User Interface Programming in C Direct Manipulation Chris
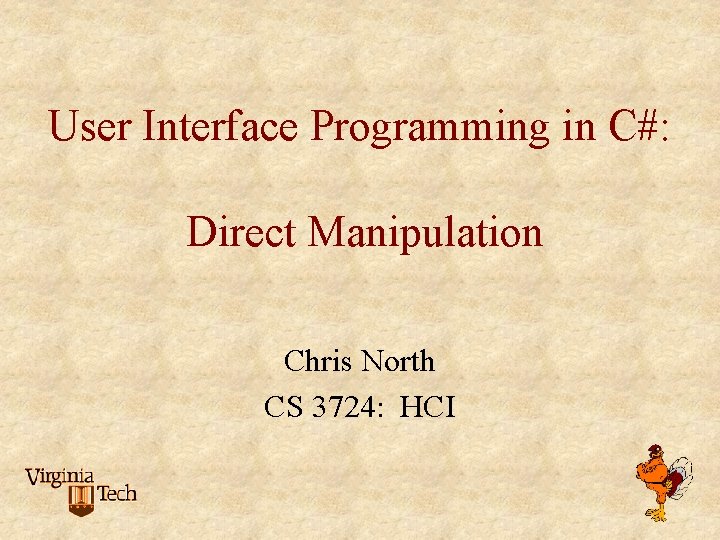
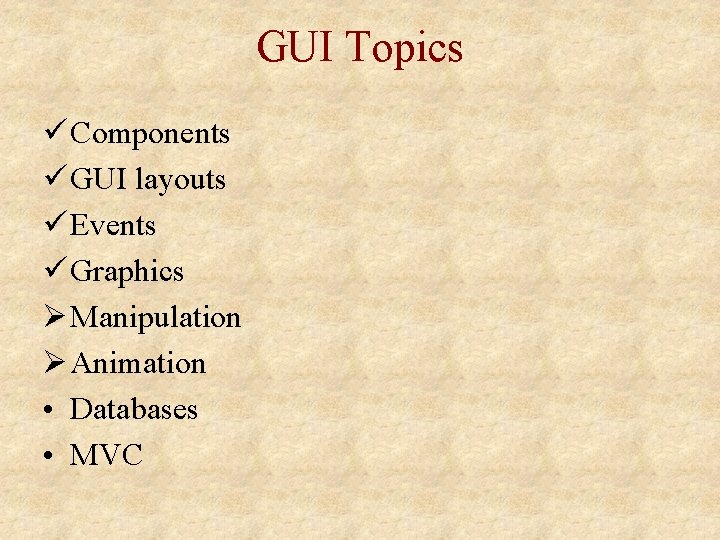
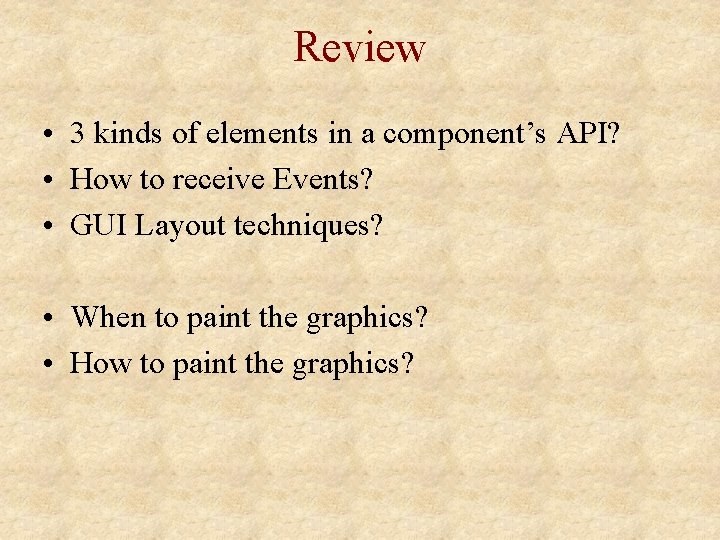
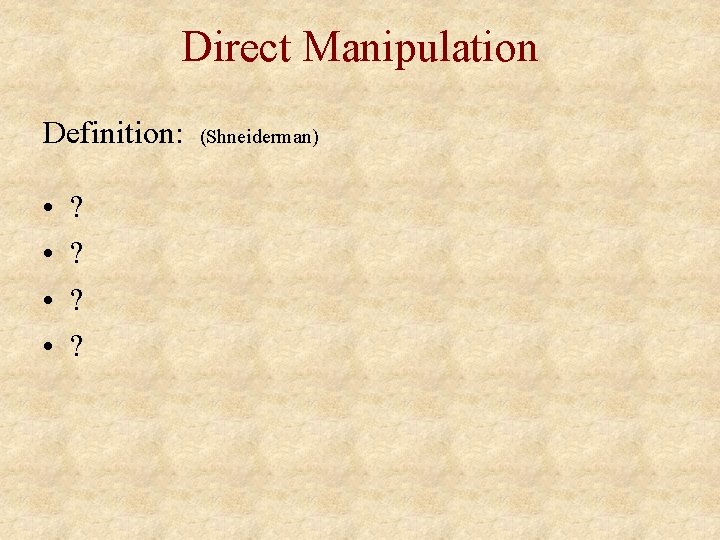
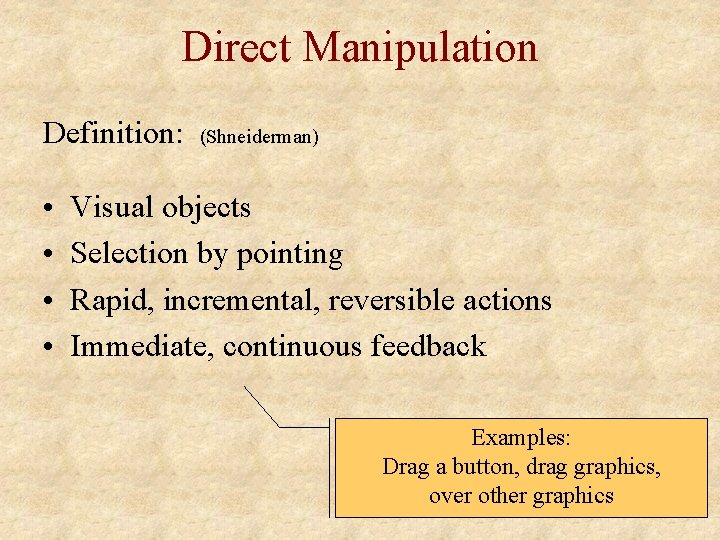
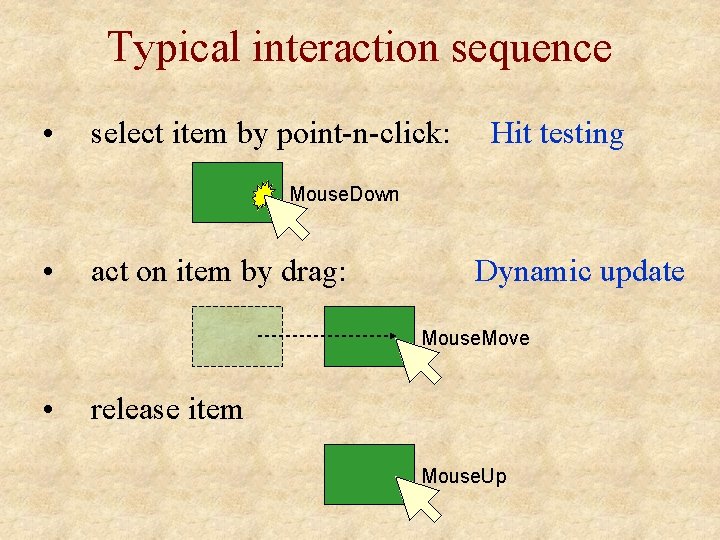
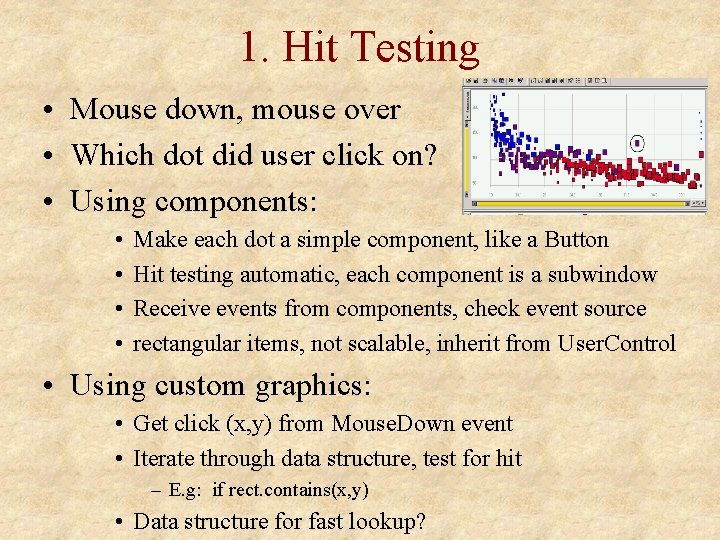
