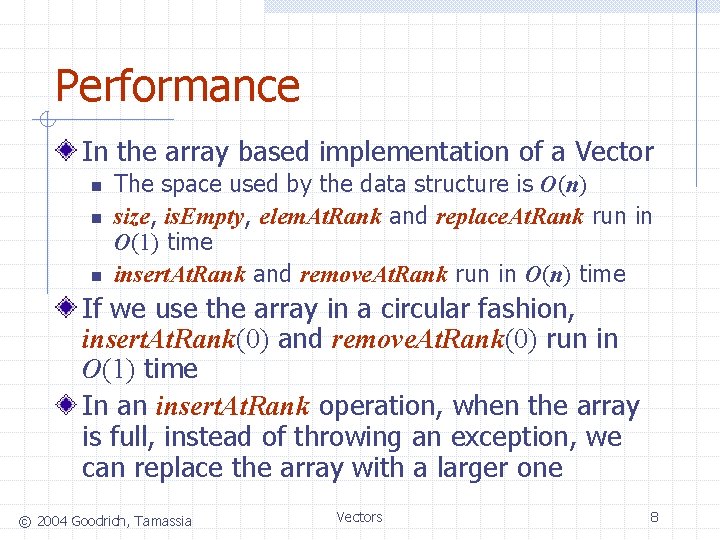
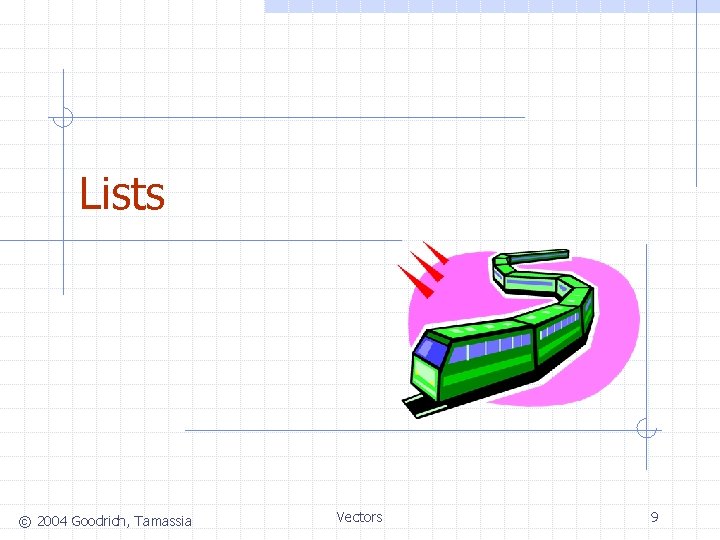
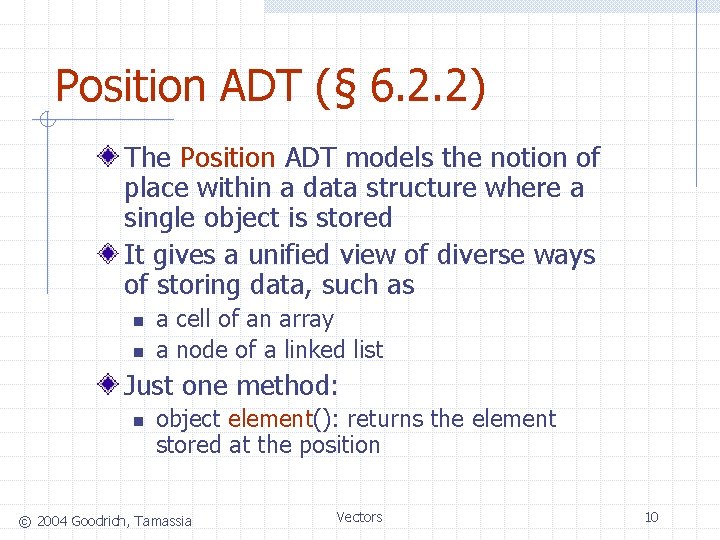
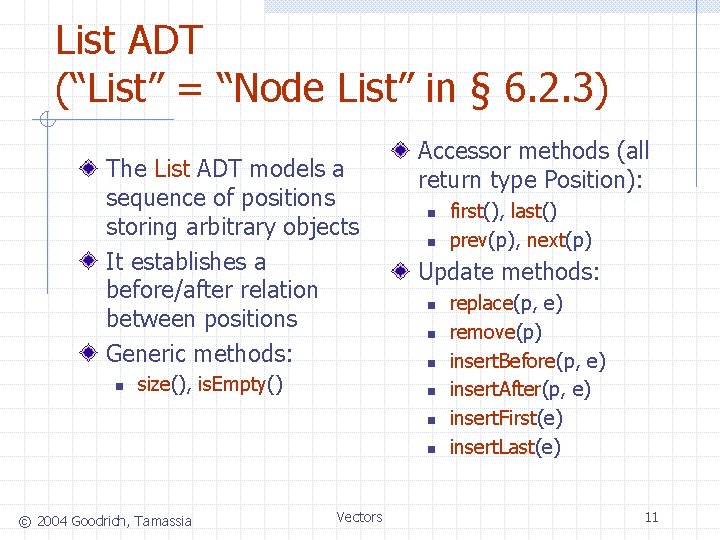
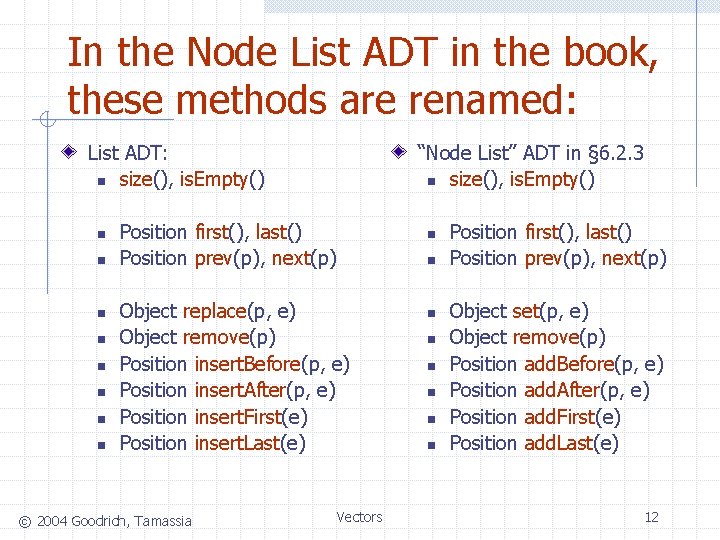
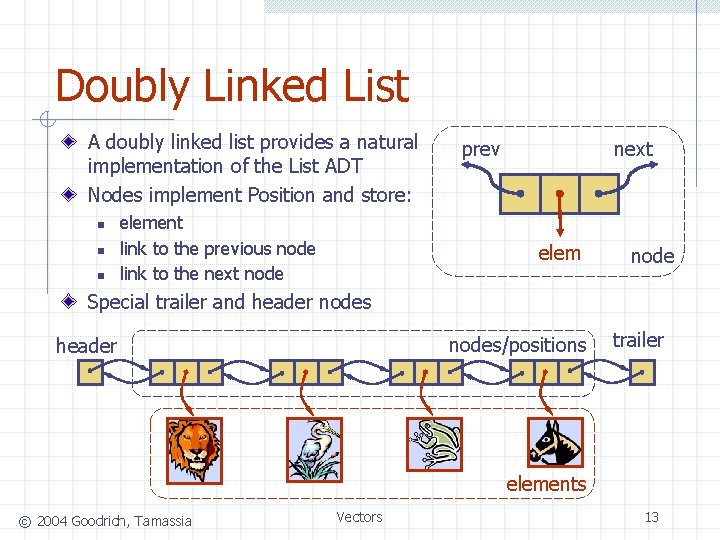
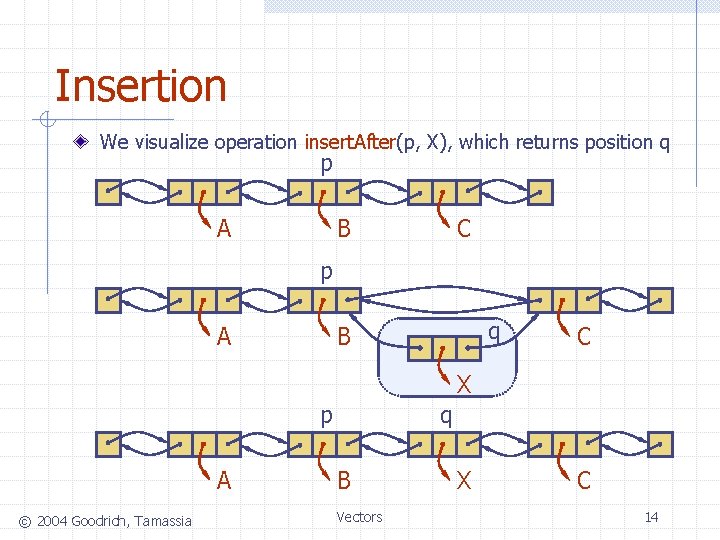
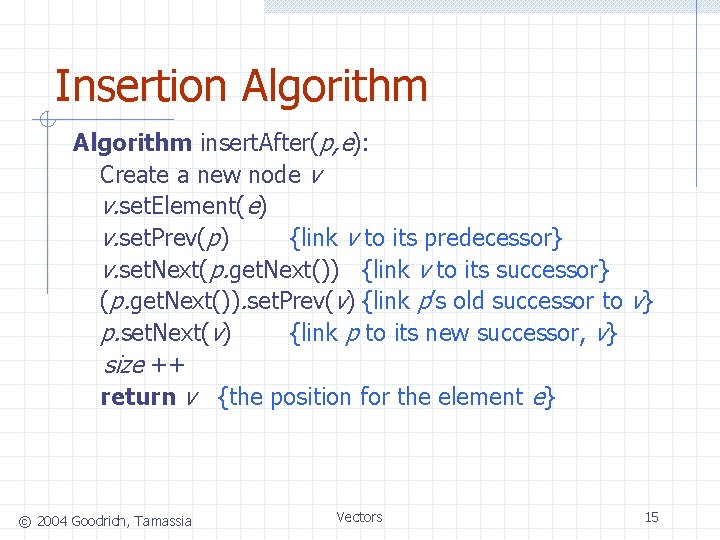
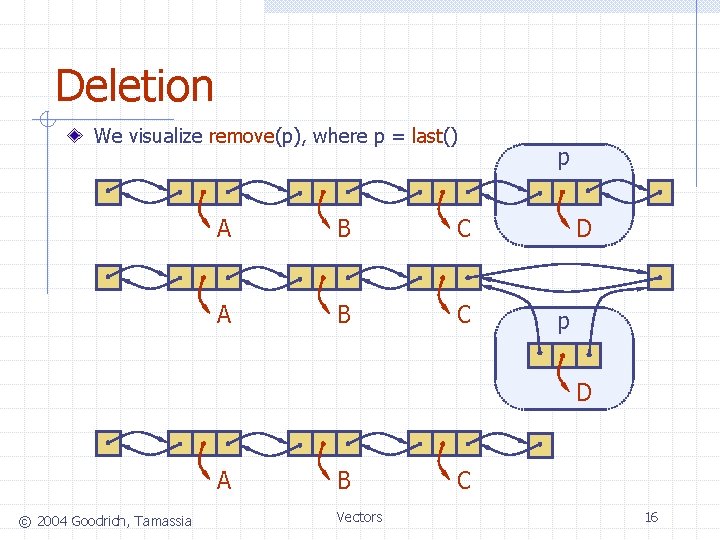
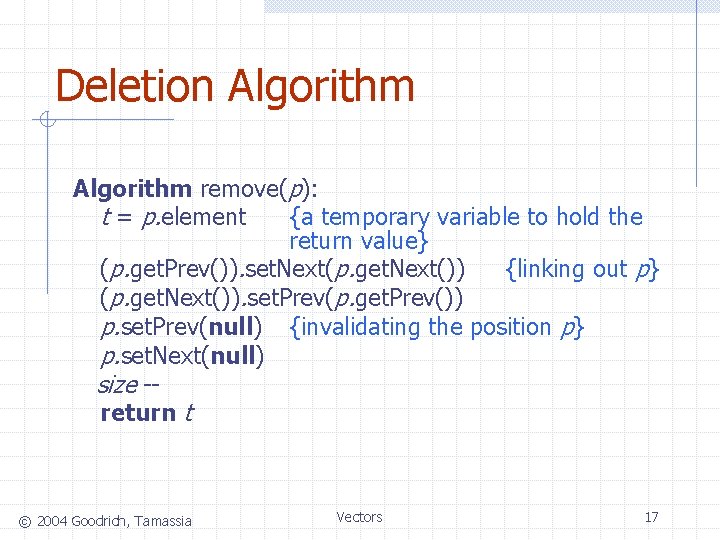
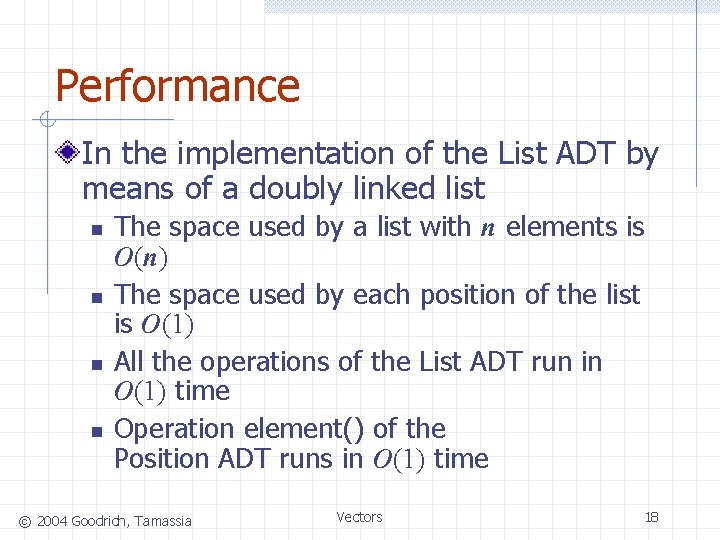
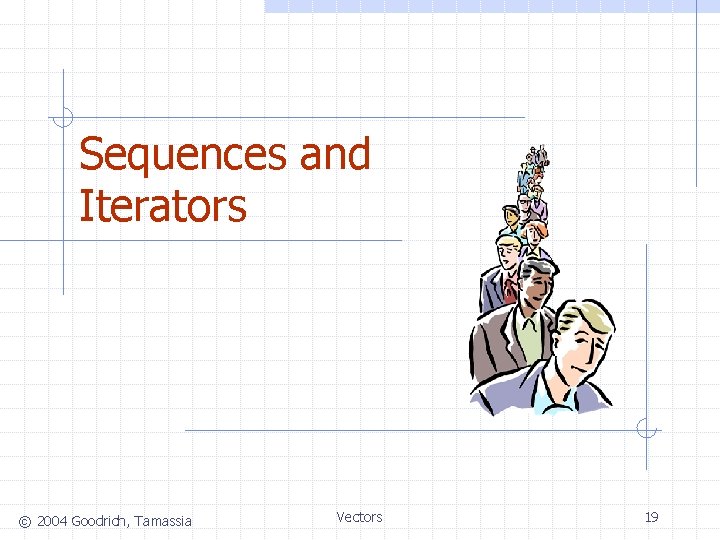
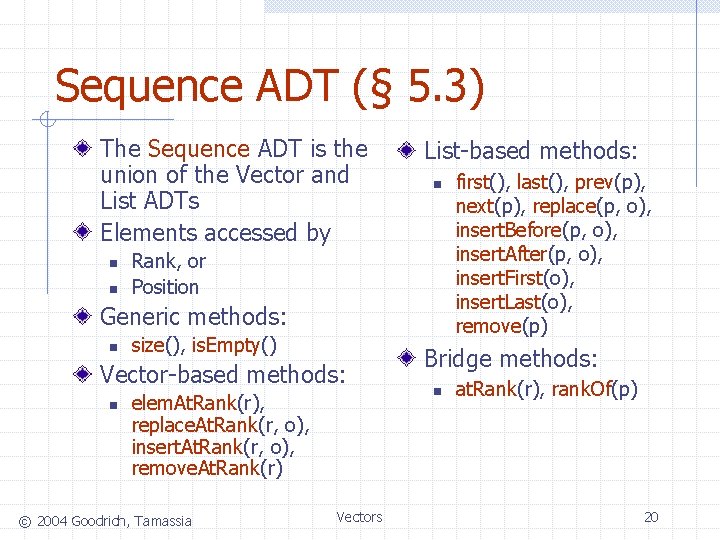
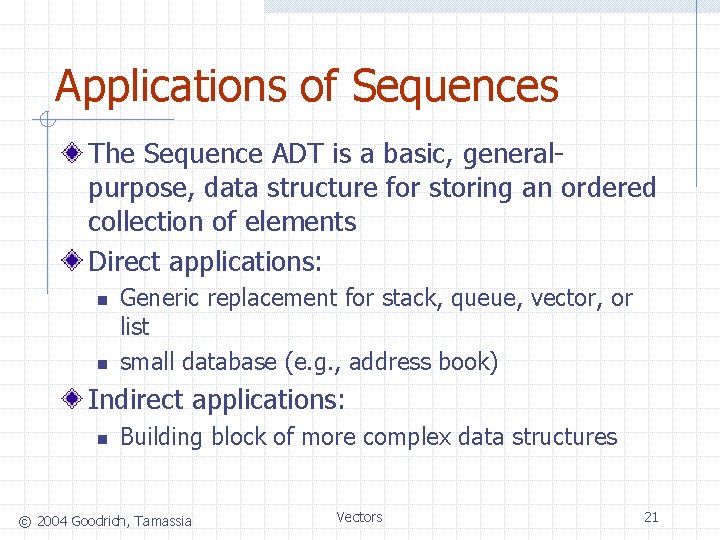
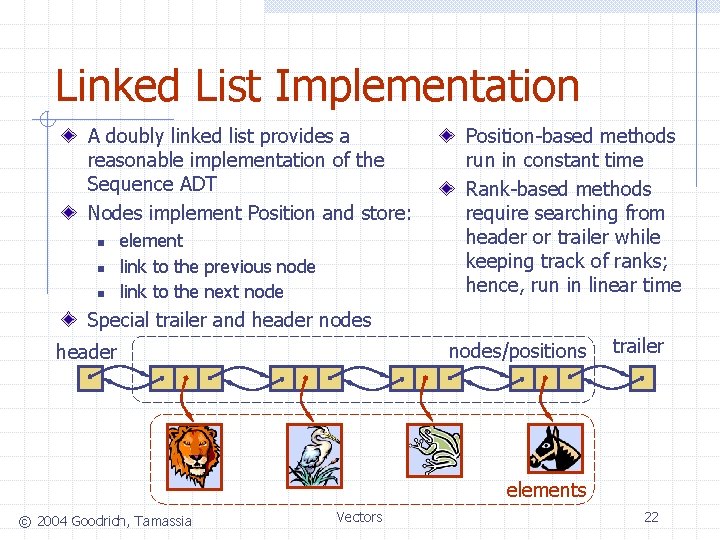
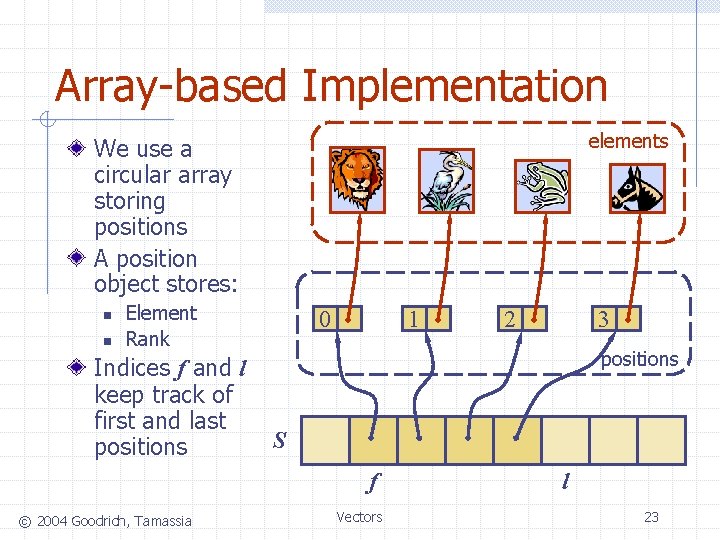
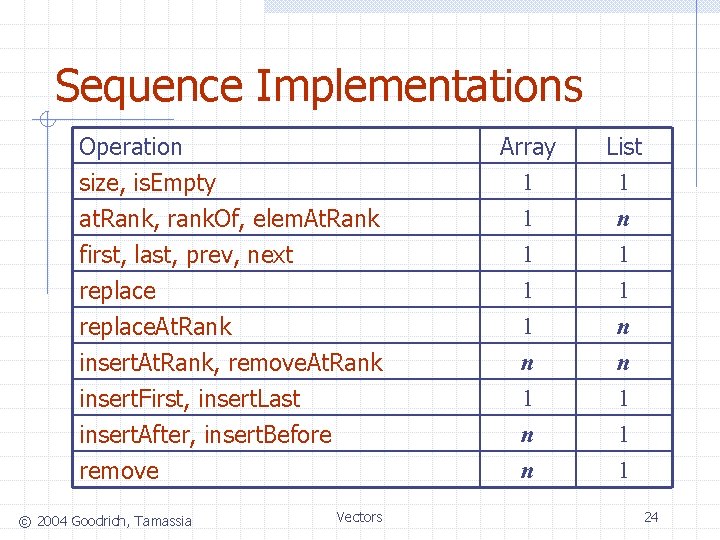
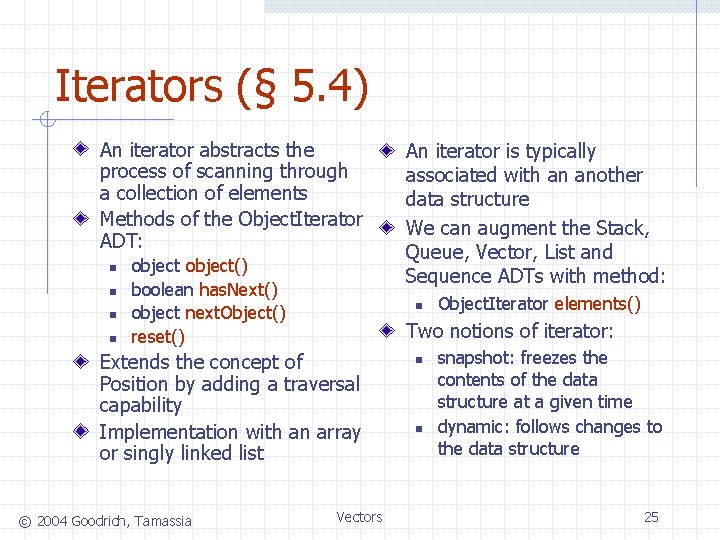
- Slides: 25
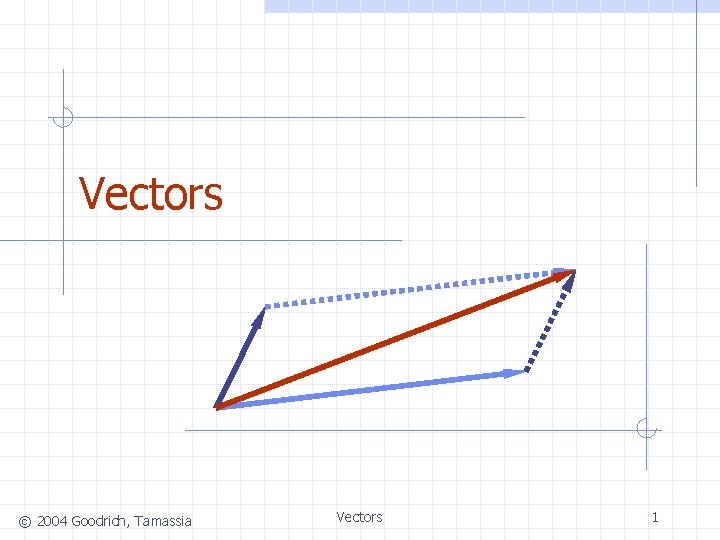
Vectors © 2004 Goodrich, Tamassia Vectors 1
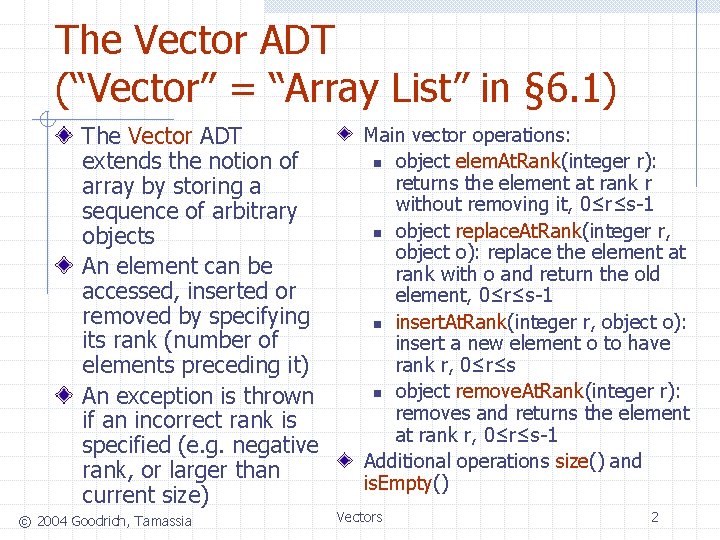
The Vector ADT (“Vector” = “Array List” in § 6. 1) The Vector ADT extends the notion of array by storing a sequence of arbitrary objects An element can be accessed, inserted or removed by specifying its rank (number of elements preceding it) An exception is thrown if an incorrect rank is specified (e. g. negative rank, or larger than current size) © 2004 Goodrich, Tamassia Main vector operations: n