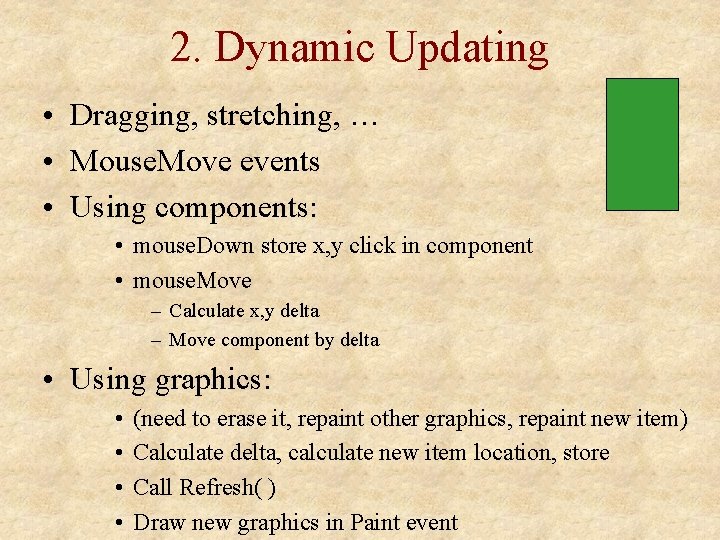
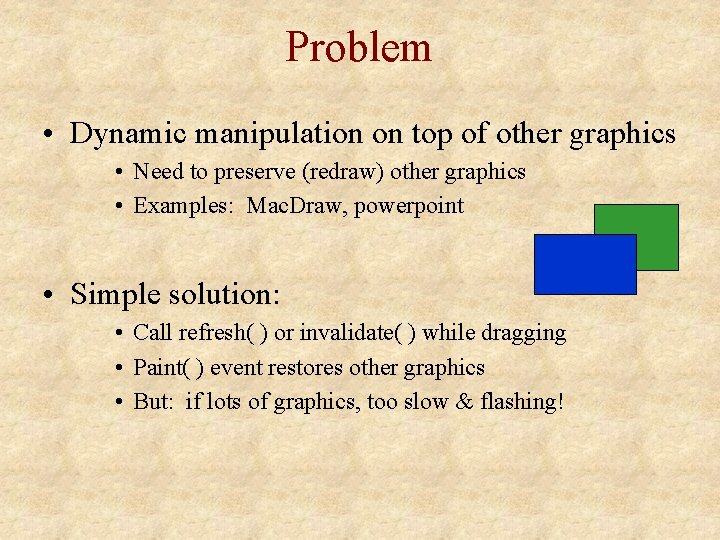
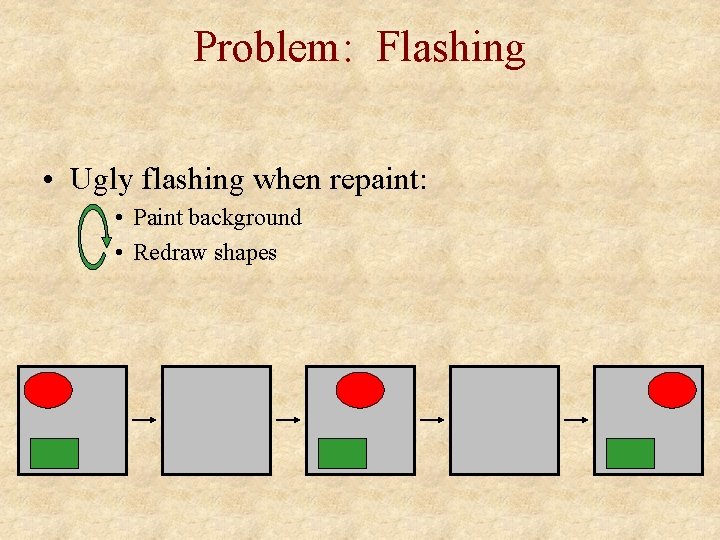
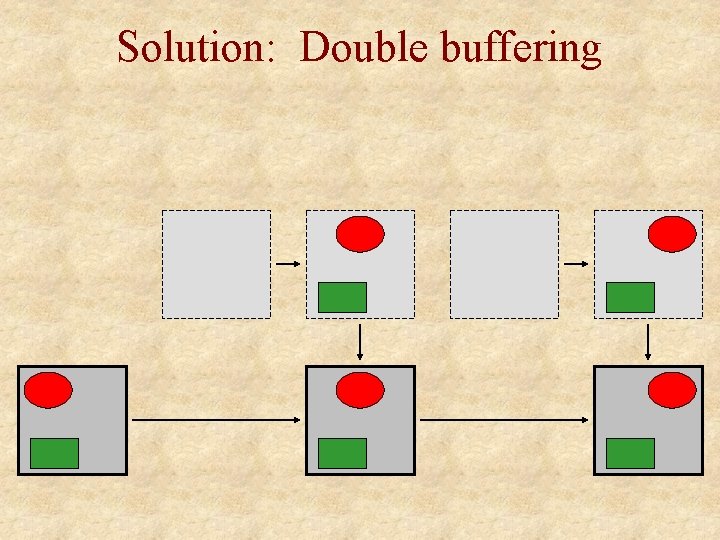
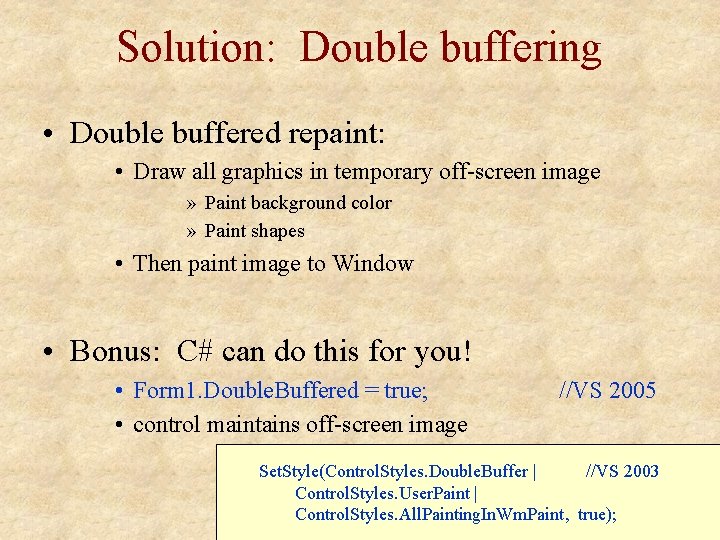
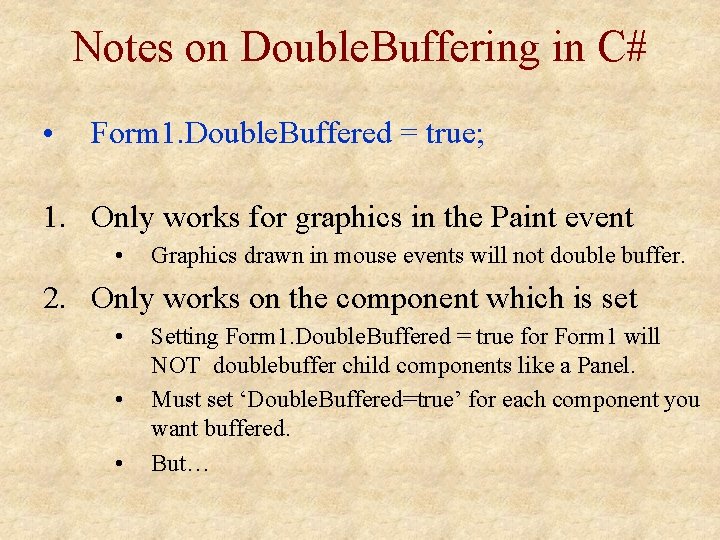
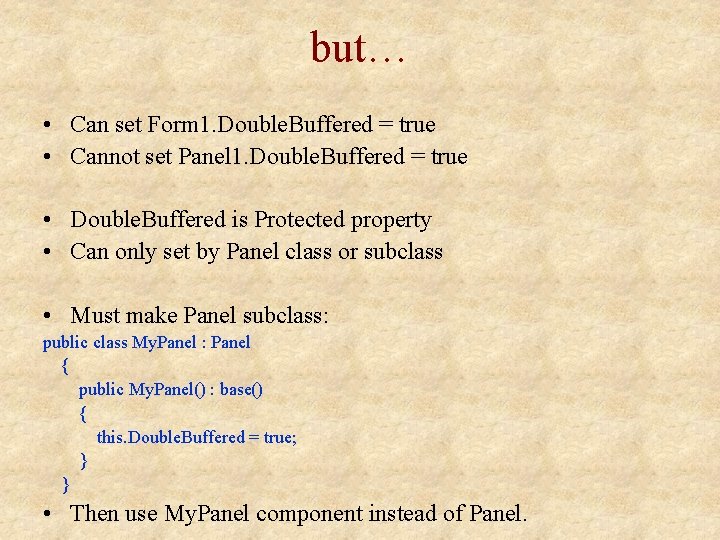
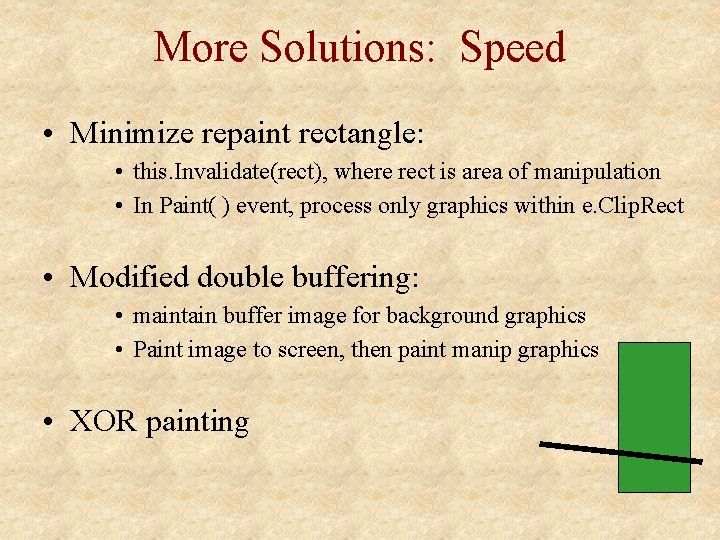