object elem. At. Rank(integer r): returns the element at rank r without removing it, 0≤r≤s-1 n object replace. At. Rank(integer r, object o): replace the element at rank with o and return the old element, 0≤r≤s-1 n insert. At. Rank(integer r, object o): insert a new element o to have rank r, 0≤r≤s n object remove. At. Rank(integer r): removes and returns the element at rank r, 0≤r≤s-1 Additional operations size() and is. Empty() Vectors 2
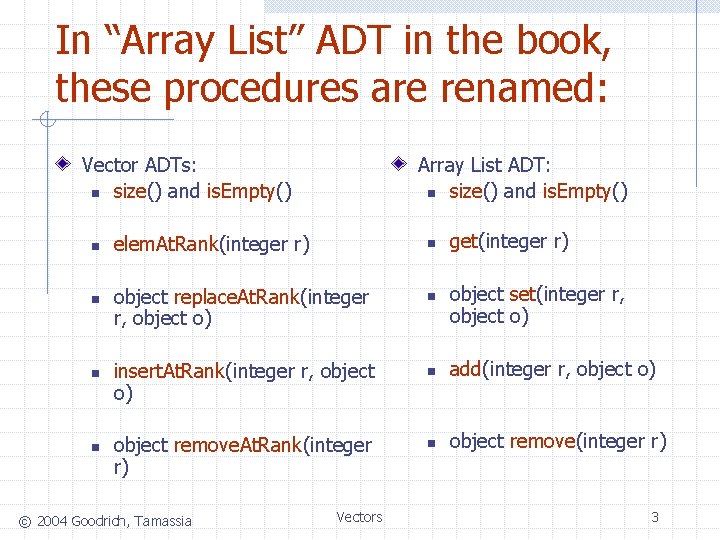
In “Array List” ADT in the book, these procedures are renamed: Vector ADTs: n size() and is. Empty() n n Array List ADT: n size() and is. Empty() elem. At. Rank(integer r) n object replace. At. Rank(integer r, object o) insert. At. Rank(integer r, object o) object remove. At. Rank(integer r) © 2004 Goodrich, Tamassia Vectors n get(integer r) object set(integer r, object o) n add(integer r, object o) n object remove(integer r) 3
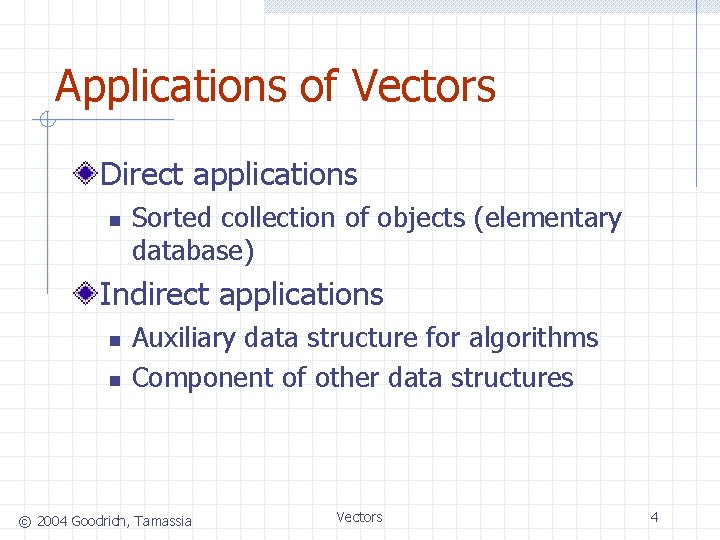
Applications of Vectors Direct applications n Sorted collection of objects (elementary database) Indirect applications n n Auxiliary data structure for algorithms Component of other data structures © 2004 Goodrich, Tamassia Vectors 4
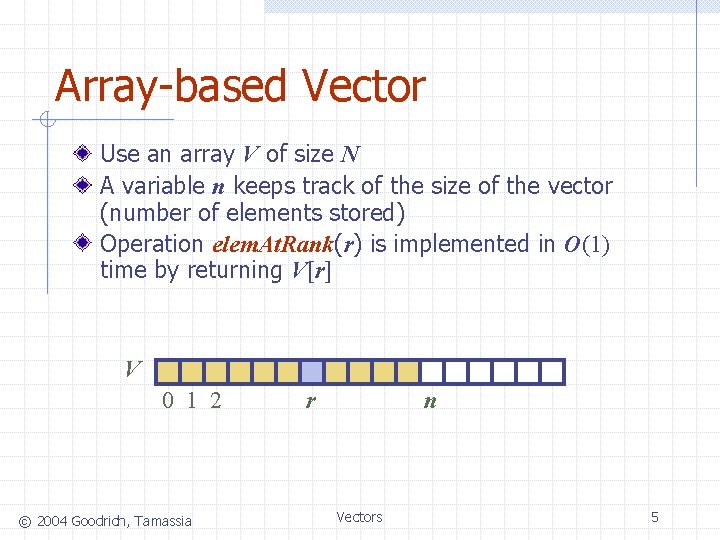