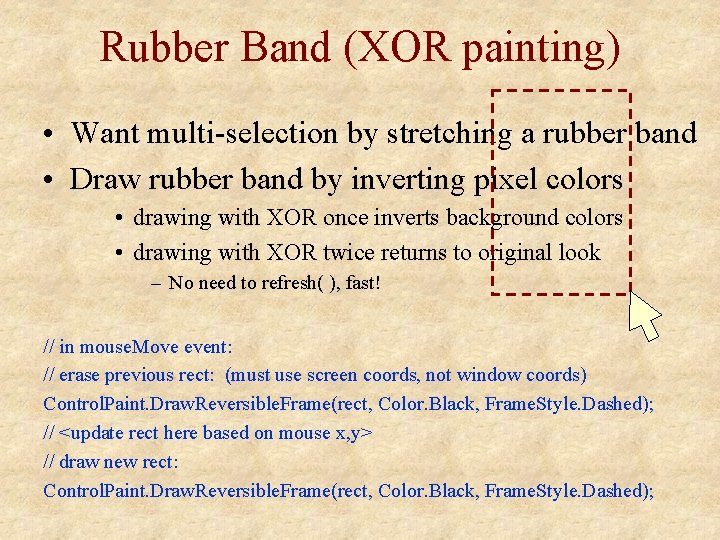
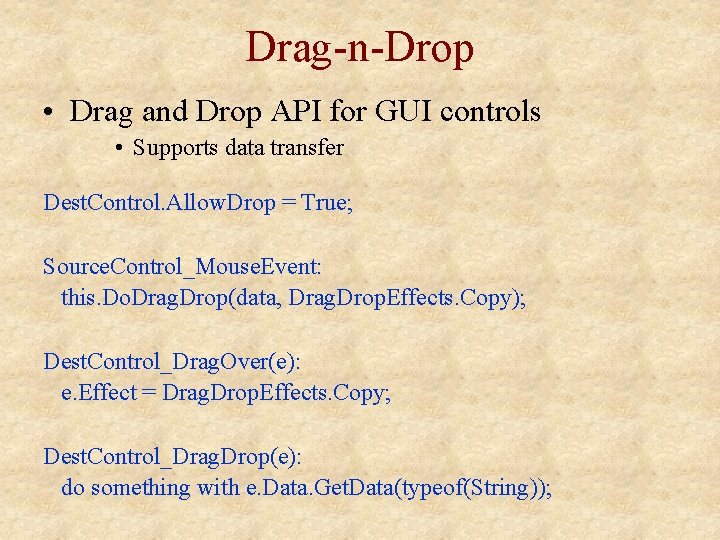
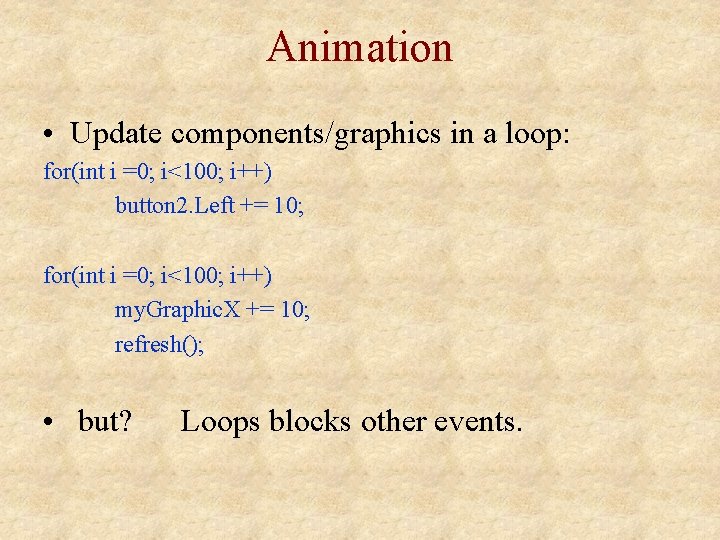
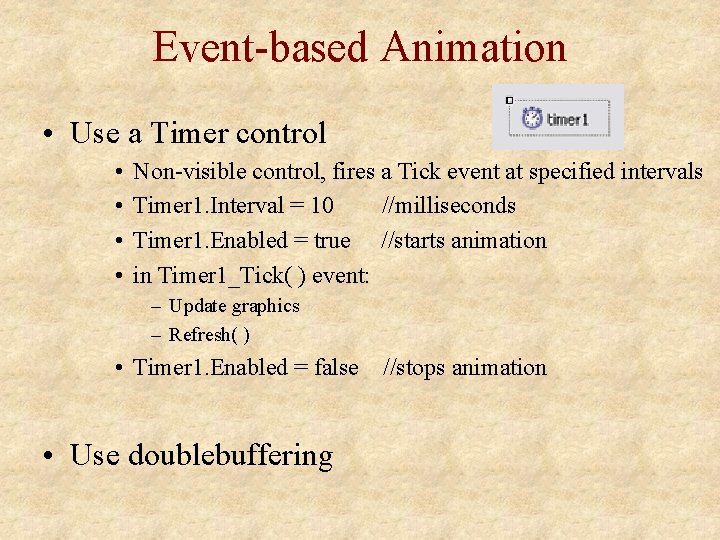
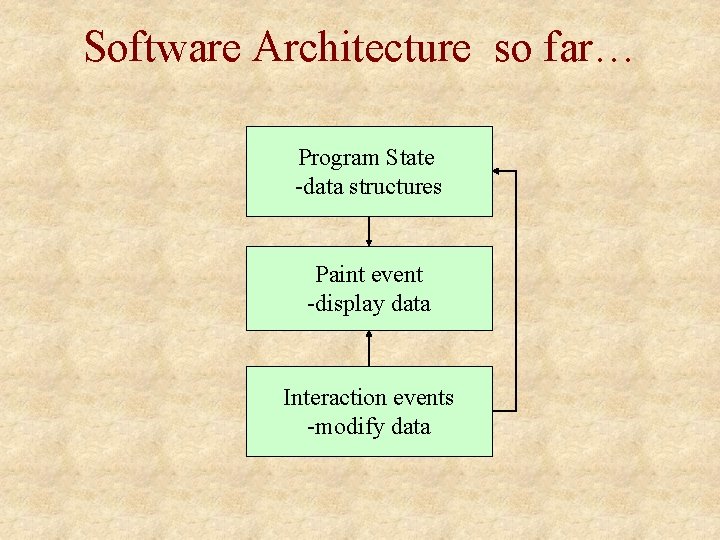
- Slides: 20
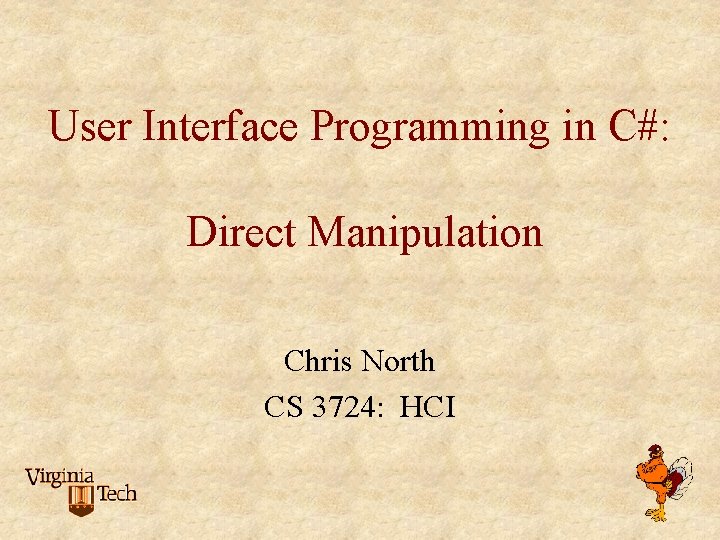
User Interface Programming in C#: Direct Manipulation Chris North CS 3724: HCI
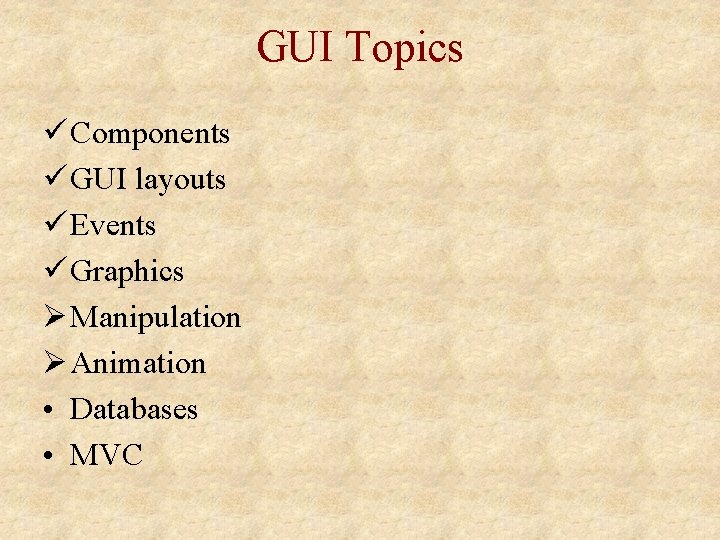
GUI Topics ü Components ü GUI layouts ü Events ü Graphics Ø Manipulation Ø Animation • Databases • MVC
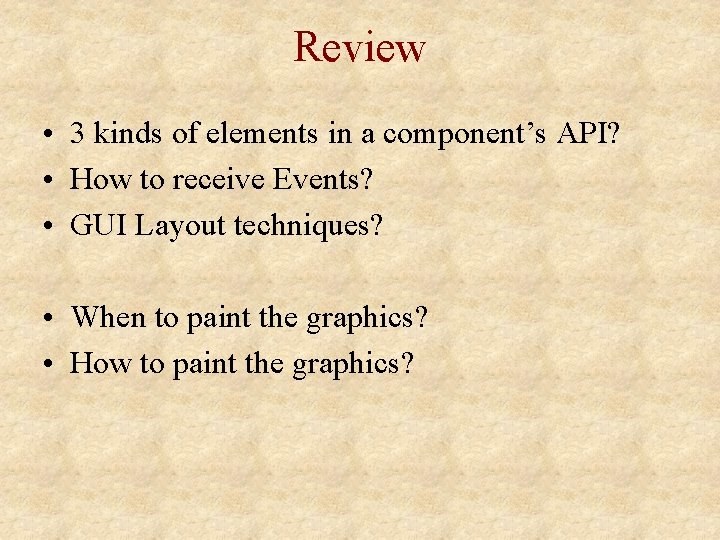
Review • 3 kinds of elements in a component’s API? • How to receive Events? • GUI Layout techniques? • When to paint the graphics? • How to paint the graphics?