Array-based Vector Use an array V of size N A variable n keeps track of the size of the vector (number of elements stored) Operation elem. At. Rank(r) is implemented in O(1) time by returning V[r] V 0 1 2 © 2004 Goodrich, Tamassia n r Vectors 5
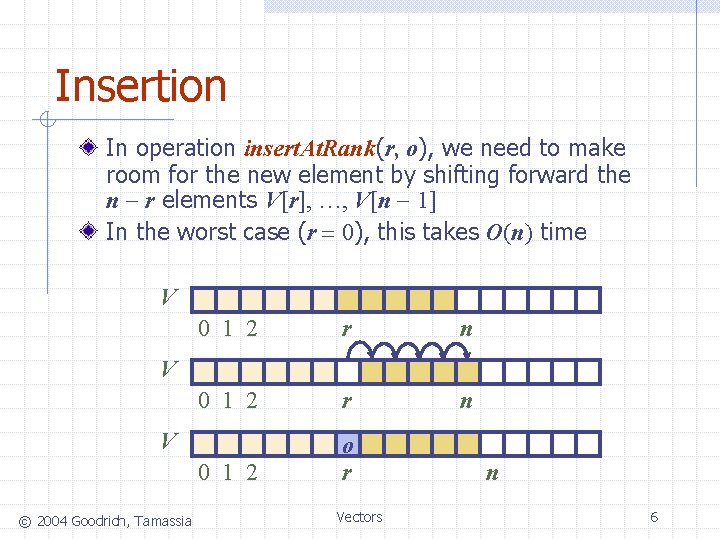
Insertion In operation insert. At. Rank(r, o), we need to make room for the new element by shifting forward the n - r elements V[r], …, V[n - 1] In the worst case (r = 0), this takes O(n) time V 0 1 2 r n 0 1 2 o r V V © 2004 Goodrich, Tamassia Vectors n 6
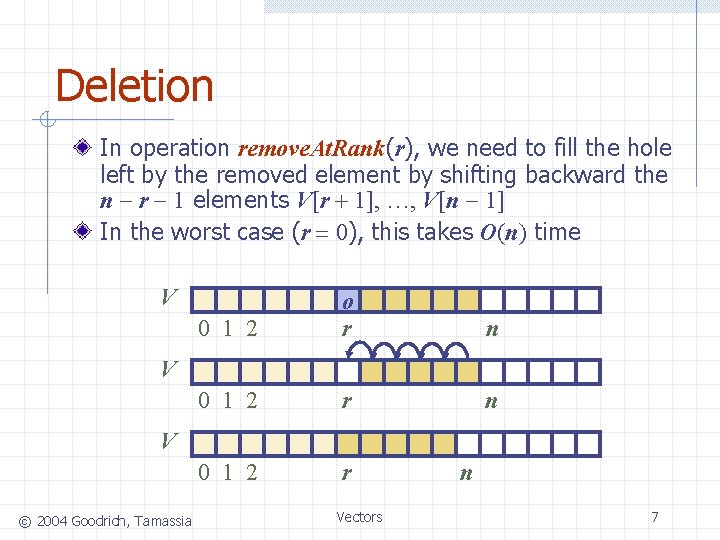
Deletion In operation remove. At. Rank(r), we need to fill the hole left by the removed element by shifting backward the n - r - 1 elements V[r + 1], …, V[n - 1] In the worst case (r = 0), this takes O(n) time V 0 1 2 o r n 0 1 2 r V V © 2004 Goodrich, Tamassia Vectors n 7
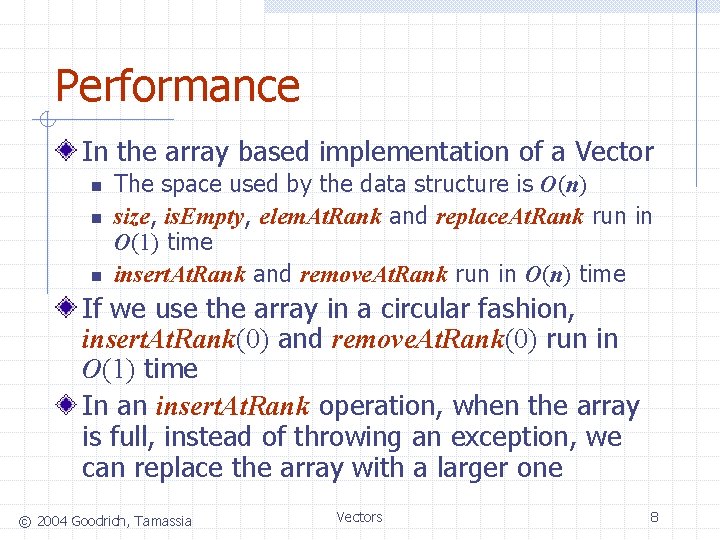
Performance In the array based implementation of a Vector n n n The space used by the data structure is O(n) size, is. Empty, elem. At. Rank and replace. At. Rank run in O(1) time insert. At. Rank and remove. At. Rank run in O(n) time If we use the array in a circular fashion, insert. At. Rank(0) and remove. At. Rank(0) run in O(1) time In an insert. At. Rank operation, when the array is full, instead of throwing an exception, we can replace the array with a larger one © 2004 Goodrich, Tamassia Vectors 8