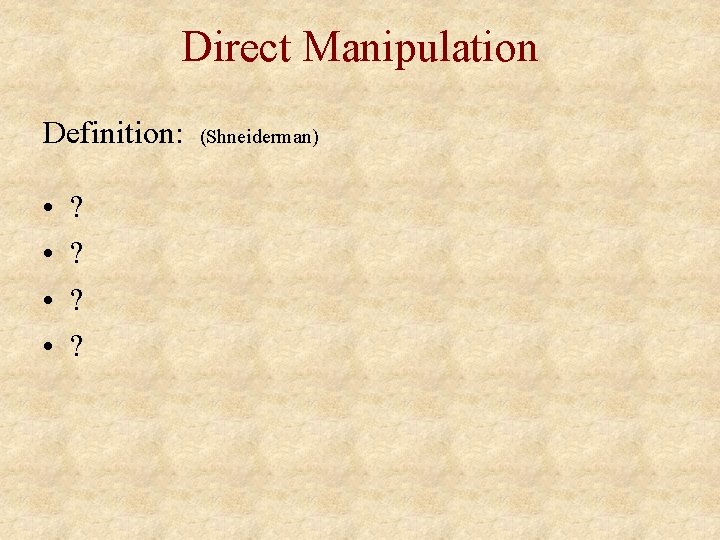
Direct Manipulation Definition: • • ? ? (Shneiderman)
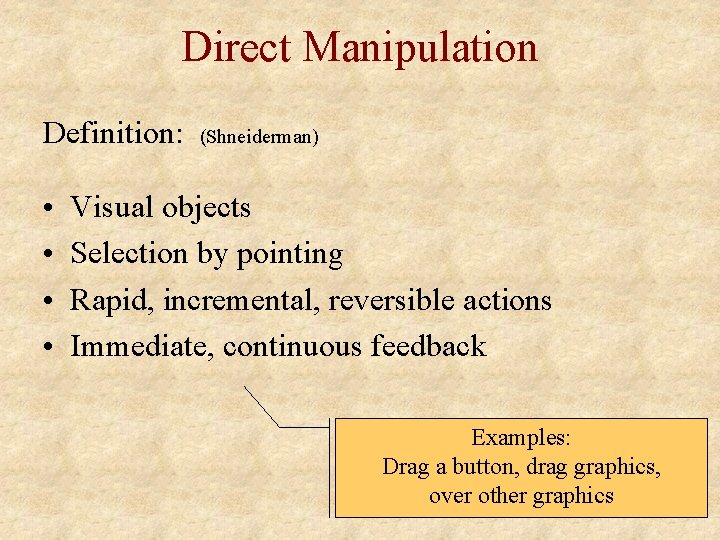
Direct Manipulation Definition: • • (Shneiderman) Visual objects Selection by pointing Rapid, incremental, reversible actions Immediate, continuous feedback Examples: Drag a button, drag graphics, over other graphics
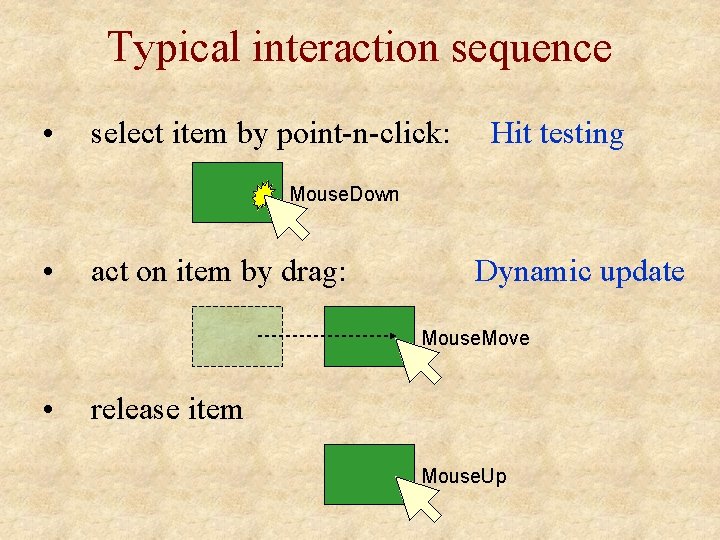
Typical interaction sequence • select item by point-n-click: Hit testing Mouse. Down • act on item by drag: Dynamic update Mouse. Move • release item Mouse. Up
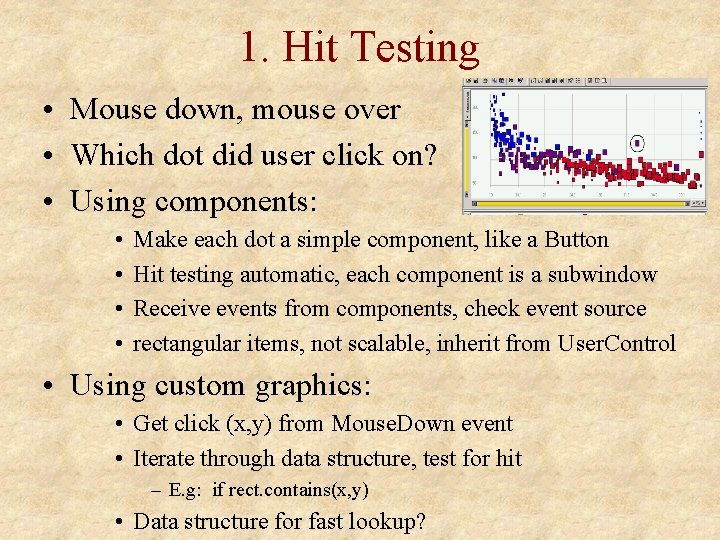
1. Hit Testing • Mouse down, mouse over • Which dot did user click on? • Using components: • • Make each dot a simple component, like a Button Hit testing automatic, each component is a subwindow Receive events from components, check event source rectangular items, not scalable, inherit from User. Control • Using custom graphics: • Get click (x, y) from Mouse. Down event • Iterate through data structure, test for hit – E. g: if rect. contains(x, y) • Data structure for fast lookup?
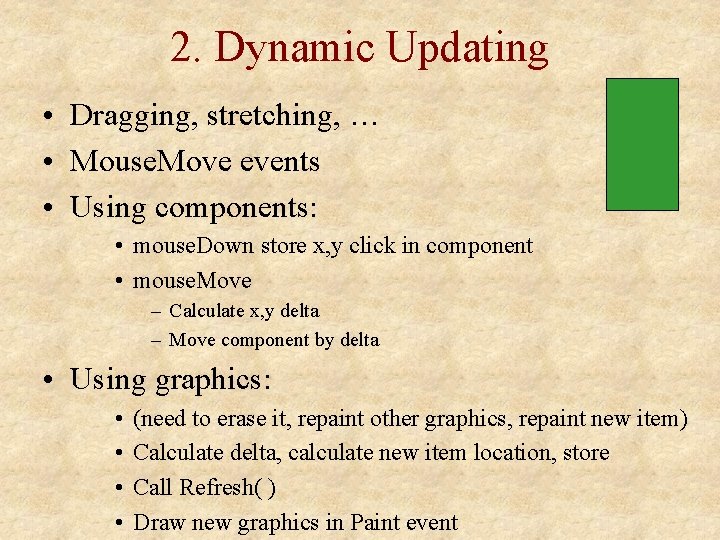
2. Dynamic Updating • Dragging, stretching, … • Mouse. Move events • Using components: • mouse. Down store x, y click in component • mouse. Move – Calculate x, y delta – Move component by delta • Using graphics: • • (need to erase it, repaint other graphics, repaint new item) Calculate delta, calculate new item location, store Call Refresh( ) Draw new graphics in Paint event
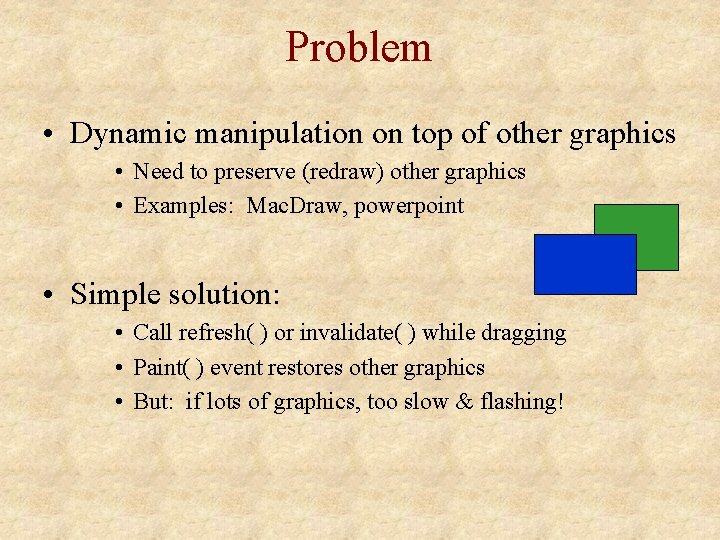
Problem • Dynamic manipulation on top of other graphics • Need to preserve (redraw) other graphics • Examples: Mac. Draw, powerpoint • Simple solution: • Call refresh( ) or invalidate( ) while dragging • Paint( ) event restores other graphics • But: if lots of graphics, too slow & flashing!
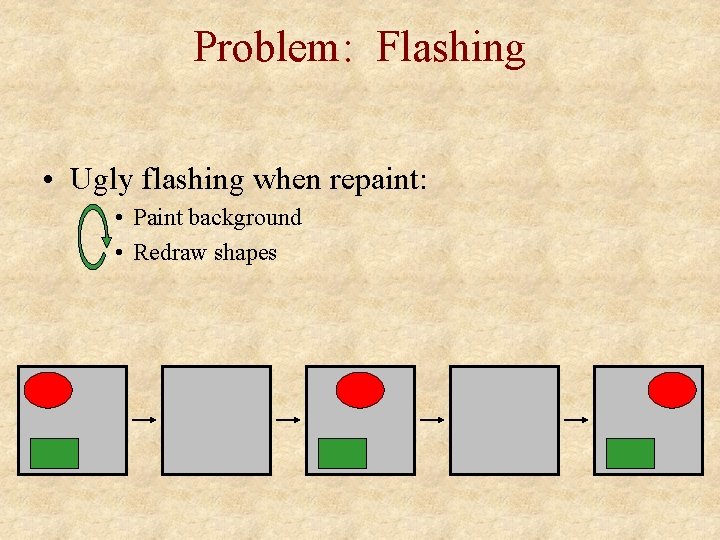
Problem: Flashing • Ugly flashing when repaint: • Paint background • Redraw shapes
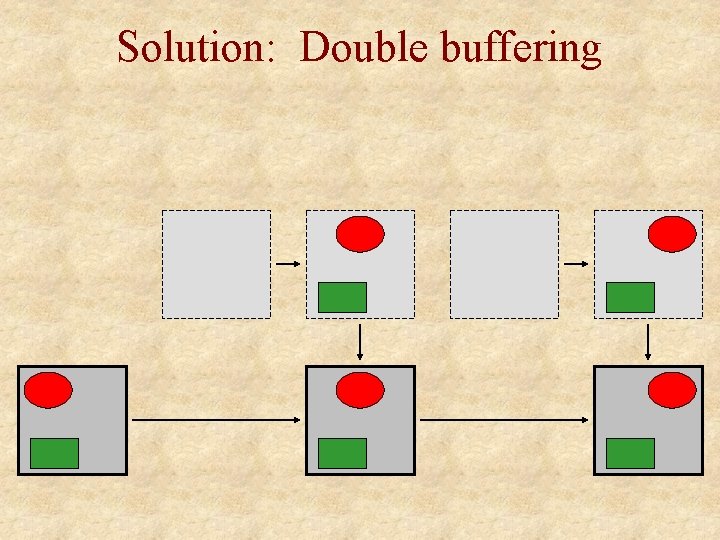
Solution: Double buffering
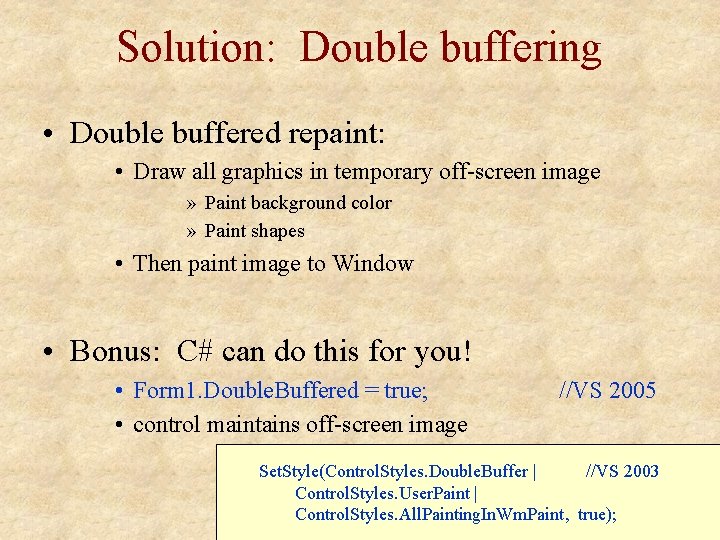
Solution: Double buffering • Double buffered repaint: • Draw all graphics in temporary off-screen image » Paint background color » Paint shapes • Then paint image to Window • Bonus: C# can do this for you! • Form 1. Double. Buffered = true; • control maintains off-screen image //VS 2005 Set. Style(Control. Styles. Double. Buffer | //VS 2003 Control. Styles. User. Paint | Control. Styles. All. Painting. In. Wm. Paint, true);
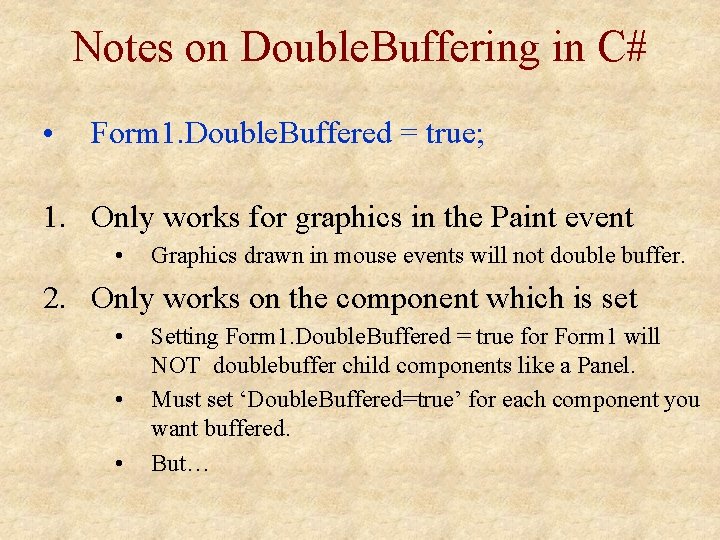