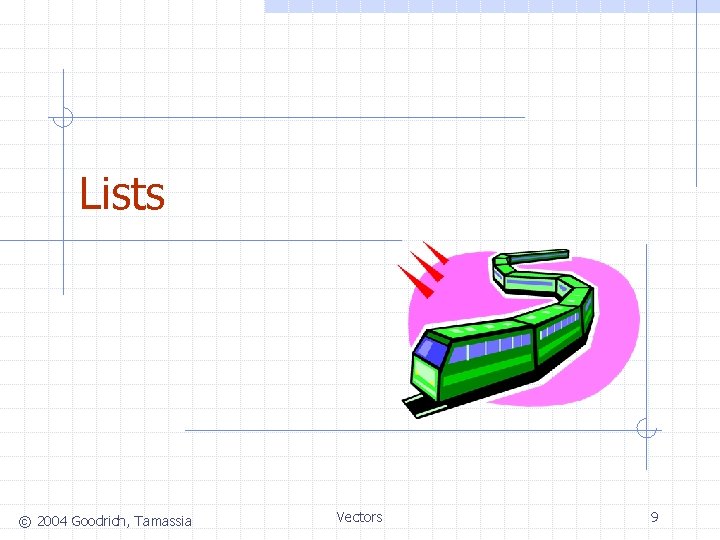
Lists © 2004 Goodrich, Tamassia Vectors 9
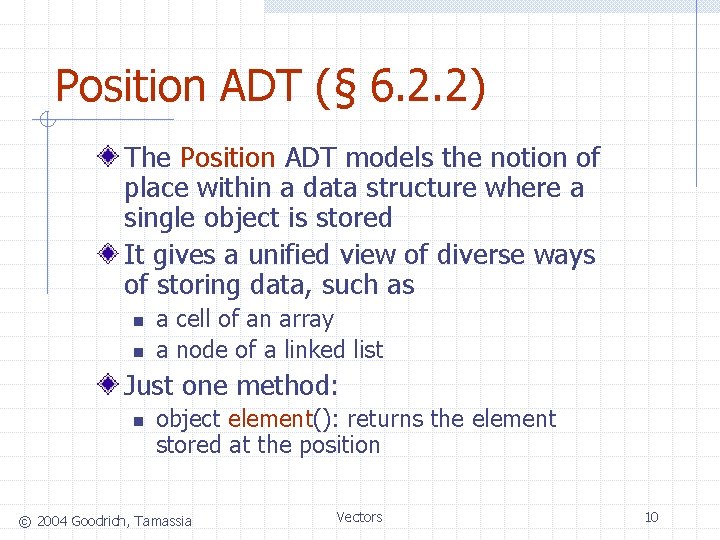
Position ADT (§ 6. 2. 2) The Position ADT models the notion of place within a data structure where a single object is stored It gives a unified view of diverse ways of storing data, such as n n a cell of an array a node of a linked list Just one method: n object element(): returns the element stored at the position © 2004 Goodrich, Tamassia Vectors 10
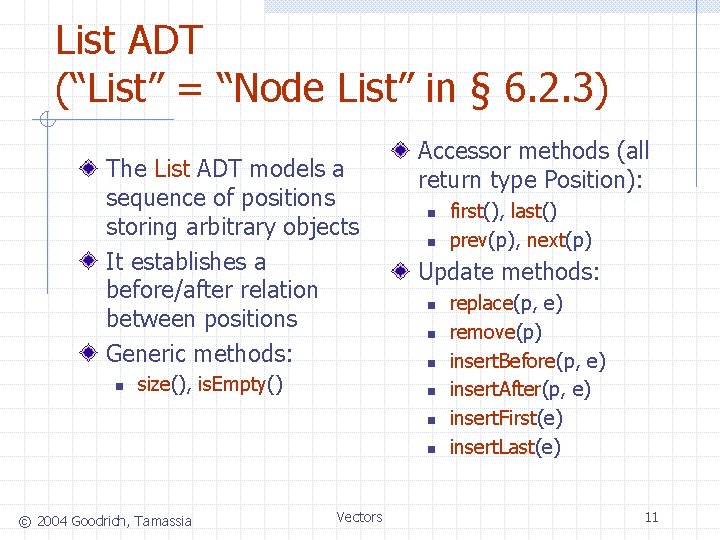
List ADT (“List” = “Node List” in § 6. 2. 3) The List ADT models a sequence of positions storing arbitrary objects It establishes a before/after relation between positions Generic methods: n size(), is. Empty() Accessor methods (all return type Position): n n Update methods: n n n © 2004 Goodrich, Tamassia Vectors first(), last() prev(p), next(p) replace(p, e) remove(p) insert. Before(p, e) insert. After(p, e) insert. First(e) insert. Last(e) 11
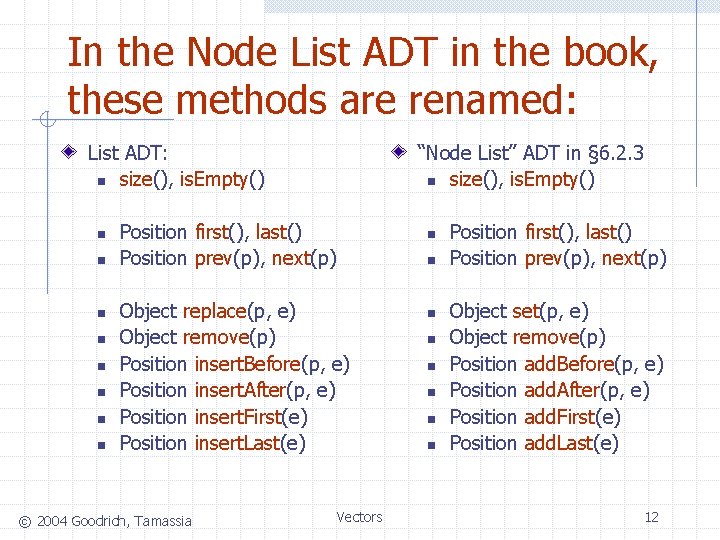