Notes on Double. Buffering in C# • Form 1. Double. Buffered = true; 1. Only works for graphics in the Paint event • Graphics drawn in mouse events will not double buffer. 2. Only works on the component which is set • • • Setting Form 1. Double. Buffered = true for Form 1 will NOT doublebuffer child components like a Panel. Must set ‘Double. Buffered=true’ for each component you want buffered. But…
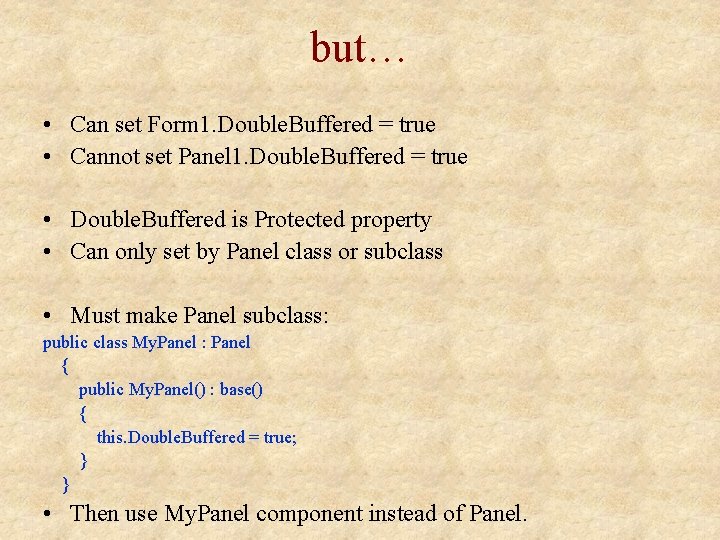
but… • Can set Form 1. Double. Buffered = true • Cannot set Panel 1. Double. Buffered = true • Double. Buffered is Protected property • Can only set by Panel class or subclass • Must make Panel subclass: public class My. Panel : Panel { public My. Panel() : base() { this. Double. Buffered = true; } } • Then use My. Panel component instead of Panel.
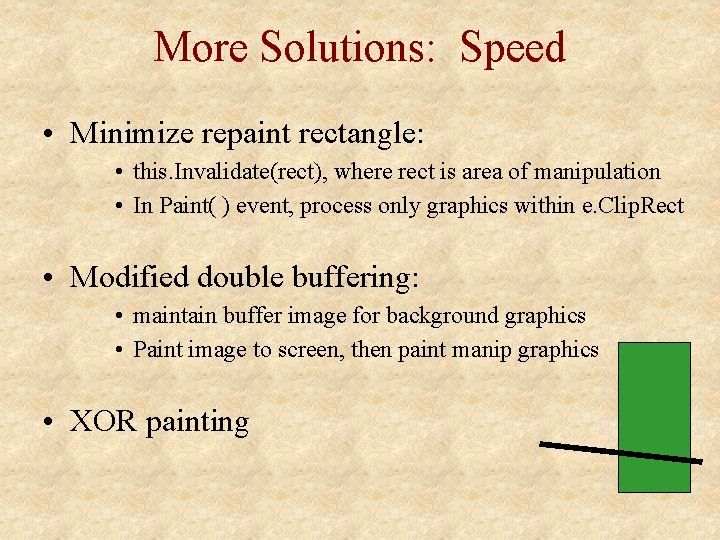
More Solutions: Speed • Minimize repaint rectangle: • this. Invalidate(rect), where rect is area of manipulation • In Paint( ) event, process only graphics within e. Clip. Rect • Modified double buffering: • maintain buffer image for background graphics • Paint image to screen, then paint manip graphics • XOR painting
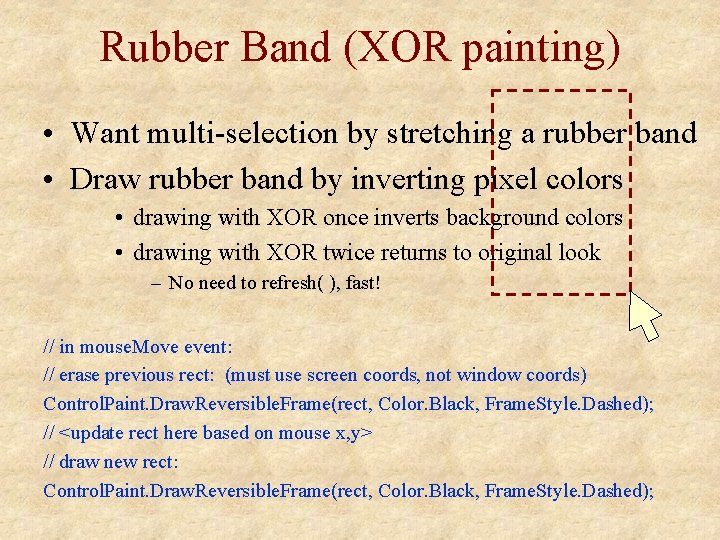
Rubber Band (XOR painting) • Want multi-selection by stretching a rubber band • Draw rubber band by inverting pixel colors • drawing with XOR once inverts background colors • drawing with XOR twice returns to original look – No need to refresh( ), fast! // in mouse. Move event: // erase previous rect: (must use screen coords, not window coords) Control. Paint. Draw. Reversible. Frame(rect, Color. Black, Frame. Style. Dashed); // <update rect here based on mouse x, y> // draw new rect: Control. Paint. Draw. Reversible. Frame(rect, Color. Black, Frame. Style. Dashed);
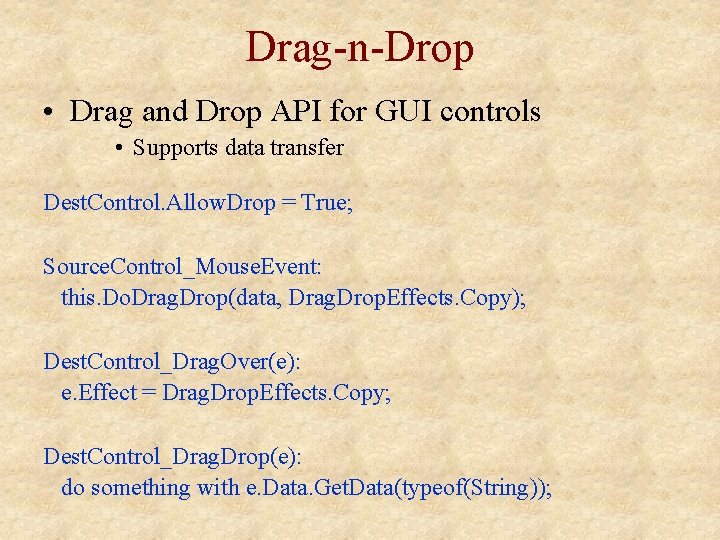
Drag-n-Drop • Drag and Drop API for GUI controls • Supports data transfer Dest. Control. Allow. Drop = True; Source. Control_Mouse. Event: this. Do. Drag. Drop(data, Drag. Drop. Effects. Copy); Dest. Control_Drag. Over(e): e. Effect = Drag. Drop. Effects. Copy; Dest. Control_Drag. Drop(e): do something with e. Data. Get. Data(typeof(String));
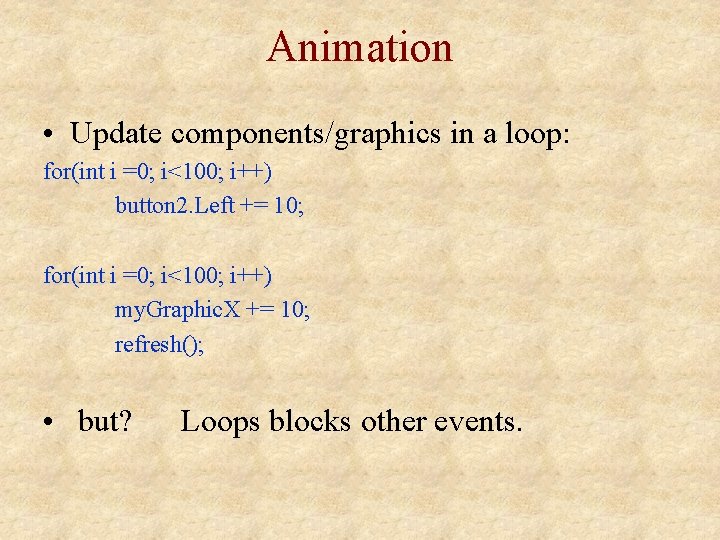
Animation • Update components/graphics in a loop: for(int i =0; i<100; i++) button 2. Left += 10; for(int i =0; i<100; i++) my. Graphic. X += 10; refresh(); • but? Loops blocks other events.
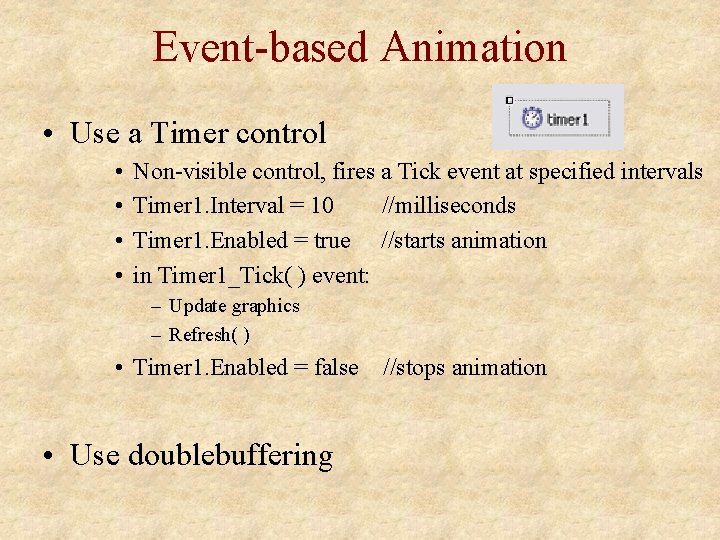
Event-based Animation • Use a Timer control • • Non-visible control, fires a Tick event at specified intervals Timer 1. Interval = 10 //milliseconds Timer 1. Enabled = true //starts animation in Timer 1_Tick( ) event: – Update graphics – Refresh( ) • Timer 1. Enabled = false //stops animation • Use doublebuffering
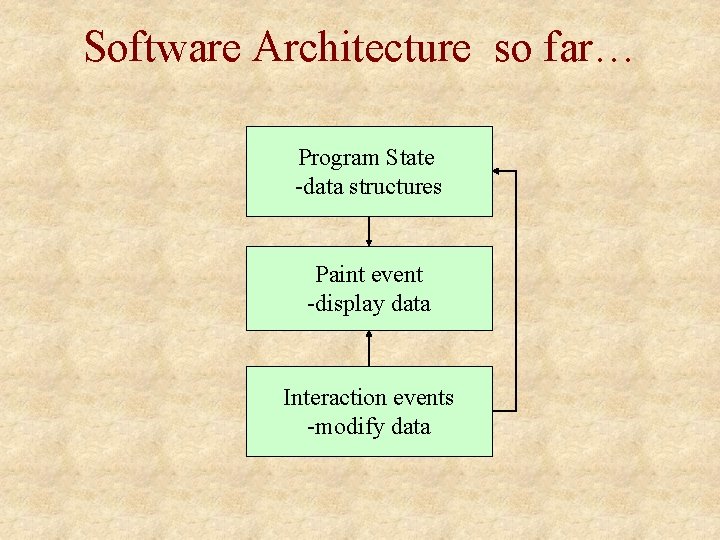
Software Architecture so far… Program State -data structures Paint event -display data Interaction events -modify data