In the Node List ADT in the book, these methods are renamed: List ADT: n size(), is. Empty() n n n n “Node List” ADT in § 6. 2. 3 n size(), is. Empty() Position first(), last() Position prev(p), next(p) n n Object replace(p, e) Object remove(p) Position insert. Before(p, e) Position insert. After(p, e) Position insert. First(e) Position insert. Last(e) © 2004 Goodrich, Tamassia Vectors n n n Position first(), last() Position prev(p), next(p) Object set(p, e) Object remove(p) Position add. Before(p, e) Position add. After(p, e) Position add. First(e) Position add. Last(e) 12
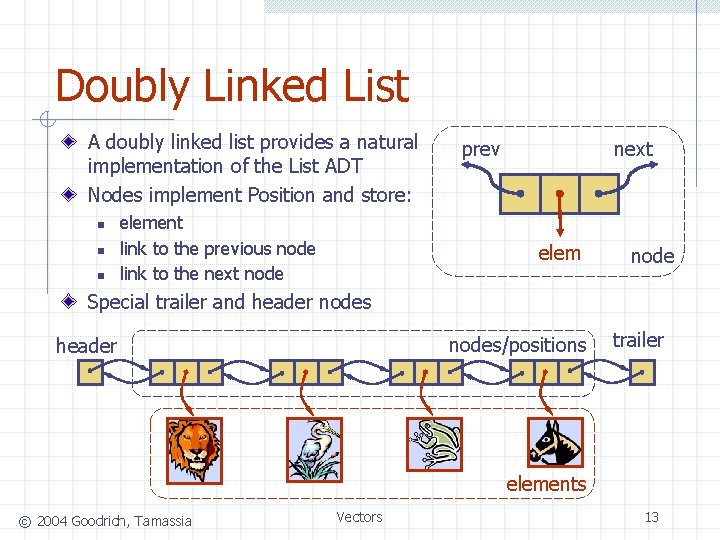
Doubly Linked List A doubly linked list provides a natural implementation of the List ADT Nodes implement Position and store: n n n element link to the previous node link to the next node prev next elem node Special trailer and header nodes/positions header trailer elements © 2004 Goodrich, Tamassia Vectors 13
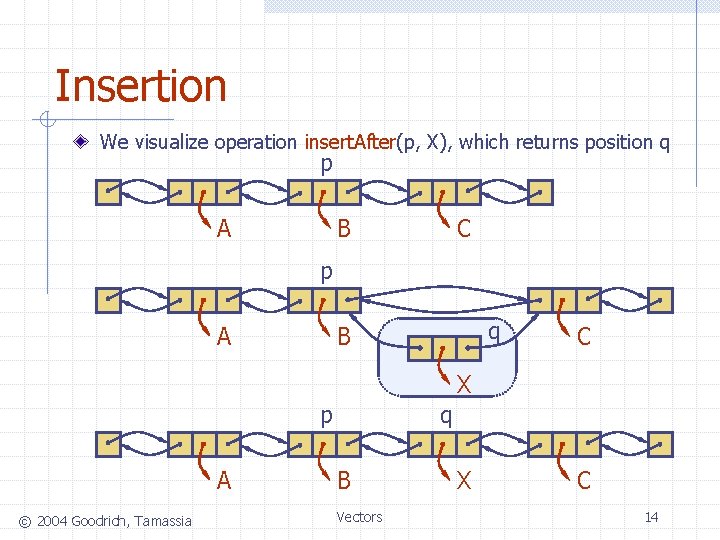
Insertion We visualize operation insert. After(p, X), which returns position q p A B C p A q B C X p A © 2004 Goodrich, Tamassia q B Vectors X C 14
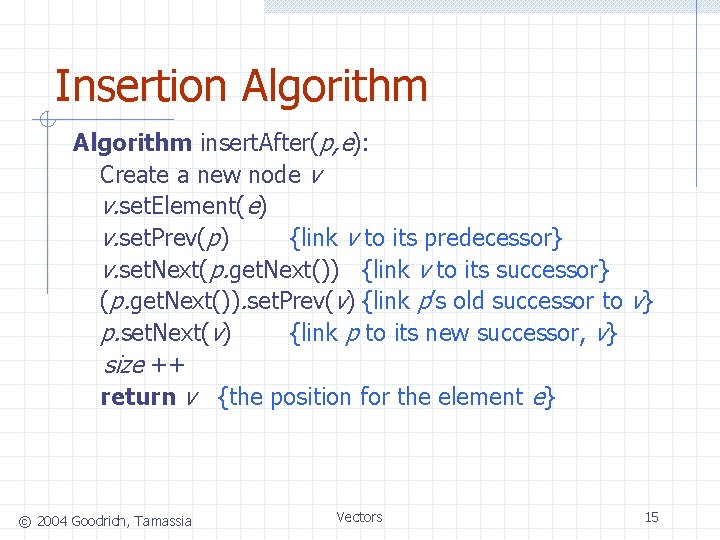
Insertion Algorithm insert. After(p, e): Create a new node v v. set. Element(e) v. set. Prev(p) {link v to its predecessor} v. set. Next(p. get. Next()) {link v to its successor} (p. get. Next()). set. Prev(v) {link p’s old successor to v} p. set. Next(v) {link p to its new successor, v} size ++ return v {the position for the element e} © 2004 Goodrich, Tamassia Vectors 15
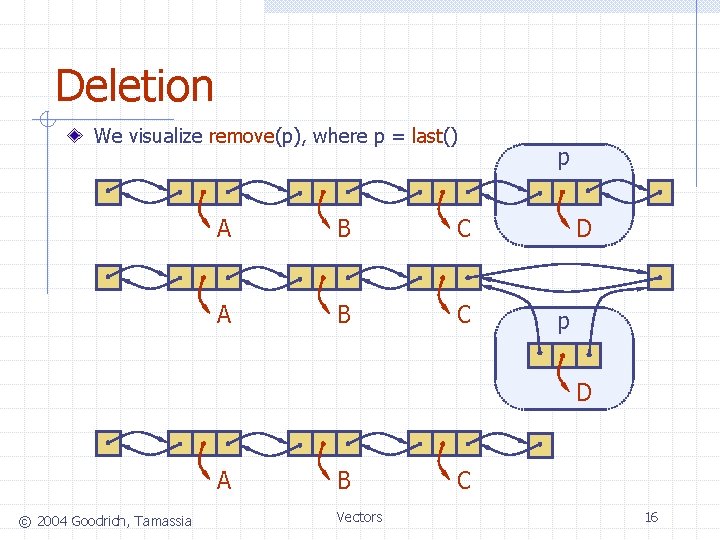
Deletion We visualize remove(p), where p = last() A B C p D A © 2004 Goodrich, Tamassia B Vectors C 16
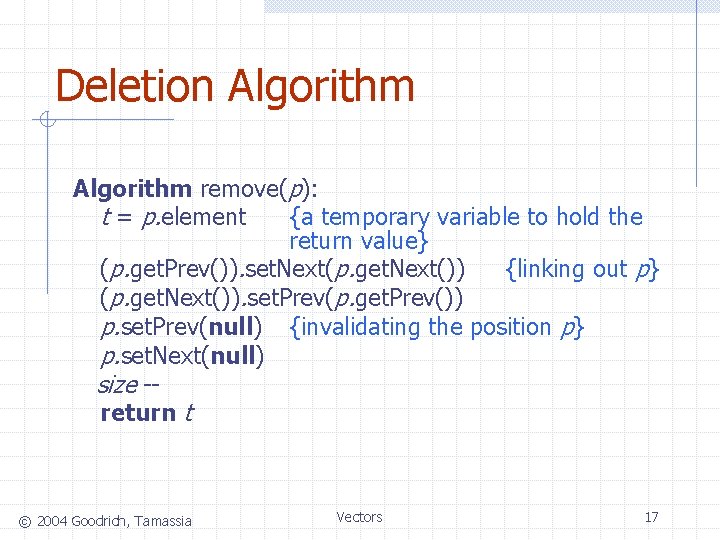
Deletion Algorithm remove(p): t = p. element {a temporary variable to hold the return value} (p. get. Prev()). set. Next(p. get. Next()) {linking out p} (p. get. Next()). set. Prev(p. get. Prev()) p. set. Prev(null) {invalidating the position p} p. set. Next(null) size -return t © 2004 Goodrich, Tamassia Vectors 17
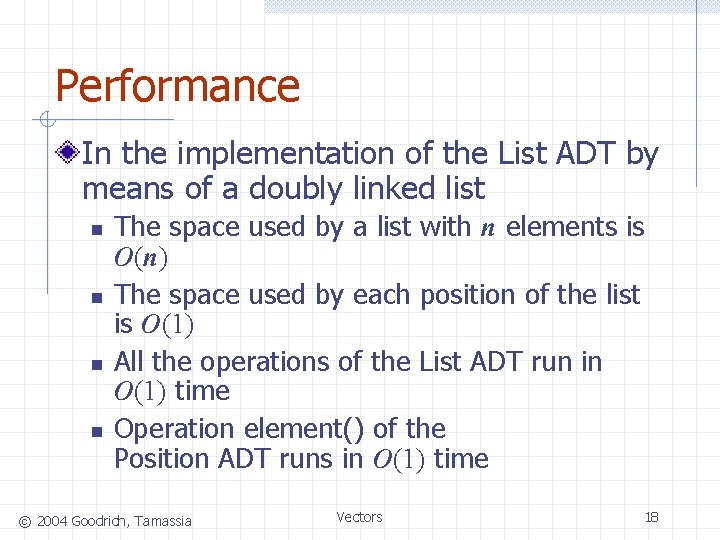
Performance In the implementation of the List ADT by means of a doubly linked list n n The space used by a list with n elements is O(n) The space used by each position of the list is O(1) All the operations of the List ADT run in O(1) time Operation element() of the Position ADT runs in O(1) time © 2004 Goodrich, Tamassia Vectors 18
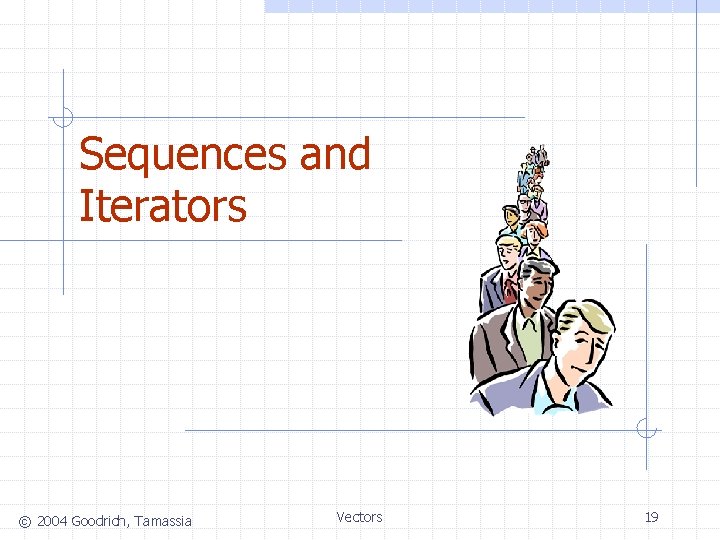
Sequences and Iterators © 2004 Goodrich, Tamassia Vectors 19
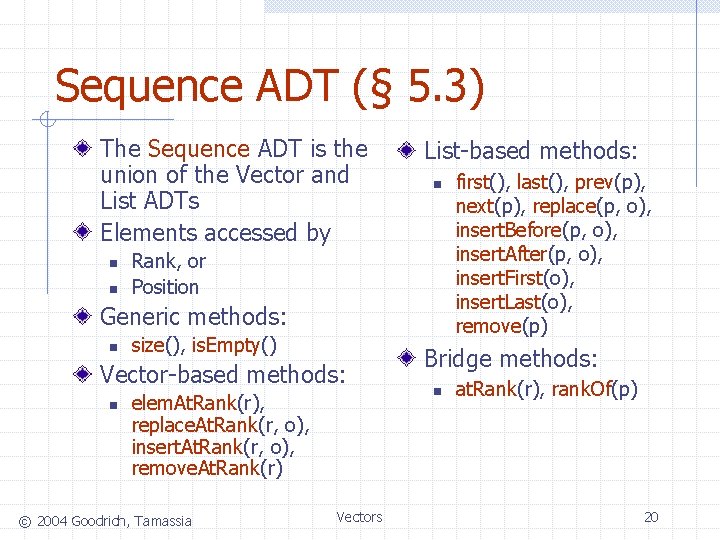
Sequence ADT (§ 5. 3) The Sequence ADT is the union of the Vector and List ADTs Elements accessed by n n List-based methods: n Rank, or Position Generic methods: n size(), is. Empty() Vector-based methods: n elem. At. Rank(r), replace. At. Rank(r, o), insert. At. Rank(r, o), remove. At. Rank(r) © 2004 Goodrich, Tamassia Vectors first(), last(), prev(p), next(p), replace(p, o), insert. Before(p, o), insert. After(p, o), insert. First(o), insert. Last(o), remove(p) Bridge methods: n at. Rank(r), rank. Of(p) 20
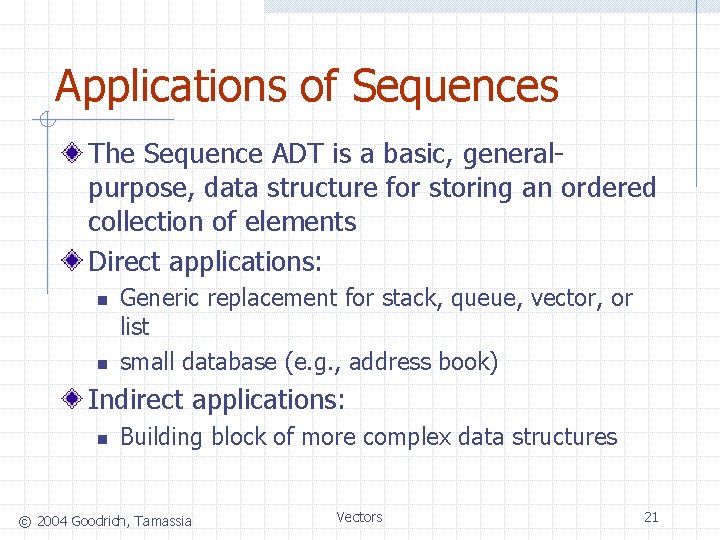
Applications of Sequences The Sequence ADT is a basic, generalpurpose, data structure for storing an ordered collection of elements Direct applications: n n Generic replacement for stack, queue, vector, or list small database (e. g. , address book) Indirect applications: n Building block of more complex data structures © 2004 Goodrich, Tamassia Vectors 21
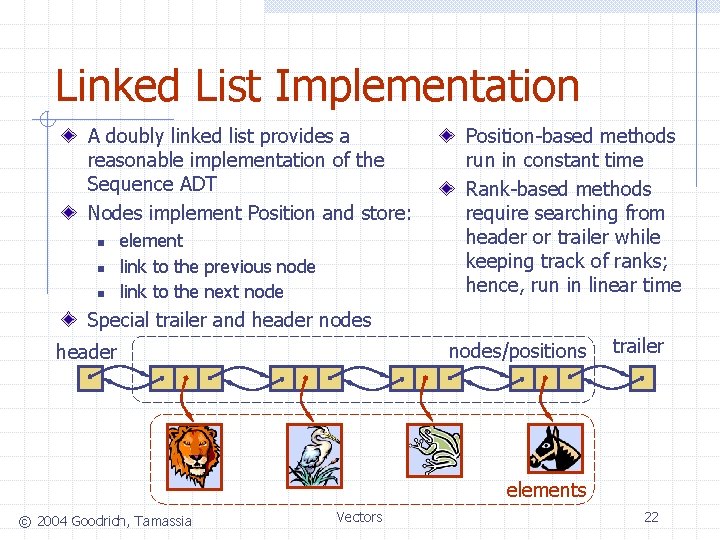
Linked List Implementation A doubly linked list provides a reasonable implementation of the Sequence ADT Nodes implement Position and store: n n n element link to the previous node link to the next node Position-based methods run in constant time Rank-based methods require searching from header or trailer while keeping track of ranks; hence, run in linear time Special trailer and header nodes/positions header trailer elements © 2004 Goodrich, Tamassia Vectors 22
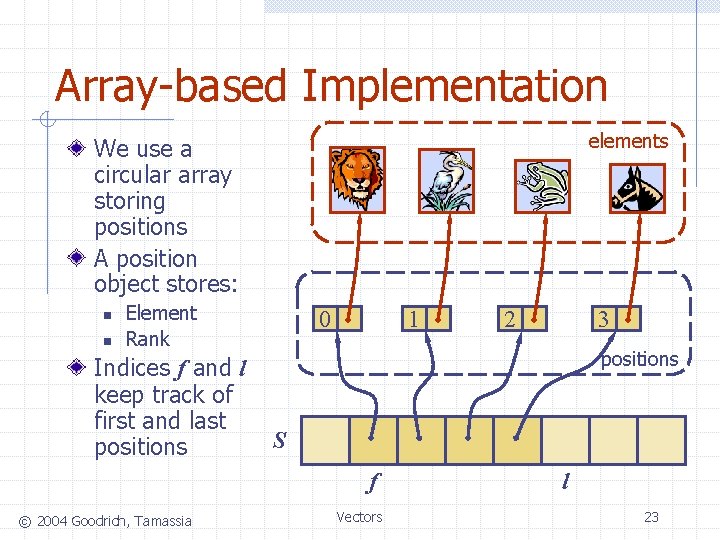
Array-based Implementation elements We use a circular array storing positions A position object stores: n n Element Rank Indices f and l keep track of first and last positions 0 1 3 positions S f © 2004 Goodrich, Tamassia 2 Vectors l 23
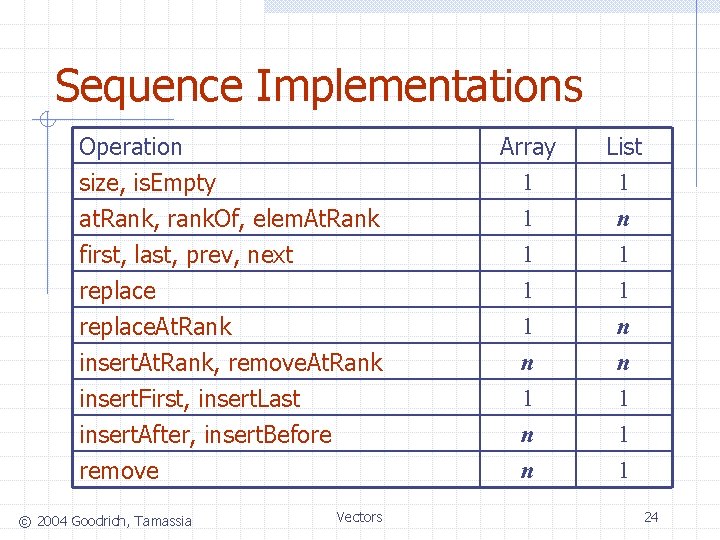
Sequence Implementations Operation size, is. Empty at. Rank, rank. Of, elem. At. Rank first, last, prev, next Array 1 1 1 List 1 n 1 replace. At. Rank insert. At. Rank, remove. At. Rank insert. First, insert. Last insert. After, insert. Before remove 1 1 n n 1 1 1 © 2004 Goodrich, Tamassia Vectors 24
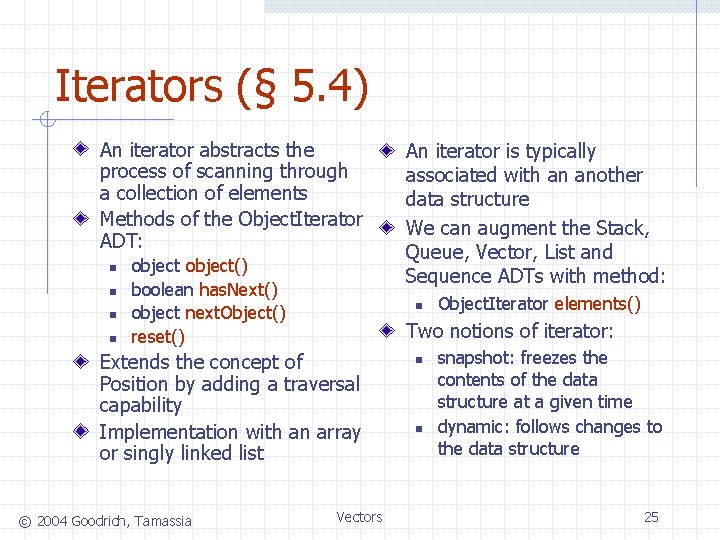
Iterators (§ 5. 4) An iterator abstracts the process of scanning through a collection of elements Methods of the Object. Iterator ADT: n n object() boolean has. Next() object next. Object() reset() n Object. Iterator elements() Two notions of iterator: Extends the concept of Position by adding a traversal capability Implementation with an array or singly linked list © 2004 Goodrich, Tamassia An iterator is typically associated with an another data structure We can augment the Stack, Queue, Vector, List and Sequence ADTs with method: Vectors n n snapshot: freezes the contents of the data structure at a given time dynamic: follows changes to the data structure 25